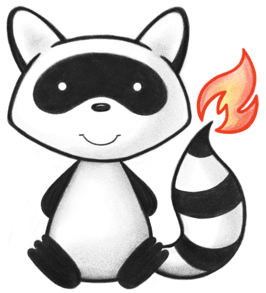
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A clinical condition, problem, diagnosis, or other event, situation, issue, 048 * or clinical concept that has risen to a level of concern. 049 */ 050@ResourceDef(name = "Condition", profile = "http://hl7.org/fhir/StructureDefinition/Condition") 051public class Condition extends DomainResource { 052 053 @Block() 054 public static class ConditionStageComponent extends BackboneElement implements IBaseBackboneElement { 055 /** 056 * A simple summary of the stage such as "Stage 3". The determination of the 057 * stage is disease-specific. 058 */ 059 @Child(name = "summary", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "Simple summary (disease specific)", formalDefinition = "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.") 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-stage") 063 protected CodeableConcept summary; 064 065 /** 066 * Reference to a formal record of the evidence on which the staging assessment 067 * is based. 068 */ 069 @Child(name = "assessment", type = { ClinicalImpression.class, DiagnosticReport.class, 070 Observation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 071 @Description(shortDefinition = "Formal record of assessment", formalDefinition = "Reference to a formal record of the evidence on which the staging assessment is based.") 072 protected List<Reference> assessment; 073 /** 074 * The actual objects that are the target of the reference (Reference to a 075 * formal record of the evidence on which the staging assessment is based.) 076 */ 077 protected List<Resource> assessmentTarget; 078 079 /** 080 * The kind of staging, such as pathological or clinical staging. 081 */ 082 @Child(name = "type", type = { 083 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 084 @Description(shortDefinition = "Kind of staging", formalDefinition = "The kind of staging, such as pathological or clinical staging.") 085 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-stage-type") 086 protected CodeableConcept type; 087 088 private static final long serialVersionUID = 668627986L; 089 090 /** 091 * Constructor 092 */ 093 public ConditionStageComponent() { 094 super(); 095 } 096 097 /** 098 * @return {@link #summary} (A simple summary of the stage such as "Stage 3". 099 * The determination of the stage is disease-specific.) 100 */ 101 public CodeableConcept getSummary() { 102 if (this.summary == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create ConditionStageComponent.summary"); 105 else if (Configuration.doAutoCreate()) 106 this.summary = new CodeableConcept(); // cc 107 return this.summary; 108 } 109 110 public boolean hasSummary() { 111 return this.summary != null && !this.summary.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #summary} (A simple summary of the stage such as "Stage 116 * 3". The determination of the stage is disease-specific.) 117 */ 118 public ConditionStageComponent setSummary(CodeableConcept value) { 119 this.summary = value; 120 return this; 121 } 122 123 /** 124 * @return {@link #assessment} (Reference to a formal record of the evidence on 125 * which the staging assessment is based.) 126 */ 127 public List<Reference> getAssessment() { 128 if (this.assessment == null) 129 this.assessment = new ArrayList<Reference>(); 130 return this.assessment; 131 } 132 133 /** 134 * @return Returns a reference to <code>this</code> for easy method chaining 135 */ 136 public ConditionStageComponent setAssessment(List<Reference> theAssessment) { 137 this.assessment = theAssessment; 138 return this; 139 } 140 141 public boolean hasAssessment() { 142 if (this.assessment == null) 143 return false; 144 for (Reference item : this.assessment) 145 if (!item.isEmpty()) 146 return true; 147 return false; 148 } 149 150 public Reference addAssessment() { // 3 151 Reference t = new Reference(); 152 if (this.assessment == null) 153 this.assessment = new ArrayList<Reference>(); 154 this.assessment.add(t); 155 return t; 156 } 157 158 public ConditionStageComponent addAssessment(Reference t) { // 3 159 if (t == null) 160 return this; 161 if (this.assessment == null) 162 this.assessment = new ArrayList<Reference>(); 163 this.assessment.add(t); 164 return this; 165 } 166 167 /** 168 * @return The first repetition of repeating field {@link #assessment}, creating 169 * it if it does not already exist 170 */ 171 public Reference getAssessmentFirstRep() { 172 if (getAssessment().isEmpty()) { 173 addAssessment(); 174 } 175 return getAssessment().get(0); 176 } 177 178 /** 179 * @deprecated Use Reference#setResource(IBaseResource) instead 180 */ 181 @Deprecated 182 public List<Resource> getAssessmentTarget() { 183 if (this.assessmentTarget == null) 184 this.assessmentTarget = new ArrayList<Resource>(); 185 return this.assessmentTarget; 186 } 187 188 /** 189 * @return {@link #type} (The kind of staging, such as pathological or clinical 190 * staging.) 191 */ 192 public CodeableConcept getType() { 193 if (this.type == null) 194 if (Configuration.errorOnAutoCreate()) 195 throw new Error("Attempt to auto-create ConditionStageComponent.type"); 196 else if (Configuration.doAutoCreate()) 197 this.type = new CodeableConcept(); // cc 198 return this.type; 199 } 200 201 public boolean hasType() { 202 return this.type != null && !this.type.isEmpty(); 203 } 204 205 /** 206 * @param value {@link #type} (The kind of staging, such as pathological or 207 * clinical staging.) 208 */ 209 public ConditionStageComponent setType(CodeableConcept value) { 210 this.type = value; 211 return this; 212 } 213 214 protected void listChildren(List<Property> children) { 215 super.listChildren(children); 216 children.add(new Property("summary", "CodeableConcept", 217 "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.", 0, 218 1, summary)); 219 children.add(new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", 220 "Reference to a formal record of the evidence on which the staging assessment is based.", 0, 221 java.lang.Integer.MAX_VALUE, assessment)); 222 children.add(new Property("type", "CodeableConcept", 223 "The kind of staging, such as pathological or clinical staging.", 0, 1, type)); 224 } 225 226 @Override 227 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 228 switch (_hash) { 229 case -1857640538: 230 /* summary */ return new Property("summary", "CodeableConcept", 231 "A simple summary of the stage such as \"Stage 3\". The determination of the stage is disease-specific.", 0, 232 1, summary); 233 case 2119382722: 234 /* assessment */ return new Property("assessment", "Reference(ClinicalImpression|DiagnosticReport|Observation)", 235 "Reference to a formal record of the evidence on which the staging assessment is based.", 0, 236 java.lang.Integer.MAX_VALUE, assessment); 237 case 3575610: 238 /* type */ return new Property("type", "CodeableConcept", 239 "The kind of staging, such as pathological or clinical staging.", 0, 1, type); 240 default: 241 return super.getNamedProperty(_hash, _name, _checkValid); 242 } 243 244 } 245 246 @Override 247 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 248 switch (hash) { 249 case -1857640538: 250 /* summary */ return this.summary == null ? new Base[0] : new Base[] { this.summary }; // CodeableConcept 251 case 2119382722: 252 /* assessment */ return this.assessment == null ? new Base[0] 253 : this.assessment.toArray(new Base[this.assessment.size()]); // Reference 254 case 3575610: 255 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 256 default: 257 return super.getProperty(hash, name, checkValid); 258 } 259 260 } 261 262 @Override 263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 264 switch (hash) { 265 case -1857640538: // summary 266 this.summary = castToCodeableConcept(value); // CodeableConcept 267 return value; 268 case 2119382722: // assessment 269 this.getAssessment().add(castToReference(value)); // Reference 270 return value; 271 case 3575610: // type 272 this.type = castToCodeableConcept(value); // CodeableConcept 273 return value; 274 default: 275 return super.setProperty(hash, name, value); 276 } 277 278 } 279 280 @Override 281 public Base setProperty(String name, Base value) throws FHIRException { 282 if (name.equals("summary")) { 283 this.summary = castToCodeableConcept(value); // CodeableConcept 284 } else if (name.equals("assessment")) { 285 this.getAssessment().add(castToReference(value)); 286 } else if (name.equals("type")) { 287 this.type = castToCodeableConcept(value); // CodeableConcept 288 } else 289 return super.setProperty(name, value); 290 return value; 291 } 292 293 @Override 294 public void removeChild(String name, Base value) throws FHIRException { 295 if (name.equals("summary")) { 296 this.summary = null; 297 } else if (name.equals("assessment")) { 298 this.getAssessment().remove(castToReference(value)); 299 } else if (name.equals("type")) { 300 this.type = null; 301 } else 302 super.removeChild(name, value); 303 304 } 305 306 @Override 307 public Base makeProperty(int hash, String name) throws FHIRException { 308 switch (hash) { 309 case -1857640538: 310 return getSummary(); 311 case 2119382722: 312 return addAssessment(); 313 case 3575610: 314 return getType(); 315 default: 316 return super.makeProperty(hash, name); 317 } 318 319 } 320 321 @Override 322 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 323 switch (hash) { 324 case -1857640538: 325 /* summary */ return new String[] { "CodeableConcept" }; 326 case 2119382722: 327 /* assessment */ return new String[] { "Reference" }; 328 case 3575610: 329 /* type */ return new String[] { "CodeableConcept" }; 330 default: 331 return super.getTypesForProperty(hash, name); 332 } 333 334 } 335 336 @Override 337 public Base addChild(String name) throws FHIRException { 338 if (name.equals("summary")) { 339 this.summary = new CodeableConcept(); 340 return this.summary; 341 } else if (name.equals("assessment")) { 342 return addAssessment(); 343 } else if (name.equals("type")) { 344 this.type = new CodeableConcept(); 345 return this.type; 346 } else 347 return super.addChild(name); 348 } 349 350 public ConditionStageComponent copy() { 351 ConditionStageComponent dst = new ConditionStageComponent(); 352 copyValues(dst); 353 return dst; 354 } 355 356 public void copyValues(ConditionStageComponent dst) { 357 super.copyValues(dst); 358 dst.summary = summary == null ? null : summary.copy(); 359 if (assessment != null) { 360 dst.assessment = new ArrayList<Reference>(); 361 for (Reference i : assessment) 362 dst.assessment.add(i.copy()); 363 } 364 ; 365 dst.type = type == null ? null : type.copy(); 366 } 367 368 @Override 369 public boolean equalsDeep(Base other_) { 370 if (!super.equalsDeep(other_)) 371 return false; 372 if (!(other_ instanceof ConditionStageComponent)) 373 return false; 374 ConditionStageComponent o = (ConditionStageComponent) other_; 375 return compareDeep(summary, o.summary, true) && compareDeep(assessment, o.assessment, true) 376 && compareDeep(type, o.type, true); 377 } 378 379 @Override 380 public boolean equalsShallow(Base other_) { 381 if (!super.equalsShallow(other_)) 382 return false; 383 if (!(other_ instanceof ConditionStageComponent)) 384 return false; 385 ConditionStageComponent o = (ConditionStageComponent) other_; 386 return true; 387 } 388 389 public boolean isEmpty() { 390 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(summary, assessment, type); 391 } 392 393 public String fhirType() { 394 return "Condition.stage"; 395 396 } 397 398 } 399 400 @Block() 401 public static class ConditionEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 402 /** 403 * A manifestation or symptom that led to the recording of this condition. 404 */ 405 @Child(name = "code", type = { 406 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 407 @Description(shortDefinition = "Manifestation/symptom", formalDefinition = "A manifestation or symptom that led to the recording of this condition.") 408 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 409 protected List<CodeableConcept> code; 410 411 /** 412 * Links to other relevant information, including pathology reports. 413 */ 414 @Child(name = "detail", type = { 415 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 416 @Description(shortDefinition = "Supporting information found elsewhere", formalDefinition = "Links to other relevant information, including pathology reports.") 417 protected List<Reference> detail; 418 /** 419 * The actual objects that are the target of the reference (Links to other 420 * relevant information, including pathology reports.) 421 */ 422 protected List<Resource> detailTarget; 423 424 private static final long serialVersionUID = 1135831276L; 425 426 /** 427 * Constructor 428 */ 429 public ConditionEvidenceComponent() { 430 super(); 431 } 432 433 /** 434 * @return {@link #code} (A manifestation or symptom that led to the recording 435 * of this condition.) 