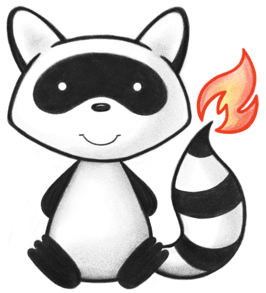
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The characteristics, operational status and capabilities of a medical-related 048 * component of a medical device. 049 */ 050@ResourceDef(name = "DeviceDefinition", profile = "http://hl7.org/fhir/StructureDefinition/DeviceDefinition") 051public class DeviceDefinition extends DomainResource { 052 053 public enum DeviceNameType { 054 /** 055 * UDI Label name. 056 */ 057 UDILABELNAME, 058 /** 059 * User Friendly name. 060 */ 061 USERFRIENDLYNAME, 062 /** 063 * Patient Reported name. 064 */ 065 PATIENTREPORTEDNAME, 066 /** 067 * Manufacturer name. 068 */ 069 MANUFACTURERNAME, 070 /** 071 * Model name. 072 */ 073 MODELNAME, 074 /** 075 * other. 076 */ 077 OTHER, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static DeviceNameType fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("udi-label-name".equals(codeString)) 087 return UDILABELNAME; 088 if ("user-friendly-name".equals(codeString)) 089 return USERFRIENDLYNAME; 090 if ("patient-reported-name".equals(codeString)) 091 return PATIENTREPORTEDNAME; 092 if ("manufacturer-name".equals(codeString)) 093 return MANUFACTURERNAME; 094 if ("model-name".equals(codeString)) 095 return MODELNAME; 096 if ("other".equals(codeString)) 097 return OTHER; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown DeviceNameType code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case UDILABELNAME: 107 return "udi-label-name"; 108 case USERFRIENDLYNAME: 109 return "user-friendly-name"; 110 case PATIENTREPORTEDNAME: 111 return "patient-reported-name"; 112 case MANUFACTURERNAME: 113 return "manufacturer-name"; 114 case MODELNAME: 115 return "model-name"; 116 case OTHER: 117 return "other"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getSystem() { 126 switch (this) { 127 case UDILABELNAME: 128 return "http://hl7.org/fhir/device-nametype"; 129 case USERFRIENDLYNAME: 130 return "http://hl7.org/fhir/device-nametype"; 131 case PATIENTREPORTEDNAME: 132 return "http://hl7.org/fhir/device-nametype"; 133 case MANUFACTURERNAME: 134 return "http://hl7.org/fhir/device-nametype"; 135 case MODELNAME: 136 return "http://hl7.org/fhir/device-nametype"; 137 case OTHER: 138 return "http://hl7.org/fhir/device-nametype"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDefinition() { 147 switch (this) { 148 case UDILABELNAME: 149 return "UDI Label name."; 150 case USERFRIENDLYNAME: 151 return "User Friendly name."; 152 case PATIENTREPORTEDNAME: 153 return "Patient Reported name."; 154 case MANUFACTURERNAME: 155 return "Manufacturer name."; 156 case MODELNAME: 157 return "Model name."; 158 case OTHER: 159 return "other."; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDisplay() { 168 switch (this) { 169 case UDILABELNAME: 170 return "UDI Label name"; 171 case USERFRIENDLYNAME: 172 return "User Friendly name"; 173 case PATIENTREPORTEDNAME: 174 return "Patient Reported name"; 175 case MANUFACTURERNAME: 176 return "Manufacturer name"; 177 case MODELNAME: 178 return "Model name"; 179 case OTHER: 180 return "other"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 } 188 189 public static class DeviceNameTypeEnumFactory implements EnumFactory<DeviceNameType> { 190 public DeviceNameType fromCode(String codeString) throws IllegalArgumentException { 191 if (codeString == null || "".equals(codeString)) 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("udi-label-name".equals(codeString)) 195 return DeviceNameType.UDILABELNAME; 196 if ("user-friendly-name".equals(codeString)) 197 return DeviceNameType.USERFRIENDLYNAME; 198 if ("patient-reported-name".equals(codeString)) 199 return DeviceNameType.PATIENTREPORTEDNAME; 200 if ("manufacturer-name".equals(codeString)) 201 return DeviceNameType.MANUFACTURERNAME; 202 if ("model-name".equals(codeString)) 203 return DeviceNameType.MODELNAME; 204 if ("other".equals(codeString)) 205 return DeviceNameType.OTHER; 206 throw new IllegalArgumentException("Unknown DeviceNameType code '" + codeString + "'"); 207 } 208 209 public Enumeration<DeviceNameType> fromType(PrimitiveType<?> code) throws FHIRException { 210 if (code == null) 211 return null; 212 if (code.isEmpty()) 213 return new Enumeration<DeviceNameType>(this, DeviceNameType.NULL, code); 214 String codeString = code.asStringValue(); 215 if (codeString == null || "".equals(codeString)) 216 return new Enumeration<DeviceNameType>(this, DeviceNameType.NULL, code); 217 if ("udi-label-name".equals(codeString)) 218 return new Enumeration<DeviceNameType>(this, DeviceNameType.UDILABELNAME, code); 219 if ("user-friendly-name".equals(codeString)) 220 return new Enumeration<DeviceNameType>(this, DeviceNameType.USERFRIENDLYNAME, code); 221 if ("patient-reported-name".equals(codeString)) 222 return new Enumeration<DeviceNameType>(this, DeviceNameType.PATIENTREPORTEDNAME, code); 223 if ("manufacturer-name".equals(codeString)) 224 return new Enumeration<DeviceNameType>(this, DeviceNameType.MANUFACTURERNAME, code); 225 if ("model-name".equals(codeString)) 226 return new Enumeration<DeviceNameType>(this, DeviceNameType.MODELNAME, code); 227 if ("other".equals(codeString)) 228 return new Enumeration<DeviceNameType>(this, DeviceNameType.OTHER, code); 229 throw new FHIRException("Unknown DeviceNameType code '" + codeString + "'"); 230 } 231 232 public String toCode(DeviceNameType code) { 233 if (code == DeviceNameType.UDILABELNAME) 234 return "udi-label-name"; 235 if (code == DeviceNameType.USERFRIENDLYNAME) 236 return "user-friendly-name"; 237 if (code == DeviceNameType.PATIENTREPORTEDNAME) 238 return "patient-reported-name"; 239 if (code == DeviceNameType.MANUFACTURERNAME) 240 return "manufacturer-name"; 241 if (code == DeviceNameType.MODELNAME) 242 return "model-name"; 243 if (code == DeviceNameType.OTHER) 244 return "other"; 245 return "?"; 246 } 247 248 public String toSystem(DeviceNameType code) { 249 return code.getSystem(); 250 } 251 } 252 253 @Block() 254 public static class DeviceDefinitionUdiDeviceIdentifierComponent extends BackboneElement 255 implements IBaseBackboneElement { 256 /** 257 * The identifier that is to be associated with every Device that references 258 * this DeviceDefintiion for the issuer and jurisdication porvided in the 259 * DeviceDefinition.udiDeviceIdentifier. 260 */ 261 @Child(name = "deviceIdentifier", type = { 262 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 263 @Description(shortDefinition = "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdication porvided in the DeviceDefinition.udiDeviceIdentifier", formalDefinition = "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdication porvided in the DeviceDefinition.udiDeviceIdentifier.") 264 protected StringType deviceIdentifier; 265 266 /** 267 * The organization that assigns the identifier algorithm. 268 */ 269 @Child(name = "issuer", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 270 @Description(shortDefinition = "The organization that assigns the identifier algorithm", formalDefinition = "The organization that assigns the identifier algorithm.") 271 protected UriType issuer; 272 273 /** 274 * The jurisdiction to which the deviceIdentifier applies. 275 */ 276 @Child(name = "jurisdiction", type = { 277 UriType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 278 @Description(shortDefinition = "The jurisdiction to which the deviceIdentifier applies", formalDefinition = "The jurisdiction to which the deviceIdentifier applies.") 279 protected UriType jurisdiction; 280 281 private static final long serialVersionUID = -1577319218L; 282 283 /** 284 * Constructor 285 */ 286 public DeviceDefinitionUdiDeviceIdentifierComponent() { 287 super(); 288 } 289 290 /** 291 * Constructor 292 */ 293 public DeviceDefinitionUdiDeviceIdentifierComponent(StringType deviceIdentifier, UriType issuer, 294 UriType jurisdiction) { 295 super(); 296 this.deviceIdentifier = deviceIdentifier; 297 this.issuer = issuer; 298 this.jurisdiction = jurisdiction; 299 } 300 301 /** 302 * @return {@link #deviceIdentifier} (The identifier that is to be associated 303 * with every Device that references this DeviceDefintiion for the 304 * issuer and jurisdication porvided in the 305 * DeviceDefinition.udiDeviceIdentifier.). This is the underlying object 306 * with id, value and extensions. The accessor "getDeviceIdentifier" 307 * gives direct access to the value 308 */ 309 public StringType getDeviceIdentifierElement() { 310 if (this.deviceIdentifier == null) 311 if (Configuration.errorOnAutoCreate()) 312 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.deviceIdentifier"); 313 else if (Configuration.doAutoCreate()) 314 this.deviceIdentifier = new StringType(); // bb 315 return this.deviceIdentifier; 316 } 317 318 public boolean hasDeviceIdentifierElement() { 319 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 320 } 321 322 public boolean hasDeviceIdentifier() { 323 return this.deviceIdentifier != null && !this.deviceIdentifier.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #deviceIdentifier} (The identifier that is to be 328 * associated with every Device that references this 329 * DeviceDefintiion for the issuer and jurisdication porvided in 330 * the DeviceDefinition.udiDeviceIdentifier.). This is the 331 * underlying object with id, value and extensions. The accessor 332 * "getDeviceIdentifier" gives direct access to the value 333 */ 334 public DeviceDefinitionUdiDeviceIdentifierComponent setDeviceIdentifierElement(StringType value) { 335 this.deviceIdentifier = value; 336 return this; 337 } 338 339 /** 340 * @return The identifier that is to be associated with every Device that 341 * references this DeviceDefintiion for the issuer and jurisdication 342 * porvided in the DeviceDefinition.udiDeviceIdentifier. 343 */ 344 public String getDeviceIdentifier() { 345 return this.deviceIdentifier == null ? null : this.deviceIdentifier.getValue(); 346 } 347 348 /** 349 * @param value The identifier that is to be associated with every Device that 350 * references this DeviceDefintiion for the issuer and 351 * jurisdication porvided in the 352 * DeviceDefinition.udiDeviceIdentifier. 353 */ 354 public DeviceDefinitionUdiDeviceIdentifierComponent setDeviceIdentifier(String value) { 355 if (this.deviceIdentifier == null) 356 this.deviceIdentifier = new StringType(); 357 this.deviceIdentifier.setValue(value); 358 return this; 359 } 360 361 /** 362 * @return {@link #issuer} (The organization that assigns the identifier 363 * algorithm.). This is the underlying object with id, value and 364 * extensions. The accessor "getIssuer" gives direct access to the value 365 */ 366 public UriType getIssuerElement() { 367 if (this.issuer == null) 368 if (Configuration.errorOnAutoCreate()) 369 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.issuer"); 370 else if (Configuration.doAutoCreate()) 371 this.issuer = new UriType(); // bb 372 return this.issuer; 373 } 374 375 public boolean hasIssuerElement() { 376 return this.issuer != null && !this.issuer.isEmpty(); 377 } 378 379 public boolean hasIssuer() { 380 return this.issuer != null && !this.issuer.isEmpty(); 381 } 382 383 /** 384 * @param value {@link #issuer} (The organization that assigns the identifier 385 * algorithm.). This is the underlying object with id, value and 386 * extensions. The accessor "getIssuer" gives direct access to the 387 * value 388 */ 389 public DeviceDefinitionUdiDeviceIdentifierComponent setIssuerElement(UriType value) { 390 this.issuer = value; 391 return this; 392 } 393 394 /** 395 * @return The organization that assigns the identifier algorithm. 396 */ 397 public String getIssuer() { 398 return this.issuer == null ? null : this.issuer.getValue(); 399 } 400 401 /** 402 * @param value The organization that assigns the identifier algorithm. 403 */ 404 public DeviceDefinitionUdiDeviceIdentifierComponent setIssuer(String value) { 405 if (this.issuer == null) 406 this.issuer = new UriType(); 407 this.issuer.setValue(value); 408 return this; 409 } 410 411 /** 412 * @return {@link #jurisdiction} (The jurisdiction to which the deviceIdentifier 413 * applies.). This is the underlying object with id, value and 414 * extensions. The accessor "getJurisdiction" gives direct access to the 415 * value 416 */ 417 public UriType getJurisdictionElement() { 418 if (this.jurisdiction == null) 419 if (Configuration.errorOnAutoCreate()) 420 throw new Error("Attempt to auto-create DeviceDefinitionUdiDeviceIdentifierComponent.jurisdiction"); 421 else if (Configuration.doAutoCreate()) 422 this.jurisdiction = new UriType(); // bb 423 return this.jurisdiction; 424 } 425 426 public boolean hasJurisdictionElement() { 427 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 428 } 429 430 public boolean hasJurisdiction() { 431 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #jurisdiction} (The jurisdiction to which the 436 * deviceIdentifier applies.). This is the underlying object with 437 * id, value and extensions. The accessor "getJurisdiction" gives 438 * direct access to the value 439 */ 440 public DeviceDefinitionUdiDeviceIdentifierComponent setJurisdictionElement(UriType value) { 441 this.jurisdiction = value; 442 return this; 443 } 444 445 /** 446 * @return The jurisdiction to which the deviceIdentifier applies. 