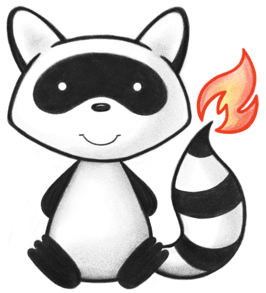
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * Details for all kinds of technology mediated contact points for a person or 045 * organization, including telephone, email, etc. 046 */ 047@DatatypeDef(name = "ContactPoint") 048public class ContactPoint extends Type implements ICompositeType { 049 050 public enum ContactPointSystem { 051 /** 052 * The value is a telephone number used for voice calls. Use of full 053 * international numbers starting with + is recommended to enable automatic 054 * dialing support but not required. 055 */ 056 PHONE, 057 /** 058 * The value is a fax machine. Use of full international numbers starting with + 059 * is recommended to enable automatic dialing support but not required. 060 */ 061 FAX, 062 /** 063 * The value is an email address. 064 */ 065 EMAIL, 066 /** 067 * The value is a pager number. These may be local pager numbers that are only 068 * usable on a particular pager system. 069 */ 070 PAGER, 071 /** 072 * A contact that is not a phone, fax, pager or email address and is expressed 073 * as a URL. This is intended for various institutional or personal contacts 074 * including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for 075 * email addresses. 076 */ 077 URL, 078 /** 079 * A contact that can be used for sending an sms message (e.g. mobile phones, 080 * some landlines). 081 */ 082 SMS, 083 /** 084 * A contact that is not a phone, fax, page or email address and is not 085 * expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for 086 * contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) 087 * Extensions may be used to distinguish "other" contact types. 088 */ 089 OTHER, 090 /** 091 * added to help the parsers with the generic types 092 */ 093 NULL; 094 095 public static ContactPointSystem fromCode(String codeString) throws FHIRException { 096 if (codeString == null || "".equals(codeString)) 097 return null; 098 if ("phone".equals(codeString)) 099 return PHONE; 100 if ("fax".equals(codeString)) 101 return FAX; 102 if ("email".equals(codeString)) 103 return EMAIL; 104 if ("pager".equals(codeString)) 105 return PAGER; 106 if ("url".equals(codeString)) 107 return URL; 108 if ("sms".equals(codeString)) 109 return SMS; 110 if ("other".equals(codeString)) 111 return OTHER; 112 if (Configuration.isAcceptInvalidEnums()) 113 return null; 114 else 115 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 116 } 117 118 public String toCode() { 119 switch (this) { 120 case PHONE: 121 return "phone"; 122 case FAX: 123 return "fax"; 124 case EMAIL: 125 return "email"; 126 case PAGER: 127 return "pager"; 128 case URL: 129 return "url"; 130 case SMS: 131 return "sms"; 132 case OTHER: 133 return "other"; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getSystem() { 142 switch (this) { 143 case PHONE: 144 return "http://hl7.org/fhir/contact-point-system"; 145 case FAX: 146 return "http://hl7.org/fhir/contact-point-system"; 147 case EMAIL: 148 return "http://hl7.org/fhir/contact-point-system"; 149 case PAGER: 150 return "http://hl7.org/fhir/contact-point-system"; 151 case URL: 152 return "http://hl7.org/fhir/contact-point-system"; 153 case SMS: 154 return "http://hl7.org/fhir/contact-point-system"; 155 case OTHER: 156 return "http://hl7.org/fhir/contact-point-system"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDefinition() { 165 switch (this) { 166 case PHONE: 167 return "The value is a telephone number used for voice calls. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 168 case FAX: 169 return "The value is a fax machine. Use of full international numbers starting with + is recommended to enable automatic dialing support but not required."; 170 case EMAIL: 171 return "The value is an email address."; 172 case PAGER: 173 return "The value is a pager number. These may be local pager numbers that are only usable on a particular pager system."; 174 case URL: 175 return "A contact that is not a phone, fax, pager or email address and is expressed as a URL. This is intended for various institutional or personal contacts including web sites, blogs, Skype, Twitter, Facebook, etc. Do not use for email addresses."; 176 case SMS: 177 return "A contact that can be used for sending an sms message (e.g. mobile phones, some landlines)."; 178 case OTHER: 179 return "A contact that is not a phone, fax, page or email address and is not expressible as a URL. E.g. Internal mail address. This SHOULD NOT be used for contacts that are expressible as a