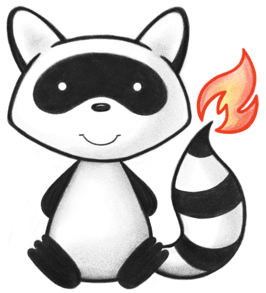
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Financial instrument which may be used to reimburse or pay for health care 048 * products and services. Includes both insurance and self-payment. 049 */ 050@ResourceDef(name = "Coverage", profile = "http://hl7.org/fhir/StructureDefinition/Coverage") 051public class Coverage extends DomainResource { 052 053 public enum CoverageStatus { 054 /** 055 * The instance is currently in-force. 056 */ 057 ACTIVE, 058 /** 059 * The instance is withdrawn, rescinded or reversed. 060 */ 061 CANCELLED, 062 /** 063 * A new instance the contents of which is not complete. 064 */ 065 DRAFT, 066 /** 067 * The instance was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 075 public static CoverageStatus fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("active".equals(codeString)) 079 return ACTIVE; 080 if ("cancelled".equals(codeString)) 081 return CANCELLED; 082 if ("draft".equals(codeString)) 083 return DRAFT; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown CoverageStatus code '" + codeString + "'"); 090 } 091 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: 095 return "active"; 096 case CANCELLED: 097 return "cancelled"; 098 case DRAFT: 099 return "draft"; 100 case ENTEREDINERROR: 101 return "entered-in-error"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case ACTIVE: 112 return "http://hl7.org/fhir/fm-status"; 113 case CANCELLED: 114 return "http://hl7.org/fhir/fm-status"; 115 case DRAFT: 116 return "http://hl7.org/fhir/fm-status"; 117 case ENTEREDINERROR: 118 return "http://hl7.org/fhir/fm-status"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case ACTIVE: 129 return "The instance is currently in-force."; 130 case CANCELLED: 131 return "The instance is withdrawn, rescinded or reversed."; 132 case DRAFT: 133 return "A new instance the contents of which is not complete."; 134 case ENTEREDINERROR: 135 return "The instance was entered in error."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case ACTIVE: 146 return "Active"; 147 case CANCELLED: 148 return "Cancelled"; 149 case DRAFT: 150 return "Draft"; 151 case ENTEREDINERROR: 152 return "Entered in Error"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 } 160 161 public static class CoverageStatusEnumFactory implements EnumFactory<CoverageStatus> { 162 public CoverageStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("active".equals(codeString)) 167 return CoverageStatus.ACTIVE; 168 if ("cancelled".equals(codeString)) 169 return CoverageStatus.CANCELLED; 170 if ("draft".equals(codeString)) 171 return CoverageStatus.DRAFT; 172 if ("entered-in-error".equals(codeString)) 173 return CoverageStatus.ENTEREDINERROR; 174 throw new IllegalArgumentException("Unknown CoverageStatus code '" + codeString + "'"); 175 } 176 177 public Enumeration<CoverageStatus> fromType(PrimitiveType<?> code) throws FHIRException { 178 if (code == null) 179 return null; 180 if (code.isEmpty()) 181 return new Enumeration<CoverageStatus>(this, CoverageStatus.NULL, code); 182 String codeString = code.asStringValue(); 183 if (codeString == null || "".equals(codeString)) 184 return new Enumeration<CoverageStatus>(this, CoverageStatus.NULL, code); 185 if ("active".equals(codeString)) 186 return new Enumeration<CoverageStatus>(this, CoverageStatus.ACTIVE, code); 187 if ("cancelled".equals(codeString)) 188 return new Enumeration<CoverageStatus>(this, CoverageStatus.CANCELLED, code); 189 if ("draft".equals(codeString)) 190 return new Enumeration<CoverageStatus>(this, CoverageStatus.DRAFT, code); 191 if ("entered-in-error".equals(codeString)) 192 return new Enumeration<CoverageStatus>(this, CoverageStatus.ENTEREDINERROR, code); 193 throw new FHIRException("Unknown CoverageStatus code '" + codeString + "'"); 194 } 195 196 public String toCode(CoverageStatus code) { 197 if (code == CoverageStatus.NULL) 198 return null; 199 if (code == CoverageStatus.ACTIVE) 200 return "active"; 201 if (code == CoverageStatus.CANCELLED) 202 return "cancelled"; 203 if (code == CoverageStatus.DRAFT) 204 return "draft"; 205 if (code == CoverageStatus.ENTEREDINERROR) 206 return "entered-in-error"; 207 return "?"; 208 } 209 210 public String toSystem(CoverageStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 @Block() 216 public static class ClassComponent extends BackboneElement implements IBaseBackboneElement { 217 /** 218 * The type of classification for which an insurer-specific class label or 219 * number and optional name is provided, for example may be used to identify a 220 * class of coverage or employer group, Policy, Plan. 221 */ 222 @Child(name = "type", type = { 223 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "Type of class such as 'group' or 'plan'", formalDefinition = "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.") 225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-class") 226 protected CodeableConcept type; 227 228 /** 229 * The alphanumeric string value associated with the insurer issued label. 230 */ 231 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 232 @Description(shortDefinition = "Value associated with the type", formalDefinition = "The alphanumeric string value associated with the insurer issued label.") 233 protected StringType value; 234 235 /** 236 * A short description for the class. 237 */ 238 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 239 @Description(shortDefinition = "Human readable description of the type and value", formalDefinition = "A short description for the class.") 240 protected StringType name; 241 242 private static final long serialVersionUID = -1501519769L; 243 244 /** 245 * Constructor 246 */ 247 public ClassComponent() { 248 super(); 249 } 250 251 /** 252 * Constructor 253 */ 254 public ClassComponent(CodeableConcept type, StringType value) { 255 super(); 256 this.type = type; 257 this.value = value; 258 } 259 260 /** 261 * @return {@link #type} (The type of classification for which an 262 * insurer-specific class label or number and optional name is provided, 263 * for example may be used to identify a class of coverage or employer 264 * group, Policy, Plan.) 265 */ 266 public CodeableConcept getType() { 267 if (this.type == null) 268 if (Configuration.errorOnAutoCreate()) 269 throw new Error("Attempt to auto-create ClassComponent.type"); 270 else if (Configuration.doAutoCreate()) 271 this.type = new CodeableConcept(); // cc 272 return this.type; 273 } 274 275 public boolean hasType() { 276 return this.type != null && !this.type.isEmpty(); 277 } 278 279 /** 280 * @param value {@link #type} (The type of classification for which an 281 * insurer-specific class label or number and optional name is 282 * provided, for example may be used to identify a class of 283 * coverage or employer group, Policy, Plan.) 284 */ 285 public ClassComponent setType(CodeableConcept value) { 286 this.type = value; 287 return this; 288 } 289 290 /** 291 * @return {@link #value} (The alphanumeric string value associated with the 292 * insurer issued label.). This is the underlying object with id, value 293 * and extensions. The accessor "getValue" gives direct access to the 294 * value 295 */ 296 public StringType getValueElement() { 297 if (this.value == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create ClassComponent.value"); 300 else if (Configuration.doAutoCreate()) 301 this.value = new StringType(); // bb 302 return this.value; 303 } 304 305 public boolean hasValueElement() { 306 return this.value != null && !this.value.isEmpty(); 307 } 308 309 public boolean hasValue() { 310 return this.value != null && !this.value.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #value} (The alphanumeric string value associated with 315 * the insurer issued label.). This is the underlying object with 316 * id, value and extensions. The accessor "getValue" gives direct 317 * access to the value 318 */ 319 public ClassComponent setValueElement(StringType value) { 320 this.value = value; 321 return this; 322 } 323 324 /** 325 * @return The alphanumeric string value associated with the insurer issued 326 * label. 327 */ 328 public String getValue() { 329 return this.value == null ? null : this.value.getValue(); 330 } 331 332 /** 333 * @param value The alphanumeric string value associated with the insurer issued 334 * label. 335 */ 336 public ClassComponent setValue(String value) { 337 if (this.value == null) 338 this.value = new StringType(); 339 this.value.setValue(value); 340 return this; 341 } 342 343 /** 344 * @return {@link #name} (A short description for the class.). This is the 345 * underlying object with id, value and extensions. The accessor 346 * "getName" gives direct access to the value 347 */ 348 public StringType getNameElement() { 349 if (this.name == null) 350 if (Configuration.errorOnAutoCreate()) 351 throw new Error("Attempt to auto-create ClassComponent.name"); 352 else if (Configuration.doAutoCreate()) 353 this.name = new StringType(); // bb 354 return this.name; 355 } 356 357 public boolean hasNameElement() { 358 return this.name != null && !this.name.isEmpty(); 359 } 360 361 public boolean hasName() { 362 return this.name != null && !this.name.isEmpty(); 363 } 364 365 /** 366 * @param value {@link #name} (A short description for the class.). This is the 367 * underlying object with id, value and extensions. The accessor 368 * "getName" gives direct access to the value 369 */ 370 public ClassComponent setNameElement(StringType value) { 371 this.name = value; 372 return this; 373 } 374 375 /** 376 * @return A short description for the class. 377 */ 378 public String getName() { 379 return this.name == null ? null : this.name.getValue(); 380 } 381 382 /** 383 * @param value A short description for the class. 384 */ 385 public ClassComponent setName(String value) { 386 if (Utilities.noString(value)) 387 this.name = null; 388 else { 389 if (this.name == null) 390 this.name = new StringType(); 391 this.name.setValue(value); 392 } 393 return this; 394 } 395 396 protected void listChildren(List<Property> children) { 397 super.listChildren(children); 398 children.add(new Property("type", "CodeableConcept", 399 "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 400 0, 1, type)); 401 children.add(new Property("value", "string", 402 "The alphanumeric string value associated with the insurer issued label.", 0, 1, value)); 403 children.add(new Property("name", "string", "A short description for the class.", 0, 1, name)); 404 } 405 406 @Override 407 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 408 switch (_hash) { 409 case 3575610: 410 /* type */ return new Property("type", "CodeableConcept", 411 "The type of classification for which an insurer-specific class label or number and optional name is provided, for example may be used to identify a class of coverage or employer group, Policy, Plan.", 412 0, 1, type); 413 case 111972721: 414 /* value */ return new Property("value", "string", 415 "The alphanumeric string value associated with the insurer issued label.", 0, 1, value); 416 case 3373707: 417 /* name */ return new Property("name", "string", "A short description for the class.", 0, 1, name); 418 default: 419 return super.getNamedProperty(_hash, _name, _checkValid); 420 } 421 422 } 423 424 @Override 425 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 426 switch (hash) { 427 case 3575610: 428 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 429 case 111972721: 430 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 431 case 3373707: 432 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 433 default: 434 return super.getProperty(hash, name, checkValid); 435 } 436 437 } 438 439 @Override 440 public Base setProperty(int hash, String name, Base value) throws FHIRException { 441 switch (hash) { 442 case 3575610: // type 443 this.type = castToCodeableConcept(value); // CodeableConcept 444 return value; 445 case 111972721: // value 446 this.value = castToString(value); // StringType 447 return value; 448 case 3373707: // name 449 this.name = castToString(value); // StringType 450 return value; 451 default: 452 return super.setProperty(hash, name, value); 453 } 454 455 } 456 457 @Override 458 public Base setProperty(String name, Base value) throws FHIRException { 459 if (name.equals("type")) { 460 this.type = castToCodeableConcept(value); // CodeableConcept 461 } else if (name.equals("value")) { 462 this.value = castToString(value); // StringType 463 } else if (name.equals("name")) { 464 this.name = castToString(value); // StringType 465 } else 466 return super.setProperty(name, value); 467 return value; 468 } 469 470 @Override 471 public void removeChild(String name, Base value) throws FHIRException { 472 if (name.equals("type")) { 473 this.type = null; 474 } else if (name.equals("value")) { 475 this.value = null; 476 } else if (name.equals("name")) { 477 this.name = null; 478 } else 479 super.removeChild(name, value); 480 481 } 482 483 @Override 484 public Base makeProperty(int hash, String name) throws FHIRException { 485 switch (hash) { 486 case 3575610: 487 return getType(); 488 case 111972721: 489 return getValueElement(); 490 case 3373707: 491 return getNameElement(); 492 default: 493 return super.makeProperty(hash, name); 494 } 495 496 } 497 498 @Override 499 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 500 switch (hash) { 501 case 3575610: 502 /* type */ return new String[] { "CodeableConcept" }; 503 case 111972721: 504 /* value */ return new String[] { "string" }; 505 case 3373707: 506 /* name */ return new String[] { "string" }; 507 default: 508 return super.getTypesForProperty(hash, name); 509 } 510 511 } 512 513 @Override 514 public Base addChild(String name) throws FHIRException { 515 if (name.equals("type")) { 516 this.type = new CodeableConcept(); 517 return this.type; 518 } else if (name.equals("value")) { 519 throw new FHIRException("Cannot call addChild on a singleton property Coverage.value"); 520 } else if (name.equals("name")) { 521 throw new FHIRException("Cannot call addChild on a singleton property Coverage.name"); 522 } else 523 return super.addChild(name); 524 } 525 526 public ClassComponent copy() { 527 ClassComponent dst = new ClassComponent(); 528 copyValues(dst); 529 return dst; 530 } 531 532 public void copyValues(ClassComponent dst) { 533 super.copyValues(dst); 534 dst.type = type == null ? null : type.copy(); 535 dst.value = value == null ? null : value.copy(); 536 dst.name = name == null ? null : name.copy(); 537 } 538 539 @Override 540 public boolean equalsDeep(Base other_) { 541 if (!super.equalsDeep(other_)) 542 return false; 543 if (!