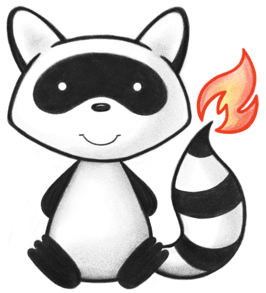
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Indicates an actual or potential clinical issue with or between one or more 049 * active or proposed clinical actions for a patient; e.g. Drug-drug 050 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 051 * etc. 052 */ 053@ResourceDef(name = "DetectedIssue", profile = "http://hl7.org/fhir/StructureDefinition/DetectedIssue") 054public class DetectedIssue extends DomainResource { 055 056 public enum DetectedIssueStatus { 057 /** 058 * The existence of the observation is registered, but there is no result yet 059 * available. 060 */ 061 REGISTERED, 062 /** 063 * This is an initial or interim observation: data may be incomplete or 064 * unverified. 065 */ 066 PRELIMINARY, 067 /** 068 * The observation is complete and there are no further actions needed. 069 * Additional information such "released", "signed", etc would be represented 070 * using [Provenance](provenance.html) which provides not only the act but also 071 * the actors and dates and other related data. These act states would be 072 * associated with an observation status of `preliminary` until they are all 073 * completed and then a status of `final` would be applied. 074 */ 075 FINAL, 076 /** 077 * Subsequent to being Final, the observation has been modified subsequent. This 078 * includes updates/new information and corrections. 079 */ 080 AMENDED, 081 /** 082 * Subsequent to being Final, the observation has been modified to correct an 083 * error in the test result. 084 */ 085 CORRECTED, 086 /** 087 * The observation is unavailable because the measurement was not started or not 088 * completed (also sometimes called "aborted"). 089 */ 090 CANCELLED, 091 /** 092 * The observation has been withdrawn following previous final release. This 093 * electronic record should never have existed, though it is possible that 094 * real-world decisions were based on it. (If real-world activity has occurred, 095 * the status should be "cancelled" rather than "entered-in-error".). 096 */ 097 ENTEREDINERROR, 098 /** 099 * The authoring/source system does not know which of the status values 100 * currently applies for this observation. Note: This concept is not to be used 101 * for "other" - one of the listed statuses is presumed to apply, but the 102 * authoring/source system does not know which. 103 */ 104 UNKNOWN, 105 /** 106 * added to help the parsers with the generic types 107 */ 108 NULL; 109 110 public static DetectedIssueStatus fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("registered".equals(codeString)) 114 return REGISTERED; 115 if ("preliminary".equals(codeString)) 116 return PRELIMINARY; 117 if ("final".equals(codeString)) 118 return FINAL; 119 if ("amended".equals(codeString)) 120 return AMENDED; 121 if ("corrected".equals(codeString)) 122 return CORRECTED; 123 if ("cancelled".equals(codeString)) 124 return CANCELLED; 125 if ("entered-in-error".equals(codeString)) 126 return ENTEREDINERROR; 127 if ("unknown".equals(codeString)) 128 return UNKNOWN; 129 if (Configuration.isAcceptInvalidEnums()) 130 return null; 131 else 132 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 133 } 134 135 public String toCode() { 136 switch (this) { 137 case REGISTERED: 138 return "registered"; 139 case PRELIMINARY: 140 return "preliminary"; 141 case FINAL: 142 return "final"; 143 case AMENDED: 144 return "amended"; 145 case CORRECTED: 146 return "corrected"; 147 case CANCELLED: 148 return "cancelled"; 149 case ENTEREDINERROR: 150 return "entered-in-error"; 151 case UNKNOWN: 152 return "unknown"; 153 case NULL: 154 return null; 155 default: 156 return "?"; 157 } 158 } 159 160 public String getSystem() { 161 switch (this) { 162 case REGISTERED: 163 return "http://hl7.org/fhir/observation-status"; 164 case PRELIMINARY: 165 return "http://hl7.org/fhir/observation-status"; 166 case FINAL: 167 return "http://hl7.org/fhir/observation-status"; 168 case AMENDED: 169 return "http://hl7.org/fhir/observation-status"; 170 case CORRECTED: 171 return "http://hl7.org/fhir/observation-status"; 172 case CANCELLED: 173 return "http://hl7.org/fhir/observation-status"; 174 case ENTEREDINERROR: 175 return "http://hl7.org/fhir/observation-status"; 176 case UNKNOWN: 177 return "http://hl7.org/fhir/observation-status"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 185 public String getDefinition() { 186 switch (this) { 187 case REGISTERED: 188 return "The existence of the observation is registered, but there is no result yet available."; 189 case PRELIMINARY: 190 return "This is an initial or interim observation: data may be incomplete or unverified."; 191 case FINAL: 192 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 193 case AMENDED: 194 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 195 case CORRECTED: 196 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 197 case CANCELLED: 198 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 199 case ENTEREDINERROR: 200 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 201 case UNKNOWN: 202 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 203 case NULL: 204 return null; 205 default: 206 return "?"; 207 } 208 } 209 210 public String getDisplay() { 211 switch (this) { 212 case REGISTERED: 213 return "Registered"; 214 case PRELIMINARY: 215 return "Preliminary"; 216 case FINAL: 217 return "Final"; 218 case AMENDED: 219 return "Amended"; 220 case CORRECTED: 221 return "Corrected"; 222 case CANCELLED: 223 return "Cancelled"; 224 case ENTEREDINERROR: 225 return "Entered in Error"; 226 case UNKNOWN: 227 return "Unknown"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class DetectedIssueStatusEnumFactory implements EnumFactory<DetectedIssueStatus> { 237 public DetectedIssueStatus fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("registered".equals(codeString)) 242 return DetectedIssueStatus.REGISTERED; 243 if ("preliminary".equals(codeString)) 244 return DetectedIssueStatus.PRELIMINARY; 245 if ("final".equals(codeString)) 246 return DetectedIssueStatus.FINAL; 247 if ("amended".equals(codeString)) 248 return DetectedIssueStatus.AMENDED; 249 if ("corrected".equals(codeString)) 250 return DetectedIssueStatus.CORRECTED; 251 if ("cancelled".equals(codeString)) 252 return DetectedIssueStatus.CANCELLED; 253 if ("entered-in-error".equals(codeString)) 254 return DetectedIssueStatus.ENTEREDINERROR; 255 if ("unknown".equals(codeString)) 256 return DetectedIssueStatus.UNKNOWN; 257 throw new IllegalArgumentException("Unknown DetectedIssueStatus code '" + codeString + "'"); 258 } 259 260 public Enumeration<DetectedIssueStatus> fromType(PrimitiveType<?> code) throws FHIRException { 261 if (code == null) 262 return null; 263 if (code.isEmpty()) 264 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 265 String codeString = code.asStringValue(); 266 if (codeString == null || "".equals(codeString)) 267 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.NULL, code); 268 if ("registered".equals(codeString)) 269 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.REGISTERED, code); 270 if ("preliminary".equals(codeString)) 271 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.PRELIMINARY, code); 272 if ("final".equals(codeString)) 273 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.FINAL, code); 274 if ("amended".equals(codeString)) 275 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.AMENDED, code); 276 if ("corrected".equals(codeString)) 277 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CORRECTED, code); 278 if ("cancelled".equals(codeString)) 279 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.CANCELLED, code); 280 if ("entered-in-error".equals(codeString)) 281 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.ENTEREDINERROR, code); 282 if ("unknown".equals(codeString)) 283 return new Enumeration<DetectedIssueStatus>(this, DetectedIssueStatus.UNKNOWN, code); 284 throw new FHIRException("Unknown DetectedIssueStatus code '" + codeString + "'"); 285 } 286 287 public String toCode(DetectedIssueStatus code) { 288 if (code == DetectedIssueStatus.NULL) 289 return null; 290 if (code == DetectedIssueStatus.REGISTERED) 291 return "registered"; 292 if (code == DetectedIssueStatus.PRELIMINARY) 293 return "preliminary"; 294 if (code == DetectedIssueStatus.FINAL) 295 return "final"; 296 if (code == DetectedIssueStatus.AMENDED) 297 return "amended"; 298 if (code == DetectedIssueStatus.CORRECTED) 299 return "corrected"; 300 if (code == DetectedIssueStatus.CANCELLED) 301 return "cancelled"; 302 if (code == DetectedIssueStatus.ENTEREDINERROR) 303 return "entered-in-error"; 304 if (code == DetectedIssueStatus.UNKNOWN) 305 return "unknown"; 306 return "?"; 307 } 308 309 public String toSystem(DetectedIssueStatus code) { 310 return code.getSystem(); 311 } 312 } 313 314 public enum DetectedIssueSeverity { 315 /** 316 * Indicates the issue may be life-threatening or has the potential to cause 317 * permanent injury. 318 */ 319 HIGH, 320 /** 321 * Indicates the issue may result in noticeable adverse consequences but is 322 * unlikely to be life-threatening or cause permanent injury. 323 */ 324 MODERATE, 325 /** 326 * Indicates the issue may result in some adverse consequences but is unlikely 327 * to substantially affect the situation of the subject. 