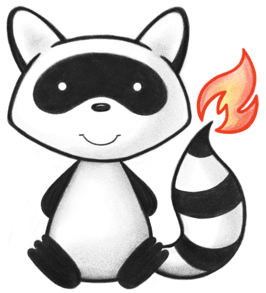
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045 046/** 047 * Describes a required data item for evaluation in terms of the type of data, 048 * and optional code or date-based filters of the data. 049 */ 050@DatatypeDef(name = "DataRequirement") 051public class DataRequirement extends Type implements ICompositeType { 052 053 public enum SortDirection { 054 /** 055 * Sort by the value ascending, so that lower values appear first. 056 */ 057 ASCENDING, 058 /** 059 * Sort by the value descending, so that lower values appear last. 060 */ 061 DESCENDING, 062 /** 063 * added to help the parsers with the generic types 064 */ 065 NULL; 066 067 public static SortDirection fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("ascending".equals(codeString)) 071 return ASCENDING; 072 if ("descending".equals(codeString)) 073 return DESCENDING; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown SortDirection code '" + codeString + "'"); 078 } 079 080 public String toCode() { 081 switch (this) { 082 case ASCENDING: 083 return "ascending"; 084 case DESCENDING: 085 return "descending"; 086 case NULL: 087 return null; 088 default: 089 return "?"; 090 } 091 } 092 093 public String getSystem() { 094 switch (this) { 095 case ASCENDING: 096 return "http://hl7.org/fhir/sort-direction"; 097 case DESCENDING: 098 return "http://hl7.org/fhir/sort-direction"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getDefinition() { 107 switch (this) { 108 case ASCENDING: 109 return "Sort by the value ascending, so that lower values appear first."; 110 case DESCENDING: 111 return "Sort by the value descending, so that lower values appear last."; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDisplay() { 120 switch (this) { 121 case ASCENDING: 122 return "Ascending"; 123 case DESCENDING: 124 return "Descending"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 } 132 133 public static class SortDirectionEnumFactory implements EnumFactory<SortDirection> { 134 public SortDirection fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("ascending".equals(codeString)) 139 return SortDirection.ASCENDING; 140 if ("descending".equals(codeString)) 141 return SortDirection.DESCENDING; 142 throw new IllegalArgumentException("Unknown SortDirection code '" + codeString + "'"); 143 } 144 145 public Enumeration<SortDirection> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<SortDirection>(this, SortDirection.NULL, code); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return new Enumeration<SortDirection>(this, SortDirection.NULL, code); 153 if ("ascending".equals(codeString)) 154 return new Enumeration<SortDirection>(this, SortDirection.ASCENDING, code); 155 if ("descending".equals(codeString)) 156 return new Enumeration<SortDirection>(this, SortDirection.DESCENDING, code); 157 throw new FHIRException("Unknown SortDirection code '" + codeString + "'"); 158 } 159 160 public String toCode(SortDirection code) { 161 if (code == SortDirection.NULL) 162 return null; 163 if (code == SortDirection.ASCENDING) 164 return "ascending"; 165 if (code == SortDirection.DESCENDING) 166 return "descending"; 167 return "?"; 168 } 169 170 public String toSystem(SortDirection code) { 171 return code.getSystem(); 172 } 173 } 174 175 @Block() 176 public static class DataRequirementCodeFilterComponent extends Element implements IBaseDatatypeElement { 177 /** 178 * The code-valued attribute of the filter. The specified path SHALL be a 179 * FHIRPath resolveable on the specified type of the DataRequirement, and SHALL 180 * consist only of identifiers, constant indexers, and .resolve(). The path is 181 * allowed to contain qualifiers (.) to traverse sub-elements, as well as 182 * indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple 183 * FHIRPath Profile](fhirpath.html#simple) for full details). Note that the 184 * index must be an integer constant. The path must resolve to an element of 185 * type code, Coding, or CodeableConcept. 186 */ 187 @Child(name = "path", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 188 @Description(shortDefinition = "A code-valued attribute to filter on", formalDefinition = "The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.") 189 protected StringType path; 190 191 /** 192 * A token parameter that refers to a search parameter defined on the specified 193 * type of the DataRequirement, and which searches on elements of type code, 194 * Coding, or CodeableConcept. 195 */ 196 @Child(name = "searchParam", type = { 197 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 198 @Description(shortDefinition = "A coded (token) parameter to search on", formalDefinition = "A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.") 199 protected StringType searchParam; 200 201 /** 202 * The valueset for the code filter. The valueSet and code elements are 203 * additive. If valueSet is specified, the filter will return only those data 204 * items for which the value of the code-valued element specified in the path is 205 * a member of the specified valueset. 206 */ 207 @Child(name = "valueSet", type = { 208 CanonicalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 209 @Description(shortDefinition = "Valueset for the filter", formalDefinition = "The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.") 210 protected CanonicalType valueSet; 211 212 /** 213 * The codes for the code filter. If values are given, the filter will return 214 * only those data items for which the code-valued attribute specified by the 215 * path has a value that is one of the specified codes. If codes are specified 216 * in addition to a value set, the filter returns items matching a code in the 217 * value set or one of the specified codes. 218 */ 219 @Child(name = "code", type = { 220 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 221 @Description(shortDefinition = "What code is expected", formalDefinition = "The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.") 222 protected List<Coding> code; 223 224 private static final long serialVersionUID = -1286212752L; 225 226 /** 227 * Constructor 228 */ 229 public DataRequirementCodeFilterComponent() { 230 super(); 231 } 232 233 /** 234 * @return {@link #path} (The code-valued attribute of the filter. The specified 235 * path SHALL be a FHIRPath resolveable on the specified type of the 236 * DataRequirement, and SHALL consist only of identifiers, constant 237 * indexers, and .resolve(). The path is allowed to contain qualifiers 238 * (.) to traverse sub-elements, as well as indexers ([x]) to traverse 239 * multiple-cardinality sub-elements (see the [Simple FHIRPath 240 * Profile](fhirpath.html#simple) for full details). Note that the index 241 * must be an integer constant. The path must resolve to an element of 242 * type code, Coding, or CodeableConcept.). This is the underlying 243 * object with id, value and extensions. The accessor "getPath" gives 244 * direct access to the value 245 */ 246 public StringType getPathElement() { 247 if (this.path == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.path"); 250 else if (Configuration.doAutoCreate()) 251 this.path = new StringType(); // bb 252 return this.path; 253 } 254 255 public boolean hasPathElement() { 256 return this.path != null && !this.path.isEmpty(); 257 } 258 259 public boolean hasPath() { 260 return this.path != null && !this.path.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #path} (The code-valued attribute of the filter. The 265 * specified path SHALL be a FHIRPath resolveable on the specified 266 * type of the DataRequirement, and SHALL consist only of 267 * identifiers, constant indexers, and .resolve(). The path is 268 * allowed to contain qualifiers (.) to traverse sub-elements, as 269 * well as indexers ([x]) to traverse multiple-cardinality 270 * sub-elements (see the [Simple FHIRPath 271 * Profile](fhirpath.html#simple) for full details). Note that the 272 * index must be an integer constant. The path must resolve to an 273 * element of type code, Coding, or CodeableConcept.). This is the 274 * underlying object with id, value and extensions. The accessor 275 * "getPath" gives direct access to the value 276 */ 277 public DataRequirementCodeFilterComponent setPathElement(StringType value) { 278 this.path = value; 279 return this; 280 } 281 282 /** 283 * @return The code-valued attribute of the filter. The specified path SHALL be 284 * a FHIRPath resolveable on the specified type of the DataRequirement, 285 * and SHALL consist only of identifiers, constant indexers, and 286 * .resolve(). The path is allowed to contain qualifiers (.) to traverse 287 * sub-elements, as well as indexers ([x]) to traverse 288 * multiple-cardinality sub-elements (see the [Simple FHIRPath 289 * Profile](fhirpath.html#simple) for full details). Note that the index 290 * must be an integer constant. The path must resolve to an element of 291 * type code, Coding, or CodeableConcept. 292 */ 293 public String getPath() { 294 return this.path == null ? null : this.path.getValue(); 295 } 296 297 /** 298 * @param value The code-valued attribute of the filter. The specified path 299 * SHALL be a FHIRPath resolveable on the specified type of the 300 * DataRequirement, and SHALL consist only of identifiers, constant 301 * indexers, and .resolve(). The path is allowed to contain 302 * qualifiers (.) to traverse sub-elements, as well as indexers 303 * ([x]) to traverse multiple-cardinality sub-elements (see the 304 * [Simple FHIRPath Profile](fhirpath.html#simple) for full 305 * details). Note that the index must be an integer constant. The 306 * path must resolve to an element of type code, Coding, or 307 * CodeableConcept. 308 */ 309 public DataRequirementCodeFilterComponent setPath(String value) { 310 if (Utilities.noString(value)) 311 this.path = null; 312 else { 313 if (this.path == null) 314 this.path = new StringType(); 315 this.path.setValue(value); 316 } 317 return this; 318 } 319 320 /** 321 * @return {@link #searchParam} (A token parameter that refers to a search 322 * parameter defined on the specified type of the DataRequirement, and 323 * which searches on elements of type code, Coding, or 324 * CodeableConcept.). This is the underlying object with id, value and 325 * extensions. The accessor "getSearchParam" gives direct access to the 326 * value 327 */ 328 public StringType getSearchParamElement() { 329 if (this.searchParam == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.searchParam"); 332 else if (Configuration.doAutoCreate()) 333 this.searchParam = new StringType(); // bb 334 return this.searchParam; 335 } 336 337 public boolean hasSearchParamElement() { 338 return this.searchParam != null && !this.searchParam.isEmpty(); 339 } 340 341 public boolean hasSearchParam() { 342 return this.searchParam != null && !this.searchParam.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #searchParam} (A token parameter that refers to a search 347 * parameter defined on the specified type of the DataRequirement, 348 * and which searches on elements of type code, Coding, or 349 * CodeableConcept.). This is the underlying object with id, value 350 * and extensions. The accessor "getSearchParam" gives direct 351 * access to the value 352 */ 353 public DataRequirementCodeFilterComponent setSearchParamElement(StringType value) { 354 this.searchParam = value; 355 return this; 356 } 357 358 /** 359 * @return A token parameter that refers to a search parameter defined on the 360 * specified type of the DataRequirement, and which searches on elements 361 * of type code, Coding, or CodeableConcept. 