URL (e.g. Skype, Twitter, Facebook, etc.) Extensions may be used to distinguish \"other\" contact types."; 180 case NULL: 181 return null; 182 default: 183 return "?"; 184 } 185 } 186 187 public String getDisplay() { 188 switch (this) { 189 case PHONE: 190 return "Phone"; 191 case FAX: 192 return "Fax"; 193 case EMAIL: 194 return "Email"; 195 case PAGER: 196 return "Pager"; 197 case URL: 198 return "URL"; 199 case SMS: 200 return "SMS"; 201 case OTHER: 202 return "Other"; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 } 210 211 public static class ContactPointSystemEnumFactory implements EnumFactory<ContactPointSystem> { 212 public ContactPointSystem fromCode(String codeString) throws IllegalArgumentException { 213 if (codeString == null || "".equals(codeString)) 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("phone".equals(codeString)) 217 return ContactPointSystem.PHONE; 218 if ("fax".equals(codeString)) 219 return ContactPointSystem.FAX; 220 if ("email".equals(codeString)) 221 return ContactPointSystem.EMAIL; 222 if ("pager".equals(codeString)) 223 return ContactPointSystem.PAGER; 224 if ("url".equals(codeString)) 225 return ContactPointSystem.URL; 226 if ("sms".equals(codeString)) 227 return ContactPointSystem.SMS; 228 if ("other".equals(codeString)) 229 return ContactPointSystem.OTHER; 230 throw new IllegalArgumentException("Unknown ContactPointSystem code '" + codeString + "'"); 231 } 232 233 public Enumeration<ContactPointSystem> fromType(PrimitiveType<?> code) throws FHIRException { 234 if (code == null) 235 return null; 236 if (code.isEmpty()) 237 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 238 String codeString = code.asStringValue(); 239 if (codeString == null || "".equals(codeString)) 240 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.NULL, code); 241 if ("phone".equals(codeString)) 242 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PHONE, code); 243 if ("fax".equals(codeString)) 244 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.FAX, code); 245 if ("email".equals(codeString)) 246 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.EMAIL, code); 247 if ("pager".equals(codeString)) 248 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.PAGER, code); 249 if ("url".equals(codeString)) 250 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.URL, code); 251 if ("sms".equals(codeString)) 252 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.SMS, code); 253 if ("other".equals(codeString)) 254 return new Enumeration<ContactPointSystem>(this, ContactPointSystem.OTHER, code); 255 throw new FHIRException("Unknown ContactPointSystem code '" + codeString + "'"); 256 } 257 258 public String toCode(ContactPointSystem code) { 259 if (code == ContactPointSystem.PHONE) 260 return "phone"; 261 if (code == ContactPointSystem.FAX) 262 return "fax"; 263 if (code == ContactPointSystem.EMAIL) 264 return "email"; 265 if (code == ContactPointSystem.PAGER) 266 return "pager"; 267 if (code == ContactPointSystem.URL) 268 return "url"; 269 if (code == ContactPointSystem.SMS) 270 return "sms"; 271 if (code == ContactPointSystem.OTHER) 272 return "other"; 273 return "?"; 274 } 275 276 public String toSystem(ContactPointSystem code) { 277 return code.getSystem(); 278 } 279 } 280 281 public enum ContactPointUse { 282 /** 283 * A communication contact point at a home; attempted contacts for business 284 * purposes might intrude privacy and chances are one will contact family or 285 * other household members instead of the person one wishes to call. Typically 286 * used with urgent cases, or if no other contacts are available. 287 */ 288 HOME, 289 /** 290 * An office contact point. First choice for business related contacts during 291 * business hours. 292 */ 293 WORK, 294 /** 295 * A temporary contact point. The period can provide more detailed information. 296 */ 297 TEMP, 298 /** 299 * This contact point is no longer in use (or was never correct, but retained 300 * for records). 301 */ 302 OLD, 303 /** 304 * A telecommunication device that moves and stays with its owner. May have 305 * characteristics of all other use codes, suitable for urgent matters, not the 306 * first choice for routine business. 