328 */ 329 LOW, 330 /** 331 * added to help the parsers with the generic types 332 */ 333 NULL; 334 335 public static DetectedIssueSeverity fromCode(String codeString) throws FHIRException { 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("high".equals(codeString)) 339 return HIGH; 340 if ("moderate".equals(codeString)) 341 return MODERATE; 342 if ("low".equals(codeString)) 343 return LOW; 344 if (Configuration.isAcceptInvalidEnums()) 345 return null; 346 else 347 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 348 } 349 350 public String toCode() { 351 switch (this) { 352 case HIGH: 353 return "high"; 354 case MODERATE: 355 return "moderate"; 356 case LOW: 357 return "low"; 358 case NULL: 359 return null; 360 default: 361 return "?"; 362 } 363 } 364 365 public String getSystem() { 366 switch (this) { 367 case HIGH: 368 return "http://hl7.org/fhir/detectedissue-severity"; 369 case MODERATE: 370 return "http://hl7.org/fhir/detectedissue-severity"; 371 case LOW: 372 return "http://hl7.org/fhir/detectedissue-severity"; 373 case NULL: 374 return null; 375 default: 376 return "?"; 377 } 378 } 379 380 public String getDefinition() { 381 switch (this) { 382 case HIGH: 383 return "Indicates the issue may be life-threatening or has the potential to cause permanent injury."; 384 case MODERATE: 385 return "Indicates the issue may result in noticeable adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 386 case LOW: 387 return "Indicates the issue may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getDisplay() { 396 switch (this) { 397 case HIGH: 398 return "High"; 399 case MODERATE: 400 return "Moderate"; 401 case LOW: 402 return "Low"; 403 case NULL: 404 return null; 405 default: 406 return "?"; 407 } 408 } 409 } 410 411 public static class DetectedIssueSeverityEnumFactory implements EnumFactory<DetectedIssueSeverity> { 412 public DetectedIssueSeverity fromCode(String codeString) throws IllegalArgumentException { 413 if (codeString == null || "".equals(codeString)) 414 if (codeString == null || "".equals(codeString)) 415 return null; 416 if ("high".equals(codeString)) 417 return DetectedIssueSeverity.HIGH; 418 if ("moderate".equals(codeString)) 419 return DetectedIssueSeverity.MODERATE; 420 if ("low".equals(codeString)) 421 return DetectedIssueSeverity.LOW; 422 throw new IllegalArgumentException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 423 } 424 425 public Enumeration<DetectedIssueSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 426 if (code == null) 427 return null; 428 if (code.isEmpty()) 429 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 430 String codeString = code.asStringValue(); 431 if (codeString == null || "".equals(codeString)) 432 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.NULL, code); 433 if ("high".equals(codeString)) 434 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.HIGH, code); 435 if ("moderate".equals(codeString)) 436 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.MODERATE, code); 437 if ("low".equals(codeString)) 438 return new Enumeration<DetectedIssueSeverity>(this, DetectedIssueSeverity.LOW, code); 439 throw new FHIRException("Unknown DetectedIssueSeverity code '" + codeString + "'"); 440 } 441 442 public String toCode(DetectedIssueSeverity code) { 443 if (code == DetectedIssueSeverity.NULL) 444 return null; 445 if (code == DetectedIssueSeverity.HIGH) 446 return "high"; 447 if (code == DetectedIssueSeverity.MODERATE) 448 return "moderate"; 449 if (code == DetectedIssueSeverity.LOW) 450 return "low"; 451 return "?"; 452 } 453 454 public String toSystem(DetectedIssueSeverity code) { 455 return code.getSystem(); 456 } 457 } 458 459 @Block() 460 public static class DetectedIssueEvidenceComponent extends BackboneElement implements IBaseBackboneElement { 461 /** 462 * A manifestation that led to the recording of this detected issue. 463 */ 464 @Child(name = "code", type = { 465 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 466 @Description(shortDefinition = "Manifestation", formalDefinition = "A manifestation that led to the recording of this detected issue.") 467 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/manifestation-or-symptom") 468 protected List<CodeableConcept> code; 469 470 /** 471 * Links to resources that constitute evidence for the detected issue such as a 472 * GuidanceResponse or MeasureReport. 473 */ 474 @Child(name = "detail", type = { 475 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 476 @Description(shortDefinition = "Supporting information", formalDefinition = "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.") 477 protected List<Reference> detail; 478 /** 479 * The actual objects that are the target of the reference (Links to resources 480 * that constitute evidence for the detected issue such as a GuidanceResponse or 481 * MeasureReport.) 482 */ 483 protected List<Resource> detailTarget; 484 485 private static final long serialVersionUID = 1135831276L; 486 487 /** 488 * Constructor 489 */ 490 public DetectedIssueEvidenceComponent() { 491 super(); 492 } 493 494 /** 495 * @return {@link #code} (A manifestation that led to the recording of this 496 * detected issue.) 497 */ 498 public List<CodeableConcept> getCode() { 499 if (this.code == null) 500 this.code = new ArrayList<CodeableConcept>(); 501 return this.code; 502 } 503 504 /** 505 * @return Returns a reference to <code>this</code> for easy method chaining 506 */ 507 public DetectedIssueEvidenceComponent setCode(List<CodeableConcept> theCode) { 508 this.code = theCode; 509 return this; 510 } 511 512 public boolean hasCode() { 513 if (this.code == null) 514 return false; 515 for (CodeableConcept item : this.code) 516 if (!item.isEmpty()) 517 return true; 518 return false; 519 } 520 521 public CodeableConcept addCode() { // 3 522 CodeableConcept t = new CodeableConcept(); 523 if (this.code == null) 524 this.code = new ArrayList<CodeableConcept>(); 525 this.code.add(t); 526 return t; 527 } 528 529 public DetectedIssueEvidenceComponent addCode(CodeableConcept t) { // 3 530 if (t == null) 531 return this; 532 if (this.code == null) 533 this.code = new ArrayList<CodeableConcept>(); 534 this.code.add(t); 535 return this; 536 } 537 538 /** 539 * @return The first repetition of repeating field {@link #code}, creating it if 540 * it does not already exist 541 */ 542 public CodeableConcept getCodeFirstRep() { 543 if (getCode().isEmpty()) { 544 addCode(); 545 } 546 return getCode().get(0); 547 } 548 549 /** 550 * @return {@link #detail} (Links to resources that constitute evidence for the 551 * detected issue such as a GuidanceResponse or MeasureReport.) 552 */ 553 public List<Reference> getDetail() { 554 if (this.detail == null) 555 this.detail = new ArrayList<Reference>(); 556 return this.detail; 557 } 558 559 /** 560 * @return Returns a reference to <code>this</code> for easy method chaining 561 */ 562 public DetectedIssueEvidenceComponent setDetail(List<Reference> theDetail) { 563 this.detail = theDetail; 564 return this; 565 } 566 567 public boolean hasDetail() { 568 if (this.detail == null) 569 return false; 570 for (Reference item : this.detail) 571 if (!item.isEmpty()) 572 return true; 573 return false; 574 } 575 576 public Reference addDetail() { // 3 577 Reference t = new Reference(); 578 if (this.detail == null) 579 this.detail = new ArrayList<Reference>(); 580 this.detail.add(t); 581 return t; 582 } 583 584 public DetectedIssueEvidenceComponent addDetail(Reference t) { // 3 585 if (t == null) 586 return this; 587 if (this.detail == null) 588 this.detail = new ArrayList<Reference>(); 589 this.detail.add(t); 590 return this; 591 } 592 593 /** 594 * @return The first repetition of repeating field {@link #detail}, creating it 595 * if it does not already exist 596 */ 597 public Reference getDetailFirstRep() { 598 if (getDetail().isEmpty()) { 599 addDetail(); 600 } 601 return getDetail().get(0); 602 } 603 604 protected void listChildren(List<Property> children) { 605 super.listChildren(children); 606 children.add(new Property("code", "CodeableConcept", 607 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code)); 608 children.add(new Property("detail", "Reference(Any)", 609 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 610 0, java.lang.Integer.MAX_VALUE, detail)); 611 } 612 613 @Override 614 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 615 switch (_hash) { 616 case 3059181: 617 /* code */ return new Property("code", "CodeableConcept", 618 "A manifestation that led to the recording of this detected issue.", 0, java.lang.Integer.MAX_VALUE, code); 619 case -1335224239: 620 /* detail */ return new Property("detail", "Reference(Any)", 621 "Links to resources that constitute evidence for the detected issue such as a GuidanceResponse or MeasureReport.", 622 0, java.lang.Integer.MAX_VALUE, detail); 623 default: 624 return super.getNamedProperty(_hash, _name, _checkValid); 625 } 626 627 } 628 629 @Override 630 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 631 switch (hash) { 632 case 3059181: 633 /* code */ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 634 case -1335224239: 635 /* detail */ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // Reference 636 default: 637 return super.getProperty(hash, name, checkValid); 638 } 639 640 } 641 642 @Override 643 public Base setProperty(int hash, String name, Base value) throws FHIRException { 644 switch (hash) { 645 case 3059181: // code 646 this.getCode().add(castToCodeableConcept(value)); // CodeableConcept 647 return value; 648 case -1335224239: // detail 649 this.getDetail().add(castToReference(value)); // Reference 650 return value; 651 default: 652 return super.setProperty(hash, name, value); 653 } 654 655 } 656 657 @Override 658 public Base setProperty(String name, Base value) throws FHIRException { 659 if (name.equals("code")) { 660 this.getCode().add(castToCodeableConcept(value)); 661 } else if (name.equals("detail")) { 662 this.getDetail().add(castToReference(value)); 663 } else 664 return super.setProperty(name, value); 665 return value; 666 } 667 668 @Override 669 public void removeChild(String name, Base value) throws FHIRException { 670 if (name.equals("code")) { 671 this.getCode().remove(castToCodeableConcept(value)); 672 } else if (name.equals("detail")) { 673 this.getDetail().remove(castToReference(value)); 674 } else 675 super.removeChild(name, value); 676 677 } 678 679 @Override 680 public Base makeProperty(int hash, String name) throws FHIRException { 681 switch (hash) { 682 case 3059181: 683 return addCode(); 684 case -1335224239: 685 return addDetail(); 686 default: 687 return super.makeProperty(hash, name); 688 } 689 690 } 691 692 @Override 693 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 694 switch (hash) { 695 case 3059181: 696 /* code */ return new String[] { "CodeableConcept" }; 697 case -1335224239: 698 /* detail */ return new String[] { "Reference" }; 699 default: 700 return super.getTypesForProperty(hash, name); 701 } 702 703 } 704 705 @Override 706 public Base addChild(String name) throws FHIRException { 707 if (name.equals("code")) { 708 return addCode(); 709 } else if (name.equals("detail")) { 710 return addDetail(); 711 } else 712 return super.addChild(name); 713 } 714 715 public DetectedIssueEvidenceComponent copy() { 716 DetectedIssueEvidenceComponent dst = new DetectedIssueEvidenceComponent(); 717 copyValues(dst); 718 return dst; 719 } 720 721 public void copyValues(DetectedIssueEvidenceComponent dst) { 722 super.copyValues(dst); 723 if (code != null) { 724 dst.code = new ArrayList<CodeableConcept>(); 725 for (CodeableConcept i : code) 726 dst.code.add(i.copy()); 727 } 728 ; 729 if (detail != null) { 730 dst.detail = new ArrayList<Reference>(); 731 for (Reference i : detail) 732 dst.detail.add(i.copy()); 733 } 734 ; 735 } 736 737 @Override 738 public boolean equalsDeep(Base other_) { 739 if (!super.equalsDeep(other_)) 740 return false; 741 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 742 return false; 743 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 744 return compareDeep(code, o.code, true) && compareDeep(detail, o.detail, true); 745 } 746 747 @Override 748 public boolean equalsShallow(Base other_) { 749 if (!super.equalsShallow(other_)) 750 return false; 751 if (!