362 */ 363 public String getSearchParam() { 364 return this.searchParam == null ? null : this.searchParam.getValue(); 365 } 366 367 /** 368 * @param value A token parameter that refers to a search parameter defined on 369 * the specified type of the DataRequirement, and which searches on 370 * elements of type code, Coding, or CodeableConcept. 371 */ 372 public DataRequirementCodeFilterComponent setSearchParam(String value) { 373 if (Utilities.noString(value)) 374 this.searchParam = null; 375 else { 376 if (this.searchParam == null) 377 this.searchParam = new StringType(); 378 this.searchParam.setValue(value); 379 } 380 return this; 381 } 382 383 /** 384 * @return {@link #valueSet} (The valueset for the code filter. The valueSet and 385 * code elements are additive. If valueSet is specified, the filter will 386 * return only those data items for which the value of the code-valued 387 * element specified in the path is a member of the specified 388 * valueset.). This is the underlying object with id, value and 389 * extensions. The accessor "getValueSet" gives direct access to the 390 * value 391 */ 392 public CanonicalType getValueSetElement() { 393 if (this.valueSet == null) 394 if (Configuration.errorOnAutoCreate()) 395 throw new Error("Attempt to auto-create DataRequirementCodeFilterComponent.valueSet"); 396 else if (Configuration.doAutoCreate()) 397 this.valueSet = new CanonicalType(); // bb 398 return this.valueSet; 399 } 400 401 public boolean hasValueSetElement() { 402 return this.valueSet != null && !this.valueSet.isEmpty(); 403 } 404 405 public boolean hasValueSet() { 406 return this.valueSet != null && !this.valueSet.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #valueSet} (The valueset for the code filter. The 411 * valueSet and code elements are additive. If valueSet is 412 * specified, the filter will return only those data items for 413 * which the value of the code-valued element specified in the path 414 * is a member of the specified valueset.). This is the underlying 415 * object with id, value and extensions. The accessor "getValueSet" 416 * gives direct access to the value 417 */ 418 public DataRequirementCodeFilterComponent setValueSetElement(CanonicalType value) { 419 this.valueSet = value; 420 return this; 421 } 422 423 /** 424 * @return The valueset for the code filter. The valueSet and code elements are 425 * additive. If valueSet is specified, the filter will return only those 426 * data items for which the value of the code-valued element specified 427 * in the path is a member of the specified valueset. 428 */ 429 public String getValueSet() { 430 return this.valueSet == null ? null : this.valueSet.getValue(); 431 } 432 433 /** 434 * @param value The valueset for the code filter. The valueSet and code elements 435 * are additive. If valueSet is specified, the filter will return 436 * only those data items for which the value of the code-valued 437 * element specified in the path is a member of the specified 438 * valueset. 439 */ 440 public DataRequirementCodeFilterComponent setValueSet(String value) { 441 if (Utilities.noString(value)) 442 this.valueSet = null; 443 else { 444 if (this.valueSet == null) 445 this.valueSet = new CanonicalType(); 446 this.valueSet.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #code} (The codes for the code filter. If values are given, 453 * the filter will return only those data items for which the 454 * code-valued attribute specified by the path has a value that is one 455 * of the specified codes. If codes are specified in addition to a value 456 * set, the filter returns items matching a code in the value set or one 457 * of the specified codes.) 458 */ 459 public List<Coding> getCode() { 460 if (this.code == null) 461 this.code = new ArrayList<Coding>(); 462 return this.code; 463 } 464 465 /** 466 * @return Returns a reference to <code>this</code> for easy method chaining 467 */ 468 public DataRequirementCodeFilterComponent setCode(List<Coding> theCode) { 469 this.code = theCode; 470 return this; 471 } 472 473 public boolean hasCode() { 474 if (this.code == null) 475 return false; 476 for (Coding item : this.code) 477 if (!item.isEmpty()) 478 return true; 479 return false; 480 } 481 482 public Coding addCode() { // 3 483 Coding t = new Coding(); 484 if (this.code == null) 485 this.code = new ArrayList<Coding>(); 486 this.code.add(t); 487 return t; 488 } 489 490 public DataRequirementCodeFilterComponent addCode(Coding t) { // 3 491 if (t == null) 492 return this; 493 if (this.code == null) 494 this.code = new ArrayList<Coding>(); 495 this.code.add(t); 496 return this; 497 } 498 499 /** 500 * @return The first repetition of repeating field {@link #code}, creating it if 501 * it does not already exist 502 */ 503 public Coding getCodeFirstRep() { 504 if (getCode().isEmpty()) { 505 addCode(); 506 } 507 return getCode().get(0); 508 } 509 510 protected void listChildren(List<Property> children) { 511 super.listChildren(children); 512 children.add(new Property("path", "string", 513 "The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 514 0, 1, path)); 515 children.add(new Property("searchParam", "string", 516 "A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.", 517 0, 1, searchParam)); 518 children.add(new Property("valueSet", "canonical(ValueSet)", 519 "The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 520 0, 1, valueSet)); 521 children.add(new Property("code", "Coding", 522 "The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.", 523 0, java.lang.Integer.MAX_VALUE, code)); 524 } 525 526 @Override 527 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 528 switch (_hash) { 529 case 3433509: 530 /* path */ return new Property("path", "string", 531 "The code-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type code, Coding, or CodeableConcept.", 532 0, 1, path); 533 case -553645115: 534 /* searchParam */ return new Property("searchParam", "string", 535 "A token parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type code, Coding, or CodeableConcept.", 536 0, 1, searchParam); 537 case -1410174671: 538 /* valueSet */ return new Property("valueSet", "canonical(ValueSet)", 539 "The valueset for the code filter. The valueSet and code elements are additive. If valueSet is specified, the filter will return only those data items for which the value of the code-valued element specified in the path is a member of the specified valueset.", 540 0, 1, valueSet); 541 case 3059181: 542 /* code */ return new Property("code", "Coding", 543 "The codes for the code filter. If values are given, the filter will return only those data items for which the code-valued attribute specified by the path has a value that is one of the specified codes. If codes are specified in addition to a value set, the filter returns items matching a code in the value set or one of the specified codes.", 544 0, java.lang.Integer.MAX_VALUE, code); 545 default: 546 return super.getNamedProperty(_hash, _name, _checkValid); 547 } 548 549 } 550 551 @Override 552 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 553 switch (hash) { 554 case 3433509: 555 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 556 case -553645115: 557 /* searchParam */ return this.searchParam == null ? new Base[0] : new Base[] { this.searchParam }; // StringType 558 case -1410174671: 559 /* valueSet */ return this.valueSet == null ? new Base[0] : new Base[] { this.valueSet }; // CanonicalType 560 case 3059181: 561 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 562 default: 563 return super.getProperty(hash, name, checkValid); 564 } 565 566 } 567 568 @Override 569 public Base setProperty(int hash, String name, Base value) throws FHIRException { 570 switch (hash) { 571 case 3433509: // path 572 this.path = castToString(value); // StringType 573 return value; 574 case -553645115: // searchParam 575 this.searchParam = castToString(value); // StringType 576 return value; 577 case -1410174671: // valueSet 578 this.valueSet = castToCanonical(value); // CanonicalType 579 return value; 580 case 3059181: // code 581 this.getCode().add(castToCoding(value)); // Coding 582 return value; 583 default: 584 return super.setProperty(hash, name, value); 585 } 586 587 } 588 589 @Override 590 public Base setProperty(String name, Base value) throws FHIRException { 591 if (name.equals("path")) { 592 this.path = castToString(value); // StringType 593 } else if (name.equals("searchParam")) { 594 this.searchParam = castToString(value); // StringType 595 } else if (name.equals("valueSet")) { 596 this.valueSet = castToCanonical(value); // CanonicalType 597 } else if (name.equals("code")) { 598 this.getCode().add(castToCoding(value)); 599 } else 600 return super.setProperty(name, value); 601 return value; 602 } 603 604 @Override 605 public void removeChild(String name, Base value) throws FHIRException { 606 if (name.equals("path")) { 607 this.path = null; 608 } else if (name.equals("searchParam")) { 609 this.searchParam = null; 610 } else if (name.equals("valueSet")) { 611 this.valueSet = null; 612 } else if (name.equals("code")) { 613 this.getCode().remove(castToCoding(value)); 614 } else 615 super.removeChild(name, value); 616 617 } 618 619 @Override 620 public Base makeProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 3433509: 623 return getPathElement(); 624 case -553645115: 625 return getSearchParamElement(); 626 case -1410174671: 627 return getValueSetElement(); 628 case 3059181: 629 return addCode(); 630 default: 631 return super.makeProperty(hash, name); 632 } 633 634 } 635 636 @Override 637 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 638 switch (hash) { 639 case 3433509: 640 /* path */ return new String[] { "string" }; 641 case -553645115: 642 /* searchParam */ return new String[] { "string" }; 643 case -1410174671: 644 /* valueSet */ return new String[] { "canonical" }; 645 case 3059181: 646 /* code */ return new String[] { "Coding" }; 647 default: 648 return super.getTypesForProperty(hash, name); 649 } 650 651 } 652 653 @Override 654 public Base addChild(String name) throws FHIRException { 655 if (name.equals("path")) { 656 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.path"); 657 } else if (name.equals("searchParam")) { 658 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.searchParam"); 659 } else if (name.equals("valueSet")) { 660 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.valueSet"); 661 } else if (name.equals("code")) { 662 return addCode(); 663 } else 664 return super.addChild(name); 665 } 666 667 public DataRequirementCodeFilterComponent copy() { 668 DataRequirementCodeFilterComponent dst = new DataRequirementCodeFilterComponent(); 669 copyValues(dst); 670 return dst; 671 } 672 673 public void copyValues(DataRequirementCodeFilterComponent dst) { 674 super.copyValues(dst); 675 dst.path = path == null ? null : path.copy(); 676 dst.searchParam = searchParam == null ? null : searchParam.copy(); 677 dst.valueSet = valueSet == null ? null : valueSet.copy(); 678 if (code != null) { 679 dst.code = new ArrayList<Coding>(); 680 for (Coding i : code) 681 dst.code.add(i.copy()); 682 } 683 ; 684 } 685 686 @Override 687 public boolean equalsDeep(Base other_) { 688 if (!super.equalsDeep(other_)) 689 return false; 690 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 691 return false; 692 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 693 return compareDeep(path, o.path, true) && compareDeep(searchParam, o.searchParam, true) 694 && compareDeep(valueSet, o.valueSet, true) && compareDeep(code, o.code, true); 695 } 696 697 @Override 698 public boolean equalsShallow(Base other_) { 699 if (!super.equalsShallow(other_)) 700 return false; 701 if (!