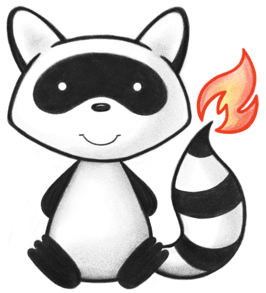
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcome; 040import org.hl7.fhir.r4.model.Enumerations.RemittanceOutcomeEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * This resource provides eligibility and plan details from the processing of an 051 * CoverageEligibilityRequest resource. 052 */ 053@ResourceDef(name = "CoverageEligibilityResponse", profile = "http://hl7.org/fhir/StructureDefinition/CoverageEligibilityResponse") 054public class CoverageEligibilityResponse extends DomainResource { 055 056 public enum EligibilityResponseStatus { 057 /** 058 * The instance is currently in-force. 059 */ 060 ACTIVE, 061 /** 062 * The instance is withdrawn, rescinded or reversed. 063 */ 064 CANCELLED, 065 /** 066 * A new instance the contents of which is not complete. 067 */ 068 DRAFT, 069 /** 070 * The instance was entered in error. 071 */ 072 ENTEREDINERROR, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static EligibilityResponseStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("cancelled".equals(codeString)) 084 return CANCELLED; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown EligibilityResponseStatus code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case ACTIVE: 098 return "active"; 099 case CANCELLED: 100 return "cancelled"; 101 case DRAFT: 102 return "draft"; 103 case ENTEREDINERROR: 104 return "entered-in-error"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case ACTIVE: 115 return "http://hl7.org/fhir/fm-status"; 116 case CANCELLED: 117 return "http://hl7.org/fhir/fm-status"; 118 case DRAFT: 119 return "http://hl7.org/fhir/fm-status"; 120 case ENTEREDINERROR: 121 return "http://hl7.org/fhir/fm-status"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case ACTIVE: 132 return "The instance is currently in-force."; 133 case CANCELLED: 134 return "The instance is withdrawn, rescinded or reversed."; 135 case DRAFT: 136 return "A new instance the contents of which is not complete."; 137 case ENTEREDINERROR: 138 return "The instance was entered in error."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case ACTIVE: 149 return "Active"; 150 case CANCELLED: 151 return "Cancelled"; 152 case DRAFT: 153 return "Draft"; 154 case ENTEREDINERROR: 155 return "Entered in Error"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 } 163 164 public static class EligibilityResponseStatusEnumFactory implements EnumFactory<EligibilityResponseStatus> { 165 public EligibilityResponseStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("active".equals(codeString)) 170 return EligibilityResponseStatus.ACTIVE; 171 if ("cancelled".equals(codeString)) 172 return EligibilityResponseStatus.CANCELLED; 173 if ("draft".equals(codeString)) 174 return EligibilityResponseStatus.DRAFT; 175 if ("entered-in-error".equals(codeString)) 176 return EligibilityResponseStatus.ENTEREDINERROR; 177 throw new IllegalArgumentException("Unknown EligibilityResponseStatus code '" + codeString + "'"); 178 } 179 180 public Enumeration<EligibilityResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.NULL, code); 188 if ("active".equals(codeString)) 189 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.ACTIVE, code); 190 if ("cancelled".equals(codeString)) 191 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.CANCELLED, code); 192 if ("draft".equals(codeString)) 193 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.DRAFT, code); 194 if ("entered-in-error".equals(codeString)) 195 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.ENTEREDINERROR, code); 196 throw new FHIRException("Unknown EligibilityResponseStatus code '" + codeString + "'"); 197 } 198 199 public String toCode(EligibilityResponseStatus code) { 200 if (code == EligibilityResponseStatus.NULL) 201 return null; 202 if (code == EligibilityResponseStatus.ACTIVE) 203 return "active"; 204 if (code == EligibilityResponseStatus.CANCELLED) 205 return "cancelled"; 206 if (code == EligibilityResponseStatus.DRAFT) 207 return "draft"; 208 if (code == EligibilityResponseStatus.ENTEREDINERROR) 209 return "entered-in-error"; 210 return "?"; 211 } 212 213 public String toSystem(EligibilityResponseStatus code) { 214 return code.getSystem(); 215 } 216 } 217 218 public enum EligibilityResponsePurpose { 219 /** 220 * The prior authorization requirements for the listed, or discovered if 221 * specified, converages for the categories of service and/or specifed biling 222 * codes are requested. 223 */ 224 AUTHREQUIREMENTS, 225 /** 226 * The plan benefits and optionally benefits consumed for the listed, or 227 * discovered if specified, converages are requested. 228 */ 229 BENEFITS, 230 /** 231 * The insurer is requested to report on any coverages which they are aware of 232 * in addition to any specifed. 233 */ 234 DISCOVERY, 235 /** 236 * A check that the specified coverages are in-force is requested. 237 */ 238 VALIDATION, 239 /** 240 * added to help the parsers with the generic types 241 */ 242 NULL; 243 244 public static EligibilityResponsePurpose fromCode(String codeString) throws FHIRException { 245 if (codeString == null || "".equals(codeString)) 246 return null; 247 if ("auth-requirements".equals(codeString)) 248 return AUTHREQUIREMENTS; 249 if ("benefits".equals(codeString)) 250 return BENEFITS; 251 if ("discovery".equals(codeString)) 252 return DISCOVERY; 253 if ("validation".equals(codeString)) 254 return VALIDATION; 255 if (Configuration.isAcceptInvalidEnums()) 256 return null; 257 else 258 throw new FHIRException("Unknown EligibilityResponsePurpose code '" + codeString + "'"); 259 } 260 261 public String toCode() { 262 switch (this) { 263 case AUTHREQUIREMENTS: 264 return "auth-requirements"; 265 case BENEFITS: 266 return "benefits"; 267 case DISCOVERY: 268 return "discovery"; 269 case VALIDATION: 270 return "validation"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 278 public String getSystem() { 279 switch (this) { 280 case AUTHREQUIREMENTS: 281 return "http://hl7.org/fhir/eligibilityresponse-purpose"; 282 case BENEFITS: 283 return "http://hl7.org/fhir/eligibilityresponse-purpose"; 284 case DISCOVERY: 285 return "http://hl7.org/fhir/eligibilityresponse-purpose"; 286 case VALIDATION: 287 return "http://hl7.org/fhir/eligibilityresponse-purpose"; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 295 public String getDefinition() { 296 switch (this) { 297 case AUTHREQUIREMENTS: 298 return "The prior authorization requirements for the listed, or discovered if specified, converages for the categories of service and/or specifed biling codes are requested."; 299 case BENEFITS: 300 return "The plan benefits and optionally benefits consumed for the listed, or discovered if specified, converages are requested."; 301 case DISCOVERY: 302 return "The insurer is requested to report on any coverages which they are aware of in addition to any specifed."; 303 case VALIDATION: 304 return "A check that the specified coverages are in-force is requested."; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 312 public String getDisplay() { 313 switch (this) { 314 case AUTHREQUIREMENTS: 315 return "Coverage auth-requirements"; 316 case BENEFITS: 317 return "Coverage benefits"; 318 case DISCOVERY: 319 return "Coverage Discovery"; 320 case VALIDATION: 321 return "Coverage Validation"; 322 case NULL: 323 return null; 324 default: 325 return "?"; 326 } 327 } 328 } 329 330 public static class EligibilityResponsePurposeEnumFactory implements EnumFactory<EligibilityResponsePurpose> { 331 public EligibilityResponsePurpose fromCode(String codeString) throws IllegalArgumentException { 332 if (codeString == null || "".equals(codeString)) 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("auth-requirements".equals(codeString)) 336 return EligibilityResponsePurpose.AUTHREQUIREMENTS; 337 if ("benefits".equals(codeString)) 338 return EligibilityResponsePurpose.BENEFITS; 339 if ("discovery".equals(codeString)) 340 return EligibilityResponsePurpose.DISCOVERY; 341 if ("validation".equals(codeString)) 342 return EligibilityResponsePurpose.VALIDATION; 343 throw new IllegalArgumentException("Unknown EligibilityResponsePurpose code '" + codeString + "'"); 344 } 345 346 public Enumeration<EligibilityResponsePurpose> fromType(PrimitiveType<?> code) throws FHIRException { 347 if (code == null) 348 return null; 349 if (code.isEmpty()) 350 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.NULL, code); 351 String codeString = code.asStringValue(); 352 if (codeString == null || "".equals(codeString)) 353 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.NULL, code); 354 if ("auth-requirements".equals(codeString)) 355 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.AUTHREQUIREMENTS, code); 356 if ("benefits".equals(codeString)) 357 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.BENEFITS, code); 358 if ("discovery".equals(codeString)) 359 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.DISCOVERY, code); 360 if ("validation".equals(codeString)) 361 return new Enumeration<EligibilityResponsePurpose>(this, EligibilityResponsePurpose.VALIDATION, code); 362 throw new FHIRException("Unknown EligibilityResponsePurpose code '" + codeString + "'"); 363 } 364 365 public String toCode(EligibilityResponsePurpose code) { 366 if (code == EligibilityResponsePurpose.NULL) 367 return null; 368 if (code == EligibilityResponsePurpose.AUTHREQUIREMENTS) 369 return "auth-requirements"; 370 if (code == EligibilityResponsePurpose.BENEFITS) 371 return "benefits"; 372 if (code == EligibilityResponsePurpose.DISCOVERY) 373 return "discovery"; 374 if (code == EligibilityResponsePurpose.VALIDATION) 375 return "validation"; 376 return "?"; 377 } 378 379 public String toSystem(EligibilityResponsePurpose code) { 380 return code.getSystem(); 381 } 382 } 383 384 @Block() 385 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 386 /** 387 * Reference to the insurance card level information contained in the Coverage 388 * resource. The coverage issuing insurer will use these details to locate the 389 * patient's actual coverage within the insurer's information system. 390 */ 391 @Child(name = "coverage", type = { Coverage.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 392 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.") 393 protected Reference coverage; 394 395 /** 396 * The actual object that is the target of the reference (Reference to the 397 * insurance card level information contained in the Coverage resource. The 398 * coverage issuing insurer will use these details to locate the patient's 399 * actual coverage within the insurer's information system.) 400 */ 401 protected Coverage coverageTarget; 402 403 /** 404 * Flag indicating if the coverage provided is inforce currently if no service 405 * date(s) specified or for the whole duration of the service dates. 406 */ 407 @Child(name = "inforce", type = { 408 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 409 @Description(shortDefinition = "Coverage inforce indicator", formalDefinition = "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.") 410 protected BooleanType inforce; 411 412 /** 413 * The term of the benefits documented in this response. 414 */ 415 @Child(name = "benefitPeriod", type = { 416 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 417 @Description(shortDefinition = "When the benefits are applicable", formalDefinition = "The term of the benefits documented in this response.") 418 protected Period benefitPeriod; 419 420 /** 421 * Benefits and optionally current balances, and authorization details by 422 * category or service. 423 */ 424 @Child(name = "item", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 425 @Description(shortDefinition = "Benefits and authorization details", formalDefinition = "Benefits and optionally current balances, and authorization details by category or service.") 426 protected List<ItemsComponent> item; 427 428 private static final long serialVersionUID = -567336701L; 429 430 /** 431 * Constructor 432 */ 433 public InsuranceComponent() { 434 super(); 435 } 436 437 /** 438 * Constructor 439 */ 440 public InsuranceComponent(Reference coverage) { 441 super(); 442 this.coverage = coverage; 443 } 444 445 /** 446 * @return {@link #coverage} (Reference to the insurance card level information 447 * contained in the Coverage resource. The coverage issuing insurer will 448 * use these details to locate the patient's actual coverage within the 449 * insurer's information system.) 450 */ 451 public Reference getCoverage() { 452 if (this.coverage == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 455 else if (Configuration.doAutoCreate()) 456 this.coverage = new Reference(); // cc 457 return this.coverage; 458 } 459 460 public boolean hasCoverage() { 461 return this.coverage != null && !this.coverage.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #coverage} (Reference to the insurance card level 466 * information contained in the Coverage resource. The coverage 467 * issuing insurer will use these details to locate the patient's 468 * actual coverage within the insurer's information system.) 469 */ 470 public InsuranceComponent setCoverage(Reference value) { 471 this.coverage = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #coverage} The actual object that is the target of the 477 * reference. The reference library doesn't populate this, but you can 478 * use it to hold the resource if you resolve it. (Reference to the 479 * insurance card level information contained in the Coverage resource. 480 * The coverage issuing insurer will use these details to locate the 481 * patient's actual coverage within the insurer's information system.) 482 */ 483 public Coverage getCoverageTarget() { 484 if (this.coverageTarget == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 487 else if (Configuration.doAutoCreate()) 488 this.coverageTarget = new Coverage(); // aa 489 return this.coverageTarget; 490 } 491 492 /** 493 * @param value {@link #coverage} The actual object that is the target of the 494 * reference. The reference library doesn't use these, but you can 495 * use it to hold the resource if you resolve it. (Reference to the 496 * insurance card level information contained in the Coverage 497 * resource. The coverage issuing insurer will use these details to 498 * locate the patient's actual coverage within the insurer's 499 * information system.) 500 */ 501 public InsuranceComponent setCoverageTarget(Coverage value) { 502 this.coverageTarget = value; 503 return this; 504 } 505 506 /** 507 * @return {@link #inforce} (Flag indicating if the coverage provided is inforce 508 * currently if no service date(s) specified or for the whole duration 509 * of the service dates.). This is the underlying object with id, value 510 * and extensions. The accessor "getInforce" gives direct access to the 511 * value 512 */ 513 public BooleanType getInforceElement() { 514 if (this.inforce == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create InsuranceComponent.inforce"); 517 else if (Configuration.doAutoCreate()) 518 this.inforce = new BooleanType(); // bb 519 return this.inforce; 520 } 521 522 public boolean hasInforceElement() { 523 return this.inforce != null && !this.inforce.isEmpty(); 524 } 525 526 public boolean hasInforce() { 527 return this.inforce != null && !this.inforce.isEmpty(); 528 } 529 530 /** 531 * @param value {@link #inforce} (Flag indicating if the coverage provided is 532 * inforce currently if no service date(s) specified or for the 533 * whole duration of the service dates.). This is the underlying 534 * object with id, value and extensions. The accessor "getInforce" 535 * gives direct access to the value 536 */ 537 public InsuranceComponent setInforceElement(BooleanType value) { 538 this.inforce = value; 539 return this; 540 } 541 542 /** 543 * @return Flag indicating if the coverage provided is inforce currently if no 544 * service date(s) specified or for the whole duration of the service 545 * dates. 546 */ 547 public boolean getInforce() { 548 return this.inforce == null || this.inforce.isEmpty() ? false : this.inforce.getValue(); 549 } 550 551 /** 552 * @param value Flag indicating if the coverage provided is inforce currently if 553 * no service date(s) specified or for the whole duration of the 554 * service dates. 555 */ 556 public InsuranceComponent setInforce(boolean value) { 557 if (this.inforce == null) 558 this.inforce = new BooleanType(); 559 this.inforce.setValue(value); 560 return this; 561 } 562 563 /** 564 * @return {@link #benefitPeriod} (The term of the benefits documented in this 565 * response.) 566 */ 567 public Period getBenefitPeriod() { 568 if (this.benefitPeriod == null) 569 if (Configuration.errorOnAutoCreate()) 570 throw new Error("Attempt to auto-create InsuranceComponent.benefitPeriod"); 571 else if (Configuration.doAutoCreate()) 572 this.benefitPeriod = new Period(); // cc 573 return this.benefitPeriod; 574 } 575 576 public boolean hasBenefitPeriod() { 577 return this.benefitPeriod != null && !this.benefitPeriod.isEmpty(); 578 } 579 580 /** 581 * @param value {@link #benefitPeriod} (The term of the benefits documented in 582 * this response.) 583 */ 584 public InsuranceComponent setBenefitPeriod(Period value) { 585 this.benefitPeriod = value; 586 return this; 587 } 588 589 /** 590 * @return {@link #item} (Benefits and optionally current balances, and 591 * authorization details by category or service.) 