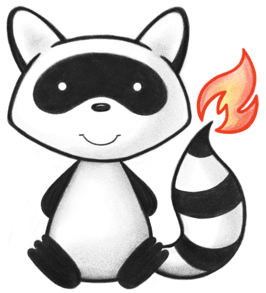
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A compartment definition that defines how resources are accessed on a server. 052 */ 053@ResourceDef(name = "CompartmentDefinition", profile = "http://hl7.org/fhir/StructureDefinition/CompartmentDefinition") 054@ChildOrder(names = { "url", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", 055 "useContext", "purpose", "code", "search", "resource" }) 056public class CompartmentDefinition extends MetadataResource { 057 058 public enum CompartmentType { 059 /** 060 * The compartment definition is for the patient compartment. 061 */ 062 PATIENT, 063 /** 064 * The compartment definition is for the encounter compartment. 065 */ 066 ENCOUNTER, 067 /** 068 * The compartment definition is for the related-person compartment. 069 */ 070 RELATEDPERSON, 071 /** 072 * The compartment definition is for the practitioner compartment. 073 */ 074 PRACTITIONER, 075 /** 076 * The compartment definition is for the device compartment. 077 */ 078 DEVICE, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static CompartmentType fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("Patient".equals(codeString)) 088 return PATIENT; 089 if ("Encounter".equals(codeString)) 090 return ENCOUNTER; 091 if ("RelatedPerson".equals(codeString)) 092 return RELATEDPERSON; 093 if ("Practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("Device".equals(codeString)) 096 return DEVICE; 097 if (Configuration.isAcceptInvalidEnums()) 098 return null; 099 else 100 throw new FHIRException("Unknown CompartmentType code '" + codeString + "'"); 101 } 102 103 public String toCode() { 104 switch (this) { 105 case PATIENT: 106 return "Patient"; 107 case ENCOUNTER: 108 return "Encounter"; 109 case RELATEDPERSON: 110 return "RelatedPerson"; 111 case PRACTITIONER: 112 return "Practitioner"; 113 case DEVICE: 114 return "Device"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case PATIENT: 125 return "http://hl7.org/fhir/compartment-type"; 126 case ENCOUNTER: 127 return "http://hl7.org/fhir/compartment-type"; 128 case RELATEDPERSON: 129 return "http://hl7.org/fhir/compartment-type"; 130 case PRACTITIONER: 131 return "http://hl7.org/fhir/compartment-type"; 132 case DEVICE: 133 return "http://hl7.org/fhir/compartment-type"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case PATIENT: 144 return "The compartment definition is for the patient compartment."; 145 case ENCOUNTER: 146 return "The compartment definition is for the encounter compartment."; 147 case RELATEDPERSON: 148 return "The compartment definition is for the related-person compartment."; 149 case PRACTITIONER: 150 return "The compartment definition is for the practitioner compartment."; 151 case DEVICE: 152 return "The compartment definition is for the device compartment."; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getDisplay() { 161 switch (this) { 162 case PATIENT: 163 return "Patient"; 164 case ENCOUNTER: 165 return "Encounter"; 166 case RELATEDPERSON: 167 return "RelatedPerson"; 168 case PRACTITIONER: 169 return "Practitioner"; 170 case DEVICE: 171 return "Device"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 } 179 180 public static class CompartmentTypeEnumFactory implements EnumFactory<CompartmentType> { 181 public CompartmentType fromCode(String codeString) throws IllegalArgumentException { 182 if (codeString == null || "".equals(codeString)) 183 if (codeString == null || "".equals(codeString)) 184 return null; 185 if ("Patient".equals(codeString)) 186 return CompartmentType.PATIENT; 187 if ("Encounter".equals(codeString)) 188 return CompartmentType.ENCOUNTER; 189 if ("RelatedPerson".equals(codeString)) 190 return CompartmentType.RELATEDPERSON; 191 if ("Practitioner".equals(codeString)) 192 return CompartmentType.PRACTITIONER; 193 if ("Device".equals(codeString)) 194 return CompartmentType.DEVICE; 195 throw new IllegalArgumentException("Unknown CompartmentType code '" + codeString + "'"); 196 } 197 198 public Enumeration<CompartmentType> fromType(PrimitiveType<?> code) throws FHIRException { 199 if (code == null) 200 return null; 201 if (code.isEmpty()) 202 return new Enumeration<CompartmentType>(this, CompartmentType.NULL, code); 203 String codeString = code.asStringValue(); 204 if (codeString == null || "".equals(codeString)) 205 return new Enumeration<CompartmentType>(this, CompartmentType.NULL, code); 206 if ("Patient".equals(codeString)) 207 return new Enumeration<CompartmentType>(this, CompartmentType.PATIENT, code); 208 if ("Encounter".equals(codeString)) 209 return new Enumeration<CompartmentType>(this, CompartmentType.ENCOUNTER, code); 210 if ("RelatedPerson".equals(codeString)) 211 return new Enumeration<CompartmentType>(this, CompartmentType.RELATEDPERSON, code); 212 if ("Practitioner".equals(codeString)) 213 return new Enumeration<CompartmentType>(this, CompartmentType.PRACTITIONER, code); 214 if ("Device".equals(codeString)) 215 return new Enumeration<CompartmentType>(this, CompartmentType.DEVICE, code); 216 throw new FHIRException("Unknown CompartmentType code '" + codeString + "'"); 217 } 218 219 public String toCode(CompartmentType code) { 220 if (code == CompartmentType.NULL) 221 return null; 222 if (code == CompartmentType.PATIENT) 223 return "Patient"; 224 if (code == CompartmentType.ENCOUNTER) 225 return "Encounter"; 226 if (code == CompartmentType.RELATEDPERSON) 227 return "RelatedPerson"; 228 if (code == CompartmentType.PRACTITIONER) 229 return "Practitioner"; 230 if (code == CompartmentType.DEVICE) 231 return "Device"; 232 return "?"; 233 } 234 235 public String toSystem(CompartmentType code) { 236 return code.getSystem(); 237 } 238 } 239 240 @Block() 241 public static class CompartmentDefinitionResourceComponent extends BackboneElement implements IBaseBackboneElement { 242 /** 243 * The name of a resource supported by the server. 244 */ 245 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 246 @Description(shortDefinition = "Name of resource type", formalDefinition = "The name of a resource supported by the server.") 247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 248 protected CodeType code; 249 250 /** 251 * The name of a search parameter that represents the link to the compartment. 252 * More than one may be listed because a resource may be linked to a compartment 253 * in more than one way,. 254 */ 255 @Child(name = "param", type = { 256 StringType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 257 @Description(shortDefinition = "Search Parameter Name, or chained parameters", formalDefinition = "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.") 258 protected List<StringType> param; 259 260 /** 261 * Additional documentation about the resource and compartment. 262 */ 263 @Child(name = "documentation", type = { 264 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 265 @Description(shortDefinition = "Additional documentation about the resource and compartment", formalDefinition = "Additional documentation about the resource and compartment.") 266 protected StringType documentation; 267 268 private static final long serialVersionUID = 988080897L; 269 270 /** 271 * Constructor 272 */ 273 public CompartmentDefinitionResourceComponent() { 274 super(); 275 } 276 277 /** 278 * Constructor 279 */ 280 public CompartmentDefinitionResourceComponent(CodeType code) { 281 super(); 282 this.code = code; 283 } 284 285 /** 286 * @return {@link #code} (The name of a resource supported by the server.). This 287 * is the underlying object with id, value and extensions. The accessor 288 * "getCode" gives direct access to the value 289 */ 290 public CodeType getCodeElement() { 291 if (this.code == null) 292 if (Configuration.errorOnAutoCreate()) 293 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.code"); 294 else if (Configuration.doAutoCreate()) 295 this.code = new CodeType(); // bb 296 return this.code; 297 } 298 299 public boolean hasCodeElement() { 300 return this.code != null && !this.code.isEmpty(); 301 } 302 303 public boolean hasCode() { 304 return this.code != null && !this.code.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #code} (The name of a resource supported by the server.). 309 * This is the underlying object with id, value and extensions. The 310 * accessor "getCode" gives direct access to the value 311 */ 312 public CompartmentDefinitionResourceComponent setCodeElement(CodeType value) { 313 this.code = value; 314 return this; 315 } 316 317 /** 318 * @return The name of a resource supported by the server. 319 */ 320 public String getCode() { 321 return this.code == null ? null : this.code.getValue(); 322 } 323 324 /** 325 * @param value The name of a resource supported by the server. 326 */ 327 public CompartmentDefinitionResourceComponent setCode(String value) { 328 if (this.code == null) 329 this.code = new CodeType(); 330 this.code.setValue(value); 331 return this; 332 } 333 334 /** 335 * @return {@link #param} (The name of a search parameter that represents the 336 * link to the compartment. More than one may be listed because a 337 * resource may be linked to a compartment in more than one way,.) 338 */ 339 public List<StringType> getParam() { 340 if (this.param == null) 341 this.param = new ArrayList<StringType>(); 342 return this.param; 343 } 344 345 /** 346 * @return Returns a reference to <code>this</code> for easy method chaining 347 */ 348 public CompartmentDefinitionResourceComponent setParam(List<StringType> theParam) { 349 this.param = theParam; 350 return this; 351 } 352 353 public boolean hasParam() { 354 if (this.param == null) 355 return false; 356 for (StringType item : this.param) 357 if (!item.isEmpty()) 358 return true; 359 return false; 360 } 361 362 /** 363 * @return {@link #param} (The name of a search parameter that represents the 364 * link to the compartment. More than one may be listed because a 365 * resource may be linked to a compartment in more than one way,.) 