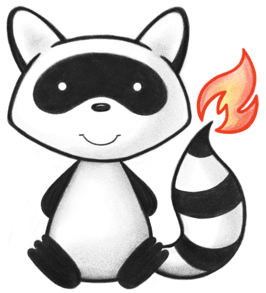
001package org.hl7.fhir.r4.model; 002 003/* 004Copyright (c) 2011+, HL7, Inc. 005All rights reserved. 006 007Redistribution and use in source and binary forms, with or without modification, 008are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032/** 033 * This class is created to help implementers deal with a change to the API that 034 * was made between versions 0.81 and 0.9 035 * 036 * The change is the behaviour of the .getX() where the cardinality of x is 0..1 037 * or 1..1. Before the change, these routines would return null if the object 038 * had not previously been assigned, and after the ' change, they will 039 * automatically create the object if it had not been assigned (unless the 040 * object is polymorphic, in which case, only the type specific getters create 041 * the object) 042 * 043 * When making the transition from the old style to the new style, the main 044 * change is that when testing for presence or abssense of the element, instead 045 * of doing one of these two: 046 * 047 * if (obj.getProperty() == null) if (obj.getProperty() != null) 048 * 049 * you instead do 050 * 051 * if (!obj.hasProperty()) if (obj.hasProperty()) 052 * 053 * or else one of these two: 054 * 055 * if (obj.getProperty().isEmpty()) if (!obj.getProperty().isEmpty()) 056 * 057 * The only way to sort this out is by finding all these things in the code, and 058 * changing them. 059 * 060 * To help with that, you can set the status field of this class to change how 061 * this API behaves. Note: the status value is tied to the way that you program. 062 * The intent of this class is to help make developers the transiition to status 063 * = 0. The old behaviour is status = 2. To make the transition, set the status 064 * code to 1. This way, any time a .getX routine needs to automatically create 065 * an object, an exception will be raised instead. The expected use of this is: 066 * - set status = 1 - test your code (all paths) - when an exception happens, 067 * change the code to use .hasX() or .isEmpty() - when all execution paths don't 068 * raise an exception, set status = 0 - start programming to the new style. 069 * 070 * You can set status = 2 and leave it like that, but this is not compatible 071 * with the utilities and validation code, nor with the HAPI code. So everyone 072 * shoul make this transition 073 * 074 * This is a difficult change to make to an API. Most users should engage with 075 * it as they migrate from DSTU1 to DSTU2, at the same time as they encounter 076 * other changes. This change was made in order to align the two java reference 077 * implementations on a common object model, which is an important outcome that 078 * justifies making this change to implementers (sorry for any pain caused) 079 * 080 * @author Grahame 081 * 082 */ 083public class Configuration { 084 085 private static int status = 0; 086 // 0: auto-create 087 // 1: error 088 // 2: return null 089 090 private static boolean acceptInvalidEnums; 091 092 public static boolean errorOnAutoCreate() { 093 return status == 1; 094 } 095 096 public static boolean doAutoCreate() { 097 return status == 0; 098 } 099 100 public static boolean isAcceptInvalidEnums() { 101 return acceptInvalidEnums; 102 } 103 104 public static void setAcceptInvalidEnums(boolean value) { 105 acceptInvalidEnums = value; 106 } 107 108}