592 */ 593 public List<ItemsComponent> getItem() { 594 if (this.item == null) 595 this.item = new ArrayList<ItemsComponent>(); 596 return this.item; 597 } 598 599 /** 600 * @return Returns a reference to <code>this</code> for easy method chaining 601 */ 602 public InsuranceComponent setItem(List<ItemsComponent> theItem) { 603 this.item = theItem; 604 return this; 605 } 606 607 public boolean hasItem() { 608 if (this.item == null) 609 return false; 610 for (ItemsComponent item : this.item) 611 if (!item.isEmpty()) 612 return true; 613 return false; 614 } 615 616 public ItemsComponent addItem() { // 3 617 ItemsComponent t = new ItemsComponent(); 618 if (this.item == null) 619 this.item = new ArrayList<ItemsComponent>(); 620 this.item.add(t); 621 return t; 622 } 623 624 public InsuranceComponent addItem(ItemsComponent t) { // 3 625 if (t == null) 626 return this; 627 if (this.item == null) 628 this.item = new ArrayList<ItemsComponent>(); 629 this.item.add(t); 630 return this; 631 } 632 633 /** 634 * @return The first repetition of repeating field {@link #item}, creating it if 635 * it does not already exist 636 */ 637 public ItemsComponent getItemFirstRep() { 638 if (getItem().isEmpty()) { 639 addItem(); 640 } 641 return getItem().get(0); 642 } 643 644 protected void listChildren(List<Property> children) { 645 super.listChildren(children); 646 children.add(new Property("coverage", "Reference(Coverage)", 647 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 648 0, 1, coverage)); 649 children.add(new Property("inforce", "boolean", 650 "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 651 0, 1, inforce)); 652 children.add(new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 653 1, benefitPeriod)); 654 children.add(new Property("item", "", 655 "Benefits and optionally current balances, and authorization details by category or service.", 0, 656 java.lang.Integer.MAX_VALUE, item)); 657 } 658 659 @Override 660 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 661 switch (_hash) { 662 case -351767064: 663 /* coverage */ return new Property("coverage", "Reference(Coverage)", 664 "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 665 0, 1, coverage); 666 case 1945431270: 667 /* inforce */ return new Property("inforce", "boolean", 668 "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 669 0, 1, inforce); 670 case -407369416: 671 /* benefitPeriod */ return new Property("benefitPeriod", "Period", 672 "The term of the benefits documented in this response.", 0, 1, benefitPeriod); 673 case 3242771: 674 /* item */ return new Property("item", "", 675 "Benefits and optionally current balances, and authorization details by category or service.", 0, 676 java.lang.Integer.MAX_VALUE, item); 677 default: 678 return super.getNamedProperty(_hash, _name, _checkValid); 679 } 680 681 } 682 683 @Override 684 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 685 switch (hash) { 686 case -351767064: 687 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 688 case 1945431270: 689 /* inforce */ return this.inforce == null ? new Base[0] : new Base[] { this.inforce }; // BooleanType 690 case -407369416: 691 /* benefitPeriod */ return this.benefitPeriod == null ? new Base[0] : new Base[] { this.benefitPeriod }; // Period 692 case 3242771: 693 /* item */ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemsComponent 694 default: 695 return super.getProperty(hash, name, checkValid); 696 } 697 698 } 699 700 @Override 701 public Base setProperty(int hash, String name, Base value) throws FHIRException { 702 switch (hash) { 703 case -351767064: // coverage 704 this.coverage = castToReference(value); // Reference 705 return value; 706 case 1945431270: // inforce 707 this.inforce = castToBoolean(value); // BooleanType 708 return value; 709 case -407369416: // benefitPeriod 710 this.benefitPeriod = castToPeriod(value); // Period 711 return value; 712 case 3242771: // item 713 this.getItem().add((ItemsComponent) value); // ItemsComponent 714 return value; 715 default: 716 return super.setProperty(hash, name, value); 717 } 718 719 } 720 721 @Override 722 public Base setProperty(String name, Base value) throws FHIRException { 723 if (name.equals("coverage")) { 724 this.coverage = castToReference(value); // Reference 725 } else if (name.equals("inforce")) { 726 this.inforce = castToBoolean(value); // BooleanType 727 } else if (name.equals("benefitPeriod")) { 728 this.benefitPeriod = castToPeriod(value); // Period 729 } else if (name.equals("item")) { 730 this.getItem().add((ItemsComponent) value); 731 } else 732 return super.setProperty(name, value); 733 return value; 734 } 735 736 @Override 737 public void removeChild(String name, Base value) throws FHIRException { 738 if (name.equals("coverage")) { 739 this.coverage = null; 740 } else if (name.equals("inforce")) { 741 this.inforce = null; 742 } else if (name.equals("benefitPeriod")) { 743 this.benefitPeriod = null; 744 } else if (name.equals("item")) { 745 this.getItem().remove((ItemsComponent) value); 746 } else 747 super.removeChild(name, value); 748 749 } 750 751 @Override 752 public Base makeProperty(int hash, String name) throws FHIRException { 753 switch (hash) { 754 case -351767064: 755 return getCoverage(); 756 case 1945431270: 757 return getInforceElement(); 758 case -407369416: 759 return getBenefitPeriod(); 760 case 3242771: 761 return addItem(); 762 default: 763 return super.makeProperty(hash, name); 764 } 765 766 } 767 768 @Override 769 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 770 switch (hash) { 771 case -351767064: 772 /* coverage */ return new String[] { "Reference" }; 773 case 1945431270: 774 /* inforce */ return new String[] { "boolean" }; 775 case -407369416: 776 /* benefitPeriod */ return new String[] { "Period" }; 777 case 3242771: 778 /* item */ return new String[] {}; 779 default: 780 return super.getTypesForProperty(hash, name); 781 } 782 783 } 784 785 @Override 786 public Base addChild(String name) throws FHIRException { 787 if (name.equals("coverage")) { 788 this.coverage = new Reference(); 789 return this.coverage; 790 } else if (name.equals("inforce")) { 791 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.inforce"); 792 } else if (name.equals("benefitPeriod")) { 793 this.benefitPeriod = new Period(); 794 return this.benefitPeriod; 795 } else if (name.equals("item")) { 796 return addItem(); 797 } else 798 return super.addChild(name); 799 } 800 801 public InsuranceComponent copy() { 802 InsuranceComponent dst = new InsuranceComponent(); 803 copyValues(dst); 804 return dst; 805 } 806 807 public void copyValues(InsuranceComponent dst) { 808 super.copyValues(dst); 809 dst.coverage = coverage == null ? null : coverage.copy(); 810 dst.inforce = inforce == null ? null : inforce.copy(); 811 dst.benefitPeriod = benefitPeriod == null ? null : benefitPeriod.copy(); 812 if (item != null) { 813 dst.item = new ArrayList<ItemsComponent>(); 814 for (ItemsComponent i : item) 815 dst.item.add(i.copy()); 816 } 817 ; 818 } 819 820 @Override 821 public boolean equalsDeep(Base other_) { 822 if (!super.equalsDeep(other_)) 823 return false; 824 if (!(other_ instanceof InsuranceComponent)) 825 return false; 826 InsuranceComponent o = (InsuranceComponent) other_; 827 return compareDeep(coverage, o.coverage, true) && compareDeep(inforce, o.inforce, true) 828 && compareDeep(benefitPeriod, o.benefitPeriod, true) && compareDeep(item, o.item, true); 829 } 830 831 @Override 832 public boolean equalsShallow(Base other_) { 833 if (!super.equalsShallow(other_)) 834 return false; 835 if (!(other_ instanceof InsuranceComponent)) 836 return false; 837 InsuranceComponent o = (InsuranceComponent) other_; 838 return compareValues(inforce, o.inforce, true); 839 } 840 841 public boolean isEmpty() { 842 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, inforce, benefitPeriod, item); 843 } 844 845 public String fhirType() { 846 return "CoverageEligibilityResponse.insurance"; 847 848 } 849 850 } 851 852 @Block() 853 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 854 /** 855 * Code to identify the general type of benefits under which products and 856 * services are provided. 857 */ 858 @Child(name = "category", type = { 859 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 860 @Description(shortDefinition = "Benefit classification", formalDefinition = "Code to identify the general type of benefits under which products and services are provided.") 861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/ex-benefitcategory") 862 protected CodeableConcept category; 863 864 /** 865 * This contains the product, service, drug or other billing code for the item. 866 */ 867 @Child(name = "productOrService", type = { 868 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 869 @Description(shortDefinition = "Billing, service, product, or drug code", formalDefinition = "This contains the product, service, drug or other billing code for the item.") 870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-uscls") 871 protected CodeableConcept productOrService; 872 873 /** 874 * Item typification or modifiers codes to convey additional context for the 875 * product or service. 876 */ 877 @Child(name = "modifier", type = { 878 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 879 @Description(shortDefinition = "Product or service billing modifiers", formalDefinition = "Item typification or modifiers codes to convey additional context for the product or service.") 880 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/claim-modifiers") 881 protected List<CodeableConcept> modifier; 882 883 /** 884 * The practitioner who is eligible for the provision of the product or service. 885 */ 886 @Child(name = "provider", type = { Practitioner.class, 887 PractitionerRole.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 888 @Description(shortDefinition = "Performing practitioner", formalDefinition = "The practitioner who is eligible for the provision of the product or service.") 889 protected Reference provider; 890 891 /** 892 * The actual object that is the target of the reference (The practitioner who 893 * is eligible for the provision of the product or service.) 894 */ 895 protected Resource providerTarget; 896 897 /** 898 * True if the indicated class of service is excluded from the plan, missing or 899 * False indicates the product or service is included in the coverage. 900 */ 901 @Child(name = "excluded", type = { 902 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 903 @Description(shortDefinition = "Excluded from the plan", formalDefinition = "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.") 904 protected BooleanType excluded; 905 906 /** 907 * A short name or tag for the benefit. 908 */ 909 @Child(name = "name", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 910 @Description(shortDefinition = "Short name for the benefit", formalDefinition = "A short name or tag for the benefit.") 911 protected StringType name; 912 913 /** 914 * A richer description of the benefit or services covered. 915 */ 916 @Child(name = "description", type = { 917 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 918 @Description(shortDefinition = "Description of the benefit or services covered", formalDefinition = "A richer description of the benefit or services covered.") 919 protected StringType description; 920 921 /** 922 * Is a flag to indicate whether the benefits refer to in-network providers or 923 * out-of-network providers. 924 */ 925 @Child(name = "network", type = { 926 CodeableConcept.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 927 @Description(shortDefinition = "In or out of network", formalDefinition = "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.") 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-network") 929 protected CodeableConcept network; 930 931 /** 932 * Indicates if the benefits apply to an individual or to the family. 933 */ 934 @Child(name = "unit", type = { 935 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 936 @Description(shortDefinition = "Individual or family", formalDefinition = "Indicates if the benefits apply to an individual or to the family.") 937 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-unit") 938 protected CodeableConcept unit; 939 940 /** 941 * The term or period of the values such as 'maximum lifetime benefit' or 942 * 'maximum annual visits'. 943 */ 944 @Child(name = "term", type = { 945 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 946 @Description(shortDefinition = "Annual or lifetime", formalDefinition = "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.") 947 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-term") 948 protected CodeableConcept term; 949 950 /** 951 * Benefits used to date. 952 */ 953 @Child(name = "benefit", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 954 @Description(shortDefinition = "Benefit Summary", formalDefinition = "Benefits used to date.") 955 protected List<BenefitComponent> benefit; 956 957 /** 958 * A boolean flag indicating whether a preauthorization is required prior to 959 * actual service delivery. 960 */ 961 @Child(name = "authorizationRequired", type = { 962 BooleanType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 963 @Description(shortDefinition = "Authorization required flag", formalDefinition = "A boolean flag indicating whether a preauthorization is required prior to actual service delivery.") 964 protected BooleanType authorizationRequired; 965 966 /** 967 * Codes or comments regarding information or actions associated with the 968 * preauthorization. 969 */ 970 @Child(name = "authorizationSupporting", type = { 971 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 972 @Description(shortDefinition = "Type of required supporting materials", formalDefinition = "Codes or comments regarding information or actions associated with the preauthorization.") 973 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/coverageeligibilityresponse-ex-auth-support") 974 protected List<CodeableConcept> authorizationSupporting; 975 976 /** 977 * A web location for obtaining requirements or descriptive information 978 * regarding the preauthorization. 979 */ 980 @Child(name = "authorizationUrl", type = { 981 UriType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 982 @Description(shortDefinition = "Preauthorization requirements endpoint", formalDefinition = "A web location for obtaining requirements or descriptive information regarding the preauthorization.") 983 protected UriType authorizationUrl; 984 985 private static final long serialVersionUID = 1779114111L; 986 987 /** 988 * Constructor 989 */ 990 public ItemsComponent() { 991 super(); 992 } 993 994 /** 995 * @return {@link #category} (Code to identify the general type of benefits 996 * under which products and services are provided.) 997 */ 998 public CodeableConcept getCategory() { 999 if (this.category == null) 1000 if (Configuration.errorOnAutoCreate()) 1001 throw new Error("Attempt to auto-create ItemsComponent.category"); 1002 else if (Configuration.doAutoCreate()) 1003 this.category = new CodeableConcept(); // cc 1004 return this.category; 1005 } 1006 1007 public boolean hasCategory() { 1008 return this.category != null && !this.category.isEmpty(); 1009 } 1010 1011 /** 1012 * @param value {@link #category} (Code to identify the general type of benefits 1013 * under which products and services are provided.) 1014 */ 1015 public ItemsComponent setCategory(CodeableConcept value) { 1016 this.category = value; 1017 return this; 1018 } 1019 1020 /** 1021 * @return {@link #productOrService} (This contains the product, service, drug 1022 * or other billing code for the item.) 1023 */ 1024 public CodeableConcept getProductOrService() { 1025 if (this.productOrService == null) 1026 if (Configuration.errorOnAutoCreate()) 1027 throw new Error("Attempt to auto-create ItemsComponent.productOrService"); 1028 else if (Configuration.doAutoCreate()) 1029 this.productOrService = new CodeableConcept(); // cc 1030 return this.productOrService; 1031 } 1032 1033 public boolean hasProductOrService() { 1034 return this.productOrService != null && !this.productOrService.isEmpty(); 1035 } 1036 1037 /** 1038 * @param value {@link #productOrService} (This contains the product, service, 1039 * drug or other billing code for the item.) 1040 */ 1041 public ItemsComponent setProductOrService(CodeableConcept value) { 1042 this.productOrService = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #modifier} (Item typification or modifiers codes to convey 1048 * additional context for the product or service.) 1049 */ 1050 public List<CodeableConcept> getModifier() { 1051 if (this.modifier == null) 1052 this.modifier = new ArrayList<CodeableConcept>(); 1053 return this.modifier; 1054 } 1055 1056 /** 1057 * @return Returns a reference to <code>this</code> for easy method chaining 1058 */ 1059 public ItemsComponent setModifier(List<CodeableConcept> theModifier) { 1060 this.modifier = theModifier; 1061 return this; 1062 } 1063 1064 public boolean hasModifier() { 1065 if (this.modifier == null) 1066 return false; 1067 for (CodeableConcept item : this.modifier) 1068 if (!item.isEmpty()) 1069 return true; 1070 return false; 1071 } 1072 1073 public CodeableConcept addModifier() { // 3 1074 CodeableConcept t = new CodeableConcept(); 1075 if (this.modifier == null) 1076 this.modifier = new ArrayList<CodeableConcept>(); 1077 this.modifier.add(t); 1078 return t; 1079 } 1080 1081 public ItemsComponent addModifier(CodeableConcept t) { // 3 1082 if (t == null) 1083 return this; 1084 if (this.modifier == null) 1085 this.modifier = new ArrayList<CodeableConcept>(); 1086 this.modifier.add(t); 1087 return this; 1088 } 1089 1090 /** 1091 * @return The first repetition of repeating field {@link #modifier}, creating 1092 * it if it does not already exist 1093 */ 1094 public CodeableConcept getModifierFirstRep() { 1095 if (getModifier().isEmpty()) { 1096 addModifier(); 1097 } 1098 return getModifier().get(0); 1099 } 1100 1101 /** 1102 * @return {@link #provider} (The practitioner who is eligible for the provision 1103 * of the product or service.) 1104 */ 1105 public Reference getProvider() { 1106 if (this.provider == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create ItemsComponent.provider"); 1109 else if (Configuration.doAutoCreate()) 1110 this.provider = new Reference(); // cc 1111 return this.provider; 1112 } 1113 1114 public boolean hasProvider() { 1115 return this.provider != null && !this.provider.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #provider} (The practitioner who is eligible for the 1120 * provision of the product or service.) 1121 */ 1122 public ItemsComponent setProvider(Reference value) { 1123 this.provider = value; 1124 return this; 1125 } 1126 1127 /** 1128 * @return {@link #provider} The actual object that is the target of the 1129 * reference. The reference library doesn't populate this, but you can 1130 * use it to hold the resource if you resolve it. (The practitioner who 1131 * is eligible for the provision of the product or service.) 1132 */ 1133 public Resource getProviderTarget() { 1134 return this.providerTarget; 1135 } 1136 1137 /** 1138 * @param value {@link #provider} The actual object that is the target of the 1139 * reference. The reference library doesn't use these, but you can 1140 * use it to hold the resource if you resolve it. (The practitioner 1141 * who is eligible for the provision of the product or service.) 1142 */ 1143 public ItemsComponent setProviderTarget(Resource value) { 1144 this.providerTarget = value; 1145 return this; 1146 } 1147 1148 /** 1149 * @return {@link #excluded} (True if the indicated class of service is excluded 1150 * from the plan, missing or False indicates the product or service is 1151 * included in the coverage.). This is the underlying object with id, 1152 * value and extensions. The accessor "getExcluded" gives direct access 1153 * to the value 1154 */ 1155 public BooleanType getExcludedElement() { 1156 if (this.excluded == null) 1157 if (Configuration.errorOnAutoCreate()) 1158 throw new Error("Attempt to auto-create ItemsComponent.excluded"); 1159 else if (Configuration.doAutoCreate()) 1160 this.excluded = new BooleanType(); // bb 1161 return this.excluded; 1162 } 1163 1164 public boolean hasExcludedElement() { 1165 return this.excluded != null && !this.excluded.isEmpty(); 1166 } 1167 1168 public boolean hasExcluded() { 1169 return this.excluded != null && !this.excluded.isEmpty(); 1170 } 1171 1172 /** 1173 * @param value {@link #excluded} (True if the indicated class of service is 1174 * excluded from the plan, missing or False indicates the product 1175 * or service is included in the coverage.). This is the underlying 1176 * object with id, value and extensions. The accessor "getExcluded" 1177 * gives direct access to the value 1178 */ 1179 public ItemsComponent setExcludedElement(BooleanType value) { 1180 this.excluded = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return True if the indicated class of service is excluded from the plan, 1186 * missing or False indicates the product or service is included in the 1187 * coverage. 1188 */ 1189 public boolean getExcluded() { 1190 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 1191 } 1192 1193 /** 1194 * @param value True if the indicated class of service is excluded from the 1195 * plan, missing or False indicates the product or service is 1196 * included in the coverage. 