366 */ 367 public StringType addParamElement() {// 2 368 StringType t = new StringType(); 369 if (this.param == null) 370 this.param = new ArrayList<StringType>(); 371 this.param.add(t); 372 return t; 373 } 374 375 /** 376 * @param value {@link #param} (The name of a search parameter that represents 377 * the link to the compartment. More than one may be listed because 378 * a resource may be linked to a compartment in more than one 379 * way,.) 380 */ 381 public CompartmentDefinitionResourceComponent addParam(String value) { // 1 382 StringType t = new StringType(); 383 t.setValue(value); 384 if (this.param == null) 385 this.param = new ArrayList<StringType>(); 386 this.param.add(t); 387 return this; 388 } 389 390 /** 391 * @param value {@link #param} (The name of a search parameter that represents 392 * the link to the compartment. More than one may be listed because 393 * a resource may be linked to a compartment in more than one 394 * way,.) 395 */ 396 public boolean hasParam(String value) { 397 if (this.param == null) 398 return false; 399 for (StringType v : this.param) 400 if (v.getValue().equals(value)) // string 401 return true; 402 return false; 403 } 404 405 /** 406 * @return {@link #documentation} (Additional documentation about the resource 407 * and compartment.). This is the underlying object with id, value and 408 * extensions. The accessor "getDocumentation" gives direct access to 409 * the value 410 */ 411 public StringType getDocumentationElement() { 412 if (this.documentation == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create CompartmentDefinitionResourceComponent.documentation"); 415 else if (Configuration.doAutoCreate()) 416 this.documentation = new StringType(); // bb 417 return this.documentation; 418 } 419 420 public boolean hasDocumentationElement() { 421 return this.documentation != null && !this.documentation.isEmpty(); 422 } 423 424 public boolean hasDocumentation() { 425 return this.documentation != null && !this.documentation.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #documentation} (Additional documentation about the 430 * resource and compartment.). This is the underlying object with 431 * id, value and extensions. The accessor "getDocumentation" gives 432 * direct access to the value 433 */ 434 public CompartmentDefinitionResourceComponent setDocumentationElement(StringType value) { 435 this.documentation = value; 436 return this; 437 } 438 439 /** 440 * @return Additional documentation about the resource and compartment. 441 */ 442 public String getDocumentation() { 443 return this.documentation == null ? null : this.documentation.getValue(); 444 } 445 446 /** 447 * @param value Additional documentation about the resource and compartment. 448 */ 449 public CompartmentDefinitionResourceComponent setDocumentation(String value) { 450 if (Utilities.noString(value)) 451 this.documentation = null; 452 else { 453 if (this.documentation == null) 454 this.documentation = new StringType(); 455 this.documentation.setValue(value); 456 } 457 return this; 458 } 459 460 protected void listChildren(List<Property> children) { 461 super.listChildren(children); 462 children.add(new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code)); 463 children.add(new Property("param", "string", 464 "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 465 0, java.lang.Integer.MAX_VALUE, param)); 466 children.add(new Property("documentation", "string", 467 "Additional documentation about the resource and compartment.", 0, 1, documentation)); 468 } 469 470 @Override 471 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 472 switch (_hash) { 473 case 3059181: 474 /* code */ return new Property("code", "code", "The name of a resource supported by the server.", 0, 1, code); 475 case 106436749: 476 /* param */ return new Property("param", "string", 477 "The name of a search parameter that represents the link to the compartment. More than one may be listed because a resource may be linked to a compartment in more than one way,.", 478 0, java.lang.Integer.MAX_VALUE, param); 479 case 1587405498: 480 /* documentation */ return new Property("documentation", "string", 481 "Additional documentation about the resource and compartment.", 0, 1, documentation); 482 default: 483 return super.getNamedProperty(_hash, _name, _checkValid); 484 } 485 486 } 487 488 @Override 489 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 490 switch (hash) { 491 case 3059181: 492 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 493 case 106436749: 494 /* param */ return this.param == null ? new Base[0] : this.param.toArray(new Base[this.param.size()]); // StringType 495 case 1587405498: 496 /* documentation */ return this.documentation == null ? new Base[0] : new Base[] { this.documentation }; // StringType 497 default: 498 return super.getProperty(hash, name, checkValid); 499 } 500 501 } 502 503 @Override 504 public Base setProperty(int hash, String name, Base value) throws FHIRException { 505 switch (hash) { 506 case 3059181: // code 507 this.code = castToCode(value); // CodeType 508 return value; 509 case 106436749: // param 510 this.getParam().add(castToString(value)); // StringType 511 return value; 512 case 1587405498: // documentation 513 this.documentation = castToString(value); // StringType 514 return value; 515 default: 516 return super.setProperty(hash, name, value); 517 } 518 519 } 520 521 @Override 522 public Base setProperty(String name, Base value) throws FHIRException { 523 if (name.equals("code")) { 524 this.code = castToCode(value); // CodeType 525 } else if (name.equals("param")) { 526 this.getParam().add(castToString(value)); 527 } else if (name.equals("documentation")) { 528 this.documentation = castToString(value); // StringType 529 } else 530 return super.setProperty(name, value); 531 return value; 532 } 533 534 @Override 535 public void removeChild(String name, Base value) throws FHIRException { 536 if (name.equals("code")) { 537 this.code = null; 538 } else if (name.equals("param")) { 539 this.getParam().remove(castToString(value)); 540 } else if (name.equals("documentation")) { 541 this.documentation = null; 542 } else 543 super.removeChild(name, value); 544 545 } 546 547 @Override 548 public Base makeProperty(int hash, String name) throws FHIRException { 549 switch (hash) { 550 case 3059181: 551 return getCodeElement(); 552 case 106436749: 553 return addParamElement(); 554 case 1587405498: 555 return getDocumentationElement(); 556 default: 557 return super.makeProperty(hash, name); 558 } 559 560 } 561 562 @Override 563 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case 3059181: 566 /* code */ return new String[] { "code" }; 567 case 106436749: 568 /* param */ return new String[] { "string" }; 569 case 1587405498: 570 /* documentation */ return new String[] { "string" }; 571 default: 572 return super.getTypesForProperty(hash, name); 573 } 574 575 } 576 577 @Override 578 public Base addChild(String name) throws FHIRException { 579 if (name.equals("code")) { 580 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 581 } else if (name.equals("param")) { 582 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.param"); 583 } else if (name.equals("documentation")) { 584 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.documentation"); 585 } else 586 return super.addChild(name); 587 } 588 589 public CompartmentDefinitionResourceComponent copy() { 590 CompartmentDefinitionResourceComponent dst = new CompartmentDefinitionResourceComponent(); 591 copyValues(dst); 592 return dst; 593 } 594 595 public void copyValues(CompartmentDefinitionResourceComponent dst) { 596 super.copyValues(dst); 597 dst.code = code == null ? null : code.copy(); 598 if (param != null) { 599 dst.param = new ArrayList<StringType>(); 600 for (StringType i : param) 601 dst.param.add(i.copy()); 602 } 603 ; 604 dst.documentation = documentation == null ? null : documentation.copy(); 605 } 606 607 @Override 608 public boolean equalsDeep(Base other_) { 609 if (!super.equalsDeep(other_)) 610 return false; 611 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 612 return false; 613 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 614 return compareDeep(code, o.code, true) && compareDeep(param, o.param, true) 615 && compareDeep(documentation, o.documentation, true); 616 } 617 618 @Override 619 public boolean equalsShallow(Base other_) { 620 if (!super.equalsShallow(other_)) 621 return false; 622 if (!(other_ instanceof CompartmentDefinitionResourceComponent)) 623 return false; 624 CompartmentDefinitionResourceComponent o = (CompartmentDefinitionResourceComponent) other_; 625 return compareValues(code, o.code, true) && compareValues(param, o.param, true) 626 && compareValues(documentation, o.documentation, true); 627 } 628 629 public boolean isEmpty() { 630 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, param, documentation); 631 } 632 633 public String fhirType() { 634 return "CompartmentDefinition.resource"; 635 636 } 637 638 } 639 640 /** 641 * Explanation of why this compartment definition is needed and why it has been 642 * designed as it has. 643 */ 644 @Child(name = "purpose", type = { 645 MarkdownType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 646 @Description(shortDefinition = "Why this compartment definition is defined", formalDefinition = "Explanation of why this compartment definition is needed and why it has been designed as it has.") 647 protected MarkdownType purpose; 648 649 /** 650 * Which compartment this definition describes. 651 */ 652 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 653 @Description(shortDefinition = "Patient | Encounter | RelatedPerson | Practitioner | Device", formalDefinition = "Which compartment this definition describes.") 654 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/compartment-type") 655 protected Enumeration<CompartmentType> code; 656 657 /** 658 * Whether the search syntax is supported,. 659 */ 660 @Child(name = "search", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 661 @Description(shortDefinition = "Whether the search syntax is supported", formalDefinition = "Whether the search syntax is supported,.") 