(other_ instanceof DataRequirementCodeFilterComponent)) 702 return false; 703 DataRequirementCodeFilterComponent o = (DataRequirementCodeFilterComponent) other_; 704 return compareValues(path, o.path, true) && compareValues(searchParam, o.searchParam, true); 705 } 706 707 public boolean isEmpty() { 708 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, searchParam, valueSet, code); 709 } 710 711 public String fhirType() { 712 return "DataRequirement.codeFilter"; 713 714 } 715 716 } 717 718 @Block() 719 public static class DataRequirementDateFilterComponent extends Element implements IBaseDatatypeElement { 720 /** 721 * The date-valued attribute of the filter. The specified path SHALL be a 722 * FHIRPath resolveable on the specified type of the DataRequirement, and SHALL 723 * consist only of identifiers, constant indexers, and .resolve(). The path is 724 * allowed to contain qualifiers (.) to traverse sub-elements, as well as 725 * indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple 726 * FHIRPath Profile](fhirpath.html#simple) for full details). Note that the 727 * index must be an integer constant. The path must resolve to an element of 728 * type date, dateTime, Period, Schedule, or Timing. 729 */ 730 @Child(name = "path", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 731 @Description(shortDefinition = "A date-valued attribute to filter on", formalDefinition = "The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.") 732 protected StringType path; 733 734 /** 735 * A date parameter that refers to a search parameter defined on the specified 736 * type of the DataRequirement, and which searches on elements of type date, 737 * dateTime, Period, Schedule, or Timing. 738 */ 739 @Child(name = "searchParam", type = { 740 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 741 @Description(shortDefinition = "A date valued parameter to search on", formalDefinition = "A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.") 742 protected StringType searchParam; 743 744 /** 745 * The value of the filter. If period is specified, the filter will return only 746 * those data items that fall within the bounds determined by the Period, 747 * inclusive of the period boundaries. If dateTime is specified, the filter will 748 * return only those data items that are equal to the specified dateTime. If a 749 * Duration is specified, the filter will return only those data items that fall 750 * within Duration before now. 751 */ 752 @Child(name = "value", type = { DateTimeType.class, Period.class, 753 Duration.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 754 @Description(shortDefinition = "The value of the filter, as a Period, DateTime, or Duration value", formalDefinition = "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.") 755 protected Type value; 756 757 private static final long serialVersionUID = 1151620053L; 758 759 /** 760 * Constructor 761 */ 762 public DataRequirementDateFilterComponent() { 763 super(); 764 } 765 766 /** 767 * @return {@link #path} (The date-valued attribute of the filter. The specified 768 * path SHALL be a FHIRPath resolveable on the specified type of the 769 * DataRequirement, and SHALL consist only of identifiers, constant 770 * indexers, and .resolve(). The path is allowed to contain qualifiers 771 * (.) to traverse sub-elements, as well as indexers ([x]) to traverse 772 * multiple-cardinality sub-elements (see the [Simple FHIRPath 773 * Profile](fhirpath.html#simple) for full details). Note that the index 774 * must be an integer constant. The path must resolve to an element of 775 * type date, dateTime, Period, Schedule, or Timing.). This is the 776 * underlying object with id, value and extensions. The accessor 777 * "getPath" gives direct access to the value 778 */ 779 public StringType getPathElement() { 780 if (this.path == null) 781 if (Configuration.errorOnAutoCreate()) 782 throw new Error("Attempt to auto-create DataRequirementDateFilterComponent.path"); 783 else if (Configuration.doAutoCreate()) 784 this.path = new StringType(); // bb 785 return this.path; 786 } 787 788 public boolean hasPathElement() { 789 return this.path != null && !this.path.isEmpty(); 790 } 791 792 public boolean hasPath() { 793 return this.path != null && !this.path.isEmpty(); 794 } 795 796 /** 797 * @param value {@link #path} (The date-valued attribute of the filter. The 798 * specified path SHALL be a FHIRPath resolveable on the specified 799 * type of the DataRequirement, and SHALL consist only of 800 * identifiers, constant indexers, and .resolve(). The path is 801 * allowed to contain qualifiers (.) to traverse sub-elements, as 802 * well as indexers ([x]) to traverse multiple-cardinality 803 * sub-elements (see the [Simple FHIRPath 804 * Profile](fhirpath.html#simple) for full details). Note that the 805 * index must be an integer constant. The path must resolve to an 806 * element of type date, dateTime, Period, Schedule, or Timing.). 807 * This is the underlying object with id, value and extensions. The 808 * accessor "getPath" gives direct access to the value 809 */ 810 public DataRequirementDateFilterComponent setPathElement(StringType value) { 811 this.path = value; 812 return this; 813 } 814 815 /** 816 * @return The date-valued attribute of the filter. The specified path SHALL be 817 * a FHIRPath resolveable on the specified type of the DataRequirement, 818 * and SHALL consist only of identifiers, constant indexers, and 819 * .resolve(). The path is allowed to contain qualifiers (.) to traverse 820 * sub-elements, as well as indexers ([x]) to traverse 821 * multiple-cardinality sub-elements (see the [Simple FHIRPath 822 * Profile](fhirpath.html#simple) for full details). Note that the index 823 * must be an integer constant. The path must resolve to an element of 824 * type date, dateTime, Period, Schedule, or Timing. 825 */ 826 public String getPath() { 827 return this.path == null ? null : this.path.getValue(); 828 } 829 830 /** 831 * @param value The date-valued attribute of the filter. The specified path 832 * SHALL be a FHIRPath resolveable on the specified type of the 833 * DataRequirement, and SHALL consist only of identifiers, constant 834 * indexers, and .resolve(). The path is allowed to contain 835 * qualifiers (.) to traverse sub-elements, as well as indexers 836 * ([x]) to traverse multiple-cardinality sub-elements (see the 837 * [Simple FHIRPath Profile](fhirpath.html#simple) for full 838 * details). Note that the index must be an integer constant. The 839 * path must resolve to an element of type date, dateTime, Period, 840 * Schedule, or Timing. 841 */ 842 public DataRequirementDateFilterComponent setPath(String value) { 843 if (Utilities.noString(value)) 844 this.path = null; 845 else { 846 if (this.path == null) 847 this.path = new StringType(); 848 this.path.setValue(value); 849 } 850 return this; 851 } 852 853 /** 854 * @return {@link #searchParam} (A date parameter that refers to a search 855 * parameter defined on the specified type of the DataRequirement, and 856 * which searches on elements of type date, dateTime, Period, Schedule, 857 * or Timing.). This is the underlying object with id, value and 858 * extensions. The accessor "getSearchParam" gives direct access to the 859 * value 860 */ 861 public StringType getSearchParamElement() { 862 if (this.searchParam == null) 863 if (Configuration.errorOnAutoCreate()) 864 throw new Error("Attempt to auto-create DataRequirementDateFilterComponent.searchParam"); 865 else if (Configuration.doAutoCreate()) 866 this.searchParam = new StringType(); // bb 867 return this.searchParam; 868 } 869 870 public boolean hasSearchParamElement() { 871 return this.searchParam != null && !this.searchParam.isEmpty(); 872 } 873 874 public boolean hasSearchParam() { 875 return this.searchParam != null && !this.searchParam.isEmpty(); 876 } 877 878 /** 879 * @param value {@link #searchParam} (A date parameter that refers to a search 880 * parameter defined on the specified type of the DataRequirement, 881 * and which searches on elements of type date, dateTime, Period, 882 * Schedule, or Timing.). This is the underlying object with id, 883 * value and extensions. The accessor "getSearchParam" gives direct 884 * access to the value 885 */ 886 public DataRequirementDateFilterComponent setSearchParamElement(StringType value) { 887 this.searchParam = value; 888 return this; 889 } 890 891 /** 892 * @return A date parameter that refers to a search parameter defined on the 893 * specified type of the DataRequirement, and which searches on elements 894 * of type date, dateTime, Period, Schedule, or Timing. 895 */ 896 public String getSearchParam() { 897 return this.searchParam == null ? null : this.searchParam.getValue(); 898 } 899 900 /** 901 * @param value A date parameter that refers to a search parameter defined on 902 * the specified type of the DataRequirement, and which searches on 903 * elements of type date, dateTime, Period, Schedule, or Timing. 904 */ 905 public DataRequirementDateFilterComponent setSearchParam(String value) { 906 if (Utilities.noString(value)) 907 this.searchParam = null; 908 else { 909 if (this.searchParam == null) 910 this.searchParam = new StringType(); 911 this.searchParam.setValue(value); 912 } 913 return this; 914 } 915 916 /** 917 * @return {@link #value} (The value of the filter. If period is specified, the 918 * filter will return only those data items that fall within the bounds 919 * determined by the Period, inclusive of the period boundaries. If 920 * dateTime is specified, the filter will return only those data items 921 * that are equal to the specified dateTime. If a Duration is specified, 922 * the filter will return only those data items that fall within 923 * Duration before now.) 924 */ 925 public Type getValue() { 926 return this.value; 927 } 928 929 /** 930 * @return {@link #value} (The value of the filter. If period is specified, the 931 * filter will return only those data items that fall within the bounds 932 * determined by the Period, inclusive of the period boundaries. If 933 * dateTime is specified, the filter will return only those data items 934 * that are equal to the specified dateTime. If a Duration is specified, 935 * the filter will return only those data items that fall within 936 * Duration before now.) 937 */ 938 public DateTimeType getValueDateTimeType() throws FHIRException { 939 if (this.value == null) 940 this.value = new DateTimeType(); 941 if (!(this.value instanceof DateTimeType)) 942 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 943 + this.value.getClass().getName() + " was encountered"); 944 return (DateTimeType) this.value; 945 } 946 947 public boolean hasValueDateTimeType() { 948 return this != null && this.value instanceof DateTimeType; 949 } 950 951 /** 952 * @return {@link #value} (The value of the filter. If period is specified, the 953 * filter will return only those data items that fall within the bounds 954 * determined by the Period, inclusive of the period boundaries. If 955 * dateTime is specified, the filter will return only those data items 956 * that are equal to the specified dateTime. If a Duration is specified, 957 * the filter will return only those data items that fall within 958 * Duration before now.) 959 */ 960 public Period getValuePeriod() throws FHIRException { 961 if (this.value == null) 962 this.value = new Period(); 963 if (!