1197 */ 1198 public ItemsComponent setExcluded(boolean value) { 1199 if (this.excluded == null) 1200 this.excluded = new BooleanType(); 1201 this.excluded.setValue(value); 1202 return this; 1203 } 1204 1205 /** 1206 * @return {@link #name} (A short name or tag for the benefit.). This is the 1207 * underlying object with id, value and extensions. The accessor 1208 * "getName" gives direct access to the value 1209 */ 1210 public StringType getNameElement() { 1211 if (this.name == null) 1212 if (Configuration.errorOnAutoCreate()) 1213 throw new Error("Attempt to auto-create ItemsComponent.name"); 1214 else if (Configuration.doAutoCreate()) 1215 this.name = new StringType(); // bb 1216 return this.name; 1217 } 1218 1219 public boolean hasNameElement() { 1220 return this.name != null && !this.name.isEmpty(); 1221 } 1222 1223 public boolean hasName() { 1224 return this.name != null && !this.name.isEmpty(); 1225 } 1226 1227 /** 1228 * @param value {@link #name} (A short name or tag for the benefit.). This is 1229 * the underlying object with id, value and extensions. The 1230 * accessor "getName" gives direct access to the value 1231 */ 1232 public ItemsComponent setNameElement(StringType value) { 1233 this.name = value; 1234 return this; 1235 } 1236 1237 /** 1238 * @return A short name or tag for the benefit. 1239 */ 1240 public String getName() { 1241 return this.name == null ? null : this.name.getValue(); 1242 } 1243 1244 /** 1245 * @param value A short name or tag for the benefit. 1246 */ 1247 public ItemsComponent setName(String value) { 1248 if (Utilities.noString(value)) 1249 this.name = null; 1250 else { 1251 if (this.name == null) 1252 this.name = new StringType(); 1253 this.name.setValue(value); 1254 } 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #description} (A richer description of the benefit or services 1260 * covered.). This is the underlying object with id, value and 1261 * extensions. The accessor "getDescription" gives direct access to the 1262 * value 1263 */ 1264 public StringType getDescriptionElement() { 1265 if (this.description == null) 1266 if (Configuration.errorOnAutoCreate()) 1267 throw new Error("Attempt to auto-create ItemsComponent.description"); 1268 else if (Configuration.doAutoCreate()) 1269 this.description = new StringType(); // bb 1270 return this.description; 1271 } 1272 1273 public boolean hasDescriptionElement() { 1274 return this.description != null && !this.description.isEmpty(); 1275 } 1276 1277 public boolean hasDescription() { 1278 return this.description != null && !this.description.isEmpty(); 1279 } 1280 1281 /** 1282 * @param value {@link #description} (A richer description of the benefit or 1283 * services covered.). This is the underlying object with id, value 1284 * and extensions. The accessor "getDescription" gives direct 1285 * access to the value 1286 */ 1287 public ItemsComponent setDescriptionElement(StringType value) { 1288 this.description = value; 1289 return this; 1290 } 1291 1292 /** 1293 * @return A richer description of the benefit or services covered. 1294 */ 1295 public String getDescription() { 1296 return this.description == null ? null : this.description.getValue(); 1297 } 1298 1299 /** 1300 * @param value A richer description of the benefit or services covered. 1301 */ 1302 public ItemsComponent setDescription(String value) { 1303 if (Utilities.noString(value)) 1304 this.description = null; 1305 else { 1306 if (this.description == null) 1307 this.description = new StringType(); 1308 this.description.setValue(value); 1309 } 1310 return this; 1311 } 1312 1313 /** 1314 * @return {@link #network} (Is a flag to indicate whether the benefits refer to 1315 * in-network providers or out-of-network providers.) 1316 */ 1317 public CodeableConcept getNetwork() { 1318 if (this.network == null) 1319 if (Configuration.errorOnAutoCreate()) 1320 throw new Error("Attempt to auto-create ItemsComponent.network"); 1321 else if (Configuration.doAutoCreate()) 1322 this.network = new CodeableConcept(); // cc 1323 return this.network; 1324 } 1325 1326 public boolean hasNetwork() { 1327 return this.network != null && !this.network.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #network} (Is a flag to indicate whether the benefits 1332 * refer to in-network providers or out-of-network providers.) 1333 */ 1334 public ItemsComponent setNetwork(CodeableConcept value) { 1335 this.network = value; 1336 return this; 1337 } 1338 1339 /** 1340 * @return {@link #unit} (Indicates if the benefits apply to an individual or to 1341 * the family.) 1342 */ 1343 public CodeableConcept getUnit() { 1344 if (this.unit == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create ItemsComponent.unit"); 1347 else if (Configuration.doAutoCreate()) 1348 this.unit = new CodeableConcept(); // cc 1349 return this.unit; 1350 } 1351 1352 public boolean hasUnit() { 1353 return this.unit != null && !this.unit.isEmpty(); 1354 } 1355 1356 /** 1357 * @param value {@link #unit} (Indicates if the benefits apply to an individual 1358 * or to the family.) 1359 */ 1360 public ItemsComponent setUnit(CodeableConcept value) { 1361 this.unit = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #term} (The term or period of the values such as 'maximum 1367 * lifetime benefit' or 'maximum annual visits'.) 1368 */ 1369 public CodeableConcept getTerm() { 1370 if (this.term == null) 1371 if (Configuration.errorOnAutoCreate()) 1372 throw new Error("Attempt to auto-create ItemsComponent.term"); 1373 else if (Configuration.doAutoCreate()) 1374 this.term = new CodeableConcept(); // cc 1375 return this.term; 1376 } 1377 1378 public boolean hasTerm() { 1379 return this.term != null && !this.term.isEmpty(); 1380 } 1381 1382 /** 1383 * @param value {@link #term} (The term or period of the values such as 'maximum 1384 * lifetime benefit' or 'maximum annual visits'.) 1385 */ 1386 public ItemsComponent setTerm(CodeableConcept value) { 1387 this.term = value; 1388 return this; 1389 } 1390 1391 /** 1392 * @return {@link #benefit} (Benefits used to date.) 1393 */ 1394 public List<BenefitComponent> getBenefit() { 1395 if (this.benefit == null) 1396 this.benefit = new ArrayList<BenefitComponent>(); 1397 return this.benefit; 1398 } 1399 1400 /** 1401 * @return Returns a reference to <code>this</code> for easy method chaining 1402 */ 1403 public ItemsComponent setBenefit(List<BenefitComponent> theBenefit) { 1404 this.benefit = theBenefit; 1405 return this; 1406 } 1407 1408 public boolean hasBenefit() { 1409 if (this.benefit == null) 1410 return false; 1411 for (BenefitComponent item : this.benefit) 1412 if (!item.isEmpty()) 1413 return true; 1414 return false; 1415 } 1416 1417 public BenefitComponent addBenefit() { // 3 1418 BenefitComponent t = new BenefitComponent(); 1419 if (this.benefit == null) 1420 this.benefit = new ArrayList<BenefitComponent>(); 1421 this.benefit.add(t); 1422 return t; 1423 } 1424 1425 public ItemsComponent addBenefit(BenefitComponent t) { // 3 1426 if (t == null) 1427 return this; 1428 if (this.benefit == null) 1429 this.benefit = new ArrayList<BenefitComponent>(); 1430 this.benefit.add(t); 1431 return this; 1432 } 1433 1434 /** 1435 * @return The first repetition of repeating field {@link #benefit}, creating it 1436 * if it does not already exist 1437 */ 1438 public BenefitComponent getBenefitFirstRep() { 1439 if (getBenefit().isEmpty()) { 1440 addBenefit(); 1441 } 1442 return getBenefit().get(0); 1443 } 1444 1445 /** 1446 * @return {@link #authorizationRequired} (A boolean flag indicating whether a 1447 * preauthorization is required prior to actual service delivery.). This 1448 * is the underlying object with id, value and extensions. The accessor 1449 * "getAuthorizationRequired" gives direct access to the value 1450 */ 1451 public BooleanType getAuthorizationRequiredElement() { 1452 if (this.authorizationRequired == null) 1453 if (Configuration.errorOnAutoCreate()) 1454 throw new Error("Attempt to auto-create ItemsComponent.authorizationRequired"); 1455 else if (Configuration.doAutoCreate()) 1456 this.authorizationRequired = new BooleanType(); // bb 1457 return this.authorizationRequired; 1458 } 1459 1460 public boolean hasAuthorizationRequiredElement() { 1461 return this.authorizationRequired != null && !this.authorizationRequired.isEmpty(); 1462 } 1463 1464 public boolean hasAuthorizationRequired() { 1465 return this.authorizationRequired != null && !this.authorizationRequired.isEmpty(); 1466 } 1467 1468 /** 1469 * @param value {@link #authorizationRequired} (A boolean flag indicating 1470 * whether a preauthorization is required prior to actual service 1471 * delivery.). This is the underlying object with id, value and 1472 * extensions. The accessor "getAuthorizationRequired" gives direct 1473 * access to the value 1474 */ 1475 public ItemsComponent setAuthorizationRequiredElement(BooleanType value) { 1476 this.authorizationRequired = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return A boolean flag indicating whether a preauthorization is required 1482 * prior to actual service delivery. 1483 */ 1484 public boolean getAuthorizationRequired() { 1485 return this.authorizationRequired == null || this.authorizationRequired.isEmpty() ? false 1486 : this.authorizationRequired.getValue(); 1487 } 1488 1489 /** 1490 * @param value A boolean flag indicating whether a preauthorization is required 1491 * prior to actual service delivery. 1492 */ 1493 public ItemsComponent setAuthorizationRequired(boolean value) { 1494 if (this.authorizationRequired == null) 1495 this.authorizationRequired = new BooleanType(); 1496 this.authorizationRequired.setValue(value); 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #authorizationSupporting} (Codes or comments regarding 1502 * information or actions associated with the preauthorization.) 1503 */ 1504 public List<CodeableConcept> getAuthorizationSupporting() { 1505 if (this.authorizationSupporting == null) 1506 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1507 return this.authorizationSupporting; 1508 } 1509 1510 /** 1511 * @return Returns a reference to <code>this</code> for easy method chaining 1512 */ 1513 public ItemsComponent setAuthorizationSupporting(List<CodeableConcept> theAuthorizationSupporting) { 1514 this.authorizationSupporting = theAuthorizationSupporting; 1515 return this; 1516 } 1517 1518 public boolean hasAuthorizationSupporting() { 1519 if (this.authorizationSupporting == null) 1520 return false; 1521 for (CodeableConcept item : this.authorizationSupporting) 1522 if (!item.isEmpty()) 1523 return true; 1524 return false; 1525 } 1526 1527 public CodeableConcept addAuthorizationSupporting() { // 3 1528 CodeableConcept t = new CodeableConcept(); 1529 if (this.authorizationSupporting == null) 1530 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1531 this.authorizationSupporting.add(t); 1532 return t; 1533 } 1534 1535 public ItemsComponent addAuthorizationSupporting(CodeableConcept t) { // 3 1536 if (t == null) 1537 return this; 1538 if (this.authorizationSupporting == null) 1539 this.authorizationSupporting = new ArrayList<CodeableConcept>(); 1540 this.authorizationSupporting.add(t); 1541 return this; 1542 } 1543 1544 /** 1545 * @return The first repetition of repeating field 1546 * {@link #authorizationSupporting}, creating it if it does not already 1547 * exist 1548 */ 1549 public CodeableConcept getAuthorizationSupportingFirstRep() { 1550 if (getAuthorizationSupporting().isEmpty()) { 1551 addAuthorizationSupporting(); 1552 } 1553 return getAuthorizationSupporting().get(0); 1554 } 1555 1556 /** 1557 * @return {@link #authorizationUrl} (A web location for obtaining requirements 1558 * or descriptive information regarding the preauthorization.). This is 1559 * the underlying object with id, value and extensions. The accessor 1560 * "getAuthorizationUrl" gives direct access to the value 1561 */ 1562 public UriType getAuthorizationUrlElement() { 1563 if (this.authorizationUrl == null) 1564 if (Configuration.errorOnAutoCreate()) 1565 throw new Error("Attempt to auto-create ItemsComponent.authorizationUrl"); 1566 else if (Configuration.doAutoCreate()) 1567 this.authorizationUrl = new UriType(); // bb 1568 return this.authorizationUrl; 1569 } 1570 1571 public boolean hasAuthorizationUrlElement() { 1572 return this.authorizationUrl != null && !this.authorizationUrl.isEmpty(); 1573 } 1574 1575 public boolean hasAuthorizationUrl() { 1576 return this.authorizationUrl != null && !this.authorizationUrl.isEmpty(); 1577 } 1578 1579 /** 1580 * @param value {@link #authorizationUrl} (A web location for obtaining 1581 * requirements or descriptive information regarding the 1582 * preauthorization.). This is the underlying object with id, value 1583 * and extensions. The accessor "getAuthorizationUrl" gives direct 1584 * access to the value 1585 */ 1586 public ItemsComponent setAuthorizationUrlElement(UriType value) { 1587 this.authorizationUrl = value; 1588 return this; 1589 } 1590 1591 /** 1592 * @return A web location for obtaining requirements or descriptive information 1593 * regarding the preauthorization. 1594 */ 1595 public String getAuthorizationUrl() { 1596 return this.authorizationUrl == null ? null : this.authorizationUrl.getValue(); 1597 } 1598 1599 /** 1600 * @param value A web location for obtaining requirements or descriptive 1601 * information regarding the preauthorization. 1602 */ 1603 public ItemsComponent setAuthorizationUrl(String value) { 1604 if (Utilities.noString(value)) 1605 this.authorizationUrl = null; 1606 else { 1607 if (this.authorizationUrl == null) 1608 this.authorizationUrl = new UriType(); 1609 this.authorizationUrl.setValue(value); 1610 } 1611 return this; 1612 } 1613 1614 protected void listChildren(List<Property> children) { 1615 super.listChildren(children); 1616 children.add(new Property("category", "CodeableConcept", 1617 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 1618 category)); 1619 children.add(new Property("productOrService", "CodeableConcept", 1620 "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService)); 1621 children.add(new Property("modifier", "CodeableConcept", 1622 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 1623 java.lang.Integer.MAX_VALUE, modifier)); 1624 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole)", 1625 "The practitioner who is eligible for the provision of the product or service.", 0, 1, provider)); 1626 children.add(new Property("excluded", "boolean", 1627 "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 1628 0, 1, excluded)); 1629 children.add(new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name)); 1630 children.add(new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1631 1, description)); 1632 children.add(new Property("network", "CodeableConcept", 1633 "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, 1634 network)); 1635 children.add(new Property("unit", "CodeableConcept", 1636 "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 1637 children.add(new Property("term", "CodeableConcept", 1638 "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, 1639 term)); 1640 children.add(new Property("benefit", "", "Benefits used to date.", 0, java.lang.Integer.MAX_VALUE, benefit)); 1641 children.add(new Property("authorizationRequired", "boolean", 1642 "A boolean flag indicating whether a preauthorization is required prior to actual service delivery.", 0, 1, 1643 authorizationRequired)); 1644 children.add(new Property("authorizationSupporting", "CodeableConcept", 1645 "Codes or comments regarding information or actions associated with the preauthorization.", 0, 1646 java.lang.Integer.MAX_VALUE, authorizationSupporting)); 1647 children.add(new Property("authorizationUrl", "uri", 1648 "A web location for obtaining requirements or descriptive information regarding the preauthorization.", 0, 1, 1649 authorizationUrl)); 1650 } 1651 1652 @Override 1653 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1654 switch (_hash) { 1655 case 50511102: 1656 /* category */ return new Property("category", "CodeableConcept", 1657 "Code to identify the general type of benefits under which products and services are provided.", 0, 1, 1658 category); 1659 case 1957227299: 1660 /* productOrService */ return new Property("productOrService", "CodeableConcept", 1661 "This contains the product, service, drug or other billing code for the item.", 0, 1, productOrService); 1662 case -615513385: 1663 /* modifier */ return new Property("modifier", "CodeableConcept", 1664 "Item typification or modifiers codes to convey additional context for the product or service.", 0, 1665 java.lang.Integer.MAX_VALUE, modifier); 1666 case -987494927: 1667 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole)", 1668 "The practitioner who is eligible for the provision of the product or service.", 0, 1, provider); 1669 case 1994055114: 1670 /* excluded */ return new Property("excluded", "boolean", 1671 "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 1672 0, 1, excluded); 1673 case 3373707: 1674 /* name */ return new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name); 1675 case -1724546052: 1676 /* description */ return new Property("description", "string", 1677 "A richer description of the benefit or services covered.", 0, 1, description); 1678 case 1843485230: 1679 /* network */ return new Property("network", "CodeableConcept", 1680 "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1681 1, network); 1682 case 3594628: 1683 /* unit */ return new Property("unit", "CodeableConcept", 1684 "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 1685 case 3556460: 1686 /* term */ return new Property("term", "CodeableConcept", 1687 "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, 1688 term); 1689 case -222710633: 1690 /* benefit */ return new Property("benefit", "", "Benefits used to date.", 0, java.lang.Integer.MAX_VALUE, 1691 benefit); 1692 case 374204216: 1693 /* authorizationRequired */ return new Property("authorizationRequired", "boolean", 1694 "A boolean flag indicating whether a preauthorization is required prior to actual service delivery.", 0, 1, 1695 authorizationRequired); 1696 case -1931146484: 1697 /* authorizationSupporting */ return new Property("authorizationSupporting", "CodeableConcept", 1698 "Codes or comments regarding information or actions associated with the preauthorization.", 0, 1699 java.lang.Integer.MAX_VALUE, authorizationSupporting); 1700 case 1409445430: 1701 /* authorizationUrl */ return new Property("authorizationUrl", "uri", 1702 "A web location for obtaining requirements or descriptive information regarding the preauthorization.", 0, 1703 1, authorizationUrl); 1704 default: 1705 return super.getNamedProperty(_hash, _name, _checkValid); 1706 } 1707 1708 } 1709 1710 @Override 1711 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1712 switch (hash) { 1713 case 50511102: 1714 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 1715 case 1957227299: 1716 /* productOrService */ return this.productOrService == null ? new Base[0] 1717 : new Base[] { this.productOrService }; // CodeableConcept 1718 case -615513385: 1719 /* modifier */ return this.modifier == null ? new Base[0] 1720 : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 1721 case -987494927: 1722 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 1723 case 1994055114: 1724 /* excluded */ return this.excluded == null ? new Base[0] : new Base[] { this.excluded }; // BooleanType 1725 case 3373707: 1726 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1727 case -1724546052: 1728 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1729 case 1843485230: 1730 /* network */ return this.network == null ? new Base[0] : new Base[] { this.network }; // CodeableConcept 1731 case 3594628: 1732 /* unit */ return this.unit == null ? new Base[0] : new Base[] { this.unit }; // CodeableConcept 1733 case 3556460: 1734 /* term */ return this.term == null ? new Base[0] : new Base[] { this.term }; // CodeableConcept 1735 case -222710633: 1736 /* benefit */ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // BenefitComponent 1737 case 374204216: 1738 /* authorizationRequired */ return this.authorizationRequired == null ? new Base[0] 1739 : new Base[] { this.authorizationRequired }; // BooleanType 1740 case -1931146484: 1741 /* authorizationSupporting */ return this.authorizationSupporting == null ? new Base[0] 1742 : this.authorizationSupporting.toArray(new Base[this.authorizationSupporting.size()]); // CodeableConcept 1743 case 1409445430: 1744 /* authorizationUrl */ return this.authorizationUrl == null ? new Base[0] 1745 : new Base[] { this.authorizationUrl }; // UriType 1746 default: 1747 return super.getProperty(hash, name, checkValid); 1748 } 1749 1750 } 1751 1752 @Override 1753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1754 switch (hash) { 1755 case 50511102: // category 1756 this.category = castToCodeableConcept(value); // CodeableConcept 1757 return value; 1758 case 1957227299: // productOrService 1759 this.productOrService = castToCodeableConcept(value); // CodeableConcept 1760 return value; 1761 case -615513385: // modifier 1762 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 1763 return value; 1764 case -987494927: // provider 1765 this.provider = castToReference(value); // Reference 1766 return value; 1767 case 1994055114: // excluded 1768 this.excluded = castToBoolean(value); // BooleanType 1769 return value; 1770 case 3373707: // name 1771 this.name = castToString(value); // StringType 1772 return value; 1773 case -1724546052: // description 1774 this.description = castToString(value); // StringType 1775 return value; 1776 case 1843485230: // network 1777 this.network = castToCodeableConcept(value); // CodeableConcept 1778 return value; 1779 case 3594628: // unit 1780 this.unit = castToCodeableConcept(value); // CodeableConcept 1781 return value; 1782 case 3556460: // term 1783 this.term = castToCodeableConcept(value); // CodeableConcept 1784 return value; 1785 case -222710633: // benefit 1786 this.getBenefit().add((BenefitComponent) value); // BenefitComponent 1787 return value; 1788 case 374204216: // authorizationRequired 1789 this.authorizationRequired = castToBoolean(value); // BooleanType 1790 return value; 1791 case -1931146484: // authorizationSupporting 1792 this.getAuthorizationSupporting().add(castToCodeableConcept(value)); // CodeableConcept 1793 return value; 1794 case 1409445430: // authorizationUrl 1795 this.authorizationUrl = castToUri(value); // UriType 1796 return value; 1797 default: 1798 return super.setProperty(hash, name, value); 1799 } 1800 1801 } 1802 1803 @Override 1804 public Base setProperty(String name, Base value) throws FHIRException { 1805 if (name.equals("category")) { 1806 this.category = castToCodeableConcept(value); // CodeableConcept 1807 } else if (name.equals("productOrService")) { 1808 this.productOrService = castToCodeableConcept(value); // CodeableConcept 1809 } else if (name.equals("modifier")) { 1810 this.getModifier().add(castToCodeableConcept(value)); 1811 } else if (name.equals("provider")) { 1812 this.provider = castToReference(value); // Reference 1813 } else if (name.equals("excluded")) { 1814 this.excluded = castToBoolean(value); // BooleanType 1815 } else if (name.equals("name")) { 1816 this.name = castToString(value); // StringType 1817 } else if (name.equals("description")) { 1818 this.description = castToString(value); // StringType 1819 } else if (name.equals("network")) { 1820 this.network = castToCodeableConcept(value); // CodeableConcept 1821 } else if (name.equals("unit")) { 1822 this.unit = castToCodeableConcept(value); // CodeableConcept 1823 } else if (name.equals("term")) { 1824 this.term = castToCodeableConcept(value); // CodeableConcept 1825 } else if (name.equals("benefit")) { 1826 this.getBenefit().add((BenefitComponent) value); 1827 } else if (name.equals("authorizationRequired")) { 1828 this.authorizationRequired = castToBoolean(value); // BooleanType 1829 } else if (name.equals("authorizationSupporting")) { 1830 this.getAuthorizationSupporting().add(castToCodeableConcept(value)); 1831 } else if (name.equals("authorizationUrl")) { 1832 this.authorizationUrl = castToUri(value); // UriType 1833 } else 1834 return super.setProperty(name, value); 1835 return value; 1836 } 1837 1838 @Override 1839 public void removeChild(String name, Base value) throws FHIRException { 1840 if (name.equals("category")) { 1841 this.category = null; 1842 } else if (name.equals("productOrService")) { 1843 this.productOrService = null; 1844 } else if (name.equals("modifier")) { 1845 this.getModifier().remove(castToCodeableConcept(value)); 1846 } else if (name.equals("provider")) { 1847 this.provider = null; 1848 } else if (name.equals("excluded")) { 1849 this.excluded = null; 1850 } else if (name.equals("name")) { 1851 this.name = null; 1852 } else if (name.equals("description")) { 1853 this.description = null; 1854 } else if (name.equals("network")) { 1855 this.network = null; 1856 } else if (name.equals("unit")) { 1857 this.unit = null; 1858 } else if (name.equals("term")) { 1859 this.term = null; 1860 } else if (name.equals("benefit")) { 1861 this.getBenefit().remove((BenefitComponent) value); 1862 } else if (name.equals("authorizationRequired")) { 1863 this.authorizationRequired = null; 1864 } else if (name.equals("authorizationSupporting")) { 1865 this.getAuthorizationSupporting().remove(castToCodeableConcept(value)); 1866 } else if (name.equals("authorizationUrl")) { 1867 this.authorizationUrl = null; 1868 } else 1869 super.removeChild(name, value); 1870 1871 } 1872 1873 @Override 1874 public Base makeProperty(int hash, String name) throws FHIRException { 1875 switch (hash) { 1876 case 50511102: 1877 return getCategory(); 1878 case 1957227299: 1879 return getProductOrService(); 1880 case -615513385: 1881 return addModifier(); 1882 case -987494927: 1883 return getProvider(); 1884 case 1994055114: 1885 return getExcludedElement(); 1886 case 3373707: 1887 return getNameElement(); 1888 case -1724546052: 1889 return getDescriptionElement(); 1890 case 1843485230: 1891 return getNetwork(); 1892 case 3594628: 1893 return getUnit(); 1894 case 3556460: 1895 return getTerm(); 1896 case -222710633: 1897 return addBenefit(); 1898 case 374204216: 1899 return getAuthorizationRequiredElement(); 1900 case -1931146484: 1901 return addAuthorizationSupporting(); 1902 case 1409445430: 1903 return getAuthorizationUrlElement(); 1904 default: 1905 return super.makeProperty(hash, name); 1906 } 1907 1908 } 1909 1910 @Override 1911 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1912 switch (hash) { 1913 case 50511102: 1914 /* category */ return new String[] { "CodeableConcept" }; 1915 case 1957227299: 1916 /* productOrService */ return new String[] { "CodeableConcept" }; 1917 case -615513385: 1918 /* modifier */ return new String[] { "CodeableConcept" }; 1919 case -987494927: 1920 /* provider */ return new String[] { "Reference" }; 1921 case 1994055114: 1922 /* excluded */ return new String[] { "boolean" }; 1923 case 3373707: 1924 /* name */ return new String[] { "string" }; 1925 case -1724546052: 1926 /* description */ return new String[] { "string" }; 1927 case 1843485230: 1928 /* network */ return new String[] { "CodeableConcept" }; 1929 case 3594628: 1930 /* unit */ return new String[] { "CodeableConcept" }; 1931 case 3556460: 1932 /* term */ return new String[] { "CodeableConcept" }; 1933 case -222710633: 1934 /* benefit */ return new String[] {}; 1935 case 374204216: 1936 /* authorizationRequired */ return new String[] { "boolean" }; 1937 case -1931146484: 1938 /* authorizationSupporting */ return new String[] { "CodeableConcept" }; 1939 case 1409445430: 1940 /* authorizationUrl */ return new String[] { "uri" }; 1941 default: 1942 return super.getTypesForProperty(hash, name); 1943 } 1944 1945 } 1946 1947 @Override 1948 public Base addChild(String name) throws FHIRException { 1949 if (name.equals("category")) { 1950 this.category = new CodeableConcept(); 1951 return this.category; 1952 } else if (name.equals("productOrService")) { 1953 this.productOrService = new CodeableConcept(); 1954 return this.productOrService; 1955 } else if (name.equals("modifier")) { 1956 return addModifier(); 1957 } else if (name.equals("provider")) { 1958 this.provider = new Reference(); 1959 return this.provider; 1960 } else if (name.equals("excluded")) { 1961 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.excluded"); 1962 } else if (name.equals("name")) { 1963 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.name"); 1964 } else if (name.equals("description")) { 1965 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.description"); 1966 } else if (name.equals("network")) { 1967 this.network = new CodeableConcept(); 1968 return this.network; 1969 } else if (name.equals("unit")) { 1970 this.unit = new CodeableConcept(); 1971 return this.unit; 1972 } else if (name.equals("term")) { 1973 this.term = new CodeableConcept(); 1974 return this.term; 1975 } else if (name.equals("benefit")) { 1976 return addBenefit(); 1977 } else if (name.equals("authorizationRequired")) { 1978 throw new FHIRException( 1979 "Cannot call addChild on a singleton property CoverageEligibilityResponse.authorizationRequired"); 1980 } else if (name.equals("authorizationSupporting")) { 1981 return addAuthorizationSupporting(); 1982 } else if (name.equals("authorizationUrl")) { 1983 throw new FHIRException( 1984 "Cannot call addChild on a singleton property CoverageEligibilityResponse.authorizationUrl"); 1985 } else 1986 return super.addChild(name); 1987 } 1988 1989 public ItemsComponent copy() { 1990 ItemsComponent dst = new ItemsComponent(); 1991 copyValues(dst); 1992 return dst; 1993 } 1994 1995 public void copyValues(ItemsComponent dst) { 1996 super.copyValues(dst); 1997 dst.category = category == null ? null : category.copy(); 1998 dst.productOrService = productOrService == null ? null : productOrService.copy(); 1999 if (modifier != null) { 2000 dst.modifier = new ArrayList<CodeableConcept>(); 2001 for (CodeableConcept i : modifier) 2002 dst.modifier.add(i.copy()); 2003 } 2004 ; 2005 dst.provider = provider == null ? null : provider.copy(); 2006 dst.excluded = excluded == null ? null : excluded.copy(); 2007 dst.name = name == null ? null : name.copy(); 2008 dst.description = description == null ? null : description.copy(); 2009 dst.network = network == null ? null : network.copy(); 2010 dst.unit = unit == null ? null : unit.copy(); 2011 dst.term = term == null ? null : term.copy(); 2012 if (benefit != null) { 2013 dst.benefit = new ArrayList<BenefitComponent>(); 2014 for (BenefitComponent i : benefit) 2015 dst.benefit.add(i.copy()); 2016 } 2017 ; 2018 dst.authorizationRequired = authorizationRequired == null ? null : authorizationRequired.copy(); 2019 if (authorizationSupporting != null) { 2020 dst.authorizationSupporting = new ArrayList<CodeableConcept>(); 2021 for (CodeableConcept i : authorizationSupporting) 2022 dst.authorizationSupporting.add(i.copy()); 2023 } 2024 ; 2025 dst.authorizationUrl = authorizationUrl == null ? null : authorizationUrl.copy(); 2026 } 2027 2028 @Override 2029 public boolean equalsDeep(Base other_) { 2030 if (!super.equalsDeep(other_)) 2031 return false; 2032 if (!(other_ instanceof ItemsComponent)) 2033 return false; 2034 ItemsComponent o = (ItemsComponent) other_; 2035 return compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 2036 && compareDeep(modifier, o.modifier, true) && compareDeep(provider, o.provider, true) 2037 && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) 2038 && compareDeep(description, o.description, true) && compareDeep(network, o.network, true) 2039 && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) && compareDeep(benefit, o.benefit, true) 2040 && compareDeep(authorizationRequired, o.authorizationRequired, true) 2041 && compareDeep(authorizationSupporting, o.authorizationSupporting, true) 2042 && compareDeep(authorizationUrl, o.authorizationUrl, true); 2043 } 2044 2045 @Override 2046 public boolean equalsShallow(Base other_) { 2047 if (!super.equalsShallow(other_)) 2048 return false; 2049 if (!(other_ instanceof ItemsComponent)) 2050 return false; 2051 ItemsComponent o = (ItemsComponent) other_; 2052 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) 2053 && compareValues(description, o.description, true) 2054 && compareValues(authorizationRequired, o.authorizationRequired, true) 2055 && compareValues(authorizationUrl, o.authorizationUrl, true); 2056 } 2057 2058 public boolean isEmpty() { 2059 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, productOrService, modifier, provider, 2060 excluded, name, description, network, unit, term, benefit, authorizationRequired, authorizationSupporting, 2061 authorizationUrl); 2062 } 2063 2064 public String fhirType() { 2065 return "CoverageEligibilityResponse.insurance.item"; 2066 2067 } 2068 2069 } 2070 2071 @Block() 2072 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 2073 /** 2074 * Classification of benefit being provided. 2075 */ 2076 @Child(name = "type", type = { 2077 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2078 @Description(shortDefinition = "Benefit classification", formalDefinition = "Classification of benefit being provided.") 2079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/benefit-type") 2080 protected CodeableConcept type; 2081 2082 /** 2083 * The quantity of the benefit which is permitted under the coverage. 2084 */ 2085 @Child(name = "allowed", type = { UnsignedIntType.class, StringType.class, 2086 Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2087 @Description(shortDefinition = "Benefits allowed", formalDefinition = "The quantity of the benefit which is permitted under the coverage.") 2088 protected Type allowed; 2089 2090 /** 2091 * The quantity of the benefit which have been consumed to date. 2092 */ 2093 @Child(name = "used", type = { UnsignedIntType.class, StringType.class, 2094 Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2095 @Description(shortDefinition = "Benefits used", formalDefinition = "The quantity of the benefit which have been consumed to date.") 2096 protected Type used; 2097 2098 private static final long serialVersionUID = -1506285314L; 2099 2100 /** 2101 * Constructor 2102 */ 2103 public BenefitComponent() { 2104 super(); 2105 } 2106 2107 /** 2108 * Constructor 2109 */ 2110 public BenefitComponent(CodeableConcept type) { 2111 super(); 2112 this.type = type; 2113 } 2114 2115 /** 2116 * @return {@link #type} (Classification of benefit being provided.) 2117 */ 2118 public CodeableConcept getType() { 2119 if (this.type == null) 2120 if (Configuration.errorOnAutoCreate()) 2121 throw new Error("Attempt to auto-create BenefitComponent.type"); 2122 else if (Configuration.doAutoCreate()) 2123 this.type = new CodeableConcept(); // cc 2124 return this.type; 2125 } 2126 2127 public boolean hasType() { 2128 return this.type != null && !this.type.isEmpty(); 2129 } 2130 2131 /** 2132 * @param value {@link #type} (Classification of benefit being provided.) 2133 */ 2134 public BenefitComponent setType(CodeableConcept value) { 2135 this.type = value; 2136 return this; 2137 } 2138 2139 /** 2140 * @return {@link #allowed} (The quantity of the benefit which is permitted 2141 * under the coverage.) 2142 */ 2143 public Type getAllowed() { 2144 return this.allowed; 2145 } 2146 2147 /** 2148 * @return {@link #allowed} (The quantity of the benefit which is permitted 2149 * under the coverage.) 2150 */ 2151 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 2152 if (this.allowed == null) 2153 this.allowed = new UnsignedIntType(); 2154 if (!(this.allowed instanceof UnsignedIntType)) 2155 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 2156 + this.allowed.getClass().getName() + " was encountered"); 2157 return (UnsignedIntType) this.allowed; 2158 } 2159 2160 public boolean hasAllowedUnsignedIntType() { 2161 return this != null && this.allowed instanceof UnsignedIntType; 2162 } 2163 2164 /** 2165 * @return {@link #allowed} (The quantity of the benefit which is permitted 2166 * under the coverage.) 2167 */ 2168 public StringType getAllowedStringType() throws FHIRException { 2169 if (this.allowed == null) 2170 this.allowed = new StringType(); 2171 if (!(this.allowed instanceof StringType)) 2172 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2173 + this.allowed.getClass().getName() + " was encountered"); 2174 return (StringType) this.allowed; 2175 } 2176 2177 public boolean hasAllowedStringType() { 2178 return this != null && this.allowed instanceof StringType; 2179 } 2180 2181 /** 2182 * @return {@link #allowed} (The quantity of the benefit which is permitted 2183 * under the coverage.) 2184 */ 2185 public Money getAllowedMoney() throws FHIRException { 2186 if (this.allowed == null) 2187 this.allowed = new Money(); 2188 if (!(this.allowed instanceof Money)) 2189 throw new FHIRException("Type mismatch: the type Money was expected, but " + this.allowed.getClass().getName() 2190 + " was encountered"); 2191 return (Money) this.allowed; 2192 } 2193 2194 public boolean hasAllowedMoney() { 2195 return this != null && this.allowed instanceof Money; 2196 } 2197 2198 public boolean hasAllowed() { 2199 return this.allowed != null && !this.allowed.isEmpty(); 2200 } 2201 2202 /** 2203 * @param value {@link #allowed} (The quantity of the benefit which is permitted 2204 * under the coverage.) 2205 */ 2206 public BenefitComponent setAllowed(Type value) { 2207 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 2208 throw new Error("Not the right type for CoverageEligibilityResponse.insurance.item.benefit.allowed[x]: " 2209 + value.fhirType()); 2210 this.allowed = value; 2211 return this; 2212 } 2213 2214 /** 2215 * @return {@link #used} (The quantity of the benefit which have been consumed 2216 * to date.) 2217 */ 2218 public Type getUsed() { 2219 return this.used; 2220 } 2221 2222 /** 2223 * @return {@link #used} (The quantity of the benefit which have been consumed 2224 * to date.) 2225 */ 2226 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 2227 if (this.used == null) 2228 this.used = new UnsignedIntType(); 2229 if (!(this.used instanceof UnsignedIntType)) 2230 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but " 2231 + this.used.getClass().getName() + " was encountered"); 2232 return (UnsignedIntType) this.used; 2233 } 2234 2235 public boolean hasUsedUnsignedIntType() { 2236 return this != null && this.used instanceof UnsignedIntType; 2237 } 2238 2239 /** 2240 * @return {@link #used} (The quantity of the benefit which have been consumed 2241 * to date.) 2242 */ 2243 public StringType getUsedStringType() throws FHIRException { 2244 if (this.used == null) 2245 this.used = new StringType(); 2246 if (!(this.used instanceof StringType)) 2247 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.used.getClass().getName() 2248 + " was encountered"); 2249 return (StringType) this.used; 2250 } 2251 2252 public boolean hasUsedStringType() { 2253 return this != null && this.used instanceof StringType; 2254 } 2255 2256 /** 2257 * @return {@link #used} (The quantity of the benefit which have been consumed 2258 * to date.) 2259 */ 2260 public Money getUsedMoney() throws FHIRException { 2261 if (this.used == null) 2262 this.used = new Money(); 2263 if (!