(other_ instanceof ClassComponent)) 544 return false; 545 ClassComponent o = (ClassComponent) other_; 546 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) && compareDeep(name, o.name, true); 547 } 548 549 @Override 550 public boolean equalsShallow(Base other_) { 551 if (!super.equalsShallow(other_)) 552 return false; 553 if (!(other_ instanceof ClassComponent)) 554 return false; 555 ClassComponent o = (ClassComponent) other_; 556 return compareValues(value, o.value, true) && compareValues(name, o.name, true); 557 } 558 559 public boolean isEmpty() { 560 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, name); 561 } 562 563 public String fhirType() { 564 return "Coverage.class"; 565 566 } 567 568 } 569 570 @Block() 571 public static class CostToBeneficiaryComponent extends BackboneElement implements IBaseBackboneElement { 572 /** 573 * The category of patient centric costs associated with treatment. 574 */ 575 @Child(name = "type", type = { 576 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 577 @Description(shortDefinition = "Cost category", formalDefinition = "The category of patient centric costs associated with treatment.") 578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-copay-type") 579 protected CodeableConcept type; 580 581 /** 582 * The amount due from the patient for the cost category. 583 */ 584 @Child(name = "value", type = { Quantity.class, 585 Money.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 586 @Description(shortDefinition = "The amount or percentage due from the beneficiary", formalDefinition = "The amount due from the patient for the cost category.") 587 protected Type value; 588 589 /** 590 * A suite of codes indicating exceptions or reductions to patient costs and 591 * their effective periods. 592 */ 593 @Child(name = "exception", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 594 @Description(shortDefinition = "Exceptions for patient payments", formalDefinition = "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.") 595 protected List<ExemptionComponent> exception; 596 597 private static final long serialVersionUID = -1302829059L; 598 599 /** 600 * Constructor 601 */ 602 public CostToBeneficiaryComponent() { 603 super(); 604 } 605 606 /** 607 * Constructor 608 */ 609 public CostToBeneficiaryComponent(Type value) { 610 super(); 611 this.value = value; 612 } 613 614 /** 615 * @return {@link #type} (The category of patient centric costs associated with 616 * treatment.) 617 */ 618 public CodeableConcept getType() { 619 if (this.type == null) 620 if (Configuration.errorOnAutoCreate()) 621 throw new Error("Attempt to auto-create CostToBeneficiaryComponent.type"); 622 else if (Configuration.doAutoCreate()) 623 this.type = new CodeableConcept(); // cc 624 return this.type; 625 } 626 627 public boolean hasType() { 628 return this.type != null && !this.type.isEmpty(); 629 } 630 631 /** 632 * @param value {@link #type} (The category of patient centric costs associated 633 * with treatment.) 634 */ 635 public CostToBeneficiaryComponent setType(CodeableConcept value) { 636 this.type = value; 637 return this; 638 } 639 640 /** 641 * @return {@link #value} (The amount due from the patient for the cost 642 * category.) 643 */ 644 public Type getValue() { 645 return this.value; 646 } 647 648 /** 649 * @return {@link #value} (The amount due from the patient for the cost 650 * category.) 651 */ 652 public Quantity getValueQuantity() throws FHIRException { 653 if (this.value == null) 654 this.value = new Quantity(); 655 if (!(this.value instanceof Quantity)) 656 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 657 + " was encountered"); 658 return (Quantity) this.value; 659 } 660 661 public boolean hasValueQuantity() { 662 return this != null && this.value instanceof Quantity; 663 } 664 665 /** 666 * @return {@link #value} (The amount due from the patient for the cost 667 * category.) 668 */ 669 public Money getValueMoney() throws FHIRException { 670 if (this.value == null) 671 this.value = new Money(); 672 if (!(this.value instanceof Money)) 673 throw new FHIRException( 674 "Type mismatch: the type Money was expected, but " + this.value.getClass().getName() + " was encountered"); 675 return (Money) this.value; 676 } 677 678 public boolean hasValueMoney() { 679 return this != null && this.value instanceof Money; 680 } 681 682 public boolean hasValue() { 683 return this.value != null && !this.value.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #value} (The amount due from the patient for the cost 688 * category.) 689 */ 690 public CostToBeneficiaryComponent setValue(Type value) { 691 if (value != null && !(value instanceof Quantity || value instanceof Money)) 692 throw new Error("Not the right type for Coverage.costToBeneficiary.value[x]: " + value.fhirType()); 693 this.value = value; 694 return this; 695 } 696 697 /** 698 * @return {@link #exception} (A suite of codes indicating exceptions or 699 * reductions to patient costs and their effective periods.) 700 */ 701 public List<ExemptionComponent> getException() { 702 if (this.exception == null) 703 this.exception = new ArrayList<ExemptionComponent>(); 704 return this.exception; 705 } 706 707 /** 708 * @return Returns a reference to <code>this</code> for easy method chaining 709 */ 710 public CostToBeneficiaryComponent setException(List<ExemptionComponent> theException) { 711 this.exception = theException; 712 return this; 713 } 714 715 public boolean hasException() { 716 if (this.exception == null) 717 return false; 718 for (ExemptionComponent item : this.exception) 719 if (!item.isEmpty()) 720 return true; 721 return false; 722 } 723 724 public ExemptionComponent addException() { // 3 725 ExemptionComponent t = new ExemptionComponent(); 726 if (this.exception == null) 727 this.exception = new ArrayList<ExemptionComponent>(); 728 this.exception.add(t); 729 return t; 730 } 731 732 public CostToBeneficiaryComponent addException(ExemptionComponent t) { // 3 733 if (t == null) 734 return this; 735 if (this.exception == null) 736 this.exception = new ArrayList<ExemptionComponent>(); 737 this.exception.add(t); 738 return this; 739 } 740 741 /** 742 * @return The first repetition of repeating field {@link #exception}, creating 743 * it if it does not already exist 744 */ 745 public ExemptionComponent getExceptionFirstRep() { 746 if (getException().isEmpty()) { 747 addException(); 748 } 749 return getException().get(0); 750 } 751 752 protected void listChildren(List<Property> children) { 753 super.listChildren(children); 754 children.add(new Property("type", "CodeableConcept", 755 "The category of patient centric costs associated with treatment.", 0, 1, type)); 756 children.add(new Property("value[x]", "SimpleQuantity|Money", 757 "The amount due from the patient for the cost category.", 0, 1, value)); 758 children.add(new Property("exception", "", 759 "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, 760 java.lang.Integer.MAX_VALUE, exception)); 761 } 762 763 @Override 764 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 765 switch (_hash) { 766 case 3575610: 767 /* type */ return new Property("type", "CodeableConcept", 768 "The category of patient centric costs associated with treatment.", 0, 1, type); 769 case -1410166417: 770 /* value[x] */ return new Property("value[x]", "SimpleQuantity|Money", 771 "The amount due from the patient for the cost category.", 0, 1, value); 772 case 111972721: 773 /* value */ return new Property("value[x]", "SimpleQuantity|Money", 774 "The amount due from the patient for the cost category.", 0, 1, value); 775 case -2029823716: 776 /* valueQuantity */ return new Property("value[x]", "SimpleQuantity|Money", 777 "The amount due from the patient for the cost category.", 0, 1, value); 778 case 2026560975: 779 /* valueMoney */ return new Property("value[x]", "SimpleQuantity|Money", 780 "The amount due from the patient for the cost category.", 0, 1, value); 781 case 1481625679: 782 /* exception */ return new Property("exception", "", 783 "A suite of codes indicating exceptions or reductions to patient costs and their effective periods.", 0, 784 java.lang.Integer.MAX_VALUE, exception); 785 default: 786 return super.getNamedProperty(_hash, _name, _checkValid); 787 } 788 789 } 790 791 @Override 792 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 793 switch (hash) { 794 case 3575610: 795 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 796 case 111972721: 797 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 798 case 1481625679: 799 /* exception */ return this.exception == null ? new Base[0] 800 : this.exception.toArray(new Base[this.exception.size()]); // ExemptionComponent 801 default: 802 return super.getProperty(hash, name, checkValid); 803 } 804 805 } 806 807 @Override 808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 809 switch (hash) { 810 case 3575610: // type 811 this.type = castToCodeableConcept(value); // CodeableConcept 812 return value; 813 case 111972721: // value 814 this.value = castToType(value); // Type 815 return value; 816 case 1481625679: // exception 817 this.getException().add((ExemptionComponent) value); // ExemptionComponent 818 return value; 819 default: 820 return super.setProperty(hash, name, value); 821 } 822 823 } 824 825 @Override 826 public Base setProperty(String name, Base value) throws FHIRException { 827 if (name.equals("type")) { 828 this.type = castToCodeableConcept(value); // CodeableConcept 829 } else if (name.equals("value[x]")) { 830 this.value = castToType(value); // Type 831 } else if (name.equals("exception")) { 832 this.getException().add((ExemptionComponent) value); 833 } else 834 return super.setProperty(name, value); 835 return value; 836 } 837 838 @Override 839 public void removeChild(String name, Base value) throws FHIRException { 840 if (name.equals("type")) { 841 this.type = null; 842 } else if (name.equals("value[x]")) { 843 this.value = null; 844 } else if (name.equals("exception")) { 845 this.getException().remove((ExemptionComponent) value); 846 } else 847 super.removeChild(name, value); 848 849 } 850 851 @Override 852 public Base makeProperty(int hash, String name) throws FHIRException { 853 switch (hash) { 854 case 3575610: 855 return getType(); 856 case -1410166417: 857 return getValue(); 858 case 111972721: 859 return getValue(); 860 case 1481625679: 861 return addException(); 862 default: 863 return super.makeProperty(hash, name); 864 } 865 866 } 867 868 @Override 869 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 870 switch (hash) { 871 case 3575610: 872 /* type */ return new String[] { "CodeableConcept" }; 873 case 111972721: 874 /* value */ return new String[] { "SimpleQuantity", "Money" }; 875 case 1481625679: 876 /* exception */ return new String[] {}; 877 default: 878 return super.getTypesForProperty(hash, name); 879 } 880 881 } 882 883 @Override 884 public Base addChild(String name) throws FHIRException { 885 if (name.equals("type")) { 886 this.type = new CodeableConcept(); 887 return this.type; 888 } else if (name.equals("valueQuantity")) { 889 this.value = new Quantity(); 890 return this.value; 891 } else if (name.equals("valueMoney")) { 892 this.value = new Money(); 893 return this.value; 894 } else if (name.equals("exception")) { 895 return addException(); 896 } else 897 return super.addChild(name); 898 } 899 900 public CostToBeneficiaryComponent copy() { 901 CostToBeneficiaryComponent dst = new CostToBeneficiaryComponent(); 902 copyValues(dst); 903 return dst; 904 } 905 906 public void copyValues(CostToBeneficiaryComponent dst) { 907 super.copyValues(dst); 908 dst.type = type == null ? null : type.copy(); 909 dst.value = value == null ? null : value.copy(); 910 if (exception != null) { 911 dst.exception = new ArrayList<ExemptionComponent>(); 912 for (ExemptionComponent i : exception) 913 dst.exception.add(i.copy()); 914 } 915 ; 916 } 917 918 @Override 919 public boolean equalsDeep(Base other_) { 920 if (!super.equalsDeep(other_)) 921 return false; 922 if (!