(this.value instanceof Period)) 964 throw new FHIRException( 965 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 966 return (Period) this.value; 967 } 968 969 public boolean hasValuePeriod() { 970 return this != null && this.value instanceof Period; 971 } 972 973 /** 974 * @return {@link #value} (The value of the filter. If period is specified, the 975 * filter will return only those data items that fall within the bounds 976 * determined by the Period, inclusive of the period boundaries. If 977 * dateTime is specified, the filter will return only those data items 978 * that are equal to the specified dateTime. If a Duration is specified, 979 * the filter will return only those data items that fall within 980 * Duration before now.) 981 */ 982 public Duration getValueDuration() throws FHIRException { 983 if (this.value == null) 984 this.value = new Duration(); 985 if (!(this.value instanceof Duration)) 986 throw new FHIRException("Type mismatch: the type Duration was expected, but " + this.value.getClass().getName() 987 + " was encountered"); 988 return (Duration) this.value; 989 } 990 991 public boolean hasValueDuration() { 992 return this != null && this.value instanceof Duration; 993 } 994 995 public boolean hasValue() { 996 return this.value != null && !this.value.isEmpty(); 997 } 998 999 /** 1000 * @param value {@link #value} (The value of the filter. If period is specified, 1001 * the filter will return only those data items that fall within 1002 * the bounds determined by the Period, inclusive of the period 1003 * boundaries. If dateTime is specified, the filter will return 1004 * only those data items that are equal to the specified dateTime. 1005 * If a Duration is specified, the filter will return only those 1006 * data items that fall within Duration before now.) 1007 */ 1008 public DataRequirementDateFilterComponent setValue(Type value) { 1009 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration)) 1010 throw new Error("Not the right type for DataRequirement.dateFilter.value[x]: " + value.fhirType()); 1011 this.value = value; 1012 return this; 1013 } 1014 1015 protected void listChildren(List<Property> children) { 1016 super.listChildren(children); 1017 children.add(new Property("path", "string", 1018 "The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.", 1019 0, 1, path)); 1020 children.add(new Property("searchParam", "string", 1021 "A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.", 1022 0, 1, searchParam)); 1023 children.add(new Property("value[x]", "dateTime|Period|Duration", 1024 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1025 0, 1, value)); 1026 } 1027 1028 @Override 1029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1030 switch (_hash) { 1031 case 3433509: 1032 /* path */ return new Property("path", "string", 1033 "The date-valued attribute of the filter. The specified path SHALL be a FHIRPath resolveable on the specified type of the DataRequirement, and SHALL consist only of identifiers, constant indexers, and .resolve(). The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details). Note that the index must be an integer constant. The path must resolve to an element of type date, dateTime, Period, Schedule, or Timing.", 1034 0, 1, path); 1035 case -553645115: 1036 /* searchParam */ return new Property("searchParam", "string", 1037 "A date parameter that refers to a search parameter defined on the specified type of the DataRequirement, and which searches on elements of type date, dateTime, Period, Schedule, or Timing.", 1038 0, 1, searchParam); 1039 case -1410166417: 1040 /* value[x] */ return new Property("value[x]", "dateTime|Period|Duration", 1041 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1042 0, 1, value); 1043 case 111972721: 1044 /* value */ return new Property("value[x]", "dateTime|Period|Duration", 1045 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1046 0, 1, value); 1047 case 1047929900: 1048 /* valueDateTime */ return new Property("value[x]", "dateTime|Period|Duration", 1049 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1050 0, 1, value); 1051 case -1524344174: 1052 /* valuePeriod */ return new Property("value[x]", "dateTime|Period|Duration", 1053 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1054 0, 1, value); 1055 case 1558135333: 1056 /* valueDuration */ return new Property("value[x]", "dateTime|Period|Duration", 1057 "The value of the filter. If period is specified, the filter will return only those data items that fall within the bounds determined by the Period, inclusive of the period boundaries. If dateTime is specified, the filter will return only those data items that are equal to the specified dateTime. If a Duration is specified, the filter will return only those data items that fall within Duration before now.", 1058 0, 1, value); 1059 default: 1060 return super.getNamedProperty(_hash, _name, _checkValid); 1061 } 1062 1063 } 1064 1065 @Override 1066 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1067 switch (hash) { 1068 case 3433509: 1069 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1070 case -553645115: 1071 /* searchParam */ return this.searchParam == null ? new Base[0] : new Base[] { this.searchParam }; // StringType 1072 case 111972721: 1073 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1074 default: 1075 return super.getProperty(hash, name, checkValid); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1082 switch (hash) { 1083 case 3433509: // path 1084 this.path = castToString(value); // StringType 1085 return value; 1086 case -553645115: // searchParam 1087 this.searchParam = castToString(value); // StringType 1088 return value; 1089 case 111972721: // value 1090 this.value = castToType(value); // Type 1091 return value; 1092 default: 1093 return super.setProperty(hash, name, value); 1094 } 1095 1096 } 1097 1098 @Override 1099 public Base setProperty(String name, Base value) throws FHIRException { 1100 if (name.equals("path")) { 1101 this.path = castToString(value); // StringType 1102 } else if (name.equals("searchParam")) { 1103 this.searchParam = castToString(value); // StringType 1104 } else if (name.equals("value[x]")) { 1105 this.value = castToType(value); // Type 1106 } else 1107 return super.setProperty(name, value); 1108 return value; 1109 } 1110 1111 @Override 1112 public void removeChild(String name, Base value) throws FHIRException { 1113 if (name.equals("path")) { 1114 this.path = null; 1115 } else if (name.equals("searchParam")) { 1116 this.searchParam = null; 1117 } else if (name.equals("value[x]")) { 1118 this.value = null; 1119 } else 1120 super.removeChild(name, value); 1121 1122 } 1123 1124 @Override 1125 public Base makeProperty(int hash, String name) throws FHIRException { 1126 switch (hash) { 1127 case 3433509: 1128 return getPathElement(); 1129 case -553645115: 1130 return getSearchParamElement(); 1131 case -1410166417: 1132 return getValue(); 1133 case 111972721: 1134 return getValue(); 1135 default: 1136 return super.makeProperty(hash, name); 1137 } 1138 1139 } 1140 1141 @Override 1142 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1143 switch (hash) { 1144 case 3433509: 1145 /* path */ return new String[] { "string" }; 1146 case -553645115: 1147 /* searchParam */ return new String[] { "string" }; 1148 case 111972721: 1149 /* value */ return new String[] { "dateTime", "Period", "Duration" }; 1150 default: 1151 return super.getTypesForProperty(hash, name); 1152 } 1153 1154 } 1155 1156 @Override 1157 public Base addChild(String name) throws FHIRException { 1158 if (name.equals("path")) { 1159 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.path"); 1160 } else if (name.equals("searchParam")) { 1161 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.searchParam"); 1162 } else if (name.equals("valueDateTime")) { 1163 this.value = new DateTimeType(); 1164 return this.value; 1165 } else if (name.equals("valuePeriod")) { 1166 this.value = new Period(); 1167 return this.value; 1168 } else if (name.equals("valueDuration")) { 1169 this.value = new Duration(); 1170 return this.value; 1171 } else 1172 return super.addChild(name); 1173 } 1174 1175 public DataRequirementDateFilterComponent copy() { 1176 DataRequirementDateFilterComponent dst = new DataRequirementDateFilterComponent(); 1177 copyValues(dst); 1178 return dst; 1179 } 1180 1181 public void copyValues(DataRequirementDateFilterComponent dst) { 1182 super.copyValues(dst); 1183 dst.path = path == null ? null : path.copy(); 1184 dst.searchParam = searchParam == null ? null : searchParam.copy(); 1185 dst.value = value == null ? null : value.copy(); 1186 } 1187 1188 @Override 1189 public boolean equalsDeep(Base other_) { 1190 if (!super.equalsDeep(other_)) 1191 return false; 1192 if (!(other_ instanceof DataRequirementDateFilterComponent)) 1193 return false; 1194 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 1195 return compareDeep(path, o.path, true) && compareDeep(searchParam, o.searchParam, true) 1196 && compareDeep(value, o.value, true); 1197 } 1198 1199 @Override 1200 public boolean equalsShallow(Base other_) { 1201 if (!super.equalsShallow(other_)) 1202 return false; 1203 if (!(other_ instanceof DataRequirementDateFilterComponent)) 1204 return false; 1205 DataRequirementDateFilterComponent o = (DataRequirementDateFilterComponent) other_; 1206 return compareValues(path, o.path, true) && compareValues(searchParam, o.searchParam, true); 1207 } 1208 1209 public boolean isEmpty() { 1210 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, searchParam, value); 1211 } 1212 1213 public String fhirType() { 1214 return "DataRequirement.dateFilter"; 1215 1216 } 1217 1218 } 1219 1220 @Block() 1221 public static class DataRequirementSortComponent extends Element implements IBaseDatatypeElement { 1222 /** 1223 * The attribute of the sort. The specified path must be resolvable from the 1224 * type of the required data. The path is allowed to contain qualifiers (.) to 1225 * traverse sub-elements, as well as indexers ([x]) to traverse 1226 * multiple-cardinality sub-elements. Note that the index must be an integer 1227 * constant. 1228 */ 1229 @Child(name = "path", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1230 @Description(shortDefinition = "The name of the attribute to perform the sort", formalDefinition = "The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.") 1231 protected StringType path; 1232 1233 /** 1234 * The direction of the sort, ascending or descending. 1235 */ 1236 @Child(name = "direction", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1237 @Description(shortDefinition = "ascending | descending", formalDefinition = "The direction of the sort, ascending or descending.") 