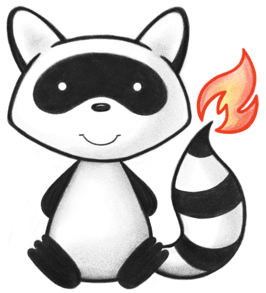
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * Legally enforceable, formally recorded unilateral or bilateral directive 051 * i.e., a policy or agreement. 052 */ 053@ResourceDef(name = "Contract", profile = "http://hl7.org/fhir/StructureDefinition/Contract") 054public class Contract extends DomainResource { 055 056 public enum ContractStatus { 057 /** 058 * Contract is augmented with additional information to correct errors in a 059 * predecessor or to updated values in a predecessor. Usage: Contract altered 060 * within effective time. Precedence Order = 9. Comparable FHIR and v.3 status 061 * codes: revised; replaced. 062 */ 063 AMENDED, 064 /** 065 * Contract is augmented with additional information that was missing from a 066 * predecessor Contract. Usage: Contract altered within effective time. 067 * Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, 068 * replaced. 069 */ 070 APPENDED, 071 /** 072 * Contract is terminated due to failure of the Grantor and/or the Grantee to 073 * fulfil one or more contract provisions. Usage: Abnormal contract termination. 074 * Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; 075 * aborted. 076 */ 077 CANCELLED, 078 /** 079 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil 080 * contract provision(s). E.g., Grantee complaint about Grantor's failure to 081 * comply with contract provisions. Usage: Contract pended. Precedence Order = 082 * 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 083 */ 084 DISPUTED, 085 /** 086 * Contract was created in error. No Precedence Order. Status may be applied to 087 * a Contract with any status. 088 */ 089 ENTEREDINERROR, 090 /** 091 * Contract execution pending; may be executed when either the Grantor or the 092 * Grantee accepts the contract provisions by signing. I.e., where either the 093 * Grantor or the Grantee has signed, but not both. E.g., when an insurance 094 * applicant signs the insurers application, which references the policy. Usage: 095 * Optional first step of contract execution activity. May be skipped and 096 * contracting activity moves directly to executed state. Precedence Order = 3. 097 * Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; 098 * active. 099 */ 100 EXECUTABLE, 101 /** 102 * Contract is activated for period stipulated when both the Grantor and Grantee 103 * have signed it. Usage: Required state for normal completion of contracting 104 * activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: 105 * accepted; completed. 106 */ 107 EXECUTED, 108 /** 109 * Contract execution is suspended while either or both the Grantor and Grantee 110 * propose and consider new or revised contract provisions. I.e., where the 111 * party which has not signed proposes changes to the terms. E .g., a life 112 * insurer declines to agree to the signed application because the life insurer 113 * has evidence that the applicant, who asserted to being younger or a 114 * non-smoker to get a lower premium rate - but offers instead to agree to a 115 * higher premium based on the applicants actual age or smoking status. Usage: 116 * Optional contract activity between executable and executed state. Precedence 117 * Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 118 */ 119 NEGOTIABLE, 120 /** 121 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract 122 * hard copy or electronic 'template', 'form' or 'application'. E.g., health 123 * insurance application; consent directive form. Usage: Beginning of contract 124 * negotiation, which may have been completed as a precondition because used for 125 * 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: 126 * requested; new. 127 */ 128 OFFERED, 129 /** 130 * Contract template is available as the basis for an application or offer by 131 * the Grantor or Grantee. E.g., health insurance policy; consent directive 132 * policy. Usage: Required initial contract activity, which may have been 133 * completed as a precondition because used for 0..* contracts. Precedence Order 134 * = 1. Comparable FHIR and v.3 status codes: proposed; intended. 135 */ 136 POLICY, 137 /** 138 * Execution of the Contract is not completed because either or both the Grantor 139 * and Grantee decline to accept some or all of the contract provisions. Usage: 140 * Optional contract activity between executable and abnormal termination. 141 * Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; 142 * cancelled. 143 */ 144 REJECTED, 145 /** 146 * Beginning of a successor Contract at the termination of predecessor Contract 147 * lifecycle. Usage: Follows termination of a preceding Contract that has 148 * reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 149 * status codes: superseded. 150 */ 151 RENEWED, 152 /** 153 * A Contract that is rescinded. May be required prior to replacing with an 154 * updated Contract. Comparable FHIR and v.3 status codes: nullified. 155 */ 156 REVOKED, 157 /** 158 * Contract is reactivated after being pended because of faulty execution. 159 * *E.g., competency of the signer(s), or where the policy is substantially 160 * different from and did not accompany the application/form so that the 161 * applicant could not compare them. Aka - ''reactivated''. Usage: Optional 162 * stage where a pended contract is reactivated. Precedence Order = 8. 163 * Comparable FHIR and v.3 status codes: reactivated. 164 */ 165 RESOLVED, 166 /** 167 * Contract reaches its expiry date. It might or might not be renewed or 168 * renegotiated. Usage: Normal end of contract period. Precedence Order = 12. 169 * Comparable FHIR and v.3 status codes: Obsoleted. 170 */ 171 TERMINATED, 172 /** 173 * added to help the parsers with the generic types 174 */ 175 NULL; 176 177 public static ContractStatus fromCode(String codeString) throws FHIRException { 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("amended".equals(codeString)) 181 return AMENDED; 182 if ("appended".equals(codeString)) 183 return APPENDED; 184 if ("cancelled".equals(codeString)) 185 return CANCELLED; 186 if ("disputed".equals(codeString)) 187 return DISPUTED; 188 if ("entered-in-error".equals(codeString)) 189 return ENTEREDINERROR; 190 if ("executable".equals(codeString)) 191 return EXECUTABLE; 192 if ("executed".equals(codeString)) 193 return EXECUTED; 194 if ("negotiable".equals(codeString)) 195 return NEGOTIABLE; 196 if ("offered".equals(codeString)) 197 return OFFERED; 198 if ("policy".equals(codeString)) 199 return POLICY; 200 if ("rejected".equals(codeString)) 201 return REJECTED; 202 if ("renewed".equals(codeString)) 203 return RENEWED; 204 if ("revoked".equals(codeString)) 205 return REVOKED; 206 if ("resolved".equals(codeString)) 207 return RESOLVED; 208 if ("terminated".equals(codeString)) 209 return TERMINATED; 210 if (Configuration.isAcceptInvalidEnums()) 211 return null; 212 else 213 throw new FHIRException("Unknown ContractStatus code '" + codeString + "'"); 214 } 215 216 public String toCode() { 217 switch (this) { 218 case AMENDED: 219 return "amended"; 220 case APPENDED: 221 return "appended"; 222 case CANCELLED: 223 return "cancelled"; 224 case DISPUTED: 225 return "disputed"; 226 case ENTEREDINERROR: 227 return "entered-in-error"; 228 case EXECUTABLE: 229 return "executable"; 230 case EXECUTED: 231 return "executed"; 232 case NEGOTIABLE: 233 return "negotiable"; 234 case OFFERED: 235 return "offered"; 236 case POLICY: 237 return "policy"; 238 case REJECTED: 239 return "rejected"; 240 case RENEWED: 241 return "renewed"; 242 case REVOKED: 243 return "revoked"; 244 case RESOLVED: 245 return "resolved"; 246 case TERMINATED: 247 return "terminated"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255 public String getSystem() { 256 switch (this) { 257 case AMENDED: 258 return "http://hl7.org/fhir/contract-status"; 259 case APPENDED: 260 return "http://hl7.org/fhir/contract-status"; 261 case CANCELLED: 262 return "http://hl7.org/fhir/contract-status"; 263 case DISPUTED: 264 return "http://hl7.org/fhir/contract-status"; 265 case ENTEREDINERROR: 266 return "http://hl7.org/fhir/contract-status"; 267 case EXECUTABLE: 268 return "http://hl7.org/fhir/contract-status"; 269 case EXECUTED: 270 return "http://hl7.org/fhir/contract-status"; 271 case NEGOTIABLE: 272 return "http://hl7.org/fhir/contract-status"; 273 case OFFERED: 274 return "http://hl7.org/fhir/contract-status"; 275 case POLICY: 276 return "http://hl7.org/fhir/contract-status"; 277 case REJECTED: 278 return "http://hl7.org/fhir/contract-status"; 279 case RENEWED: 280 return "http://hl7.org/fhir/contract-status"; 281 case REVOKED: 282 return "http://hl7.org/fhir/contract-status"; 283 case RESOLVED: 284 return "http://hl7.org/fhir/contract-status"; 285 case TERMINATED: 286 return "http://hl7.org/fhir/contract-status"; 287 case NULL: 288 return null; 289 default: 290 return "?"; 291 } 292 } 293 294 public String getDefinition() { 295 switch (this) { 296 case AMENDED: 297 return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 298 case APPENDED: 299 return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 300 case CANCELLED: 301 return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 302 case DISPUTED: 303 return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 304 case ENTEREDINERROR: 305 return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 306 case EXECUTABLE: 307 return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 308 case EXECUTED: 309 return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 310 case NEGOTIABLE: 311 return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 312 case OFFERED: 313 return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 314 case POLICY: 315 return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 316 case REJECTED: 317 return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 318 case RENEWED: 319 return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 320 case REVOKED: 321 return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 322 case RESOLVED: 323 return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 324 case TERMINATED: 325 return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 326 case NULL: 327 return null; 328 default: 329 return "?"; 330 } 331 } 332 333 public String getDisplay() { 334 switch (this) { 335 case AMENDED: 336 return "Amended"; 337 case APPENDED: 338 return "Appended"; 339 case CANCELLED: 340 return "Cancelled"; 341 case DISPUTED: 342 return "Disputed"; 343 case ENTEREDINERROR: 344 return "Entered in Error"; 345 case EXECUTABLE: 346 return "Executable"; 347 case EXECUTED: 348 return "Executed"; 349 case NEGOTIABLE: 350 return "Negotiable"; 351 case OFFERED: 352 return "Offered"; 353 case POLICY: 354 return "Policy"; 355 case REJECTED: 356 return "Rejected"; 357 case RENEWED: 358 return "Renewed"; 359 case REVOKED: 360 return "Revoked"; 361 case RESOLVED: 362 return "Resolved"; 363 case TERMINATED: 364 return "Terminated"; 365 case NULL: 366 return null; 367 default: 368 return "?"; 369 } 370 } 371 } 372 373 public static class ContractStatusEnumFactory implements EnumFactory<ContractStatus> { 374 public ContractStatus fromCode(String codeString) throws IllegalArgumentException { 375 if (codeString == null || "".equals(codeString)) 376 if (codeString == null || "".equals(codeString)) 377 return null; 378 if ("amended".equals(codeString)) 379 return ContractStatus.AMENDED; 380 if ("appended".equals(codeString)) 381 return ContractStatus.APPENDED; 382 if ("cancelled".equals(codeString)) 383 return ContractStatus.CANCELLED; 384 if ("disputed".equals(codeString)) 385 return ContractStatus.DISPUTED; 386 if ("entered-in-error".equals(codeString)) 387 return ContractStatus.ENTEREDINERROR; 388 if ("executable".equals(codeString)) 389 return ContractStatus.EXECUTABLE; 390 if ("executed".equals(codeString)) 391 return ContractStatus.EXECUTED; 392 if ("negotiable".equals(codeString)) 393 return ContractStatus.NEGOTIABLE; 394 if ("offered".equals(codeString)) 395 return ContractStatus.OFFERED; 396 if ("policy".equals(codeString)) 397 return ContractStatus.POLICY; 398 if ("rejected".equals(codeString)) 399 return ContractStatus.REJECTED; 400 if ("renewed".equals(codeString)) 401 return ContractStatus.RENEWED; 402 if ("revoked".equals(codeString)) 403 return ContractStatus.REVOKED; 404 if ("resolved".equals(codeString)) 405 return ContractStatus.RESOLVED; 406 if ("terminated".equals(codeString)) 407 return ContractStatus.TERMINATED; 408 throw new IllegalArgumentException("Unknown ContractStatus code '" + codeString + "'"); 409 } 410 411 public Enumeration<ContractStatus> fromType(PrimitiveType<?> code) throws FHIRException { 412 if (code == null) 413 return null; 414 if (code.isEmpty()) 415 return new Enumeration<ContractStatus>(this, ContractStatus.NULL, code); 416 String codeString = code.asStringValue(); 417 if (codeString == null || "".equals(codeString)) 418 return new Enumeration<ContractStatus>(this, ContractStatus.NULL, code); 419 if ("amended".equals(codeString)) 420 return new Enumeration<ContractStatus>(this, ContractStatus.AMENDED, code); 421 if ("appended".equals(codeString)) 422 return new Enumeration<ContractStatus>(this, ContractStatus.APPENDED, code); 423 if ("cancelled".equals(codeString)) 424 return new Enumeration<ContractStatus>(this, ContractStatus.CANCELLED, code); 425 if ("disputed".equals(codeString)) 426 return new Enumeration<ContractStatus>(this, ContractStatus.DISPUTED, code); 427 if ("entered-in-error".equals(codeString)) 428 return new Enumeration<ContractStatus>(this, ContractStatus.ENTEREDINERROR, code); 429 if ("executable".equals(codeString)) 430 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTABLE, code); 431 if ("executed".equals(codeString)) 432 return new Enumeration<ContractStatus>(this, ContractStatus.EXECUTED, code); 433 if ("negotiable".equals(codeString)) 434 return new Enumeration<ContractStatus>(this, ContractStatus.NEGOTIABLE, code); 435 if ("offered".equals(codeString)) 436 return new Enumeration<ContractStatus>(this, ContractStatus.OFFERED, code); 437 if ("policy".equals(codeString)) 438 return new Enumeration<ContractStatus>(this, ContractStatus.POLICY, code); 439 if ("rejected".equals(codeString)) 440 return new Enumeration<ContractStatus>(this, ContractStatus.REJECTED, code); 441 if ("renewed".equals(codeString)) 442 return new Enumeration<ContractStatus>(this, ContractStatus.RENEWED, code); 443 if ("revoked".equals(codeString)) 444 return new Enumeration<ContractStatus>(this, ContractStatus.REVOKED, code); 445 if ("resolved".equals(codeString)) 446 return new Enumeration<ContractStatus>(this, ContractStatus.RESOLVED, code); 447 if ("terminated".equals(codeString)) 448 return new Enumeration<ContractStatus>(this, ContractStatus.TERMINATED, code); 449 throw new FHIRException("Unknown ContractStatus code '" + codeString + "'"); 450 } 451 452 public String toCode(ContractStatus code) { 453 if (code == ContractStatus.NULL) 454 return null; 455 if (code == ContractStatus.AMENDED) 456 return "amended"; 457 if (code == ContractStatus.APPENDED) 458 return "appended"; 459 if (code == ContractStatus.CANCELLED) 460 return "cancelled"; 461 if (code == ContractStatus.DISPUTED) 462 return "disputed"; 463 if (code == ContractStatus.ENTEREDINERROR) 464 return "entered-in-error"; 465 if (code == ContractStatus.EXECUTABLE) 466 return "executable"; 467 if (code == ContractStatus.EXECUTED) 468 return "executed"; 469 if (code == ContractStatus.NEGOTIABLE) 470 return "negotiable"; 471 if (code == ContractStatus.OFFERED) 472 return "offered"; 473 if (code == ContractStatus.POLICY) 474 return "policy"; 475 if (code == ContractStatus.REJECTED) 476 return "rejected"; 477 if (code == ContractStatus.RENEWED) 478 return "renewed"; 479 if (code == ContractStatus.REVOKED) 480 return "revoked"; 481 if (code == ContractStatus.RESOLVED) 482 return "resolved"; 483 if (code == ContractStatus.TERMINATED) 484 return "terminated"; 485 return "?"; 486 } 487 488 public String toSystem(ContractStatus code) { 489 return code.getSystem(); 490 } 491 } 492 493 public enum ContractPublicationStatus { 494 /** 495 * Contract is augmented with additional information to correct errors in a 496 * predecessor or to updated values in a predecessor. Usage: Contract altered 497 * within effective time. Precedence Order = 9. Comparable FHIR and v.3 status 498 * codes: revised; replaced. 499 */ 500 AMENDED, 501 /** 502 * Contract is augmented with additional information that was missing from a 503 * predecessor Contract. Usage: Contract altered within effective time. 504 * Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, 505 * replaced. 506 */ 507 APPENDED, 508 /** 509 * Contract is terminated due to failure of the Grantor and/or the Grantee to 510 * fulfil one or more contract provisions. Usage: Abnormal contract termination. 511 * Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; 512 * aborted. 513 */ 514 CANCELLED, 515 /** 516 * Contract is pended to rectify failure of the Grantor or the Grantee to fulfil 517 * contract provision(s). E.g., Grantee complaint about Grantor's failure to 518 * comply with contract provisions. Usage: Contract pended. Precedence Order = 519 * 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended. 520 */ 521 DISPUTED, 522 /** 523 * Contract was created in error. No Precedence Order. Status may be applied to 524 * a Contract with any status. 525 */ 526 ENTEREDINERROR, 527 /** 528 * Contract execution pending; may be executed when either the Grantor or the 529 * Grantee accepts the contract provisions by signing. I.e., where either the 530 * Grantor or the Grantee has signed, but not both. E.g., when an insurance 531 * applicant signs the insurers application, which references the policy. Usage: 532 * Optional first step of contract execution activity. May be skipped and 533 * contracting activity moves directly to executed state. Precedence Order = 3. 534 * Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; 535 * active. 536 */ 537 EXECUTABLE, 538 /** 539 * Contract is activated for period stipulated when both the Grantor and Grantee 540 * have signed it. Usage: Required state for normal completion of contracting 541 * activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: 542 * accepted; completed. 543 */ 544 EXECUTED, 545 /** 546 * Contract execution is suspended while either or both the Grantor and Grantee 547 * propose and consider new or revised contract provisions. I.e., where the 548 * party which has not signed proposes changes to the terms. E .g., a life 549 * insurer declines to agree to the signed application because the life insurer 550 * has evidence that the applicant, who asserted to being younger or a 551 * non-smoker to get a lower premium rate - but offers instead to agree to a 552 * higher premium based on the applicants actual age or smoking status. Usage: 553 * Optional contract activity between executable and executed state. Precedence 554 * Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held. 555 */ 556 NEGOTIABLE, 557 /** 558 * Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract 559 * hard copy or electronic 'template', 'form' or 'application'. E.g., health 560 * insurance application; consent directive form. Usage: Beginning of contract 561 * negotiation, which may have been completed as a precondition because used for 562 * 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: 563 * requested; new. 564 */ 565 OFFERED, 566 /** 567 * Contract template is available as the basis for an application or offer by 568 * the Grantor or Grantee. E.g., health insurance policy; consent directive 569 * policy. Usage: Required initial contract activity, which may have been 570 * completed as a precondition because used for 0..* contracts. Precedence Order 571 * = 1. Comparable FHIR and v.3 status codes: proposed; intended. 572 */ 573 POLICY, 574 /** 575 * Execution of the Contract is not completed because either or both the Grantor 576 * and Grantee decline to accept some or all of the contract provisions. Usage: 577 * Optional contract activity between executable and abnormal termination. 578 * Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; 579 * cancelled. 580 */ 581 REJECTED, 582 /** 583 * Beginning of a successor Contract at the termination of predecessor Contract 584 * lifecycle. Usage: Follows termination of a preceding Contract that has 585 * reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 586 * status codes: superseded. 587 */ 588 RENEWED, 589 /** 590 * A Contract that is rescinded. May be required prior to replacing with an 591 * updated Contract. Comparable FHIR and v.3 status codes: nullified. 592 */ 593 REVOKED, 594 /** 595 * Contract is reactivated after being pended because of faulty execution. 596 * *E.g., competency of the signer(s), or where the policy is substantially 597 * different from and did not accompany the application/form so that the 598 * applicant could not compare them. Aka - ''reactivated''. Usage: Optional 599 * stage where a pended contract is reactivated. Precedence Order = 8. 600 * Comparable FHIR and v.3 status codes: reactivated. 601 */ 602 RESOLVED, 603 /** 604 * Contract reaches its expiry date. It might or might not be renewed or 605 * renegotiated. Usage: Normal end of contract period. Precedence Order = 12. 606 * Comparable FHIR and v.3 status codes: Obsoleted. 607 */ 608 TERMINATED, 609 /** 610 * added to help the parsers with the generic types 611 */ 612 NULL; 613 614 public static ContractPublicationStatus fromCode(String codeString) throws FHIRException { 615 if (codeString == null || "".equals(codeString)) 616 return null; 617 if ("amended".equals(codeString)) 618 return AMENDED; 619 if ("appended".equals(codeString)) 620 return APPENDED; 621 if ("cancelled".equals(codeString)) 622 return CANCELLED; 623 if ("disputed".equals(codeString)) 624 return DISPUTED; 625 if ("entered-in-error".equals(codeString)) 626 return ENTEREDINERROR; 627 if ("executable".equals(codeString)) 628 return EXECUTABLE; 629 if ("executed".equals(codeString)) 630 return EXECUTED; 631 if ("negotiable".equals(codeString)) 632 return NEGOTIABLE; 633 if ("offered".equals(codeString)) 634 return OFFERED; 635 if ("policy".equals(codeString)) 636 return POLICY; 637 if ("rejected".equals(codeString)) 638 return REJECTED; 639 if ("renewed".equals(codeString)) 640 return RENEWED; 641 if ("revoked".equals(codeString)) 642 return REVOKED; 643 if ("resolved".equals(codeString)) 644 return RESOLVED; 645 if ("terminated".equals(codeString)) 646 return TERMINATED; 647 if (Configuration.isAcceptInvalidEnums()) 648 return null; 649 else 650 throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'"); 651 } 652 653 public String toCode() { 654 switch (this) { 655 case AMENDED: 656 return "amended"; 657 case APPENDED: 658 return "appended"; 659 case CANCELLED: 660 return "cancelled"; 661 case DISPUTED: 662 return "disputed"; 663 case ENTEREDINERROR: 664 return "entered-in-error"; 665 case EXECUTABLE: 666 return "executable"; 667 case EXECUTED: 668 return "executed"; 669 case NEGOTIABLE: 670 return "negotiable"; 671 case OFFERED: 672 return "offered"; 673 case POLICY: 674 return "policy"; 675 case REJECTED: 676 return "rejected"; 677 case RENEWED: 678 return "renewed"; 679 case REVOKED: 680 return "revoked"; 681 case RESOLVED: 682 return "resolved"; 683 case TERMINATED: 684 return "terminated"; 685 case NULL: 686 return null; 687 default: 688 return "?"; 689 } 690 } 691 692 public String getSystem() { 693 switch (this) { 694 case AMENDED: 695 return "http://hl7.org/fhir/contract-publicationstatus"; 696 case APPENDED: 697 return "http://hl7.org/fhir/contract-publicationstatus"; 698 case CANCELLED: 699 return "http://hl7.org/fhir/contract-publicationstatus"; 700 case DISPUTED: 701 return "http://hl7.org/fhir/contract-publicationstatus"; 702 case ENTEREDINERROR: 703 return "http://hl7.org/fhir/contract-publicationstatus"; 704 case EXECUTABLE: 705 return "http://hl7.org/fhir/contract-publicationstatus"; 706 case EXECUTED: 707 return "http://hl7.org/fhir/contract-publicationstatus"; 708 case NEGOTIABLE: 709 return "http://hl7.org/fhir/contract-publicationstatus"; 710 case OFFERED: 711 return "http://hl7.org/fhir/contract-publicationstatus"; 712 case POLICY: 713 return "http://hl7.org/fhir/contract-publicationstatus"; 714 case REJECTED: 715 return "http://hl7.org/fhir/contract-publicationstatus"; 716 case RENEWED: 717 return "http://hl7.org/fhir/contract-publicationstatus"; 718 case REVOKED: 719 return "http://hl7.org/fhir/contract-publicationstatus"; 720 case RESOLVED: 721 return "http://hl7.org/fhir/contract-publicationstatus"; 722 case TERMINATED: 723 return "http://hl7.org/fhir/contract-publicationstatus"; 724 case NULL: 725 return null; 726 default: 727 return "?"; 728 } 729 } 730 731 public String getDefinition() { 732 switch (this) { 733 case AMENDED: 734 return "Contract is augmented with additional information to correct errors in a predecessor or to updated values in a predecessor. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: revised; replaced."; 735 case APPENDED: 736 return "Contract is augmented with additional information that was missing from a predecessor Contract. Usage: Contract altered within effective time. Precedence Order = 9. Comparable FHIR and v.3 status codes: updated, replaced."; 737 case CANCELLED: 738 return "Contract is terminated due to failure of the Grantor and/or the Grantee to fulfil one or more contract provisions. Usage: Abnormal contract termination. Precedence Order = 10. Comparable FHIR and v.3 status codes: stopped; failed; aborted."; 739 case DISPUTED: 740 return "Contract is pended to rectify failure of the Grantor or the Grantee to fulfil contract provision(s). E.g., Grantee complaint about Grantor's failure to comply with contract provisions. Usage: Contract pended. Precedence Order = 7. Comparable FHIR and v.3 status codes: on hold; pended; suspended."; 741 case ENTEREDINERROR: 742 return "Contract was created in error. No Precedence Order. Status may be applied to a Contract with any status."; 743 case EXECUTABLE: 744 return "Contract execution pending; may be executed when either the Grantor or the Grantee accepts the contract provisions by signing. I.e., where either the Grantor or the Grantee has signed, but not both. E.g., when an insurance applicant signs the insurers application, which references the policy. Usage: Optional first step of contract execution activity. May be skipped and contracting activity moves directly to executed state. Precedence Order = 3. Comparable FHIR and v.3 status codes: draft; preliminary; planned; intended; active."; 745 case EXECUTED: 746 return "Contract is activated for period stipulated when both the Grantor and Grantee have signed it. Usage: Required state for normal completion of contracting activity. Precedence Order = 6. Comparable FHIR and v.3 status codes: accepted; completed."; 747 case NEGOTIABLE: 748 return "Contract execution is suspended while either or both the Grantor and Grantee propose and consider new or revised contract provisions. I.e., where the party which has not signed proposes changes to the terms. E .g., a life insurer declines to agree to the signed application because the life insurer has evidence that the applicant, who asserted to being younger or a non-smoker to get a lower premium rate - but offers instead to agree to a higher premium based on the applicants actual age or smoking status. Usage: Optional contract activity between executable and executed state. Precedence Order = 4. Comparable FHIR and v.3 status codes: in progress; review; held."; 749 case OFFERED: 750 return "Contract is a proposal by either the Grantor or the Grantee. Aka - A Contract hard copy or electronic 'template', 'form' or 'application'. E.g., health insurance application; consent directive form. Usage: Beginning of contract negotiation, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 2. Comparable FHIR and v.3 status codes: requested; new."; 751 case POLICY: 752 return "Contract template is available as the basis for an application or offer by the Grantor or Grantee. E.g., health insurance policy; consent directive policy. Usage: Required initial contract activity, which may have been completed as a precondition because used for 0..* contracts. Precedence Order = 1. Comparable FHIR and v.3 status codes: proposed; intended."; 753 case REJECTED: 754 return " Execution of the Contract is not completed because either or both the Grantor and Grantee decline to accept some or all of the contract provisions. Usage: Optional contract activity between executable and abnormal termination. Precedence Order = 5. Comparable FHIR and v.3 status codes: stopped; cancelled."; 755 case RENEWED: 756 return "Beginning of a successor Contract at the termination of predecessor Contract lifecycle. Usage: Follows termination of a preceding Contract that has reached its expiry date. Precedence Order = 13. Comparable FHIR and v.3 status codes: superseded."; 757 case REVOKED: 758 return "A Contract that is rescinded. May be required prior to replacing with an updated Contract. Comparable FHIR and v.3 status codes: nullified."; 759 case RESOLVED: 760 return "Contract is reactivated after being pended because of faulty execution. *E.g., competency of the signer(s), or where the policy is substantially different from and did not accompany the application/form so that the applicant could not compare them. Aka - ''reactivated''. Usage: Optional stage where a pended contract is reactivated. Precedence Order = 8. Comparable FHIR and v.3 status codes: reactivated."; 761 case TERMINATED: 762 return "Contract reaches its expiry date. It might or might not be renewed or renegotiated. Usage: Normal end of contract period. Precedence Order = 12. Comparable FHIR and v.3 status codes: Obsoleted."; 763 case NULL: 764 return null; 765 default: 766 return "?"; 767 } 768 } 769 770 public String getDisplay() { 771 switch (this) { 772 case AMENDED: 773 return "Amended"; 774 case APPENDED: 775 return "Appended"; 776 case CANCELLED: 777 return "Cancelled"; 778 case DISPUTED: 779 return "Disputed"; 780 case ENTEREDINERROR: 781 return "Entered in Error"; 782 case EXECUTABLE: 783 return "Executable"; 784 case EXECUTED: 785 return "Executed"; 786 case NEGOTIABLE: 787 return "Negotiable"; 788 case OFFERED: 789 return "Offered"; 790 case POLICY: 791 return "Policy"; 792 case REJECTED: 793 return "Rejected"; 794 case RENEWED: 795 return "Renewed"; 796 case REVOKED: 797 return "Revoked"; 798 case RESOLVED: 799 return "Resolved"; 800 case TERMINATED: 801 return "Terminated"; 802 case NULL: 803 return null; 804 default: 805 return "?"; 806 } 807 } 808 } 809 810 public static class ContractPublicationStatusEnumFactory implements EnumFactory<ContractPublicationStatus> { 811 public ContractPublicationStatus fromCode(String codeString) throws IllegalArgumentException { 812 if (codeString == null || "".equals(codeString)) 813 if (codeString == null || "".equals(codeString)) 814 return null; 815 if ("amended".equals(codeString)) 816 return ContractPublicationStatus.AMENDED; 817 if ("appended".equals(codeString)) 818 return ContractPublicationStatus.APPENDED; 819 if ("cancelled".equals(codeString)) 820 return ContractPublicationStatus.CANCELLED; 821 if ("disputed".equals(codeString)) 822 return ContractPublicationStatus.DISPUTED; 823 if ("entered-in-error".equals(codeString)) 824 return ContractPublicationStatus.ENTEREDINERROR; 825 if ("executable".equals(codeString)) 826 return ContractPublicationStatus.EXECUTABLE; 827 if ("executed".equals(codeString)) 828 return ContractPublicationStatus.EXECUTED; 829 if ("negotiable".equals(codeString)) 830 return ContractPublicationStatus.NEGOTIABLE; 831 if ("offered".equals(codeString)) 832 return ContractPublicationStatus.OFFERED; 833 if ("policy".equals(codeString)) 834 return ContractPublicationStatus.POLICY; 835 if ("rejected".equals(codeString)) 836 return ContractPublicationStatus.REJECTED; 837 if ("renewed".equals(codeString)) 838 return ContractPublicationStatus.RENEWED; 839 if ("revoked".equals(codeString)) 840 return ContractPublicationStatus.REVOKED; 841 if ("resolved".equals(codeString)) 842 return ContractPublicationStatus.RESOLVED; 843 if ("terminated".equals(codeString)) 844 return ContractPublicationStatus.TERMINATED; 845 throw new IllegalArgumentException("Unknown ContractPublicationStatus code '" + codeString + "'"); 846 } 847 848 public Enumeration<ContractPublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 849 if (code == null) 850 return null; 851 if (code.isEmpty()) 852 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NULL, code); 853 String codeString = code.asStringValue(); 854 if (codeString == null || "".equals(codeString)) 855 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NULL, code); 856 if ("amended".equals(codeString)) 857 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.AMENDED, code); 858 if ("appended".equals(codeString)) 859 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.APPENDED, code); 860 if ("cancelled".equals(codeString)) 861 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.CANCELLED, code); 862 if ("disputed".equals(codeString)) 863 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.DISPUTED, code); 864 if ("entered-in-error".equals(codeString)) 865 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.ENTEREDINERROR, code); 866 if ("executable".equals(codeString)) 867 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.EXECUTABLE, code); 868 if ("executed".equals(codeString)) 869 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.EXECUTED, code); 870 if ("negotiable".equals(codeString)) 871 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.NEGOTIABLE, code); 872 if ("offered".equals(codeString)) 873 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.OFFERED, code); 874 if ("policy".equals(codeString)) 875 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.POLICY, code); 876 if ("rejected".equals(codeString)) 877 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.REJECTED, code); 878 if ("renewed".equals(codeString)) 879 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.RENEWED, code); 880 if ("revoked".equals(codeString)) 881 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.REVOKED, code); 882 if ("resolved".equals(codeString)) 883 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.RESOLVED, code); 884 if ("terminated".equals(codeString)) 885 return new Enumeration<ContractPublicationStatus>(this, ContractPublicationStatus.TERMINATED, code); 886 throw new FHIRException("Unknown ContractPublicationStatus code '" + codeString + "'"); 887 } 888 889 public String toCode(ContractPublicationStatus code) { 890 if (code == ContractPublicationStatus.NULL) 891 return null; 892 if (code == ContractPublicationStatus.AMENDED) 893 return "amended"; 894 if (code == ContractPublicationStatus.APPENDED) 895 return "appended"; 896 if (code == ContractPublicationStatus.CANCELLED) 897 return "cancelled"; 898 if (code == ContractPublicationStatus.DISPUTED) 899 return "disputed"; 900 if (code == ContractPublicationStatus.ENTEREDINERROR) 901 return "entered-in-error"; 902 if (code == ContractPublicationStatus.EXECUTABLE) 903 return "executable"; 904 if (code == ContractPublicationStatus.EXECUTED) 905 return "executed"; 906 if (code == ContractPublicationStatus.NEGOTIABLE) 907 return "negotiable"; 908 if (code == ContractPublicationStatus.OFFERED) 909 return "offered"; 910 if (code == ContractPublicationStatus.POLICY) 911 return "policy"; 912 if (code == ContractPublicationStatus.REJECTED) 913 return "rejected"; 914 if (code == ContractPublicationStatus.RENEWED) 915 return "renewed"; 916 if (code == ContractPublicationStatus.REVOKED) 917 return "revoked"; 918 if (code == ContractPublicationStatus.RESOLVED) 919 return "resolved"; 920 if (code == ContractPublicationStatus.TERMINATED) 921 return "terminated"; 922 return "?"; 923 } 924 925 public String toSystem(ContractPublicationStatus code) { 926 return code.getSystem(); 927 } 928 } 929 930 @Block() 931 public static class ContentDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 932 /** 933 * Precusory content structure and use, i.e., a boilerplate, template, 934 * application for a contract such as an insurance policy or benefits under a 935 * program, e.g., workers compensation. 936 */ 937 @Child(name = "type", type = { 938 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 939 @Description(shortDefinition = "Content structure and use", formalDefinition = "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.") 940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-type") 941 protected CodeableConcept type; 942 943 /** 944 * Detailed Precusory content type. 945 */ 946 @Child(name = "subType", type = { 947 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 948 @Description(shortDefinition = "Detailed Content Type Definition", formalDefinition = "Detailed Precusory content type.") 949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-definition-subtype") 950 protected CodeableConcept subType; 951 952 /** 953 * The individual or organization that published the Contract precursor content. 954 */ 955 @Child(name = "publisher", type = { Practitioner.class, PractitionerRole.class, 956 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 957 @Description(shortDefinition = "Publisher Entity", formalDefinition = "The individual or organization that published the Contract precursor content.") 958 protected Reference publisher; 959 960 /** 961 * The actual object that is the target of the reference (The individual or 962 * organization that published the Contract precursor content.) 963 */ 964 protected Resource publisherTarget; 965 966 /** 967 * The date (and optionally time) when the contract was published. The date must 968 * change when the business version changes and it must change if the status 969 * code changes. In addition, it should change when the substantive content of 970 * the contract changes. 971 */ 972 @Child(name = "publicationDate", type = { 973 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 974 @Description(shortDefinition = "When published", formalDefinition = "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.") 975 protected DateTimeType publicationDate; 976 977 /** 978 * amended | appended | cancelled | disputed | entered-in-error | executable | 979 * executed | negotiable | offered | policy | rejected | renewed | revoked | 980 * resolved | terminated. 981 */ 982 @Child(name = "publicationStatus", type = { 983 CodeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = false) 984 @Description(shortDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.") 985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-publicationstatus") 986 protected Enumeration<ContractPublicationStatus> publicationStatus; 987 988 /** 989 * A copyright statement relating to Contract precursor content. Copyright 990 * statements are generally legal restrictions on the use and publishing of the 991 * Contract precursor content. 992 */ 993 @Child(name = "copyright", type = { 994 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 995 @Description(shortDefinition = "Publication Ownership", formalDefinition = "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.") 996 protected MarkdownType copyright; 997 998 private static final long serialVersionUID = -699592864L; 999 1000 /** 1001 * Constructor 1002 */ 1003 public ContentDefinitionComponent() { 1004 super(); 1005 } 1006 1007 /** 1008 * Constructor 1009 */ 1010 public ContentDefinitionComponent(CodeableConcept type, Enumeration<ContractPublicationStatus> publicationStatus) { 1011 super(); 1012 this.type = type; 1013 this.publicationStatus = publicationStatus; 1014 } 1015 1016 /** 1017 * @return {@link #type} (Precusory content structure and use, i.e., a 1018 * boilerplate, template, application for a contract such as an 1019 * insurance policy or benefits under a program, e.g., workers 1020 * compensation.) 1021 */ 1022 public CodeableConcept getType() { 1023 if (this.type == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create ContentDefinitionComponent.type"); 1026 else if (Configuration.doAutoCreate()) 1027 this.type = new CodeableConcept(); // cc 1028 return this.type; 1029 } 1030 1031 public boolean hasType() { 1032 return this.type != null && !this.type.isEmpty(); 1033 } 1034 1035 /** 1036 * @param value {@link #type} (Precusory content structure and use, i.e., a 1037 * boilerplate, template, application for a contract such as an 1038 * insurance policy or benefits under a program, e.g., workers 1039 * compensation.) 1040 */ 1041 public ContentDefinitionComponent setType(CodeableConcept value) { 1042 this.type = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #subType} (Detailed Precusory content type.) 1048 */ 1049 public CodeableConcept getSubType() { 1050 if (this.subType == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create ContentDefinitionComponent.subType"); 1053 else if (Configuration.doAutoCreate()) 1054 this.subType = new CodeableConcept(); // cc 1055 return this.subType; 1056 } 1057 1058 public boolean hasSubType() { 1059 return this.subType != null && !this.subType.isEmpty(); 1060 } 1061 1062 /** 1063 * @param value {@link #subType} (Detailed Precusory content type.) 1064 */ 1065 public ContentDefinitionComponent setSubType(CodeableConcept value) { 1066 this.subType = value; 1067 return this; 1068 } 1069 1070 /** 1071 * @return {@link #publisher} (The individual or organization that published the 1072 * Contract precursor content.) 1073 */ 1074 public Reference getPublisher() { 1075 if (this.publisher == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create ContentDefinitionComponent.publisher"); 1078 else if (Configuration.doAutoCreate()) 1079 this.publisher = new Reference(); // cc 1080 return this.publisher; 1081 } 1082 1083 public boolean hasPublisher() { 1084 return this.publisher != null && !this.publisher.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #publisher} (The individual or organization that 1089 * published the Contract precursor content.) 1090 */ 1091 public ContentDefinitionComponent setPublisher(Reference value) { 1092 this.publisher = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #publisher} The actual object that is the target of the 1098 * reference. The reference library doesn't populate this, but you can 1099 * use it to hold the resource if you resolve it. (The individual or 1100 * organization that published the Contract precursor content.) 1101 */ 1102 public Resource getPublisherTarget() { 1103 return this.publisherTarget; 1104 } 1105 1106 /** 1107 * @param value {@link #publisher} The actual object that is the target of the 1108 * reference. The reference library doesn't use these, but you can 1109 * use it to hold the resource if you resolve it. (The individual 1110 * or organization that published the Contract precursor content.) 1111 */ 1112 public ContentDefinitionComponent setPublisherTarget(Resource value) { 1113 this.publisherTarget = value; 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #publicationDate} (The date (and optionally time) when the 1119 * contract was published. The date must change when the business 1120 * version changes and it must change if the status code changes. In 1121 * addition, it should change when the substantive content of the 1122 * contract changes.). This is the underlying object with id, value and 1123 * extensions. The accessor "getPublicationDate" gives direct access to 1124 * the value 1125 */ 1126 public DateTimeType getPublicationDateElement() { 1127 if (this.publicationDate == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationDate"); 1130 else if (Configuration.doAutoCreate()) 1131 this.publicationDate = new DateTimeType(); // bb 1132 return this.publicationDate; 1133 } 1134 1135 public boolean hasPublicationDateElement() { 1136 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1137 } 1138 1139 public boolean hasPublicationDate() { 1140 return this.publicationDate != null && !this.publicationDate.isEmpty(); 1141 } 1142 1143 /** 1144 * @param value {@link #publicationDate} (The date (and optionally time) when 1145 * the contract was published. The date must change when the 1146 * business version changes and it must change if the status code 1147 * changes. In addition, it should change when the substantive 1148 * content of the contract changes.). This is the underlying object 1149 * with id, value and extensions. The accessor "getPublicationDate" 1150 * gives direct access to the value 1151 */ 1152 public ContentDefinitionComponent setPublicationDateElement(DateTimeType value) { 1153 this.publicationDate = value; 1154 return this; 1155 } 1156 1157 /** 1158 * @return The date (and optionally time) when the contract was published. The 1159 * date must change when the business version changes and it must change 1160 * if the status code changes. In addition, it should change when the 1161 * substantive content of the contract changes. 1162 */ 1163 public Date getPublicationDate() { 1164 return this.publicationDate == null ? null : this.publicationDate.getValue(); 1165 } 1166 1167 /** 1168 * @param value The date (and optionally time) when the contract was published. 1169 * The date must change when the business version changes and it 1170 * must change if the status code changes. In addition, it should 1171 * change when the substantive content of the contract changes. 1172 */ 1173 public ContentDefinitionComponent setPublicationDate(Date value) { 1174 if (value == null) 1175 this.publicationDate = null; 1176 else { 1177 if (this.publicationDate == null) 1178 this.publicationDate = new DateTimeType(); 1179 this.publicationDate.setValue(value); 1180 } 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #publicationStatus} (amended | appended | cancelled | disputed 1186 * | entered-in-error | executable | executed | negotiable | offered | 1187 * policy | rejected | renewed | revoked | resolved | terminated.). This 1188 * is the underlying object with id, value and extensions. The accessor 1189 * "getPublicationStatus" gives direct access to the value 1190 */ 1191 public Enumeration<ContractPublicationStatus> getPublicationStatusElement() { 1192 if (this.publicationStatus == null) 1193 if (Configuration.errorOnAutoCreate()) 1194 throw new Error("Attempt to auto-create ContentDefinitionComponent.publicationStatus"); 1195 else if (Configuration.doAutoCreate()) 1196 this.publicationStatus = new Enumeration<ContractPublicationStatus>( 1197 new ContractPublicationStatusEnumFactory()); // bb 1198 return this.publicationStatus; 1199 } 1200 1201 public boolean hasPublicationStatusElement() { 1202 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1203 } 1204 1205 public boolean hasPublicationStatus() { 1206 return this.publicationStatus != null && !this.publicationStatus.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #publicationStatus} (amended | appended | cancelled | 1211 * disputed | entered-in-error | executable | executed | negotiable 1212 * | offered | policy | rejected | renewed | revoked | resolved | 1213 * terminated.). This is the underlying object with id, value and 1214 * extensions. The accessor "getPublicationStatus" gives direct 1215 * access to the value 1216 */ 1217 public ContentDefinitionComponent setPublicationStatusElement(Enumeration<ContractPublicationStatus> value) { 1218 this.publicationStatus = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return amended | appended | cancelled | disputed | entered-in-error | 1224 * executable | executed | negotiable | offered | policy | rejected | 1225 * renewed | revoked | resolved | terminated. 1226 */ 1227 public ContractPublicationStatus getPublicationStatus() { 1228 return this.publicationStatus == null ? null : this.publicationStatus.getValue(); 1229 } 1230 1231 /** 1232 * @param value amended | appended | cancelled | disputed | entered-in-error | 1233 * executable | executed | negotiable | offered | policy | rejected 1234 * | renewed | revoked | resolved | terminated. 1235 */ 1236 public ContentDefinitionComponent setPublicationStatus(ContractPublicationStatus value) { 1237 if (this.publicationStatus == null) 1238 this.publicationStatus = new Enumeration<ContractPublicationStatus>(new ContractPublicationStatusEnumFactory()); 1239 this.publicationStatus.setValue(value); 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #copyright} (A copyright statement relating to Contract 1245 * precursor content. Copyright statements are generally legal 1246 * restrictions on the use and publishing of the Contract precursor 1247 * content.). This is the underlying object with id, value and 1248 * extensions. The accessor "getCopyright" gives direct access to the 1249 * value 1250 */ 1251 public MarkdownType getCopyrightElement() { 1252 if (this.copyright == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create ContentDefinitionComponent.copyright"); 1255 else if (Configuration.doAutoCreate()) 1256 this.copyright = new MarkdownType(); // bb 1257 return this.copyright; 1258 } 1259 1260 public boolean hasCopyrightElement() { 1261 return this.copyright != null && !this.copyright.isEmpty(); 1262 } 1263 1264 public boolean hasCopyright() { 1265 return this.copyright != null && !this.copyright.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #copyright} (A copyright statement relating to Contract 1270 * precursor content. Copyright statements are generally legal 1271 * restrictions on the use and publishing of the Contract precursor 1272 * content.). This is the underlying object with id, value and 1273 * extensions. The accessor "getCopyright" gives direct access to 1274 * the value 1275 */ 1276 public ContentDefinitionComponent setCopyrightElement(MarkdownType value) { 1277 this.copyright = value; 1278 return this; 1279 } 1280 1281 /** 1282 * @return A copyright statement relating to Contract precursor content. 1283 * Copyright statements are generally legal restrictions on the use and 1284 * publishing of the Contract precursor content. 1285 */ 1286 public String getCopyright() { 1287 return this.copyright == null ? null : this.copyright.getValue(); 1288 } 1289 1290 /** 1291 * @param value A copyright statement relating to Contract precursor content. 1292 * Copyright statements are generally legal restrictions on the use 1293 * and publishing of the Contract precursor content. 1294 */ 1295 public ContentDefinitionComponent setCopyright(String value) { 1296 if (value == null) 1297 this.copyright = null; 1298 else { 1299 if (this.copyright == null) 1300 this.copyright = new MarkdownType(); 1301 this.copyright.setValue(value); 1302 } 1303 return this; 1304 } 1305 1306 protected void listChildren(List<Property> children) { 1307 super.listChildren(children); 1308 children.add(new Property("type", "CodeableConcept", 1309 "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 1310 0, 1, type)); 1311 children.add(new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, subType)); 1312 children.add(new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", 1313 "The individual or organization that published the Contract precursor content.", 0, 1, publisher)); 1314 children.add(new Property("publicationDate", "dateTime", 1315 "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 1316 0, 1, publicationDate)); 1317 children.add(new Property("publicationStatus", "code", 1318 "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.", 1319 0, 1, publicationStatus)); 1320 children.add(new Property("copyright", "markdown", 1321 "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 1322 0, 1, copyright)); 1323 } 1324 1325 @Override 1326 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1327 switch (_hash) { 1328 case 3575610: 1329 /* type */ return new Property("type", "CodeableConcept", 1330 "Precusory content structure and use, i.e., a boilerplate, template, application for a contract such as an insurance policy or benefits under a program, e.g., workers compensation.", 1331 0, 1, type); 1332 case -1868521062: 1333 /* subType */ return new Property("subType", "CodeableConcept", "Detailed Precusory content type.", 0, 1, 1334 subType); 1335 case 1447404028: 1336 /* publisher */ return new Property("publisher", "Reference(Practitioner|PractitionerRole|Organization)", 1337 "The individual or organization that published the Contract precursor content.", 0, 1, publisher); 1338 case 1470566394: 1339 /* publicationDate */ return new Property("publicationDate", "dateTime", 1340 "The date (and optionally time) when the contract was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the contract changes.", 1341 0, 1, publicationDate); 1342 case 616500542: 1343 /* publicationStatus */ return new Property("publicationStatus", "code", 1344 "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated.", 1345 0, 1, publicationStatus); 1346 case 1522889671: 1347 /* copyright */ return new Property("copyright", "markdown", 1348 "A copyright statement relating to Contract precursor content. Copyright statements are generally legal restrictions on the use and publishing of the Contract precursor content.", 1349 0, 1, copyright); 1350 default: 1351 return super.getNamedProperty(_hash, _name, _checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1358 switch (hash) { 1359 case 3575610: 1360 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1361 case -1868521062: 1362 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 1363 case 1447404028: 1364 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // Reference 1365 case 1470566394: 1366 /* publicationDate */ return this.publicationDate == null ? new Base[0] : new Base[] { this.publicationDate }; // DateTimeType 1367 case 616500542: 1368 /* publicationStatus */ return this.publicationStatus == null ? new Base[0] 1369 : new Base[] { this.publicationStatus }; // Enumeration<ContractPublicationStatus> 1370 case 1522889671: 1371 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 1372 default: 1373 return super.getProperty(hash, name, checkValid); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1380 switch (hash) { 1381 case 3575610: // type 1382 this.type = castToCodeableConcept(value); // CodeableConcept 1383 return value; 1384 case -1868521062: // subType 1385 this.subType = castToCodeableConcept(value); // CodeableConcept 1386 return value; 1387 case 1447404028: // publisher 1388 this.publisher = castToReference(value); // Reference 1389 return value; 1390 case 1470566394: // publicationDate 1391 this.publicationDate = castToDateTime(value); // DateTimeType 1392 return value; 1393 case 616500542: // publicationStatus 1394 value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value)); 1395 this.publicationStatus = (Enumeration) value; // Enumeration<ContractPublicationStatus> 1396 return value; 1397 case 1522889671: // copyright 1398 this.copyright = castToMarkdown(value); // MarkdownType 1399 return value; 1400 default: 1401 return super.setProperty(hash, name, value); 1402 } 1403 1404 } 1405 1406 @Override 1407 public Base setProperty(String name, Base value) throws FHIRException { 1408 if (name.equals("type")) { 1409 this.type = castToCodeableConcept(value); // CodeableConcept 1410 } else if (name.equals("subType")) { 1411 this.subType = castToCodeableConcept(value); // CodeableConcept 1412 } else if (name.equals("publisher")) { 1413 this.publisher = castToReference(value); // Reference 1414 } else if (name.equals("publicationDate")) { 1415 this.publicationDate = castToDateTime(value); // DateTimeType 1416 } else if (name.equals("publicationStatus")) { 1417 value = new ContractPublicationStatusEnumFactory().fromType(castToCode(value)); 1418 this.publicationStatus = (Enumeration) value; // Enumeration<ContractPublicationStatus> 1419 } else if (name.equals("copyright")) { 1420 this.copyright = castToMarkdown(value); // MarkdownType 1421 } else 1422 return super.setProperty(name, value); 1423 return value; 1424 } 1425 1426 @Override 1427 public void removeChild(String name, Base value) throws FHIRException { 1428 if (name.equals("type")) { 1429 this.type = null; 1430 } else if (name.equals("subType")) { 1431 this.subType = null; 1432 } else if (name.equals("publisher")) { 1433 this.publisher = null; 1434 } else if (name.equals("publicationDate")) { 1435 this.publicationDate = null; 1436 } else if (name.equals("publicationStatus")) { 1437 this.publicationStatus = null; 1438 } else if (name.equals("copyright")) { 1439 this.copyright = null; 1440 } else 1441 super.removeChild(name, value); 1442 1443 } 1444 1445 @Override 1446 public Base makeProperty(int hash, String name) throws FHIRException { 1447 switch (hash) { 1448 case 3575610: 1449 return getType(); 1450 case -1868521062: 1451 return getSubType(); 1452 case 1447404028: 1453 return getPublisher(); 1454 case 1470566394: 1455 return getPublicationDateElement(); 1456 case 616500542: 1457 return getPublicationStatusElement(); 1458 case 1522889671: 1459 return getCopyrightElement(); 1460 default: 1461 return super.makeProperty(hash, name); 1462 } 1463 1464 } 1465 1466 @Override 1467 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1468 switch (hash) { 1469 case 3575610: 1470 /* type */ return new String[] { "CodeableConcept" }; 1471 case -1868521062: 1472 /* subType */ return new String[] { "CodeableConcept" }; 1473 case 1447404028: 1474 /* publisher */ return new String[] { "Reference" }; 1475 case 1470566394: 1476 /* publicationDate */ return new String[] { "dateTime" }; 1477 case 616500542: 1478 /* publicationStatus */ return new String[] { "code" }; 1479 case 1522889671: 1480 /* copyright */ return new String[] { "markdown" }; 1481 default: 1482 return super.getTypesForProperty(hash, name); 1483 } 1484 1485 } 1486 1487 @Override 1488 public Base addChild(String name) throws FHIRException { 1489 if (name.equals("type")) { 1490 this.type = new CodeableConcept(); 1491 return this.type; 1492 } else if (name.equals("subType")) { 1493 this.subType = new CodeableConcept(); 1494 return this.subType; 1495 } else if (name.equals("publisher")) { 1496 this.publisher = new Reference(); 1497 return this.publisher; 1498 } else if (name.equals("publicationDate")) { 1499 throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationDate"); 1500 } else if (name.equals("publicationStatus")) { 1501 throw new FHIRException("Cannot call addChild on a singleton property Contract.publicationStatus"); 1502 } else if (name.equals("copyright")) { 1503 throw new FHIRException("Cannot call addChild on a singleton property Contract.copyright"); 1504 } else 1505 return super.addChild(name); 1506 } 1507 1508 public ContentDefinitionComponent copy() { 1509 ContentDefinitionComponent dst = new ContentDefinitionComponent(); 1510 copyValues(dst); 1511 return dst; 1512 } 1513 1514 public void copyValues(ContentDefinitionComponent dst) { 1515 super.copyValues(dst); 1516 dst.type = type == null ? null : type.copy(); 1517 dst.subType = subType == null ? null : subType.copy(); 1518 dst.publisher = publisher == null ? null : publisher.copy(); 1519 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 1520 dst.publicationStatus = publicationStatus == null ? null : publicationStatus.copy(); 1521 dst.copyright = copyright == null ? null : copyright.copy(); 1522 } 1523 1524 @Override 1525 public boolean equalsDeep(Base other_) { 1526 if (!super.equalsDeep(other_)) 1527 return false; 1528 if (!(other_ instanceof ContentDefinitionComponent)) 1529 return false; 1530 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1531 return compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 1532 && compareDeep(publisher, o.publisher, true) && compareDeep(publicationDate, o.publicationDate, true) 1533 && compareDeep(publicationStatus, o.publicationStatus, true) && compareDeep(copyright, o.copyright, true); 1534 } 1535 1536 @Override 1537 public boolean equalsShallow(Base other_) { 1538 if (!super.equalsShallow(other_)) 1539 return false; 1540 if (!(other_ instanceof ContentDefinitionComponent)) 1541 return false; 1542 ContentDefinitionComponent o = (ContentDefinitionComponent) other_; 1543 return compareValues(publicationDate, o.publicationDate, true) 1544 && compareValues(publicationStatus, o.publicationStatus, true) && compareValues(copyright, o.copyright, true); 1545 } 1546 1547 public boolean isEmpty() { 1548 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, subType, publisher, publicationDate, 1549 publicationStatus, copyright); 1550 } 1551 1552 public String fhirType() { 1553 return "Contract.contentDefinition"; 1554 1555 } 1556 1557 } 1558 1559 @Block() 1560 public static class TermComponent extends BackboneElement implements IBaseBackboneElement { 1561 /** 1562 * Unique identifier for this particular Contract Provision. 1563 */ 1564 @Child(name = "identifier", type = { 1565 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1566 @Description(shortDefinition = "Contract Term Number", formalDefinition = "Unique identifier for this particular Contract Provision.") 1567 protected Identifier identifier; 1568 1569 /** 1570 * When this Contract Provision was issued. 1571 */ 1572 @Child(name = "issued", type = { 1573 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1574 @Description(shortDefinition = "Contract Term Issue Date Time", formalDefinition = "When this Contract Provision was issued.") 1575 protected DateTimeType issued; 1576 1577 /** 1578 * Relevant time or time-period when this Contract Provision is applicable. 1579 */ 1580 @Child(name = "applies", type = { Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1581 @Description(shortDefinition = "Contract Term Effective Time", formalDefinition = "Relevant time or time-period when this Contract Provision is applicable.") 1582 protected Period applies; 1583 1584 /** 1585 * The entity that the term applies to. 1586 */ 1587 @Child(name = "topic", type = { CodeableConcept.class, 1588 Reference.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1589 @Description(shortDefinition = "Term Concern", formalDefinition = "The entity that the term applies to.") 1590 protected Type topic; 1591 1592 /** 1593 * A legal clause or condition contained within a contract that requires one or 1594 * both parties to perform a particular requirement by some specified time or 1595 * prevents one or both parties from performing a particular requirement by some 1596 * specified time. 1597 */ 1598 @Child(name = "type", type = { 1599 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1600 @Description(shortDefinition = "Contract Term Type or Form", formalDefinition = "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.") 1601 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type") 1602 protected CodeableConcept type; 1603 1604 /** 1605 * A specialized legal clause or condition based on overarching contract type. 1606 */ 1607 @Child(name = "subType", type = { 1608 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1609 @Description(shortDefinition = "Contract Term Type specific classification", formalDefinition = "A specialized legal clause or condition based on overarching contract type.") 1610 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-subtype") 1611 protected CodeableConcept subType; 1612 1613 /** 1614 * Statement of a provision in a policy or a contract. 1615 */ 1616 @Child(name = "text", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1617 @Description(shortDefinition = "Term Statement", formalDefinition = "Statement of a provision in a policy or a contract.") 1618 protected StringType text; 1619 1620 /** 1621 * Security labels that protect the handling of information about the term and 1622 * its elements, which may be specifically identified.. 1623 */ 1624 @Child(name = "securityLabel", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1625 @Description(shortDefinition = "Protection for the Term", formalDefinition = "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..") 1626 protected List<SecurityLabelComponent> securityLabel; 1627 1628 /** 1629 * The matter of concern in the context of this provision of the agrement. 1630 */ 1631 @Child(name = "offer", type = {}, order = 9, min = 1, max = 1, modifier = false, summary = false) 1632 @Description(shortDefinition = "Context of the Contract term", formalDefinition = "The matter of concern in the context of this provision of the agrement.") 1633 protected ContractOfferComponent offer; 1634 1635 /** 1636 * Contract Term Asset List. 1637 */ 1638 @Child(name = "asset", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1639 @Description(shortDefinition = "Contract Term Asset List", formalDefinition = "Contract Term Asset List.") 1640 protected List<ContractAssetComponent> asset; 1641 1642 /** 1643 * An actor taking a role in an activity for which it can be assigned some 1644 * degree of responsibility for the activity taking place. 1645 */ 1646 @Child(name = "action", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1647 @Description(shortDefinition = "Entity being ascribed responsibility", formalDefinition = "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.") 1648 protected List<ActionComponent> action; 1649 1650 /** 1651 * Nested group of Contract Provisions. 1652 */ 1653 @Child(name = "group", type = { 1654 TermComponent.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1655 @Description(shortDefinition = "Nested Contract Term Group", formalDefinition = "Nested group of Contract Provisions.") 1656 protected List<TermComponent> group; 1657 1658 private static final long serialVersionUID = -460907186L; 1659 1660 /** 1661 * Constructor 1662 */ 1663 public TermComponent() { 1664 super(); 1665 } 1666 1667 /** 1668 * Constructor 1669 */ 1670 public TermComponent(ContractOfferComponent offer) { 1671 super(); 1672 this.offer = offer; 1673 } 1674 1675 /** 1676 * @return {@link #identifier} (Unique identifier for this particular Contract 1677 * Provision.) 1678 */ 1679 public Identifier getIdentifier() { 1680 if (this.identifier == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create TermComponent.identifier"); 1683 else if (Configuration.doAutoCreate()) 1684 this.identifier = new Identifier(); // cc 1685 return this.identifier; 1686 } 1687 1688 public boolean hasIdentifier() { 1689 return this.identifier != null && !this.identifier.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #identifier} (Unique identifier for this particular 1694 * Contract Provision.) 1695 */ 1696 public TermComponent setIdentifier(Identifier value) { 1697 this.identifier = value; 1698 return this; 1699 } 1700 1701 /** 1702 * @return {@link #issued} (When this Contract Provision was issued.). This is 1703 * the underlying object with id, value and extensions. The accessor 1704 * "getIssued" gives direct access to the value 1705 */ 1706 public DateTimeType getIssuedElement() { 1707 if (this.issued == null) 1708 if (Configuration.errorOnAutoCreate()) 1709 throw new Error("Attempt to auto-create TermComponent.issued"); 1710 else if (Configuration.doAutoCreate()) 1711 this.issued = new DateTimeType(); // bb 1712 return this.issued; 1713 } 1714 1715 public boolean hasIssuedElement() { 1716 return this.issued != null && !this.issued.isEmpty(); 1717 } 1718 1719 public boolean hasIssued() { 1720 return this.issued != null && !this.issued.isEmpty(); 1721 } 1722 1723 /** 1724 * @param value {@link #issued} (When this Contract Provision was issued.). This 1725 * is the underlying object with id, value and extensions. The 1726 * accessor "getIssued" gives direct access to the value 1727 */ 1728 public TermComponent setIssuedElement(DateTimeType value) { 1729 this.issued = value; 1730 return this; 1731 } 1732 1733 /** 1734 * @return When this Contract Provision was issued. 