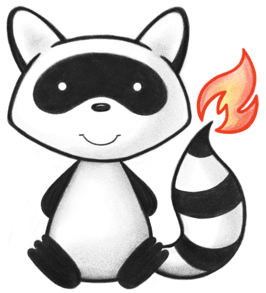
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.IBaseCoding; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * A reference to a code defined by a terminology system. 046 */ 047@DatatypeDef(name = "Coding") 048public class Coding extends Type implements IBaseCoding, ICompositeType, ICoding { 049 050 /** 051 * The identification of the code system that defines the meaning of the symbol 052 * in the code. 053 */ 054 @Child(name = "system", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Identity of the terminology system", formalDefinition = "The identification of the code system that defines the meaning of the symbol in the code.") 056 protected UriType system; 057 058 /** 059 * The version of the code system which was used when choosing this code. Note 060 * that a well-maintained code system does not need the version reported, 061 * because the meaning of codes is consistent across versions. However this 062 * cannot consistently be assured, and when the meaning is not guaranteed to be 063 * consistent, the version SHOULD be exchanged. 064 */ 065 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "Version of the system - if relevant", formalDefinition = "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.") 067 protected StringType version; 068 069 /** 070 * A symbol in syntax defined by the system. The symbol may be a predefined code 071 * or an expression in a syntax defined by the coding system (e.g. 072 * post-coordination). 073 */ 074 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Symbol in syntax defined by the system", formalDefinition = "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).") 076 protected CodeType code; 077 078 /** 079 * A representation of the meaning of the code in the system, following the 080 * rules of the system. 081 */ 082 @Child(name = "display", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 083 @Description(shortDefinition = "Representation defined by the system", formalDefinition = "A representation of the meaning of the code in the system, following the rules of the system.") 084 protected StringType display; 085 086 /** 087 * Indicates that this coding was chosen by a user directly - e.g. off a pick 088 * list of available items (codes or displays). 089 */ 090 @Child(name = "userSelected", type = { 091 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 092 @Description(shortDefinition = "If this coding was chosen directly by the user", formalDefinition = "Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).") 093 protected BooleanType userSelected; 094 095 private static final long serialVersionUID = -1417514061L; 096 097 /** 098 * Constructor 099 */ 100 public Coding() { 101 super(); 102 } 103 104 /** 105 * Convenience constructor 106 * 107 * @param theSystem The {@link #setSystem(String) code system} 108 * @param theCode The {@link #setCode(String) code} 109 * @param theDisplay The {@link #setDisplay(String) human readable display} 110 */ 111 public Coding(String theSystem, String theCode, String theDisplay) { 112 setSystem(theSystem); 113 setCode(theCode); 114 setDisplay(theDisplay); 115 } 116 117 /** 118 * @return {@link #system} (The identification of the code system that defines 119 * the meaning of the symbol in the code.). This is the underlying 120 * object with id, value and extensions. The accessor "getSystem" gives 121 * direct access to the value 122 */ 123 public UriType getSystemElement() { 124 if (this.system == null) 125 if (Configuration.errorOnAutoCreate()) 126 throw new Error("Attempt to auto-create Coding.system"); 127 else if (Configuration.doAutoCreate()) 128 this.system = new UriType(); // bb 129 return this.system; 130 } 131 132 public boolean hasSystemElement() { 133 return this.system != null && !this.system.isEmpty(); 134 } 135 136 public boolean hasSystem() { 137 return this.system != null && !this.system.isEmpty(); 138 } 139 140 /** 141 * @param value {@link #system} (The identification of the code system that 142 * defines the meaning of the symbol in the code.). This is the 143 * underlying object with id, value and extensions. The accessor 144 * "getSystem" gives direct access to the value 145 */ 146 public Coding setSystemElement(UriType value) { 147 this.system = value; 148 return this; 149 } 150 151 /** 152 * @return The