447 */ 448 public String getJurisdiction() { 449 return this.jurisdiction == null ? null : this.jurisdiction.getValue(); 450 } 451 452 /** 453 * @param value The jurisdiction to which the deviceIdentifier applies. 454 */ 455 public DeviceDefinitionUdiDeviceIdentifierComponent setJurisdiction(String value) { 456 if (this.jurisdiction == null) 457 this.jurisdiction = new UriType(); 458 this.jurisdiction.setValue(value); 459 return this; 460 } 461 462 protected void listChildren(List<Property> children) { 463 super.listChildren(children); 464 children.add(new Property("deviceIdentifier", "string", 465 "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdication porvided in the DeviceDefinition.udiDeviceIdentifier.", 466 0, 1, deviceIdentifier)); 467 children 468 .add(new Property("issuer", "uri", "The organization that assigns the identifier algorithm.", 0, 1, issuer)); 469 children.add(new Property("jurisdiction", "uri", "The jurisdiction to which the deviceIdentifier applies.", 0, 1, 470 jurisdiction)); 471 } 472 473 @Override 474 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 475 switch (_hash) { 476 case 1322005407: 477 /* deviceIdentifier */ return new Property("deviceIdentifier", "string", 478 "The identifier that is to be associated with every Device that references this DeviceDefintiion for the issuer and jurisdication porvided in the DeviceDefinition.udiDeviceIdentifier.", 479 0, 1, deviceIdentifier); 480 case -1179159879: 481 /* issuer */ return new Property("issuer", "uri", "The organization that assigns the identifier algorithm.", 0, 482 1, issuer); 483 case -507075711: 484 /* jurisdiction */ return new Property("jurisdiction", "uri", 485 "The jurisdiction to which the deviceIdentifier applies.", 0, 1, jurisdiction); 486 default: 487 return super.getNamedProperty(_hash, _name, _checkValid); 488 } 489 490 } 491 492 @Override 493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 494 switch (hash) { 495 case 1322005407: 496 /* deviceIdentifier */ return this.deviceIdentifier == null ? new Base[0] 497 : new Base[] { this.deviceIdentifier }; // StringType 498 case -1179159879: 499 /* issuer */ return this.issuer == null ? new Base[0] : new Base[] { this.issuer }; // UriType 500 case -507075711: 501 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] : new Base[] { this.jurisdiction }; // UriType 502 default: 503 return super.getProperty(hash, name, checkValid); 504 } 505 506 } 507 508 @Override 509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 510 switch (hash) { 511 case 1322005407: // deviceIdentifier 512 this.deviceIdentifier = castToString(value); // StringType 513 return value; 514 case -1179159879: // issuer 515 this.issuer = castToUri(value); // UriType 516 return value; 517 case -507075711: // jurisdiction 518 this.jurisdiction = castToUri(value); // UriType 519 return value; 520 default: 521 return super.setProperty(hash, name, value); 522 } 523 524 } 525 526 @Override 527 public Base setProperty(String name, Base value) throws FHIRException { 528 if (name.equals("deviceIdentifier")) { 529 this.deviceIdentifier = castToString(value); // StringType 530 } else if (name.equals("issuer")) { 531 this.issuer = castToUri(value); // UriType 532 } else if (name.equals("jurisdiction")) { 533 this.jurisdiction = castToUri(value); // UriType 534 } else 535 return super.setProperty(name, value); 536 return value; 537 } 538 539 @Override 540 public Base makeProperty(int hash, String name) throws FHIRException { 541 switch (hash) { 542 case 1322005407: 543 return getDeviceIdentifierElement(); 544 case -1179159879: 545 return getIssuerElement(); 546 case -507075711: 547 return getJurisdictionElement(); 548 default: 549 return super.makeProperty(hash, name); 550 } 551 552 } 553 554 @Override 555 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 556 switch (hash) { 557 case 1322005407: 558 /* deviceIdentifier */ return new String[] { "string" }; 559 case -1179159879: 560 /* issuer */ return new String[] { "uri" }; 561 case -507075711: 562 /* jurisdiction */ return new String[] { "uri" }; 563 default: 564 return super.getTypesForProperty(hash, name); 565 } 566 567 } 568 569 @Override 570 public Base addChild(String name) throws FHIRException { 571 if (name.equals("deviceIdentifier")) { 572 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.deviceIdentifier"); 573 } else if (name.equals("issuer")) { 574 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.issuer"); 575 } else if (name.equals("jurisdiction")) { 576 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.jurisdiction"); 577 } else 578 return super.addChild(name); 579 } 580 581 public DeviceDefinitionUdiDeviceIdentifierComponent copy() { 582 DeviceDefinitionUdiDeviceIdentifierComponent dst = new DeviceDefinitionUdiDeviceIdentifierComponent(); 583 copyValues(dst); 584 return dst; 585 } 586 587 public void copyValues(DeviceDefinitionUdiDeviceIdentifierComponent dst) { 588 super.copyValues(dst); 589 dst.deviceIdentifier = deviceIdentifier == null ? null : deviceIdentifier.copy(); 590 dst.issuer = issuer == null ? null : issuer.copy(); 591 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 592 } 593 594 @Override 595 public boolean equalsDeep(Base other_) { 596 if (!super.equalsDeep(other_)) 597 return false; 598 if (!(other_ instanceof DeviceDefinitionUdiDeviceIdentifierComponent)) 599 return false; 600 DeviceDefinitionUdiDeviceIdentifierComponent o = (DeviceDefinitionUdiDeviceIdentifierComponent) other_; 601 return compareDeep(deviceIdentifier, o.deviceIdentifier, true) && compareDeep(issuer, o.issuer, true) 602 && compareDeep(jurisdiction, o.jurisdiction, true); 603 } 604 605 @Override 606 public boolean equalsShallow(Base other_) { 607 if (!super.equalsShallow(other_)) 608 return false; 609 if (!(other_ instanceof DeviceDefinitionUdiDeviceIdentifierComponent)) 610 return false; 611 DeviceDefinitionUdiDeviceIdentifierComponent o = (DeviceDefinitionUdiDeviceIdentifierComponent) other_; 612 return compareValues(deviceIdentifier, o.deviceIdentifier, true) && compareValues(issuer, o.issuer, true) 613 && compareValues(jurisdiction, o.jurisdiction, true); 614 } 615 616 public boolean isEmpty() { 617 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(deviceIdentifier, issuer, jurisdiction); 618 } 619 620 public String fhirType() { 621 return "DeviceDefinition.udiDeviceIdentifier"; 622 623 } 624 625 } 626 627 @Block() 628 public static class DeviceDefinitionDeviceNameComponent extends BackboneElement implements IBaseBackboneElement { 629 /** 630 * The name of the device. 631 */ 632 @Child(name = "name", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 633 @Description(shortDefinition = "The name of the device", formalDefinition = "The name of the device.") 634 protected StringType name; 635 636 /** 637 * The type of deviceName. UDILabelName | UserFriendlyName | PatientReportedName 638 * | ManufactureDeviceName | ModelName. 639 */ 640 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 641 @Description(shortDefinition = "udi-label-name | user-friendly-name | patient-reported-name | manufacturer-name | model-name | other", formalDefinition = "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.") 642 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-nametype") 643 protected Enumeration<DeviceNameType> type; 644 645 private static final long serialVersionUID = 918983440L; 646 647 /** 648 * Constructor 649 */ 650 public DeviceDefinitionDeviceNameComponent() { 651 super(); 652 } 653 654 /** 655 * Constructor 656 */ 657 public DeviceDefinitionDeviceNameComponent(StringType name, Enumeration<DeviceNameType> type) { 658 super(); 659 this.name = name; 660 this.type = type; 661 } 662 663 /** 664 * @return {@link #name} (The name of the device.). This is the underlying 665 * object with id, value and extensions. The accessor "getName" gives 666 * direct access to the value 667 */ 668 public StringType getNameElement() { 669 if (this.name == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create DeviceDefinitionDeviceNameComponent.name"); 672 else if (Configuration.doAutoCreate()) 673 this.name = new StringType(); // bb 674 return this.name; 675 } 676 677 public boolean hasNameElement() { 678 return this.name != null && !this.name.isEmpty(); 679 } 680 681 public boolean hasName() { 682 return this.name != null && !this.name.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #name} (The name of the device.). This is the underlying 687 * object with id, value and extensions. The accessor "getName" 688 * gives direct access to the value 689 */ 690 public DeviceDefinitionDeviceNameComponent setNameElement(StringType value) { 691 this.name = value; 692 return this; 693 } 694 695 /** 696 * @return The name of the device. 697 */ 698 public String getName() { 699 return this.name == null ? null : this.name.getValue(); 700 } 701 702 /** 703 * @param value The name of the device. 704 */ 705 public DeviceDefinitionDeviceNameComponent setName(String value) { 706 if (this.name == null) 707 this.name = new StringType(); 708 this.name.setValue(value); 709 return this; 710 } 711 712 /** 713 * @return {@link #type} (The type of deviceName. UDILabelName | 714 * UserFriendlyName | PatientReportedName | ManufactureDeviceName | 715 * ModelName.). This is the underlying object with id, value and 716 * extensions. The accessor "getType" gives direct access to the value 717 */ 718 public Enumeration<DeviceNameType> getTypeElement() { 719 if (this.type == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create DeviceDefinitionDeviceNameComponent.type"); 722 else if (Configuration.doAutoCreate()) 723 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); // bb 724 return this.type; 725 } 726 727 public boolean hasTypeElement() { 728 return this.type != null && !this.type.isEmpty(); 729 } 730 731 public boolean hasType() { 732 return this.type != null && !this.type.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #type} (The type of deviceName. UDILabelName | 737 * UserFriendlyName | PatientReportedName | ManufactureDeviceName | 738 * ModelName.). This is the underlying object with id, value and 739 * extensions. The accessor "getType" gives direct access to the 740 * value 741 */ 742 public DeviceDefinitionDeviceNameComponent setTypeElement(Enumeration<DeviceNameType> value) { 743 this.type = value; 744 return this; 745 } 746 747 /** 748 * @return The type of deviceName. UDILabelName | UserFriendlyName | 749 * PatientReportedName | ManufactureDeviceName | ModelName. 750 */ 751 public DeviceNameType getType() { 752 return this.type == null ? null : this.type.getValue(); 753 } 754 755 /** 756 * @param value The type of deviceName. UDILabelName | UserFriendlyName | 757 * PatientReportedName | ManufactureDeviceName | ModelName. 758 */ 759 public DeviceDefinitionDeviceNameComponent setType(DeviceNameType value) { 760 if (this.type == null) 761 this.type = new Enumeration<DeviceNameType>(new DeviceNameTypeEnumFactory()); 762 this.type.setValue(value); 763 return this; 764 } 765 766 protected void listChildren(List<Property> children) { 767 super.listChildren(children); 768 children.add(new Property("name", "string", "The name of the device.", 0, 1, name)); 769 children.add(new Property("type", "code", 770 "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.", 771 0, 1, type)); 772 } 773 774 @Override 775 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 776 switch (_hash) { 777 case 3373707: 778 /* name */ return new Property("name", "string", "The name of the device.", 0, 1, name); 779 case 3575610: 780 /* type */ return new Property("type", "code", 781 "The type of deviceName.