1735 */ 1736 public Date getIssued() { 1737 return this.issued == null ? null : this.issued.getValue(); 1738 } 1739 1740 /** 1741 * @param value When this Contract Provision was issued. 1742 */ 1743 public TermComponent setIssued(Date value) { 1744 if (value == null) 1745 this.issued = null; 1746 else { 1747 if (this.issued == null) 1748 this.issued = new DateTimeType(); 1749 this.issued.setValue(value); 1750 } 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #applies} (Relevant time or time-period when this Contract 1756 * Provision is applicable.) 1757 */ 1758 public Period getApplies() { 1759 if (this.applies == null) 1760 if (Configuration.errorOnAutoCreate()) 1761 throw new Error("Attempt to auto-create TermComponent.applies"); 1762 else if (Configuration.doAutoCreate()) 1763 this.applies = new Period(); // cc 1764 return this.applies; 1765 } 1766 1767 public boolean hasApplies() { 1768 return this.applies != null && !this.applies.isEmpty(); 1769 } 1770 1771 /** 1772 * @param value {@link #applies} (Relevant time or time-period when this 1773 * Contract Provision is applicable.) 1774 */ 1775 public TermComponent setApplies(Period value) { 1776 this.applies = value; 1777 return this; 1778 } 1779 1780 /** 1781 * @return {@link #topic} (The entity that the term applies to.) 1782 */ 1783 public Type getTopic() { 1784 return this.topic; 1785 } 1786 1787 /** 1788 * @return {@link #topic} (The entity that the term applies to.) 1789 */ 1790 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 1791 if (this.topic == null) 1792 this.topic = new CodeableConcept(); 1793 if (!(this.topic instanceof CodeableConcept)) 1794 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1795 + this.topic.getClass().getName() + " was encountered"); 1796 return (CodeableConcept) this.topic; 1797 } 1798 1799 public boolean hasTopicCodeableConcept() { 1800 return this.topic instanceof CodeableConcept; 1801 } 1802 1803 /** 1804 * @return {@link #topic} (The entity that the term applies to.) 1805 */ 1806 public Reference getTopicReference() throws FHIRException { 1807 if (this.topic == null) 1808 this.topic = new Reference(); 1809 if (!(this.topic instanceof Reference)) 1810 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.topic.getClass().getName() 1811 + " was encountered"); 1812 return (Reference) this.topic; 1813 } 1814 1815 public boolean hasTopicReference() { 1816 return this.topic instanceof Reference; 1817 } 1818 1819 public boolean hasTopic() { 1820 return this.topic != null && !this.topic.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #topic} (The entity that the term applies to.) 1825 */ 1826 public TermComponent setTopic(Type value) { 1827 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1828 throw new Error("Not the right type for Contract.term.topic[x]: " + value.fhirType()); 1829 this.topic = value; 1830 return this; 1831 } 1832 1833 /** 1834 * @return {@link #type} (A legal clause or condition contained within a 1835 * contract that requires one or both parties to perform a particular 1836 * requirement by some specified time or prevents one or both parties 1837 * from performing a particular requirement by some specified time.) 1838 */ 1839 public CodeableConcept getType() { 1840 if (this.type == null) 1841 if (Configuration.errorOnAutoCreate()) 1842 throw new Error("Attempt to auto-create TermComponent.type"); 1843 else if (Configuration.doAutoCreate()) 1844 this.type = new CodeableConcept(); // cc 1845 return this.type; 1846 } 1847 1848 public boolean hasType() { 1849 return this.type != null && !this.type.isEmpty(); 1850 } 1851 1852 /** 1853 * @param value {@link #type} (A legal clause or condition contained within a 1854 * contract that requires one or both parties to perform a 1855 * particular requirement by some specified time or prevents one or 1856 * both parties from performing a particular requirement by some 1857 * specified time.) 1858 */ 1859 public TermComponent setType(CodeableConcept value) { 1860 this.type = value; 1861 return this; 1862 } 1863 1864 /** 1865 * @return {@link #subType} (A specialized legal clause or condition based on 1866 * overarching contract type.) 1867 */ 1868 public CodeableConcept getSubType() { 1869 if (this.subType == null) 1870 if (Configuration.errorOnAutoCreate()) 1871 throw new Error("Attempt to auto-create TermComponent.subType"); 1872 else if (Configuration.doAutoCreate()) 1873 this.subType = new CodeableConcept(); // cc 1874 return this.subType; 1875 } 1876 1877 public boolean hasSubType() { 1878 return this.subType != null && !this.subType.isEmpty(); 1879 } 1880 1881 /** 1882 * @param value {@link #subType} (A specialized legal clause or condition based 1883 * on overarching contract type.) 1884 */ 1885 public TermComponent setSubType(CodeableConcept value) { 1886 this.subType = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #text} (Statement of a provision in a policy or a contract.). 1892 * This is the underlying object with id, value and extensions. The 1893 * accessor "getText" gives direct access to the value 1894 */ 1895 public StringType getTextElement() { 1896 if (this.text == null) 1897 if (Configuration.errorOnAutoCreate()) 1898 throw new Error("Attempt to auto-create TermComponent.text"); 1899 else if (Configuration.doAutoCreate()) 1900 this.text = new StringType(); // bb 1901 return this.text; 1902 } 1903 1904 public boolean hasTextElement() { 1905 return this.text != null && !this.text.isEmpty(); 1906 } 1907 1908 public boolean hasText() { 1909 return this.text != null && !this.text.isEmpty(); 1910 } 1911 1912 /** 1913 * @param value {@link #text} (Statement of a provision in a policy or a 1914 * contract.). This is the underlying object with id, value and 1915 * extensions. The accessor "getText" gives direct access to the 1916 * value 1917 */ 1918 public TermComponent setTextElement(StringType value) { 1919 this.text = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return Statement of a provision in a policy or a contract. 1925 */ 1926 public String getText() { 1927 return this.text == null ? null : this.text.getValue(); 1928 } 1929 1930 /** 1931 * @param value Statement of a provision in a policy or a contract. 1932 */ 1933 public TermComponent setText(String value) { 1934 if (Utilities.noString(value)) 1935 this.text = null; 1936 else { 1937 if (this.text == null) 1938 this.text = new StringType(); 1939 this.text.setValue(value); 1940 } 1941 return this; 1942 } 1943 1944 /** 1945 * @return {@link #securityLabel} (Security labels that protect the handling of 1946 * information about the term and its elements, which may be 1947 * specifically identified..) 1948 */ 1949 public List<SecurityLabelComponent> getSecurityLabel() { 1950 if (this.securityLabel == null) 1951 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1952 return this.securityLabel; 1953 } 1954 1955 /** 1956 * @return Returns a reference to <code>this</code> for easy method chaining 1957 */ 1958 public TermComponent setSecurityLabel(List<SecurityLabelComponent> theSecurityLabel) { 1959 this.securityLabel = theSecurityLabel; 1960 return this; 1961 } 1962 1963 public boolean hasSecurityLabel() { 1964 if (this.securityLabel == null) 1965 return false; 1966 for (SecurityLabelComponent item : this.securityLabel) 1967 if (!item.isEmpty()) 1968 return true; 1969 return false; 1970 } 1971 1972 public SecurityLabelComponent addSecurityLabel() { // 3 1973 SecurityLabelComponent t = new SecurityLabelComponent(); 1974 if (this.securityLabel == null) 1975 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1976 this.securityLabel.add(t); 1977 return t; 1978 } 1979 1980 public TermComponent addSecurityLabel(SecurityLabelComponent t) { // 3 1981 if (t == null) 1982 return this; 1983 if (this.securityLabel == null) 1984 this.securityLabel = new ArrayList<SecurityLabelComponent>(); 1985 this.securityLabel.add(t); 1986 return this; 1987 } 1988 1989 /** 1990 * @return The first repetition of repeating field {@link #securityLabel}, 1991 * creating it if it does not already exist 1992 */ 1993 public SecurityLabelComponent getSecurityLabelFirstRep() { 1994 if (getSecurityLabel().isEmpty()) { 1995 addSecurityLabel(); 1996 } 1997 return getSecurityLabel().get(0); 1998 } 1999 2000 /** 2001 * @return {@link #offer} (The matter of concern in the context of this 2002 * provision of the agrement.) 2003 */ 2004 public ContractOfferComponent getOffer() { 2005 if (this.offer == null) 2006 if (Configuration.errorOnAutoCreate()) 2007 throw new Error("Attempt to auto-create TermComponent.offer"); 2008 else if (Configuration.doAutoCreate()) 2009 this.offer = new ContractOfferComponent(); // cc 2010 return this.offer; 2011 } 2012 2013 public boolean hasOffer() { 2014 return this.offer != null && !this.offer.isEmpty(); 2015 } 2016 2017 /** 2018 * @param value {@link #offer} (The matter of concern in the context of this 2019 * provision of the agrement.) 2020 */ 2021 public TermComponent setOffer(ContractOfferComponent value) { 2022 this.offer = value; 2023 return this; 2024 } 2025 2026 /** 2027 * @return {@link #asset} (Contract Term Asset List.) 2028 */ 2029 public List<ContractAssetComponent> getAsset() { 2030 if (this.asset == null) 2031 this.asset = new ArrayList<ContractAssetComponent>(); 2032 return this.asset; 2033 } 2034 2035 /** 2036 * @return Returns a reference to <code>this</code> for easy method chaining 2037 */ 2038 public TermComponent setAsset(List<ContractAssetComponent> theAsset) { 2039 this.asset = theAsset; 2040 return this; 2041 } 2042 2043 public boolean hasAsset() { 2044 if (this.asset == null) 2045 return false; 2046 for (ContractAssetComponent item : this.asset) 2047 if (!item.isEmpty()) 2048 return true; 2049 return false; 2050 } 2051 2052 public ContractAssetComponent addAsset() { // 3 2053 ContractAssetComponent t = new ContractAssetComponent(); 2054 if (this.asset == null) 2055 this.asset = new ArrayList<ContractAssetComponent>(); 2056 this.asset.add(t); 2057 return t; 2058 } 2059 2060 public TermComponent addAsset(ContractAssetComponent t) { // 3 2061 if (t == null) 2062 return this; 2063 if (this.asset == null) 2064 this.asset = new ArrayList<ContractAssetComponent>(); 2065 this.asset.add(t); 2066 return this; 2067 } 2068 2069 /** 2070 * @return The first repetition of repeating field {@link #asset}, creating it 2071 * if it does not already exist 2072 */ 2073 public ContractAssetComponent getAssetFirstRep() { 2074 if (getAsset().isEmpty()) { 2075 addAsset(); 2076 } 2077 return getAsset().get(0); 2078 } 2079 2080 /** 2081 * @return {@link #action} (An actor taking a role in an activity for which it 2082 * can be assigned some degree of responsibility for the activity taking 2083 * place.) 2084 */ 2085 public List<ActionComponent> getAction() { 2086 if (this.action == null) 2087 this.action = new ArrayList<ActionComponent>(); 2088 return this.action; 2089 } 2090 2091 /** 2092 * @return Returns a reference to <code>this</code> for easy method chaining 2093 */ 2094 public TermComponent setAction(List<ActionComponent> theAction) { 2095 this.action = theAction; 2096 return this; 2097 } 2098 2099 public boolean hasAction() { 2100 if (this.action == null) 2101 return false; 2102 for (ActionComponent item : this.action) 2103 if (!item.isEmpty()) 2104 return true; 2105 return false; 2106 } 2107 2108 public ActionComponent addAction() { // 3 2109 ActionComponent t = new ActionComponent(); 2110 if (this.action == null) 2111 this.action = new ArrayList<ActionComponent>(); 2112 this.action.add(t); 2113 return t; 2114 } 2115 2116 public TermComponent addAction(ActionComponent t) { // 3 2117 if (t == null) 2118 return this; 2119 if (this.action == null) 2120 this.action = new ArrayList<ActionComponent>(); 2121 this.action.add(t); 2122 return this; 2123 } 2124 2125 /** 2126 * @return The first repetition of repeating field {@link #action}, creating it 2127 * if it does not already exist 2128 */ 2129 public ActionComponent getActionFirstRep() { 2130 if (getAction().isEmpty()) { 2131 addAction(); 2132 } 2133 return getAction().get(0); 2134 } 2135 2136 /** 2137 * @return {@link #group} (Nested group of Contract Provisions.) 2138 */ 2139 public List<TermComponent> getGroup() { 2140 if (this.group == null) 2141 this.group = new ArrayList<TermComponent>(); 2142 return this.group; 2143 } 2144 2145 /** 2146 * @return Returns a reference to <code>this</code> for easy method chaining 2147 */ 2148 public TermComponent setGroup(List<TermComponent> theGroup) { 2149 this.group = theGroup; 2150 return this; 2151 } 2152 2153 public boolean hasGroup() { 2154 if (this.group == null) 2155 return false; 2156 for (TermComponent item : this.group) 2157 if (!item.isEmpty()) 2158 return true; 2159 return false; 2160 } 2161 2162 public TermComponent addGroup() { // 3 2163 TermComponent t = new TermComponent(); 2164 if (this.group == null) 2165 this.group = new ArrayList<TermComponent>(); 2166 this.group.add(t); 2167 return t; 2168 } 2169 2170 public TermComponent addGroup(TermComponent t) { // 3 2171 if (t == null) 2172 return this; 2173 if (this.group == null) 2174 this.group = new ArrayList<TermComponent>(); 2175 this.group.add(t); 2176 return this; 2177 } 2178 2179 /** 2180 * @return The first repetition of repeating field {@link #group}, creating it 2181 * if it does not already exist 2182 */ 2183 public TermComponent getGroupFirstRep() { 2184 if (getGroup().isEmpty()) { 2185 addGroup(); 2186 } 2187 return getGroup().get(0); 2188 } 2189 2190 protected void listChildren(List<Property> children) { 2191 super.listChildren(children); 2192 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 2193 0, 1, identifier)); 2194 children.add(new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, issued)); 2195 children.add(new Property("applies", "Period", 2196 "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies)); 2197 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", "The entity that the term applies to.", 0, 2198 1, topic)); 2199 children.add(new Property("type", "CodeableConcept", 2200 "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 2201 0, 1, type)); 2202 children.add(new Property("subType", "CodeableConcept", 2203 "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType)); 2204 children.add(new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, text)); 2205 children.add(new Property("securityLabel", "", 2206 "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..", 2207 0, java.lang.Integer.MAX_VALUE, securityLabel)); 2208 children.add(new Property("offer", "", "The matter of concern in the context of this provision of the agrement.", 2209 0, 1, offer)); 2210 children.add(new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, asset)); 2211 children.add(new Property("action", "", 2212 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 2213 0, java.lang.Integer.MAX_VALUE, action)); 2214 children.add(new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, 2215 java.lang.Integer.MAX_VALUE, group)); 2216 } 2217 2218 @Override 2219 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2220 switch (_hash) { 2221 case -1618432855: 2222 /* identifier */ return new Property("identifier", "Identifier", 2223 "Unique identifier for this particular Contract Provision.", 0, 1, identifier); 2224 case -1179159893: 2225 /* issued */ return new Property("issued", "dateTime", "When this Contract Provision was issued.", 0, 1, 2226 issued); 2227 case -793235316: 2228 /* applies */ return new Property("applies", "Period", 2229 "Relevant time or time-period when this Contract Provision is applicable.", 0, 1, applies); 2230 case -957295375: 2231 /* topic[x] */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2232 "The entity that the term applies to.", 0, 1, topic); 2233 case 110546223: 2234 /* topic */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2235 "The entity that the term applies to.", 0, 1, topic); 2236 case 777778802: 2237 /* topicCodeableConcept */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2238 "The entity that the term applies to.", 0, 1, topic); 2239 case -343345444: 2240 /* topicReference */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 2241 "The entity that the term applies to.", 0, 1, topic); 2242 case 3575610: 2243 /* type */ return new Property("type", "CodeableConcept", 2244 "A legal clause or condition contained within a contract that requires one or both parties to perform a particular requirement by some specified time or prevents one or both parties from performing a particular requirement by some specified time.", 2245 0, 1, type); 2246 case -1868521062: 2247 /* subType */ return new Property("subType", "CodeableConcept", 2248 "A specialized legal clause or condition based on overarching contract type.", 0, 1, subType); 2249 case 3556653: 2250 /* text */ return new Property("text", "string", "Statement of a provision in a policy or a contract.", 0, 1, 2251 text); 2252 case -722296940: 2253 /* securityLabel */ return new Property("securityLabel", "", 2254 "Security labels that protect the handling of information about the term and its elements, which may be specifically identified..", 2255 0, java.lang.Integer.MAX_VALUE, securityLabel); 2256 case 105650780: 2257 /* offer */ return new Property("offer", "", 2258 "The matter of concern in the context of this provision of the agrement.", 0, 1, offer); 2259 case 93121264: 2260 /* asset */ return new Property("asset", "", "Contract Term Asset List.", 0, java.lang.Integer.MAX_VALUE, 2261 asset); 2262 case -1422950858: 2263 /* action */ return new Property("action", "", 2264 "An actor taking a role in an activity for which it can be assigned some degree of responsibility for the activity taking place.", 2265 0, java.lang.Integer.MAX_VALUE, action); 2266 case 98629247: 2267 /* group */ return new Property("group", "@Contract.term", "Nested group of Contract Provisions.", 0, 2268 java.lang.Integer.MAX_VALUE, group); 2269 default: 2270 return super.getNamedProperty(_hash, _name, _checkValid); 2271 } 2272 2273 } 2274 2275 @Override 2276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2277 switch (hash) { 2278 case -1618432855: 2279 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2280 case -1179159893: 2281 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType 2282 case -793235316: 2283 /* applies */ return this.applies == null ? new Base[0] : new Base[] { this.applies }; // Period 2284 case 110546223: 2285 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Type 2286 case 3575610: 2287 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2288 case -1868521062: 2289 /* subType */ return this.subType == null ? new Base[0] : new Base[] { this.subType }; // CodeableConcept 2290 case 3556653: 2291 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 2292 case -722296940: 2293 /* securityLabel */ return this.securityLabel == null ? new Base[0] 2294 : this.securityLabel.toArray(new Base[this.securityLabel.size()]); // SecurityLabelComponent 2295 case 105650780: 2296 /* offer */ return this.offer == null ? new Base[0] : new Base[] { this.offer }; // ContractOfferComponent 2297 case 93121264: 2298 /* asset */ return this.asset == null ? new Base[0] : this.asset.toArray(new Base[this.asset.size()]); // ContractAssetComponent 2299 case -1422950858: 2300 /* action */ return this.action == null ? new Base[0] : this.action.toArray(new Base[this.action.size()]); // ActionComponent 2301 case 98629247: 2302 /* group */ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // TermComponent 2303 default: 2304 return super.getProperty(hash, name, checkValid); 2305 } 2306 2307 } 2308 2309 @Override 2310 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2311 switch (hash) { 2312 case -1618432855: // identifier 2313 this.identifier = castToIdentifier(value); // Identifier 2314 return value; 2315 case -1179159893: // issued 2316 this.issued = castToDateTime(value); // DateTimeType 2317 return value; 2318 case -793235316: // applies 2319 this.applies = castToPeriod(value); // Period 2320 return value; 2321 case 110546223: // topic 2322 this.topic = castToType(value); // Type 2323 return value; 2324 case 3575610: // type 2325 this.type = castToCodeableConcept(value); // CodeableConcept 2326 return value; 2327 case -1868521062: // subType 2328 this.subType = castToCodeableConcept(value); // CodeableConcept 2329 return value; 2330 case 3556653: // text 2331 this.text = castToString(value); // StringType 2332 return value; 2333 case -722296940: // securityLabel 2334 this.getSecurityLabel().add((SecurityLabelComponent) value); // SecurityLabelComponent 2335 return value; 2336 case 105650780: // offer 2337 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2338 return value; 2339 case 93121264: // asset 2340 this.getAsset().add((ContractAssetComponent) value); // ContractAssetComponent 2341 return value; 2342 case -1422950858: // action 2343 this.getAction().add((ActionComponent) value); // ActionComponent 2344 return value; 2345 case 98629247: // group 2346 this.getGroup().add((TermComponent) value); // TermComponent 2347 return value; 2348 default: 2349 return super.setProperty(hash, name, value); 2350 } 2351 2352 } 2353 2354 @Override 2355 public Base setProperty(String name, Base value) throws FHIRException { 2356 if (name.equals("identifier")) { 2357 this.identifier = castToIdentifier(value); // Identifier 2358 } else if (name.equals("issued")) { 2359 this.issued = castToDateTime(value); // DateTimeType 2360 } else if (name.equals("applies")) { 2361 this.applies = castToPeriod(value); // Period 2362 } else if (name.equals("topic[x]")) { 2363 this.topic = castToType(value); // Type 2364 } else if (name.equals("type")) { 2365 this.type = castToCodeableConcept(value); // CodeableConcept 2366 } else if (name.equals("subType")) { 2367 this.subType = castToCodeableConcept(value); // CodeableConcept 2368 } else if (name.equals("text")) { 2369 this.text = castToString(value); // StringType 2370 } else if (name.equals("securityLabel")) { 2371 this.getSecurityLabel().add((SecurityLabelComponent) value); 2372 } else if (name.equals("offer")) { 2373 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2374 } else if (name.equals("asset")) { 2375 this.getAsset().add((ContractAssetComponent) value); 2376 } else if (name.equals("action")) { 2377 this.getAction().add((ActionComponent) value); 2378 } else if (name.equals("group")) { 2379 this.getGroup().add((TermComponent) value); 2380 } else 2381 return super.setProperty(name, value); 2382 return value; 2383 } 2384 2385 @Override 2386 public void removeChild(String name, Base value) throws FHIRException { 2387 if (name.equals("identifier")) { 2388 this.identifier = null; 2389 } else if (name.equals("issued")) { 2390 this.issued = null; 2391 } else if (name.equals("applies")) { 2392 this.applies = null; 2393 } else if (name.equals("topic[x]")) { 2394 this.topic = null; 2395 } else if (name.equals("type")) { 2396 this.type = null; 2397 } else if (name.equals("subType")) { 2398 this.subType = null; 2399 } else if (name.equals("text")) { 2400 this.text = null; 2401 } else if (name.equals("securityLabel")) { 2402 this.getSecurityLabel().remove((SecurityLabelComponent) value); 2403 } else if (name.equals("offer")) { 2404 this.offer = (ContractOfferComponent) value; // ContractOfferComponent 2405 } else if (name.equals("asset")) { 2406 this.getAsset().remove((ContractAssetComponent) value); 2407 } else if (name.equals("action")) { 2408 this.getAction().remove((ActionComponent) value); 2409 } else if (name.equals("group")) { 2410 this.getGroup().remove((TermComponent) value); 2411 } else 2412 super.removeChild(name, value); 2413 2414 } 2415 2416 @Override 2417 public Base makeProperty(int hash, String name) throws FHIRException { 2418 switch (hash) { 2419 case -1618432855: 2420 return getIdentifier(); 2421 case -1179159893: 2422 return getIssuedElement(); 2423 case -793235316: 2424 return getApplies(); 2425 case -957295375: 2426 return getTopic(); 2427 case 110546223: 2428 return getTopic(); 2429 case 3575610: 2430 return getType(); 2431 case -1868521062: 2432 return getSubType(); 2433 case 3556653: 2434 return getTextElement(); 2435 case -722296940: 2436 return addSecurityLabel(); 2437 case 105650780: 2438 return getOffer(); 2439 case 93121264: 2440 return addAsset(); 2441 case -1422950858: 2442 return addAction(); 2443 case 98629247: 2444 return addGroup(); 2445 default: 2446 return super.makeProperty(hash, name); 2447 } 2448 2449 } 2450 2451 @Override 2452 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2453 switch (hash) { 2454 case -1618432855: 2455 /* identifier */ return new String[] { "Identifier" }; 2456 case -1179159893: 2457 /* issued */ return new String[] { "dateTime" }; 2458 case -793235316: 2459 /* applies */ return new String[] { "Period" }; 2460 case 110546223: 2461 /* topic */ return new String[] { "CodeableConcept", "Reference" }; 2462 case 3575610: 2463 /* type */ return new String[] { "CodeableConcept" }; 2464 case -1868521062: 2465 /* subType */ return new String[] { "CodeableConcept" }; 2466 case 3556653: 2467 /* text */ return new String[] { "string" }; 2468 case -722296940: 2469 /* securityLabel */ return new String[] {}; 2470 case 105650780: 2471 /* offer */ return new String[] {}; 2472 case 93121264: 2473 /* asset */ return new String[] {}; 2474 case -1422950858: 2475 /* action */ return new String[] {}; 2476 case 98629247: 2477 /* group */ return new String[] { "@Contract.term" }; 2478 default: 2479 return super.getTypesForProperty(hash, name); 2480 } 2481 2482 } 2483 2484 @Override 2485 public Base addChild(String name) throws FHIRException { 2486 if (name.equals("identifier")) { 2487 this.identifier = new Identifier(); 2488 return this.identifier; 2489 } else if (name.equals("issued")) { 2490 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 2491 } else if (name.equals("applies")) { 2492 this.applies = new Period(); 2493 return this.applies; 2494 } else if (name.equals("topicCodeableConcept")) { 2495 this.topic = new CodeableConcept(); 2496 return this.topic; 2497 } else if (name.equals("topicReference")) { 2498 this.topic = new Reference(); 2499 return this.topic; 2500 } else if (name.equals("type")) { 2501 this.type = new CodeableConcept(); 2502 return this.type; 2503 } else if (name.equals("subType")) { 2504 this.subType = new CodeableConcept(); 2505 return this.subType; 2506 } else if (name.equals("text")) { 2507 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 2508 } else if (name.equals("securityLabel")) { 2509 return addSecurityLabel(); 2510 } else if (name.equals("offer")) { 2511 this.offer = new ContractOfferComponent(); 2512 return this.offer; 2513 } else if (name.equals("asset")) { 2514 return addAsset(); 2515 } else if (name.equals("action")) { 2516 return addAction(); 2517 } else if (name.equals("group")) { 2518 return addGroup(); 2519 } else 2520 return super.addChild(name); 2521 } 2522 2523 public TermComponent copy() { 2524 TermComponent dst = new TermComponent(); 2525 copyValues(dst); 2526 return dst; 2527 } 2528 2529 public void copyValues(TermComponent dst) { 2530 super.copyValues(dst); 2531 dst.identifier = identifier == null ? null : identifier.copy(); 2532 dst.issued = issued == null ? null : issued.copy(); 2533 dst.applies = applies == null ? null : applies.copy(); 2534 dst.topic = topic == null ? null : topic.copy(); 2535 dst.type = type == null ? null : type.copy(); 2536 dst.subType = subType == null ? null : subType.copy(); 2537 dst.text = text == null ? null : text.copy(); 2538 if (securityLabel != null) { 2539 dst.securityLabel = new ArrayList<SecurityLabelComponent>(); 2540 for (SecurityLabelComponent i : securityLabel) 2541 dst.securityLabel.add(i.copy()); 2542 } 2543 ; 2544 dst.offer = offer == null ? null : offer.copy(); 2545 if (asset != null) { 2546 dst.asset = new ArrayList<ContractAssetComponent>(); 2547 for (ContractAssetComponent i : asset) 2548 dst.asset.add(i.copy()); 2549 } 2550 ; 2551 if (action != null) { 2552 dst.action = new ArrayList<ActionComponent>(); 2553 for (ActionComponent i : action) 2554 dst.action.add(i.copy()); 2555 } 2556 ; 2557 if (group != null) { 2558 dst.group = new ArrayList<TermComponent>(); 2559 for (TermComponent i : group) 2560 dst.group.add(i.copy()); 2561 } 2562 ; 2563 } 2564 2565 @Override 2566 public boolean equalsDeep(Base other_) { 2567 if (!super.equalsDeep(other_)) 2568 return false; 2569 if (!(other_ instanceof TermComponent)) 2570 return false; 2571 TermComponent o = (TermComponent) other_; 2572 return compareDeep(identifier, o.identifier, true) && compareDeep(issued, o.issued, true) 2573 && compareDeep(applies, o.applies, true) && compareDeep(topic, o.topic, true) 2574 && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) && compareDeep(text, o.text, true) 2575 && compareDeep(securityLabel, o.securityLabel, true) && compareDeep(offer, o.offer, true) 2576 && compareDeep(asset, o.asset, true) && compareDeep(action, o.action, true) 2577 && compareDeep(group, o.group, true); 2578 } 2579 2580 @Override 2581 public boolean equalsShallow(Base other_) { 2582 if (!super.equalsShallow(other_)) 2583 return false; 2584 if (!(other_ instanceof TermComponent)) 2585 return false; 2586 TermComponent o = (TermComponent) other_; 2587 return compareValues(issued, o.issued, true) && compareValues(text, o.text, true); 2588 } 2589 2590 public boolean isEmpty() { 2591 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, issued, applies, topic, type, subType, 2592 text, securityLabel, offer, asset, action, group); 2593 } 2594 2595 public String fhirType() { 2596 return "Contract.term"; 2597 2598 } 2599 2600 } 2601 2602 @Block() 2603 public static class SecurityLabelComponent extends BackboneElement implements IBaseBackboneElement { 2604 /** 2605 * Number used to link this term or term element to the applicable Security 2606 * Label. 2607 */ 2608 @Child(name = "number", type = { 2609 UnsignedIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2610 @Description(shortDefinition = "Link to Security Labels", formalDefinition = "Number used to link this term or term element to the applicable Security Label.") 2611 protected List<UnsignedIntType> number; 2612 2613 /** 2614 * Security label privacy tag that species the level of confidentiality 2615 * protection required for this term and/or term elements. 2616 */ 2617 @Child(name = "classification", type = { 2618 Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 2619 @Description(shortDefinition = "Confidentiality Protection", formalDefinition = "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.") 2620 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-classification") 2621 protected Coding classification; 2622 2623 /** 2624 * Security label privacy tag that species the applicable privacy and security 2625 * policies governing this term and/or term elements. 2626 */ 2627 @Child(name = "category", type = { 2628 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2629 @Description(shortDefinition = "Applicable Policy", formalDefinition = "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.") 2630 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-category") 2631 protected List<Coding> category; 2632 2633 /** 2634 * Security label privacy tag that species the manner in which term and/or term 2635 * elements are to be protected. 2636 */ 2637 @Child(name = "control", type = { 2638 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2639 @Description(shortDefinition = "Handling Instructions", formalDefinition = "Security label privacy tag that species the manner in which term and/or term elements are to be protected.") 2640 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-security-control") 2641 protected List<Coding> control; 2642 2643 private static final long serialVersionUID = 788281758L; 2644 2645 /** 2646 * Constructor 2647 */ 2648 public SecurityLabelComponent() { 2649 super(); 2650 } 2651 2652 /** 2653 * Constructor 2654 */ 2655 public SecurityLabelComponent(Coding classification) { 2656 super(); 2657 this.classification = classification; 2658 } 2659 2660 /** 2661 * @return {@link #number} (Number used to link this term or term element to the 2662 * applicable Security Label.) 2663 */ 2664 public List<UnsignedIntType> getNumber() { 2665 if (this.number == null) 2666 this.number = new ArrayList<UnsignedIntType>(); 2667 return this.number; 2668 } 2669 2670 /** 2671 * @return Returns a reference to <code>this</code> for easy method chaining 2672 */ 2673 public SecurityLabelComponent setNumber(List<UnsignedIntType> theNumber) { 2674 this.number = theNumber; 2675 return this; 2676 } 2677 2678 public boolean hasNumber() { 2679 if (this.number == null) 2680 return false; 2681 for (UnsignedIntType item : this.number) 2682 if (!item.isEmpty()) 2683 return true; 2684 return false; 2685 } 2686 2687 /** 2688 * @return {@link #number} (Number used to link this term or term element to the 2689 * applicable Security Label.) 2690 */ 2691 public UnsignedIntType addNumberElement() {// 2 2692 UnsignedIntType t = new UnsignedIntType(); 2693 if (this.number == null) 2694 this.number = new ArrayList<UnsignedIntType>(); 2695 this.number.add(t); 2696 return t; 2697 } 2698 2699 /** 2700 * @param value {@link #number} (Number used to link this term or term element 2701 * to the applicable Security Label.) 2702 */ 2703 public SecurityLabelComponent addNumber(int value) { // 1 2704 UnsignedIntType t = new UnsignedIntType(); 2705 t.setValue(value); 2706 if (this.number == null) 2707 this.number = new ArrayList<UnsignedIntType>(); 2708 this.number.add(t); 2709 return this; 2710 } 2711 2712 /** 2713 * @param value {@link #number} (Number used to link this term or term element 2714 * to the applicable Security Label.) 2715 */ 2716 public boolean hasNumber(int value) { 2717 if (this.number == null) 2718 return false; 2719 for (UnsignedIntType v : this.number) 2720 if (v.getValue().equals(value)) // unsignedInt 2721 return true; 2722 return false; 2723 } 2724 2725 /** 2726 * @return {@link #classification} (Security label privacy tag that species the 2727 * level of confidentiality protection required for this term and/or 2728 * term elements.) 2729 */ 2730 public Coding getClassification() { 2731 if (this.classification == null) 2732 if (Configuration.errorOnAutoCreate()) 2733 throw new Error("Attempt to auto-create SecurityLabelComponent.classification"); 2734 else if (Configuration.doAutoCreate()) 2735 this.classification = new Coding(); // cc 2736 return this.classification; 2737 } 2738 2739 public boolean hasClassification() { 2740 return this.classification != null && !this.classification.isEmpty(); 2741 } 2742 2743 /** 2744 * @param value {@link #classification} (Security label privacy tag that species 2745 * the level of confidentiality protection required for this term 2746 * and/or term elements.) 2747 */ 2748 public SecurityLabelComponent setClassification(Coding value) { 2749 this.classification = value; 2750 return this; 2751 } 2752 2753 /** 2754 * @return {@link #category} (Security label privacy tag that species the 2755 * applicable privacy and security policies governing this term and/or 2756 * term elements.) 2757 */ 2758 public List<Coding> getCategory() { 2759 if (this.category == null) 2760 this.category = new ArrayList<Coding>(); 2761 return this.category; 2762 } 2763 2764 /** 2765 * @return Returns a reference to <code>this</code> for easy method chaining 2766 */ 2767 public SecurityLabelComponent setCategory(List<Coding> theCategory) { 2768 this.category = theCategory; 2769 return this; 2770 } 2771 2772 public boolean hasCategory() { 2773 if (this.category == null) 2774 return false; 2775 for (Coding item : this.category) 2776 if (!item.isEmpty()) 2777 return true; 2778 return false; 2779 } 2780 2781 public Coding addCategory() { // 3 2782 Coding t = new Coding(); 2783 if (this.category == null) 2784 this.category = new ArrayList<Coding>(); 2785 this.category.add(t); 2786 return t; 2787 } 2788 2789 public SecurityLabelComponent addCategory(Coding t) { // 3 2790 if (t == null) 2791 return this; 2792 if (this.category == null) 2793 this.category = new ArrayList<Coding>(); 2794 this.category.add(t); 2795 return this; 2796 } 2797 2798 /** 2799 * @return The first repetition of repeating field {@link #category}, creating 2800 * it if it does not already exist 2801 */ 2802 public Coding getCategoryFirstRep() { 2803 if (getCategory().isEmpty()) { 2804 addCategory(); 2805 } 2806 return getCategory().get(0); 2807 } 2808 2809 /** 2810 * @return {@link #control} (Security label privacy tag that species the manner 2811 * in which term and/or term elements are to be protected.) 2812 */ 2813 public List<Coding> getControl() { 2814 if (this.control == null) 2815 this.control = new ArrayList<Coding>(); 2816 return this.control; 2817 } 2818 2819 /** 2820 * @return Returns a reference to <code>this</code> for easy method chaining 2821 */ 2822 public SecurityLabelComponent setControl(List<Coding> theControl) { 2823 this.control = theControl; 2824 return this; 2825 } 2826 2827 public boolean hasControl() { 2828 if (this.control == null) 2829 return false; 2830 for (Coding item : this.control) 2831 if (!item.isEmpty()) 2832 return true; 2833 return false; 2834 } 2835 2836 public Coding addControl() { // 3 2837 Coding t = new Coding(); 2838 if (this.control == null) 2839 this.control = new ArrayList<Coding>(); 2840 this.control.add(t); 2841 return t; 2842 } 2843 2844 public SecurityLabelComponent addControl(Coding t) { // 3 2845 if (t == null) 2846 return this; 2847 if (this.control == null) 2848 this.control = new ArrayList<Coding>(); 2849 this.control.add(t); 2850 return this; 2851 } 2852 2853 /** 2854 * @return The first repetition of repeating field {@link #control}, creating it 2855 * if it does not already exist 2856 */ 2857 public Coding getControlFirstRep() { 2858 if (getControl().isEmpty()) { 2859 addControl(); 2860 } 2861 return getControl().get(0); 2862 } 2863 2864 protected void listChildren(List<Property> children) { 2865 super.listChildren(children); 2866 children.add(new Property("number", "unsignedInt", 2867 "Number used to link this term or term element to the applicable Security Label.", 0, 2868 java.lang.Integer.MAX_VALUE, number)); 2869 children.add(new Property("classification", "Coding", 2870 "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.", 2871 0, 1, classification)); 2872 children.add(new Property("category", "Coding", 2873 "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.", 2874 0, java.lang.Integer.MAX_VALUE, category)); 2875 children.add(new Property("control", "Coding", 2876 "Security label privacy tag that species the manner in which term and/or term elements are to be protected.", 2877 0, java.lang.Integer.MAX_VALUE, control)); 2878 } 2879 2880 @Override 2881 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2882 switch (_hash) { 2883 case -1034364087: 2884 /* number */ return new Property("number", "unsignedInt", 2885 "Number used to link this term or term element to the applicable Security Label.", 0, 2886 java.lang.Integer.MAX_VALUE, number); 2887 case 382350310: 2888 /* classification */ return new Property("classification", "Coding", 2889 "Security label privacy tag that species the level of confidentiality protection required for this term and/or term elements.", 2890 0, 1, classification); 2891 case 50511102: 2892 /* category */ return new Property("category", "Coding", 2893 "Security label privacy tag that species the applicable privacy and security policies governing this term and/or term elements.", 2894 0, java.lang.Integer.MAX_VALUE, category); 2895 case 951543133: 2896 /* control */ return new Property("control", "Coding", 2897 "Security label privacy tag that species the manner in which term and/or term elements are to be protected.", 2898 0, java.lang.Integer.MAX_VALUE, control); 2899 default: 2900 return super.getNamedProperty(_hash, _name, _checkValid); 2901 } 2902 2903 } 2904 2905 @Override 2906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2907 switch (hash) { 2908 case -1034364087: 2909 /* number */ return this.number == null ? new Base[0] : this.number.toArray(new Base[this.number.size()]); // UnsignedIntType 2910 case 382350310: 2911 /* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // Coding 2912 case 50511102: 2913 /* category */ return this.category == null ? new Base[0] 2914 : this.category.toArray(new Base[this.category.size()]); // Coding 2915 case 951543133: 2916 /* control */ return this.control == null ? new Base[0] : this.control.toArray(new Base[this.control.size()]); // Coding 2917 default: 2918 return super.getProperty(hash, name, checkValid); 2919 } 2920 2921 } 2922 2923 @Override 2924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2925 switch (hash) { 2926 case -1034364087: // number 2927 this.getNumber().add(castToUnsignedInt(value)); // UnsignedIntType 2928 return value; 2929 case 382350310: // classification 2930 this.classification = castToCoding(value); // Coding 2931 return value; 2932 case 50511102: // category 2933 this.getCategory().add(castToCoding(value)); // Coding 2934 return value; 2935 case 951543133: // control 2936 this.getControl().add(castToCoding(value)); // Coding 2937 return value; 2938 default: 2939 return super.setProperty(hash, name, value); 2940 } 2941 2942 } 2943 2944 @Override 2945 public Base setProperty(String name, Base value) throws FHIRException { 2946 if (name.equals("number")) { 2947 this.getNumber().add(castToUnsignedInt(value)); 2948 } else if (name.equals("classification")) { 2949 this.classification = castToCoding(value); // Coding 2950 } else if (name.equals("category")) { 2951 this.getCategory().add(castToCoding(value)); 2952 } else if (name.equals("control")) { 2953 this.getControl().add(castToCoding(value)); 2954 } else 2955 return super.setProperty(name, value); 2956 return value; 2957 } 2958 2959 @Override 2960 public void removeChild(String name, Base value) throws FHIRException { 2961 if (name.equals("number")) { 2962 this.getNumber().remove(castToUnsignedInt(value)); 2963 } else if (name.equals("classification")) { 2964 this.classification = null; 2965 } else if (name.equals("category")) { 2966 this.getCategory().remove(castToCoding(value)); 2967 } else if (name.equals("control")) { 2968 this.getControl().remove(castToCoding(value)); 2969 } else 2970 super.removeChild(name, value); 2971 2972 } 2973 2974 @Override 2975 public Base makeProperty(int hash, String name) throws FHIRException { 2976 switch (hash) { 2977 case -1034364087: 2978 return addNumberElement(); 2979 case 382350310: 2980 return getClassification(); 2981 case 50511102: 2982 return addCategory(); 2983 case 951543133: 2984 return addControl(); 2985 default: 2986 return super.makeProperty(hash, name); 2987 } 2988 2989 } 2990 2991 @Override 2992 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2993 switch (hash) { 2994 case -1034364087: 2995 /* number */ return new String[] { "unsignedInt" }; 2996 case 382350310: 2997 /* classification */ return new String[] { "Coding" }; 2998 case 50511102: 2999 /* category */ return new String[] { "Coding" }; 3000 case 951543133: 3001 /* control */ return new String[] { "Coding" }; 3002 default: 3003 return super.getTypesForProperty(hash, name); 3004 } 3005 3006 } 3007 3008 @Override 3009 public Base addChild(String name) throws FHIRException { 3010 if (name.equals("number")) { 3011 throw new FHIRException("Cannot call addChild on a singleton property Contract.number"); 3012 } else if (name.equals("classification")) { 3013 this.classification = new Coding(); 3014 return this.classification; 3015 } else if (name.equals("category")) { 3016 return addCategory(); 3017 } else if (name.equals("control")) { 3018 return addControl(); 3019 } else 3020 return super.addChild(name); 3021 } 3022 3023 public SecurityLabelComponent copy() { 3024 SecurityLabelComponent dst = new SecurityLabelComponent(); 3025 copyValues(dst); 3026 return dst; 3027 } 3028 3029 public void copyValues(SecurityLabelComponent dst) { 3030 super.copyValues(dst); 3031 if (number != null) { 3032 dst.number = new ArrayList<UnsignedIntType>(); 3033 for (UnsignedIntType i : number) 3034 dst.number.add(i.copy()); 3035 } 3036 ; 3037 dst.classification = classification == null ? null : classification.copy(); 3038 if (category != null) { 3039 dst.category = new ArrayList<Coding>(); 3040 for (Coding i : category) 3041 dst.category.add(i.copy()); 3042 } 3043 ; 3044 if (control != null) { 3045 dst.control = new ArrayList<Coding>(); 3046 for (Coding i : control) 3047 dst.control.add(i.copy()); 3048 } 3049 ; 3050 } 3051 3052 @Override 3053 public boolean equalsDeep(Base other_) { 3054 if (!super.equalsDeep(other_)) 3055 return false; 3056 if (!(other_ instanceof SecurityLabelComponent)) 3057 return false; 3058 SecurityLabelComponent o = (SecurityLabelComponent) other_; 3059 return compareDeep(number, o.number, true) && compareDeep(classification, o.classification, true) 3060 && compareDeep(category, o.category, true) && compareDeep(control, o.control, true); 3061 } 3062 3063 @Override 3064 public boolean equalsShallow(Base other_) { 3065 if (!super.equalsShallow(other_)) 3066 return false; 3067 if (!(other_ instanceof SecurityLabelComponent)) 3068 return false; 3069 SecurityLabelComponent o = (SecurityLabelComponent) other_; 3070 return compareValues(number, o.number, true); 3071 } 3072 3073 public boolean isEmpty() { 3074 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, classification, category, control); 3075 } 3076 3077 public String fhirType() { 3078 return "Contract.term.securityLabel"; 3079 3080 } 3081 3082 } 3083 3084 @Block() 3085 public static class ContractOfferComponent extends BackboneElement implements IBaseBackboneElement { 3086 /** 3087 * Unique identifier for this particular Contract Provision. 3088 */ 3089 @Child(name = "identifier", type = { 3090 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3091 @Description(shortDefinition = "Offer business ID", formalDefinition = "Unique identifier for this particular Contract Provision.") 3092 protected List<Identifier> identifier; 3093 3094 /** 3095 * Offer Recipient. 3096 */ 3097 @Child(name = "party", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3098 @Description(shortDefinition = "Offer Recipient", formalDefinition = "Offer Recipient.") 3099 protected List<ContractPartyComponent> party; 3100 3101 /** 3102 * The owner of an asset has the residual control rights over the asset: the 3103 * right to decide all usages of the asset in any way not inconsistent with a 3104 * prior contract, custom, or law (Hart, 1995, p. 30). 3105 */ 3106 @Child(name = "topic", type = { Reference.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3107 @Description(shortDefinition = "Negotiable offer asset", formalDefinition = "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).") 3108 protected Reference topic; 3109 3110 /** 3111 * The actual object that is the target of the reference (The owner of an asset 3112 * has the residual control rights over the asset: the right to decide all 3113 * usages of the asset in any way not inconsistent with a prior contract, 3114 * custom, or law (Hart, 1995, p. 30).) 3115 */ 3116 protected Resource topicTarget; 3117 3118 /** 3119 * Type of Contract Provision such as specific requirements, purposes for 3120 * actions, obligations, prohibitions, e.g. life time maximum benefit. 3121 */ 3122 @Child(name = "type", type = { 3123 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3124 @Description(shortDefinition = "Contract Offer Type or Form", formalDefinition = "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.") 3125 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-term-type") 3126 protected CodeableConcept type; 3127 3128 /** 3129 * Type of choice made by accepting party with respect to an offer made by an 3130 * offeror/ grantee. 3131 */ 3132 @Child(name = "decision", type = { 3133 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3134 @Description(shortDefinition = "Accepting party choice", formalDefinition = "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.") 3135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActConsentDirective") 3136 protected CodeableConcept decision; 3137 3138 /** 3139 * How the decision about a Contract was conveyed. 3140 */ 3141 @Child(name = "decisionMode", type = { 3142 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3143 @Description(shortDefinition = "How decision is conveyed", formalDefinition = "How the decision about a Contract was conveyed.") 3144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-decision-mode") 3145 protected List<CodeableConcept> decisionMode; 3146 3147 /** 3148 * Response to offer text. 3149 */ 3150 @Child(name = "answer", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3151 @Description(shortDefinition = "Response to offer text", formalDefinition = "Response to offer text.") 3152 protected List<AnswerComponent> answer; 3153 3154 /** 3155 * Human readable form of this Contract Offer. 3156 */ 3157 @Child(name = "text", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 3158 @Description(shortDefinition = "Human readable offer text", formalDefinition = "Human readable form of this Contract Offer.") 3159 protected StringType text; 3160 3161 /** 3162 * The id of the clause or question text of the offer in the referenced 3163 * questionnaire/response. 3164 */ 3165 @Child(name = "linkId", type = { 3166 StringType.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3167 @Description(shortDefinition = "Pointer to text", formalDefinition = "The id of the clause or question text of the offer in the referenced questionnaire/response.") 3168 protected List<StringType> linkId; 3169 3170 /** 3171 * Security labels that protects the offer. 3172 */ 3173 @Child(name = "securityLabelNumber", type = { 3174 UnsignedIntType.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3175 @Description(shortDefinition = "Offer restriction numbers", formalDefinition = "Security labels that protects the offer.") 3176 protected List<UnsignedIntType> securityLabelNumber; 3177 3178 private static final long serialVersionUID = -395674449L; 3179 3180 /** 3181 * Constructor 3182 */ 3183 public ContractOfferComponent() { 3184 super(); 3185 } 3186 3187 /** 3188 * @return {@link #identifier} (Unique identifier for this particular Contract 3189 * Provision.) 3190 */ 3191 public List<Identifier> getIdentifier() { 3192 if (this.identifier == null) 3193 this.identifier = new ArrayList<Identifier>(); 3194 return this.identifier; 3195 } 3196 3197 /** 3198 * @return Returns a reference to <code>this</code> for easy method chaining 3199 */ 3200 public ContractOfferComponent setIdentifier(List<Identifier> theIdentifier) { 3201 this.identifier = theIdentifier; 3202 return this; 3203 } 3204 3205 public boolean hasIdentifier() { 3206 if (this.identifier == null) 3207 return false; 3208 for (Identifier item : this.identifier) 3209 if (!item.isEmpty()) 3210 return true; 3211 return false; 3212 } 3213 3214 public Identifier addIdentifier() { // 3 3215 Identifier t = new Identifier(); 3216 if (this.identifier == null) 3217 this.identifier = new ArrayList<Identifier>(); 3218 this.identifier.add(t); 3219 return t; 3220 } 3221 3222 public ContractOfferComponent addIdentifier(Identifier t) { // 3 3223 if (t == null) 3224 return this; 3225 if (this.identifier == null) 3226 this.identifier = new ArrayList<Identifier>(); 3227 this.identifier.add(t); 3228 return this; 3229 } 3230 3231 /** 3232 * @return The first repetition of repeating field {@link #identifier}, creating 3233 * it if it does not already exist 3234 */ 3235 public Identifier getIdentifierFirstRep() { 3236 if (getIdentifier().isEmpty()) { 3237 addIdentifier(); 3238 } 3239 return getIdentifier().get(0); 3240 } 3241 3242 /** 3243 * @return {@link #party} (Offer Recipient.) 3244 */ 3245 public List<ContractPartyComponent> getParty() { 3246 if (this.party == null) 3247 this.party = new ArrayList<ContractPartyComponent>(); 3248 return this.party; 3249 } 3250 3251 /** 3252 * @return Returns a reference to <code>this</code> for easy method chaining 3253 */ 3254 public ContractOfferComponent setParty(List<ContractPartyComponent> theParty) { 3255 this.party = theParty; 3256 return this; 3257 } 3258 3259 public boolean hasParty() { 3260 if (this.party == null) 3261 return false; 3262 for (ContractPartyComponent item : this.party) 3263 if (!item.isEmpty()) 3264 return true; 3265 return false; 3266 } 3267 3268 public ContractPartyComponent addParty() { // 3 3269 ContractPartyComponent t = new ContractPartyComponent(); 3270 if (this.party == null) 3271 this.party = new ArrayList<ContractPartyComponent>(); 3272 this.party.add(t); 3273 return t; 3274 } 3275 3276 public ContractOfferComponent addParty(ContractPartyComponent t) { // 3 3277 if (t == null) 3278 return this; 3279 if (this.party == null) 3280 this.party = new ArrayList<ContractPartyComponent>(); 3281 this.party.add(t); 3282 return this; 3283 } 3284 3285 /** 3286 * @return The first repetition of repeating field {@link #party}, creating it 3287 * if it does not already exist 3288 */ 3289 public ContractPartyComponent getPartyFirstRep() { 3290 if (getParty().isEmpty()) { 3291 addParty(); 3292 } 3293 return getParty().get(0); 3294 } 3295 3296 /** 3297 * @return {@link #topic} (The owner of an asset has the residual control rights 3298 * over the asset: the right to decide all usages of the asset in any 3299 * way not inconsistent with a prior contract, custom, or law (Hart, 3300 * 1995, p. 30).) 3301 */ 3302 public Reference getTopic() { 3303 if (this.topic == null) 3304 if (Configuration.errorOnAutoCreate()) 3305 throw new Error("Attempt to auto-create ContractOfferComponent.topic"); 3306 else if (Configuration.doAutoCreate()) 3307 this.topic = new Reference(); // cc 3308 return this.topic; 3309 } 3310 3311 public boolean hasTopic() { 3312 return this.topic != null && !this.topic.isEmpty(); 3313 } 3314 3315 /** 3316 * @param value {@link #topic} (The owner of an asset has the residual control 3317 * rights over the asset: the right to decide all usages of the 3318 * asset in any way not inconsistent with a prior contract, custom, 3319 * or law (Hart, 1995, p. 30).) 3320 */ 3321 public ContractOfferComponent setTopic(Reference value) { 3322 this.topic = value; 3323 return this; 3324 } 3325 3326 /** 3327 * @return {@link #topic} The actual object that is the target of the reference. 3328 * The reference library doesn't populate this, but you can use it to 3329 * hold the resource if you resolve it. (The owner of an asset has the 3330 * residual control rights over the asset: the right to decide all 3331 * usages of the asset in any way not inconsistent with a prior 3332 * contract, custom, or law (Hart, 1995, p. 30).) 3333 */ 3334 public Resource getTopicTarget() { 3335 return this.topicTarget; 3336 } 3337 3338 /** 3339 * @param value {@link #topic} The actual object that is the target of the 3340 * reference. The reference library doesn't use these, but you can 3341 * use it to hold the resource if you resolve it. (The owner of an 3342 * asset has the residual control rights over the asset: the right 3343 * to decide all usages of the asset in any way not inconsistent 3344 * with a prior contract, custom, or law (Hart, 1995, p. 30).) 3345 */ 3346 public ContractOfferComponent setTopicTarget(Resource value) { 3347 this.topicTarget = value; 3348 return this; 3349 } 3350 3351 /** 3352 * @return {@link #type} (Type of Contract Provision such as specific 3353 * requirements, purposes for actions, obligations, prohibitions, e.g. 3354 * life time maximum benefit.) 3355 */ 3356 public CodeableConcept getType() { 3357 if (this.type == null) 3358 if (Configuration.errorOnAutoCreate()) 3359 throw new Error("Attempt to auto-create ContractOfferComponent.type"); 3360 else if (Configuration.doAutoCreate()) 3361 this.type = new CodeableConcept(); // cc 3362 return this.type; 3363 } 3364 3365 public boolean hasType() { 3366 return this.type != null && !this.type.isEmpty(); 3367 } 3368 3369 /** 3370 * @param value {@link #type} (Type of Contract Provision such as specific 3371 * requirements, purposes for actions, obligations, prohibitions, 3372 * e.g. life time maximum benefit.) 3373 */ 3374 public ContractOfferComponent setType(CodeableConcept value) { 3375 this.type = value; 3376 return this; 3377 } 3378 3379 /** 3380 * @return {@link #decision} (Type of choice made by accepting party with 3381 * respect to an offer made by an offeror/ grantee.) 3382 */ 3383 public CodeableConcept getDecision() { 3384 if (this.decision == null) 3385 if (Configuration.errorOnAutoCreate()) 3386 throw new Error("Attempt to auto-create ContractOfferComponent.decision"); 3387 else if (Configuration.doAutoCreate()) 3388 this.decision = new CodeableConcept(); // cc 3389 return this.decision; 3390 } 3391 3392 public boolean hasDecision() { 3393 return this.decision != null && !this.decision.isEmpty(); 3394 } 3395 3396 /** 3397 * @param value {@link #decision} (Type of choice made by accepting party with 3398 * respect to an offer made by an offeror/ grantee.) 3399 */ 3400 public ContractOfferComponent setDecision(CodeableConcept value) { 3401 this.decision = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #decisionMode} (How the decision about a Contract was 3407 * conveyed.) 3408 */ 3409 public List<CodeableConcept> getDecisionMode() { 3410 if (this.decisionMode == null) 3411 this.decisionMode = new ArrayList<CodeableConcept>(); 3412 return this.decisionMode; 3413 } 3414 3415 /** 3416 * @return Returns a reference to <code>this</code> for easy method chaining 3417 */ 3418 public ContractOfferComponent setDecisionMode(List<CodeableConcept> theDecisionMode) { 3419 this.decisionMode = theDecisionMode; 3420 return this; 3421 } 3422 3423 public boolean hasDecisionMode() { 3424 if (this.decisionMode == null) 3425 return false; 3426 for (CodeableConcept item : this.decisionMode) 3427 if (!item.isEmpty()) 3428 return true; 3429 return false; 3430 } 3431 3432 public CodeableConcept addDecisionMode() { // 3 3433 CodeableConcept t = new CodeableConcept(); 3434 if (this.decisionMode == null) 3435 this.decisionMode = new ArrayList<CodeableConcept>(); 3436 this.decisionMode.add(t); 3437 return t; 3438 } 3439 3440 public ContractOfferComponent addDecisionMode(CodeableConcept t) { // 3 3441 if (t == null) 3442 return this; 3443 if (this.decisionMode == null) 3444 this.decisionMode = new ArrayList<CodeableConcept>(); 3445 this.decisionMode.add(t); 3446 return this; 3447 } 3448 3449 /** 3450 * @return The first repetition of repeating field {@link #decisionMode}, 3451 * creating it if it does not already exist 3452 */ 3453 public CodeableConcept getDecisionModeFirstRep() { 3454 if (getDecisionMode().isEmpty()) { 3455 addDecisionMode(); 3456 } 3457 return getDecisionMode().get(0); 3458 } 3459 3460 /** 3461 * @return {@link #answer} (Response to offer text.) 3462 */ 3463 public List<AnswerComponent> getAnswer() { 3464 if (this.answer == null) 3465 this.answer = new ArrayList<AnswerComponent>(); 3466 return this.answer; 3467 } 3468 3469 /** 3470 * @return Returns a reference to <code>this</code> for easy method chaining 3471 */ 3472 public ContractOfferComponent setAnswer(List<AnswerComponent> theAnswer) { 3473 this.answer = theAnswer; 3474 return this; 3475 } 3476 3477 public boolean hasAnswer() { 3478 if (this.answer == null) 3479 return false; 3480 for (AnswerComponent item : this.answer) 3481 if (!item.isEmpty()) 3482 return true; 3483 return false; 3484 } 3485 3486 public AnswerComponent addAnswer() { // 3 3487 AnswerComponent t = new AnswerComponent(); 3488 if (this.answer == null) 3489 this.answer = new ArrayList<AnswerComponent>(); 3490 this.answer.add(t); 3491 return t; 3492 } 3493 3494 public ContractOfferComponent addAnswer(AnswerComponent t) { // 3 3495 if (t == null) 3496 return this; 3497 if (this.answer == null) 3498 this.answer = new ArrayList<AnswerComponent>(); 3499 this.answer.add(t); 3500 return this; 3501 } 3502 3503 /** 3504 * @return The first repetition of repeating field {@link #answer}, creating it 3505 * if it does not already exist 3506 */ 3507 public AnswerComponent getAnswerFirstRep() { 3508 if (getAnswer().isEmpty()) { 3509 addAnswer(); 3510 } 3511 return getAnswer().get(0); 3512 } 3513 3514 /** 3515 * @return {@link #text} (Human readable form of this Contract Offer.). This is 3516 * the underlying object with id, value and extensions. The accessor 3517 * "getText" gives direct access to the value 3518 */ 3519 public StringType getTextElement() { 3520 if (this.text == null) 3521 if (Configuration.errorOnAutoCreate()) 3522 throw new Error("Attempt to auto-create ContractOfferComponent.text"); 3523 else if (Configuration.doAutoCreate()) 3524 this.text = new StringType(); // bb 3525 return this.text; 3526 } 3527 3528 public boolean hasTextElement() { 3529 return this.text != null && !this.text.isEmpty(); 3530 } 3531 3532 public boolean hasText() { 3533 return this.text != null && !this.text.isEmpty(); 3534 } 3535 3536 /** 3537 * @param value {@link #text} (Human readable form of this Contract Offer.). 3538 * This is the underlying object with id, value and extensions. The 3539 * accessor "getText" gives direct access to the value 3540 */ 3541 public ContractOfferComponent setTextElement(StringType value) { 3542 this.text = value; 3543 return this; 3544 } 3545 3546 /** 3547 * @return Human readable form of this Contract Offer. 3548 */ 3549 public String getText() { 3550 return this.text == null ? null : this.text.getValue(); 3551 } 3552 3553 /** 3554 * @param value Human readable form of this Contract Offer. 3555 */ 3556 public ContractOfferComponent setText(String value) { 3557 if (Utilities.noString(value)) 3558 this.text = null; 3559 else { 3560 if (this.text == null) 3561 this.text = new StringType(); 3562 this.text.setValue(value); 3563 } 3564 return this; 3565 } 3566 3567 /** 3568 * @return {@link #linkId} (The id of the clause or question text of the offer 3569 * in the referenced questionnaire/response.) 3570 */ 3571 public List<StringType> getLinkId() { 3572 if (this.linkId == null) 3573 this.linkId = new ArrayList<StringType>(); 3574 return this.linkId; 3575 } 3576 3577 /** 3578 * @return Returns a reference to <code>this</code> for easy method chaining 3579 */ 3580 public ContractOfferComponent setLinkId(List<StringType> theLinkId) { 3581 this.linkId = theLinkId; 3582 return this; 3583 } 3584 3585 public boolean hasLinkId() { 3586 if (this.linkId == null) 3587 return false; 3588 for (StringType item : this.linkId) 3589 if (!item.isEmpty()) 3590 return true; 3591 return false; 3592 } 3593 3594 /** 3595 * @return {@link #linkId} (The id of the clause or question text of the offer 3596 * in the referenced questionnaire/response.) 3597 */ 3598 public StringType addLinkIdElement() {// 2 3599 StringType t = new StringType(); 3600 if (this.linkId == null) 3601 this.linkId = new ArrayList<StringType>(); 3602 this.linkId.add(t); 3603 return t; 3604 } 3605 3606 /** 3607 * @param value {@link #linkId} (The id of the clause or question text of the 3608 * offer in the referenced questionnaire/response.) 3609 */ 3610 public ContractOfferComponent addLinkId(String value) { // 1 3611 StringType t = new StringType(); 3612 t.setValue(value); 3613 if (this.linkId == null) 3614 this.linkId = new ArrayList<StringType>(); 3615 this.linkId.add(t); 3616 return this; 3617 } 3618 3619 /** 3620 * @param value {@link #linkId} (The id of the clause or question text of the 3621 * offer in the referenced questionnaire/response.) 3622 */ 3623 public boolean hasLinkId(String value) { 3624 if (this.linkId == null) 3625 return false; 3626 for (StringType v : this.linkId) 3627 if (v.getValue().equals(value)) // string 3628 return true; 3629 return false; 3630 } 3631 3632 /** 3633 * @return {@link #securityLabelNumber} (Security labels that protects the 3634 * offer.) 3635 */ 3636 public List<UnsignedIntType> getSecurityLabelNumber() { 3637 if (this.securityLabelNumber == null) 3638 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3639 return this.securityLabelNumber; 3640 } 3641 3642 /** 3643 * @return Returns a reference to <code>this</code> for easy method chaining 3644 */ 3645 public ContractOfferComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 3646 this.securityLabelNumber = theSecurityLabelNumber; 3647 return this; 3648 } 3649 3650 public boolean hasSecurityLabelNumber() { 3651 if (this.securityLabelNumber == null) 3652 return false; 3653 for (UnsignedIntType item : this.securityLabelNumber) 3654 if (!item.isEmpty()) 3655 return true; 3656 return false; 3657 } 3658 3659 /** 3660 * @return {@link #securityLabelNumber} (Security labels that protects the 3661 * offer.) 3662 */ 3663 public UnsignedIntType addSecurityLabelNumberElement() {// 2 3664 UnsignedIntType t = new UnsignedIntType(); 3665 if (this.securityLabelNumber == null) 3666 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3667 this.securityLabelNumber.add(t); 3668 return t; 3669 } 3670 3671 /** 3672 * @param value {@link #securityLabelNumber} (Security labels that protects the 3673 * offer.) 3674 */ 3675 public ContractOfferComponent addSecurityLabelNumber(int value) { // 1 3676 UnsignedIntType t = new UnsignedIntType(); 3677 t.setValue(value); 3678 if (this.securityLabelNumber == null) 3679 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 3680 this.securityLabelNumber.add(t); 3681 return this; 3682 } 3683 3684 /** 3685 * @param value {@link #securityLabelNumber} (Security labels that protects the 3686 * offer.) 3687 */ 3688 public boolean hasSecurityLabelNumber(int value) { 3689 if (this.securityLabelNumber == null) 3690 return false; 3691 for (UnsignedIntType v : this.securityLabelNumber) 3692 if (v.getValue().equals(value)) // unsignedInt 3693 return true; 3694 return false; 3695 } 3696 3697 protected void listChildren(List<Property> children) { 3698 super.listChildren(children); 3699 children.add(new Property("identifier", "Identifier", "Unique identifier for this particular Contract Provision.", 3700 0, java.lang.Integer.MAX_VALUE, identifier)); 3701 children.add(new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party)); 3702 children.add(new Property("topic", "Reference(Any)", 3703 "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 3704 0, 1, topic)); 3705 children.add(new Property("type", "CodeableConcept", 3706 "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 3707 0, 1, type)); 3708 children.add(new Property("decision", "CodeableConcept", 3709 "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, 3710 decision)); 3711 children.add(new Property("decisionMode", "CodeableConcept", "How the decision about a Contract was conveyed.", 0, 3712 java.lang.Integer.MAX_VALUE, decisionMode)); 3713 children.add(new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, answer)); 3714 children.add(new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text)); 3715 children.add(new Property("linkId", "string", 3716 "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, 3717 java.lang.Integer.MAX_VALUE, linkId)); 3718 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the offer.", 0, 3719 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 3720 } 3721 3722 @Override 3723 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3724 switch (_hash) { 3725 case -1618432855: 3726 /* identifier */ return new Property("identifier", "Identifier", 3727 "Unique identifier for this particular Contract Provision.", 0, java.lang.Integer.MAX_VALUE, identifier); 3728 case 106437350: 3729 /* party */ return new Property("party", "", "Offer Recipient.", 0, java.lang.Integer.MAX_VALUE, party); 3730 case 110546223: 3731 /* topic */ return new Property("topic", "Reference(Any)", 3732 "The owner of an asset has the residual control rights over the asset: the right to decide all usages of the asset in any way not inconsistent with a prior contract, custom, or law (Hart, 1995, p. 30).", 3733 0, 1, topic); 3734 case 3575610: 3735 /* type */ return new Property("type", "CodeableConcept", 3736 "Type of Contract Provision such as specific requirements, purposes for actions, obligations, prohibitions, e.g. life time maximum benefit.", 3737 0, 1, type); 3738 case 565719004: 3739 /* decision */ return new Property("decision", "CodeableConcept", 3740 "Type of choice made by accepting party with respect to an offer made by an offeror/ grantee.", 0, 1, 3741 decision); 3742 case 675909535: 3743 /* decisionMode */ return new Property("decisionMode", "CodeableConcept", 3744 "How the decision about a Contract was conveyed.", 0, java.lang.Integer.MAX_VALUE, decisionMode); 3745 case -1412808770: 3746 /* answer */ return new Property("answer", "", "Response to offer text.", 0, java.lang.Integer.MAX_VALUE, 3747 answer); 3748 case 3556653: 3749 /* text */ return new Property("text", "string", "Human readable form of this Contract Offer.", 0, 1, text); 3750 case -1102667083: 3751 /* linkId */ return new Property("linkId", "string", 3752 "The id of the clause or question text of the offer in the referenced questionnaire/response.", 0, 3753 java.lang.Integer.MAX_VALUE, linkId); 3754 case -149460995: 3755 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 3756 "Security labels that protects the offer.