identification of the code system that defines the meaning of the 153 * symbol in the code. 154 */ 155 public String getSystem() { 156 return this.system == null ? null : this.system.getValue(); 157 } 158 159 /** 160 * @param value The identification of the code system that defines the meaning 161 * of the symbol in the code. 162 */ 163 public Coding setSystem(String value) { 164 if (Utilities.noString(value)) 165 this.system = null; 166 else { 167 if (this.system == null) 168 this.system = new UriType(); 169 this.system.setValue(value); 170 } 171 return this; 172 } 173 174 /** 175 * @return {@link #version} (The version of the code system which was used when 176 * choosing this code. Note that a well-maintained code system does not 177 * need the version reported, because the meaning of codes is consistent 178 * across versions. However this cannot consistently be assured, and 179 * when the meaning is not guaranteed to be consistent, the version 180 * SHOULD be exchanged.). This is the underlying object with id, value 181 * and extensions. The accessor "getVersion" gives direct access to the 182 * value 183 */ 184 public StringType getVersionElement() { 185 if (this.version == null) 186 if (Configuration.errorOnAutoCreate()) 187 throw new Error("Attempt to auto-create Coding.version"); 188 else if (Configuration.doAutoCreate()) 189 this.version = new StringType(); // bb 190 return this.version; 191 } 192 193 public boolean hasVersionElement() { 194 return this.version != null && !this.version.isEmpty(); 195 } 196 197 public boolean hasVersion() { 198 return this.version != null && !this.version.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #version} (The version of the code system which was used 203 * when choosing this code. Note that a well-maintained code system 204 * does not need the version reported, because the meaning of codes 205 * is consistent across versions. However this cannot consistently 206 * be assured, and when the meaning is not guaranteed to be 207 * consistent, the version SHOULD be exchanged.). This is the 208 * underlying object with id, value and extensions. The accessor 209 * "getVersion" gives direct access to the value 210 */ 211 public Coding setVersionElement(StringType value) { 212 this.version = value; 213 return this; 214 } 215 216 /** 217 * @return The version of the code system which was used when choosing this 218 * code. Note that a well-maintained code system does not need the 219 * version reported, because the meaning of codes is consistent across 220 * versions. However this cannot consistently be assured, and when the 221 * meaning is not guaranteed to be consistent, the version SHOULD be 222 * exchanged. 223 */ 224 public String getVersion() { 225 return this.version == null ? null : this.version.getValue(); 226 } 227 228 /** 229 * @param value The version of the code system which was used when choosing this 230 * code. Note that a well-maintained code system does not need the 231 * version reported, because the meaning of codes is consistent 232 * across versions. However this cannot consistently be assured, 233 * and when the meaning is not guaranteed to be consistent, the 234 * version SHOULD be exchanged. 235 */ 236 public Coding setVersion(String value) { 237 if (Utilities.noString(value)) 238 this.version = null; 239 else { 240 if (this.version == null) 241 this.version = new StringType(); 242 this.version.setValue(value); 243 } 244 return this; 245 } 246 247 /** 248 * @return {@link #code} (A symbol in syntax defined by the system. The symbol 249 * may be a predefined code or an expression in a syntax defined by the 250 * coding system (e.g. post-coordination).). This is the underlying 251 * object with id, value and extensions. The accessor "getCode" gives 252 * direct access to the value 253 */ 254 public CodeType getCodeElement() { 255 if (this.code == null) 256 if (Configuration.errorOnAutoCreate()) 257 throw new Error("Attempt to auto-create Coding.code"); 258 else if (Configuration.doAutoCreate()) 259 this.code = new CodeType(); // bb 260 return this.code; 261 } 262 263 public boolean hasCodeElement() { 264 return this.code != null && !this.code.isEmpty(); 265 } 266 267 public boolean hasCode() { 268 return this.code != null && !this.code.isEmpty(); 269 } 270 271 /** 272 * @param value {@link #code} (A symbol in syntax defined by the system. The 273 * symbol may be a predefined code or an expression in a syntax 274 * defined by the coding system (e.g. post-coordination).). This is 275 * the underlying object with id, value and extensions. The 276 * accessor "getCode" gives direct access to the value 277 */ 278 public Coding setCodeElement(CodeType value) { 279 this.code = value; 280 return this; 281 } 282 283 /** 284 * @return A symbol in syntax defined by the system. The symbol may be a 285 * predefined code or an expression in a syntax defined by the coding 286 * system (e.g. post-coordination). 