1238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sort-direction") 1239 protected Enumeration<SortDirection> direction; 1240 1241 private static final long serialVersionUID = -694498683L; 1242 1243 /** 1244 * Constructor 1245 */ 1246 public DataRequirementSortComponent() { 1247 super(); 1248 } 1249 1250 /** 1251 * Constructor 1252 */ 1253 public DataRequirementSortComponent(StringType path, Enumeration<SortDirection> direction) { 1254 super(); 1255 this.path = path; 1256 this.direction = direction; 1257 } 1258 1259 /** 1260 * @return {@link #path} (The attribute of the sort. The specified path must be 1261 * resolvable from the type of the required data. The path is allowed to 1262 * contain qualifiers (.) to traverse sub-elements, as well as indexers 1263 * ([x]) to traverse multiple-cardinality sub-elements. Note that the 1264 * index must be an integer constant.). This is the underlying object 1265 * with id, value and extensions. The accessor "getPath" gives direct 1266 * access to the value 1267 */ 1268 public StringType getPathElement() { 1269 if (this.path == null) 1270 if (Configuration.errorOnAutoCreate()) 1271 throw new Error("Attempt to auto-create DataRequirementSortComponent.path"); 1272 else if (Configuration.doAutoCreate()) 1273 this.path = new StringType(); // bb 1274 return this.path; 1275 } 1276 1277 public boolean hasPathElement() { 1278 return this.path != null && !this.path.isEmpty(); 1279 } 1280 1281 public boolean hasPath() { 1282 return this.path != null && !this.path.isEmpty(); 1283 } 1284 1285 /** 1286 * @param value {@link #path} (The attribute of the sort. The specified path 1287 * must be resolvable from the type of the required data. The path 1288 * is allowed to contain qualifiers (.) to traverse sub-elements, 1289 * as well as indexers ([x]) to traverse multiple-cardinality 1290 * sub-elements. Note that the index must be an integer constant.). 1291 * This is the underlying object with id, value and extensions. The 1292 * accessor "getPath" gives direct access to the value 1293 */ 1294 public DataRequirementSortComponent setPathElement(StringType value) { 1295 this.path = value; 1296 return this; 1297 } 1298 1299 /** 1300 * @return The attribute of the sort. The specified path must be resolvable from 1301 * the type of the required data. The path is allowed to contain 1302 * qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to 1303 * traverse multiple-cardinality sub-elements. Note that the index must 1304 * be an integer constant. 1305 */ 1306 public String getPath() { 1307 return this.path == null ? null : this.path.getValue(); 1308 } 1309 1310 /** 1311 * @param value The attribute of the sort. The specified path must be resolvable 1312 * from the type of the required data. The path is allowed to 1313 * contain qualifiers (.) to traverse sub-elements, as well as 1314 * indexers ([x]) to traverse multiple-cardinality sub-elements. 1315 * Note that the index must be an integer constant. 1316 */ 1317 public DataRequirementSortComponent setPath(String value) { 1318 if (this.path == null) 1319 this.path = new StringType(); 1320 this.path.setValue(value); 1321 return this; 1322 } 1323 1324 /** 1325 * @return {@link #direction} (The direction of the sort, ascending or 1326 * descending.). This is the underlying object with id, value and 1327 * extensions. The accessor "getDirection" gives direct access to the 1328 * value 1329 */ 1330 public Enumeration<SortDirection> getDirectionElement() { 1331 if (this.direction == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create DataRequirementSortComponent.direction"); 1334 else if (Configuration.doAutoCreate()) 1335 this.direction = new Enumeration<SortDirection>(new SortDirectionEnumFactory()); // bb 1336 return this.direction; 1337 } 1338 1339 public boolean hasDirectionElement() { 1340 return this.direction != null && !this.direction.isEmpty(); 1341 } 1342 1343 public boolean hasDirection() { 1344 return this.direction != null && !this.direction.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #direction} (The direction of the sort, ascending or 1349 * descending.). This is the underlying object with id, value and 1350 * extensions. The accessor "getDirection" gives direct access to 1351 * the value 1352 */ 1353 public DataRequirementSortComponent setDirectionElement(Enumeration<SortDirection> value) { 1354 this.direction = value; 1355 return this; 1356 } 1357 1358 /** 1359 * @return The direction of the sort, ascending or descending. 1360 */ 1361 public SortDirection getDirection() { 1362 return this.direction == null ? null : this.direction.getValue(); 1363 } 1364 1365 /** 1366 * @param value The direction of the sort, ascending or descending. 1367 */ 1368 public DataRequirementSortComponent setDirection(SortDirection value) { 1369 if (this.direction == null) 1370 this.direction = new Enumeration<SortDirection>(new SortDirectionEnumFactory()); 1371 this.direction.setValue(value); 1372 return this; 1373 } 1374 1375 protected void listChildren(List<Property> children) { 1376 super.listChildren(children); 1377 children.add(new Property("path", "string", 1378 "The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.", 1379 0, 1, path)); 1380 children.add( 1381 new Property("direction", "code", "The direction of the sort, ascending or descending.", 0, 1, direction)); 1382 } 1383 1384 @Override 1385 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1386 switch (_hash) { 1387 case 3433509: 1388 /* path */ return new Property("path", "string", 1389 "The attribute of the sort. The specified path must be resolvable from the type of the required data. The path is allowed to contain qualifiers (.) to traverse sub-elements, as well as indexers ([x]) to traverse multiple-cardinality sub-elements. Note that the index must be an integer constant.", 1390 0, 1, path); 1391 case -962590849: 1392 /* direction */ return new Property("direction", "code", "The direction of the sort, ascending or descending.", 1393 0, 1, direction); 1394 default: 1395 return super.getNamedProperty(_hash, _name, _checkValid); 1396 } 1397 1398 } 1399 1400 @Override 1401 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1402 switch (hash) { 1403 case 3433509: 1404 /* path */ return this.path == null ? new Base[0] : new Base[] { this.path }; // StringType 1405 case -962590849: 1406 /* direction */ return this.direction == null ? new Base[0] : new Base[] { this.direction }; // Enumeration<SortDirection> 1407 default: 1408 return super.getProperty(hash, name, checkValid); 1409 } 1410 1411 } 1412 1413 @Override 1414 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1415 switch (hash) { 1416 case 3433509: // path 1417 this.path = castToString(value); // StringType 1418 return value; 1419 case -962590849: // direction 1420 value = new SortDirectionEnumFactory().fromType(castToCode(value)); 1421 this.direction = (Enumeration) value; // Enumeration<SortDirection> 1422 return value; 1423 default: 1424 return super.setProperty(hash, name, value); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base setProperty(String name, Base value) throws FHIRException { 1431 if (name.equals("path")) { 1432 this.path = castToString(value); // StringType 1433 } else if (name.equals("direction")) { 1434 value = new SortDirectionEnumFactory().fromType(castToCode(value)); 1435 this.direction = (Enumeration) value; // Enumeration<SortDirection> 1436 } else 1437 return super.setProperty(name, value); 1438 return value; 1439 } 1440 1441 @Override 1442 public void removeChild(String name, Base value) throws FHIRException { 1443 if (name.equals("path")) { 1444 this.path = null; 1445 } else if (name.equals("direction")) { 1446 this.direction = null; 1447 } else 1448 super.removeChild(name, value); 1449 1450 } 1451 1452 @Override 1453 public Base makeProperty(int hash, String name) throws FHIRException { 1454 switch (hash) { 1455 case 3433509: 1456 return getPathElement(); 1457 case -962590849: 1458 return getDirectionElement(); 1459 default: 1460 return super.makeProperty(hash, name); 1461 } 1462 1463 } 1464 1465 @Override 1466 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1467 switch (hash) { 1468 case 3433509: 1469 /* path */ return new String[] { "string" }; 1470 case -962590849: 1471 /* direction */ return new String[] { "code" }; 1472 default: 1473 return super.getTypesForProperty(hash, name); 1474 } 1475 1476 } 1477 1478 @Override 1479 public Base addChild(String name) throws FHIRException { 1480 if (name.equals("path")) { 1481 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.path"); 1482 } else if (name.equals("direction")) { 1483 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.direction"); 1484 } else 1485 return super.addChild(name); 1486 } 1487 1488 public DataRequirementSortComponent copy() { 1489 DataRequirementSortComponent dst = new DataRequirementSortComponent(); 1490 copyValues(dst); 1491 return dst; 1492 } 1493 1494 public void copyValues(DataRequirementSortComponent dst) { 1495 super.copyValues(dst); 1496 dst.path = path == null ? null : path.copy(); 1497 dst.direction = direction == null ? null : direction.copy(); 1498 } 1499 1500 @Override 1501 public boolean equalsDeep(Base other_) { 1502 if (!super.equalsDeep(other_)) 1503 return false; 1504 if (!(other_ instanceof DataRequirementSortComponent)) 1505 return false; 1506 DataRequirementSortComponent o = (DataRequirementSortComponent) other_; 1507 return compareDeep(path, o.path, true) && compareDeep(direction, o.direction, true); 1508 } 1509 1510 @Override 1511 public boolean equalsShallow(Base other_) { 1512 if (!super.equalsShallow(other_)) 1513 return false; 1514 if (!(other_ instanceof DataRequirementSortComponent)) 1515 return false; 1516 DataRequirementSortComponent o = (DataRequirementSortComponent) other_; 1517 return compareValues(path, o.path, true) && compareValues(direction, o.direction, true); 1518 } 1519 1520 public boolean isEmpty() { 1521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, direction); 1522 } 1523 1524 public String fhirType() { 1525 return "DataRequirement.sort"; 1526 1527 } 1528 1529 } 1530 1531 /** 1532 * The type of the required data, specified as the type name of a resource. For 1533 * profiles, this value is set to the type of the base resource of the profile. 1534 */ 1535 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 1536 @Description(shortDefinition = "The type of the required data", formalDefinition = "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.") 1537 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/all-types") 1538 protected CodeType type; 1539 1540 /** 1541 * The profile of the required data, specified as the uri of the profile 1542 * definition. 1543 */ 1544 @Child(name = "profile", type = { 1545 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1546 @Description(shortDefinition = "The profile of the required data", formalDefinition = "The profile of the required data, specified as the uri of the profile definition.") 1547 protected List<CanonicalType> profile; 1548 1549 /** 1550 * The intended subjects of the data requirement. If this element is not 1551 * provided, a Patient subject is assumed. 1552 */ 1553 @Child(name = "subject", type = { CodeableConcept.class, 1554 Group.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1555 @Description(shortDefinition = "E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition = "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.") 1556 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/subject-type") 1557 protected Type subject; 1558 1559 /** 1560 * Indicates that specific elements of the type are referenced by the knowledge 1561 * module and must be supported by the consumer in order to obtain an effective 1562 * evaluation. This does not mean that a value is required for this element, 1563 * only that the consuming system must understand the element and be able to 1564 * provide values for it if they are available. 