\nUDILabelName | UserFriendlyName | PatientReportedName | ManufactureDeviceName | ModelName.", 782 0, 1, type); 783 default: 784 return super.getNamedProperty(_hash, _name, _checkValid); 785 } 786 787 } 788 789 @Override 790 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 791 switch (hash) { 792 case 3373707: 793 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 794 case 3575610: 795 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<DeviceNameType> 796 default: 797 return super.getProperty(hash, name, checkValid); 798 } 799 800 } 801 802 @Override 803 public Base setProperty(int hash, String name, Base value) throws FHIRException { 804 switch (hash) { 805 case 3373707: // name 806 this.name = castToString(value); // StringType 807 return value; 808 case 3575610: // type 809 value = new DeviceNameTypeEnumFactory().fromType(castToCode(value)); 810 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 811 return value; 812 default: 813 return super.setProperty(hash, name, value); 814 } 815 816 } 817 818 @Override 819 public Base setProperty(String name, Base value) throws FHIRException { 820 if (name.equals("name")) { 821 this.name = castToString(value); // StringType 822 } else if (name.equals("type")) { 823 value = new DeviceNameTypeEnumFactory().fromType(castToCode(value)); 824 this.type = (Enumeration) value; // Enumeration<DeviceNameType> 825 } else 826 return super.setProperty(name, value); 827 return value; 828 } 829 830 @Override 831 public Base makeProperty(int hash, String name) throws FHIRException { 832 switch (hash) { 833 case 3373707: 834 return getNameElement(); 835 case 3575610: 836 return getTypeElement(); 837 default: 838 return super.makeProperty(hash, name); 839 } 840 841 } 842 843 @Override 844 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 845 switch (hash) { 846 case 3373707: 847 /* name */ return new String[] { "string" }; 848 case 3575610: 849 /* type */ return new String[] { "code" }; 850 default: 851 return super.getTypesForProperty(hash, name); 852 } 853 854 } 855 856 @Override 857 public Base addChild(String name) throws FHIRException { 858 if (name.equals("name")) { 859 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.name"); 860 } else if (name.equals("type")) { 861 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.type"); 862 } else 863 return super.addChild(name); 864 } 865 866 public DeviceDefinitionDeviceNameComponent copy() { 867 DeviceDefinitionDeviceNameComponent dst = new DeviceDefinitionDeviceNameComponent(); 868 copyValues(dst); 869 return dst; 870 } 871 872 public void copyValues(DeviceDefinitionDeviceNameComponent dst) { 873 super.copyValues(dst); 874 dst.name = name == null ? null : name.copy(); 875 dst.type = type == null ? null : type.copy(); 876 } 877 878 @Override 879 public boolean equalsDeep(Base other_) { 880 if (!super.equalsDeep(other_)) 881 return false; 882 if (!(other_ instanceof DeviceDefinitionDeviceNameComponent)) 883 return false; 884 DeviceDefinitionDeviceNameComponent o = (DeviceDefinitionDeviceNameComponent) other_; 885 return compareDeep(name, o.name, true) && compareDeep(type, o.type, true); 886 } 887 888 @Override 889 public boolean equalsShallow(Base other_) { 890 if (!super.equalsShallow(other_)) 891 return false; 892 if (!(other_ instanceof DeviceDefinitionDeviceNameComponent)) 893 return false; 894 DeviceDefinitionDeviceNameComponent o = (DeviceDefinitionDeviceNameComponent) other_; 895 return compareValues(name, o.name, true) && compareValues(type, o.type, true); 896 } 897 898 public boolean isEmpty() { 899 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, type); 900 } 901 902 public String fhirType() { 903 return "DeviceDefinition.deviceName"; 904 905 } 906 907 } 908 909 @Block() 910 public static class DeviceDefinitionSpecializationComponent extends BackboneElement implements IBaseBackboneElement { 911 /** 912 * The standard that is used to operate and communicate. 913 */ 914 @Child(name = "systemType", type = { 915 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 916 @Description(shortDefinition = "The standard that is used to operate and communicate", formalDefinition = "The standard that is used to operate and communicate.") 917 protected StringType systemType; 918 919 /** 920 * The version of the standard that is used to operate and communicate. 921 */ 922 @Child(name = "version", type = { 923 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 924 @Description(shortDefinition = "The version of the standard that is used to operate and communicate", formalDefinition = "The version of the standard that is used to operate and communicate.") 925 protected StringType version; 926 927 private static final long serialVersionUID = -249304393L; 928 929 /** 930 * Constructor 931 */ 932 public DeviceDefinitionSpecializationComponent() { 933 super(); 934 } 935 936 /** 937 * Constructor 938 */ 939 public DeviceDefinitionSpecializationComponent(StringType systemType) { 940 super(); 941 this.systemType = systemType; 942 } 943 944 /** 945 * @return {@link #systemType} (The standard that is used to operate and 946 * communicate.). This is the underlying object with id, value and 947 * extensions. The accessor "getSystemType" gives direct access to the 948 * value 949 */ 950 public StringType getSystemTypeElement() { 951 if (this.systemType == null) 952 if (Configuration.errorOnAutoCreate()) 953 throw new Error("Attempt to auto-create DeviceDefinitionSpecializationComponent.systemType"); 954 else if (Configuration.doAutoCreate()) 955 this.systemType = new StringType(); // bb 956 return this.systemType; 957 } 958 959 public boolean hasSystemTypeElement() { 960 return this.systemType != null && !this.systemType.isEmpty(); 961 } 962 963 public boolean hasSystemType() { 964 return this.systemType != null && !this.systemType.isEmpty(); 965 } 966 967 /** 968 * @param value {@link #systemType} (The standard that is used to operate and 969 * communicate.). This is the underlying object with id, value and 970 * extensions. The accessor "getSystemType" gives direct access to 971 * the value 972 */ 973 public DeviceDefinitionSpecializationComponent setSystemTypeElement(StringType value) { 974 this.systemType = value; 975 return this; 976 } 977 978 /** 979 * @return The standard that is used to operate and communicate. 980 */ 981 public String getSystemType() { 982 return this.systemType == null ? null : this.systemType.getValue(); 983 } 984 985 /** 986 * @param value The standard that is used to operate and communicate. 987 */ 988 public DeviceDefinitionSpecializationComponent setSystemType(String value) { 989 if (this.systemType == null) 990 this.systemType = new StringType(); 991 this.systemType.setValue(value); 992 return this; 993 } 994 995 /** 996 * @return {@link #version} (The version of the standard that is used to operate 997 * and communicate.). This is the underlying object with id, value and 998 * extensions. The accessor "getVersion" gives direct access to the 999 * value 1000 */ 1001 public StringType getVersionElement() { 1002 if (this.version == null) 1003 if (Configuration.errorOnAutoCreate()) 1004 throw new Error("Attempt to auto-create DeviceDefinitionSpecializationComponent.version"); 1005 else if (Configuration.doAutoCreate()) 1006 this.version = new StringType(); // bb 1007 return this.version; 1008 } 1009 1010 public boolean hasVersionElement() { 1011 return this.version != null && !this.version.isEmpty(); 1012 } 1013 1014 public boolean hasVersion() { 1015 return this.version != null && !this.version.isEmpty(); 1016 } 1017 1018 /** 1019 * @param value {@link #version} (The version of the standard that is used to 1020 * operate and communicate.). This is the underlying object with 1021 * id, value and extensions. The accessor "getVersion" gives direct 1022 * access to the value 1023 */ 1024 public DeviceDefinitionSpecializationComponent setVersionElement(StringType value) { 1025 this.version = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return The version of the standard that is used to operate and communicate. 1031 */ 1032 public String getVersion() { 1033 return this.version == null ? null : this.version.getValue(); 1034 } 1035 1036 /** 1037 * @param value The version of the standard that is used to operate and 1038 * communicate. 1039 */ 1040 public DeviceDefinitionSpecializationComponent setVersion(String value) { 1041 if (Utilities.noString(value)) 1042 this.version = null; 1043 else { 1044 if (this.version == null) 1045 this.version = new StringType(); 1046 this.version.setValue(value); 1047 } 1048 return this; 1049 } 1050 1051 protected void listChildren(List<Property> children) { 1052 super.listChildren(children); 1053 children.add(new Property("systemType", "string", "The standard that is used to operate and communicate.", 0, 1, 1054 systemType)); 1055 children.add(new Property("version", "string", 1056 "The version of the standard that is used to operate and communicate.", 0, 1, version)); 1057 } 1058 1059 @Override 1060 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1061 switch (_hash) { 1062 case 642893321: 1063 /* systemType */ return new Property("systemType", "string", 1064 "The standard that is used to operate and communicate.", 0, 1, systemType); 1065 case 351608024: 1066 /* version */ return new Property("version", "string", 1067 "The version of the standard that is used to operate and communicate.", 0, 1, version); 1068 default: 1069 return super.getNamedProperty(_hash, _name, _checkValid); 1070 } 1071 1072 } 1073 1074 @Override 1075 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1076 switch (hash) { 1077 case 642893321: 1078 /* systemType */ return this.systemType == null ? new Base[0] : new Base[] { this.systemType }; // StringType 1079 case 351608024: 1080 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 1081 default: 1082 return super.getProperty(hash, name, checkValid); 1083 } 1084 1085 } 1086 1087 @Override 1088 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1089 switch (hash) { 1090 case 642893321: // systemType 1091 this.systemType = castToString(value); // StringType 1092 return value; 1093 case 351608024: // version 1094 this.version = castToString(value); // StringType 1095 return value; 1096 default: 1097 return super.setProperty(hash, name, value); 1098 } 1099 1100 } 1101 1102 @Override 1103 public Base setProperty(String name, Base value) throws FHIRException { 1104 if (name.equals("systemType")) { 1105 this.systemType = castToString(value); // StringType 1106 } else if (name.equals("version")) { 1107 this.version = castToString(value); // StringType 1108 } else 1109 return super.setProperty(name, value); 1110 return value; 1111 } 1112 1113 @Override 1114 public Base makeProperty(int hash, String name) throws FHIRException { 1115 switch (hash) { 1116 case 642893321: 1117 return getSystemTypeElement(); 1118 case 351608024: 1119 return getVersionElement(); 1120 default: 1121 return super.makeProperty(hash, name); 1122 } 1123 1124 } 1125 1126 @Override 1127 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1128 switch (hash) { 1129 case 642893321: 1130 /* systemType */ return new String[] { "string" }; 1131 case 351608024: 1132 /* version */ return new String[] { "string" }; 1133 default: 1134 return super.getTypesForProperty(hash, name); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base addChild(String name) throws FHIRException { 1141 if (name.equals("systemType")) { 1142 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.systemType"); 1143 } else if (name.equals("version")) { 1144 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.version"); 1145 } else 1146 return super.addChild(name); 1147 } 1148 1149 public DeviceDefinitionSpecializationComponent copy() { 1150 DeviceDefinitionSpecializationComponent dst = new DeviceDefinitionSpecializationComponent(); 1151 copyValues(dst); 1152 return dst; 1153 } 1154 1155 public void copyValues(DeviceDefinitionSpecializationComponent dst) { 1156 super.copyValues(dst); 1157 dst.systemType = systemType == null ? null : systemType.copy(); 1158 dst.version = version == null ? null : version.copy(); 1159 } 1160 1161 @Override 1162 public boolean equalsDeep(Base other_) { 1163 if (!super.equalsDeep(other_)) 1164 return false; 1165 if (!(other_ instanceof DeviceDefinitionSpecializationComponent)) 1166 return false; 1167 DeviceDefinitionSpecializationComponent o = (DeviceDefinitionSpecializationComponent) other_; 1168 return compareDeep(systemType, o.systemType, true) && compareDeep(version, o.version, true); 1169 } 1170 1171 @Override 1172 public boolean equalsShallow(Base other_) { 1173 if (!super.equalsShallow(other_)) 1174 return false; 1175 if (!(other_ instanceof DeviceDefinitionSpecializationComponent)) 1176 return false; 1177 DeviceDefinitionSpecializationComponent o = (DeviceDefinitionSpecializationComponent) other_; 1178 return compareValues(systemType, o.systemType, true) && compareValues(version, o.version, true); 1179 } 1180 1181 public boolean isEmpty() { 1182 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(systemType, version); 1183 } 1184 1185 public String fhirType() { 1186 return "DeviceDefinition.specialization"; 1187 1188 } 1189 1190 } 1191 1192 @Block() 1193 public static class DeviceDefinitionCapabilityComponent extends BackboneElement implements IBaseBackboneElement { 1194 /** 1195 * Type of capability. 