(this.used instanceof Money)) 2264 throw new FHIRException( 2265 "Type mismatch: the type Money was expected, but " + this.used.getClass().getName() + " was encountered"); 2266 return (Money) this.used; 2267 } 2268 2269 public boolean hasUsedMoney() { 2270 return this != null && this.used instanceof Money; 2271 } 2272 2273 public boolean hasUsed() { 2274 return this.used != null && !this.used.isEmpty(); 2275 } 2276 2277 /** 2278 * @param value {@link #used} (The quantity of the benefit which have been 2279 * consumed to date.) 2280 */ 2281 public BenefitComponent setUsed(Type value) { 2282 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 2283 throw new Error( 2284 "Not the right type for CoverageEligibilityResponse.insurance.item.benefit.used[x]: " + value.fhirType()); 2285 this.used = value; 2286 return this; 2287 } 2288 2289 protected void listChildren(List<Property> children) { 2290 super.listChildren(children); 2291 children.add(new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type)); 2292 children.add(new Property("allowed[x]", "unsignedInt|string|Money", 2293 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed)); 2294 children.add(new Property("used[x]", "unsignedInt|string|Money", 2295 "The quantity of the benefit which have been consumed to date.", 0, 1, used)); 2296 } 2297 2298 @Override 2299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2300 switch (_hash) { 2301 case 3575610: 2302 /* type */ return new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, 2303 type); 2304 case -1336663592: 2305 /* allowed[x] */ return new Property("allowed[x]", "unsignedInt|string|Money", 2306 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2307 case -911343192: 2308 /* allowed */ return new Property("allowed[x]", "unsignedInt|string|Money", 2309 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2310 case 1668802034: 2311 /* allowedUnsignedInt */ return new Property("allowed[x]", "unsignedInt|string|Money", 2312 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2313 case -2135265319: 2314 /* allowedString */ return new Property("allowed[x]", "unsignedInt|string|Money", 2315 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2316 case -351668232: 2317 /* allowedMoney */ return new Property("allowed[x]", "unsignedInt|string|Money", 2318 "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 2319 case -147553373: 2320 /* used[x] */ return new Property("used[x]", "unsignedInt|string|Money", 2321 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2322 case 3599293: 2323 /* used */ return new Property("used[x]", "unsignedInt|string|Money", 2324 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2325 case 1252740285: 2326 /* usedUnsignedInt */ return new Property("used[x]", "unsignedInt|string|Money", 2327 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2328 case 2051978798: 2329 /* usedString */ return new Property("used[x]", "unsignedInt|string|Money", 2330 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2331 case -78048509: 2332 /* usedMoney */ return new Property("used[x]", "unsignedInt|string|Money", 2333 "The quantity of the benefit which have been consumed to date.", 0, 1, used); 2334 default: 2335 return super.getNamedProperty(_hash, _name, _checkValid); 2336 } 2337 2338 } 2339 2340 @Override 2341 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2342 switch (hash) { 2343 case 3575610: 2344 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2345 case -911343192: 2346 /* allowed */ return this.allowed == null ? new Base[0] : new Base[] { this.allowed }; // Type 2347 case 3599293: 2348 /* used */ return this.used == null ? new Base[0] : new Base[] { this.used }; // Type 2349 default: 2350 return super.getProperty(hash, name, checkValid); 2351 } 2352 2353 } 2354 2355 @Override 2356 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2357 switch (hash) { 2358 case 3575610: // type 2359 this.type = castToCodeableConcept(value); // CodeableConcept 2360 return value; 2361 case -911343192: // allowed 2362 this.allowed = castToType(value); // Type 2363 return value; 2364 case 3599293: // used 2365 this.used = castToType(value); // Type 2366 return value; 2367 default: 2368 return super.setProperty(hash, name, value); 2369 } 2370 2371 } 2372 2373 @Override 2374 public Base setProperty(String name, Base value) throws FHIRException { 2375 if (name.equals("type")) { 2376 this.type = castToCodeableConcept(value); // CodeableConcept 2377 } else if (name.equals("allowed[x]")) { 2378 this.allowed = castToType(value); // Type 2379 } else if (name.equals("used[x]")) { 2380 this.used = castToType(value); // Type 2381 } else 2382 return super.setProperty(name, value); 2383 return value; 2384 } 2385 2386 @Override 2387 public void removeChild(String name, Base value) throws FHIRException { 2388 if (name.equals("type")) { 2389 this.type = null; 2390 } else if (name.equals("allowed[x]")) { 2391 this.allowed = null; 2392 } else if (name.equals("used[x]")) { 2393 this.used = null; 2394 } else 2395 super.removeChild(name, value); 2396 2397 } 2398 2399 @Override 2400 public Base makeProperty(int hash, String name) throws FHIRException { 2401 switch (hash) { 2402 case 3575610: 2403 return getType(); 2404 case -1336663592: 2405 return getAllowed(); 2406 case -911343192: 2407 return getAllowed(); 2408 case -147553373: 2409 return getUsed(); 2410 case 3599293: 2411 return getUsed(); 2412 default: 2413 return super.makeProperty(hash, name); 2414 } 2415 2416 } 2417 2418 @Override 2419 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2420 switch (hash) { 2421 case 3575610: 2422 /* type */ return new String[] { "CodeableConcept" }; 2423 case -911343192: 2424 /* allowed */ return new String[] { "unsignedInt", "string", "Money" }; 2425 case 3599293: 2426 /* used */ return new String[] { "unsignedInt", "string", "Money" }; 2427 default: 2428 return super.getTypesForProperty(hash, name); 2429 } 2430 2431 } 2432 2433 @Override 2434 public Base addChild(String name) throws FHIRException { 2435 if (name.equals("type")) { 2436 this.type = new CodeableConcept(); 2437 return this.type; 2438 } else if (name.equals("allowedUnsignedInt")) { 2439 this.allowed = new UnsignedIntType(); 2440 return this.allowed; 2441 } else if (name.equals("allowedString")) { 2442 this.allowed = new StringType(); 2443 return this.allowed; 2444 } else if (name.equals("allowedMoney")) { 2445 this.allowed = new Money(); 2446 return this.allowed; 2447 } else if (name.equals("usedUnsignedInt")) { 2448 this.used = new UnsignedIntType(); 2449 return this.used; 2450 } else if (name.equals("usedString")) { 2451 this.used = new StringType(); 2452 return this.used; 2453 } else if (name.equals("usedMoney")) { 2454 this.used = new Money(); 2455 return this.used; 2456 } else 2457 return super.addChild(name); 2458 } 2459 2460 public BenefitComponent copy() { 2461 BenefitComponent dst = new BenefitComponent(); 2462 copyValues(dst); 2463 return dst; 2464 } 2465 2466 public void copyValues(BenefitComponent dst) { 2467 super.copyValues(dst); 2468 dst.type = type == null ? null : type.copy(); 2469 dst.allowed = allowed == null ? null : allowed.copy(); 2470 dst.used = used == null ? null : used.copy(); 2471 } 2472 2473 @Override 2474 public boolean equalsDeep(Base other_) { 2475 if (!super.equalsDeep(other_)) 2476 return false; 2477 if (!(other_ instanceof BenefitComponent)) 2478 return false; 2479 BenefitComponent o = (BenefitComponent) other_; 2480 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) 2481 && compareDeep(used, o.used, true); 2482 } 2483 2484 @Override 2485 public boolean equalsShallow(Base other_) { 2486 if (!super.equalsShallow(other_)) 2487 return false; 2488 if (!(other_ instanceof BenefitComponent)) 2489 return false; 2490 BenefitComponent o = (BenefitComponent) other_; 2491 return true; 2492 } 2493 2494 public boolean isEmpty() { 2495 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 2496 } 2497 2498 public String fhirType() { 2499 return "CoverageEligibilityResponse.insurance.item.benefit"; 2500 2501 } 2502 2503 } 2504 2505 @Block() 2506 public static class ErrorsComponent extends BackboneElement implements IBaseBackboneElement { 2507 /** 2508 * An error code,from a specified code system, which details why the eligibility 2509 * check could not be performed. 2510 */ 2511 @Child(name = "code", type = { 2512 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2513 @Description(shortDefinition = "Error code detailing processing issues", formalDefinition = "An error code,from a specified code system, which details why the eligibility check could not be performed.") 2514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/adjudication-error") 2515 protected CodeableConcept code; 2516 2517 private static final long serialVersionUID = -1048343046L; 2518 2519 /** 2520 * Constructor 2521 */ 2522 public ErrorsComponent() { 2523 super(); 2524 } 2525 2526 /** 2527 * Constructor 2528 */ 2529 public ErrorsComponent(CodeableConcept code) { 2530 super(); 2531 this.code = code; 2532 } 2533 2534 /** 2535 * @return {@link #code} (An error code,from a specified code system, which 2536 * details why the eligibility check could not be performed.) 2537 */ 2538 public CodeableConcept getCode() { 2539 if (this.code == null) 2540 if (Configuration.errorOnAutoCreate()) 2541 throw new Error("Attempt to auto-create ErrorsComponent.code"); 2542 else if (Configuration.doAutoCreate()) 2543 this.code = new CodeableConcept(); // cc 2544 return this.code; 2545 } 2546 2547 public boolean hasCode() { 2548 return this.code != null && !this.code.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #code} (An error code,from a specified code system, which 2553 * details why the eligibility check could not be performed.) 2554 */ 2555 public ErrorsComponent setCode(CodeableConcept value) { 2556 this.code = value; 2557 return this; 2558 } 2559 2560 protected void listChildren(List<Property> children) { 2561 super.listChildren(children); 2562 children.add(new Property("code", "CodeableConcept", 2563 "An error code,from a specified code system, which details why the eligibility check could not be performed.", 2564 0, 1, code)); 2565 } 2566 2567 @Override 2568 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2569 switch (_hash) { 2570 case 3059181: 2571 /* code */ return new Property("code", "CodeableConcept", 2572 "An error code,from a specified code system, which details why the eligibility check could not be performed.", 2573 0, 1, code); 2574 default: 2575 return super.getNamedProperty(_hash, _name, _checkValid); 2576 } 2577 2578 } 2579 2580 @Override 2581 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2582 switch (hash) { 2583 case 3059181: 2584 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 2585 default: 2586 return super.getProperty(hash, name, checkValid); 2587 } 2588 2589 } 2590 2591 @Override 2592 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2593 switch (hash) { 2594 case 3059181: // code 2595 this.code = castToCodeableConcept(value); // CodeableConcept 2596 return value; 2597 default: 2598 return super.setProperty(hash, name, value); 2599 } 2600 2601 } 2602 2603 @Override 2604 public Base setProperty(String name, Base value) throws FHIRException { 2605 if (name.equals("code")) { 2606 this.code = castToCodeableConcept(value); // CodeableConcept 2607 } else 2608 return super.setProperty(name, value); 2609 return value; 2610 } 2611 2612 @Override 2613 public void removeChild(String name, Base value) throws FHIRException { 2614 if (name.equals("code")) { 2615 this.code = null; 2616 } else 2617 super.removeChild(name, value); 2618 2619 } 2620 2621 @Override 2622 public Base makeProperty(int hash, String name) throws FHIRException { 2623 switch (hash) { 2624 case 3059181: 2625 return getCode(); 2626 default: 2627 return super.makeProperty(hash, name); 2628 } 2629 2630 } 2631 2632 @Override 2633 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2634 switch (hash) { 2635 case 3059181: 2636 /* code */ return new String[] { "CodeableConcept" }; 2637 default: 2638 return super.getTypesForProperty(hash, name); 2639 } 2640 2641 } 2642 2643 @Override 2644 public Base addChild(String name) throws FHIRException { 2645 if (name.equals("code")) { 2646 this.code = new CodeableConcept(); 2647 return this.code; 2648 } else 2649 return super.addChild(name); 2650 } 2651 2652 public ErrorsComponent copy() { 2653 ErrorsComponent dst = new ErrorsComponent(); 2654 copyValues(dst); 2655 return dst; 2656 } 2657 2658 public void copyValues(ErrorsComponent dst) { 2659 super.copyValues(dst); 2660 dst.code = code == null ? null : code.copy(); 2661 } 2662 2663 @Override 2664 public boolean equalsDeep(Base other_) { 2665 if (!super.equalsDeep(other_)) 2666 return false; 2667 if (!(other_ instanceof ErrorsComponent)) 2668 return false; 2669 ErrorsComponent o = (ErrorsComponent) other_; 2670 return compareDeep(code, o.code, true); 2671 } 2672 2673 @Override 2674 public boolean equalsShallow(Base other_) { 2675 if (!super.equalsShallow(other_)) 2676 return false; 2677 if (!(other_ instanceof ErrorsComponent)) 2678 return false; 2679 ErrorsComponent o = (ErrorsComponent) other_; 2680 return true; 2681 } 2682 2683 public boolean isEmpty() { 2684 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code); 2685 } 2686 2687 public String fhirType() { 2688 return "CoverageEligibilityResponse.error"; 2689 2690 } 2691 2692 } 2693 2694 /** 2695 * A unique identifier assigned to this coverage eligiblity request. 2696 */ 2697 @Child(name = "identifier", type = { 2698 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2699 @Description(shortDefinition = "Business Identifier for coverage eligiblity request", formalDefinition = "A unique identifier assigned to this coverage eligiblity request.") 2700 protected List<Identifier> identifier; 2701 2702 /** 2703 * The status of the resource instance. 2704 */ 2705 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2706 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 2707 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 2708 protected Enumeration<EligibilityResponseStatus> status; 2709 2710 /** 2711 * Code to specify whether requesting: prior authorization requirements for some 2712 * service categories or billing codes; benefits for coverages specified or 2713 * discovered; discovery and return of coverages for the patient; and/or 2714 * validation that the specified coverage is in-force at the date/period 2715 * specified or 'now' if not specified. 2716 */ 2717 @Child(name = "purpose", type = { 2718 CodeType.class }, order = 2, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2719 @Description(shortDefinition = "auth-requirements | benefits | discovery | validation", formalDefinition = "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.") 2720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/eligibilityresponse-purpose") 2721 protected List<Enumeration<EligibilityResponsePurpose>> purpose; 2722 2723 /** 2724 * The party who is the beneficiary of the supplied coverage and for whom 2725 * eligibility is sought. 2726 */ 2727 @Child(name = "patient", type = { Patient.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2728 @Description(shortDefinition = "Intended recipient of products and services", formalDefinition = "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.") 2729 protected Reference patient; 2730 2731 /** 2732 * The actual object that is the target of the reference (The party who is the 2733 * beneficiary of the supplied coverage and for whom eligibility is sought.) 2734 */ 2735 protected Patient patientTarget; 2736 2737 /** 2738 * The date or dates when the enclosed suite of services were performed or 2739 * completed. 2740 */ 2741 @Child(name = "serviced", type = { DateType.class, 2742 Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2743 @Description(shortDefinition = "Estimated date or dates of service", formalDefinition = "The date or dates when the enclosed suite of services were performed or completed.") 2744 protected Type serviced; 2745 2746 /** 2747 * The date this resource was created. 2748 */ 2749 @Child(name = "created", type = { DateTimeType.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 2750 @Description(shortDefinition = "Response creation date", formalDefinition = "The date this resource was created.") 2751 protected DateTimeType created; 2752 2753 /** 2754 * The provider which is responsible for the request. 2755 */ 2756 @Child(name = "requestor", type = { Practitioner.class, PractitionerRole.class, 2757 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 2758 @Description(shortDefinition = "Party responsible for the request", formalDefinition = "The provider which is responsible for the request.") 2759 protected Reference requestor; 2760 2761 /** 2762 * The actual object that is the target of the reference (The provider which is 2763 * responsible for the request.) 2764 */ 2765 protected Resource requestorTarget; 2766 2767 /** 2768 * Reference to the original request resource. 2769 */ 2770 @Child(name = "request", type = { 2771 CoverageEligibilityRequest.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 2772 @Description(shortDefinition = "Eligibility request reference", formalDefinition = "Reference to the original request resource.") 2773 protected Reference request; 2774 2775 /** 2776 * The actual object that is the target of the reference (Reference to the 2777 * original request resource.) 2778 */ 2779 protected CoverageEligibilityRequest requestTarget; 2780 2781 /** 2782 * The outcome of the request processing. 2783 */ 2784 @Child(name = "outcome", type = { CodeType.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 2785 @Description(shortDefinition = "queued | complete | error | partial", formalDefinition = "The outcome of the request processing.") 2786 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/remittance-outcome") 2787 protected Enumeration<RemittanceOutcome> outcome; 2788 2789 /** 2790 * A human readable description of the status of the adjudication. 2791 */ 2792 @Child(name = "disposition", type = { 2793 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 2794 @Description(shortDefinition = "Disposition Message", formalDefinition = "A human readable description of the status of the adjudication.") 2795 protected StringType disposition; 2796 2797 /** 2798 * The Insurer who issued the coverage in question and is the author of the 2799 * response. 2800 */ 2801 @Child(name = "insurer", type = { 2802 Organization.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 2803 @Description(shortDefinition = "Coverage issuer", formalDefinition = "The Insurer who issued the coverage in question and is the author of the response.") 2804 protected Reference insurer; 2805 2806 /** 2807 * The actual object that is the target of the reference (The Insurer who issued 2808 * the coverage in question and is the author of the response.) 2809 */ 2810 protected Organization insurerTarget; 2811 2812 /** 2813 * Financial instruments for reimbursement for the health care products and 2814 * services. 2815 */ 2816 @Child(name = "insurance", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2817 @Description(shortDefinition = "Patient insurance information", formalDefinition = "Financial instruments for reimbursement for the health care products and services.") 