307 */ 308 MOBILE, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 314 public static ContactPointUse fromCode(String codeString) throws FHIRException { 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("home".equals(codeString)) 318 return HOME; 319 if ("work".equals(codeString)) 320 return WORK; 321 if ("temp".equals(codeString)) 322 return TEMP; 323 if ("old".equals(codeString)) 324 return OLD; 325 if ("mobile".equals(codeString)) 326 return MOBILE; 327 if (Configuration.isAcceptInvalidEnums()) 328 return null; 329 else 330 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 331 } 332 333 public String toCode() { 334 switch (this) { 335 case HOME: 336 return "home"; 337 case WORK: 338 return "work"; 339 case TEMP: 340 return "temp"; 341 case OLD: 342 return "old"; 343 case MOBILE: 344 return "mobile"; 345 case NULL: 346 return null; 347 default: 348 return "?"; 349 } 350 } 351 352 public String getSystem() { 353 switch (this) { 354 case HOME: 355 return "http://hl7.org/fhir/contact-point-use"; 356 case WORK: 357 return "http://hl7.org/fhir/contact-point-use"; 358 case TEMP: 359 return "http://hl7.org/fhir/contact-point-use"; 360 case OLD: 361 return "http://hl7.org/fhir/contact-point-use"; 362 case MOBILE: 363 return "http://hl7.org/fhir/contact-point-use"; 364 case NULL: 365 return null; 366 default: 367 return "?"; 368 } 369 } 370 371 public String getDefinition() { 372 switch (this) { 373 case HOME: 374 return "A communication contact point at a home; attempted contacts for business purposes might intrude privacy and chances are one will contact family or other household members instead of the person one wishes to call. Typically used with urgent cases, or if no other contacts are available."; 375 case WORK: 376 return "An office contact point. First choice for business related contacts during business hours."; 377 case TEMP: 378 return "A temporary contact point. The period can provide more detailed information."; 379 case OLD: 380 return "This contact point is no longer in use (or was never correct, but retained for records)."; 381 case MOBILE: 382 return "A telecommunication device that moves and stays with its owner. May have characteristics of all other use codes, suitable for urgent matters, not the first choice for routine business."; 383 case NULL: 384 return null; 385 default: 386 return "?"; 387 } 388 } 389 390 public String getDisplay() { 391 switch (this) { 392 case HOME: 393 return "Home"; 394 case WORK: 395 return "Work"; 396 case TEMP: 397 return "Temp"; 398 case OLD: 399 return "Old"; 400 case MOBILE: 401 return "Mobile"; 402 case NULL: 403 return null; 404 default: 405 return "?"; 406 } 407 } 408 } 409 410 public static class ContactPointUseEnumFactory implements EnumFactory<ContactPointUse> { 411 public ContactPointUse fromCode(String codeString) throws IllegalArgumentException { 412 if (codeString == null || "".equals(codeString)) 413 if (codeString == null || "".equals(codeString)) 414 return null; 415 if ("home".equals(codeString)) 416 return ContactPointUse.HOME; 417 if ("work".equals(codeString)) 418 return ContactPointUse.WORK; 419 if ("temp".equals(codeString)) 420 return ContactPointUse.TEMP; 421 if ("old".equals(codeString)) 422 return ContactPointUse.OLD; 423 if ("mobile".equals(codeString)) 424 return ContactPointUse.MOBILE; 425 throw new IllegalArgumentException("Unknown ContactPointUse code '" + codeString + "'"); 426 } 427 428 public Enumeration<ContactPointUse> fromType(PrimitiveType<?> code) throws FHIRException { 429 if (code == null) 430 return null; 431 if (code.isEmpty()) 432 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 433 String codeString = code.asStringValue(); 434 if (codeString == null || "".equals(codeString)) 435 return new Enumeration<ContactPointUse>(this, ContactPointUse.NULL, code); 436 if ("home".equals(codeString)) 437 return new Enumeration<ContactPointUse>(this, ContactPointUse.HOME, code); 438 if ("work".equals(codeString)) 439 return new Enumeration<ContactPointUse>(this, ContactPointUse.WORK, code); 440 if ("temp".equals(codeString)) 441 return new Enumeration<ContactPointUse>(this, ContactPointUse.TEMP, code); 442 if ("old".equals(codeString)) 443 return new Enumeration<ContactPointUse>(this, ContactPointUse.OLD, code); 444 if ("mobile".equals(codeString)) 445 return new Enumeration<ContactPointUse>(this, ContactPointUse.MOBILE, code); 446 throw new FHIRException("Unknown ContactPointUse code '" + codeString + "'"); 447 } 448 449 public String toCode(ContactPointUse code) { 450 if (code == ContactPointUse.HOME) 451 return "home"; 452 if (code == ContactPointUse.WORK) 453 return "work"; 454 if (code == ContactPointUse.TEMP) 455 return "temp"; 456 if (code == ContactPointUse.OLD) 457 return "old"; 458 if (code == ContactPointUse.MOBILE) 459 return "mobile"; 460 return "?"; 461 } 462 463 public String toSystem(ContactPointUse code) { 464 return code.getSystem(); 465 } 466 } 467 468 /** 469 * Telecommunications form for contact point - what communications system is 470 * required to make use of the contact. 