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 3757 default: 3758 return super.getNamedProperty(_hash, _name, _checkValid); 3759 } 3760 3761 } 3762 3763 @Override 3764 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3765 switch (hash) { 3766 case -1618432855: 3767 /* identifier */ return this.identifier == null ? new Base[0] 3768 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3769 case 106437350: 3770 /* party */ return this.party == null ? new Base[0] : this.party.toArray(new Base[this.party.size()]); // ContractPartyComponent 3771 case 110546223: 3772 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Reference 3773 case 3575610: 3774 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3775 case 565719004: 3776 /* decision */ return this.decision == null ? new Base[0] : new Base[] { this.decision }; // CodeableConcept 3777 case 675909535: 3778 /* decisionMode */ return this.decisionMode == null ? new Base[0] 3779 : this.decisionMode.toArray(new Base[this.decisionMode.size()]); // CodeableConcept 3780 case -1412808770: 3781 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 3782 case 3556653: 3783 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 3784 case -1102667083: 3785 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 3786 case -149460995: 3787 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 3788 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 3789 default: 3790 return super.getProperty(hash, name, checkValid); 3791 } 3792 3793 } 3794 3795 @Override 3796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3797 switch (hash) { 3798 case -1618432855: // identifier 3799 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3800 return value; 3801 case 106437350: // party 3802 this.getParty().add((ContractPartyComponent) value); // ContractPartyComponent 3803 return value; 3804 case 110546223: // topic 3805 this.topic = castToReference(value); // Reference 3806 return value; 3807 case 3575610: // type 3808 this.type = castToCodeableConcept(value); // CodeableConcept 3809 return value; 3810 case 565719004: // decision 3811 this.decision = castToCodeableConcept(value); // CodeableConcept 3812 return value; 3813 case 675909535: // decisionMode 3814 this.getDecisionMode().add(castToCodeableConcept(value)); // CodeableConcept 3815 return value; 3816 case -1412808770: // answer 3817 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 3818 return value; 3819 case 3556653: // text 3820 this.text = castToString(value); // StringType 3821 return value; 3822 case -1102667083: // linkId 3823 this.getLinkId().add(castToString(value)); // StringType 3824 return value; 3825 case -149460995: // securityLabelNumber 3826 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 3827 return value; 3828 default: 3829 return super.setProperty(hash, name, value); 3830 } 3831 3832 } 3833 3834 @Override 3835 public Base setProperty(String name, Base value) throws FHIRException { 3836 if (name.equals("identifier")) { 3837 this.getIdentifier().add(castToIdentifier(value)); 3838 } else if (name.equals("party")) { 3839 this.getParty().add((ContractPartyComponent) value); 3840 } else if (name.equals("topic")) { 3841 this.topic = castToReference(value); // Reference 3842 } else if (name.equals("type")) { 3843 this.type = castToCodeableConcept(value); // CodeableConcept 3844 } else if (name.equals("decision")) { 3845 this.decision = castToCodeableConcept(value); // CodeableConcept 3846 } else if (name.equals("decisionMode")) { 3847 this.getDecisionMode().add(castToCodeableConcept(value)); 3848 } else if (name.equals("answer")) { 3849 this.getAnswer().add((AnswerComponent) value); 3850 } else if (name.equals("text")) { 3851 this.text = castToString(value); // StringType 3852 } else if (name.equals("linkId")) { 3853 this.getLinkId().add(castToString(value)); 3854 } else if (name.equals("securityLabelNumber")) { 3855 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 3856 } else 3857 return super.setProperty(name, value); 3858 return value; 3859 } 3860 3861 @Override 3862 public void removeChild(String name, Base value) throws FHIRException { 3863 if (name.equals("identifier")) { 3864 this.getIdentifier().remove(castToIdentifier(value)); 3865 } else if (name.equals("party")) { 3866 this.getParty().remove((ContractPartyComponent) value); 3867 } else if (name.equals("topic")) { 3868 this.topic = null; 3869 } else if (name.equals("type")) { 3870 this.type = null; 3871 } else if (name.equals("decision")) { 3872 this.decision = null; 3873 } else if (name.equals("decisionMode")) { 3874 this.getDecisionMode().remove(castToCodeableConcept(value)); 3875 } else if (name.equals("answer")) { 3876 this.getAnswer().remove((AnswerComponent) value); 3877 } else if (name.equals("text")) { 3878 this.text = null; 3879 } else if (name.equals("linkId")) { 3880 this.getLinkId().remove(castToString(value)); 3881 } else if (name.equals("securityLabelNumber")) { 3882 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 3883 } else 3884 super.removeChild(name, value); 3885 3886 } 3887 3888 @Override 3889 public Base makeProperty(int hash, String name) throws FHIRException { 3890 switch (hash) { 3891 case -1618432855: 3892 return addIdentifier(); 3893 case 106437350: 3894 return addParty(); 3895 case 110546223: 3896 return getTopic(); 3897 case 3575610: 3898 return getType(); 3899 case 565719004: 3900 return getDecision(); 3901 case 675909535: 3902 return addDecisionMode(); 3903 case -1412808770: 3904 return addAnswer(); 3905 case 3556653: 3906 return getTextElement(); 3907 case -1102667083: 3908 return addLinkIdElement(); 3909 case -149460995: 3910 return addSecurityLabelNumberElement(); 3911 default: 3912 return super.makeProperty(hash, name); 3913 } 3914 3915 } 3916 3917 @Override 3918 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3919 switch (hash) { 3920 case -1618432855: 3921 /* identifier */ return new String[] { "Identifier" }; 3922 case 106437350: 3923 /* party */ return new String[] {}; 3924 case 110546223: 3925 /* topic */ return new String[] { "Reference" }; 3926 case 3575610: 3927 /* type */ return new String[] { "CodeableConcept" }; 3928 case 565719004: 3929 /* decision */ return new String[] { "CodeableConcept" }; 3930 case 675909535: 3931 /* decisionMode */ return new String[] { "CodeableConcept" }; 3932 case -1412808770: 3933 /* answer */ return new String[] {}; 3934 case 3556653: 3935 /* text */ return new String[] { "string" }; 3936 case -1102667083: 3937 /* linkId */ return new String[] { "string" }; 3938 case -149460995: 3939 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 3940 default: 3941 return super.getTypesForProperty(hash, name); 3942 } 3943 3944 } 3945 3946 @Override 3947 public Base addChild(String name) throws FHIRException { 3948 if (name.equals("identifier")) { 3949 return addIdentifier(); 3950 } else if (name.equals("party")) { 3951 return addParty(); 3952 } else if (name.equals("topic")) { 3953 this.topic = new Reference(); 3954 return this.topic; 3955 } else if (name.equals("type")) { 3956 this.type = new CodeableConcept(); 3957 return this.type; 3958 } else if (name.equals("decision")) { 3959 this.decision = new CodeableConcept(); 3960 return this.decision; 3961 } else if (name.equals("decisionMode")) { 3962 return addDecisionMode(); 3963 } else if (name.equals("answer")) { 3964 return addAnswer(); 3965 } else if (name.equals("text")) { 3966 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 3967 } else if (name.equals("linkId")) { 3968 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 3969 } else if (name.equals("securityLabelNumber")) { 3970 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 3971 } else 3972 return super.addChild(name); 3973 } 3974 3975 public ContractOfferComponent copy() { 3976 ContractOfferComponent dst = new ContractOfferComponent(); 3977 copyValues(dst); 3978 return dst; 3979 } 3980 3981 public void copyValues(ContractOfferComponent dst) { 3982 super.copyValues(dst); 3983 if (identifier != null) { 3984 dst.identifier = new ArrayList<Identifier>(); 3985 for (Identifier i : identifier) 3986 dst.identifier.add(i.copy()); 3987 } 3988 ; 3989 if (party != null) { 3990 dst.party = new ArrayList<ContractPartyComponent>(); 3991 for (ContractPartyComponent i : party) 3992 dst.party.add(i.copy()); 3993 } 3994 ; 3995 dst.topic = topic == null ? null : topic.copy(); 3996 dst.type = type == null ? null : type.copy(); 3997 dst.decision = decision == null ? null : decision.copy(); 3998 if (decisionMode != null) { 3999 dst.decisionMode = new ArrayList<CodeableConcept>(); 4000 for (CodeableConcept i : decisionMode) 4001 dst.decisionMode.add(i.copy()); 4002 } 4003 ; 4004 if (answer != null) { 4005 dst.answer = new ArrayList<AnswerComponent>(); 4006 for (AnswerComponent i : answer) 4007 dst.answer.add(i.copy()); 4008 } 4009 ; 4010 dst.text = text == null ? null : text.copy(); 4011 if (linkId != null) { 4012 dst.linkId = new ArrayList<StringType>(); 4013 for (StringType i : linkId) 4014 dst.linkId.add(i.copy()); 4015 } 4016 ; 4017 if (securityLabelNumber != null) { 4018 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 4019 for (UnsignedIntType i : securityLabelNumber) 4020 dst.securityLabelNumber.add(i.copy()); 4021 } 4022 ; 4023 } 4024 4025 @Override 4026 public boolean equalsDeep(Base other_) { 4027 if (!super.equalsDeep(other_)) 4028 return false; 4029 if (!(other_ instanceof ContractOfferComponent)) 4030 return false; 4031 ContractOfferComponent o = (ContractOfferComponent) other_; 4032 return compareDeep(identifier, o.identifier, true) && compareDeep(party, o.party, true) 4033 && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) 4034 && compareDeep(decision, o.decision, true) && compareDeep(decisionMode, o.decisionMode, true) 4035 && compareDeep(answer, o.answer, true) && compareDeep(text, o.text, true) 4036 && compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 4037 } 4038 4039 @Override 4040 public boolean equalsShallow(Base other_) { 4041 if (!super.equalsShallow(other_)) 4042 return false; 4043 if (!(other_ instanceof ContractOfferComponent)) 4044 return false; 4045 ContractOfferComponent o = (ContractOfferComponent) other_; 4046 return compareValues(text, o.text, true) && compareValues(linkId, o.linkId, true) 4047 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 4048 } 4049 4050 public boolean isEmpty() { 4051 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, party, topic, type, decision, 4052 decisionMode, answer, text, linkId, securityLabelNumber); 4053 } 4054 4055 public String fhirType() { 4056 return "Contract.term.offer"; 4057 4058 } 4059 4060 } 4061 4062 @Block() 4063 public static class ContractPartyComponent extends BackboneElement implements IBaseBackboneElement { 4064 /** 4065 * Participant in the offer. 4066 */ 4067 @Child(name = "reference", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 4068 Device.class, Group.class, 4069 Organization.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4070 @Description(shortDefinition = "Referenced entity", formalDefinition = "Participant in the offer.") 4071 protected List<Reference> reference; 4072 /** 4073 * The actual objects that are the target of the reference (Participant in the 4074 * offer.) 4075 */ 4076 protected List<Resource> referenceTarget; 4077 4078 /** 4079 * How the party participates in the offer. 4080 */ 4081 @Child(name = "role", type = { 4082 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4083 @Description(shortDefinition = "Participant engagement type", formalDefinition = "How the party participates in the offer.") 4084 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-party-role") 4085 protected CodeableConcept role; 4086 4087 private static final long serialVersionUID = 128949255L; 4088 4089 /** 4090 * Constructor 4091 */ 4092 public ContractPartyComponent() { 4093 super(); 4094 } 4095 4096 /** 4097 * Constructor 4098 */ 4099 public ContractPartyComponent(CodeableConcept role) { 4100 super(); 4101 this.role = role; 4102 } 4103 4104 /** 4105 * @return {@link #reference} (Participant in the offer.) 4106 */ 4107 public List<Reference> getReference() { 4108 if (this.reference == null) 4109 this.reference = new ArrayList<Reference>(); 4110 return this.reference; 4111 } 4112 4113 /** 4114 * @return Returns a reference to <code>this</code> for easy method chaining 4115 */ 4116 public ContractPartyComponent setReference(List<Reference> theReference) { 4117 this.reference = theReference; 4118 return this; 4119 } 4120 4121 public boolean hasReference() { 4122 if (this.reference == null) 4123 return false; 4124 for (Reference item : this.reference) 4125 if (!item.isEmpty()) 4126 return true; 4127 return false; 4128 } 4129 4130 public Reference addReference() { // 3 4131 Reference t = new Reference(); 4132 if (this.reference == null) 4133 this.reference = new ArrayList<Reference>(); 4134 this.reference.add(t); 4135 return t; 4136 } 4137 4138 public ContractPartyComponent addReference(Reference t) { // 3 4139 if (t == null) 4140 return this; 4141 if (this.reference == null) 4142 this.reference = new ArrayList<Reference>(); 4143 this.reference.add(t); 4144 return this; 4145 } 4146 4147 /** 4148 * @return The first repetition of repeating field {@link #reference}, creating 4149 * it if it does not already exist 4150 */ 4151 public Reference getReferenceFirstRep() { 4152 if (getReference().isEmpty()) { 4153 addReference(); 4154 } 4155 return getReference().get(0); 4156 } 4157 4158 /** 4159 * @return {@link #role} (How the party participates in the offer.) 4160 */ 4161 public CodeableConcept getRole() { 4162 if (this.role == null) 4163 if (Configuration.errorOnAutoCreate()) 4164 throw new Error("Attempt to auto-create ContractPartyComponent.role"); 4165 else if (Configuration.doAutoCreate()) 4166 this.role = new CodeableConcept(); // cc 4167 return this.role; 4168 } 4169 4170 public boolean hasRole() { 4171 return this.role != null && !this.role.isEmpty(); 4172 } 4173 4174 /** 4175 * @param value {@link #role} (How the party participates in the offer.) 4176 */ 4177 public ContractPartyComponent setRole(CodeableConcept value) { 4178 this.role = value; 4179 return this; 4180 } 4181 4182 protected void listChildren(List<Property> children) { 4183 super.listChildren(children); 4184 children.add(new Property("reference", 4185 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 4186 "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference)); 4187 children.add(new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, role)); 4188 } 4189 4190 @Override 4191 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4192 switch (_hash) { 4193 case -925155509: 4194 /* reference */ return new Property("reference", 4195 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 4196 "Participant in the offer.", 0, java.lang.Integer.MAX_VALUE, reference); 4197 case 3506294: 4198 /* role */ return new Property("role", "CodeableConcept", "How the party participates in the offer.", 0, 1, 4199 role); 4200 default: 4201 return super.getNamedProperty(_hash, _name, _checkValid); 4202 } 4203 4204 } 4205 4206 @Override 4207 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4208 switch (hash) { 4209 case -925155509: 4210 /* reference */ return this.reference == null ? new Base[0] 4211 : this.reference.toArray(new Base[this.reference.size()]); // Reference 4212 case 3506294: 4213 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 4214 default: 4215 return super.getProperty(hash, name, checkValid); 4216 } 4217 4218 } 4219 4220 @Override 4221 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4222 switch (hash) { 4223 case -925155509: // reference 4224 this.getReference().add(castToReference(value)); // Reference 4225 return value; 4226 case 3506294: // role 4227 this.role = castToCodeableConcept(value); // CodeableConcept 4228 return value; 4229 default: 4230 return super.setProperty(hash, name, value); 4231 } 4232 4233 } 4234 4235 @Override 4236 public Base setProperty(String name, Base value) throws FHIRException { 4237 if (name.equals("reference")) { 4238 this.getReference().add(castToReference(value)); 4239 } else if (name.equals("role")) { 4240 this.role = castToCodeableConcept(value); // CodeableConcept 4241 } else 4242 return super.setProperty(name, value); 4243 return value; 4244 } 4245 4246 @Override 4247 public void removeChild(String name, Base value) throws FHIRException { 4248 if (name.equals("reference")) { 4249 this.getReference().remove(castToReference(value)); 4250 } else if (name.equals("role")) { 4251 this.role = null; 4252 } else 4253 super.removeChild(name, value); 4254 4255 } 4256 4257 @Override 4258 public Base makeProperty(int hash, String name) throws FHIRException { 4259 switch (hash) { 4260 case -925155509: 4261 return addReference(); 4262 case 3506294: 4263 return getRole(); 4264 default: 4265 return super.makeProperty(hash, name); 4266 } 4267 4268 } 4269 4270 @Override 4271 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4272 switch (hash) { 4273 case -925155509: 4274 /* reference */ return new String[] { "Reference" }; 4275 case 3506294: 4276 /* role */ return new String[] { "CodeableConcept" }; 4277 default: 4278 return super.getTypesForProperty(hash, name); 4279 } 4280 4281 } 4282 4283 @Override 4284 public Base addChild(String name) throws FHIRException { 4285 if (name.equals("reference")) { 4286 return addReference(); 4287 } else if (name.equals("role")) { 4288 this.role = new CodeableConcept(); 4289 return this.role; 4290 } else 4291 return super.addChild(name); 4292 } 4293 4294 public ContractPartyComponent copy() { 4295 ContractPartyComponent dst = new ContractPartyComponent(); 4296 copyValues(dst); 4297 return dst; 4298 } 4299 4300 public void copyValues(ContractPartyComponent dst) { 4301 super.copyValues(dst); 4302 if (reference != null) { 4303 dst.reference = new ArrayList<Reference>(); 4304 for (Reference i : reference) 4305 dst.reference.add(i.copy()); 4306 } 4307 ; 4308 dst.role = role == null ? null : role.copy(); 4309 } 4310 4311 @Override 4312 public boolean equalsDeep(Base other_) { 4313 if (!super.equalsDeep(other_)) 4314 return false; 4315 if (!(other_ instanceof ContractPartyComponent)) 4316 return false; 4317 ContractPartyComponent o = (ContractPartyComponent) other_; 4318 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 4319 } 4320 4321 @Override 4322 public boolean equalsShallow(Base other_) { 4323 if (!super.equalsShallow(other_)) 4324 return false; 4325 if (!(other_ instanceof ContractPartyComponent)) 4326 return false; 4327 ContractPartyComponent o = (ContractPartyComponent) other_; 4328 return true; 4329 } 4330 4331 public boolean isEmpty() { 4332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 4333 } 4334 4335 public String fhirType() { 4336 return "Contract.term.offer.party"; 4337 4338 } 4339 4340 } 4341 4342 @Block() 4343 public static class AnswerComponent extends BackboneElement implements IBaseBackboneElement { 4344 /** 4345 * Response to an offer clause or question text, which enables selection of 4346 * values to be agreed to, e.g., the period of participation, the date of 4347 * occupancy of a rental, warrently duration, or whether biospecimen may be used 4348 * for further research. 4349 */ 4350 @Child(name = "value", type = { BooleanType.class, DecimalType.class, IntegerType.class, DateType.class, 4351 DateTimeType.class, TimeType.class, StringType.class, UriType.class, Attachment.class, Coding.class, 4352 Quantity.class, Reference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4353 @Description(shortDefinition = "The actual answer response", formalDefinition = "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.") 4354 protected Type value; 4355 4356 private static final long serialVersionUID = -732981989L; 4357 4358 /** 4359 * Constructor 4360 */ 4361 public AnswerComponent() { 4362 super(); 4363 } 4364 4365 /** 4366 * Constructor 4367 */ 4368 public AnswerComponent(Type value) { 4369 super(); 4370 this.value = value; 4371 } 4372 4373 /** 4374 * @return {@link #value} (Response to an offer clause or question text, which 4375 * enables selection of values to be agreed to, e.g., the period of 4376 * participation, the date of occupancy of a rental, warrently duration, 4377 * or whether biospecimen may be used for further research.) 4378 */ 4379 public Type getValue() { 4380 return this.value; 4381 } 4382 4383 /** 4384 * @return {@link #value} (Response to an offer clause or question text, which 4385 * enables selection of values to be agreed to, e.g., the period of 4386 * participation, the date of occupancy of a rental, warrently duration, 4387 * or whether biospecimen may be used for further research.) 4388 */ 4389 public BooleanType getValueBooleanType() throws FHIRException { 4390 if (this.value == null) 4391 this.value = new BooleanType(); 4392 if (!(this.value instanceof BooleanType)) 4393 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 4394 + this.value.getClass().getName() + " was encountered"); 4395 return (BooleanType) this.value; 4396 } 4397 4398 public boolean hasValueBooleanType() { 4399 return this.value instanceof BooleanType; 4400 } 4401 4402 /** 4403 * @return {@link #value} (Response to an offer clause or question text, which 4404 * enables selection of values to be agreed to, e.g., the period of 4405 * participation, the date of occupancy of a rental, warrently duration, 4406 * or whether biospecimen may be used for further research.) 4407 */ 4408 public DecimalType getValueDecimalType() throws FHIRException { 4409 if (this.value == null) 4410 this.value = new DecimalType(); 4411 if (!(this.value instanceof DecimalType)) 4412 throw new FHIRException("Type mismatch: the type DecimalType was expected, but " 4413 + this.value.getClass().getName() + " was encountered"); 4414 return (DecimalType) this.value; 4415 } 4416 4417 public boolean hasValueDecimalType() { 4418 return this.value instanceof DecimalType; 4419 } 4420 4421 /** 4422 * @return {@link #value} (Response to an offer clause or question text, which 4423 * enables selection of values to be agreed to, e.g., the period of 4424 * participation, the date of occupancy of a rental, warrently duration, 4425 * or whether biospecimen may be used for further research.) 4426 */ 4427 public IntegerType getValueIntegerType() throws FHIRException { 4428 if (this.value == null) 4429 this.value = new IntegerType(); 4430 if (!(this.value instanceof IntegerType)) 4431 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 4432 + this.value.getClass().getName() + " was encountered"); 4433 return (IntegerType) this.value; 4434 } 4435 4436 public boolean hasValueIntegerType() { 4437 return this.value instanceof IntegerType; 4438 } 4439 4440 /** 4441 * @return {@link #value} (Response to an offer clause or question text, which 4442 * enables selection of values to be agreed to, e.g., the period of 4443 * participation, the date of occupancy of a rental, warrently duration, 4444 * or whether biospecimen may be used for further research.) 4445 */ 4446 public DateType getValueDateType() throws FHIRException { 4447 if (this.value == null) 4448 this.value = new DateType(); 4449 if (!(this.value instanceof DateType)) 4450 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.value.getClass().getName() 4451 + " was encountered"); 4452 return (DateType) this.value; 4453 } 4454 4455 public boolean hasValueDateType() { 4456 return this.value instanceof DateType; 4457 } 4458 4459 /** 4460 * @return {@link #value} (Response to an offer clause or question text, which 4461 * enables selection of values to be agreed to, e.g., the period of 4462 * participation, the date of occupancy of a rental, warrently duration, 4463 * or whether biospecimen may be used for further research.) 4464 */ 4465 public DateTimeType getValueDateTimeType() throws FHIRException { 4466 if (this.value == null) 4467 this.value = new DateTimeType(); 4468 if (!(this.value instanceof DateTimeType)) 4469 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 4470 + this.value.getClass().getName() + " was encountered"); 4471 return (DateTimeType) this.value; 4472 } 4473 4474 public boolean hasValueDateTimeType() { 4475 return this.value instanceof DateTimeType; 4476 } 4477 4478 /** 4479 * @return {@link #value} (Response to an offer clause or question text, which 4480 * enables selection of values to be agreed to, e.g., the period of 4481 * participation, the date of occupancy of a rental, warrently duration, 4482 * or whether biospecimen may be used for further research.) 4483 */ 4484 public TimeType getValueTimeType() throws FHIRException { 4485 if (this.value == null) 4486 this.value = new TimeType(); 4487 if (!(this.value instanceof TimeType)) 4488 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 4489 + " was encountered"); 4490 return (TimeType) this.value; 4491 } 4492 4493 public boolean hasValueTimeType() { 4494 return this.value instanceof TimeType; 4495 } 4496 4497 /** 4498 * @return {@link #value} (Response to an offer clause or question text, which 4499 * enables selection of values to be agreed to, e.g., the period of 4500 * participation, the date of occupancy of a rental, warrently duration, 4501 * or whether biospecimen may be used for further research.) 4502 */ 4503 public StringType getValueStringType() throws FHIRException { 4504 if (this.value == null) 4505 this.value = new StringType(); 4506 if (!(this.value instanceof StringType)) 4507 throw new FHIRException("Type mismatch: the type StringType was expected, but " 4508 + this.value.getClass().getName() + " was encountered"); 4509 return (StringType) this.value; 4510 } 4511 4512 public boolean hasValueStringType() { 4513 return this.value instanceof StringType; 4514 } 4515 4516 /** 4517 * @return {@link #value} (Response to an offer clause or question text, which 4518 * enables selection of values to be agreed to, e.g., the period of 4519 * participation, the date of occupancy of a rental, warrently duration, 4520 * or whether biospecimen may be used for further research.) 4521 */ 4522 public UriType getValueUriType() throws FHIRException { 4523 if (this.value == null) 4524 this.value = new UriType(); 4525 if (!(this.value instanceof UriType)) 4526 throw new FHIRException("Type mismatch: the type UriType was expected, but " + this.value.getClass().getName() 4527 + " was encountered"); 4528 return (UriType) this.value; 4529 } 4530 4531 public boolean hasValueUriType() { 4532 return this.value instanceof UriType; 4533 } 4534 4535 /** 4536 * @return {@link #value} (Response to an offer clause or question text, which 4537 * enables selection of values to be agreed to, e.g., the period of 4538 * participation, the date of occupancy of a rental, warrently duration, 4539 * or whether biospecimen may be used for further research.) 4540 */ 4541 public Attachment getValueAttachment() throws FHIRException { 4542 if (this.value == null) 4543 this.value = new Attachment(); 4544 if (!(this.value instanceof Attachment)) 4545 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 4546 + this.value.getClass().getName() + " was encountered"); 4547 return (Attachment) this.value; 4548 } 4549 4550 public boolean hasValueAttachment() { 4551 return this.value instanceof Attachment; 4552 } 4553 4554 /** 4555 * @return {@link #value} (Response to an offer clause or question text, which 4556 * enables selection of values to be agreed to, e.g., the period of 4557 * participation, the date of occupancy of a rental, warrently duration, 4558 * or whether biospecimen may be used for further research.) 4559 */ 4560 public Coding getValueCoding() throws FHIRException { 4561 if (this.value == null) 4562 this.value = new Coding(); 4563 if (!(this.value instanceof Coding)) 4564 throw new FHIRException( 4565 "Type mismatch: the type Coding was expected, but " + this.value.getClass().getName() + " was encountered"); 4566 return (Coding) this.value; 4567 } 4568 4569 public boolean hasValueCoding() { 4570 return this.value instanceof Coding; 4571 } 4572 4573 /** 4574 * @return {@link #value} (Response to an offer clause or question text, which 4575 * enables selection of values to be agreed to, e.g., the period of 4576 * participation, the date of occupancy of a rental, warrently duration, 4577 * or whether biospecimen may be used for further research.) 4578 */ 4579 public Quantity getValueQuantity() throws FHIRException { 4580 if (this.value == null) 4581 this.value = new Quantity(); 4582 if (!(this.value instanceof Quantity)) 4583 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 4584 + " was encountered"); 4585 return (Quantity) this.value; 4586 } 4587 4588 public boolean hasValueQuantity() { 4589 return this.value instanceof Quantity; 4590 } 4591 4592 /** 4593 * @return {@link #value} (Response to an offer clause or question text, which 4594 * enables selection of values to be agreed to, e.g., the period of 4595 * participation, the date of occupancy of a rental, warrently duration, 4596 * or whether biospecimen may be used for further research.) 4597 */ 4598 public Reference getValueReference() throws FHIRException { 4599 if (this.value == null) 4600 this.value = new Reference(); 4601 if (!(this.value instanceof Reference)) 4602 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 4603 + " was encountered"); 4604 return (Reference) this.value; 4605 } 4606 4607 public boolean hasValueReference() { 4608 return this.value instanceof Reference; 4609 } 4610 4611 public boolean hasValue() { 4612 return this.value != null && !this.value.isEmpty(); 4613 } 4614 4615 /** 4616 * @param value {@link #value} (Response to an offer clause or question text, 4617 * which enables selection of values to be agreed to, e.g., the 4618 * period of participation, the date of occupancy of a rental, 4619 * warrently duration, or whether biospecimen may be used for 4620 * further research.) 4621 */ 4622 public AnswerComponent setValue(Type value) { 4623 if (value != null 4624 && !(value instanceof BooleanType || value instanceof DecimalType || value instanceof IntegerType 4625 || value instanceof DateType || value instanceof DateTimeType || value instanceof TimeType 4626 || value instanceof StringType || value instanceof UriType || value instanceof Attachment 4627 || value instanceof Coding || value instanceof Quantity || value instanceof Reference)) 4628 throw new Error("Not the right type for Contract.term.offer.answer.value[x]: " + value.fhirType()); 4629 this.value = value; 4630 return this; 4631 } 4632 4633 protected void listChildren(List<Property> children) { 4634 super.listChildren(children); 4635 children.add(new Property("value[x]", 4636 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4637 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4638 0, 1, value)); 4639 } 4640 4641 @Override 4642 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4643 switch (_hash) { 4644 case -1410166417: 4645 /* value[x] */ return new Property("value[x]", 4646 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4647 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4648 0, 1, value); 4649 case 111972721: 4650 /* value */ return new Property("value[x]", 4651 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4652 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4653 0, 1, value); 4654 case 733421943: 4655 /* valueBoolean */ return new Property("value[x]", 4656 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4657 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4658 0, 1, value); 4659 case -2083993440: 4660 /* valueDecimal */ return new Property("value[x]", 4661 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4662 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4663 0, 1, value); 4664 case -1668204915: 4665 /* valueInteger */ return new Property("value[x]", 4666 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4667 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4668 0, 1, value); 4669 case -766192449: 4670 /* valueDate */ return new Property("value[x]", 4671 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4672 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4673 0, 1, value); 4674 case 1047929900: 4675 /* valueDateTime */ return new Property("value[x]", 4676 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4677 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4678 0, 1, value); 4679 case -765708322: 4680 /* valueTime */ return new Property("value[x]", 4681 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4682 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4683 0, 1, value); 4684 case -1424603934: 4685 /* valueString */ return new Property("value[x]", 4686 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4687 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4688 0, 1, value); 4689 case -1410172357: 4690 /* valueUri */ return new Property("value[x]", 4691 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4692 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4693 0, 1, value); 4694 case -475566732: 4695 /* valueAttachment */ return new Property("value[x]", 4696 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4697 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4698 0, 1, value); 4699 case -1887705029: 4700 /* valueCoding */ return new Property("value[x]", 4701 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4702 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4703 0, 1, value); 4704 case -2029823716: 4705 /* valueQuantity */ return new Property("value[x]", 4706 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4707 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4708 0, 1, value); 4709 case 1755241690: 4710 /* valueReference */ return new Property("value[x]", 4711 "boolean|decimal|integer|date|dateTime|time|string|uri|Attachment|Coding|Quantity|Reference(Any)", 4712 "Response to an offer clause or question text, which enables selection of values to be agreed to, e.g., the period of participation, the date of occupancy of a rental, warrently duration, or whether biospecimen may be used for further research.", 4713 0, 1, value); 4714 default: 4715 return super.getNamedProperty(_hash, _name, _checkValid); 4716 } 4717 4718 } 4719 4720 @Override 4721 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4722 switch (hash) { 4723 case 111972721: 4724 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 4725 default: 4726 return super.getProperty(hash, name, checkValid); 4727 } 4728 4729 } 4730 4731 @Override 4732 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4733 switch (hash) { 4734 case 111972721: // value 4735 this.value = castToType(value); // Type 4736 return value; 4737 default: 4738 return super.setProperty(hash, name, value); 4739 } 4740 4741 } 4742 4743 @Override 4744 public Base setProperty(String name, Base value) throws FHIRException { 4745 if (name.equals("value[x]")) { 4746 this.value = castToType(value); // Type 4747 } else 4748 return super.setProperty(name, value); 4749 return value; 4750 } 4751 4752 @Override 4753 public void removeChild(String name, Base value) throws FHIRException { 4754 if (name.equals("value[x]")) { 4755 this.value = null; 4756 } else 4757 super.removeChild(name, value); 4758 4759 } 4760 4761 @Override 4762 public Base makeProperty(int hash, String name) throws FHIRException { 4763 switch (hash) { 4764 case -1410166417: 4765 return getValue(); 4766 case 111972721: 4767 return getValue(); 4768 default: 4769 return super.makeProperty(hash, name); 4770 } 4771 4772 } 4773 4774 @Override 4775 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4776 switch (hash) { 4777 case 111972721: 4778 /* value */ return new String[] { "boolean", "decimal", "integer", "date", "dateTime", "time", "string", "uri", 4779 "Attachment", "Coding", "Quantity", "Reference" }; 4780 default: 4781 return super.getTypesForProperty(hash, name); 4782 } 4783 4784 } 4785 4786 @Override 4787 public Base addChild(String name) throws FHIRException { 4788 if (name.equals("valueBoolean")) { 4789 this.value = new BooleanType(); 4790 return this.value; 4791 } else if (name.equals("valueDecimal")) { 4792 this.value = new DecimalType(); 4793 return this.value; 4794 } else if (name.equals("valueInteger")) { 4795 this.value = new IntegerType(); 4796 return this.value; 4797 } else if (name.equals("valueDate")) { 4798 this.value = new DateType(); 4799 return this.value; 4800 } else if (name.equals("valueDateTime")) { 4801 this.value = new DateTimeType(); 4802 return this.value; 4803 } else if (name.equals("valueTime")) { 4804 this.value = new TimeType(); 4805 return this.value; 4806 } else if (name.equals("valueString")) { 4807 this.value = new StringType(); 4808 return this.value; 4809 } else if (name.equals("valueUri")) { 4810 this.value = new UriType(); 4811 return this.value; 4812 } else if (name.equals("valueAttachment")) { 4813 this.value = new Attachment(); 4814 return this.value; 4815 } else if (name.equals("valueCoding")) { 4816 this.value = new Coding(); 4817 return this.value; 4818 } else if (name.equals("valueQuantity")) { 4819 this.value = new Quantity(); 4820 return this.value; 4821 } else if (name.equals("valueReference")) { 4822 this.value = new Reference(); 4823 return this.value; 4824 } else 4825 return super.addChild(name); 4826 } 4827 4828 public AnswerComponent copy() { 4829 AnswerComponent dst = new AnswerComponent(); 4830 copyValues(dst); 4831 return dst; 4832 } 4833 4834 public void copyValues(AnswerComponent dst) { 4835 super.copyValues(dst); 4836 dst.value = value == null ? null : value.copy(); 4837 } 4838 4839 @Override 4840 public boolean equalsDeep(Base other_) { 4841 if (!super.equalsDeep(other_)) 4842 return false; 4843 if (!(other_ instanceof AnswerComponent)) 4844 return false; 4845 AnswerComponent o = (AnswerComponent) other_; 4846 return compareDeep(value, o.value, true); 4847 } 4848 4849 @Override 4850 public boolean equalsShallow(Base other_) { 4851 if (!super.equalsShallow(other_)) 4852 return false; 4853 if (!(other_ instanceof AnswerComponent)) 4854 return false; 4855 AnswerComponent o = (AnswerComponent) other_; 4856 return true; 4857 } 4858 4859 public boolean isEmpty() { 4860 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value); 4861 } 4862 4863 public String fhirType() { 4864 return "Contract.term.offer.answer"; 4865 4866 } 4867 4868 } 4869 4870 @Block() 4871 public static class ContractAssetComponent extends BackboneElement implements IBaseBackboneElement { 4872 /** 4873 * Differentiates the kind of the asset . 4874 */ 4875 @Child(name = "scope", type = { 4876 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 4877 @Description(shortDefinition = "Range of asset", formalDefinition = "Differentiates the kind of the asset .") 4878 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetscope") 4879 protected CodeableConcept scope; 4880 4881 /** 4882 * Target entity type about which the term may be concerned. 4883 */ 4884 @Child(name = "type", type = { 4885 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4886 @Description(shortDefinition = "Asset category", formalDefinition = "Target entity type about which the term may be concerned.") 4887 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assettype") 4888 protected List<CodeableConcept> type; 4889 4890 /** 4891 * Associated entities. 4892 */ 4893 @Child(name = "typeReference", type = { 4894 Reference.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4895 @Description(shortDefinition = "Associated entities", formalDefinition = "Associated entities.") 4896 protected List<Reference> typeReference; 4897 /** 4898 * The actual objects that are the target of the reference (Associated 4899 * entities.) 4900 */ 4901 protected List<Resource> typeReferenceTarget; 4902 4903 /** 4904 * May be a subtype or part of an offered asset. 4905 */ 4906 @Child(name = "subtype", type = { 4907 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4908 @Description(shortDefinition = "Asset sub-category", formalDefinition = "May be a subtype or part of an offered asset.") 4909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetsubtype") 4910 protected List<CodeableConcept> subtype; 4911 4912 /** 4913 * Specifies the applicability of the term to an asset resource instance, and 4914 * instances it refers to orinstances that refer to it, and/or are owned by the 4915 * offeree. 4916 */ 4917 @Child(name = "relationship", type = { 4918 Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 4919 @Description(shortDefinition = "Kinship of the asset", formalDefinition = "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.") 4920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/consent-content-class") 4921 protected Coding relationship; 4922 4923 /** 4924 * Circumstance of the asset. 4925 */ 4926 @Child(name = "context", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4927 @Description(shortDefinition = "Circumstance of the asset", formalDefinition = "Circumstance of the asset.") 4928 protected List<AssetContextComponent> context; 4929 4930 /** 4931 * Description of the quality and completeness of the asset that imay be a 4932 * factor in its valuation. 4933 */ 4934 @Child(name = "condition", type = { 4935 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 4936 @Description(shortDefinition = "Quality desctiption of asset", formalDefinition = "Description of the quality and completeness of the asset that imay be a factor in its valuation.") 4937 protected StringType condition; 4938 4939 /** 4940 * Type of Asset availability for use or ownership. 4941 */ 4942 @Child(name = "periodType", type = { 4943 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4944 @Description(shortDefinition = "Asset availability types", formalDefinition = "Type of Asset availability for use or ownership.") 4945 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/asset-availability") 4946 protected List<CodeableConcept> periodType; 4947 4948 /** 4949 * Asset relevant contractual time period. 4950 */ 4951 @Child(name = "period", type = { 4952 Period.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4953 @Description(shortDefinition = "Time period of the asset", formalDefinition = "Asset relevant contractual time period.") 4954 protected List<Period> period; 4955 4956 /** 4957 * Time period of asset use. 4958 */ 4959 @Child(name = "usePeriod", type = { 4960 Period.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4961 @Description(shortDefinition = "Time period", formalDefinition = "Time period of asset use.") 4962 protected List<Period> usePeriod; 4963 4964 /** 4965 * Clause or question text (Prose Object) concerning the asset in a linked form, 4966 * such as a QuestionnaireResponse used in the formation of the contract. 4967 */ 4968 @Child(name = "text", type = { StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 4969 @Description(shortDefinition = "Asset clause or question text", formalDefinition = "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.") 4970 protected StringType text; 4971 4972 /** 4973 * Id [identifier??] of the clause or question text about the asset in the 4974 * referenced form or QuestionnaireResponse. 4975 */ 4976 @Child(name = "linkId", type = { 4977 StringType.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4978 @Description(shortDefinition = "Pointer to asset text", formalDefinition = "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.") 4979 protected List<StringType> linkId; 4980 4981 /** 4982 * Response to assets. 4983 */ 4984 @Child(name = "answer", type = { 4985 AnswerComponent.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4986 @Description(shortDefinition = "Response to assets", formalDefinition = "Response to assets.") 4987 protected List<AnswerComponent> answer; 4988 4989 /** 4990 * Security labels that protects the asset. 4991 */ 4992 @Child(name = "securityLabelNumber", type = { 4993 UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 4994 @Description(shortDefinition = "Asset restriction numbers", formalDefinition = "Security labels that protects the asset.") 4995 protected List<UnsignedIntType> securityLabelNumber; 4996 4997 /** 4998 * Contract Valued Item List. 4999 */ 5000 @Child(name = "valuedItem", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5001 @Description(shortDefinition = "Contract Valued Item List", formalDefinition = "Contract Valued Item List.") 5002 protected List<ValuedItemComponent> valuedItem; 5003 5004 private static final long serialVersionUID = -1080398792L; 5005 5006 /** 5007 * Constructor 5008 */ 5009 public ContractAssetComponent() { 5010 super(); 5011 } 5012 5013 /** 5014 * @return {@link #scope} (Differentiates the kind of the asset .) 5015 */ 5016 public CodeableConcept getScope() { 5017 if (this.scope == null) 5018 if (Configuration.errorOnAutoCreate()) 5019 throw new Error("Attempt to auto-create ContractAssetComponent.scope"); 5020 else if (Configuration.doAutoCreate()) 5021 this.scope = new CodeableConcept(); // cc 5022 return this.scope; 5023 } 5024 5025 public boolean hasScope() { 5026 return this.scope != null && !this.scope.isEmpty(); 5027 } 5028 5029 /** 5030 * @param value {@link #scope} (Differentiates the kind of the asset .) 5031 */ 5032 public ContractAssetComponent setScope(CodeableConcept value) { 5033 this.scope = value; 5034 return this; 5035 } 5036 5037 /** 5038 * @return {@link #type} (Target entity type about which the term may be 5039 * concerned.) 5040 */ 5041 public List<CodeableConcept> getType() { 5042 if (this.type == null) 5043 this.type = new ArrayList<CodeableConcept>(); 5044 return this.type; 5045 } 5046 5047 /** 5048 * @return Returns a reference to <code>this</code> for easy method chaining 5049 */ 5050 public ContractAssetComponent setType(List<CodeableConcept> theType) { 5051 this.type = theType; 5052 return this; 5053 } 5054 5055 public boolean hasType() { 5056 if (this.type == null) 5057 return false; 5058 for (CodeableConcept item : this.type) 5059 if (!item.isEmpty()) 5060 return true; 5061 return false; 5062 } 5063 5064 public CodeableConcept addType() { // 3 5065 CodeableConcept t = new CodeableConcept(); 5066 if (this.type == null) 5067 this.type = new ArrayList<CodeableConcept>(); 5068 this.type.add(t); 5069 return t; 5070 } 5071 5072 public ContractAssetComponent addType(CodeableConcept t) { // 3 5073 if (t == null) 5074 return this; 5075 if (this.type == null) 5076 this.type = new ArrayList<CodeableConcept>(); 5077 this.type.add(t); 5078 return this; 5079 } 5080 5081 /** 5082 * @return The first repetition of repeating field {@link #type}, creating it if 5083 * it does not already exist 5084 */ 5085 public CodeableConcept getTypeFirstRep() { 5086 if (getType().isEmpty()) { 5087 addType(); 5088 } 5089 return getType().get(0); 5090 } 5091 5092 /** 5093 * @return {@link #typeReference} (Associated entities.) 5094 */ 5095 public List<Reference> getTypeReference() { 5096 if (this.typeReference == null) 5097 this.typeReference = new ArrayList<Reference>(); 5098 return this.typeReference; 5099 } 5100 5101 /** 5102 * @return Returns a reference to <code>this</code> for easy method chaining 5103 */ 5104 public ContractAssetComponent setTypeReference(List<Reference> theTypeReference) { 5105 this.typeReference = theTypeReference; 5106 return this; 5107 } 5108 5109 public boolean hasTypeReference() { 5110 if (this.typeReference == null) 5111 return false; 5112 for (Reference item : this.typeReference) 5113 if (!item.isEmpty()) 5114 return true; 5115 return false; 5116 } 5117 5118 public Reference addTypeReference() { // 3 5119 Reference t = new Reference(); 5120 if (this.typeReference == null) 5121 this.typeReference = new ArrayList<Reference>(); 5122 this.typeReference.add(t); 5123 return t; 5124 } 5125 5126 public ContractAssetComponent addTypeReference(Reference t) { // 3 5127 if (t == null) 5128 return this; 5129 if (this.typeReference == null) 5130 this.typeReference = new ArrayList<Reference>(); 5131 this.typeReference.add(t); 5132 return this; 5133 } 5134 5135 /** 5136 * @return The first repetition of repeating field {@link #typeReference}, 5137 * creating it if it does not already exist 5138 */ 5139 public Reference getTypeReferenceFirstRep() { 5140 if (getTypeReference().isEmpty()) { 5141 addTypeReference(); 5142 } 5143 return getTypeReference().get(0); 5144 } 5145 5146 /** 5147 * @return {@link #subtype} (May be a subtype or part of an offered asset.) 5148 */ 5149 public List<CodeableConcept> getSubtype() { 5150 if (this.subtype == null) 5151 this.subtype = new ArrayList<CodeableConcept>(); 5152 return this.subtype; 5153 } 5154 5155 /** 5156 * @return Returns a reference to <code>this</code> for easy method chaining 5157 */ 5158 public ContractAssetComponent setSubtype(List<CodeableConcept> theSubtype) { 5159 this.subtype = theSubtype; 5160 return this; 5161 } 5162 5163 public boolean hasSubtype() { 5164 if (this.subtype == null) 5165 return false; 5166 for (CodeableConcept item : this.subtype) 5167 if (!item.isEmpty()) 5168 return true; 5169 return false; 5170 } 5171 5172 public CodeableConcept addSubtype() { // 3 5173 CodeableConcept t = new CodeableConcept(); 5174 if (this.subtype == null) 5175 this.subtype = new ArrayList<CodeableConcept>(); 5176 this.subtype.add(t); 5177 return t; 5178 } 5179 5180 public ContractAssetComponent addSubtype(CodeableConcept t) { // 3 5181 if (t == null) 5182 return this; 5183 if (this.subtype == null) 5184 this.subtype = new ArrayList<CodeableConcept>(); 5185 this.subtype.add(t); 5186 return this; 5187 } 5188 5189 /** 5190 * @return The first repetition of repeating field {@link #subtype}, creating it 5191 * if it does not already exist 5192 */ 5193 public CodeableConcept getSubtypeFirstRep() { 5194 if (getSubtype().isEmpty()) { 5195 addSubtype(); 5196 } 5197 return getSubtype().get(0); 5198 } 5199 5200 /** 5201 * @return {@link #relationship} (Specifies the applicability of the term to an 5202 * asset resource instance, and instances it refers to orinstances that 5203 * refer to it, and/or are owned by the offeree.) 5204 */ 5205 public Coding getRelationship() { 5206 if (this.relationship == null) 5207 if (Configuration.errorOnAutoCreate()) 5208 throw new Error("Attempt to auto-create ContractAssetComponent.relationship"); 5209 else if (Configuration.doAutoCreate()) 5210 this.relationship = new Coding(); // cc 5211 return this.relationship; 5212 } 5213 5214 public boolean hasRelationship() { 5215 return this.relationship != null && !this.relationship.isEmpty(); 5216 } 5217 5218 /** 5219 * @param value {@link #relationship} (Specifies the applicability of the term 5220 * to an asset resource instance, and instances it refers to 5221 * orinstances that refer to it, and/or are owned by the offeree.) 5222 */ 5223 public ContractAssetComponent setRelationship(Coding value) { 5224 this.relationship = value; 5225 return this; 5226 } 5227 5228 /** 5229 * @return {@link #context} (Circumstance of the asset.) 5230 */ 5231 public List<AssetContextComponent> getContext() { 5232 if (this.context == null) 5233 this.context = new ArrayList<AssetContextComponent>(); 5234 return this.context; 5235 } 5236 5237 /** 5238 * @return Returns a reference to <code>this</code> for easy method chaining 5239 */ 5240 public ContractAssetComponent setContext(List<AssetContextComponent> theContext) { 5241 this.context = theContext; 5242 return this; 5243 } 5244 5245 public boolean hasContext() { 5246 if (this.context == null) 5247 return false; 5248 for (AssetContextComponent item : this.context) 5249 if (!item.isEmpty()) 5250 return true; 5251 return false; 5252 } 5253 5254 public AssetContextComponent addContext() { // 3 5255 AssetContextComponent t = new AssetContextComponent(); 5256 if (this.context == null) 5257 this.context = new ArrayList<AssetContextComponent>(); 5258 this.context.add(t); 5259 return t; 5260 } 5261 5262 public ContractAssetComponent addContext(AssetContextComponent t) { // 3 5263 if (t == null) 5264 return this; 5265 if (this.context == null) 5266 this.context = new ArrayList<AssetContextComponent>(); 5267 this.context.add(t); 5268 return this; 5269 } 5270 5271 /** 5272 * @return The first repetition of repeating field {@link #context}, creating it 5273 * if it does not already exist 5274 */ 5275 public AssetContextComponent getContextFirstRep() { 5276 if (getContext().isEmpty()) { 5277 addContext(); 5278 } 5279 return getContext().get(0); 5280 } 5281 5282 /** 5283 * @return {@link #condition} (Description of the quality and completeness of 5284 * the asset that imay be a factor in its valuation.). This is the 5285 * underlying object with id, value and extensions. The accessor 5286 * "getCondition" gives direct access to the value 5287 */ 5288 public StringType getConditionElement() { 5289 if (this.condition == null) 5290 if (Configuration.errorOnAutoCreate()) 5291 throw new Error("Attempt to auto-create ContractAssetComponent.condition"); 5292 else if (Configuration.doAutoCreate()) 5293 this.condition = new StringType(); // bb 5294 return this.condition; 5295 } 5296 5297 public boolean hasConditionElement() { 5298 return this.condition != null && !this.condition.isEmpty(); 5299 } 5300 5301 public boolean hasCondition() { 5302 return this.condition != null && !this.condition.isEmpty(); 5303 } 5304 5305 /** 5306 * @param value {@link #condition} (Description of the quality and completeness 5307 * of the asset that imay be a factor in its valuation.). This is 5308 * the underlying object with id, value and extensions. The 5309 * accessor "getCondition" gives direct access to the value 5310 */ 5311 public ContractAssetComponent setConditionElement(StringType value) { 5312 this.condition = value; 5313 return this; 5314 } 5315 5316 /** 5317 * @return Description of the quality and completeness of the asset that imay be 5318 * a factor in its valuation. 5319 */ 5320 public String getCondition() { 5321 return this.condition == null ? null : this.condition.getValue(); 5322 } 5323 5324 /** 5325 * @param value Description of the quality and completeness of the asset that 5326 * imay be a factor in its valuation. 5327 */ 5328 public ContractAssetComponent setCondition(String value) { 5329 if (Utilities.noString(value)) 5330 this.condition = null; 5331 else { 5332 if (this.condition == null) 5333 this.condition = new StringType(); 5334 this.condition.setValue(value); 5335 } 5336 return this; 5337 } 5338 5339 /** 5340 * @return {@link #periodType} (Type of Asset availability for use or 5341 * ownership.) 5342 */ 5343 public List<CodeableConcept> getPeriodType() { 5344 if (this.periodType == null) 5345 this.periodType = new ArrayList<CodeableConcept>(); 5346 return this.periodType; 5347 } 5348 5349 /** 5350 * @return Returns a reference to <code>this</code> for easy method chaining 5351 */ 5352 public ContractAssetComponent setPeriodType(List<CodeableConcept> thePeriodType) { 5353 this.periodType = thePeriodType; 5354 return this; 5355 } 5356 5357 public boolean hasPeriodType() { 5358 if (this.periodType == null) 5359 return false; 5360 for (CodeableConcept item : this.periodType) 5361 if (!item.isEmpty()) 5362 return true; 5363 return false; 5364 } 5365 5366 public CodeableConcept addPeriodType() { // 3 5367 CodeableConcept t = new CodeableConcept(); 5368 if (this.periodType == null) 5369 this.periodType = new ArrayList<CodeableConcept>(); 5370 this.periodType.add(t); 5371 return t; 5372 } 5373 5374 public ContractAssetComponent addPeriodType(CodeableConcept t) { // 3 5375 if (t == null) 5376 return this; 5377 if (this.periodType == null) 5378 this.periodType = new ArrayList<CodeableConcept>(); 5379 this.periodType.add(t); 5380 return this; 5381 } 5382 5383 /** 5384 * @return The first repetition of repeating field {@link #periodType}, creating 5385 * it if it does not already exist 5386 */ 5387 public CodeableConcept getPeriodTypeFirstRep() { 5388 if (getPeriodType().isEmpty()) { 5389 addPeriodType(); 5390 } 5391 return getPeriodType().get(0); 5392 } 5393 5394 /** 5395 * @return {@link #period} (Asset relevant contractual time period.) 5396 */ 5397 public List<Period> getPeriod() { 5398 if (this.period == null) 5399 this.period = new ArrayList<Period>(); 5400 return this.period; 5401 } 5402 5403 /** 5404 * @return Returns a reference to <code>this</code> for easy method chaining 5405 */ 5406 public ContractAssetComponent setPeriod(List<Period> thePeriod) { 5407 this.period = thePeriod; 5408 return this; 5409 } 5410 5411 public boolean hasPeriod() { 5412 if (this.period == null) 5413 return false; 5414 for (Period item : this.period) 5415 if (!item.isEmpty()) 5416 return true; 5417 return false; 5418 } 5419 5420 public Period addPeriod() { // 3 5421 Period t = new Period(); 5422 if (this.period == null) 5423 this.period = new ArrayList<Period>(); 5424 this.period.add(t); 5425 return t; 5426 } 5427 5428 public ContractAssetComponent addPeriod(Period t) { // 3 5429 if (t == null) 5430 return this; 5431 if (this.period == null) 5432 this.period = new ArrayList<Period>(); 5433 this.period.add(t); 5434 return this; 5435 } 5436 5437 /** 5438 * @return The first repetition of repeating field {@link #period}, creating it 5439 * if it does not already exist 5440 */ 5441 public Period getPeriodFirstRep() { 5442 if (getPeriod().isEmpty()) { 5443 addPeriod(); 5444 } 5445 return getPeriod().get(0); 5446 } 5447 5448 /** 5449 * @return {@link #usePeriod} (Time period of asset use.) 5450 */ 5451 public List<Period> getUsePeriod() { 5452 if (this.usePeriod == null) 5453 this.usePeriod = new ArrayList<Period>(); 5454 return this.usePeriod; 5455 } 5456 5457 /** 5458 * @return Returns a reference to <code>this</code> for easy method chaining 5459 */ 5460 public ContractAssetComponent setUsePeriod(List<Period> theUsePeriod) { 5461 this.usePeriod = theUsePeriod; 5462 return this; 5463 } 5464 5465 public boolean hasUsePeriod() { 5466 if (this.usePeriod == null) 5467 return false; 5468 for (Period item : this.usePeriod) 5469 if (!item.isEmpty()) 5470 return true; 5471 return false; 5472 } 5473 5474 public Period addUsePeriod() { // 3 5475 Period t = new Period(); 5476 if (this.usePeriod == null) 5477 this.usePeriod = new ArrayList<Period>(); 5478 this.usePeriod.add(t); 5479 return t; 5480 } 5481 5482 public ContractAssetComponent addUsePeriod(Period t) { // 3 5483 if (t == null) 5484 return this; 5485 if (this.usePeriod == null) 5486 this.usePeriod = new ArrayList<Period>(); 5487 this.usePeriod.add(t); 5488 return this; 5489 } 5490 5491 /** 5492 * @return The first repetition of repeating field {@link #usePeriod}, creating 5493 * it if it does not already exist 5494 */ 5495 public Period getUsePeriodFirstRep() { 5496 if (getUsePeriod().isEmpty()) { 5497 addUsePeriod(); 5498 } 5499 return getUsePeriod().get(0); 5500 } 5501 5502 /** 5503 * @return {@link #text} (Clause or question text (Prose Object) concerning the 5504 * asset in a linked form, such as a QuestionnaireResponse used in the 5505 * formation of the contract.). This is the underlying object with id, 5506 * value and extensions. The accessor "getText" gives direct access to 5507 * the value 5508 */ 5509 public StringType getTextElement() { 5510 if (this.text == null) 5511 if (Configuration.errorOnAutoCreate()) 5512 throw new Error("Attempt to auto-create ContractAssetComponent.text"); 5513 else if (Configuration.doAutoCreate()) 5514 this.text = new StringType(); // bb 5515 return this.text; 5516 } 5517 5518 public boolean hasTextElement() { 5519 return this.text != null && !this.text.isEmpty(); 5520 } 5521 5522 public boolean hasText() { 5523 return this.text != null && !this.text.isEmpty(); 5524 } 5525 5526 /** 5527 * @param value {@link #text} (Clause or question text (Prose Object) concerning 5528 * the asset in a linked form, such as a QuestionnaireResponse used 5529 * in the formation of the contract.). This is the underlying 5530 * object with id, value and extensions. The accessor "getText" 5531 * gives direct access to the value 5532 */ 5533 public ContractAssetComponent setTextElement(StringType value) { 5534 this.text = value; 5535 return this; 5536 } 5537 5538 /** 5539 * @return Clause or question text (Prose Object) concerning the asset in a 5540 * linked form, such as a QuestionnaireResponse used in the formation of 5541 * the contract. 5542 */ 5543 public String getText() { 5544 return this.text == null ? null : this.text.getValue(); 5545 } 5546 5547 /** 5548 * @param value Clause or question text (Prose Object) concerning the asset in a 5549 * linked form, such as a QuestionnaireResponse used in the 5550 * formation of the contract. 5551 */ 5552 public ContractAssetComponent setText(String value) { 5553 if (Utilities.noString(value)) 5554 this.text = null; 5555 else { 5556 if (this.text == null) 5557 this.text = new StringType(); 5558 this.text.setValue(value); 5559 } 5560 return this; 5561 } 5562 5563 /** 5564 * @return {@link #linkId} (Id [identifier??] of the clause or question text 5565 * about the asset in the referenced form or QuestionnaireResponse.) 5566 */ 5567 public List<StringType> getLinkId() { 5568 if (this.linkId == null) 5569 this.linkId = new ArrayList<StringType>(); 5570 return this.linkId; 5571 } 5572 5573 /** 5574 * @return Returns a reference to <code>this</code> for easy method chaining 5575 */ 5576 public ContractAssetComponent setLinkId(List<StringType> theLinkId) { 5577 this.linkId = theLinkId; 5578 return this; 5579 } 5580 5581 public boolean hasLinkId() { 5582 if (this.linkId == null) 5583 return false; 5584 for (StringType item : this.linkId) 5585 if (!item.isEmpty()) 5586 return true; 5587 return false; 5588 } 5589 5590 /** 5591 * @return {@link #linkId} (Id [identifier??] of the clause or question text 5592 * about the asset in the referenced form or QuestionnaireResponse.) 5593 */ 5594 public StringType addLinkIdElement() {// 2 5595 StringType t = new StringType(); 5596 if (this.linkId == null) 5597 this.linkId = new ArrayList<StringType>(); 5598 this.linkId.add(t); 5599 return t; 5600 } 5601 5602 /** 5603 * @param value {@link #linkId} (Id [identifier??] of the clause or question 5604 * text about the asset in the referenced form or 5605 * QuestionnaireResponse.) 5606 */ 5607 public ContractAssetComponent addLinkId(String value) { // 1 5608 StringType t = new StringType(); 5609 t.setValue(value); 5610 if (this.linkId == null) 5611 this.linkId = new ArrayList<StringType>(); 5612 this.linkId.add(t); 5613 return this; 5614 } 5615 5616 /** 5617 * @param value {@link #linkId} (Id [identifier??] of the clause or question 5618 * text about the asset in the referenced form or 5619 * QuestionnaireResponse.) 5620 */ 5621 public boolean hasLinkId(String value) { 5622 if (this.linkId == null) 5623 return false; 5624 for (StringType v : this.linkId) 5625 if (v.getValue().equals(value)) // string 5626 return true; 5627 return false; 5628 } 5629 5630 /** 5631 * @return {@link #answer} (Response to assets.) 5632 */ 5633 public List<AnswerComponent> getAnswer() { 5634 if (this.answer == null) 5635 this.answer = new ArrayList<AnswerComponent>(); 5636 return this.answer; 5637 } 5638 5639 /** 5640 * @return Returns a reference to <code>this</code> for easy method chaining 5641 */ 5642 public ContractAssetComponent setAnswer(List<AnswerComponent> theAnswer) { 5643 this.answer = theAnswer; 5644 return this; 5645 } 5646 5647 public boolean hasAnswer() { 5648 if (this.answer == null) 5649 return false; 5650 for (AnswerComponent item : this.answer) 5651 if (!item.isEmpty()) 5652 return true; 5653 return false; 5654 } 5655 5656 public AnswerComponent addAnswer() { // 3 5657 AnswerComponent t = new AnswerComponent(); 5658 if (this.answer == null) 5659 this.answer = new ArrayList<AnswerComponent>(); 5660 this.answer.add(t); 5661 return t; 5662 } 5663 5664 public ContractAssetComponent addAnswer(AnswerComponent t) { // 3 5665 if (t == null) 5666 return this; 5667 if (this.answer == null) 5668 this.answer = new ArrayList<AnswerComponent>(); 5669 this.answer.add(t); 5670 return this; 5671 } 5672 5673 /** 5674 * @return The first repetition of repeating field {@link #answer}, creating it 5675 * if it does not already exist 5676 */ 5677 public AnswerComponent getAnswerFirstRep() { 5678 if (getAnswer().isEmpty()) { 5679 addAnswer(); 5680 } 5681 return getAnswer().get(0); 5682 } 5683 5684 /** 5685 * @return {@link #securityLabelNumber} (Security labels that protects the 5686 * asset.) 5687 */ 5688 public List<UnsignedIntType> getSecurityLabelNumber() { 5689 if (this.securityLabelNumber == null) 5690 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5691 return this.securityLabelNumber; 5692 } 5693 5694 /** 5695 * @return Returns a reference to <code>this</code> for easy method chaining 5696 */ 5697 public ContractAssetComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 5698 this.securityLabelNumber = theSecurityLabelNumber; 5699 return this; 5700 } 5701 5702 public boolean hasSecurityLabelNumber() { 5703 if (this.securityLabelNumber == null) 5704 return false; 5705 for (UnsignedIntType item : this.securityLabelNumber) 5706 if (!item.isEmpty()) 5707 return true; 5708 return false; 5709 } 5710 5711 /** 5712 * @return {@link #securityLabelNumber} (Security labels that protects the 5713 * asset.) 5714 */ 5715 public UnsignedIntType addSecurityLabelNumberElement() {// 2 5716 UnsignedIntType t = new UnsignedIntType(); 5717 if (this.securityLabelNumber == null) 5718 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5719 this.securityLabelNumber.add(t); 5720 return t; 5721 } 5722 5723 /** 5724 * @param value {@link #securityLabelNumber} (Security labels that protects the 5725 * asset.) 5726 */ 5727 public ContractAssetComponent addSecurityLabelNumber(int value) { // 1 5728 UnsignedIntType t = new UnsignedIntType(); 5729 t.setValue(value); 5730 if (this.securityLabelNumber == null) 5731 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 5732 this.securityLabelNumber.add(t); 5733 return this; 5734 } 5735 5736 /** 5737 * @param value {@link #securityLabelNumber} (Security labels that protects the 5738 * asset.) 