(other_ instanceof CostToBeneficiaryComponent)) 923 return false; 924 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 925 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 926 && compareDeep(exception, o.exception, true); 927 } 928 929 @Override 930 public boolean equalsShallow(Base other_) { 931 if (!super.equalsShallow(other_)) 932 return false; 933 if (!(other_ instanceof CostToBeneficiaryComponent)) 934 return false; 935 CostToBeneficiaryComponent o = (CostToBeneficiaryComponent) other_; 936 return true; 937 } 938 939 public boolean isEmpty() { 940 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, exception); 941 } 942 943 public String fhirType() { 944 return "Coverage.costToBeneficiary"; 945 946 } 947 948 } 949 950 @Block() 951 public static class ExemptionComponent extends BackboneElement implements IBaseBackboneElement { 952 /** 953 * The code for the specific exception. 954 */ 955 @Child(name = "type", type = { 956 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 957 @Description(shortDefinition = "Exception category", formalDefinition = "The code for the specific exception.") 958 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-financial-exception") 959 protected CodeableConcept type; 960 961 /** 962 * The timeframe during when the exception is in force. 963 */ 964 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 965 @Description(shortDefinition = "The effective period of the exception", formalDefinition = "The timeframe during when the exception is in force.") 966 protected Period period; 967 968 private static final long serialVersionUID = 523191991L; 969 970 /** 971 * Constructor 972 */ 973 public ExemptionComponent() { 974 super(); 975 } 976 977 /** 978 * Constructor 979 */ 980 public ExemptionComponent(CodeableConcept type) { 981 super(); 982 this.type = type; 983 } 984 985 /** 986 * @return {@link #type} (The code for the specific exception.) 987 */ 988 public CodeableConcept getType() { 989 if (this.type == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create ExemptionComponent.type"); 992 else if (Configuration.doAutoCreate()) 993 this.type = new CodeableConcept(); // cc 994 return this.type; 995 } 996 997 public boolean hasType() { 998 return this.type != null && !this.type.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #type} (The code for the specific exception.) 1003 */ 1004 public ExemptionComponent setType(CodeableConcept value) { 1005 this.type = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #period} (The timeframe during when the exception is in 1011 * force.) 1012 */ 1013 public Period getPeriod() { 1014 if (this.period == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create ExemptionComponent.period"); 1017 else if (Configuration.doAutoCreate()) 1018 this.period = new Period(); // cc 1019 return this.period; 1020 } 1021 1022 public boolean hasPeriod() { 1023 return this.period != null && !this.period.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #period} (The timeframe during when the exception is in 1028 * force.) 1029 */ 1030 public ExemptionComponent setPeriod(Period value) { 1031 this.period = value; 1032 return this; 1033 } 1034 1035 protected void listChildren(List<Property> children) { 1036 super.listChildren(children); 1037 children.add(new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type)); 1038 children 1039 .add(new Property("period", "Period", "The timeframe during when the exception is in force.", 0, 1, period)); 1040 } 1041 1042 @Override 1043 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1044 switch (_hash) { 1045 case 3575610: 1046 /* type */ return new Property("type", "CodeableConcept", "The code for the specific exception.", 0, 1, type); 1047 case -991726143: 1048 /* period */ return new Property("period", "Period", "The timeframe during when the exception is in force.", 0, 1049 1, period); 1050 default: 1051 return super.getNamedProperty(_hash, _name, _checkValid); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1058 switch (hash) { 1059 case 3575610: 1060 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1061 case -991726143: 1062 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1063 default: 1064 return super.getProperty(hash, name, checkValid); 1065 } 1066 1067 } 1068 1069 @Override 1070 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1071 switch (hash) { 1072 case 3575610: // type 1073 this.type = castToCodeableConcept(value); // CodeableConcept 1074 return value; 1075 case -991726143: // period 1076 this.period = castToPeriod(value); // Period 1077 return value; 1078 default: 1079 return super.setProperty(hash, name, value); 1080 } 1081 1082 } 1083 1084 @Override 1085 public Base setProperty(String name, Base value) throws FHIRException { 1086 if (name.equals("type")) { 1087 this.type = castToCodeableConcept(value); // CodeableConcept 1088 } else if (name.equals("period")) { 1089 this.period = castToPeriod(value); // Period 1090 } else 1091 return super.setProperty(name, value); 1092 return value; 1093 } 1094 1095 @Override 1096 public void removeChild(String name, Base value) throws FHIRException { 1097 if (name.equals("type")) { 1098 this.type = null; 1099 } else if (name.equals("period")) { 1100 this.period = null; 1101 } else 1102 super.removeChild(name, value); 1103 1104 } 1105 1106 @Override 1107 public Base makeProperty(int hash, String name) throws FHIRException { 1108 switch (hash) { 1109 case 3575610: 1110 return getType(); 1111 case -991726143: 1112 return getPeriod(); 1113 default: 1114 return super.makeProperty(hash, name); 1115 } 1116 1117 } 1118 1119 @Override 1120 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1121 switch (hash) { 1122 case 3575610: 1123 /* type */ return new String[] { "CodeableConcept" }; 1124 case -991726143: 1125 /* period */ return new String[] { "Period" }; 1126 default: 1127 return super.getTypesForProperty(hash, name); 1128 } 1129 1130 } 1131 1132 @Override 1133 public Base addChild(String name) throws FHIRException { 1134 if (name.equals("type")) { 1135 this.type = new CodeableConcept(); 1136 return this.type; 1137 } else if (name.equals("period")) { 1138 this.period = new Period(); 1139 return this.period; 1140 } else 1141 return super.addChild(name); 1142 } 1143 1144 public ExemptionComponent copy() { 1145 ExemptionComponent dst = new ExemptionComponent(); 1146 copyValues(dst); 1147 return dst; 1148 } 1149 1150 public void copyValues(ExemptionComponent dst) { 1151 super.copyValues(dst); 1152 dst.type = type == null ? null : type.copy(); 1153 dst.period = period == null ? null : period.copy(); 1154 } 1155 1156 @Override 1157 public boolean equalsDeep(Base other_) { 1158 if (!super.equalsDeep(other_)) 1159 return false; 1160 if (!(other_ instanceof ExemptionComponent)) 1161 return false; 1162 ExemptionComponent o = (ExemptionComponent) other_; 1163 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true); 1164 } 1165 1166 @Override 1167 public boolean equalsShallow(Base other_) { 1168 if (!super.equalsShallow(other_)) 1169 return false; 1170 if (!(other_ instanceof ExemptionComponent)) 1171 return false; 1172 ExemptionComponent o = (ExemptionComponent) other_; 1173 return true; 1174 } 1175 1176 public boolean isEmpty() { 1177 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period); 1178 } 1179 1180 public String fhirType() { 1181 return "Coverage.costToBeneficiary.exception"; 1182 1183 } 1184 1185 } 1186 1187 /** 1188 * A unique identifier assigned to this coverage. 1189 */ 1190 @Child(name = "identifier", type = { 1191 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1192 @Description(shortDefinition = "Business Identifier for the coverage", formalDefinition = "A unique identifier assigned to this coverage.") 1193 protected List<Identifier> identifier; 1194 1195 /** 1196 * The status of the resource instance. 1197 */ 1198 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1199 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 1200 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 1201 protected Enumeration<CoverageStatus> status; 1202 1203 /** 1204 * The type of coverage: social program, medical plan, accident coverage 1205 * (workers compensation, auto), group health or payment by an individual or 1206 * organization. 1207 */ 1208 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1209 @Description(shortDefinition = "Coverage category such as medical or accident", formalDefinition = "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.") 1210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverage-type") 1211 protected CodeableConcept type; 1212 1213 /** 1214 * The party who 'owns' the insurance policy. 1215 */ 1216 @Child(name = "policyHolder", type = { Patient.class, RelatedPerson.class, 1217 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1218 @Description(shortDefinition = "Owner of the policy", formalDefinition = "The party who 'owns' the insurance policy.") 1219 protected Reference policyHolder; 1220 1221 /** 1222 * The actual object that is the target of the reference (The party who 'owns' 1223 * the insurance policy.) 1224 */ 1225 protected Resource policyHolderTarget; 1226 1227 /** 1228 * The party who has signed-up for or 'owns' the contractual relationship to the 1229 * policy or to whom the benefit of the policy for services rendered to them or 1230 * their family is due. 1231 */ 1232 @Child(name = "subscriber", type = { Patient.class, 1233 RelatedPerson.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1234 @Description(shortDefinition = "Subscriber to the policy", formalDefinition = "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.") 1235 protected Reference subscriber; 1236 1237 /** 1238 * The actual object that is the target of the reference (The party who has 1239 * signed-up for or 'owns' the contractual relationship to the policy or to whom 1240 * the benefit of the policy for services rendered to them or their family is 1241 * due.) 1242 */ 1243 protected Resource subscriberTarget; 1244 1245 /** 1246 * The insurer assigned ID for the Subscriber. 1247 */ 1248 @Child(name = "subscriberId", type = { 1249 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1250 @Description(shortDefinition = "ID assigned to the subscriber", formalDefinition = "The insurer assigned ID for the Subscriber.") 1251 protected StringType subscriberId; 1252 1253 /** 1254 * The party who benefits from the insurance coverage; the patient when products 1255 * and/or services are provided. 1256 */ 1257 @Child(name = "beneficiary", type = { Patient.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1258 @Description(shortDefinition = "Plan beneficiary", formalDefinition = "The party who benefits from the insurance coverage; the patient when products and/or services are provided.") 1259 protected Reference beneficiary; 1260 1261 /** 1262 * The actual object that is the target of the reference (The party who benefits 1263 * from the insurance coverage; the patient when products and/or services are 1264 * provided.) 1265 */ 1266 protected Patient beneficiaryTarget; 1267 1268 /** 1269 * A unique identifier for a dependent under the coverage. 1270 */ 1271 @Child(name = "dependent", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1272 @Description(shortDefinition = "Dependent number", formalDefinition = "A unique identifier for a dependent under the coverage.") 1273 protected StringType dependent; 1274 1275 /** 1276 * The relationship of beneficiary (patient) to the subscriber. 1277 */ 1278 @Child(name = "relationship", type = { 1279 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1280 @Description(shortDefinition = "Beneficiary relationship to the subscriber", formalDefinition = "The relationship of beneficiary (patient) to the subscriber.") 1281 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subscriber-relationship") 1282 protected CodeableConcept relationship; 1283 1284 /** 1285 * Time period during which the coverage is in force. A missing start date 1286 * indicates the start date isn't known, a missing end date means the coverage 1287 * is continuing to be in force. 1288 */ 1289 @Child(name = "period", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1290 @Description(shortDefinition = "Coverage start and end dates", formalDefinition = "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.") 1291 protected Period period; 1292 1293 /** 1294 * The program or plan underwriter or payor including both insurance and 1295 * non-insurance agreements, such as patient-pay agreements. 1296 */ 1297 @Child(name = "payor", type = { Organization.class, Patient.class, 1298 RelatedPerson.class }, order = 10, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1299 @Description(shortDefinition = "Issuer of the policy", formalDefinition = "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.") 1300 protected List<Reference> payor; 1301 /** 1302 * The actual objects that are the target of the reference (The program or plan 1303 * underwriter or payor including both insurance and non-insurance agreements, 1304 * such as patient-pay agreements.) 1305 */ 1306 protected List<Resource> payorTarget; 1307 1308 /** 1309 * A suite of underwriter specific classifiers. 