436 */ 437 public List<CodeableConcept> getCode() { 438 if (this.code == null) 439 this.code = new ArrayList<CodeableConcept>(); 440 return this.code; 441 } 442 443 /** 444 * @return Returns a reference to <code>this</code> for easy method chaining 445 */ 446 public ConditionEvidenceComponent setCode(List<CodeableConcept> theCode) { 447 this.code = theCode; 448 return this; 449 } 450 451 public boolean hasCode() { 452 if (this.code == null) 453 return false; 454 for (CodeableConcept item : this.code) 455 if (!item.isEmpty()) 456 return true; 457 return false; 458 } 459 460 public CodeableConcept addCode() { // 3 461 CodeableConcept t = new CodeableConcept(); 462 if (this.code == null) 463 this.code = new ArrayList<CodeableConcept>(); 464 this.code.add(t); 465 return t; 466 } 467 468 public ConditionEvidenceComponent addCode(CodeableConcept t) { // 3 469 if (t == null) 470 return this; 471 if (this.code == null) 472 this.code = new ArrayList<CodeableConcept>(); 473 this.code.add(t); 474 return this; 475 } 476 477 /** 478 * @return The first repetition of repeating field {@link #code}, creating it if 479 * it does not already exist 480 */ 481 public CodeableConcept getCodeFirstRep() { 482 if (getCode().isEmpty()) { 483 addCode(); 484 } 485 return getCode().get(0); 486 } 487 488 /** 489 * @return {@link #detail} (Links to other relevant information, including 490 * pathology reports.) 491 */ 492 public List<Reference> getDetail() { 493 if (this.detail == null) 494 this.detail = new ArrayList<Reference>(); 495 return this.detail; 496 } 497 498 /** 499 * @return Returns a reference to <code>this</code> for easy method chaining 500 */ 501 public ConditionEvidenceComponent setDetail(List<Reference> theDetail) { 502 this.detail = theDetail; 503 return this; 504 } 505 506 public boolean hasDetail() { 507 if (this.detail == null) 508 return false; 509 for (Reference item : this.detail) 510 if (!item.isEmpty()) 511 return true; 512 return false; 513 } 514 515 public Reference addDetail() { // 3 516 Reference t = new Reference(); 517 if (this.detail == null) 518 this.detail = new ArrayList<Reference>(); 519 this.detail.add(t); 520 return t; 521 } 522 523 public ConditionEvidenceComponent addDetail(Reference t) { // 3 524 if (t == null) 525 return this; 526 if (this.detail == null) 527 this.detail = new ArrayList<Reference>(); 528 this.detail.add(t); 529 return this; 530 } 531 532 /** 533 * @return The first repetition of repeating field {@link #detail}, creating it 534 * if it does not already exist 535 */ 536 public Reference getDetailFirstRep() { 537 if (getDetail().isEmpty()) { 538 addDetail(); 539 } 540 return getDetail().get(0); 541 } 542 543 /** 544 * @deprecated Use Reference#setResource(IBaseResource) instead 545 */ 546 @Deprecated 547 public List<Resource> getDetailTarget() { 548 if (this.detailTarget == null) 549 this.detailTarget = new ArrayList<Resource>(); 550 return this.detailTarget; 551 } 552 553 protected void listChildren(List<Property> children) { 554 super.listChildren(children); 555 children.add(new Property("code", "CodeableConcept", 556 "A manifestation or symptom that led to the recording of this condition.", 0, java.lang.Integer.MAX_VALUE, 557 code)); 558 children.add(new Property("detail", "Reference(Any)", 559 "Links to other relevant information, including pathology reports.", 0, java.lang.Integer.MAX_VALUE, detail)); 560 } 561 562 @Override 563 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 564 switch (_hash) { 565 case 3059181: 566 /* code */ return new Property("code", "CodeableConcept", 567 "A manifestation or symptom that led to the recording of this condition.", 0, java.lang.Integer.MAX_VALUE, 568 code); 569 case -1335224239: 570 /* detail */ return new Property("detail", "Reference(Any)", 571 "Links to other relevant information, including pathology reports.", 0, java.lang.Integer.MAX_VALUE, 572 detail); 573 default: 574 return super.getNamedProperty(_hash, _name, _checkValid); 575 } 576 577 } 578 579 @Override 580 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 581 switch (hash) { 582 case 3059181: 583 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 584 case -1335224239: 585 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 586 default: 587 return super.getProperty(hash, name, checkValid); 588 } 589 590 } 591 592 @Override 593 public Base setProperty(int hash, String name, Base value) throws FHIRException { 594 switch (hash) { 595 case 3059181: // code 596 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 597 return value; 598 case -1335224239: // detail 599 this.getDetail().add(castToReference(value)); // Reference 600 return value; 601 default: 602 return super.setProperty(hash, name, value); 603 } 604 605 } 606 607 @Override 608 public Base setProperty(String name, Base value) throws FHIRException { 609 if (name.equals("code")) { 610 this.getCode().add(castToCodeableConcept(value)); 611 } else if (name.equals("detail")) { 612 this.getDetail().add(castToReference(value)); 613 } else 614 return super.setProperty(name, value); 615 return value; 616 } 617 618 @Override 619 public void removeChild(String name, Base value) throws FHIRException { 620 if (name.equals("code")) { 621 this.getCode().remove(castToCodeableConcept(value)); 622 } else if (name.equals("detail")) { 623 this.getDetail().remove(castToReference(value)); 624 } else 625 super.removeChild(name, value); 626 627 } 628 629 @Override 630 public Base makeProperty(int hash, String name) throws FHIRException { 631 switch (hash) { 632 case 3059181: 633 return addCode(); 634 case -1335224239: 635 return addDetail(); 636 default: 637 return super.makeProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 644 switch (hash) { 645 case 3059181: 646 /* code */ return new String[] { "CodeableConcept" }; 647 case -1335224239: 648 /* detail */ return new String[] { "Reference" }; 649 default: 650 return super.getTypesForProperty(hash, name); 651 } 652 653 } 654 655 @Override 656 public Base addChild(String name) throws FHIRException { 657 if (name.equals("code")) { 658 return addCode(); 659 } else if (name.equals("detail")) { 660 return addDetail(); 661 } else 662 return super.addChild(name); 663 } 664 665 public ConditionEvidenceComponent copy() { 666 ConditionEvidenceComponent dst = new ConditionEvidenceComponent(); 667 copyValues(dst); 668 return dst; 669 } 670 671 public void copyValues(ConditionEvidenceComponent dst) { 672 super.copyValues(dst); 673 if (code != null) { 674 dst.code = new ArrayList<CodeableConcept>(); 675 for (CodeableConcept i : code) 676 dst.code.add(i.copy()); 677 } 678 ; 679 if (detail != null) { 680 dst.detail = new ArrayList<Reference>(); 681 for (Reference i : detail) 682 dst.detail.add(i.copy()); 683 } 684 ; 685 } 686 687 @Override 688 public boolean equalsDeep(Base other_) { 689 if (!super.equalsDeep(other_)) 690 return false; 691 if (!(other_ instanceof ConditionEvidenceComponent)) 692 return false; 693 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other_; 694 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 695 } 696 697 @Override 698 public boolean equalsShallow(Base other_) { 699 if (!super.equalsShallow(other_)) 700 return false; 701 if (!(other_ instanceof ConditionEvidenceComponent)) 702 return false; 703 ConditionEvidenceComponent o = (ConditionEvidenceComponent) other_; 704 return true; 705 } 706 707 public boolean isEmpty() { 708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 709 } 710 711 public String fhirType() { 712 return "Condition.evidence"; 713 714 } 715 716 } 717 718 /** 719 * Business identifiers assigned to this condition by the performer or other 720 * systems which remain constant as the resource is updated and propagates from 721 * server to server. 722 */ 723 @Child(name = "identifier", type = { 724 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 725 @Description(shortDefinition = "External Ids for this condition", formalDefinition = "Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 726 protected List<Identifier> identifier; 727 728 /** 729 * The clinical status of the condition. 730 */ 731 @Child(name = "clinicalStatus", type = { 732 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 733 @Description(shortDefinition = "active | recurrence | relapse | inactive | remission | resolved", formalDefinition = "The clinical status of the condition.") 734 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-clinical") 735 protected CodeableConcept clinicalStatus; 736 737 /** 738 * The verification status to support the clinical status of the condition. 739 */ 740 @Child(name = "verificationStatus", type = { 741 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 742 @Description(shortDefinition = "unconfirmed | provisional | differential | confirmed | refuted | entered-in-error", formalDefinition = "The verification status to support the clinical status of the condition.") 743 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-ver-status") 744 protected CodeableConcept verificationStatus; 745 746 /** 747 * A category assigned to the condition. 748 */ 749 @Child(name = "category", type = { 750 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 751 @Description(shortDefinition = "problem-list-item | encounter-diagnosis", formalDefinition = "A category assigned to the condition.") 752 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-category") 753 protected List<CodeableConcept> category; 754 755 /** 756 * A subjective assessment of the severity of the condition as evaluated by the 757 * clinician. 758 */ 759 @Child(name = "severity", type = { 760 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 761 @Description(shortDefinition = "Subjective severity of condition", formalDefinition = "A subjective assessment of the severity of the condition as evaluated by the clinician.") 762 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-severity") 763 protected CodeableConcept severity; 764 765 /** 766 * Identification of the condition, problem or diagnosis. 767 */ 768 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 769 @Description(shortDefinition = "Identification of the condition, problem or diagnosis", formalDefinition = "Identification of the condition, problem or diagnosis.") 770 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 771 protected CodeableConcept code; 772 773 /** 774 * The anatomical location where this condition manifests itself. 775 */ 776 @Child(name = "bodySite", type = { 777 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 778 @Description(shortDefinition = "Anatomical location, if relevant", formalDefinition = "The anatomical location where this condition manifests itself.") 779 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 780 protected List<CodeableConcept> bodySite; 781 782 /** 783 * Indicates the patient or group who the condition record is associated with. 784 */ 785 @Child(name = "subject", type = { Patient.class, 786 Group.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 787 @Description(shortDefinition = "Who has the condition?", formalDefinition = "Indicates the patient or group who the condition record is associated with.") 788 protected Reference subject; 789 790 /** 791 * The actual object that is the target of the reference (Indicates the patient 792 * or group who the condition record is associated with.) 793 */ 794 protected Resource subjectTarget; 795 796 /** 797 * The Encounter during which this Condition was created or to which the 798 * creation of this record is tightly associated. 799 */ 800 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 801 @Description(shortDefinition = "Encounter created as part of", formalDefinition = "The Encounter during which this Condition was created or to which the creation of this record is tightly associated.") 802 protected Reference encounter; 803 804 /** 805 * The actual object that is the target of the reference (The Encounter during 806 * which this Condition was created or to which the creation of this record is 807 * tightly associated.) 808 */ 809 protected Encounter encounterTarget; 810 811 /** 812 * Estimated or actual date or date-time the condition began, in the opinion of 813 * the clinician. 814 */ 815 @Child(name = "onset", type = { DateTimeType.class, Age.class, Period.class, Range.class, 816 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 817 @Description(shortDefinition = "Estimated or actual date, date-time, or age", formalDefinition = "Estimated or actual date or date-time the condition began, in the opinion of the clinician.") 818 protected Type onset; 819 820 /** 821 * The date or estimated date that the condition resolved or went into 822 * remission. This is called "abatement" because of the many overloaded 823 * connotations associated with "remission" or "resolution" - Conditions are 824 * never really resolved, but they can abate. 825 */ 826 @Child(name = "abatement", type = { DateTimeType.class, Age.class, Period.class, Range.class, 827 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 828 @Description(shortDefinition = "When in resolution/remission", formalDefinition = "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.") 