(other_ instanceof DetectedIssueEvidenceComponent)) 752 return false; 753 DetectedIssueEvidenceComponent o = (DetectedIssueEvidenceComponent) other_; 754 return true; 755 } 756 757 public boolean isEmpty() { 758 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, detail); 759 } 760 761 public String fhirType() { 762 return "DetectedIssue.evidence"; 763 764 } 765 766 } 767 768 @Block() 769 public static class DetectedIssueMitigationComponent extends BackboneElement implements IBaseBackboneElement { 770 /** 771 * Describes the action that was taken or the observation that was made that 772 * reduces/eliminates the risk associated with the identified issue. 773 */ 774 @Child(name = "action", type = { 775 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 776 @Description(shortDefinition = "What mitigation?", formalDefinition = "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.") 777 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-mitigation-action") 778 protected CodeableConcept action; 779 780 /** 781 * Indicates when the mitigating action was documented. 782 */ 783 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 784 @Description(shortDefinition = "Date committed", formalDefinition = "Indicates when the mitigating action was documented.") 785 protected DateTimeType date; 786 787 /** 788 * Identifies the practitioner who determined the mitigation and takes 789 * responsibility for the mitigation step occurring. 790 */ 791 @Child(name = "author", type = { Practitioner.class, 792 PractitionerRole.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 793 @Description(shortDefinition = "Who is committing?", formalDefinition = "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.") 794 protected Reference author; 795 796 /** 797 * The actual object that is the target of the reference (Identifies the 798 * practitioner who determined the mitigation and takes responsibility for the 799 * mitigation step occurring.) 800 */ 801 protected Resource authorTarget; 802 803 private static final long serialVersionUID = -1928864832L; 804 805 /** 806 * Constructor 807 */ 808 public DetectedIssueMitigationComponent() { 809 super(); 810 } 811 812 /** 813 * Constructor 814 */ 815 public DetectedIssueMitigationComponent(CodeableConcept action) { 816 super(); 817 this.action = action; 818 } 819 820 /** 821 * @return {@link #action} (Describes the action that was taken or the 822 * observation that was made that reduces/eliminates the risk associated 823 * with the identified issue.) 824 */ 825 public CodeableConcept getAction() { 826 if (this.action == null) 827 if (Configuration.errorOnAutoCreate()) 828 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.action"); 829 else if (Configuration.doAutoCreate()) 830 this.action = new CodeableConcept(); // cc 831 return this.action; 832 } 833 834 public boolean hasAction() { 835 return this.action != null && !this.action.isEmpty(); 836 } 837 838 /** 839 * @param value {@link #action} (Describes the action that was taken or the 840 * observation that was made that reduces/eliminates the risk 841 * associated with the identified issue.) 842 */ 843 public DetectedIssueMitigationComponent setAction(CodeableConcept value) { 844 this.action = value; 845 return this; 846 } 847 848 /** 849 * @return {@link #date} (Indicates when the mitigating action was documented.). 850 * This is the underlying object with id, value and extensions. The 851 * accessor "getDate" gives direct access to the value 852 */ 853 public DateTimeType getDateElement() { 854 if (this.date == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.date"); 857 else if (Configuration.doAutoCreate()) 858 this.date = new DateTimeType(); // bb 859 return this.date; 860 } 861 862 public boolean hasDateElement() { 863 return this.date != null && !this.date.isEmpty(); 864 } 865 866 public boolean hasDate() { 867 return this.date != null && !this.date.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #date} (Indicates when the mitigating action was 872 * documented.). This is the underlying object with id, value and 873 * extensions. The accessor "getDate" gives direct access to the 874 * value 875 */ 876 public DetectedIssueMitigationComponent setDateElement(DateTimeType value) { 877 this.date = value; 878 return this; 879 } 880 881 /** 882 * @return Indicates when the mitigating action was documented. 883 */ 884 public Date getDate() { 885 return this.date == null ? null : this.date.getValue(); 886 } 887 888 /** 889 * @param value Indicates when the mitigating action was documented. 890 */ 891 public DetectedIssueMitigationComponent setDate(Date value) { 892 if (value == null) 893 this.date = null; 894 else { 895 if (this.date == null) 896 this.date = new DateTimeType(); 897 this.date.setValue(value); 898 } 899 return this; 900 } 901 902 /** 903 * @return {@link #author} (Identifies the practitioner who determined the 904 * mitigation and takes responsibility for the mitigation step 905 * occurring.) 906 */ 907 public Reference getAuthor() { 908 if (this.author == null) 909 if (Configuration.errorOnAutoCreate()) 910 throw new Error("Attempt to auto-create DetectedIssueMitigationComponent.author"); 911 else if (Configuration.doAutoCreate()) 912 this.author = new Reference(); // cc 913 return this.author; 914 } 915 916 public boolean hasAuthor() { 917 return this.author != null && !this.author.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #author} (Identifies the practitioner who determined the 922 * mitigation and takes responsibility for the mitigation step 923 * occurring.) 924 */ 925 public DetectedIssueMitigationComponent setAuthor(Reference value) { 926 this.author = value; 927 return this; 928 } 929 930 /** 931 * @return {@link #author} The actual object that is the target of the 932 * reference. The reference library doesn't populate this, but you can 933 * use it to hold the resource if you resolve it. (Identifies the 934 * practitioner who determined the mitigation and takes responsibility 935 * for the mitigation step occurring.) 936 */ 937 public Resource getAuthorTarget() { 938 return this.authorTarget; 939 } 940 941 /** 942 * @param value {@link #author} The actual object that is the target of the 943 * reference. The reference library doesn't use these, but you can 944 * use it to hold the resource if you resolve it. (Identifies the 945 * practitioner who determined the mitigation and takes 946 * responsibility for the mitigation step occurring.) 947 */ 948 public DetectedIssueMitigationComponent setAuthorTarget(Resource value) { 949 this.authorTarget = value; 950 return this; 951 } 952 953 protected void listChildren(List<Property> children) { 954 super.listChildren(children); 955 children.add(new Property("action", "CodeableConcept", 956 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 957 0, 1, action)); 958 children 959 .add(new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, date)); 960 children.add(new Property("author", "Reference(Practitioner|PractitionerRole)", 961 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 962 0, 1, author)); 963 } 964 965 @Override 966 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 967 switch (_hash) { 968 case -1422950858: 969 /* action */ return new Property("action", "CodeableConcept", 970 "Describes the action that was taken or the observation that was made that reduces/eliminates the risk associated with the identified issue.", 971 0, 1, action); 972 case 3076014: 973 /* date */ return new Property("date", "dateTime", "Indicates when the mitigating action was documented.", 0, 1, 974 date); 975 case -1406328437: 976 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole)", 977 "Identifies the practitioner who determined the mitigation and takes responsibility for the mitigation step occurring.", 978 0, 1, author); 979 default: 980 return super.getNamedProperty(_hash, _name, _checkValid); 981 } 982 983 } 984 985 @Override 986 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 987 switch (hash) { 988 case -1422950858: 989 /* action */ return this.action == null ? new Base[0] : new Base[] { this.action }; // CodeableConcept 990 case 3076014: 991 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 992 case -1406328437: 993 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 994 default: 995 return super.getProperty(hash, name, checkValid); 996 } 997 998 } 999 1000 @Override 1001 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1002 switch (hash) { 1003 case -1422950858: // action 1004 this.action = castToCodeableConcept(value); // CodeableConcept 1005 return value; 1006 case 3076014: // date 1007 this.date = castToDateTime(value); // DateTimeType 1008 return value; 1009 case -1406328437: // author 1010 this.author = castToReference(value); // Reference 1011 return value; 1012 default: 1013 return super.setProperty(hash, name, value); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(String name, Base value) throws FHIRException { 1020 if (name.equals("action")) { 1021 this.action = castToCodeableConcept(value); // CodeableConcept 1022 } else if (name.equals("date")) { 1023 this.date = castToDateTime(value); // DateTimeType 1024 } else if (name.equals("author")) { 1025 this.author = castToReference(value); // Reference 1026 } else 1027 return super.setProperty(name, value); 1028 return value; 1029 } 1030 1031 @Override 1032 public void removeChild(String name, Base value) throws FHIRException { 1033 if (name.equals("action")) { 1034 this.action = null; 1035 } else if (name.equals("date")) { 1036 this.date = null; 1037 } else if (name.equals("author")) { 1038 this.author = null; 1039 } else 1040 super.removeChild(name, value); 1041 1042 } 1043 1044 @Override 1045 public Base makeProperty(int hash, String name) throws FHIRException { 1046 switch (hash) { 1047 case -1422950858: 1048 return getAction(); 1049 case 3076014: 1050 return getDateElement(); 1051 case -1406328437: 1052 return getAuthor(); 1053 default: 1054 return super.makeProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1061 