1310 */ 1311 @Child(name = "class", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1312 @Description(shortDefinition = "Additional coverage classifications", formalDefinition = "A suite of underwriter specific classifiers.") 1313 protected List<ClassComponent> class_; 1314 1315 /** 1316 * The order of applicability of this coverage relative to other coverages which 1317 * are currently in force. Note, there may be gaps in the numbering and this 1318 * does not imply primary, secondary etc. as the specific positioning of 1319 * coverages depends upon the episode of care. 1320 */ 1321 @Child(name = "order", type = { 1322 PositiveIntType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1323 @Description(shortDefinition = "Relative order of the coverage", formalDefinition = "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.") 1324 protected PositiveIntType order; 1325 1326 /** 1327 * The insurer-specific identifier for the insurer-defined network of providers 1328 * to which the beneficiary may seek treatment which will be covered at the 1329 * 'in-network' rate, otherwise 'out of network' terms and conditions apply. 1330 */ 1331 @Child(name = "network", type = { StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1332 @Description(shortDefinition = "Insurer network", formalDefinition = "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.") 1333 protected StringType network; 1334 1335 /** 1336 * A suite of codes indicating the cost category and associated amount which 1337 * have been detailed in the policy and may have been included on the health 1338 * card. 1339 */ 1340 @Child(name = "costToBeneficiary", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1341 @Description(shortDefinition = "Patient payments for services/products", formalDefinition = "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.") 1342 protected List<CostToBeneficiaryComponent> costToBeneficiary; 1343 1344 /** 1345 * When 'subrogation=true' this insurance instance has been included not for 1346 * adjudication but to provide insurers with the details to recover costs. 1347 */ 1348 @Child(name = "subrogation", type = { 1349 BooleanType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1350 @Description(shortDefinition = "Reimbursement to insurer", formalDefinition = "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.") 1351 protected BooleanType subrogation; 1352 1353 /** 1354 * The policy(s) which constitute this insurance coverage. 1355 */ 1356 @Child(name = "contract", type = { 1357 Contract.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1358 @Description(shortDefinition = "Contract details", formalDefinition = "The policy(s) which constitute this insurance coverage.") 1359 protected List<Reference> contract; 1360 /** 1361 * The actual objects that are the target of the reference (The policy(s) which 1362 * constitute this insurance coverage.) 1363 */ 1364 protected List<Contract> contractTarget; 1365 1366 private static final long serialVersionUID = 212315315L; 1367 1368 /** 1369 * Constructor 1370 */ 1371 public Coverage() { 1372 super(); 1373 } 1374 1375 /** 1376 * Constructor 1377 */ 1378 public Coverage(Enumeration<CoverageStatus> status, Reference beneficiary) { 1379 super(); 1380 this.status = status; 1381 this.beneficiary = beneficiary; 1382 } 1383 1384 /** 1385 * @return {@link #identifier} (A unique identifier assigned to this coverage.) 1386 */ 1387 public List<Identifier> getIdentifier() { 1388 if (this.identifier == null) 1389 this.identifier = new ArrayList<Identifier>(); 1390 return this.identifier; 1391 } 1392 1393 /** 1394 * @return Returns a reference to <code>this</code> for easy method chaining 1395 */ 1396 public Coverage setIdentifier(List<Identifier> theIdentifier) { 1397 this.identifier = theIdentifier; 1398 return this; 1399 } 1400 1401 public boolean hasIdentifier() { 1402 if (this.identifier == null) 1403 return false; 1404 for (Identifier item : this.identifier) 1405 if (!item.isEmpty()) 1406 return true; 1407 return false; 1408 } 1409 1410 public Identifier addIdentifier() { // 3 1411 Identifier t = new Identifier(); 1412 if (this.identifier == null) 1413 this.identifier = new ArrayList<Identifier>(); 1414 this.identifier.add(t); 1415 return t; 1416 } 1417 1418 public Coverage addIdentifier(Identifier t) { // 3 1419 if (t == null) 1420 return this; 1421 if (this.identifier == null) 1422 this.identifier = new ArrayList<Identifier>(); 1423 this.identifier.add(t); 1424 return this; 1425 } 1426 1427 /** 1428 * @return The first repetition of repeating field {@link #identifier}, creating 1429 * it if it does not already exist 1430 */ 1431 public Identifier getIdentifierFirstRep() { 1432 if (getIdentifier().isEmpty()) { 1433 addIdentifier(); 1434 } 1435 return getIdentifier().get(0); 1436 } 1437 1438 /** 1439 * @return {@link #status} (The status of the resource instance.). This is the 1440 * underlying object with id, value and extensions. The accessor 1441 * "getStatus" gives direct access to the value 1442 */ 1443 public Enumeration<CoverageStatus> getStatusElement() { 1444 if (this.status == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create Coverage.status"); 1447 else if (Configuration.doAutoCreate()) 1448 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); // bb 1449 return this.status; 1450 } 1451 1452 public boolean hasStatusElement() { 1453 return this.status != null && !this.status.isEmpty(); 1454 } 1455 1456 public boolean hasStatus() { 1457 return this.status != null && !this.status.isEmpty(); 1458 } 1459 1460 /** 1461 * @param value {@link #status} (The status of the resource instance.). This is 1462 * the underlying object with id, value and extensions. The 1463 * accessor "getStatus" gives direct access to the value 1464 */ 1465 public Coverage setStatusElement(Enumeration<CoverageStatus> value) { 1466 this.status = value; 1467 return this; 1468 } 1469 1470 /** 1471 * @return The status of the resource instance. 1472 */ 1473 public CoverageStatus getStatus() { 1474 return this.status == null ? null : this.status.getValue(); 1475 } 1476 1477 /** 1478 * @param value The status of the resource instance. 1479 */ 1480 public Coverage setStatus(CoverageStatus value) { 1481 if (this.status == null) 1482 this.status = new Enumeration<CoverageStatus>(new CoverageStatusEnumFactory()); 1483 this.status.setValue(value); 1484 return this; 1485 } 1486 1487 /** 1488 * @return {@link #type} (The type of coverage: social program, medical plan, 1489 * accident coverage (workers compensation, auto), group health or 1490 * payment by an individual or organization.) 1491 */ 1492 public CodeableConcept getType() { 1493 if (this.type == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create Coverage.type"); 1496 else if (Configuration.doAutoCreate()) 1497 this.type = new CodeableConcept(); // cc 1498 return this.type; 1499 } 1500 1501 public boolean hasType() { 1502 return this.type != null && !this.type.isEmpty(); 1503 } 1504 1505 /** 1506 * @param value {@link #type} (The type of coverage: social program, medical 1507 * plan, accident coverage (workers compensation, auto), group 1508 * health or payment by an individual or organization.) 1509 */ 1510 public Coverage setType(CodeableConcept value) { 1511 this.type = value; 1512 return this; 1513 } 1514 1515 /** 1516 * @return {@link #policyHolder} (The party who 'owns' the insurance policy.) 1517 */ 1518 public Reference getPolicyHolder() { 1519 if (this.policyHolder == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create Coverage.policyHolder"); 1522 else if (Configuration.doAutoCreate()) 1523 this.policyHolder = new Reference(); // cc 1524 return this.policyHolder; 1525 } 1526 1527 public boolean hasPolicyHolder() { 1528 return this.policyHolder != null && !this.policyHolder.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #policyHolder} (The party who 'owns' the insurance 1533 * policy.) 1534 */ 1535 public Coverage setPolicyHolder(Reference value) { 1536 this.policyHolder = value; 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #policyHolder} The actual object that is the target of the 1542 * reference. The reference library doesn't populate this, but you can 1543 * use it to hold the resource if you resolve it. (The party who 'owns' 1544 * the insurance policy.) 1545 */ 1546 public Resource getPolicyHolderTarget() { 1547 return this.policyHolderTarget; 1548 } 1549 1550 /** 1551 * @param value {@link #policyHolder} The actual object that is the target of 1552 * the reference. The reference library doesn't use these, but you 1553 * can use it to hold the resource if you resolve it. (The party 1554 * who 'owns' the insurance policy.) 1555 */ 1556 public Coverage setPolicyHolderTarget(Resource value) { 1557 this.policyHolderTarget = value; 1558 return this; 1559 } 1560 1561 /** 1562 * @return {@link #subscriber} (The party who has signed-up for or 'owns' the 1563 * contractual relationship to the policy or to whom the benefit of the 1564 * policy for services rendered to them or their family is due.) 1565 */ 1566 public Reference getSubscriber() { 1567 if (this.subscriber == null) 1568 if (Configuration.errorOnAutoCreate()) 1569 throw new Error("Attempt to auto-create Coverage.subscriber"); 1570 else if (Configuration.doAutoCreate()) 1571 this.subscriber = new Reference(); // cc 1572 return this.subscriber; 1573 } 1574 1575 public boolean hasSubscriber() { 1576 return this.subscriber != null && !this.subscriber.isEmpty(); 1577 } 1578 1579 /** 1580 * @param value {@link #subscriber} (The party who has signed-up for or 'owns' 1581 * the contractual relationship to the policy or to whom the 1582 * benefit of the policy for services rendered to them or their 1583 * family is due.) 1584 */ 1585 public Coverage setSubscriber(Reference value) { 1586 this.subscriber = value; 1587 return this; 1588 } 1589 1590 /** 1591 * @return {@link #subscriber} The actual object that is the target of the 1592 * reference. The reference library doesn't populate this, but you can 1593 * use it to hold the resource if you resolve it. (The party who has 1594 * signed-up for or 'owns' the contractual relationship to the policy or 1595 * to whom the benefit of the policy for services rendered to them or 1596 * their family is due.) 1597 */ 1598 public Resource getSubscriberTarget() { 1599 return this.subscriberTarget; 1600 } 1601 1602 /** 1603 * @param value {@link #subscriber} The actual object that is the target of the 1604 * reference. The reference library doesn't use these, but you can 1605 * use it to hold the resource if you resolve it. (The party who 1606 * has signed-up for or 'owns' the contractual relationship to the 1607 * policy or to whom the benefit of the policy for services 1608 * rendered to them or their family is due.) 1609 */ 1610 public Coverage setSubscriberTarget(Resource value) { 1611 this.subscriberTarget = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #subscriberId} (The insurer assigned ID for the Subscriber.). 1617 * This is the underlying object with id, value and extensions. The 1618 * accessor "getSubscriberId" gives direct access to the value 1619 */ 1620 public StringType getSubscriberIdElement() { 1621 if (this.subscriberId == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create Coverage.subscriberId"); 1624 else if (Configuration.doAutoCreate()) 1625 this.subscriberId = new StringType(); // bb 1626 return this.subscriberId; 1627 } 1628 1629 public boolean hasSubscriberIdElement() { 1630 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1631 } 1632 1633 public boolean hasSubscriberId() { 1634 return this.subscriberId != null && !this.subscriberId.isEmpty(); 1635 } 1636 1637 /** 1638 * @param value {@link #subscriberId} (The insurer assigned ID for the 1639 * Subscriber.). This is the underlying object with id, value and 1640 * extensions. The accessor "getSubscriberId" gives direct access 1641 * to the value 1642 */ 1643 public Coverage setSubscriberIdElement(StringType value) { 1644 this.subscriberId = value; 1645 return this; 1646 } 1647 1648 /** 1649 * @return The insurer assigned ID for the Subscriber. 1650 */ 1651 public String getSubscriberId() { 1652 return this.subscriberId == null ? null : this.subscriberId.getValue(); 1653 } 1654 1655 /** 1656 * @param value The insurer assigned ID for the Subscriber. 1657 */ 1658 public Coverage setSubscriberId(String value) { 1659 if (Utilities.noString(value)) 1660 this.subscriberId = null; 1661 else { 1662 if (this.subscriberId == null) 1663 this.subscriberId = new StringType(); 1664 this.subscriberId.setValue(value); 1665 } 1666 return this; 1667 } 1668 1669 /** 1670 * @return {@link #beneficiary} (The party who benefits from the insurance 1671 * coverage; the patient when products and/or services are provided.) 1672 */ 1673 public Reference getBeneficiary() { 1674 if (this.beneficiary == null) 1675 if (Configuration.errorOnAutoCreate()) 1676 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1677 else if (Configuration.doAutoCreate()) 1678 this.beneficiary = new Reference(); // cc 1679 return this.beneficiary; 1680 } 1681 1682 public boolean hasBeneficiary() { 1683 return this.beneficiary != null && !this.beneficiary.isEmpty(); 1684 } 1685 1686 /** 1687 * @param value {@link #beneficiary} (The party who benefits from the insurance 1688 * coverage; the patient when products and/or services are 1689 * provided.) 