5739 */ 5740 public boolean hasSecurityLabelNumber(int value) { 5741 if (this.securityLabelNumber == null) 5742 return false; 5743 for (UnsignedIntType v : this.securityLabelNumber) 5744 if (v.getValue().equals(value)) // unsignedInt 5745 return true; 5746 return false; 5747 } 5748 5749 /** 5750 * @return {@link #valuedItem} (Contract Valued Item List.) 5751 */ 5752 public List<ValuedItemComponent> getValuedItem() { 5753 if (this.valuedItem == null) 5754 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5755 return this.valuedItem; 5756 } 5757 5758 /** 5759 * @return Returns a reference to <code>this</code> for easy method chaining 5760 */ 5761 public ContractAssetComponent setValuedItem(List<ValuedItemComponent> theValuedItem) { 5762 this.valuedItem = theValuedItem; 5763 return this; 5764 } 5765 5766 public boolean hasValuedItem() { 5767 if (this.valuedItem == null) 5768 return false; 5769 for (ValuedItemComponent item : this.valuedItem) 5770 if (!item.isEmpty()) 5771 return true; 5772 return false; 5773 } 5774 5775 public ValuedItemComponent addValuedItem() { // 3 5776 ValuedItemComponent t = new ValuedItemComponent(); 5777 if (this.valuedItem == null) 5778 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5779 this.valuedItem.add(t); 5780 return t; 5781 } 5782 5783 public ContractAssetComponent addValuedItem(ValuedItemComponent t) { // 3 5784 if (t == null) 5785 return this; 5786 if (this.valuedItem == null) 5787 this.valuedItem = new ArrayList<ValuedItemComponent>(); 5788 this.valuedItem.add(t); 5789 return this; 5790 } 5791 5792 /** 5793 * @return The first repetition of repeating field {@link #valuedItem}, creating 5794 * it if it does not already exist 5795 */ 5796 public ValuedItemComponent getValuedItemFirstRep() { 5797 if (getValuedItem().isEmpty()) { 5798 addValuedItem(); 5799 } 5800 return getValuedItem().get(0); 5801 } 5802 5803 protected void listChildren(List<Property> children) { 5804 super.listChildren(children); 5805 children.add(new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, scope)); 5806 children.add(new Property("type", "CodeableConcept", "Target entity type about which the term may be concerned.", 5807 0, java.lang.Integer.MAX_VALUE, type)); 5808 children.add(new Property("typeReference", "Reference(Any)", "Associated entities.", 0, 5809 java.lang.Integer.MAX_VALUE, typeReference)); 5810 children.add(new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 0, 5811 java.lang.Integer.MAX_VALUE, subtype)); 5812 children.add(new Property("relationship", "Coding", 5813 "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 5814 0, 1, relationship)); 5815 children.add(new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, context)); 5816 children.add(new Property("condition", "string", 5817 "Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1, 5818 condition)); 5819 children.add(new Property("periodType", "CodeableConcept", "Type of Asset availability for use or ownership.", 0, 5820 java.lang.Integer.MAX_VALUE, periodType)); 5821 children.add(new Property("period", "Period", "Asset relevant contractual time period.", 0, 5822 java.lang.Integer.MAX_VALUE, period)); 5823 children.add( 5824 new Property("usePeriod", "Period", "Time period of asset use.", 0, java.lang.Integer.MAX_VALUE, usePeriod)); 5825 children.add(new Property("text", "string", 5826 "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 5827 0, 1, text)); 5828 children.add(new Property("linkId", "string", 5829 "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 5830 0, java.lang.Integer.MAX_VALUE, linkId)); 5831 children.add(new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, 5832 java.lang.Integer.MAX_VALUE, answer)); 5833 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the asset.", 0, 5834 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 5835 children.add( 5836 new Property("valuedItem", "", "Contract Valued Item List.", 0, java.lang.Integer.MAX_VALUE, valuedItem)); 5837 } 5838 5839 @Override 5840 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5841 switch (_hash) { 5842 case 109264468: 5843 /* scope */ return new Property("scope", "CodeableConcept", "Differentiates the kind of the asset .", 0, 1, 5844 scope); 5845 case 3575610: 5846 /* type */ return new Property("type", "CodeableConcept", 5847 "Target entity type about which the term may be concerned.", 0, java.lang.Integer.MAX_VALUE, type); 5848 case 2074825009: 5849 /* typeReference */ return new Property("typeReference", "Reference(Any)", "Associated entities.", 0, 5850 java.lang.Integer.MAX_VALUE, typeReference); 5851 case -1867567750: 5852 /* subtype */ return new Property("subtype", "CodeableConcept", "May be a subtype or part of an offered asset.", 5853 0, java.lang.Integer.MAX_VALUE, subtype); 5854 case -261851592: 5855 /* relationship */ return new Property("relationship", "Coding", 5856 "Specifies the applicability of the term to an asset resource instance, and instances it refers to orinstances that refer to it, and/or are owned by the offeree.", 5857 0, 1, relationship); 5858 case 951530927: 5859 /* context */ return new Property("context", "", "Circumstance of the asset.", 0, java.lang.Integer.MAX_VALUE, 5860 context); 5861 case -861311717: 5862 /* condition */ return new Property("condition", "string", 5863 "Description of the quality and completeness of the asset that imay be a factor in its valuation.", 0, 1, 5864 condition); 5865 case 384348315: 5866 /* periodType */ return new Property("periodType", "CodeableConcept", 5867 "Type of Asset availability for use or ownership.", 0, java.lang.Integer.MAX_VALUE, periodType); 5868 case -991726143: 5869 /* period */ return new Property("period", "Period", "Asset relevant contractual time period.", 0, 5870 java.lang.Integer.MAX_VALUE, period); 5871 case -628382168: 5872 /* usePeriod */ return new Property("usePeriod", "Period", "Time period of asset use.", 0, 5873 java.lang.Integer.MAX_VALUE, usePeriod); 5874 case 3556653: 5875 /* text */ return new Property("text", "string", 5876 "Clause or question text (Prose Object) concerning the asset in a linked form, such as a QuestionnaireResponse used in the formation of the contract.", 5877 0, 1, text); 5878 case -1102667083: 5879 /* linkId */ return new Property("linkId", "string", 5880 "Id [identifier??] of the clause or question text about the asset in the referenced form or QuestionnaireResponse.", 5881 0, java.lang.Integer.MAX_VALUE, linkId); 5882 case -1412808770: 5883 /* answer */ return new Property("answer", "@Contract.term.offer.answer", "Response to assets.", 0, 5884 java.lang.Integer.MAX_VALUE, answer); 5885 case -149460995: 5886 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 5887 "Security labels that protects the asset.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 5888 case 2046675654: 5889 /* valuedItem */ return new Property("valuedItem", "", "Contract Valued Item List.", 0, 5890 java.lang.Integer.MAX_VALUE, valuedItem); 5891 default: 5892 return super.getNamedProperty(_hash, _name, _checkValid); 5893 } 5894 5895 } 5896 5897 @Override 5898 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5899 switch (hash) { 5900 case 109264468: 5901 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept 5902 case 3575610: 5903 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 5904 case 2074825009: 5905 /* typeReference */ return this.typeReference == null ? new Base[0] 5906 : this.typeReference.toArray(new Base[this.typeReference.size()]); // Reference 5907 case -1867567750: 5908 /* subtype */ return this.subtype == null ? new Base[0] : this.subtype.toArray(new Base[this.subtype.size()]); // CodeableConcept 5909 case -261851592: 5910 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // Coding 5911 case 951530927: 5912 /* context */ return this.context == null ? new Base[0] : this.context.toArray(new Base[this.context.size()]); // AssetContextComponent 5913 case -861311717: 5914 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // StringType 5915 case 384348315: 5916 /* periodType */ return this.periodType == null ? new Base[0] 5917 : this.periodType.toArray(new Base[this.periodType.size()]); // CodeableConcept 5918 case -991726143: 5919 /* period */ return this.period == null ? new Base[0] : this.period.toArray(new Base[this.period.size()]); // Period 5920 case -628382168: 5921 /* usePeriod */ return this.usePeriod == null ? new Base[0] 5922 : this.usePeriod.toArray(new Base[this.usePeriod.size()]); // Period 5923 case 3556653: 5924 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 5925 case -1102667083: 5926 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 5927 case -1412808770: 5928 /* answer */ return this.answer == null ? new Base[0] : this.answer.toArray(new Base[this.answer.size()]); // AnswerComponent 5929 case -149460995: 5930 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 5931 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 5932 case 2046675654: 5933 /* valuedItem */ return this.valuedItem == null ? new Base[0] 5934 : this.valuedItem.toArray(new Base[this.valuedItem.size()]); // ValuedItemComponent 5935 default: 5936 return super.getProperty(hash, name, checkValid); 5937 } 5938 5939 } 5940 5941 @Override 5942 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5943 switch (hash) { 5944 case 109264468: // scope 5945 this.scope = castToCodeableConcept(value); // CodeableConcept 5946 return value; 5947 case 3575610: // type 5948 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 5949 return value; 5950 case 2074825009: // typeReference 5951 this.getTypeReference().add(castToReference(value)); // Reference 5952 return value; 5953 case -1867567750: // subtype 5954 this.getSubtype().add(castToCodeableConcept(value)); // CodeableConcept 5955 return value; 5956 case -261851592: // relationship 5957 this.relationship = castToCoding(value); // Coding 5958 return value; 5959 case 951530927: // context 5960 this.getContext().add((AssetContextComponent) value); // AssetContextComponent 5961 return value; 5962 case -861311717: // condition 5963 this.condition = castToString(value); // StringType 5964 return value; 5965 case 384348315: // periodType 5966 this.getPeriodType().add(castToCodeableConcept(value)); // CodeableConcept 5967 return value; 5968 case -991726143: // period 5969 this.getPeriod().add(castToPeriod(value)); // Period 5970 return value; 5971 case -628382168: // usePeriod 5972 this.getUsePeriod().add(castToPeriod(value)); // Period 5973 return value; 5974 case 3556653: // text 5975 this.text = castToString(value); // StringType 5976 return value; 5977 case -1102667083: // linkId 5978 this.getLinkId().add(castToString(value)); // StringType 5979 return value; 5980 case -1412808770: // answer 5981 this.getAnswer().add((AnswerComponent) value); // AnswerComponent 5982 return value; 5983 case -149460995: // securityLabelNumber 5984 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 5985 return value; 5986 case 2046675654: // valuedItem 5987 this.getValuedItem().add((ValuedItemComponent) value); // ValuedItemComponent 5988 return value; 5989 default: 5990 return super.setProperty(hash, name, value); 5991 } 5992 5993 } 5994 5995 @Override 5996 public Base setProperty(String name, Base value) throws FHIRException { 5997 if (name.equals("scope")) { 5998 this.scope = castToCodeableConcept(value); // CodeableConcept 5999 } else if (name.equals("type")) { 6000 this.getType().add(castToCodeableConcept(value)); 6001 } else if (name.equals("typeReference")) { 6002 this.getTypeReference().add(castToReference(value)); 6003 } else if (name.equals("subtype")) { 6004 this.getSubtype().add(castToCodeableConcept(value)); 6005 } else if (name.equals("relationship")) { 6006 this.relationship = castToCoding(value); // Coding 6007 } else if (name.equals("context")) { 6008 this.getContext().add((AssetContextComponent) value); 6009 } else if (name.equals("condition")) { 6010 this.condition = castToString(value); // StringType 6011 } else if (name.equals("periodType")) { 6012 this.getPeriodType().add(castToCodeableConcept(value)); 6013 } else if (name.equals("period")) { 6014 this.getPeriod().add(castToPeriod(value)); 6015 } else if (name.equals("usePeriod")) { 6016 this.getUsePeriod().add(castToPeriod(value)); 6017 } else if (name.equals("text")) { 6018 this.text = castToString(value); // StringType 6019 } else if (name.equals("linkId")) { 6020 this.getLinkId().add(castToString(value)); 6021 } else if (name.equals("answer")) { 6022 this.getAnswer().add((AnswerComponent) value); 6023 } else if (name.equals("securityLabelNumber")) { 6024 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 6025 } else if (name.equals("valuedItem")) { 6026 this.getValuedItem().add((ValuedItemComponent) value); 6027 } else 6028 return super.setProperty(name, value); 6029 return value; 6030 } 6031 6032 @Override 6033 public void removeChild(String name, Base value) throws FHIRException { 6034 if (name.equals("scope")) { 6035 this.scope = null; 6036 } else if (name.equals("type")) { 6037 this.getType().remove(castToCodeableConcept(value)); 6038 } else if (name.equals("typeReference")) { 6039 this.getTypeReference().remove(castToReference(value)); 6040 } else if (name.equals("subtype")) { 6041 this.getSubtype().remove(castToCodeableConcept(value)); 6042 } else if (name.equals("relationship")) { 6043 this.relationship = null; 6044 } else if (name.equals("context")) { 6045 this.getContext().remove((AssetContextComponent) value); 6046 } else if (name.equals("condition")) { 6047 this.condition = null; 6048 } else if (name.equals("periodType")) { 6049 this.getPeriodType().remove(castToCodeableConcept(value)); 6050 } else if (name.equals("period")) { 6051 this.getPeriod().remove(castToPeriod(value)); 6052 } else if (name.equals("usePeriod")) { 6053 this.getUsePeriod().remove(castToPeriod(value)); 6054 } else if (name.equals("text")) { 6055 this.text = null; 6056 } else if (name.equals("linkId")) { 6057 this.getLinkId().remove(castToString(value)); 6058 } else if (name.equals("answer")) { 6059 this.getAnswer().remove((AnswerComponent) value); 6060 } else if (name.equals("securityLabelNumber")) { 6061 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 6062 } else if (name.equals("valuedItem")) { 6063 this.getValuedItem().remove((ValuedItemComponent) value); 6064 } else 6065 super.removeChild(name, value); 6066 6067 } 6068 6069 @Override 6070 public Base makeProperty(int hash, String name) throws FHIRException { 6071 switch (hash) { 6072 case 109264468: 6073 return getScope(); 6074 case 3575610: 6075 return addType(); 6076 case 2074825009: 6077 return addTypeReference(); 6078 case -1867567750: 6079 return addSubtype(); 6080 case -261851592: 6081 return getRelationship(); 6082 case 951530927: 6083 return addContext(); 6084 case -861311717: 6085 return getConditionElement(); 6086 case 384348315: 6087 return addPeriodType(); 6088 case -991726143: 6089 return addPeriod(); 6090 case -628382168: 6091 return addUsePeriod(); 6092 case 3556653: 6093 return getTextElement(); 6094 case -1102667083: 6095 return addLinkIdElement(); 6096 case -1412808770: 6097 return addAnswer(); 6098 case -149460995: 6099 return addSecurityLabelNumberElement(); 6100 case 2046675654: 6101 return addValuedItem(); 6102 default: 6103 return super.makeProperty(hash, name); 6104 } 6105 6106 } 6107 6108 @Override 6109 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6110 switch (hash) { 6111 case 109264468: 6112 /* scope */ return new String[] { "CodeableConcept" }; 6113 case 3575610: 6114 /* type */ return new String[] { "CodeableConcept" }; 6115 case 2074825009: 6116 /* typeReference */ return new String[] { "Reference" }; 6117 case -1867567750: 6118 /* subtype */ return new String[] { "CodeableConcept" }; 6119 case -261851592: 6120 /* relationship */ return new String[] { "Coding" }; 6121 case 951530927: 6122 /* context */ return new String[] {}; 6123 case -861311717: 6124 /* condition */ return new String[] { "string" }; 6125 case 384348315: 6126 /* periodType */ return new String[] { "CodeableConcept" }; 6127 case -991726143: 6128 /* period */ return new String[] { "Period" }; 6129 case -628382168: 6130 /* usePeriod */ return new String[] { "Period" }; 6131 case 3556653: 6132 /* text */ return new String[] { "string" }; 6133 case -1102667083: 6134 /* linkId */ return new String[] { "string" }; 6135 case -1412808770: 6136 /* answer */ return new String[] { "@Contract.term.offer.answer" }; 6137 case -149460995: 6138 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 6139 case 2046675654: 6140 /* valuedItem */ return new String[] {}; 6141 default: 6142 return super.getTypesForProperty(hash, name); 6143 } 6144 6145 } 6146 6147 @Override 6148 public Base addChild(String name) throws FHIRException { 6149 if (name.equals("scope")) { 6150 this.scope = new CodeableConcept(); 6151 return this.scope; 6152 } else if (name.equals("type")) { 6153 return addType(); 6154 } else if (name.equals("typeReference")) { 6155 return addTypeReference(); 6156 } else if (name.equals("subtype")) { 6157 return addSubtype(); 6158 } else if (name.equals("relationship")) { 6159 this.relationship = new Coding(); 6160 return this.relationship; 6161 } else if (name.equals("context")) { 6162 return addContext(); 6163 } else if (name.equals("condition")) { 6164 throw new FHIRException("Cannot call addChild on a singleton property Contract.condition"); 6165 } else if (name.equals("periodType")) { 6166 return addPeriodType(); 6167 } else if (name.equals("period")) { 6168 return addPeriod(); 6169 } else if (name.equals("usePeriod")) { 6170 return addUsePeriod(); 6171 } else if (name.equals("text")) { 6172 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 6173 } else if (name.equals("linkId")) { 6174 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 6175 } else if (name.equals("answer")) { 6176 return addAnswer(); 6177 } else if (name.equals("securityLabelNumber")) { 6178 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 6179 } else if (name.equals("valuedItem")) { 6180 return addValuedItem(); 6181 } else 6182 return super.addChild(name); 6183 } 6184 6185 public ContractAssetComponent copy() { 6186 ContractAssetComponent dst = new ContractAssetComponent(); 6187 copyValues(dst); 6188 return dst; 6189 } 6190 6191 public void copyValues(ContractAssetComponent dst) { 6192 super.copyValues(dst); 6193 dst.scope = scope == null ? null : scope.copy(); 6194 if (type != null) { 6195 dst.type = new ArrayList<CodeableConcept>(); 6196 for (CodeableConcept i : type) 6197 dst.type.add(i.copy()); 6198 } 6199 ; 6200 if (typeReference != null) { 6201 dst.typeReference = new ArrayList<Reference>(); 6202 for (Reference i : typeReference) 6203 dst.typeReference.add(i.copy()); 6204 } 6205 ; 6206 if (subtype != null) { 6207 dst.subtype = new ArrayList<CodeableConcept>(); 6208 for (CodeableConcept i : subtype) 6209 dst.subtype.add(i.copy()); 6210 } 6211 ; 6212 dst.relationship = relationship == null ? null : relationship.copy(); 6213 if (context != null) { 6214 dst.context = new ArrayList<AssetContextComponent>(); 6215 for (AssetContextComponent i : context) 6216 dst.context.add(i.copy()); 6217 } 6218 ; 6219 dst.condition = condition == null ? null : condition.copy(); 6220 if (periodType != null) { 6221 dst.periodType = new ArrayList<CodeableConcept>(); 6222 for (CodeableConcept i : periodType) 6223 dst.periodType.add(i.copy()); 6224 } 6225 ; 6226 if (period != null) { 6227 dst.period = new ArrayList<Period>(); 6228 for (Period i : period) 6229 dst.period.add(i.copy()); 6230 } 6231 ; 6232 if (usePeriod != null) { 6233 dst.usePeriod = new ArrayList<Period>(); 6234 for (Period i : usePeriod) 6235 dst.usePeriod.add(i.copy()); 6236 } 6237 ; 6238 dst.text = text == null ? null : text.copy(); 6239 if (linkId != null) { 6240 dst.linkId = new ArrayList<StringType>(); 6241 for (StringType i : linkId) 6242 dst.linkId.add(i.copy()); 6243 } 6244 ; 6245 if (answer != null) { 6246 dst.answer = new ArrayList<AnswerComponent>(); 6247 for (AnswerComponent i : answer) 6248 dst.answer.add(i.copy()); 6249 } 6250 ; 6251 if (securityLabelNumber != null) { 6252 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 6253 for (UnsignedIntType i : securityLabelNumber) 6254 dst.securityLabelNumber.add(i.copy()); 6255 } 6256 ; 6257 if (valuedItem != null) { 6258 dst.valuedItem = new ArrayList<ValuedItemComponent>(); 6259 for (ValuedItemComponent i : valuedItem) 6260 dst.valuedItem.add(i.copy()); 6261 } 6262 ; 6263 } 6264 6265 @Override 6266 public boolean equalsDeep(Base other_) { 6267 if (!super.equalsDeep(other_)) 6268 return false; 6269 if (!(other_ instanceof ContractAssetComponent)) 6270 return false; 6271 ContractAssetComponent o = (ContractAssetComponent) other_; 6272 return compareDeep(scope, o.scope, true) && compareDeep(type, o.type, true) 6273 && compareDeep(typeReference, o.typeReference, true) && compareDeep(subtype, o.subtype, true) 6274 && compareDeep(relationship, o.relationship, true) && compareDeep(context, o.context, true) 6275 && compareDeep(condition, o.condition, true) && compareDeep(periodType, o.periodType, true) 6276 && compareDeep(period, o.period, true) && compareDeep(usePeriod, o.usePeriod, true) 6277 && compareDeep(text, o.text, true) && compareDeep(linkId, o.linkId, true) 6278 && compareDeep(answer, o.answer, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true) 6279 && compareDeep(valuedItem, o.valuedItem, true); 6280 } 6281 6282 @Override 6283 public boolean equalsShallow(Base other_) { 6284 if (!super.equalsShallow(other_)) 6285 return false; 6286 if (!(other_ instanceof ContractAssetComponent)) 6287 return false; 6288 ContractAssetComponent o = (ContractAssetComponent) other_; 6289 return compareValues(condition, o.condition, true) && compareValues(text, o.text, true) 6290 && compareValues(linkId, o.linkId, true) && compareValues(securityLabelNumber, o.securityLabelNumber, true); 6291 } 6292 6293 public boolean isEmpty() { 6294 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(scope, type, typeReference, subtype, relationship, 6295 context, condition, periodType, period, usePeriod, text, linkId, answer, securityLabelNumber, valuedItem); 6296 } 6297 6298 public String fhirType() { 6299 return "Contract.term.asset"; 6300 6301 } 6302 6303 } 6304 6305 @Block() 6306 public static class AssetContextComponent extends BackboneElement implements IBaseBackboneElement { 6307 /** 6308 * Asset context reference may include the creator, custodian, or owning Person 6309 * or Organization (e.g., bank, repository), location held, e.g., building, 6310 * jurisdiction. 6311 */ 6312 @Child(name = "reference", type = { 6313 Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6314 @Description(shortDefinition = "Creator,custodian or owner", formalDefinition = "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.") 6315 protected Reference reference; 6316 6317 /** 6318 * The actual object that is the target of the reference (Asset context 6319 * reference may include the creator, custodian, or owning Person or 6320 * Organization (e.g., bank, repository), location held, e.g., building, 6321 * jurisdiction.) 6322 */ 6323 protected Resource referenceTarget; 6324 6325 /** 6326 * Coded representation of the context generally or of the Referenced entity, 6327 * such as the asset holder type or location. 6328 */ 6329 @Child(name = "code", type = { 6330 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6331 @Description(shortDefinition = "Codeable asset context", formalDefinition = "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.") 6332 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-assetcontext") 6333 protected List<CodeableConcept> code; 6334 6335 /** 6336 * Context description. 6337 */ 6338 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6339 @Description(shortDefinition = "Context description", formalDefinition = "Context description.") 6340 protected StringType text; 6341 6342 private static final long serialVersionUID = -634115628L; 6343 6344 /** 6345 * Constructor 6346 */ 6347 public AssetContextComponent() { 6348 super(); 6349 } 6350 6351 /** 6352 * @return {@link #reference} (Asset context reference may include the creator, 6353 * custodian, or owning Person or Organization (e.g., bank, repository), 6354 * location held, e.g., building, jurisdiction.) 6355 */ 6356 public Reference getReference() { 6357 if (this.reference == null) 6358 if (Configuration.errorOnAutoCreate()) 6359 throw new Error("Attempt to auto-create AssetContextComponent.reference"); 6360 else if (Configuration.doAutoCreate()) 6361 this.reference = new Reference(); // cc 6362 return this.reference; 6363 } 6364 6365 public boolean hasReference() { 6366 return this.reference != null && !this.reference.isEmpty(); 6367 } 6368 6369 /** 6370 * @param value {@link #reference} (Asset context reference may include the 6371 * creator, custodian, or owning Person or Organization (e.g., 6372 * bank, repository), location held, e.g., building, jurisdiction.) 6373 */ 6374 public AssetContextComponent setReference(Reference value) { 6375 this.reference = value; 6376 return this; 6377 } 6378 6379 /** 6380 * @return {@link #reference} The actual object that is the target of the 6381 * reference. The reference library doesn't populate this, but you can 6382 * use it to hold the resource if you resolve it. (Asset context 6383 * reference may include the creator, custodian, or owning Person or 6384 * Organization (e.g., bank, repository), location held, e.g., building, 6385 * jurisdiction.) 6386 */ 6387 public Resource getReferenceTarget() { 6388 return this.referenceTarget; 6389 } 6390 6391 /** 6392 * @param value {@link #reference} The actual object that is the target of the 6393 * reference. The reference library doesn't use these, but you can 6394 * use it to hold the resource if you resolve it. (Asset context 6395 * reference may include the creator, custodian, or owning Person 6396 * or Organization (e.g., bank, repository), location held, e.g., 6397 * building, jurisdiction.) 6398 */ 6399 public AssetContextComponent setReferenceTarget(Resource value) { 6400 this.referenceTarget = value; 6401 return this; 6402 } 6403 6404 /** 6405 * @return {@link #code} (Coded representation of the context generally or of 6406 * the Referenced entity, such as the asset holder type or location.) 6407 */ 6408 public List<CodeableConcept> getCode() { 6409 if (this.code == null) 6410 this.code = new ArrayList<CodeableConcept>(); 6411 return this.code; 6412 } 6413 6414 /** 6415 * @return Returns a reference to <code>this</code> for easy method chaining 6416 */ 6417 public AssetContextComponent setCode(List<CodeableConcept> theCode) { 6418 this.code = theCode; 6419 return this; 6420 } 6421 6422 public boolean hasCode() { 6423 if (this.code == null) 6424 return false; 6425 for (CodeableConcept item : this.code) 6426 if (!item.isEmpty()) 6427 return true; 6428 return false; 6429 } 6430 6431 public CodeableConcept addCode() { // 3 6432 CodeableConcept t = new CodeableConcept(); 6433 if (this.code == null) 6434 this.code = new ArrayList<CodeableConcept>(); 6435 this.code.add(t); 6436 return t; 6437 } 6438 6439 public AssetContextComponent addCode(CodeableConcept t) { // 3 6440 if (t == null) 6441 return this; 6442 if (this.code == null) 6443 this.code = new ArrayList<CodeableConcept>(); 6444 this.code.add(t); 6445 return this; 6446 } 6447 6448 /** 6449 * @return The first repetition of repeating field {@link #code}, creating it if 6450 * it does not already exist 6451 */ 6452 public CodeableConcept getCodeFirstRep() { 6453 if (getCode().isEmpty()) { 6454 addCode(); 6455 } 6456 return getCode().get(0); 6457 } 6458 6459 /** 6460 * @return {@link #text} (Context description.). This is the underlying object 6461 * with id, value and extensions. The accessor "getText" gives direct 6462 * access to the value 6463 */ 6464 public StringType getTextElement() { 6465 if (this.text == null) 6466 if (Configuration.errorOnAutoCreate()) 6467 throw new Error("Attempt to auto-create AssetContextComponent.text"); 6468 else if (Configuration.doAutoCreate()) 6469 this.text = new StringType(); // bb 6470 return this.text; 6471 } 6472 6473 public boolean hasTextElement() { 6474 return this.text != null && !this.text.isEmpty(); 6475 } 6476 6477 public boolean hasText() { 6478 return this.text != null && !this.text.isEmpty(); 6479 } 6480 6481 /** 6482 * @param value {@link #text} (Context description.). This is the underlying 6483 * object with id, value and extensions. The accessor "getText" 6484 * gives direct access to the value 6485 */ 6486 public AssetContextComponent setTextElement(StringType value) { 6487 this.text = value; 6488 return this; 6489 } 6490 6491 /** 6492 * @return Context description. 6493 */ 6494 public String getText() { 6495 return this.text == null ? null : this.text.getValue(); 6496 } 6497 6498 /** 6499 * @param value Context description. 6500 */ 6501 public AssetContextComponent setText(String value) { 6502 if (Utilities.noString(value)) 6503 this.text = null; 6504 else { 6505 if (this.text == null) 6506 this.text = new StringType(); 6507 this.text.setValue(value); 6508 } 6509 return this; 6510 } 6511 6512 protected void listChildren(List<Property> children) { 6513 super.listChildren(children); 6514 children.add(new Property("reference", "Reference(Any)", 6515 "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 6516 0, 1, reference)); 6517 children.add(new Property("code", "CodeableConcept", 6518 "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 6519 0, java.lang.Integer.MAX_VALUE, code)); 6520 children.add(new Property("text", "string", "Context description.", 0, 1, text)); 6521 } 6522 6523 @Override 6524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6525 switch (_hash) { 6526 case -925155509: 6527 /* reference */ return new Property("reference", "Reference(Any)", 6528 "Asset context reference may include the creator, custodian, or owning Person or Organization (e.g., bank, repository), location held, e.g., building, jurisdiction.", 6529 0, 1, reference); 6530 case 3059181: 6531 /* code */ return new Property("code", "CodeableConcept", 6532 "Coded representation of the context generally or of the Referenced entity, such as the asset holder type or location.", 6533 0, java.lang.Integer.MAX_VALUE, code); 6534 case 3556653: 6535 /* text */ return new Property("text", "string", "Context description.", 0, 1, text); 6536 default: 6537 return super.getNamedProperty(_hash, _name, _checkValid); 6538 } 6539 6540 } 6541 6542 @Override 6543 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6544 switch (hash) { 6545 case -925155509: 6546 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // Reference 6547 case 3059181: 6548 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 6549 case 3556653: 6550 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 6551 default: 6552 return super.getProperty(hash, name, checkValid); 6553 } 6554 6555 } 6556 6557 @Override 6558 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6559 switch (hash) { 6560 case -925155509: // reference 6561 this.reference = castToReference(value); // Reference 6562 return value; 6563 case 3059181: // code 6564 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 6565 return value; 6566 case 3556653: // text 6567 this.text = castToString(value); // StringType 6568 return value; 6569 default: 6570 return super.setProperty(hash, name, value); 6571 } 6572 6573 } 6574 6575 @Override 6576 public Base setProperty(String name, Base value) throws FHIRException { 6577 if (name.equals("reference")) { 6578 this.reference = castToReference(value); // Reference 6579 } else if (name.equals("code")) { 6580 this.getCode().add(castToCodeableConcept(value)); 6581 } else if (name.equals("text")) { 6582 this.text = castToString(value); // StringType 6583 } else 6584 return super.setProperty(name, value); 6585 return value; 6586 } 6587 6588 @Override 6589 public void removeChild(String name, Base value) throws FHIRException { 6590 if (name.equals("reference")) { 6591 this.reference = null; 6592 } else if (name.equals("code")) { 6593 this.getCode().remove(castToCodeableConcept(value)); 6594 } else if (name.equals("text")) { 6595 this.text = null; 6596 } else 6597 super.removeChild(name, value); 6598 6599 } 6600 6601 @Override 6602 public Base makeProperty(int hash, String name) throws FHIRException { 6603 switch (hash) { 6604 case -925155509: 6605 return getReference(); 6606 case 3059181: 6607 return addCode(); 6608 case 3556653: 6609 return getTextElement(); 6610 default: 6611 return super.makeProperty(hash, name); 6612 } 6613 6614 } 6615 6616 @Override 6617 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6618 switch (hash) { 6619 case -925155509: 6620 /* reference */ return new String[] { "Reference" }; 6621 case 3059181: 6622 /* code */ return new String[] { "CodeableConcept" }; 6623 case 3556653: 6624 /* text */ return new String[] { "string" }; 6625 default: 6626 return super.getTypesForProperty(hash, name); 6627 } 6628 6629 } 6630 6631 @Override 6632 public Base addChild(String name) throws FHIRException { 6633 if (name.equals("reference")) { 6634 this.reference = new Reference(); 6635 return this.reference; 6636 } else if (name.equals("code")) { 6637 return addCode(); 6638 } else if (name.equals("text")) { 6639 throw new FHIRException("Cannot call addChild on a singleton property Contract.text"); 6640 } else 6641 return super.addChild(name); 6642 } 6643 6644 public AssetContextComponent copy() { 6645 AssetContextComponent dst = new AssetContextComponent(); 6646 copyValues(dst); 6647 return dst; 6648 } 6649 6650 public void copyValues(AssetContextComponent dst) { 6651 super.copyValues(dst); 6652 dst.reference = reference == null ? null : reference.copy(); 6653 if (code != null) { 6654 dst.code = new ArrayList<CodeableConcept>(); 6655 for (CodeableConcept i : code) 6656 dst.code.add(i.copy()); 6657 } 6658 ; 6659 dst.text = text == null ? null : text.copy(); 6660 } 6661 6662 @Override 6663 public boolean equalsDeep(Base other_) { 6664 if (!super.equalsDeep(other_)) 6665 return false; 6666 if (!(other_ instanceof AssetContextComponent)) 6667 return false; 6668 AssetContextComponent o = (AssetContextComponent) other_; 6669 return compareDeep(reference, o.reference, true) && compareDeep(code, o.code, true) 6670 && compareDeep(text, o.text, true); 6671 } 6672 6673 @Override 6674 public boolean equalsShallow(Base other_) { 6675 if (!super.equalsShallow(other_)) 6676 return false; 6677 if (!(other_ instanceof AssetContextComponent)) 6678 return false; 6679 AssetContextComponent o = (AssetContextComponent) other_; 6680 return compareValues(text, o.text, true); 6681 } 6682 6683 public boolean isEmpty() { 6684 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, code, text); 6685 } 6686 6687 public String fhirType() { 6688 return "Contract.term.asset.context"; 6689 6690 } 6691 6692 } 6693 6694 @Block() 6695 public static class ValuedItemComponent extends BackboneElement implements IBaseBackboneElement { 6696 /** 6697 * Specific type of Contract Valued Item that may be priced. 6698 */ 6699 @Child(name = "entity", type = { CodeableConcept.class, 6700 Reference.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 6701 @Description(shortDefinition = "Contract Valued Item Type", formalDefinition = "Specific type of Contract Valued Item that may be priced.") 6702 protected Type entity; 6703 6704 /** 6705 * Identifies a Contract Valued Item instance. 6706 */ 6707 @Child(name = "identifier", type = { 6708 Identifier.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6709 @Description(shortDefinition = "Contract Valued Item Number", formalDefinition = "Identifies a Contract Valued Item instance.") 6710 protected Identifier identifier; 6711 6712 /** 6713 * Indicates the time during which this Contract ValuedItem information is 6714 * effective. 6715 */ 6716 @Child(name = "effectiveTime", type = { 6717 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6718 @Description(shortDefinition = "Contract Valued Item Effective Tiem", formalDefinition = "Indicates the time during which this Contract ValuedItem information is effective.") 6719 protected DateTimeType effectiveTime; 6720 6721 /** 6722 * Specifies the units by which the Contract Valued Item is measured or counted, 6723 * and quantifies the countable or measurable Contract Valued Item instances. 6724 */ 6725 @Child(name = "quantity", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6726 @Description(shortDefinition = "Count of Contract Valued Items", formalDefinition = "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.") 6727 protected Quantity quantity; 6728 6729 /** 6730 * A Contract Valued Item unit valuation measure. 6731 */ 6732 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6733 @Description(shortDefinition = "Contract Valued Item fee, charge, or cost", formalDefinition = "A Contract Valued Item unit valuation measure.") 6734 protected Money unitPrice; 6735 6736 /** 6737 * A real number that represents a multiplier used in determining the overall 6738 * value of the Contract Valued Item delivered. The concept of a Factor allows 6739 * for a discount or surcharge multiplier to be applied to a monetary amount. 6740 */ 6741 @Child(name = "factor", type = { 6742 DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6743 @Description(shortDefinition = "Contract Valued Item Price Scaling Factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 6744 protected DecimalType factor; 6745 6746 /** 6747 * An amount that expresses the weighting (based on difficulty, cost and/or 6748 * resource intensiveness) associated with the Contract Valued Item delivered. 6749 * The concept of Points allows for assignment of point values for a Contract 6750 * Valued Item, such that a monetary amount can be assigned to each point. 6751 */ 6752 @Child(name = "points", type = { 6753 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6754 @Description(shortDefinition = "Contract Valued Item Difficulty Scaling Factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.") 6755 protected DecimalType points; 6756 6757 /** 6758 * Expresses the product of the Contract Valued Item unitQuantity and the 6759 * unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per 6760 * Point) * factor Number * points = net Amount. Quantity, factor and points are 6761 * assumed to be 1 if not supplied. 6762 */ 6763 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6764 @Description(shortDefinition = "Total Contract Valued Item Value", formalDefinition = "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 6765 protected Money net; 6766 6767 /** 6768 * Terms of valuation. 6769 */ 6770 @Child(name = "payment", type = { 6771 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6772 @Description(shortDefinition = "Terms of valuation", formalDefinition = "Terms of valuation.") 6773 protected StringType payment; 6774 6775 /** 6776 * When payment is due. 6777 */ 6778 @Child(name = "paymentDate", type = { 6779 DateTimeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6780 @Description(shortDefinition = "When payment is due", formalDefinition = "When payment is due.") 6781 protected DateTimeType paymentDate; 6782 6783 /** 6784 * Who will make payment. 6785 */ 6786 @Child(name = "responsible", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 6787 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 6788 @Description(shortDefinition = "Who will make payment", formalDefinition = "Who will make payment.") 6789 protected Reference responsible; 6790 6791 /** 6792 * The actual object that is the target of the reference (Who will make 6793 * payment.) 6794 */ 6795 protected Resource responsibleTarget; 6796 6797 /** 6798 * Who will receive payment. 6799 */ 6800 @Child(name = "recipient", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 6801 RelatedPerson.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 6802 @Description(shortDefinition = "Who will receive payment", formalDefinition = "Who will receive payment.") 6803 protected Reference recipient; 6804 6805 /** 6806 * The actual object that is the target of the reference (Who will receive 6807 * payment.) 6808 */ 6809 protected Resource recipientTarget; 6810 6811 /** 6812 * Id of the clause or question text related to the context of this valuedItem 6813 * in the referenced form or QuestionnaireResponse. 6814 */ 6815 @Child(name = "linkId", type = { 6816 StringType.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6817 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.") 6818 protected List<StringType> linkId; 6819 6820 /** 6821 * A set of security labels that define which terms are controlled by this 6822 * condition. 6823 */ 6824 @Child(name = "securityLabelNumber", type = { 6825 UnsignedIntType.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 6826 @Description(shortDefinition = "Security Labels that define affected terms", formalDefinition = "A set of security labels that define which terms are controlled by this condition.") 6827 protected List<UnsignedIntType> securityLabelNumber; 6828 6829 private static final long serialVersionUID = 1894951601L; 6830 6831 /** 6832 * Constructor 6833 */ 6834 public ValuedItemComponent() { 6835 super(); 6836 } 6837 6838 /** 6839 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6840 * priced.) 6841 */ 6842 public Type getEntity() { 6843 return this.entity; 6844 } 6845 6846 /** 6847 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6848 * priced.) 6849 */ 6850 public CodeableConcept getEntityCodeableConcept() throws FHIRException { 6851 if (this.entity == null) 6852 this.entity = new CodeableConcept(); 6853 if (!(this.entity instanceof CodeableConcept)) 6854 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 6855 + this.entity.getClass().getName() + " was encountered"); 6856 return (CodeableConcept) this.entity; 6857 } 6858 6859 public boolean hasEntityCodeableConcept() { 6860 return this.entity instanceof CodeableConcept; 6861 } 6862 6863 /** 6864 * @return {@link #entity} (Specific type of Contract Valued Item that may be 6865 * priced.) 6866 */ 6867 public Reference getEntityReference() throws FHIRException { 6868 if (this.entity == null) 6869 this.entity = new Reference(); 6870 if (!(this.entity instanceof Reference)) 6871 throw new FHIRException("Type mismatch: the type Reference was expected, but " 6872 + this.entity.getClass().getName() + " was encountered"); 6873 return (Reference) this.entity; 6874 } 6875 6876 public boolean hasEntityReference() { 6877 return this.entity instanceof Reference; 6878 } 6879 6880 public boolean hasEntity() { 6881 return this.entity != null && !this.entity.isEmpty(); 6882 } 6883 6884 /** 6885 * @param value {@link #entity} (Specific type of Contract Valued Item that may 6886 * be priced.) 6887 */ 6888 public ValuedItemComponent setEntity(Type value) { 6889 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 6890 throw new Error("Not the right type for Contract.term.asset.valuedItem.entity[x]: " + value.fhirType()); 6891 this.entity = value; 6892 return this; 6893 } 6894 6895 /** 6896 * @return {@link #identifier} (Identifies a Contract Valued Item instance.) 6897 */ 6898 public Identifier getIdentifier() { 6899 if (this.identifier == null) 6900 if (Configuration.errorOnAutoCreate()) 6901 throw new Error("Attempt to auto-create ValuedItemComponent.identifier"); 6902 else if (Configuration.doAutoCreate()) 6903 this.identifier = new Identifier(); // cc 6904 return this.identifier; 6905 } 6906 6907 public boolean hasIdentifier() { 6908 return this.identifier != null && !this.identifier.isEmpty(); 6909 } 6910 6911 /** 6912 * @param value {@link #identifier} (Identifies a Contract Valued Item 6913 * instance.) 6914 */ 6915 public ValuedItemComponent setIdentifier(Identifier value) { 6916 this.identifier = value; 6917 return this; 6918 } 6919 6920 /** 6921 * @return {@link #effectiveTime} (Indicates the time during which this Contract 6922 * ValuedItem information is effective.). This is the underlying object 6923 * with id, value and extensions. The accessor "getEffectiveTime" gives 6924 * direct access to the value 6925 */ 6926 public DateTimeType getEffectiveTimeElement() { 6927 if (this.effectiveTime == null) 6928 if (Configuration.errorOnAutoCreate()) 6929 throw new Error("Attempt to auto-create ValuedItemComponent.effectiveTime"); 6930 else if (Configuration.doAutoCreate()) 6931 this.effectiveTime = new DateTimeType(); // bb 6932 return this.effectiveTime; 6933 } 6934 6935 public boolean hasEffectiveTimeElement() { 6936 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 6937 } 6938 6939 public boolean hasEffectiveTime() { 6940 return this.effectiveTime != null && !this.effectiveTime.isEmpty(); 6941 } 6942 6943 /** 6944 * @param value {@link #effectiveTime} (Indicates the time during which this 6945 * Contract ValuedItem information is effective.). This is the 6946 * underlying object with id, value and extensions. The accessor 6947 * "getEffectiveTime" gives direct access to the value 6948 */ 6949 public ValuedItemComponent setEffectiveTimeElement(DateTimeType value) { 6950 this.effectiveTime = value; 6951 return this; 6952 } 6953 6954 /** 6955 * @return Indicates the time during which this Contract ValuedItem information 6956 * is effective. 6957 */ 6958 public Date getEffectiveTime() { 6959 return this.effectiveTime == null ? null : this.effectiveTime.getValue(); 6960 } 6961 6962 /** 6963 * @param value Indicates the time during which this Contract ValuedItem 6964 * information is effective. 6965 */ 6966 public ValuedItemComponent setEffectiveTime(Date value) { 6967 if (value == null) 6968 this.effectiveTime = null; 6969 else { 6970 if (this.effectiveTime == null) 6971 this.effectiveTime = new DateTimeType(); 6972 this.effectiveTime.setValue(value); 6973 } 6974 return this; 6975 } 6976 6977 /** 6978 * @return {@link #quantity} (Specifies the units by which the Contract Valued 6979 * Item is measured or counted, and quantifies the countable or 6980 * measurable Contract Valued Item instances.) 6981 */ 6982 public Quantity getQuantity() { 6983 if (this.quantity == null) 6984 if (Configuration.errorOnAutoCreate()) 6985 throw new Error("Attempt to auto-create ValuedItemComponent.quantity"); 6986 else if (Configuration.doAutoCreate()) 6987 this.quantity = new Quantity(); // cc 6988 return this.quantity; 6989 } 6990 6991 public boolean hasQuantity() { 6992 return this.quantity != null && !this.quantity.isEmpty(); 6993 } 6994 6995 /** 6996 * @param value {@link #quantity} (Specifies the units by which the Contract 6997 * Valued Item is measured or counted, and quantifies the countable 6998 * or measurable Contract Valued Item instances.) 6999 */ 7000 public ValuedItemComponent setQuantity(Quantity value) { 7001 this.quantity = value; 7002 return this; 7003 } 7004 7005 /** 7006 * @return {@link #unitPrice} (A Contract Valued Item unit valuation measure.) 7007 */ 7008 public Money getUnitPrice() { 7009 if (this.unitPrice == null) 7010 if (Configuration.errorOnAutoCreate()) 7011 throw new Error("Attempt to auto-create ValuedItemComponent.unitPrice"); 7012 else if (Configuration.doAutoCreate()) 7013 this.unitPrice = new Money(); // cc 7014 return this.unitPrice; 7015 } 7016 7017 public boolean hasUnitPrice() { 7018 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7019 } 7020 7021 /** 7022 * @param value {@link #unitPrice} (A Contract Valued Item unit valuation 7023 * measure.) 7024 */ 7025 public ValuedItemComponent setUnitPrice(Money value) { 7026 this.unitPrice = value; 7027 return this; 7028 } 7029 7030 /** 7031 * @return {@link #factor} (A real number that represents a multiplier used in 7032 * determining the overall value of the Contract Valued Item delivered. 7033 * The concept of a Factor allows for a discount or surcharge multiplier 7034 * to be applied to a monetary amount.). This is the underlying object 7035 * with id, value and extensions. The accessor "getFactor" gives direct 7036 * access to the value 7037 */ 7038 public DecimalType getFactorElement() { 7039 if (this.factor == null) 7040 if (Configuration.errorOnAutoCreate()) 7041 throw new Error("Attempt to auto-create ValuedItemComponent.factor"); 7042 else if (Configuration.doAutoCreate()) 7043 this.factor = new DecimalType(); // bb 7044 return this.factor; 7045 } 7046 7047 public boolean hasFactorElement() { 7048 return this.factor != null && !this.factor.isEmpty(); 7049 } 7050 7051 public boolean hasFactor() { 7052 return this.factor != null && !this.factor.isEmpty(); 7053 } 7054 7055 /** 7056 * @param value {@link #factor} (A real number that represents a multiplier used 7057 * in determining the overall value of the Contract Valued Item 7058 * delivered. The concept of a Factor allows for a discount or 7059 * surcharge multiplier to be applied to a monetary amount.). This 7060 * is the underlying object with id, value and extensions. The 7061 * accessor "getFactor" gives direct access to the value 7062 */ 7063 public ValuedItemComponent setFactorElement(DecimalType value) { 7064 this.factor = value; 7065 return this; 7066 } 7067 7068 /** 7069 * @return A real number that represents a multiplier used in determining the 7070 * overall value of the Contract Valued Item delivered. The concept of a 7071 * Factor allows for a discount or surcharge multiplier to be applied to 7072 * a monetary amount. 7073 */ 7074 public BigDecimal getFactor() { 7075 return this.factor == null ? null : this.factor.getValue(); 7076 } 7077 7078 /** 7079 * @param value A real number that represents a multiplier used in determining 7080 * the overall value of the Contract Valued Item delivered. The 7081 * concept of a Factor allows for a discount or surcharge 7082 * multiplier to be applied to a monetary amount. 7083 */ 7084 public ValuedItemComponent setFactor(BigDecimal value) { 7085 if (value == null) 7086 this.factor = null; 7087 else { 7088 if (this.factor == null) 7089 this.factor = new DecimalType(); 7090 this.factor.setValue(value); 7091 } 7092 return this; 7093 } 7094 7095 /** 7096 * @param value A real number that represents a multiplier used in determining 7097 * the overall value of the Contract Valued Item delivered. The 7098 * concept of a Factor allows for a discount or surcharge 7099 * multiplier to be applied to a monetary amount. 7100 */ 7101 public ValuedItemComponent setFactor(long value) { 7102 this.factor = new DecimalType(); 7103 this.factor.setValue(value); 7104 return this; 7105 } 7106 7107 /** 7108 * @param value A real number that represents a multiplier used in determining 7109 * the overall value of the Contract Valued Item delivered. The 7110 * concept of a Factor allows for a discount or surcharge 7111 * multiplier to be applied to a monetary amount. 7112 */ 7113 public ValuedItemComponent setFactor(double value) { 7114 this.factor = new DecimalType(); 7115 this.factor.setValue(value); 7116 return this; 7117 } 7118 7119 /** 7120 * @return {@link #points} (An amount that expresses the weighting (based on 7121 * difficulty, cost and/or resource intensiveness) associated with the 7122 * Contract Valued Item delivered. The concept of Points allows for 7123 * assignment of point values for a Contract Valued Item, such that a 7124 * monetary amount can be assigned to each point.). This is the 7125 * underlying object with id, value and extensions. The accessor 7126 * "getPoints" gives direct access to the value 7127 */ 7128 public DecimalType getPointsElement() { 7129 if (this.points == null) 7130 if (Configuration.errorOnAutoCreate()) 7131 throw new Error("Attempt to auto-create ValuedItemComponent.points"); 7132 else if (Configuration.doAutoCreate()) 7133 this.points = new DecimalType(); // bb 7134 return this.points; 7135 } 7136 7137 public boolean hasPointsElement() { 7138 return this.points != null && !this.points.isEmpty(); 7139 } 7140 7141 public boolean hasPoints() { 7142 return this.points != null && !this.points.isEmpty(); 7143 } 7144 7145 /** 7146 * @param value {@link #points} (An amount that expresses the weighting (based 7147 * on difficulty, cost and/or resource intensiveness) associated 7148 * with the Contract Valued Item delivered. The concept of Points 7149 * allows for assignment of point values for a Contract Valued 7150 * Item, such that a monetary amount can be assigned to each 7151 * point.). This is the underlying object with id, value and 7152 * extensions. The accessor "getPoints" gives direct access to the 7153 * value 7154 */ 7155 public ValuedItemComponent setPointsElement(DecimalType value) { 7156 this.points = value; 7157 return this; 7158 } 7159 7160 /** 7161 * @return An amount that expresses the weighting (based on difficulty, cost 7162 * and/or resource intensiveness) associated with the Contract Valued 7163 * Item delivered. The concept of Points allows for assignment of point 7164 * values for a Contract Valued Item, such that a monetary amount can be 7165 * assigned to each point. 7166 */ 7167 public BigDecimal getPoints() { 7168 return this.points == null ? null : this.points.getValue(); 7169 } 7170 7171 /** 7172 * @param value An amount that expresses the weighting (based on difficulty, 7173 * cost and/or resource intensiveness) associated with the Contract 7174 * Valued Item delivered. The concept of Points allows for 7175 * assignment of point values for a Contract Valued Item, such that 7176 * a monetary amount can be assigned to each point. 7177 */ 7178 public ValuedItemComponent setPoints(BigDecimal value) { 7179 if (value == null) 7180 this.points = null; 7181 else { 7182 if (this.points == null) 7183 this.points = new DecimalType(); 7184 this.points.setValue(value); 7185 } 7186 return this; 7187 } 7188 7189 /** 7190 * @param value An amount that expresses the weighting (based on difficulty, 7191 * cost and/or resource intensiveness) associated with the Contract 7192 * Valued Item delivered. The concept of Points allows for 7193 * assignment of point values for a Contract Valued Item, such that 7194 * a monetary amount can be assigned to each point. 7195 */ 7196 public ValuedItemComponent setPoints(long value) { 7197 this.points = new DecimalType(); 7198 this.points.setValue(value); 7199 return this; 7200 } 7201 7202 /** 7203 * @param value An amount that expresses the weighting (based on difficulty, 7204 * cost and/or resource intensiveness) associated with the Contract 7205 * Valued Item delivered. The concept of Points allows for 7206 * assignment of point values for a Contract Valued Item, such that 7207 * a monetary amount can be assigned to each point. 7208 */ 7209 public ValuedItemComponent setPoints(double value) { 7210 this.points = new DecimalType(); 7211 this.points.setValue(value); 7212 return this; 7213 } 7214 7215 /** 7216 * @return {@link #net} (Expresses the product of the Contract Valued Item 7217 * unitQuantity and the unitPriceAmt. For example, the formula: unit 7218 * Quantity * unit Price (Cost per Point) * factor Number * points = net 7219 * Amount. Quantity, factor and points are assumed to be 1 if not 7220 * supplied.) 7221 */ 7222 public Money getNet() { 7223 if (this.net == null) 7224 if (Configuration.errorOnAutoCreate()) 7225 throw new Error("Attempt to auto-create ValuedItemComponent.net"); 7226 else if (Configuration.doAutoCreate()) 7227 this.net = new Money(); // cc 7228 return this.net; 7229 } 7230 7231 public boolean hasNet() { 7232 return this.net != null && !this.net.isEmpty(); 7233 } 7234 7235 /** 7236 * @param value {@link #net} (Expresses the product of the Contract Valued Item 7237 * unitQuantity and the unitPriceAmt. For example, the formula: 7238 * unit Quantity * unit Price (Cost per Point) * factor Number * 7239 * points = net Amount. Quantity, factor and points are assumed to 7240 * be 1 if not supplied.) 7241 */ 7242 public ValuedItemComponent setNet(Money value) { 7243 this.net = value; 7244 return this; 7245 } 7246 7247 /** 7248 * @return {@link #payment} (Terms of valuation.). This is the underlying object 7249 * with id, value and extensions. The accessor "getPayment" gives direct 7250 * access to the value 7251 */ 7252 public StringType getPaymentElement() { 7253 if (this.payment == null) 7254 if (Configuration.errorOnAutoCreate()) 7255 throw new Error("Attempt to auto-create ValuedItemComponent.payment"); 7256 else if (Configuration.doAutoCreate()) 7257 this.payment = new StringType(); // bb 7258 return this.payment; 7259 } 7260 7261 public boolean hasPaymentElement() { 7262 return this.payment != null && !this.payment.isEmpty(); 7263 } 7264 7265 public boolean hasPayment() { 7266 return this.payment != null && !this.payment.isEmpty(); 7267 } 7268 7269 /** 7270 * @param value {@link #payment} (Terms of valuation.). This is the underlying 7271 * object with id, value and extensions. The accessor "getPayment" 7272 * gives direct access to the value 7273 */ 7274 public ValuedItemComponent setPaymentElement(StringType value) { 7275 this.payment = value; 7276 return this; 7277 } 7278 7279 /** 7280 * @return Terms of valuation. 7281 */ 7282 public String getPayment() { 7283 return this.payment == null ? null : this.payment.getValue(); 7284 } 7285 7286 /** 7287 * @param value Terms of valuation. 7288 */ 7289 public ValuedItemComponent setPayment(String value) { 7290 if (Utilities.noString(value)) 7291 this.payment = null; 7292 else { 7293 if (this.payment == null) 7294 this.payment = new StringType(); 7295 this.payment.setValue(value); 7296 } 7297 return this; 7298 } 7299 7300 /** 7301 * @return {@link #paymentDate} (When payment is due.). This is the underlying 7302 * object with id, value and extensions. The accessor "getPaymentDate" 7303 * gives direct access to the value 7304 */ 7305 public DateTimeType getPaymentDateElement() { 7306 if (this.paymentDate == null) 7307 if (Configuration.errorOnAutoCreate()) 7308 throw new Error("Attempt to auto-create ValuedItemComponent.paymentDate"); 7309 else if (Configuration.doAutoCreate()) 7310 this.paymentDate = new DateTimeType(); // bb 7311 return this.paymentDate; 7312 } 7313 7314 public boolean hasPaymentDateElement() { 7315 return this.paymentDate != null && !this.paymentDate.isEmpty(); 7316 } 7317 7318 public boolean hasPaymentDate() { 7319 return this.paymentDate != null && !this.paymentDate.isEmpty(); 7320 } 7321 7322 /** 7323 * @param value {@link #paymentDate} (When payment is due.). This is the 7324 * underlying object with id, value and extensions. The accessor 7325 * "getPaymentDate" gives direct access to the value 7326 */ 7327 public ValuedItemComponent setPaymentDateElement(DateTimeType value) { 7328 this.paymentDate = value; 7329 return this; 7330 } 7331 7332 /** 7333 * @return When payment is due. 7334 */ 7335 public Date getPaymentDate() { 7336 return this.paymentDate == null ? null : this.paymentDate.getValue(); 7337 } 7338 7339 /** 7340 * @param value When payment is due. 7341 */ 7342 public ValuedItemComponent setPaymentDate(Date value) { 7343 if (value == null) 7344 this.paymentDate = null; 7345 else { 7346 if (this.paymentDate == null) 7347 this.paymentDate = new DateTimeType(); 7348 this.paymentDate.setValue(value); 7349 } 7350 return this; 7351 } 7352 7353 /** 7354 * @return {@link #responsible} (Who will make payment.) 7355 */ 7356 public Reference getResponsible() { 7357 if (this.responsible == null) 7358 if (Configuration.errorOnAutoCreate()) 7359 throw new Error("Attempt to auto-create ValuedItemComponent.responsible"); 7360 else if (Configuration.doAutoCreate()) 7361 this.responsible = new Reference(); // cc 7362 return this.responsible; 7363 } 7364 7365 public boolean hasResponsible() { 7366 return this.responsible != null && !this.responsible.isEmpty(); 7367 } 7368 7369 /** 7370 * @param value {@link #responsible} (Who will make payment.) 7371 */ 7372 public ValuedItemComponent setResponsible(Reference value) { 7373 this.responsible = value; 7374 return this; 7375 } 7376 7377 /** 7378 * @return {@link #responsible} The actual object that is the target of the 7379 * reference. The reference library doesn't populate this, but you can 7380 * use it to hold the resource if you resolve it. (Who will make 7381 * payment.) 7382 */ 7383 public Resource getResponsibleTarget() { 7384 return this.responsibleTarget; 7385 } 7386 7387 /** 7388 * @param value {@link #responsible} The actual object that is the target of the 7389 * reference. The reference library doesn't use these, but you can 7390 * use it to hold the resource if you resolve it. (Who will make 7391 * payment.) 7392 */ 7393 public ValuedItemComponent setResponsibleTarget(Resource value) { 7394 this.responsibleTarget = value; 7395 return this; 7396 } 7397 7398 /** 7399 * @return {@link #recipient} (Who will receive payment.) 7400 */ 7401 public Reference getRecipient() { 7402 if (this.recipient == null) 7403 if (Configuration.errorOnAutoCreate()) 7404 throw new Error("Attempt to auto-create ValuedItemComponent.recipient"); 7405 else if (Configuration.doAutoCreate()) 7406 this.recipient = new Reference(); // cc 7407 return this.recipient; 7408 } 7409 7410 public boolean hasRecipient() { 7411 return this.recipient != null && !this.recipient.isEmpty(); 7412 } 7413 7414 /** 7415 * @param value {@link #recipient} (Who will receive payment.) 7416 */ 7417 public ValuedItemComponent setRecipient(Reference value) { 7418 this.recipient = value; 7419 return this; 7420 } 7421 7422 /** 7423 * @return {@link #recipient} The actual object that is the target of the 7424 * reference. The reference library doesn't populate this, but you can 7425 * use it to hold the resource if you resolve it. (Who will receive 7426 * payment.) 7427 */ 7428 public Resource getRecipientTarget() { 7429 return this.recipientTarget; 7430 } 7431 7432 /** 7433 * @param value {@link #recipient} The actual object that is the target of the 7434 * reference. The reference library doesn't use these, but you can 7435 * use it to hold the resource if you resolve it. (Who will receive 7436 * payment.) 7437 */ 7438 public ValuedItemComponent setRecipientTarget(Resource value) { 7439 this.recipientTarget = value; 7440 return this; 7441 } 7442 7443 /** 7444 * @return {@link #linkId} (Id of the clause or question text related to the 7445 * context of this valuedItem in the referenced form or 7446 * QuestionnaireResponse.) 7447 */ 7448 public List<StringType> getLinkId() { 7449 if (this.linkId == null) 7450 this.linkId = new ArrayList<StringType>(); 7451 return this.linkId; 7452 } 7453 7454 /** 7455 * @return Returns a reference to <code>this</code> for easy method chaining 7456 */ 7457 public ValuedItemComponent setLinkId(List<StringType> theLinkId) { 7458 this.linkId = theLinkId; 7459 return this; 7460 } 7461 7462 public boolean hasLinkId() { 7463 if (this.linkId == null) 7464 return false; 7465 for (StringType item : this.linkId) 7466 if (!item.isEmpty()) 7467 return true; 7468 return false; 7469 } 7470 7471 /** 7472 * @return {@link #linkId} (Id of the clause or question text related to the 7473 * context of this valuedItem in the referenced form or 7474 * QuestionnaireResponse.) 7475 */ 7476 public StringType addLinkIdElement() {// 2 7477 StringType t = new StringType(); 7478 if (this.linkId == null) 7479 this.linkId = new ArrayList<StringType>(); 7480 this.linkId.add(t); 7481 return t; 7482 } 7483 7484 /** 7485 * @param value {@link #linkId} (Id of the clause or question text related to 7486 * the context of this valuedItem in the referenced form or 7487 * QuestionnaireResponse.) 7488 */ 7489 public ValuedItemComponent addLinkId(String value) { // 1 7490 StringType t = new StringType(); 7491 t.setValue(value); 7492 if (this.linkId == null) 7493 this.linkId = new ArrayList<StringType>(); 7494 this.linkId.add(t); 7495 return this; 7496 } 7497 7498 /** 7499 * @param value {@link #linkId} (Id of the clause or question text related to 7500 * the context of this valuedItem in the referenced form or 7501 * QuestionnaireResponse.) 7502 */ 7503 public boolean hasLinkId(String value) { 7504 if (this.linkId == null) 7505 return false; 7506 for (StringType v : this.linkId) 7507 if (v.getValue().equals(value)) // string 7508 return true; 7509 return false; 7510 } 7511 7512 /** 7513 * @return {@link #securityLabelNumber} (A set of security labels that define 7514 * which terms are controlled by this condition.) 7515 */ 7516 public List<UnsignedIntType> getSecurityLabelNumber() { 7517 if (this.securityLabelNumber == null) 7518 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7519 return this.securityLabelNumber; 7520 } 7521 7522 /** 7523 * @return Returns a reference to <code>this</code> for easy method chaining 7524 */ 7525 public ValuedItemComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 7526 this.securityLabelNumber = theSecurityLabelNumber; 7527 return this; 7528 } 7529 7530 public boolean hasSecurityLabelNumber() { 7531 if (this.securityLabelNumber == null) 7532 return false; 7533 for (UnsignedIntType item : this.securityLabelNumber) 7534 if (!item.isEmpty()) 7535 return true; 7536 return false; 7537 } 7538 7539 /** 7540 * @return {@link #securityLabelNumber} (A set of security labels that define 7541 * which terms are controlled by this condition.) 7542 */ 7543 public UnsignedIntType addSecurityLabelNumberElement() {// 2 7544 UnsignedIntType t = new UnsignedIntType(); 7545 if (this.securityLabelNumber == null) 7546 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7547 this.securityLabelNumber.add(t); 7548 return t; 7549 } 7550 7551 /** 7552 * @param value {@link #securityLabelNumber} (A set of security labels that 7553 * define which terms are controlled by this condition.) 7554 */ 7555 public ValuedItemComponent addSecurityLabelNumber(int value) { // 1 7556 UnsignedIntType t = new UnsignedIntType(); 7557 t.setValue(value); 7558 if (this.securityLabelNumber == null) 7559 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7560 this.securityLabelNumber.add(t); 7561 return this; 7562 } 7563 7564 /** 7565 * @param value {@link #securityLabelNumber} (A set of security labels that 7566 * define which terms are controlled by this condition.) 