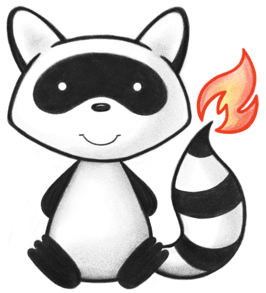
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A request to convey information; e.g. the CDS system proposes that an alert 048 * be sent to a responsible provider, the CDS system proposes that the public 049 * health agency be notified about a reportable condition. 050 */ 051@ResourceDef(name = "CommunicationRequest", profile = "http://hl7.org/fhir/StructureDefinition/CommunicationRequest") 052public class CommunicationRequest extends DomainResource { 053 054 public enum CommunicationRequestStatus { 055 /** 056 * The request has been created but is not yet complete or ready for action. 057 */ 058 DRAFT, 059 /** 060 * The request is in force and ready to be acted upon. 061 */ 062 ACTIVE, 063 /** 064 * The request (and any implicit authorization to act) has been temporarily 065 * withdrawn but is expected to resume in the future. 066 */ 067 ONHOLD, 068 /** 069 * The request (and any implicit authorization to act) has been terminated prior 070 * to the known full completion of the intended actions. No further activity 071 * should occur. 072 */ 073 REVOKED, 074 /** 075 * The activity described by the request has been fully performed. No further 076 * activity will occur. 077 */ 078 COMPLETED, 079 /** 080 * This request should never have existed and should be considered 'void'. (It 081 * is possible that real-world decisions were based on it. If real-world 082 * activity has occurred, the status should be "revoked" rather than 083 * "entered-in-error".). 084 */ 085 ENTEREDINERROR, 086 /** 087 * The authoring/source system does not know which of the status values 088 * currently applies for this request. Note: This concept is not to be used for 089 * "other" - one of the listed statuses is presumed to apply, but the 090 * authoring/source system does not know which. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static CommunicationRequestStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("draft".equals(codeString)) 102 return DRAFT; 103 if ("active".equals(codeString)) 104 return ACTIVE; 105 if ("on-hold".equals(codeString)) 106 return ONHOLD; 107 if ("revoked".equals(codeString)) 108 return REVOKED; 109 if ("completed".equals(codeString)) 110 return COMPLETED; 111 if ("entered-in-error".equals(codeString)) 112 return ENTEREDINERROR; 113 if ("unknown".equals(codeString)) 114 return UNKNOWN; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case DRAFT: 124 return "draft"; 125 case ACTIVE: 126 return "active"; 127 case ONHOLD: 128 return "on-hold"; 129 case REVOKED: 130 return "revoked"; 131 case COMPLETED: 132 return "completed"; 133 case ENTEREDINERROR: 134 return "entered-in-error"; 135 case UNKNOWN: 136 return "unknown"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case DRAFT: 147 return "http://hl7.org/fhir/request-status"; 148 case ACTIVE: 149 return "http://hl7.org/fhir/request-status"; 150 case ONHOLD: 151 return "http://hl7.org/fhir/request-status"; 152 case REVOKED: 153 return "http://hl7.org/fhir/request-status"; 154 case COMPLETED: 155 return "http://hl7.org/fhir/request-status"; 156 case ENTEREDINERROR: 157 return "http://hl7.org/fhir/request-status"; 158 case UNKNOWN: 159 return "http://hl7.org/fhir/request-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case DRAFT: 170 return "The request has been created but is not yet complete or ready for action."; 171 case ACTIVE: 172 return "The request is in force and ready to be acted upon."; 173 case ONHOLD: 174 return "The request (and any implicit authorization to act) has been temporarily withdrawn but is expected to resume in the future."; 175 case REVOKED: 176 return "The request (and any implicit authorization to act) has been terminated prior to the known full completion of the intended actions. No further activity should occur."; 177 case COMPLETED: 178 return "The activity described by the request has been fully performed. No further activity will occur."; 179 case ENTEREDINERROR: 180 return "This request should never have existed and should be considered 'void'. (It is possible that real-world decisions were based on it. If real-world activity has occurred, the status should be \"revoked\" rather than \"entered-in-error\".)."; 181 case UNKNOWN: 182 return "The authoring/source system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case DRAFT: 193 return "Draft"; 194 case ACTIVE: 195 return "Active"; 196 case ONHOLD: 197 return "On Hold"; 198 case REVOKED: 199 return "Revoked"; 200 case COMPLETED: 201 return "Completed"; 202 case ENTEREDINERROR: 203 return "Entered in Error"; 204 case UNKNOWN: 205 return "Unknown"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class CommunicationRequestStatusEnumFactory implements EnumFactory<CommunicationRequestStatus> { 215 public CommunicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("draft".equals(codeString)) 220 return CommunicationRequestStatus.DRAFT; 221 if ("active".equals(codeString)) 222 return CommunicationRequestStatus.ACTIVE; 223 if ("on-hold".equals(codeString)) 224 return CommunicationRequestStatus.ONHOLD; 225 if ("revoked".equals(codeString)) 226 return CommunicationRequestStatus.REVOKED; 227 if ("completed".equals(codeString)) 228 return CommunicationRequestStatus.COMPLETED; 229 if ("entered-in-error".equals(codeString)) 230 return CommunicationRequestStatus.ENTEREDINERROR; 231 if ("unknown".equals(codeString)) 232 return CommunicationRequestStatus.UNKNOWN; 233 throw new IllegalArgumentException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<CommunicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.NULL, code); 244 if ("draft".equals(codeString)) 245 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.DRAFT, code); 246 if ("active".equals(codeString)) 247 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ACTIVE, code); 248 if ("on-hold".equals(codeString)) 249 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ONHOLD, code); 250 if ("revoked".equals(codeString)) 251 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.REVOKED, code); 252 if ("completed".equals(codeString)) 253 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.COMPLETED, code); 254 if ("entered-in-error".equals(codeString)) 255 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.ENTEREDINERROR, code); 256 if ("unknown".equals(codeString)) 257 return new Enumeration<CommunicationRequestStatus>(this, CommunicationRequestStatus.UNKNOWN, code); 258 throw new FHIRException("Unknown CommunicationRequestStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(CommunicationRequestStatus code) { 262 if (code == CommunicationRequestStatus.NULL) 263 return null; 264 if (code == CommunicationRequestStatus.DRAFT) 265 return "draft"; 266 if (code == CommunicationRequestStatus.ACTIVE) 267 return "active"; 268 if (code == CommunicationRequestStatus.ONHOLD) 269 return "on-hold"; 270 if (code == CommunicationRequestStatus.REVOKED) 271 return "revoked"; 272 if (code == CommunicationRequestStatus.COMPLETED) 273 return "completed"; 274 if (code == CommunicationRequestStatus.ENTEREDINERROR) 275 return "entered-in-error"; 276 if (code == CommunicationRequestStatus.UNKNOWN) 277 return "unknown"; 278 return "?"; 279 } 280 281 public String toSystem(CommunicationRequestStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 public enum CommunicationPriority { 287 /** 288 * The request has normal priority. 289 */ 290 ROUTINE, 291 /** 292 * The request should be actioned promptly - higher priority than routine. 293 */ 294 URGENT, 295 /** 296 * The request should be actioned as soon as possible - higher priority than 297 * urgent. 298 */ 299 ASAP, 300 /** 301 * The request should be actioned immediately - highest possible priority. E.g. 302 * an emergency. 303 */ 304 STAT, 305 /** 306 * added to help the parsers with the generic types 307 */ 308 NULL; 309 310 public static CommunicationPriority fromCode(String codeString) throws FHIRException { 311 if (codeString == null || "".equals(codeString)) 312 return null; 313 if ("routine".equals(codeString)) 314 return ROUTINE; 315 if ("urgent".equals(codeString)) 316 return URGENT; 317 if ("asap".equals(codeString)) 318 return ASAP; 319 if ("stat".equals(codeString)) 320 return STAT; 321 if (Configuration.isAcceptInvalidEnums()) 322 return null; 323 else 324 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 325 } 326 327 public String toCode() { 328 switch (this) { 329 case ROUTINE: 330 return "routine"; 331 case URGENT: 332 return "urgent"; 333 case ASAP: 334 return "asap"; 335 case STAT: 336 return "stat"; 337 case NULL: 338 return null; 339 default: 340 return "?"; 341 } 342 } 343 344 public String getSystem() { 345 switch (this) { 346 case ROUTINE: 347 return "http://hl7.org/fhir/request-priority"; 348 case URGENT: 349 return "http://hl7.org/fhir/request-priority"; 350 case ASAP: 351 return "http://hl7.org/fhir/request-priority"; 352 case STAT: 353 return "http://hl7.org/fhir/request-priority"; 354 case NULL: 355 return null; 356 default: 357 return "?"; 358 } 359 } 360 361 public String getDefinition() { 362 switch (this) { 363 case ROUTINE: 364 return "The request has normal priority."; 365 case URGENT: 366 return "The request should be actioned promptly - higher priority than routine."; 367 case ASAP: 368 return "The request should be actioned as soon as possible - higher priority than urgent."; 369 case STAT: 370 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 371 case NULL: 372 return null; 373 default: 374 return "?"; 375 } 376 } 377 378 public String getDisplay() { 379 switch (this) { 380 case ROUTINE: 381 return "Routine"; 382 case URGENT: 383 return "Urgent"; 384 case ASAP: 385 return "ASAP"; 386 case STAT: 387 return "STAT"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 } 395 396 public static class CommunicationPriorityEnumFactory implements EnumFactory<CommunicationPriority> { 397 public CommunicationPriority fromCode(String codeString) throws IllegalArgumentException { 398 if (codeString == null || "".equals(codeString)) 399 if (codeString == null || "".equals(codeString)) 400 return null; 401 if ("routine".equals(codeString)) 402 return CommunicationPriority.ROUTINE; 403 if ("urgent".equals(codeString)) 404 return CommunicationPriority.URGENT; 405 if ("asap".equals(codeString)) 406 return CommunicationPriority.ASAP; 407 if ("stat".equals(codeString)) 408 return CommunicationPriority.STAT; 409 throw new IllegalArgumentException("Unknown CommunicationPriority code '" + codeString + "'"); 410 } 411 412 public Enumeration<CommunicationPriority> fromType(PrimitiveType<?> code) throws FHIRException { 413 if (code == null) 414 return null; 415 if (code.isEmpty()) 416 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 417 String codeString = code.asStringValue(); 418 if (codeString == null || "".equals(codeString)) 419 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.NULL, code); 420 if ("routine".equals(codeString)) 421 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ROUTINE, code); 422 if ("urgent".equals(codeString)) 423 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.URGENT, code); 424 if ("asap".equals(codeString)) 425 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.ASAP, code); 426 if ("stat".equals(codeString)) 427 return new Enumeration<CommunicationPriority>(this, CommunicationPriority.STAT, code); 428 throw new FHIRException("Unknown CommunicationPriority code '" + codeString + "'"); 429 } 430 431 public String toCode(CommunicationPriority code) { 432 if (code == CommunicationPriority.NULL) 433 return null; 434 if (code == CommunicationPriority.ROUTINE) 435 return "routine"; 436 if (code == CommunicationPriority.URGENT) 437 return "urgent"; 438 if (code == CommunicationPriority.ASAP) 439 return "asap"; 440 if (code == CommunicationPriority.STAT) 441 return "stat"; 442 return "?"; 443 } 444 445 public String toSystem(CommunicationPriority code) { 446 return code.getSystem(); 447 } 448 } 449 450 @Block() 451 public static class CommunicationRequestPayloadComponent extends BackboneElement implements IBaseBackboneElement { 452 /** 453 * The communicated content (or for multi-part communications, one portion of 454 * the communication). 455 */ 456 @Child(name = "content", type = { StringType.class, Attachment.class, 457 Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 458 @Description(shortDefinition = "Message part content", formalDefinition = "The communicated content (or for multi-part communications, one portion of the communication).") 459 protected Type content; 460 461 private static final long serialVersionUID = -1763459053L; 462 463 /** 464 * Constructor 465 */ 466 public CommunicationRequestPayloadComponent() { 467 super(); 468 } 469 470 /** 471 * Constructor 472 */ 473 public CommunicationRequestPayloadComponent(Type content) { 474 super(); 475 this.content = content; 476 } 477 478 /** 479 * @return {@link #content} (The communicated content (or for multi-part 480 * communications, one portion of the communication).) 481 */ 482 public Type getContent() { 483 return this.content; 484 } 485 486 /** 487 * @return {@link #content} (The communicated content (or for multi-part 488 * communications, one portion of the communication).) 489 */ 490 public StringType getContentStringType() throws FHIRException { 491 if (this.content == null) 492 this.content = new StringType(); 493 if (!(this.content instanceof StringType)) 494 throw new FHIRException("Type mismatch: the type StringType was expected, but " 495 + this.content.getClass().getName() + " was encountered"); 496 return (StringType) this.content; 497 } 498 499 public boolean hasContentStringType() { 500 return this.content instanceof StringType; 501 } 502 503 /** 504 * @return {@link #content} (The communicated content (or for multi-part 505 * communications, one portion of the communication).) 506 */ 507 public Attachment getContentAttachment() throws FHIRException { 508 if (this.content == null) 509 this.content = new Attachment(); 510 if (!(this.content instanceof Attachment)) 511 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 512 + this.content.getClass().getName() + " was encountered"); 513 return (Attachment) this.content; 514 } 515 516 public boolean hasContentAttachment() { 517 return this.content instanceof Attachment; 518 } 519 520 /** 521 * @return {@link #content} (The communicated content (or for multi-part 522 * communications, one portion of the communication).) 