287 */ 288 public String getCode() { 289 return this.code == null ? null : this.code.getValue(); 290 } 291 292 /** 293 * @param value A symbol in syntax defined by the system. The symbol may be a 294 * predefined code or an expression in a syntax defined by the 295 * coding system (e.g. post-coordination). 296 */ 297 public Coding setCode(String value) { 298 if (Utilities.noString(value)) 299 this.code = null; 300 else { 301 if (this.code == null) 302 this.code = new CodeType(); 303 this.code.setValue(value); 304 } 305 return this; 306 } 307 308 /** 309 * @return {@link #display} (A representation of the meaning of the code in the 310 * system, following the rules of the system.). This is the underlying 311 * object with id, value and extensions. The accessor "getDisplay" gives 312 * direct access to the value 313 */ 314 public StringType getDisplayElement() { 315 if (this.display == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create Coding.display"); 318 else if (Configuration.doAutoCreate()) 319 this.display = new StringType(); // bb 320 return this.display; 321 } 322 323 public boolean hasDisplayElement() { 324 return this.display != null && !this.display.isEmpty(); 325 } 326 327 public boolean hasDisplay() { 328 return this.display != null && !this.display.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #display} (A representation of the meaning of the code in 333 * the system, following the rules of the system.). This is the 334 * underlying object with id, value and extensions. The accessor 335 * "getDisplay" gives direct access to the value 336 */ 337 public Coding setDisplayElement(StringType value) { 338 this.display = value; 339 return this; 340 } 341 342 /** 343 * @return A representation of the meaning of the code in the system, following 344 * the rules of the system. 345 */ 346 public String getDisplay() { 347 return this.display == null ? null : this.display.getValue(); 348 } 349 350 /** 351 * @param value A representation of the meaning of the code in the system, 352 * following the rules of the system. 353 */ 354 public Coding setDisplay(String value) { 355 if (Utilities.noString(value)) 356 this.display = null; 357 else { 358 if (this.display == null) 359 this.display = new StringType(); 360 this.display.setValue(value); 361 } 362 return this; 363 } 364 365 /** 366 * @return {@link #userSelected} (Indicates that this coding was chosen by a 367 * user directly - e.g. off a pick list of available items (codes or 368 * displays).). This is the underlying object with id, value and 369 * extensions. The accessor "getUserSelected" gives direct access to the 370 * value 371 */ 372 public BooleanType getUserSelectedElement() { 373 if (this.userSelected == null) 374 if (Configuration.errorOnAutoCreate()) 375 throw new Error("Attempt to auto-create Coding.userSelected"); 376 else if (Configuration.doAutoCreate()) 377 this.userSelected = new BooleanType(); // bb 378 return this.userSelected; 379 } 380 381 public boolean hasUserSelectedElement() { 382 return this.userSelected != null && !this.userSelected.isEmpty(); 383 } 384 385 public boolean hasUserSelected() { 386 return this.userSelected != null && !this.userSelected.isEmpty(); 387 } 388 389 /** 390 * @param value {@link #userSelected} (Indicates that this coding was chosen by 391 * a user directly - e.g. off a pick list of available items (codes 392 * or displays).). This is the underlying object with id, value and 393 * extensions. The accessor "getUserSelected" gives direct access 394 * to the value 395 */ 396 public Coding setUserSelectedElement(BooleanType value) { 397 this.userSelected = value; 398 return this; 399 } 400 401 /** 402 * @return Indicates that this coding was chosen by a user directly - e.g. off a 403 * pick list of available items (codes or displays). 