662 protected BooleanType search; 663 664 /** 665 * Information about how a resource is related to the compartment. 666 */ 667 @Child(name = "resource", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 668 @Description(shortDefinition = "How a resource is related to the compartment", formalDefinition = "Information about how a resource is related to the compartment.") 669 protected List<CompartmentDefinitionResourceComponent> resource; 670 671 private static final long serialVersionUID = -1159172945L; 672 673 /** 674 * Constructor 675 */ 676 public CompartmentDefinition() { 677 super(); 678 } 679 680 /** 681 * Constructor 682 */ 683 public CompartmentDefinition(UriType url, StringType name, Enumeration<PublicationStatus> status, 684 Enumeration<CompartmentType> code, BooleanType search) { 685 super(); 686 this.url = url; 687 this.name = name; 688 this.status = status; 689 this.code = code; 690 this.search = search; 691 } 692 693 /** 694 * @return {@link #url} (An absolute URI that is used to identify this 695 * compartment definition when it is referenced in a specification, 696 * model, design or an instance; also called its canonical identifier. 697 * This SHOULD be globally unique and SHOULD be a literal address at 698 * which at which an authoritative instance of this compartment 699 * definition is (or will be) published. This URL can be the target of a 700 * canonical reference. It SHALL remain the same when the compartment 701 * definition is stored on different servers.). This is the underlying 702 * object with id, value and extensions. The accessor "getUrl" gives 703 * direct access to the value 704 */ 705 public UriType getUrlElement() { 706 if (this.url == null) 707 if (Configuration.errorOnAutoCreate()) 708 throw new Error("Attempt to auto-create CompartmentDefinition.url"); 709 else if (Configuration.doAutoCreate()) 710 this.url = new UriType(); // bb 711 return this.url; 712 } 713 714 public boolean hasUrlElement() { 715 return this.url != null && !this.url.isEmpty(); 716 } 717 718 public boolean hasUrl() { 719 return this.url != null && !this.url.isEmpty(); 720 } 721 722 /** 723 * @param value {@link #url} (An absolute URI that is used to identify this 724 * compartment definition when it is referenced in a specification, 725 * model, design or an instance; also called its canonical 726 * identifier. This SHOULD be globally unique and SHOULD be a 727 * literal address at which at which an authoritative instance of 728 * this compartment definition is (or will be) published. This URL 729 * can be the target of a canonical reference. It SHALL remain the 730 * same when the compartment definition is stored on different 731 * servers.). This is the underlying object with id, value and 732 * extensions. The accessor "getUrl" gives direct access to the 733 * value 734 */ 735 public CompartmentDefinition setUrlElement(UriType value) { 736 this.url = value; 737 return this; 738 } 739 740 /** 741 * @return An absolute URI that is used to identify this compartment definition 742 * when it is referenced in a specification, model, design or an 743 * instance; also called its canonical identifier. This SHOULD be 744 * globally unique and SHOULD be a literal address at which at which an 745 * authoritative instance of this compartment definition is (or will be) 746 * published. This URL can be the target of a canonical reference. It 747 * SHALL remain the same when the compartment definition is stored on 748 * different servers. 749 */ 750 public String getUrl() { 751 return this.url == null ? null : this.url.getValue(); 752 } 753 754 /** 755 * @param value An absolute URI that is used to identify this compartment 756 * definition when it is referenced in a specification, model, 757 * design or an instance; also called its canonical identifier. 758 * This SHOULD be globally unique and SHOULD be a literal address 759 * at which at which an authoritative instance of this compartment 760 * definition is (or will be) published. This URL can be the target 761 * of a canonical reference. It SHALL remain the same when the 762 * compartment definition is stored on different servers. 763 */ 764 public CompartmentDefinition setUrl(String value) { 765 if (this.url == null) 766 this.url = new UriType(); 767 this.url.setValue(value); 768 return this; 769 } 770 771 /** 772 * @return {@link #version} (The identifier that is used to identify this 773 * version of the compartment definition when it is referenced in a 774 * specification, model, design or instance. This is an arbitrary value 775 * managed by the compartment definition author and is not expected to 776 * be globally unique. For example, it might be a timestamp (e.g. 777 * yyyymmdd) if a managed version is not available. There is also no 778 * expectation that versions can be placed in a lexicographical 779 * sequence.). This is the underlying object with id, value and 780 * extensions. The accessor "getVersion" gives direct access to the 781 * value 782 */ 783 public StringType getVersionElement() { 784 if (this.version == null) 785 if (Configuration.errorOnAutoCreate()) 786 throw new Error("Attempt to auto-create CompartmentDefinition.version"); 787 else if (Configuration.doAutoCreate()) 788 this.version = new StringType(); // bb 789 return this.version; 790 } 791 792 public boolean hasVersionElement() { 793 return this.version != null && !this.version.isEmpty(); 794 } 795 796 public boolean hasVersion() { 797 return this.version != null && !this.version.isEmpty(); 798 } 799 800 /** 801 * @param value {@link #version} (The identifier that is used to identify this 802 * version of the compartment definition when it is referenced in a 803 * specification, model, design or instance. This is an arbitrary 804 * value managed by the compartment definition author and is not 805 * expected to be globally unique. For example, it might be a 806 * timestamp (e.g. yyyymmdd) if a managed version is not available. 807 * There is also no expectation that versions can be placed in a 808 * lexicographical sequence.). This is the underlying object with 809 * id, value and extensions. The accessor "getVersion" gives direct 810 * access to the value 811 */ 812 public CompartmentDefinition setVersionElement(StringType value) { 813 this.version = value; 814 return this; 815 } 816 817 /** 818 * @return The identifier that is used to identify this version of the 819 * compartment definition when it is referenced in a specification, 820 * model, design or instance. This is an arbitrary value managed by the 821 * compartment definition author and is not expected to be globally 822 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if a 823 * managed version is not available. There is also no expectation that 824 * versions can be placed in a lexicographical sequence. 825 */ 826 public String getVersion() { 827 return this.version == null ? null : this.version.getValue(); 828 } 829 830 /** 831 * @param value The identifier that is used to identify this version of the 832 * compartment definition when it is referenced in a specification, 833 * model, design or instance. This is an arbitrary value managed by 834 * the compartment definition author and is not expected to be 835 * globally unique. For example, it might be a timestamp (e.g. 836 * yyyymmdd) if a managed version is not available. There is also 837 * no expectation that versions can be placed in a lexicographical 838 * sequence. 839 */ 840 public CompartmentDefinition setVersion(String value) { 841 if (Utilities.noString(value)) 842 this.version = null; 843 else { 844 if (this.version == null) 845 this.version = new StringType(); 846 this.version.setValue(value); 847 } 848 return this; 849 } 850 851 /** 852 * @return {@link #name} (A natural language name identifying the compartment 853 * definition. This name should be usable as an identifier for the 854 * module by machine processing applications such as code generation.). 855 * This is the underlying object with id, value and extensions. The 856 * accessor "getName" gives direct access to the value 857 */ 858 public StringType getNameElement() { 859 if (this.name == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create CompartmentDefinition.name"); 862 else if (Configuration.doAutoCreate()) 863 this.name = new StringType(); // bb 864 return this.name; 865 } 866 867 public boolean hasNameElement() { 868 return this.name != null && !this.name.isEmpty(); 869 } 870 871 public boolean hasName() { 872 return this.name != null && !this.name.isEmpty(); 873 } 874 875 /** 876 * @param value {@link #name} (A natural language name identifying the 877 * compartment definition. This name should be usable as an 878 * identifier for the module by machine processing applications 879 * such as code generation.). This is the underlying object with 880 * id, value and extensions. The accessor "getName" gives direct 881 * access to the value 882 */ 883 public CompartmentDefinition setNameElement(StringType value) { 884 this.name = value; 885 return this; 886 } 887 888 /** 889 * @return A natural language name identifying the compartment definition. This 890 * name should be usable as an identifier for the module by machine 891 * processing applications such as code generation. 892 */ 893 public String getName() { 894 return this.name == null ? null : this.name.getValue(); 895 } 896 897 /** 898 * @param value A natural language name identifying the compartment definition. 899 * This name should be usable as an identifier for the module by 900 * machine processing applications such as code generation. 901 */ 902 public CompartmentDefinition setName(String value) { 903 if (this.name == null) 904 this.name = new StringType(); 905 this.name.setValue(value); 906 return this; 907 } 908 909 /** 910 * @return {@link #status} (The status of this compartment definition. Enables 911 * tracking the life-cycle of the content.). This is the underlying 912 * object with id, value and extensions. The accessor "getStatus" gives 913 * direct access to the value 914 */ 915 public Enumeration<PublicationStatus> getStatusElement() { 916 if (this.status == null) 917 if (Configuration.errorOnAutoCreate()) 918 throw new Error("Attempt to auto-create CompartmentDefinition.status"); 919 else if (Configuration.doAutoCreate()) 920 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 921 return this.status; 922 } 923 924 public boolean hasStatusElement() { 925 return this.status != null && !this.status.isEmpty(); 926 } 927 928 public boolean hasStatus() { 929 return this.status != null && !this.status.isEmpty(); 930 } 931 932 /** 933 * @param value {@link #status} (The status of this compartment definition. 