523 */ 524 public Reference getContentReference() throws FHIRException { 525 if (this.content == null) 526 this.content = new Reference(); 527 if (!(this.content instanceof Reference)) 528 throw new FHIRException("Type mismatch: the type Reference was expected, but " 529 + this.content.getClass().getName() + " was encountered"); 530 return (Reference) this.content; 531 } 532 533 public boolean hasContentReference() { 534 return this.content instanceof Reference; 535 } 536 537 public boolean hasContent() { 538 return this.content != null && !this.content.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #content} (The communicated content (or for multi-part 543 * communications, one portion of the communication).) 544 */ 545 public CommunicationRequestPayloadComponent setContent(Type value) { 546 if (value != null && !(value instanceof StringType || value instanceof Attachment || value instanceof Reference)) 547 throw new Error("Not the right type for CommunicationRequest.payload.content[x]: " + value.fhirType()); 548 this.content = value; 549 return this; 550 } 551 552 protected void listChildren(List<Property> children) { 553 super.listChildren(children); 554 children.add(new Property("content[x]", "string|Attachment|Reference(Any)", 555 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 556 content)); 557 } 558 559 @Override 560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 561 switch (_hash) { 562 case 264548711: 563 /* content[x] */ return new Property("content[x]", "string|Attachment|Reference(Any)", 564 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 565 content); 566 case 951530617: 567 /* content */ return new Property("content[x]", "string|Attachment|Reference(Any)", 568 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 569 content); 570 case -326336022: 571 /* contentString */ return new Property("content[x]", "string|Attachment|Reference(Any)", 572 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 573 content); 574 case -702028164: 575 /* contentAttachment */ return new Property("content[x]", "string|Attachment|Reference(Any)", 576 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 577 content); 578 case 1193747154: 579 /* contentReference */ return new Property("content[x]", "string|Attachment|Reference(Any)", 580 "The communicated content (or for multi-part communications, one portion of the communication).", 0, 1, 581 content); 582 default: 583 return super.getNamedProperty(_hash, _name, _checkValid); 584 } 585 586 } 587 588 @Override 589 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 590 switch (hash) { 591 case 951530617: 592 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 593 default: 594 return super.getProperty(hash, name, checkValid); 595 } 596 597 } 598 599 @Override 600 public Base setProperty(int hash, String name, Base value) throws FHIRException { 601 switch (hash) { 602 case 951530617: // content 603 this.content = castToType(value); // Type 604 return value; 605 default: 606 return super.setProperty(hash, name, value); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(String name, Base value) throws FHIRException { 613 if (name.equals("content[x]")) { 614 this.content = castToType(value); // Type 615 } else 616 return super.setProperty(name, value); 617 return value; 618 } 619 620 @Override 621 public void removeChild(String name, Base value) throws FHIRException { 622 if (name.equals("content[x]")) { 623 this.content = null; 624 } else 625 super.removeChild(name, value); 626 627 } 628 629 @Override 630 public Base makeProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case 264548711: 633 return getContent(); 634 case 951530617: 635 return getContent(); 636 default: 637 return super.makeProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 644 switch (hash) { 645 case 951530617: 646 /* content */ return new String[] { "string", "Attachment", "Reference" }; 647 default: 648 return super.getTypesForProperty(hash, name); 649 } 650 651 } 652 653 @Override 654 public Base addChild(String name) throws FHIRException { 655 if (name.equals("contentString")) { 656 this.content = new StringType(); 657 return this.content; 658 } else if (name.equals("contentAttachment")) { 659 this.content = new Attachment(); 660 return this.content; 661 } else if (name.equals("contentReference")) { 662 this.content = new Reference(); 663 return this.content; 664 } else 665 return super.addChild(name); 666 } 667 668 public CommunicationRequestPayloadComponent copy() { 669 CommunicationRequestPayloadComponent dst = new CommunicationRequestPayloadComponent(); 670 copyValues(dst); 671 return dst; 672 } 673 674 public void copyValues(CommunicationRequestPayloadComponent dst) { 675 super.copyValues(dst); 676 dst.content = content == null ? null : content.copy(); 677 } 678 679 @Override 680 public boolean equalsDeep(Base other_) { 681 if (!super.equalsDeep(other_)) 682 return false; 683 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 684 return false; 685 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 686 return compareDeep(content, o.content, true); 687 } 688 689 @Override 690 public boolean equalsShallow(Base other_) { 691 if (!super.equalsShallow(other_)) 692 return false; 693 if (!(other_ instanceof CommunicationRequestPayloadComponent)) 694 return false; 695 CommunicationRequestPayloadComponent o = (CommunicationRequestPayloadComponent) other_; 696 return true; 697 } 698 699 public boolean isEmpty() { 700 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 701 } 702 703 public String fhirType() { 704 return "CommunicationRequest.payload"; 705 706 } 707 708 } 709 710 /** 711 * Business identifiers assigned to this communication request by the performer 712 * or other systems which remain constant as the resource is updated and 713 * propagates from server to server. 714 */ 715 @Child(name = "identifier", type = { 716 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 717 @Description(shortDefinition = "Unique identifier", formalDefinition = "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 718 protected List<Identifier> identifier; 719 720 /** 721 * A plan or proposal that is fulfilled in whole or in part by this request. 722 */ 723 @Child(name = "basedOn", type = { 724 Reference.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 725 @Description(shortDefinition = "Fulfills plan or proposal", formalDefinition = "A plan or proposal that is fulfilled in whole or in part by this request.") 726 protected List<Reference> basedOn; 727 /** 728 * The actual objects that are the target of the reference (A plan or proposal 729 * that is fulfilled in whole or in part by this request.) 730 */ 731 protected List<Resource> basedOnTarget; 732 733 /** 734 * Completed or terminated request(s) whose function is taken by this new 735 * request. 736 */ 737 @Child(name = "replaces", type = { 738 CommunicationRequest.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 739 @Description(shortDefinition = "Request(s) replaced by this request", formalDefinition = "Completed or terminated request(s) whose function is taken by this new request.") 740 protected List<Reference> replaces; 741 /** 742 * The actual objects that are the target of the reference (Completed or 743 * terminated request(s) whose function is taken by this new request.) 744 */ 745 protected List<CommunicationRequest> replacesTarget; 746 747 /** 748 * A shared identifier common to all requests that were authorized more or less 749 * simultaneously by a single author, representing the identifier of the 750 * requisition, prescription or similar form. 751 */ 752 @Child(name = "groupIdentifier", type = { 753 Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 754 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.") 755 protected Identifier groupIdentifier; 756 757 /** 758 * The status of the proposal or order. 759 */ 760 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 761 @Description(shortDefinition = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", formalDefinition = "The status of the proposal or order.") 762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-status") 763 protected Enumeration<CommunicationRequestStatus> status; 764 765 /** 766 * Captures the reason for the current state of the CommunicationRequest. 767 */ 768 @Child(name = "statusReason", type = { 769 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 770 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the CommunicationRequest.") 771 protected CodeableConcept statusReason; 772 773 /** 774 * The type of message to be sent such as alert, notification, reminder, 775 * instruction, etc. 776 */ 777 @Child(name = "category", type = { 778 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 779 @Description(shortDefinition = "Message category", formalDefinition = "The type of message to be sent such as alert, notification, reminder, instruction, etc.") 780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/communication-category") 781 protected List<CodeableConcept> category; 782 783 /** 784 * Characterizes how quickly the proposed act must be initiated. Includes 785 * concepts such as stat, urgent, routine. 786 */ 787 @Child(name = "priority", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 788 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.") 789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 790 protected Enumeration<CommunicationPriority> priority; 791 792 /** 793 * If true indicates that the CommunicationRequest is asking for the specified 794 * action to *not* occur. 795 */ 796 @Child(name = "doNotPerform", type = { 797 BooleanType.class }, order = 8, min = 0, max = 1, modifier = true, summary = true) 798 @Description(shortDefinition = "True if request is prohibiting action", formalDefinition = "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.") 799 protected BooleanType doNotPerform; 800 801 /** 802 * A channel that was used for this communication (e.g. email, fax). 803 */ 804 @Child(name = "medium", type = { 805 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 806 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 807 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ParticipationMode") 808 protected List<CodeableConcept> medium; 809 810 /** 811 * The patient or group that is the focus of this communication request. 812 */ 813 @Child(name = "subject", type = { Patient.class, 814 Group.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 815 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient or group that is the focus of this communication request.") 816 protected Reference subject; 817 818 /** 819 * The actual object that is the target of the reference (The patient or group 820 * that is the focus of this communication request.) 821 */ 822 protected Resource subjectTarget; 823 824 /** 825 * Other resources that pertain to this communication request and to which this 826 * communication request should be associated. 827 */ 828 @Child(name = "about", type = { 829 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 830 @Description(shortDefinition = "Resources that pertain to this communication request", formalDefinition = "Other resources that pertain to this communication request and to which this communication request should be associated.") 831 protected List<Reference> about; 832 /** 833 * The actual objects that are the target of the reference (Other resources that 834 * pertain to this communication request and to which this communication request 835 * should be associated.) 836 */ 837 protected List<Resource> aboutTarget; 838 839 /** 840 * The Encounter during which this CommunicationRequest was created or to which 841 * the creation of this record is tightly associated. 842 */ 843 @Child(name = "encounter", type = { Encounter.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 844 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.") 845 protected Reference encounter; 846 847 /** 848 * The actual object that is the target of the reference (The Encounter during 849 * which this CommunicationRequest was created or to which the creation of this 850 * record is tightly associated.) 851 */ 852 protected Encounter encounterTarget; 853 854 /** 855 * Text, attachment(s), or resource(s) to be communicated to the recipient. 856 */ 857 @Child(name = "payload", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 858 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) to be communicated to the recipient.") 859 protected List<CommunicationRequestPayloadComponent> payload; 860 861 /** 862 * The time when this communication is to occur. 863 */ 864 @Child(name = "occurrence", type = { DateTimeType.class, 865 Period.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 866 @Description(shortDefinition = "When scheduled", formalDefinition = "The time when this communication is to occur.") 867 protected Type occurrence; 868 869 /** 870 * For draft requests, indicates the date of initial creation. For requests with 871 * other statuses, indicates the date of activation. 872 */ 873 @Child(name = "authoredOn", type = { 874 DateTimeType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 875 @Description(shortDefinition = "When request transitioned to being actionable", formalDefinition = "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.") 876 protected DateTimeType authoredOn; 877 878 /** 879 * The device, individual, or organization who initiated the request and has 880 * responsibility for its activation. 881 */ 882 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 883 RelatedPerson.class, Device.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 884 @Description(shortDefinition = "Who/what is requesting service", formalDefinition = "The device, individual, or organization who initiated the request and has responsibility for its activation.") 885 protected Reference requester; 886 887 /** 888 * The actual object that is the target of the reference (The device, 889 * individual, or organization who initiated the request and has responsibility 890 * for its activation.) 