829 protected Type abatement; 830 831 /** 832 * The recordedDate represents when this particular Condition record was created 833 * in the system, which is often a system-generated date. 834 */ 835 @Child(name = "recordedDate", type = { 836 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 837 @Description(shortDefinition = "Date record was first recorded", formalDefinition = "The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.") 838 protected DateTimeType recordedDate; 839 840 /** 841 * Individual who recorded the record and takes responsibility for its content. 842 */ 843 @Child(name = "recorder", type = { Practitioner.class, PractitionerRole.class, Patient.class, 844 RelatedPerson.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 845 @Description(shortDefinition = "Who recorded the condition", formalDefinition = "Individual who recorded the record and takes responsibility for its content.") 846 protected Reference recorder; 847 848 /** 849 * The actual object that is the target of the reference (Individual who 850 * recorded the record and takes responsibility for its content.) 851 */ 852 protected Resource recorderTarget; 853 854 /** 855 * Individual who is making the condition statement. 856 */ 857 @Child(name = "asserter", type = { Practitioner.class, PractitionerRole.class, Patient.class, 858 RelatedPerson.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 859 @Description(shortDefinition = "Person who asserts this condition", formalDefinition = "Individual who is making the condition statement.") 860 protected Reference asserter; 861 862 /** 863 * The actual object that is the target of the reference (Individual who is 864 * making the condition statement.) 865 */ 866 protected Resource asserterTarget; 867 868 /** 869 * Clinical stage or grade of a condition. May include formal severity 870 * assessments. 871 */ 872 @Child(name = "stage", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 873 @Description(shortDefinition = "Stage/grade, usually assessed formally", formalDefinition = "Clinical stage or grade of a condition. May include formal severity assessments.") 874 protected List<ConditionStageComponent> stage; 875 876 /** 877 * Supporting evidence / manifestations that are the basis of the Condition's 878 * verification status, such as evidence that confirmed or refuted the 879 * condition. 880 */ 881 @Child(name = "evidence", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 882 @Description(shortDefinition = "Supporting evidence", formalDefinition = "Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.") 883 protected List<ConditionEvidenceComponent> evidence; 884 885 /** 886 * Additional information about the Condition. This is a general notes/comments 887 * entry for description of the Condition, its diagnosis and prognosis. 888 */ 889 @Child(name = "note", type = { 890 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 891 @Description(shortDefinition = "Additional information about the Condition", formalDefinition = "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.") 892 protected List<Annotation> note; 893 894 private static final long serialVersionUID = 186776568L; 895 896 /** 897 * Constructor 898 */ 899 public Condition() { 900 super(); 901 } 902 903 /** 904 * Constructor 905 */ 906 public Condition(Reference subject) { 907 super(); 908 this.subject = subject; 909 } 910 911 /** 912 * @return {@link #identifier} (Business identifiers assigned to this condition 913 * by the performer or other systems which remain constant as the 914 * resource is updated and propagates from server to server.) 915 */ 916 public List<Identifier> getIdentifier() { 917 if (this.identifier == null) 918 this.identifier = new ArrayList<Identifier>(); 919 return this.identifier; 920 } 921 922 /** 923 * @return Returns a reference to <code>this</code> for easy method chaining 924 */ 925 public Condition setIdentifier(List<Identifier> theIdentifier) { 926 this.identifier = theIdentifier; 927 return this; 928 } 929 930 public boolean hasIdentifier() { 931 if (this.identifier == null) 932 return false; 933 for (Identifier item : this.identifier) 934 if (!item.isEmpty()) 935 return true; 936 return false; 937 } 938 939 public Identifier addIdentifier() { // 3 940 Identifier t = new Identifier(); 941 if (this.identifier == null) 942 this.identifier = new ArrayList<Identifier>(); 943 this.identifier.add(t); 944 return t; 945 } 946 947 public Condition addIdentifier(Identifier t) { // 3 948 if (t == null) 949 return this; 950 if (this.identifier == null) 951 this.identifier = new ArrayList<Identifier>(); 952 this.identifier.add(t); 953 return this; 954 } 955 956 /** 957 * @return The first repetition of repeating field {@link #identifier}, creating 958 * it if it does not already exist 959 */ 960 public Identifier getIdentifierFirstRep() { 961 if (getIdentifier().isEmpty()) { 962 addIdentifier(); 963 } 964 return getIdentifier().get(0); 965 } 966 967 /** 968 * @return {@link #clinicalStatus} (The clinical status of the condition.) 969 */ 970 public CodeableConcept getClinicalStatus() { 971 if (this.clinicalStatus == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create Condition.clinicalStatus"); 974 else if (Configuration.doAutoCreate()) 975 this.clinicalStatus = new CodeableConcept(); // cc 976 return this.clinicalStatus; 977 } 978 979 public boolean hasClinicalStatus() { 980 return this.clinicalStatus != null && !this.clinicalStatus.isEmpty(); 981 } 982 983 /** 984 * @param value {@link #clinicalStatus} (The clinical status of the condition.) 985 */ 986 public Condition setClinicalStatus(CodeableConcept value) { 987 this.clinicalStatus = value; 988 return this; 989 } 990 991 /** 992 * @return {@link #verificationStatus} (The verification status to support the 993 * clinical status of the condition.) 994 */ 995 public CodeableConcept getVerificationStatus() { 996 if (this.verificationStatus == null) 997 if (Configuration.errorOnAutoCreate()) 998 throw new Error("Attempt to auto-create Condition.verificationStatus"); 999 else if (Configuration.doAutoCreate()) 1000 this.verificationStatus = new CodeableConcept(); // cc 1001 return this.verificationStatus; 1002 } 1003 1004 public boolean hasVerificationStatus() { 1005 return this.verificationStatus != null && !this.verificationStatus.isEmpty(); 1006 } 1007 1008 /** 1009 * @param value {@link #verificationStatus} (The verification status to support 1010 * the clinical status of the condition.) 1011 */ 1012 public Condition setVerificationStatus(CodeableConcept value) { 1013 this.verificationStatus = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #category} (A category assigned to the condition.) 1019 */ 1020 public List<CodeableConcept> getCategory() { 1021 if (this.category == null) 1022 this.category = new ArrayList<CodeableConcept>(); 1023 return this.category; 1024 } 1025 1026 /** 1027 * @return Returns a reference to <code>this</code> for easy method chaining 1028 */ 1029 public Condition setCategory(List<CodeableConcept> theCategory) { 1030 this.category = theCategory; 1031 return this; 1032 } 1033 1034 public boolean hasCategory() { 1035 if (this.category == null) 1036 return false; 1037 for (CodeableConcept item : this.category) 1038 if (!item.isEmpty()) 1039 return true; 1040 return false; 1041 } 1042 1043 public CodeableConcept addCategory() { // 3 1044 CodeableConcept t = new CodeableConcept(); 1045 if (this.category == null) 1046 this.category = new ArrayList<CodeableConcept>(); 1047 this.category.add(t); 1048 return t; 1049 } 1050 1051 public Condition addCategory(CodeableConcept t) { // 3 1052 if (t == null) 1053 return this; 1054 if (this.category == null) 1055 this.category = new ArrayList<CodeableConcept>(); 1056 this.category.add(t); 1057 return this; 1058 } 1059 1060 /** 1061 * @return The first repetition of repeating field {@link #category}, creating 1062 * it if it does not already exist 1063 */ 1064 public CodeableConcept getCategoryFirstRep() { 1065 if (getCategory().isEmpty()) { 1066 addCategory(); 1067 } 1068 return getCategory().get(0); 1069 } 1070 1071 /** 1072 * @return {@link #severity} (A subjective assessment of the severity of the 1073 * condition as evaluated by the clinician.) 1074 */ 1075 public CodeableConcept getSeverity() { 1076 if (this.severity == null) 1077 if (Configuration.errorOnAutoCreate()) 1078 throw new Error("Attempt to auto-create Condition.severity"); 1079 else if (Configuration.doAutoCreate()) 1080 this.severity = new CodeableConcept(); // cc 1081 return this.severity; 1082 } 1083 1084 public boolean hasSeverity() { 1085 return this.severity != null && !this.severity.isEmpty(); 1086 } 1087 1088 /** 1089 * @param value {@link #severity} (A subjective assessment of the severity of 1090 * the condition as evaluated by the clinician.) 1091 */ 1092 public Condition setSeverity(CodeableConcept value) { 1093 this.severity = value; 1094 return this; 1095 } 1096 1097 /** 1098 * @return {@link #code} (Identification of the condition, problem or 1099 * diagnosis.) 1100 */ 1101 public CodeableConcept getCode() { 1102 if (this.code == null) 1103 if (Configuration.errorOnAutoCreate()) 1104 throw new Error("Attempt to auto-create Condition.code"); 1105 else if (Configuration.doAutoCreate()) 1106 this.code = new CodeableConcept(); // cc 1107 return this.code; 1108 } 1109 1110 public boolean hasCode() { 1111 return this.code != null && !this.code.isEmpty(); 1112 } 1113 1114 /** 1115 * @param value {@link #code} (Identification of the condition, problem or 1116 * diagnosis.) 1117 */ 1118 public Condition setCode(CodeableConcept value) { 1119 this.code = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #bodySite} (The anatomical location where this condition 1125 * manifests itself.) 1126 */ 1127 public List<CodeableConcept> getBodySite() { 1128 if (this.bodySite == null) 1129 this.bodySite = new ArrayList<CodeableConcept>(); 1130 return this.bodySite; 1131 } 1132 1133 /** 1134 * @return Returns a reference to <code>this</code> for easy method chaining 1135 */ 1136 public Condition setBodySite(List<CodeableConcept> theBodySite) { 1137 this.bodySite = theBodySite; 1138 return this; 1139 } 1140 1141 public boolean hasBodySite() { 1142 if (this.bodySite == null) 1143 return false; 1144 for (CodeableConcept item : this.bodySite) 1145 if (!item.isEmpty()) 1146 return true; 1147 return false; 1148 } 1149 1150 public CodeableConcept addBodySite() { // 3 1151 CodeableConcept t = new CodeableConcept(); 1152 if (this.bodySite == null) 1153 this.bodySite = new ArrayList<CodeableConcept>(); 1154 this.bodySite.add(t); 1155 return t; 1156 } 1157 1158 public Condition addBodySite(CodeableConcept t) { // 3 1159 if (t == null) 1160 return this; 1161 if (this.bodySite == null) 1162 this.bodySite = new ArrayList<CodeableConcept>(); 1163 this.bodySite.add(t); 1164 return this; 1165 } 1166 1167 /** 1168 * @return The first repetition of repeating field {@link #bodySite}, creating 1169 * it if it does not already exist 1170 */ 1171 public CodeableConcept getBodySiteFirstRep() { 1172 if (getBodySite().isEmpty()) { 1173 addBodySite(); 1174 } 1175 return getBodySite().get(0); 1176 } 1177 1178 /** 1179 * @return {@link #subject} (Indicates the patient or group who the condition 1180 * record is associated with.) 1181 */ 1182 public Reference getSubject() { 1183 if (this.subject == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create Condition.subject"); 1186 else if (Configuration.doAutoCreate()) 1187 this.subject = new Reference(); // cc 1188 return this.subject; 1189 } 1190 1191 public boolean hasSubject() { 1192 return this.subject != null && !this.subject.isEmpty(); 1193 } 1194 1195 /** 1196 * @param value {@link #subject} (Indicates the patient or group who the 1197 * condition record is associated with.) 1198 */ 1199 public Condition setSubject(Reference value) { 1200 this.subject = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #subject} The actual object that is the target of the 1206 * reference. The reference library doesn't populate this, but you can 1207 * use it to hold the resource if you resolve it. (Indicates the patient 1208 * or group who the condition record is associated with.) 1209 */ 1210 public Resource getSubjectTarget() { 1211 return this.subjectTarget; 1212 } 1213 1214 /** 1215 * @param value {@link #subject} The actual object that is the target of the 1216 * reference. The reference library doesn't use these, but you can 1217 * use it to hold the resource if you resolve it. (Indicates the 1218 * patient or group who the condition record is associated with.) 1219 */ 1220 public Condition setSubjectTarget(Resource value) { 1221 this.subjectTarget = value; 1222 return this; 1223 } 1224 1225 /** 1226 * @return {@link #encounter} (The Encounter during which this Condition was 1227 * created or to which the creation of this record is tightly 1228 * associated.) 1229 */ 1230 public Reference getEncounter() { 1231 if (this.encounter == null) 1232 if (Configuration.errorOnAutoCreate()) 1233 throw new Error("Attempt to auto-create Condition.encounter"); 1234 else if (Configuration.doAutoCreate()) 1235 this.encounter = new Reference(); // cc 1236 return this.encounter; 1237 } 1238 1239 public boolean hasEncounter() { 1240 return this.encounter != null && !this.encounter.