934 * Enables tracking the life-cycle of the content.). This is the 935 * underlying object with id, value and extensions. The accessor 936 * "getStatus" gives direct access to the value 937 */ 938 public CompartmentDefinition setStatusElement(Enumeration<PublicationStatus> value) { 939 this.status = value; 940 return this; 941 } 942 943 /** 944 * @return The status of this compartment definition. Enables tracking the 945 * life-cycle of the content. 946 */ 947 public PublicationStatus getStatus() { 948 return this.status == null ? null : this.status.getValue(); 949 } 950 951 /** 952 * @param value The status of this compartment definition. Enables tracking the 953 * life-cycle of the content. 954 */ 955 public CompartmentDefinition setStatus(PublicationStatus value) { 956 if (this.status == null) 957 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 958 this.status.setValue(value); 959 return this; 960 } 961 962 /** 963 * @return {@link #experimental} (A Boolean value to indicate that this 964 * compartment definition is authored for testing purposes (or 965 * education/evaluation/marketing) and is not intended to be used for 966 * genuine usage.). This is the underlying object with id, value and 967 * extensions. The accessor "getExperimental" gives direct access to the 968 * value 969 */ 970 public BooleanType getExperimentalElement() { 971 if (this.experimental == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create CompartmentDefinition.experimental"); 974 else if (Configuration.doAutoCreate()) 975 this.experimental = new BooleanType(); // bb 976 return this.experimental; 977 } 978 979 public boolean hasExperimentalElement() { 980 return this.experimental != null && !this.experimental.isEmpty(); 981 } 982 983 public boolean hasExperimental() { 984 return this.experimental != null && !this.experimental.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #experimental} (A Boolean value to indicate that this 989 * compartment definition is authored for testing purposes (or 990 * education/evaluation/marketing) and is not intended to be used 991 * for genuine usage.). This is the underlying object with id, 992 * value and extensions. The accessor "getExperimental" gives 993 * direct access to the value 994 */ 995 public CompartmentDefinition setExperimentalElement(BooleanType value) { 996 this.experimental = value; 997 return this; 998 } 999 1000 /** 1001 * @return A Boolean value to indicate that this compartment definition is 1002 * authored for testing purposes (or education/evaluation/marketing) and 1003 * is not intended to be used for genuine usage. 1004 */ 1005 public boolean getExperimental() { 1006 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1007 } 1008 1009 /** 1010 * @param value A Boolean value to indicate that this compartment definition is 1011 * authored for testing purposes (or 1012 * education/evaluation/marketing) and is not intended to be used 1013 * for genuine usage. 1014 */ 1015 public CompartmentDefinition setExperimental(boolean value) { 1016 if (this.experimental == null) 1017 this.experimental = new BooleanType(); 1018 this.experimental.setValue(value); 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #date} (The date (and optionally time) when the compartment 1024 * definition was published. The date must change when the business 1025 * version changes and it must change if the status code changes. In 1026 * addition, it should change when the substantive content of the 1027 * compartment definition changes.). This is the underlying object with 1028 * id, value and extensions. The accessor "getDate" gives direct access 1029 * to the value 1030 */ 1031 public DateTimeType getDateElement() { 1032 if (this.date == null) 1033 if (Configuration.errorOnAutoCreate()) 1034 throw new Error("Attempt to auto-create CompartmentDefinition.date"); 1035 else if (Configuration.doAutoCreate()) 1036 this.date = new DateTimeType(); // bb 1037 return this.date; 1038 } 1039 1040 public boolean hasDateElement() { 1041 return this.date != null && !this.date.isEmpty(); 1042 } 1043 1044 public boolean hasDate() { 1045 return this.date != null && !this.date.isEmpty(); 1046 } 1047 1048 /** 1049 * @param value {@link #date} (The date (and optionally time) when the 1050 * compartment definition was published. The date must change when 1051 * the business version changes and it must change if the status 1052 * code changes. In addition, it should change when the substantive 1053 * content of the compartment definition changes.). This is the 1054 * underlying object with id, value and extensions. The accessor 1055 * "getDate" gives direct access to the value 1056 */ 1057 public CompartmentDefinition setDateElement(DateTimeType value) { 1058 this.date = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return The date (and optionally time) when the compartment definition was 1064 * published. The date must change when the business version changes and 1065 * it must change if the status code changes. In addition, it should 1066 * change when the substantive content of the compartment definition 1067 * changes. 1068 */ 1069 public Date getDate() { 1070 return this.date == null ? null : this.date.getValue(); 1071 } 1072 1073 /** 1074 * @param value The date (and optionally time) when the compartment definition 1075 * was published. The date must change when the business version 1076 * changes and it must change if the status code changes. In 1077 * addition, it should change when the substantive content of the 1078 * compartment definition changes. 1079 */ 1080 public CompartmentDefinition setDate(Date value) { 1081 if (value == null) 1082 this.date = null; 1083 else { 1084 if (this.date == null) 1085 this.date = new DateTimeType(); 1086 this.date.setValue(value); 1087 } 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #publisher} (The name of the organization or individual that 1093 * published the compartment definition.). This is the underlying object 1094 * with id, value and extensions. The accessor "getPublisher" gives 1095 * direct access to the value 1096 */ 1097 public StringType getPublisherElement() { 1098 if (this.publisher == null) 1099 if (Configuration.errorOnAutoCreate()) 1100 throw new Error("Attempt to auto-create CompartmentDefinition.publisher"); 1101 else if (Configuration.doAutoCreate()) 1102 this.publisher = new StringType(); // bb 1103 return this.publisher; 1104 } 1105 1106 public boolean hasPublisherElement() { 1107 return this.publisher != null && !this.publisher.isEmpty(); 1108 } 1109 1110 public boolean hasPublisher() { 1111 return this.publisher != null && !this.publisher.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #publisher} (The name of the organization or individual 1116 * that published the compartment definition.). This is the 1117 * underlying object with id, value and extensions. The accessor 1118 * "getPublisher" gives direct access to the value 1119 */ 1120 public CompartmentDefinition setPublisherElement(StringType value) { 1121 this.publisher = value; 1122 return this; 1123 } 1124 1125 /** 1126 * @return The name of the organization or individual that published the 1127 * compartment definition. 1128 */ 1129 public String getPublisher() { 1130 return this.publisher == null ? null : this.publisher.getValue(); 1131 } 1132 1133 /** 1134 * @param value The name of the organization or individual that published the 1135 * compartment definition. 1136 */ 1137 public CompartmentDefinition setPublisher(String value) { 1138 if (Utilities.noString(value)) 1139 this.publisher = null; 1140 else { 1141 if (this.publisher == null) 1142 this.publisher = new StringType(); 1143 this.publisher.setValue(value); 1144 } 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #contact} (Contact details to assist a user in finding and 1150 * communicating with the publisher.) 1151 */ 1152 public List<ContactDetail> getContact() { 1153 if (this.contact == null) 1154 this.contact = new ArrayList<ContactDetail>(); 1155 return this.contact; 1156 } 1157 1158 /** 1159 * @return Returns a reference to <code>this</code> for easy method chaining 1160 */ 1161 public CompartmentDefinition setContact(List<ContactDetail> theContact) { 1162 this.contact = theContact; 1163 return this; 1164 } 1165 1166 public boolean hasContact() { 1167 if (this.contact == null) 1168 return false; 1169 for (ContactDetail item : this.contact) 1170 if (!item.isEmpty()) 1171 return true; 1172 return false; 1173 } 1174 1175 public ContactDetail addContact() { // 3 1176 ContactDetail t = new ContactDetail(); 1177 if (this.contact == null) 1178 this.contact = new ArrayList<ContactDetail>(); 1179 this.contact.add(t); 1180 return t; 1181 } 1182 1183 public CompartmentDefinition addContact(ContactDetail t) { // 3 1184 if (t == null) 1185 return this; 1186 if (this.contact == null) 1187 this.contact = new ArrayList<ContactDetail>(); 1188 this.contact.add(t); 1189 return this; 1190 } 1191 1192 /** 1193 * @return The first repetition of repeating field {@link #contact}, creating it 1194 * if it does not already exist 1195 */ 1196 public ContactDetail getContactFirstRep() { 1197 if (getContact().isEmpty()) { 1198 addContact(); 1199 } 1200 return getContact().get(0); 1201 } 1202 1203 /** 1204 * @return {@link #description} (A free text natural language description of the 1205 * compartment definition from a consumer's perspective.). This is the 1206 * underlying object with id, value and extensions. The accessor 1207 * "getDescription" gives direct access to the value 1208 */ 1209 public MarkdownType getDescriptionElement() { 1210 if (this.description == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create CompartmentDefinition.description"); 1213 else if (Configuration.doAutoCreate()) 1214 this.description = new MarkdownType(); // bb 1215 return this.description; 1216 } 1217 1218 public boolean hasDescriptionElement() { 1219 return this.description != null && !this.description.isEmpty(); 1220 } 1221 1222 public boolean hasDescription() { 1223 return this.description != null && !this.description.