1196 */ 1197 @Child(name = "type", type = { 1198 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1199 @Description(shortDefinition = "Type of capability", formalDefinition = "Type of capability.") 1200 protected CodeableConcept type; 1201 1202 /** 1203 * Description of capability. 1204 */ 1205 @Child(name = "description", type = { 1206 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1207 @Description(shortDefinition = "Description of capability", formalDefinition = "Description of capability.") 1208 protected List<CodeableConcept> description; 1209 1210 private static final long serialVersionUID = -192945344L; 1211 1212 /** 1213 * Constructor 1214 */ 1215 public DeviceDefinitionCapabilityComponent() { 1216 super(); 1217 } 1218 1219 /** 1220 * Constructor 1221 */ 1222 public DeviceDefinitionCapabilityComponent(CodeableConcept type) { 1223 super(); 1224 this.type = type; 1225 } 1226 1227 /** 1228 * @return {@link #type} (Type of capability.) 1229 */ 1230 public CodeableConcept getType() { 1231 if (this.type == null) 1232 if (Configuration.errorOnAutoCreate()) 1233 throw new Error("Attempt to auto-create DeviceDefinitionCapabilityComponent.type"); 1234 else if (Configuration.doAutoCreate()) 1235 this.type = new CodeableConcept(); // cc 1236 return this.type; 1237 } 1238 1239 public boolean hasType() { 1240 return this.type != null && !this.type.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #type} (Type of capability.) 1245 */ 1246 public DeviceDefinitionCapabilityComponent setType(CodeableConcept value) { 1247 this.type = value; 1248 return this; 1249 } 1250 1251 /** 1252 * @return {@link #description} (Description of capability.) 1253 */ 1254 public List<CodeableConcept> getDescription() { 1255 if (this.description == null) 1256 this.description = new ArrayList<CodeableConcept>(); 1257 return this.description; 1258 } 1259 1260 /** 1261 * @return Returns a reference to <code>this</code> for easy method chaining 1262 */ 1263 public DeviceDefinitionCapabilityComponent setDescription(List<CodeableConcept> theDescription) { 1264 this.description = theDescription; 1265 return this; 1266 } 1267 1268 public boolean hasDescription() { 1269 if (this.description == null) 1270 return false; 1271 for (CodeableConcept item : this.description) 1272 if (!item.isEmpty()) 1273 return true; 1274 return false; 1275 } 1276 1277 public CodeableConcept addDescription() { // 3 1278 CodeableConcept t = new CodeableConcept(); 1279 if (this.description == null) 1280 this.description = new ArrayList<CodeableConcept>(); 1281 this.description.add(t); 1282 return t; 1283 } 1284 1285 public DeviceDefinitionCapabilityComponent addDescription(CodeableConcept t) { // 3 1286 if (t == null) 1287 return this; 1288 if (this.description == null) 1289 this.description = new ArrayList<CodeableConcept>(); 1290 this.description.add(t); 1291 return this; 1292 } 1293 1294 /** 1295 * @return The first repetition of repeating field {@link #description}, 1296 * creating it if it does not already exist 1297 */ 1298 public CodeableConcept getDescriptionFirstRep() { 1299 if (getDescription().isEmpty()) { 1300 addDescription(); 1301 } 1302 return getDescription().get(0); 1303 } 1304 1305 protected void listChildren(List<Property> children) { 1306 super.listChildren(children); 1307 children.add(new Property("type", "CodeableConcept", "Type of capability.", 0, 1, type)); 1308 children.add(new Property("description", "CodeableConcept", "Description of capability.", 0, 1309 java.lang.Integer.MAX_VALUE, description)); 1310 } 1311 1312 @Override 1313 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1314 switch (_hash) { 1315 case 3575610: 1316 /* type */ return new Property("type", "CodeableConcept", "Type of capability.", 0, 1, type); 1317 case -1724546052: 1318 /* description */ return new Property("description", "CodeableConcept", "Description of capability.", 0, 1319 java.lang.Integer.MAX_VALUE, description); 1320 default: 1321 return super.getNamedProperty(_hash, _name, _checkValid); 1322 } 1323 1324 } 1325 1326 @Override 1327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1328 switch (hash) { 1329 case 3575610: 1330 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1331 case -1724546052: 1332 /* description */ return this.description == null ? new Base[0] 1333 : this.description.toArray(new Base[this.description.size()]); // CodeableConcept 1334 default: 1335 return super.getProperty(hash, name, checkValid); 1336 } 1337 1338 } 1339 1340 @Override 1341 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1342 switch (hash) { 1343 case 3575610: // type 1344 this.type = castToCodeableConcept(value); // CodeableConcept 1345 return value; 1346 case -1724546052: // description 1347 this.getDescription().add(castToCodeableConcept(value)); // CodeableConcept 1348 return value; 1349 default: 1350 return super.setProperty(hash, name, value); 1351 } 1352 1353 } 1354 1355 @Override 1356 public Base setProperty(String name, Base value) throws FHIRException { 1357 if (name.equals("type")) { 1358 this.type = castToCodeableConcept(value); // CodeableConcept 1359 } else if (name.equals("description")) { 1360 this.getDescription().add(castToCodeableConcept(value)); 1361 } else 1362 return super.setProperty(name, value); 1363 return value; 1364 } 1365 1366 @Override 1367 public Base makeProperty(int hash, String name) throws FHIRException { 1368 switch (hash) { 1369 case 3575610: 1370 return getType(); 1371 case -1724546052: 1372 return addDescription(); 1373 default: 1374 return super.makeProperty(hash, name); 1375 } 1376 1377 } 1378 1379 @Override 1380 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1381 switch (hash) { 1382 case 3575610: 1383 /* type */ return new String[] { "CodeableConcept" }; 1384 case -1724546052: 1385 /* description */ return new String[] { "CodeableConcept" }; 1386 default: 1387 return super.getTypesForProperty(hash, name); 1388 } 1389 1390 } 1391 1392 @Override 1393 public Base addChild(String name) throws FHIRException { 1394 if (name.equals("type")) { 1395 this.type = new CodeableConcept(); 1396 return this.type; 1397 } else if (name.equals("description")) { 1398 return addDescription(); 1399 } else 1400 return super.addChild(name); 1401 } 1402 1403 public DeviceDefinitionCapabilityComponent copy() { 1404 DeviceDefinitionCapabilityComponent dst = new DeviceDefinitionCapabilityComponent(); 1405 copyValues(dst); 1406 return dst; 1407 } 1408 1409 public void copyValues(DeviceDefinitionCapabilityComponent dst) { 1410 super.copyValues(dst); 1411 dst.type = type == null ? null : type.copy(); 1412 if (description != null) { 1413 dst.description = new ArrayList<CodeableConcept>(); 1414 for (CodeableConcept i : description) 1415 dst.description.add(i.copy()); 1416 } 1417 ; 1418 } 1419 1420 @Override 1421 public boolean equalsDeep(Base other_) { 1422 if (!super.equalsDeep(other_)) 1423 return false; 1424 if (!(other_ instanceof DeviceDefinitionCapabilityComponent)) 1425 return false; 1426 DeviceDefinitionCapabilityComponent o = (DeviceDefinitionCapabilityComponent) other_; 1427 return compareDeep(type, o.type, true) && compareDeep(description, o.description, true); 1428 } 1429 1430 @Override 1431 public boolean equalsShallow(Base other_) { 1432 if (!super.equalsShallow(other_)) 1433 return false; 1434 if (!(other_ instanceof DeviceDefinitionCapabilityComponent)) 1435 return false; 1436 DeviceDefinitionCapabilityComponent o = (DeviceDefinitionCapabilityComponent) other_; 1437 return true; 1438 } 1439 1440 public boolean isEmpty() { 1441 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, description); 1442 } 1443 1444 public String fhirType() { 1445 return "DeviceDefinition.capability"; 1446 1447 } 1448 1449 } 1450 1451 @Block() 1452 public static class DeviceDefinitionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 1453 /** 1454 * Code that specifies the property DeviceDefinitionPropetyCode (Extensible). 1455 */ 1456 @Child(name = "type", type = { 1457 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1458 @Description(shortDefinition = "Code that specifies the property DeviceDefinitionPropetyCode (Extensible)", formalDefinition = "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).") 1459 protected CodeableConcept type; 1460 1461 /** 1462 * Property value as a quantity. 1463 */ 1464 @Child(name = "valueQuantity", type = { 1465 Quantity.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1466 @Description(shortDefinition = "Property value as a quantity", formalDefinition = "Property value as a quantity.") 1467 protected List<Quantity> valueQuantity; 1468 1469 /** 1470 * Property value as a code, e.g., NTP4 (synced to NTP). 1471 */ 1472 @Child(name = "valueCode", type = { 1473 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1474 @Description(shortDefinition = "Property value as a code, e.g., NTP4 (synced to NTP)", formalDefinition = "Property value as a code, e.g., NTP4 (synced to NTP).") 1475 protected List<CodeableConcept> valueCode; 1476 1477 private static final long serialVersionUID = 1512172633L; 1478 1479 /** 1480 * Constructor 1481 */ 1482 public DeviceDefinitionPropertyComponent() { 1483 super(); 1484 } 1485 1486 /** 1487 * Constructor 1488 */ 1489 public DeviceDefinitionPropertyComponent(CodeableConcept type) { 1490 super(); 1491 this.type = type; 1492 } 1493 1494 /** 1495 * @return {@link #type} (Code that specifies the property 1496 * DeviceDefinitionPropetyCode (Extensible).) 1497 */ 1498 public CodeableConcept getType() { 1499 if (this.type == null) 1500 if (Configuration.errorOnAutoCreate()) 1501 throw new Error("Attempt to auto-create DeviceDefinitionPropertyComponent.type"); 1502 else if (Configuration.doAutoCreate()) 1503 this.type = new CodeableConcept(); // cc 1504 return this.type; 1505 } 1506 1507 public boolean hasType() { 1508 return this.type != null && !this.type.isEmpty(); 1509 } 1510 1511 /** 1512 * @param value {@link #type} (Code that specifies the property 1513 * DeviceDefinitionPropetyCode (Extensible).) 1514 */ 1515 public DeviceDefinitionPropertyComponent setType(CodeableConcept value) { 1516 this.type = value; 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #valueQuantity} (Property value as a quantity.) 1522 */ 1523 public List<Quantity> getValueQuantity() { 1524 if (this.valueQuantity == null) 1525 this.valueQuantity = new ArrayList<Quantity>(); 1526 return this.valueQuantity; 1527 } 1528 1529 /** 1530 * @return Returns a reference to <code>this</code> for easy method chaining 1531 */ 1532 public DeviceDefinitionPropertyComponent setValueQuantity(List<Quantity> theValueQuantity) { 1533 this.valueQuantity = theValueQuantity; 1534 return this; 1535 } 1536 1537 public boolean hasValueQuantity() { 1538 if (this.valueQuantity == null) 1539 return false; 1540 for (Quantity item : this.valueQuantity) 1541 if (!item.isEmpty()) 1542 return true; 1543 return false; 1544 } 1545 1546 public Quantity addValueQuantity() { // 3 1547 Quantity t = new Quantity(); 1548 if (this.valueQuantity == null) 1549 this.valueQuantity = new ArrayList<Quantity>(); 1550 this.valueQuantity.add(t); 1551 return t; 1552 } 1553 1554 public DeviceDefinitionPropertyComponent addValueQuantity(Quantity t) { // 3 1555 if (t == null) 1556 return this; 1557 if (this.valueQuantity == null) 1558 this.valueQuantity = new ArrayList<Quantity>(); 1559 this.valueQuantity.add(t); 1560 return this; 1561 } 1562 1563 /** 1564 * @return The first repetition of repeating field {@link #valueQuantity}, 1565 * creating it if it does not already exist 1566 */ 1567 public Quantity getValueQuantityFirstRep() { 1568 if (getValueQuantity().isEmpty()) { 1569 addValueQuantity(); 1570 } 1571 return getValueQuantity().get(0); 1572 } 1573 1574 /** 1575 * @return {@link #valueCode} (Property value as a code, e.g., NTP4 (synced to 1576 * NTP).) 