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #encounter} (The Encounter during which this Condition 1245 * was created or to which the creation of this record is tightly 1246 * associated.) 1247 */ 1248 public Condition setEncounter(Reference value) { 1249 this.encounter = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #encounter} The actual object that is the target of the 1255 * reference. The reference library doesn't populate this, but you can 1256 * use it to hold the resource if you resolve it. (The Encounter during 1257 * which this Condition was created or to which the creation of this 1258 * record is tightly associated.) 1259 */ 1260 public Encounter getEncounterTarget() { 1261 if (this.encounterTarget == null) 1262 if (Configuration.errorOnAutoCreate()) 1263 throw new Error("Attempt to auto-create Condition.encounter"); 1264 else if (Configuration.doAutoCreate()) 1265 this.encounterTarget = new Encounter(); // aa 1266 return this.encounterTarget; 1267 } 1268 1269 /** 1270 * @param value {@link #encounter} The actual object that is the target of the 1271 * reference. The reference library doesn't use these, but you can 1272 * use it to hold the resource if you resolve it. (The Encounter 1273 * during which this Condition was created or to which the creation 1274 * of this record is tightly associated.) 1275 */ 1276 public Condition setEncounterTarget(Encounter value) { 1277 this.encounterTarget = value; 1278 return this; 1279 } 1280 1281 /** 1282 * @return {@link #onset} (Estimated or actual date or date-time the condition 1283 * began, in the opinion of the clinician.) 1284 */ 1285 public Type getOnset() { 1286 return this.onset; 1287 } 1288 1289 /** 1290 * @return {@link #onset} (Estimated or actual date or date-time the condition 1291 * began, in the opinion of the clinician.) 1292 */ 1293 public DateTimeType getOnsetDateTimeType() throws FHIRException { 1294 if (this.onset == null) 1295 this.onset = new DateTimeType(); 1296 if (!(this.onset instanceof DateTimeType)) 1297 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1298 + this.onset.getClass().getName() + " was encountered"); 1299 return (DateTimeType) this.onset; 1300 } 1301 1302 public boolean hasOnsetDateTimeType() { 1303 return this != null && this.onset instanceof DateTimeType; 1304 } 1305 1306 /** 1307 * @return {@link #onset} (Estimated or actual date or date-time the condition 1308 * began, in the opinion of the clinician.) 1309 */ 1310 public Age getOnsetAge() throws FHIRException { 1311 if (this.onset == null) 1312 this.onset = new Age(); 1313 if (!(this.onset instanceof Age)) 1314 throw new FHIRException( 1315 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 1316 return (Age) this.onset; 1317 } 1318 1319 public boolean hasOnsetAge() { 1320 return this != null && this.onset instanceof Age; 1321 } 1322 1323 /** 1324 * @return {@link #onset} (Estimated or actual date or date-time the condition 1325 * began, in the opinion of the clinician.) 1326 */ 1327 public Period getOnsetPeriod() throws FHIRException { 1328 if (this.onset == null) 1329 this.onset = new Period(); 1330 if (!(this.onset instanceof Period)) 1331 throw new FHIRException( 1332 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 1333 return (Period) this.onset; 1334 } 1335 1336 public boolean hasOnsetPeriod() { 1337 return this != null && this.onset instanceof Period; 1338 } 1339 1340 /** 1341 * @return {@link #onset} (Estimated or actual date or date-time the condition 1342 * began, in the opinion of the clinician.) 1343 */ 1344 public Range getOnsetRange() throws FHIRException { 1345 if (this.onset == null) 1346 this.onset = new Range(); 1347 if (!(this.onset instanceof Range)) 1348 throw new FHIRException( 1349 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 1350 return (Range) this.onset; 1351 } 1352 1353 public boolean hasOnsetRange() { 1354 return this != null && this.onset instanceof Range; 1355 } 1356 1357 /** 1358 * @return {@link #onset} (Estimated or actual date or date-time the condition 1359 * began, in the opinion of the clinician.) 1360 */ 1361 public StringType getOnsetStringType() throws FHIRException { 1362 if (this.onset == null) 1363 this.onset = new StringType(); 1364 if (!(this.onset instanceof StringType)) 1365 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.onset.getClass().getName() 1366 + " was encountered"); 1367 return (StringType) this.onset; 1368 } 1369 1370 public boolean hasOnsetStringType() { 1371 return this != null && this.onset instanceof StringType; 1372 } 1373 1374 public boolean hasOnset() { 1375 return this.onset != null && !this.onset.isEmpty(); 1376 } 1377 1378 /** 1379 * @param value {@link #onset} (Estimated or actual date or date-time the 1380 * condition began, in the opinion of the clinician.) 1381 */ 1382 public Condition setOnset(Type value) { 1383 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 1384 || value instanceof Range || value instanceof StringType)) 1385 throw new Error("Not the right type for Condition.onset[x]: " + value.fhirType()); 1386 this.onset = value; 1387 return this; 1388 } 1389 1390 /** 1391 * @return {@link #abatement} (The date or estimated date that the condition 1392 * resolved or went into remission. This is called "abatement" because 1393 * of the many overloaded connotations associated with "remission" or 1394 * "resolution" - Conditions are never really resolved, but they can 1395 * abate.) 1396 */ 1397 public Type getAbatement() { 1398 return this.abatement; 1399 } 1400 1401 /** 1402 * @return {@link #abatement} (The date or estimated date that the condition 1403 * resolved or went into remission. This is called "abatement" because 1404 * of the many overloaded connotations associated with "remission" or 1405 * "resolution" - Conditions are never really resolved, but they can 1406 * abate.) 1407 */ 1408 public DateTimeType getAbatementDateTimeType() throws FHIRException { 1409 if (this.abatement == null) 1410 this.abatement = new DateTimeType(); 1411 if (!(this.abatement instanceof DateTimeType)) 1412 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1413 + this.abatement.getClass().getName() + " was encountered"); 1414 return (DateTimeType) this.abatement; 1415 } 1416 1417 public boolean hasAbatementDateTimeType() { 1418 return this != null && this.abatement instanceof DateTimeType; 1419 } 1420 1421 /** 1422 * @return {@link #abatement} (The date or estimated date that the condition 1423 * resolved or went into remission. This is called "abatement" because 1424 * of the many overloaded connotations associated with "remission" or 1425 * "resolution" - Conditions are never really resolved, but they can 1426 * abate.) 1427 */ 1428 public Age getAbatementAge() throws FHIRException { 1429 if (this.abatement == null) 1430 this.abatement = new Age(); 1431 if (!(this.abatement instanceof Age)) 1432 throw new FHIRException( 1433 "Type mismatch: the type Age was expected, but " + this.abatement.getClass().getName() + " was encountered"); 1434 return (Age) this.abatement; 1435 } 1436 1437 public boolean hasAbatementAge() { 1438 return this != null && this.abatement instanceof Age; 1439 } 1440 1441 /** 1442 * @return {@link #abatement} (The date or estimated date that the condition 1443 * resolved or went into remission. This is called "abatement" because 1444 * of the many overloaded connotations associated with "remission" or 1445 * "resolution" - Conditions are never really resolved, but they can 1446 * abate.) 1447 */ 1448 public Period getAbatementPeriod() throws FHIRException { 1449 if (this.abatement == null) 1450 this.abatement = new Period(); 1451 if (!(this.abatement instanceof Period)) 1452 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.abatement.getClass().getName() 1453 + " was encountered"); 1454 return (Period) this.abatement; 1455 } 1456 1457 public boolean hasAbatementPeriod() { 1458 return this != null && this.abatement instanceof Period; 1459 } 1460 1461 /** 1462 * @return {@link #abatement} (The date or estimated date that the condition 1463 * resolved or went into remission. This is called "abatement" because 1464 * of the many overloaded connotations associated with "remission" or 1465 * "resolution" - Conditions are never really resolved, but they can 1466 * abate.) 1467 */ 1468 public Range getAbatementRange() throws FHIRException { 1469 if (this.abatement == null) 1470 this.abatement = new Range(); 1471 if (!(this.abatement instanceof Range)) 1472 throw new FHIRException("Type mismatch: the type Range was expected, but " + this.abatement.getClass().getName() 1473 + " was encountered"); 1474 return (Range) this.abatement; 1475 } 1476 1477 public boolean hasAbatementRange() { 1478 return this != null && this.abatement instanceof Range; 1479 } 1480 1481 /** 1482 * @return {@link #abatement} (The date or estimated date that the condition 1483 * resolved or went into remission. This is called "abatement" because 1484 * of the many overloaded connotations associated with "remission" or 1485 * "resolution" - Conditions are never really resolved, but they can 1486 * abate.) 1487 */ 1488 public StringType getAbatementStringType() throws FHIRException { 1489 if (this.abatement == null) 1490 this.abatement = new StringType(); 1491 if (!(this.abatement instanceof StringType)) 1492 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1493 + this.abatement.getClass().getName() + " was encountered"); 1494 return (StringType) this.abatement; 1495 } 1496 1497 public boolean hasAbatementStringType() { 1498 return this != null && this.abatement instanceof StringType; 1499 } 1500 1501 public boolean hasAbatement() { 1502 return this.abatement != null && !this.abatement.isEmpty(); 1503 } 1504 1505 /** 1506 * @param value {@link #abatement} (The date or estimated date that the 1507 * condition resolved or went into remission. This is called 1508 * "abatement" because of the many overloaded connotations 1509 * associated with "remission" or "resolution" - Conditions are 1510 * never really resolved, but they can abate.) 1511 */ 1512 public Condition setAbatement(Type value) { 1513 if (value != null && !(value instanceof DateTimeType || value instanceof Age || value instanceof Period 1514 || value instanceof Range || value instanceof StringType)) 1515 throw new Error("Not the right type for Condition.abatement[x]: " + value.fhirType()); 1516 this.abatement = value; 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #recordedDate} (The recordedDate represents when this 1522 * particular Condition record was created in the system, which is often 1523 * a system-generated date.). This is the underlying object with id, 1524 * value and extensions. The accessor "getRecordedDate" gives direct 1525 * access to the value 1526 */ 1527 public DateTimeType getRecordedDateElement() { 1528 if (this.recordedDate == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create Condition.recordedDate"); 1531 else if (Configuration.doAutoCreate()) 1532 this.recordedDate = new DateTimeType(); // bb 1533 return this.recordedDate; 1534 } 1535 1536 public boolean hasRecordedDateElement() { 1537 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1538 } 1539 1540 public boolean hasRecordedDate() { 1541 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #recordedDate} (The recordedDate represents when this 1546 * particular Condition record was created in the system, which is 1547 * often a system-generated date.). This is the underlying object 1548 * with id, value and extensions. The accessor "getRecordedDate" 1549 * gives direct access to the value 1550 */ 1551 public Condition setRecordedDateElement(DateTimeType value) { 1552 this.recordedDate = value; 1553 return this; 1554 } 1555 1556 /** 1557 * @return The recordedDate represents when this particular Condition record was 1558 * created in the system, which is often a system-generated date. 1559 */ 1560 public Date getRecordedDate() { 1561 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1562 } 1563 1564 /** 1565 * @param value The recordedDate represents when this particular Condition 1566 * record was created in the system, which is often a 1567 * system-generated date. 1568 */ 1569 public Condition setRecordedDate(Date value) { 1570 if (value == null) 1571 this.recordedDate = null; 1572 else { 1573 if (this.recordedDate == null) 1574 this.recordedDate = new DateTimeType(); 1575 this.recordedDate.setValue(value); 1576 } 1577 return this; 1578 } 1579 1580 /** 1581 * @return {@link #recorder} (Individual who recorded the record and takes 1582 * responsibility for its content.) 1583 */ 1584 public Reference getRecorder() { 1585 if (this.recorder == null) 1586 if (Configuration.errorOnAutoCreate()) 1587 throw new Error("Attempt to auto-create Condition.recorder"); 1588 else if (Configuration.doAutoCreate()) 1589 this.recorder = new Reference(); // cc 1590 return this.recorder; 1591 } 1592 1593 public boolean hasRecorder() { 1594 return this.recorder != null && !this.recorder.isEmpty(); 1595 } 1596 1597 /** 1598 * @param value {@link #recorder} (Individual who recorded the record and takes 1599 * responsibility for its content.) 