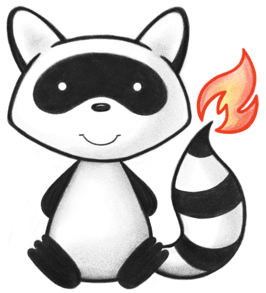
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.ICompositeType; 037 038import ca.uhn.fhir.model.api.annotation.DatatypeDef; 039 040/** 041 * A measured amount (or an amount that can potentially be measured). Note that 042 * measured amounts include amounts that are not precisely quantified, including 043 * amounts involving arbitrary units and floating currencies. 044 */ 045@DatatypeDef(name = "Count") 046public class Count extends Quantity implements ICompositeType { 047 048 private static final long serialVersionUID = 0L; 049 050 /** 051 * Constructor 052 */ 053 public Count() { 054 super(); 055 } 056 057 protected void listChildren(List<Property> children) { 058 super.listChildren(children); 059 } 060 061 @Override 062 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 063 switch (_hash) { 064 default: 065 return super.getNamedProperty(_hash, _name, _checkValid); 066 } 067 068 } 069 070 @Override 071 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 072 switch (hash) { 073 default: 074 return super.getProperty(hash, name, checkValid); 075 } 076 077 } 078 079 @Override 080 public Base setProperty(int hash, String name, Base value) throws FHIRException { 081 switch (hash) { 082 default: 083 return super.setProperty(hash, name, value); 084 } 085 086 } 087 088 @Override 089 public Base setProperty(String name, Base value) throws FHIRException { 090 return super.setProperty(name, value); 091 } 092 093 @Override 094 public void removeChild(String name, Base value) throws FHIRException { 095 super.removeChild(name, value); 096 } 097 098 @Override 099 public Base makeProperty(int hash, String name) throws FHIRException { 100 switch (hash) { 101 default: 102 return super.makeProperty(hash, name); 103 } 104 105 } 106 107 @Override 108 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 109 switch (hash) { 110 default: 111 return super.getTypesForProperty(hash, name); 112 } 113 114 } 115 116 @Override 117 public Base addChild(String name) throws FHIRException { 118 return super.addChild(name); 119 } 120 121 public String fhirType() { 122 return "Count"; 123 124 } 125 126 public Count copy() { 127 Count dst = new Count(); 128 copyValues(dst); 129 return dst; 130 } 131 132 public void copyValues(Count dst) { 133 super.copyValues(dst); 134 } 135 136 protected Count typedCopy() { 137 return copy(); 138 } 139 140 @Override 141 public boolean equalsDeep(Base other_) { 142 if (!super.equalsDeep(other_)) 143 return false; 144 if (!(other_ instanceof Count)) 145 return false; 146 Count o = (Count) other_; 147 return true; 148 } 149 150 @Override 151 public boolean equalsShallow(Base other_) { 152 if (!super.equalsShallow(other_)) 153 return false; 154 if (!(other_ instanceof Count)) 155 return false; 156 Count o = (Count) other_; 157 return true; 158 } 159 160 public boolean isEmpty() { 161 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 162 } 163 164}