7567 */ 7568 public boolean hasSecurityLabelNumber(int value) { 7569 if (this.securityLabelNumber == null) 7570 return false; 7571 for (UnsignedIntType v : this.securityLabelNumber) 7572 if (v.getValue().equals(value)) // unsignedInt 7573 return true; 7574 return false; 7575 } 7576 7577 protected void listChildren(List<Property> children) { 7578 super.listChildren(children); 7579 children.add(new Property("entity[x]", "CodeableConcept|Reference(Any)", 7580 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity)); 7581 children.add( 7582 new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 0, 1, identifier)); 7583 children.add(new Property("effectiveTime", "dateTime", 7584 "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime)); 7585 children.add(new Property("quantity", "SimpleQuantity", 7586 "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 7587 0, 1, quantity)); 7588 children 7589 .add(new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 1, unitPrice)); 7590 children.add(new Property("factor", "decimal", 7591 "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7592 0, 1, factor)); 7593 children.add(new Property("points", "decimal", 7594 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 7595 0, 1, points)); 7596 children.add(new Property("net", "Money", 7597 "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 7598 0, 1, net)); 7599 children.add(new Property("payment", "string", "Terms of valuation.", 0, 1, payment)); 7600 children.add(new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate)); 7601 children.add( 7602 new Property("responsible", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 7603 "Who will make payment.", 0, 1, responsible)); 7604 children 7605 .add(new Property("recipient", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 7606 "Who will receive payment.", 0, 1, recipient)); 7607 children.add(new Property("linkId", "string", 7608 "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 7609 0, java.lang.Integer.MAX_VALUE, linkId)); 7610 children.add(new Property("securityLabelNumber", "unsignedInt", 7611 "A set of security labels that define which terms are controlled by this condition.", 0, 7612 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 7613 } 7614 7615 @Override 7616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7617 switch (_hash) { 7618 case -740568643: 7619 /* entity[x] */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7620 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7621 case -1298275357: 7622 /* entity */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7623 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7624 case 924197182: 7625 /* entityCodeableConcept */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7626 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7627 case -356635992: 7628 /* entityReference */ return new Property("entity[x]", "CodeableConcept|Reference(Any)", 7629 "Specific type of Contract Valued Item that may be priced.", 0, 1, entity); 7630 case -1618432855: 7631 /* identifier */ return new Property("identifier", "Identifier", "Identifies a Contract Valued Item instance.", 7632 0, 1, identifier); 7633 case -929905388: 7634 /* effectiveTime */ return new Property("effectiveTime", "dateTime", 7635 "Indicates the time during which this Contract ValuedItem information is effective.", 0, 1, effectiveTime); 7636 case -1285004149: 7637 /* quantity */ return new Property("quantity", "SimpleQuantity", 7638 "Specifies the units by which the Contract Valued Item is measured or counted, and quantifies the countable or measurable Contract Valued Item instances.", 7639 0, 1, quantity); 7640 case -486196699: 7641 /* unitPrice */ return new Property("unitPrice", "Money", "A Contract Valued Item unit valuation measure.", 0, 7642 1, unitPrice); 7643 case -1282148017: 7644 /* factor */ return new Property("factor", "decimal", 7645 "A real number that represents a multiplier used in determining the overall value of the Contract Valued Item delivered. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 7646 0, 1, factor); 7647 case -982754077: 7648 /* points */ return new Property("points", "decimal", 7649 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the Contract Valued Item delivered. The concept of Points allows for assignment of point values for a Contract Valued Item, such that a monetary amount can be assigned to each point.", 7650 0, 1, points); 7651 case 108957: 7652 /* net */ return new Property("net", "Money", 7653 "Expresses the product of the Contract Valued Item unitQuantity and the unitPriceAmt. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 7654 0, 1, net); 7655 case -786681338: 7656 /* payment */ return new Property("payment", "string", "Terms of valuation.", 0, 1, payment); 7657 case -1540873516: 7658 /* paymentDate */ return new Property("paymentDate", "dateTime", "When payment is due.", 0, 1, paymentDate); 7659 case 1847674614: 7660 /* responsible */ return new Property("responsible", 7661 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will make payment.", 0, 7662 1, responsible); 7663 case 820081177: 7664 /* recipient */ return new Property("recipient", 7665 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Who will receive payment.", 7666 0, 1, recipient); 7667 case -1102667083: 7668 /* linkId */ return new Property("linkId", "string", 7669 "Id of the clause or question text related to the context of this valuedItem in the referenced form or QuestionnaireResponse.", 7670 0, java.lang.Integer.MAX_VALUE, linkId); 7671 case -149460995: 7672 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 7673 "A set of security labels that define which terms are controlled by this condition.", 0, 7674 java.lang.Integer.MAX_VALUE, securityLabelNumber); 7675 default: 7676 return super.getNamedProperty(_hash, _name, _checkValid); 7677 } 7678 7679 } 7680 7681 @Override 7682 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7683 switch (hash) { 7684 case -1298275357: 7685 /* entity */ return this.entity == null ? new Base[0] : new Base[] { this.entity }; // Type 7686 case -1618432855: 7687 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 7688 case -929905388: 7689 /* effectiveTime */ return this.effectiveTime == null ? new Base[0] : new Base[] { this.effectiveTime }; // DateTimeType 7690 case -1285004149: 7691 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7692 case -486196699: 7693 /* unitPrice */ return this.unitPrice == null ? new Base[0] : new Base[] { this.unitPrice }; // Money 7694 case -1282148017: 7695 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 7696 case -982754077: 7697 /* points */ return this.points == null ? new Base[0] : new Base[] { this.points }; // DecimalType 7698 case 108957: 7699 /* net */ return this.net == null ? new Base[0] : new Base[] { this.net }; // Money 7700 case -786681338: 7701 /* payment */ return this.payment == null ? new Base[0] : new Base[] { this.payment }; // StringType 7702 case -1540873516: 7703 /* paymentDate */ return this.paymentDate == null ? new Base[0] : new Base[] { this.paymentDate }; // DateTimeType 7704 case 1847674614: 7705 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // Reference 7706 case 820081177: 7707 /* recipient */ return this.recipient == null ? new Base[0] : new Base[] { this.recipient }; // Reference 7708 case -1102667083: 7709 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 7710 case -149460995: 7711 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 7712 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 7713 default: 7714 return super.getProperty(hash, name, checkValid); 7715 } 7716 7717 } 7718 7719 @Override 7720 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7721 switch (hash) { 7722 case -1298275357: // entity 7723 this.entity = castToType(value); // Type 7724 return value; 7725 case -1618432855: // identifier 7726 this.identifier = castToIdentifier(value); // Identifier 7727 return value; 7728 case -929905388: // effectiveTime 7729 this.effectiveTime = castToDateTime(value); // DateTimeType 7730 return value; 7731 case -1285004149: // quantity 7732 this.quantity = castToQuantity(value); // Quantity 7733 return value; 7734 case -486196699: // unitPrice 7735 this.unitPrice = castToMoney(value); // Money 7736 return value; 7737 case -1282148017: // factor 7738 this.factor = castToDecimal(value); // DecimalType 7739 return value; 7740 case -982754077: // points 7741 this.points = castToDecimal(value); // DecimalType 7742 return value; 7743 case 108957: // net 7744 this.net = castToMoney(value); // Money 7745 return value; 7746 case -786681338: // payment 7747 this.payment = castToString(value); // StringType 7748 return value; 7749 case -1540873516: // paymentDate 7750 this.paymentDate = castToDateTime(value); // DateTimeType 7751 return value; 7752 case 1847674614: // responsible 7753 this.responsible = castToReference(value); // Reference 7754 return value; 7755 case 820081177: // recipient 7756 this.recipient = castToReference(value); // Reference 7757 return value; 7758 case -1102667083: // linkId 7759 this.getLinkId().add(castToString(value)); // StringType 7760 return value; 7761 case -149460995: // securityLabelNumber 7762 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 7763 return value; 7764 default: 7765 return super.setProperty(hash, name, value); 7766 } 7767 7768 } 7769 7770 @Override 7771 public Base setProperty(String name, Base value) throws FHIRException { 7772 if (name.equals("entity[x]")) { 7773 this.entity = castToType(value); // Type 7774 } else if (name.equals("identifier")) { 7775 this.identifier = castToIdentifier(value); // Identifier 7776 } else if (name.equals("effectiveTime")) { 7777 this.effectiveTime = castToDateTime(value); // DateTimeType 7778 } else if (name.equals("quantity")) { 7779 this.quantity = castToQuantity(value); // Quantity 7780 } else if (name.equals("unitPrice")) { 7781 this.unitPrice = castToMoney(value); // Money 7782 } else if (name.equals("factor")) { 7783 this.factor = castToDecimal(value); // DecimalType 7784 } else if (name.equals("points")) { 7785 this.points = castToDecimal(value); // DecimalType 7786 } else if (name.equals("net")) { 7787 this.net = castToMoney(value); // Money 7788 } else if (name.equals("payment")) { 7789 this.payment = castToString(value); // StringType 7790 } else if (name.equals("paymentDate")) { 7791 this.paymentDate = castToDateTime(value); // DateTimeType 7792 } else if (name.equals("responsible")) { 7793 this.responsible = castToReference(value); // Reference 7794 } else if (name.equals("recipient")) { 7795 this.recipient = castToReference(value); // Reference 7796 } else if (name.equals("linkId")) { 7797 this.getLinkId().add(castToString(value)); 7798 } else if (name.equals("securityLabelNumber")) { 7799 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 7800 } else 7801 return super.setProperty(name, value); 7802 return value; 7803 } 7804 7805 @Override 7806 public void removeChild(String name, Base value) throws FHIRException { 7807 if (name.equals("entity[x]")) { 7808 this.entity = null; 7809 } else if (name.equals("identifier")) { 7810 this.identifier = null; 7811 } else if (name.equals("effectiveTime")) { 7812 this.effectiveTime = null; 7813 } else if (name.equals("quantity")) { 7814 this.quantity = null; 7815 } else if (name.equals("unitPrice")) { 7816 this.unitPrice = null; 7817 } else if (name.equals("factor")) { 7818 this.factor = null; 7819 } else if (name.equals("points")) { 7820 this.points = null; 7821 } else if (name.equals("net")) { 7822 this.net = null; 7823 } else if (name.equals("payment")) { 7824 this.payment = null; 7825 } else if (name.equals("paymentDate")) { 7826 this.paymentDate = null; 7827 } else if (name.equals("responsible")) { 7828 this.responsible = null; 7829 } else if (name.equals("recipient")) { 7830 this.recipient = null; 7831 } else if (name.equals("linkId")) { 7832 this.getLinkId().remove(castToString(value)); 7833 } else if (name.equals("securityLabelNumber")) { 7834 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 7835 } else 7836 super.removeChild(name, value); 7837 7838 } 7839 7840 @Override 7841 public Base makeProperty(int hash, String name) throws FHIRException { 7842 switch (hash) { 7843 case -740568643: 7844 return getEntity(); 7845 case -1298275357: 7846 return getEntity(); 7847 case -1618432855: 7848 return getIdentifier(); 7849 case -929905388: 7850 return getEffectiveTimeElement(); 7851 case -1285004149: 7852 return getQuantity(); 7853 case -486196699: 7854 return getUnitPrice(); 7855 case -1282148017: 7856 return getFactorElement(); 7857 case -982754077: 7858 return getPointsElement(); 7859 case 108957: 7860 return getNet(); 7861 case -786681338: 7862 return getPaymentElement(); 7863 case -1540873516: 7864 return getPaymentDateElement(); 7865 case 1847674614: 7866 return getResponsible(); 7867 case 820081177: 7868 return getRecipient(); 7869 case -1102667083: 7870 return addLinkIdElement(); 7871 case -149460995: 7872 return addSecurityLabelNumberElement(); 7873 default: 7874 return super.makeProperty(hash, name); 7875 } 7876 7877 } 7878 7879 @Override 7880 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7881 switch (hash) { 7882 case -1298275357: 7883 /* entity */ return new String[] { "CodeableConcept", "Reference" }; 7884 case -1618432855: 7885 /* identifier */ return new String[] { "Identifier" }; 7886 case -929905388: 7887 /* effectiveTime */ return new String[] { "dateTime" }; 7888 case -1285004149: 7889 /* quantity */ return new String[] { "SimpleQuantity" }; 7890 case -486196699: 7891 /* unitPrice */ return new String[] { "Money" }; 7892 case -1282148017: 7893 /* factor */ return new String[] { "decimal" }; 7894 case -982754077: 7895 /* points */ return new String[] { "decimal" }; 7896 case 108957: 7897 /* net */ return new String[] { "Money" }; 7898 case -786681338: 7899 /* payment */ return new String[] { "string" }; 7900 case -1540873516: 7901 /* paymentDate */ return new String[] { "dateTime" }; 7902 case 1847674614: 7903 /* responsible */ return new String[] { "Reference" }; 7904 case 820081177: 7905 /* recipient */ return new String[] { "Reference" }; 7906 case -1102667083: 7907 /* linkId */ return new String[] { "string" }; 7908 case -149460995: 7909 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 7910 default: 7911 return super.getTypesForProperty(hash, name); 7912 } 7913 7914 } 7915 7916 @Override 7917 public Base addChild(String name) throws FHIRException { 7918 if (name.equals("entityCodeableConcept")) { 7919 this.entity = new CodeableConcept(); 7920 return this.entity; 7921 } else if (name.equals("entityReference")) { 7922 this.entity = new Reference(); 7923 return this.entity; 7924 } else if (name.equals("identifier")) { 7925 this.identifier = new Identifier(); 7926 return this.identifier; 7927 } else if (name.equals("effectiveTime")) { 7928 throw new FHIRException("Cannot call addChild on a singleton property Contract.effectiveTime"); 7929 } else if (name.equals("quantity")) { 7930 this.quantity = new Quantity(); 7931 return this.quantity; 7932 } else if (name.equals("unitPrice")) { 7933 this.unitPrice = new Money(); 7934 return this.unitPrice; 7935 } else if (name.equals("factor")) { 7936 throw new FHIRException("Cannot call addChild on a singleton property Contract.factor"); 7937 } else if (name.equals("points")) { 7938 throw new FHIRException("Cannot call addChild on a singleton property Contract.points"); 7939 } else if (name.equals("net")) { 7940 this.net = new Money(); 7941 return this.net; 7942 } else if (name.equals("payment")) { 7943 throw new FHIRException("Cannot call addChild on a singleton property Contract.payment"); 7944 } else if (name.equals("paymentDate")) { 7945 throw new FHIRException("Cannot call addChild on a singleton property Contract.paymentDate"); 7946 } else if (name.equals("responsible")) { 7947 this.responsible = new Reference(); 7948 return this.responsible; 7949 } else if (name.equals("recipient")) { 7950 this.recipient = new Reference(); 7951 return this.recipient; 7952 } else if (name.equals("linkId")) { 7953 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 7954 } else if (name.equals("securityLabelNumber")) { 7955 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 7956 } else 7957 return super.addChild(name); 7958 } 7959 7960 public ValuedItemComponent copy() { 7961 ValuedItemComponent dst = new ValuedItemComponent(); 7962 copyValues(dst); 7963 return dst; 7964 } 7965 7966 public void copyValues(ValuedItemComponent dst) { 7967 super.copyValues(dst); 7968 dst.entity = entity == null ? null : entity.copy(); 7969 dst.identifier = identifier == null ? null : identifier.copy(); 7970 dst.effectiveTime = effectiveTime == null ? null : effectiveTime.copy(); 7971 dst.quantity = quantity == null ? null : quantity.copy(); 7972 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7973 dst.factor = factor == null ? null : factor.copy(); 7974 dst.points = points == null ? null : points.copy(); 7975 dst.net = net == null ? null : net.copy(); 7976 dst.payment = payment == null ? null : payment.copy(); 7977 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 7978 dst.responsible = responsible == null ? null : responsible.copy(); 7979 dst.recipient = recipient == null ? null : recipient.copy(); 7980 if (linkId != null) { 7981 dst.linkId = new ArrayList<StringType>(); 7982 for (StringType i : linkId) 7983 dst.linkId.add(i.copy()); 7984 } 7985 ; 7986 if (securityLabelNumber != null) { 7987 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 7988 for (UnsignedIntType i : securityLabelNumber) 7989 dst.securityLabelNumber.add(i.copy()); 7990 } 7991 ; 7992 } 7993 7994 @Override 7995 public boolean equalsDeep(Base other_) { 7996 if (!super.equalsDeep(other_)) 7997 return false; 7998 if (!(other_ instanceof ValuedItemComponent)) 7999 return false; 8000 ValuedItemComponent o = (ValuedItemComponent) other_; 8001 return compareDeep(entity, o.entity, true) && compareDeep(identifier, o.identifier, true) 8002 && compareDeep(effectiveTime, o.effectiveTime, true) && compareDeep(quantity, o.quantity, true) 8003 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 8004 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) 8005 && compareDeep(payment, o.payment, true) && compareDeep(paymentDate, o.paymentDate, true) 8006 && compareDeep(responsible, o.responsible, true) && compareDeep(recipient, o.recipient, true) 8007 && compareDeep(linkId, o.linkId, true) && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 8008 } 8009 8010 @Override 8011 public boolean equalsShallow(Base other_) { 8012 if (!super.equalsShallow(other_)) 8013 return false; 8014 if (!(other_ instanceof ValuedItemComponent)) 8015 return false; 8016 ValuedItemComponent o = (ValuedItemComponent) other_; 8017 return compareValues(effectiveTime, o.effectiveTime, true) && compareValues(factor, o.factor, true) 8018 && compareValues(points, o.points, true) && compareValues(payment, o.payment, true) 8019 && compareValues(paymentDate, o.paymentDate, true) && compareValues(linkId, o.linkId, true) 8020 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 8021 } 8022 8023 public boolean isEmpty() { 8024 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, identifier, effectiveTime, quantity, 8025 unitPrice, factor, points, net, payment, paymentDate, responsible, recipient, linkId, securityLabelNumber); 8026 } 8027 8028 public String fhirType() { 8029 return "Contract.term.asset.valuedItem"; 8030 8031 } 8032 8033 } 8034 8035 @Block() 8036 public static class ActionComponent extends BackboneElement implements IBaseBackboneElement { 8037 /** 8038 * True if the term prohibits the action. 8039 */ 8040 @Child(name = "doNotPerform", type = { 8041 BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = false) 8042 @Description(shortDefinition = "True if the term prohibits the action", formalDefinition = "True if the term prohibits the action.") 8043 protected BooleanType doNotPerform; 8044 8045 /** 8046 * Activity or service obligation to be done or not done, performed or not 8047 * performed, effectuated or not by this Contract term. 8048 */ 8049 @Child(name = "type", type = { 8050 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 8051 @Description(shortDefinition = "Type or form of the action", formalDefinition = "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.") 8052 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-action") 8053 protected CodeableConcept type; 8054 8055 /** 8056 * Entity of the action. 8057 */ 8058 @Child(name = "subject", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8059 @Description(shortDefinition = "Entity of the action", formalDefinition = "Entity of the action.") 8060 protected List<ActionSubjectComponent> subject; 8061 8062 /** 8063 * Reason or purpose for the action stipulated by this Contract Provision. 8064 */ 8065 @Child(name = "intent", type = { 8066 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 8067 @Description(shortDefinition = "Purpose for the Contract Term Action", formalDefinition = "Reason or purpose for the action stipulated by this Contract Provision.") 8068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 8069 protected CodeableConcept intent; 8070 8071 /** 8072 * Id [identifier??] of the clause or question text related to this action in 8073 * the referenced form or QuestionnaireResponse. 8074 */ 8075 @Child(name = "linkId", type = { 8076 StringType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8077 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.") 8078 protected List<StringType> linkId; 8079 8080 /** 8081 * Current state of the term action. 8082 */ 8083 @Child(name = "status", type = { 8084 CodeableConcept.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 8085 @Description(shortDefinition = "State of the action", formalDefinition = "Current state of the term action.") 8086 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-actionstatus") 8087 protected CodeableConcept status; 8088 8089 /** 8090 * Encounter or Episode with primary association to specified term activity. 8091 */ 8092 @Child(name = "context", type = { Encounter.class, 8093 EpisodeOfCare.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 8094 @Description(shortDefinition = "Episode associated with action", formalDefinition = "Encounter or Episode with primary association to specified term activity.") 8095 protected Reference context; 8096 8097 /** 8098 * The actual object that is the target of the reference (Encounter or Episode 8099 * with primary association to specified term activity.) 8100 */ 8101 protected Resource contextTarget; 8102 8103 /** 8104 * Id [identifier??] of the clause or question text related to the requester of 8105 * this action in the referenced form or QuestionnaireResponse. 8106 */ 8107 @Child(name = "contextLinkId", type = { 8108 StringType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8109 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.") 8110 protected List<StringType> contextLinkId; 8111 8112 /** 8113 * When action happens. 8114 */ 8115 @Child(name = "occurrence", type = { DateTimeType.class, Period.class, 8116 Timing.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 8117 @Description(shortDefinition = "When action happens", formalDefinition = "When action happens.") 8118 protected Type occurrence; 8119 8120 /** 8121 * Who or what initiated the action and has responsibility for its activation. 8122 */ 8123 @Child(name = "requester", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 8124 Device.class, Group.class, 8125 Organization.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8126 @Description(shortDefinition = "Who asked for action", formalDefinition = "Who or what initiated the action and has responsibility for its activation.") 8127 protected List<Reference> requester; 8128 /** 8129 * The actual objects that are the target of the reference (Who or what 8130 * initiated the action and has responsibility for its activation.) 8131 */ 8132 protected List<Resource> requesterTarget; 8133 8134 /** 8135 * Id [identifier??] of the clause or question text related to the requester of 8136 * this action in the referenced form or QuestionnaireResponse. 8137 */ 8138 @Child(name = "requesterLinkId", type = { 8139 StringType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8140 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.") 8141 protected List<StringType> requesterLinkId; 8142 8143 /** 8144 * The type of individual that is desired or required to perform or not perform 8145 * the action. 8146 */ 8147 @Child(name = "performerType", type = { 8148 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8149 @Description(shortDefinition = "Kind of service performer", formalDefinition = "The type of individual that is desired or required to perform or not perform the action.") 8150 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-agent-type") 8151 protected List<CodeableConcept> performerType; 8152 8153 /** 8154 * The type of role or competency of an individual desired or required to 8155 * perform or not perform the action. 8156 */ 8157 @Child(name = "performerRole", type = { 8158 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 8159 @Description(shortDefinition = "Competency of the performer", formalDefinition = "The type of role or competency of an individual desired or required to perform or not perform the action.") 8160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/provenance-agent-role") 8161 protected CodeableConcept performerRole; 8162 8163 /** 8164 * Indicates who or what is being asked to perform (or not perform) the ction. 8165 */ 8166 @Child(name = "performer", type = { RelatedPerson.class, Patient.class, Practitioner.class, PractitionerRole.class, 8167 CareTeam.class, Device.class, Substance.class, Organization.class, 8168 Location.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 8169 @Description(shortDefinition = "Actor that wil execute (or not) the action", formalDefinition = "Indicates who or what is being asked to perform (or not perform) the ction.") 8170 protected Reference performer; 8171 8172 /** 8173 * The actual object that is the target of the reference (Indicates who or what 8174 * is being asked to perform (or not perform) the ction.) 8175 */ 8176 protected Resource performerTarget; 8177 8178 /** 8179 * Id [identifier??] of the clause or question text related to the reason type 8180 * or reference of this action in the referenced form or QuestionnaireResponse. 8181 */ 8182 @Child(name = "performerLinkId", type = { 8183 StringType.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8184 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.") 8185 protected List<StringType> performerLinkId; 8186 8187 /** 8188 * Rationale for the action to be performed or not performed. Describes why the 8189 * action is permitted or prohibited. 8190 */ 8191 @Child(name = "reasonCode", type = { 8192 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8193 @Description(shortDefinition = "Why is action (not) needed?", formalDefinition = "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.") 8194 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-PurposeOfUse") 8195 protected List<CodeableConcept> reasonCode; 8196 8197 /** 8198 * Indicates another resource whose existence justifies permitting or not 8199 * permitting this action. 8200 */ 8201 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 8202 DocumentReference.class, Questionnaire.class, 8203 QuestionnaireResponse.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8204 @Description(shortDefinition = "Why is action (not) needed?", formalDefinition = "Indicates another resource whose existence justifies permitting or not permitting this action.") 8205 protected List<Reference> reasonReference; 8206 /** 8207 * The actual objects that are the target of the reference (Indicates another 8208 * resource whose existence justifies permitting or not permitting this action.) 8209 */ 8210 protected List<Resource> reasonReferenceTarget; 8211 8212 /** 8213 * Describes why the action is to be performed or not performed in textual form. 8214 */ 8215 @Child(name = "reason", type = { 8216 StringType.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8217 @Description(shortDefinition = "Why action is to be performed", formalDefinition = "Describes why the action is to be performed or not performed in textual form.") 8218 protected List<StringType> reason; 8219 8220 /** 8221 * Id [identifier??] of the clause or question text related to the reason type 8222 * or reference of this action in the referenced form or QuestionnaireResponse. 8223 */ 8224 @Child(name = "reasonLinkId", type = { 8225 StringType.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8226 @Description(shortDefinition = "Pointer to specific item", formalDefinition = "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.") 8227 protected List<StringType> reasonLinkId; 8228 8229 /** 8230 * Comments made about the term action made by the requester, performer, subject 8231 * or other participants. 8232 */ 8233 @Child(name = "note", type = { 8234 Annotation.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8235 @Description(shortDefinition = "Comments about the action", formalDefinition = "Comments made about the term action made by the requester, performer, subject or other participants.") 8236 protected List<Annotation> note; 8237 8238 /** 8239 * Security labels that protects the action. 8240 */ 8241 @Child(name = "securityLabelNumber", type = { 8242 UnsignedIntType.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 8243 @Description(shortDefinition = "Action restriction numbers", formalDefinition = "Security labels that protects the action.") 8244 protected List<UnsignedIntType> securityLabelNumber; 8245 8246 private static final long serialVersionUID = -178728180L; 8247 8248 /** 8249 * Constructor 8250 */ 8251 public ActionComponent() { 8252 super(); 8253 } 8254 8255 /** 8256 * Constructor 8257 */ 8258 public ActionComponent(CodeableConcept type, CodeableConcept intent, CodeableConcept status) { 8259 super(); 8260 this.type = type; 8261 this.intent = intent; 8262 this.status = status; 8263 } 8264 8265 /** 8266 * @return {@link #doNotPerform} (True if the term prohibits the action.). This 8267 * is the underlying object with id, value and extensions. The accessor 8268 * "getDoNotPerform" gives direct access to the value 8269 */ 8270 public BooleanType getDoNotPerformElement() { 8271 if (this.doNotPerform == null) 8272 if (Configuration.errorOnAutoCreate()) 8273 throw new Error("Attempt to auto-create ActionComponent.doNotPerform"); 8274 else if (Configuration.doAutoCreate()) 8275 this.doNotPerform = new BooleanType(); // bb 8276 return this.doNotPerform; 8277 } 8278 8279 public boolean hasDoNotPerformElement() { 8280 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 8281 } 8282 8283 public boolean hasDoNotPerform() { 8284 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 8285 } 8286 8287 /** 8288 * @param value {@link #doNotPerform} (True if the term prohibits the action.). 8289 * This is the underlying object with id, value and extensions. The 8290 * accessor "getDoNotPerform" gives direct access to the value 8291 */ 8292 public ActionComponent setDoNotPerformElement(BooleanType value) { 8293 this.doNotPerform = value; 8294 return this; 8295 } 8296 8297 /** 8298 * @return True if the term prohibits the action. 8299 */ 8300 public boolean getDoNotPerform() { 8301 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 8302 } 8303 8304 /** 8305 * @param value True if the term prohibits the action. 8306 */ 8307 public ActionComponent setDoNotPerform(boolean value) { 8308 if (this.doNotPerform == null) 8309 this.doNotPerform = new BooleanType(); 8310 this.doNotPerform.setValue(value); 8311 return this; 8312 } 8313 8314 /** 8315 * @return {@link #type} (Activity or service obligation to be done or not done, 8316 * performed or not performed, effectuated or not by this Contract 8317 * term.) 8318 */ 8319 public CodeableConcept getType() { 8320 if (this.type == null) 8321 if (Configuration.errorOnAutoCreate()) 8322 throw new Error("Attempt to auto-create ActionComponent.type"); 8323 else if (Configuration.doAutoCreate()) 8324 this.type = new CodeableConcept(); // cc 8325 return this.type; 8326 } 8327 8328 public boolean hasType() { 8329 return this.type != null && !this.type.isEmpty(); 8330 } 8331 8332 /** 8333 * @param value {@link #type} (Activity or service obligation to be done or not 8334 * done, performed or not performed, effectuated or not by this 8335 * Contract term.) 8336 */ 8337 public ActionComponent setType(CodeableConcept value) { 8338 this.type = value; 8339 return this; 8340 } 8341 8342 /** 8343 * @return {@link #subject} (Entity of the action.) 8344 */ 8345 public List<ActionSubjectComponent> getSubject() { 8346 if (this.subject == null) 8347 this.subject = new ArrayList<ActionSubjectComponent>(); 8348 return this.subject; 8349 } 8350 8351 /** 8352 * @return Returns a reference to <code>this</code> for easy method chaining 8353 */ 8354 public ActionComponent setSubject(List<ActionSubjectComponent> theSubject) { 8355 this.subject = theSubject; 8356 return this; 8357 } 8358 8359 public boolean hasSubject() { 8360 if (this.subject == null) 8361 return false; 8362 for (ActionSubjectComponent item : this.subject) 8363 if (!item.isEmpty()) 8364 return true; 8365 return false; 8366 } 8367 8368 public ActionSubjectComponent addSubject() { // 3 8369 ActionSubjectComponent t = new ActionSubjectComponent(); 8370 if (this.subject == null) 8371 this.subject = new ArrayList<ActionSubjectComponent>(); 8372 this.subject.add(t); 8373 return t; 8374 } 8375 8376 public ActionComponent addSubject(ActionSubjectComponent t) { // 3 8377 if (t == null) 8378 return this; 8379 if (this.subject == null) 8380 this.subject = new ArrayList<ActionSubjectComponent>(); 8381 this.subject.add(t); 8382 return this; 8383 } 8384 8385 /** 8386 * @return The first repetition of repeating field {@link #subject}, creating it 8387 * if it does not already exist 8388 */ 8389 public ActionSubjectComponent getSubjectFirstRep() { 8390 if (getSubject().isEmpty()) { 8391 addSubject(); 8392 } 8393 return getSubject().get(0); 8394 } 8395 8396 /** 8397 * @return {@link #intent} (Reason or purpose for the action stipulated by this 8398 * Contract Provision.) 8399 */ 8400 public CodeableConcept getIntent() { 8401 if (this.intent == null) 8402 if (Configuration.errorOnAutoCreate()) 8403 throw new Error("Attempt to auto-create ActionComponent.intent"); 8404 else if (Configuration.doAutoCreate()) 8405 this.intent = new CodeableConcept(); // cc 8406 return this.intent; 8407 } 8408 8409 public boolean hasIntent() { 8410 return this.intent != null && !this.intent.isEmpty(); 8411 } 8412 8413 /** 8414 * @param value {@link #intent} (Reason or purpose for the action stipulated by 8415 * this Contract Provision.) 8416 */ 8417 public ActionComponent setIntent(CodeableConcept value) { 8418 this.intent = value; 8419 return this; 8420 } 8421 8422 /** 8423 * @return {@link #linkId} (Id [identifier??] of the clause or question text 8424 * related to this action in the referenced form or 8425 * QuestionnaireResponse.) 8426 */ 8427 public List<StringType> getLinkId() { 8428 if (this.linkId == null) 8429 this.linkId = new ArrayList<StringType>(); 8430 return this.linkId; 8431 } 8432 8433 /** 8434 * @return Returns a reference to <code>this</code> for easy method chaining 8435 */ 8436 public ActionComponent setLinkId(List<StringType> theLinkId) { 8437 this.linkId = theLinkId; 8438 return this; 8439 } 8440 8441 public boolean hasLinkId() { 8442 if (this.linkId == null) 8443 return false; 8444 for (StringType item : this.linkId) 8445 if (!item.isEmpty()) 8446 return true; 8447 return false; 8448 } 8449 8450 /** 8451 * @return {@link #linkId} (Id [identifier??] of the clause or question text 8452 * related to this action in the referenced form or 8453 * QuestionnaireResponse.) 8454 */ 8455 public StringType addLinkIdElement() {// 2 8456 StringType t = new StringType(); 8457 if (this.linkId == null) 8458 this.linkId = new ArrayList<StringType>(); 8459 this.linkId.add(t); 8460 return t; 8461 } 8462 8463 /** 8464 * @param value {@link #linkId} (Id [identifier??] of the clause or question 8465 * text related to this action in the referenced form or 8466 * QuestionnaireResponse.) 8467 */ 8468 public ActionComponent addLinkId(String value) { // 1 8469 StringType t = new StringType(); 8470 t.setValue(value); 8471 if (this.linkId == null) 8472 this.linkId = new ArrayList<StringType>(); 8473 this.linkId.add(t); 8474 return this; 8475 } 8476 8477 /** 8478 * @param value {@link #linkId} (Id [identifier??] of the clause or question 8479 * text related to this action in the referenced form or 8480 * QuestionnaireResponse.) 8481 */ 8482 public boolean hasLinkId(String value) { 8483 if (this.linkId == null) 8484 return false; 8485 for (StringType v : this.linkId) 8486 if (v.getValue().equals(value)) // string 8487 return true; 8488 return false; 8489 } 8490 8491 /** 8492 * @return {@link #status} (Current state of the term action.) 8493 */ 8494 public CodeableConcept getStatus() { 8495 if (this.status == null) 8496 if (Configuration.errorOnAutoCreate()) 8497 throw new Error("Attempt to auto-create ActionComponent.status"); 8498 else if (Configuration.doAutoCreate()) 8499 this.status = new CodeableConcept(); // cc 8500 return this.status; 8501 } 8502 8503 public boolean hasStatus() { 8504 return this.status != null && !this.status.isEmpty(); 8505 } 8506 8507 /** 8508 * @param value {@link #status} (Current state of the term action.) 8509 */ 8510 public ActionComponent setStatus(CodeableConcept value) { 8511 this.status = value; 8512 return this; 8513 } 8514 8515 /** 8516 * @return {@link #context} (Encounter or Episode with primary association to 8517 * specified term activity.) 8518 */ 8519 public Reference getContext() { 8520 if (this.context == null) 8521 if (Configuration.errorOnAutoCreate()) 8522 throw new Error("Attempt to auto-create ActionComponent.context"); 8523 else if (Configuration.doAutoCreate()) 8524 this.context = new Reference(); // cc 8525 return this.context; 8526 } 8527 8528 public boolean hasContext() { 8529 return this.context != null && !this.context.isEmpty(); 8530 } 8531 8532 /** 8533 * @param value {@link #context} (Encounter or Episode with primary association 8534 * to specified term activity.) 8535 */ 8536 public ActionComponent setContext(Reference value) { 8537 this.context = value; 8538 return this; 8539 } 8540 8541 /** 8542 * @return {@link #context} The actual object that is the target of the 8543 * reference. The reference library doesn't populate this, but you can 8544 * use it to hold the resource if you resolve it. (Encounter or Episode 8545 * with primary association to specified term activity.) 8546 */ 8547 public Resource getContextTarget() { 8548 return this.contextTarget; 8549 } 8550 8551 /** 8552 * @param value {@link #context} The actual object that is the target of the 8553 * reference. The reference library doesn't use these, but you can 8554 * use it to hold the resource if you resolve it. (Encounter or 8555 * Episode with primary association to specified term activity.) 8556 */ 8557 public ActionComponent setContextTarget(Resource value) { 8558 this.contextTarget = value; 8559 return this; 8560 } 8561 8562 /** 8563 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question 8564 * text related to the requester of this action in the referenced form 8565 * or QuestionnaireResponse.) 8566 */ 8567 public List<StringType> getContextLinkId() { 8568 if (this.contextLinkId == null) 8569 this.contextLinkId = new ArrayList<StringType>(); 8570 return this.contextLinkId; 8571 } 8572 8573 /** 8574 * @return Returns a reference to <code>this</code> for easy method chaining 8575 */ 8576 public ActionComponent setContextLinkId(List<StringType> theContextLinkId) { 8577 this.contextLinkId = theContextLinkId; 8578 return this; 8579 } 8580 8581 public boolean hasContextLinkId() { 8582 if (this.contextLinkId == null) 8583 return false; 8584 for (StringType item : this.contextLinkId) 8585 if (!item.isEmpty()) 8586 return true; 8587 return false; 8588 } 8589 8590 /** 8591 * @return {@link #contextLinkId} (Id [identifier??] of the clause or question 8592 * text related to the requester of this action in the referenced form 8593 * or QuestionnaireResponse.) 8594 */ 8595 public StringType addContextLinkIdElement() {// 2 8596 StringType t = new StringType(); 8597 if (this.contextLinkId == null) 8598 this.contextLinkId = new ArrayList<StringType>(); 8599 this.contextLinkId.add(t); 8600 return t; 8601 } 8602 8603 /** 8604 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or 8605 * question text related to the requester of this action in the 8606 * referenced form or QuestionnaireResponse.) 8607 */ 8608 public ActionComponent addContextLinkId(String value) { // 1 8609 StringType t = new StringType(); 8610 t.setValue(value); 8611 if (this.contextLinkId == null) 8612 this.contextLinkId = new ArrayList<StringType>(); 8613 this.contextLinkId.add(t); 8614 return this; 8615 } 8616 8617 /** 8618 * @param value {@link #contextLinkId} (Id [identifier??] of the clause or 8619 * question text related to the requester of this action in the 8620 * referenced form or QuestionnaireResponse.) 8621 */ 8622 public boolean hasContextLinkId(String value) { 8623 if (this.contextLinkId == null) 8624 return false; 8625 for (StringType v : this.contextLinkId) 8626 if (v.getValue().equals(value)) // string 8627 return true; 8628 return false; 8629 } 8630 8631 /** 8632 * @return {@link #occurrence} (When action happens.) 8633 */ 8634 public Type getOccurrence() { 8635 return this.occurrence; 8636 } 8637 8638 /** 8639 * @return {@link #occurrence} (When action happens.) 8640 */ 8641 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 8642 if (this.occurrence == null) 8643 this.occurrence = new DateTimeType(); 8644 if (!(this.occurrence instanceof DateTimeType)) 8645 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 8646 + this.occurrence.getClass().getName() + " was encountered"); 8647 return (DateTimeType) this.occurrence; 8648 } 8649 8650 public boolean hasOccurrenceDateTimeType() { 8651 return this.occurrence instanceof DateTimeType; 8652 } 8653 8654 /** 8655 * @return {@link #occurrence} (When action happens.) 8656 */ 8657 public Period getOccurrencePeriod() throws FHIRException { 8658 if (this.occurrence == null) 8659 this.occurrence = new Period(); 8660 if (!(this.occurrence instanceof Period)) 8661 throw new FHIRException("Type mismatch: the type Period was expected, but " 8662 + this.occurrence.getClass().getName() + " was encountered"); 8663 return (Period) this.occurrence; 8664 } 8665 8666 public boolean hasOccurrencePeriod() { 8667 return this.occurrence instanceof Period; 8668 } 8669 8670 /** 8671 * @return {@link #occurrence} (When action happens.) 8672 */ 8673 public Timing getOccurrenceTiming() throws FHIRException { 8674 if (this.occurrence == null) 8675 this.occurrence = new Timing(); 8676 if (!(this.occurrence instanceof Timing)) 8677 throw new FHIRException("Type mismatch: the type Timing was expected, but " 8678 + this.occurrence.getClass().getName() + " was encountered"); 8679 return (Timing) this.occurrence; 8680 } 8681 8682 public boolean hasOccurrenceTiming() { 8683 return this.occurrence instanceof Timing; 8684 } 8685 8686 public boolean hasOccurrence() { 8687 return this.occurrence != null && !this.occurrence.isEmpty(); 8688 } 8689 8690 /** 8691 * @param value {@link #occurrence} (When action happens.) 8692 */ 8693 public ActionComponent setOccurrence(Type value) { 8694 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 8695 throw new Error("Not the right type for Contract.term.action.occurrence[x]: " + value.fhirType()); 8696 this.occurrence = value; 8697 return this; 8698 } 8699 8700 /** 8701 * @return {@link #requester} (Who or what initiated the action and has 8702 * responsibility for its activation.) 8703 */ 8704 public List<Reference> getRequester() { 8705 if (this.requester == null) 8706 this.requester = new ArrayList<Reference>(); 8707 return this.requester; 8708 } 8709 8710 /** 8711 * @return Returns a reference to <code>this</code> for easy method chaining 8712 */ 8713 public ActionComponent setRequester(List<Reference> theRequester) { 8714 this.requester = theRequester; 8715 return this; 8716 } 8717 8718 public boolean hasRequester() { 8719 if (this.requester == null) 8720 return false; 8721 for (Reference item : this.requester) 8722 if (!item.isEmpty()) 8723 return true; 8724 return false; 8725 } 8726 8727 public Reference addRequester() { // 3 8728 Reference t = new Reference(); 8729 if (this.requester == null) 8730 this.requester = new ArrayList<Reference>(); 8731 this.requester.add(t); 8732 return t; 8733 } 8734 8735 public ActionComponent addRequester(Reference t) { // 3 8736 if (t == null) 8737 return this; 8738 if (this.requester == null) 8739 this.requester = new ArrayList<Reference>(); 8740 this.requester.add(t); 8741 return this; 8742 } 8743 8744 /** 8745 * @return The first repetition of repeating field {@link #requester}, creating 8746 * it if it does not already exist 8747 */ 8748 public Reference getRequesterFirstRep() { 8749 if (getRequester().isEmpty()) { 8750 addRequester(); 8751 } 8752 return getRequester().get(0); 8753 } 8754 8755 /** 8756 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question 8757 * text related to the requester of this action in the referenced form 8758 * or QuestionnaireResponse.) 8759 */ 8760 public List<StringType> getRequesterLinkId() { 8761 if (this.requesterLinkId == null) 8762 this.requesterLinkId = new ArrayList<StringType>(); 8763 return this.requesterLinkId; 8764 } 8765 8766 /** 8767 * @return Returns a reference to <code>this</code> for easy method chaining 8768 */ 8769 public ActionComponent setRequesterLinkId(List<StringType> theRequesterLinkId) { 8770 this.requesterLinkId = theRequesterLinkId; 8771 return this; 8772 } 8773 8774 public boolean hasRequesterLinkId() { 8775 if (this.requesterLinkId == null) 8776 return false; 8777 for (StringType item : this.requesterLinkId) 8778 if (!item.isEmpty()) 8779 return true; 8780 return false; 8781 } 8782 8783 /** 8784 * @return {@link #requesterLinkId} (Id [identifier??] of the clause or question 8785 * text related to the requester of this action in the referenced form 8786 * or QuestionnaireResponse.) 8787 */ 8788 public StringType addRequesterLinkIdElement() {// 2 8789 StringType t = new StringType(); 8790 if (this.requesterLinkId == null) 8791 this.requesterLinkId = new ArrayList<StringType>(); 8792 this.requesterLinkId.add(t); 8793 return t; 8794 } 8795 8796 /** 8797 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or 8798 * question text related to the requester of this action in the 8799 * referenced form or QuestionnaireResponse.) 8800 */ 8801 public ActionComponent addRequesterLinkId(String value) { // 1 8802 StringType t = new StringType(); 8803 t.setValue(value); 8804 if (this.requesterLinkId == null) 8805 this.requesterLinkId = new ArrayList<StringType>(); 8806 this.requesterLinkId.add(t); 8807 return this; 8808 } 8809 8810 /** 8811 * @param value {@link #requesterLinkId} (Id [identifier??] of the clause or 8812 * question text related to the requester of this action in the 8813 * referenced form or QuestionnaireResponse.) 8814 */ 8815 public boolean hasRequesterLinkId(String value) { 8816 if (this.requesterLinkId == null) 8817 return false; 8818 for (StringType v : this.requesterLinkId) 8819 if (v.getValue().equals(value)) // string 8820 return true; 8821 return false; 8822 } 8823 8824 /** 8825 * @return {@link #performerType} (The type of individual that is desired or 8826 * required to perform or not perform the action.) 8827 */ 8828 public List<CodeableConcept> getPerformerType() { 8829 if (this.performerType == null) 8830 this.performerType = new ArrayList<CodeableConcept>(); 8831 return this.performerType; 8832 } 8833 8834 /** 8835 * @return Returns a reference to <code>this</code> for easy method chaining 8836 */ 8837 public ActionComponent setPerformerType(List<CodeableConcept> thePerformerType) { 8838 this.performerType = thePerformerType; 8839 return this; 8840 } 8841 8842 public boolean hasPerformerType() { 8843 if (this.performerType == null) 8844 return false; 8845 for (CodeableConcept item : this.performerType) 8846 if (!item.isEmpty()) 8847 return true; 8848 return false; 8849 } 8850 8851 public CodeableConcept addPerformerType() { // 3 8852 CodeableConcept t = new CodeableConcept(); 8853 if (this.performerType == null) 8854 this.performerType = new ArrayList<CodeableConcept>(); 8855 this.performerType.add(t); 8856 return t; 8857 } 8858 8859 public ActionComponent addPerformerType(CodeableConcept t) { // 3 8860 if (t == null) 8861 return this; 8862 if (this.performerType == null) 8863 this.performerType = new ArrayList<CodeableConcept>(); 8864 this.performerType.add(t); 8865 return this; 8866 } 8867 8868 /** 8869 * @return The first repetition of repeating field {@link #performerType}, 8870 * creating it if it does not already exist 8871 */ 8872 public CodeableConcept getPerformerTypeFirstRep() { 8873 if (getPerformerType().isEmpty()) { 8874 addPerformerType(); 8875 } 8876 return getPerformerType().get(0); 8877 } 8878 8879 /** 8880 * @return {@link #performerRole} (The type of role or competency of an 8881 * individual desired or required to perform or not perform the action.) 8882 */ 8883 public CodeableConcept getPerformerRole() { 8884 if (this.performerRole == null) 8885 if (Configuration.errorOnAutoCreate()) 8886 throw new Error("Attempt to auto-create ActionComponent.performerRole"); 8887 else if (Configuration.doAutoCreate()) 8888 this.performerRole = new CodeableConcept(); // cc 8889 return this.performerRole; 8890 } 8891 8892 public boolean hasPerformerRole() { 8893 return this.performerRole != null && !this.performerRole.isEmpty(); 8894 } 8895 8896 /** 8897 * @param value {@link #performerRole} (The type of role or competency of an 8898 * individual desired or required to perform or not perform the 8899 * action.) 8900 */ 8901 public ActionComponent setPerformerRole(CodeableConcept value) { 8902 this.performerRole = value; 8903 return this; 8904 } 8905 8906 /** 8907 * @return {@link #performer} (Indicates who or what is being asked to perform 8908 * (or not perform) the ction.) 8909 */ 8910 public Reference getPerformer() { 8911 if (this.performer == null) 8912 if (Configuration.errorOnAutoCreate()) 8913 throw new Error("Attempt to auto-create ActionComponent.performer"); 8914 else if (Configuration.doAutoCreate()) 8915 this.performer = new Reference(); // cc 8916 return this.performer; 8917 } 8918 8919 public boolean hasPerformer() { 8920 return this.performer != null && !this.performer.isEmpty(); 8921 } 8922 8923 /** 8924 * @param value {@link #performer} (Indicates who or what is being asked to 8925 * perform (or not perform) the ction.) 8926 */ 8927 public ActionComponent setPerformer(Reference value) { 8928 this.performer = value; 8929 return this; 8930 } 8931 8932 /** 8933 * @return {@link #performer} The actual object that is the target of the 8934 * reference. The reference library doesn't populate this, but you can 8935 * use it to hold the resource if you resolve it. (Indicates who or what 8936 * is being asked to perform (or not perform) the ction.) 8937 */ 8938 public Resource getPerformerTarget() { 8939 return this.performerTarget; 8940 } 8941 8942 /** 8943 * @param value {@link #performer} The actual object that is the target of the 8944 * reference. The reference library doesn't use these, but you can 8945 * use it to hold the resource if you resolve it. (Indicates who or 8946 * what is being asked to perform (or not perform) the ction.) 8947 */ 8948 public ActionComponent setPerformerTarget(Resource value) { 8949 this.performerTarget = value; 8950 return this; 8951 } 8952 8953 /** 8954 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question 8955 * text related to the reason type or reference of this action in the 8956 * referenced form or QuestionnaireResponse.) 8957 */ 8958 public List<StringType> getPerformerLinkId() { 8959 if (this.performerLinkId == null) 8960 this.performerLinkId = new ArrayList<StringType>(); 8961 return this.performerLinkId; 8962 } 8963 8964 /** 8965 * @return Returns a reference to <code>this</code> for easy method chaining 8966 */ 8967 public ActionComponent setPerformerLinkId(List<StringType> thePerformerLinkId) { 8968 this.performerLinkId = thePerformerLinkId; 8969 return this; 8970 } 8971 8972 public boolean hasPerformerLinkId() { 8973 if (this.performerLinkId == null) 8974 return false; 8975 for (StringType item : this.performerLinkId) 8976 if (!item.isEmpty()) 8977 return true; 8978 return false; 8979 } 8980 8981 /** 8982 * @return {@link #performerLinkId} (Id [identifier??] of the clause or question 8983 * text related to the reason type or reference of this action in the 8984 * referenced form or QuestionnaireResponse.) 8985 */ 8986 public StringType addPerformerLinkIdElement() {// 2 8987 StringType t = new StringType(); 8988 if (this.performerLinkId == null) 8989 this.performerLinkId = new ArrayList<StringType>(); 8990 this.performerLinkId.add(t); 8991 return t; 8992 } 8993 8994 /** 8995 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or 8996 * question text related to the reason type or reference of this 8997 * action in the referenced form or QuestionnaireResponse.) 8998 */ 8999 public ActionComponent addPerformerLinkId(String value) { // 1 9000 StringType t = new StringType(); 9001 t.setValue(value); 9002 if (this.performerLinkId == null) 9003 this.performerLinkId = new ArrayList<StringType>(); 9004 this.performerLinkId.add(t); 9005 return this; 9006 } 9007 9008 /** 9009 * @param value {@link #performerLinkId} (Id [identifier??] of the clause or 9010 * question text related to the reason type or reference of this 9011 * action in the referenced form or QuestionnaireResponse.) 9012 */ 9013 public boolean hasPerformerLinkId(String value) { 9014 if (this.performerLinkId == null) 9015 return false; 9016 for (StringType v : this.performerLinkId) 9017 if (v.getValue().equals(value)) // string 9018 return true; 9019 return false; 9020 } 9021 9022 /** 9023 * @return {@link #reasonCode} (Rationale for the action to be performed or not 9024 * performed. Describes why the action is permitted or prohibited.) 9025 */ 9026 public List<CodeableConcept> getReasonCode() { 9027 if (this.reasonCode == null) 9028 this.reasonCode = new ArrayList<CodeableConcept>(); 9029 return this.reasonCode; 9030 } 9031 9032 /** 9033 * @return Returns a reference to <code>this</code> for easy method chaining 9034 */ 9035 public ActionComponent setReasonCode(List<CodeableConcept> theReasonCode) { 9036 this.reasonCode = theReasonCode; 9037 return this; 9038 } 9039 9040 public boolean hasReasonCode() { 9041 if (this.reasonCode == null) 9042 return false; 9043 for (CodeableConcept item : this.reasonCode) 9044 if (!item.isEmpty()) 9045 return true; 9046 return false; 9047 } 9048 9049 public CodeableConcept addReasonCode() { // 3 9050 CodeableConcept t = new CodeableConcept(); 9051 if (this.reasonCode == null) 9052 this.reasonCode = new ArrayList<CodeableConcept>(); 9053 this.reasonCode.add(t); 9054 return t; 9055 } 9056 9057 public ActionComponent addReasonCode(CodeableConcept t) { // 3 9058 if (t == null) 9059 return this; 9060 if (this.reasonCode == null) 9061 this.reasonCode = new ArrayList<CodeableConcept>(); 9062 this.reasonCode.add(t); 9063 return this; 9064 } 9065 9066 /** 9067 * @return The first repetition of repeating field {@link #reasonCode}, creating 9068 * it if it does not already exist 9069 */ 9070 public CodeableConcept getReasonCodeFirstRep() { 9071 if (getReasonCode().isEmpty()) { 9072 addReasonCode(); 9073 } 9074 return getReasonCode().get(0); 9075 } 9076 9077 /** 9078 * @return {@link #reasonReference} (Indicates another resource whose existence 9079 * justifies permitting or not permitting this action.) 9080 */ 9081 public List<Reference> getReasonReference() { 9082 if (this.reasonReference == null) 9083 this.reasonReference = new ArrayList<Reference>(); 9084 return this.reasonReference; 9085 } 9086 9087 /** 9088 * @return Returns a reference to <code>this</code> for easy method chaining 9089 */ 9090 public ActionComponent setReasonReference(List<Reference> theReasonReference) { 9091 this.reasonReference = theReasonReference; 9092 return this; 9093 } 9094 9095 public boolean hasReasonReference() { 9096 if (this.reasonReference == null) 9097 return false; 9098 for (Reference item : this.reasonReference) 9099 if (!item.isEmpty()) 9100 return true; 9101 return false; 9102 } 9103 9104 public Reference addReasonReference() { // 3 9105 Reference t = new Reference(); 9106 if (this.reasonReference == null) 9107 this.reasonReference = new ArrayList<Reference>(); 9108 this.reasonReference.add(t); 9109 return t; 9110 } 9111 9112 public ActionComponent addReasonReference(Reference t) { // 3 9113 if (t == null) 9114 return this; 9115 if (this.reasonReference == null) 9116 this.reasonReference = new ArrayList<Reference>(); 9117 this.reasonReference.add(t); 9118 return this; 9119 } 9120 9121 /** 9122 * @return The first repetition of repeating field {@link #reasonReference}, 9123 * creating it if it does not already exist 9124 */ 9125 public Reference getReasonReferenceFirstRep() { 9126 if (getReasonReference().isEmpty()) { 9127 addReasonReference(); 9128 } 9129 return getReasonReference().get(0); 9130 } 9131 9132 /** 9133 * @return {@link #reason} (Describes why the action is to be performed or not 9134 * performed in textual form.) 9135 */ 9136 public List<StringType> getReason() { 9137 if (this.reason == null) 9138 this.reason = new ArrayList<StringType>(); 9139 return this.reason; 9140 } 9141 9142 /** 9143 * @return Returns a reference to <code>this</code> for easy method chaining 9144 */ 9145 public ActionComponent setReason(List<StringType> theReason) { 9146 this.reason = theReason; 9147 return this; 9148 } 9149 9150 public boolean hasReason() { 9151 if (this.reason == null) 9152 return false; 9153 for (StringType item : this.reason) 9154 if (!item.isEmpty()) 9155 return true; 9156 return false; 9157 } 9158 9159 /** 9160 * @return {@link #reason} (Describes why the action is to be performed or not 9161 * performed in textual form.) 9162 */ 9163 public StringType addReasonElement() {// 2 9164 StringType t = new StringType(); 9165 if (this.reason == null) 9166 this.reason = new ArrayList<StringType>(); 9167 this.reason.add(t); 9168 return t; 9169 } 9170 9171 /** 9172 * @param value {@link #reason} (Describes why the action is to be performed or 9173 * not performed in textual form.) 9174 */ 9175 public ActionComponent addReason(String value) { // 1 9176 StringType t = new StringType(); 9177 t.setValue(value); 9178 if (this.reason == null) 9179 this.reason = new ArrayList<StringType>(); 9180 this.reason.add(t); 9181 return this; 9182 } 9183 9184 /** 9185 * @param value {@link #reason} (Describes why the action is to be performed or 9186 * not performed in textual form.) 9187 */ 9188 public boolean hasReason(String value) { 9189 if (this.reason == null) 9190 return false; 9191 for (StringType v : this.reason) 9192 if (v.getValue().equals(value)) // string 9193 return true; 9194 return false; 9195 } 9196 9197 /** 9198 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question 9199 * text related to the reason type or reference of this action in the 9200 * referenced form or QuestionnaireResponse.) 9201 */ 9202 public List<StringType> getReasonLinkId() { 9203 if (this.reasonLinkId == null) 9204 this.reasonLinkId = new ArrayList<StringType>(); 9205 return this.reasonLinkId; 9206 } 9207 9208 /** 9209 * @return Returns a reference to <code>this</code> for easy method chaining 9210 */ 9211 public ActionComponent setReasonLinkId(List<StringType> theReasonLinkId) { 9212 this.reasonLinkId = theReasonLinkId; 9213 return this; 9214 } 9215 9216 public boolean hasReasonLinkId() { 9217 if (this.reasonLinkId == null) 9218 return false; 9219 for (StringType item : this.reasonLinkId) 9220 if (!item.isEmpty()) 9221 return true; 9222 return false; 9223 } 9224 9225 /** 9226 * @return {@link #reasonLinkId} (Id [identifier??] of the clause or question 9227 * text related to the reason type or reference of this action in the 9228 * referenced form or QuestionnaireResponse.) 9229 */ 9230 public StringType addReasonLinkIdElement() {// 2 9231 StringType t = new StringType(); 9232 if (this.reasonLinkId == null) 9233 this.reasonLinkId = new ArrayList<StringType>(); 9234 this.reasonLinkId.add(t); 9235 return t; 9236 } 9237 9238 /** 9239 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or 9240 * question text related to the reason type or reference of this 9241 * action in the referenced form or QuestionnaireResponse.) 9242 */ 9243 public ActionComponent addReasonLinkId(String value) { // 1 9244 StringType t = new StringType(); 9245 t.setValue(value); 9246 if (this.reasonLinkId == null) 9247 this.reasonLinkId = new ArrayList<StringType>(); 9248 this.reasonLinkId.add(t); 9249 return this; 9250 } 9251 9252 /** 9253 * @param value {@link #reasonLinkId} (Id [identifier??] of the clause or 9254 * question text related to the reason type or reference of this 9255 * action in the referenced form or QuestionnaireResponse.) 9256 */ 9257 public boolean hasReasonLinkId(String value) { 9258 if (this.reasonLinkId == null) 9259 return false; 9260 for (StringType v : this.reasonLinkId) 9261 if (v.getValue().equals(value)) // string 9262 return true; 9263 return false; 9264 } 9265 9266 /** 9267 * @return {@link #note} (Comments made about the term action made by the 9268 * requester, performer, subject or other participants.) 9269 */ 9270 public List<Annotation> getNote() { 9271 if (this.note == null) 9272 this.note = new ArrayList<Annotation>(); 9273 return this.note; 9274 } 9275 9276 /** 9277 * @return Returns a reference to <code>this</code> for easy method chaining 9278 */ 9279 public ActionComponent setNote(List<Annotation> theNote) { 9280 this.note = theNote; 9281 return this; 9282 } 9283 9284 public boolean hasNote() { 9285 if (this.note == null) 9286 return false; 9287 for (Annotation item : this.note) 9288 if (!item.isEmpty()) 9289 return true; 9290 return false; 9291 } 9292 9293 public Annotation addNote() { // 3 9294 Annotation t = new Annotation(); 9295 if (this.note == null) 9296 this.note = new ArrayList<Annotation>(); 9297 this.note.add(t); 9298 return t; 9299 } 9300 9301 public ActionComponent addNote(Annotation t) { // 3 9302 if (t == null) 9303 return this; 9304 if (this.note == null) 9305 this.note = new ArrayList<Annotation>(); 9306 this.note.add(t); 9307 return this; 9308 } 9309 9310 /** 9311 * @return The first repetition of repeating field {@link #note}, creating it if 9312 * it does not already exist 9313 */ 9314 public Annotation getNoteFirstRep() { 9315 if (getNote().isEmpty()) { 9316 addNote(); 9317 } 9318 return getNote().get(0); 9319 } 9320 9321 /** 9322 * @return {@link #securityLabelNumber} (Security labels that protects the 9323 * action.) 9324 */ 9325 public List<UnsignedIntType> getSecurityLabelNumber() { 9326 if (this.securityLabelNumber == null) 9327 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9328 return this.securityLabelNumber; 9329 } 9330 9331 /** 9332 * @return Returns a reference to <code>this</code> for easy method chaining 9333 */ 9334 public ActionComponent setSecurityLabelNumber(List<UnsignedIntType> theSecurityLabelNumber) { 9335 this.securityLabelNumber = theSecurityLabelNumber; 9336 return this; 9337 } 9338 9339 public boolean hasSecurityLabelNumber() { 9340 if (this.securityLabelNumber == null) 9341 return false; 9342 for (UnsignedIntType item : this.securityLabelNumber) 9343 if (!item.isEmpty()) 9344 return true; 9345 return false; 9346 } 9347 9348 /** 9349 * @return {@link #securityLabelNumber} (Security labels that protects the 9350 * action.) 9351 */ 9352 public UnsignedIntType addSecurityLabelNumberElement() {// 2 9353 UnsignedIntType t = new UnsignedIntType(); 9354 if (this.securityLabelNumber == null) 9355 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9356 this.securityLabelNumber.add(t); 9357 return t; 9358 } 9359 9360 /** 9361 * @param value {@link #securityLabelNumber} (Security labels that protects the 9362 * action.) 9363 */ 9364 public ActionComponent addSecurityLabelNumber(int value) { // 1 9365 UnsignedIntType t = new UnsignedIntType(); 9366 t.setValue(value); 9367 if (this.securityLabelNumber == null) 9368 this.securityLabelNumber = new ArrayList<UnsignedIntType>(); 9369 this.securityLabelNumber.add(t); 9370 return this; 9371 } 9372 9373 /** 9374 * @param value {@link #securityLabelNumber} (Security labels that protects the 9375 * action.) 