471 */ 472 @Child(name = "system", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 473 @Description(shortDefinition = "phone | fax | email | pager | url | sms | other", formalDefinition = "Telecommunications form for contact point - what communications system is required to make use of the contact.") 474 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-system") 475 protected Enumeration<ContactPointSystem> system; 476 477 /** 478 * The actual contact point details, in a form that is meaningful to the 479 * designated communication system (i.e. phone number or email address). 480 */ 481 @Child(name = "value", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 482 @Description(shortDefinition = "The actual contact point details", formalDefinition = "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).") 483 protected StringType value; 484 485 /** 486 * Identifies the purpose for the contact point. 487 */ 488 @Child(name = "use", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 489 @Description(shortDefinition = "home | work | temp | old | mobile - purpose of this contact point", formalDefinition = "Identifies the purpose for the contact point.") 490 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contact-point-use") 491 protected Enumeration<ContactPointUse> use; 492 493 /** 494 * Specifies a preferred order in which to use a set of contacts. ContactPoints 495 * with lower rank values are more preferred than those with higher rank values. 496 */ 497 @Child(name = "rank", type = { PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 498 @Description(shortDefinition = "Specify preferred order of use (1 = highest)", formalDefinition = "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.") 499 protected PositiveIntType rank; 500 501 /** 502 * Time period when the contact point was/is in use. 503 */ 504 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 505 @Description(shortDefinition = "Time period when the contact point was/is in use", formalDefinition = "Time period when the contact point was/is in use.") 506 protected Period period; 507 508 private static final long serialVersionUID = 1509610874L; 509 510 /** 511 * Constructor 512 */ 513 public ContactPoint() { 514 super(); 515 } 516 517 /** 518 * @return {@link #system} (Telecommunications form for contact point - what 519 * communications system is required to make use of the contact.). This 520 * is the underlying object with id, value and extensions. The accessor 521 * "getSystem" gives direct access to the value 522 */ 523 public Enumeration<ContactPointSystem> getSystemElement() { 524 if (this.system == null) 525 if (Configuration.errorOnAutoCreate()) 526 throw new Error("Attempt to auto-create ContactPoint.system"); 527 else if (Configuration.doAutoCreate()) 528 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); // bb 529 return this.system; 530 } 531 532 public boolean hasSystemElement() { 533 return this.system != null && !this.system.isEmpty(); 534 } 535 536 public boolean hasSystem() { 537 return this.system != null && !this.system.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #system} (Telecommunications form for contact point - 542 * what communications system is required to make use of the 543 * contact.). This is the underlying object with id, value and 544 * extensions. The accessor "getSystem" gives direct access to the 545 * value 546 */ 547 public ContactPoint setSystemElement(Enumeration<ContactPointSystem> value) { 548 this.system = value; 549 return this; 550 } 551 552 /** 553 * @return Telecommunications form for contact point - what communications 554 * system is required to make use of the contact. 555 */ 556 public ContactPointSystem getSystem() { 557 return this.system == null ? null : this.system.getValue(); 558 } 559 560 /** 561 * @param value Telecommunications form for contact point - what communications 562 * system is required to make use of the contact. 