1577 */ 1578 public List<CodeableConcept> getValueCode() { 1579 if (this.valueCode == null) 1580 this.valueCode = new ArrayList<CodeableConcept>(); 1581 return this.valueCode; 1582 } 1583 1584 /** 1585 * @return Returns a reference to <code>this</code> for easy method chaining 1586 */ 1587 public DeviceDefinitionPropertyComponent setValueCode(List<CodeableConcept> theValueCode) { 1588 this.valueCode = theValueCode; 1589 return this; 1590 } 1591 1592 public boolean hasValueCode() { 1593 if (this.valueCode == null) 1594 return false; 1595 for (CodeableConcept item : this.valueCode) 1596 if (!item.isEmpty()) 1597 return true; 1598 return false; 1599 } 1600 1601 public CodeableConcept addValueCode() { // 3 1602 CodeableConcept t = new CodeableConcept(); 1603 if (this.valueCode == null) 1604 this.valueCode = new ArrayList<CodeableConcept>(); 1605 this.valueCode.add(t); 1606 return t; 1607 } 1608 1609 public DeviceDefinitionPropertyComponent addValueCode(CodeableConcept t) { // 3 1610 if (t == null) 1611 return this; 1612 if (this.valueCode == null) 1613 this.valueCode = new ArrayList<CodeableConcept>(); 1614 this.valueCode.add(t); 1615 return this; 1616 } 1617 1618 /** 1619 * @return The first repetition of repeating field {@link #valueCode}, creating 1620 * it if it does not already exist 1621 */ 1622 public CodeableConcept getValueCodeFirstRep() { 1623 if (getValueCode().isEmpty()) { 1624 addValueCode(); 1625 } 1626 return getValueCode().get(0); 1627 } 1628 1629 protected void listChildren(List<Property> children) { 1630 super.listChildren(children); 1631 children.add(new Property("type", "CodeableConcept", 1632 "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).", 0, 1, type)); 1633 children.add(new Property("valueQuantity", "Quantity", "Property value as a quantity.", 0, 1634 java.lang.Integer.MAX_VALUE, valueQuantity)); 1635 children.add(new Property("valueCode", "CodeableConcept", "Property value as a code, e.g., NTP4 (synced to NTP).", 1636 0, java.lang.Integer.MAX_VALUE, valueCode)); 1637 } 1638 1639 @Override 1640 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1641 switch (_hash) { 1642 case 3575610: 1643 /* type */ return new Property("type", "CodeableConcept", 1644 "Code that specifies the property DeviceDefinitionPropetyCode (Extensible).", 0, 1, type); 1645 case -2029823716: 1646 /* valueQuantity */ return new Property("valueQuantity", "Quantity", "Property value as a quantity.", 0, 1647 java.lang.Integer.MAX_VALUE, valueQuantity); 1648 case -766209282: 1649 /* valueCode */ return new Property("valueCode", "CodeableConcept", 1650 "Property value as a code, e.g., NTP4 (synced to NTP).", 0, java.lang.Integer.MAX_VALUE, valueCode); 1651 default: 1652 return super.getNamedProperty(_hash, _name, _checkValid); 1653 } 1654 1655 } 1656 1657 @Override 1658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1659 switch (hash) { 1660 case 3575610: 1661 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1662 case -2029823716: 1663 /* valueQuantity */ return this.valueQuantity == null ? new Base[0] 1664 : this.valueQuantity.toArray(new Base[this.valueQuantity.size()]); // Quantity 1665 case -766209282: 1666 /* valueCode */ return this.valueCode == null ? new Base[0] 1667 : this.valueCode.toArray(new Base[this.valueCode.size()]); // CodeableConcept 1668 default: 1669 return super.getProperty(hash, name, checkValid); 1670 } 1671 1672 } 1673 1674 @Override 1675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1676 switch (hash) { 1677 case 3575610: // type 1678 this.type = castToCodeableConcept(value); // CodeableConcept 1679 return value; 1680 case -2029823716: // valueQuantity 1681 this.getValueQuantity().add(castToQuantity(value)); // Quantity 1682 return value; 1683 case -766209282: // valueCode 1684 this.getValueCode().add(castToCodeableConcept(value)); // CodeableConcept 1685 return value; 1686 default: 1687 return super.setProperty(hash, name, value); 1688 } 1689 1690 } 1691 1692 @Override 1693 public Base setProperty(String name, Base value) throws FHIRException { 1694 if (name.equals("type")) { 1695 this.type = castToCodeableConcept(value); // CodeableConcept 1696 } else if (name.equals("valueQuantity")) { 1697 this.getValueQuantity().add(castToQuantity(value)); 1698 } else if (name.equals("valueCode")) { 1699 this.getValueCode().add(castToCodeableConcept(value)); 1700 } else 1701 return super.setProperty(name, value); 1702 return value; 1703 } 1704 1705 @Override 1706 public Base makeProperty(int hash, String name) throws FHIRException { 1707 switch (hash) { 1708 case 3575610: 1709 return getType(); 1710 case -2029823716: 1711 return addValueQuantity(); 1712 case -766209282: 1713 return addValueCode(); 1714 default: 1715 return super.makeProperty(hash, name); 1716 } 1717 1718 } 1719 1720 @Override 1721 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1722 switch (hash) { 1723 case 3575610: 1724 /* type */ return new String[] { "CodeableConcept" }; 1725 case -2029823716: 1726 /* valueQuantity */ return new String[] { "Quantity" }; 1727 case -766209282: 1728 /* valueCode */ return new String[] { "CodeableConcept" }; 1729 default: 1730 return super.getTypesForProperty(hash, name); 1731 } 1732 1733 } 1734 1735 @Override 1736 public Base addChild(String name) throws FHIRException { 1737 if (name.equals("type")) { 1738 this.type = new CodeableConcept(); 1739 return this.type; 1740 } else if (name.equals("valueQuantity")) { 1741 return addValueQuantity(); 1742 } else if (name.equals("valueCode")) { 1743 return addValueCode(); 1744 } else 1745 return super.addChild(name); 1746 } 1747 1748 public DeviceDefinitionPropertyComponent copy() { 1749 DeviceDefinitionPropertyComponent dst = new DeviceDefinitionPropertyComponent(); 1750 copyValues(dst); 1751 return dst; 1752 } 1753 1754 public void copyValues(DeviceDefinitionPropertyComponent dst) { 1755 super.copyValues(dst); 1756 dst.type = type == null ? null : type.copy(); 1757 if (valueQuantity != null) { 1758 dst.valueQuantity = new ArrayList<Quantity>(); 1759 for (Quantity i : valueQuantity) 1760 dst.valueQuantity.add(i.copy()); 1761 } 1762 ; 1763 if (valueCode != null) { 1764 dst.valueCode = new ArrayList<CodeableConcept>(); 1765 for (CodeableConcept i : valueCode) 1766 dst.valueCode.add(i.copy()); 1767 } 1768 ; 1769 } 1770 1771 @Override 1772 public boolean equalsDeep(Base other_) { 1773 if (!super.equalsDeep(other_)) 1774 return false; 1775 if (!(other_ instanceof DeviceDefinitionPropertyComponent)) 1776 return false; 1777 DeviceDefinitionPropertyComponent o = (DeviceDefinitionPropertyComponent) other_; 1778 return compareDeep(type, o.type, true) && compareDeep(valueQuantity, o.valueQuantity, true) 1779 && compareDeep(valueCode, o.valueCode, true); 1780 } 1781 1782 @Override 1783 public boolean equalsShallow(Base other_) { 1784 if (!super.equalsShallow(other_)) 1785 return false; 1786 if (!(other_ instanceof DeviceDefinitionPropertyComponent)) 1787 return false; 1788 DeviceDefinitionPropertyComponent o = (DeviceDefinitionPropertyComponent) other_; 1789 return true; 1790 } 1791 1792 public boolean isEmpty() { 1793 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, valueQuantity, valueCode); 1794 } 1795 1796 public String fhirType() { 1797 return "DeviceDefinition.property"; 1798 1799 } 1800 1801 } 1802 1803 @Block() 1804 public static class DeviceDefinitionMaterialComponent extends BackboneElement implements IBaseBackboneElement { 1805 /** 1806 * The substance. 1807 */ 1808 @Child(name = "substance", type = { 1809 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1810 @Description(shortDefinition = "The substance", formalDefinition = "The substance.") 1811 protected CodeableConcept substance; 1812 1813 /** 1814 * Indicates an alternative material of the device. 1815 */ 1816 @Child(name = "alternate", type = { 1817 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1818 @Description(shortDefinition = "Indicates an alternative material of the device", formalDefinition = "Indicates an alternative material of the device.") 1819 protected BooleanType alternate; 1820 1821 /** 1822 * Whether the substance is a known or suspected allergen. 1823 */ 1824 @Child(name = "allergenicIndicator", type = { 1825 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1826 @Description(shortDefinition = "Whether the substance is a known or suspected allergen", formalDefinition = "Whether the substance is a known or suspected allergen.") 1827 protected BooleanType allergenicIndicator; 1828 1829 private static final long serialVersionUID = 1232736508L; 1830 1831 /** 1832 * Constructor 1833 */ 1834 public DeviceDefinitionMaterialComponent() { 1835 super(); 1836 } 1837 1838 /** 1839 * Constructor 1840 */ 1841 public DeviceDefinitionMaterialComponent(CodeableConcept substance) { 1842 super(); 1843 this.substance = substance; 1844 } 1845 1846 /** 1847 * @return {@link #substance} (The substance.) 1848 */ 1849 public CodeableConcept getSubstance() { 1850 if (this.substance == null) 1851 if (Configuration.errorOnAutoCreate()) 1852 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.substance"); 1853 else if (Configuration.doAutoCreate()) 1854 this.substance = new CodeableConcept(); // cc 1855 return this.substance; 1856 } 1857 1858 public boolean hasSubstance() { 1859 return this.substance != null && !this.substance.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #substance} (The substance.) 1864 */ 1865 public DeviceDefinitionMaterialComponent setSubstance(CodeableConcept value) { 1866 this.substance = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return {@link #alternate} (Indicates an alternative material of the 1872 * device.). This is the underlying object with id, value and 1873 * extensions. The accessor "getAlternate" gives direct access to the 1874 * value 1875 */ 1876 public BooleanType getAlternateElement() { 1877 if (this.alternate == null) 1878 if (Configuration.errorOnAutoCreate()) 1879 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.alternate"); 1880 else if (Configuration.doAutoCreate()) 1881 this.alternate = new BooleanType(); // bb 1882 return this.alternate; 1883 } 1884 1885 public boolean hasAlternateElement() { 1886 return this.alternate != null && !this.alternate.isEmpty(); 1887 } 1888 1889 public boolean hasAlternate() { 1890 return this.alternate != null && !this.alternate.isEmpty(); 1891 } 1892 1893 /** 1894 * @param value {@link #alternate} (Indicates an alternative material of the 1895 * device.). This is the underlying object with id, value and 1896 * extensions. The accessor "getAlternate" gives direct access to 1897 * the value 1898 */ 1899 public DeviceDefinitionMaterialComponent setAlternateElement(BooleanType value) { 1900 this.alternate = value; 1901 return this; 1902 } 1903 1904 /** 1905 * @return Indicates an alternative material of the device. 1906 */ 1907 public boolean getAlternate() { 1908 return this.alternate == null || this.alternate.isEmpty() ? false : this.alternate.getValue(); 1909 } 1910 1911 /** 1912 * @param value Indicates an alternative material of the device. 1913 */ 1914 public DeviceDefinitionMaterialComponent setAlternate(boolean value) { 1915 if (this.alternate == null) 1916 this.alternate = new BooleanType(); 1917 this.alternate.setValue(value); 1918 return this; 1919 } 1920 1921 /** 1922 * @return {@link #allergenicIndicator} (Whether the substance is a known or 1923 * suspected allergen.). This is the underlying object with id, value 1924 * and extensions. The accessor "getAllergenicIndicator" gives direct 1925 * access to the value 1926 */ 1927 public BooleanType getAllergenicIndicatorElement() { 1928 if (this.allergenicIndicator == null) 1929 if (Configuration.errorOnAutoCreate()) 1930 throw new Error("Attempt to auto-create DeviceDefinitionMaterialComponent.allergenicIndicator"); 1931 else if (Configuration.doAutoCreate()) 1932 this.allergenicIndicator = new BooleanType(); // bb 1933 return this.allergenicIndicator; 1934 } 1935 1936 public boolean hasAllergenicIndicatorElement() { 1937 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 1938 } 1939 1940 public boolean hasAllergenicIndicator() { 1941 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #allergenicIndicator} (Whether the substance is a known 1946 * or suspected allergen.). This is the underlying object with id, 1947 * value and extensions. The accessor "getAllergenicIndicator" 1948 * gives direct access to the value 1949 */ 1950 public DeviceDefinitionMaterialComponent setAllergenicIndicatorElement(BooleanType value) { 1951 this.allergenicIndicator = value; 1952 return this; 1953 } 1954 1955 /** 1956 * @return Whether the substance is a known or suspected allergen. 1957 */ 1958 public boolean getAllergenicIndicator() { 1959 return this.allergenicIndicator == null || this.allergenicIndicator.isEmpty() ? false 1960 : this.allergenicIndicator.getValue(); 1961 } 1962 1963 /** 1964 * @param value Whether the substance is a known or suspected allergen. 