891 */ 892 protected Resource requesterTarget; 893 894 /** 895 * The entity (e.g. person, organization, clinical information system, device, 896 * group, or care team) which is the intended target of the communication. 897 */ 898 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 899 PractitionerRole.class, RelatedPerson.class, Group.class, CareTeam.class, 900 HealthcareService.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 901 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.") 902 protected List<Reference> recipient; 903 /** 904 * The actual objects that are the target of the reference (The entity (e.g. 905 * person, organization, clinical information system, device, group, or care 906 * team) which is the intended target of the communication.) 907 */ 908 protected List<Resource> recipientTarget; 909 910 /** 911 * The entity (e.g. person, organization, clinical information system, or 912 * device) which is to be the source of the communication. 913 */ 914 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 915 PractitionerRole.class, RelatedPerson.class, 916 HealthcareService.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 917 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.") 918 protected Reference sender; 919 920 /** 921 * The actual object that is the target of the reference (The entity (e.g. 922 * person, organization, clinical information system, or device) which is to be 923 * the source of the communication.) 924 */ 925 protected Resource senderTarget; 926 927 /** 928 * Describes why the request is being made in coded or textual form. 929 */ 930 @Child(name = "reasonCode", type = { 931 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 932 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Describes why the request is being made in coded or textual form.") 933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActReason") 934 protected List<CodeableConcept> reasonCode; 935 936 /** 937 * Indicates another resource whose existence justifies this request. 938 */ 939 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 940 DocumentReference.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 941 @Description(shortDefinition = "Why is communication needed?", formalDefinition = "Indicates another resource whose existence justifies this request.") 942 protected List<Reference> reasonReference; 943 /** 944 * The actual objects that are the target of the reference (Indicates another 945 * resource whose existence justifies this request.) 946 */ 947 protected List<Resource> reasonReferenceTarget; 948 949 /** 950 * Comments made about the request by the requester, sender, recipient, subject 951 * or other participants. 952 */ 953 @Child(name = "note", type = { 954 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 955 @Description(shortDefinition = "Comments made about communication request", formalDefinition = "Comments made about the request by the requester, sender, recipient, subject or other participants.") 956 protected List<Annotation> note; 957 958 private static final long serialVersionUID = 2131096857L; 959 960 /** 961 * Constructor 962 */ 963 public CommunicationRequest() { 964 super(); 965 } 966 967 /** 968 * Constructor 969 */ 970 public CommunicationRequest(Enumeration<CommunicationRequestStatus> status) { 971 super(); 972 this.status = status; 973 } 974 975 /** 976 * @return {@link #identifier} (Business identifiers assigned to this 977 * communication request by the performer or other systems which remain 978 * constant as the resource is updated and propagates from server to 979 * server.) 980 */ 981 public List<Identifier> getIdentifier() { 982 if (this.identifier == null) 983 this.identifier = new ArrayList<Identifier>(); 984 return this.identifier; 985 } 986 987 /** 988 * @return Returns a reference to <code>this</code> for easy method chaining 989 */ 990 public CommunicationRequest setIdentifier(List<Identifier> theIdentifier) { 991 this.identifier = theIdentifier; 992 return this; 993 } 994 995 public boolean hasIdentifier() { 996 if (this.identifier == null) 997 return false; 998 for (Identifier item : this.identifier) 999 if (!item.isEmpty()) 1000 return true; 1001 return false; 1002 } 1003 1004 public Identifier addIdentifier() { // 3 1005 Identifier t = new Identifier(); 1006 if (this.identifier == null) 1007 this.identifier = new ArrayList<Identifier>(); 1008 this.identifier.add(t); 1009 return t; 1010 } 1011 1012 public CommunicationRequest addIdentifier(Identifier t) { // 3 1013 if (t == null) 1014 return this; 1015 if (this.identifier == null) 1016 this.identifier = new ArrayList<Identifier>(); 1017 this.identifier.add(t); 1018 return this; 1019 } 1020 1021 /** 1022 * @return The first repetition of repeating field {@link #identifier}, creating 1023 * it if it does not already exist 1024 */ 1025 public Identifier getIdentifierFirstRep() { 1026 if (getIdentifier().isEmpty()) { 1027 addIdentifier(); 1028 } 1029 return getIdentifier().get(0); 1030 } 1031 1032 /** 1033 * @return {@link #basedOn} (A plan or proposal that is fulfilled in whole or in 1034 * part by this request.) 1035 */ 1036 public List<Reference> getBasedOn() { 1037 if (this.basedOn == null) 1038 this.basedOn = new ArrayList<Reference>(); 1039 return this.basedOn; 1040 } 1041 1042 /** 1043 * @return Returns a reference to <code>this</code> for easy method chaining 1044 */ 1045 public CommunicationRequest setBasedOn(List<Reference> theBasedOn) { 1046 this.basedOn = theBasedOn; 1047 return this; 1048 } 1049 1050 public boolean hasBasedOn() { 1051 if (this.basedOn == null) 1052 return false; 1053 for (Reference item : this.basedOn) 1054 if (!item.isEmpty()) 1055 return true; 1056 return false; 1057 } 1058 1059 public Reference addBasedOn() { // 3 1060 Reference t = new Reference(); 1061 if (this.basedOn == null) 1062 this.basedOn = new ArrayList<Reference>(); 1063 this.basedOn.add(t); 1064 return t; 1065 } 1066 1067 public CommunicationRequest addBasedOn(Reference t) { // 3 1068 if (t == null) 1069 return this; 1070 if (this.basedOn == null) 1071 this.basedOn = new ArrayList<Reference>(); 1072 this.basedOn.add(t); 1073 return this; 1074 } 1075 1076 /** 1077 * @return The first repetition of repeating field {@link #basedOn}, creating it 1078 * if it does not already exist 1079 */ 1080 public Reference getBasedOnFirstRep() { 1081 if (getBasedOn().isEmpty()) { 1082 addBasedOn(); 1083 } 1084 return getBasedOn().get(0); 1085 } 1086 1087 /** 1088 * @return {@link #replaces} (Completed or terminated request(s) whose function 1089 * is taken by this new request.) 1090 */ 1091 public List<Reference> getReplaces() { 1092 if (this.replaces == null) 1093 this.replaces = new ArrayList<Reference>(); 1094 return this.replaces; 1095 } 1096 1097 /** 1098 * @return Returns a reference to <code>this</code> for easy method chaining 1099 */ 1100 public CommunicationRequest setReplaces(List<Reference> theReplaces) { 1101 this.replaces = theReplaces; 1102 return this; 1103 } 1104 1105 public boolean hasReplaces() { 1106 if (this.replaces == null) 1107 return false; 1108 for (Reference item : this.replaces) 1109 if (!item.isEmpty()) 1110 return true; 1111 return false; 1112 } 1113 1114 public Reference addReplaces() { // 3 1115 Reference t = new Reference(); 1116 if (this.replaces == null) 1117 this.replaces = new ArrayList<Reference>(); 1118 this.replaces.add(t); 1119 return t; 1120 } 1121 1122 public CommunicationRequest addReplaces(Reference t) { // 3 1123 if (t == null) 1124 return this; 1125 if (this.replaces == null) 1126 this.replaces = new ArrayList<Reference>(); 1127 this.replaces.add(t); 1128 return this; 1129 } 1130 1131 /** 1132 * @return The first repetition of repeating field {@link #replaces}, creating 1133 * it if it does not already exist 1134 */ 1135 public Reference getReplacesFirstRep() { 1136 if (getReplaces().isEmpty()) { 1137 addReplaces(); 1138 } 1139 return getReplaces().get(0); 1140 } 1141 1142 /** 1143 * @return {@link #groupIdentifier} (A shared identifier common to all requests 1144 * that were authorized more or less simultaneously by a single author, 1145 * representing the identifier of the requisition, prescription or 1146 * similar form.) 1147 */ 1148 public Identifier getGroupIdentifier() { 1149 if (this.groupIdentifier == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create CommunicationRequest.groupIdentifier"); 1152 else if (Configuration.doAutoCreate()) 1153 this.groupIdentifier = new Identifier(); // cc 1154 return this.groupIdentifier; 1155 } 1156 1157 public boolean hasGroupIdentifier() { 1158 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #groupIdentifier} (A shared identifier common to all 1163 * requests that were authorized more or less simultaneously by a 1164 * single author, representing the identifier of the requisition, 1165 * prescription or similar form.) 1166 */ 1167 public CommunicationRequest setGroupIdentifier(Identifier value) { 1168 this.groupIdentifier = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #status} (The status of the proposal or order.). This is the 1174 * underlying object with id, value and extensions. The accessor 1175 * "getStatus" gives direct access to the value 1176 */ 1177 public Enumeration<CommunicationRequestStatus> getStatusElement() { 1178 if (this.status == null) 1179 if (Configuration.errorOnAutoCreate()) 1180 throw new Error("Attempt to auto-create CommunicationRequest.status"); 1181 else if (Configuration.doAutoCreate()) 1182 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); // bb 1183 return this.status; 1184 } 1185 1186 public boolean hasStatusElement() { 1187 return this.status != null && !this.status.isEmpty(); 1188 } 1189 1190 public boolean hasStatus() { 1191 return this.status != null && !this.status.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #status} (The status of the proposal or order.). This is 1196 * the underlying object with id, value and extensions. The 1197 * accessor "getStatus" gives direct access to the value 1198 */ 1199 public CommunicationRequest setStatusElement(Enumeration<CommunicationRequestStatus> value) { 1200 this.status = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return The status of the proposal or order. 1206 */ 1207 public CommunicationRequestStatus getStatus() { 1208 return this.status == null ? null : this.status.getValue(); 1209 } 1210 1211 /** 1212 * @param value The status of the proposal or order. 1213 */ 1214 public CommunicationRequest setStatus(CommunicationRequestStatus value) { 1215 if (this.status == null) 1216 this.status = new Enumeration<CommunicationRequestStatus>(new CommunicationRequestStatusEnumFactory()); 1217 this.status.setValue(value); 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #statusReason} (Captures the reason for the current state of 1223 * the CommunicationRequest.) 1224 */ 1225 public CodeableConcept getStatusReason() { 1226 if (this.statusReason == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create CommunicationRequest.statusReason"); 1229 else if (Configuration.doAutoCreate()) 1230 this.statusReason = new CodeableConcept(); // cc 1231 return this.statusReason; 1232 } 1233 1234 public boolean hasStatusReason() { 1235 return this.statusReason != null && !this.statusReason.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #statusReason} (Captures the reason for the current state 1240 * of the CommunicationRequest.) 1241 */ 1242 public CommunicationRequest setStatusReason(CodeableConcept value) { 1243 this.statusReason = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #category} (The type of message to be sent such as alert, 1249 * notification, reminder, instruction, etc.) 1250 */ 1251 public List<CodeableConcept> getCategory() { 1252 if (this.category == null) 1253 this.category = new ArrayList<CodeableConcept>(); 1254 return this.category; 1255 } 1256 1257 /** 1258 * @return Returns a reference to <code>this</code> for easy method chaining 1259 */ 1260 public CommunicationRequest setCategory(List<CodeableConcept> theCategory) { 1261 this.category = theCategory; 1262 return this; 1263 } 1264 1265 public boolean hasCategory() { 1266 if (this.category == null) 1267 return false; 1268 for (CodeableConcept item : this.category) 1269 if (!item.isEmpty()) 1270 return true; 1271 return false; 1272 } 1273 1274 public CodeableConcept addCategory() { // 3 1275 CodeableConcept t = new CodeableConcept(); 1276 if (this.category == null) 1277 this.category = new ArrayList<CodeableConcept>(); 1278 this.category.add(t); 1279 return t; 1280 } 1281 1282 public CommunicationRequest addCategory(CodeableConcept t) { // 3 1283 if (t == null) 1284 return this; 1285 if (this.category == null) 1286 this.category = new ArrayList<CodeableConcept>(); 1287 this.category.add(t); 1288 return this; 1289 } 1290 1291 /** 1292 * @return The first repetition of repeating field {@link #category}, creating 1293 * it if it does not already exist 1294 */ 1295 public CodeableConcept getCategoryFirstRep() { 1296 if (getCategory().isEmpty()) { 1297 addCategory(); 1298 } 1299 return getCategory().get(0); 1300 } 1301 1302 /** 1303 * @return {@link #priority} (Characterizes how quickly the proposed act must be 1304 * initiated. Includes concepts such as stat, urgent, routine.). This is 1305 * the underlying object with id, value and extensions. The accessor 1306 * "getPriority" gives direct access to the value 1307 */ 1308 public Enumeration<CommunicationPriority> getPriorityElement() { 1309 if (this.priority == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create CommunicationRequest.priority"); 1312 else if (Configuration.doAutoCreate()) 1313 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); // bb 1314 return this.priority; 1315 } 1316 1317 public boolean hasPriorityElement() { 1318 return this.priority != null && !this.priority.isEmpty(); 1319 } 1320 1321 public boolean hasPriority() { 1322 return this.priority != null && !this.priority.isEmpty(); 1323 } 1324 1325 /** 1326 * @param value {@link #priority} (Characterizes how quickly the proposed act 1327 * must be initiated. Includes concepts such as stat, urgent, 1328 * routine.). This is the underlying object with id, value and 1329 * extensions. The accessor "getPriority" gives direct access to 1330 * the value 1331 */ 1332 public CommunicationRequest setPriorityElement(Enumeration<CommunicationPriority> value) { 1333 this.priority = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return Characterizes how quickly the proposed act must be initiated. 