switch (hash) { 1062 case -1422950858: 1063 /* action */ return new String[] { "CodeableConcept" }; 1064 case 3076014: 1065 /* date */ return new String[] { "dateTime" }; 1066 case -1406328437: 1067 /* author */ return new String[] { "Reference" }; 1068 default: 1069 return super.getTypesForProperty(hash, name); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base addChild(String name) throws FHIRException { 1076 if (name.equals("action")) { 1077 this.action = new CodeableConcept(); 1078 return this.action; 1079 } else if (name.equals("date")) { 1080 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.date"); 1081 } else if (name.equals("author")) { 1082 this.author = new Reference(); 1083 return this.author; 1084 } else 1085 return super.addChild(name); 1086 } 1087 1088 public DetectedIssueMitigationComponent copy() { 1089 DetectedIssueMitigationComponent dst = new DetectedIssueMitigationComponent(); 1090 copyValues(dst); 1091 return dst; 1092 } 1093 1094 public void copyValues(DetectedIssueMitigationComponent dst) { 1095 super.copyValues(dst); 1096 dst.action = action == null ? null : action.copy(); 1097 dst.date = date == null ? null : date.copy(); 1098 dst.author = author == null ? null : author.copy(); 1099 } 1100 1101 @Override 1102 public boolean equalsDeep(Base other_) { 1103 if (!super.equalsDeep(other_)) 1104 return false; 1105 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1106 return false; 1107 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1108 return compareDeep(action, o.action, true) && compareDeep(date, o.date, true) 1109 && compareDeep(author, o.author, true); 1110 } 1111 1112 @Override 1113 public boolean equalsShallow(Base other_) { 1114 if (!super.equalsShallow(other_)) 1115 return false; 1116 if (!(other_ instanceof DetectedIssueMitigationComponent)) 1117 return false; 1118 DetectedIssueMitigationComponent o = (DetectedIssueMitigationComponent) other_; 1119 return compareValues(date, o.date, true); 1120 } 1121 1122 public boolean isEmpty() { 1123 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(action, date, author); 1124 } 1125 1126 public String fhirType() { 1127 return "DetectedIssue.mitigation"; 1128 1129 } 1130 1131 } 1132 1133 /** 1134 * Business identifier associated with the detected issue record. 1135 */ 1136 @Child(name = "identifier", type = { 1137 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1138 @Description(shortDefinition = "Unique id for the detected issue", formalDefinition = "Business identifier associated with the detected issue record.") 1139 protected List<Identifier> identifier; 1140 1141 /** 1142 * Indicates the status of the detected issue. 1143 */ 1144 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1145 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "Indicates the status of the detected issue.") 1146 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1147 protected Enumeration<DetectedIssueStatus> status; 1148 1149 /** 1150 * Identifies the general type of issue identified. 1151 */ 1152 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1153 @Description(shortDefinition = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", formalDefinition = "Identifies the general type of issue identified.") 1154 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-category") 1155 protected CodeableConcept code; 1156 1157 /** 1158 * Indicates the degree of importance associated with the identified issue based 1159 * on the potential impact on the patient. 1160 */ 1161 @Child(name = "severity", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1162 @Description(shortDefinition = "high | moderate | low", formalDefinition = "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.") 1163 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/detectedissue-severity") 1164 protected Enumeration<DetectedIssueSeverity> severity; 1165 1166 /** 1167 * Indicates the patient whose record the detected issue is associated with. 1168 */ 1169 @Child(name = "patient", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1170 @Description(shortDefinition = "Associated patient", formalDefinition = "Indicates the patient whose record the detected issue is associated with.") 1171 protected Reference patient; 1172 1173 /** 1174 * The actual object that is the target of the reference (Indicates the patient 1175 * whose record the detected issue is associated with.) 1176 */ 1177 protected Patient patientTarget; 1178 1179 /** 1180 * The date or period when the detected issue was initially identified. 1181 */ 1182 @Child(name = "identified", type = { DateTimeType.class, 1183 Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1184 @Description(shortDefinition = "When identified", formalDefinition = "The date or period when the detected issue was initially identified.") 1185 protected Type identified; 1186 1187 /** 1188 * Individual or device responsible for the issue being raised. For example, a 1189 * decision support application or a pharmacist conducting a medication review. 1190 */ 1191 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, 1192 Device.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1193 @Description(shortDefinition = "The provider or device that identified the issue", formalDefinition = "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.") 1194 protected Reference author; 1195 1196 /** 1197 * The actual object that is the target of the reference (Individual or device 1198 * responsible for the issue being raised. For example, a decision support 1199 * application or a pharmacist conducting a medication review.) 1200 */ 1201 protected Resource authorTarget; 1202 1203 /** 1204 * Indicates the resource representing the current activity or proposed activity 1205 * that is potentially problematic. 1206 */ 1207 @Child(name = "implicated", type = { 1208 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1209 @Description(shortDefinition = "Problem resource", formalDefinition = "Indicates the resource representing the current activity or proposed activity that is potentially problematic.") 1210 protected List<Reference> implicated; 1211 /** 1212 * The actual objects that are the target of the reference (Indicates the 1213 * resource representing the current activity or proposed activity that is 1214 * potentially problematic.) 1215 */ 1216 protected List<Resource> implicatedTarget; 1217 1218 /** 1219 * Supporting evidence or manifestations that provide the basis for identifying 1220 * the detected issue such as a GuidanceResponse or MeasureReport. 1221 */ 1222 @Child(name = "evidence", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1223 @Description(shortDefinition = "Supporting evidence", formalDefinition = "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.") 1224 protected List<DetectedIssueEvidenceComponent> evidence; 1225 1226 /** 1227 * A textual explanation of the detected issue. 1228 */ 1229 @Child(name = "detail", type = { StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1230 @Description(shortDefinition = "Description and context", formalDefinition = "A textual explanation of the detected issue.") 1231 protected StringType detail; 1232 1233 /** 1234 * The literature, knowledge-base or similar reference that describes the 1235 * propensity for the detected issue identified. 1236 */ 1237 @Child(name = "reference", type = { UriType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1238 @Description(shortDefinition = "Authority for issue", formalDefinition = "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.") 1239 protected UriType reference; 1240 1241 /** 1242 * Indicates an action that has been taken or is committed to reduce or 1243 * eliminate the likelihood of the risk identified by the detected issue from 1244 * manifesting. Can also reflect an observation of known mitigating factors that 1245 * may reduce/eliminate the need for any action. 1246 */ 1247 @Child(name = "mitigation", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1248 @Description(shortDefinition = "Step taken to address", formalDefinition = "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.") 1249 protected List<DetectedIssueMitigationComponent> mitigation; 1250 1251 private static final long serialVersionUID = 1404426283L; 1252 1253 /** 1254 * Constructor 1255 */ 1256 public DetectedIssue() { 1257 super(); 1258 } 1259 1260 /** 1261 * Constructor 1262 */ 1263 public DetectedIssue(Enumeration<DetectedIssueStatus> status) { 1264 super(); 1265 this.status = status; 1266 } 1267 1268 /** 1269 * @return {@link #identifier} (Business identifier associated with the detected 1270 * issue record.) 1271 */ 1272 public List<Identifier> getIdentifier() { 1273 if (this.identifier == null) 1274 this.identifier = new ArrayList<Identifier>(); 1275 return this.identifier; 1276 } 1277 1278 /** 1279 * @return Returns a reference to <code>this</code> for easy method chaining 1280 */ 1281 public DetectedIssue setIdentifier(List<Identifier> theIdentifier) { 1282 this.identifier = theIdentifier; 1283 return this; 1284 } 1285 1286 public boolean hasIdentifier() { 1287 if (this.identifier == null) 1288 return false; 1289 for (Identifier item : this.identifier) 1290 if (!item.isEmpty()) 1291 return true; 1292 return false; 1293 } 1294 1295 public Identifier addIdentifier() { // 3 1296 Identifier t = new Identifier(); 1297 if (this.identifier == null) 1298 this.identifier = new ArrayList<Identifier>(); 1299 this.identifier.add(t); 1300 return t; 1301 } 1302 1303 public DetectedIssue addIdentifier(Identifier t) { // 3 1304 if (t == null) 1305 return this; 1306 if (this.identifier == null) 1307 this.identifier = new ArrayList<Identifier>(); 1308 this.identifier.add(t); 1309 return this; 1310 } 1311 1312 /** 1313 * @return The first repetition of repeating field {@link #identifier}, creating 1314 * it if it does not already exist 1315 */ 1316 public Identifier getIdentifierFirstRep() { 1317 if (getIdentifier().isEmpty()) { 1318 addIdentifier(); 1319 } 1320 return getIdentifier().get(0); 1321 } 1322 1323 /** 1324 * @return {@link #status} (Indicates the status of the detected issue.). This 1325 * is the underlying object with id, value and extensions. The accessor 1326 * "getStatus" gives direct access to the value 1327 */ 1328 public Enumeration<DetectedIssueStatus> getStatusElement() { 1329 if (this.status == null) 1330 if (Configuration.errorOnAutoCreate()) 1331 throw new Error("Attempt to auto-create DetectedIssue.status"); 1332 else if (Configuration.doAutoCreate()) 1333 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); // bb 1334 return this.status; 1335 } 1336 1337 public boolean hasStatusElement() { 1338 return this.status != null && !this.status.isEmpty(); 1339 } 1340 1341 public boolean hasStatus() { 1342 return this.status != null && !this.status.isEmpty(); 1343 } 1344 1345 /** 1346 * @param value {@link #status} (Indicates the status of the detected issue.). 