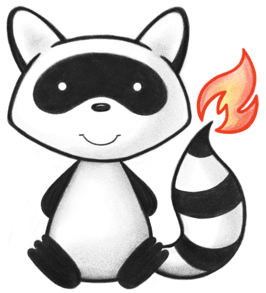
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * A concept that may be defined by a formal reference to a terminology or 046 * ontology or may be provided by text. 047 */ 048@DatatypeDef(name = "CodeableConcept") 049public class CodeableConcept extends Type implements ICompositeType { 050 051 /** 052 * A reference to a code defined by a terminology system. 053 */ 054 @Child(name = "coding", type = { 055 Coding.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 056 @Description(shortDefinition = "Code defined by a terminology system", formalDefinition = "A reference to a code defined by a terminology system.") 057 protected List<Coding> coding; 058 059 /** 060 * A human language representation of the concept as seen/selected/uttered by 061 * the user who entered the data and/or which represents the intended meaning of 062 * the user. 063 */ 064 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "Plain text representation of the concept", formalDefinition = "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.") 066 protected StringType text; 067 068 private static final long serialVersionUID = 760353246L; 069 070 /** 071 * Constructor 072 */ 073 public CodeableConcept() { 074 super(); 075 } 076 077 /** 078 * @return {@link #coding} (A reference to a code defined by a terminology 079 * system.) 080 */ 081 public List<Coding> getCoding() { 082 if (this.coding == null) 083 this.coding = new ArrayList<Coding>(); 084 return this.coding; 085 } 086 087 /** 088 * @return Returns a reference to <code>this</code> for easy method chaining 089 */ 090 public CodeableConcept setCoding(List<Coding> theCoding) { 091 this.coding = theCoding; 092 return this; 093 } 094 095 public boolean hasCoding() { 096 if (this.coding == null) 097 return false; 098 for (Coding item : this.coding) 099 if (!item.isEmpty()) 100 return true; 101 return false; 102 } 103 104 public Coding addCoding() { // 3 105 Coding t = new Coding(); 106 if (this.coding == null) 107 this.coding = new ArrayList<Coding>(); 108 this.coding.add(t); 109 return t; 110 } 111 112 public CodeableConcept addCoding(Coding t) { // 3 113 if (t == null) 114 return this; 115 if (this.coding == null) 116 this.coding = new ArrayList<Coding>(); 117 this.coding.add(t); 118 return this; 119 } 120 121 /** 122 * @return The first repetition of repeating field {@link #coding}, creating it 123 * if it does not already exist 124 */ 125 public Coding getCodingFirstRep() { 126 if (getCoding().isEmpty()) { 127 addCoding(); 128 } 129 return getCoding().get(0); 130 } 131 132 /** 133 * @return {@link #text} (A human language representation of the concept as 134 * seen/selected/uttered by the user who entered the data and/or which 135 * represents the intended meaning of the user.). This is the underlying 136 * object with id, value and extensions. The accessor "getText" gives 137 * direct access to the value 138 */ 139 public StringType getTextElement() { 140 if (this.text == null) 141 if (Configuration.errorOnAutoCreate()) 142 throw new Error("Attempt to auto-create CodeableConcept.text"); 143 else if (Configuration.doAutoCreate()) 144 this.text = new StringType(); // bb 145 return this.text; 146 } 147 148 public boolean hasTextElement() { 149 return this.text != null && !this.text.isEmpty(); 150 } 151 152 public boolean hasText() { 153 return this.text != null && !this.text.isEmpty(); 154 } 155 156 /** 157 * @param value {@link #text} (A human language representation of the concept as 158 * seen/selected/uttered by the user who entered the data and/or 159 * which represents the intended meaning of the user.). This is the 160 * underlying object with id, value and extensions. The accessor 161 * "getText" gives direct access to the value 162 */ 163 public CodeableConcept setTextElement(StringType value) { 164 this.text = value; 165 return this; 166 } 167 168 /** 169 * @return A human language representation of the concept as 170 * seen/selected/uttered by the user who entered the data and/or which 171 * represents the intended meaning of the user. 172 */ 173 public String getText() { 174 return this.text == null ? null : this.text.getValue(); 175 } 176 177 /** 178 * @param value A human language representation of the concept as 179 * seen/selected/uttered by the user who entered the data and/or 180 * which represents the intended meaning of the user. 181 */ 182 public CodeableConcept setText(String value) { 183 if (Utilities.noString(value)) 184 this.text = null; 185 else { 186 if (this.text == null) 187 this.text = new StringType(); 188 this.text.setValue(value); 189 } 190 return this; 191 } 192 193 protected void listChildren(List<Property> children) { 194 super.listChildren(children); 195 children.add(new