1339 * Includes concepts such as stat, urgent, routine. 1340 */ 1341 public CommunicationPriority getPriority() { 1342 return this.priority == null ? null : this.priority.getValue(); 1343 } 1344 1345 /** 1346 * @param value Characterizes how quickly the proposed act must be initiated. 1347 * Includes concepts such as stat, urgent, routine. 1348 */ 1349 public CommunicationRequest setPriority(CommunicationPriority value) { 1350 if (value == null) 1351 this.priority = null; 1352 else { 1353 if (this.priority == null) 1354 this.priority = new Enumeration<CommunicationPriority>(new CommunicationPriorityEnumFactory()); 1355 this.priority.setValue(value); 1356 } 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #doNotPerform} (If true indicates that the 1362 * CommunicationRequest is asking for the specified action to *not* 1363 * occur.). This is the underlying object with id, value and extensions. 1364 * The accessor "getDoNotPerform" gives direct access to the value 1365 */ 1366 public BooleanType getDoNotPerformElement() { 1367 if (this.doNotPerform == null) 1368 if (Configuration.errorOnAutoCreate()) 1369 throw new Error("Attempt to auto-create CommunicationRequest.doNotPerform"); 1370 else if (Configuration.doAutoCreate()) 1371 this.doNotPerform = new BooleanType(); // bb 1372 return this.doNotPerform; 1373 } 1374 1375 public boolean hasDoNotPerformElement() { 1376 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1377 } 1378 1379 public boolean hasDoNotPerform() { 1380 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 1381 } 1382 1383 /** 1384 * @param value {@link #doNotPerform} (If true indicates that the 1385 * CommunicationRequest is asking for the specified action to *not* 1386 * occur.). This is the underlying object with id, value and 1387 * extensions. The accessor "getDoNotPerform" gives direct access 1388 * to the value 1389 */ 1390 public CommunicationRequest setDoNotPerformElement(BooleanType value) { 1391 this.doNotPerform = value; 1392 return this; 1393 } 1394 1395 /** 1396 * @return If true indicates that the CommunicationRequest is asking for the 1397 * specified action to *not* occur. 1398 */ 1399 public boolean getDoNotPerform() { 1400 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 1401 } 1402 1403 /** 1404 * @param value If true indicates that the CommunicationRequest is asking for 1405 * the specified action to *not* occur. 1406 */ 1407 public CommunicationRequest setDoNotPerform(boolean value) { 1408 if (this.doNotPerform == null) 1409 this.doNotPerform = new BooleanType(); 1410 this.doNotPerform.setValue(value); 1411 return this; 1412 } 1413 1414 /** 1415 * @return {@link #medium} (A channel that was used for this communication (e.g. 1416 * email, fax).) 1417 */ 1418 public List<CodeableConcept> getMedium() { 1419 if (this.medium == null) 1420 this.medium = new ArrayList<CodeableConcept>(); 1421 return this.medium; 1422 } 1423 1424 /** 1425 * @return Returns a reference to <code>this</code> for easy method chaining 1426 */ 1427 public CommunicationRequest setMedium(List<CodeableConcept> theMedium) { 1428 this.medium = theMedium; 1429 return this; 1430 } 1431 1432 public boolean hasMedium() { 1433 if (this.medium == null) 1434 return false; 1435 for (CodeableConcept item : this.medium) 1436 if (!item.isEmpty()) 1437 return true; 1438 return false; 1439 } 1440 1441 public CodeableConcept addMedium() { // 3 1442 CodeableConcept t = new CodeableConcept(); 1443 if (this.medium == null) 1444 this.medium = new ArrayList<CodeableConcept>(); 1445 this.medium.add(t); 1446 return t; 1447 } 1448 1449 public CommunicationRequest addMedium(CodeableConcept t) { // 3 1450 if (t == null) 1451 return this; 1452 if (this.medium == null) 1453 this.medium = new ArrayList<CodeableConcept>(); 1454 this.medium.add(t); 1455 return this; 1456 } 1457 1458 /** 1459 * @return The first repetition of repeating field {@link #medium}, creating it 1460 * if it does not already exist 1461 */ 1462 public CodeableConcept getMediumFirstRep() { 1463 if (getMedium().isEmpty()) { 1464 addMedium(); 1465 } 1466 return getMedium().get(0); 1467 } 1468 1469 /** 1470 * @return {@link #subject} (The patient or group that is the focus of this 1471 * communication request.) 1472 */ 1473 public Reference getSubject() { 1474 if (this.subject == null) 1475 if (Configuration.errorOnAutoCreate()) 1476 throw new Error("Attempt to auto-create CommunicationRequest.subject"); 1477 else if (Configuration.doAutoCreate()) 1478 this.subject = new Reference(); // cc 1479 return this.subject; 1480 } 1481 1482 public boolean hasSubject() { 1483 return this.subject != null && !this.subject.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #subject} (The patient or group that is the focus of this 1488 * communication request.) 1489 */ 1490 public CommunicationRequest setSubject(Reference value) { 1491 this.subject = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #subject} The actual object that is the target of the 1497 * reference. The reference library doesn't populate this, but you can 1498 * use it to hold the resource if you resolve it. (The patient or group 1499 * that is the focus of this communication request.) 1500 */ 1501 public Resource getSubjectTarget() { 1502 return this.subjectTarget; 1503 } 1504 1505 /** 1506 * @param value {@link #subject} The actual object that is the target of the 1507 * reference. The reference library doesn't use these, but you can 1508 * use it to hold the resource if you resolve it. (The patient or 1509 * group that is the focus of this communication request.) 1510 */ 1511 public CommunicationRequest setSubjectTarget(Resource value) { 1512 this.subjectTarget = value; 1513 return this; 1514 } 1515 1516 /** 1517 * @return {@link #about} (Other resources that pertain to this communication 1518 * request and to which this communication request should be 1519 * associated.) 1520 */ 1521 public List<Reference> getAbout() { 1522 if (this.about == null) 1523 this.about = new ArrayList<Reference>(); 1524 return this.about; 1525 } 1526 1527 /** 1528 * @return Returns a reference to <code>this</code> for easy method chaining 1529 */ 1530 public CommunicationRequest setAbout(List<Reference> theAbout) { 1531 this.about = theAbout; 1532 return this; 1533 } 1534 1535 public boolean hasAbout() { 1536 if (this.about == null) 1537 return false; 1538 for (Reference item : this.about) 1539 if (!item.isEmpty()) 1540 return true; 1541 return false; 1542 } 1543 1544 public Reference addAbout() { // 3 1545 Reference t = new Reference(); 1546 if (this.about == null) 1547 this.about = new ArrayList<Reference>(); 1548 this.about.add(t); 1549 return t; 1550 } 1551 1552 public CommunicationRequest addAbout(Reference t) { // 3 1553 if (t == null) 1554 return this; 1555 if (this.about == null) 1556 this.about = new ArrayList<Reference>(); 1557 this.about.add(t); 1558 return this; 1559 } 1560 1561 /** 1562 * @return The first repetition of repeating field {@link #about}, creating it 1563 * if it does not already exist 1564 */ 1565 public Reference getAboutFirstRep() { 1566 if (getAbout().isEmpty()) { 1567 addAbout(); 1568 } 1569 return getAbout().get(0); 1570 } 1571 1572 /** 1573 * @return {@link #encounter} (The Encounter during which this 1574 * CommunicationRequest was created or to which the creation of this 1575 * record is tightly associated.) 1576 */ 1577 public Reference getEncounter() { 1578 if (this.encounter == null) 1579 if (Configuration.errorOnAutoCreate()) 1580 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1581 else if (Configuration.doAutoCreate()) 1582 this.encounter = new Reference(); // cc 1583 return this.encounter; 1584 } 1585 1586 public boolean hasEncounter() { 1587 return this.encounter != null && !this.encounter.isEmpty(); 1588 } 1589 1590 /** 1591 * @param value {@link #encounter} (The Encounter during which this 1592 * CommunicationRequest was created or to which the creation of 1593 * this record is tightly associated.) 1594 */ 1595 public CommunicationRequest setEncounter(Reference value) { 1596 this.encounter = value; 1597 return this; 1598 } 1599 1600 /** 1601 * @return {@link #encounter} The actual object that is the target of the 1602 * reference. The reference library doesn't populate this, but you can 1603 * use it to hold the resource if you resolve it. (The Encounter during 1604 * which this CommunicationRequest was created or to which the creation 1605 * of this record is tightly associated.) 1606 */ 1607 public Encounter getEncounterTarget() { 1608 if (this.encounterTarget == null) 1609 if (Configuration.errorOnAutoCreate()) 1610 throw new Error("Attempt to auto-create CommunicationRequest.encounter"); 1611 else if (Configuration.doAutoCreate()) 1612 this.encounterTarget = new Encounter(); // aa 1613 return this.encounterTarget; 1614 } 1615 1616 /** 1617 * @param value {@link #encounter} The actual object that is the target of the 1618 * reference. The reference library doesn't use these, but you can 1619 * use it to hold the resource if you resolve it. (The Encounter 1620 * during which this CommunicationRequest was created or to which 1621 * the creation of this record is tightly associated.) 1622 */ 1623 public CommunicationRequest setEncounterTarget(Encounter value) { 1624 this.encounterTarget = value; 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #payload} (Text, attachment(s), or resource(s) to be 1630 * communicated to the recipient.) 1631 */ 1632 public List<CommunicationRequestPayloadComponent> getPayload() { 1633 if (this.payload == null) 1634 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1635 return this.payload; 1636 } 1637 1638 /** 1639 * @return Returns a reference to <code>this</code> for easy method chaining 1640 */ 1641 public CommunicationRequest setPayload(List<CommunicationRequestPayloadComponent> thePayload) { 1642 this.payload = thePayload; 1643 return this; 1644 } 1645 1646 public boolean hasPayload() { 1647 if (this.payload == null) 1648 return false; 1649 for (CommunicationRequestPayloadComponent item : this.payload) 1650 if (!item.isEmpty()) 1651 return true; 1652 return false; 1653 } 1654 1655 public CommunicationRequestPayloadComponent addPayload() { // 3 1656 CommunicationRequestPayloadComponent t = new CommunicationRequestPayloadComponent(); 1657 if (this.payload == null) 1658 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1659 this.payload.add(t); 1660 return t; 1661 } 1662 1663 public CommunicationRequest addPayload(CommunicationRequestPayloadComponent t) { // 3 1664 if (t == null) 1665 return this; 1666 if (this.payload == null) 1667 this.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 1668 this.payload.add(t); 1669 return this; 1670 } 1671 1672 /** 1673 * @return The first repetition of repeating field {@link #payload}, creating it 1674 * if it does not already exist 1675 */ 1676 public CommunicationRequestPayloadComponent getPayloadFirstRep() { 1677 if (getPayload().isEmpty()) { 1678 addPayload(); 1679 } 1680 return getPayload().get(0); 1681 } 1682 1683 /** 1684 * @return {@link #occurrence} (The time when this communication is to occur.) 1685 */ 1686 public Type getOccurrence() { 1687 return this.occurrence; 1688 } 1689 1690 /** 1691 * @return {@link #occurrence} (The time when this communication is to occur.) 1692 */ 1693 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 1694 if (this.occurrence == null) 1695 this.occurrence = new DateTimeType(); 1696 if (!(this.occurrence instanceof DateTimeType)) 1697 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1698 + this.occurrence.getClass().getName() + " was encountered"); 1699 return (DateTimeType) this.occurrence; 1700 } 1701 1702 public boolean hasOccurrenceDateTimeType() { 1703 return this.occurrence instanceof DateTimeType; 1704 } 1705 1706 /** 1707 * @return {@link #occurrence} (The time when this communication is to occur.) 1708 */ 1709 public Period getOccurrencePeriod() throws FHIRException { 1710 if (this.occurrence == null) 1711 this.occurrence = new Period(); 1712 if (!(this.occurrence instanceof Period)) 1713 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.occurrence.getClass().getName() 1714 + " was encountered"); 1715 return (Period) this.occurrence; 1716 } 1717 1718 public boolean hasOccurrencePeriod() { 1719 return this.occurrence instanceof Period; 1720 } 1721 1722 public boolean hasOccurrence() { 1723 return this.occurrence != null && !this.occurrence.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #occurrence} (The time when this communication is to 1728 * occur.) 1729 */ 1730 public CommunicationRequest setOccurrence(Type value) { 1731 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1732 throw new Error("Not the right type for CommunicationRequest.occurrence[x]: " + value.fhirType()); 1733 this.occurrence = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return {@link #authoredOn} (For draft requests, indicates the date of 1739 * initial creation. For requests with other statuses, indicates the 1740 * date of activation.). This is the underlying object with id, value 1741 * and extensions. The accessor "getAuthoredOn" gives direct access to 1742 * the value 1743 */ 1744 public DateTimeType getAuthoredOnElement() { 1745 if (this.authoredOn == null) 1746 if (Configuration.errorOnAutoCreate()) 1747 throw new Error("Attempt to auto-create CommunicationRequest.authoredOn"); 1748 else if (Configuration.doAutoCreate()) 1749 this.authoredOn = new DateTimeType(); // bb 1750 return this.authoredOn; 1751 } 1752 1753 public boolean hasAuthoredOnElement() { 1754 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1755 } 1756 1757 public boolean hasAuthoredOn() { 1758 return this.authoredOn != null && !this.authoredOn.isEmpty(); 1759 } 1760 1761 /** 1762 * @param value {@link #authoredOn} (For draft requests, indicates the date of 1763 * initial creation. For requests with other statuses, indicates 1764 * the date of activation.). This is the underlying object with id, 1765 * value and extensions. The accessor "getAuthoredOn" gives direct 1766 * access to the value 1767 */ 1768 public CommunicationRequest setAuthoredOnElement(DateTimeType value) { 1769 this.authoredOn = value; 1770 return this; 1771 } 1772 1773 /** 1774 * @return For draft requests, indicates the date of initial creation. For 1775 * requests with other statuses, indicates the date of activation. 