1600 */ 1601 public Condition setRecorder(Reference value) { 1602 this.recorder = value; 1603 return this; 1604 } 1605 1606 /** 1607 * @return {@link #recorder} The actual object that is the target of the 1608 * reference. The reference library doesn't populate this, but you can 1609 * use it to hold the resource if you resolve it. (Individual who 1610 * recorded the record and takes responsibility for its content.) 1611 */ 1612 public Resource getRecorderTarget() { 1613 return this.recorderTarget; 1614 } 1615 1616 /** 1617 * @param value {@link #recorder} The actual object that is the target of the 1618 * reference. The reference library doesn't use these, but you can 1619 * use it to hold the resource if you resolve it. (Individual who 1620 * recorded the record and takes responsibility for its content.) 1621 */ 1622 public Condition setRecorderTarget(Resource value) { 1623 this.recorderTarget = value; 1624 return this; 1625 } 1626 1627 /** 1628 * @return {@link #asserter} (Individual who is making the condition statement.) 1629 */ 1630 public Reference getAsserter() { 1631 if (this.asserter == null) 1632 if (Configuration.errorOnAutoCreate()) 1633 throw new Error("Attempt to auto-create Condition.asserter"); 1634 else if (Configuration.doAutoCreate()) 1635 this.asserter = new Reference(); // cc 1636 return this.asserter; 1637 } 1638 1639 public boolean hasAsserter() { 1640 return this.asserter != null && !this.asserter.isEmpty(); 1641 } 1642 1643 /** 1644 * @param value {@link #asserter} (Individual who is making the condition 1645 * statement.) 1646 */ 1647 public Condition setAsserter(Reference value) { 1648 this.asserter = value; 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #asserter} The actual object that is the target of the 1654 * reference. The reference library doesn't populate this, but you can 1655 * use it to hold the resource if you resolve it. (Individual who is 1656 * making the condition statement.) 1657 */ 1658 public Resource getAsserterTarget() { 1659 return this.asserterTarget; 1660 } 1661 1662 /** 1663 * @param value {@link #asserter} The actual object that is the target of the 1664 * reference. The reference library doesn't use these, but you can 1665 * use it to hold the resource if you resolve it. (Individual who 1666 * is making the condition statement.) 1667 */ 1668 public Condition setAsserterTarget(Resource value) { 1669 this.asserterTarget = value; 1670 return this; 1671 } 1672 1673 /** 1674 * @return {@link #stage} (Clinical stage or grade of a condition. May include 1675 * formal severity assessments.) 1676 */ 1677 public List<ConditionStageComponent> getStage() { 1678 if (this.stage == null) 1679 this.stage = new ArrayList<ConditionStageComponent>(); 1680 return this.stage; 1681 } 1682 1683 /** 1684 * @return Returns a reference to <code>this</code> for easy method chaining 1685 */ 1686 public Condition setStage(List<ConditionStageComponent> theStage) { 1687 this.stage = theStage; 1688 return this; 1689 } 1690 1691 public boolean hasStage() { 1692 if (this.stage == null) 1693 return false; 1694 for (ConditionStageComponent item : this.stage) 1695 if (!item.isEmpty()) 1696 return true; 1697 return false; 1698 } 1699 1700 public ConditionStageComponent addStage() { // 3 1701 ConditionStageComponent t = new ConditionStageComponent(); 1702 if (this.stage == null) 1703 this.stage = new ArrayList<ConditionStageComponent>(); 1704 this.stage.add(t); 1705 return t; 1706 } 1707 1708 public Condition addStage(ConditionStageComponent t) { // 3 1709 if (t == null) 1710 return this; 1711 if (this.stage == null) 1712 this.stage = new ArrayList<ConditionStageComponent>(); 1713 this.stage.add(t); 1714 return this; 1715 } 1716 1717 /** 1718 * @return The first repetition of repeating field {@link #stage}, creating it 1719 * if it does not already exist 1720 */ 1721 public ConditionStageComponent getStageFirstRep() { 1722 if (getStage().isEmpty()) { 1723 addStage(); 1724 } 1725 return getStage().get(0); 1726 } 1727 1728 /** 1729 * @return {@link #evidence} (Supporting evidence / manifestations that are the 1730 * basis of the Condition's verification status, such as evidence that 1731 * confirmed or refuted the condition.) 1732 */ 1733 public List<ConditionEvidenceComponent> getEvidence() { 1734 if (this.evidence == null) 1735 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1736 return this.evidence; 1737 } 1738 1739 /** 1740 * @return Returns a reference to <code>this</code> for easy method chaining 1741 */ 1742 public Condition setEvidence(List<ConditionEvidenceComponent> theEvidence) { 1743 this.evidence = theEvidence; 1744 return this; 1745 } 1746 1747 public boolean hasEvidence() { 1748 if (this.evidence == null) 1749 return false; 1750 for (ConditionEvidenceComponent item : this.evidence) 1751 if (!item.isEmpty()) 1752 return true; 1753 return false; 1754 } 1755 1756 public ConditionEvidenceComponent addEvidence() { // 3 1757 ConditionEvidenceComponent t = new ConditionEvidenceComponent(); 1758 if (this.evidence == null) 1759 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1760 this.evidence.add(t); 1761 return t; 1762 } 1763 1764 public Condition addEvidence(ConditionEvidenceComponent t) { // 3 1765 if (t == null) 1766 return this; 1767 if (this.evidence == null) 1768 this.evidence = new ArrayList<ConditionEvidenceComponent>(); 1769 this.evidence.add(t); 1770 return this; 1771 } 1772 1773 /** 1774 * @return The first repetition of repeating field {@link #evidence}, creating 1775 * it if it does not already exist 1776 */ 1777 public ConditionEvidenceComponent getEvidenceFirstRep() { 1778 if (getEvidence().isEmpty()) { 1779 addEvidence(); 1780 } 1781 return getEvidence().get(0); 1782 } 1783 1784 /** 1785 * @return {@link #note} (Additional information about the Condition. This is a 1786 * general notes/comments entry for description of the Condition, its 1787 * diagnosis and prognosis.) 1788 */ 1789 public List<Annotation> getNote() { 1790 if (this.note == null) 1791 this.note = new ArrayList<Annotation>(); 1792 return this.note; 1793 } 1794 1795 /** 1796 * @return Returns a reference to <code>this</code> for easy method chaining 1797 */ 1798 public Condition setNote(List<Annotation> theNote) { 1799 this.note = theNote; 1800 return this; 1801 } 1802 1803 public boolean hasNote() { 1804 if (this.note == null) 1805 return false; 1806 for (Annotation item : this.note) 1807 if (!item.isEmpty()) 1808 return true; 1809 return false; 1810 } 1811 1812 public Annotation addNote() { // 3 1813 Annotation t = new Annotation(); 1814 if (this.note == null) 1815 this.note = new ArrayList<Annotation>(); 1816 this.note.add(t); 1817 return t; 1818 } 1819 1820 public Condition addNote(Annotation t) { // 3 1821 if (t == null) 1822 return this; 1823 if (this.note == null) 1824 this.note = new ArrayList<Annotation>(); 1825 this.note.add(t); 1826 return this; 1827 } 1828 1829 /** 1830 * @return The first repetition of repeating field {@link #note}, creating it if 1831 * it does not already exist 1832 */ 1833 public Annotation getNoteFirstRep() { 1834 if (getNote().isEmpty()) { 1835 addNote(); 1836 } 1837 return getNote().get(0); 1838 } 1839 1840 protected void listChildren(List<Property> children) { 1841 super.listChildren(children); 1842 children.add(new Property("identifier", "Identifier", 1843 "Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1844 0, java.lang.Integer.MAX_VALUE, identifier)); 1845 children.add(new Property("clinicalStatus", "CodeableConcept", "The clinical status of the condition.", 0, 1, 1846 clinicalStatus)); 1847 children.add(new Property("verificationStatus", "CodeableConcept", 1848 "The verification status to support the clinical status of the condition.", 0, 1, verificationStatus)); 1849 children.add(new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, 1850 java.lang.Integer.MAX_VALUE, category)); 1851 children.add(new Property("severity", "CodeableConcept", 1852 "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity)); 1853 children.add( 1854 new Property("code", "CodeableConcept", "Identification of the condition, problem or diagnosis.", 0, 1, code)); 1855 children.add(new Property("bodySite", "CodeableConcept", 1856 "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 1857 children.add(new Property("subject", "Reference(Patient|Group)", 1858 "Indicates the patient or group who the condition record is associated with.", 0, 1, subject)); 1859 children.add(new Property("encounter", "Reference(Encounter)", 1860 "The Encounter during which this Condition was created or to which the creation of this record is tightly associated.", 1861 0, 1, encounter)); 1862 children.add(new Property("onset[x]", "dateTime|Age|Period|Range|string", 1863 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset)); 1864 children.add(new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1865 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1866 0, 1, abatement)); 1867 children.add(new Property("recordedDate", "dateTime", 1868 "The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.", 1869 0, 1, recordedDate)); 1870 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 1871 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder)); 1872 children.add(new Property("asserter", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 1873 "Individual who is making the condition statement.", 0, 1, asserter)); 1874 children.add( 1875 new Property("stage", "", "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1876 java.lang.Integer.MAX_VALUE, stage)); 1877 children.add(new Property("evidence", "", 1878 "Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.", 1879 0, java.lang.Integer.MAX_VALUE, evidence)); 1880 children.add(new Property("note", "Annotation", 1881 "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 1882 0, java.lang.Integer.MAX_VALUE, note)); 1883 } 1884 1885 @Override 1886 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1887 switch (_hash) { 1888 case -1618432855: 1889 /* identifier */ return new Property("identifier", "Identifier", 1890 "Business identifiers assigned to this condition by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 1891 0, java.lang.Integer.MAX_VALUE, identifier); 1892 case -462853915: 1893 /* clinicalStatus */ return new Property("clinicalStatus", "CodeableConcept", 1894 "The clinical status of the condition.", 0, 1, clinicalStatus); 1895 case -842509843: 1896 /* verificationStatus */ return new Property("verificationStatus", "CodeableConcept", 1897 "The verification status to support the clinical status of the condition.", 0, 1, verificationStatus); 1898 case 50511102: 1899 /* category */ return new Property("category", "CodeableConcept", "A category assigned to the condition.", 0, 1900 java.lang.Integer.MAX_VALUE, category); 1901 case 1478300413: 1902 /* severity */ return new Property("severity", "CodeableConcept", 1903 "A subjective assessment of the severity of the condition as evaluated by the clinician.", 0, 1, severity); 1904 case 3059181: 1905 /* code */ return new Property("code", "CodeableConcept", 1906 "Identification of the condition, problem or diagnosis.", 0, 1, code); 1907 case 1702620169: 1908 /* bodySite */ return new Property("bodySite", "CodeableConcept", 1909 "The anatomical location where this condition manifests itself.", 0, java.lang.Integer.MAX_VALUE, bodySite); 1910 case -1867885268: 1911 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1912 "Indicates the patient or group who the condition record is associated with.", 0, 1, subject); 1913 case 1524132147: 1914 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1915 "The Encounter during which this Condition was created or to which the creation of this record is tightly associated.", 1916 0, 1, encounter); 1917 case -1886216323: 1918 /* onset[x] */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1919 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1920 case 105901603: 1921 /* onset */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1922 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1923 case -1701663010: 1924 /* onsetDateTime */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1925 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1926 case -1886241828: 1927 /* onsetAge */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1928 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1929 case -1545082428: 1930 /* onsetPeriod */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1931 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1932 case -186664742: 1933 /* onsetRange */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1934 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1935 case -1445342188: 1936 /* onsetString */ return new Property("onset[x]", "dateTime|Age|Period|Range|string", 