Property("coding", "Coding", "A reference to a code defined by a terminology system.", 0, 196 java.lang.Integer.MAX_VALUE, coding)); 197 children.add(new Property("text", "string", 198 "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.", 199 0, 1, text)); 200 } 201 202 @Override 203 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 204 switch (_hash) { 205 case -1355086998: 206 /* coding */ return new Property("coding", "Coding", "A reference to a code defined by a terminology system.", 0, 207 java.lang.Integer.MAX_VALUE, coding); 208 case 3556653: 209 /* text */ return new Property("text", "string", 210 "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.", 211 0, 1, text); 212 default: 213 return super.getNamedProperty(_hash, _name, _checkValid); 214 } 215 216 } 217 218 @Override 219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 220 switch (hash) { 221 case -1355086998: 222 /* coding */ return this.coding == null ? new Base[0] : this.coding.toArray(new Base[this.coding.size()]); // Coding 223 case 3556653: 224 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 225 default: 226 return super.getProperty(hash, name, checkValid); 227 } 228 229 } 230 231 @Override 232 public Base setProperty(int hash, String name, Base value) throws FHIRException { 233 switch (hash) { 234 case -1355086998: // coding 235 this.getCoding().add(castToCoding(value)); // Coding 236 return value; 237 case 3556653: // text 238 this.text = castToString(value); // StringType 239 return value; 240 default: 241 return super.setProperty(hash, name, value); 242 } 243 244 } 245 246 @Override 247 public Base setProperty(String name, Base value) throws FHIRException { 248 if (name.equals("coding")) { 249 this.getCoding().add(castToCoding(value)); 250 } else if (name.equals("text")) { 251 this.text = castToString(value); // StringType 252 } else 253 return super.setProperty(name, value); 254 return value; 255 } 256 257 @Override 258 public void removeChild(String name, Base value) throws FHIRException { 259 if (name.equals("coding")) { 260 this.getCoding().remove(castToCoding(value)); 261 } else if (name.equals("text")) { 262 this.text = null; 263 } else 264 super.removeChild(name, value); 265 266 } 267 268 @Override 269 public Base makeProperty(int hash, String name) throws FHIRException { 270 switch (hash) { 271 case -1355086998: 272 return addCoding(); 273 case 3556653: 274 return getTextElement(); 275 default: 276 return super.makeProperty(hash, name); 277 } 278 279 } 280 281 @Override 282 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 283 switch (hash) { 284 case -1355086998: 285 /* coding */ return new String[] { "Coding" }; 286 case 3556653: 287 /* text */ return new String[] { "string" }; 288 default: 289 return super.getTypesForProperty(hash, name); 290 } 291 292 } 293 294 @Override 295 public Base addChild(String name) throws FHIRException { 296 if (name.equals("coding")) { 297 return addCoding(); 298 } else if (name.equals("text")) { 299 throw new FHIRException("Cannot call addChild on a singleton property CodeableConcept.text"); 300 } else 301 return super.addChild(name); 302 } 303 304 public String fhirType() { 305 return "CodeableConcept"; 306 307 } 308 309 public CodeableConcept copy() { 310 CodeableConcept dst = new CodeableConcept(); 311 copyValues(dst); 312 return dst; 313 } 314 315 public void copyValues(CodeableConcept dst) { 316 super.copyValues(dst); 317 if (coding != null) { 318 dst.coding = new ArrayList<Coding>(); 319 for (Coding i : coding) 320 dst.coding.add(i.copy()); 321 } 322 ; 323 dst.text = text == null ? null : text.copy(); 324 } 325 326 protected CodeableConcept typedCopy() { 327 return copy(); 328 } 329 330 @Override 331 public boolean equalsDeep(Base other_) { 332 if (!super.equalsDeep(other_)) 333 return false; 334 if (!(other_ instanceof CodeableConcept)) 335 return false; 336 CodeableConcept o = (CodeableConcept) other_; 337 return compareDeep(coding, o.coding, true) && compareDeep(text, o.text, true); 338 } 339 340 @Override 341 public boolean equalsShallow(Base other_) { 342 if (!super.equalsShallow(other_)) 343 return false; 344 if (!(other_ instanceof CodeableConcept)) 345 return false; 346 CodeableConcept o = (CodeableConcept) other_; 347 return compareValues(text, o.text, true); 348 } 349 350 public boolean isEmpty() { 351 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coding, text); 352 } 353 354// added from java-adornments.txt: 355 356 public boolean hasCoding(String system, String code) { 357 for (Coding c : getCoding()) { 358 if (system.equals(c.getSystem()) && code.equals(c.getCode())) 359 return true; 360 } 361 return false; 362 } 363 364 public CodeableConcept(Coding code) { 365 super(); 366 addCoding(code); 367 } 368 369// end addition 370 371}