1776 */ 1777 public Date getAuthoredOn() { 1778 return this.authoredOn == null ? null : this.authoredOn.getValue(); 1779 } 1780 1781 /** 1782 * @param value For draft requests, indicates the date of initial creation. For 1783 * requests with other statuses, indicates the date of activation. 1784 */ 1785 public CommunicationRequest setAuthoredOn(Date value) { 1786 if (value == null) 1787 this.authoredOn = null; 1788 else { 1789 if (this.authoredOn == null) 1790 this.authoredOn = new DateTimeType(); 1791 this.authoredOn.setValue(value); 1792 } 1793 return this; 1794 } 1795 1796 /** 1797 * @return {@link #requester} (The device, individual, or organization who 1798 * initiated the request and has responsibility for its activation.) 1799 */ 1800 public Reference getRequester() { 1801 if (this.requester == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create CommunicationRequest.requester"); 1804 else if (Configuration.doAutoCreate()) 1805 this.requester = new Reference(); // cc 1806 return this.requester; 1807 } 1808 1809 public boolean hasRequester() { 1810 return this.requester != null && !this.requester.isEmpty(); 1811 } 1812 1813 /** 1814 * @param value {@link #requester} (The device, individual, or organization who 1815 * initiated the request and has responsibility for its 1816 * activation.) 1817 */ 1818 public CommunicationRequest setRequester(Reference value) { 1819 this.requester = value; 1820 return this; 1821 } 1822 1823 /** 1824 * @return {@link #requester} The actual object that is the target of the 1825 * reference. The reference library doesn't populate this, but you can 1826 * use it to hold the resource if you resolve it. (The device, 1827 * individual, or organization who initiated the request and has 1828 * responsibility for its activation.) 1829 */ 1830 public Resource getRequesterTarget() { 1831 return this.requesterTarget; 1832 } 1833 1834 /** 1835 * @param value {@link #requester} The actual object that is the target of the 1836 * reference. The reference library doesn't use these, but you can 1837 * use it to hold the resource if you resolve it. (The device, 1838 * individual, or organization who initiated the request and has 1839 * responsibility for its activation.) 1840 */ 1841 public CommunicationRequest setRequesterTarget(Resource value) { 1842 this.requesterTarget = value; 1843 return this; 1844 } 1845 1846 /** 1847 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 1848 * information system, device, group, or care team) which is the 1849 * intended target of the communication.) 1850 */ 1851 public List<Reference> getRecipient() { 1852 if (this.recipient == null) 1853 this.recipient = new ArrayList<Reference>(); 1854 return this.recipient; 1855 } 1856 1857 /** 1858 * @return Returns a reference to <code>this</code> for easy method chaining 1859 */ 1860 public CommunicationRequest setRecipient(List<Reference> theRecipient) { 1861 this.recipient = theRecipient; 1862 return this; 1863 } 1864 1865 public boolean hasRecipient() { 1866 if (this.recipient == null) 1867 return false; 1868 for (Reference item : this.recipient) 1869 if (!item.isEmpty()) 1870 return true; 1871 return false; 1872 } 1873 1874 public Reference addRecipient() { // 3 1875 Reference t = new Reference(); 1876 if (this.recipient == null) 1877 this.recipient = new ArrayList<Reference>(); 1878 this.recipient.add(t); 1879 return t; 1880 } 1881 1882 public CommunicationRequest addRecipient(Reference t) { // 3 1883 if (t == null) 1884 return this; 1885 if (this.recipient == null) 1886 this.recipient = new ArrayList<Reference>(); 1887 this.recipient.add(t); 1888 return this; 1889 } 1890 1891 /** 1892 * @return The first repetition of repeating field {@link #recipient}, creating 1893 * it if it does not already exist 1894 */ 1895 public Reference getRecipientFirstRep() { 1896 if (getRecipient().isEmpty()) { 1897 addRecipient(); 1898 } 1899 return getRecipient().get(0); 1900 } 1901 1902 /** 1903 * @return {@link #sender} (The entity (e.g. person, organization, clinical 1904 * information system, or device) which is to be the source of the 1905 * communication.) 1906 */ 1907 public Reference getSender() { 1908 if (this.sender == null) 1909 if (Configuration.errorOnAutoCreate()) 1910 throw new Error("Attempt to auto-create CommunicationRequest.sender"); 1911 else if (Configuration.doAutoCreate()) 1912 this.sender = new Reference(); // cc 1913 return this.sender; 1914 } 1915 1916 public boolean hasSender() { 1917 return this.sender != null && !this.sender.isEmpty(); 1918 } 1919 1920 /** 1921 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 1922 * information system, or device) which is to be the source of the 1923 * communication.) 1924 */ 1925 public CommunicationRequest setSender(Reference value) { 1926 this.sender = value; 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #sender} The actual object that is the target of the 1932 * reference. The reference library doesn't populate this, but you can 1933 * use it to hold the resource if you resolve it. (The entity (e.g. 1934 * person, organization, clinical information system, or device) which 1935 * is to be the source of the communication.) 1936 */ 1937 public Resource getSenderTarget() { 1938 return this.senderTarget; 1939 } 1940 1941 /** 1942 * @param value {@link #sender} The actual object that is the target of the 1943 * reference. The reference library doesn't use these, but you can 1944 * use it to hold the resource if you resolve it. (The entity (e.g. 1945 * person, organization, clinical information system, or device) 1946 * which is to be the source of the communication.) 1947 */ 1948 public CommunicationRequest setSenderTarget(Resource value) { 1949 this.senderTarget = value; 1950 return this; 1951 } 1952 1953 /** 1954 * @return {@link #reasonCode} (Describes why the request is being made in coded 1955 * or textual form.) 1956 */ 1957 public List<CodeableConcept> getReasonCode() { 1958 if (this.reasonCode == null) 1959 this.reasonCode = new ArrayList<CodeableConcept>(); 1960 return this.reasonCode; 1961 } 1962 1963 /** 1964 * @return Returns a reference to <code>this</code> for easy method chaining 1965 */ 1966 public CommunicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 1967 this.reasonCode = theReasonCode; 1968 return this; 1969 } 1970 1971 public boolean hasReasonCode() { 1972 if (this.reasonCode == null) 1973 return false; 1974 for (CodeableConcept item : this.reasonCode) 1975 if (!item.isEmpty()) 1976 return true; 1977 return false; 1978 } 1979 1980 public CodeableConcept addReasonCode() { // 3 1981 CodeableConcept t = new CodeableConcept(); 1982 if (this.reasonCode == null) 1983 this.reasonCode = new ArrayList<CodeableConcept>(); 1984 this.reasonCode.add(t); 1985 return t; 1986 } 1987 1988 public CommunicationRequest addReasonCode(CodeableConcept t) { // 3 1989 if (t == null) 1990 return this; 1991 if (this.reasonCode == null) 1992 this.reasonCode = new ArrayList<CodeableConcept>(); 1993 this.reasonCode.add(t); 1994 return this; 1995 } 1996 1997 /** 1998 * @return The first repetition of repeating field {@link #reasonCode}, creating 1999 * it if it does not already exist 2000 */ 2001 public CodeableConcept getReasonCodeFirstRep() { 2002 if (getReasonCode().isEmpty()) { 2003 addReasonCode(); 2004 } 2005 return getReasonCode().get(0); 2006 } 2007 2008 /** 2009 * @return {@link #reasonReference} (Indicates another resource whose existence 2010 * justifies this request.) 2011 */ 2012 public List<Reference> getReasonReference() { 2013 if (this.reasonReference == null) 2014 this.reasonReference = new ArrayList<Reference>(); 2015 return this.reasonReference; 2016 } 2017 2018 /** 2019 * @return Returns a reference to <code>this</code> for easy method chaining 2020 */ 2021 public CommunicationRequest setReasonReference(List<Reference> theReasonReference) { 2022 this.reasonReference = theReasonReference; 2023 return this; 2024 } 2025 2026 public boolean hasReasonReference() { 2027 if (this.reasonReference == null) 2028 return false; 2029 for (Reference item : this.reasonReference) 2030 if (!item.isEmpty()) 2031 return true; 2032 return false; 2033 } 2034 2035 public Reference addReasonReference() { // 3 2036 Reference t = new Reference(); 2037 if (this.reasonReference == null) 2038 this.reasonReference = new ArrayList<Reference>(); 2039 this.reasonReference.add(t); 2040 return t; 2041 } 2042 2043 public CommunicationRequest addReasonReference(Reference t) { // 3 2044 if (t == null) 2045 return this; 2046 if (this.reasonReference == null) 2047 this.reasonReference = new ArrayList<Reference>(); 2048 this.reasonReference.add(t); 2049 return this; 2050 } 2051 2052 /** 2053 * @return The first repetition of repeating field {@link #reasonReference}, 2054 * creating it if it does not already exist 2055 */ 2056 public Reference getReasonReferenceFirstRep() { 2057 if (getReasonReference().isEmpty()) { 2058 addReasonReference(); 2059 } 2060 return getReasonReference().get(0); 2061 } 2062 2063 /** 2064 * @return {@link #note} (Comments made about the request by the requester, 2065 * sender, recipient, subject or other participants.) 2066 */ 2067 public List<Annotation> getNote() { 2068 if (this.note == null) 2069 this.note = new ArrayList<Annotation>(); 2070 return this.note; 2071 } 2072 2073 /** 2074 * @return Returns a reference to <code>this</code> for easy method chaining 2075 */ 2076 public CommunicationRequest setNote(List<Annotation> theNote) { 2077 this.note = theNote; 2078 return this; 2079 } 2080 2081 public boolean hasNote() { 2082 if (this.note == null) 2083 return false; 2084 for (Annotation item : this.note) 2085 if (!item.isEmpty()) 2086 return true; 2087 return false; 2088 } 2089 2090 public Annotation addNote() { // 3 2091 Annotation t = new Annotation(); 2092 if (this.note == null) 2093 this.note = new ArrayList<Annotation>(); 2094 this.note.add(t); 2095 return t; 2096 } 2097 2098 public CommunicationRequest addNote(Annotation t) { // 3 2099 if (t == null) 2100 return this; 2101 if (this.note == null) 2102 this.note = new ArrayList<Annotation>(); 2103 this.note.add(t); 2104 return this; 2105 } 2106 2107 /** 2108 * @return The first repetition of repeating field {@link #note}, creating it if 2109 * it does not already exist 2110 */ 2111 public Annotation getNoteFirstRep() { 2112 if (getNote().isEmpty()) { 2113 addNote(); 2114 } 2115 return getNote().get(0); 2116 } 2117 2118 protected void listChildren(List<Property> children) { 2119 super.listChildren(children); 2120 children.add(new Property("identifier", "Identifier", 2121 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2122 0, java.lang.Integer.MAX_VALUE, identifier)); 2123 children.add(new Property("basedOn", "Reference(Any)", 2124 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2125 basedOn)); 2126 children.add(new Property("replaces", "Reference(CommunicationRequest)", 2127 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2128 java.lang.Integer.MAX_VALUE, replaces)); 2129 children.add(new Property("groupIdentifier", "Identifier", 2130 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2131 0, 1, groupIdentifier)); 2132 children.add(new Property("status", "code", "The status of the proposal or order.", 0, 1, status)); 2133 children.add(new Property("statusReason", "CodeableConcept", 2134 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason)); 2135 children.add(new Property("category", "CodeableConcept", 2136 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2137 java.lang.Integer.MAX_VALUE, category)); 2138 children.add(new Property("priority", "code", 2139 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2140 0, 1, priority)); 2141 children.add(new Property("doNotPerform", "boolean", 2142 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2143 doNotPerform)); 2144 children.add(new Property("medium", "CodeableConcept", 2145 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 2146 children.add(new Property("subject", "Reference(Patient|Group)", 2147 "The patient or group that is the focus of this communication request.", 0, 1, subject)); 2148 children.add(new Property("about", "Reference(Any)", 2149 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2150 0, java.lang.Integer.MAX_VALUE, about)); 2151 children.add(new Property("encounter", "Reference(Encounter)", 2152 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2153 0, 1, encounter)); 2154 children.add(new Property("payload", "", "Text, attachment(s), or resource(s) to be communicated to the recipient.", 2155 0, java.lang.Integer.MAX_VALUE, payload)); 2156 children.add(new Property("occurrence[x]", "dateTime|Period", "The time when this communication is to occur.", 0, 1, 2157 occurrence)); 2158 children.add(new Property("authoredOn", "dateTime", 2159 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2160 0, 1, authoredOn)); 2161 children.add(new Property("requester", 2162 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2163 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2164 0, 1, requester)); 2165 children.add(new Property("recipient", 2166 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2167 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2168 0, java.lang.Integer.MAX_VALUE, recipient)); 2169 children.add(new Property("sender", 2170 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2171 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2172 0, 1, sender)); 2173 children.add(new Property("reasonCode", "CodeableConcept", 2174 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2175 reasonCode)); 2176 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2177 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2178 reasonReference)); 