1965 */ 1966 public DeviceDefinitionMaterialComponent setAllergenicIndicator(boolean value) { 1967 if (this.allergenicIndicator == null) 1968 this.allergenicIndicator = new BooleanType(); 1969 this.allergenicIndicator.setValue(value); 1970 return this; 1971 } 1972 1973 protected void listChildren(List<Property> children) { 1974 super.listChildren(children); 1975 children.add(new Property("substance", "CodeableConcept", "The substance.", 0, 1, substance)); 1976 children.add( 1977 new Property("alternate", "boolean", "Indicates an alternative material of the device.", 0, 1, alternate)); 1978 children.add(new Property("allergenicIndicator", "boolean", 1979 "Whether the substance is a known or suspected allergen.", 0, 1, allergenicIndicator)); 1980 } 1981 1982 @Override 1983 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1984 switch (_hash) { 1985 case 530040176: 1986 /* substance */ return new Property("substance", "CodeableConcept", "The substance.", 0, 1, substance); 1987 case -1408024454: 1988 /* alternate */ return new Property("alternate", "boolean", "Indicates an alternative material of the device.", 1989 0, 1, alternate); 1990 case 75406931: 1991 /* allergenicIndicator */ return new Property("allergenicIndicator", "boolean", 1992 "Whether the substance is a known or suspected allergen.", 0, 1, allergenicIndicator); 1993 default: 1994 return super.getNamedProperty(_hash, _name, _checkValid); 1995 } 1996 1997 } 1998 1999 @Override 2000 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2001 switch (hash) { 2002 case 530040176: 2003 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // CodeableConcept 2004 case -1408024454: 2005 /* alternate */ return this.alternate == null ? new Base[0] : new Base[] { this.alternate }; // BooleanType 2006 case 75406931: 2007 /* allergenicIndicator */ return this.allergenicIndicator == null ? new Base[0] 2008 : new Base[] { this.allergenicIndicator }; // BooleanType 2009 default: 2010 return super.getProperty(hash, name, checkValid); 2011 } 2012 2013 } 2014 2015 @Override 2016 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2017 switch (hash) { 2018 case 530040176: // substance 2019 this.substance = castToCodeableConcept(value); // CodeableConcept 2020 return value; 2021 case -1408024454: // alternate 2022 this.alternate = castToBoolean(value); // BooleanType 2023 return value; 2024 case 75406931: // allergenicIndicator 2025 this.allergenicIndicator = castToBoolean(value); // BooleanType 2026 return value; 2027 default: 2028 return super.setProperty(hash, name, value); 2029 } 2030 2031 } 2032 2033 @Override 2034 public Base setProperty(String name, Base value) throws FHIRException { 2035 if (name.equals("substance")) { 2036 this.substance = castToCodeableConcept(value); // CodeableConcept 2037 } else if (name.equals("alternate")) { 2038 this.alternate = castToBoolean(value); // BooleanType 2039 } else if (name.equals("allergenicIndicator")) { 2040 this.allergenicIndicator = castToBoolean(value); // BooleanType 2041 } else 2042 return super.setProperty(name, value); 2043 return value; 2044 } 2045 2046 @Override 2047 public Base makeProperty(int hash, String name) throws FHIRException { 2048 switch (hash) { 2049 case 530040176: 2050 return getSubstance(); 2051 case -1408024454: 2052 return getAlternateElement(); 2053 case 75406931: 2054 return getAllergenicIndicatorElement(); 2055 default: 2056 return super.makeProperty(hash, name); 2057 } 2058 2059 } 2060 2061 @Override 2062 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2063 switch (hash) { 2064 case 530040176: 2065 /* substance */ return new String[] { "CodeableConcept" }; 2066 case -1408024454: 2067 /* alternate */ return new String[] { "boolean" }; 2068 case 75406931: 2069 /* allergenicIndicator */ return new String[] { "boolean" }; 2070 default: 2071 return super.getTypesForProperty(hash, name); 2072 } 2073 2074 } 2075 2076 @Override 2077 public Base addChild(String name) throws FHIRException { 2078 if (name.equals("substance")) { 2079 this.substance = new CodeableConcept(); 2080 return this.substance; 2081 } else if (name.equals("alternate")) { 2082 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.alternate"); 2083 } else if (name.equals("allergenicIndicator")) { 2084 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.allergenicIndicator"); 2085 } else 2086 return super.addChild(name); 2087 } 2088 2089 public DeviceDefinitionMaterialComponent copy() { 2090 DeviceDefinitionMaterialComponent dst = new DeviceDefinitionMaterialComponent(); 2091 copyValues(dst); 2092 return dst; 2093 } 2094 2095 public void copyValues(DeviceDefinitionMaterialComponent dst) { 2096 super.copyValues(dst); 2097 dst.substance = substance == null ? null : substance.copy(); 2098 dst.alternate = alternate == null ? null : alternate.copy(); 2099 dst.allergenicIndicator = allergenicIndicator == null ? null : allergenicIndicator.copy(); 2100 } 2101 2102 @Override 2103 public boolean equalsDeep(Base other_) { 2104 if (!super.equalsDeep(other_)) 2105 return false; 2106 if (!(other_ instanceof DeviceDefinitionMaterialComponent)) 2107 return false; 2108 DeviceDefinitionMaterialComponent o = (DeviceDefinitionMaterialComponent) other_; 2109 return compareDeep(substance, o.substance, true) && compareDeep(alternate, o.alternate, true) 2110 && compareDeep(allergenicIndicator, o.allergenicIndicator, true); 2111 } 2112 2113 @Override 2114 public boolean equalsShallow(Base other_) { 2115 if (!super.equalsShallow(other_)) 2116 return false; 2117 if (!(other_ instanceof DeviceDefinitionMaterialComponent)) 2118 return false; 2119 DeviceDefinitionMaterialComponent o = (DeviceDefinitionMaterialComponent) other_; 2120 return compareValues(alternate, o.alternate, true) 2121 && compareValues(allergenicIndicator, o.allergenicIndicator, true); 2122 } 2123 2124 public boolean isEmpty() { 2125 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, alternate, allergenicIndicator); 2126 } 2127 2128 public String fhirType() { 2129 return "DeviceDefinition.material"; 2130 2131 } 2132 2133 } 2134 2135 /** 2136 * Unique instance identifiers assigned to a device by the software, 2137 * manufacturers, other organizations or owners. For example: handle ID. 2138 */ 2139 @Child(name = "identifier", type = { 2140 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2141 @Description(shortDefinition = "Instance identifier", formalDefinition = "Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID.") 2142 protected List<Identifier> identifier; 2143 2144 /** 2145 * Unique device identifier (UDI) assigned to device label or package. Note that 2146 * the Device may include multiple udiCarriers as it either may include just the 2147 * udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it 2148 * could have been sold. 2149 */ 2150 @Child(name = "udiDeviceIdentifier", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2151 @Description(shortDefinition = "Unique Device Identifier (UDI) Barcode string", formalDefinition = "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.") 2152 protected List<DeviceDefinitionUdiDeviceIdentifierComponent> udiDeviceIdentifier; 2153 2154 /** 2155 * A name of the manufacturer. 2156 */ 2157 @Child(name = "manufacturer", type = { StringType.class, 2158 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2159 @Description(shortDefinition = "Name of device manufacturer", formalDefinition = "A name of the manufacturer.") 2160 protected Type manufacturer; 2161 2162 /** 2163 * A name given to the device to identify it. 2164 */ 2165 @Child(name = "deviceName", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2166 @Description(shortDefinition = "A name given to the device to identify it", formalDefinition = "A name given to the device to identify it.") 2167 protected List<DeviceDefinitionDeviceNameComponent> deviceName; 2168 2169 /** 2170 * The model number for the device. 2171 */ 2172 @Child(name = "modelNumber", type = { 2173 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2174 @Description(shortDefinition = "The model number for the device", formalDefinition = "The model number for the device.") 2175 protected StringType modelNumber; 2176 2177 /** 2178 * What kind of device or device system this is. 2179 */ 2180 @Child(name = "type", type = { 2181 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2182 @Description(shortDefinition = "What kind of device or device system this is", formalDefinition = "What kind of device or device system this is.") 2183 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-kind") 2184 protected CodeableConcept type; 2185 2186 /** 2187 * The capabilities supported on a device, the standards to which the device 2188 * conforms for a particular purpose, and used for the communication. 2189 */ 2190 @Child(name = "specialization", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2191 @Description(shortDefinition = "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication", formalDefinition = "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.") 2192 protected List<DeviceDefinitionSpecializationComponent> specialization; 2193 2194 /** 2195 * The available versions of the device, e.g., software versions. 2196 */ 2197 @Child(name = "version", type = { 2198 StringType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2199 @Description(shortDefinition = "Available versions", formalDefinition = "The available versions of the device, e.g., software versions.") 2200 protected List<StringType> version; 2201 2202 /** 2203 * Safety characteristics of the device. 2204 */ 2205 @Child(name = "safety", type = { 2206 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2207 @Description(shortDefinition = "Safety characteristics of the device", formalDefinition = "Safety characteristics of the device.") 2208 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-safety") 2209 protected List<CodeableConcept> safety; 2210 2211 /** 2212 * Shelf Life and storage information. 2213 */ 2214 @Child(name = "shelfLifeStorage", type = { 2215 ProductShelfLife.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2216 @Description(shortDefinition = "Shelf Life and storage information", formalDefinition = "Shelf Life and storage information.") 2217 protected List<ProductShelfLife> shelfLifeStorage; 2218 2219 /** 2220 * Dimensions, color etc. 2221 */ 2222 @Child(name = "physicalCharacteristics", type = { 2223 ProdCharacteristic.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 2224 @Description(shortDefinition = "Dimensions, color etc.", formalDefinition = "Dimensions, color etc.") 2225 protected ProdCharacteristic physicalCharacteristics; 2226 2227 /** 2228 * Language code for the human-readable text strings produced by the device (all 2229 * supported). 2230 */ 2231 @Child(name = "languageCode", type = { 2232 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2233 @Description(shortDefinition = "Language code for the human-readable text strings produced by the device (all supported)", formalDefinition = "Language code for the human-readable text strings produced by the device (all supported).") 2234 protected List<CodeableConcept> languageCode; 2235 2236 /** 2237 * Device capabilities. 2238 */ 2239 @Child(name = "capability", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2240 @Description(shortDefinition = "Device capabilities", formalDefinition = "Device capabilities.") 2241 protected List<DeviceDefinitionCapabilityComponent> capability; 2242 2243 /** 2244 * The actual configuration settings of a device as it actually operates, e.g., 2245 * regulation status, time properties. 2246 */ 2247 @Child(name = "property", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2248 @Description(shortDefinition = "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties", formalDefinition = "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.") 2249 protected List<DeviceDefinitionPropertyComponent> property; 2250 2251 /** 2252 * An organization that is responsible for the provision and ongoing maintenance 2253 * of the device. 2254 */ 2255 @Child(name = "owner", type = { Organization.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2256 @Description(shortDefinition = "Organization responsible for device", formalDefinition = "An organization that is responsible for the provision and ongoing maintenance of the device.") 2257 protected Reference owner; 2258 2259 /** 2260 * The actual object that is the target of the reference (An organization that 2261 * is responsible for the provision and ongoing maintenance of the device.) 2262 */ 2263 protected Organization ownerTarget; 2264 2265 /** 2266 * Contact details for an organization or a particular human that is responsible 2267 * for the device. 2268 */ 2269 @Child(name = "contact", type = { 2270 ContactPoint.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2271 @Description(shortDefinition = "Details for human/organization for support", formalDefinition = "Contact details for an organization or a particular human that is responsible for the device.") 2272 protected List<ContactPoint> contact; 2273 2274 /** 2275 * A network address on which the device may be contacted directly. 2276 */ 2277 @Child(name = "url", type = { UriType.