9376 */ 9377 public boolean hasSecurityLabelNumber(int value) { 9378 if (this.securityLabelNumber == null) 9379 return false; 9380 for (UnsignedIntType v : this.securityLabelNumber) 9381 if (v.getValue().equals(value)) // unsignedInt 9382 return true; 9383 return false; 9384 } 9385 9386 protected void listChildren(List<Property> children) { 9387 super.listChildren(children); 9388 children 9389 .add(new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 1, doNotPerform)); 9390 children.add(new Property("type", "CodeableConcept", 9391 "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 9392 0, 1, type)); 9393 children.add(new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, subject)); 9394 children.add(new Property("intent", "CodeableConcept", 9395 "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent)); 9396 children.add(new Property("linkId", "string", 9397 "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 9398 0, java.lang.Integer.MAX_VALUE, linkId)); 9399 children.add(new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, status)); 9400 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 9401 "Encounter or Episode with primary association to specified term activity.", 0, 1, context)); 9402 children.add(new Property("contextLinkId", "string", 9403 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9404 0, java.lang.Integer.MAX_VALUE, contextLinkId)); 9405 children.add(new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, occurrence)); 9406 children.add(new Property("requester", 9407 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 9408 "Who or what initiated the action and has responsibility for its activation.", 0, java.lang.Integer.MAX_VALUE, 9409 requester)); 9410 children.add(new Property("requesterLinkId", "string", 9411 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9412 0, java.lang.Integer.MAX_VALUE, requesterLinkId)); 9413 children.add(new Property("performerType", "CodeableConcept", 9414 "The type of individual that is desired or required to perform or not perform the action.", 0, 9415 java.lang.Integer.MAX_VALUE, performerType)); 9416 children.add(new Property("performerRole", "CodeableConcept", 9417 "The type of role or competency of an individual desired or required to perform or not perform the action.", 9418 0, 1, performerRole)); 9419 children.add(new Property("performer", 9420 "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", 9421 "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer)); 9422 children.add(new Property("performerLinkId", "string", 9423 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9424 0, java.lang.Integer.MAX_VALUE, performerLinkId)); 9425 children.add(new Property("reasonCode", "CodeableConcept", 9426 "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.", 9427 0, java.lang.Integer.MAX_VALUE, reasonCode)); 9428 children.add(new Property("reasonReference", 9429 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", 9430 "Indicates another resource whose existence justifies permitting or not permitting this action.", 0, 9431 java.lang.Integer.MAX_VALUE, reasonReference)); 9432 children.add(new Property("reason", "string", 9433 "Describes why the action is to be performed or not performed in textual form.", 0, 9434 java.lang.Integer.MAX_VALUE, reason)); 9435 children.add(new Property("reasonLinkId", "string", 9436 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9437 0, java.lang.Integer.MAX_VALUE, reasonLinkId)); 9438 children.add(new Property("note", "Annotation", 9439 "Comments made about the term action made by the requester, performer, subject or other participants.", 0, 9440 java.lang.Integer.MAX_VALUE, note)); 9441 children.add(new Property("securityLabelNumber", "unsignedInt", "Security labels that protects the action.", 0, 9442 java.lang.Integer.MAX_VALUE, securityLabelNumber)); 9443 } 9444 9445 @Override 9446 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9447 switch (_hash) { 9448 case -1788508167: 9449 /* doNotPerform */ return new Property("doNotPerform", "boolean", "True if the term prohibits the action.", 0, 9450 1, doNotPerform); 9451 case 3575610: 9452 /* type */ return new Property("type", "CodeableConcept", 9453 "Activity or service obligation to be done or not done, performed or not performed, effectuated or not by this Contract term.", 9454 0, 1, type); 9455 case -1867885268: 9456 /* subject */ return new Property("subject", "", "Entity of the action.", 0, java.lang.Integer.MAX_VALUE, 9457 subject); 9458 case -1183762788: 9459 /* intent */ return new Property("intent", "CodeableConcept", 9460 "Reason or purpose for the action stipulated by this Contract Provision.", 0, 1, intent); 9461 case -1102667083: 9462 /* linkId */ return new Property("linkId", "string", 9463 "Id [identifier??] of the clause or question text related to this action in the referenced form or QuestionnaireResponse.", 9464 0, java.lang.Integer.MAX_VALUE, linkId); 9465 case -892481550: 9466 /* status */ return new Property("status", "CodeableConcept", "Current state of the term action.", 0, 1, 9467 status); 9468 case 951530927: 9469 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 9470 "Encounter or Episode with primary association to specified term activity.", 0, 1, context); 9471 case -288783036: 9472 /* contextLinkId */ return new Property("contextLinkId", "string", 9473 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9474 0, java.lang.Integer.MAX_VALUE, contextLinkId); 9475 case -2022646513: 9476 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, 9477 occurrence); 9478 case 1687874001: 9479 /* occurrence */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 1, 9480 occurrence); 9481 case -298443636: 9482 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 9483 0, 1, occurrence); 9484 case 1397156594: 9485 /* occurrencePeriod */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 9486 1, occurrence); 9487 case 1515218299: 9488 /* occurrenceTiming */ return new Property("occurrence[x]", "dateTime|Period|Timing", "When action happens.", 0, 9489 1, occurrence); 9490 case 693933948: 9491 /* requester */ return new Property("requester", 9492 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 9493 "Who or what initiated the action and has responsibility for its activation.", 0, 9494 java.lang.Integer.MAX_VALUE, requester); 9495 case -1468032687: 9496 /* requesterLinkId */ return new Property("requesterLinkId", "string", 9497 "Id [identifier??] of the clause or question text related to the requester of this action in the referenced form or QuestionnaireResponse.", 9498 0, java.lang.Integer.MAX_VALUE, requesterLinkId); 9499 case -901444568: 9500 /* performerType */ return new Property("performerType", "CodeableConcept", 9501 "The type of individual that is desired or required to perform or not perform the action.", 0, 9502 java.lang.Integer.MAX_VALUE, performerType); 9503 case -901513884: 9504 /* performerRole */ return new Property("performerRole", "CodeableConcept", 9505 "The type of role or competency of an individual desired or required to perform or not perform the action.", 9506 0, 1, performerRole); 9507 case 481140686: 9508 /* performer */ return new Property("performer", 9509 "Reference(RelatedPerson|Patient|Practitioner|PractitionerRole|CareTeam|Device|Substance|Organization|Location)", 9510 "Indicates who or what is being asked to perform (or not perform) the ction.", 0, 1, performer); 9511 case 1051302947: 9512 /* performerLinkId */ return new Property("performerLinkId", "string", 9513 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9514 0, java.lang.Integer.MAX_VALUE, performerLinkId); 9515 case 722137681: 9516 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 9517 "Rationale for the action to be performed or not performed. Describes why the action is permitted or prohibited.", 9518 0, java.lang.Integer.MAX_VALUE, reasonCode); 9519 case -1146218137: 9520 /* reasonReference */ return new Property("reasonReference", 9521 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Questionnaire|QuestionnaireResponse)", 9522 "Indicates another resource whose existence justifies permitting or not permitting this action.", 0, 9523 java.lang.Integer.MAX_VALUE, reasonReference); 9524 case -934964668: 9525 /* reason */ return new Property("reason", "string", 9526 "Describes why the action is to be performed or not performed in textual form.", 0, 9527 java.lang.Integer.MAX_VALUE, reason); 9528 case -1557963239: 9529 /* reasonLinkId */ return new Property("reasonLinkId", "string", 9530 "Id [identifier??] of the clause or question text related to the reason type or reference of this action in the referenced form or QuestionnaireResponse.", 9531 0, java.lang.Integer.MAX_VALUE, reasonLinkId); 9532 case 3387378: 9533 /* note */ return new Property("note", "Annotation", 9534 "Comments made about the term action made by the requester, performer, subject or other participants.", 0, 9535 java.lang.Integer.MAX_VALUE, note); 9536 case -149460995: 9537 /* securityLabelNumber */ return new Property("securityLabelNumber", "unsignedInt", 9538 "Security labels that protects the action.", 0, java.lang.Integer.MAX_VALUE, securityLabelNumber); 9539 default: 9540 return super.getNamedProperty(_hash, _name, _checkValid); 9541 } 9542 9543 } 9544 9545 @Override 9546 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9547 switch (hash) { 9548 case -1788508167: 9549 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 9550 case 3575610: 9551 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 9552 case -1867885268: 9553 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // ActionSubjectComponent 9554 case -1183762788: 9555 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // CodeableConcept 9556 case -1102667083: 9557 /* linkId */ return this.linkId == null ? new Base[0] : this.linkId.toArray(new Base[this.linkId.size()]); // StringType 9558 case -892481550: 9559 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 9560 case 951530927: 9561 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 9562 case -288783036: 9563 /* contextLinkId */ return this.contextLinkId == null ? new Base[0] 9564 : this.contextLinkId.toArray(new Base[this.contextLinkId.size()]); // StringType 9565 case 1687874001: 9566 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 9567 case 693933948: 9568 /* requester */ return this.requester == null ? new Base[0] 9569 : this.requester.toArray(new Base[this.requester.size()]); // Reference 9570 case -1468032687: 9571 /* requesterLinkId */ return this.requesterLinkId == null ? new Base[0] 9572 : this.requesterLinkId.toArray(new Base[this.requesterLinkId.size()]); // StringType 9573 case -901444568: 9574 /* performerType */ return this.performerType == null ? new Base[0] 9575 : this.performerType.toArray(new Base[this.performerType.size()]); // CodeableConcept 9576 case -901513884: 9577 /* performerRole */ return this.performerRole == null ? new Base[0] : new Base[] { this.performerRole }; // CodeableConcept 9578 case 481140686: 9579 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 9580 case 1051302947: 9581 /* performerLinkId */ return this.performerLinkId == null ? new Base[0] 9582 : this.performerLinkId.toArray(new Base[this.performerLinkId.size()]); // StringType 9583 case 722137681: 9584 /* reasonCode */ return this.reasonCode == null ? new Base[0] 9585 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 9586 case -1146218137: 9587 /* reasonReference */ return this.reasonReference == null ? new Base[0] 9588 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 9589 case -934964668: 9590 /* reason */ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // StringType 9591 case -1557963239: 9592 /* reasonLinkId */ return this.reasonLinkId == null ? new Base[0] 9593 : this.reasonLinkId.toArray(new Base[this.reasonLinkId.size()]); // StringType 9594 case 3387378: 9595 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 9596 case -149460995: 9597 /* securityLabelNumber */ return this.securityLabelNumber == null ? new Base[0] 9598 : this.securityLabelNumber.toArray(new Base[this.securityLabelNumber.size()]); // UnsignedIntType 9599 default: 9600 return super.getProperty(hash, name, checkValid); 9601 } 9602 9603 } 9604 9605 @Override 9606 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9607 switch (hash) { 9608 case -1788508167: // doNotPerform 9609 this.doNotPerform = castToBoolean(value); // BooleanType 9610 return value; 9611 case 3575610: // type 9612 this.type = castToCodeableConcept(value); // CodeableConcept 9613 return value; 9614 case -1867885268: // subject 9615 this.getSubject().add((ActionSubjectComponent) value); // ActionSubjectComponent 9616 return value; 9617 case -1183762788: // intent 9618 this.intent = castToCodeableConcept(value); // CodeableConcept 9619 return value; 9620 case -1102667083: // linkId 9621 this.getLinkId().add(castToString(value)); // StringType 9622 return value; 9623 case -892481550: // status 9624 this.status = castToCodeableConcept(value); // CodeableConcept 9625 return value; 9626 case 951530927: // context 9627 this.context = castToReference(value); // Reference 9628 return value; 9629 case -288783036: // contextLinkId 9630 this.getContextLinkId().add(castToString(value)); // StringType 9631 return value; 9632 case 1687874001: // occurrence 9633 this.occurrence = castToType(value); // Type 9634 return value; 9635 case 693933948: // requester 9636 this.getRequester().add(castToReference(value)); // Reference 9637 return value; 9638 case -1468032687: // requesterLinkId 9639 this.getRequesterLinkId().add(castToString(value)); // StringType 9640 return value; 9641 case -901444568: // performerType 9642 this.getPerformerType().add(castToCodeableConcept(value)); // CodeableConcept 9643 return value; 9644 case -901513884: // performerRole 9645 this.performerRole = castToCodeableConcept(value); // CodeableConcept 9646 return value; 9647 case 481140686: // performer 9648 this.performer = castToReference(value); // Reference 9649 return value; 9650 case 1051302947: // performerLinkId 9651 this.getPerformerLinkId().add(castToString(value)); // StringType 9652 return value; 9653 case 722137681: // reasonCode 9654 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 9655 return value; 9656 case -1146218137: // reasonReference 9657 this.getReasonReference().add(castToReference(value)); // Reference 9658 return value; 9659 case -934964668: // reason 9660 this.getReason().add(castToString(value)); // StringType 9661 return value; 9662 case -1557963239: // reasonLinkId 9663 this.getReasonLinkId().add(castToString(value)); // StringType 9664 return value; 9665 case 3387378: // note 9666 this.getNote().add(castToAnnotation(value)); // Annotation 9667 return value; 9668 case -149460995: // securityLabelNumber 9669 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); // UnsignedIntType 9670 return value; 9671 default: 9672 return super.setProperty(hash, name, value); 9673 } 9674 9675 } 9676 9677 @Override 9678 public Base setProperty(String name, Base value) throws FHIRException { 9679 if (name.equals("doNotPerform")) { 9680 this.doNotPerform = castToBoolean(value); // BooleanType 9681 } else if (name.equals("type")) { 9682 this.type = castToCodeableConcept(value); // CodeableConcept 9683 } else if (name.equals("subject")) { 9684 this.getSubject().add((ActionSubjectComponent) value); 9685 } else if (name.equals("intent")) { 9686 this.intent = castToCodeableConcept(value); // CodeableConcept 9687 } else if (name.equals("linkId")) { 9688 this.getLinkId().add(castToString(value)); 9689 } else if (name.equals("status")) { 9690 this.status = castToCodeableConcept(value); // CodeableConcept 9691 } else if (name.equals("context")) { 9692 this.context = castToReference(value); // Reference 9693 } else if (name.equals("contextLinkId")) { 9694 this.getContextLinkId().add(castToString(value)); 9695 } else if (name.equals("occurrence[x]")) { 9696 this.occurrence = castToType(value); // Type 9697 } else if (name.equals("requester")) { 9698 this.getRequester().add(castToReference(value)); 9699 } else if (name.equals("requesterLinkId")) { 9700 this.getRequesterLinkId().add(castToString(value)); 9701 } else if (name.equals("performerType")) { 9702 this.getPerformerType().add(castToCodeableConcept(value)); 9703 } else if (name.equals("performerRole")) { 9704 this.performerRole = castToCodeableConcept(value); // CodeableConcept 9705 } else if (name.equals("performer")) { 9706 this.performer = castToReference(value); // Reference 9707 } else if (name.equals("performerLinkId")) { 9708 this.getPerformerLinkId().add(castToString(value)); 9709 } else if (name.equals("reasonCode")) { 9710 this.getReasonCode().add(castToCodeableConcept(value)); 9711 } else if (name.equals("reasonReference")) { 9712 this.getReasonReference().add(castToReference(value)); 9713 } else if (name.equals("reason")) { 9714 this.getReason().add(castToString(value)); 9715 } else if (name.equals("reasonLinkId")) { 9716 this.getReasonLinkId().add(castToString(value)); 9717 } else if (name.equals("note")) { 9718 this.getNote().add(castToAnnotation(value)); 9719 } else if (name.equals("securityLabelNumber")) { 9720 this.getSecurityLabelNumber().add(castToUnsignedInt(value)); 9721 } else 9722 return super.setProperty(name, value); 9723 return value; 9724 } 9725 9726 @Override 9727 public void removeChild(String name, Base value) throws FHIRException { 9728 if (name.equals("doNotPerform")) { 9729 this.doNotPerform = null; 9730 } else if (name.equals("type")) { 9731 this.type = null; 9732 } else if (name.equals("subject")) { 9733 this.getSubject().remove((ActionSubjectComponent) value); 9734 } else if (name.equals("intent")) { 9735 this.intent = null; 9736 } else if (name.equals("linkId")) { 9737 this.getLinkId().remove(castToString(value)); 9738 } else if (name.equals("status")) { 9739 this.status = null; 9740 } else if (name.equals("context")) { 9741 this.context = null; 9742 } else if (name.equals("contextLinkId")) { 9743 this.getContextLinkId().remove(castToString(value)); 9744 } else if (name.equals("occurrence[x]")) { 9745 this.occurrence = null; 9746 } else if (name.equals("requester")) { 9747 this.getRequester().remove(castToReference(value)); 9748 } else if (name.equals("requesterLinkId")) { 9749 this.getRequesterLinkId().remove(castToString(value)); 9750 } else if (name.equals("performerType")) { 9751 this.getPerformerType().remove(castToCodeableConcept(value)); 9752 } else if (name.equals("performerRole")) { 9753 this.performerRole = null; 9754 } else if (name.equals("performer")) { 9755 this.performer = null; 9756 } else if (name.equals("performerLinkId")) { 9757 this.getPerformerLinkId().remove(castToString(value)); 9758 } else if (name.equals("reasonCode")) { 9759 this.getReasonCode().remove(castToCodeableConcept(value)); 9760 } else if (name.equals("reasonReference")) { 9761 this.getReasonReference().remove(castToReference(value)); 9762 } else if (name.equals("reason")) { 9763 this.getReason().remove(castToString(value)); 9764 } else if (name.equals("reasonLinkId")) { 9765 this.getReasonLinkId().remove(castToString(value)); 9766 } else if (name.equals("note")) { 9767 this.getNote().remove(castToAnnotation(value)); 9768 } else if (name.equals("securityLabelNumber")) { 9769 this.getSecurityLabelNumber().remove(castToUnsignedInt(value)); 9770 } else 9771 super.removeChild(name, value); 9772 9773 } 9774 9775 @Override 9776 public Base makeProperty(int hash, String name) throws FHIRException { 9777 switch (hash) { 9778 case -1788508167: 9779 return getDoNotPerformElement(); 9780 case 3575610: 9781 return getType(); 9782 case -1867885268: 9783 return addSubject(); 9784 case -1183762788: 9785 return getIntent(); 9786 case -1102667083: 9787 return addLinkIdElement(); 9788 case -892481550: 9789 return getStatus(); 9790 case 951530927: 9791 return getContext(); 9792 case -288783036: 9793 return addContextLinkIdElement(); 9794 case -2022646513: 9795 return getOccurrence(); 9796 case 1687874001: 9797 return getOccurrence(); 9798 case 693933948: 9799 return addRequester(); 9800 case -1468032687: 9801 return addRequesterLinkIdElement(); 9802 case -901444568: 9803 return addPerformerType(); 9804 case -901513884: 9805 return getPerformerRole(); 9806 case 481140686: 9807 return getPerformer(); 9808 case 1051302947: 9809 return addPerformerLinkIdElement(); 9810 case 722137681: 9811 return addReasonCode(); 9812 case -1146218137: 9813 return addReasonReference(); 9814 case -934964668: 9815 return addReasonElement(); 9816 case -1557963239: 9817 return addReasonLinkIdElement(); 9818 case 3387378: 9819 return addNote(); 9820 case -149460995: 9821 return addSecurityLabelNumberElement(); 9822 default: 9823 return super.makeProperty(hash, name); 9824 } 9825 9826 } 9827 9828 @Override 9829 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9830 switch (hash) { 9831 case -1788508167: 9832 /* doNotPerform */ return new String[] { "boolean" }; 9833 case 3575610: 9834 /* type */ return new String[] { "CodeableConcept" }; 9835 case -1867885268: 9836 /* subject */ return new String[] {}; 9837 case -1183762788: 9838 /* intent */ return new String[] { "CodeableConcept" }; 9839 case -1102667083: 9840 /* linkId */ return new String[] { "string" }; 9841 case -892481550: 9842 /* status */ return new String[] { "CodeableConcept" }; 9843 case 951530927: 9844 /* context */ return new String[] { "Reference" }; 9845 case -288783036: 9846 /* contextLinkId */ return new String[] { "string" }; 9847 case 1687874001: 9848 /* occurrence */ return new String[] { "dateTime", "Period", "Timing" }; 9849 case 693933948: 9850 /* requester */ return new String[] { "Reference" }; 9851 case -1468032687: 9852 /* requesterLinkId */ return new String[] { "string" }; 9853 case -901444568: 9854 /* performerType */ return new String[] { "CodeableConcept" }; 9855 case -901513884: 9856 /* performerRole */ return new String[] { "CodeableConcept" }; 9857 case 481140686: 9858 /* performer */ return new String[] { "Reference" }; 9859 case 1051302947: 9860 /* performerLinkId */ return new String[] { "string" }; 9861 case 722137681: 9862 /* reasonCode */ return new String[] { "CodeableConcept" }; 9863 case -1146218137: 9864 /* reasonReference */ return new String[] { "Reference" }; 9865 case -934964668: 9866 /* reason */ return new String[] { "string" }; 9867 case -1557963239: 9868 /* reasonLinkId */ return new String[] { "string" }; 9869 case 3387378: 9870 /* note */ return new String[] { "Annotation" }; 9871 case -149460995: 9872 /* securityLabelNumber */ return new String[] { "unsignedInt" }; 9873 default: 9874 return super.getTypesForProperty(hash, name); 9875 } 9876 9877 } 9878 9879 @Override 9880 public Base addChild(String name) throws FHIRException { 9881 if (name.equals("doNotPerform")) { 9882 throw new FHIRException("Cannot call addChild on a singleton property Contract.doNotPerform"); 9883 } else if (name.equals("type")) { 9884 this.type = new CodeableConcept(); 9885 return this.type; 9886 } else if (name.equals("subject")) { 9887 return addSubject(); 9888 } else if (name.equals("intent")) { 9889 this.intent = new CodeableConcept(); 9890 return this.intent; 9891 } else if (name.equals("linkId")) { 9892 throw new FHIRException("Cannot call addChild on a singleton property Contract.linkId"); 9893 } else if (name.equals("status")) { 9894 this.status = new CodeableConcept(); 9895 return this.status; 9896 } else if (name.equals("context")) { 9897 this.context = new Reference(); 9898 return this.context; 9899 } else if (name.equals("contextLinkId")) { 9900 throw new FHIRException("Cannot call addChild on a singleton property Contract.contextLinkId"); 9901 } else if (name.equals("occurrenceDateTime")) { 9902 this.occurrence = new DateTimeType(); 9903 return this.occurrence; 9904 } else if (name.equals("occurrencePeriod")) { 9905 this.occurrence = new Period(); 9906 return this.occurrence; 9907 } else if (name.equals("occurrenceTiming")) { 9908 this.occurrence = new Timing(); 9909 return this.occurrence; 9910 } else if (name.equals("requester")) { 9911 return addRequester(); 9912 } else if (name.equals("requesterLinkId")) { 9913 throw new FHIRException("Cannot call addChild on a singleton property Contract.requesterLinkId"); 9914 } else if (name.equals("performerType")) { 9915 return addPerformerType(); 9916 } else if (name.equals("performerRole")) { 9917 this.performerRole = new CodeableConcept(); 9918 return this.performerRole; 9919 } else if (name.equals("performer")) { 9920 this.performer = new Reference(); 9921 return this.performer; 9922 } else if (name.equals("performerLinkId")) { 9923 throw new FHIRException("Cannot call addChild on a singleton property Contract.performerLinkId"); 9924 } else if (name.equals("reasonCode")) { 9925 return addReasonCode(); 9926 } else if (name.equals("reasonReference")) { 9927 return addReasonReference(); 9928 } else if (name.equals("reason")) { 9929 throw new FHIRException("Cannot call addChild on a singleton property Contract.reason"); 9930 } else if (name.equals("reasonLinkId")) { 9931 throw new FHIRException("Cannot call addChild on a singleton property Contract.reasonLinkId"); 9932 } else if (name.equals("note")) { 9933 return addNote(); 9934 } else if (name.equals("securityLabelNumber")) { 9935 throw new FHIRException("Cannot call addChild on a singleton property Contract.securityLabelNumber"); 9936 } else 9937 return super.addChild(name); 9938 } 9939 9940 public ActionComponent copy() { 9941 ActionComponent dst = new ActionComponent(); 9942 copyValues(dst); 9943 return dst; 9944 } 9945 9946 public void copyValues(ActionComponent dst) { 9947 super.copyValues(dst); 9948 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 9949 dst.type = type == null ? null : type.copy(); 9950 if (subject != null) { 9951 dst.subject = new ArrayList<ActionSubjectComponent>(); 9952 for (ActionSubjectComponent i : subject) 9953 dst.subject.add(i.copy()); 9954 } 9955 ; 9956 dst.intent = intent == null ? null : intent.copy(); 9957 if (linkId != null) { 9958 dst.linkId = new ArrayList<StringType>(); 9959 for (StringType i : linkId) 9960 dst.linkId.add(i.copy()); 9961 } 9962 ; 9963 dst.status = status == null ? null : status.copy(); 9964 dst.context = context == null ? null : context.copy(); 9965 if (contextLinkId != null) { 9966 dst.contextLinkId = new ArrayList<StringType>(); 9967 for (StringType i : contextLinkId) 9968 dst.contextLinkId.add(i.copy()); 9969 } 9970 ; 9971 dst.occurrence = occurrence == null ? null : occurrence.copy(); 9972 if (requester != null) { 9973 dst.requester = new ArrayList<Reference>(); 9974 for (Reference i : requester) 9975 dst.requester.add(i.copy()); 9976 } 9977 ; 9978 if (requesterLinkId != null) { 9979 dst.requesterLinkId = new ArrayList<StringType>(); 9980 for (StringType i : requesterLinkId) 9981 dst.requesterLinkId.add(i.copy()); 9982 } 9983 ; 9984 if (performerType != null) { 9985 dst.performerType = new ArrayList<CodeableConcept>(); 9986 for (CodeableConcept i : performerType) 9987 dst.performerType.add(i.copy()); 9988 } 9989 ; 9990 dst.performerRole = performerRole == null ? null : performerRole.copy(); 9991 dst.performer = performer == null ? null : performer.copy(); 9992 if (performerLinkId != null) { 9993 dst.performerLinkId = new ArrayList<StringType>(); 9994 for (StringType i : performerLinkId) 9995 dst.performerLinkId.add(i.copy()); 9996 } 9997 ; 9998 if (reasonCode != null) { 9999 dst.reasonCode = new ArrayList<CodeableConcept>(); 10000 for (CodeableConcept i : reasonCode) 10001 dst.reasonCode.add(i.copy()); 10002 } 10003 ; 10004 if (reasonReference != null) { 10005 dst.reasonReference = new ArrayList<Reference>(); 10006 for (Reference i : reasonReference) 10007 dst.reasonReference.add(i.copy()); 10008 } 10009 ; 10010 if (reason != null) { 10011 dst.reason = new ArrayList<StringType>(); 10012 for (StringType i : reason) 10013 dst.reason.add(i.copy()); 10014 } 10015 ; 10016 if (reasonLinkId != null) { 10017 dst.reasonLinkId = new ArrayList<StringType>(); 10018 for (StringType i : reasonLinkId) 10019 dst.reasonLinkId.add(i.copy()); 10020 } 10021 ; 10022 if (note != null) { 10023 dst.note = new ArrayList<Annotation>(); 10024 for (Annotation i : note) 10025 dst.note.add(i.copy()); 10026 } 10027 ; 10028 if (securityLabelNumber != null) { 10029 dst.securityLabelNumber = new ArrayList<UnsignedIntType>(); 10030 for (UnsignedIntType i : securityLabelNumber) 10031 dst.securityLabelNumber.add(i.copy()); 10032 } 10033 ; 10034 } 10035 10036 @Override 10037 public boolean equalsDeep(Base other_) { 10038 if (!super.equalsDeep(other_)) 10039 return false; 10040 if (!(other_ instanceof ActionComponent)) 10041 return false; 10042 ActionComponent o = (ActionComponent) other_; 10043 return compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(type, o.type, true) 10044 && compareDeep(subject, o.subject, true) && compareDeep(intent, o.intent, true) 10045 && compareDeep(linkId, o.linkId, true) && compareDeep(status, o.status, true) 10046 && compareDeep(context, o.context, true) && compareDeep(contextLinkId, o.contextLinkId, true) 10047 && compareDeep(occurrence, o.occurrence, true) && compareDeep(requester, o.requester, true) 10048 && compareDeep(requesterLinkId, o.requesterLinkId, true) && compareDeep(performerType, o.performerType, true) 10049 && compareDeep(performerRole, o.performerRole, true) && compareDeep(performer, o.performer, true) 10050 && compareDeep(performerLinkId, o.performerLinkId, true) && compareDeep(reasonCode, o.reasonCode, true) 10051 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(reason, o.reason, true) 10052 && compareDeep(reasonLinkId, o.reasonLinkId, true) && compareDeep(note, o.note, true) 10053 && compareDeep(securityLabelNumber, o.securityLabelNumber, true); 10054 } 10055 10056 @Override 10057 public boolean equalsShallow(Base other_) { 10058 if (!super.equalsShallow(other_)) 10059 return false; 10060 if (!(other_ instanceof ActionComponent)) 10061 return false; 10062 ActionComponent o = (ActionComponent) other_; 10063 return compareValues(doNotPerform, o.doNotPerform, true) && compareValues(linkId, o.linkId, true) 10064 && compareValues(contextLinkId, o.contextLinkId, true) 10065 && compareValues(requesterLinkId, o.requesterLinkId, true) 10066 && compareValues(performerLinkId, o.performerLinkId, true) && compareValues(reason, o.reason, true) 10067 && compareValues(reasonLinkId, o.reasonLinkId, true) 10068 && compareValues(securityLabelNumber, o.securityLabelNumber, true); 10069 } 10070 10071 public boolean isEmpty() { 10072 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doNotPerform, type, subject, intent, linkId, 10073 status, context, contextLinkId, occurrence, requester, requesterLinkId, performerType, performerRole, 10074 performer, performerLinkId, reasonCode, reasonReference, reason, reasonLinkId, note, securityLabelNumber); 10075 } 10076 10077 public String fhirType() { 10078 return "Contract.term.action"; 10079 10080 } 10081 10082 } 10083 10084 @Block() 10085 public static class ActionSubjectComponent extends BackboneElement implements IBaseBackboneElement { 10086 /** 10087 * The entity the action is performed or not performed on or for. 10088 */ 10089 @Child(name = "reference", type = { Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class, 10090 Device.class, Group.class, 10091 Organization.class }, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 10092 @Description(shortDefinition = "Entity of the action", formalDefinition = "The entity the action is performed or not performed on or for.") 10093 protected List<Reference> reference; 10094 /** 10095 * The actual objects that are the target of the reference (The entity the 10096 * action is performed or not performed on or for.) 10097 */ 10098 protected List<Resource> referenceTarget; 10099 10100 /** 10101 * Role type of agent assigned roles in this Contract. 10102 */ 10103 @Child(name = "role", type = { 10104 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 10105 @Description(shortDefinition = "Role type of the agent", formalDefinition = "Role type of agent assigned roles in this Contract.") 10106 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-actorrole") 10107 protected CodeableConcept role; 10108 10109 private static final long serialVersionUID = 128949255L; 10110 10111 /** 10112 * Constructor 10113 */ 10114 public ActionSubjectComponent() { 10115 super(); 10116 } 10117 10118 /** 10119 * @return {@link #reference} (The entity the action is performed or not 10120 * performed on or for.) 10121 */ 10122 public List<Reference> getReference() { 10123 if (this.reference == null) 10124 this.reference = new ArrayList<Reference>(); 10125 return this.reference; 10126 } 10127 10128 /** 10129 * @return Returns a reference to <code>this</code> for easy method chaining 10130 */ 10131 public ActionSubjectComponent setReference(List<Reference> theReference) { 10132 this.reference = theReference; 10133 return this; 10134 } 10135 10136 public boolean hasReference() { 10137 if (this.reference == null) 10138 return false; 10139 for (Reference item : this.reference) 10140 if (!item.isEmpty()) 10141 return true; 10142 return false; 10143 } 10144 10145 public Reference addReference() { // 3 10146 Reference t = new Reference(); 10147 if (this.reference == null) 10148 this.reference = new ArrayList<Reference>(); 10149 this.reference.add(t); 10150 return t; 10151 } 10152 10153 public ActionSubjectComponent addReference(Reference t) { // 3 10154 if (t == null) 10155 return this; 10156 if (this.reference == null) 10157 this.reference = new ArrayList<Reference>(); 10158 this.reference.add(t); 10159 return this; 10160 } 10161 10162 /** 10163 * @return The first repetition of repeating field {@link #reference}, creating 10164 * it if it does not already exist 10165 */ 10166 public Reference getReferenceFirstRep() { 10167 if (getReference().isEmpty()) { 10168 addReference(); 10169 } 10170 return getReference().get(0); 10171 } 10172 10173 /** 10174 * @return {@link #role} (Role type of agent assigned roles in this Contract.) 10175 */ 10176 public CodeableConcept getRole() { 10177 if (this.role == null) 10178 if (Configuration.errorOnAutoCreate()) 10179 throw new Error("Attempt to auto-create ActionSubjectComponent.role"); 10180 else if (Configuration.doAutoCreate()) 10181 this.role = new CodeableConcept(); // cc 10182 return this.role; 10183 } 10184 10185 public boolean hasRole() { 10186 return this.role != null && !this.role.isEmpty(); 10187 } 10188 10189 /** 10190 * @param value {@link #role} (Role type of agent assigned roles in this 10191 * Contract.) 10192 */ 10193 public ActionSubjectComponent setRole(CodeableConcept value) { 10194 this.role = value; 10195 return this; 10196 } 10197 10198 protected void listChildren(List<Property> children) { 10199 super.listChildren(children); 10200 children.add(new Property("reference", 10201 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 10202 "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, reference)); 10203 children.add( 10204 new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 0, 1, role)); 10205 } 10206 10207 @Override 10208 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10209 switch (_hash) { 10210 case -925155509: 10211 /* reference */ return new Property("reference", 10212 "Reference(Patient|RelatedPerson|Practitioner|PractitionerRole|Device|Group|Organization)", 10213 "The entity the action is performed or not performed on or for.", 0, java.lang.Integer.MAX_VALUE, 10214 reference); 10215 case 3506294: 10216 /* role */ return new Property("role", "CodeableConcept", "Role type of agent assigned roles in this Contract.", 10217 0, 1, role); 10218 default: 10219 return super.getNamedProperty(_hash, _name, _checkValid); 10220 } 10221 10222 } 10223 10224 @Override 10225 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10226 switch (hash) { 10227 case -925155509: 10228 /* reference */ return this.reference == null ? new Base[0] 10229 : this.reference.toArray(new Base[this.reference.size()]); // Reference 10230 case 3506294: 10231 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 10232 default: 10233 return super.getProperty(hash, name, checkValid); 10234 } 10235 10236 } 10237 10238 @Override 10239 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10240 switch (hash) { 10241 case -925155509: // reference 10242 this.getReference().add(castToReference(value)); // Reference 10243 return value; 10244 case 3506294: // role 10245 this.role = castToCodeableConcept(value); // CodeableConcept 10246 return value; 10247 default: 10248 return super.setProperty(hash, name, value); 10249 } 10250 10251 } 10252 10253 @Override 10254 public Base setProperty(String name, Base value) throws FHIRException { 10255 if (name.equals("reference")) { 10256 this.getReference().add(castToReference(value)); 10257 } else if (name.equals("role")) { 10258 this.role = castToCodeableConcept(value); // CodeableConcept 10259 } else 10260 return super.setProperty(name, value); 10261 return value; 10262 } 10263 10264 @Override 10265 public void removeChild(String name, Base value) throws FHIRException { 10266 if (name.equals("reference")) { 10267 this.getReference().remove(castToReference(value)); 10268 } else if (name.equals("role")) { 10269 this.role = null; 10270 } else 10271 super.removeChild(name, value); 10272 10273 } 10274 10275 @Override 10276 public Base makeProperty(int hash, String name) throws FHIRException { 10277 switch (hash) { 10278 case -925155509: 10279 return addReference(); 10280 case 3506294: 10281 return getRole(); 10282 default: 10283 return super.makeProperty(hash, name); 10284 } 10285 10286 } 10287 10288 @Override 10289 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10290 switch (hash) { 10291 case -925155509: 10292 /* reference */ return new String[] { "Reference" }; 10293 case 3506294: 10294 /* role */ return new String[] { "CodeableConcept" }; 10295 default: 10296 return super.getTypesForProperty(hash, name); 10297 } 10298 10299 } 10300 10301 @Override 10302 public Base addChild(String name) throws FHIRException { 10303 if (name.equals("reference")) { 10304 return addReference(); 10305 } else if (name.equals("role")) { 10306 this.role = new CodeableConcept(); 10307 return this.role; 10308 } else 10309 return super.addChild(name); 10310 } 10311 10312 public ActionSubjectComponent copy() { 10313 ActionSubjectComponent dst = new ActionSubjectComponent(); 10314 copyValues(dst); 10315 return dst; 10316 } 10317 10318 public void copyValues(ActionSubjectComponent dst) { 10319 super.copyValues(dst); 10320 if (reference != null) { 10321 dst.reference = new ArrayList<Reference>(); 10322 for (Reference i : reference) 10323 dst.reference.add(i.copy()); 10324 } 10325 ; 10326 dst.role = role == null ? null : role.copy(); 10327 } 10328 10329 @Override 10330 public boolean equalsDeep(Base other_) { 10331 if (!super.equalsDeep(other_)) 10332 return false; 10333 if (!(other_ instanceof ActionSubjectComponent)) 10334 return false; 10335 ActionSubjectComponent o = (ActionSubjectComponent) other_; 10336 return compareDeep(reference, o.reference, true) && compareDeep(role, o.role, true); 10337 } 10338 10339 @Override 10340 public boolean equalsShallow(Base other_) { 10341 if (!super.equalsShallow(other_)) 10342 return false; 10343 if (!(other_ instanceof ActionSubjectComponent)) 10344 return false; 10345 ActionSubjectComponent o = (ActionSubjectComponent) other_; 10346 return true; 10347 } 10348 10349 public boolean isEmpty() { 10350 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, role); 10351 } 10352 10353 public String fhirType() { 10354 return "Contract.term.action.subject"; 10355 10356 } 10357 10358 } 10359 10360 @Block() 10361 public static class SignatoryComponent extends BackboneElement implements IBaseBackboneElement { 10362 /** 10363 * Role of this Contract signer, e.g. notary, grantee. 10364 */ 10365 @Child(name = "type", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 10366 @Description(shortDefinition = "Contract Signatory Role", formalDefinition = "Role of this Contract signer, e.g. notary, grantee.") 10367 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-signer-type") 10368 protected Coding type; 10369 10370 /** 10371 * Party which is a signator to this Contract. 10372 */ 10373 @Child(name = "party", type = { Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 10374 RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 10375 @Description(shortDefinition = "Contract Signatory Party", formalDefinition = "Party which is a signator to this Contract.") 10376 protected Reference party; 10377 10378 /** 10379 * The actual object that is the target of the reference (Party which is a 10380 * signator to this Contract.) 10381 */ 10382 protected Resource partyTarget; 10383 10384 /** 10385 * Legally binding Contract DSIG signature contents in Base64. 10386 */ 10387 @Child(name = "signature", type = { 10388 Signature.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 10389 @Description(shortDefinition = "Contract Documentation Signature", formalDefinition = "Legally binding Contract DSIG signature contents in Base64.") 10390 protected List<Signature> signature; 10391 10392 private static final long serialVersionUID = 1948139228L; 10393 10394 /** 10395 * Constructor 10396 */ 10397 public SignatoryComponent() { 10398 super(); 10399 } 10400 10401 /** 10402 * Constructor 10403 */ 10404 public SignatoryComponent(Coding type, Reference party) { 10405 super(); 10406 this.type = type; 10407 this.party = party; 10408 } 10409 10410 /** 10411 * @return {@link #type} (Role of this Contract signer, e.g. notary, grantee.) 10412 */ 10413 public Coding getType() { 10414 if (this.type == null) 10415 if (Configuration.errorOnAutoCreate()) 10416 throw new Error("Attempt to auto-create SignatoryComponent.type"); 10417 else if (Configuration.doAutoCreate()) 10418 this.type = new Coding(); // cc 10419 return this.type; 10420 } 10421 10422 public boolean hasType() { 10423 return this.type != null && !this.type.isEmpty(); 10424 } 10425 10426 /** 10427 * @param value {@link #type} (Role of this Contract signer, e.g. notary, 10428 * grantee.) 10429 */ 10430 public SignatoryComponent setType(Coding value) { 10431 this.type = value; 10432 return this; 10433 } 10434 10435 /** 10436 * @return {@link #party} (Party which is a signator to this Contract.) 10437 */ 10438 public Reference getParty() { 10439 if (this.party == null) 10440 if (Configuration.errorOnAutoCreate()) 10441 throw new Error("Attempt to auto-create SignatoryComponent.party"); 10442 else if (Configuration.doAutoCreate()) 10443 this.party = new Reference(); // cc 10444 return this.party; 10445 } 10446 10447 public boolean hasParty() { 10448 return this.party != null && !this.party.isEmpty(); 10449 } 10450 10451 /** 10452 * @param value {@link #party} (Party which is a signator to this Contract.) 10453 */ 10454 public SignatoryComponent setParty(Reference value) { 10455 this.party = value; 10456 return this; 10457 } 10458 10459 /** 10460 * @return {@link #party} The actual object that is the target of the reference. 10461 * The reference library doesn't populate this, but you can use it to 10462 * hold the resource if you resolve it. (Party which is a signator to 10463 * this Contract.) 10464 */ 10465 public Resource getPartyTarget() { 10466 return this.partyTarget; 10467 } 10468 10469 /** 10470 * @param value {@link #party} The actual object that is the target of the 10471 * reference. The reference library doesn't use these, but you can 10472 * use it to hold the resource if you resolve it. (Party which is a 10473 * signator to this Contract.) 10474 */ 10475 public SignatoryComponent setPartyTarget(Resource value) { 10476 this.partyTarget = value; 10477 return this; 10478 } 10479 10480 /** 10481 * @return {@link #signature} (Legally binding Contract DSIG signature contents 10482 * in Base64.) 10483 */ 10484 public List<Signature> getSignature() { 10485 if (this.signature == null) 10486 this.signature = new ArrayList<Signature>(); 10487 return this.signature; 10488 } 10489 10490 /** 10491 * @return Returns a reference to <code>this</code> for easy method chaining 10492 */ 10493 public SignatoryComponent setSignature(List<Signature> theSignature) { 10494 this.signature = theSignature; 10495 return this; 10496 } 10497 10498 public boolean hasSignature() { 10499 if (this.signature == null) 10500 return false; 10501 for (Signature item : this.signature) 10502 if (!item.isEmpty()) 10503 return true; 10504 return false; 10505 } 10506 10507 public Signature addSignature() { // 3 10508 Signature t = new Signature(); 10509 if (this.signature == null) 10510 this.signature = new ArrayList<Signature>(); 10511 this.signature.add(t); 10512 return t; 10513 } 10514 10515 public SignatoryComponent addSignature(Signature t) { // 3 10516 if (t == null) 10517 return this; 10518 if (this.signature == null) 10519 this.signature = new ArrayList<Signature>(); 10520 this.signature.add(t); 10521 return this; 10522 } 10523 10524 /** 10525 * @return The first repetition of repeating field {@link #signature}, creating 10526 * it if it does not already exist 10527 */ 10528 public Signature getSignatureFirstRep() { 10529 if (getSignature().isEmpty()) { 10530 addSignature(); 10531 } 10532 return getSignature().get(0); 10533 } 10534 10535 protected void listChildren(List<Property> children) { 10536 super.listChildren(children); 10537 children.add(new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, type)); 10538 children.add(new Property("party", "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 10539 "Party which is a signator to this Contract.", 0, 1, party)); 10540 children.add(new Property("signature", "Signature", "Legally binding Contract DSIG signature contents in Base64.", 10541 0, java.lang.Integer.MAX_VALUE, signature)); 10542 } 10543 10544 @Override 10545 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10546 switch (_hash) { 10547 case 3575610: 10548 /* type */ return new Property("type", "Coding", "Role of this Contract signer, e.g. notary, grantee.", 0, 1, 10549 type); 10550 case 106437350: 10551 /* party */ return new Property("party", 10552 "Reference(Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", 10553 "Party which is a signator to this Contract.", 0, 1, party); 10554 case 1073584312: 10555 /* signature */ return new Property("signature", "Signature", 10556 "Legally binding Contract DSIG signature contents in Base64.", 0, java.lang.Integer.MAX_VALUE, signature); 10557 default: 10558 return super.getNamedProperty(_hash, _name, _checkValid); 10559 } 10560 10561 } 10562 10563 @Override 10564 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10565 switch (hash) { 10566 case 3575610: 10567 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Coding 10568 case 106437350: 10569 /* party */ return this.party == null ? new Base[0] : new Base[] { this.party }; // Reference 10570 case 1073584312: 10571 /* signature */ return this.signature == null ? new Base[0] 10572 : this.signature.toArray(new Base[this.signature.size()]); // Signature 10573 default: 10574 return super.getProperty(hash, name, checkValid); 10575 } 10576 10577 } 10578 10579 @Override 10580 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10581 switch (hash) { 10582 case 3575610: // type 10583 this.type = castToCoding(value); // Coding 10584 return value; 10585 case 106437350: // party 10586 this.party = castToReference(value); // Reference 10587 return value; 10588 case 1073584312: // signature 10589 this.getSignature().add(castToSignature(value)); // Signature 10590 return value; 10591 default: 10592 return super.setProperty(hash, name, value); 10593 } 10594 10595 } 10596 10597 @Override 10598 public Base setProperty(String name, Base value) throws FHIRException { 10599 if (name.equals("type")) { 10600 this.type = castToCoding(value); // Coding 10601 } else if (name.equals("party")) { 10602 this.party = castToReference(value); // Reference 10603 } else if (name.equals("signature")) { 10604 this.getSignature().add(castToSignature(value)); 10605 } else 10606 return super.setProperty(name, value); 10607 return value; 10608 } 10609 10610 @Override 10611 public void removeChild(String name, Base value) throws FHIRException { 10612 if (name.equals("type")) { 10613 this.type = null; 10614 } else if (name.equals("party")) { 10615 this.party = null; 10616 } else if (name.equals("signature")) { 10617 this.getSignature().remove(castToSignature(value)); 10618 } else 10619 super.removeChild(name, value); 10620 10621 } 10622 10623 @Override 10624 public Base makeProperty(int hash, String name) throws FHIRException { 10625 switch (hash) { 10626 case 3575610: 10627 return getType(); 10628 case 106437350: 10629 return getParty(); 10630 case 1073584312: 10631 return addSignature(); 10632 default: 10633 return super.makeProperty(hash, name); 10634 } 10635 10636 } 10637 10638 @Override 10639 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10640 switch (hash) { 10641 case 3575610: 10642 /* type */ return new String[] { "Coding" }; 10643 case 106437350: 10644 /* party */ return new String[] { "Reference" }; 10645 case 1073584312: 10646 /* signature */ return new String[] { "Signature" }; 10647 default: 10648 return super.getTypesForProperty(hash, name); 10649 } 10650 10651 } 10652 10653 @Override 10654 public Base addChild(String name) throws FHIRException { 10655 if (name.equals("type")) { 10656 this.type = new Coding(); 10657 return this.type; 10658 } else if (name.equals("party")) { 10659 this.party = new Reference(); 10660 return this.party; 10661 } else if (name.equals("signature")) { 10662 return addSignature(); 10663 } else 10664 return super.addChild(name); 10665 } 10666 10667 public SignatoryComponent copy() { 10668 SignatoryComponent dst = new SignatoryComponent(); 10669 copyValues(dst); 10670 return dst; 10671 } 10672 10673 public void copyValues(SignatoryComponent dst) { 10674 super.copyValues(dst); 10675 dst.type = type == null ? null : type.copy(); 10676 dst.party = party == null ? null : party.copy(); 10677 if (signature != null) { 10678 dst.signature = new ArrayList<Signature>(); 10679 for (Signature i : signature) 10680 dst.signature.add(i.copy()); 10681 } 10682 ; 10683 } 10684 10685 @Override 10686 public boolean equalsDeep(Base other_) { 10687 if (!super.equalsDeep(other_)) 10688 return false; 10689 if (!(other_ instanceof SignatoryComponent)) 10690 return false; 10691 SignatoryComponent o = (SignatoryComponent) other_; 10692 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true) 10693 && compareDeep(signature, o.signature, true); 10694 } 10695 10696 @Override 10697 public boolean equalsShallow(Base other_) { 10698 if (!super.equalsShallow(other_)) 10699 return false; 10700 if (!(other_ instanceof SignatoryComponent)) 10701 return false; 10702 SignatoryComponent o = (SignatoryComponent) other_; 10703 return true; 10704 } 10705 10706 public boolean isEmpty() { 10707 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party, signature); 10708 } 10709 10710 public String fhirType() { 10711 return "Contract.signer"; 10712 10713 } 10714 10715 } 10716 10717 @Block() 10718 public static class FriendlyLanguageComponent extends BackboneElement implements IBaseBackboneElement { 10719 /** 10720 * Human readable rendering of this Contract in a format and representation 10721 * intended to enhance comprehension and ensure understandability. 10722 */ 10723 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 10724 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 10725 @Description(shortDefinition = "Easily comprehended representation of this Contract", formalDefinition = "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.") 10726 protected Type content; 10727 10728 private static final long serialVersionUID = -1763459053L; 10729 10730 /** 10731 * Constructor 10732 */ 10733 public FriendlyLanguageComponent() { 10734 super(); 10735 } 10736 10737 /** 10738 * Constructor 10739 */ 10740 public FriendlyLanguageComponent(Type content) { 10741 super(); 10742 this.content = content; 10743 } 10744 10745 /** 10746 * @return {@link #content} (Human readable rendering of this Contract in a 10747 * format and representation intended to enhance comprehension and 10748 * ensure understandability.) 10749 */ 10750 public Type getContent() { 10751 return this.content; 10752 } 10753 10754 /** 10755 * @return {@link #content} (Human readable rendering of this Contract in a 10756 * format and representation intended to enhance comprehension and 10757 * ensure understandability.) 10758 */ 10759 public Attachment getContentAttachment() throws FHIRException { 10760 if (this.content == null) 10761 this.content = new Attachment(); 10762 if (!(this.content instanceof Attachment)) 10763 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 10764 + this.content.getClass().getName() + " was encountered"); 10765 return (Attachment) this.content; 10766 } 10767 10768 public boolean hasContentAttachment() { 10769 return this.content instanceof Attachment; 10770 } 10771 10772 /** 10773 * @return {@link #content} (Human readable rendering of this Contract in a 10774 * format and representation intended to enhance comprehension and 10775 * ensure understandability.) 10776 */ 10777 public Reference getContentReference() throws FHIRException { 10778 if (this.content == null) 10779 this.content = new Reference(); 10780 if (!(this.content instanceof Reference)) 10781 throw new FHIRException("Type mismatch: the type Reference was expected, but " 10782 + this.content.getClass().getName() + " was encountered"); 10783 return (Reference) this.content; 10784 } 10785 10786 public boolean hasContentReference() { 10787 return this.content instanceof Reference; 10788 } 10789 10790 public boolean hasContent() { 10791 return this.content != null && !this.content.isEmpty(); 10792 } 10793 10794 /** 10795 * @param value {@link #content} (Human readable rendering of this Contract in a 10796 * format and representation intended to enhance comprehension and 10797 * ensure understandability.) 10798 */ 10799 public FriendlyLanguageComponent setContent(Type value) { 10800 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 10801 throw new Error("Not the right type for Contract.friendly.content[x]: " + value.fhirType()); 10802 this.content = value; 10803 return this; 10804 } 10805 10806 protected void listChildren(List<Property> children) { 10807 super.listChildren(children); 10808 children.add(new Property("content[x]", 10809 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10810 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10811 0, 1, content)); 10812 } 10813 10814 @Override 10815 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10816 switch (_hash) { 10817 case 264548711: 10818 /* content[x] */ return new Property("content[x]", 10819 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10820 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10821 0, 1, content); 10822 case 951530617: 10823 /* content */ return new Property("content[x]", 10824 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10825 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10826 0, 1, content); 10827 case -702028164: 10828 /* contentAttachment */ return new Property("content[x]", 10829 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10830 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10831 0, 1, content); 10832 case 1193747154: 10833 /* contentReference */ return new Property("content[x]", 10834 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 10835 "Human readable rendering of this Contract in a format and representation intended to enhance comprehension and ensure understandability.", 10836 0, 1, content); 10837 default: 10838 return super.getNamedProperty(_hash, _name, _checkValid); 10839 } 10840 10841 } 10842 10843 @Override 10844 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10845 switch (hash) { 10846 case 951530617: 10847 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 10848 default: 10849 return super.getProperty(hash, name, checkValid); 10850 } 10851 10852 } 10853 10854 @Override 10855 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10856 switch (hash) { 10857 case 951530617: // content 10858 this.content = castToType(value); // Type 10859 return value; 10860 default: 10861 return super.setProperty(hash, name, value); 10862 } 10863 10864 } 10865 10866 @Override 10867 public Base setProperty(String name, Base value) throws FHIRException { 10868 if (name.equals("content[x]")) { 10869 this.content = castToType(value); // Type 10870 } else 10871 return super.setProperty(name, value); 10872 return value; 10873 } 10874 10875 @Override 10876 public void removeChild(String name, Base value) throws FHIRException { 10877 if (name.equals("content[x]")) { 10878 this.content = null; 10879 } else 10880 super.removeChild(name, value); 10881 10882 } 10883 10884 @Override 10885 public Base makeProperty(int hash, String name) throws FHIRException { 10886 switch (hash) { 10887 case 264548711: 10888 return getContent(); 10889 case 951530617: 10890 return getContent(); 10891 default: 10892 return super.makeProperty(hash, name); 10893 } 10894 10895 } 10896 10897 @Override 10898 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10899 switch (hash) { 10900 case 951530617: 10901 /* content */ return new String[] { "Attachment", "Reference" }; 10902 default: 10903 return super.getTypesForProperty(hash, name); 10904 } 10905 10906 } 10907 10908 @Override 10909 public Base addChild(String name) throws FHIRException { 10910 if (name.equals("contentAttachment")) { 10911 this.content = new Attachment(); 10912 return this.content; 10913 } else if (name.equals("contentReference")) { 10914 this.content = new Reference(); 10915 return this.content; 10916 } else 10917 return super.addChild(name); 10918 } 10919 10920 public FriendlyLanguageComponent copy() { 10921 FriendlyLanguageComponent dst = new FriendlyLanguageComponent(); 10922 copyValues(dst); 10923 return dst; 10924 } 10925 10926 public void copyValues(FriendlyLanguageComponent dst) { 10927 super.copyValues(dst); 10928 dst.content = content == null ? null : content.copy(); 10929 } 10930 10931 @Override 10932 public boolean equalsDeep(Base other_) { 10933 if (!super.equalsDeep(other_)) 10934 return false; 10935 if (!(other_ instanceof FriendlyLanguageComponent)) 10936 return false; 10937 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 10938 return compareDeep(content, o.content, true); 10939 } 10940 10941 @Override 10942 public boolean equalsShallow(Base other_) { 10943 if (!super.equalsShallow(other_)) 10944 return false; 10945 if (!(other_ instanceof FriendlyLanguageComponent)) 10946 return false; 10947 FriendlyLanguageComponent o = (FriendlyLanguageComponent) other_; 10948 return true; 10949 } 10950 10951 public boolean isEmpty() { 10952 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 10953 } 10954 10955 public String fhirType() { 10956 return "Contract.friendly"; 10957 10958 } 10959 10960 } 10961 10962 @Block() 10963 public static class LegalLanguageComponent extends BackboneElement implements IBaseBackboneElement { 10964 /** 10965 * Contract legal text in human renderable form. 10966 */ 10967 @Child(name = "content", type = { Attachment.class, Composition.class, DocumentReference.class, 10968 QuestionnaireResponse.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 10969 @Description(shortDefinition = "Contract Legal Text", formalDefinition = "Contract legal text in human renderable form.") 10970 protected Type content; 10971 10972 private static final long serialVersionUID = -1763459053L; 10973 10974 /** 10975 * Constructor 10976 */ 10977 public LegalLanguageComponent() { 10978 super(); 10979 } 10980 10981 /** 10982 * Constructor 10983 */ 10984 public LegalLanguageComponent(Type content) { 10985 super(); 10986 this.content = content; 10987 } 10988 10989 /** 10990 * @return {@link #content} (Contract legal text in human renderable form.) 10991 */ 10992 public Type getContent() { 10993 return this.content; 10994 } 10995 10996 /** 10997 * @return {@link #content} (Contract legal text in human renderable form.) 10998 */ 10999 public Attachment getContentAttachment() throws FHIRException { 11000 if (this.content == null) 11001 this.content = new Attachment(); 11002 if (!(this.content instanceof Attachment)) 11003 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 11004 + this.content.getClass().getName() + " was encountered"); 11005 return (Attachment) this.content; 11006 } 11007 11008 public boolean hasContentAttachment() { 11009 return this.content instanceof Attachment; 11010 } 11011 11012 /** 11013 * @return {@link #content} (Contract legal text in human renderable form.) 11014 */ 11015 public Reference getContentReference() throws FHIRException { 11016 if (this.content == null) 11017 this.content = new Reference(); 11018 if (!(this.content instanceof Reference)) 11019 throw new FHIRException("Type mismatch: the type Reference was expected, but " 11020 + this.content.getClass().getName() + " was encountered"); 11021 return (Reference) this.content; 11022 } 11023 11024 public boolean hasContentReference() { 11025 return this.content instanceof Reference; 11026 } 11027 11028 public boolean hasContent() { 11029 return this.content != null && !this.content.isEmpty(); 11030 } 11031 11032 /** 11033 * @param value {@link #content} (Contract legal text in human renderable form.) 11034 */ 11035 public LegalLanguageComponent setContent(Type value) { 11036 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 11037 throw new Error("Not the right type for Contract.legal.content[x]: " + value.fhirType()); 11038 this.content = value; 11039 return this; 11040 } 11041 11042 protected void listChildren(List<Property> children) { 11043 super.listChildren(children); 11044 children 11045 .add(new Property("content[x]", "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11046 "Contract legal text in human renderable form.", 0, 1, content)); 11047 } 11048 11049 @Override 11050 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11051 switch (_hash) { 11052 case 264548711: 11053 /* content[x] */ return new Property("content[x]", 11054 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11055 "Contract legal text in human renderable form.", 0, 1, content); 11056 case 951530617: 11057 /* content */ return new Property("content[x]", 11058 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11059 "Contract legal text in human renderable form.", 0, 1, content); 11060 case -702028164: 11061 /* contentAttachment */ return new Property("content[x]", 11062 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11063 "Contract legal text in human renderable form.", 0, 1, content); 11064 case 1193747154: 11065 /* contentReference */ return new Property("content[x]", 11066 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse)", 11067 "Contract legal text in human renderable form.", 0, 1, content); 11068 default: 11069 return super.getNamedProperty(_hash, _name, _checkValid); 11070 } 11071 11072 } 11073 11074 @Override 11075 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11076 switch (hash) { 11077 case 951530617: 11078 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 11079 default: 11080 return super.getProperty(hash, name, checkValid); 11081 } 11082 11083 } 11084 11085 @Override 11086 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11087 switch (hash) { 11088 case 951530617: // content 11089 this.content = castToType(value); // Type 11090 return value; 11091 default: 11092 return super.setProperty(hash, name, value); 11093 } 11094 11095 } 11096 11097 @Override 11098 public Base setProperty(String name, Base value) throws FHIRException { 11099 if (name.equals("content[x]")) { 11100 this.content = castToType(value); // Type 11101 } else 11102 return super.setProperty(name, value); 11103 return value; 11104 } 11105 11106 @Override 11107 public void removeChild(String name, Base value) throws FHIRException { 11108 if (name.equals("content[x]")) { 11109 this.content = null; 11110 } else 11111 super.removeChild(name, value); 11112 11113 } 11114 11115 @Override 11116 public Base makeProperty(int hash, String name) throws FHIRException { 11117 switch (hash) { 11118 case 264548711: 11119 return getContent(); 11120 case 951530617: 11121 return getContent(); 11122 default: 11123 return super.makeProperty(hash, name); 11124 } 11125 11126 } 11127 11128 @Override 11129 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11130 switch (hash) { 11131 case 951530617: 11132 /* content */ return new String[] { "Attachment", "Reference" }; 11133 default: 11134 return super.getTypesForProperty(hash, name); 11135 } 11136 11137 } 11138 11139 @Override 11140 public Base addChild(String name) throws FHIRException { 11141 if (name.equals("contentAttachment")) { 11142 this.content = new Attachment(); 11143 return this.content; 11144 } else if (name.equals("contentReference")) { 11145 this.content = new Reference(); 11146 return this.content; 11147 } else 11148 return super.addChild(name); 11149 } 11150 11151 public LegalLanguageComponent copy() { 11152 LegalLanguageComponent dst = new LegalLanguageComponent(); 11153 copyValues(dst); 11154 return dst; 11155 } 11156 11157 public void copyValues(LegalLanguageComponent dst) { 11158 super.copyValues(dst); 11159 dst.content = content == null ? null : content.copy(); 11160 } 11161 11162 @Override 11163 public boolean equalsDeep(Base other_) { 11164 if (!super.equalsDeep(other_)) 11165 return false; 11166 if (!(other_ instanceof LegalLanguageComponent)) 11167 return false; 11168 LegalLanguageComponent o = (LegalLanguageComponent) other_; 11169 return compareDeep(content, o.content, true); 11170 } 11171 11172 @Override 11173 public boolean equalsShallow(Base other_) { 11174 if (!super.equalsShallow(other_)) 11175 return false; 11176 if (!(other_ instanceof LegalLanguageComponent)) 11177 return false; 11178 LegalLanguageComponent o = (LegalLanguageComponent) other_; 11179 return true; 11180 } 11181 11182 public boolean isEmpty() { 11183 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 11184 } 11185 11186 public String fhirType() { 11187 return "Contract.legal"; 11188 11189 } 11190 11191 } 11192 11193 @Block() 11194 public static class ComputableLanguageComponent extends BackboneElement implements IBaseBackboneElement { 11195 /** 11196 * Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, 11197 * SecPal). 11198 */ 11199 @Child(name = "content", type = { Attachment.class, 11200 DocumentReference.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 11201 @Description(shortDefinition = "Computable Contract Rules", formalDefinition = "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).") 11202 protected Type content; 11203 11204 private static final long serialVersionUID = -1763459053L; 11205 11206 /** 11207 * Constructor 11208 */ 11209 public ComputableLanguageComponent() { 11210 super(); 11211 } 11212 11213 /** 11214 * Constructor 11215 */ 11216 public ComputableLanguageComponent(Type content) { 11217 super(); 11218 this.content = content; 11219 } 11220 11221 /** 11222 * @return {@link #content} (Computable Contract conveyed using a policy rule 11223 * language (e.g. XACML, DKAL, SecPal).) 11224 */ 11225 public Type getContent() { 11226 return this.content; 11227 } 11228 11229 /** 11230 * @return {@link #content} (Computable Contract conveyed using a policy rule 11231 * language (e.g. XACML, DKAL, SecPal).) 11232 */ 11233 public Attachment getContentAttachment() throws FHIRException { 11234 if (this.content == null) 11235 this.content = new Attachment(); 11236 if (!(this.content instanceof Attachment)) 11237 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 11238 + this.content.getClass().getName() + " was encountered"); 11239 return (Attachment) this.content; 11240 } 11241 11242 public boolean hasContentAttachment() { 11243 return this.content instanceof Attachment; 11244 } 11245 11246 /** 11247 * @return {@link #content} (Computable Contract conveyed using a policy rule 11248 * language (e.g. XACML, DKAL, SecPal).) 11249 */ 11250 public Reference getContentReference() throws FHIRException { 11251 if (this.content == null) 11252 this.content = new Reference(); 11253 if (!(this.content instanceof Reference)) 11254 throw new FHIRException("Type mismatch: the type Reference was expected, but " 11255 + this.content.getClass().getName() + " was encountered"); 11256 return (Reference) this.content; 11257 } 11258 11259 public boolean hasContentReference() { 11260 return this.content instanceof Reference; 11261 } 11262 11263 public boolean hasContent() { 11264 return this.content != null && !this.content.isEmpty(); 11265 } 11266 11267 /** 11268 * @param value {@link #content} (Computable Contract conveyed using a policy 11269 * rule language (e.g. XACML, DKAL, SecPal).) 11270 */ 11271 public ComputableLanguageComponent setContent(Type value) { 11272 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 11273 throw new Error("Not the right type for Contract.rule.content[x]: " + value.fhirType()); 11274 this.content = value; 11275 return this; 11276 } 11277 11278 protected void listChildren(List<Property> children) { 11279 super.listChildren(children); 11280 children.add(new Property("content[x]", "Attachment|Reference(DocumentReference)", 11281 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content)); 11282 } 11283 11284 @Override 11285 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11286 switch (_hash) { 11287 case 264548711: 11288 /* content[x] */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11289 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11290 case 951530617: 11291 /* content */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11292 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11293 case -702028164: 11294 /* contentAttachment */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11295 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11296 case 1193747154: 11297 /* contentReference */ return new Property("content[x]", "Attachment|Reference(DocumentReference)", 11298 "Computable Contract conveyed using a policy rule language (e.g. XACML, DKAL, SecPal).", 0, 1, content); 11299 default: 11300 return super.getNamedProperty(_hash, _name, _checkValid); 11301 } 11302 11303 } 11304 11305 @Override 11306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11307 switch (hash) { 11308 case 951530617: 11309 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Type 11310 default: 11311 return super.getProperty(hash, name, checkValid); 11312 } 11313 11314 } 11315 11316 @Override 11317 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11318 switch (hash) { 11319 case 951530617: // content 11320 this.content = castToType(value); // Type 11321 return value; 11322 default: 11323 return super.setProperty(hash, name, value); 11324 } 11325 11326 } 11327 11328 @Override 11329 public Base setProperty(String name, Base value) throws FHIRException { 11330 if (name.equals("content[x]")) { 11331 this.content = castToType(value); // Type 11332 } else 11333 return super.setProperty(name, value); 11334 return value; 11335 } 11336 11337 @Override 11338 public void removeChild(String name, Base value) throws FHIRException { 11339 if (name.equals("content[x]")) { 11340 this.content = null; 11341 } else 11342 super.removeChild(name, value); 11343 11344 } 11345 11346 @Override 11347 public Base makeProperty(int hash, String name) throws FHIRException { 11348 switch (hash) { 11349 case 264548711: 11350 return getContent(); 11351 case 951530617: 11352 return getContent(); 11353 default: 11354 return super.makeProperty(hash, name); 11355 } 11356 11357 } 11358 11359 @Override 11360 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11361 switch (hash) { 11362 case 951530617: 11363 /* content */ return new String[] { "Attachment", "Reference" }; 11364 default: 11365 return super.getTypesForProperty(hash, name); 11366 } 11367 11368 } 11369 11370 @Override 11371 public Base addChild(String name) throws FHIRException { 11372 if (name.equals("contentAttachment")) { 11373 this.content = new Attachment(); 11374 return this.content; 11375 } else if (name.equals("contentReference")) { 11376 this.content = new Reference(); 11377 return this.content; 11378 } else 11379 return super.addChild(name); 11380 } 11381 11382 public ComputableLanguageComponent copy() { 11383 ComputableLanguageComponent dst = new ComputableLanguageComponent(); 11384 copyValues(dst); 11385 return dst; 11386 } 11387 11388 public void copyValues(ComputableLanguageComponent dst) { 11389 super.copyValues(dst); 11390 dst.content = content == null ? null : content.copy(); 11391 } 11392 11393 @Override 11394 public boolean equalsDeep(Base other_) { 11395 if (!super.equalsDeep(other_)) 11396 return false; 11397 if (!