2179 children.add(new Property("note", "Annotation", 2180 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2181 java.lang.Integer.MAX_VALUE, note)); 2182 } 2183 2184 @Override 2185 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2186 switch (_hash) { 2187 case -1618432855: 2188 /* identifier */ return new Property("identifier", "Identifier", 2189 "Business identifiers assigned to this communication request by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2190 0, java.lang.Integer.MAX_VALUE, identifier); 2191 case -332612366: 2192 /* basedOn */ return new Property("basedOn", "Reference(Any)", 2193 "A plan or proposal that is fulfilled in whole or in part by this request.", 0, java.lang.Integer.MAX_VALUE, 2194 basedOn); 2195 case -430332865: 2196 /* replaces */ return new Property("replaces", "Reference(CommunicationRequest)", 2197 "Completed or terminated request(s) whose function is taken by this new request.", 0, 2198 java.lang.Integer.MAX_VALUE, replaces); 2199 case -445338488: 2200 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 2201 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition, prescription or similar form.", 2202 0, 1, groupIdentifier); 2203 case -892481550: 2204 /* status */ return new Property("status", "code", "The status of the proposal or order.", 0, 1, status); 2205 case 2051346646: 2206 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2207 "Captures the reason for the current state of the CommunicationRequest.", 0, 1, statusReason); 2208 case 50511102: 2209 /* category */ return new Property("category", "CodeableConcept", 2210 "The type of message to be sent such as alert, notification, reminder, instruction, etc.", 0, 2211 java.lang.Integer.MAX_VALUE, category); 2212 case -1165461084: 2213 /* priority */ return new Property("priority", "code", 2214 "Characterizes how quickly the proposed act must be initiated. Includes concepts such as stat, urgent, routine.", 2215 0, 1, priority); 2216 case -1788508167: 2217 /* doNotPerform */ return new Property("doNotPerform", "boolean", 2218 "If true indicates that the CommunicationRequest is asking for the specified action to *not* occur.", 0, 1, 2219 doNotPerform); 2220 case -1078030475: 2221 /* medium */ return new Property("medium", "CodeableConcept", 2222 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium); 2223 case -1867885268: 2224 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2225 "The patient or group that is the focus of this communication request.", 0, 1, subject); 2226 case 92611469: 2227 /* about */ return new Property("about", "Reference(Any)", 2228 "Other resources that pertain to this communication request and to which this communication request should be associated.", 2229 0, java.lang.Integer.MAX_VALUE, about); 2230 case 1524132147: 2231 /* encounter */ return new Property("encounter", "Reference(Encounter)", 2232 "The Encounter during which this CommunicationRequest was created or to which the creation of this record is tightly associated.", 2233 0, 1, encounter); 2234 case -786701938: 2235 /* payload */ return new Property("payload", "", 2236 "Text, attachment(s), or resource(s) to be communicated to the recipient.", 0, java.lang.Integer.MAX_VALUE, 2237 payload); 2238 case -2022646513: 2239 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period", 2240 "The time when this communication is to occur.", 0, 1, occurrence); 2241 case 1687874001: 2242 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period", 2243 "The time when this communication is to occur.", 0, 1, occurrence); 2244 case -298443636: 2245 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period", 2246 "The time when this communication is to occur.", 0, 1, occurrence); 2247 case 1397156594: 2248 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period", 2249 "The time when this communication is to occur.", 0, 1, occurrence); 2250 case -1500852503: 2251 /* authoredOn */ return new Property("authoredOn", "dateTime", 2252 "For draft requests, indicates the date of initial creation. For requests with other statuses, indicates the date of activation.", 2253 0, 1, authoredOn); 2254 case 693933948: 2255 /* requester */ return new Property("requester", 2256 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 2257 "The device, individual, or organization who initiated the request and has responsibility for its activation.", 2258 0, 1, requester); 2259 case 820081177: 2260 /* recipient */ return new Property("recipient", 2261 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Group|CareTeam|HealthcareService)", 2262 "The entity (e.g. person, organization, clinical information system, device, group, or care team) which is the intended target of the communication.", 2263 0, java.lang.Integer.MAX_VALUE, recipient); 2264 case -905962955: 2265 /* sender */ return new Property("sender", 2266 "Reference(Device|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|HealthcareService)", 2267 "The entity (e.g. person, organization, clinical information system, or device) which is to be the source of the communication.", 2268 0, 1, sender); 2269 case 722137681: 2270 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2271 "Describes why the request is being made in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2272 reasonCode); 2273 case -1146218137: 2274 /* reasonReference */ return new Property("reasonReference", 2275 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 2276 "Indicates another resource whose existence justifies this request.", 0, java.lang.Integer.MAX_VALUE, 2277 reasonReference); 2278 case 3387378: 2279 /* note */ return new Property("note", "Annotation", 2280 "Comments made about the request by the requester, sender, recipient, subject or other participants.", 0, 2281 java.lang.Integer.MAX_VALUE, note); 2282 default: 2283 return super.getNamedProperty(_hash, _name, _checkValid); 2284 } 2285 2286 } 2287 2288 @Override 2289 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2290 switch (hash) { 2291 case -1618432855: 2292 /* identifier */ return this.identifier == null ? new Base[0] 2293 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2294 case -332612366: 2295 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2296 case -430332865: 2297 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2298 case -445338488: 2299 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 2300 case -892481550: 2301 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CommunicationRequestStatus> 2302 case 2051346646: 2303 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 2304 case 50511102: 2305 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2306 case -1165461084: 2307 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<CommunicationPriority> 2308 case -1788508167: 2309 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 2310 case -1078030475: 2311 /* medium */ return this.medium == null ? new Base[0] : this.medium.toArray(new Base[this.medium.size()]); // CodeableConcept 2312 case -1867885268: 2313 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2314 case 92611469: 2315 /* about */ return this.about == null ? new Base[0] : this.about.toArray(new Base[this.about.size()]); // Reference 2316 case 1524132147: 2317 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2318 case -786701938: 2319 /* payload */ return this.payload == null ? new Base[0] : this.payload.toArray(new Base[this.payload.size()]); // CommunicationRequestPayloadComponent 2320 case 1687874001: 2321 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 2322 case -1500852503: 2323 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 2324 case 693933948: 2325 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 2326 case 820081177: 2327 /* recipient */ return this.recipient == null ? new Base[0] 2328 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 2329 case -905962955: 2330 /* sender */ return this.sender == null ? new Base[0] : new Base[] { this.sender }; // Reference 2331 case 722137681: 2332 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2333 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2334 case -1146218137: 2335 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2336 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2337 case 3387378: 2338 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2339 default: 2340 return super.getProperty(hash, name, checkValid); 2341 } 2342 2343 } 2344 2345 @Override 2346 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2347 switch (hash) { 2348 case -1618432855: // identifier 2349 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2350 return value; 2351 case -332612366: // basedOn 2352 this.getBasedOn().add(castToReference(value)); // Reference 2353 return value; 2354 case -430332865: // replaces 2355 this.getReplaces().add(castToReference(value)); // Reference 2356 return value; 2357 case -445338488: // groupIdentifier 2358 this.groupIdentifier = castToIdentifier(value); // Identifier 2359 return value; 2360 case -892481550: // status 2361 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2362 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2363 return value; 2364 case 2051346646: // statusReason 2365 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2366 return value; 2367 case 50511102: // category 2368 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2369 return value; 2370 case -1165461084: // priority 2371 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2372 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2373 return value; 2374 case -1788508167: // doNotPerform 2375 this.doNotPerform = castToBoolean(value); // BooleanType 2376 return value; 2377 case -1078030475: // medium 2378 this.getMedium().add(castToCodeableConcept(value)); // CodeableConcept 2379 return value; 2380 case -1867885268: // subject 2381 this.subject = castToReference(value); // Reference 2382 return value; 2383 case 92611469: // about 2384 this.getAbout().add(castToReference(value)); // Reference 2385 return value; 2386 case 1524132147: // encounter 2387 this.encounter = castToReference(value); // Reference 2388 return value; 2389 case -786701938: // payload 2390 this.getPayload().add((CommunicationRequestPayloadComponent) value); // CommunicationRequestPayloadComponent 2391 return value; 2392 case 1687874001: // occurrence 2393 this.occurrence = castToType(value); // Type 2394 return value; 2395 case -1500852503: // authoredOn 2396 this.authoredOn = castToDateTime(value); // DateTimeType 2397 return value; 2398 case 693933948: // requester 2399 this.requester = castToReference(value); // Reference 2400 return value; 2401 case 820081177: // recipient 2402 this.getRecipient().add(castToReference(value)); // Reference 2403 return value; 2404 case -905962955: // sender 2405 this.sender = castToReference(value); // Reference 2406 return value; 2407 case 722137681: // reasonCode 2408 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2409 return value; 2410 case -1146218137: // reasonReference 2411 this.getReasonReference().add(castToReference(value)); // Reference 2412 return value; 2413 case 3387378: // note 2414 this.getNote().add(castToAnnotation(value)); // Annotation 2415 return value; 2416 default: 2417 return super.setProperty(hash, name, value); 2418 } 2419 2420 } 2421 2422 @Override 2423 public Base setProperty(String name, Base value) throws FHIRException { 2424 if (name.equals("identifier")) { 2425 this.getIdentifier().add(castToIdentifier(value)); 2426 } else if (name.equals("basedOn")) { 2427 this.getBasedOn().add(castToReference(value)); 2428 } else if (name.equals("replaces")) { 2429 this.getReplaces().add(castToReference(value)); 2430 } else if (name.equals("groupIdentifier")) { 2431 this.groupIdentifier = castToIdentifier(value); // Identifier 2432 } else if (name.equals("status")) { 2433 value = new CommunicationRequestStatusEnumFactory().fromType(castToCode(value)); 2434 this.status = (Enumeration) value; // Enumeration<CommunicationRequestStatus> 2435 } else if (name.equals("statusReason")) { 2436 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2437 } else if (name.equals("category")) { 2438 this.getCategory().add(castToCodeableConcept(value)); 2439 } else if (name.equals("priority")) { 2440 value = new CommunicationPriorityEnumFactory().fromType(castToCode(value)); 2441 this.priority = (Enumeration) value; // Enumeration<CommunicationPriority> 2442 } else if (name.equals("doNotPerform")) { 2443 this.doNotPerform = castToBoolean(value); // BooleanType 2444 } else if (name.equals("medium")) { 2445 this.getMedium().add(castToCodeableConcept(value)); 2446 } else if (name.equals("subject")) { 2447 this.subject = castToReference(value); // Reference 2448 } else if (name.equals("about")) { 2449 this.getAbout().add(castToReference(value)); 2450 } else if (name.equals("encounter")) { 2451 this.encounter = castToReference(value); // Reference 2452 } else if (name.equals("payload")) { 2453 this.getPayload().add((CommunicationRequestPayloadComponent) value); 2454 } else if (name.equals("occurrence[x]")) { 2455 this.occurrence = castToType(value); // Type 2456 } else if (name.equals("authoredOn")) { 2457 this.authoredOn = castToDateTime(value); // DateTimeType 2458 } else if (name.equals("requester")) { 2459 this.requester = castToReference(value); // Reference 2460 } else if (name.equals("recipient")) { 2461 this.getRecipient().add(castToReference(value)); 2462 } else if (name.equals("sender")) { 2463 this.sender = castToReference(value); // Reference 2464 } else if (name.equals("reasonCode")) { 2465 this.getReasonCode().add(castToCodeableConcept(value)); 2466 } else if (name.equals("reasonReference")) { 2467 this.getReasonReference().add(castToReference(value)); 2468 } else if (name.equals("note")) { 2469 this.getNote().add(castToAnnotation(value)); 2470 } else 2471 return super.setProperty(name, value); 2472 return value; 2473 } 2474 2475 @Override 2476 public void removeChild(String name, Base value) throws FHIRException { 2477 if (name.equals("identifier")) { 2478 this.getIdentifier().remove(castToIdentifier(value)); 2479 } else if (name.equals("basedOn")) { 2480 this.getBasedOn().remove(castToReference(value)); 2481 } else if (name.equals("replaces")) { 2482 this.getReplaces().remove(castToReference(value)); 2483 } else if (name.equals("groupIdentifier")) { 2484 this.groupIdentifier = null; 2485 } else if (name.equals("status")) { 2486 this.status = null; 2487 } else if (name.equals("statusReason")) { 2488 this.statusReason = null; 2489 } else if (name.equals("category")) { 2490 this.getCategory().remove(castToCodeableConcept(value)); 2491 } else if (name.equals("priority")) { 2492 this.priority = null; 2493 } else if (name.equals("doNotPerform")) { 2494 this.doNotPerform = null; 2495 } else if (name.equals("medium")) { 2496 this.getMedium().remove(castToCodeableConcept(value)); 2497 } else if (name.equals("subject")) { 2498 this.subject = null; 2499 } else if (name.equals("about")) { 2500 this.getAbout().remove(castToReference(value)); 2501 } else if (name.equals("encounter")) { 2502 this.encounter = null; 2503 } else if (name.equals("payload")) { 2504 this.getPayload().remove((CommunicationRequestPayloadComponent) value); 2505 } else if (name.equals("occurrence[x]")) { 2506 this.occurrence = null; 2507 } else if (name.equals("authoredOn")) { 2508 this.authoredOn = null; 2509 } else if (name.equals("requester")) { 2510 this.requester = null; 2511 } else if (name.equals("recipient")) { 2512 this.getRecipient().remove(castToReference(value)); 2513 } else if (name.equals("sender")) { 2514 this.sender = null; 2515 } else if (name.equals("reasonCode")) { 2516 this.getReasonCode().remove(castToCodeableConcept(value)); 2517 } else if (name.equals("reasonReference")) { 2518 this.getReasonReference().remove(castToReference(value)); 2519 } else if (name.equals("note")) { 2520 this.getNote().remove(castToAnnotation(value)); 2521 } else 2522 super.removeChild(name, value); 2523 2524 } 2525 2526 @Override 2527 public Base makeProperty(int hash, String name) throws FHIRException { 2528 switch (hash) { 2529 case -1618432855: 2530 return addIdentifier(); 2531 case -332612366: 2532 return addBasedOn(); 2533 case -430332865: 2534 return addReplaces(); 2535 case -445338488: 2536 return getGroupIdentifier(); 2537 case -892481550: 2538 return getStatusElement(); 2539 case 2051346646: 2540 return getStatusReason(); 2541 case 50511102: 2542 return addCategory(); 2543 case -1165461084: 2544 return getPriorityElement(); 2545 case -1788508167: 2546 return getDoNotPerformElement(); 2547 case -1078030475: 2548 return addMedium(); 2549 case -1867885268: 2550 return getSubject(); 2551 case 92611469: 2552 return addAbout(); 2553 case 1524132147: 2554 return getEncounter(); 2555 case -786701938: 2556 return addPayload(); 2557 case -2022646513: 2558 return getOccurrence(); 2559 case 1687874001: 2560 return getOccurrence(); 2561 case -1500852503: 2562 return getAuthoredOnElement(); 2563 case 693933948: 2564 return getRequester(); 2565 case 820081177: 2566 return addRecipient(); 2567 case -905962955: 2568 return getSender(); 2569 case 722137681: 2570 return addReasonCode(); 2571 case -1146218137: 2572 return addReasonReference(); 2573 case 3387378: 2574 return addNote(); 2575 default: 2576 return super.makeProperty(hash, name); 2577 } 2578 2579 } 2580 2581 @Override 2582 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2583 switch (hash) { 2584 case -1618432855: 2585 /* identifier */ return new String[] { "Identifier" }; 2586 case -332612366: 2587 /* basedOn */ return new String[] { "Reference" }; 2588 case -430332865: 2589 /* replaces */ return new String[] { "Reference" }; 2590 case -445338488: 2591 /* groupIdentifier */ return new String[] { "Identifier" }; 2592 case -892481550: 2593 /* status */ return new String[] { "code" }; 2594 case 2051346646: 2595 /* statusReason */ return new String[] { "CodeableConcept" }; 2596 case 50511102: 2597 /* category */ return new String[] { "CodeableConcept" }; 2598 case -1165461084: 2599 /* priority */ return new String[] { "code" }; 2600 case -1788508167: 2601 /* doNotPerform */ return new String[] { "boolean" }; 2602 case -1078030475: 2603 /* medium */ return new String[] { "CodeableConcept" }; 2604 case -1867885268: 2605 /* subject */ return new String[] { "Reference" }; 2606 case 92611469: 2607 /* about */ return new String[] { "Reference" }; 2608 case 1524132147: 2609 /* encounter */ return new String[] { "Reference" }; 2610 case -786701938: 2611 /* payload */ return new String[] {}; 2612 case 1687874001: 2613 /* occurrence */ return new String[] { "dateTime", "Period" }; 2614 case -1500852503: 2615 /* authoredOn */ return new String[] { "dateTime" }; 2616 case 693933948: 2617 /* requester */ return new String[] { "Reference" }; 2618 case 820081177: 2619 /* recipient */ return new String[] { "Reference" }; 2620 case -905962955: 2621 /* sender */ return new String[] { "Reference" }; 2622 case 722137681: 2623 /* reasonCode */ return new String[] { "CodeableConcept" }; 2624 case -1146218137: 2625 /* reasonReference */ return new String[] { "Reference" }; 2626 case 3387378: 2627 /* note */ return new String[] { "Annotation" }; 2628 default: 2629 return super.getTypesForProperty(hash, name); 2630 } 2631 2632 } 2633 2634 @Override 2635 public Base addChild(String name) throws FHIRException { 2636 if (name.equals("identifier")) { 2637 return addIdentifier(); 2638 } else if (name.equals("basedOn")) { 2639 return addBasedOn(); 2640 } else if (name.equals("replaces")) { 2641 return addReplaces(); 2642 } else if (name.equals("groupIdentifier")) { 2643 this.groupIdentifier = new Identifier(); 2644 return this.groupIdentifier; 2645 } else if (name.equals("status")) { 2646 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.status"); 2647 } else if (name.equals("statusReason")) { 2648 this.statusReason = new CodeableConcept(); 2649 return this.statusReason; 2650 } else if (name.equals("category")) { 2651 return addCategory(); 2652 } else if (name.equals("priority")) { 2653 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.priority"); 2654 } else if (name.equals("doNotPerform")) { 2655 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.doNotPerform"); 2656 } else if (name.equals("medium")) { 2657 return addMedium(); 2658 } else if (name.equals("subject")) { 2659 this.subject = new Reference(); 2660 return this.subject; 2661 } else if (name.equals("about")) { 2662 return addAbout(); 2663 } else if (name.equals("encounter")) { 2664 this.encounter = new Reference(); 2665 return this.encounter; 2666 } else if (name.equals("payload")) { 2667 return addPayload(); 2668 } else if (name.equals("occurrenceDateTime")) { 2669 this.occurrence = new DateTimeType(); 2670 return this.occurrence; 2671 } else if (name.equals("occurrencePeriod")) { 2672 this.occurrence = new Period(); 2673 return this.occurrence; 2674 } else if (name.equals("authoredOn")) { 2675 throw new FHIRException("Cannot call addChild on a singleton property CommunicationRequest.authoredOn"); 2676 } else if (name.equals("requester")) { 2677 this.requester = new Reference(); 2678 return this.requester; 2679 } else if (name.equals("recipient")) { 2680 return addRecipient(); 2681 } else if (name.equals("sender")) { 2682 this.sender = new Reference(); 2683 return this.sender; 2684 } else if (name.equals("reasonCode")) { 2685 return addReasonCode(); 2686 } else if (name.equals("reasonReference")) { 2687 return addReasonReference(); 2688 } else if (name.equals("note")) { 2689 return addNote(); 2690 } else 2691 return super.addChild(name); 2692 } 2693 2694 public String fhirType() { 2695 return "CommunicationRequest"; 2696 2697 } 2698 2699 public CommunicationRequest copy() { 2700 CommunicationRequest dst = new CommunicationRequest(); 2701 copyValues(dst); 2702 return dst; 2703 } 2704 2705 public void copyValues(CommunicationRequest dst) { 2706 super.copyValues(dst); 2707 if (identifier != null) { 2708 dst.identifier = new ArrayList<Identifier>(); 2709 for (Identifier i : identifier) 2710 dst.identifier.add(i.copy()); 2711 } 2712 ; 2713 if (basedOn != null) { 2714 dst.basedOn = new ArrayList<Reference>(); 2715 for (Reference i : basedOn) 2716 dst.basedOn.add(i.copy()); 2717 } 2718 ; 2719 if (replaces != null) { 2720 dst.replaces = new ArrayList<Reference>(); 2721 for (Reference i : replaces) 2722 dst.replaces.add(i.copy()); 2723 } 2724 ; 2725 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 2726 dst.status = status == null ? null : status.copy(); 2727 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2728 if (category != null) { 2729 dst.category = new ArrayList<CodeableConcept>(); 2730 for (CodeableConcept i : category) 2731 dst.category.add(i.copy()); 2732 } 2733 ; 2734 dst.priority = priority == null ? null : priority.copy(); 2735 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 2736 if (medium != null) { 2737 dst.medium = new ArrayList<CodeableConcept>(); 2738 for (CodeableConcept i : medium) 2739 dst.medium.add(i.copy()); 2740 } 2741 ; 2742 dst.subject = subject == null ? null : subject.copy(); 2743 if (about != null) { 2744 dst.about = new ArrayList<Reference>(); 2745 for (Reference i : about) 2746 dst.about.add(i.copy()); 2747 } 2748 ; 2749 dst.encounter = encounter == null ? null : encounter.copy(); 2750 if (payload != null) { 2751 dst.payload = new ArrayList<CommunicationRequestPayloadComponent>(); 2752 for (CommunicationRequestPayloadComponent i : payload) 2753 dst.payload.add(i.copy()); 2754 } 2755 ; 2756 dst.occurrence = occurrence == null ? null : occurrence.copy(); 2757 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 2758 dst.requester = requester == null ? null : requester.copy(); 2759 if (recipient != null) { 2760 dst.recipient = new ArrayList<Reference>(); 2761 for (Reference i : recipient) 2762 dst.recipient.add(i.copy()); 2763 } 2764 ; 2765 dst.sender = sender == null ? null : sender.copy(); 2766 if (reasonCode != null) { 2767 dst.reasonCode = new ArrayList<CodeableConcept>(); 2768 for (CodeableConcept i : reasonCode) 2769 dst.reasonCode.add(i.copy()); 2770 } 2771 ; 2772 if (reasonReference != null) { 2773 dst.reasonReference = new ArrayList<Reference>(); 2774 for (Reference i : reasonReference) 2775 dst.reasonReference.add(i.copy()); 2776 } 2777 ; 2778 if (note != null) { 2779 dst.note = new ArrayList<Annotation>(); 2780 for (Annotation i : note) 2781 dst.note.add(i.copy()); 2782 } 2783 ; 2784 } 2785 2786 protected CommunicationRequest typedCopy() { 2787 return copy(); 2788 } 2789 2790 @Override 2791 public boolean equalsDeep(Base other_) { 2792 if (!super.equalsDeep(other_)) 2793 return false; 2794 if (!(other_ instanceof CommunicationRequest)) 2795 return false; 2796 CommunicationRequest o = (CommunicationRequest) other_; 2797 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2798 && compareDeep(replaces, o.replaces, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 2799 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) 2800 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 2801 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(medium, o.medium, true) 2802 && compareDeep(subject, o.subject, true) && compareDeep(about, o.about, true) 2803 && compareDeep(encounter, o.encounter, true) && compareDeep(payload, o.payload, true) 2804 && compareDeep(occurrence, o.occurrence, true) && compareDeep(authoredOn, o.authoredOn, true) 2805 && compareDeep(requester, o.requester, true) && compareDeep(recipient, o.recipient, true) 2806 && compareDeep(sender, o.sender, true) && compareDeep(reasonCode, o.reasonCode, true) 2807 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(note, o.note, true); 2808 } 2809 2810 @Override 2811 public boolean equalsShallow(Base other_) { 2812 if (!super.equalsShallow(other_)) 2813 return false; 2814 if (!(other_ instanceof CommunicationRequest)) 2815 return false; 2816 CommunicationRequest o = (CommunicationRequest) other_; 2817 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 2818 && compareValues(doNotPerform, o.doNotPerform, true) && compareValues(authoredOn, o.authoredOn, true); 2819 } 2820 2821 public boolean isEmpty() { 2822 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, replaces, groupIdentifier, 2823 status, statusReason, category, priority, doNotPerform, medium, subject, about, encounter, payload, occurrence, 2824 authoredOn, requester, recipient, sender, reasonCode, reasonReference, note); 2825 } 2826 2827 @Override 2828 public ResourceType getResourceType() { 2829 return ResourceType.CommunicationRequest; 2830 } 2831 2832 /** 2833 * Search parameter: <b>requester</b> 2834 * <p> 2835 * Description: <b>Who/what is requesting service</b><br> 2836 * Type: <b>reference</b><br> 2837 * Path: <b>CommunicationRequest.requester</b><br> 2838 * </p> 2839 */ 2840 @SearchParamDefinition(name = "requester", path = "CommunicationRequest.requester", description = "Who/what is requesting service", type = "reference", providesMembershipIn = { 2841 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2842 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2843 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 2844 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2845 public static final String SP_REQUESTER = "requester"; 2846 /** 2847 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 2848 * <p> 2849 * Description: <b>Who/what is requesting service</b><br> 2850 * Type: <b>reference</b><br> 2851 * Path: <b>CommunicationRequest.requester</b><br> 2852 * </p> 2853 */ 2854 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2855 SP_REQUESTER); 2856 2857 /** 2858 * Constant for fluent queries to be used to add include statements. Specifies 2859 * the path value of "<b>CommunicationRequest:requester</b>". 