1565 * 1566 * The value of mustSupport SHALL be a FHIRPath resolveable on the type of the 1567 * DataRequirement. The path SHALL consist only of identifiers, constant 1568 * indexers, and .resolve() (see the [Simple FHIRPath 1569 * Profile](fhirpath.html#simple) for full details). 1570 */ 1571 @Child(name = "mustSupport", type = { 1572 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1573 @Description(shortDefinition = "Indicates specific structure elements that are referenced by the knowledge module", formalDefinition = "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolveable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).") 1574 protected List<StringType> mustSupport; 1575 1576 /** 1577 * Code filters specify additional constraints on the data, specifying the value 1578 * set of interest for a particular element of the data. Each code filter 1579 * defines an additional constraint on the data, i.e. code filters are AND'ed, 1580 * not OR'ed. 1581 */ 1582 @Child(name = "codeFilter", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1583 @Description(shortDefinition = "What codes are expected", formalDefinition = "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.") 1584 protected List<DataRequirementCodeFilterComponent> codeFilter; 1585 1586 /** 1587 * Date filters specify additional constraints on the data in terms of the 1588 * applicable date range for specific elements. Each date filter specifies an 1589 * additional constraint on the data, i.e. date filters are AND'ed, not OR'ed. 1590 */ 1591 @Child(name = "dateFilter", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1592 @Description(shortDefinition = "What dates/date ranges are expected", formalDefinition = "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.") 1593 protected List<DataRequirementDateFilterComponent> dateFilter; 1594 1595 /** 1596 * Specifies a maximum number of results that are required (uses the _count 1597 * search parameter). 1598 */ 1599 @Child(name = "limit", type = { 1600 PositiveIntType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1601 @Description(shortDefinition = "Number of results", formalDefinition = "Specifies a maximum number of results that are required (uses the _count search parameter).") 1602 protected PositiveIntType limit; 1603 1604 /** 1605 * Specifies the order of the results to be returned. 1606 */ 1607 @Child(name = "sort", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1608 @Description(shortDefinition = "Order of the results", formalDefinition = "Specifies the order of the results to be returned.") 1609 protected List<DataRequirementSortComponent> sort; 1610 1611 private static final long serialVersionUID = 74042278L; 1612 1613 /** 1614 * Constructor 1615 */ 1616 public DataRequirement() { 1617 super(); 1618 } 1619 1620 /** 1621 * Constructor 1622 */ 1623 public DataRequirement(CodeType type) { 1624 super(); 1625 this.type = type; 1626 } 1627 1628 /** 1629 * @return {@link #type} (The type of the required data, specified as the type 1630 * name of a resource. For profiles, this value is set to the type of 1631 * the base resource of the profile.). This is the underlying object 1632 * with id, value and extensions. The accessor "getType" gives direct 1633 * access to the value 1634 */ 1635 public CodeType getTypeElement() { 1636 if (this.type == null) 1637 if (Configuration.errorOnAutoCreate()) 1638 throw new Error("Attempt to auto-create DataRequirement.type"); 1639 else if (Configuration.doAutoCreate()) 1640 this.type = new CodeType(); // bb 1641 return this.type; 1642 } 1643 1644 public boolean hasTypeElement() { 1645 return this.type != null && !this.type.isEmpty(); 1646 } 1647 1648 public boolean hasType() { 1649 return this.type != null && !this.type.isEmpty(); 1650 } 1651 1652 /** 1653 * @param value {@link #type} (The type of the required data, specified as the 1654 * type name of a resource. For profiles, this value is set to the 1655 * type of the base resource of the profile.). This is the 1656 * underlying object with id, value and extensions. The accessor 1657 * "getType" gives direct access to the value 1658 */ 1659 public DataRequirement setTypeElement(CodeType value) { 1660 this.type = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return The type of the required data, specified as the type name of a 1666 * resource. For profiles, this value is set to the type of the base 1667 * resource of the profile. 1668 */ 1669 public String getType() { 1670 return this.type == null ? null : this.type.getValue(); 1671 } 1672 1673 /** 1674 * @param value The type of the required data, specified as the type name of a 1675 * resource. For profiles, this value is set to the type of the 1676 * base resource of the profile. 1677 */ 1678 public DataRequirement setType(String value) { 1679 if (this.type == null) 1680 this.type = new CodeType(); 1681 this.type.setValue(value); 1682 return this; 1683 } 1684 1685 /** 1686 * @return {@link #profile} (The profile of the required data, specified as the 1687 * uri of the profile definition.) 1688 */ 1689 public List<CanonicalType> getProfile() { 1690 if (this.profile == null) 1691 this.profile = new ArrayList<CanonicalType>(); 1692 return this.profile; 1693 } 1694 1695 /** 1696 * @return Returns a reference to <code>this</code> for easy method chaining 1697 */ 1698 public DataRequirement setProfile(List<CanonicalType> theProfile) { 1699 this.profile = theProfile; 1700 return this; 1701 } 1702 1703 public boolean hasProfile() { 1704 if (this.profile == null) 1705 return false; 1706 for (CanonicalType item : this.profile) 1707 if (!item.isEmpty()) 1708 return true; 1709 return false; 1710 } 1711 1712 /** 1713 * @return {@link #profile} (The profile of the required data, specified as the 1714 * uri of the profile definition.) 1715 */ 1716 public CanonicalType addProfileElement() {// 2 1717 CanonicalType t = new CanonicalType(); 1718 if (this.profile == null) 1719 this.profile = new ArrayList<CanonicalType>(); 1720 this.profile.add(t); 1721 return t; 1722 } 1723 1724 /** 1725 * @param value {@link #profile} (The profile of the required data, specified as 1726 * the uri of the profile definition.) 1727 */ 1728 public DataRequirement addProfile(String value) { // 1 1729 CanonicalType t = new CanonicalType(); 1730 t.setValue(value); 1731 if (this.profile == null) 1732 this.profile = new ArrayList<CanonicalType>(); 1733 this.profile.add(t); 1734 return this; 1735 } 1736 1737 /** 1738 * @param value {@link #profile} (The profile of the required data, specified as 1739 * the uri of the profile definition.) 1740 */ 1741 public boolean hasProfile(String value) { 1742 if (this.profile == null) 1743 return false; 1744 for (CanonicalType v : this.profile) 1745 if (v.getValue().equals(value)) // canonical(StructureDefinition) 1746 return true; 1747 return false; 1748 } 1749 1750 /** 1751 * @return {@link #subject} (The intended subjects of the data requirement. If 1752 * this element is not provided, a Patient subject is assumed.) 1753 */ 1754 public Type getSubject() { 1755 return this.subject; 1756 } 1757 1758 /** 1759 * @return {@link #subject} (The intended subjects of the data requirement. If 1760 * this element is not provided, a Patient subject is assumed.) 1761 */ 1762 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 1763 if (this.subject == null) 1764 this.subject = new CodeableConcept(); 1765 if (!(this.subject instanceof CodeableConcept)) 1766 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1767 + this.subject.getClass().getName() + " was encountered"); 1768 return (CodeableConcept) this.subject; 1769 } 1770 1771 public boolean hasSubjectCodeableConcept() { 1772 return this != null && this.subject instanceof CodeableConcept; 1773 } 1774 1775 /** 1776 * @return {@link #subject} (The intended subjects of the data requirement. If 1777 * this element is not provided, a Patient subject is assumed.) 1778 */ 1779 public Reference getSubjectReference() throws FHIRException { 1780 if (this.subject == null) 1781 this.subject = new Reference(); 1782 if (!(this.subject instanceof Reference)) 1783 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.subject.getClass().getName() 1784 + " was encountered"); 1785 return (Reference) this.subject; 1786 } 1787 1788 public boolean hasSubjectReference() { 1789 return this != null && this.subject instanceof Reference; 1790 } 1791 1792 public boolean hasSubject() { 1793 return this.subject != null && !this.subject.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #subject} (The intended subjects of the data requirement. 1798 * If this element is not provided, a Patient subject is assumed.) 1799 */ 1800 public DataRequirement setSubject(Type value) { 1801 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1802 throw new Error("Not the right type for DataRequirement.subject[x]: " + value.fhirType()); 1803 this.subject = value; 1804 return this; 1805 } 1806 1807 /** 1808 * @return {@link #mustSupport} (Indicates that specific elements of the type 1809 * are referenced by the knowledge module and must be supported by the 1810 * consumer in order to obtain an effective evaluation. This does not 1811 * mean that a value is required for this element, only that the 1812 * consuming system must understand the element and be able to provide 1813 * values for it if they are available. 1814 * 1815 * The value of mustSupport SHALL be a FHIRPath resolveable on the type 1816 * of the DataRequirement. The path SHALL consist only of identifiers, 1817 * constant indexers, and .resolve() (see the [Simple FHIRPath 1818 * Profile](fhirpath.html#simple) for full details).) 1819 */ 1820 public List<StringType> getMustSupport() { 1821 if (this.mustSupport == null) 1822 this.mustSupport = new ArrayList<StringType>(); 1823 return this.mustSupport; 1824 } 1825 1826 /** 1827 * @return Returns a reference to <code>this</code> for easy method chaining 1828 */ 1829 public DataRequirement setMustSupport(List<StringType> theMustSupport) { 1830 this.mustSupport = theMustSupport; 1831 return this; 1832 } 1833 1834 public boolean hasMustSupport() { 1835 if (this.mustSupport == null) 1836 return false; 1837 for (StringType item : this.mustSupport) 1838 if (!item.isEmpty()) 1839 return true; 1840 return false; 1841 } 1842 1843 /** 1844 * @return {@link #mustSupport} (Indicates that specific elements of the type 1845 * are referenced by the knowledge module and must be supported by the 1846 * consumer in order to obtain an effective evaluation. This does not 1847 * mean that a value is required for this element, only that the 1848 * consuming system must understand the element and be able to provide 1849 * values for it if they are available. 1850 * 1851 * The value of mustSupport SHALL be a FHIRPath resolveable on the type 1852 * of the DataRequirement. The path SHALL consist only of identifiers, 1853 * constant indexers, and .resolve() (see the [Simple FHIRPath 1854 * Profile](fhirpath.html#simple) for full details).) 1855 */ 1856 public StringType addMustSupportElement() {// 2 1857 StringType t = new StringType(); 1858 if (this.mustSupport == null) 1859 this.mustSupport = new ArrayList<StringType>(); 1860 this.mustSupport.add(t); 1861 return t; 1862 } 1863 1864 /** 1865 * @param value {@link #mustSupport} (Indicates that specific elements of the 1866 * type are referenced by the knowledge module and must be 1867 * supported by the consumer in order to obtain an effective 1868 * evaluation. This does not mean that a value is required for this 1869 * element, only that the consuming system must understand the 1870 * element and be able to provide values for it if they are 1871 * available. 