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #description} (A free text natural language description 1228 * of the compartment definition from a consumer's perspective.). 1229 * This is the underlying object with id, value and extensions. The 1230 * accessor "getDescription" gives direct access to the value 1231 */ 1232 public CompartmentDefinition setDescriptionElement(MarkdownType value) { 1233 this.description = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return A free text natural language description of the compartment 1239 * definition from a consumer's perspective. 1240 */ 1241 public String getDescription() { 1242 return this.description == null ? null : this.description.getValue(); 1243 } 1244 1245 /** 1246 * @param value A free text natural language description of the compartment 1247 * definition from a consumer's perspective. 1248 */ 1249 public CompartmentDefinition setDescription(String value) { 1250 if (value == null) 1251 this.description = null; 1252 else { 1253 if (this.description == null) 1254 this.description = new MarkdownType(); 1255 this.description.setValue(value); 1256 } 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #useContext} (The content was developed with a focus and 1262 * intent of supporting the contexts that are listed. These contexts may 1263 * be general categories (gender, age, ...) or may be references to 1264 * specific programs (insurance plans, studies, ...) and may be used to 1265 * assist with indexing and searching for appropriate compartment 1266 * definition instances.) 1267 */ 1268 public List<UsageContext> getUseContext() { 1269 if (this.useContext == null) 1270 this.useContext = new ArrayList<UsageContext>(); 1271 return this.useContext; 1272 } 1273 1274 /** 1275 * @return Returns a reference to <code>this</code> for easy method chaining 1276 */ 1277 public CompartmentDefinition setUseContext(List<UsageContext> theUseContext) { 1278 this.useContext = theUseContext; 1279 return this; 1280 } 1281 1282 public boolean hasUseContext() { 1283 if (this.useContext == null) 1284 return false; 1285 for (UsageContext item : this.useContext) 1286 if (!item.isEmpty()) 1287 return true; 1288 return false; 1289 } 1290 1291 public UsageContext addUseContext() { // 3 1292 UsageContext t = new UsageContext(); 1293 if (this.useContext == null) 1294 this.useContext = new ArrayList<UsageContext>(); 1295 this.useContext.add(t); 1296 return t; 1297 } 1298 1299 public CompartmentDefinition addUseContext(UsageContext t) { // 3 1300 if (t == null) 1301 return this; 1302 if (this.useContext == null) 1303 this.useContext = new ArrayList<UsageContext>(); 1304 this.useContext.add(t); 1305 return this; 1306 } 1307 1308 /** 1309 * @return The first repetition of repeating field {@link #useContext}, creating 1310 * it if it does not already exist 1311 */ 1312 public UsageContext getUseContextFirstRep() { 1313 if (getUseContext().isEmpty()) { 1314 addUseContext(); 1315 } 1316 return getUseContext().get(0); 1317 } 1318 1319 /** 1320 * @return {@link #purpose} (Explanation of why this compartment definition is 1321 * needed and why it has been designed as it has.). This is the 1322 * underlying object with id, value and extensions. The accessor 1323 * "getPurpose" gives direct access to the value 1324 */ 1325 public MarkdownType getPurposeElement() { 1326 if (this.purpose == null) 1327 if (Configuration.errorOnAutoCreate()) 1328 throw new Error("Attempt to auto-create CompartmentDefinition.purpose"); 1329 else if (Configuration.doAutoCreate()) 1330 this.purpose = new MarkdownType(); // bb 1331 return this.purpose; 1332 } 1333 1334 public boolean hasPurposeElement() { 1335 return this.purpose != null && !this.purpose.isEmpty(); 1336 } 1337 1338 public boolean hasPurpose() { 1339 return this.purpose != null && !this.purpose.isEmpty(); 1340 } 1341 1342 /** 1343 * @param value {@link #purpose} (Explanation of why this compartment definition 1344 * is needed and why it has been designed as it has.). This is the 1345 * underlying object with id, value and extensions. The accessor 1346 * "getPurpose" gives direct access to the value 1347 */ 1348 public CompartmentDefinition setPurposeElement(MarkdownType value) { 1349 this.purpose = value; 1350 return this; 1351 } 1352 1353 /** 1354 * @return Explanation of why this compartment definition is needed and why it 1355 * has been designed as it has. 1356 */ 1357 public String getPurpose() { 1358 return this.purpose == null ? null : this.purpose.getValue(); 1359 } 1360 1361 /** 1362 * @param value Explanation of why this compartment definition is needed and why 1363 * it has been designed as it has. 1364 */ 1365 public CompartmentDefinition setPurpose(String value) { 1366 if (value == null) 1367 this.purpose = null; 1368 else { 1369 if (this.purpose == null) 1370 this.purpose = new MarkdownType(); 1371 this.purpose.setValue(value); 1372 } 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #code} (Which compartment this definition describes.). This is 1378 * the underlying object with id, value and extensions. The accessor 1379 * "getCode" gives direct access to the value 1380 */ 1381 public Enumeration<CompartmentType> getCodeElement() { 1382 if (this.code == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create CompartmentDefinition.code"); 1385 else if (Configuration.doAutoCreate()) 1386 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); // bb 1387 return this.code; 1388 } 1389 1390 public boolean hasCodeElement() { 1391 return this.code != null && !this.code.isEmpty(); 1392 } 1393 1394 public boolean hasCode() { 1395 return this.code != null && !this.code.isEmpty(); 1396 } 1397 1398 /** 1399 * @param value {@link #code} (Which compartment this definition describes.). 1400 * This is the underlying object with id, value and extensions. The 1401 * accessor "getCode" gives direct access to the value 1402 */ 1403 public CompartmentDefinition setCodeElement(Enumeration<CompartmentType> value) { 1404 this.code = value; 1405 return this; 1406 } 1407 1408 /** 1409 * @return Which compartment this definition describes. 1410 */ 1411 public CompartmentType getCode() { 1412 return this.code == null ? null : this.code.getValue(); 1413 } 1414 1415 /** 1416 * @param value Which compartment this definition describes. 1417 */ 1418 public CompartmentDefinition setCode(CompartmentType value) { 1419 if (this.code == null) 1420 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); 1421 this.code.setValue(value); 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #search} (Whether the search syntax is supported,.). This is 1427 * the underlying object with id, value and extensions. The accessor 1428 * "getSearch" gives direct access to the value 1429 */ 1430 public BooleanType getSearchElement() { 1431 if (this.search == null) 1432 if (Configuration.errorOnAutoCreate()) 1433 throw new Error("Attempt to auto-create CompartmentDefinition.search"); 1434 else if (Configuration.doAutoCreate()) 1435 this.search = new BooleanType(); // bb 1436 return this.search; 1437 } 1438 1439 public boolean hasSearchElement() { 1440 return this.search != null && !this.search.isEmpty(); 1441 } 1442 1443 public boolean hasSearch() { 1444 return this.search != null && !this.search.isEmpty(); 1445 } 1446 1447 /** 1448 * @param value {@link #search} (Whether the search syntax is supported,.). This 1449 * is the underlying object with id, value and extensions. The 1450 * accessor "getSearch" gives direct access to the value 1451 */ 1452 public CompartmentDefinition setSearchElement(BooleanType value) { 1453 this.search = value; 1454 return this; 1455 } 1456 1457 /** 1458 * @return Whether the search syntax is supported,. 1459 */ 1460 public boolean getSearch() { 1461 return this.search == null || this.search.isEmpty() ? false : this.search.getValue(); 1462 } 1463 1464 /** 1465 * @param value Whether the search syntax is supported,. 1466 */ 1467 public CompartmentDefinition setSearch(boolean value) { 1468 if (this.search == null) 1469 this.search = new BooleanType(); 1470 this.search.setValue(value); 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #resource} (Information about how a resource is related to the 1476 * compartment.) 1477 */ 1478 public List<CompartmentDefinitionResourceComponent> getResource() { 1479 if (this.resource == null) 1480 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1481 return this.resource; 1482 } 1483 1484 /** 1485 * @return Returns a reference to <code>this</code> for easy method chaining 1486 */ 1487 public CompartmentDefinition setResource(List<CompartmentDefinitionResourceComponent> theResource) { 1488 this.resource = theResource; 1489 return this; 1490 } 1491 1492 public boolean hasResource() { 1493 if (this.resource == null) 1494 return false; 1495 for (CompartmentDefinitionResourceComponent item : this.resource) 1496 if (!item.isEmpty()) 1497 return true; 1498 return false; 1499 } 1500 1501 public CompartmentDefinitionResourceComponent addResource() { // 3 1502 CompartmentDefinitionResourceComponent t = new CompartmentDefinitionResourceComponent(); 1503 if (this.resource == null) 1504 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1505 this.resource.add(t); 1506 return t; 1507 } 1508 1509 public CompartmentDefinition addResource(CompartmentDefinitionResourceComponent t) { // 3 1510 if (t == null) 1511 return this; 1512 if (this.resource == null) 1513 this.