2818 protected List<InsuranceComponent> insurance; 2819 2820 /** 2821 * A reference from the Insurer to which these services pertain to be used on 2822 * further communication and as proof that the request occurred. 2823 */ 2824 @Child(name = "preAuthRef", type = { 2825 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 2826 @Description(shortDefinition = "Preauthorization reference", formalDefinition = "A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.") 2827 protected StringType preAuthRef; 2828 2829 /** 2830 * A code for the form to be used for printing the content. 2831 */ 2832 @Child(name = "form", type = { 2833 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 2834 @Description(shortDefinition = "Printed form identifier", formalDefinition = "A code for the form to be used for printing the content.") 2835 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/forms") 2836 protected CodeableConcept form; 2837 2838 /** 2839 * Errors encountered during the processing of the request. 2840 */ 2841 @Child(name = "error", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2842 @Description(shortDefinition = "Processing errors", formalDefinition = "Errors encountered during the processing of the request.") 2843 protected List<ErrorsComponent> error; 2844 2845 private static final long serialVersionUID = -266280848L; 2846 2847 /** 2848 * Constructor 2849 */ 2850 public CoverageEligibilityResponse() { 2851 super(); 2852 } 2853 2854 /** 2855 * Constructor 2856 */ 2857 public CoverageEligibilityResponse(Enumeration<EligibilityResponseStatus> status, Reference patient, 2858 DateTimeType created, Reference request, Enumeration<RemittanceOutcome> outcome, Reference insurer) { 2859 super(); 2860 this.status = status; 2861 this.patient = patient; 2862 this.created = created; 2863 this.request = request; 2864 this.outcome = outcome; 2865 this.insurer = insurer; 2866 } 2867 2868 /** 2869 * @return {@link #identifier} (A unique identifier assigned to this coverage 2870 * eligiblity request.) 2871 */ 2872 public List<Identifier> getIdentifier() { 2873 if (this.identifier == null) 2874 this.identifier = new ArrayList<Identifier>(); 2875 return this.identifier; 2876 } 2877 2878 /** 2879 * @return Returns a reference to <code>this</code> for easy method chaining 2880 */ 2881 public CoverageEligibilityResponse setIdentifier(List<Identifier> theIdentifier) { 2882 this.identifier = theIdentifier; 2883 return this; 2884 } 2885 2886 public boolean hasIdentifier() { 2887 if (this.identifier == null) 2888 return false; 2889 for (Identifier item : this.identifier) 2890 if (!item.isEmpty()) 2891 return true; 2892 return false; 2893 } 2894 2895 public Identifier addIdentifier() { // 3 2896 Identifier t = new Identifier(); 2897 if (this.identifier == null) 2898 this.identifier = new ArrayList<Identifier>(); 2899 this.identifier.add(t); 2900 return t; 2901 } 2902 2903 public CoverageEligibilityResponse addIdentifier(Identifier t) { // 3 2904 if (t == null) 2905 return this; 2906 if (this.identifier == null) 2907 this.identifier = new ArrayList<Identifier>(); 2908 this.identifier.add(t); 2909 return this; 2910 } 2911 2912 /** 2913 * @return The first repetition of repeating field {@link #identifier}, creating 2914 * it if it does not already exist 2915 */ 2916 public Identifier getIdentifierFirstRep() { 2917 if (getIdentifier().isEmpty()) { 2918 addIdentifier(); 2919 } 2920 return getIdentifier().get(0); 2921 } 2922 2923 /** 2924 * @return {@link #status} (The status of the resource instance.). This is the 2925 * underlying object with id, value and extensions. The accessor 2926 * "getStatus" gives direct access to the value 2927 */ 2928 public Enumeration<EligibilityResponseStatus> getStatusElement() { 2929 if (this.status == null) 2930 if (Configuration.errorOnAutoCreate()) 2931 throw new Error("Attempt to auto-create CoverageEligibilityResponse.status"); 2932 else if (Configuration.doAutoCreate()) 2933 this.status = new Enumeration<EligibilityResponseStatus>(new EligibilityResponseStatusEnumFactory()); // bb 2934 return this.status; 2935 } 2936 2937 public boolean hasStatusElement() { 2938 return this.status != null && !this.status.isEmpty(); 2939 } 2940 2941 public boolean hasStatus() { 2942 return this.status != null && !this.status.isEmpty(); 2943 } 2944 2945 /** 2946 * @param value {@link #status} (The status of the resource instance.). This is 2947 * the underlying object with id, value and extensions. The 2948 * accessor "getStatus" gives direct access to the value 2949 */ 2950 public CoverageEligibilityResponse setStatusElement(Enumeration<EligibilityResponseStatus> value) { 2951 this.status = value; 2952 return this; 2953 } 2954 2955 /** 2956 * @return The status of the resource instance. 2957 */ 2958 public EligibilityResponseStatus getStatus() { 2959 return this.status == null ? null : this.status.getValue(); 2960 } 2961 2962 /** 2963 * @param value The status of the resource instance. 2964 */ 2965 public CoverageEligibilityResponse setStatus(EligibilityResponseStatus value) { 2966 if (this.status == null) 2967 this.status = new Enumeration<EligibilityResponseStatus>(new EligibilityResponseStatusEnumFactory()); 2968 this.status.setValue(value); 2969 return this; 2970 } 2971 2972 /** 2973 * @return {@link #purpose} (Code to specify whether requesting: prior 2974 * authorization requirements for some service categories or billing 2975 * codes; benefits for coverages specified or discovered; discovery and 2976 * return of coverages for the patient; and/or validation that the 2977 * specified coverage is in-force at the date/period specified or 'now' 2978 * if not specified.) 2979 */ 2980 public List<Enumeration<EligibilityResponsePurpose>> getPurpose() { 2981 if (this.purpose == null) 2982 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 2983 return this.purpose; 2984 } 2985 2986 /** 2987 * @return Returns a reference to <code>this</code> for easy method chaining 2988 */ 2989 public CoverageEligibilityResponse setPurpose(List<Enumeration<EligibilityResponsePurpose>> thePurpose) { 2990 this.purpose = thePurpose; 2991 return this; 2992 } 2993 2994 public boolean hasPurpose() { 2995 if (this.purpose == null) 2996 return false; 2997 for (Enumeration<EligibilityResponsePurpose> item : this.purpose) 2998 if (!item.isEmpty()) 2999 return true; 3000 return false; 3001 } 3002 3003 /** 3004 * @return {@link #purpose} (Code to specify whether requesting: prior 3005 * authorization requirements for some service categories or billing 3006 * codes; benefits for coverages specified or discovered; discovery and 3007 * return of coverages for the patient; and/or validation that the 3008 * specified coverage is in-force at the date/period specified or 'now' 3009 * if not specified.) 3010 */ 3011 public Enumeration<EligibilityResponsePurpose> addPurposeElement() {// 2 3012 Enumeration<EligibilityResponsePurpose> t = new Enumeration<EligibilityResponsePurpose>( 3013 new EligibilityResponsePurposeEnumFactory()); 3014 if (this.purpose == null) 3015 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 3016 this.purpose.add(t); 3017 return t; 3018 } 3019 3020 /** 3021 * @param value {@link #purpose} (Code to specify whether requesting: prior 3022 * authorization requirements for some service categories or 3023 * billing codes; benefits for coverages specified or discovered; 3024 * discovery and return of coverages for the patient; and/or 3025 * validation that the specified coverage is in-force at the 3026 * date/period specified or 'now' if not specified.) 3027 */ 3028 public CoverageEligibilityResponse addPurpose(EligibilityResponsePurpose value) { // 1 3029 Enumeration<EligibilityResponsePurpose> t = new Enumeration<EligibilityResponsePurpose>( 3030 new EligibilityResponsePurposeEnumFactory()); 3031 t.setValue(value); 3032 if (this.purpose == null) 3033 this.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 3034 this.purpose.add(t); 3035 return this; 3036 } 3037 3038 /** 3039 * @param value {@link #purpose} (Code to specify whether requesting: prior 3040 * authorization requirements for some service categories or 3041 * billing codes; benefits for coverages specified or discovered; 3042 * discovery and return of coverages for the patient; and/or 3043 * validation that the specified coverage is in-force at the 3044 * date/period specified or 'now' if not specified.) 3045 */ 3046 public boolean hasPurpose(EligibilityResponsePurpose value) { 3047 if (this.purpose == null) 3048 return false; 3049 for (Enumeration<EligibilityResponsePurpose> v : this.purpose) 3050 if (v.getValue().equals(value)) // code 3051 return true; 3052 return false; 3053 } 3054 3055 /** 3056 * @return {@link #patient} (The party who is the beneficiary of the supplied 3057 * coverage and for whom eligibility is sought.) 3058 */ 3059 public Reference getPatient() { 3060 if (this.patient == null) 3061 if (Configuration.errorOnAutoCreate()) 3062 throw new Error("Attempt to auto-create CoverageEligibilityResponse.patient"); 3063 else if (Configuration.doAutoCreate()) 3064 this.patient = new Reference(); // cc 3065 return this.patient; 3066 } 3067 3068 public boolean hasPatient() { 3069 return this.patient != null && !this.patient.isEmpty(); 3070 } 3071 3072 /** 3073 * @param value {@link #patient} (The party who is the beneficiary of the 3074 * supplied coverage and for whom eligibility is sought.) 3075 */ 3076 public CoverageEligibilityResponse setPatient(Reference value) { 3077 this.patient = value; 3078 return this; 3079 } 3080 3081 /** 3082 * @return {@link #patient} The actual object that is the target of the 3083 * reference. The reference library doesn't populate this, but you can 3084 * use it to hold the resource if you resolve it. (The party who is the 3085 * beneficiary of the supplied coverage and for whom eligibility is 3086 * sought.) 3087 */ 3088 public Patient getPatientTarget() { 3089 if (this.patientTarget == null) 3090 if (Configuration.errorOnAutoCreate()) 3091 throw new Error("Attempt to auto-create CoverageEligibilityResponse.patient"); 3092 else if (Configuration.doAutoCreate()) 3093 this.patientTarget = new Patient(); // aa 3094 return this.patientTarget; 3095 } 3096 3097 /** 3098 * @param value {@link #patient} The actual object that is the target of the 3099 * reference. The reference library doesn't use these, but you can 3100 * use it to hold the resource if you resolve it. (The party who is 3101 * the beneficiary of the supplied coverage and for whom 3102 * eligibility is sought.) 3103 */ 3104 public CoverageEligibilityResponse setPatientTarget(Patient value) { 3105 this.patientTarget = value; 3106 return this; 3107 } 3108 3109 /** 3110 * @return {@link #serviced} (The date or dates when the enclosed suite of 3111 * services were performed or completed.) 3112 */ 3113 public Type getServiced() { 3114 return this.serviced; 3115 } 3116 3117 /** 3118 * @return {@link #serviced} (The date or dates when the enclosed suite of 3119 * services were performed or completed.) 3120 */ 3121 public DateType getServicedDateType() throws FHIRException { 3122 if (this.serviced == null) 3123 this.serviced = new DateType(); 3124 if (!(this.serviced instanceof DateType)) 3125 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.serviced.getClass().getName() 3126 + " was encountered"); 3127 return (DateType) this.serviced; 3128 } 3129 3130 public boolean hasServicedDateType() { 3131 return this != null && this.serviced instanceof DateType; 3132 } 3133 3134 /** 3135 * @return {@link #serviced} (The date or dates when the enclosed suite of 3136 * services were performed or completed.) 3137 */ 3138 public Period getServicedPeriod() throws FHIRException { 3139 if (this.serviced == null) 3140 this.serviced = new Period(); 3141 if (!(this.serviced instanceof Period)) 3142 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.serviced.getClass().getName() 3143 + " was encountered"); 3144 return (Period) this.serviced; 3145 } 3146 3147 public boolean hasServicedPeriod() { 3148 return this != null && this.serviced instanceof Period; 3149 } 3150 3151 public boolean hasServiced() { 3152 return this.serviced != null && !this.serviced.isEmpty(); 3153 } 3154 3155 /** 3156 * @param value {@link #serviced} (The date or dates when the enclosed suite of 3157 * services were performed or completed.) 3158 */ 3159 public CoverageEligibilityResponse setServiced(Type value) { 3160 if (value != null && !(value instanceof DateType || value instanceof Period)) 3161 throw new Error("Not the right type for CoverageEligibilityResponse.serviced[x]: " + value.fhirType()); 3162 this.serviced = value; 3163 return this; 3164 } 3165 3166 /** 3167 * @return {@link #created} (The date this resource was created.). This is the 3168 * underlying object with id, value and extensions. The accessor 3169 * "getCreated" gives direct access to the value 3170 */ 3171 public DateTimeType getCreatedElement() { 3172 if (this.created == null) 3173 if (Configuration.errorOnAutoCreate()) 3174 throw new Error("Attempt to auto-create CoverageEligibilityResponse.created"); 3175 else if (Configuration.doAutoCreate()) 3176 this.created = new DateTimeType(); // bb 3177 return this.created; 3178 } 3179 3180 public boolean hasCreatedElement() { 3181 return this.created != null && !this.created.isEmpty(); 3182 } 3183 3184 public boolean hasCreated() { 3185 return this.created != null && !this.created.isEmpty(); 3186 } 3187 3188 /** 3189 * @param value {@link #created} (The date this resource was created.). This is 3190 * the underlying object with id, value and extensions. The 3191 * accessor "getCreated" gives direct access to the value 3192 */ 3193 public CoverageEligibilityResponse setCreatedElement(DateTimeType value) { 3194 this.created = value; 3195 return this; 3196 } 3197 3198 /** 3199 * @return The date this resource was created. 3200 */ 3201 public Date getCreated() { 3202 return this.created == null ? null : this.created.getValue(); 3203 } 3204 3205 /** 3206 * @param value The date this resource was created. 3207 */ 3208 public CoverageEligibilityResponse setCreated(Date value) { 3209 if (this.created == null) 3210 this.created = new DateTimeType(); 3211 this.created.setValue(value); 3212 return this; 3213 } 3214 3215 /** 3216 * @return {@link #requestor} (The provider which is responsible for the 3217 * request.) 3218 */ 3219 public Reference getRequestor() { 3220 if (this.requestor == null) 3221 if (Configuration.errorOnAutoCreate()) 3222 throw new Error("Attempt to auto-create CoverageEligibilityResponse.requestor"); 3223 else if (Configuration.doAutoCreate()) 3224 this.requestor = new Reference(); // cc 3225 return this.requestor; 3226 } 3227 3228 public boolean hasRequestor() { 3229 return this.requestor != null && !this.requestor.isEmpty(); 3230 } 3231 3232 /** 3233 * @param value {@link #requestor} (The provider which is responsible for the 3234 * request.) 3235 */ 3236 public CoverageEligibilityResponse setRequestor(Reference value) { 3237 this.requestor = value; 3238 return this; 3239 } 3240 3241 /** 3242 * @return {@link #requestor} The actual object that is the target of the 3243 * reference. The reference library doesn't populate this, but you can 3244 * use it to hold the resource if you resolve it. (The provider which is 3245 * responsible for the request.) 3246 */ 3247 public Resource getRequestorTarget() { 3248 return this.requestorTarget; 3249 } 3250 3251 /** 3252 * @param value {@link #requestor} The actual object that is the target of the 3253 * reference. The reference library doesn't use these, but you can 3254 * use it to hold the resource if you resolve it. (The provider 3255 * which is responsible for the request.) 3256 */ 3257 public CoverageEligibilityResponse setRequestorTarget(Resource value) { 3258 this.requestorTarget = value; 3259 return this; 3260 } 3261 3262 /** 3263 * @return {@link #request} (Reference to the original request resource.) 3264 */ 3265 public Reference getRequest() { 3266 if (this.request == null) 3267 if (Configuration.errorOnAutoCreate()) 3268 throw new Error("Attempt to auto-create CoverageEligibilityResponse.request"); 3269 else if (Configuration.doAutoCreate()) 3270 this.request = new Reference(); // cc 3271 return this.request; 3272 } 3273 3274 public boolean hasRequest() { 3275 return this.request != null && !this.request.isEmpty(); 3276 } 3277 3278 /** 3279 * @param value {@link #request} (Reference to the original request resource.) 3280 */ 3281 public CoverageEligibilityResponse setRequest(Reference value) { 3282 this.request = value; 3283 return this; 3284 } 3285 3286 /** 3287 * @return {@link #request} The actual object that is the target of the 3288 * reference. The reference library doesn't populate this, but you can 3289 * use it to hold the resource if you resolve it. (Reference to the 3290 * original request resource.) 3291 */ 3292 public CoverageEligibilityRequest getRequestTarget() { 3293 if (this.requestTarget == null) 3294 if (Configuration.errorOnAutoCreate()) 3295 throw new Error("Attempt to auto-create CoverageEligibilityResponse.request"); 3296 else if (Configuration.doAutoCreate()) 3297 this.requestTarget = new CoverageEligibilityRequest(); // aa 3298 return this.requestTarget; 3299 } 3300 3301 /** 3302 * @param value {@link #request} The actual object that is the target of the 3303 * reference. The reference library doesn't use these, but you can 3304 * use it to hold the resource if you resolve it. (Reference to the 3305 * original request resource.) 3306 */ 3307 public CoverageEligibilityResponse setRequestTarget(CoverageEligibilityRequest value) { 3308 this.requestTarget = value; 3309 return this; 3310 } 3311 3312 /** 3313 * @return {@link #outcome} (The outcome of the request processing.). This is 3314 * the underlying object with id, value and extensions. The accessor 3315 * "getOutcome" gives direct access to the value 3316 */ 3317 public Enumeration<RemittanceOutcome> getOutcomeElement() { 3318 if (this.outcome == null) 3319 if (Configuration.errorOnAutoCreate()) 3320 throw new Error("Attempt to auto-create CoverageEligibilityResponse.outcome"); 3321 else if (Configuration.doAutoCreate()) 3322 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 3323 return this.outcome; 3324 } 3325 3326 public boolean hasOutcomeElement() { 3327 return this.outcome != null && !this.outcome.isEmpty(); 3328 } 3329 3330 public boolean hasOutcome() { 3331 return this.outcome != null && !this.outcome.isEmpty(); 3332 } 3333 3334 /** 3335 * @param value {@link #outcome} (The outcome of the request processing.). This 3336 * is the underlying object with id, value and extensions. The 3337 * accessor "getOutcome" gives direct access to the value 3338 */ 3339 public CoverageEligibilityResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 3340 this.outcome = value; 3341 return this; 3342 } 3343 3344 /** 3345 * @return The outcome of the request processing. 3346 */ 3347 public RemittanceOutcome getOutcome() { 3348 return this.outcome == null ? null : this.outcome.getValue(); 3349 } 3350 3351 /** 3352 * @param value The outcome of the request processing. 