1872 * 1873 * The value of mustSupport SHALL be a FHIRPath resolveable on the 1874 * type of the DataRequirement. The path SHALL consist only of 1875 * identifiers, constant indexers, and .resolve() (see the [Simple 1876 * FHIRPath Profile](fhirpath.html#simple) for full details).) 1877 */ 1878 public DataRequirement addMustSupport(String value) { // 1 1879 StringType t = new StringType(); 1880 t.setValue(value); 1881 if (this.mustSupport == null) 1882 this.mustSupport = new ArrayList<StringType>(); 1883 this.mustSupport.add(t); 1884 return this; 1885 } 1886 1887 /** 1888 * @param value {@link #mustSupport} (Indicates that specific elements of the 1889 * type are referenced by the knowledge module and must be 1890 * supported by the consumer in order to obtain an effective 1891 * evaluation. This does not mean that a value is required for this 1892 * element, only that the consuming system must understand the 1893 * element and be able to provide values for it if they are 1894 * available. 1895 * 1896 * The value of mustSupport SHALL be a FHIRPath resolveable on the 1897 * type of the DataRequirement. The path SHALL consist only of 1898 * identifiers, constant indexers, and .resolve() (see the [Simple 1899 * FHIRPath Profile](fhirpath.html#simple) for full details).) 1900 */ 1901 public boolean hasMustSupport(String value) { 1902 if (this.mustSupport == null) 1903 return false; 1904 for (StringType v : this.mustSupport) 1905 if (v.getValue().equals(value)) // string 1906 return true; 1907 return false; 1908 } 1909 1910 /** 1911 * @return {@link #codeFilter} (Code filters specify additional constraints on 1912 * the data, specifying the value set of interest for a particular 1913 * element of the data. Each code filter defines an additional 1914 * constraint on the data, i.e. code filters are AND'ed, not OR'ed.) 1915 */ 1916 public List<DataRequirementCodeFilterComponent> getCodeFilter() { 1917 if (this.codeFilter == null) 1918 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1919 return this.codeFilter; 1920 } 1921 1922 /** 1923 * @return Returns a reference to <code>this</code> for easy method chaining 1924 */ 1925 public DataRequirement setCodeFilter(List<DataRequirementCodeFilterComponent> theCodeFilter) { 1926 this.codeFilter = theCodeFilter; 1927 return this; 1928 } 1929 1930 public boolean hasCodeFilter() { 1931 if (this.codeFilter == null) 1932 return false; 1933 for (DataRequirementCodeFilterComponent item : this.codeFilter) 1934 if (!item.isEmpty()) 1935 return true; 1936 return false; 1937 } 1938 1939 public DataRequirementCodeFilterComponent addCodeFilter() { // 3 1940 DataRequirementCodeFilterComponent t = new DataRequirementCodeFilterComponent(); 1941 if (this.codeFilter == null) 1942 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1943 this.codeFilter.add(t); 1944 return t; 1945 } 1946 1947 public DataRequirement addCodeFilter(DataRequirementCodeFilterComponent t) { // 3 1948 if (t == null) 1949 return this; 1950 if (this.codeFilter == null) 1951 this.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 1952 this.codeFilter.add(t); 1953 return this; 1954 } 1955 1956 /** 1957 * @return The first repetition of repeating field {@link #codeFilter}, creating 1958 * it if it does not already exist 1959 */ 1960 public DataRequirementCodeFilterComponent getCodeFilterFirstRep() { 1961 if (getCodeFilter().isEmpty()) { 1962 addCodeFilter(); 1963 } 1964 return getCodeFilter().get(0); 1965 } 1966 1967 /** 1968 * @return {@link #dateFilter} (Date filters specify additional constraints on 1969 * the data in terms of the applicable date range for specific elements. 1970 * Each date filter specifies an additional constraint on the data, i.e. 1971 * date filters are AND'ed, not OR'ed.) 1972 */ 1973 public List<DataRequirementDateFilterComponent> getDateFilter() { 1974 if (this.dateFilter == null) 1975 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 1976 return this.dateFilter; 1977 } 1978 1979 /** 1980 * @return Returns a reference to <code>this</code> for easy method chaining 1981 */ 1982 public DataRequirement setDateFilter(List<DataRequirementDateFilterComponent> theDateFilter) { 1983 this.dateFilter = theDateFilter; 1984 return this; 1985 } 1986 1987 public boolean hasDateFilter() { 1988 if (this.dateFilter == null) 1989 return false; 1990 for (DataRequirementDateFilterComponent item : this.dateFilter) 1991 if (!item.isEmpty()) 1992 return true; 1993 return false; 1994 } 1995 1996 public DataRequirementDateFilterComponent addDateFilter() { // 3 1997 DataRequirementDateFilterComponent t = new DataRequirementDateFilterComponent(); 1998 if (this.dateFilter == null) 1999 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2000 this.dateFilter.add(t); 2001 return t; 2002 } 2003 2004 public DataRequirement addDateFilter(DataRequirementDateFilterComponent t) { // 3 2005 if (t == null) 2006 return this; 2007 if (this.dateFilter == null) 2008 this.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2009 this.dateFilter.add(t); 2010 return this; 2011 } 2012 2013 /** 2014 * @return The first repetition of repeating field {@link #dateFilter}, creating 2015 * it if it does not already exist 2016 */ 2017 public DataRequirementDateFilterComponent getDateFilterFirstRep() { 2018 if (getDateFilter().isEmpty()) { 2019 addDateFilter(); 2020 } 2021 return getDateFilter().get(0); 2022 } 2023 2024 /** 2025 * @return {@link #limit} (Specifies a maximum number of results that are 2026 * required (uses the _count search parameter).). This is the underlying 2027 * object with id, value and extensions. The accessor "getLimit" gives 2028 * direct access to the value 2029 */ 2030 public PositiveIntType getLimitElement() { 2031 if (this.limit == null) 2032 if (Configuration.errorOnAutoCreate()) 2033 throw new Error("Attempt to auto-create DataRequirement.limit"); 2034 else if (Configuration.doAutoCreate()) 2035 this.limit = new PositiveIntType(); // bb 2036 return this.limit; 2037 } 2038 2039 public boolean hasLimitElement() { 2040 return this.limit != null && !this.limit.isEmpty(); 2041 } 2042 2043 public boolean hasLimit() { 2044 return this.limit != null && !this.limit.isEmpty(); 2045 } 2046 2047 /** 2048 * @param value {@link #limit} (Specifies a maximum number of results that are 2049 * required (uses the _count search parameter).). This is the 2050 * underlying object with id, value and extensions. The accessor 2051 * "getLimit" gives direct access to the value 2052 */ 2053 public DataRequirement setLimitElement(PositiveIntType value) { 2054 this.limit = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return Specifies a maximum number of results that are required (uses the 2060 * _count search parameter). 2061 */ 2062 public int getLimit() { 2063 return this.limit == null || this.limit.isEmpty() ? 0 : this.limit.getValue(); 2064 } 2065 2066 /** 2067 * @param value Specifies a maximum number of results that are required (uses 2068 * the _count search parameter). 2069 */ 2070 public DataRequirement setLimit(int value) { 2071 if (this.limit == null) 2072 this.limit = new PositiveIntType(); 2073 this.limit.setValue(value); 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #sort} (Specifies the order of the results to be returned.) 2079 */ 2080 public List<DataRequirementSortComponent> getSort() { 2081 if (this.sort == null) 2082 this.sort = new ArrayList<DataRequirementSortComponent>(); 2083 return this.sort; 2084 } 2085 2086 /** 2087 * @return Returns a reference to <code>this</code> for easy method chaining 2088 */ 2089 public DataRequirement setSort(List<DataRequirementSortComponent> theSort) { 2090 this.sort = theSort; 2091 return this; 2092 } 2093 2094 public boolean hasSort() { 2095 if (this.sort == null) 2096 return false; 2097 for (DataRequirementSortComponent item : this.sort) 2098 if (!item.isEmpty()) 2099 return true; 2100 return false; 2101 } 2102 2103 public DataRequirementSortComponent addSort() { // 3 2104 DataRequirementSortComponent t = new DataRequirementSortComponent(); 2105 if (this.sort == null) 2106 this.sort = new ArrayList<DataRequirementSortComponent>(); 2107 this.sort.add(t); 2108 return t; 2109 } 2110 2111 public DataRequirement addSort(DataRequirementSortComponent t) { // 3 2112 if (t == null) 2113 return this; 2114 if (this.sort == null) 2115 this.sort = new ArrayList<DataRequirementSortComponent>(); 2116 this.sort.add(t); 2117 return this; 2118 } 2119 2120 /** 2121 * @return The first repetition of repeating field {@link #sort}, creating it if 2122 * it does not already exist 2123 */ 2124 public DataRequirementSortComponent getSortFirstRep() { 2125 if (getSort().isEmpty()) { 2126 addSort(); 2127 } 2128 return getSort().get(0); 2129 } 2130 2131 protected void listChildren(List<Property> children) { 2132 super.listChildren(children); 2133 children.add(new Property("type", "code", 2134 "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 2135 0, 1, type)); 2136 children.add(new Property("profile", "canonical(StructureDefinition)", 2137 "The profile of the required data, specified as the uri of the profile definition.", 0, 2138 java.lang.Integer.MAX_VALUE, profile)); 2139 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", 2140 "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 2141 0, 1, subject)); 2142 children.add(new Property("mustSupport", "string", 2143 "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolveable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 2144 0, java.lang.Integer.MAX_VALUE, mustSupport)); 2145 children.add(new Property("codeFilter", "", 2146 "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.", 2147 0, java.lang.Integer.MAX_VALUE, codeFilter)); 2148 children.add(new Property("dateFilter", "", 2149 "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.", 2150 0, java.lang.Integer.MAX_VALUE, dateFilter)); 2151 children.add(new Property("limit", "positiveInt", 2152 "Specifies a maximum number of results that are required (uses the _count search parameter).", 0, 1, limit)); 2153 children.add(new Property("sort", "", "Specifies the order of the results to be returned.", 0, 2154 java.lang.Integer.MAX_VALUE, sort)); 2155 } 2156 2157 @Override 2158 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2159 switch (_hash) { 2160 case 3575610: 2161 /* type */ return new Property("type", "code", 2162 "The type of the required data, specified as the type name of a resource. For profiles, this value is set to the type of the base resource of the profile.", 2163 0, 1, type); 2164 case -309425751: 2165 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 2166 "The profile of the required data, specified as the uri of the profile definition.", 0, 2167 java.lang.Integer.MAX_VALUE, profile); 2168 case -573640748: 2169 /* subject[x] */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2170 "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 2171 0, 1, subject); 2172 case -1867885268: 2173 /* subject */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2174 "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 2175 0, 1, subject); 2176 case -1257122603: 2177 /* subjectCodeableConcept */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2178 "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 2179 0, 1, subject); 2180 case 772938623: 2181 /* subjectReference */ return new Property("subject[x]", "CodeableConcept|Reference(Group)", 2182 "The intended subjects of the data requirement. If