1690 */ 1691 public Coverage setBeneficiary(Reference value) { 1692 this.beneficiary = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #beneficiary} The actual object that is the target of the 1698 * reference. The reference library doesn't populate this, but you can 1699 * use it to hold the resource if you resolve it. (The party who 1700 * benefits from the insurance coverage; the patient when products 1701 * and/or services are provided.) 1702 */ 1703 public Patient getBeneficiaryTarget() { 1704 if (this.beneficiaryTarget == null) 1705 if (Configuration.errorOnAutoCreate()) 1706 throw new Error("Attempt to auto-create Coverage.beneficiary"); 1707 else if (Configuration.doAutoCreate()) 1708 this.beneficiaryTarget = new Patient(); // aa 1709 return this.beneficiaryTarget; 1710 } 1711 1712 /** 1713 * @param value {@link #beneficiary} The actual object that is the target of the 1714 * reference. The reference library doesn't use these, but you can 1715 * use it to hold the resource if you resolve it. (The party who 1716 * benefits from the insurance coverage; the patient when products 1717 * and/or services are provided.) 1718 */ 1719 public Coverage setBeneficiaryTarget(Patient value) { 1720 this.beneficiaryTarget = value; 1721 return this; 1722 } 1723 1724 /** 1725 * @return {@link #dependent} (A unique identifier for a dependent under the 1726 * coverage.). This is the underlying object with id, value and 1727 * extensions. The accessor "getDependent" gives direct access to the 1728 * value 1729 */ 1730 public StringType getDependentElement() { 1731 if (this.dependent == null) 1732 if (Configuration.errorOnAutoCreate()) 1733 throw new Error("Attempt to auto-create Coverage.dependent"); 1734 else if (Configuration.doAutoCreate()) 1735 this.dependent = new StringType(); // bb 1736 return this.dependent; 1737 } 1738 1739 public boolean hasDependentElement() { 1740 return this.dependent != null && !this.dependent.isEmpty(); 1741 } 1742 1743 public boolean hasDependent() { 1744 return this.dependent != null && !this.dependent.isEmpty(); 1745 } 1746 1747 /** 1748 * @param value {@link #dependent} (A unique identifier for a dependent under 1749 * the coverage.). This is the underlying object with id, value and 1750 * extensions. The accessor "getDependent" gives direct access to 1751 * the value 1752 */ 1753 public Coverage setDependentElement(StringType value) { 1754 this.dependent = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return A unique identifier for a dependent under the coverage. 1760 */ 1761 public String getDependent() { 1762 return this.dependent == null ? null : this.dependent.getValue(); 1763 } 1764 1765 /** 1766 * @param value A unique identifier for a dependent under the coverage. 1767 */ 1768 public Coverage setDependent(String value) { 1769 if (Utilities.noString(value)) 1770 this.dependent = null; 1771 else { 1772 if (this.dependent == null) 1773 this.dependent = new StringType(); 1774 this.dependent.setValue(value); 1775 } 1776 return this; 1777 } 1778 1779 /** 1780 * @return {@link #relationship} (The relationship of beneficiary (patient) to 1781 * the subscriber.) 1782 */ 1783 public CodeableConcept getRelationship() { 1784 if (this.relationship == null) 1785 if (Configuration.errorOnAutoCreate()) 1786 throw new Error("Attempt to auto-create Coverage.relationship"); 1787 else if (Configuration.doAutoCreate()) 1788 this.relationship = new CodeableConcept(); // cc 1789 return this.relationship; 1790 } 1791 1792 public boolean hasRelationship() { 1793 return this.relationship != null && !this.relationship.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #relationship} (The relationship of beneficiary (patient) 1798 * to the subscriber.) 1799 */ 1800 public Coverage setRelationship(CodeableConcept value) { 1801 this.relationship = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #period} (Time period during which the coverage is in force. A 1807 * missing start date indicates the start date isn't known, a missing 1808 * end date means the coverage is continuing to be in force.) 1809 */ 1810 public Period getPeriod() { 1811 if (this.period == null) 1812 if (Configuration.errorOnAutoCreate()) 1813 throw new Error("Attempt to auto-create Coverage.period"); 1814 else if (Configuration.doAutoCreate()) 1815 this.period = new Period(); // cc 1816 return this.period; 1817 } 1818 1819 public boolean hasPeriod() { 1820 return this.period != null && !this.period.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #period} (Time period during which the coverage is in 1825 * force. A missing start date indicates the start date isn't 1826 * known, a missing end date means the coverage is continuing to be 1827 * in force.) 1828 */ 1829 public Coverage setPeriod(Period value) { 1830 this.period = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return {@link #payor} (The program or plan underwriter or payor including 1836 * both insurance and non-insurance agreements, such as patient-pay 1837 * agreements.) 1838 */ 1839 public List<Reference> getPayor() { 1840 if (this.payor == null) 1841 this.payor = new ArrayList<Reference>(); 1842 return this.payor; 1843 } 1844 1845 /** 1846 * @return Returns a reference to <code>this</code> for easy method chaining 1847 */ 1848 public Coverage setPayor(List<Reference> thePayor) { 1849 this.payor = thePayor; 1850 return this; 1851 } 1852 1853 public boolean hasPayor() { 1854 if (this.payor == null) 1855 return false; 1856 for (Reference item : this.payor) 1857 if (!item.isEmpty()) 1858 return true; 1859 return false; 1860 } 1861 1862 public Reference addPayor() { // 3 1863 Reference t = new Reference(); 1864 if (this.payor == null) 1865 this.payor = new ArrayList<Reference>(); 1866 this.payor.add(t); 1867 return t; 1868 } 1869 1870 public Coverage addPayor(Reference t) { // 3 1871 if (t == null) 1872 return this; 1873 if (this.payor == null) 1874 this.payor = new ArrayList<Reference>(); 1875 this.payor.add(t); 1876 return this; 1877 } 1878 1879 /** 1880 * @return The first repetition of repeating field {@link #payor}, creating it 1881 * if it does not already exist 1882 */ 1883 public Reference getPayorFirstRep() { 1884 if (getPayor().isEmpty()) { 1885 addPayor(); 1886 } 1887 return getPayor().get(0); 1888 } 1889 1890 /** 1891 * @deprecated Use Reference#setResource(IBaseResource) instead 1892 */ 1893 @Deprecated 1894 public List<Resource> getPayorTarget() { 1895 if (this.payorTarget == null) 1896 this.payorTarget = new ArrayList<Resource>(); 1897 return this.payorTarget; 1898 } 1899 1900 /** 1901 * @return {@link #class_} (A suite of underwriter specific classifiers.) 1902 */ 1903 public List<ClassComponent> getClass_() { 1904 if (this.class_ == null) 1905 this.class_ = new ArrayList<ClassComponent>(); 1906 return this.class_; 1907 } 1908 1909 /** 1910 * @return Returns a reference to <code>this</code> for easy method chaining 1911 */ 1912 public Coverage setClass_(List<ClassComponent> theClass_) { 1913 this.class_ = theClass_; 1914 return this; 1915 } 1916 1917 public boolean hasClass_() { 1918 if (this.class_ == null) 1919 return false; 1920 for (ClassComponent item : this.class_) 1921 if (!item.isEmpty()) 1922 return true; 1923 return false; 1924 } 1925 1926 public ClassComponent addClass_() { // 3 1927 ClassComponent t = new ClassComponent(); 1928 if (this.class_ == null) 1929 this.class_ = new ArrayList<ClassComponent>(); 1930 this.class_.add(t); 1931 return t; 1932 } 1933 1934 public Coverage addClass_(ClassComponent t) { // 3 1935 if (t == null) 1936 return this; 1937 if (this.class_ == null) 1938 this.class_ = new ArrayList<ClassComponent>(); 1939 this.class_.add(t); 1940 return this; 1941 } 1942 1943 /** 1944 * @return The first repetition of repeating field {@link #class_}, creating it 1945 * if it does not already exist 1946 */ 1947 public ClassComponent getClass_FirstRep() { 1948 if (getClass_().isEmpty()) { 1949 addClass_(); 1950 } 1951 return getClass_().get(0); 1952 } 1953 1954 /** 1955 * @return {@link #order} (The order of applicability of this coverage relative 1956 * to other coverages which are currently in force. Note, there may be 1957 * gaps in the numbering and this does not imply primary, secondary etc. 1958 * as the specific positioning of coverages depends upon the episode of 1959 * care.). This is the underlying object with id, value and extensions. 1960 * The accessor "getOrder" gives direct access to the value 1961 */ 1962 public PositiveIntType getOrderElement() { 1963 if (this.order == null) 1964 if (Configuration.errorOnAutoCreate()) 1965 throw new Error("Attempt to auto-create Coverage.order"); 1966 else if (Configuration.doAutoCreate()) 1967 this.order = new PositiveIntType(); // bb 1968 return this.order; 1969 } 1970 1971 public boolean hasOrderElement() { 1972 return this.order != null && !this.order.isEmpty(); 1973 } 1974 1975 public boolean hasOrder() { 1976 return this.order != null && !this.order.isEmpty(); 1977 } 1978 1979 /** 1980 * @param value {@link #order} (The order of applicability of this coverage 1981 * relative to other coverages which are currently in force. Note, 1982 * there may be gaps in the numbering and this does not imply 1983 * primary, secondary etc. as the specific positioning of coverages 1984 * depends upon the episode of care.). This is the underlying 1985 * object with id, value and extensions. The accessor "getOrder" 1986 * gives direct access to the value 1987 */ 1988 public Coverage setOrderElement(PositiveIntType value) { 1989 this.order = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return The order of applicability of this coverage relative to other 1995 * coverages which are currently in force. Note, there may be gaps in 1996 * the numbering and this does not imply primary, secondary etc. as the 1997 * specific positioning of coverages depends upon the episode of care. 1998 */ 1999 public int getOrder() { 2000 return this.order == null || this.order.isEmpty() ? 0 : this.order.getValue(); 2001 } 2002 2003 /** 2004 * @param value The order of applicability of this coverage relative to other 2005 * coverages which are currently in force. Note, there may be gaps 2006 * in the numbering and this does not imply primary, secondary etc. 2007 * as the specific positioning of coverages depends upon the 2008 * episode of care. 2009 */ 2010 public Coverage setOrder(int value) { 2011 if (this.order == null) 2012 this.order = new PositiveIntType(); 2013 this.order.setValue(value); 2014 return this; 2015 } 2016 2017 /** 2018 * @return {@link #network} (The insurer-specific identifier for the 2019 * insurer-defined network of providers to which the beneficiary may 2020 * seek treatment which will be covered at the 'in-network' rate, 2021 * otherwise 'out of network' terms and conditions apply.). This is the 2022 * underlying object with id, value and extensions. The accessor 2023 * "getNetwork" gives direct access to the value 2024 */ 2025 public StringType getNetworkElement() { 2026 if (this.network == null) 2027 if (Configuration.errorOnAutoCreate()) 2028 throw new Error("Attempt to auto-create Coverage.network"); 2029 else if (Configuration.doAutoCreate()) 2030 this.network = new StringType(); // bb 2031 return this.network; 2032 } 2033 2034 public boolean hasNetworkElement() { 2035 return this.network != null && !this.network.isEmpty(); 2036 } 2037 2038 public boolean hasNetwork() { 2039 return this.network != null && !this.network.isEmpty(); 2040 } 2041 2042 /** 2043 * @param value {@link #network} (The insurer-specific identifier for the 2044 * insurer-defined network of providers to which the beneficiary 2045 * may seek treatment which will be covered at the 'in-network' 2046 * rate, otherwise 'out of network' terms and conditions apply.). 2047 * This is the underlying object with id, value and extensions. The 2048 * accessor "getNetwork" gives direct access to the value 2049 */ 2050 public Coverage setNetworkElement(StringType value) { 2051 this.network = value; 2052 return this; 2053 } 2054 2055 /** 2056 * @return The insurer-specific identifier for the insurer-defined network of 2057 * providers to which the beneficiary may seek treatment which will be 2058 * covered at the 'in-network' rate, otherwise 'out of network' terms 2059 * and conditions apply. 2060 */ 2061 public String getNetwork() { 2062 return this.network == null ? null : this.network.getValue(); 2063 } 2064 2065 /** 2066 * @param value The insurer-specific identifier for the insurer-defined network 2067 * of providers to which the beneficiary may seek treatment which 2068 * will be covered at the 'in-network' rate, otherwise 'out of 2069 * network' terms and conditions apply. 