1937 "Estimated or actual date or date-time the condition began, in the opinion of the clinician.", 0, 1, onset); 1938 case -584196495: 1939 /* abatement[x] */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1940 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1941 0, 1, abatement); 1942 case -921554001: 1943 /* abatement */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1944 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1945 0, 1, abatement); 1946 case 44869738: 1947 /* abatementDateTime */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1948 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1949 0, 1, abatement); 1950 case -584222000: 1951 /* abatementAge */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1952 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1953 0, 1, abatement); 1954 case -922036656: 1955 /* abatementPeriod */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1956 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1957 0, 1, abatement); 1958 case 1218906830: 1959 /* abatementRange */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1960 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1961 0, 1, abatement); 1962 case -822296416: 1963 /* abatementString */ return new Property("abatement[x]", "dateTime|Age|Period|Range|string", 1964 "The date or estimated date that the condition resolved or went into remission. This is called \"abatement\" because of the many overloaded connotations associated with \"remission\" or \"resolution\" - Conditions are never really resolved, but they can abate.", 1965 0, 1, abatement); 1966 case -1952893826: 1967 /* recordedDate */ return new Property("recordedDate", "dateTime", 1968 "The recordedDate represents when this particular Condition record was created in the system, which is often a system-generated date.", 1969 0, 1, recordedDate); 1970 case -799233858: 1971 /* recorder */ return new Property("recorder", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 1972 "Individual who recorded the record and takes responsibility for its content.", 0, 1, recorder); 1973 case -373242253: 1974 /* asserter */ return new Property("asserter", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", 1975 "Individual who is making the condition statement.", 0, 1, asserter); 1976 case 109757182: 1977 /* stage */ return new Property("stage", "", 1978 "Clinical stage or grade of a condition. May include formal severity assessments.", 0, 1979 java.lang.Integer.MAX_VALUE, stage); 1980 case 382967383: 1981 /* evidence */ return new Property("evidence", "", 1982 "Supporting evidence / manifestations that are the basis of the Condition's verification status, such as evidence that confirmed or refuted the condition.", 1983 0, java.lang.Integer.MAX_VALUE, evidence); 1984 case 3387378: 1985 /* note */ return new Property("note", "Annotation", 1986 "Additional information about the Condition. This is a general notes/comments entry for description of the Condition, its diagnosis and prognosis.", 1987 0, java.lang.Integer.MAX_VALUE, note); 1988 default: 1989 return super.getNamedProperty(_hash, _name, _checkValid); 1990 } 1991 1992 } 1993 1994 @Override 1995 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1996 switch (hash) { 1997 case -1618432855: 1998 /* identifier */ return this.identifier == null ? new Base[0] 1999 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2000 case -462853915: 2001 /* clinicalStatus */ return this.clinicalStatus == null ? new Base[0] : new Base[] { this.clinicalStatus }; // CodeableConcept 2002 case -842509843: 2003 /* verificationStatus */ return this.verificationStatus == null ? new Base[0] 2004 : new Base[] { this.verificationStatus }; // CodeableConcept 2005 case 50511102: 2006 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2007 case 1478300413: 2008 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // CodeableConcept 2009 case 3059181: 2010 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2011 case 1702620169: 2012 /* bodySite */ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // CodeableConcept 2013 case -1867885268: 2014 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2015 case 1524132147: 2016 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 2017 case 105901603: 2018 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // Type 2019 case -921554001: 2020 /* abatement */ return this.abatement == null ? new Base[0] : new Base[] { this.abatement }; // Type 2021 case -1952893826: 2022 /* recordedDate */ return this.recordedDate == null ? new Base[0] : new Base[] { this.recordedDate }; // DateTimeType 2023 case -799233858: 2024 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 2025 case -373242253: 2026 /* asserter */ return this.asserter == null ? new Base[0] : new Base[] { this.asserter }; // Reference 2027 case 109757182: 2028 /* stage */ return this.stage == null ? new Base[0] : this.stage.toArray(new Base[this.stage.size()]); // ConditionStageComponent 2029 case 382967383: 2030 /* evidence */ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // ConditionEvidenceComponent 2031 case 3387378: 2032 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2033 default: 2034 return super.getProperty(hash, name, checkValid); 2035 } 2036 2037 } 2038 2039 @Override 2040 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2041 switch (hash) { 2042 case -1618432855: // identifier 2043 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2044 return value; 2045 case -462853915: // clinicalStatus 2046 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2047 return value; 2048 case -842509843: // verificationStatus 2049 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2050 return value; 2051 case 50511102: // category 2052 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 2053 return value; 2054 case 1478300413: // severity 2055 this.severity = castToCodeableConcept(value); // CodeableConcept 2056 return value; 2057 case 3059181: // code 2058 this.code = castToCodeableConcept(value); // CodeableConcept 2059 return value; 2060 case 1702620169: // bodySite 2061 this.getBodySite().add(castToCodeableConcept(value)); // CodeableConcept 2062 return value; 2063 case -1867885268: // subject 2064 this.subject = castToReference(value); // Reference 2065 return value; 2066 case 1524132147: // encounter 2067 this.encounter = castToReference(value); // Reference 2068 return value; 2069 case 105901603: // onset 2070 this.onset = castToType(value); // Type 2071 return value; 2072 case -921554001: // abatement 2073 this.abatement = castToType(value); // Type 2074 return value; 2075 case -1952893826: // recordedDate 2076 this.recordedDate = castToDateTime(value); // DateTimeType 2077 return value; 2078 case -799233858: // recorder 2079 this.recorder = castToReference(value); // Reference 2080 return value; 2081 case -373242253: // asserter 2082 this.asserter = castToReference(value); // Reference 2083 return value; 2084 case 109757182: // stage 2085 this.getStage().add((ConditionStageComponent) value); // ConditionStageComponent 2086 return value; 2087 case 382967383: // evidence 2088 this.getEvidence().add((ConditionEvidenceComponent) value); // ConditionEvidenceComponent 2089 return value; 2090 case 3387378: // note 2091 this.getNote().add(castToAnnotation(value)); // Annotation 2092 return value; 2093 default: 2094 return super.setProperty(hash, name, value); 2095 } 2096 2097 } 2098 2099 @Override 2100 public Base setProperty(String name, Base value) throws FHIRException { 2101 if (name.equals("identifier")) { 2102 this.getIdentifier().add(castToIdentifier(value)); 2103 } else if (name.equals("clinicalStatus")) { 2104 this.clinicalStatus = castToCodeableConcept(value); // CodeableConcept 2105 } else if (name.equals("verificationStatus")) { 2106 this.verificationStatus = castToCodeableConcept(value); // CodeableConcept 2107 } else if (name.equals("category")) { 2108 this.getCategory().add(castToCodeableConcept(value)); 2109 } else if (name.equals("severity")) { 2110 this.severity = castToCodeableConcept(value); // CodeableConcept 2111 } else if (name.equals("code")) { 2112 this.code = castToCodeableConcept(value); // CodeableConcept 2113 } else if (name.equals("bodySite")) { 2114 this.getBodySite().add(castToCodeableConcept(value)); 2115 } else if (name.equals("subject")) { 2116 this.subject = castToReference(value); // Reference 2117 } else if (name.equals("encounter")) { 2118 this.encounter = castToReference(value); // Reference 2119 } else if (name.equals("onset[x]")) { 2120 this.onset = castToType(value); // Type 2121 } else if (name.equals("abatement[x]")) { 2122 this.abatement = castToType(value); // Type 2123 } else if (name.equals("recordedDate")) { 2124 this.recordedDate = castToDateTime(value); // DateTimeType 2125 } else if (name.equals("recorder")) { 2126 this.recorder = castToReference(value); // Reference 2127 } else if (name.equals("asserter")) { 2128 this.asserter = castToReference(value); // Reference 2129 } else if (name.equals("stage")) { 2130 this.getStage().add((ConditionStageComponent) value); 2131 } else if (name.equals("evidence")) { 2132 this.getEvidence().add((ConditionEvidenceComponent) value); 2133 } else if (name.equals("note")) { 2134 this.getNote().add(castToAnnotation(value)); 2135 } else 2136 return super.setProperty(name, value); 2137 return value; 2138 } 2139 2140 @Override 2141 public void removeChild(String name, Base value) throws FHIRException { 2142 if (name.equals("identifier")) { 2143 this.getIdentifier().remove(castToIdentifier(value)); 2144 } else if (name.equals("clinicalStatus")) { 2145 this.clinicalStatus = null; 2146 } else if (name.equals("verificationStatus")) { 2147 this.verificationStatus = null; 2148 } else if (name.equals("category")) { 2149 this.getCategory().remove(castToCodeableConcept(value)); 2150 } else if (name.equals("severity")) { 2151 this.severity = null; 2152 } else if (name.equals("code")) { 2153 this.code = null; 2154 } else if (name.equals("bodySite")) { 2155 this.getBodySite().remove(castToCodeableConcept(value)); 2156 } else if (name.equals("subject")) { 2157 this.subject = null; 2158 } else if (name.equals("encounter")) { 2159 this.encounter = null; 2160 } else if (name.equals("onset[x]")) { 2161 this.onset = null; 2162 } else if (name.equals("abatement[x]")) { 2163 this.abatement = null; 2164 } else if (name.equals("recordedDate")) { 2165 this.recordedDate = null; 2166 } else if (name.equals("recorder")) { 2167 this.recorder = null; 2168 } else if (name.equals("asserter")) { 2169 this.asserter = null; 2170 } else if (name.equals("stage")) { 2171 this.getStage().remove((ConditionStageComponent) value); 2172 } else if (name.equals("evidence")) { 2173 this.getEvidence().remove((ConditionEvidenceComponent) value); 2174 } else if (name.equals("note")) { 2175 this.getNote().remove(castToAnnotation(value)); 2176 } else 2177 super.removeChild(name, value); 2178 2179 } 2180 2181 @Override 2182 public Base makeProperty(int hash, String name) throws FHIRException { 2183 switch (hash) { 2184 case -1618432855: 2185 return addIdentifier(); 2186 case -462853915: 2187 return getClinicalStatus(); 2188 case -842509843: 2189 return getVerificationStatus(); 2190 case 50511102: 2191 return addCategory(); 2192 case 1478300413: 2193 return getSeverity(); 2194 case 3059181: 2195 return getCode(); 2196 case 1702620169: 2197 return addBodySite(); 2198 case -1867885268: 2199 return getSubject(); 2200 case 1524132147: 2201 return getEncounter(); 2202 case -1886216323: 2203 return getOnset(); 2204 case 105901603: 2205 return getOnset(); 2206 case -584196495: 2207 return getAbatement(); 2208 case -921554001: 2209 return getAbatement(); 2210 case -1952893826: 2211 return getRecordedDateElement(); 2212 case -799233858: 2213 return getRecorder(); 2214 case -373242253: 2215 return getAsserter(); 2216 case 109757182: 2217 return addStage(); 2218 case 382967383: 2219 return addEvidence(); 2220 case 3387378: 2221 return addNote(); 2222 default: 2223 return super.makeProperty(hash, name); 2224 } 2225 2226 } 2227 2228 @Override 2229 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2230 switch (hash) { 2231 case -1618432855: 2232 /* identifier */ return new String[] { "Identifier" }; 2233 case -462853915: 2234 /* clinicalStatus */ return new String[] { "CodeableConcept" }; 2235 case -842509843: 2236 /* verificationStatus */ return new String[] { "CodeableConcept" }; 2237 case 50511102: 2238 /* category */ return new String[] { "CodeableConcept" }; 2239 case 1478300413: 2240 /* severity */ return new String[] { "CodeableConcept" }; 2241 case 3059181: 2242 /* code */ return new String[] { "CodeableConcept" }; 2243 case 1702620169: 2244 /* bodySite */ return new String[] { "CodeableConcept" }; 2245 case -1867885268: 2246 /* subject */ return new String[] { "Reference" }; 2247 case 1524132147: 2248 /* encounter */ return new String[] { "Reference" }; 2249 case 105901603: 2250 /* onset */ return new String[] { "dateTime", "Age", "Period", "Range", "string" }; 2251 case -921554001: 2252 /* abatement */ return new String[] { "dateTime", "Age", "Period", "Range", "string" }; 2253 case -1952893826: 2254 /* recordedDate */ return new String[] { "dateTime" }; 2255 case -799233858: 2256 /* recorder */ return new String[] { "Reference" }; 2257 case -373242253: 2258 /* asserter */ return new String[] { "Reference" }; 2259 case 109757182: 2260 /* stage */ return new String[] {}; 2261 case 382967383: 2262 /* evidence */ return new String[] {}; 2263 case 3387378: 2264 /* note */ return new String[] { "Annotation" }; 2265 default: 2266 return super.getTypesForProperty(hash, name); 2267 } 2268 2269 } 2270 2271 @Override 2272 public Base addChild(String name) throws FHIRException { 2273 if (name.equals("identifier")) { 2274 return addIdentifier(); 2275 } else if (name.equals("clinicalStatus")) { 2276 this.clinicalStatus = new CodeableConcept(); 2277 return this.clinicalStatus; 2278 } else if (name.equals("verificationStatus")) { 2279 this.verificationStatus = new CodeableConcept(); 2280 return this.verificationStatus; 2281 } else if (name.equals("category")) { 2282 return addCategory(); 2283 } else if (name.equals("severity")) { 2284 this.severity = new CodeableConcept(); 2285 return this.severity; 2286 } else if (name.equals("code")) { 2287 this.code = new CodeableConcept(); 2288 return this.code; 2289 } else if (name.equals("bodySite")) { 2290 return addBodySite(); 2291 } else if (name.equals("subject")) { 2292 this.subject = new Reference(); 2293 return this.subject; 2294 } else if (name.equals("encounter")) { 2295 this.encounter = new Reference(); 2296 return this.encounter; 2297 } else if (name.equals("onsetDateTime")) { 2298 this.onset = new DateTimeType(); 2299 return this.onset; 2300 } else if (name.equals("onsetAge")) { 2301 this.onset = new Age(); 2302 return this.onset; 2303 } else if (name.equals("onsetPeriod")) { 2304 this.onset = new Period(); 2305 return this.onset; 2306 } else if (name.equals("onsetRange")) { 2307 this.onset = new Range(); 2308 return this.onset; 2309 } else if (name.equals("onsetString")) { 2310 this.onset = new StringType(); 2311 return this.onset; 2312 } else if (name.equals("abatementDateTime")) { 2313 this.abatement = new DateTimeType(); 2314 return this.abatement; 2315 } else if (name.equals("abatementAge")) { 2316 this.abatement = new Age(); 2317 return this.abatement; 2318 } else if (name.equals("abatementPeriod")) { 2319 this.abatement = new Period(); 2320 return this.abatement; 2321 } else if (name.equals("abatementRange")) { 2322 this.abatement = new Range(); 2323 return this.abatement; 2324 } else if (name.equals("abatementString")) { 2325 this.abatement = new StringType(); 2326 return this.abatement; 2327 } else if (name.equals("recordedDate")) { 2328 throw new FHIRException("Cannot call addChild on a singleton property Condition.recordedDate"); 2329 } else if (name.equals("recorder")) { 2330 this.recorder = new Reference(); 2331 return this.recorder; 2332 } else if (name.equals("asserter")) { 2333 this.asserter = new Reference(); 2334 return this.asserter; 2335 } else if (name.equals("stage")) { 2336 return addStage(); 2337 } else if (name.equals("evidence")) { 2338 return addEvidence(); 2339 } else if (name.equals("note")) { 2340 return addNote(); 2341 } else 2342 return super.addChild(name); 2343 } 2344 2345 public String fhirType() { 2346 return "Condition"; 2347 2348 } 2349 2350 public Condition copy() { 2351 Condition dst = new Condition(); 2352 copyValues(dst); 2353 return dst; 2354 } 2355 2356 public void copyValues(Condition dst) { 2357 super.copyValues(dst); 2358 if (identifier != null) { 2359 dst.identifier = new ArrayList<Identifier>(); 2360 for (Identifier i : identifier) 2361 dst.identifier.add(i.copy()); 2362 } 2363 ; 2364 dst.clinicalStatus = clinicalStatus == null ? null : clinicalStatus.copy(); 2365 dst.verificationStatus = verificationStatus == null ? null : verificationStatus.copy(); 2366 if (category != null) { 2367 dst.category = new ArrayList<CodeableConcept>(); 2368 for (CodeableConcept i : category) 2369 dst.category.add(i.copy()); 2370 } 2371 ; 2372 dst.severity = severity == null ? null : severity.copy(); 2373 dst.code = code == null ? null : code.copy(); 2374 if (bodySite != null) { 2375 dst.bodySite = new ArrayList<CodeableConcept>(); 2376 for (CodeableConcept i : bodySite) 2377 dst.bodySite.add(i.copy()); 2378 } 2379 ; 2380 dst.subject = subject == null ? null : subject.copy(); 2381 dst.encounter = encounter == null ? null : encounter.copy(); 2382 dst.onset = onset == null ? null : onset.copy(); 2383 dst.abatement = abatement == null ? null : abatement.copy(); 2384 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2385 dst.recorder = recorder == null ? null : recorder.copy(); 2386 dst.asserter = asserter == null ? null : asserter.copy(); 2387 if (stage != null) { 2388 dst.stage = new ArrayList<ConditionStageComponent>(); 2389 for (ConditionStageComponent i : stage) 2390 dst.stage.add(i.copy()); 2391 } 2392 ; 2393 if (evidence != null) { 2394 dst.evidence = new ArrayList<ConditionEvidenceComponent>(); 2395 for (ConditionEvidenceComponent i : evidence) 2396 dst.evidence.add(i.copy()); 2397 } 2398 ; 2399 if (note != null) { 2400 dst.note = new ArrayList<Annotation>(); 2401 for (Annotation i : note) 2402 dst.note.add(i.copy()); 2403 } 2404 ; 2405 } 2406 2407 protected Condition typedCopy() { 2408 return copy(); 2409 } 2410 2411 @Override 2412 public boolean equalsDeep(Base other_) { 2413 if (!super.equalsDeep(other_)) 2414 return false; 2415 if (!(other_ instanceof Condition)) 2416 return false; 2417 Condition o = (Condition) other_; 2418 return compareDeep(identifier, o.identifier, true) && compareDeep(clinicalStatus, o.clinicalStatus, true) 2419 && compareDeep(verificationStatus, o.verificationStatus, true) && compareDeep(category, o.category, true) 2420 && compareDeep(severity, o.severity, true) && compareDeep(code, o.code, true) 2421 && compareDeep(bodySite, o.bodySite, true) && compareDeep(subject, o.subject, true) 2422 && compareDeep(encounter, o.encounter, true) && compareDeep(onset, o.onset, true) 2423 && compareDeep(abatement, o.abatement, true) && compareDeep(recordedDate, o.recordedDate, true) 2424 && compareDeep(recorder, o.recorder, true) && compareDeep(asserter, o.asserter, true) 2425 && compareDeep(stage, o.stage, true) && compareDeep(evidence, o.evidence, true) 2426 && compareDeep(note, o.note, true); 2427 } 2428 2429 @Override 2430 public boolean equalsShallow(Base other_) { 2431 if (!super.equalsShallow(other_)) 2432 return false; 2433 if (!(other_ instanceof Condition)) 2434 return false; 2435 Condition o = (Condition) other_; 2436 return compareValues(recordedDate, o.recordedDate, true); 2437 } 2438 2439 public boolean isEmpty() { 2440 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, clinicalStatus, verificationStatus, 2441 category, severity, code, bodySite, subject, encounter, onset, abatement, recordedDate, recorder, asserter, 2442 stage, evidence, note); 2443 } 2444 2445 @Override 2446 public ResourceType getResourceType() { 2447 return ResourceType.Condition; 2448 } 2449 2450 /** 2451 * Search parameter: <b>severity</b> 2452 * <p> 2453 * Description: <b>The severity of the condition</b><br> 2454 * Type: <b>token</b><br> 2455 * Path: <b>Condition.severity</b><br> 2456 * </p> 2457 */ 2458 @SearchParamDefinition(name = "severity", path = "Condition.severity", description = "The severity of the condition", type = "token") 2459 public static final String SP_SEVERITY = "severity"; 2460 /** 2461 * <b>Fluent Client</b> search parameter constant for <b>severity</b> 2462 * <p> 2463 * Description: <b>The severity of the condition</b><br> 2464 * Type: <b>token</b><br> 2465 * Path: <b>Condition.severity</b><br> 2466 * </p> 2467 */ 2468 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEVERITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2469 SP_SEVERITY); 2470 2471 /** 2472 * Search parameter: <b>evidence-detail</b> 2473 * <p> 2474 * Description: <b>Supporting information found elsewhere</b><br> 2475 * Type: <b>reference</b><br> 2476 * Path: <b>Condition.evidence.detail</b><br> 2477 * </p> 2478 */ 2479 @SearchParamDefinition(name = "evidence-detail", path = "Condition.evidence.detail", description = "Supporting information found elsewhere", type = "reference") 2480 public static final String SP_EVIDENCE_DETAIL = "evidence-detail"; 2481 /** 2482 * <b>Fluent Client</b> search parameter constant for <b>evidence-detail</b> 2483 * <p> 2484 * Description: <b>Supporting information found elsewhere</b><br> 2485 * Type: <b>reference</b><br> 2486 * Path: <b>Condition.evidence.detail</b><br> 2487 * </p> 2488 */ 2489 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVIDENCE_DETAIL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2490 SP_EVIDENCE_DETAIL); 2491 2492 /** 2493 * Constant for fluent queries to be used to add include statements. Specifies 2494 * the path value of "<b>Condition:evidence-detail</b>". 2495 */ 2496 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVIDENCE_DETAIL = new ca.uhn.fhir.model.api.Include( 2497 "Condition:evidence-detail").toLocked(); 2498 2499 /** 2500 * Search parameter: <b>identifier</b> 2501 * <p> 2502 * Description: <b>A unique identifier of the condition record</b><br> 2503 * Type: <b>token</b><br> 2504 * Path: <b>Condition.identifier</b><br> 2505 * </p> 2506 */ 2507 @SearchParamDefinition(name = "identifier", path = "Condition.identifier", description = "A unique identifier of the condition record", type = "token") 2508 public static final String SP_IDENTIFIER = "identifier"; 2509 /** 2510 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2511 * <p> 2512 * Description: <b>A unique identifier of the condition record</b><br> 2513 * Type: <b>token</b><br> 2514 * Path: <b>Condition.identifier</b><br> 2515 * </p> 2516 */ 2517 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2518 SP_IDENTIFIER); 2519 2520 /** 2521 * Search parameter: <b>onset-info</b> 2522 * <p> 2523 * Description: <b>Onsets as a string</b><br> 2524 * Type: <b>string</b><br> 2525 * Path: <b>Condition.onset[x]</b><br> 2526 * </p> 2527 */ 2528 @SearchParamDefinition(name = "onset-info", path = "Condition.onset.as(string)", description = "Onsets as a string", type = "string") 2529 public static final String SP_ONSET_INFO = "onset-info"; 2530 /** 2531 * <b>Fluent Client</b> search parameter constant for <b>onset-info</b> 2532 * <p> 2533 * Description: <b>Onsets as a string</b><br> 2534 * Type: <b>string</b><br> 2535 * Path: <b>Condition.onset[x]</b><br> 2536 * </p> 2537 */ 2538 public static final ca.uhn.fhir.rest.gclient.StringClientParam ONSET_INFO = new ca.uhn.fhir.rest.gclient.StringClientParam( 2539 SP_ONSET_INFO); 2540 2541 /** 2542 * Search parameter: <b>recorded-date</b> 2543 * <p> 2544 * Description: <b>Date record was first recorded</b><br> 2545 * Type: <b>date</b><br> 2546 * Path: <b>Condition.recordedDate</b><br> 2547 * </p> 2548 */ 2549 @SearchParamDefinition(name = "recorded-date", path = "Condition.recordedDate", description = "Date record was first recorded", type = "date") 2550 public static final String SP_RECORDED_DATE = "recorded-date"; 2551 /** 2552 * <b>Fluent Client</b> search parameter constant for <b>recorded-date</b> 2553 * <p> 2554 * Description: <b>Date record was first recorded</b><br> 2555 * Type: <b>date</b><br> 2556 * Path: <b>Condition.recordedDate</b><br> 2557 * </p> 2558 */ 2559 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2560 SP_RECORDED_DATE); 2561 2562 /** 2563 * Search parameter: <b>code</b> 2564 * <p> 2565 * Description: <b>Code for the condition</b><br> 2566 * Type: <b>token</b><br> 2567 * Path: <b>Condition.code</b><br> 2568 * </p> 2569 */ 2570 @SearchParamDefinition(name = "code", path = "Condition.code", description = "Code for the condition", type = "token") 2571 public static final String SP_CODE = "code"; 2572 /** 2573 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2574 * <p> 2575 * Description: <b>Code for the condition</b><br> 2576 * Type: <b>token</b><br> 2577 * Path: <b>Condition.code</b><br> 2578 * </p> 2579 */ 2580 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2581 SP_CODE); 2582 2583 /** 2584 * Search parameter: <b>evidence</b> 2585 * <p> 2586 * Description: <b>Manifestation/symptom</b><br> 2587 * Type: <b>token</b><br> 2588 * Path: <b>Condition.evidence.code</b><br> 2589 * </p> 2590 */ 2591 @SearchParamDefinition(name = "evidence", path = "Condition.evidence.code", description = "Manifestation/symptom", type = "token") 2592 public static final String SP_EVIDENCE = "evidence"; 2593 /** 2594 * <b>Fluent Client</b> search parameter constant for <b>evidence</b> 2595 * <p> 2596 * Description: <b>Manifestation/symptom</b><br> 2597 * Type: <b>token</b><br> 2598 * Path: <b>Condition.evidence.code</b><br> 2599 * </p> 2600 */ 2601 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVIDENCE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2602 SP_EVIDENCE); 2603 2604 /** 2605 * Search parameter: <b>subject</b> 2606 * <p> 2607 * Description: <b>Who has the condition?