404 */ 405 public boolean getUserSelected() { 406 return this.userSelected == null || this.userSelected.isEmpty() ? false : this.userSelected.getValue(); 407 } 408 409 /** 410 * @param value Indicates that this coding was chosen by a user directly - e.g. 411 * off a pick list of available items (codes or displays). 412 */ 413 public Coding setUserSelected(boolean value) { 414 if (this.userSelected == null) 415 this.userSelected = new BooleanType(); 416 this.userSelected.setValue(value); 417 return this; 418 } 419 420 protected void listChildren(List<Property> children) { 421 super.listChildren(children); 422 children.add(new Property("system", "uri", 423 "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system)); 424 children.add(new Property("version", "string", 425 "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 426 0, 1, version)); 427 children.add(new Property("code", "code", 428 "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 429 0, 1, code)); 430 children.add(new Property("display", "string", 431 "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, 432 display)); 433 children.add(new Property("userSelected", "boolean", 434 "Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).", 435 0, 1, userSelected)); 436 } 437 438 @Override 439 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 440 switch (_hash) { 441 case -887328209: 442 /* system */ return new Property("system", "uri", 443 "The identification of the code system that defines the meaning of the symbol in the code.", 0, 1, system); 444 case 351608024: 445 /* version */ return new Property("version", "string", 446 "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured, and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 447 0, 1, version); 448 case 3059181: 449 /* code */ return new Property("code", "code", 450 "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 451 0, 1, code); 452 case 1671764162: 453 /* display */ return new Property("display", "string", 454 "A representation of the meaning of the code in the system, following the rules of the system.", 0, 1, 455 display); 456 case 423643014: 457 /* userSelected */ return new Property("userSelected", "boolean", 458 "Indicates that this coding was chosen by a user directly - e.g. off a pick list of available items (codes or displays).", 459 0, 1, userSelected); 460 default: 461 return super.getNamedProperty(_hash, _name, _checkValid); 462 } 463 464 } 465 466 @Override 467 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 468 switch (hash) { 469 case -887328209: 470 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // UriType 471 case 351608024: 472 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 473 case 3059181: 474 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 475 case 1671764162: 476 /* display */ return this.display == null ? new Base[0] : new Base[] { this.display }; // StringType 477 case 423643014: 478 /* userSelected */ return this.userSelected == null ? new Base[0] : new Base[] { this.userSelected }; // BooleanType 479 default: 480 return super.getProperty(hash, name, checkValid); 481 } 482 483 } 484 485 @Override 486 public Base setProperty(int hash, String name, Base value) throws FHIRException { 487 switch (hash) { 488 case -887328209: // system 489 this.system = castToUri(value); // UriType 490 return value; 491 case 351608024: // version 492 this.version = castToString(value); // StringType 493 return value; 494 case 3059181: // code 495 this.code = castToCode(value); // CodeType 496 return value; 497 case 1671764162: // display 498 this.display = castToString(value); // StringType 499 return value; 500 case 423643014: // userSelected 501 this.userSelected = castToBoolean(value); // BooleanType 502 return value; 503 default: 504 return super.setProperty(hash, name, value); 505 } 506 507 } 508 509 @Override 510 public Base setProperty(String name, Base value) throws FHIRException { 511 if (name.equals("system")) { 512 this.system = castToUri(value); // UriType 513 } else if (name.equals("version")) { 514 this.version = castToString(value); // StringType 515 } else if (name.equals("code")) { 516 this.code = castToCode(value); // CodeType 517 } else if (name.equals("display")) { 518 this.display = castToString(value); // StringType 519 } else if (name.equals("userSelected")) { 520 this.userSelected = castToBoolean(value); // BooleanType 521 } else 522 return super.setProperty(name, value); 523 return value; 524 } 525 526 @Override 527 public void removeChild(String name, Base value) throws FHIRException { 528 if (name.equals("system")) { 529 this.system = null; 530 } else if (name.equals("version")) { 531 this.version = null; 532 } else if (name.equals("code")) { 533 this.code = null; 534 } else if (name.equals("display")) { 535 this.display = null; 536 } else if (name.equals("userSelected")) { 537 this.userSelected = null; 538 } else 539 super.removeChild(name, value); 540 541 } 542 543 @Override 544 public Base makeProperty(int hash, String name) throws FHIRException { 545 switch (hash) { 546 case -887328209: 547 return getSystemElement(); 548 case 351608024: 549 return getVersionElement(); 550 case 3059181: 551 return getCodeElement(); 552 case 1671764162: 553 return getDisplayElement(); 554 case 423643014: 555 return getUserSelectedElement(); 556 default: 557 return super.makeProperty(hash, name); 558 } 559 560 } 561 562 @Override 563 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case -887328209: 566 /* system */ return new String[] { "uri" }; 567 case 351608024: 568 /* version */ return new String[] { "string" }; 569 case 3059181: 570 /* code */ return new String[] { "code" }; 571 case 1671764162: 572 /* display */ return new String[] { "string" }; 573 case 423643014: 574 /* userSelected */ return new String[] { "boolean" }; 575 default: 576 return super.getTypesForProperty(hash, name); 577 } 578 579 } 580 581 @Override 582 public Base addChild(String name) throws FHIRException { 583 if (name.equals("system")) { 584 throw new FHIRException("Cannot call addChild on a singleton property Coding.system"); 585 } else if (name.equals("version")) { 586 throw new FHIRException("Cannot call addChild on a singleton property Coding.version"); 587 } else if (name.equals("code")) { 588 throw new FHIRException("Cannot call addChild on a singleton property Coding.code"); 589 } else if (name.equals("display")) { 590 throw new FHIRException("Cannot call addChild on a singleton property Coding.display"); 591 } else if (name.equals("userSelected")) { 592 throw new FHIRException("Cannot call addChild on a singleton property Coding.userSelected"); 593 } else 594 return super.addChild(name); 595 } 596 597 public String fhirType() { 598 return "Coding"; 599 600 } 601 602 public Coding copy() { 603 Coding dst = new Coding(); 604 copyValues(dst); 605 return dst; 606 } 607 608 public void copyValues(Coding dst) { 609 super.copyValues(dst); 610 dst.system = system == null ? null : system.copy(); 611 dst.version = version == null ? null : version.copy(); 612 dst.code = code == null ? null : code.copy(); 613 dst.display = display == null ? null : display.copy(); 614 dst.userSelected = userSelected == null ? null : userSelected.copy(); 615 } 616 617 protected Coding typedCopy() { 618 return copy(); 619 } 620 621 @Override 622 public boolean equalsDeep(Base other_) { 623 if (!super.equalsDeep(other_)) 624 return false; 625 if (!(other_ instanceof Coding)) 626 return false; 627 Coding o = (Coding) other_; 628 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) 629 && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 630 && compareDeep(userSelected, o.userSelected, true); 631 } 632 633 @Override 634 public boolean equalsShallow(Base other_) { 635 if (!super.equalsShallow(other_)) 636 return false; 637 if (!(other_ instanceof Coding)) 638 return false; 639 Coding o = (Coding) other_; 640 return compareValues(system, o.system, true) && compareValues(version, o.version, true) 641 && compareValues(code, o.code, true) && compareValues(display, o.display, true) 642 && compareValues(userSelected, o.userSelected, true); 643 } 644 645 public boolean isEmpty() { 646 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, code, display, userSelected); 647 } 648 649// added from java-adornments.txt: 650 @Override 651 public boolean supportsVersion() { 652 return true; 653 } 654 655 @Override 656 public boolean supportsDisplay() { 657 return true; 658 } 659 660 public boolean is(String system, String code) { 661 return hasSystem() && hasCode() && this.getSystem().equals(system) && this.getCode().equals(code); 662 } 663 664// end addition 665 666}