3353 */ 3354 public CoverageEligibilityResponse setOutcome(RemittanceOutcome value) { 3355 if (this.outcome == null) 3356 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 3357 this.outcome.setValue(value); 3358 return this; 3359 } 3360 3361 /** 3362 * @return {@link #disposition} (A human readable description of the status of 3363 * the adjudication.). This is the underlying object with id, value and 3364 * extensions. The accessor "getDisposition" gives direct access to the 3365 * value 3366 */ 3367 public StringType getDispositionElement() { 3368 if (this.disposition == null) 3369 if (Configuration.errorOnAutoCreate()) 3370 throw new Error("Attempt to auto-create CoverageEligibilityResponse.disposition"); 3371 else if (Configuration.doAutoCreate()) 3372 this.disposition = new StringType(); // bb 3373 return this.disposition; 3374 } 3375 3376 public boolean hasDispositionElement() { 3377 return this.disposition != null && !this.disposition.isEmpty(); 3378 } 3379 3380 public boolean hasDisposition() { 3381 return this.disposition != null && !this.disposition.isEmpty(); 3382 } 3383 3384 /** 3385 * @param value {@link #disposition} (A human readable description of the status 3386 * of the adjudication.). This is the underlying object with id, 3387 * value and extensions. The accessor "getDisposition" gives direct 3388 * access to the value 3389 */ 3390 public CoverageEligibilityResponse setDispositionElement(StringType value) { 3391 this.disposition = value; 3392 return this; 3393 } 3394 3395 /** 3396 * @return A human readable description of the status of the adjudication. 3397 */ 3398 public String getDisposition() { 3399 return this.disposition == null ? null : this.disposition.getValue(); 3400 } 3401 3402 /** 3403 * @param value A human readable description of the status of the adjudication. 3404 */ 3405 public CoverageEligibilityResponse setDisposition(String value) { 3406 if (Utilities.noString(value)) 3407 this.disposition = null; 3408 else { 3409 if (this.disposition == null) 3410 this.disposition = new StringType(); 3411 this.disposition.setValue(value); 3412 } 3413 return this; 3414 } 3415 3416 /** 3417 * @return {@link #insurer} (The Insurer who issued the coverage in question and 3418 * is the author of the response.) 3419 */ 3420 public Reference getInsurer() { 3421 if (this.insurer == null) 3422 if (Configuration.errorOnAutoCreate()) 3423 throw new Error("Attempt to auto-create CoverageEligibilityResponse.insurer"); 3424 else if (Configuration.doAutoCreate()) 3425 this.insurer = new Reference(); // cc 3426 return this.insurer; 3427 } 3428 3429 public boolean hasInsurer() { 3430 return this.insurer != null && !this.insurer.isEmpty(); 3431 } 3432 3433 /** 3434 * @param value {@link #insurer} (The Insurer who issued the coverage in 3435 * question and is the author of the response.) 3436 */ 3437 public CoverageEligibilityResponse setInsurer(Reference value) { 3438 this.insurer = value; 3439 return this; 3440 } 3441 3442 /** 3443 * @return {@link #insurer} The actual object that is the target of the 3444 * reference. The reference library doesn't populate this, but you can 3445 * use it to hold the resource if you resolve it. (The Insurer who 3446 * issued the coverage in question and is the author of the response.) 3447 */ 3448 public Organization getInsurerTarget() { 3449 if (this.insurerTarget == null) 3450 if (Configuration.errorOnAutoCreate()) 3451 throw new Error("Attempt to auto-create CoverageEligibilityResponse.insurer"); 3452 else if (Configuration.doAutoCreate()) 3453 this.insurerTarget = new Organization(); // aa 3454 return this.insurerTarget; 3455 } 3456 3457 /** 3458 * @param value {@link #insurer} The actual object that is the target of the 3459 * reference. The reference library doesn't use these, but you can 3460 * use it to hold the resource if you resolve it. (The Insurer who 3461 * issued the coverage in question and is the author of the 3462 * response.) 3463 */ 3464 public CoverageEligibilityResponse setInsurerTarget(Organization value) { 3465 this.insurerTarget = value; 3466 return this; 3467 } 3468 3469 /** 3470 * @return {@link #insurance} (Financial instruments for reimbursement for the 3471 * health care products and services.) 3472 */ 3473 public List<InsuranceComponent> getInsurance() { 3474 if (this.insurance == null) 3475 this.insurance = new ArrayList<InsuranceComponent>(); 3476 return this.insurance; 3477 } 3478 3479 /** 3480 * @return Returns a reference to <code>this</code> for easy method chaining 3481 */ 3482 public CoverageEligibilityResponse setInsurance(List<InsuranceComponent> theInsurance) { 3483 this.insurance = theInsurance; 3484 return this; 3485 } 3486 3487 public boolean hasInsurance() { 3488 if (this.insurance == null) 3489 return false; 3490 for (InsuranceComponent item : this.insurance) 3491 if (!item.isEmpty()) 3492 return true; 3493 return false; 3494 } 3495 3496 public InsuranceComponent addInsurance() { // 3 3497 InsuranceComponent t = new InsuranceComponent(); 3498 if (this.insurance == null) 3499 this.insurance = new ArrayList<InsuranceComponent>(); 3500 this.insurance.add(t); 3501 return t; 3502 } 3503 3504 public CoverageEligibilityResponse addInsurance(InsuranceComponent t) { // 3 3505 if (t == null) 3506 return this; 3507 if (this.insurance == null) 3508 this.insurance = new ArrayList<InsuranceComponent>(); 3509 this.insurance.add(t); 3510 return this; 3511 } 3512 3513 /** 3514 * @return The first repetition of repeating field {@link #insurance}, creating 3515 * it if it does not already exist 3516 */ 3517 public InsuranceComponent getInsuranceFirstRep() { 3518 if (getInsurance().isEmpty()) { 3519 addInsurance(); 3520 } 3521 return getInsurance().get(0); 3522 } 3523 3524 /** 3525 * @return {@link #preAuthRef} (A reference from the Insurer to which these 3526 * services pertain to be used on further communication and as proof 3527 * that the request occurred.). This is the underlying object with id, 3528 * value and extensions. The accessor "getPreAuthRef" gives direct 3529 * access to the value 3530 */ 3531 public StringType getPreAuthRefElement() { 3532 if (this.preAuthRef == null) 3533 if (Configuration.errorOnAutoCreate()) 3534 throw new Error("Attempt to auto-create CoverageEligibilityResponse.preAuthRef"); 3535 else if (Configuration.doAutoCreate()) 3536 this.preAuthRef = new StringType(); // bb 3537 return this.preAuthRef; 3538 } 3539 3540 public boolean hasPreAuthRefElement() { 3541 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 3542 } 3543 3544 public boolean hasPreAuthRef() { 3545 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 3546 } 3547 3548 /** 3549 * @param value {@link #preAuthRef} (A reference from the Insurer to which these 3550 * services pertain to be used on further communication and as 3551 * proof that the request occurred.). This is the underlying object 3552 * with id, value and extensions. The accessor "getPreAuthRef" 3553 * gives direct access to the value 3554 */ 3555 public CoverageEligibilityResponse setPreAuthRefElement(StringType value) { 3556 this.preAuthRef = value; 3557 return this; 3558 } 3559 3560 /** 3561 * @return A reference from the Insurer to which these services pertain to be 3562 * used on further communication and as proof that the request occurred. 3563 */ 3564 public String getPreAuthRef() { 3565 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 3566 } 3567 3568 /** 3569 * @param value A reference from the Insurer to which these services pertain to 3570 * be used on further communication and as proof that the request 3571 * occurred. 3572 */ 3573 public CoverageEligibilityResponse setPreAuthRef(String value) { 3574 if (Utilities.noString(value)) 3575 this.preAuthRef = null; 3576 else { 3577 if (this.preAuthRef == null) 3578 this.preAuthRef = new StringType(); 3579 this.preAuthRef.setValue(value); 3580 } 3581 return this; 3582 } 3583 3584 /** 3585 * @return {@link #form} (A code for the form to be used for printing the 3586 * content.) 3587 */ 3588 public CodeableConcept getForm() { 3589 if (this.form == null) 3590 if (Configuration.errorOnAutoCreate()) 3591 throw new Error("Attempt to auto-create CoverageEligibilityResponse.form"); 3592 else if (Configuration.doAutoCreate()) 3593 this.form = new CodeableConcept(); // cc 3594 return this.form; 3595 } 3596 3597 public boolean hasForm() { 3598 return this.form != null && !this.form.isEmpty(); 3599 } 3600 3601 /** 3602 * @param value {@link #form} (A code for the form to be used for printing the 3603 * content.) 3604 */ 3605 public CoverageEligibilityResponse setForm(CodeableConcept value) { 3606 this.form = value; 3607 return this; 3608 } 3609 3610 /** 3611 * @return {@link #error} (Errors encountered during the processing of the 3612 * request.) 3613 */ 3614 public List<ErrorsComponent> getError() { 3615 if (this.error == null) 3616 this.error = new ArrayList<ErrorsComponent>(); 3617 return this.error; 3618 } 3619 3620 /** 3621 * @return Returns a reference to <code>this</code> for easy method chaining 3622 */ 3623 public CoverageEligibilityResponse setError(List<ErrorsComponent> theError) { 3624 this.error = theError; 3625 return this; 3626 } 3627 3628 public boolean hasError() { 3629 if (this.error == null) 3630 return false; 3631 for (ErrorsComponent item : this.error) 3632 if (!item.isEmpty()) 3633 return true; 3634 return false; 3635 } 3636 3637 public ErrorsComponent addError() { // 3 3638 ErrorsComponent t = new ErrorsComponent(); 3639 if (this.error == null) 3640 this.error = new ArrayList<ErrorsComponent>(); 3641 this.error.add(t); 3642 return t; 3643 } 3644 3645 public CoverageEligibilityResponse addError(ErrorsComponent t) { // 3 3646 if (t == null) 3647 return this; 3648 if (this.error == null) 3649 this.error = new ArrayList<ErrorsComponent>(); 3650 this.error.add(t); 3651 return this; 3652 } 3653 3654 /** 3655 * @return The first repetition of repeating field {@link #error}, creating it 3656 * if it does not already exist 3657 */ 3658 public ErrorsComponent getErrorFirstRep() { 3659 if (getError().isEmpty()) { 3660 addError(); 3661 } 3662 return getError().get(0); 3663 } 3664 3665 protected void listChildren(List<Property> children) { 3666 super.listChildren(children); 3667 children.add( 3668 new Property("identifier", "Identifier", "A unique identifier assigned to this coverage eligiblity request.", 0, 3669 java.lang.Integer.MAX_VALUE, identifier)); 3670 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 3671 children.add(new Property("purpose", "code", 3672 "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 3673 0, java.lang.Integer.MAX_VALUE, purpose)); 3674 children.add(new Property("patient", "Reference(Patient)", 3675 "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, 3676 patient)); 3677 children.add(new Property("serviced[x]", "date|Period", 3678 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 3679 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 3680 children.add(new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 3681 "The provider which is responsible for the request.", 0, 1, requestor)); 3682 children.add(new Property("request", "Reference(CoverageEligibilityRequest)", 3683 "Reference to the original request resource.", 0, 1, request)); 3684 children.add(new Property("outcome", "code", "The outcome of the request processing.", 0, 1, outcome)); 3685 children.add(new Property("disposition", "string", 3686 "A human readable description of the status of the adjudication.", 0, 1, disposition)); 3687 children.add(new Property("insurer", "Reference(Organization)", 3688 "The Insurer who issued the coverage in question and is the author of the response.", 0, 1, insurer)); 3689 children.add(new Property("insurance", "", 3690 "Financial instruments for reimbursement for the health care products and services.", 0, 3691 java.lang.Integer.MAX_VALUE, insurance)); 3692 children.add(new Property("preAuthRef", "string", 3693 "A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.", 3694 0, 1, preAuthRef)); 3695 children.add(new Property("form", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 3696 1, form)); 3697 children.add(new Property("error", "", "Errors encountered during the processing of the request.", 0, 3698 java.lang.Integer.MAX_VALUE, error)); 3699 } 3700 3701 @Override 3702 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3703 switch (_hash) { 3704 case -1618432855: 3705 /* identifier */ return new Property("identifier", "Identifier", 3706 "A unique identifier assigned to this coverage eligiblity request.", 0, java.lang.Integer.MAX_VALUE, 3707 identifier); 3708 case -892481550: 3709 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 3710 case -220463842: 3711 /* purpose */ return new Property("purpose", "code", 3712 "Code to specify whether requesting: prior authorization requirements for some service categories or billing codes; benefits for coverages specified or discovered; discovery and return of coverages for the patient; and/or validation that the specified coverage is in-force at the date/period specified or 'now' if not specified.", 3713 0, java.lang.Integer.MAX_VALUE, purpose); 3714 case -791418107: 3715 /* patient */ return new Property("patient", "Reference(Patient)", 3716 "The party who is the beneficiary of the supplied coverage and for whom eligibility is sought.", 0, 1, 3717 patient); 3718 case -1927922223: 3719 /* serviced[x] */ return new Property("serviced[x]", "date|Period", 3720 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3721 case 1379209295: 3722 /* serviced */ return new Property("serviced[x]", "date|Period", 3723 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3724 case 363246749: 3725 /* servicedDate */ return new Property("serviced[x]", "date|Period", 3726 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3727 case 1534966512: 3728 /* servicedPeriod */ return new Property("serviced[x]", "date|Period", 3729 "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 3730 case 1028554472: 3731 /* created */ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 3732 case 693934258: 3733 /* requestor */ return new Property("requestor", "Reference(Practitioner|PractitionerRole|Organization)", 3734 "The provider which is responsible for the request.", 0, 1, requestor); 3735 case 1095692943: 3736 /* request */ return new Property("request", "Reference(CoverageEligibilityRequest)", 3737 "Reference to the original request resource.", 0, 1, request); 3738 case -1106507950: 3739 /* outcome */ return new Property("outcome", "code", "The outcome of the request processing.", 0, 1, outcome); 3740 case 583380919: 3741 /* disposition */ return new Property("disposition", "string", 3742 "A human readable description of the status of the adjudication.", 0, 1, disposition); 3743 case 1957615864: 3744 /* insurer */ return new Property("insurer", "Reference(Organization)", 3745 "The Insurer who issued the coverage in question and is the author of the response.", 0, 1, insurer); 3746 case 73049818: 3747 /* insurance */ return new Property("insurance", "", 3748 "Financial instruments for reimbursement for the health care products and services.", 0, 3749 java.lang.Integer.MAX_VALUE, insurance); 3750 case 522246568: 3751 /* preAuthRef */ return new Property("preAuthRef", "string", 3752 "A reference from the Insurer to which these services pertain to be used on further communication and as proof that the request occurred.", 3753 0, 1, preAuthRef); 3754 case 3148996: 3755 /* form */ return new Property("form", "CodeableConcept", 3756 "A code for the form to be used for printing the content.", 0, 1, form); 3757 case 96784904: 3758 /* error */ return new Property("error", "", "Errors encountered during the processing of the request.", 0, 3759 java.lang.Integer.MAX_VALUE, error); 3760 default: 3761 return super.getNamedProperty(_hash, _name, _checkValid); 3762 } 3763 3764 } 3765 3766 @Override 3767 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3768 switch (hash) { 3769 case -1618432855: 3770 /* identifier */ return this.identifier == null ? new Base[0] 3771 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3772 case -892481550: 3773 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EligibilityResponseStatus> 3774 case -220463842: 3775 /* purpose */ return this.purpose == null ? new Base[0] : this.purpose.toArray(new Base[this.purpose.size()]); // Enumeration<EligibilityResponsePurpose> 3776 case -791418107: 3777 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 3778 case 1379209295: 3779 /* serviced */ return this.serviced == null ? new Base[0] : new Base[] { this.serviced }; // Type 3780 case 1028554472: 3781 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 3782 case 693934258: 3783 /* requestor */ return this.requestor == null ? new Base[0] : new Base[] { this.requestor }; // Reference 3784 case 1095692943: 3785 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 3786 case -1106507950: 3787 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // Enumeration<RemittanceOutcome> 3788 case 583380919: 3789 /* disposition */ return this.disposition == null ? new Base[0] : new Base[] { this.disposition }; // StringType 3790 case 1957615864: 3791 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 3792 case 73049818: 3793 /* insurance */ return this.insurance == null ? new Base[0] 3794 : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 3795 case 522246568: 3796 /* preAuthRef */ return this.preAuthRef == null ? new Base[0] : new Base[] { this.preAuthRef }; // StringType 3797 case 3148996: 3798 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // CodeableConcept 3799 case 96784904: 3800 /* error */ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorsComponent 3801 default: 3802 return super.getProperty(hash, name, checkValid); 3803 } 3804 3805 } 3806 3807 @Override 3808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3809 switch (hash) { 3810 case -1618432855: // identifier 3811 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3812 return value; 3813 case -892481550: // status 3814 value = new EligibilityResponseStatusEnumFactory().fromType(castToCode(value)); 3815 this.status = (Enumeration) value; // Enumeration<EligibilityResponseStatus> 3816 return value; 3817 case -220463842: // purpose 3818 value = new EligibilityResponsePurposeEnumFactory().fromType(castToCode(value)); 3819 this.getPurpose().add((Enumeration) value); // Enumeration<EligibilityResponsePurpose> 3820 return value; 3821 case -791418107: // patient 3822 this.patient = castToReference(value); // Reference 3823 return value; 3824 case 1379209295: // serviced 3825 this.serviced = castToType(value); // Type 3826 return value; 3827 case 1028554472: // created 3828 this.created = castToDateTime(value); // DateTimeType 3829 return value; 3830 case 693934258: // requestor 3831 this.requestor = castToReference(value); // Reference 3832 return value; 3833 case 1095692943: // request 3834 this.request = castToReference(value); // Reference 3835 return value; 3836 case -1106507950: // outcome 3837 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 3838 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 3839 return value; 3840 case 583380919: // disposition 3841 this.disposition = castToString(value); // StringType 3842 return value; 3843 case 1957615864: // insurer 3844 this.insurer = castToReference(value); // Reference 3845 return value; 3846 case 73049818: // insurance 3847 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 3848 return value; 3849 case 522246568: // preAuthRef 3850 this.preAuthRef = castToString(value); // StringType 3851 return value; 3852 case 3148996: // form 3853 this.form = castToCodeableConcept(value); // CodeableConcept 3854 return value; 3855 case 96784904: // error 3856 this.getError().add((ErrorsComponent) value); // ErrorsComponent 3857 return value; 3858 default: 3859 return super.setProperty(hash, name, value); 3860 } 3861 3862 } 3863 3864 @Override 3865 public Base setProperty(String name, Base