563 */ 564 public ContactPoint setSystem(ContactPointSystem value) { 565 if (value == null) 566 this.system = null; 567 else { 568 if (this.system == null) 569 this.system = new Enumeration<ContactPointSystem>(new ContactPointSystemEnumFactory()); 570 this.system.setValue(value); 571 } 572 return this; 573 } 574 575 /** 576 * @return {@link #value} (The actual contact point details, in a form that is 577 * meaningful to the designated communication system (i.e. phone number 578 * or email address).). This is the underlying object with id, value and 579 * extensions. The accessor "getValue" gives direct access to the value 580 */ 581 public StringType getValueElement() { 582 if (this.value == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create ContactPoint.value"); 585 else if (Configuration.doAutoCreate()) 586 this.value = new StringType(); // bb 587 return this.value; 588 } 589 590 public boolean hasValueElement() { 591 return this.value != null && !this.value.isEmpty(); 592 } 593 594 public boolean hasValue() { 595 return this.value != null && !this.value.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #value} (The actual contact point details, in a form that 600 * is meaningful to the designated communication system (i.e. phone 601 * number or email address).). This is the underlying object with 602 * id, value and extensions. The accessor "getValue" gives direct 603 * access to the value 604 */ 605 public ContactPoint setValueElement(StringType value) { 606 this.value = value; 607 return this; 608 } 609 610 /** 611 * @return The actual contact point details, in a form that is meaningful to the 612 * designated communication system (i.e. phone number or email address). 613 */ 614 public String getValue() { 615 return this.value == null ? null : this.value.getValue(); 616 } 617 618 /** 619 * @param value The actual contact point details, in a form that is meaningful 620 * to the designated communication system (i.e. phone number or 621 * email address). 622 */ 623 public ContactPoint setValue(String value) { 624 if (Utilities.noString(value)) 625 this.value = null; 626 else { 627 if (this.value == null) 628 this.value = new StringType(); 629 this.value.setValue(value); 630 } 631 return this; 632 } 633 634 /** 635 * @return {@link #use} (Identifies the purpose for the contact point.). This is 636 * the underlying object with id, value and extensions. The accessor 637 * "getUse" gives direct access to the value 638 */ 639 public Enumeration<ContactPointUse> getUseElement() { 640 if (this.use == null) 641 if (Configuration.errorOnAutoCreate()) 642 throw new Error("Attempt to auto-create ContactPoint.use"); 643 else if (Configuration.doAutoCreate()) 644 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); // bb 645 return this.use; 646 } 647 648 public boolean hasUseElement() { 649 return this.use != null && !this.use.isEmpty(); 650 } 651 652 public boolean hasUse() { 653 return this.use != null && !this.use.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #use} (Identifies the purpose for the contact point.). 658 * This is the underlying object with id, value and extensions. The 659 * accessor "getUse" gives direct access to the value 660 */ 661 public ContactPoint setUseElement(Enumeration<ContactPointUse> value) { 662 this.use = value; 663 return this; 664 } 665 666 /** 667 * @return Identifies the purpose for the contact point. 668 */ 669 public ContactPointUse getUse() { 670 return this.use == null ? null : this.use.getValue(); 671 } 672 673 /** 674 * @param value Identifies the purpose for the contact point. 675 */ 676 public ContactPoint setUse(ContactPointUse value) { 677 if (value == null) 678 this.use = null; 679 else { 680 if (this.use == null) 681 this.use = new Enumeration<ContactPointUse>(new ContactPointUseEnumFactory()); 682 this.use.setValue(value); 683 } 684 return this; 685 } 686 687 /** 688 * @return {@link #rank} (Specifies a preferred order in which to use a set of 689 * contacts. ContactPoints with lower rank values are more preferred 690 * than those with higher rank values.). This is the underlying object 691 * with id, value and extensions. The accessor "getRank" gives direct 692 * access to the value 693 */ 694 public PositiveIntType getRankElement() { 695 if (this.rank == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create ContactPoint.rank"); 698 else if (Configuration.doAutoCreate()) 699 this.rank = new PositiveIntType(); // bb 700 return this.rank; 701 } 702 703 public boolean hasRankElement() { 704 return this.rank != null && !this.rank.isEmpty(); 705 } 706 707 public boolean hasRank() { 708 return this.rank != null && !this.rank.isEmpty(); 709 } 710 711 /** 712 * @param value {@link #rank} (Specifies a preferred order in which to use a set 713 * of contacts. ContactPoints with lower rank values are more 714 * preferred than those with higher rank values.). This is the 715 * underlying object with id, value and extensions. The accessor 716 * "getRank" gives direct access to the value 717 */ 718 public ContactPoint setRankElement(PositiveIntType value) { 719 this.rank = value; 720 return this; 721 } 722 723 /** 724 * @return Specifies a preferred order in which to use a set of contacts. 