</b><br> 2608 * Type: <b>reference</b><br> 2609 * Path: <b>Condition.subject</b><br> 2610 * </p> 2611 */ 2612 @SearchParamDefinition(name = "subject", path = "Condition.subject", description = "Who has the condition?", type = "reference", target = { 2613 Group.class, Patient.class }) 2614 public static final String SP_SUBJECT = "subject"; 2615 /** 2616 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2617 * <p> 2618 * Description: <b>Who has the condition?</b><br> 2619 * Type: <b>reference</b><br> 2620 * Path: <b>Condition.subject</b><br> 2621 * </p> 2622 */ 2623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2624 SP_SUBJECT); 2625 2626 /** 2627 * Constant for fluent queries to be used to add include statements. Specifies 2628 * the path value of "<b>Condition:subject</b>". 2629 */ 2630 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 2631 "Condition:subject").toLocked(); 2632 2633 /** 2634 * Search parameter: <b>verification-status</b> 2635 * <p> 2636 * Description: <b>unconfirmed | provisional | differential | confirmed | 2637 * refuted | entered-in-error</b><br> 2638 * Type: <b>token</b><br> 2639 * Path: <b>Condition.verificationStatus</b><br> 2640 * </p> 2641 */ 2642 @SearchParamDefinition(name = "verification-status", path = "Condition.verificationStatus", description = "unconfirmed | provisional | differential | confirmed | refuted | entered-in-error", type = "token") 2643 public static final String SP_VERIFICATION_STATUS = "verification-status"; 2644 /** 2645 * <b>Fluent Client</b> search parameter constant for <b>verification-status</b> 2646 * <p> 2647 * Description: <b>unconfirmed | provisional | differential | confirmed | 2648 * refuted | entered-in-error</b><br> 2649 * Type: <b>token</b><br> 2650 * Path: <b>Condition.verificationStatus</b><br> 2651 * </p> 2652 */ 2653 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERIFICATION_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2654 SP_VERIFICATION_STATUS); 2655 2656 /** 2657 * Search parameter: <b>clinical-status</b> 2658 * <p> 2659 * Description: <b>The clinical status of the condition</b><br> 2660 * Type: <b>token</b><br> 2661 * Path: <b>Condition.clinicalStatus</b><br> 2662 * </p> 2663 */ 2664 @SearchParamDefinition(name = "clinical-status", path = "Condition.clinicalStatus", description = "The clinical status of the condition", type = "token") 2665 public static final String SP_CLINICAL_STATUS = "clinical-status"; 2666 /** 2667 * <b>Fluent Client</b> search parameter constant for <b>clinical-status</b> 2668 * <p> 2669 * Description: <b>The clinical status of the condition</b><br> 2670 * Type: <b>token</b><br> 2671 * Path: <b>Condition.clinicalStatus</b><br> 2672 * </p> 2673 */ 2674 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLINICAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2675 SP_CLINICAL_STATUS); 2676 2677 /** 2678 * Search parameter: <b>encounter</b> 2679 * <p> 2680 * Description: <b>Encounter created as part of</b><br> 2681 * Type: <b>reference</b><br> 2682 * Path: <b>Condition.encounter</b><br> 2683 * </p> 2684 */ 2685 @SearchParamDefinition(name = "encounter", path = "Condition.encounter", description = "Encounter created as part of", type = "reference", providesMembershipIn = { 2686 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2687 public static final String SP_ENCOUNTER = "encounter"; 2688 /** 2689 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2690 * <p> 2691 * Description: <b>Encounter created as part of</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>Condition.encounter</b><br> 2694 * </p> 2695 */ 2696 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2697 SP_ENCOUNTER); 2698 2699 /** 2700 * Constant for fluent queries to be used to add include statements. Specifies 2701 * the path value of "<b>Condition:encounter</b>". 2702 */ 2703 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2704 "Condition:encounter").toLocked(); 2705 2706 /** 2707 * Search parameter: <b>onset-date</b> 2708 * <p> 2709 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2710 * Type: <b>date</b><br> 2711 * Path: <b>Condition.onset[x]</b><br> 2712 * </p> 2713 */ 2714 @SearchParamDefinition(name = "onset-date", path = "Condition.onset.as(dateTime) | Condition.onset.as(Period)", description = "Date related onsets (dateTime and Period)", type = "date") 2715 public static final String SP_ONSET_DATE = "onset-date"; 2716 /** 2717 * <b>Fluent Client</b> search parameter constant for <b>onset-date</b> 2718 * <p> 2719 * Description: <b>Date related onsets (dateTime and Period)</b><br> 2720 * Type: <b>date</b><br> 2721 * Path: <b>Condition.onset[x]</b><br> 2722 * </p> 2723 */ 2724 public static final ca.uhn.fhir.rest.gclient.DateClientParam ONSET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2725 SP_ONSET_DATE); 2726 2727 /** 2728 * Search parameter: <b>abatement-date</b> 2729 * <p> 2730 * Description: <b>Date-related abatements (dateTime and period)</b><br> 2731 * Type: <b>date</b><br> 2732 * Path: <b>Condition.abatement[x]</b><br> 2733 * </p> 2734 */ 2735 @SearchParamDefinition(name = "abatement-date", path = "Condition.abatement.as(dateTime) | Condition.abatement.as(Period)", description = "Date-related abatements (dateTime and period)", type = "date") 2736 public static final String SP_ABATEMENT_DATE = "abatement-date"; 2737 /** 2738 * <b>Fluent Client</b> search parameter constant for <b>abatement-date</b> 2739 * <p> 2740 * Description: <b>Date-related abatements (dateTime and period)</b><br> 2741 * Type: <b>date</b><br> 2742 * Path: <b>Condition.abatement[x]</b><br> 2743 * </p> 2744 */ 2745 public static final ca.uhn.fhir.rest.gclient.DateClientParam ABATEMENT_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2746 SP_ABATEMENT_DATE); 2747 2748 /** 2749 * Search parameter: <b>asserter</b> 2750 * <p> 2751 * Description: <b>Person who asserts this condition</b><br> 2752 * Type: <b>reference</b><br> 2753 * Path: <b>Condition.asserter</b><br> 2754 * </p> 2755 */ 2756 @SearchParamDefinition(name = "asserter", path = "Condition.asserter", description = "Person who asserts this condition", type = "reference", providesMembershipIn = { 2757 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2758 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 2759 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2760 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2761 public static final String SP_ASSERTER = "asserter"; 2762 /** 2763 * <b>Fluent Client</b> search parameter constant for <b>asserter</b> 2764 * <p> 2765 * Description: <b>Person who asserts this condition</b><br> 2766 * Type: <b>reference</b><br> 2767 * Path: <b>Condition.asserter</b><br> 2768 * </p> 2769 */ 2770 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ASSERTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2771 SP_ASSERTER); 2772 2773 /** 2774 * Constant for fluent queries to be used to add include statements. Specifies 2775 * the path value of "<b>Condition:asserter</b>". 2776 */ 2777 public static final ca.uhn.fhir.model.api.Include INCLUDE_ASSERTER = new ca.uhn.fhir.model.api.Include( 2778 "Condition:asserter").toLocked(); 2779 2780 /** 2781 * Search parameter: <b>stage</b> 2782 * <p> 2783 * Description: <b>Simple summary (disease specific)</b><br> 2784 * Type: <b>token</b><br> 2785 * Path: <b>Condition.stage.summary</b><br> 2786 * </p> 2787 */ 2788 @SearchParamDefinition(name = "stage", path = "Condition.stage.summary", description = "Simple summary (disease specific)", type = "token") 2789 public static final String SP_STAGE = "stage"; 2790 /** 2791 * <b>Fluent Client</b> search parameter constant for <b>stage</b> 2792 * <p> 2793 * Description: <b>Simple summary (disease specific)</b><br> 2794 * Type: <b>token</b><br> 2795 * Path: <b>Condition.stage.summary</b><br> 2796 * </p> 2797 */ 2798 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STAGE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2799 SP_STAGE); 2800 2801 /** 2802 * Search parameter: <b>abatement-string</b> 2803 * <p> 2804 * Description: <b>Abatement as a string</b><br> 2805 * Type: <b>string</b><br> 2806 * Path: <b>Condition.abatement[x]</b><br> 2807 * </p> 2808 */ 2809 @SearchParamDefinition(name = "abatement-string", path = "Condition.abatement.as(string)", description = "Abatement as a string", type = "string") 2810 public static final String SP_ABATEMENT_STRING = "abatement-string"; 2811 /** 2812 * <b>Fluent Client</b> search parameter constant for <b>abatement-string</b> 2813 * <p> 2814 * Description: <b>Abatement as a string</b><br> 2815 * Type: <b>string</b><br> 2816 * Path: <b>Condition.abatement[x]</b><br> 2817 * </p> 2818 */ 2819 public static final ca.uhn.fhir.rest.gclient.StringClientParam ABATEMENT_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam( 2820 SP_ABATEMENT_STRING); 2821 2822 /** 2823 * Search parameter: <b>patient</b> 2824 * <p> 2825 * Description: <b>Who has the condition?</b><br> 2826 * Type: <b>reference</b><br> 2827 * Path: <b>Condition.subject</b><br> 2828 * </p> 2829 */ 2830 @SearchParamDefinition(name = "patient", path = "Condition.subject.where(resolve() is Patient)", description = "Who has the condition?", type = "reference", providesMembershipIn = { 2831 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2832 public static final String SP_PATIENT = "patient"; 2833 /** 2834 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2835 * <p> 2836 * Description: <b>Who has the condition?</b><br> 2837 * Type: <b>reference</b><br> 2838 * Path: <b>Condition.subject</b><br> 2839 * </p> 2840 */ 2841 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2842 SP_PATIENT); 2843 2844 /** 2845 * Constant for fluent queries to be used to add include statements. Specifies 2846 * the path value of "<b>Condition:patient</b>". 2847 */ 2848 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2849 "Condition:patient").toLocked(); 2850 2851 /** 2852 * Search parameter: <b>onset-age</b> 2853 * <p> 2854 * Description: <b>Onsets as age or age range</b><br> 2855 * Type: <b>quantity</b><br> 2856 * Path: <b>Condition.onset[x]</b><br> 2857 * </p> 2858 */ 2859 @SearchParamDefinition(name = "onset-age", path = "Condition.onset.as(Age) | Condition.onset.as(Range)", description = "Onsets as age or age range", type = "quantity") 2860 public static final String SP_ONSET_AGE = "onset-age"; 2861 /** 2862 * <b>Fluent Client</b> search parameter constant for <b>onset-age</b> 2863 * <p> 2864 * Description: <b>Onsets as age or age range</b><br> 2865 * Type: <b>quantity</b><br> 2866 * Path: <b>Condition.onset[x]</b><br> 2867 * </p> 2868 */ 2869 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ONSET_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2870 SP_ONSET_AGE); 2871 2872 /** 2873 * Search parameter: <b>abatement-age</b> 2874 * <p> 2875 * Description: <b>Abatement as age or age range</b><br> 2876 * Type: <b>quantity</b><br> 2877 * Path: <b>Condition.abatement[x]</b><br> 2878 * </p> 2879 */ 2880 @SearchParamDefinition(name = "abatement-age", path = "Condition.abatement.as(Age) | Condition.abatement.as(Range)", description = "Abatement as age or age range", type = "quantity") 2881 public static final String SP_ABATEMENT_AGE = "abatement-age"; 2882 /** 2883 * <b>Fluent Client</b> search parameter constant for <b>abatement-age</b> 2884 * <p> 2885 * Description: <b>Abatement as age or age range</b><br> 2886 * Type: <b>quantity</b><br> 2887 * Path: <b>Condition.abatement[x]</b><br> 2888 * </p> 2889 */ 2890 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam ABATEMENT_AGE = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2891 SP_ABATEMENT_AGE); 2892 2893 /** 2894 * Search parameter: <b>category</b> 2895 * <p> 2896 * Description: <b>The category of the condition</b><br> 2897 * Type: <b>token</b><br> 2898 * Path: <b>Condition.category</b><br> 2899 * </p> 2900 */ 2901 @SearchParamDefinition(name = "category", path = "Condition.category", description = "The category of the condition", type = "token") 2902 public static final String SP_CATEGORY = "category"; 2903 /** 2904 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2905 * <p> 2906 * Description: <b>The category of the condition</b><br> 2907 * Type: <b>token</b><br> 2908 * Path: <b>Condition.category</b><br> 2909 * </p> 2910 */ 2911 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2912 SP_CATEGORY); 2913 2914 /** 2915 * Search parameter: <b>body-site</b> 2916 * <p> 2917 * Description: <b>Anatomical location, if relevant</b><br> 2918 * Type: <b>token</b><br> 2919 * Path: <b>Condition.bodySite</b><br> 2920 * </p> 2921 */ 2922 @SearchParamDefinition(name = "body-site", path = "Condition.bodySite", description = "Anatomical location, if relevant", type = "token") 2923 public static final String SP_BODY_SITE = "body-site"; 2924 /** 2925 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 2926 * <p> 2927 * Description: <b>Anatomical location, if relevant</b><br> 2928 * Type: <b>token</b><br> 2929 * Path: <b>Condition.bodySite</b><br> 2930 * </p> 2931 */ 2932 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2933 SP_BODY_SITE); 2934 2935}