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2278 @Description(shortDefinition = "Network address to contact device", formalDefinition = "A network address on which the device may be contacted directly.") 2279 protected UriType url; 2280 2281 /** 2282 * Access to on-line information about the device. 2283 */ 2284 @Child(name = "onlineInformation", type = { 2285 UriType.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 2286 @Description(shortDefinition = "Access to on-line information", formalDefinition = "Access to on-line information about the device.") 2287 protected UriType onlineInformation; 2288 2289 /** 2290 * Descriptive information, usage information or implantation information that 2291 * is not captured in an existing element. 2292 */ 2293 @Child(name = "note", type = { 2294 Annotation.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2295 @Description(shortDefinition = "Device notes and comments", formalDefinition = "Descriptive information, usage information or implantation information that is not captured in an existing element.") 2296 protected List<Annotation> note; 2297 2298 /** 2299 * The quantity of the device present in the packaging (e.g. the number of 2300 * devices present in a pack, or the number of devices in the same package of 2301 * the medicinal product). 2302 */ 2303 @Child(name = "quantity", type = { Quantity.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 2304 @Description(shortDefinition = "The quantity of the device present in the packaging (e.g. the number of devices present in a pack, or the number of devices in the same package of the medicinal product)", formalDefinition = "The quantity of the device present in the packaging (e.g. the number of devices present in a pack, or the number of devices in the same package of the medicinal product).") 2305 protected Quantity quantity; 2306 2307 /** 2308 * The parent device it can be part of. 2309 */ 2310 @Child(name = "parentDevice", type = { 2311 DeviceDefinition.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 2312 @Description(shortDefinition = "The parent device it can be part of", formalDefinition = "The parent device it can be part of.") 2313 protected Reference parentDevice; 2314 2315 /** 2316 * The actual object that is the target of the reference (The parent device it 2317 * can be part of.) 2318 */ 2319 protected DeviceDefinition parentDeviceTarget; 2320 2321 /** 2322 * A substance used to create the material(s) of which the device is made. 2323 */ 2324 @Child(name = "material", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2325 @Description(shortDefinition = "A substance used to create the material(s) of which the device is made", formalDefinition = "A substance used to create the material(s) of which the device is made.") 2326 protected List<DeviceDefinitionMaterialComponent> material; 2327 2328 private static final long serialVersionUID = -2041532433L; 2329 2330 /** 2331 * Constructor 2332 */ 2333 public DeviceDefinition() { 2334 super(); 2335 } 2336 2337 /** 2338 * @return {@link #identifier} (Unique instance identifiers assigned to a device 2339 * by the software, manufacturers, other organizations or owners. For 2340 * example: handle ID.) 2341 */ 2342 public List<Identifier> getIdentifier() { 2343 if (this.identifier == null) 2344 this.identifier = new ArrayList<Identifier>(); 2345 return this.identifier; 2346 } 2347 2348 /** 2349 * @return Returns a reference to <code>this</code> for easy method chaining 2350 */ 2351 public DeviceDefinition setIdentifier(List<Identifier> theIdentifier) { 2352 this.identifier = theIdentifier; 2353 return this; 2354 } 2355 2356 public boolean hasIdentifier() { 2357 if (this.identifier == null) 2358 return false; 2359 for (Identifier item : this.identifier) 2360 if (!item.isEmpty()) 2361 return true; 2362 return false; 2363 } 2364 2365 public Identifier addIdentifier() { // 3 2366 Identifier t = new Identifier(); 2367 if (this.identifier == null) 2368 this.identifier = new ArrayList<Identifier>(); 2369 this.identifier.add(t); 2370 return t; 2371 } 2372 2373 public DeviceDefinition addIdentifier(Identifier t) { // 3 2374 if (t == null) 2375 return this; 2376 if (this.identifier == null) 2377 this.identifier = new ArrayList<Identifier>(); 2378 this.identifier.add(t); 2379 return this; 2380 } 2381 2382 /** 2383 * @return The first repetition of repeating field {@link #identifier}, creating 2384 * it if it does not already exist 2385 */ 2386 public Identifier getIdentifierFirstRep() { 2387 if (getIdentifier().isEmpty()) { 2388 addIdentifier(); 2389 } 2390 return getIdentifier().get(0); 2391 } 2392 2393 /** 2394 * @return {@link #udiDeviceIdentifier} (Unique device identifier (UDI) assigned 2395 * to device label or package. Note that the Device may include multiple 2396 * udiCarriers as it either may include just the udiCarrier for the 2397 * jurisdiction it is sold, or for multiple jurisdictions it could have 2398 * been sold.) 2399 */ 2400 public List<DeviceDefinitionUdiDeviceIdentifierComponent> getUdiDeviceIdentifier() { 2401 if (this.udiDeviceIdentifier == null) 2402 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2403 return this.udiDeviceIdentifier; 2404 } 2405 2406 /** 2407 * @return Returns a reference to <code>this</code> for easy method chaining 2408 */ 2409 public DeviceDefinition setUdiDeviceIdentifier( 2410 List<DeviceDefinitionUdiDeviceIdentifierComponent> theUdiDeviceIdentifier) { 2411 this.udiDeviceIdentifier = theUdiDeviceIdentifier; 2412 return this; 2413 } 2414 2415 public boolean hasUdiDeviceIdentifier() { 2416 if (this.udiDeviceIdentifier == null) 2417 return false; 2418 for (DeviceDefinitionUdiDeviceIdentifierComponent item : this.udiDeviceIdentifier) 2419 if (!item.isEmpty()) 2420 return true; 2421 return false; 2422 } 2423 2424 public DeviceDefinitionUdiDeviceIdentifierComponent addUdiDeviceIdentifier() { // 3 2425 DeviceDefinitionUdiDeviceIdentifierComponent t = new DeviceDefinitionUdiDeviceIdentifierComponent(); 2426 if (this.udiDeviceIdentifier == null) 2427 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2428 this.udiDeviceIdentifier.add(t); 2429 return t; 2430 } 2431 2432 public DeviceDefinition addUdiDeviceIdentifier(DeviceDefinitionUdiDeviceIdentifierComponent t) { // 3 2433 if (t == null) 2434 return this; 2435 if (this.udiDeviceIdentifier == null) 2436 this.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 2437 this.udiDeviceIdentifier.add(t); 2438 return this; 2439 } 2440 2441 /** 2442 * @return The first repetition of repeating field {@link #udiDeviceIdentifier}, 2443 * creating it if it does not already exist 2444 */ 2445 public DeviceDefinitionUdiDeviceIdentifierComponent getUdiDeviceIdentifierFirstRep() { 2446 if (getUdiDeviceIdentifier().isEmpty()) { 2447 addUdiDeviceIdentifier(); 2448 } 2449 return getUdiDeviceIdentifier().get(0); 2450 } 2451 2452 /** 2453 * @return {@link #manufacturer} (A name of the manufacturer.) 2454 */ 2455 public Type getManufacturer() { 2456 return this.manufacturer; 2457 } 2458 2459 /** 2460 * @return {@link #manufacturer} (A name of the manufacturer.) 2461 */ 2462 public StringType getManufacturerStringType() throws FHIRException { 2463 if (this.manufacturer == null) 2464 this.manufacturer = new StringType(); 2465 if (!(this.manufacturer instanceof StringType)) 2466 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2467 + this.manufacturer.getClass().getName() + " was encountered"); 2468 return (StringType) this.manufacturer; 2469 } 2470 2471 public boolean hasManufacturerStringType() { 2472 return this != null && this.manufacturer instanceof StringType; 2473 } 2474 2475 /** 2476 * @return {@link #manufacturer} (A name of the manufacturer.) 2477 */ 2478 public Reference getManufacturerReference() throws FHIRException { 2479 if (this.manufacturer == null) 2480 this.manufacturer = new Reference(); 2481 if (!(this.manufacturer instanceof Reference)) 2482 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2483 + this.manufacturer.getClass().getName() + " was encountered"); 2484 return (Reference) this.manufacturer; 2485 } 2486 2487 public boolean hasManufacturerReference() { 2488 return this != null && this.manufacturer instanceof Reference; 2489 } 2490 2491 public boolean hasManufacturer() { 2492 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2493 } 2494 2495 /** 2496 * @param value {@link #manufacturer} (A name of the manufacturer.) 2497 */ 2498 public DeviceDefinition setManufacturer(Type value) { 2499 if (value != null && !(value instanceof StringType || value instanceof Reference)) 2500 throw new Error("Not the right type for DeviceDefinition.manufacturer[x]: " + value.fhirType()); 2501 this.manufacturer = value; 2502 return this; 2503 } 2504 2505 /** 2506 * @return {@link #deviceName} (A name given to the device to identify it.) 2507 */ 2508 public List<DeviceDefinitionDeviceNameComponent> getDeviceName() { 2509 if (this.deviceName == null) 2510 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 2511 return this.deviceName; 2512 } 2513 2514 /** 2515 * @return Returns a reference to <code>this</code> for easy method chaining 2516 */ 2517 public DeviceDefinition setDeviceName(List<DeviceDefinitionDeviceNameComponent> theDeviceName) { 2518 this.deviceName = theDeviceName; 2519 return this; 2520 } 2521 2522 public boolean hasDeviceName() { 2523 if (this.deviceName == null) 2524 return false; 2525 for (DeviceDefinitionDeviceNameComponent item : this.deviceName) 2526 if (!item.isEmpty()) 2527 return true; 2528 return false; 2529 } 2530 2531 public DeviceDefinitionDeviceNameComponent addDeviceName() { // 3 2532 DeviceDefinitionDeviceNameComponent t = new DeviceDefinitionDeviceNameComponent(); 2533 if (this.deviceName == null) 2534 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 2535 this.deviceName.add(t); 2536 return t; 2537 } 2538 2539 public DeviceDefinition addDeviceName(DeviceDefinitionDeviceNameComponent t) { // 3 2540 if (t == null) 2541 return this; 2542 if (this.deviceName == null) 2543 this.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 2544 this.deviceName.add(t); 2545 return this; 2546 } 2547 2548 /** 2549 * @return The first repetition of repeating field {@link #deviceName}, creating 2550 * it if it does not already exist 2551 */ 2552 public DeviceDefinitionDeviceNameComponent getDeviceNameFirstRep() { 2553 if (getDeviceName().isEmpty()) { 2554 addDeviceName(); 2555 } 2556 return getDeviceName().get(0); 2557 } 2558 2559 /** 2560 * @return {@link #modelNumber} (The model number for the device.). This is the 2561 * underlying object with id, value and extensions. The accessor 2562 * "getModelNumber" gives direct access to the value 2563 */ 2564 public StringType getModelNumberElement() { 2565 if (this.modelNumber == null) 2566 if (Configuration.errorOnAutoCreate()) 2567 throw new Error("Attempt to auto-create DeviceDefinition.modelNumber"); 2568 else if (Configuration.doAutoCreate()) 2569 this.modelNumber = new StringType(); // bb 2570 return this.modelNumber; 2571 } 2572 2573 public boolean hasModelNumberElement() { 2574 return this.modelNumber != null && !this.modelNumber.isEmpty(); 2575 } 2576 2577 public boolean hasModelNumber() { 2578 return this.modelNumber != null && !this.modelNumber.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #modelNumber} (The model number for the device.). This is 2583 * the underlying object with id, value and extensions. The 2584 * accessor "getModelNumber" gives direct access to the value 2585 */ 2586 public DeviceDefinition setModelNumberElement(StringType value) { 2587 this.modelNumber = value; 2588 return this; 2589 } 2590 2591 /** 2592 * @return The model number for the device. 2593 */ 2594 public String getModelNumber() { 2595 return this.modelNumber == null ? null : this.modelNumber.getValue(); 2596 } 2597 2598 /** 2599 * @param value The model number for the device. 2600 */ 2601 public DeviceDefinition setModelNumber(String value) { 2602 if (Utilities.noString(value)) 2603 this.modelNumber = null; 2604 else { 2605 if (this.modelNumber == null) 2606 this.modelNumber = new StringType(); 2607 this.modelNumber.setValue(value); 2608 } 2609 return this; 2610 } 2611 2612 /** 2613 * @return {@link #type} (What kind of device or device system this is.) 2614 */ 2615 public CodeableConcept getType() { 2616 if (this.type == null) 2617 if (Configuration.errorOnAutoCreate()) 2618 throw new Error("Attempt to auto-create DeviceDefinition.type"); 2619 else if (Configuration.doAutoCreate()) 2620 this.type = new CodeableConcept(); // cc 2621 return this.type; 2622 } 2623 2624 public boolean hasType() { 2625 return this.type != null && !this.type.isEmpty(); 2626 } 2627 2628 /** 2629 * @param value {@link #type} (What kind of device or device system this is.) 2630 */ 2631 public DeviceDefinition setType(CodeableConcept value) { 2632 this.type = value; 2633 return this; 2634 } 2635 2636 /** 2637 * @return {@link #specialization} (The capabilities supported on a device, the 2638 * standards to which the device conforms for a particular purpose, and 2639 * used for the communication.) 