2070 */ 2071 public Coverage setNetwork(String value) { 2072 if (Utilities.noString(value)) 2073 this.network = null; 2074 else { 2075 if (this.network == null) 2076 this.network = new StringType(); 2077 this.network.setValue(value); 2078 } 2079 return this; 2080 } 2081 2082 /** 2083 * @return {@link #costToBeneficiary} (A suite of codes indicating the cost 2084 * category and associated amount which have been detailed in the policy 2085 * and may have been included on the health card.) 2086 */ 2087 public List<CostToBeneficiaryComponent> getCostToBeneficiary() { 2088 if (this.costToBeneficiary == null) 2089 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2090 return this.costToBeneficiary; 2091 } 2092 2093 /** 2094 * @return Returns a reference to <code>this</code> for easy method chaining 2095 */ 2096 public Coverage setCostToBeneficiary(List<CostToBeneficiaryComponent> theCostToBeneficiary) { 2097 this.costToBeneficiary = theCostToBeneficiary; 2098 return this; 2099 } 2100 2101 public boolean hasCostToBeneficiary() { 2102 if (this.costToBeneficiary == null) 2103 return false; 2104 for (CostToBeneficiaryComponent item : this.costToBeneficiary) 2105 if (!item.isEmpty()) 2106 return true; 2107 return false; 2108 } 2109 2110 public CostToBeneficiaryComponent addCostToBeneficiary() { // 3 2111 CostToBeneficiaryComponent t = new CostToBeneficiaryComponent(); 2112 if (this.costToBeneficiary == null) 2113 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2114 this.costToBeneficiary.add(t); 2115 return t; 2116 } 2117 2118 public Coverage addCostToBeneficiary(CostToBeneficiaryComponent t) { // 3 2119 if (t == null) 2120 return this; 2121 if (this.costToBeneficiary == null) 2122 this.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2123 this.costToBeneficiary.add(t); 2124 return this; 2125 } 2126 2127 /** 2128 * @return The first repetition of repeating field {@link #costToBeneficiary}, 2129 * creating it if it does not already exist 2130 */ 2131 public CostToBeneficiaryComponent getCostToBeneficiaryFirstRep() { 2132 if (getCostToBeneficiary().isEmpty()) { 2133 addCostToBeneficiary(); 2134 } 2135 return getCostToBeneficiary().get(0); 2136 } 2137 2138 /** 2139 * @return {@link #subrogation} (When 'subrogation=true' this insurance instance 2140 * has been included not for adjudication but to provide insurers with 2141 * the details to recover costs.). This is the underlying object with 2142 * id, value and extensions. The accessor "getSubrogation" gives direct 2143 * access to the value 2144 */ 2145 public BooleanType getSubrogationElement() { 2146 if (this.subrogation == null) 2147 if (Configuration.errorOnAutoCreate()) 2148 throw new Error("Attempt to auto-create Coverage.subrogation"); 2149 else if (Configuration.doAutoCreate()) 2150 this.subrogation = new BooleanType(); // bb 2151 return this.subrogation; 2152 } 2153 2154 public boolean hasSubrogationElement() { 2155 return this.subrogation != null && !this.subrogation.isEmpty(); 2156 } 2157 2158 public boolean hasSubrogation() { 2159 return this.subrogation != null && !this.subrogation.isEmpty(); 2160 } 2161 2162 /** 2163 * @param value {@link #subrogation} (When 'subrogation=true' this insurance 2164 * instance has been included not for adjudication but to provide 2165 * insurers with the details to recover costs.). This is the 2166 * underlying object with id, value and extensions. The accessor 2167 * "getSubrogation" gives direct access to the value 2168 */ 2169 public Coverage setSubrogationElement(BooleanType value) { 2170 this.subrogation = value; 2171 return this; 2172 } 2173 2174 /** 2175 * @return When 'subrogation=true' this insurance instance has been included not 2176 * for adjudication but to provide insurers with the details to recover 2177 * costs. 2178 */ 2179 public boolean getSubrogation() { 2180 return this.subrogation == null || this.subrogation.isEmpty() ? false : this.subrogation.getValue(); 2181 } 2182 2183 /** 2184 * @param value When 'subrogation=true' this insurance instance has been 2185 * included not for adjudication but to provide insurers with the 2186 * details to recover costs. 2187 */ 2188 public Coverage setSubrogation(boolean value) { 2189 if (this.subrogation == null) 2190 this.subrogation = new BooleanType(); 2191 this.subrogation.setValue(value); 2192 return this; 2193 } 2194 2195 /** 2196 * @return {@link #contract} (The policy(s) which constitute this insurance 2197 * coverage.) 2198 */ 2199 public List<Reference> getContract() { 2200 if (this.contract == null) 2201 this.contract = new ArrayList<Reference>(); 2202 return this.contract; 2203 } 2204 2205 /** 2206 * @return Returns a reference to <code>this</code> for easy method chaining 2207 */ 2208 public Coverage setContract(List<Reference> theContract) { 2209 this.contract = theContract; 2210 return this; 2211 } 2212 2213 public boolean hasContract() { 2214 if (this.contract == null) 2215 return false; 2216 for (Reference item : this.contract) 2217 if (!item.isEmpty()) 2218 return true; 2219 return false; 2220 } 2221 2222 public Reference addContract() { // 3 2223 Reference t = new Reference(); 2224 if (this.contract == null) 2225 this.contract = new ArrayList<Reference>(); 2226 this.contract.add(t); 2227 return t; 2228 } 2229 2230 public Coverage addContract(Reference t) { // 3 2231 if (t == null) 2232 return this; 2233 if (this.contract == null) 2234 this.contract = new ArrayList<Reference>(); 2235 this.contract.add(t); 2236 return this; 2237 } 2238 2239 /** 2240 * @return The first repetition of repeating field {@link #contract}, creating 2241 * it if it does not already exist 2242 */ 2243 public Reference getContractFirstRep() { 2244 if (getContract().isEmpty()) { 2245 addContract(); 2246 } 2247 return getContract().get(0); 2248 } 2249 2250 /** 2251 * @deprecated Use Reference#setResource(IBaseResource) instead 2252 */ 2253 @Deprecated 2254 public List<Contract> getContractTarget() { 2255 if (this.contractTarget == null) 2256 this.contractTarget = new ArrayList<Contract>(); 2257 return this.contractTarget; 2258 } 2259 2260 /** 2261 * @deprecated Use Reference#setResource(IBaseResource) instead 2262 */ 2263 @Deprecated 2264 public Contract addContractTarget() { 2265 Contract r = new Contract(); 2266 if (this.contractTarget == null) 2267 this.contractTarget = new ArrayList<Contract>(); 2268 this.contractTarget.add(r); 2269 return r; 2270 } 2271 2272 protected void listChildren(List<Property> children) { 2273 super.listChildren(children); 2274 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this coverage.", 0, 2275 java.lang.Integer.MAX_VALUE, identifier)); 2276 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2277 children.add(new Property("type", "CodeableConcept", 2278 "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 2279 0, 1, type)); 2280 children.add(new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", 2281 "The party who 'owns' the insurance policy.", 0, 1, policyHolder)); 2282 children.add(new Property("subscriber", "Reference(Patient|RelatedPerson)", 2283 "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 2284 0, 1, subscriber)); 2285 children 2286 .add(new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 1, subscriberId)); 2287 children.add(new Property("beneficiary", "Reference(Patient)", 2288 "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 2289 0, 1, beneficiary)); 2290 children.add(new Property("dependent", "string", "A unique identifier for a dependent under the coverage.", 0, 1, 2291 dependent)); 2292 children.add(new Property("relationship", "CodeableConcept", 2293 "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship)); 2294 children.add(new Property("period", "Period", 2295 "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 2296 0, 1, period)); 2297 children.add(new Property("payor", "Reference(Organization|Patient|RelatedPerson)", 2298 "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.", 2299 0, java.lang.Integer.MAX_VALUE, payor)); 2300 children.add(new Property("class", "", "A suite of underwriter specific classifiers.", 0, 2301 java.lang.Integer.MAX_VALUE, class_)); 2302 children.add(new Property("order", "positiveInt", 2303 "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.", 2304 0, 1, order)); 2305 children.add(new Property("network", "string", 2306 "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 2307 0, 1, network)); 2308 children.add(new Property("costToBeneficiary", "", 2309 "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 2310 0, java.lang.Integer.MAX_VALUE, costToBeneficiary)); 2311 children.add(new Property("subrogation", "boolean", 2312 "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 2313 0, 1, subrogation)); 2314 children.add(new Property("contract", "Reference(Contract)", 2315 "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract)); 2316 } 2317 2318 @Override 2319 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2320 switch (_hash) { 2321 case -1618432855: 2322 /* identifier */ return new Property("identifier", "Identifier", "A unique identifier assigned to this coverage.", 2323 0, java.lang.Integer.MAX_VALUE, identifier); 2324 case -892481550: 2325 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2326 case 3575610: 2327 /* type */ return new Property("type", "CodeableConcept", 2328 "The type of coverage: social program, medical plan, accident coverage (workers compensation, auto), group health or payment by an individual or organization.", 2329 0, 1, type); 2330 case 2046898558: 2331 /* policyHolder */ return new Property("policyHolder", "Reference(Patient|RelatedPerson|Organization)", 2332 "The party who 'owns' the insurance policy.", 0, 1, policyHolder); 2333 case -1219769240: 2334 /* subscriber */ return new Property("subscriber", "Reference(Patient|RelatedPerson)", 2335 "The party who has signed-up for or 'owns' the contractual relationship to the policy or to whom the benefit of the policy for services rendered to them or their family is due.", 2336 0, 1, subscriber); 2337 case 327834531: 2338 /* subscriberId */ return new Property("subscriberId", "string", "The insurer assigned ID for the Subscriber.", 0, 2339 1, subscriberId); 2340 case -565102875: 2341 /* beneficiary */ return new Property("beneficiary", "Reference(Patient)", 2342 "The party who benefits from the insurance coverage; the patient when products and/or services are provided.", 2343 0, 1, beneficiary); 2344 case -1109226753: 2345 /* dependent */ return new Property("dependent", "string", 2346 "A unique identifier for a dependent under the coverage.", 0, 1, dependent); 2347 case -261851592: 2348 /* relationship */ return new Property("relationship", "CodeableConcept", 2349 "The relationship of beneficiary (patient) to the subscriber.", 0, 1, relationship); 2350 case -991726143: 2351 /* period */ return new Property("period", "Period", 2352 "Time period during which the coverage is in force. A missing start date indicates the start date isn't known, a missing end date means the coverage is continuing to be in force.", 2353 0, 1, period); 2354 case 106443915: 2355 /* payor */ return new Property("payor", "Reference(Organization|Patient|RelatedPerson)", 2356 "The program or plan underwriter or payor including both insurance and non-insurance agreements, such as patient-pay agreements.", 2357 0, java.lang.Integer.MAX_VALUE, payor); 2358 case 94742904: 2359 /* class */ return new Property("class", "", "A suite of underwriter specific classifiers.", 0, 2360 java.lang.Integer.MAX_VALUE, class_); 2361 case 106006350: 2362 /* order */ return new Property("order", "positiveInt", 2363 "The order of applicability of this coverage relative to other coverages which are currently in force. Note, there may be gaps in the numbering and this does not imply primary, secondary etc. as the specific positioning of coverages depends upon the episode of care.", 2364 0, 1, order); 2365 case 1843485230: 2366 /* network */ return new Property("network", "string", 2367 "The insurer-specific identifier for the insurer-defined network of providers to which the beneficiary may seek treatment which will be covered at the 'in-network' rate, otherwise 'out of network' terms and conditions apply.", 2368 0, 1, network); 2369 case -1866474851: 2370 /* costToBeneficiary */ return new Property("costToBeneficiary", "", 2371 "A suite of codes indicating the cost category and associated amount which have been detailed in the policy and may have been included on the health card.", 2372 0, java.lang.Integer.MAX_VALUE, costToBeneficiary); 2373 case 837389739: 2374 /* subrogation */ return new Property("subrogation", "boolean", 2375 "When 'subrogation=true' this insurance instance has been included not for adjudication but to provide insurers with the details to recover costs.", 2376 0, 1, subrogation); 2377 case -566947566: 2378 /* contract */ return new Property("contract", "Reference(Contract)", 2379 "The policy(s) which constitute this insurance coverage.", 0, java.lang.Integer.MAX_VALUE, contract); 2380 default: 2381 return super.getNamedProperty(_hash, _name, _checkValid); 2382 } 2383 2384 } 2385 2386 @Override 2387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2388 switch (hash) { 2389 case -1618432855: 2390 /* identifier */ return this.identifier == null ? new Base[0] 2391 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2392 case -892481550: 2393 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<CoverageStatus> 2394 case 3575610: 2395 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2396 case 2046898558: 2397 /* policyHolder */ return this.policyHolder == null ? new Base[0] : new Base[] { this.policyHolder }; // Reference 2398 case -1219769240: 2399 /* subscriber */ return this.subscriber == null ? new Base[0] : new Base[] { this.subscriber }; // Reference 2400 case 327834531: 2401 /* subscriberId */ return this.subscriberId == null ? new Base[0] : new Base[] { this.subscriberId }; // StringType 