(other_ instanceof ComputableLanguageComponent)) 11398 return false; 11399 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 11400 return compareDeep(content, o.content, true); 11401 } 11402 11403 @Override 11404 public boolean equalsShallow(Base other_) { 11405 if (!super.equalsShallow(other_)) 11406 return false; 11407 if (!(other_ instanceof ComputableLanguageComponent)) 11408 return false; 11409 ComputableLanguageComponent o = (ComputableLanguageComponent) other_; 11410 return true; 11411 } 11412 11413 public boolean isEmpty() { 11414 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(content); 11415 } 11416 11417 public String fhirType() { 11418 return "Contract.rule"; 11419 11420 } 11421 11422 } 11423 11424 /** 11425 * Unique identifier for this Contract or a derivative that references a Source 11426 * Contract. 11427 */ 11428 @Child(name = "identifier", type = { 11429 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11430 @Description(shortDefinition = "Contract number", formalDefinition = "Unique identifier for this Contract or a derivative that references a Source Contract.") 11431 protected List<Identifier> identifier; 11432 11433 /** 11434 * Canonical identifier for this contract, represented as a URI (globally 11435 * unique). 11436 */ 11437 @Child(name = "url", type = { UriType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 11438 @Description(shortDefinition = "Basal definition", formalDefinition = "Canonical identifier for this contract, represented as a URI (globally unique).") 11439 protected UriType url; 11440 11441 /** 11442 * An edition identifier used for business purposes to label business 11443 * significant variants. 11444 */ 11445 @Child(name = "version", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 11446 @Description(shortDefinition = "Business edition", formalDefinition = "An edition identifier used for business purposes to label business significant variants.") 11447 protected StringType version; 11448 11449 /** 11450 * The status of the resource instance. 11451 */ 11452 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 11453 @Description(shortDefinition = "amended | appended | cancelled | disputed | entered-in-error | executable | executed | negotiable | offered | policy | rejected | renewed | revoked | resolved | terminated", formalDefinition = "The status of the resource instance.") 11454 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-status") 11455 protected Enumeration<ContractStatus> status; 11456 11457 /** 11458 * Legal states of the formation of a legal instrument, which is a formally 11459 * executed written document that can be formally attributed to its author, 11460 * records and formally expresses a legally enforceable act, process, or 11461 * contractual duty, obligation, or right, and therefore evidences that act, 11462 * process, or agreement. 11463 */ 11464 @Child(name = "legalState", type = { 11465 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 11466 @Description(shortDefinition = "Negotiation status", formalDefinition = "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.") 11467 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-legalstate") 11468 protected CodeableConcept legalState; 11469 11470 /** 11471 * The URL pointing to a FHIR-defined Contract Definition that is adhered to in 11472 * whole or part by this Contract. 11473 */ 11474 @Child(name = "instantiatesCanonical", type = { 11475 Contract.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 11476 @Description(shortDefinition = "Source Contract Definition", formalDefinition = "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.") 11477 protected Reference instantiatesCanonical; 11478 11479 /** 11480 * The actual object that is the target of the reference (The URL pointing to a 11481 * FHIR-defined Contract Definition that is adhered to in whole or part by this 11482 * Contract.) 11483 */ 11484 protected Contract instantiatesCanonicalTarget; 11485 11486 /** 11487 * The URL pointing to an externally maintained definition that is adhered to in 11488 * whole or in part by this Contract. 11489 */ 11490 @Child(name = "instantiatesUri", type = { 11491 UriType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 11492 @Description(shortDefinition = "External Contract Definition", formalDefinition = "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.") 11493 protected UriType instantiatesUri; 11494 11495 /** 11496 * The minimal content derived from the basal information source at a specific 11497 * stage in its lifecycle. 11498 */ 11499 @Child(name = "contentDerivative", type = { 11500 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 11501 @Description(shortDefinition = "Content derived from the basal information", formalDefinition = "The minimal content derived from the basal information source at a specific stage in its lifecycle.") 11502 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-content-derivative") 11503 protected CodeableConcept contentDerivative; 11504 11505 /** 11506 * When this Contract was issued. 11507 */ 11508 @Child(name = "issued", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 11509 @Description(shortDefinition = "When this Contract was issued", formalDefinition = "When this Contract was issued.") 11510 protected DateTimeType issued; 11511 11512 /** 11513 * Relevant time or time-period when this Contract is applicable. 11514 */ 11515 @Child(name = "applies", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 11516 @Description(shortDefinition = "Effective time", formalDefinition = "Relevant time or time-period when this Contract is applicable.") 11517 protected Period applies; 11518 11519 /** 11520 * Event resulting in discontinuation or termination of this Contract instance 11521 * by one or more parties to the contract. 11522 */ 11523 @Child(name = "expirationType", type = { 11524 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 11525 @Description(shortDefinition = "Contract cessation cause", formalDefinition = "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.") 11526 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-expiration-type") 11527 protected CodeableConcept expirationType; 11528 11529 /** 11530 * The target entity impacted by or of interest to parties to the agreement. 11531 */ 11532 @Child(name = "subject", type = { 11533 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11534 @Description(shortDefinition = "Contract Target Entity", formalDefinition = "The target entity impacted by or of interest to parties to the agreement.") 11535 protected List<Reference> subject; 11536 /** 11537 * The actual objects that are the target of the reference (The target entity 11538 * impacted by or of interest to parties to the agreement.) 11539 */ 11540 protected List<Resource> subjectTarget; 11541 11542 /** 11543 * A formally or informally recognized grouping of people, principals, 11544 * organizations, or jurisdictions formed for the purpose of achieving some form 11545 * of collective action such as the promulgation, administration and enforcement 11546 * of contracts and policies. 11547 */ 11548 @Child(name = "authority", type = { 11549 Organization.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11550 @Description(shortDefinition = "Authority under which this Contract has standing", formalDefinition = "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.") 11551 protected List<Reference> authority; 11552 /** 11553 * The actual objects that are the target of the reference (A formally or 11554 * informally recognized grouping of people, principals, organizations, or 11555 * jurisdictions formed for the purpose of achieving some form of collective 11556 * action such as the promulgation, administration and enforcement of contracts 11557 * and policies.) 11558 */ 11559 protected List<Organization> authorityTarget; 11560 11561 /** 11562 * Recognized governance framework or system operating with a circumscribed 11563 * scope in accordance with specified principles, policies, processes or 11564 * procedures for managing rights, actions, or behaviors of parties or 11565 * principals relative to resources. 11566 */ 11567 @Child(name = "domain", type = { 11568 Location.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11569 @Description(shortDefinition = "A sphere of control governed by an authoritative jurisdiction, organization, or person", formalDefinition = "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.") 11570 protected List<Reference> domain; 11571 /** 11572 * The actual objects that are the target of the reference (Recognized 11573 * governance framework or system operating with a circumscribed scope in 11574 * accordance with specified principles, policies, processes or procedures for 11575 * managing rights, actions, or behaviors of parties or principals relative to 11576 * resources.) 11577 */ 11578 protected List<Location> domainTarget; 11579 11580 /** 11581 * Sites in which the contract is complied with, exercised, or in force. 11582 */ 11583 @Child(name = "site", type = { 11584 Location.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11585 @Description(shortDefinition = "Specific Location", formalDefinition = "Sites in which the contract is complied with, exercised, or in force.") 11586 protected List<Reference> site; 11587 /** 11588 * The actual objects that are the target of the reference (Sites in which the 11589 * contract is complied with, exercised, or in force.) 11590 */ 11591 protected List<Location> siteTarget; 11592 11593 /** 11594 * A natural language name identifying this Contract definition, derivative, or 11595 * instance in any legal state. Provides additional information about its 11596 * content. This name should be usable as an identifier for the module by 11597 * machine processing applications such as code generation. 11598 */ 11599 @Child(name = "name", type = { StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 11600 @Description(shortDefinition = "Computer friendly designation", formalDefinition = "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.") 11601 protected StringType name; 11602 11603 /** 11604 * A short, descriptive, user-friendly title for this Contract definition, 11605 * derivative, or instance in any legal state.t giving additional information 11606 * about its content. 11607 */ 11608 @Child(name = "title", type = { StringType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 11609 @Description(shortDefinition = "Human Friendly name", formalDefinition = "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.") 11610 protected StringType title; 11611 11612 /** 11613 * An explanatory or alternate user-friendly title for this Contract definition, 11614 * derivative, or instance in any legal state.t giving additional information 11615 * about its content. 11616 */ 11617 @Child(name = "subtitle", type = { 11618 StringType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 11619 @Description(shortDefinition = "Subordinate Friendly name", formalDefinition = "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.") 11620 protected StringType subtitle; 11621 11622 /** 11623 * Alternative representation of the title for this Contract definition, 11624 * derivative, or instance in any legal state., e.g., a domain specific contract 11625 * number related to legislation. 11626 */ 11627 @Child(name = "alias", type = { 11628 StringType.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11629 @Description(shortDefinition = "Acronym or short name", formalDefinition = "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.") 11630 protected List<StringType> alias; 11631 11632 /** 11633 * The individual or organization that authored the Contract definition, 11634 * derivative, or instance in any legal state. 11635 */ 11636 @Child(name = "author", type = { Patient.class, Practitioner.class, PractitionerRole.class, 11637 Organization.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 11638 @Description(shortDefinition = "Source of Contract", formalDefinition = "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.") 11639 protected Reference author; 11640 11641 /** 11642 * The actual object that is the target of the reference (The individual or 11643 * organization that authored the Contract definition, derivative, or instance 11644 * in any legal state.) 11645 */ 11646 protected Resource authorTarget; 11647 11648 /** 11649 * A selector of legal concerns for this Contract definition, derivative, or 11650 * instance in any legal state. 11651 */ 11652 @Child(name = "scope", type = { 11653 CodeableConcept.class }, order = 20, min = 0, max = 1, modifier = false, summary = false) 11654 @Description(shortDefinition = "Range of Legal Concerns", formalDefinition = "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.") 11655 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-scope") 11656 protected CodeableConcept scope; 11657 11658 /** 11659 * Narrows the range of legal concerns to focus on the achievement of specific 11660 * contractual objectives. 11661 */ 11662 @Child(name = "topic", type = { CodeableConcept.class, 11663 Reference.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 11664 @Description(shortDefinition = "Focus of contract interest", formalDefinition = "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.") 11665 protected Type topic; 11666 11667 /** 11668 * A high-level category for the legal instrument, whether constructed as a 11669 * Contract definition, derivative, or instance in any legal state. Provides 11670 * additional information about its content within the context of the Contract's 11671 * scope to distinguish the kinds of systems that would be interested in the 11672 * contract. 11673 */ 11674 @Child(name = "type", type = { 11675 CodeableConcept.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 11676 @Description(shortDefinition = "Legal instrument category", formalDefinition = "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.") 11677 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-type") 11678 protected CodeableConcept type; 11679 11680 /** 11681 * Sub-category for the Contract that distinguishes the kinds of systems that 11682 * would be interested in the Contract within the context of the Contract's 11683 * scope. 11684 */ 11685 @Child(name = "subType", type = { 11686 CodeableConcept.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 11687 @Description(shortDefinition = "Subtype within the context of type", formalDefinition = "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.") 11688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contract-subtype") 11689 protected List<CodeableConcept> subType; 11690 11691 /** 11692 * Precusory content developed with a focus and intent of supporting the 11693 * formation a Contract instance, which may be associated with and transformable 11694 * into a Contract. 11695 */ 11696 @Child(name = "contentDefinition", type = {}, order = 24, min = 0, max = 1, modifier = false, summary = false) 11697 @Description(shortDefinition = "Contract precursor content", formalDefinition = "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.") 11698 protected ContentDefinitionComponent contentDefinition; 11699 11700 /** 11701 * One or more Contract Provisions, which may be related and conveyed as a 11702 * group, and may contain nested groups. 11703 */ 11704 @Child(name = "term", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11705 @Description(shortDefinition = "Contract Term List", formalDefinition = "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.") 11706 protected List<TermComponent> term; 11707 11708 /** 11709 * Information that may be needed by/relevant to the performer in their 11710 * execution of this term action. 11711 */ 11712 @Child(name = "supportingInfo", type = { 11713 Reference.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11714 @Description(shortDefinition = "Extra Information", formalDefinition = "Information that may be needed by/relevant to the performer in their execution of this term action.") 11715 protected List<Reference> supportingInfo; 11716 /** 11717 * The actual objects that are the target of the reference (Information that may 11718 * be needed by/relevant to the performer in their execution of this term 11719 * action.) 11720 */ 11721 protected List<Resource> supportingInfoTarget; 11722 11723 /** 11724 * Links to Provenance records for past versions of this Contract definition, 11725 * derivative, or instance, which identify key state transitions or updates that 11726 * are likely to be relevant to a user looking at the current version of the 11727 * Contract. The Provence.entity indicates the target that was changed in the 11728 * update. http://build.fhir.org/provenance-definitions.html#Provenance.entity. 11729 */ 11730 @Child(name = "relevantHistory", type = { 11731 Provenance.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11732 @Description(shortDefinition = "Key event in Contract History", formalDefinition = "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.") 11733 protected List<Reference> relevantHistory; 11734 /** 11735 * The actual objects that are the target of the reference (Links to Provenance 11736 * records for past versions of this Contract definition, derivative, or 11737 * instance, which identify key state transitions or updates that are likely to 11738 * be relevant to a user looking at the current version of the Contract. The 11739 * Provence.entity indicates the target that was changed in the update. 11740 * http://build.fhir.org/provenance-definitions.html#Provenance.entity.) 11741 */ 11742 protected List<Provenance> relevantHistoryTarget; 11743 11744 /** 11745 * Parties with legal standing in the Contract, including the principal parties, 11746 * the grantor(s) and grantee(s), which are any person or organization bound by 11747 * the contract, and any ancillary parties, which facilitate the execution of 11748 * the contract such as a notary or witness. 11749 */ 11750 @Child(name = "signer", type = {}, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11751 @Description(shortDefinition = "Contract Signatory", formalDefinition = "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.") 11752 protected List<SignatoryComponent> signer; 11753 11754 /** 11755 * The "patient friendly language" versionof the Contract in whole or in parts. 11756 * "Patient friendly language" means the representation of the Contract and 11757 * Contract Provisions in a manner that is readily accessible and understandable 11758 * by a layperson in accordance with best practices for communication styles 11759 * that ensure that those agreeing to or signing the Contract understand the 11760 * roles, actions, obligations, responsibilities, and implication of the 11761 * agreement. 11762 */ 11763 @Child(name = "friendly", type = {}, order = 29, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11764 @Description(shortDefinition = "Contract Friendly Language", formalDefinition = "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.") 11765 protected List<FriendlyLanguageComponent> friendly; 11766 11767 /** 11768 * List of Legal expressions or representations of this Contract. 11769 */ 11770 @Child(name = "legal", type = {}, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11771 @Description(shortDefinition = "Contract Legal Language", formalDefinition = "List of Legal expressions or representations of this Contract.") 11772 protected List<LegalLanguageComponent> legal; 11773 11774 /** 11775 * List of Computable Policy Rule Language Representations of this Contract. 11776 */ 11777 @Child(name = "rule", type = {}, order = 31, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 11778 @Description(shortDefinition = "Computable Contract Language", formalDefinition = "List of Computable Policy Rule Language Representations of this Contract.") 11779 protected List<ComputableLanguageComponent> rule; 11780 11781 /** 11782 * Legally binding Contract: This is the signed and legally recognized 11783 * representation of the Contract, which is considered the "source of truth" and 11784 * which would be the basis for legal action related to enforcement of this 11785 * Contract. 11786 */ 11787 @Child(name = "legallyBinding", type = { Attachment.class, Composition.class, DocumentReference.class, 11788 QuestionnaireResponse.class, Contract.class }, order = 32, min = 0, max = 1, modifier = false, summary = false) 11789 @Description(shortDefinition = "Binding Contract", formalDefinition = "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.") 11790 protected Type legallyBinding; 11791 11792 private static final long serialVersionUID = -1388892487L; 11793 11794 /** 11795 * Constructor 11796 */ 11797 public Contract() { 11798 super(); 11799 } 11800 11801 /** 11802 * @return {@link #identifier} (Unique identifier for this Contract or a 11803 * derivative that references a Source Contract.) 11804 */ 11805 public List<Identifier> getIdentifier() { 11806 if (this.identifier == null) 11807 this.identifier = new ArrayList<Identifier>(); 11808 return this.identifier; 11809 } 11810 11811 /** 11812 * @return Returns a reference to <code>this</code> for easy method chaining 11813 */ 11814 public Contract setIdentifier(List<Identifier> theIdentifier) { 11815 this.identifier = theIdentifier; 11816 return this; 11817 } 11818 11819 public boolean hasIdentifier() { 11820 if (this.identifier == null) 11821 return false; 11822 for (Identifier item : this.identifier) 11823 if (!item.isEmpty()) 11824 return true; 11825 return false; 11826 } 11827 11828 public Identifier addIdentifier() { // 3 11829 Identifier t = new Identifier(); 11830 if (this.identifier == null) 11831 this.identifier = new ArrayList<Identifier>(); 11832 this.identifier.add(t); 11833 return t; 11834 } 11835 11836 public Contract addIdentifier(Identifier t) { // 3 11837 if (t == null) 11838 return this; 11839 if (this.identifier == null) 11840 this.identifier = new ArrayList<Identifier>(); 11841 this.identifier.add(t); 11842 return this; 11843 } 11844 11845 /** 11846 * @return The first repetition of repeating field {@link #identifier}, creating 11847 * it if it does not already exist 11848 */ 11849 public Identifier getIdentifierFirstRep() { 11850 if (getIdentifier().isEmpty()) { 11851 addIdentifier(); 11852 } 11853 return getIdentifier().get(0); 11854 } 11855 11856 /** 11857 * @return {@link #url} (Canonical identifier for this contract, represented as 11858 * a URI (globally unique).). This is the underlying object with id, 11859 * value and extensions. The accessor "getUrl" gives direct access to 11860 * the value 11861 */ 11862 public UriType getUrlElement() { 11863 if (this.url == null) 11864 if (Configuration.errorOnAutoCreate()) 11865 throw new Error("Attempt to auto-create Contract.url"); 11866 else if (Configuration.doAutoCreate()) 11867 this.url = new UriType(); // bb 11868 return this.url; 11869 } 11870 11871 public boolean hasUrlElement() { 11872 return this.url != null && !this.url.isEmpty(); 11873 } 11874 11875 public boolean hasUrl() { 11876 return this.url != null && !this.url.isEmpty(); 11877 } 11878 11879 /** 11880 * @param value {@link #url} (Canonical identifier for this contract, 11881 * represented as a URI (globally unique).). This is the underlying 11882 * object with id, value and extensions. The accessor "getUrl" 11883 * gives direct access to the value 11884 */ 11885 public Contract setUrlElement(UriType value) { 11886 this.url = value; 11887 return this; 11888 } 11889 11890 /** 11891 * @return Canonical identifier for this contract, represented as a URI 11892 * (globally unique). 11893 */ 11894 public String getUrl() { 11895 return this.url == null ? null : this.url.getValue(); 11896 } 11897 11898 /** 11899 * @param value Canonical identifier for this contract, represented as a URI 11900 * (globally unique). 11901 */ 11902 public Contract setUrl(String value) { 11903 if (Utilities.noString(value)) 11904 this.url = null; 11905 else { 11906 if (this.url == null) 11907 this.url = new UriType(); 11908 this.url.setValue(value); 11909 } 11910 return this; 11911 } 11912 11913 /** 11914 * @return {@link #version} (An edition identifier used for business purposes to 11915 * label business significant variants.). This is the underlying object 11916 * with id, value and extensions. The accessor "getVersion" gives direct 11917 * access to the value 11918 */ 11919 public StringType getVersionElement() { 11920 if (this.version == null) 11921 if (Configuration.errorOnAutoCreate()) 11922 throw new Error("Attempt to auto-create Contract.version"); 11923 else if (Configuration.doAutoCreate()) 11924 this.version = new StringType(); // bb 11925 return this.version; 11926 } 11927 11928 public boolean hasVersionElement() { 11929 return this.version != null && !this.version.isEmpty(); 11930 } 11931 11932 public boolean hasVersion() { 11933 return this.version != null && !this.version.isEmpty(); 11934 } 11935 11936 /** 11937 * @param value {@link #version} (An edition identifier used for business 11938 * purposes to label business significant variants.). This is the 11939 * underlying object with id, value and extensions. The accessor 11940 * "getVersion" gives direct access to the value 11941 */ 11942 public Contract setVersionElement(StringType value) { 11943 this.version = value; 11944 return this; 11945 } 11946 11947 /** 11948 * @return An edition identifier used for business purposes to label business 11949 * significant variants. 11950 */ 11951 public String getVersion() { 11952 return this.version == null ? null : this.version.getValue(); 11953 } 11954 11955 /** 11956 * @param value An edition identifier used for business purposes to label 11957 * business significant variants. 11958 */ 11959 public Contract setVersion(String value) { 11960 if (Utilities.noString(value)) 11961 this.version = null; 11962 else { 11963 if (this.version == null) 11964 this.version = new StringType(); 11965 this.version.setValue(value); 11966 } 11967 return this; 11968 } 11969 11970 /** 11971 * @return {@link #status} (The status of the resource instance.). This is the 11972 * underlying object with id, value and extensions. The accessor 11973 * "getStatus" gives direct access to the value 11974 */ 11975 public Enumeration<ContractStatus> getStatusElement() { 11976 if (this.status == null) 11977 if (Configuration.errorOnAutoCreate()) 11978 throw new Error("Attempt to auto-create Contract.status"); 11979 else if (Configuration.doAutoCreate()) 11980 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); // bb 11981 return this.status; 11982 } 11983 11984 public boolean hasStatusElement() { 11985 return this.status != null && !this.status.isEmpty(); 11986 } 11987 11988 public boolean hasStatus() { 11989 return this.status != null && !this.status.isEmpty(); 11990 } 11991 11992 /** 11993 * @param value {@link #status} (The status of the resource instance.). This is 11994 * the underlying object with id, value and extensions. The 11995 * accessor "getStatus" gives direct access to the value 11996 */ 11997 public Contract setStatusElement(Enumeration<ContractStatus> value) { 11998 this.status = value; 11999 return this; 12000 } 12001 12002 /** 12003 * @return The status of the resource instance. 12004 */ 12005 public ContractStatus getStatus() { 12006 return this.status == null ? null : this.status.getValue(); 12007 } 12008 12009 /** 12010 * @param value The status of the resource instance. 12011 */ 12012 public Contract setStatus(ContractStatus value) { 12013 if (value == null) 12014 this.status = null; 12015 else { 12016 if (this.status == null) 12017 this.status = new Enumeration<ContractStatus>(new ContractStatusEnumFactory()); 12018 this.status.setValue(value); 12019 } 12020 return this; 12021 } 12022 12023 /** 12024 * @return {@link #legalState} (Legal states of the formation of a legal 12025 * instrument, which is a formally executed written document that can be 12026 * formally attributed to its author, records and formally expresses a 12027 * legally enforceable act, process, or contractual duty, obligation, or 12028 * right, and therefore evidences that act, process, or agreement.) 12029 */ 12030 public CodeableConcept getLegalState() { 12031 if (this.legalState == null) 12032 if (Configuration.errorOnAutoCreate()) 12033 throw new Error("Attempt to auto-create Contract.legalState"); 12034 else if (Configuration.doAutoCreate()) 12035 this.legalState = new CodeableConcept(); // cc 12036 return this.legalState; 12037 } 12038 12039 public boolean hasLegalState() { 12040 return this.legalState != null && !this.legalState.isEmpty(); 12041 } 12042 12043 /** 12044 * @param value {@link #legalState} (Legal states of the formation of a legal 12045 * instrument, which is a formally executed written document that 12046 * can be formally attributed to its author, records and formally 12047 * expresses a legally enforceable act, process, or contractual 12048 * duty, obligation, or right, and therefore evidences that act, 12049 * process, or agreement.) 12050 */ 12051 public Contract setLegalState(CodeableConcept value) { 12052 this.legalState = value; 12053 return this; 12054 } 12055 12056 /** 12057 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 12058 * Contract Definition that is adhered to in whole or part by this 12059 * Contract.) 12060 */ 12061 public Reference getInstantiatesCanonical() { 12062 if (this.instantiatesCanonical == null) 12063 if (Configuration.errorOnAutoCreate()) 12064 throw new Error("Attempt to auto-create Contract.instantiatesCanonical"); 12065 else if (Configuration.doAutoCreate()) 12066 this.instantiatesCanonical = new Reference(); // cc 12067 return this.instantiatesCanonical; 12068 } 12069 12070 public boolean hasInstantiatesCanonical() { 12071 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 12072 } 12073 12074 /** 12075 * @param value {@link #instantiatesCanonical} (The URL pointing to a 12076 * FHIR-defined Contract Definition that is adhered to in whole or 12077 * part by this Contract.) 12078 */ 12079 public Contract setInstantiatesCanonical(Reference value) { 12080 this.instantiatesCanonical = value; 12081 return this; 12082 } 12083 12084 /** 12085 * @return {@link #instantiatesCanonical} The actual object that is the target 12086 * of the reference. The reference library doesn't populate this, but 12087 * you can use it to hold the resource if you resolve it. (The URL 12088 * pointing to a FHIR-defined Contract Definition that is adhered to in 12089 * whole or part by this Contract.) 12090 */ 12091 public Contract getInstantiatesCanonicalTarget() { 12092 if (this.instantiatesCanonicalTarget == null) 12093 if (Configuration.errorOnAutoCreate()) 12094 throw new Error("Attempt to auto-create Contract.instantiatesCanonical"); 12095 else if (Configuration.doAutoCreate()) 12096 this.instantiatesCanonicalTarget = new Contract(); // aa 12097 return this.instantiatesCanonicalTarget; 12098 } 12099 12100 /** 12101 * @param value {@link #instantiatesCanonical} The actual object that is the 12102 * target of the reference. The reference library doesn't use 12103 * these, but you can use it to hold the resource if you resolve 12104 * it. (The URL pointing to a FHIR-defined Contract Definition that 12105 * is adhered to in whole or part by this Contract.) 12106 */ 12107 public Contract setInstantiatesCanonicalTarget(Contract value) { 12108 this.instantiatesCanonicalTarget = value; 12109 return this; 12110 } 12111 12112 /** 12113 * @return {@link #instantiatesUri} (The URL pointing to an externally 12114 * maintained definition that is adhered to in whole or in part by this 12115 * Contract.). This is the underlying object with id, value and 12116 * extensions. The accessor "getInstantiatesUri" gives direct access to 12117 * the value 12118 */ 12119 public UriType getInstantiatesUriElement() { 12120 if (this.instantiatesUri == null) 12121 if (Configuration.errorOnAutoCreate()) 12122 throw new Error("Attempt to auto-create Contract.instantiatesUri"); 12123 else if (Configuration.doAutoCreate()) 12124 this.instantiatesUri = new UriType(); // bb 12125 return this.instantiatesUri; 12126 } 12127 12128 public boolean hasInstantiatesUriElement() { 12129 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 12130 } 12131 12132 public boolean hasInstantiatesUri() { 12133 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 12134 } 12135 12136 /** 12137 * @param value {@link #instantiatesUri} (The URL pointing to an externally 12138 * maintained definition that is adhered to in whole or in part by 12139 * this Contract.). This is the underlying object with id, value 12140 * and extensions. The accessor "getInstantiatesUri" gives direct 12141 * access to the value 12142 */ 12143 public Contract setInstantiatesUriElement(UriType value) { 12144 this.instantiatesUri = value; 12145 return this; 12146 } 12147 12148 /** 12149 * @return The URL pointing to an externally maintained definition that is 12150 * adhered to in whole or in part by this Contract. 12151 */ 12152 public String getInstantiatesUri() { 12153 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 12154 } 12155 12156 /** 12157 * @param value The URL pointing to an externally maintained definition that is 12158 * adhered to in whole or in part by this Contract. 12159 */ 12160 public Contract setInstantiatesUri(String value) { 12161 if (Utilities.noString(value)) 12162 this.instantiatesUri = null; 12163 else { 12164 if (this.instantiatesUri == null) 12165 this.instantiatesUri = new UriType(); 12166 this.instantiatesUri.setValue(value); 12167 } 12168 return this; 12169 } 12170 12171 /** 12172 * @return {@link #contentDerivative} (The minimal content derived from the 12173 * basal information source at a specific stage in its lifecycle.) 12174 */ 12175 public CodeableConcept getContentDerivative() { 12176 if (this.contentDerivative == null) 12177 if (Configuration.errorOnAutoCreate()) 12178 throw new Error("Attempt to auto-create Contract.contentDerivative"); 12179 else if (Configuration.doAutoCreate()) 12180 this.contentDerivative = new CodeableConcept(); // cc 12181 return this.contentDerivative; 12182 } 12183 12184 public boolean hasContentDerivative() { 12185 return this.contentDerivative != null && !this.contentDerivative.isEmpty(); 12186 } 12187 12188 /** 12189 * @param value {@link #contentDerivative} (The minimal content derived from the 12190 * basal information source at a specific stage in its lifecycle.) 12191 */ 12192 public Contract setContentDerivative(CodeableConcept value) { 12193 this.contentDerivative = value; 12194 return this; 12195 } 12196 12197 /** 12198 * @return {@link #issued} (When this Contract was issued.). This is the 12199 * underlying object with id, value and extensions. The accessor 12200 * "getIssued" gives direct access to the value 12201 */ 12202 public DateTimeType getIssuedElement() { 12203 if (this.issued == null) 12204 if (Configuration.errorOnAutoCreate()) 12205 throw new Error("Attempt to auto-create Contract.issued"); 12206 else if (Configuration.doAutoCreate()) 12207 this.issued = new DateTimeType(); // bb 12208 return this.issued; 12209 } 12210 12211 public boolean hasIssuedElement() { 12212 return this.issued != null && !this.issued.isEmpty(); 12213 } 12214 12215 public boolean hasIssued() { 12216 return this.issued != null && !this.issued.isEmpty(); 12217 } 12218 12219 /** 12220 * @param value {@link #issued} (When this Contract was issued.). This is the 12221 * underlying object with id, value and extensions. The accessor 12222 * "getIssued" gives direct access to the value 12223 */ 12224 public Contract setIssuedElement(DateTimeType value) { 12225 this.issued = value; 12226 return this; 12227 } 12228 12229 /** 12230 * @return When this Contract was issued. 12231 */ 12232 public Date getIssued() { 12233 return this.issued == null ? null : this.issued.getValue(); 12234 } 12235 12236 /** 12237 * @param value When this Contract was issued. 12238 */ 12239 public Contract setIssued(Date value) { 12240 if (value == null) 12241 this.issued = null; 12242 else { 12243 if (this.issued == null) 12244 this.issued = new DateTimeType(); 12245 this.issued.setValue(value); 12246 } 12247 return this; 12248 } 12249 12250 /** 12251 * @return {@link #applies} (Relevant time or time-period when this Contract is 12252 * applicable.) 12253 */ 12254 public Period getApplies() { 12255 if (this.applies == null) 12256 if (Configuration.errorOnAutoCreate()) 12257 throw new Error("Attempt to auto-create Contract.applies"); 12258 else if (Configuration.doAutoCreate()) 12259 this.applies = new Period(); // cc 12260 return this.applies; 12261 } 12262 12263 public boolean hasApplies() { 12264 return this.applies != null && !this.applies.isEmpty(); 12265 } 12266 12267 /** 12268 * @param value {@link #applies} (Relevant time or time-period when this 12269 * Contract is applicable.) 12270 */ 12271 public Contract setApplies(Period value) { 12272 this.applies = value; 12273 return this; 12274 } 12275 12276 /** 12277 * @return {@link #expirationType} (Event resulting in discontinuation or 12278 * termination of this Contract instance by one or more parties to the 12279 * contract.) 12280 */ 12281 public CodeableConcept getExpirationType() { 12282 if (this.expirationType == null) 12283 if (Configuration.errorOnAutoCreate()) 12284 throw new Error("Attempt to auto-create Contract.expirationType"); 12285 else if (Configuration.doAutoCreate()) 12286 this.expirationType = new CodeableConcept(); // cc 12287 return this.expirationType; 12288 } 12289 12290 public boolean hasExpirationType() { 12291 return this.expirationType != null && !this.expirationType.isEmpty(); 12292 } 12293 12294 /** 12295 * @param value {@link #expirationType} (Event resulting in discontinuation or 12296 * termination of this Contract instance by one or more parties to 12297 * the contract.) 12298 */ 12299 public Contract setExpirationType(CodeableConcept value) { 12300 this.expirationType = value; 12301 return this; 12302 } 12303 12304 /** 12305 * @return {@link #subject} (The target entity impacted by or of interest to 12306 * parties to the agreement.) 12307 */ 12308 public List<Reference> getSubject() { 12309 if (this.subject == null) 12310 this.subject = new ArrayList<Reference>(); 12311 return this.subject; 12312 } 12313 12314 /** 12315 * @return Returns a reference to <code>this</code> for easy method chaining 12316 */ 12317 public Contract setSubject(List<Reference> theSubject) { 12318 this.subject = theSubject; 12319 return this; 12320 } 12321 12322 public boolean hasSubject() { 12323 if (this.subject == null) 12324 return false; 12325 for (Reference item : this.subject) 12326 if (!item.isEmpty()) 12327 return true; 12328 return false; 12329 } 12330 12331 public Reference addSubject() { // 3 12332 Reference t = new Reference(); 12333 if (this.subject == null) 12334 this.subject = new ArrayList<Reference>(); 12335 this.subject.add(t); 12336 return t; 12337 } 12338 12339 public Contract addSubject(Reference t) { // 3 12340 if (t == null) 12341 return this; 12342 if (this.subject == null) 12343 this.subject = new ArrayList<Reference>(); 12344 this.subject.add(t); 12345 return this; 12346 } 12347 12348 /** 12349 * @return The first repetition of repeating field {@link #subject}, creating it 12350 * if it does not already exist 12351 */ 12352 public Reference getSubjectFirstRep() { 12353 if (getSubject().isEmpty()) { 12354 addSubject(); 12355 } 12356 return getSubject().get(0); 12357 } 12358 12359 /** 12360 * @return {@link #authority} (A formally or informally recognized grouping of 12361 * people, principals, organizations, or jurisdictions formed for the 12362 * purpose of achieving some form of collective action such as the 12363 * promulgation, administration and enforcement of contracts and 12364 * policies.) 12365 */ 12366 public List<Reference> getAuthority() { 12367 if (this.authority == null) 12368 this.authority = new ArrayList<Reference>(); 12369 return this.authority; 12370 } 12371 12372 /** 12373 * @return Returns a reference to <code>this</code> for easy method chaining 12374 */ 12375 public Contract setAuthority(List<Reference> theAuthority) { 12376 this.authority = theAuthority; 12377 return this; 12378 } 12379 12380 public boolean hasAuthority() { 12381 if (this.authority == null) 12382 return false; 12383 for (Reference item : this.authority) 12384 if (!item.isEmpty()) 12385 return true; 12386 return false; 12387 } 12388 12389 public Reference addAuthority() { // 3 12390 Reference t = new Reference(); 12391 if (this.authority == null) 12392 this.authority = new ArrayList<Reference>(); 12393 this.authority.add(t); 12394 return t; 12395 } 12396 12397 public Contract addAuthority(Reference t) { // 3 12398 if (t == null) 12399 return this; 12400 if (this.authority == null) 12401 this.authority = new ArrayList<Reference>(); 12402 this.authority.add(t); 12403 return this; 12404 } 12405 12406 /** 12407 * @return The first repetition of repeating field {@link #authority}, creating 12408 * it if it does not already exist 12409 */ 12410 public Reference getAuthorityFirstRep() { 12411 if (getAuthority().isEmpty()) { 12412 addAuthority(); 12413 } 12414 return getAuthority().get(0); 12415 } 12416 12417 /** 12418 * @return {@link #domain} (Recognized governance framework or system operating 12419 * with a circumscribed scope in accordance with specified principles, 12420 * policies, processes or procedures for managing rights, actions, or 12421 * behaviors of parties or principals relative to resources.) 12422 */ 12423 public List<Reference> getDomain() { 12424 if (this.domain == null) 12425 this.domain = new ArrayList<Reference>(); 12426 return this.domain; 12427 } 12428 12429 /** 12430 * @return Returns a reference to <code>this</code> for easy method chaining 12431 */ 12432 public Contract setDomain(List<Reference> theDomain) { 12433 this.domain = theDomain; 12434 return this; 12435 } 12436 12437 public boolean hasDomain() { 12438 if (this.domain == null) 12439 return false; 12440 for (Reference item : this.domain) 12441 if (!item.isEmpty()) 12442 return true; 12443 return false; 12444 } 12445 12446 public Reference addDomain() { // 3 12447 Reference t = new Reference(); 12448 if (this.domain == null) 12449 this.domain = new ArrayList<Reference>(); 12450 this.domain.add(t); 12451 return t; 12452 } 12453 12454 public Contract addDomain(Reference t) { // 3 12455 if (t == null) 12456 return this; 12457 if (this.domain == null) 12458 this.domain = new ArrayList<Reference>(); 12459 this.domain.add(t); 12460 return this; 12461 } 12462 12463 /** 12464 * @return The first repetition of repeating field {@link #domain}, creating it 12465 * if it does not already exist 12466 */ 12467 public Reference getDomainFirstRep() { 12468 if (getDomain().isEmpty()) { 12469 addDomain(); 12470 } 12471 return getDomain().get(0); 12472 } 12473 12474 /** 12475 * @return {@link #site} (Sites in which the contract is complied with, 12476 * exercised, or in force.) 12477 */ 12478 public List<Reference> getSite() { 12479 if (this.site == null) 12480 this.site = new ArrayList<Reference>(); 12481 return this.site; 12482 } 12483 12484 /** 12485 * @return Returns a reference to <code>this</code> for easy method chaining 12486 */ 12487 public Contract setSite(List<Reference> theSite) { 12488 this.site = theSite; 12489 return this; 12490 } 12491 12492 public boolean hasSite() { 12493 if (this.site == null) 12494 return false; 12495 for (Reference item : this.site) 12496 if (!item.isEmpty()) 12497 return true; 12498 return false; 12499 } 12500 12501 public Reference addSite() { // 3 12502 Reference t = new Reference(); 12503 if (this.site == null) 12504 this.site = new ArrayList<Reference>(); 12505 this.site.add(t); 12506 return t; 12507 } 12508 12509 public Contract addSite(Reference t) { // 3 12510 if (t == null) 12511 return this; 12512 if (this.site == null) 12513 this.site = new ArrayList<Reference>(); 12514 this.site.add(t); 12515 return this; 12516 } 12517 12518 /** 12519 * @return The first repetition of repeating field {@link #site}, creating it if 12520 * it does not already exist 12521 */ 12522 public Reference getSiteFirstRep() { 12523 if (getSite().isEmpty()) { 12524 addSite(); 12525 } 12526 return getSite().get(0); 12527 } 12528 12529 /** 12530 * @return {@link #name} (A natural language name identifying this Contract 12531 * definition, derivative, or instance in any legal state. Provides 12532 * additional information about its content. This name should be usable 12533 * as an identifier for the module by machine processing applications 12534 * such as code generation.). This is the underlying object with id, 12535 * value and extensions. The accessor "getName" gives direct access to 12536 * the value 12537 */ 12538 public StringType getNameElement() { 12539 if (this.name == null) 12540 if (Configuration.errorOnAutoCreate()) 12541 throw new Error("Attempt to auto-create Contract.name"); 12542 else if (Configuration.doAutoCreate()) 12543 this.name = new StringType(); // bb 12544 return this.name; 12545 } 12546 12547 public boolean hasNameElement() { 12548 return this.name != null && !this.name.isEmpty(); 12549 } 12550 12551 public boolean hasName() { 12552 return this.name != null && !this.name.isEmpty(); 12553 } 12554 12555 /** 12556 * @param value {@link #name} (A natural language name identifying this Contract 12557 * definition, derivative, or instance in any legal state. Provides 12558 * additional information about its content. This name should be 12559 * usable as an identifier for the module by machine processing 12560 * applications such as code generation.). This is the underlying 12561 * object with id, value and extensions. The accessor "getName" 12562 * gives direct access to the value 12563 */ 12564 public Contract setNameElement(StringType value) { 12565 this.name = value; 12566 return this; 12567 } 12568 12569 /** 12570 * @return A natural language name identifying this Contract definition, 12571 * derivative, or instance in any legal state. Provides additional 12572 * information about its content. This name should be usable as an 12573 * identifier for the module by machine processing applications such as 12574 * code generation. 12575 */ 12576 public String getName() { 12577 return this.name == null ? null : this.name.getValue(); 12578 } 12579 12580 /** 12581 * @param value A natural language name identifying this Contract definition, 12582 * derivative, or instance in any legal state. Provides additional 12583 * information about its content. This name should be usable as an 12584 * identifier for the module by machine processing applications 12585 * such as code generation. 12586 */ 12587 public Contract setName(String value) { 12588 if (Utilities.noString(value)) 12589 this.name = null; 12590 else { 12591 if (this.name == null) 12592 this.name = new StringType(); 12593 this.name.setValue(value); 12594 } 12595 return this; 12596 } 12597 12598 /** 12599 * @return {@link #title} (A short, descriptive, user-friendly title for this 12600 * Contract definition, derivative, or instance in any legal state.t 12601 * giving additional information about its content.). This is the 12602 * underlying object with id, value and extensions. The accessor 12603 * "getTitle" gives direct access to the value 12604 */ 12605 public StringType getTitleElement() { 12606 if (this.title == null) 12607 if (Configuration.errorOnAutoCreate()) 12608 throw new Error("Attempt to auto-create Contract.title"); 12609 else if (Configuration.doAutoCreate()) 12610 this.title = new StringType(); // bb 12611 return this.title; 12612 } 12613 12614 public boolean hasTitleElement() { 12615 return this.title != null && !this.title.isEmpty(); 12616 } 12617 12618 public boolean hasTitle() { 12619 return this.title != null && !this.title.isEmpty(); 12620 } 12621 12622 /** 12623 * @param value {@link #title} (A short, descriptive, user-friendly title for 12624 * this Contract definition, derivative, or instance in any legal 12625 * state.t giving additional information about its content.). This 12626 * is the underlying object with id, value and extensions. The 12627 * accessor "getTitle" gives direct access to the value 12628 */ 12629 public Contract setTitleElement(StringType value) { 12630 this.title = value; 12631 return this; 12632 } 12633 12634 /** 12635 * @return A short, descriptive, user-friendly title for this Contract 12636 * definition, derivative, or instance in any legal state.t giving 12637 * additional information about its content. 12638 */ 12639 public String getTitle() { 12640 return this.title == null ? null : this.title.getValue(); 12641 } 12642 12643 /** 12644 * @param value A short, descriptive, user-friendly title for this Contract 12645 * definition, derivative, or instance in any legal state.t giving 12646 * additional information about its content. 12647 */ 12648 public Contract setTitle(String value) { 12649 if (Utilities.noString(value)) 12650 this.title = null; 12651 else { 12652 if (this.title == null) 12653 this.title = new StringType(); 12654 this.title.setValue(value); 12655 } 12656 return this; 12657 } 12658 12659 /** 12660 * @return {@link #subtitle} (An explanatory or alternate user-friendly title 12661 * for this Contract definition, derivative, or instance in any legal 12662 * state.t giving additional information about its content.). This is 12663 * the underlying object with id, value and extensions. The accessor 12664 * "getSubtitle" gives direct access to the value 12665 */ 12666 public StringType getSubtitleElement() { 12667 if (this.subtitle == null) 12668 if (Configuration.errorOnAutoCreate()) 12669 throw new Error("Attempt to auto-create Contract.subtitle"); 12670 else if (Configuration.doAutoCreate()) 12671 this.subtitle = new StringType(); // bb 12672 return this.subtitle; 12673 } 12674 12675 public boolean hasSubtitleElement() { 12676 return this.subtitle != null && !this.subtitle.isEmpty(); 12677 } 12678 12679 public boolean hasSubtitle() { 12680 return this.subtitle != null && !this.subtitle.isEmpty(); 12681 } 12682 12683 /** 12684 * @param value {@link #subtitle} (An explanatory or alternate user-friendly 12685 * title for this Contract definition, derivative, or instance in 12686 * any legal state.t giving additional information about its 12687 * content.). This is the underlying object with id, value and 12688 * extensions. The accessor "getSubtitle" gives direct access to 12689 * the value 12690 */ 12691 public Contract setSubtitleElement(StringType value) { 12692 this.subtitle = value; 12693 return this; 12694 } 12695 12696 /** 12697 * @return An explanatory or alternate user-friendly title for this Contract 12698 * definition, derivative, or instance in any legal state.t giving 12699 * additional information about its content. 12700 */ 12701 public String getSubtitle() { 12702 return this.subtitle == null ? null : this.subtitle.getValue(); 12703 } 12704 12705 /** 12706 * @param value An explanatory or alternate user-friendly title for this 12707 * Contract definition, derivative, or instance in any legal 12708 * state.t giving additional information about its content. 12709 */ 12710 public Contract setSubtitle(String value) { 12711 if (Utilities.noString(value)) 12712 this.subtitle = null; 12713 else { 12714 if (this.subtitle == null) 12715 this.subtitle = new StringType(); 12716 this.subtitle.setValue(value); 12717 } 12718 return this; 12719 } 12720 12721 /** 12722 * @return {@link #alias} (Alternative representation of the title for this 12723 * Contract definition, derivative, or instance in any legal state., 12724 * e.g., a domain specific contract number related to legislation.) 12725 */ 12726 public List<StringType> getAlias() { 12727 if (this.alias == null) 12728 this.alias = new ArrayList<StringType>(); 12729 return this.alias; 12730 } 12731 12732 /** 12733 * @return Returns a reference to <code>this</code> for easy method chaining 12734 */ 12735 public Contract setAlias(List<StringType> theAlias) { 12736 this.alias = theAlias; 12737 return this; 12738 } 12739 12740 public boolean hasAlias() { 12741 if (this.alias == null) 12742 return false; 12743 for (StringType item : this.alias) 12744 if (!item.isEmpty()) 12745 return true; 12746 return false; 12747 } 12748 12749 /** 12750 * @return {@link #alias} (Alternative representation of the title for this 12751 * Contract definition, derivative, or instance in any legal state., 12752 * e.g., a domain specific contract number related to legislation.) 12753 */ 12754 public StringType addAliasElement() {// 2 12755 StringType t = new StringType(); 12756 if (this.alias == null) 12757 this.alias = new ArrayList<StringType>(); 12758 this.alias.add(t); 12759 return t; 12760 } 12761 12762 /** 12763 * @param value {@link #alias} (Alternative representation of the title for this 12764 * Contract definition, derivative, or instance in any legal 12765 * state., e.g., a domain specific contract number related to 12766 * legislation.) 12767 */ 12768 public Contract addAlias(String value) { // 1 12769 StringType t = new StringType(); 12770 t.setValue(value); 12771 if (this.alias == null) 12772 this.alias = new ArrayList<StringType>(); 12773 this.alias.add(t); 12774 return this; 12775 } 12776 12777 /** 12778 * @param value {@link #alias} (Alternative representation of the title for this 12779 * Contract definition, derivative, or instance in any legal 12780 * state., e.g., a domain specific contract number related to 12781 * legislation.) 12782 */ 12783 public boolean hasAlias(String value) { 12784 if (this.alias == null) 12785 return false; 12786 for (StringType v : this.alias) 12787 if (v.getValue().equals(value)) // string 12788 return true; 12789 return false; 12790 } 12791 12792 /** 12793 * @return {@link #author} (The individual or organization that authored the 12794 * Contract definition, derivative, or instance in any legal state.) 12795 */ 12796 public Reference getAuthor() { 12797 if (this.author == null) 12798 if (Configuration.errorOnAutoCreate()) 12799 throw new Error("Attempt to auto-create Contract.author"); 12800 else if (Configuration.doAutoCreate()) 12801 this.author = new Reference(); // cc 12802 return this.author; 12803 } 12804 12805 public boolean hasAuthor() { 12806 return this.author != null && !this.author.isEmpty(); 12807 } 12808 12809 /** 12810 * @param value {@link #author} (The individual or organization that authored 12811 * the Contract definition, derivative, or instance in any legal 12812 * state.) 12813 */ 12814 public Contract setAuthor(Reference value) { 12815 this.author = value; 12816 return this; 12817 } 12818 12819 /** 12820 * @return {@link #author} The actual object that is the target of the 12821 * reference. The reference library doesn't populate this, but you can 12822 * use it to hold the resource if you resolve it. (The individual or 12823 * organization that authored the Contract definition, derivative, or 12824 * instance in any legal state.) 12825 */ 12826 public Resource getAuthorTarget() { 12827 return this.authorTarget; 12828 } 12829 12830 /** 12831 * @param value {@link #author} The actual object that is the target of the 12832 * reference. The reference library doesn't use these, but you can 12833 * use it to hold the resource if you resolve it. (The individual 12834 * or organization that authored the Contract definition, 12835 * derivative, or instance in any legal state.) 12836 */ 12837 public Contract setAuthorTarget(Resource value) { 12838 this.authorTarget = value; 12839 return this; 12840 } 12841 12842 /** 12843 * @return {@link #scope} (A selector of legal concerns for this Contract 12844 * definition, derivative, or instance in any legal state.) 12845 */ 12846 public CodeableConcept getScope() { 12847 if (this.scope == null) 12848 if (Configuration.errorOnAutoCreate()) 12849 throw new Error("Attempt to auto-create Contract.scope"); 12850 else if (Configuration.doAutoCreate()) 12851 this.scope = new CodeableConcept(); // cc 12852 return this.scope; 12853 } 12854 12855 public boolean hasScope() { 12856 return this.scope != null && !this.scope.isEmpty(); 12857 } 12858 12859 /** 12860 * @param value {@link #scope} (A selector of legal concerns for this Contract 12861 * definition, derivative, or instance in any legal state.) 12862 */ 12863 public Contract setScope(CodeableConcept value) { 12864 this.scope = value; 12865 return this; 12866 } 12867 12868 /** 12869 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 12870 * achievement of specific contractual objectives.) 12871 */ 12872 public Type getTopic() { 12873 return this.topic; 12874 } 12875 12876 /** 12877 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 12878 * achievement of specific contractual objectives.) 12879 */ 12880 public CodeableConcept getTopicCodeableConcept() throws FHIRException { 12881 if (this.topic == null) 12882 this.topic = new CodeableConcept(); 12883 if (!(this.topic instanceof CodeableConcept)) 12884 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 12885 + this.topic.getClass().getName() + " was encountered"); 12886 return (CodeableConcept) this.topic; 12887 } 12888 12889 public boolean hasTopicCodeableConcept() { 12890 return this.topic instanceof CodeableConcept; 12891 } 12892 12893 /** 12894 * @return {@link #topic} (Narrows the range of legal concerns to focus on the 12895 * achievement of specific contractual objectives.) 12896 */ 12897 public Reference getTopicReference() throws FHIRException { 12898 if (this.topic == null) 12899 this.topic = new Reference(); 12900 if (!(this.topic instanceof Reference)) 12901 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.topic.getClass().getName() 12902 + " was encountered"); 12903 return (Reference) this.topic; 12904 } 12905 12906 public boolean hasTopicReference() { 12907 return this.topic instanceof Reference; 12908 } 12909 12910 public boolean hasTopic() { 12911 return this.topic != null && !this.topic.isEmpty(); 12912 } 12913 12914 /** 12915 * @param value {@link #topic} (Narrows the range of legal concerns to focus on 12916 * the achievement of specific contractual objectives.) 12917 */ 12918 public Contract setTopic(Type value) { 12919 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 12920 throw new Error("Not the right type for Contract.topic[x]: " + value.fhirType()); 12921 this.topic = value; 12922 return this; 12923 } 12924 12925 /** 12926 * @return {@link #type} (A high-level category for the legal instrument, 12927 * whether constructed as a Contract definition, derivative, or instance 12928 * in any legal state. Provides additional information about its content 12929 * within the context of the Contract's scope to distinguish the kinds 12930 * of systems that would be interested in the contract.) 12931 */ 12932 public CodeableConcept getType() { 12933 if (this.type == null) 12934 if (Configuration.errorOnAutoCreate()) 12935 throw new Error("Attempt to auto-create Contract.type"); 12936 else if (Configuration.doAutoCreate()) 12937 this.type = new CodeableConcept(); // cc 12938 return this.type; 12939 } 12940 12941 public boolean hasType() { 12942 return this.type != null && !this.type.isEmpty(); 12943 } 12944 12945 /** 12946 * @param value {@link #type} (A high-level category for the legal instrument, 12947 * whether constructed as a Contract definition, derivative, or 12948 * instance in any legal state. Provides additional information 12949 * about its content within the context of the Contract's scope to 12950 * distinguish the kinds of systems that would be interested in the 12951 * contract.) 12952 */ 12953 public Contract setType(CodeableConcept value) { 12954 this.type = value; 12955 return this; 12956 } 12957 12958 /** 12959 * @return {@link #subType} (Sub-category for the Contract that distinguishes 12960 * the kinds of systems that would be interested in the Contract within 12961 * the context of the Contract's scope.) 12962 */ 12963 public List<CodeableConcept> getSubType() { 12964 if (this.subType == null) 12965 this.subType = new ArrayList<CodeableConcept>(); 12966 return this.subType; 12967 } 12968 12969 /** 12970 * @return Returns a reference to <code>this</code> for easy method chaining 12971 */ 12972 public Contract setSubType(List<CodeableConcept> theSubType) { 12973 this.subType = theSubType; 12974 return this; 12975 } 12976 12977 public boolean hasSubType() { 12978 if (this.subType == null) 12979 return false; 12980 for (CodeableConcept item : this.subType) 12981 if (!item.isEmpty()) 12982 return true; 12983 return false; 12984 } 12985 12986 public CodeableConcept addSubType() { // 3 12987 CodeableConcept t = new CodeableConcept(); 12988 if (this.subType == null) 12989 this.subType = new ArrayList<CodeableConcept>(); 12990 this.subType.add(t); 12991 return t; 12992 } 12993 12994 public Contract addSubType(CodeableConcept t) { // 3 12995 if (t == null) 12996 return this; 12997 if (this.subType == null) 12998 this.subType = new ArrayList<CodeableConcept>(); 12999 this.subType.add(t); 13000 return this; 13001 } 13002 13003 /** 13004 * @return The first repetition of repeating field {@link #subType}, creating it 13005 * if it does not already exist 13006 */ 13007 public CodeableConcept getSubTypeFirstRep() { 13008 if (getSubType().isEmpty()) { 13009 addSubType(); 13010 } 13011 return getSubType().get(0); 13012 } 13013 13014 /** 13015 * @return {@link #contentDefinition} (Precusory content developed with a focus 13016 * and intent of supporting the formation a Contract instance, which may 13017 * be associated with and transformable into a Contract.) 13018 */ 13019 public ContentDefinitionComponent getContentDefinition() { 13020 if (this.contentDefinition == null) 13021 if (Configuration.errorOnAutoCreate()) 13022 throw new Error("Attempt to auto-create Contract.contentDefinition"); 13023 else if (Configuration.doAutoCreate()) 13024 this.contentDefinition = new ContentDefinitionComponent(); // cc 13025 return this.contentDefinition; 13026 } 13027 13028 public boolean hasContentDefinition() { 13029 return this.contentDefinition != null && !this.contentDefinition.isEmpty(); 13030 } 13031 13032 /** 13033 * @param value {@link #contentDefinition} (Precusory content developed with a 13034 * focus and intent of supporting the formation a Contract 13035 * instance, which may be associated with and transformable into a 13036 * Contract.) 13037 */ 13038 public Contract setContentDefinition(ContentDefinitionComponent value) { 13039 this.contentDefinition = value; 13040 return this; 13041 } 13042 13043 /** 13044 * @return {@link #term} (One or more Contract Provisions, which may be related 13045 * and conveyed as a group, and may contain nested groups.) 13046 */ 13047 public List<TermComponent> getTerm() { 13048 if (this.term == null) 13049 this.term = new ArrayList<TermComponent>(); 13050 return this.term; 13051 } 13052 13053 /** 13054 * @return Returns a reference to <code>this</code> for easy method chaining 13055 */ 13056 public Contract setTerm(List<TermComponent> theTerm) { 13057 this.term = theTerm; 13058 return this; 13059 } 13060 13061 public boolean hasTerm() { 13062 if (this.term == null) 13063 return false; 13064 for (TermComponent item : this.term) 13065 if (!item.isEmpty()) 13066 return true; 13067 return false; 13068 } 13069 13070 public TermComponent addTerm() { // 3 13071 TermComponent t = new TermComponent(); 13072 if (this.term == null) 13073 this.term = new ArrayList<TermComponent>(); 13074 this.term.add(t); 13075 return t; 13076 } 13077 13078 public Contract addTerm(TermComponent t) { // 3 13079 if (t == null) 13080 return this; 13081 if (this.term == null) 13082 this.term = new ArrayList<TermComponent>(); 13083 this.term.add(t); 13084 return this; 13085 } 13086 13087 /** 13088 * @return The first repetition of repeating field {@link #term}, creating it if 13089 * it does not already exist 13090 */ 13091 public TermComponent getTermFirstRep() { 13092 if (getTerm().isEmpty()) { 13093 addTerm(); 13094 } 13095 return getTerm().get(0); 13096 } 13097 13098 /** 13099 * @return {@link #supportingInfo} (Information that may be needed by/relevant 13100 * to the performer in their execution of this term action.) 13101 */ 13102 public List<Reference> getSupportingInfo() { 13103 if (this.supportingInfo == null) 13104 this.supportingInfo = new ArrayList<Reference>(); 13105 return this.supportingInfo; 13106 } 13107 13108 /** 13109 * @return Returns a reference to <code>this</code> for easy method chaining 13110 */ 13111 public Contract setSupportingInfo(List<Reference> theSupportingInfo) { 13112 this.supportingInfo = theSupportingInfo; 13113 return this; 13114 } 13115 13116 public boolean hasSupportingInfo() { 13117 if (this.supportingInfo == null) 13118 return false; 13119 for (Reference item : this.supportingInfo) 13120 if (!item.isEmpty()) 13121 return true; 13122 return false; 13123 } 13124 13125 public Reference addSupportingInfo() { // 3 13126 Reference t = new Reference(); 13127 if (this.supportingInfo == null) 13128 this.supportingInfo = new ArrayList<Reference>(); 13129 this.supportingInfo.add(t); 13130 return t; 13131 } 13132 13133 public Contract addSupportingInfo(Reference t) { // 3 13134 if (t == null) 13135 return this; 13136 if (this.supportingInfo == null) 13137 this.supportingInfo = new ArrayList<Reference>(); 13138 this.supportingInfo.add(t); 13139 return this; 13140 } 13141 13142 /** 13143 * @return The first repetition of repeating field {@link #supportingInfo}, 13144 * creating it if it does not already exist 13145 */ 13146 public Reference getSupportingInfoFirstRep() { 13147 if (getSupportingInfo().isEmpty()) { 13148 addSupportingInfo(); 13149 } 13150 return getSupportingInfo().get(0); 13151 } 13152 13153 /** 13154 * @return {@link #relevantHistory} (Links to Provenance records for past 13155 * versions of this Contract definition, derivative, or instance, which 13156 * identify key state transitions or updates that are likely to be 13157 * relevant to a user looking at the current version of the Contract. 13158 * The Provence.entity indicates the target that was changed in the 13159 * update. 13160 * http://build.fhir.org/provenance-definitions.html#Provenance.entity.) 