1347 * This is the underlying object with id, value and extensions. The 1348 * accessor "getStatus" gives direct access to the value 1349 */ 1350 public DetectedIssue setStatusElement(Enumeration<DetectedIssueStatus> value) { 1351 this.status = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return Indicates the status of the detected issue. 1357 */ 1358 public DetectedIssueStatus getStatus() { 1359 return this.status == null ? null : this.status.getValue(); 1360 } 1361 1362 /** 1363 * @param value Indicates the status of the detected issue. 1364 */ 1365 public DetectedIssue setStatus(DetectedIssueStatus value) { 1366 if (this.status == null) 1367 this.status = new Enumeration<DetectedIssueStatus>(new DetectedIssueStatusEnumFactory()); 1368 this.status.setValue(value); 1369 return this; 1370 } 1371 1372 /** 1373 * @return {@link #code} (Identifies the general type of issue identified.) 1374 */ 1375 public CodeableConcept getCode() { 1376 if (this.code == null) 1377 if (Configuration.errorOnAutoCreate()) 1378 throw new Error("Attempt to auto-create DetectedIssue.code"); 1379 else if (Configuration.doAutoCreate()) 1380 this.code = new CodeableConcept(); // cc 1381 return this.code; 1382 } 1383 1384 public boolean hasCode() { 1385 return this.code != null && !this.code.isEmpty(); 1386 } 1387 1388 /** 1389 * @param value {@link #code} (Identifies the general type of issue identified.) 1390 */ 1391 public DetectedIssue setCode(CodeableConcept value) { 1392 this.code = value; 1393 return this; 1394 } 1395 1396 /** 1397 * @return {@link #severity} (Indicates the degree of importance associated with 1398 * the identified issue based on the potential impact on the patient.). 1399 * This is the underlying object with id, value and extensions. The 1400 * accessor "getSeverity" gives direct access to the value 1401 */ 1402 public Enumeration<DetectedIssueSeverity> getSeverityElement() { 1403 if (this.severity == null) 1404 if (Configuration.errorOnAutoCreate()) 1405 throw new Error("Attempt to auto-create DetectedIssue.severity"); 1406 else if (Configuration.doAutoCreate()) 1407 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); // bb 1408 return this.severity; 1409 } 1410 1411 public boolean hasSeverityElement() { 1412 return this.severity != null && !this.severity.isEmpty(); 1413 } 1414 1415 public boolean hasSeverity() { 1416 return this.severity != null && !this.severity.isEmpty(); 1417 } 1418 1419 /** 1420 * @param value {@link #severity} (Indicates the degree of importance associated 1421 * with the identified issue based on the potential impact on the 1422 * patient.). This is the underlying object with id, value and 1423 * extensions. The accessor "getSeverity" gives direct access to 1424 * the value 1425 */ 1426 public DetectedIssue setSeverityElement(Enumeration<DetectedIssueSeverity> value) { 1427 this.severity = value; 1428 return this; 1429 } 1430 1431 /** 1432 * @return Indicates the degree of importance associated with the identified 1433 * issue based on the potential impact on the patient. 1434 */ 1435 public DetectedIssueSeverity getSeverity() { 1436 return this.severity == null ? null : this.severity.getValue(); 1437 } 1438 1439 /** 1440 * @param value Indicates the degree of importance associated with the 1441 * identified issue based on the potential impact on the patient. 1442 */ 1443 public DetectedIssue setSeverity(DetectedIssueSeverity value) { 1444 if (value == null) 1445 this.severity = null; 1446 else { 1447 if (this.severity == null) 1448 this.severity = new Enumeration<DetectedIssueSeverity>(new DetectedIssueSeverityEnumFactory()); 1449 this.severity.setValue(value); 1450 } 1451 return this; 1452 } 1453 1454 /** 1455 * @return {@link #patient} (Indicates the patient whose record the detected 1456 * issue is associated with.) 1457 */ 1458 public Reference getPatient() { 1459 if (this.patient == null) 1460 if (Configuration.errorOnAutoCreate()) 1461 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1462 else if (Configuration.doAutoCreate()) 1463 this.patient = new Reference(); // cc 1464 return this.patient; 1465 } 1466 1467 public boolean hasPatient() { 1468 return this.patient != null && !this.patient.isEmpty(); 1469 } 1470 1471 /** 1472 * @param value {@link #patient} (Indicates the patient whose record the 1473 * detected issue is associated with.) 1474 */ 1475 public DetectedIssue setPatient(Reference value) { 1476 this.patient = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return {@link #patient} The actual object that is the target of the 1482 * reference. The reference library doesn't populate this, but you can 1483 * use it to hold the resource if you resolve it. (Indicates the patient 1484 * whose record the detected issue is associated with.) 1485 */ 1486 public Patient getPatientTarget() { 1487 if (this.patientTarget == null) 1488 if (Configuration.errorOnAutoCreate()) 1489 throw new Error("Attempt to auto-create DetectedIssue.patient"); 1490 else if (Configuration.doAutoCreate()) 1491 this.patientTarget = new Patient(); // aa 1492 return this.patientTarget; 1493 } 1494 1495 /** 1496 * @param value {@link #patient} The actual object that is the target of the 1497 * reference. The reference library doesn't use these, but you can 1498 * use it to hold the resource if you resolve it. (Indicates the 1499 * patient whose record the detected issue is associated with.) 1500 */ 1501 public DetectedIssue setPatientTarget(Patient value) { 1502 this.patientTarget = value; 1503 return this; 1504 } 1505 1506 /** 1507 * @return {@link #identified} (The date or period when the detected issue was 1508 * initially identified.) 1509 */ 1510 public Type getIdentified() { 1511 return this.identified; 1512 } 1513 1514 /** 1515 * @return {@link #identified} (The date or period when the detected issue was 1516 * initially identified.) 1517 */ 1518 public DateTimeType getIdentifiedDateTimeType() throws FHIRException { 1519 if (this.identified == null) 1520 this.identified = new DateTimeType(); 1521 if (!(this.identified instanceof DateTimeType)) 1522 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1523 + this.identified.getClass().getName() + " was encountered"); 1524 return (DateTimeType) this.identified; 1525 } 1526 1527 public boolean hasIdentifiedDateTimeType() { 1528 return this.identified instanceof DateTimeType; 1529 } 1530 1531 /** 1532 * @return {@link #identified} (The date or period when the detected issue was 1533 * initially identified.) 1534 */ 1535 public Period getIdentifiedPeriod() throws FHIRException { 1536 if (this.identified == null) 1537 this.identified = new Period(); 1538 if (!(this.identified instanceof Period)) 1539 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.identified.getClass().getName() 1540 + " was encountered"); 1541 return (Period) this.identified; 1542 } 1543 1544 public boolean hasIdentifiedPeriod() { 1545 return this.identified instanceof Period; 1546 } 1547 1548 public boolean hasIdentified() { 1549 return this.identified != null && !this.identified.isEmpty(); 1550 } 1551 1552 /** 1553 * @param value {@link #identified} (The date or period when the detected issue 1554 * was initially identified.) 1555 */ 1556 public DetectedIssue setIdentified(Type value) { 1557 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1558 throw new Error("Not the right type for DetectedIssue.identified[x]: " + value.fhirType()); 1559 this.identified = value; 1560 return this; 1561 } 1562 1563 /** 1564 * @return {@link #author} (Individual or device responsible for the issue being 1565 * raised. For example, a decision support application or a pharmacist 1566 * conducting a medication review.) 1567 */ 1568 public Reference getAuthor() { 1569 if (this.author == null) 1570 if (Configuration.errorOnAutoCreate()) 1571 throw new Error("Attempt to auto-create DetectedIssue.author"); 1572 else if (Configuration.doAutoCreate()) 1573 this.author = new Reference(); // cc 1574 return this.author; 1575 } 1576 1577 public boolean hasAuthor() { 1578 return this.author != null && !this.author.isEmpty(); 1579 } 1580 1581 /** 1582 * @param value {@link #author} (Individual or device responsible for the issue 1583 * being raised. For example, a decision support application or a 1584 * pharmacist conducting a medication review.) 1585 */ 1586 public DetectedIssue setAuthor(Reference value) { 1587 this.author = value; 1588 return this; 1589 } 1590 1591 /** 1592 * @return {@link #author} The actual object that is the target of the 1593 * reference. The reference library doesn't populate this, but you can 1594 * use it to hold the resource if you resolve it. (Individual or device 1595 * responsible for the issue being raised. For example, a decision 1596 * support application or a pharmacist conducting a medication review.) 1597 */ 1598 public Resource getAuthorTarget() { 1599 return this.authorTarget; 1600 } 1601 1602 /** 1603 * @param value {@link #author} The actual object that is the target of the 1604 * reference. The reference library doesn't use these, but you can 1605 * use it to hold the resource if you resolve it. (Individual or 1606 * device responsible for the issue being raised. For example, a 1607 * decision support application or a pharmacist conducting a 1608 * medication review.) 1609 */ 1610 public DetectedIssue setAuthorTarget(Resource value) { 1611 this.authorTarget = value; 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #implicated} (Indicates the resource representing the current 1617 * activity or proposed activity that is potentially problematic.) 