2402 case -565102875: 2403 /* beneficiary */ return this.beneficiary == null ? new Base[0] : new Base[] { this.beneficiary }; // Reference 2404 case -1109226753: 2405 /* dependent */ return this.dependent == null ? new Base[0] : new Base[] { this.dependent }; // StringType 2406 case -261851592: 2407 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 2408 case -991726143: 2409 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2410 case 106443915: 2411 /* payor */ return this.payor == null ? new Base[0] : this.payor.toArray(new Base[this.payor.size()]); // Reference 2412 case 94742904: 2413 /* class */ return this.class_ == null ? new Base[0] : this.class_.toArray(new Base[this.class_.size()]); // ClassComponent 2414 case 106006350: 2415 /* order */ return this.order == null ? new Base[0] : new Base[] { this.order }; // PositiveIntType 2416 case 1843485230: 2417 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // StringType 2418 case -1866474851: 2419 /* costToBeneficiary */ return this.costToBeneficiary == null ? new Base[0] 2420 : this.costToBeneficiary.toArray(new Base[this.costToBeneficiary.size()]); // CostToBeneficiaryComponent 2421 case 837389739: 2422 /* subrogation */ return this.subrogation == null ? new Base[0] : new Base[] { this.subrogation }; // BooleanType 2423 case -566947566: 2424 /* contract */ return this.contract == null ? new Base[0] : this.contract.toArray(new Base[this.contract.size()]); // Reference 2425 default: 2426 return super.getProperty(hash, name, checkValid); 2427 } 2428 2429 } 2430 2431 @Override 2432 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2433 switch (hash) { 2434 case -1618432855: // identifier 2435 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2436 return value; 2437 case -892481550: // status 2438 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2439 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2440 return value; 2441 case 3575610: // type 2442 this.type = castToCodeableConcept(value); // CodeableConcept 2443 return value; 2444 case 2046898558: // policyHolder 2445 this.policyHolder = castToReference(value); // Reference 2446 return value; 2447 case -1219769240: // subscriber 2448 this.subscriber = castToReference(value); // Reference 2449 return value; 2450 case 327834531: // subscriberId 2451 this.subscriberId = castToString(value); // StringType 2452 return value; 2453 case -565102875: // beneficiary 2454 this.beneficiary = castToReference(value); // Reference 2455 return value; 2456 case -1109226753: // dependent 2457 this.dependent = castToString(value); // StringType 2458 return value; 2459 case -261851592: // relationship 2460 this.relationship = castToCodeableConcept(value); // CodeableConcept 2461 return value; 2462 case -991726143: // period 2463 this.period = castToPeriod(value); // Period 2464 return value; 2465 case 106443915: // payor 2466 this.getPayor().add(castToReference(value)); // Reference 2467 return value; 2468 case 94742904: // class 2469 this.getClass_().add((ClassComponent) value); // ClassComponent 2470 return value; 2471 case 106006350: // order 2472 this.order = castToPositiveInt(value); // PositiveIntType 2473 return value; 2474 case 1843485230: // network 2475 this.network = castToString(value); // StringType 2476 return value; 2477 case -1866474851: // costToBeneficiary 2478 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); // CostToBeneficiaryComponent 2479 return value; 2480 case 837389739: // subrogation 2481 this.subrogation = castToBoolean(value); // BooleanType 2482 return value; 2483 case -566947566: // contract 2484 this.getContract().add(castToReference(value)); // Reference 2485 return value; 2486 default: 2487 return super.setProperty(hash, name, value); 2488 } 2489 2490 } 2491 2492 @Override 2493 public Base setProperty(String name, Base value) throws FHIRException { 2494 if (name.equals("identifier")) { 2495 this.getIdentifier().add(castToIdentifier(value)); 2496 } else if (name.equals("status")) { 2497 value = new CoverageStatusEnumFactory().fromType(castToCode(value)); 2498 this.status = (Enumeration) value; // Enumeration<CoverageStatus> 2499 } else if (name.equals("type")) { 2500 this.type = castToCodeableConcept(value); // CodeableConcept 2501 } else if (name.equals("policyHolder")) { 2502 this.policyHolder = castToReference(value); // Reference 2503 } else if (name.equals("subscriber")) { 2504 this.subscriber = castToReference(value); // Reference 2505 } else if (name.equals("subscriberId")) { 2506 this.subscriberId = castToString(value); // StringType 2507 } else if (name.equals("beneficiary")) { 2508 this.beneficiary = castToReference(value); // Reference 2509 } else if (name.equals("dependent")) { 2510 this.dependent = castToString(value); // StringType 2511 } else if (name.equals("relationship")) { 2512 this.relationship = castToCodeableConcept(value); // CodeableConcept 2513 } else if (name.equals("period")) { 2514 this.period = castToPeriod(value); // Period 2515 } else if (name.equals("payor")) { 2516 this.getPayor().add(castToReference(value)); 2517 } else if (name.equals("class")) { 2518 this.getClass_().add((ClassComponent) value); 2519 } else if (name.equals("order")) { 2520 this.order = castToPositiveInt(value); // PositiveIntType 2521 } else if (name.equals("network")) { 2522 this.network = castToString(value); // StringType 2523 } else if (name.equals("costToBeneficiary")) { 2524 this.getCostToBeneficiary().add((CostToBeneficiaryComponent) value); 2525 } else if (name.equals("subrogation")) { 2526 this.subrogation = castToBoolean(value); // BooleanType 2527 } else if (name.equals("contract")) { 2528 this.getContract().add(castToReference(value)); 2529 } else 2530 return super.setProperty(name, value); 2531 return value; 2532 } 2533 2534 @Override 2535 public void removeChild(String name, Base value) throws FHIRException { 2536 if (name.equals("identifier")) { 2537 this.getIdentifier().remove(castToIdentifier(value)); 2538 } else if (name.equals("status")) { 2539 this.status = null; 2540 } else if (name.equals("type")) { 2541 this.type = null; 2542 } else if (name.equals("policyHolder")) { 2543 this.policyHolder = null; 2544 } else if (name.equals("subscriber")) { 2545 this.subscriber = null; 2546 } else if (name.equals("subscriberId")) { 2547 this.subscriberId = null; 2548 } else if (name.equals("beneficiary")) { 2549 this.beneficiary = null; 2550 } else if (name.equals("dependent")) { 2551 this.dependent = null; 2552 } else if (name.equals("relationship")) { 2553 this.relationship = null; 2554 } else if (name.equals("period")) { 2555 this.period = null; 2556 } else if (name.equals("payor")) { 2557 this.getPayor().remove(castToReference(value)); 2558 } else if (name.equals("class")) { 2559 this.getClass_().remove((ClassComponent) value); 2560 } else if (name.equals("order")) { 2561 this.order = null; 2562 } else if (name.equals("network")) { 2563 this.network = null; 2564 } else if (name.equals("costToBeneficiary")) { 2565 this.getCostToBeneficiary().remove((CostToBeneficiaryComponent) value); 2566 } else if (name.equals("subrogation")) { 2567 this.subrogation = null; 2568 } else if (name.equals("contract")) { 2569 this.getContract().remove(castToReference(value)); 2570 } else 2571 super.removeChild(name, value); 2572 2573 } 2574 2575 @Override 2576 public Base makeProperty(int hash, String name) throws FHIRException { 2577 switch (hash) { 2578 case -1618432855: 2579 return addIdentifier(); 2580 case -892481550: 2581 return getStatusElement(); 2582 case 3575610: 2583 return getType(); 2584 case 2046898558: 2585 return getPolicyHolder(); 2586 case -1219769240: 2587 return getSubscriber(); 2588 case 327834531: 2589 return getSubscriberIdElement(); 2590 case -565102875: 2591 return getBeneficiary(); 2592 case -1109226753: 2593 return getDependentElement(); 2594 case -261851592: 2595 return getRelationship(); 2596 case -991726143: 2597 return getPeriod(); 2598 case 106443915: 2599 return addPayor(); 2600 case 94742904: 2601 return addClass_(); 2602 case 106006350: 2603 return getOrderElement(); 2604 case 1843485230: 2605 return getNetworkElement(); 2606 case -1866474851: 2607 return addCostToBeneficiary(); 2608 case 837389739: 2609 return getSubrogationElement(); 2610 case -566947566: 2611 return addContract(); 2612 default: 2613 return super.makeProperty(hash, name); 2614 } 2615 2616 } 2617 2618 @Override 2619 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2620 switch (hash) { 2621 case -1618432855: 2622 /* identifier */ return new String[] { "Identifier" }; 2623 case -892481550: 2624 /* status */ return new String[] { "code" }; 2625 case 3575610: 2626 /* type */ return new String[] { "CodeableConcept" }; 2627 case 2046898558: 2628 /* policyHolder */ return new String[] { "Reference" }; 2629 case -1219769240: 2630 /* subscriber */ return new String[] { "Reference" }; 2631 case 327834531: 2632 /* subscriberId */ return new String[] { "string" }; 2633 case -565102875: 2634 /* beneficiary */ return new String[] { "Reference" }; 2635 case -1109226753: 2636 /* dependent */ return new String[] { "string" }; 2637 case -261851592: 2638 /* relationship */ return new String[] { "CodeableConcept" }; 2639 case -991726143: 2640 /* period */ return new String[] { "Period" }; 2641 case 106443915: 2642 /* payor */ return new String[] { "Reference" }; 2643 case 94742904: 2644 /* class */ return new String[] {}; 2645 case 106006350: 2646 /* order */ return new String[] { "positiveInt" }; 2647 case 1843485230: 2648 /* network */ return new String[] { "string" }; 2649 case -1866474851: 2650 /* costToBeneficiary */ return new String[] {}; 2651 case 837389739: 2652 /* subrogation */ return new String[] { "boolean" }; 2653 case -566947566: 2654 /* contract */ return new String[] { "Reference" }; 2655 default: 2656 return super.getTypesForProperty(hash, name); 2657 } 2658 2659 } 2660 2661 @Override 2662 public Base addChild(String name) throws FHIRException { 2663 if (name.equals("identifier")) { 2664 return addIdentifier(); 2665 } else if (name.equals("status")) { 2666 throw new FHIRException("Cannot call addChild on a singleton property Coverage.status"); 2667 } else if (name.equals("type")) { 2668 this.type = new CodeableConcept(); 2669 return this.type; 2670 } else if (name.equals("policyHolder")) { 2671 this.policyHolder = new Reference(); 2672 return this.policyHolder; 2673 } else if (name.equals("subscriber")) { 2674 this.subscriber = new Reference(); 2675 return this.subscriber; 2676 } else if (name.equals("subscriberId")) { 2677 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subscriberId"); 2678 } else if (name.equals("beneficiary")) { 2679 this.beneficiary = new Reference(); 2680 return this.beneficiary; 2681 } else if (name.equals("dependent")) { 2682 throw new FHIRException("Cannot call addChild on a singleton property Coverage.dependent"); 2683 } else if (name.equals("relationship")) { 2684 this.relationship = new CodeableConcept(); 2685 return this.relationship; 2686 } else if (name.equals("period")) { 2687 this.period = new Period(); 2688 return this.period; 2689 } else if (name.equals("payor")) { 2690 return addPayor(); 2691 } else if (name.equals("class")) { 2692 return addClass_(); 2693 } else if (name.equals("order")) { 2694 throw new FHIRException("Cannot call addChild on a singleton property Coverage.order"); 2695 } else if (name.equals("network")) { 2696 throw new FHIRException("Cannot call addChild on a singleton property Coverage.network"); 2697 } else if (name.equals("costToBeneficiary")) { 2698 return addCostToBeneficiary(); 2699 } else if (name.equals("subrogation")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property Coverage.subrogation"); 2701 } else if (name.equals("contract")) { 2702 return addContract(); 2703 } else 2704 return super.addChild(name); 2705 } 2706 2707 public String fhirType() { 2708 return "Coverage"; 2709 2710 } 2711 2712 public Coverage copy() { 2713 Coverage dst = new Coverage(); 2714 copyValues(dst); 2715 return dst; 2716 } 2717 2718 public void copyValues(Coverage dst) { 2719 super.copyValues(dst); 2720 if (identifier != null) { 2721 dst.identifier = new ArrayList<Identifier>(); 2722 for (Identifier i : identifier) 2723 dst.identifier.add(i.copy()); 2724 } 2725 ; 2726 dst.status = status == null ? null : status.copy(); 2727 dst.type = type == null ? null : type.copy(); 2728 dst.policyHolder = policyHolder == null ? null : policyHolder.copy(); 2729 dst.subscriber = subscriber == null ? null : subscriber.copy(); 2730 dst.subscriberId = subscriberId == null ? null : subscriberId.copy(); 2731 dst.beneficiary = beneficiary == null ? null : beneficiary.copy(); 2732 dst.dependent = dependent == null ? null : dependent.copy(); 2733 dst.relationship = relationship == null ? null : relationship.copy(); 2734 dst.period = period == null ? null : period.copy(); 2735 if (payor != null) { 2736 dst.payor = new ArrayList<Reference>(); 2737 for (Reference i : payor) 2738 dst.payor.add(i.copy()); 2739 } 2740 ; 2741 if (class_ != null) { 2742 dst.class_ = new ArrayList<ClassComponent>(); 2743 for (ClassComponent i : class_) 2744 dst.class_.add(i.copy()); 2745 } 2746 ; 2747 dst.order = order == null ? null : order.copy(); 2748 dst.network = network == null ? null : network.copy(); 2749 if (costToBeneficiary != null) { 2750 dst.costToBeneficiary = new ArrayList<CostToBeneficiaryComponent>(); 2751 for (CostToBeneficiaryComponent i : costToBeneficiary) 2752 dst.costToBeneficiary.add(i.copy()); 2753 } 2754 ; 2755 dst.subrogation = subrogation == null ? null : subrogation.copy(); 2756 if (contract != null) { 2757 dst.contract = new ArrayList<Reference>(); 2758 for (Reference i : contract) 2759 dst.contract.add(i.copy()); 2760 } 2761 ; 2762 } 2763 2764 protected Coverage typedCopy() { 2765 return copy(); 2766 } 2767 2768 @Override 2769 public boolean equalsDeep(Base other_) { 2770 if (!super.equalsDeep(other_)) 2771 return false; 2772 if (!