13161 */ 13162 public List<Reference> getRelevantHistory() { 13163 if (this.relevantHistory == null) 13164 this.relevantHistory = new ArrayList<Reference>(); 13165 return this.relevantHistory; 13166 } 13167 13168 /** 13169 * @return Returns a reference to <code>this</code> for easy method chaining 13170 */ 13171 public Contract setRelevantHistory(List<Reference> theRelevantHistory) { 13172 this.relevantHistory = theRelevantHistory; 13173 return this; 13174 } 13175 13176 public boolean hasRelevantHistory() { 13177 if (this.relevantHistory == null) 13178 return false; 13179 for (Reference item : this.relevantHistory) 13180 if (!item.isEmpty()) 13181 return true; 13182 return false; 13183 } 13184 13185 public Reference addRelevantHistory() { // 3 13186 Reference t = new Reference(); 13187 if (this.relevantHistory == null) 13188 this.relevantHistory = new ArrayList<Reference>(); 13189 this.relevantHistory.add(t); 13190 return t; 13191 } 13192 13193 public Contract addRelevantHistory(Reference t) { // 3 13194 if (t == null) 13195 return this; 13196 if (this.relevantHistory == null) 13197 this.relevantHistory = new ArrayList<Reference>(); 13198 this.relevantHistory.add(t); 13199 return this; 13200 } 13201 13202 /** 13203 * @return The first repetition of repeating field {@link #relevantHistory}, 13204 * creating it if it does not already exist 13205 */ 13206 public Reference getRelevantHistoryFirstRep() { 13207 if (getRelevantHistory().isEmpty()) { 13208 addRelevantHistory(); 13209 } 13210 return getRelevantHistory().get(0); 13211 } 13212 13213 /** 13214 * @return {@link #signer} (Parties with legal standing in the Contract, 13215 * including the principal parties, the grantor(s) and grantee(s), which 13216 * are any person or organization bound by the contract, and any 13217 * ancillary parties, which facilitate the execution of the contract 13218 * such as a notary or witness.) 13219 */ 13220 public List<SignatoryComponent> getSigner() { 13221 if (this.signer == null) 13222 this.signer = new ArrayList<SignatoryComponent>(); 13223 return this.signer; 13224 } 13225 13226 /** 13227 * @return Returns a reference to <code>this</code> for easy method chaining 13228 */ 13229 public Contract setSigner(List<SignatoryComponent> theSigner) { 13230 this.signer = theSigner; 13231 return this; 13232 } 13233 13234 public boolean hasSigner() { 13235 if (this.signer == null) 13236 return false; 13237 for (SignatoryComponent item : this.signer) 13238 if (!item.isEmpty()) 13239 return true; 13240 return false; 13241 } 13242 13243 public SignatoryComponent addSigner() { // 3 13244 SignatoryComponent t = new SignatoryComponent(); 13245 if (this.signer == null) 13246 this.signer = new ArrayList<SignatoryComponent>(); 13247 this.signer.add(t); 13248 return t; 13249 } 13250 13251 public Contract addSigner(SignatoryComponent t) { // 3 13252 if (t == null) 13253 return this; 13254 if (this.signer == null) 13255 this.signer = new ArrayList<SignatoryComponent>(); 13256 this.signer.add(t); 13257 return this; 13258 } 13259 13260 /** 13261 * @return The first repetition of repeating field {@link #signer}, creating it 13262 * if it does not already exist 13263 */ 13264 public SignatoryComponent getSignerFirstRep() { 13265 if (getSigner().isEmpty()) { 13266 addSigner(); 13267 } 13268 return getSigner().get(0); 13269 } 13270 13271 /** 13272 * @return {@link #friendly} (The "patient friendly language" versionof the 13273 * Contract in whole or in parts. "Patient friendly language" means the 13274 * representation of the Contract and Contract Provisions in a manner 13275 * that is readily accessible and understandable by a layperson in 13276 * accordance with best practices for communication styles that ensure 13277 * that those agreeing to or signing the Contract understand the roles, 13278 * actions, obligations, responsibilities, and implication of the 13279 * agreement.) 13280 */ 13281 public List<FriendlyLanguageComponent> getFriendly() { 13282 if (this.friendly == null) 13283 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13284 return this.friendly; 13285 } 13286 13287 /** 13288 * @return Returns a reference to <code>this</code> for easy method chaining 13289 */ 13290 public Contract setFriendly(List<FriendlyLanguageComponent> theFriendly) { 13291 this.friendly = theFriendly; 13292 return this; 13293 } 13294 13295 public boolean hasFriendly() { 13296 if (this.friendly == null) 13297 return false; 13298 for (FriendlyLanguageComponent item : this.friendly) 13299 if (!item.isEmpty()) 13300 return true; 13301 return false; 13302 } 13303 13304 public FriendlyLanguageComponent addFriendly() { // 3 13305 FriendlyLanguageComponent t = new FriendlyLanguageComponent(); 13306 if (this.friendly == null) 13307 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13308 this.friendly.add(t); 13309 return t; 13310 } 13311 13312 public Contract addFriendly(FriendlyLanguageComponent t) { // 3 13313 if (t == null) 13314 return this; 13315 if (this.friendly == null) 13316 this.friendly = new ArrayList<FriendlyLanguageComponent>(); 13317 this.friendly.add(t); 13318 return this; 13319 } 13320 13321 /** 13322 * @return The first repetition of repeating field {@link #friendly}, creating 13323 * it if it does not already exist 13324 */ 13325 public FriendlyLanguageComponent getFriendlyFirstRep() { 13326 if (getFriendly().isEmpty()) { 13327 addFriendly(); 13328 } 13329 return getFriendly().get(0); 13330 } 13331 13332 /** 13333 * @return {@link #legal} (List of Legal expressions or representations of this 13334 * Contract.) 13335 */ 13336 public List<LegalLanguageComponent> getLegal() { 13337 if (this.legal == null) 13338 this.legal = new ArrayList<LegalLanguageComponent>(); 13339 return this.legal; 13340 } 13341 13342 /** 13343 * @return Returns a reference to <code>this</code> for easy method chaining 13344 */ 13345 public Contract setLegal(List<LegalLanguageComponent> theLegal) { 13346 this.legal = theLegal; 13347 return this; 13348 } 13349 13350 public boolean hasLegal() { 13351 if (this.legal == null) 13352 return false; 13353 for (LegalLanguageComponent item : this.legal) 13354 if (!item.isEmpty()) 13355 return true; 13356 return false; 13357 } 13358 13359 public LegalLanguageComponent addLegal() { // 3 13360 LegalLanguageComponent t = new LegalLanguageComponent(); 13361 if (this.legal == null) 13362 this.legal = new ArrayList<LegalLanguageComponent>(); 13363 this.legal.add(t); 13364 return t; 13365 } 13366 13367 public Contract addLegal(LegalLanguageComponent t) { // 3 13368 if (t == null) 13369 return this; 13370 if (this.legal == null) 13371 this.legal = new ArrayList<LegalLanguageComponent>(); 13372 this.legal.add(t); 13373 return this; 13374 } 13375 13376 /** 13377 * @return The first repetition of repeating field {@link #legal}, creating it 13378 * if it does not already exist 13379 */ 13380 public LegalLanguageComponent getLegalFirstRep() { 13381 if (getLegal().isEmpty()) { 13382 addLegal(); 13383 } 13384 return getLegal().get(0); 13385 } 13386 13387 /** 13388 * @return {@link #rule} (List of Computable Policy Rule Language 13389 * Representations of this Contract.) 13390 */ 13391 public List<ComputableLanguageComponent> getRule() { 13392 if (this.rule == null) 13393 this.rule = new ArrayList<ComputableLanguageComponent>(); 13394 return this.rule; 13395 } 13396 13397 /** 13398 * @return Returns a reference to <code>this</code> for easy method chaining 13399 */ 13400 public Contract setRule(List<ComputableLanguageComponent> theRule) { 13401 this.rule = theRule; 13402 return this; 13403 } 13404 13405 public boolean hasRule() { 13406 if (this.rule == null) 13407 return false; 13408 for (ComputableLanguageComponent item : this.rule) 13409 if (!item.isEmpty()) 13410 return true; 13411 return false; 13412 } 13413 13414 public ComputableLanguageComponent addRule() { // 3 13415 ComputableLanguageComponent t = new ComputableLanguageComponent(); 13416 if (this.rule == null) 13417 this.rule = new ArrayList<ComputableLanguageComponent>(); 13418 this.rule.add(t); 13419 return t; 13420 } 13421 13422 public Contract addRule(ComputableLanguageComponent t) { // 3 13423 if (t == null) 13424 return this; 13425 if (this.rule == null) 13426 this.rule = new ArrayList<ComputableLanguageComponent>(); 13427 this.rule.add(t); 13428 return this; 13429 } 13430 13431 /** 13432 * @return The first repetition of repeating field {@link #rule}, creating it if 13433 * it does not already exist 13434 */ 13435 public ComputableLanguageComponent getRuleFirstRep() { 13436 if (getRule().isEmpty()) { 13437 addRule(); 13438 } 13439 return getRule().get(0); 13440 } 13441 13442 /** 13443 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13444 * and legally recognized representation of the Contract, which is 13445 * considered the "source of truth" and which would be the basis for 13446 * legal action related to enforcement of this Contract.) 13447 */ 13448 public Type getLegallyBinding() { 13449 return this.legallyBinding; 13450 } 13451 13452 /** 13453 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13454 * and legally recognized representation of the Contract, which is 13455 * considered the "source of truth" and which would be the basis for 13456 * legal action related to enforcement of this Contract.) 13457 */ 13458 public Attachment getLegallyBindingAttachment() throws FHIRException { 13459 if (this.legallyBinding == null) 13460 this.legallyBinding = new Attachment(); 13461 if (!(this.legallyBinding instanceof Attachment)) 13462 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 13463 + this.legallyBinding.getClass().getName() + " was encountered"); 13464 return (Attachment) this.legallyBinding; 13465 } 13466 13467 public boolean hasLegallyBindingAttachment() { 13468 return this.legallyBinding instanceof Attachment; 13469 } 13470 13471 /** 13472 * @return {@link #legallyBinding} (Legally binding Contract: This is the signed 13473 * and legally recognized representation of the Contract, which is 13474 * considered the "source of truth" and which would be the basis for 13475 * legal action related to enforcement of this Contract.) 13476 */ 13477 public Reference getLegallyBindingReference() throws FHIRException { 13478 if (this.legallyBinding == null) 13479 this.legallyBinding = new Reference(); 13480 if (!(this.legallyBinding instanceof Reference)) 13481 throw new FHIRException("Type mismatch: the type Reference was expected, but " 13482 + this.legallyBinding.getClass().getName() + " was encountered"); 13483 return (Reference) this.legallyBinding; 13484 } 13485 13486 public boolean hasLegallyBindingReference() { 13487 return this.legallyBinding instanceof Reference; 13488 } 13489 13490 public boolean hasLegallyBinding() { 13491 return this.legallyBinding != null && !this.legallyBinding.isEmpty(); 13492 } 13493 13494 /** 13495 * @param value {@link #legallyBinding} (Legally binding Contract: This is the 13496 * signed and legally recognized representation of the Contract, 13497 * which is considered the "source of truth" and which would be the 13498 * basis for legal action related to enforcement of this Contract.) 13499 */ 13500 public Contract setLegallyBinding(Type value) { 13501 if (value != null && !(value instanceof Attachment || value instanceof Reference)) 13502 throw new Error("Not the right type for Contract.legallyBinding[x]: " + value.fhirType()); 13503 this.legallyBinding = value; 13504 return this; 13505 } 13506 13507 protected void listChildren(List<Property> children) { 13508 super.listChildren(children); 13509 children.add(new Property("identifier", "Identifier", 13510 "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, 13511 java.lang.Integer.MAX_VALUE, identifier)); 13512 children.add(new Property("url", "uri", 13513 "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url)); 13514 children.add(new Property("version", "string", 13515 "An edition identifier used for business purposes to label business significant variants.", 0, 1, version)); 13516 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 13517 children.add(new Property("legalState", "CodeableConcept", 13518 "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 13519 0, 1, legalState)); 13520 children.add(new Property("instantiatesCanonical", "Reference(Contract)", 13521 "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 13522 0, 1, instantiatesCanonical)); 13523 children.add(new Property("instantiatesUri", "uri", 13524 "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 13525 0, 1, instantiatesUri)); 13526 children.add(new Property("contentDerivative", "CodeableConcept", 13527 "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, 13528 contentDerivative)); 13529 children.add(new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued)); 13530 children.add(new Property("applies", "Period", "Relevant time or time-period when this Contract is applicable.", 0, 13531 1, applies)); 13532 children.add(new Property("expirationType", "CodeableConcept", 13533 "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 13534 0, 1, expirationType)); 13535 children.add(new Property("subject", "Reference(Any)", 13536 "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, 13537 subject)); 13538 children.add(new Property("authority", "Reference(Organization)", 13539 "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 13540 0, java.lang.Integer.MAX_VALUE, authority)); 13541 children.add(new Property("domain", "Reference(Location)", 13542 "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 13543 0, java.lang.Integer.MAX_VALUE, domain)); 13544 children.add(new Property("site", "Reference(Location)", 13545 "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, 13546 site)); 13547 children.add(new Property("name", "string", 13548 "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 13549 0, 1, name)); 13550 children.add(new Property("title", "string", 13551 "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13552 0, 1, title)); 13553 children.add(new Property("subtitle", "string", 13554 "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13555 0, 1, subtitle)); 13556 children.add(new Property("alias", "string", 13557 "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 13558 0, java.lang.Integer.MAX_VALUE, alias)); 13559 children.add(new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", 13560 "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 13561 0, 1, author)); 13562 children.add(new Property("scope", "CodeableConcept", 13563 "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 1, 13564 scope)); 13565 children.add(new Property("topic[x]", "CodeableConcept|Reference(Any)", 13566 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13567 topic)); 13568 children.add(new Property("type", "CodeableConcept", 13569 "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 13570 0, 1, type)); 13571 children.add(new Property("subType", "CodeableConcept", 13572 "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 13573 0, java.lang.Integer.MAX_VALUE, subType)); 13574 children.add(new Property("contentDefinition", "", 13575 "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 13576 0, 1, contentDefinition)); 13577 children.add(new Property("term", "", 13578 "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 13579 0, java.lang.Integer.MAX_VALUE, term)); 13580 children.add(new Property("supportingInfo", "Reference(Any)", 13581 "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, 13582 java.lang.Integer.MAX_VALUE, supportingInfo)); 13583 children.add(new Property("relevantHistory", "Reference(Provenance)", 13584 "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.", 13585 0, java.lang.Integer.MAX_VALUE, relevantHistory)); 13586 children.add(new Property("signer", "", 13587 "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 13588 0, java.lang.Integer.MAX_VALUE, signer)); 13589 children.add(new Property("friendly", "", 13590 "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 13591 0, java.lang.Integer.MAX_VALUE, friendly)); 13592 children.add(new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, 13593 java.lang.Integer.MAX_VALUE, legal)); 13594 children.add(new Property("rule", "", "List of Computable Policy Rule Language Representations of this Contract.", 13595 0, java.lang.Integer.MAX_VALUE, rule)); 13596 children.add(new Property("legallyBinding[x]", 13597 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13598 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13599 0, 1, legallyBinding)); 13600 } 13601 13602 @Override 13603 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13604 switch (_hash) { 13605 case -1618432855: 13606 /* identifier */ return new Property("identifier", "Identifier", 13607 "Unique identifier for this Contract or a derivative that references a Source Contract.", 0, 13608 java.lang.Integer.MAX_VALUE, identifier); 13609 case 116079: 13610 /* url */ return new Property("url", "uri", 13611 "Canonical identifier for this contract, represented as a URI (globally unique).", 0, 1, url); 13612 case 351608024: 13613 /* version */ return new Property("version", "string", 13614 "An edition identifier used for business purposes to label business significant variants.", 0, 1, version); 13615 case -892481550: 13616 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 13617 case 568606040: 13618 /* legalState */ return new Property("legalState", "CodeableConcept", 13619 "Legal states of the formation of a legal instrument, which is a formally executed written document that can be formally attributed to its author, records and formally expresses a legally enforceable act, process, or contractual duty, obligation, or right, and therefore evidences that act, process, or agreement.", 13620 0, 1, legalState); 13621 case 8911915: 13622 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "Reference(Contract)", 13623 "The URL pointing to a FHIR-defined Contract Definition that is adhered to in whole or part by this Contract.", 13624 0, 1, instantiatesCanonical); 13625 case -1926393373: 13626 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 13627 "The URL pointing to an externally maintained definition that is adhered to in whole or in part by this Contract.", 13628 0, 1, instantiatesUri); 13629 case -92412192: 13630 /* contentDerivative */ return new Property("contentDerivative", "CodeableConcept", 13631 "The minimal content derived from the basal information source at a specific stage in its lifecycle.", 0, 1, 13632 contentDerivative); 13633 case -1179159893: 13634 /* issued */ return new Property("issued", "dateTime", "When this Contract was issued.", 0, 1, issued); 13635 case -793235316: 13636 /* applies */ return new Property("applies", "Period", 13637 "Relevant time or time-period when this Contract is applicable.", 0, 1, applies); 13638 case -668311927: 13639 /* expirationType */ return new Property("expirationType", "CodeableConcept", 13640 "Event resulting in discontinuation or termination of this Contract instance by one or more parties to the contract.", 13641 0, 1, expirationType); 13642 case -1867885268: 13643 /* subject */ return new Property("subject", "Reference(Any)", 13644 "The target entity impacted by or of interest to parties to the agreement.", 0, java.lang.Integer.MAX_VALUE, 13645 subject); 13646 case 1475610435: 13647 /* authority */ return new Property("authority", "Reference(Organization)", 13648 "A formally or informally recognized grouping of people, principals, organizations, or jurisdictions formed for the purpose of achieving some form of collective action such as the promulgation, administration and enforcement of contracts and policies.", 13649 0, java.lang.Integer.MAX_VALUE, authority); 13650 case -1326197564: 13651 /* domain */ return new Property("domain", "Reference(Location)", 13652 "Recognized governance framework or system operating with a circumscribed scope in accordance with specified principles, policies, processes or procedures for managing rights, actions, or behaviors of parties or principals relative to resources.", 13653 0, java.lang.Integer.MAX_VALUE, domain); 13654 case 3530567: 13655 /* site */ return new Property("site", "Reference(Location)", 13656 "Sites in which the contract is complied with, exercised, or in force.", 0, java.lang.Integer.MAX_VALUE, 13657 site); 13658 case 3373707: 13659 /* name */ return new Property("name", "string", 13660 "A natural language name identifying this Contract definition, derivative, or instance in any legal state. Provides additional information about its content. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 13661 0, 1, name); 13662 case 110371416: 13663 /* title */ return new Property("title", "string", 13664 "A short, descriptive, user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13665 0, 1, title); 13666 case -2060497896: 13667 /* subtitle */ return new Property("subtitle", "string", 13668 "An explanatory or alternate user-friendly title for this Contract definition, derivative, or instance in any legal state.t giving additional information about its content.", 13669 0, 1, subtitle); 13670 case 92902992: 13671 /* alias */ return new Property("alias", "string", 13672 "Alternative representation of the title for this Contract definition, derivative, or instance in any legal state., e.g., a domain specific contract number related to legislation.", 13673 0, java.lang.Integer.MAX_VALUE, alias); 13674 case -1406328437: 13675 /* author */ return new Property("author", "Reference(Patient|Practitioner|PractitionerRole|Organization)", 13676 "The individual or organization that authored the Contract definition, derivative, or instance in any legal state.", 13677 0, 1, author); 13678 case 109264468: 13679 /* scope */ return new Property("scope", "CodeableConcept", 13680 "A selector of legal concerns for this Contract definition, derivative, or instance in any legal state.", 0, 13681 1, scope); 13682 case -957295375: 13683 /* topic[x] */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13684 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13685 topic); 13686 case 110546223: 13687 /* topic */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13688 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13689 topic); 13690 case 777778802: 13691 /* topicCodeableConcept */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13692 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13693 topic); 13694 case -343345444: 13695 /* topicReference */ return new Property("topic[x]", "CodeableConcept|Reference(Any)", 13696 "Narrows the range of legal concerns to focus on the achievement of specific contractual objectives.", 0, 1, 13697 topic); 13698 case 3575610: 13699 /* type */ return new Property("type", "CodeableConcept", 13700 "A high-level category for the legal instrument, whether constructed as a Contract definition, derivative, or instance in any legal state. Provides additional information about its content within the context of the Contract's scope to distinguish the kinds of systems that would be interested in the contract.", 13701 0, 1, type); 13702 case -1868521062: 13703 /* subType */ return new Property("subType", "CodeableConcept", 13704 "Sub-category for the Contract that distinguishes the kinds of systems that would be interested in the Contract within the context of the Contract's scope.", 13705 0, java.lang.Integer.MAX_VALUE, subType); 13706 case 247055020: 13707 /* contentDefinition */ return new Property("contentDefinition", "", 13708 "Precusory content developed with a focus and intent of supporting the formation a Contract instance, which may be associated with and transformable into a Contract.", 13709 0, 1, contentDefinition); 13710 case 3556460: 13711 /* term */ return new Property("term", "", 13712 "One or more Contract Provisions, which may be related and conveyed as a group, and may contain nested groups.", 13713 0, java.lang.Integer.MAX_VALUE, term); 13714 case 1922406657: 13715 /* supportingInfo */ return new Property("supportingInfo", "Reference(Any)", 13716 "Information that may be needed by/relevant to the performer in their execution of this term action.", 0, 13717 java.lang.Integer.MAX_VALUE, supportingInfo); 13718 case 1538891575: 13719 /* relevantHistory */ return new Property("relevantHistory", "Reference(Provenance)", 13720 "Links to Provenance records for past versions of this Contract definition, derivative, or instance, which identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the Contract. The Provence.entity indicates the target that was changed in the update. http://build.fhir.org/provenance-definitions.html#Provenance.entity.", 13721 0, java.lang.Integer.MAX_VALUE, relevantHistory); 13722 case -902467798: 13723 /* signer */ return new Property("signer", "", 13724 "Parties with legal standing in the Contract, including the principal parties, the grantor(s) and grantee(s), which are any person or organization bound by the contract, and any ancillary parties, which facilitate the execution of the contract such as a notary or witness.", 13725 0, java.lang.Integer.MAX_VALUE, signer); 13726 case -1423054677: 13727 /* friendly */ return new Property("friendly", "", 13728 "The \"patient friendly language\" versionof the Contract in whole or in parts. \"Patient friendly language\" means the representation of the Contract and Contract Provisions in a manner that is readily accessible and understandable by a layperson in accordance with best practices for communication styles that ensure that those agreeing to or signing the Contract understand the roles, actions, obligations, responsibilities, and implication of the agreement.", 13729 0, java.lang.Integer.MAX_VALUE, friendly); 13730 case 102851257: 13731 /* legal */ return new Property("legal", "", "List of Legal expressions or representations of this Contract.", 0, 13732 java.lang.Integer.MAX_VALUE, legal); 13733 case 3512060: 13734 /* rule */ return new Property("rule", "", 13735 "List of Computable Policy Rule Language Representations of this Contract.", 0, java.lang.Integer.MAX_VALUE, 13736 rule); 13737 case -772497791: 13738 /* legallyBinding[x] */ return new Property("legallyBinding[x]", 13739 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13740 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13741 0, 1, legallyBinding); 13742 case -126751329: 13743 /* legallyBinding */ return new Property("legallyBinding[x]", 13744 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13745 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13746 0, 1, legallyBinding); 13747 case 344057890: 13748 /* legallyBindingAttachment */ return new Property("legallyBinding[x]", 13749 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13750 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13751 0, 1, legallyBinding); 13752 case -296528788: 13753 /* legallyBindingReference */ return new Property("legallyBinding[x]", 13754 "Attachment|Reference(Composition|DocumentReference|QuestionnaireResponse|Contract)", 13755 "Legally binding Contract: This is the signed and legally recognized representation of the Contract, which is considered the \"source of truth\" and which would be the basis for legal action related to enforcement of this Contract.", 13756 0, 1, legallyBinding); 13757 default: 13758 return super.getNamedProperty(_hash, _name, _checkValid); 13759 } 13760 13761 } 13762 13763 @Override 13764 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13765 switch (hash) { 13766 case -1618432855: 13767 /* identifier */ return this.identifier == null ? new Base[0] 13768 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 13769 case 116079: 13770 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 13771 case 351608024: 13772 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 13773 case -892481550: 13774 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ContractStatus> 13775 case 568606040: 13776 /* legalState */ return this.legalState == null ? new Base[0] : new Base[] { this.legalState }; // CodeableConcept 13777 case 8911915: 13778 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 13779 : new Base[] { this.instantiatesCanonical }; // Reference 13780 case -1926393373: 13781 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] : new Base[] { this.instantiatesUri }; // UriType 13782 case -92412192: 13783 /* contentDerivative */ return this.contentDerivative == null ? new Base[0] 13784 : new Base[] { this.contentDerivative }; // CodeableConcept 13785 case -1179159893: 13786 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // DateTimeType 13787 case -793235316: 13788 /* applies */ return this.applies == null ? new Base[0] : new Base[] { this.applies }; // Period 13789 case -668311927: 13790 /* expirationType */ return this.expirationType == null ? new Base[0] : new Base[] { this.expirationType }; // CodeableConcept 13791 case -1867885268: 13792 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 13793 case 1475610435: 13794 /* authority */ return this.authority == null ? new Base[0] 13795 : this.authority.toArray(new Base[this.authority.size()]); // Reference 13796 case -1326197564: 13797 /* domain */ return this.domain == null ? new Base[0] : this.domain.toArray(new Base[this.domain.size()]); // Reference 13798 case 3530567: 13799 /* site */ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // Reference 13800 case 3373707: 13801 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 13802 case 110371416: 13803 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 13804 case -2060497896: 13805 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 13806 case 92902992: 13807 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 13808 case -1406328437: 13809 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 13810 case 109264468: 13811 /* scope */ return this.scope == null ? new Base[0] : new Base[] { this.scope }; // CodeableConcept 13812 case 110546223: 13813 /* topic */ return this.topic == null ? new Base[0] : new Base[] { this.topic }; // Type 13814 case 3575610: 13815 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 13816 case -1868521062: 13817 /* subType */ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 13818 case 247055020: 13819 /* contentDefinition */ return this.contentDefinition == null ? new Base[0] 13820 : new Base[] { this.contentDefinition }; // ContentDefinitionComponent 13821 case 3556460: 13822 /* term */ return this.term == null ? new Base[0] : this.term.toArray(new Base[this.term.size()]); // TermComponent 13823 case 1922406657: 13824 /* supportingInfo */ return this.supportingInfo == null ? new Base[0] 13825 : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // Reference 13826 case 1538891575: 13827 /* relevantHistory */ return this.relevantHistory == null ? new Base[0] 13828 : this.relevantHistory.toArray(new Base[this.relevantHistory.size()]); // Reference 13829 case -902467798: 13830 /* signer */ return this.signer == null ? new Base[0] : this.signer.toArray(new Base[this.signer.size()]); // SignatoryComponent 13831 case -1423054677: 13832 /* friendly */ return this.friendly == null ? new Base[0] : this.friendly.toArray(new Base[this.friendly.size()]); // FriendlyLanguageComponent 13833 case 102851257: 13834 /* legal */ return this.legal == null ? new Base[0] : this.legal.toArray(new Base[this.legal.size()]); // LegalLanguageComponent 13835 case 3512060: 13836 /* rule */ return this.rule == null ? new Base[0] : this.rule.toArray(new Base[this.rule.size()]); // ComputableLanguageComponent 13837 case -126751329: 13838 /* legallyBinding */ return this.legallyBinding == null ? new Base[0] : new Base[] { this.legallyBinding }; // Type 13839 default: 13840 return super.getProperty(hash, name, checkValid); 13841 } 13842 13843 } 13844 13845 @Override 13846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13847 switch (hash) { 13848 case -1618432855: // identifier 13849 this.getIdentifier().add(castToIdentifier(value)); // Identifier 13850 return value; 13851 case 116079: // url 13852 this.url = castToUri(value); // UriType 13853 return value; 13854 case 351608024: // version 13855 this.version = castToString(value); // StringType 13856 return value; 13857 case -892481550: // status 13858 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 13859 this.status = (Enumeration) value; // Enumeration<ContractStatus> 13860 return value; 13861 case 568606040: // legalState 13862 this.legalState = castToCodeableConcept(value); // CodeableConcept 13863 return value; 13864 case 8911915: // instantiatesCanonical 13865 this.instantiatesCanonical = castToReference(value); // Reference 13866 return value; 13867 case -1926393373: // instantiatesUri 13868 this.instantiatesUri = castToUri(value); // UriType 13869 return value; 13870 case -92412192: // contentDerivative 13871 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 13872 return value; 13873 case -1179159893: // issued 13874 this.issued = castToDateTime(value); // DateTimeType 13875 return value; 13876 case -793235316: // applies 13877 this.applies = castToPeriod(value); // Period 13878 return value; 13879 case -668311927: // expirationType 13880 this.expirationType = castToCodeableConcept(value); // CodeableConcept 13881 return value; 13882 case -1867885268: // subject 13883 this.getSubject().add(castToReference(value)); // Reference 13884 return value; 13885 case 1475610435: // authority 13886 this.getAuthority().add(castToReference(value)); // Reference 13887 return value; 13888 case -1326197564: // domain 13889 this.getDomain().add(castToReference(value)); // Reference 13890 return value; 13891 case 3530567: // site 13892 this.getSite().add(castToReference(value)); // Reference 13893 return value; 13894 case 3373707: // name 13895 this.name = castToString(value); // StringType 13896 return value; 13897 case 110371416: // title 13898 this.title = castToString(value); // StringType 13899 return value; 13900 case -2060497896: // subtitle 13901 this.subtitle = castToString(value); // StringType 13902 return value; 13903 case 92902992: // alias 13904 this.getAlias().add(castToString(value)); // StringType 13905 return value; 13906 case -1406328437: // author 13907 this.author = castToReference(value); // Reference 13908 return value; 13909 case 109264468: // scope 13910 this.scope = castToCodeableConcept(value); // CodeableConcept 13911 return value; 13912 case 110546223: // topic 13913 this.topic = castToType(value); // Type 13914 return value; 13915 case 3575610: // type 13916 this.type = castToCodeableConcept(value); // CodeableConcept 13917 return value; 13918 case -1868521062: // subType 13919 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 13920 return value; 13921 case 247055020: // contentDefinition 13922 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 13923 return value; 13924 case 3556460: // term 13925 this.getTerm().add((TermComponent) value); // TermComponent 13926 return value; 13927 case 1922406657: // supportingInfo 13928 this.getSupportingInfo().add(castToReference(value)); // Reference 13929 return value; 13930 case 1538891575: // relevantHistory 13931 this.getRelevantHistory().add(castToReference(value)); // Reference 13932 return value; 13933 case -902467798: // signer 13934 this.getSigner().add((SignatoryComponent) value); // SignatoryComponent 13935 return value; 13936 case -1423054677: // friendly 13937 this.getFriendly().add((FriendlyLanguageComponent) value); // FriendlyLanguageComponent 13938 return value; 13939 case 102851257: // legal 13940 this.getLegal().add((LegalLanguageComponent) value); // LegalLanguageComponent 13941 return value; 13942 case 3512060: // rule 13943 this.getRule().add((ComputableLanguageComponent) value); // ComputableLanguageComponent 13944 return value; 13945 case -126751329: // legallyBinding 13946 this.legallyBinding = castToType(value); // Type 13947 return value; 13948 default: 13949 return super.setProperty(hash, name, value); 13950 } 13951 13952 } 13953 13954 @Override 13955 public Base setProperty(String name, Base value) throws FHIRException { 13956 if (name.equals("identifier")) { 13957 this.getIdentifier().add(castToIdentifier(value)); 13958 } else if (name.equals("url")) { 13959 this.url = castToUri(value); // UriType 13960 } else if (name.equals("version")) { 13961 this.version = castToString(value); // StringType 13962 } else if (name.equals("status")) { 13963 value = new ContractStatusEnumFactory().fromType(castToCode(value)); 13964 this.status = (Enumeration) value; // Enumeration<ContractStatus> 13965 } else if (name.equals("legalState")) { 13966 this.legalState = castToCodeableConcept(value); // CodeableConcept 13967 } else if (name.equals("instantiatesCanonical")) { 13968 this.instantiatesCanonical = castToReference(value); // Reference 13969 } else if (name.equals("instantiatesUri")) { 13970 this.instantiatesUri = castToUri(value); // UriType 13971 } else if (name.equals("contentDerivative")) { 13972 this.contentDerivative = castToCodeableConcept(value); // CodeableConcept 13973 } else if (name.equals("issued")) { 13974 this.issued = castToDateTime(value); // DateTimeType 13975 } else if (name.equals("applies")) { 13976 this.applies = castToPeriod(value); // Period 13977 } else if (name.equals("expirationType")) { 13978 this.expirationType = castToCodeableConcept(value); // CodeableConcept 13979 } else if (name.equals("subject")) { 13980 this.getSubject().add(castToReference(value)); 13981 } else if (name.equals("authority")) { 13982 this.getAuthority().add(castToReference(value)); 13983 } else if (name.equals("domain")) { 13984 this.getDomain().add(castToReference(value)); 13985 } else if (name.equals("site")) { 13986 this.getSite().add(castToReference(value)); 13987 } else if (name.equals("name")) { 13988 this.name = castToString(value); // StringType 13989 } else if (name.equals("title")) { 13990 this.title = castToString(value); // StringType 13991 } else if (name.equals("subtitle")) { 13992 this.subtitle = castToString(value); // StringType 13993 } else if (name.equals("alias")) { 13994 this.getAlias().add(castToString(value)); 13995 } else if (name.equals("author")) { 13996 this.author = castToReference(value); // Reference 13997 } else if (name.equals("scope")) { 13998 this.scope = castToCodeableConcept(value); // CodeableConcept 13999 } else if (name.equals("topic[x]")) { 14000 this.topic = castToType(value); // Type 14001 } else if (name.equals("type")) { 14002 this.type = castToCodeableConcept(value); // CodeableConcept 14003 } else if (name.equals("subType")) { 14004 this.getSubType().add(castToCodeableConcept(value)); 14005 } else if (name.equals("contentDefinition")) { 14006 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 14007 } else if (name.equals("term")) { 14008 this.getTerm().add((TermComponent) value); 14009 } else if (name.equals("supportingInfo")) { 14010 this.getSupportingInfo().add(castToReference(value)); 14011 } else if (name.equals("relevantHistory")) { 14012 this.getRelevantHistory().add(castToReference(value)); 14013 } else if (name.equals("signer")) { 14014 this.getSigner().add((SignatoryComponent) value); 14015 } else if (name.equals("friendly")) { 14016 this.getFriendly().add((FriendlyLanguageComponent) value); 14017 } else if (name.equals("legal")) { 14018 this.getLegal().add((LegalLanguageComponent) value); 14019 } else if (name.equals("rule")) { 14020 this.getRule().add((ComputableLanguageComponent) value); 14021 } else if (name.equals("legallyBinding[x]")) { 14022 this.legallyBinding = castToType(value); // Type 14023 } else 14024 return super.setProperty(name, value); 14025 return value; 14026 } 14027 14028 @Override 14029 public void removeChild(String name, Base value) throws FHIRException { 14030 if (name.equals("identifier")) { 14031 this.getIdentifier().remove(castToIdentifier(value)); 14032 } else if (name.equals("url")) { 14033 this.url = null; 14034 } else if (name.equals("version")) { 14035 this.version = null; 14036 } else if (name.equals("status")) { 14037 this.status = null; 14038 } else if (name.equals("legalState")) { 14039 this.legalState = null; 14040 } else if (name.equals("instantiatesCanonical")) { 14041 this.instantiatesCanonical = null; 14042 } else if (name.equals("instantiatesUri")) { 14043 this.instantiatesUri = null; 14044 } else if (name.equals("contentDerivative")) { 14045 this.contentDerivative = null; 14046 } else if (name.equals("issued")) { 14047 this.issued = null; 14048 } else if (name.equals("applies")) { 14049 this.applies = null; 14050 } else if (name.equals("expirationType")) { 14051 this.expirationType = null; 14052 } else if (name.equals("subject")) { 14053 this.getSubject().remove(castToReference(value)); 14054 } else if (name.equals("authority")) { 14055 this.getAuthority().remove(castToReference(value)); 14056 } else if (name.equals("domain")) { 14057 this.getDomain().remove(castToReference(value)); 14058 } else if (name.equals("site")) { 14059 this.getSite().remove(castToReference(value)); 14060 } else if (name.equals("name")) { 14061 this.name = null; 14062 } else if (name.equals("title")) { 14063 this.title = null; 14064 } else if (name.equals("subtitle")) { 14065 this.subtitle = null; 14066 } else if (name.equals("alias")) { 14067 this.getAlias().remove(castToString(value)); 14068 } else if (name.equals("author")) { 14069 this.author = null; 14070 } else if (name.equals("scope")) { 14071 this.scope = null; 14072 } else if (name.equals("topic[x]")) { 14073 this.topic = null; 14074 } else if (name.equals("type")) { 14075 this.type = null; 14076 } else if (name.equals("subType")) { 14077 this.getSubType().remove(castToCodeableConcept(value)); 14078 } else if (name.equals("contentDefinition")) { 14079 this.contentDefinition = (ContentDefinitionComponent) value; // ContentDefinitionComponent 14080 } else if (name.equals("term")) { 14081 this.getTerm().remove((TermComponent) value); 14082 } else if (name.equals("supportingInfo")) { 14083 this.getSupportingInfo().remove(castToReference(value)); 14084 } else if (name.equals("relevantHistory")) { 14085 this.getRelevantHistory().remove(castToReference(value)); 14086 } else if (name.equals("signer")) { 14087 this.getSigner().remove((SignatoryComponent) value); 14088 } else if (name.equals("friendly")) { 14089 this.getFriendly().remove((FriendlyLanguageComponent) value); 14090 } else if (name.equals("legal")) { 14091 this.getLegal().remove((LegalLanguageComponent) value); 14092 } else if (name.equals("rule")) { 14093 this.getRule().remove((ComputableLanguageComponent) value); 14094 } else if (name.equals("legallyBinding[x]")) { 14095 this.legallyBinding = null; 14096 } else 14097 super.removeChild(name, value); 14098 14099 } 14100 14101 @Override 14102 public Base makeProperty(int hash, String name) throws FHIRException { 14103 switch (hash) { 14104 case -1618432855: 14105 return addIdentifier(); 14106 case 116079: 14107 return getUrlElement(); 14108 case 351608024: 14109 return getVersionElement(); 14110 case -892481550: 14111 return getStatusElement(); 14112 case 568606040: 14113 return getLegalState(); 14114 case 8911915: 14115 return getInstantiatesCanonical(); 14116 case -1926393373: 14117 return getInstantiatesUriElement(); 14118 case -92412192: 14119 return getContentDerivative(); 14120 case -1179159893: 14121 return getIssuedElement(); 14122 case -793235316: 14123 return getApplies(); 14124 case -668311927: 14125 return getExpirationType(); 14126 case -1867885268: 14127 return addSubject(); 14128 case 1475610435: 14129 return addAuthority(); 14130 case -1326197564: 14131 return addDomain(); 14132 case 3530567: 14133 return addSite(); 14134 case 3373707: 14135 return getNameElement(); 14136 case 110371416: 14137 return getTitleElement(); 14138 case -2060497896: 14139 return getSubtitleElement(); 14140 case 92902992: 14141 return addAliasElement(); 14142 case -1406328437: 14143 return getAuthor(); 14144 case 109264468: 14145 return getScope(); 14146 case -957295375: 14147 return getTopic(); 14148 case 110546223: 14149 return getTopic(); 14150 case 3575610: 14151 return getType(); 14152 case -1868521062: 14153 return addSubType(); 14154 case 247055020: 14155 return getContentDefinition(); 14156 case 3556460: 14157 return addTerm(); 14158 case 1922406657: 14159 return addSupportingInfo(); 14160 case 1538891575: 14161 return addRelevantHistory(); 14162 case -902467798: 14163 return addSigner(); 14164 case -1423054677: 14165 return addFriendly(); 14166 case 102851257: 14167 return addLegal(); 14168 case 3512060: 14169 return addRule(); 14170 case -772497791: 14171 return getLegallyBinding(); 14172 case -126751329: 14173 return getLegallyBinding(); 14174 default: 14175 return super.makeProperty(hash, name); 14176 } 14177 14178 } 14179 14180 @Override 14181 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14182 switch (hash) { 14183 case -1618432855: 14184 /* identifier */ return new String[] { "Identifier" }; 14185 case 116079: 14186 /* url */ return new String[] { "uri" }; 14187 case 351608024: 14188 /* version */ return new String[] { "string" }; 14189 case -892481550: 14190 /* status */ return new String[] { "code" }; 14191 case 568606040: 14192 /* legalState */ return new String[] { "CodeableConcept" }; 14193 case 8911915: 14194 /* instantiatesCanonical */ return new String[] { "Reference" }; 14195 case -1926393373: 14196 /* instantiatesUri */ return new String[] { "uri" }; 14197 case -92412192: 14198 /* contentDerivative */ return new String[] { "CodeableConcept" }; 14199 case -1179159893: 14200 /* issued */ return new String[] { "dateTime" }; 14201 case -793235316: 14202 /* applies */ return new String[] { "Period" }; 14203 case -668311927: 14204 /* expirationType */ return new String[] { "CodeableConcept" }; 14205 case -1867885268: 14206 /* subject */ return new String[] { "Reference" }; 14207 case 1475610435: 14208 /* authority */ return new String[] { "Reference" }; 14209 case -1326197564: 14210 /* domain */ return new String[] { "Reference" }; 14211 case 3530567: 14212 /* site */ return new String[] { "Reference" }; 14213 case 3373707: 14214 /* name */ return new String[] { "string" }; 14215 case 110371416: 14216 /* title */ return new String[] { "string" }; 14217 case -2060497896: 14218 /* subtitle */ return new String[] { "string" }; 14219 case 92902992: 14220 /* alias */ return new String[] { "string" }; 14221 case -1406328437: 14222 /* author */ return new String[] { "Reference" }; 14223 case 109264468: 14224 /* scope */ return new String[] { "CodeableConcept" }; 14225 case 110546223: 14226 /* topic */ return new String[] { "CodeableConcept", "Reference" }; 14227 case 3575610: 14228 /* type */ return new String[] { "CodeableConcept" }; 14229 case -1868521062: 14230 /* subType */ return new String[] { "CodeableConcept" }; 14231 case 247055020: 14232 /* contentDefinition */ return new String[] {}; 14233 case 3556460: 14234 /* term */ return new String[] {}; 14235 case 1922406657: 14236 /* supportingInfo */ return new String[] { "Reference" }; 14237 case 1538891575: 14238 /* relevantHistory */ return new String[] { "Reference" }; 14239 case -902467798: 14240 /* signer */ return new String[] {}; 14241 case -1423054677: 14242 /* friendly */ return new String[] {}; 14243 case 102851257: 14244 /* legal */ return new String[] {}; 14245 case 3512060: 14246 /* rule */ return new String[] {}; 14247 case -126751329: 14248 /* legallyBinding */ return new String[] { "Attachment", "Reference" }; 14249 default: 14250 return super.getTypesForProperty(hash, name); 14251 } 14252 14253 } 14254 14255 @Override 14256 public Base addChild(String name) throws FHIRException { 14257 if (name.equals("identifier")) { 14258 return addIdentifier(); 14259 } else if (name.equals("url")) { 14260 throw new FHIRException("Cannot call addChild on a singleton property Contract.url"); 14261 } else if (name.equals("version")) { 14262 throw new FHIRException("Cannot call addChild on a singleton property Contract.version"); 14263 } else if (name.equals("status")) { 14264 throw new FHIRException("Cannot call addChild on a singleton property Contract.status"); 14265 } else if (name.equals("legalState")) { 14266 this.legalState = new CodeableConcept(); 14267 return this.legalState; 14268 } else if (name.equals("instantiatesCanonical")) { 14269 this.instantiatesCanonical = new Reference(); 14270 return this.instantiatesCanonical; 14271 } else if (name.equals("instantiatesUri")) { 14272 throw new FHIRException("Cannot call addChild on a singleton property Contract.instantiatesUri"); 14273 } else if (name.equals("contentDerivative")) { 14274 this.contentDerivative = new CodeableConcept(); 14275 return this.contentDerivative; 14276 } else if (name.equals("issued")) { 14277 throw new FHIRException("Cannot call addChild on a singleton property Contract.issued"); 14278 } else if (name.equals("applies")) { 14279 this.applies = new Period(); 14280 return this.applies; 14281 } else if (name.equals("expirationType")) { 14282 this.expirationType = new CodeableConcept(); 14283 return this.expirationType; 14284 } else if (name.equals("subject")) { 14285 return addSubject(); 14286 } else if (name.equals("authority")) { 14287 return addAuthority(); 14288 } else if (name.equals("domain")) { 14289 return addDomain(); 14290 } else if (name.equals("site")) { 14291 return addSite(); 14292 } else if (name.equals("name")) { 14293 throw new FHIRException("Cannot call addChild on a singleton property Contract.name"); 14294 } else if (name.equals("title")) { 14295 throw new FHIRException("Cannot call addChild on a singleton property Contract.title"); 14296 } else if (name.equals("subtitle")) { 14297 throw new FHIRException("Cannot call addChild on a singleton property Contract.subtitle"); 14298 } else if (name.equals("alias")) { 14299 throw new FHIRException("Cannot call addChild on a singleton property Contract.alias"); 14300 } else if (name.equals("author")) { 14301 this.author = new Reference(); 14302 return this.author; 14303 } else if (name.equals("scope")) { 14304 this.scope = new CodeableConcept(); 14305 return this.scope; 14306 } else if (name.equals("topicCodeableConcept")) { 14307 this.topic = new CodeableConcept(); 14308 return this.topic; 14309 } else if (name.equals("topicReference")) { 14310 this.topic = new Reference(); 14311 return this.topic; 14312 } else if (name.equals("type")) { 14313 this.type = new CodeableConcept(); 14314 return this.type; 14315 } else if (name.equals("subType")) { 14316 return addSubType(); 14317 } else if (name.equals("contentDefinition")) { 14318 this.contentDefinition = new ContentDefinitionComponent(); 14319 return this.contentDefinition; 14320 } else if (name.equals("term")) { 14321 return addTerm(); 14322 } else if (name.equals("supportingInfo")) { 14323 return addSupportingInfo(); 14324 } else if (name.equals("relevantHistory")) { 14325 return addRelevantHistory(); 14326 } else if (name.equals("signer")) { 14327 return addSigner(); 14328 } else if (name.equals("friendly")) { 14329 return addFriendly(); 14330 } else if (name.equals("legal")) { 14331 return addLegal(); 14332 } else if (name.equals("rule")) { 14333 return addRule(); 14334 } else if (name.equals("legallyBindingAttachment")) { 14335 this.legallyBinding = new Attachment(); 14336 return this.legallyBinding; 14337 } else if (name.equals("legallyBindingReference")) { 14338 this.legallyBinding = new Reference(); 14339 return this.legallyBinding; 14340 } else 14341 return super.addChild(name); 14342 } 14343 14344 public String fhirType() { 14345 return "Contract"; 14346 14347 } 14348 14349 public Contract copy() { 14350 Contract dst = new Contract(); 14351 copyValues(dst); 14352 return dst; 14353 } 14354 14355 public void copyValues(Contract dst) { 14356 super.copyValues(dst); 14357 if (identifier != null) { 14358 dst.identifier = new ArrayList<Identifier>(); 14359 for (Identifier i : identifier) 14360 dst.identifier.add(i.copy()); 14361 } 14362 ; 14363 dst.url = url == null ? null : url.copy(); 14364 dst.version = version == null ? null : version.copy(); 14365 dst.status = status == null ? null : status.copy(); 14366 dst.legalState = legalState == null ? null : legalState.copy(); 14367 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 14368 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 14369 dst.contentDerivative = contentDerivative == null ? null : contentDerivative.copy(); 14370 dst.issued = issued == null ? null : issued.copy(); 14371 dst.applies = applies == null ? null : applies.copy(); 14372 dst.expirationType = expirationType == null ? null : expirationType.copy(); 14373 if (subject != null) { 14374 dst.subject = new ArrayList<Reference>(); 14375 for (Reference i : subject) 14376 dst.subject.add(i.copy()); 14377 } 14378 ; 14379 if (authority != null) { 14380 dst.authority = new ArrayList<Reference>(); 14381 for (Reference i : authority) 14382 dst.authority.add(i.copy()); 14383 } 14384 ; 14385 if (domain != null) { 14386 dst.domain = new ArrayList<Reference>(); 14387 for (Reference i : domain) 14388 dst.domain.add(i.copy()); 14389 } 14390 ; 14391 if (site != null) { 14392 dst.site = new ArrayList<Reference>(); 14393 for (Reference i : site) 14394 dst.site.add(i.copy()); 14395 } 14396 ; 14397 dst.name = name == null ? null : name.copy(); 14398 dst.title = title == null ? null : title.copy(); 14399 dst.subtitle = subtitle == null ? null : subtitle.copy(); 14400 if (alias != null) { 14401 dst.alias = new ArrayList<StringType>(); 14402 for (StringType i : alias) 14403 dst.alias.add(i.copy()); 14404 } 14405 ; 14406 dst.author = author == null ? null : author.copy(); 14407 dst.scope = scope == null ? null : scope.copy(); 14408 dst.topic = topic == null ? null : topic.copy(); 14409 dst.type = type == null ? null : type.copy(); 14410 if (subType != null) { 14411 dst.subType = new ArrayList<CodeableConcept>(); 14412 for (CodeableConcept i : subType) 14413 dst.subType.add(i.copy()); 14414 } 14415 ; 14416 dst.contentDefinition = contentDefinition == null ? null : contentDefinition.copy(); 14417 if (term != null) { 14418 dst.term = new ArrayList<TermComponent>(); 14419 for (TermComponent i : term) 14420 dst.term.add(i.copy()); 14421 } 14422 ; 14423 if (supportingInfo != null) { 14424 dst.supportingInfo = new ArrayList<Reference>(); 14425 for (Reference i : supportingInfo) 14426 dst.supportingInfo.add(i.copy()); 14427 } 14428 ; 14429 if (relevantHistory != null) { 14430 dst.relevantHistory = new ArrayList<Reference>(); 14431 for (Reference i : relevantHistory) 14432 dst.relevantHistory.add(i.copy()); 14433 } 14434 ; 14435 if (signer != null) { 14436 dst.signer = new ArrayList<SignatoryComponent>(); 14437 for (SignatoryComponent i : signer) 14438 dst.signer.add(i.copy()); 14439 } 14440 ; 14441 if (friendly != null) { 14442 dst.friendly = new ArrayList<FriendlyLanguageComponent>(); 14443 for (FriendlyLanguageComponent i : friendly) 14444 dst.friendly.add(i.copy()); 14445 } 14446 ; 14447 if (legal != null) { 14448 dst.legal = new ArrayList<LegalLanguageComponent>(); 14449 for (LegalLanguageComponent i : legal) 14450 dst.legal.add(i.copy()); 14451 } 14452 ; 14453 if (rule != null) { 14454 dst.rule = new ArrayList<ComputableLanguageComponent>(); 14455 for (ComputableLanguageComponent i : rule) 14456 dst.rule.add(i.copy()); 14457 } 14458 ; 14459 dst.legallyBinding = legallyBinding == null ? null : legallyBinding.copy(); 14460 } 14461 14462 protected Contract typedCopy() { 14463 return copy(); 14464 } 14465 14466 @Override 14467 public boolean equalsDeep(Base other_) { 14468 if (!super.equalsDeep(other_)) 14469 return false; 14470 if (!(other_ instanceof Contract)) 14471 return false; 14472 Contract o = (Contract) other_; 14473 return compareDeep(identifier, o.identifier, true) && compareDeep(url, o.url, true) 14474 && compareDeep(version, o.version, true) && compareDeep(status, o.status, true) 14475 && compareDeep(legalState, o.legalState, true) 14476 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 14477 && compareDeep(instantiatesUri, o.instantiatesUri, true) 14478 && compareDeep(contentDerivative, o.contentDerivative, true) && compareDeep(issued, o.issued, true) 14479 && compareDeep(applies, o.applies, true) && compareDeep(expirationType, o.expirationType, true) 14480 && compareDeep(subject, o.subject, true) && compareDeep(authority, o.authority, true) 14481 && compareDeep(domain, o.domain, true) && compareDeep(site, o.site, true) && compareDeep(name, o.name, true) 14482 && compareDeep(title, o.title, true) && compareDeep(subtitle, o.subtitle, true) 14483 && compareDeep(alias, o.alias, true) && compareDeep(author, o.author, true) && compareDeep(scope, o.scope, true) 14484 && compareDeep(topic, o.topic, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 14485 && compareDeep(contentDefinition, o.contentDefinition, true) && compareDeep(term, o.term, true) 14486 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(relevantHistory, o.relevantHistory, true) 14487 && compareDeep(signer, o.signer, true) && compareDeep(friendly, o.friendly, true) 14488 && compareDeep(legal, o.legal, true) && compareDeep(rule, o.rule, true) 14489 && compareDeep(legallyBinding, o.legallyBinding, true); 14490 } 14491 14492 @Override 14493 public boolean equalsShallow(Base other_) { 14494 if (!super.equalsShallow(other_)) 14495 return false; 14496 if (!(other_ instanceof Contract)) 14497 return false; 14498 Contract o = (Contract) other_; 14499 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 14500 && compareValues(status, o.status, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 14501 && compareValues(issued, o.issued, true) && compareValues(name, o.name, true) 14502 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) 14503 && compareValues(alias, o.alias, true); 14504 } 14505 14506 public boolean isEmpty() { 14507 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, url, version, status, legalState, 14508 instantiatesCanonical, instantiatesUri, contentDerivative, issued, applies, expirationType, subject, authority, 14509 domain, site, name, title, subtitle, alias, author, scope, topic, type, subType, contentDefinition, term, 14510 supportingInfo, relevantHistory, signer, friendly, legal, rule, legallyBinding); 14511 } 14512 14513 @Override 14514 public ResourceType getResourceType() { 14515 return ResourceType.Contract; 14516 } 14517 14518 /** 14519 * Search parameter: <b>identifier</b> 14520 * <p> 14521 * Description: <b>The identity of the contract</b><br> 14522 * Type: <b>token</b><br> 14523 * Path: <b>Contract.identifier</b><br> 14524 * </p> 14525 */ 14526 @SearchParamDefinition(name = "identifier", path = "Contract.identifier", description = "The identity of the contract", type = "token") 14527 public static final String SP_IDENTIFIER = "identifier"; 14528 /** 14529 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 14530 * <p> 14531 * Description: <b>The identity of the contract</b><br> 14532 * Type: <b>token</b><br> 14533 * Path: <b>Contract.identifier</b><br> 14534 * </p> 14535 */ 14536 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 14537 SP_IDENTIFIER); 14538 14539 /** 14540 * Search parameter: <b>instantiates</b> 14541 * <p> 14542 * Description: <b>A source definition of the contract</b><br> 14543 * Type: <b>uri</b><br> 14544 * Path: <b>Contract.instantiatesUri</b><br> 14545 * </p> 14546 */ 14547 @SearchParamDefinition(name = "instantiates", path = "Contract.instantiatesUri", description = "A source definition of the contract", type = "uri") 14548 public static final String SP_INSTANTIATES = "instantiates"; 14549 /** 14550 * <b>Fluent Client</b> search parameter constant for <b>instantiates</b> 14551 * <p> 14552 * Description: <b>A source definition of the contract</b><br> 14553 * Type: <b>uri</b><br> 14554 * Path: <b>Contract.instantiatesUri</b><br> 14555 * </p> 14556 */ 14557 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES = new ca.uhn.fhir.rest.gclient.UriClientParam( 14558 SP_INSTANTIATES); 14559 14560 /** 14561 * Search parameter: <b>patient</b> 14562 * <p> 14563 * Description: <b>The identity of the subject of the contract (if a 14564 * patient)</b><br> 14565 * Type: <b>reference</b><br> 14566 * Path: <b>Contract.subject</b><br> 14567 * </p> 14568 */ 14569 @SearchParamDefinition(name = "patient", path = "Contract.subject.where(resolve() is Patient)", description = "The identity of the subject of the contract (if a patient)", type = "reference", target = { 14570 Patient.class }) 14571 public static final String SP_PATIENT = "patient"; 14572 /** 14573 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 14574 * <p> 14575 * Description: <b>The identity of the subject of the contract (if a 14576 * patient)</b><br> 14577 * Type: <b>reference</b><br> 14578 * Path: <b>Contract.subject</b><br> 14579 * </p> 14580 */ 14581 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14582 SP_PATIENT); 14583 14584 /** 14585 * Constant for fluent queries to be used to add include statements. Specifies 14586 * the path value of "<b>Contract:patient</b>". 14587 */ 14588 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 14589 "Contract:patient").toLocked(); 14590 14591 /** 14592 * Search parameter: <b>subject</b> 14593 * <p> 14594 * Description: <b>The identity of the subject of the contract</b><br> 14595 * Type: <b>reference</b><br> 14596 * Path: <b>Contract.subject</b><br> 14597 * </p> 14598 */ 14599 @SearchParamDefinition(name = "subject", path = "Contract.subject", description = "The identity of the subject of the contract", type = "reference") 14600 public static final String SP_SUBJECT = "subject"; 14601 /** 14602 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 14603 * <p> 14604 * Description: <b>The identity of the subject of the contract</b><br> 14605 * Type: <b>reference</b><br> 14606 * Path: <b>Contract.subject</b><br> 14607 * </p> 14608 */ 14609 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14610 SP_SUBJECT); 14611 14612 /** 14613 * Constant for fluent queries to be used to add include statements. Specifies 14614 * the path value of "<b>Contract:subject</b>". 14615 */ 14616 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 14617 "Contract:subject").toLocked(); 14618 14619 /** 14620 * Search parameter: <b>authority</b> 14621 * <p> 14622 * Description: <b>The authority of the contract</b><br> 14623 * Type: <b>reference</b><br> 14624 * Path: <b>Contract.authority</b><br> 14625 * </p> 14626 */ 14627 @SearchParamDefinition(name = "authority", path = "Contract.authority", description = "The authority of the contract", type = "reference", target = { 14628 Organization.class }) 14629 public static final String SP_AUTHORITY = "authority"; 14630 /** 14631 * <b>Fluent Client</b> search parameter constant for <b>authority</b> 14632 * <p> 14633 * Description: <b>The authority of the contract</b><br> 14634 * Type: <b>reference</b><br> 14635 * Path: <b>Contract.authority</b><br> 14636 * </p> 14637 */ 14638 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHORITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14639 SP_AUTHORITY); 14640 14641 /** 14642 * Constant for fluent queries to be used to add include statements. Specifies 14643 * the path value of "<b>Contract:authority</b>". 14644 */ 14645 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHORITY = new ca.uhn.fhir.model.api.Include( 14646 "Contract:authority").toLocked(); 14647 14648 /** 14649 * Search parameter: <b>domain</b> 14650 * <p> 14651 * Description: <b>The domain of the contract</b><br> 14652 * Type: <b>reference</b><br> 14653 * Path: <b>Contract.domain</b><br> 14654 * </p> 14655 */ 14656 @SearchParamDefinition(name = "domain", path = "Contract.domain", description = "The domain of the contract", type = "reference", target = { 14657 Location.class }) 14658 public static final String SP_DOMAIN = "domain"; 14659 /** 14660 * <b>Fluent Client</b> search parameter constant for <b>domain</b> 14661 * <p> 14662 * Description: <b>The domain of the contract</b><br> 14663 * Type: <b>reference</b><br> 14664 * Path: <b>Contract.domain</b><br> 14665 * </p> 14666 */ 14667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DOMAIN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14668 SP_DOMAIN); 14669 14670 /** 14671 * Constant for fluent queries to be used to add include statements. Specifies 14672 * the path value of "<b>Contract:domain</b>". 14673 */ 14674 public static final ca.uhn.fhir.model.api.Include INCLUDE_DOMAIN = new ca.uhn.fhir.model.api.Include( 14675 "Contract:domain").toLocked(); 14676 14677 /** 14678 * Search parameter: <b>issued</b> 14679 * <p> 14680 * Description: <b>The date/time the contract was issued</b><br> 14681 * Type: <b>date</b><br> 14682 * Path: <b>Contract.issued</b><br> 14683 * </p> 14684 */ 14685 @SearchParamDefinition(name = "issued", path = "Contract.issued", description = "The date/time the contract was issued", type = "date") 14686 public static final String SP_ISSUED = "issued"; 14687 /** 14688 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 14689 * <p> 14690 * Description: <b>The date/time the contract was issued</b><br> 14691 * Type: <b>date</b><br> 14692 * Path: <b>Contract.issued</b><br> 14693 * </p> 14694 */ 14695 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam( 14696 SP_ISSUED); 14697 14698 /** 14699 * Search parameter: <b>url</b> 14700 * <p> 14701 * Description: <b>The basal contract definition</b><br> 14702 * Type: <b>uri</b><br> 14703 * Path: <b>Contract.url</b><br> 14704 * </p> 14705 */ 14706 @SearchParamDefinition(name = "url", path = "Contract.url", description = "The basal contract definition", type = "uri") 14707 public static final String SP_URL = "url"; 14708 /** 14709 * <b>Fluent Client</b> search parameter constant for <b>url</b> 14710 * <p> 14711 * Description: <b>The basal contract definition</b><br> 14712 * Type: <b>uri</b><br> 14713 * Path: <b>Contract.url</b><br> 14714 * </p> 14715 */ 14716 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 14717 14718 /** 14719 * Search parameter: <b>signer</b> 14720 * <p> 14721 * Description: <b>Contract Signatory Party</b><br> 14722 * Type: <b>reference</b><br> 14723 * Path: <b>Contract.signer.party</b><br> 14724 * </p> 14725 */ 14726 @SearchParamDefinition(name = "signer", path = "Contract.signer.party", description = "Contract Signatory Party", type = "reference", target = { 14727 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 14728 public static final String SP_SIGNER = "signer"; 14729 /** 14730 * <b>Fluent Client</b> search parameter constant for <b>signer</b> 14731 * <p> 14732 * Description: <b>Contract Signatory Party</b><br> 14733 * Type: <b>reference</b><br> 14734 * Path: <b>Contract.signer.party</b><br> 14735 * </p> 14736 */ 14737 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SIGNER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 14738 SP_SIGNER); 14739 14740 /** 14741 * Constant for fluent queries to be used to add include statements. Specifies 14742 * the path value of "<b>Contract:signer</b>". 14743 */ 14744 public static final ca.uhn.fhir.model.api.Include INCLUDE_SIGNER = new ca.uhn.fhir.model.api.Include( 14745 "Contract:signer").toLocked(); 14746 14747 /** 14748 * Search parameter: <b>status</b> 14749 * <p> 14750 * Description: <b>The status of the contract</b><br> 14751 * Type: <b>token</b><br> 14752 * Path: <b>Contract.status</b><br> 14753 * </p> 14754 */ 14755 @SearchParamDefinition(name = "status", path = "Contract.status", description = "The status of the contract", type = "token") 14756 public static final String SP_STATUS = "status"; 14757 /** 14758 * <b>Fluent Client</b> search parameter constant for <b>status</b> 14759 * <p> 14760 * Description: <b>The status of the contract</b><br> 14761 * Type: <b>token</b><br> 14762 * Path: <b>Contract.status</b><br> 14763 * </p> 14764 */ 14765 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 14766 SP_STATUS); 14767 14768}