1618 */ 1619 public List<Reference> getImplicated() { 1620 if (this.implicated == null) 1621 this.implicated = new ArrayList<Reference>(); 1622 return this.implicated; 1623 } 1624 1625 /** 1626 * @return Returns a reference to <code>this</code> for easy method chaining 1627 */ 1628 public DetectedIssue setImplicated(List<Reference> theImplicated) { 1629 this.implicated = theImplicated; 1630 return this; 1631 } 1632 1633 public boolean hasImplicated() { 1634 if (this.implicated == null) 1635 return false; 1636 for (Reference item : this.implicated) 1637 if (!item.isEmpty()) 1638 return true; 1639 return false; 1640 } 1641 1642 public Reference addImplicated() { // 3 1643 Reference t = new Reference(); 1644 if (this.implicated == null) 1645 this.implicated = new ArrayList<Reference>(); 1646 this.implicated.add(t); 1647 return t; 1648 } 1649 1650 public DetectedIssue addImplicated(Reference t) { // 3 1651 if (t == null) 1652 return this; 1653 if (this.implicated == null) 1654 this.implicated = new ArrayList<Reference>(); 1655 this.implicated.add(t); 1656 return this; 1657 } 1658 1659 /** 1660 * @return The first repetition of repeating field {@link #implicated}, creating 1661 * it if it does not already exist 1662 */ 1663 public Reference getImplicatedFirstRep() { 1664 if (getImplicated().isEmpty()) { 1665 addImplicated(); 1666 } 1667 return getImplicated().get(0); 1668 } 1669 1670 /** 1671 * @return {@link #evidence} (Supporting evidence or manifestations that provide 1672 * the basis for identifying the detected issue such as a 1673 * GuidanceResponse or MeasureReport.) 1674 */ 1675 public List<DetectedIssueEvidenceComponent> getEvidence() { 1676 if (this.evidence == null) 1677 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1678 return this.evidence; 1679 } 1680 1681 /** 1682 * @return Returns a reference to <code>this</code> for easy method chaining 1683 */ 1684 public DetectedIssue setEvidence(List<DetectedIssueEvidenceComponent> theEvidence) { 1685 this.evidence = theEvidence; 1686 return this; 1687 } 1688 1689 public boolean hasEvidence() { 1690 if (this.evidence == null) 1691 return false; 1692 for (DetectedIssueEvidenceComponent item : this.evidence) 1693 if (!item.isEmpty()) 1694 return true; 1695 return false; 1696 } 1697 1698 public DetectedIssueEvidenceComponent addEvidence() { // 3 1699 DetectedIssueEvidenceComponent t = new DetectedIssueEvidenceComponent(); 1700 if (this.evidence == null) 1701 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1702 this.evidence.add(t); 1703 return t; 1704 } 1705 1706 public DetectedIssue addEvidence(DetectedIssueEvidenceComponent t) { // 3 1707 if (t == null) 1708 return this; 1709 if (this.evidence == null) 1710 this.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 1711 this.evidence.add(t); 1712 return this; 1713 } 1714 1715 /** 1716 * @return The first repetition of repeating field {@link #evidence}, creating 1717 * it if it does not already exist 1718 */ 1719 public DetectedIssueEvidenceComponent getEvidenceFirstRep() { 1720 if (getEvidence().isEmpty()) { 1721 addEvidence(); 1722 } 1723 return getEvidence().get(0); 1724 } 1725 1726 /** 1727 * @return {@link #detail} (A textual explanation of the detected issue.). This 1728 * is the underlying object with id, value and extensions. The accessor 1729 * "getDetail" gives direct access to the value 1730 */ 1731 public StringType getDetailElement() { 1732 if (this.detail == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create DetectedIssue.detail"); 1735 else if (Configuration.doAutoCreate()) 1736 this.detail = new StringType(); // bb 1737 return this.detail; 1738 } 1739 1740 public boolean hasDetailElement() { 1741 return this.detail != null && !this.detail.isEmpty(); 1742 } 1743 1744 public boolean hasDetail() { 1745 return this.detail != null && !this.detail.isEmpty(); 1746 } 1747 1748 /** 1749 * @param value {@link #detail} (A textual explanation of the detected issue.). 1750 * This is the underlying object with id, value and extensions. The 1751 * accessor "getDetail" gives direct access to the value 1752 */ 1753 public DetectedIssue setDetailElement(StringType value) { 1754 this.detail = value; 1755 return this; 1756 } 1757 1758 /** 1759 * @return A textual explanation of the detected issue. 1760 */ 1761 public String getDetail() { 1762 return this.detail == null ? null : this.detail.getValue(); 1763 } 1764 1765 /** 1766 * @param value A textual explanation of the detected issue. 1767 */ 1768 public DetectedIssue setDetail(String value) { 1769 if (Utilities.noString(value)) 1770 this.detail = null; 1771 else { 1772 if (this.detail == null) 1773 this.detail = new StringType(); 1774 this.detail.setValue(value); 1775 } 1776 return this; 1777 } 1778 1779 /** 1780 * @return {@link #reference} (The literature, knowledge-base or similar 1781 * reference that describes the propensity for the detected issue 1782 * identified.). This is the underlying object with id, value and 1783 * extensions. The accessor "getReference" gives direct access to the 1784 * value 1785 */ 1786 public UriType getReferenceElement() { 1787 if (this.reference == null) 1788 if (Configuration.errorOnAutoCreate()) 1789 throw new Error("Attempt to auto-create DetectedIssue.reference"); 1790 else if (Configuration.doAutoCreate()) 1791 this.reference = new UriType(); // bb 1792 return this.reference; 1793 } 1794 1795 public boolean hasReferenceElement() { 1796 return this.reference != null && !this.reference.isEmpty(); 1797 } 1798 1799 public boolean hasReference() { 1800 return this.reference != null && !this.reference.isEmpty(); 1801 } 1802 1803 /** 1804 * @param value {@link #reference} (The literature, knowledge-base or similar 1805 * reference that describes the propensity for the detected issue 1806 * identified.). This is the underlying object with id, value and 1807 * extensions. The accessor "getReference" gives direct access to 1808 * the value 1809 */ 1810 public DetectedIssue setReferenceElement(UriType value) { 1811 this.reference = value; 1812 return this; 1813 } 1814 1815 /** 1816 * @return The literature, knowledge-base or similar reference that describes 1817 * the propensity for the detected issue identified. 1818 */ 1819 public String getReference() { 1820 return this.reference == null ? null : this.reference.getValue(); 1821 } 1822 1823 /** 1824 * @param value The literature, knowledge-base or similar reference that 1825 * describes the propensity for the detected issue identified. 1826 */ 1827 public DetectedIssue setReference(String value) { 1828 if (Utilities.noString(value)) 1829 this.reference = null; 1830 else { 1831 if (this.reference == null) 1832 this.reference = new UriType(); 1833 this.reference.setValue(value); 1834 } 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #mitigation} (Indicates an action that has been taken or is 1840 * committed to reduce or eliminate the likelihood of the risk 1841 * identified by the detected issue from manifesting. Can also reflect 1842 * an observation of known mitigating factors that may reduce/eliminate 1843 * the need for any action.) 1844 */ 1845 public List<DetectedIssueMitigationComponent> getMitigation() { 1846 if (this.mitigation == null) 1847 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1848 return this.mitigation; 1849 } 1850 1851 /** 1852 * @return Returns a reference to <code>this</code> for easy method chaining 1853 */ 1854 public DetectedIssue setMitigation(List<DetectedIssueMitigationComponent> theMitigation) { 1855 this.mitigation = theMitigation; 1856 return this; 1857 } 1858 1859 public boolean hasMitigation() { 1860 if (this.mitigation == null) 1861 return false; 1862 for (DetectedIssueMitigationComponent item : this.mitigation) 1863 if (!item.isEmpty()) 1864 return true; 1865 return false; 1866 } 1867 1868 public DetectedIssueMitigationComponent addMitigation() { // 3 1869 DetectedIssueMitigationComponent t = new DetectedIssueMitigationComponent(); 1870 if (this.mitigation == null) 1871 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1872 this.mitigation.add(t); 1873 return t; 1874 } 1875 1876 public DetectedIssue addMitigation(DetectedIssueMitigationComponent t) { // 3 1877 if (t == null) 1878 return this; 1879 if (this.mitigation == null) 1880 this.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 1881 this.mitigation.add(t); 1882 return this; 1883 } 1884 1885 /** 1886 * @return The first repetition of repeating field {@link #mitigation}, creating 1887 * it if it does not already exist 1888 */ 1889 public DetectedIssueMitigationComponent getMitigationFirstRep() { 1890 if (getMitigation().isEmpty()) { 1891 addMitigation(); 1892 } 1893 return getMitigation().get(0); 1894 } 1895 1896 protected void listChildren(List<Property> children) { 1897 super.listChildren(children); 1898 children.add(new Property("identifier", "Identifier", 1899 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1900 children.add(new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status)); 1901 children 1902 .add(new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1, code)); 1903 children.add(new Property("severity", "code", 1904 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1905 0, 1, severity)); 1906 children.add(new Property("patient", "Reference(Patient)", 1907 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient)); 1908 children.add(new Property("identified[x]", "dateTime|Period", 1909 "The date or period when the detected issue was initially identified.", 0, 1, identified)); 1910 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1911 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1912 0, 1, author)); 1913 children.add(new Property("implicated", "Reference(Any)", 1914 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1915 0, java.lang.Integer.MAX_VALUE, implicated)); 1916 children.add(new Property("evidence", "", 1917 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1918 0, java.lang.Integer.MAX_VALUE, evidence)); 1919 children.add(new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, detail)); 1920 children.add(new Property("reference", "uri", 1921 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1922 0, 1, reference)); 1923 children.add(new Property("mitigation", "", 1924 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1925 0, java.lang.Integer.MAX_VALUE, mitigation)); 1926 } 1927 1928 @Override 1929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1930 switch (_hash) { 1931 case -1618432855: 1932 /* identifier */ return new Property("identifier", "Identifier", 1933 "Business identifier associated with the detected issue record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1934 case -892481550: 1935 /* status */ return new Property("status", "code", "Indicates the status of the detected issue.", 0, 1, status); 1936 case 3059181: 1937 /* code */ return new Property("code", "CodeableConcept", "Identifies the general type of issue identified.", 0, 1938 1, code); 1939 case 1478300413: 1940 /* severity */ return new Property("severity", "code", 1941 "Indicates the degree of importance associated with