this element is not provided, a Patient subject is assumed.", 2183 0, 1, subject); 2184 case -1402857082: 2185 /* mustSupport */ return new Property("mustSupport", "string", 2186 "Indicates that specific elements of the type are referenced by the knowledge module and must be supported by the consumer in order to obtain an effective evaluation. This does not mean that a value is required for this element, only that the consuming system must understand the element and be able to provide values for it if they are available. \n\nThe value of mustSupport SHALL be a FHIRPath resolveable on the type of the DataRequirement. The path SHALL consist only of identifiers, constant indexers, and .resolve() (see the [Simple FHIRPath Profile](fhirpath.html#simple) for full details).", 2187 0, java.lang.Integer.MAX_VALUE, mustSupport); 2188 case -1303674939: 2189 /* codeFilter */ return new Property("codeFilter", "", 2190 "Code filters specify additional constraints on the data, specifying the value set of interest for a particular element of the data. Each code filter defines an additional constraint on the data, i.e. code filters are AND'ed, not OR'ed.", 2191 0, java.lang.Integer.MAX_VALUE, codeFilter); 2192 case 149531846: 2193 /* dateFilter */ return new Property("dateFilter", "", 2194 "Date filters specify additional constraints on the data in terms of the applicable date range for specific elements. Each date filter specifies an additional constraint on the data, i.e. date filters are AND'ed, not OR'ed.", 2195 0, java.lang.Integer.MAX_VALUE, dateFilter); 2196 case 102976443: 2197 /* limit */ return new Property("limit", "positiveInt", 2198 "Specifies a maximum number of results that are required (uses the _count search parameter).", 0, 1, limit); 2199 case 3536286: 2200 /* sort */ return new Property("sort", "", "Specifies the order of the results to be returned.", 0, 2201 java.lang.Integer.MAX_VALUE, sort); 2202 default: 2203 return super.getNamedProperty(_hash, _name, _checkValid); 2204 } 2205 2206 } 2207 2208 @Override 2209 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2210 switch (hash) { 2211 case 3575610: 2212 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeType 2213 case -309425751: 2214 /* profile */ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 2215 case -1867885268: 2216 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Type 2217 case -1402857082: 2218 /* mustSupport */ return this.mustSupport == null ? new Base[0] 2219 : this.mustSupport.toArray(new Base[this.mustSupport.size()]); // StringType 2220 case -1303674939: 2221 /* codeFilter */ return this.codeFilter == null ? new Base[0] 2222 : this.codeFilter.toArray(new Base[this.codeFilter.size()]); // DataRequirementCodeFilterComponent 2223 case 149531846: 2224 /* dateFilter */ return this.dateFilter == null ? new Base[0] 2225 : this.dateFilter.toArray(new Base[this.dateFilter.size()]); // DataRequirementDateFilterComponent 2226 case 102976443: 2227 /* limit */ return this.limit == null ? new Base[0] : new Base[] { this.limit }; // PositiveIntType 2228 case 3536286: 2229 /* sort */ return this.sort == null ? new Base[0] : this.sort.toArray(new Base[this.sort.size()]); // DataRequirementSortComponent 2230 default: 2231 return super.getProperty(hash, name, checkValid); 2232 } 2233 2234 } 2235 2236 @Override 2237 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2238 switch (hash) { 2239 case 3575610: // type 2240 this.type = castToCode(value); // CodeType 2241 return value; 2242 case -309425751: // profile 2243 this.getProfile().add(castToCanonical(value)); // CanonicalType 2244 return value; 2245 case -1867885268: // subject 2246 this.subject = castToType(value); // Type 2247 return value; 2248 case -1402857082: // mustSupport 2249 this.getMustSupport().add(castToString(value)); // StringType 2250 return value; 2251 case -1303674939: // codeFilter 2252 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); // DataRequirementCodeFilterComponent 2253 return value; 2254 case 149531846: // dateFilter 2255 this.getDateFilter().add((DataRequirementDateFilterComponent) value); // DataRequirementDateFilterComponent 2256 return value; 2257 case 102976443: // limit 2258 this.limit = castToPositiveInt(value); // PositiveIntType 2259 return value; 2260 case 3536286: // sort 2261 this.getSort().add((DataRequirementSortComponent) value); // DataRequirementSortComponent 2262 return value; 2263 default: 2264 return super.setProperty(hash, name, value); 2265 } 2266 2267 } 2268 2269 @Override 2270 public Base setProperty(String name, Base value) throws FHIRException { 2271 if (name.equals("type")) { 2272 this.type = castToCode(value); // CodeType 2273 } else if (name.equals("profile")) { 2274 this.getProfile().add(castToCanonical(value)); 2275 } else if (name.equals("subject[x]")) { 2276 this.subject = castToType(value); // Type 2277 } else if (name.equals("mustSupport")) { 2278 this.getMustSupport().add(castToString(value)); 2279 } else if (name.equals("codeFilter")) { 2280 this.getCodeFilter().add((DataRequirementCodeFilterComponent) value); 2281 } else if (name.equals("dateFilter")) { 2282 this.getDateFilter().add((DataRequirementDateFilterComponent) value); 2283 } else if (name.equals("limit")) { 2284 this.limit = castToPositiveInt(value); // PositiveIntType 2285 } else if (name.equals("sort")) { 2286 this.getSort().add((DataRequirementSortComponent) value); 2287 } else 2288 return super.setProperty(name, value); 2289 return value; 2290 } 2291 2292 @Override 2293 public void removeChild(String name, Base value) throws FHIRException { 2294 if (name.equals("type")) { 2295 this.type = null; 2296 } else if (name.equals("profile")) { 2297 this.getProfile().remove(castToCanonical(value)); 2298 } else if (name.equals("subject[x]")) { 2299 this.subject = null; 2300 } else if (name.equals("mustSupport")) { 2301 this.getMustSupport().remove(castToString(value)); 2302 } else if (name.equals("codeFilter")) { 2303 this.getCodeFilter().remove((DataRequirementCodeFilterComponent) value); 2304 } else if (name.equals("dateFilter")) { 2305 this.getDateFilter().remove((DataRequirementDateFilterComponent) value); 2306 } else if (name.equals("limit")) { 2307 this.limit = null; 2308 } else if (name.equals("sort")) { 2309 this.getSort().remove((DataRequirementSortComponent) value); 2310 } else 2311 super.removeChild(name, value); 2312 2313 } 2314 2315 @Override 2316 public Base makeProperty(int hash, String name) throws FHIRException { 2317 switch (hash) { 2318 case 3575610: 2319 return getTypeElement(); 2320 case -309425751: 2321 return addProfileElement(); 2322 case -573640748: 2323 return getSubject(); 2324 case -1867885268: 2325 return getSubject(); 2326 case -1402857082: 2327 return addMustSupportElement(); 2328 case -1303674939: 2329 return addCodeFilter(); 2330 case 149531846: 2331 return addDateFilter(); 2332 case 102976443: 2333 return getLimitElement(); 2334 case 3536286: 2335 return addSort(); 2336 default: 2337 return super.makeProperty(hash, name); 2338 } 2339 2340 } 2341 2342 @Override 2343 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2344 switch (hash) { 2345 case 3575610: 2346 /* type */ return new String[] { "code" }; 2347 case -309425751: 2348 /* profile */ return new String[] { "canonical" }; 2349 case -1867885268: 2350 /* subject */ return new String[] { "CodeableConcept", "Reference" }; 2351 case -1402857082: 2352 /* mustSupport */ return new String[] { "string" }; 2353 case -1303674939: 2354 /* codeFilter */ return new String[] {}; 2355 case 149531846: 2356 /* dateFilter */ return new String[] {}; 2357 case 102976443: 2358 /* limit */ return new String[] { "positiveInt" }; 2359 case 3536286: 2360 /* sort */ return new String[] {}; 2361 default: 2362 return super.getTypesForProperty(hash, name); 2363 } 2364 2365 } 2366 2367 @Override 2368 public Base addChild(String name) throws FHIRException { 2369 if (name.equals("type")) { 2370 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.type"); 2371 } else if (name.equals("profile")) { 2372 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.profile"); 2373 } else if (name.equals("subjectCodeableConcept")) { 2374 this.subject = new CodeableConcept(); 2375 return this.subject; 2376 } else if (name.equals("subjectReference")) { 2377 this.subject = new Reference(); 2378 return this.subject; 2379 } else if (name.equals("mustSupport")) { 2380 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.mustSupport"); 2381 } else if (name.equals("codeFilter")) { 2382 return addCodeFilter(); 2383 } else if (name.equals("dateFilter")) { 2384 return addDateFilter(); 2385 } else if (name.equals("limit")) { 2386 throw new FHIRException("Cannot call addChild on a singleton property DataRequirement.limit"); 2387 } else if (name.equals("sort")) { 2388 return addSort(); 2389 } else 2390 return super.addChild(name); 2391 } 2392 2393 public String fhirType() { 2394 return "DataRequirement"; 2395 2396 } 2397 2398 public DataRequirement copy() { 2399 DataRequirement dst = new DataRequirement(); 2400 copyValues(dst); 2401 return dst; 2402 } 2403 2404 public void copyValues(DataRequirement dst) { 2405 super.copyValues(dst); 2406 dst.type = type == null ? null : type.copy(); 2407 if (profile != null) { 2408 dst.profile = new ArrayList<CanonicalType>(); 2409 for (CanonicalType i : profile) 2410 dst.profile.add(i.copy()); 2411 } 2412 ; 2413 dst.subject = subject == null ? null : subject.copy(); 2414 if (mustSupport != null) { 2415 dst.mustSupport = new ArrayList<StringType>(); 2416 for (StringType i : mustSupport) 2417 dst.mustSupport.add(i.copy()); 2418 } 2419 ; 2420 if (codeFilter != null) { 2421 dst.codeFilter = new ArrayList<DataRequirementCodeFilterComponent>(); 2422 for (DataRequirementCodeFilterComponent i : codeFilter) 2423 dst.codeFilter.add(i.copy()); 2424 } 2425 ; 2426 if (dateFilter != null) { 2427 dst.dateFilter = new ArrayList<DataRequirementDateFilterComponent>(); 2428 for (DataRequirementDateFilterComponent i : dateFilter) 2429 dst.dateFilter.add(i.copy()); 2430 } 2431 ; 2432 dst.limit = limit == null ? null : limit.copy(); 2433 if (sort != null) { 2434 dst.sort = new ArrayList<DataRequirementSortComponent>(); 2435 for (DataRequirementSortComponent i : sort) 2436 dst.sort.add(i.copy()); 2437 } 2438 ; 2439 } 2440 2441 protected DataRequirement typedCopy() { 2442 return copy(); 2443 } 2444 2445 @Override 2446 public boolean equalsDeep(Base other_) { 2447 if (!super.equalsDeep(other_)) 2448 return false; 2449 if (!(other_ instanceof DataRequirement)) 2450 return false; 2451 DataRequirement o = (DataRequirement) other_; 2452 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) 2453 && compareDeep(subject, o.subject, true) && compareDeep(mustSupport, o.mustSupport, true) 2454 && compareDeep(codeFilter, o.codeFilter, true) && compareDeep(dateFilter, o.dateFilter, true) 2455 && compareDeep(limit, o.limit, true) && compareDeep(sort, o.sort, true); 2456 } 2457 2458 @Override 2459 public boolean equalsShallow(Base other_) { 2460 if (!super.equalsShallow(other_)) 2461 return false; 2462 if (!(other_ instanceof DataRequirement)) 2463 return false; 2464 DataRequirement o = (DataRequirement) other_; 2465 return compareValues(type, o.type, true) && compareValues(mustSupport, o.mustSupport, true) 2466 && compareValues(limit, o.limit, true); 2467 } 2468 2469 public boolean isEmpty() { 2470 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, subject, mustSupport, codeFilter, 2471 dateFilter, limit, sort); 2472 } 2473 2474}