725 * ContactPoints with lower rank values are more preferred than those 726 * with higher rank values. 727 */ 728 public int getRank() { 729 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 730 } 731 732 /** 733 * @param value Specifies a preferred order in which to use a set of contacts. 734 * ContactPoints with lower rank values are more preferred than 735 * those with higher rank values. 736 */ 737 public ContactPoint setRank(int value) { 738 if (this.rank == null) 739 this.rank = new PositiveIntType(); 740 this.rank.setValue(value); 741 return this; 742 } 743 744 /** 745 * @return {@link #period} (Time period when the contact point was/is in use.) 746 */ 747 public Period getPeriod() { 748 if (this.period == null) 749 if (Configuration.errorOnAutoCreate()) 750 throw new Error("Attempt to auto-create ContactPoint.period"); 751 else if (Configuration.doAutoCreate()) 752 this.period = new Period(); // cc 753 return this.period; 754 } 755 756 public boolean hasPeriod() { 757 return this.period != null && !this.period.isEmpty(); 758 } 759 760 /** 761 * @param value {@link #period} (Time period when the contact point was/is in 762 * use.) 763 */ 764 public ContactPoint setPeriod(Period value) { 765 this.period = value; 766 return this; 767 } 768 769 protected void listChildren(List<Property> children) { 770 super.listChildren(children); 771 children.add(new Property("system", "code", 772 "Telecommunications form for contact point - what communications system is required to make use of the contact.", 773 0, 1, system)); 774 children.add(new Property("value", "string", 775 "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 776 0, 1, value)); 777 children.add(new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use)); 778 children.add(new Property("rank", "positiveInt", 779 "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 780 0, 1, rank)); 781 children.add(new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, period)); 782 } 783 784 @Override 785 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 786 switch (_hash) { 787 case -887328209: 788 /* system */ return new Property("system", "code", 789 "Telecommunications form for contact point - what communications system is required to make use of the contact.", 790 0, 1, system); 791 case 111972721: 792 /* value */ return new Property("value", "string", 793 "The actual contact point details, in a form that is meaningful to the designated communication system (i.e. phone number or email address).", 794 0, 1, value); 795 case 116103: 796 /* use */ return new Property("use", "code", "Identifies the purpose for the contact point.", 0, 1, use); 797 case 3492908: 798 /* rank */ return new Property("rank", "positiveInt", 799 "Specifies a preferred order in which to use a set of contacts. ContactPoints with lower rank values are more preferred than those with higher rank values.", 800 0, 1, rank); 801 case -991726143: 802 /* period */ return new Property("period", "Period", "Time period when the contact point was/is in use.", 0, 1, 803 period); 804 default: 805 return super.getNamedProperty(_hash, _name, _checkValid); 806 } 807 808 } 809 810 @Override 811 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 812 switch (hash) { 813 case -887328209: 814 /* system */ return this.system == null ? new Base[0] : new Base[] { this.system }; // Enumeration<ContactPointSystem> 815 case 111972721: 816 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 817 case 116103: 818 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // Enumeration<ContactPointUse> 819 case 3492908: 820 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 821 case -991726143: 822 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 823 default: 824 return super.getProperty(hash, name, checkValid); 825 } 826 827 } 828 829 @Override 830 public Base setProperty(int hash, String name, Base value) throws FHIRException { 831 switch (hash) { 832 case -887328209: // system 833 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 834 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 835 return value; 836 case 