the identified issue based on the potential impact on the patient.", 1942 0, 1, severity); 1943 case -791418107: 1944 /* patient */ return new Property("patient", "Reference(Patient)", 1945 "Indicates the patient whose record the detected issue is associated with.", 0, 1, patient); 1946 case 569355781: 1947 /* identified[x] */ return new Property("identified[x]", "dateTime|Period", 1948 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1949 case -1618432869: 1950 /* identified */ return new Property("identified[x]", "dateTime|Period", 1951 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1952 case -968788650: 1953 /* identifiedDateTime */ return new Property("identified[x]", "dateTime|Period", 1954 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1955 case 520482364: 1956 /* identifiedPeriod */ return new Property("identified[x]", "dateTime|Period", 1957 "The date or period when the detected issue was initially identified.", 0, 1, identified); 1958 case -1406328437: 1959 /* author */ return new Property("author", "Reference(Practitioner|PractitionerRole|Device)", 1960 "Individual or device responsible for the issue being raised. For example, a decision support application or a pharmacist conducting a medication review.", 1961 0, 1, author); 1962 case -810216884: 1963 /* implicated */ return new Property("implicated", "Reference(Any)", 1964 "Indicates the resource representing the current activity or proposed activity that is potentially problematic.", 1965 0, java.lang.Integer.MAX_VALUE, implicated); 1966 case 382967383: 1967 /* evidence */ return new Property("evidence", "", 1968 "Supporting evidence or manifestations that provide the basis for identifying the detected issue such as a GuidanceResponse or MeasureReport.", 1969 0, java.lang.Integer.MAX_VALUE, evidence); 1970 case -1335224239: 1971 /* detail */ return new Property("detail", "string", "A textual explanation of the detected issue.", 0, 1, 1972 detail); 1973 case -925155509: 1974 /* reference */ return new Property("reference", "uri", 1975 "The literature, knowledge-base or similar reference that describes the propensity for the detected issue identified.", 1976 0, 1, reference); 1977 case 1293793087: 1978 /* mitigation */ return new Property("mitigation", "", 1979 "Indicates an action that has been taken or is committed to reduce or eliminate the likelihood of the risk identified by the detected issue from manifesting. Can also reflect an observation of known mitigating factors that may reduce/eliminate the need for any action.", 1980 0, java.lang.Integer.MAX_VALUE, mitigation); 1981 default: 1982 return super.getNamedProperty(_hash, _name, _checkValid); 1983 } 1984 1985 } 1986 1987 @Override 1988 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1989 switch (hash) { 1990 case -1618432855: 1991 /* identifier */ return this.identifier == null ? new Base[0] 1992 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1993 case -892481550: 1994 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DetectedIssueStatus> 1995 case 3059181: 1996 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1997 case 1478300413: 1998 /* severity */ return this.severity == null ? new Base[0] : new Base[] { this.severity }; // Enumeration<DetectedIssueSeverity> 1999 case -791418107: 2000 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2001 case -1618432869: 2002 /* identified */ return this.identified == null ? new Base[0] : new Base[] { this.identified }; // Type 2003 case -1406328437: 2004 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 2005 case -810216884: 2006 /* implicated */ return this.implicated == null ? new Base[0] 2007 : this.implicated.toArray(new Base[this.implicated.size()]); // Reference 2008 case 382967383: 2009 /* evidence */ return this.evidence == null ? new Base[0] : this.evidence.toArray(new Base[this.evidence.size()]); // DetectedIssueEvidenceComponent 2010 case -1335224239: 2011 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // StringType 2012 case -925155509: 2013 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 2014 case 1293793087: 2015 /* mitigation */ return this.mitigation == null ? new Base[0] 2016 : this.mitigation.toArray(new Base[this.mitigation.size()]); // DetectedIssueMitigationComponent 2017 default: 2018 return super.getProperty(hash, name, checkValid); 2019 } 2020 2021 } 2022 2023 @Override 2024 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2025 switch (hash) { 2026 case -1618432855: // identifier 2027 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2028 return value; 2029 case -892481550: // status 2030 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2031 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2032 return value; 2033 case 3059181: // code 2034 this.code = castToCodeableConcept(value); // CodeableConcept 2035 return value; 2036 case 1478300413: // severity 2037 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2038 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2039 return value; 2040 case -791418107: // patient 2041 this.patient = castToReference(value); // Reference 2042 return value; 2043 case -1618432869: // identified 2044 this.identified = castToType(value); // Type 2045 return value; 2046 case -1406328437: // author 2047 this.author = castToReference(value); // Reference 2048 return value; 2049 case -810216884: // implicated 2050 this.getImplicated().add(castToReference(value)); // Reference 2051 return value; 2052 case 382967383: // evidence 2053 this.getEvidence().add((DetectedIssueEvidenceComponent) value); // DetectedIssueEvidenceComponent 2054 return value; 2055 case -1335224239: // detail 2056 this.detail = castToString(value); // StringType 2057 return value; 2058 case -925155509: // reference 2059 this.reference = castToUri(value); // UriType 2060 return value; 2061 case 1293793087: // mitigation 2062 this.getMitigation().add((DetectedIssueMitigationComponent) value); // DetectedIssueMitigationComponent 2063 return value; 2064 default: 2065 return super.setProperty(hash, name, value); 2066 } 2067 2068 } 2069 2070 @Override 2071 public Base setProperty(String name, Base value) throws FHIRException { 2072 if (name.equals("identifier")) { 2073 this.getIdentifier().add(castToIdentifier(value)); 2074 } else if (name.equals("status")) { 2075 value = new DetectedIssueStatusEnumFactory().fromType(castToCode(value)); 2076 this.status = (Enumeration) value; // Enumeration<DetectedIssueStatus> 2077 } else if (name.equals("code")) { 2078 this.code = castToCodeableConcept(value); // CodeableConcept 2079 } else if (name.equals("severity")) { 2080 value = new DetectedIssueSeverityEnumFactory().fromType(castToCode(value)); 2081 this.severity = (Enumeration) value; // Enumeration<DetectedIssueSeverity> 2082 } else if (name.equals("patient")) { 2083 this.patient = castToReference(value); // Reference 2084 } else if (name.equals("identified[x]")) { 2085 this.identified = castToType(value); // Type 2086 } else if (name.equals("author")) { 2087 this.author = castToReference(value); // Reference 2088 } else if (name.equals("implicated")) { 2089 this.getImplicated().add(castToReference(value)); 2090 } else if (name.equals("evidence")) { 2091 this.getEvidence().add((DetectedIssueEvidenceComponent) value); 2092 } else if (name.equals("detail")) { 2093 this.detail = castToString(value); // StringType 2094 } else if (name.equals("reference")) { 2095 this.reference = castToUri(value); // UriType 2096 } else if (name.equals("mitigation")) { 2097 this.getMitigation().add((DetectedIssueMitigationComponent) value); 2098 } else 2099 return super.setProperty(name, value); 2100 return value; 2101 } 2102 2103 @Override 2104 public void removeChild(String name, Base value) throws FHIRException { 2105 if (name.equals("identifier")) { 2106 this.getIdentifier().remove(castToIdentifier(value)); 2107 } else if (name.equals("status")) { 2108 this.status = null; 2109 } else if (name.equals("code")) { 2110 this.code = null; 2111 } else if (name.equals("severity")) { 2112 this.severity = null; 2113 } else if (name.equals("patient")) { 2114 this.patient = null; 2115 } else if (name.equals("identified[x]")) { 2116 this.identified = null; 2117 } else if (name.equals("author")) { 2118 this.author = null; 2119 } else if (name.equals("implicated")) { 2120 this.getImplicated().remove(castToReference(value)); 2121 } else if (name.equals("evidence")) { 2122 this.getEvidence().remove((DetectedIssueEvidenceComponent) value); 2123 } else if (name.equals("detail")) { 2124 this.detail = null; 2125 } else if (name.equals("reference")) { 2126 this.reference = null; 2127 } else if (name.equals("mitigation")) { 2128 this.getMitigation().remove((DetectedIssueMitigationComponent) value); 2129 } else 2130 super.removeChild(name, value); 2131 2132 } 2133 2134 @Override 2135 public Base makeProperty(int hash, String name) throws FHIRException { 2136 switch (hash) { 2137 case -1618432855: 2138 return addIdentifier(); 2139 case -892481550: 2140 return getStatusElement(); 2141 case 3059181: 2142 return getCode(); 2143 case 1478300413: 2144 return getSeverityElement(); 2145 case -791418107: 2146 return getPatient(); 2147 case 569355781: 2148 return getIdentified(); 2149 case -1618432869: 2150 return getIdentified(); 2151 case -1406328437: 2152 return getAuthor(); 2153 case -810216884: 2154 return addImplicated(); 2155 case 382967383: 2156 return addEvidence(); 2157 case -1335224239: 2158 return getDetailElement(); 2159 case -925155509: 2160 return getReferenceElement(); 2161 case 1293793087: 2162 return addMitigation(); 2163 default: 2164 return super.makeProperty(hash, name); 2165 } 2166 2167 } 2168 2169 @Override 2170 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2171 switch (hash) { 2172 case -1618432855: 2173 /* identifier */ return new String[] { "Identifier" }; 2174 case -892481550: 2175 /* status */ return new String[] { "code" }; 2176 case 3059181: 2177 /* code */ return new String[] { "CodeableConcept" }; 2178 case 1478300413: 2179 /* severity */ return new String[] { "code" }; 2180 case -791418107: 2181 /* patient */ return new String[] { "Reference" }; 2182 case -1618432869: 2183 /* identified */ return new String[] { "dateTime", "Period" }; 2184 case -1406328437: 2185 /* author */ return new String[] { "Reference" }; 2186 case -810216884: 2187 /* implicated */ return new String[] { "Reference" }; 2188 case 382967383: 2189 /* evidence */ return new String[] {}; 2190 case -1335224239: 2191 /* detail */ return new String[] { "string" }; 2192 case -925155509: 2193 /* reference */ return new String[] { "uri" }; 2194 case 1293793087: 2195 /* mitigation */ return new String[] {}; 2196 default: 2197 return super.getTypesForProperty(hash, name); 2198 } 2199 2200 } 2201 2202 @Override 2203 public Base addChild(String name) throws FHIRException { 2204 if (name.equals("identifier")) { 2205 return addIdentifier(); 2206 } else if (name.equals("status")) { 2207 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.status"); 2208 } else if (name.equals("code")) { 2209 this.code = new CodeableConcept(); 2210 return this.code; 2211 } else if (name.equals("severity")) { 2212 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.severity"); 2213 } else if (name.equals("patient")) { 2214 this.patient = new Reference(); 2215 return this.patient; 2216 } else if (name.equals("identifiedDateTime")) { 2217 this.identified = new DateTimeType(); 2218 return this.identified; 2219 } else if (name.equals("identifiedPeriod")) { 2220 this.identified = new Period(); 2221 return this.identified; 2222 } else if (name.equals("author")) { 2223 this.author = new Reference(); 2224 return this.author; 2225 } else if (name.equals("implicated")) { 2226 return addImplicated(); 2227 } else if (name.equals("evidence")) { 2228 return addEvidence(); 2229 } else if (name.equals("detail")) { 2230 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.detail"); 2231 } else if (name.equals("reference")) { 2232 throw new FHIRException("Cannot call addChild on a singleton property DetectedIssue.reference"); 2233 } else if (name.equals("mitigation")) { 2234 return addMitigation(); 2235 } else 2236 return super.addChild(name); 2237 } 2238 2239 public String fhirType() { 2240 return "DetectedIssue"; 2241 2242 } 2243 2244 public DetectedIssue copy() { 2245 DetectedIssue dst = new DetectedIssue(); 2246 copyValues(dst); 2247 return dst; 2248 } 2249 2250 public void copyValues(DetectedIssue dst) { 2251 super.copyValues(dst); 2252 if (identifier != null) { 2253 dst.identifier = new ArrayList<Identifier>(); 2254 for (Identifier i : identifier) 2255 dst.identifier.add(i.copy()); 2256 } 2257 ; 2258 dst.status = status == null ? null : status.copy(); 2259 dst.code = code == null ? null : code.copy(); 2260 dst.severity = severity == null ? null : severity.copy(); 2261 dst.patient = patient == null ? null : patient.copy(); 2262 dst.identified = identified == null ? null : identified.copy(); 2263 dst.author = author == null ? null : author.copy(); 2264 if (implicated != null) { 2265 dst.implicated = new ArrayList<Reference>(); 2266 for (Reference i : implicated) 2267 dst.implicated.add(i.copy()); 2268 } 2269 ; 2270 if (evidence != null) { 2271 dst.evidence = new ArrayList<DetectedIssueEvidenceComponent>(); 2272 for (DetectedIssueEvidenceComponent i : evidence) 2273 dst.evidence.add(i.copy()); 2274 } 2275 ; 2276 dst.detail = detail == null ? null : detail.copy(); 2277 dst.reference = reference == null ? null : reference.copy(); 2278 if (mitigation != null) { 2279 dst.mitigation = new ArrayList<DetectedIssueMitigationComponent>(); 2280 for (DetectedIssueMitigationComponent i : mitigation) 2281 dst.mitigation.add(i.copy()); 2282 } 2283 ; 2284 } 2285 2286 protected DetectedIssue typedCopy() { 2287 return copy(); 2288 } 2289 2290 @Override 2291 public boolean equalsDeep(Base other_) { 2292 if (!super.equalsDeep(other_)) 2293 return false; 2294 if (!(other_ instanceof DetectedIssue)) 2295 return false; 2296 DetectedIssue o = (DetectedIssue) other_; 2297 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2298 && compareDeep(code, o.code, true) && compareDeep(severity, o.severity, true) 2299 && compareDeep(patient, o.patient, true) && compareDeep(identified, o.identified, true) 2300 && compareDeep(author, o.author, true) && compareDeep(implicated, o.implicated, true) 2301 && compareDeep(evidence, o.evidence, true) && compareDeep(detail, o.detail, true) 2302 && compareDeep(reference, o.reference, true) && compareDeep(mitigation, o.mitigation, true); 2303 } 2304 2305 @Override 2306 public boolean equalsShallow(Base other_) { 2307 if (!super.equalsShallow(other_)) 2308 return false; 2309 if (!(other_ instanceof DetectedIssue)) 2310 return false; 2311 DetectedIssue o = (DetectedIssue) other_; 2312 return compareValues(status, o.status, true) && compareValues(severity, o.severity, true) 2313 && compareValues(detail, o.detail, true) && compareValues(reference, o.reference, true); 2314 } 2315 2316 public boolean isEmpty() { 2317 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, code, severity, patient, 2318 identified, author, implicated, evidence, detail, reference, mitigation); 2319 } 2320 2321 @Override 2322 public ResourceType getResourceType() { 2323 return ResourceType.DetectedIssue; 2324 } 2325 2326 /** 2327 * Search parameter: <b>identifier</b> 2328 * <p> 2329 * Description: <b>Unique id for the detected issue</b><br> 2330 * Type: <b>token</b><br> 2331 * Path: <b>DetectedIssue.identifier</b><br> 2332 * </p> 2333 */ 2334 @SearchParamDefinition(name = "identifier", path = "DetectedIssue.identifier", description = "Unique id for the detected issue", type = "token") 2335 public static final String SP_IDENTIFIER = "identifier"; 2336 /** 2337 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2338 * <p> 2339 * Description: <b>Unique id for the detected issue</b><br> 2340 * Type: <b>token</b><br> 2341 * Path: <b>DetectedIssue.identifier</b><br> 2342 * </p> 2343 */ 2344 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2345 SP_IDENTIFIER); 2346 2347 /** 2348 * Search parameter: <b>code</b> 2349 * <p> 2350 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2351 * etc.</b><br> 2352 * Type: <b>token</b><br> 2353 * Path: <b>DetectedIssue.code</b><br> 2354 * </p> 2355 */ 2356 @SearchParamDefinition(name = "code", path = "DetectedIssue.code", description = "Issue Category, e.g. drug-drug, duplicate therapy, etc.", type = "token") 2357 public static final String SP_CODE = "code"; 2358 /** 2359 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2360 * <p> 2361 * Description: <b>Issue Category, e.g. drug-drug, duplicate therapy, 2362 * etc.</b><br> 2363 * Type: <b>token</b><br> 2364 * Path: <b>DetectedIssue.code</b><br> 2365 * </p> 2366 */ 2367 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2368 SP_CODE); 2369 2370 /** 2371 * Search parameter: <b>identified</b> 2372 * <p> 2373 * Description: <b>When identified</b><br> 2374 * Type: <b>date</b><br> 2375 * Path: <b>DetectedIssue.identified[x]</b><br> 2376 * </p> 2377 */ 2378 @SearchParamDefinition(name = "identified", path = "DetectedIssue.identified", description = "When identified", type = "date") 2379 public static final String SP_IDENTIFIED = "identified"; 2380 /** 2381 * <b>Fluent Client</b> search parameter constant for <b>identified</b> 2382 * <p> 2383 * Description: <b>When identified</b><br> 2384 * Type: <b>date</b><br> 2385 * Path: <b>DetectedIssue.identified[x]</b><br> 2386 * </p> 2387 */ 2388 public static final ca.uhn.fhir.rest.gclient.DateClientParam IDENTIFIED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2389 SP_IDENTIFIED); 2390 2391 /** 2392 * Search parameter: <b>patient</b> 2393 * <p> 2394 * Description: <b>Associated patient</b><br> 2395 * Type: <b>reference</b><br> 2396 * Path: <b>DetectedIssue.patient</b><br> 2397 * </p> 2398 */ 2399 @SearchParamDefinition(name = "patient", path = "DetectedIssue.patient", description = "Associated patient", type = "reference", providesMembershipIn = { 2400 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2401 public static final String SP_PATIENT = "patient"; 2402 /** 2403 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2404 * <p> 2405 * Description: <b>Associated patient</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>DetectedIssue.patient</b><br> 2408 * </p> 2409 */ 2410 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2411 SP_PATIENT); 2412 2413 /** 2414 * Constant for fluent queries to be used to add include statements. Specifies 2415 * the path value of "<b>DetectedIssue:patient</b>". 2416 */ 2417 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2418 "DetectedIssue:patient").toLocked(); 2419 2420 /** 2421 * Search parameter: <b>author</b> 2422 * <p> 2423 * Description: <b>The provider or device that identified the issue</b><br> 2424 * Type: <b>reference</b><br> 2425 * Path: <b>DetectedIssue.author</b><br> 2426 * </p> 2427 */ 2428 @SearchParamDefinition(name = "author", path = "DetectedIssue.author", description = "The provider or device that identified the issue", type = "reference", providesMembershipIn = { 2429 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2430 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 2431 Practitioner.class, PractitionerRole.class }) 2432 public static final String SP_AUTHOR = "author"; 2433 /** 2434 * <b>Fluent Client</b> search parameter constant for <b>author</b> 2435 * <p> 2436 * Description: <b>The provider or device that identified the issue</b><br> 2437 * Type: <b>reference</b><br> 2438 * Path: <b>DetectedIssue.author</b><br> 2439 * </p> 2440 */ 2441 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2442 SP_AUTHOR); 2443 2444 /** 2445 * Constant for fluent queries to be used to add include statements. Specifies 2446 * the path value of "<b>DetectedIssue:author</b>". 2447 */ 2448 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 2449 "DetectedIssue:author").toLocked(); 2450 2451 /** 2452 * Search parameter: <b>implicated</b> 2453 * <p> 2454 * Description: <b>Problem resource</b><br> 2455 * Type: <b>reference</b><br> 2456 * Path: <b>DetectedIssue.implicated</b><br> 2457 * </p> 2458 */ 2459 @SearchParamDefinition(name = "implicated", path = "DetectedIssue.implicated", description = "Problem resource", type = "reference") 2460 public static final String SP_IMPLICATED = "implicated"; 2461 /** 2462 * <b>Fluent Client</b> search parameter constant for <b>implicated</b> 2463 * <p> 2464 * Description: <b>Problem resource</b><br> 2465 * Type: <b>reference</b><br> 2466 * Path: <b>DetectedIssue.implicated</b><br> 2467 * </p> 2468 */ 2469 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMPLICATED = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2470 SP_IMPLICATED); 2471 2472 /** 2473 * Constant for fluent queries to be used to add include statements. Specifies 2474 * the path value of "<b>DetectedIssue:implicated</b>". 2475 */ 2476 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMPLICATED = new ca.uhn.fhir.model.api.Include( 2477 "DetectedIssue:implicated").toLocked(); 2478 2479}