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1514 this.resource.add(t); 1515 return this; 1516 } 1517 1518 /** 1519 * @return The first repetition of repeating field {@link #resource}, creating 1520 * it if it does not already exist 1521 */ 1522 public CompartmentDefinitionResourceComponent getResourceFirstRep() { 1523 if (getResource().isEmpty()) { 1524 addResource(); 1525 } 1526 return getResource().get(0); 1527 } 1528 1529 protected void listChildren(List<Property> children) { 1530 super.listChildren(children); 1531 children.add(new Property("url", "uri", 1532 "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 1533 0, 1, url)); 1534 children.add(new Property("version", "string", 1535 "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1536 0, 1, version)); 1537 children.add(new Property("name", "string", 1538 "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1539 0, 1, name)); 1540 children.add(new Property("status", "code", 1541 "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 1542 children.add(new Property("experimental", "boolean", 1543 "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1544 0, 1, experimental)); 1545 children.add(new Property("date", "dateTime", 1546 "The date (and optionally time) when the compartment definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 1547 0, 1, date)); 1548 children.add(new Property("publisher", "string", 1549 "The name of the organization or individual that published the compartment definition.", 0, 1, publisher)); 1550 children.add(new Property("contact", "ContactDetail", 1551 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1552 java.lang.Integer.MAX_VALUE, contact)); 1553 children.add(new Property("description", "markdown", 1554 "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, 1555 description)); 1556 children.add(new Property("useContext", "UsageContext", 1557 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 1558 0, java.lang.Integer.MAX_VALUE, useContext)); 1559 children.add(new Property("purpose", "markdown", 1560 "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, 1561 purpose)); 1562 children.add(new Property("code", "code", "Which compartment this definition describes.", 0, 1, code)); 1563 children.add(new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search)); 1564 children.add(new Property("resource", "", "Information about how a resource is related to the compartment.", 0, 1565 java.lang.Integer.MAX_VALUE, resource)); 1566 } 1567 1568 @Override 1569 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1570 switch (_hash) { 1571 case 116079: 1572 /* url */ return new Property("url", "uri", 1573 "An absolute URI that is used to identify this compartment definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this compartment definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the compartment definition is stored on different servers.", 1574 0, 1, url); 1575 case 351608024: 1576 /* version */ return new Property("version", "string", 1577 "The identifier that is used to identify this version of the compartment definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the compartment definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 1578 0, 1, version); 1579 case 3373707: 1580 /* name */ return new Property("name", "string", 1581 "A natural language name identifying the compartment definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1582 0, 1, name); 1583 case -892481550: 1584 /* status */ return new Property("status", "code", 1585 "The status of this compartment definition. Enables tracking the life-cycle of the content.", 0, 1, status); 1586 case -404562712: 1587 /* experimental */ return new Property("experimental", "boolean", 1588 "A Boolean value to indicate that this compartment definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 1589 0, 1, experimental); 1590 case 3076014: 1591 /* date */ return new Property("date", "dateTime", 1592 "The date (and optionally time) when the compartment definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the compartment definition changes.", 1593 0, 1, date); 1594 case 1447404028: 1595 /* publisher */ return new Property("publisher", "string", 1596 "The name of the organization or individual that published the compartment definition.", 0, 1, publisher); 1597 case 951526432: 1598 /* contact */ return new Property("contact", "ContactDetail", 1599 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1600 java.lang.Integer.MAX_VALUE, contact); 1601 case -1724546052: 1602 /* description */ return new Property("description", "markdown", 1603 "A free text natural language description of the compartment definition from a consumer's perspective.", 0, 1, 1604 description); 1605 case -669707736: 1606 /* useContext */ return new Property("useContext", "UsageContext", 1607 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate compartment definition instances.", 1608 0, java.lang.Integer.MAX_VALUE, useContext); 1609 case -220463842: 1610 /* purpose */ return new Property("purpose", "markdown", 1611 "Explanation of why this compartment definition is needed and why it has been designed as it has.", 0, 1, 1612 purpose); 1613 case 3059181: 1614 /* code */ return new Property("code", "code", "Which compartment this definition describes.", 0, 1, code); 1615 case -906336856: 1616 /* search */ return new Property("search", "boolean", "Whether the search syntax is supported,.", 0, 1, search); 1617 case -341064690: 1618 /* resource */ return new Property("resource", "", 1619 "Information about how a resource is related to the compartment.", 0, java.lang.Integer.MAX_VALUE, resource); 1620 default: 1621 return super.getNamedProperty(_hash, _name, _checkValid); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1628 switch (hash) { 1629 case 116079: 1630 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 1631 case 351608024: 1632 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1633 case 3373707: 1634 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1635 case -892481550: 1636 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 1637 case -404562712: 1638 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 1639 case 3076014: 1640 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1641 case 1447404028: 1642 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 1643 case 951526432: 1644 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1645 case -1724546052: 1646 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 1647 case -669707736: 1648 /* useContext */ return this.useContext == null ? new Base[0] 1649 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1650 case -220463842: 1651 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 1652 case 3059181: 1653 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // Enumeration<CompartmentType> 1654 case -906336856: 1655 /* search */ return this.search == null ? new Base[0] : new Base[] { this.search }; // BooleanType 1656 case -341064690: 1657 /* resource */ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // CompartmentDefinitionResourceComponent 1658 default: 1659 return super.getProperty(hash, name, checkValid); 1660 } 1661 1662 } 1663 1664 @Override 1665 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1666 switch (hash) { 1667 case 116079: // url 1668 this.url = castToUri(value); // UriType 1669 return value; 1670 case 351608024: // version 1671 this.version = castToString(value); // StringType 1672 return value; 1673 case 3373707: // name 1674 this.name = castToString(value); // StringType 1675 return value; 1676 case -892481550: // status 1677 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1678 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1679 return value; 1680 case -404562712: // experimental 1681 this.experimental = castToBoolean(value); // BooleanType 1682 return value; 1683 case 3076014: // date 1684 this.date = castToDateTime(value); // DateTimeType 1685 return value; 1686 case 1447404028: // publisher 1687 this.publisher = castToString(value); // StringType 1688 return value; 1689 case 951526432: // contact 1690 this.getContact().add(castToContactDetail(value)); // ContactDetail 1691 return value; 1692 case -1724546052: // description 1693 this.description = castToMarkdown(value); // MarkdownType 1694 return value; 1695 case -669707736: // useContext 1696 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1697 return value; 1698 case -220463842: // purpose 1699 this.purpose = castToMarkdown(value); // MarkdownType 1700 return value; 1701 case 3059181: // code 1702 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1703 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1704 return value; 1705 case -906336856: // search 1706 this.search = castToBoolean(value); // BooleanType 1707 return value; 1708 case -341064690: // resource 1709 this.getResource().add((CompartmentDefinitionResourceComponent) value); // CompartmentDefinitionResourceComponent 1710 return value; 1711 default: 1712 return super.setProperty(hash, name, value); 1713 } 1714 1715 } 1716 1717 @Override 1718 public Base setProperty(String name, Base value) throws FHIRException { 1719 if (name.equals("url")) { 1720 this.url = castToUri(value); // UriType 1721 } else if (name.equals("version")) { 1722 this.version = castToString(value); // StringType 1723 } else if (name.equals("name")) { 1724 this.name = castToString(value); // StringType 1725 } else if (name.equals("status")) { 1726 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1727 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1728 } else if (name.equals("experimental")) { 1729 this.experimental = castToBoolean(value); // BooleanType 1730 } else if (name.equals("date")) { 1731 this.date = castToDateTime(value); // DateTimeType 1732 } else if (name.equals("publisher")) { 1733 this.publisher = castToString(value); // StringType 1734 } else if (name.equals("contact")) { 1735 this.getContact().add(castToContactDetail(value)); 1736 } else if (name.equals("description")) { 1737 this.description = castToMarkdown(value); // MarkdownType 1738 } else if (name.equals("useContext")) { 1739 this.getUseContext().add(castToUsageContext(value)); 1740 } else if (name.equals("purpose")) { 1741 this.purpose = castToMarkdown(value); // MarkdownType 1742 } else if (name.equals("code")) { 1743 value = new CompartmentTypeEnumFactory().fromType(castToCode(value)); 1744 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1745 } else if (name.equals("search")) { 1746 this.search = castToBoolean(value); // BooleanType 1747 } else if (name.equals("resource")) { 1748 this.getResource().add((CompartmentDefinitionResourceComponent) value); 1749 } else 1750 return super.setProperty(name, value); 1751 return value; 1752 } 1753 1754 @Override 1755 public void removeChild(String name, Base value) throws FHIRException { 1756 if (name.equals("url")) { 1757 this.url = null; 1758 } else if (name.equals("version")) { 1759 this.version = null; 1760 } else if (name.equals("name")) { 1761 this.name = null; 1762 } else if (name.equals("status")) { 1763 this.status = null; 1764 } else if (name.equals("experimental")) { 1765 this.experimental = null; 1766 } else if (name.equals("date")) { 1767 this.date = null; 1768 } else if (name.equals("publisher")) { 1769 this.publisher = null; 1770 } else if (name.equals("contact")) { 1771 this.getContact().remove(castToContactDetail(value)); 1772 } else if (name.equals("description")) { 1773 this.description = null; 1774 } else if (name.equals("useContext")) { 1775 this.getUseContext().remove(castToUsageContext(value)); 1776 } else if (name.equals("purpose")) { 1777 this.purpose = null; 1778 } else if (name.equals("code")) { 1779 this.code = null; 1780 } else if (name.equals("search")) { 1781 this.search = null; 1782 } else if (name.equals("resource")) { 1783 this.getResource().remove((CompartmentDefinitionResourceComponent) value); 1784 } else 1785 super.removeChild(name, value); 1786 1787 } 1788 1789 @Override 1790 public Base makeProperty(int hash, String name) throws FHIRException { 1791 switch (hash) { 1792 case 116079: 1793 return getUrlElement(); 1794 case 351608024: 1795 return getVersionElement(); 1796 case 3373707: 1797 return getNameElement(); 1798 case -892481550: 1799 return getStatusElement(); 1800 case -404562712: 1801 return getExperimentalElement(); 1802 case 3076014: 1803 return getDateElement(); 1804 case 1447404028: 1805 return getPublisherElement(); 1806 case 951526432: 1807 return addContact(); 1808 case -1724546052: 1809 return getDescriptionElement(); 1810 case -669707736: 1811 return addUseContext(); 1812 case -220463842: 1813 return getPurposeElement(); 1814 case 3059181: 1815 return getCodeElement(); 1816 case -906336856: 1817 return getSearchElement(); 1818 case -341064690: 1819 return addResource(); 1820 default: 1821 return super.makeProperty(hash, name); 1822 } 1823 1824 } 1825 1826 @Override 1827 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1828 switch (hash) { 1829 case 116079: 1830 /* url */ return new String[] { "uri" }; 1831 case 351608024: 1832 /* version */ return new String[] { "string" }; 1833 case 3373707: 1834 /* name */ return new String[] { "string" }; 1835 case -892481550: 1836 /* status */ return new String[] { "code" }; 1837 case -404562712: 1838 /* experimental */ return new String[] { "boolean" }; 1839 case 3076014: 1840 /* date */ return new String[] { "dateTime" }; 1841 case 1447404028: 1842 /* publisher */ return new String[] { "string" }; 1843 case 951526432: 1844 /* contact */ return new String[] { "ContactDetail" }; 1845 case -1724546052: 1846 /* description */ return new String[] { "markdown" }; 1847 case -669707736: 1848 /* useContext */ return new String[] { "UsageContext" }; 1849 case -220463842: 1850 /* purpose */ return new String[] { "markdown" }; 1851 case 3059181: 1852 /* code */ return new String[] { "code" }; 1853 case -906336856: 1854 /* search */ return new String[] { "boolean" }; 1855 case -341064690: 1856 /* resource */ return new String[] {}; 1857 default: 1858 return super.getTypesForProperty(hash, name); 1859 } 1860 1861 } 1862 1863 @Override 1864 public Base addChild(String name) throws FHIRException { 1865 if (name.equals("url")) { 1866 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.url"); 1867 } else if (name.equals("version")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.version"); 1869 } else if (name.equals("name")) { 1870 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.name"); 1871 } else if (name.equals("status")) { 1872 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.status"); 1873 } else if (name.equals("experimental")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.experimental"); 1875 } else if (name.equals("date")) { 1876 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.date"); 1877 } else if (name.equals("publisher")) { 1878 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.publisher"); 1879 } else if (name.equals("contact")) { 1880 return addContact(); 1881 } else if (name.equals("description")) { 1882 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.description"); 1883 } else if (name.equals("useContext")) { 1884 return addUseContext(); 1885 } else if (name.equals("purpose")) { 1886 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.purpose"); 1887 } else if (name.equals("code")) { 1888 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.code"); 1889 } else if (name.equals("search")) { 1890 throw new FHIRException("Cannot call addChild on a singleton property CompartmentDefinition.search"); 1891 } else if (name.equals("resource")) { 1892 return addResource(); 1893 } else 1894 return super.addChild(name); 1895 } 1896 1897 public String fhirType() { 1898 return "CompartmentDefinition"; 1899 1900 } 1901 1902 public CompartmentDefinition copy() { 1903 CompartmentDefinition dst = new CompartmentDefinition(); 1904 copyValues(dst); 1905 return dst; 1906 } 1907 1908 public void copyValues(CompartmentDefinition dst) { 1909 super.copyValues(dst); 1910 dst.url = url == null ? null : url.copy(); 1911 dst.version = version == null ? null : version.copy(); 1912 dst.name = name == null ? null : name.copy(); 1913 dst.status = status == null ? null : status.copy(); 1914 dst.experimental = experimental == null ? null : experimental.copy(); 1915 dst.date = date == null ? null : date.copy(); 1916 dst.publisher = publisher == null ? null : publisher.copy(); 1917 if (contact != null) { 1918 dst.contact = new ArrayList<ContactDetail>(); 1919 for (ContactDetail i : contact) 1920 dst.contact.add(i.copy()); 1921 } 1922 ; 1923 dst.description = description == null ? null : description.copy(); 1924 if (useContext != null) { 1925 dst.useContext = new ArrayList<UsageContext>(); 1926 for (UsageContext i : useContext) 1927 dst.useContext.add(i.copy()); 1928 } 1929 ; 1930 dst.purpose = purpose == null ? null : purpose.copy(); 1931 dst.code = code == null ? null : code.copy(); 1932 dst.search = search == null ? null : search.copy(); 1933 if (resource != null) { 1934 dst.resource = new ArrayList<CompartmentDefinitionResourceComponent>(); 1935 for (CompartmentDefinitionResourceComponent i : resource) 1936 dst.resource.add(i.copy()); 1937 } 1938 ; 1939 } 1940 1941 protected CompartmentDefinition typedCopy() { 1942 return copy(); 1943 } 1944 1945 @Override 1946 public boolean equalsDeep(Base other_) { 1947 if (!super.equalsDeep(other_)) 1948 return false; 1949 if (!(other_ instanceof CompartmentDefinition)) 1950 return false; 1951 CompartmentDefinition o = (CompartmentDefinition) other_; 1952 return compareDeep(purpose, o.purpose, true) && compareDeep(code, o.code, true) 1953 && compareDeep(search, o.search, true) && compareDeep(resource, o.resource, true); 1954 } 1955 1956 @Override 1957 public boolean equalsShallow(Base other_) { 1958 if (!super.equalsShallow(other_)) 1959 return false; 1960 if (!(other_ instanceof CompartmentDefinition)) 1961 return false; 1962 CompartmentDefinition o = (CompartmentDefinition) other_; 1963 return compareValues(purpose, o.purpose, true) && compareValues(code, o.code, true) 1964 && compareValues(search, o.search, true); 1965 } 1966 1967 public boolean isEmpty() { 1968 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, code, search, resource); 1969 } 1970 1971 @Override 1972 public ResourceType getResourceType() { 1973 return ResourceType.CompartmentDefinition; 1974 } 1975 1976 /** 1977 * Search parameter: <b>date</b> 1978 * <p> 1979 * Description: <b>The compartment definition publication date</b><br> 1980 * Type: <b>date</b><br> 1981 * Path: <b>CompartmentDefinition.date</b><br> 1982 * </p> 1983 */ 1984 @SearchParamDefinition(name = "date", path = "CompartmentDefinition.date", description = "The compartment definition publication date", type = "date") 1985 public static final String SP_DATE = "date"; 1986 /** 1987 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1988 * <p> 1989 * Description: <b>The compartment definition publication date</b><br> 1990 * Type: <b>date</b><br> 1991 * Path: <b>CompartmentDefinition.date</b><br> 1992 * </p> 1993 */ 1994 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1995 SP_DATE); 1996 1997 /** 1998 * Search parameter: <b>code</b> 1999 * <p> 2000 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | 2001 * Device</b><br> 2002 * Type: <b>token</b><br> 2003 * Path: <b>CompartmentDefinition.code</b><br> 2004 * </p> 2005 */ 2006 @SearchParamDefinition(name = "code", path = "CompartmentDefinition.code", description = "Patient | Encounter | RelatedPerson | Practitioner | Device", type = "token") 2007 public static final String SP_CODE = "code"; 2008 /** 2009 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2010 * <p> 2011 * Description: <b>Patient | Encounter | RelatedPerson | Practitioner | 2012 * Device</b><br> 2013 * Type: <b>token</b><br> 2014 * Path: <b>CompartmentDefinition.code</b><br> 2015 * </p> 2016 */ 2017 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2018 SP_CODE); 2019 2020 /** 2021 * Search parameter: <b>context-type-value</b> 2022 * <p> 2023 * Description: <b>A use context type and value assigned to the compartment 2024 * definition</b><br> 2025 * Type: <b>composite</b><br> 2026 * Path: <b></b><br> 2027 * </p> 2028 */ 2029 @SearchParamDefinition(name = "context-type-value", path = "CompartmentDefinition.useContext", description = "A use context type and value assigned to the compartment definition", type = "composite", compositeOf = { 2030 "context-type", "context" }) 2031 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2032 /** 2033 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2034 * <p> 2035 * Description: <b>A use context type and value assigned to the compartment 2036 * definition</b><br> 2037 * Type: <b>composite</b><br> 2038 * Path: <b></b><br> 2039 * </p> 2040 */ 2041 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2042 SP_CONTEXT_TYPE_VALUE); 2043 2044 /** 2045 * Search parameter: <b>resource</b> 2046 * <p> 2047 * Description: <b>Name of resource type</b><br> 2048 * Type: <b>token</b><br> 2049 * Path: <b>CompartmentDefinition.resource.code</b><br> 2050 * </p> 2051 */ 2052 @SearchParamDefinition(name = "resource", path = "CompartmentDefinition.resource.code", description = "Name of resource type", type = "token") 2053 public static final String SP_RESOURCE = "resource"; 2054 /** 2055 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 2056 * <p> 2057 * Description: <b>Name of resource type</b><br> 2058 * Type: <b>token</b><br> 2059 * Path: <b>CompartmentDefinition.resource.code</b><br> 2060 * </p> 2061 */ 2062 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2063 SP_RESOURCE); 2064 2065 /** 2066 * Search parameter: <b>description</b> 2067 * <p> 2068 * Description: <b>The description of the compartment definition</b><br> 2069 * Type: <b>string</b><br> 2070 * Path: <b>CompartmentDefinition.description</b><br> 2071 * </p> 2072 */ 2073 @SearchParamDefinition(name = "description", path = "CompartmentDefinition.description", description = "The description of the compartment definition", type = "string") 2074 public static final String SP_DESCRIPTION = "description"; 2075 /** 2076 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2077 * <p> 2078 * Description: <b>The description of the compartment definition</b><br> 2079 * Type: <b>string</b><br> 2080 * Path: <b>CompartmentDefinition.description</b><br> 2081 * </p> 2082 */ 2083 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2084 SP_DESCRIPTION); 2085 2086 /** 2087 * Search parameter: <b>context-type</b> 2088 * <p> 2089 * Description: <b>A type of use context assigned to the compartment 2090 * definition</b><br> 2091 * Type: <b>token</b><br> 2092 * Path: <b>CompartmentDefinition.useContext.code</b><br> 2093 * </p> 2094 */ 2095 @SearchParamDefinition(name = "context-type", path = "CompartmentDefinition.useContext.code", description = "A type of use context assigned to the compartment definition", type = "token") 2096 public static final String SP_CONTEXT_TYPE = "context-type"; 2097 /** 2098 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2099 * <p> 2100 * Description: <b>A type of use context assigned to the compartment 2101 * definition</b><br> 2102 * Type: <b>token</b><br> 2103 * Path: <b>CompartmentDefinition.useContext.code</b><br> 2104 * </p> 2105 */ 2106 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2107 SP_CONTEXT_TYPE); 2108 2109 /** 2110 * Search parameter: <b>version</b> 2111 * <p> 2112 * Description: <b>The business version of the compartment definition</b><br> 2113 * Type: <b>token</b><br> 2114 * Path: <b>CompartmentDefinition.version</b><br> 2115 * </p> 2116 */ 2117 @SearchParamDefinition(name = "version", path = "CompartmentDefinition.version", description = "The business version of the compartment definition", type = "token") 2118 public static final String SP_VERSION = "version"; 2119 /** 2120 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2121 * <p> 2122 * Description: <b>The business version of the compartment definition</b><br> 2123 * Type: <b>token</b><br> 2124 * Path: <b>CompartmentDefinition.version</b><br> 2125 * </p> 2126 */ 2127 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2128 SP_VERSION); 2129 2130 /** 2131 * Search parameter: <b>url</b> 2132 * <p> 2133 * Description: <b>The uri that identifies the compartment definition</b><br> 2134 * Type: <b>uri</b><br> 2135 * Path: <b>CompartmentDefinition.url</b><br> 2136 * </p> 2137 */ 2138 @SearchParamDefinition(name = "url", path = "CompartmentDefinition.url", description = "The uri that identifies the compartment definition", type = "uri") 2139 public static final String SP_URL = "url"; 2140 /** 2141 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2142 * <p> 2143 * Description: <b>The uri that identifies the compartment definition</b><br> 2144 * Type: <b>uri</b><br> 2145 * Path: <b>CompartmentDefinition.url</b><br> 2146 * </p> 2147 */ 2148 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2149 2150 /** 2151 * Search parameter: <b>context-quantity</b> 2152 * <p> 2153 * Description: <b>A quantity- or range-valued use context assigned to the 2154 * compartment definition</b><br> 2155 * Type: <b>quantity</b><br> 2156 * Path: <b>CompartmentDefinition.useContext.valueQuantity, 2157 * CompartmentDefinition.useContext.valueRange</b><br> 2158 * </p> 2159 */ 2160 @SearchParamDefinition(name = "context-quantity", path = "(CompartmentDefinition.useContext.value as Quantity) | (CompartmentDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the compartment definition", type = "quantity") 2161 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2162 /** 2163 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2164 * <p> 2165 * Description: <b>A quantity- or range-valued use context assigned to the 2166 * compartment definition</b><br> 2167 * Type: <b>quantity</b><br> 2168 * Path: <b>CompartmentDefinition.useContext.valueQuantity, 2169 * CompartmentDefinition.useContext.valueRange</b><br> 2170 * </p> 2171 */ 2172 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2173 SP_CONTEXT_QUANTITY); 2174 2175 /** 2176 * Search parameter: <b>name</b> 2177 * <p> 2178 * Description: <b>Computationally friendly name of the compartment 2179 * definition</b><br> 2180 * Type: <b>string</b><br> 2181 * Path: <b>CompartmentDefinition.name</b><br> 2182 * </p> 2183 */ 2184 @SearchParamDefinition(name = "name", path = "CompartmentDefinition.name", description = "Computationally friendly name of the compartment definition", type = "string") 2185 public static final String SP_NAME = "name"; 2186 /** 2187 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2188 * <p> 2189 * Description: <b>Computationally friendly name of the compartment 2190 * definition</b><br> 2191 * Type: <b>string</b><br> 2192 * Path: <b>CompartmentDefinition.name</b><br> 2193 * </p> 2194 */ 2195 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2196 SP_NAME); 2197 2198 /** 2199 * Search parameter: <b>context</b> 2200 * <p> 2201 * Description: <b>A use context assigned to the compartment definition</b><br> 2202 * Type: <b>token</b><br> 2203 * Path: <b>CompartmentDefinition.useContext.valueCodeableConcept</b><br> 2204 * </p> 2205 */ 2206 @SearchParamDefinition(name = "context", path = "(CompartmentDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the compartment definition", type = "token") 2207 public static final String SP_CONTEXT = "context"; 2208 /** 2209 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2210 * <p> 2211 * Description: <b>A use context assigned to the compartment definition</b><br> 2212 * Type: <b>token</b><br> 2213 * Path: <b>CompartmentDefinition.useContext.valueCodeableConcept</b><br> 2214 * </p> 2215 */ 2216 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2217 SP_CONTEXT); 2218 2219 /** 2220 * Search parameter: <b>publisher</b> 2221 * <p> 2222 * Description: <b>Name of the publisher of the compartment definition</b><br> 2223 * Type: <b>string</b><br> 2224 * Path: <b>CompartmentDefinition.publisher</b><br> 2225 * </p> 2226 */ 2227 @SearchParamDefinition(name = "publisher", path = "CompartmentDefinition.publisher", description = "Name of the publisher of the compartment definition", type = "string") 2228 public static final String SP_PUBLISHER = "publisher"; 2229 /** 2230 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2231 * <p> 2232 * Description: <b>Name of the publisher of the compartment definition</b><br> 2233 * Type: <b>string</b><br> 2234 * Path: <b>CompartmentDefinition.publisher</b><br> 2235 * </p> 2236 */ 2237 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 2238 SP_PUBLISHER); 2239 2240 /** 2241 * Search parameter: <b>context-type-quantity</b> 2242 * <p> 2243 * Description: <b>A use context type and quantity- or range-based value 2244 * assigned to the compartment definition</b><br> 2245 * Type: <b>composite</b><br> 2246 * Path: <b></b><br> 2247 * </p> 2248 */ 2249 @SearchParamDefinition(name = "context-type-quantity", path = "CompartmentDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the compartment definition", type = "composite", compositeOf = { 2250 "context-type", "context-quantity" }) 2251 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2252 /** 2253 * <b>Fluent Client</b> search parameter constant for 2254 * <b>context-type-quantity</b> 2255 * <p> 2256 * Description: <b>A use context type and quantity- or range-based value 2257 * assigned to the compartment definition</b><br> 2258 * Type: <b>composite</b><br> 2259 * Path: <b></b><br> 2260 * </p> 2261 */ 2262 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 2263 SP_CONTEXT_TYPE_QUANTITY); 2264 2265 /** 2266 * Search parameter: <b>status</b> 2267 * <p> 2268 * Description: <b>The current status of the compartment definition</b><br> 2269 * Type: <b>token</b><br> 2270 * Path: <b>CompartmentDefinition.status</b><br> 2271 * </p> 2272 */ 2273 @SearchParamDefinition(name = "status", path = "CompartmentDefinition.status", description = "The current status of the compartment definition", type = "token") 2274 public static final String SP_STATUS = "status"; 2275 /** 2276 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2277 * <p> 2278 * Description: <b>The current status of the compartment definition</b><br> 2279 * Type: <b>token</b><br> 2280 * Path: <b>CompartmentDefinition.status</b><br> 2281 * </p> 2282 */ 2283 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2284 SP_STATUS); 2285 2286}