111972721: // value 837 this.value = castToString(value); // StringType 838 return value; 839 case 116103: // use 840 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 841 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 842 return value; 843 case 3492908: // rank 844 this.rank = castToPositiveInt(value); // PositiveIntType 845 return value; 846 case -991726143: // period 847 this.period = castToPeriod(value); // Period 848 return value; 849 default: 850 return super.setProperty(hash, name, value); 851 } 852 853 } 854 855 @Override 856 public Base setProperty(String name, Base value) throws FHIRException { 857 if (name.equals("system")) { 858 value = new ContactPointSystemEnumFactory().fromType(castToCode(value)); 859 this.system = (Enumeration) value; // Enumeration<ContactPointSystem> 860 } else if (name.equals("value")) { 861 this.value = castToString(value); // StringType 862 } else if (name.equals("use")) { 863 value = new ContactPointUseEnumFactory().fromType(castToCode(value)); 864 this.use = (Enumeration) value; // Enumeration<ContactPointUse> 865 } else if (name.equals("rank")) { 866 this.rank = castToPositiveInt(value); // PositiveIntType 867 } else if (name.equals("period")) { 868 this.period = castToPeriod(value); // Period 869 } else 870 return super.setProperty(name, value); 871 return value; 872 } 873 874 @Override 875 public Base makeProperty(int hash, String name) throws FHIRException { 876 switch (hash) { 877 case -887328209: 878 return getSystemElement(); 879 case 111972721: 880 return getValueElement(); 881 case 116103: 882 return getUseElement(); 883 case 3492908: 884 return getRankElement(); 885 case -991726143: 886 return getPeriod(); 887 default: 888 return super.makeProperty(hash, name); 889 } 890 891 } 892 893 @Override 894 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 895 switch (hash) { 896 case -887328209: 897 /* system */ return new String[] { "code" }; 898 case 111972721: 899 /* value */ return new String[] { "string" }; 900 case 116103: 901 /* use */ return new String[] { "code" }; 902 case 3492908: 903 /* rank */ return new String[] { "positiveInt" }; 904 case -991726143: 905 /* period */ return new String[] { "Period" }; 906 default: 907 return super.getTypesForProperty(hash, name); 908 } 909 910 } 911 912 @Override 913 public Base addChild(String name) throws FHIRException { 914 if (name.equals("system")) { 915 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.system"); 916 } else if (name.equals("value")) { 917 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.value"); 918 } else if (name.equals("use")) { 919 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.use"); 920 } else if (name.equals("rank")) { 921 throw new FHIRException("Cannot call addChild on a singleton property ContactPoint.rank"); 922 } else if (name.equals("period")) { 923 this.period = new Period(); 924 return this.period; 925 } else 926 return super.addChild(name); 927 } 928 929 public String fhirType() { 930 return "ContactPoint"; 931 932 } 933 934 public ContactPoint copy() { 935 ContactPoint dst = new ContactPoint(); 936 copyValues(dst); 937 return dst; 938 } 939 940 public void copyValues(ContactPoint dst) { 941 super.copyValues(dst); 942 dst.system = system == null ? null : system.copy(); 943 dst.value = value == null ? null : value.copy(); 944 dst.use = use == null ? null : use.copy(); 945 dst.rank = rank == null ? null : rank.copy(); 946 dst.period = period == null ? null : period.copy(); 947 } 948 949 protected ContactPoint typedCopy() { 950 return copy(); 951 } 952 953 @Override 954 public boolean equalsDeep(Base other_) { 955 if (!super.equalsDeep(other_)) 956 return false; 957 if (!(other_ instanceof ContactPoint)) 958 return false; 959 ContactPoint o = (ContactPoint) other_; 960 return compareDeep(system, o.system, true) && compareDeep(value, o.value, true) && compareDeep(use, o.use, true) 961 && compareDeep(rank, o.rank, true) && compareDeep(period, o.period, true); 962 } 963 964 @Override 965 public boolean equalsShallow(Base other_) { 966 if (!super.equalsShallow(other_)) 967 return false; 968 if (!(other_ instanceof ContactPoint)) 969 return false; 970 ContactPoint o = (ContactPoint) other_; 971 return compareValues(system, o.system, true) && compareValues(value, o.value, true) 972 && compareValues(use, o.use, true) && compareValues(rank, o.rank, true); 973 } 974 975 public boolean isEmpty() { 976 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, value, use, rank, period); 977 } 978 979}