2860 */ 2861 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 2862 "CommunicationRequest:requester").toLocked(); 2863 2864 /** 2865 * Search parameter: <b>authored</b> 2866 * <p> 2867 * Description: <b>When request transitioned to being actionable</b><br> 2868 * Type: <b>date</b><br> 2869 * Path: <b>CommunicationRequest.authoredOn</b><br> 2870 * </p> 2871 */ 2872 @SearchParamDefinition(name = "authored", path = "CommunicationRequest.authoredOn", description = "When request transitioned to being actionable", type = "date") 2873 public static final String SP_AUTHORED = "authored"; 2874 /** 2875 * <b>Fluent Client</b> search parameter constant for <b>authored</b> 2876 * <p> 2877 * Description: <b>When request transitioned to being actionable</b><br> 2878 * Type: <b>date</b><br> 2879 * Path: <b>CommunicationRequest.authoredOn</b><br> 2880 * </p> 2881 */ 2882 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2883 SP_AUTHORED); 2884 2885 /** 2886 * Search parameter: <b>identifier</b> 2887 * <p> 2888 * Description: <b>Unique identifier</b><br> 2889 * Type: <b>token</b><br> 2890 * Path: <b>CommunicationRequest.identifier</b><br> 2891 * </p> 2892 */ 2893 @SearchParamDefinition(name = "identifier", path = "CommunicationRequest.identifier", description = "Unique identifier", type = "token") 2894 public static final String SP_IDENTIFIER = "identifier"; 2895 /** 2896 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2897 * <p> 2898 * Description: <b>Unique identifier</b><br> 2899 * Type: <b>token</b><br> 2900 * Path: <b>CommunicationRequest.identifier</b><br> 2901 * </p> 2902 */ 2903 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2904 SP_IDENTIFIER); 2905 2906 /** 2907 * Search parameter: <b>subject</b> 2908 * <p> 2909 * Description: <b>Focus of message</b><br> 2910 * Type: <b>reference</b><br> 2911 * Path: <b>CommunicationRequest.subject</b><br> 2912 * </p> 2913 */ 2914 @SearchParamDefinition(name = "subject", path = "CommunicationRequest.subject", description = "Focus of message", type = "reference", providesMembershipIn = { 2915 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 2916 public static final String SP_SUBJECT = "subject"; 2917 /** 2918 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2919 * <p> 2920 * Description: <b>Focus of message</b><br> 2921 * Type: <b>reference</b><br> 2922 * Path: <b>CommunicationRequest.subject</b><br> 2923 * </p> 2924 */ 2925 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2926 SP_SUBJECT); 2927 2928 /** 2929 * Constant for fluent queries to be used to add include statements. Specifies 2930 * the path value of "<b>CommunicationRequest:subject</b>". 2931 */ 2932 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2933 "CommunicationRequest:subject").toLocked(); 2934 2935 /** 2936 * Search parameter: <b>replaces</b> 2937 * <p> 2938 * Description: <b>Request(s) replaced by this request</b><br> 2939 * Type: <b>reference</b><br> 2940 * Path: <b>CommunicationRequest.replaces</b><br> 2941 * </p> 2942 */ 2943 @SearchParamDefinition(name = "replaces", path = "CommunicationRequest.replaces", description = "Request(s) replaced by this request", type = "reference", target = { 2944 CommunicationRequest.class }) 2945 public static final String SP_REPLACES = "replaces"; 2946 /** 2947 * <b>Fluent Client</b> search parameter constant for <b>replaces</b> 2948 * <p> 2949 * Description: <b>Request(s) replaced by this request</b><br> 2950 * Type: <b>reference</b><br> 2951 * Path: <b>CommunicationRequest.replaces</b><br> 2952 * </p> 2953 */ 2954 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPLACES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2955 SP_REPLACES); 2956 2957 /** 2958 * Constant for fluent queries to be used to add include statements. Specifies 2959 * the path value of "<b>CommunicationRequest:replaces</b>". 2960 */ 2961 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPLACES = new ca.uhn.fhir.model.api.Include( 2962 "CommunicationRequest:replaces").toLocked(); 2963 2964 /** 2965 * Search parameter: <b>medium</b> 2966 * <p> 2967 * Description: <b>A channel of communication</b><br> 2968 * Type: <b>token</b><br> 2969 * Path: <b>CommunicationRequest.medium</b><br> 2970 * </p> 2971 */ 2972 @SearchParamDefinition(name = "medium", path = "CommunicationRequest.medium", description = "A channel of communication", type = "token") 2973 public static final String SP_MEDIUM = "medium"; 2974 /** 2975 * <b>Fluent Client</b> search parameter constant for <b>medium</b> 2976 * <p> 2977 * Description: <b>A channel of communication</b><br> 2978 * Type: <b>token</b><br> 2979 * Path: <b>CommunicationRequest.medium</b><br> 2980 * </p> 2981 */ 2982 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEDIUM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2983 SP_MEDIUM); 2984 2985 /** 2986 * Search parameter: <b>encounter</b> 2987 * <p> 2988 * Description: <b>Encounter created as part of</b><br> 2989 * Type: <b>reference</b><br> 2990 * Path: <b>CommunicationRequest.encounter</b><br> 2991 * </p> 2992 */ 2993 @SearchParamDefinition(name = "encounter", path = "CommunicationRequest.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2994 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2995 public static final String SP_ENCOUNTER = "encounter"; 2996 /** 2997 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2998 * <p> 2999 * Description: <b>Encounter created as part of</b><br> 3000 * Type: <b>reference</b><br> 3001 * Path: <b>CommunicationRequest.encounter</b><br> 3002 * </p> 3003 */ 3004 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3005 SP_ENCOUNTER); 3006 3007 /** 3008 * Constant for fluent queries to be used to add include statements. Specifies 3009 * the path value of "<b>CommunicationRequest:encounter</b>". 3010 */ 3011 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 3012 "CommunicationRequest:encounter").toLocked(); 3013 3014 /** 3015 * Search parameter: <b>occurrence</b> 3016 * <p> 3017 * Description: <b>When scheduled</b><br> 3018 * Type: <b>date</b><br> 3019 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3020 * </p> 3021 */ 3022 @SearchParamDefinition(name = "occurrence", path = "(CommunicationRequest.occurrence as dateTime)", description = "When scheduled", type = "date") 3023 public static final String SP_OCCURRENCE = "occurrence"; 3024 /** 3025 * <b>Fluent Client</b> search parameter constant for <b>occurrence</b> 3026 * <p> 3027 * Description: <b>When scheduled</b><br> 3028 * Type: <b>date</b><br> 3029 * Path: <b>CommunicationRequest.occurrenceDateTime</b><br> 3030 * </p> 3031 */ 3032 public static final ca.uhn.fhir.rest.gclient.DateClientParam OCCURRENCE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3033 SP_OCCURRENCE); 3034 3035 /** 3036 * Search parameter: <b>priority</b> 3037 * <p> 3038 * Description: <b>routine | urgent | asap | stat</b><br> 3039 * Type: <b>token</b><br> 3040 * Path: <b>CommunicationRequest.priority</b><br> 3041 * </p> 3042 */ 3043 @SearchParamDefinition(name = "priority", path = "CommunicationRequest.priority", description = "routine | urgent | asap | stat", type = "token") 3044 public static final String SP_PRIORITY = "priority"; 3045 /** 3046 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3047 * <p> 3048 * Description: <b>routine | urgent | asap | stat</b><br> 3049 * Type: <b>token</b><br> 3050 * Path: <b>CommunicationRequest.priority</b><br> 3051 * </p> 3052 */ 3053 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3054 SP_PRIORITY); 3055 3056 /** 3057 * Search parameter: <b>group-identifier</b> 3058 * <p> 3059 * Description: <b>Composite request this is part of</b><br> 3060 * Type: <b>token</b><br> 3061 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3062 * </p> 3063 */ 3064 @SearchParamDefinition(name = "group-identifier", path = "CommunicationRequest.groupIdentifier", description = "Composite request this is part of", type = "token") 3065 public static final String SP_GROUP_IDENTIFIER = "group-identifier"; 3066 /** 3067 * <b>Fluent Client</b> search parameter constant for <b>group-identifier</b> 3068 * <p> 3069 * Description: <b>Composite request this is part of</b><br> 3070 * Type: <b>token</b><br> 3071 * Path: <b>CommunicationRequest.groupIdentifier</b><br> 3072 * </p> 3073 */ 3074 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GROUP_IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3075 SP_GROUP_IDENTIFIER); 3076 3077 /** 3078 * Search parameter: <b>based-on</b> 3079 * <p> 3080 * Description: <b>Fulfills plan or proposal</b><br> 3081 * Type: <b>reference</b><br> 3082 * Path: <b>CommunicationRequest.basedOn</b><br> 3083 * </p> 3084 */ 3085 @SearchParamDefinition(name = "based-on", path = "CommunicationRequest.basedOn", description = "Fulfills plan or proposal", type = "reference") 3086 public static final String SP_BASED_ON = "based-on"; 3087 /** 3088 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3089 * <p> 3090 * Description: <b>Fulfills plan or proposal</b><br> 3091 * Type: <b>reference</b><br> 3092 * Path: <b>CommunicationRequest.basedOn</b><br> 3093 * </p> 3094 */ 3095 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3096 SP_BASED_ON); 3097 3098 /** 3099 * Constant for fluent queries to be used to add include statements. Specifies 3100 * the path value of "<b>CommunicationRequest:based-on</b>". 3101 */ 3102 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 3103 "CommunicationRequest:based-on").toLocked(); 3104 3105 /** 3106 * Search parameter: <b>sender</b> 3107 * <p> 3108 * Description: <b>Message sender</b><br> 3109 * Type: <b>reference</b><br> 3110 * Path: <b>CommunicationRequest.sender</b><br> 3111 * </p> 3112 */ 3113 @SearchParamDefinition(name = "sender", path = "CommunicationRequest.sender", description = "Message sender", type = "reference", providesMembershipIn = { 3114 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3115 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3116 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3117 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 3118 HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 3119 RelatedPerson.class }) 3120 public static final String SP_SENDER = "sender"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>sender</b> 3123 * <p> 3124 * Description: <b>Message sender</b><br> 3125 * Type: <b>reference</b><br> 3126 * Path: <b>CommunicationRequest.sender</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SENDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3130 SP_SENDER); 3131 3132 /** 3133 * Constant for fluent queries to be used to add include statements. Specifies 3134 * the path value of "<b>CommunicationRequest:sender</b>". 3135 */ 3136 public static final ca.uhn.fhir.model.api.Include INCLUDE_SENDER = new ca.uhn.fhir.model.api.Include( 3137 "CommunicationRequest:sender").toLocked(); 3138 3139 /** 3140 * Search parameter: <b>patient</b> 3141 * <p> 3142 * Description: <b>Focus of message</b><br> 3143 * Type: <b>reference</b><br> 3144 * Path: <b>CommunicationRequest.subject</b><br> 3145 * </p> 3146 */ 3147 @SearchParamDefinition(name = "patient", path = "CommunicationRequest.subject.where(resolve() is Patient)", description = "Focus of message", type = "reference", target = { 3148 Patient.class }) 3149 public static final String SP_PATIENT = "patient"; 3150 /** 3151 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3152 * <p> 3153 * Description: <b>Focus of message</b><br> 3154 * Type: <b>reference</b><br> 3155 * Path: <b>CommunicationRequest.subject</b><br> 3156 * </p> 3157 */ 3158 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3159 SP_PATIENT); 3160 3161 /** 3162 * Constant for fluent queries to be used to add include statements. Specifies 3163 * the path value of "<b>CommunicationRequest:patient</b>". 3164 */ 3165 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3166 "CommunicationRequest:patient").toLocked(); 3167 3168 /** 3169 * Search parameter: <b>recipient</b> 3170 * <p> 3171 * Description: <b>Message recipient</b><br> 3172 * Type: <b>reference</b><br> 3173 * Path: <b>CommunicationRequest.recipient</b><br> 3174 * </p> 3175 */ 3176 @SearchParamDefinition(name = "recipient", path = "CommunicationRequest.recipient", description = "Message recipient", type = "reference", providesMembershipIn = { 3177 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3178 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3179 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3180 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, Device.class, 3181 Group.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, 3182 PractitionerRole.class, RelatedPerson.class }) 3183 public static final String SP_RECIPIENT = "recipient"; 3184 /** 3185 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3186 * <p> 3187 * Description: <b>Message recipient</b><br> 3188 * Type: <b>reference</b><br> 3189 * Path: <b>CommunicationRequest.recipient</b><br> 3190 * </p> 3191 */ 3192 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3193 SP_RECIPIENT); 3194 3195 /** 3196 * Constant for fluent queries to be used to add include statements. Specifies 3197 * the path value of "<b>CommunicationRequest:recipient</b>". 3198 */ 3199 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 3200 "CommunicationRequest:recipient").toLocked(); 3201 3202 /** 3203 * Search parameter: <b>category</b> 3204 * <p> 3205 * Description: <b>Message category</b><br> 3206 * Type: <b>token</b><br> 3207 * Path: <b>CommunicationRequest.category</b><br> 3208 * </p> 3209 */ 3210 @SearchParamDefinition(name = "category", path = "CommunicationRequest.category", description = "Message category", type = "token") 3211 public static final String SP_CATEGORY = "category"; 3212 /** 3213 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3214 * <p> 3215 * Description: <b>Message category</b><br> 3216 * Type: <b>token</b><br> 3217 * Path: <b>CommunicationRequest.category</b><br> 3218 * </p> 3219 */ 3220 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3221 SP_CATEGORY); 3222 3223 /** 3224 * Search parameter: <b>status</b> 3225 * <p> 3226 * Description: <b>draft | active | on-hold | revoked | completed | 3227 * entered-in-error | unknown</b><br> 3228 * Type: <b>token</b><br> 3229 * Path: <b>CommunicationRequest.status</b><br> 3230 * </p> 3231 */ 3232 @SearchParamDefinition(name = "status", path = "CommunicationRequest.status", description = "draft | active | on-hold | revoked | completed | entered-in-error | unknown", type = "token") 3233 public static final String SP_STATUS = "status"; 3234 /** 3235 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3236 * <p> 3237 * Description: <b>draft | active | on-hold | revoked | completed | 3238 * entered-in-error | unknown</b><br> 3239 * Type: <b>token</b><br> 3240 * Path: <b>CommunicationRequest.status</b><br> 3241 * </p> 3242 */ 3243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3244 SP_STATUS); 3245 3246}