(other_ instanceof Coverage)) 2773 return false; 2774 Coverage o = (Coverage) other_; 2775 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2776 && compareDeep(type, o.type, true) && compareDeep(policyHolder, o.policyHolder, true) 2777 && compareDeep(subscriber, o.subscriber, true) && compareDeep(subscriberId, o.subscriberId, true) 2778 && compareDeep(beneficiary, o.beneficiary, true) && compareDeep(dependent, o.dependent, true) 2779 && compareDeep(relationship, o.relationship, true) && compareDeep(period, o.period, true) 2780 && compareDeep(payor, o.payor, true) && compareDeep(class_, o.class_, true) && compareDeep(order, o.order, true) 2781 && compareDeep(network, o.network, true) && compareDeep(costToBeneficiary, o.costToBeneficiary, true) 2782 && compareDeep(subrogation, o.subrogation, true) && compareDeep(contract, o.contract, true); 2783 } 2784 2785 @Override 2786 public boolean equalsShallow(Base other_) { 2787 if (!super.equalsShallow(other_)) 2788 return false; 2789 if (!(other_ instanceof Coverage)) 2790 return false; 2791 Coverage o = (Coverage) other_; 2792 return compareValues(status, o.status, true) && compareValues(subscriberId, o.subscriberId, true) 2793 && compareValues(dependent, o.dependent, true) && compareValues(order, o.order, true) 2794 && compareValues(network, o.network, true) && compareValues(subrogation, o.subrogation, true); 2795 } 2796 2797 public boolean isEmpty() { 2798 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, policyHolder, subscriber, 2799 subscriberId, beneficiary, dependent, relationship, period, payor, class_, order, network, costToBeneficiary, 2800 subrogation, contract); 2801 } 2802 2803 @Override 2804 public ResourceType getResourceType() { 2805 return ResourceType.Coverage; 2806 } 2807 2808 /** 2809 * Search parameter: <b>identifier</b> 2810 * <p> 2811 * Description: <b>The primary identifier of the insured and the 2812 * coverage</b><br> 2813 * Type: <b>token</b><br> 2814 * Path: <b>Coverage.identifier</b><br> 2815 * </p> 2816 */ 2817 @SearchParamDefinition(name = "identifier", path = "Coverage.identifier", description = "The primary identifier of the insured and the coverage", type = "token") 2818 public static final String SP_IDENTIFIER = "identifier"; 2819 /** 2820 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2821 * <p> 2822 * Description: <b>The primary identifier of the insured and the 2823 * coverage</b><br> 2824 * Type: <b>token</b><br> 2825 * Path: <b>Coverage.identifier</b><br> 2826 * </p> 2827 */ 2828 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2829 SP_IDENTIFIER); 2830 2831 /** 2832 * Search parameter: <b>payor</b> 2833 * <p> 2834 * Description: <b>The identity of the insurer or party paying for 2835 * services</b><br> 2836 * Type: <b>reference</b><br> 2837 * Path: <b>Coverage.payor</b><br> 2838 * </p> 2839 */ 2840 @SearchParamDefinition(name = "payor", path = "Coverage.payor", description = "The identity of the insurer or party paying for services", type = "reference", providesMembershipIn = { 2841 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2842 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 2843 Patient.class, RelatedPerson.class }) 2844 public static final String SP_PAYOR = "payor"; 2845 /** 2846 * <b>Fluent Client</b> search parameter constant for <b>payor</b> 2847 * <p> 2848 * Description: <b>The identity of the insurer or party paying for 2849 * services</b><br> 2850 * Type: <b>reference</b><br> 2851 * Path: <b>Coverage.payor</b><br> 2852 * </p> 2853 */ 2854 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2855 SP_PAYOR); 2856 2857 /** 2858 * Constant for fluent queries to be used to add include statements. Specifies 2859 * the path value of "<b>Coverage:payor</b>". 2860 */ 2861 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYOR = new ca.uhn.fhir.model.api.Include("Coverage:payor") 2862 .toLocked(); 2863 2864 /** 2865 * Search parameter: <b>subscriber</b> 2866 * <p> 2867 * Description: <b>Reference to the subscriber</b><br> 2868 * Type: <b>reference</b><br> 2869 * Path: <b>Coverage.subscriber</b><br> 2870 * </p> 2871 */ 2872 @SearchParamDefinition(name = "subscriber", path = "Coverage.subscriber", description = "Reference to the subscriber", type = "reference", providesMembershipIn = { 2873 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2874 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Patient.class, 2875 RelatedPerson.class }) 2876 public static final String SP_SUBSCRIBER = "subscriber"; 2877 /** 2878 * <b>Fluent Client</b> search parameter constant for <b>subscriber</b> 2879 * <p> 2880 * Description: <b>Reference to the subscriber</b><br> 2881 * Type: <b>reference</b><br> 2882 * Path: <b>Coverage.subscriber</b><br> 2883 * </p> 2884 */ 2885 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSCRIBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2886 SP_SUBSCRIBER); 2887 2888 /** 2889 * Constant for fluent queries to be used to add include statements. Specifies 2890 * the path value of "<b>Coverage:subscriber</b>". 2891 */ 2892 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSCRIBER = new ca.uhn.fhir.model.api.Include( 2893 "Coverage:subscriber").toLocked(); 2894 2895 /** 2896 * Search parameter: <b>beneficiary</b> 2897 * <p> 2898 * Description: <b>Covered party</b><br> 2899 * Type: <b>reference</b><br> 2900 * Path: <b>Coverage.beneficiary</b><br> 2901 * </p> 2902 */ 2903 @SearchParamDefinition(name = "beneficiary", path = "Coverage.beneficiary", description = "Covered party", type = "reference", providesMembershipIn = { 2904 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2905 public static final String SP_BENEFICIARY = "beneficiary"; 2906 /** 2907 * <b>Fluent Client</b> search parameter constant for <b>beneficiary</b> 2908 * <p> 2909 * Description: <b>Covered party</b><br> 2910 * Type: <b>reference</b><br> 2911 * Path: <b>Coverage.beneficiary</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BENEFICIARY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2915 SP_BENEFICIARY); 2916 2917 /** 2918 * Constant for fluent queries to be used to add include statements. Specifies 2919 * the path value of "<b>Coverage:beneficiary</b>". 2920 */ 2921 public static final ca.uhn.fhir.model.api.Include INCLUDE_BENEFICIARY = new ca.uhn.fhir.model.api.Include( 2922 "Coverage:beneficiary").toLocked(); 2923 2924 /** 2925 * Search parameter: <b>patient</b> 2926 * <p> 2927 * Description: <b>Retrieve coverages for a patient</b><br> 2928 * Type: <b>reference</b><br> 2929 * Path: <b>Coverage.beneficiary</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name = "patient", path = "Coverage.beneficiary", description = "Retrieve coverages for a patient", type = "reference", target = { 2933 Patient.class }) 2934 public static final String SP_PATIENT = "patient"; 2935 /** 2936 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2937 * <p> 2938 * Description: <b>Retrieve coverages for a patient</b><br> 2939 * Type: <b>reference</b><br> 2940 * Path: <b>Coverage.beneficiary</b><br> 2941 * </p> 2942 */ 2943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2944 SP_PATIENT); 2945 2946 /** 2947 * Constant for fluent queries to be used to add include statements. Specifies 2948 * the path value of "<b>Coverage:patient</b>". 2949 */ 2950 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2951 "Coverage:patient").toLocked(); 2952 2953 /** 2954 * Search parameter: <b>class-value</b> 2955 * <p> 2956 * Description: <b>Value of the class (eg. Plan number, group number)</b><br> 2957 * Type: <b>string</b><br> 2958 * Path: <b>Coverage.class.value</b><br> 2959 * </p> 2960 */ 2961 @SearchParamDefinition(name = "class-value", path = "Coverage.class.value", description = "Value of the class (eg. Plan number, group number)", type = "string") 2962 public static final String SP_CLASS_VALUE = "class-value"; 2963 /** 2964 * <b>Fluent Client</b> search parameter constant for <b>class-value</b> 2965 * <p> 2966 * Description: <b>Value of the class (eg. Plan number, group number)</b><br> 2967 * Type: <b>string</b><br> 2968 * Path: <b>Coverage.class.value</b><br> 2969 * </p> 2970 */ 2971 public static final ca.uhn.fhir.rest.gclient.StringClientParam CLASS_VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2972 SP_CLASS_VALUE); 2973 2974 /** 2975 * Search parameter: <b>type</b> 2976 * <p> 2977 * Description: <b>The kind of coverage (health plan, auto, Workers 2978 * Compensation)</b><br> 2979 * Type: <b>token</b><br> 2980 * Path: <b>Coverage.type</b><br> 2981 * </p> 2982 */ 2983 @SearchParamDefinition(name = "type", path = "Coverage.type", description = "The kind of coverage (health plan, auto, Workers Compensation)", type = "token") 2984 public static final String SP_TYPE = "type"; 2985 /** 2986 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2987 * <p> 2988 * Description: <b>The kind of coverage (health plan, auto, Workers 2989 * Compensation)</b><br> 2990 * Type: <b>token</b><br> 2991 * Path: <b>Coverage.type</b><br> 2992 * </p> 2993 */ 2994 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2995 SP_TYPE); 2996 2997 /** 2998 * Search parameter: <b>dependent</b> 2999 * <p> 3000 * Description: <b>Dependent number</b><br> 3001 * Type: <b>string</b><br> 3002 * Path: <b>Coverage.dependent</b><br> 3003 * </p> 3004 */ 3005 @SearchParamDefinition(name = "dependent", path = "Coverage.dependent", description = "Dependent number", type = "string") 3006 public static final String SP_DEPENDENT = "dependent"; 3007 /** 3008 * <b>Fluent Client</b> search parameter constant for <b>dependent</b> 3009 * <p> 3010 * Description: <b>Dependent number</b><br> 3011 * Type: <b>string</b><br> 3012 * Path: <b>Coverage.dependent</b><br> 3013 * </p> 3014 */ 3015 public static final ca.uhn.fhir.rest.gclient.StringClientParam DEPENDENT = new ca.uhn.fhir.rest.gclient.StringClientParam( 3016 SP_DEPENDENT); 3017 3018 /** 3019 * Search parameter: <b>class-type</b> 3020 * <p> 3021 * Description: <b>Coverage class (eg. plan, group)</b><br> 3022 * Type: <b>token</b><br> 3023 * Path: <b>Coverage.class.type</b><br> 3024 * </p> 3025 */ 3026 @SearchParamDefinition(name = "class-type", path = "Coverage.class.type", description = "Coverage class (eg. plan, group)", type = "token") 3027 public static final String SP_CLASS_TYPE = "class-type"; 3028 /** 3029 * <b>Fluent Client</b> search parameter constant for <b>class-type</b> 3030 * <p> 3031 * Description: <b>Coverage class (eg. plan, group)</b><br> 3032 * Type: <b>token</b><br> 3033 * Path: <b>Coverage.class.type</b><br> 3034 * </p> 3035 */ 3036 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3037 SP_CLASS_TYPE); 3038 3039 /** 3040 * Search parameter: <b>policy-holder</b> 3041 * <p> 3042 * Description: <b>Reference to the policyholder</b><br> 3043 * Type: <b>reference</b><br> 3044 * Path: <b>Coverage.policyHolder</b><br> 3045 * </p> 3046 */ 3047 @SearchParamDefinition(name = "policy-holder", path = "Coverage.policyHolder", description = "Reference to the policyholder", type = "reference", providesMembershipIn = { 3048 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3049 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 3050 Patient.class, RelatedPerson.class }) 3051 public static final String SP_POLICY_HOLDER = "policy-holder"; 3052 /** 3053 * <b>Fluent Client</b> search parameter constant for <b>policy-holder</b> 3054 * <p> 3055 * Description: <b>Reference to the policyholder</b><br> 3056 * Type: <b>reference</b><br> 3057 * Path: <b>Coverage.policyHolder</b><br> 3058 * </p> 3059 */ 3060 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam POLICY_HOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3061 SP_POLICY_HOLDER); 3062 3063 /** 3064 * Constant for fluent queries to be used to add include statements. Specifies 3065 * the path value of "<b>Coverage:policy-holder</b>". 3066 */ 3067 public static final ca.uhn.fhir.model.api.Include INCLUDE_POLICY_HOLDER = new ca.uhn.fhir.model.api.Include( 3068 "Coverage:policy-holder").toLocked(); 3069 3070 /** 3071 * Search parameter: <b>status</b> 3072 * <p> 3073 * Description: <b>The status of the Coverage</b><br> 3074 * Type: <b>token</b><br> 3075 * Path: <b>Coverage.status</b><br> 3076 * </p> 3077 */ 3078 @SearchParamDefinition(name = "status", path = "Coverage.status", description = "The status of the Coverage", type = "token") 3079 public static final String SP_STATUS = "status"; 3080 /** 3081 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3082 * <p> 3083 * Description: <b>The status of the Coverage</b><br> 3084 * Type: <b>token</b><br> 3085 * Path: <b>Coverage.status</b><br> 3086 * </p> 3087 */ 3088 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3089 SP_STATUS); 3090 3091}