value) throws FHIRException { 3866 if (name.equals("identifier")) { 3867 this.getIdentifier().add(castToIdentifier(value)); 3868 } else if (name.equals("status")) { 3869 value = new EligibilityResponseStatusEnumFactory().fromType(castToCode(value)); 3870 this.status = (Enumeration) value; // Enumeration<EligibilityResponseStatus> 3871 } else if (name.equals("purpose")) { 3872 value = new EligibilityResponsePurposeEnumFactory().fromType(castToCode(value)); 3873 this.getPurpose().add((Enumeration) value); 3874 } else if (name.equals("patient")) { 3875 this.patient = castToReference(value); // Reference 3876 } else if (name.equals("serviced[x]")) { 3877 this.serviced = castToType(value); // Type 3878 } else if (name.equals("created")) { 3879 this.created = castToDateTime(value); // DateTimeType 3880 } else if (name.equals("requestor")) { 3881 this.requestor = castToReference(value); // Reference 3882 } else if (name.equals("request")) { 3883 this.request = castToReference(value); // Reference 3884 } else if (name.equals("outcome")) { 3885 value = new RemittanceOutcomeEnumFactory().fromType(castToCode(value)); 3886 this.outcome = (Enumeration) value; // Enumeration<RemittanceOutcome> 3887 } else if (name.equals("disposition")) { 3888 this.disposition = castToString(value); // StringType 3889 } else if (name.equals("insurer")) { 3890 this.insurer = castToReference(value); // Reference 3891 } else if (name.equals("insurance")) { 3892 this.getInsurance().add((InsuranceComponent) value); 3893 } else if (name.equals("preAuthRef")) { 3894 this.preAuthRef = castToString(value); // StringType 3895 } else if (name.equals("form")) { 3896 this.form = castToCodeableConcept(value); // CodeableConcept 3897 } else if (name.equals("error")) { 3898 this.getError().add((ErrorsComponent) value); 3899 } else 3900 return super.setProperty(name, value); 3901 return value; 3902 } 3903 3904 @Override 3905 public void removeChild(String name, Base value) throws FHIRException { 3906 if (name.equals("identifier")) { 3907 this.getIdentifier().remove(castToIdentifier(value)); 3908 } else if (name.equals("status")) { 3909 this.status = null; 3910 } else if (name.equals("purpose")) { 3911 this.getPurpose().remove((Enumeration) value); 3912 } else if (name.equals("patient")) { 3913 this.patient = null; 3914 } else if (name.equals("serviced[x]")) { 3915 this.serviced = null; 3916 } else if (name.equals("created")) { 3917 this.created = null; 3918 } else if (name.equals("requestor")) { 3919 this.requestor = null; 3920 } else if (name.equals("request")) { 3921 this.request = null; 3922 } else if (name.equals("outcome")) { 3923 this.outcome = null; 3924 } else if (name.equals("disposition")) { 3925 this.disposition = null; 3926 } else if (name.equals("insurer")) { 3927 this.insurer = null; 3928 } else if (name.equals("insurance")) { 3929 this.getInsurance().remove((InsuranceComponent) value); 3930 } else if (name.equals("preAuthRef")) { 3931 this.preAuthRef = null; 3932 } else if (name.equals("form")) { 3933 this.form = null; 3934 } else if (name.equals("error")) { 3935 this.getError().remove((ErrorsComponent) value); 3936 } else 3937 super.removeChild(name, value); 3938 3939 } 3940 3941 @Override 3942 public Base makeProperty(int hash, String name) throws FHIRException { 3943 switch (hash) { 3944 case -1618432855: 3945 return addIdentifier(); 3946 case -892481550: 3947 return getStatusElement(); 3948 case -220463842: 3949 return addPurposeElement(); 3950 case -791418107: 3951 return getPatient(); 3952 case -1927922223: 3953 return getServiced(); 3954 case 1379209295: 3955 return getServiced(); 3956 case 1028554472: 3957 return getCreatedElement(); 3958 case 693934258: 3959 return getRequestor(); 3960 case 1095692943: 3961 return getRequest(); 3962 case -1106507950: 3963 return getOutcomeElement(); 3964 case 583380919: 3965 return getDispositionElement(); 3966 case 1957615864: 3967 return getInsurer(); 3968 case 73049818: 3969 return addInsurance(); 3970 case 522246568: 3971 return getPreAuthRefElement(); 3972 case 3148996: 3973 return getForm(); 3974 case 96784904: 3975 return addError(); 3976 default: 3977 return super.makeProperty(hash, name); 3978 } 3979 3980 } 3981 3982 @Override 3983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3984 switch (hash) { 3985 case -1618432855: 3986 /* identifier */ return new String[] { "Identifier" }; 3987 case -892481550: 3988 /* status */ return new String[] { "code" }; 3989 case -220463842: 3990 /* purpose */ return new String[] { "code" }; 3991 case -791418107: 3992 /* patient */ return new String[] { "Reference" }; 3993 case 1379209295: 3994 /* serviced */ return new String[] { "date", "Period" }; 3995 case 1028554472: 3996 /* created */ return new String[] { "dateTime" }; 3997 case 693934258: 3998 /* requestor */ return new String[] { "Reference" }; 3999 case 1095692943: 4000 /* request */ return new String[] { "Reference" }; 4001 case -1106507950: 4002 /* outcome */ return new String[] { "code" }; 4003 case 583380919: 4004 /* disposition */ return new String[] { "string" }; 4005 case 1957615864: 4006 /* insurer */ return new String[] { "Reference" }; 4007 case 73049818: 4008 /* insurance */ return new String[] {}; 4009 case 522246568: 4010 /* preAuthRef */ return new String[] { "string" }; 4011 case 3148996: 4012 /* form */ return new String[] { "CodeableConcept" }; 4013 case 96784904: 4014 /* error */ return new String[] {}; 4015 default: 4016 return super.getTypesForProperty(hash, name); 4017 } 4018 4019 } 4020 4021 @Override 4022 public Base addChild(String name) throws FHIRException { 4023 if (name.equals("identifier")) { 4024 return addIdentifier(); 4025 } else if (name.equals("status")) { 4026 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.status"); 4027 } else if (name.equals("purpose")) { 4028 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.purpose"); 4029 } else if (name.equals("patient")) { 4030 this.patient = new Reference(); 4031 return this.patient; 4032 } else if (name.equals("servicedDate")) { 4033 this.serviced = new DateType(); 4034 return this.serviced; 4035 } else if (name.equals("servicedPeriod")) { 4036 this.serviced = new Period(); 4037 return this.serviced; 4038 } else if (name.equals("created")) { 4039 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.created"); 4040 } else if (name.equals("requestor")) { 4041 this.requestor = new Reference(); 4042 return this.requestor; 4043 } else if (name.equals("request")) { 4044 this.request = new Reference(); 4045 return this.request; 4046 } else if (name.equals("outcome")) { 4047 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.outcome"); 4048 } else if (name.equals("disposition")) { 4049 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.disposition"); 4050 } else if (name.equals("insurer")) { 4051 this.insurer = new Reference(); 4052 return this.insurer; 4053 } else if (name.equals("insurance")) { 4054 return addInsurance(); 4055 } else if (name.equals("preAuthRef")) { 4056 throw new FHIRException("Cannot call addChild on a singleton property CoverageEligibilityResponse.preAuthRef"); 4057 } else if (name.equals("form")) { 4058 this.form = new CodeableConcept(); 4059 return this.form; 4060 } else if (name.equals("error")) { 4061 return addError(); 4062 } else 4063 return super.addChild(name); 4064 } 4065 4066 public String fhirType() { 4067 return "CoverageEligibilityResponse"; 4068 4069 } 4070 4071 public CoverageEligibilityResponse copy() { 4072 CoverageEligibilityResponse dst = new CoverageEligibilityResponse(); 4073 copyValues(dst); 4074 return dst; 4075 } 4076 4077 public void copyValues(CoverageEligibilityResponse dst) { 4078 super.copyValues(dst); 4079 if (identifier != null) { 4080 dst.identifier = new ArrayList<Identifier>(); 4081 for (Identifier i : identifier) 4082 dst.identifier.add(i.copy()); 4083 } 4084 ; 4085 dst.status = status == null ? null : status.copy(); 4086 if (purpose != null) { 4087 dst.purpose = new ArrayList<Enumeration<EligibilityResponsePurpose>>(); 4088 for (Enumeration<EligibilityResponsePurpose> i : purpose) 4089 dst.purpose.add(i.copy()); 4090 } 4091 ; 4092 dst.patient = patient == null ? null : patient.copy(); 4093 dst.serviced = serviced == null ? null : serviced.copy(); 4094 dst.created = created == null ? null : created.copy(); 4095 dst.requestor = requestor == null ? null : requestor.copy(); 4096 dst.request = request == null ? null : request.copy(); 4097 dst.outcome = outcome == null ? null : outcome.copy(); 4098 dst.disposition = disposition == null ? null : disposition.copy(); 4099 dst.insurer = insurer == null ? null : insurer.copy(); 4100 if (insurance != null) { 4101 dst.insurance = new ArrayList<InsuranceComponent>(); 4102 for (InsuranceComponent i : insurance) 4103 dst.insurance.add(i.copy()); 4104 } 4105 ; 4106 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 4107 dst.form = form == null ? null : form.copy(); 4108 if (error != null) { 4109 dst.error = new ArrayList<ErrorsComponent>(); 4110 for (ErrorsComponent i : error) 4111 dst.error.add(i.copy()); 4112 } 4113 ; 4114 } 4115 4116 protected CoverageEligibilityResponse typedCopy() { 4117 return copy(); 4118 } 4119 4120 @Override 4121 public boolean equalsDeep(Base other_) { 4122 if (!super.equalsDeep(other_)) 4123 return false; 4124 if (!(other_ instanceof CoverageEligibilityResponse)) 4125 return false; 4126 CoverageEligibilityResponse o = (CoverageEligibilityResponse) other_; 4127 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4128 && compareDeep(purpose, o.purpose, true) && compareDeep(patient, o.patient, true) 4129 && compareDeep(serviced, o.serviced, true) && compareDeep(created, o.created, true) 4130 && compareDeep(requestor, o.requestor, true) && compareDeep(request, o.request, true) 4131 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 4132 && compareDeep(insurer, o.insurer, true) && compareDeep(insurance, o.insurance, true) 4133 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(form, o.form, true) 4134 && compareDeep(error, o.error, true); 4135 } 4136 4137 @Override 4138 public boolean equalsShallow(Base other_) { 4139 if (!super.equalsShallow(other_)) 4140 return false; 4141 if (!(other_ instanceof CoverageEligibilityResponse)) 4142 return false; 4143 CoverageEligibilityResponse o = (CoverageEligibilityResponse) other_; 4144 return compareValues(status, o.status, true) && compareValues(purpose, o.purpose, true) 4145 && compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 4146 && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true); 4147 } 4148 4149 public boolean isEmpty() { 4150 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, purpose, patient, serviced, 4151 created, requestor, request, outcome, disposition, insurer, insurance, preAuthRef, form, error); 4152 } 4153 4154 @Override 4155 public ResourceType getResourceType() { 4156 return ResourceType.CoverageEligibilityResponse; 4157 } 4158 4159 /** 4160 * Search parameter: <b>identifier</b> 4161 * <p> 4162 * Description: <b>The business identifier</b><br> 4163 * Type: <b>token</b><br> 4164 * Path: <b>CoverageEligibilityResponse.identifier</b><br> 4165 * </p> 4166 */ 4167 @SearchParamDefinition(name = "identifier", path = "CoverageEligibilityResponse.identifier", description = "The business identifier", type = "token") 4168 public static final String SP_IDENTIFIER = "identifier"; 4169 /** 4170 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4171 * <p> 4172 * Description: <b>The business identifier</b><br> 4173 * Type: <b>token</b><br> 4174 * Path: <b>CoverageEligibilityResponse.identifier</b><br> 4175 * </p> 4176 */ 4177 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4178 SP_IDENTIFIER); 4179 4180 /** 4181 * Search parameter: <b>request</b> 4182 * <p> 4183 * Description: <b>The EligibilityRequest reference</b><br> 4184 * Type: <b>reference</b><br> 4185 * Path: <b>CoverageEligibilityResponse.request</b><br> 4186 * </p> 4187 */ 4188 @SearchParamDefinition(name = "request", path = "CoverageEligibilityResponse.request", description = "The EligibilityRequest reference", type = "reference", target = { 4189 CoverageEligibilityRequest.class }) 4190 public static final String SP_REQUEST = "request"; 4191 /** 4192 * <b>Fluent Client</b> search parameter constant for <b>request</b> 4193 * <p> 4194 * Description: <b>The EligibilityRequest reference</b><br> 4195 * Type: <b>reference</b><br> 4196 * Path: <b>CoverageEligibilityResponse.request</b><br> 4197 * </p> 4198 */ 4199 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4200 SP_REQUEST); 4201 4202 /** 4203 * Constant for fluent queries to be used to add include statements. Specifies 4204 * the path value of "<b>CoverageEligibilityResponse:request</b>". 4205 */ 4206 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 4207 "CoverageEligibilityResponse:request").toLocked(); 4208 4209 /** 4210 * Search parameter: <b>disposition</b> 4211 * <p> 4212 * Description: <b>The contents of the disposition message</b><br> 4213 * Type: <b>string</b><br> 4214 * Path: <b>CoverageEligibilityResponse.disposition</b><br> 4215 * </p> 4216 */ 4217 @SearchParamDefinition(name = "disposition", path = "CoverageEligibilityResponse.disposition", description = "The contents of the disposition message", type = "string") 4218 public static final String SP_DISPOSITION = "disposition"; 4219 /** 4220 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 4221 * <p> 4222 * Description: <b>The contents of the disposition message</b><br> 4223 * Type: <b>string</b><br> 4224 * Path: <b>CoverageEligibilityResponse.disposition</b><br> 4225 * </p> 4226 */ 4227 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4228 SP_DISPOSITION); 4229 4230 /** 4231 * Search parameter: <b>patient</b> 4232 * <p> 4233 * Description: <b>The reference to the patient</b><br> 4234 * Type: <b>reference</b><br> 4235 * Path: <b>CoverageEligibilityResponse.patient</b><br> 4236 * </p> 4237 */ 4238 @SearchParamDefinition(name = "patient", path = "CoverageEligibilityResponse.patient", description = "The reference to the patient", type = "reference", providesMembershipIn = { 4239 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4240 public static final String SP_PATIENT = "patient"; 4241 /** 4242 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4243 * <p> 4244 * Description: <b>The reference to the patient</b><br> 4245 * Type: <b>reference</b><br> 4246 * Path: <b>CoverageEligibilityResponse.patient</b><br> 4247 * </p> 4248 */ 4249 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4250 SP_PATIENT); 4251 4252 /** 4253 * Constant for fluent queries to be used to add include statements. Specifies 4254 * the path value of "<b>CoverageEligibilityResponse:patient</b>". 4255 */ 4256 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4257 "CoverageEligibilityResponse:patient").toLocked(); 4258 4259 /** 4260 * Search parameter: <b>insurer</b> 4261 * <p> 4262 * Description: <b>The organization which generated this resource</b><br> 4263 * Type: <b>reference</b><br> 4264 * Path: <b>CoverageEligibilityResponse.insurer</b><br> 4265 * </p> 4266 */ 4267 @SearchParamDefinition(name = "insurer", path = "CoverageEligibilityResponse.insurer", description = "The organization which generated this resource", type = "reference", target = { 4268 Organization.class }) 4269 public static final String SP_INSURER = "insurer"; 4270 /** 4271 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 4272 * <p> 4273 * Description: <b>The organization which generated this resource</b><br> 4274 * Type: <b>reference</b><br> 4275 * Path: <b>CoverageEligibilityResponse.insurer</b><br> 4276 * </p> 4277 */ 4278 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4279 SP_INSURER); 4280 4281 /** 4282 * Constant for fluent queries to be used to add include statements. Specifies 4283 * the path value of "<b>CoverageEligibilityResponse:insurer</b>". 4284 */ 4285 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include( 4286 "CoverageEligibilityResponse:insurer").toLocked(); 4287 4288 /** 4289 * Search parameter: <b>created</b> 4290 * <p> 4291 * Description: <b>The creation date</b><br> 4292 * Type: <b>date</b><br> 4293 * Path: <b>CoverageEligibilityResponse.created</b><br> 4294 * </p> 4295 */ 4296 @SearchParamDefinition(name = "created", path = "CoverageEligibilityResponse.created", description = "The creation date", type = "date") 4297 public static final String SP_CREATED = "created"; 4298 /** 4299 * <b>Fluent Client</b> search parameter constant for <b>created</b> 4300 * <p> 4301 * Description: <b>The creation date</b><br> 4302 * Type: <b>date</b><br> 4303 * Path: <b>CoverageEligibilityResponse.created</b><br> 4304 * </p> 4305 */ 4306 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 4307 SP_CREATED); 4308 4309 /** 4310 * Search parameter: <b>outcome</b> 4311 * <p> 4312 * Description: <b>The processing outcome</b><br> 4313 * Type: <b>token</b><br> 4314 * Path: <b>CoverageEligibilityResponse.outcome</b><br> 4315 * </p> 4316 */ 4317 @SearchParamDefinition(name = "outcome", path = "CoverageEligibilityResponse.outcome", description = "The processing outcome", type = "token") 4318 public static final String SP_OUTCOME = "outcome"; 4319 /** 4320 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 4321 * <p> 4322 * Description: <b>The processing outcome</b><br> 4323 * Type: <b>token</b><br> 4324 * Path: <b>CoverageEligibilityResponse.outcome</b><br> 4325 * </p> 4326 */ 4327 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4328 SP_OUTCOME); 4329 4330 /** 4331 * Search parameter: <b>requestor</b> 4332 * <p> 4333 * Description: <b>The EligibilityRequest provider</b><br> 4334 * Type: <b>reference</b><br> 4335 * Path: <b>CoverageEligibilityResponse.requestor</b><br> 4336 * </p> 4337 */ 4338 @SearchParamDefinition(name = "requestor", path = "CoverageEligibilityResponse.requestor", description = "The EligibilityRequest provider", type = "reference", providesMembershipIn = { 4339 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 4340 Practitioner.class, PractitionerRole.class }) 4341 public static final String SP_REQUESTOR = "requestor"; 4342 /** 4343 * <b>Fluent Client</b> search parameter constant for <b>requestor</b> 4344 * <p> 4345 * Description: <b>The EligibilityRequest provider</b><br> 4346 * Type: <b>reference</b><br> 4347 * Path: <b>CoverageEligibilityResponse.requestor</b><br> 4348 * </p> 4349 */ 4350 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4351 SP_REQUESTOR); 4352 4353 /** 4354 * Constant for fluent queries to be used to add include statements. Specifies 4355 * the path value of "<b>CoverageEligibilityResponse:requestor</b>". 4356 */ 4357 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTOR = new ca.uhn.fhir.model.api.Include( 4358 "CoverageEligibilityResponse:requestor").toLocked(); 4359 4360 /** 4361 * Search parameter: <b>status</b> 4362 * <p> 4363 * Description: <b>The EligibilityRequest status</b><br> 4364 * Type: <b>token</b><br> 4365 * Path: <b>CoverageEligibilityResponse.status</b><br> 4366 * </p> 4367 */ 4368 @SearchParamDefinition(name = "status", path = "CoverageEligibilityResponse.status", description = "The EligibilityRequest status", type = "token") 4369 public static final String SP_STATUS = "status"; 4370 /** 4371 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4372 * <p> 4373 * Description: <b>The EligibilityRequest status</b><br> 4374 * Type: <b>token</b><br> 4375 * Path: <b>CoverageEligibilityResponse.status</b><br> 4376 * </p> 4377 */ 4378 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4379 SP_STATUS); 4380 4381}