2640 */ 2641 public List<DeviceDefinitionSpecializationComponent> getSpecialization() { 2642 if (this.specialization == null) 2643 this.specialization = new ArrayList<DeviceDefinitionSpecializationComponent>(); 2644 return this.specialization; 2645 } 2646 2647 /** 2648 * @return Returns a reference to <code>this</code> for easy method chaining 2649 */ 2650 public DeviceDefinition setSpecialization(List<DeviceDefinitionSpecializationComponent> theSpecialization) { 2651 this.specialization = theSpecialization; 2652 return this; 2653 } 2654 2655 public boolean hasSpecialization() { 2656 if (this.specialization == null) 2657 return false; 2658 for (DeviceDefinitionSpecializationComponent item : this.specialization) 2659 if (!item.isEmpty()) 2660 return true; 2661 return false; 2662 } 2663 2664 public DeviceDefinitionSpecializationComponent addSpecialization() { // 3 2665 DeviceDefinitionSpecializationComponent t = new DeviceDefinitionSpecializationComponent(); 2666 if (this.specialization == null) 2667 this.specialization = new ArrayList<DeviceDefinitionSpecializationComponent>(); 2668 this.specialization.add(t); 2669 return t; 2670 } 2671 2672 public DeviceDefinition addSpecialization(DeviceDefinitionSpecializationComponent t) { // 3 2673 if (t == null) 2674 return this; 2675 if (this.specialization == null) 2676 this.specialization = new ArrayList<DeviceDefinitionSpecializationComponent>(); 2677 this.specialization.add(t); 2678 return this; 2679 } 2680 2681 /** 2682 * @return The first repetition of repeating field {@link #specialization}, 2683 * creating it if it does not already exist 2684 */ 2685 public DeviceDefinitionSpecializationComponent getSpecializationFirstRep() { 2686 if (getSpecialization().isEmpty()) { 2687 addSpecialization(); 2688 } 2689 return getSpecialization().get(0); 2690 } 2691 2692 /** 2693 * @return {@link #version} (The available versions of the device, e.g., 2694 * software versions.) 2695 */ 2696 public List<StringType> getVersion() { 2697 if (this.version == null) 2698 this.version = new ArrayList<StringType>(); 2699 return this.version; 2700 } 2701 2702 /** 2703 * @return Returns a reference to <code>this</code> for easy method chaining 2704 */ 2705 public DeviceDefinition setVersion(List<StringType> theVersion) { 2706 this.version = theVersion; 2707 return this; 2708 } 2709 2710 public boolean hasVersion() { 2711 if (this.version == null) 2712 return false; 2713 for (StringType item : this.version) 2714 if (!item.isEmpty()) 2715 return true; 2716 return false; 2717 } 2718 2719 /** 2720 * @return {@link #version} (The available versions of the device, e.g., 2721 * software versions.) 2722 */ 2723 public StringType addVersionElement() {// 2 2724 StringType t = new StringType(); 2725 if (this.version == null) 2726 this.version = new ArrayList<StringType>(); 2727 this.version.add(t); 2728 return t; 2729 } 2730 2731 /** 2732 * @param value {@link #version} (The available versions of the device, e.g., 2733 * software versions.) 2734 */ 2735 public DeviceDefinition addVersion(String value) { // 1 2736 StringType t = new StringType(); 2737 t.setValue(value); 2738 if (this.version == null) 2739 this.version = new ArrayList<StringType>(); 2740 this.version.add(t); 2741 return this; 2742 } 2743 2744 /** 2745 * @param value {@link #version} (The available versions of the device, e.g., 2746 * software versions.) 2747 */ 2748 public boolean hasVersion(String value) { 2749 if (this.version == null) 2750 return false; 2751 for (StringType v : this.version) 2752 if (v.getValue().equals(value)) // string 2753 return true; 2754 return false; 2755 } 2756 2757 /** 2758 * @return {@link #safety} (Safety characteristics of the device.) 2759 */ 2760 public List<CodeableConcept> getSafety() { 2761 if (this.safety == null) 2762 this.safety = new ArrayList<CodeableConcept>(); 2763 return this.safety; 2764 } 2765 2766 /** 2767 * @return Returns a reference to <code>this</code> for easy method chaining 2768 */ 2769 public DeviceDefinition setSafety(List<CodeableConcept> theSafety) { 2770 this.safety = theSafety; 2771 return this; 2772 } 2773 2774 public boolean hasSafety() { 2775 if (this.safety == null) 2776 return false; 2777 for (CodeableConcept item : this.safety) 2778 if (!item.isEmpty()) 2779 return true; 2780 return false; 2781 } 2782 2783 public CodeableConcept addSafety() { // 3 2784 CodeableConcept t = new CodeableConcept(); 2785 if (this.safety == null) 2786 this.safety = new ArrayList<CodeableConcept>(); 2787 this.safety.add(t); 2788 return t; 2789 } 2790 2791 public DeviceDefinition addSafety(CodeableConcept t) { // 3 2792 if (t == null) 2793 return this; 2794 if (this.safety == null) 2795 this.safety = new ArrayList<CodeableConcept>(); 2796 this.safety.add(t); 2797 return this; 2798 } 2799 2800 /** 2801 * @return The first repetition of repeating field {@link #safety}, creating it 2802 * if it does not already exist 2803 */ 2804 public CodeableConcept getSafetyFirstRep() { 2805 if (getSafety().isEmpty()) { 2806 addSafety(); 2807 } 2808 return getSafety().get(0); 2809 } 2810 2811 /** 2812 * @return {@link #shelfLifeStorage} (Shelf Life and storage information.) 2813 */ 2814 public List<ProductShelfLife> getShelfLifeStorage() { 2815 if (this.shelfLifeStorage == null) 2816 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 2817 return this.shelfLifeStorage; 2818 } 2819 2820 /** 2821 * @return Returns a reference to <code>this</code> for easy method chaining 2822 */ 2823 public DeviceDefinition setShelfLifeStorage(List<ProductShelfLife> theShelfLifeStorage) { 2824 this.shelfLifeStorage = theShelfLifeStorage; 2825 return this; 2826 } 2827 2828 public boolean hasShelfLifeStorage() { 2829 if (this.shelfLifeStorage == null) 2830 return false; 2831 for (ProductShelfLife item : this.shelfLifeStorage) 2832 if (!item.isEmpty()) 2833 return true; 2834 return false; 2835 } 2836 2837 public ProductShelfLife addShelfLifeStorage() { // 3 2838 ProductShelfLife t = new ProductShelfLife(); 2839 if (this.shelfLifeStorage == null) 2840 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 2841 this.shelfLifeStorage.add(t); 2842 return t; 2843 } 2844 2845 public DeviceDefinition addShelfLifeStorage(ProductShelfLife t) { // 3 2846 if (t == null) 2847 return this; 2848 if (this.shelfLifeStorage == null) 2849 this.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 2850 this.shelfLifeStorage.add(t); 2851 return this; 2852 } 2853 2854 /** 2855 * @return The first repetition of repeating field {@link #shelfLifeStorage}, 2856 * creating it if it does not already exist 2857 */ 2858 public ProductShelfLife getShelfLifeStorageFirstRep() { 2859 if (getShelfLifeStorage().isEmpty()) { 2860 addShelfLifeStorage(); 2861 } 2862 return getShelfLifeStorage().get(0); 2863 } 2864 2865 /** 2866 * @return {@link #physicalCharacteristics} (Dimensions, color etc.) 2867 */ 2868 public ProdCharacteristic getPhysicalCharacteristics() { 2869 if (this.physicalCharacteristics == null) 2870 if (Configuration.errorOnAutoCreate()) 2871 throw new Error("Attempt to auto-create DeviceDefinition.physicalCharacteristics"); 2872 else if (Configuration.doAutoCreate()) 2873 this.physicalCharacteristics = new ProdCharacteristic(); // cc 2874 return this.physicalCharacteristics; 2875 } 2876 2877 public boolean hasPhysicalCharacteristics() { 2878 return this.physicalCharacteristics != null && !this.physicalCharacteristics.isEmpty(); 2879 } 2880 2881 /** 2882 * @param value {@link #physicalCharacteristics} (Dimensions, color etc.) 2883 */ 2884 public DeviceDefinition setPhysicalCharacteristics(ProdCharacteristic value) { 2885 this.physicalCharacteristics = value; 2886 return this; 2887 } 2888 2889 /** 2890 * @return {@link #languageCode} (Language code for the human-readable text 2891 * strings produced by the device (all supported).) 2892 */ 2893 public List<CodeableConcept> getLanguageCode() { 2894 if (this.languageCode == null) 2895 this.languageCode = new ArrayList<CodeableConcept>(); 2896 return this.languageCode; 2897 } 2898 2899 /** 2900 * @return Returns a reference to <code>this</code> for easy method chaining 2901 */ 2902 public DeviceDefinition setLanguageCode(List<CodeableConcept> theLanguageCode) { 2903 this.languageCode = theLanguageCode; 2904 return this; 2905 } 2906 2907 public boolean hasLanguageCode() { 2908 if (this.languageCode == null) 2909 return false; 2910 for (CodeableConcept item : this.languageCode) 2911 if (!item.isEmpty()) 2912 return true; 2913 return false; 2914 } 2915 2916 public CodeableConcept addLanguageCode() { // 3 2917 CodeableConcept t = new CodeableConcept(); 2918 if (this.languageCode == null) 2919 this.languageCode = new ArrayList<CodeableConcept>(); 2920 this.languageCode.add(t); 2921 return t; 2922 } 2923 2924 public DeviceDefinition addLanguageCode(CodeableConcept t) { // 3 2925 if (t == null) 2926 return this; 2927 if (this.languageCode == null) 2928 this.languageCode = new ArrayList<CodeableConcept>(); 2929 this.languageCode.add(t); 2930 return this; 2931 } 2932 2933 /** 2934 * @return The first repetition of repeating field {@link #languageCode}, 2935 * creating it if it does not already exist 2936 */ 2937 public CodeableConcept getLanguageCodeFirstRep() { 2938 if (getLanguageCode().isEmpty()) { 2939 addLanguageCode(); 2940 } 2941 return getLanguageCode().get(0); 2942 } 2943 2944 /** 2945 * @return {@link #capability} (Device capabilities.) 2946 */ 2947 public List<DeviceDefinitionCapabilityComponent> getCapability() { 2948 if (this.capability == null) 2949 this.capability = new ArrayList<DeviceDefinitionCapabilityComponent>(); 2950 return this.capability; 2951 } 2952 2953 /** 2954 * @return Returns a reference to <code>this</code> for easy method chaining 2955 */ 2956 public DeviceDefinition setCapability(List<DeviceDefinitionCapabilityComponent> theCapability) { 2957 this.capability = theCapability; 2958 return this; 2959 } 2960 2961 public boolean hasCapability() { 2962 if (this.capability == null) 2963 return false; 2964 for (DeviceDefinitionCapabilityComponent item : this.capability) 2965 if (!item.isEmpty()) 2966 return true; 2967 return false; 2968 } 2969 2970 public DeviceDefinitionCapabilityComponent addCapability() { // 3 2971 DeviceDefinitionCapabilityComponent t = new DeviceDefinitionCapabilityComponent(); 2972 if (this.capability == null) 2973 this.capability = new ArrayList<DeviceDefinitionCapabilityComponent>(); 2974 this.capability.add(t); 2975 return t; 2976 } 2977 2978 public DeviceDefinition addCapability(DeviceDefinitionCapabilityComponent t) { // 3 2979 if (t == null) 2980 return this; 2981 if (this.capability == null) 2982 this.capability = new ArrayList<DeviceDefinitionCapabilityComponent>(); 2983 this.capability.add(t); 2984 return this; 2985 } 2986 2987 /** 2988 * @return The first repetition of repeating field {@link #capability}, creating 2989 * it if it does not already exist 2990 */ 2991 public DeviceDefinitionCapabilityComponent getCapabilityFirstRep() { 2992 if (getCapability().isEmpty()) { 2993 addCapability(); 2994 } 2995 return getCapability().get(0); 2996 } 2997 2998 /** 2999 * @return {@link #property} (The actual configuration settings of a device as 3000 * it actually operates, e.g., regulation status, time properties.) 3001 */ 3002 public List<DeviceDefinitionPropertyComponent> getProperty() { 3003 if (this.property == null) 3004 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 3005 return this.property; 3006 } 3007 3008 /** 3009 * @return Returns a reference to <code>this</code> for easy method chaining 3010 */ 3011 public DeviceDefinition setProperty(List<DeviceDefinitionPropertyComponent> theProperty) { 3012 this.property = theProperty; 3013 return this; 3014 } 3015 3016 public boolean hasProperty() { 3017 if (this.property == null) 3018 return false; 3019 for (DeviceDefinitionPropertyComponent item : this.property) 3020 if (!item.isEmpty()) 3021 return true; 3022 return false; 3023 } 3024 3025 public DeviceDefinitionPropertyComponent addProperty() { // 3 3026 DeviceDefinitionPropertyComponent t = new DeviceDefinitionPropertyComponent(); 3027 if (this.property == null) 3028 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 3029 this.property.add(t); 3030 return t; 3031 } 3032 3033 public DeviceDefinition addProperty(DeviceDefinitionPropertyComponent t) { // 3 3034 if (t == null) 3035 return this; 3036 if (this.property == null) 3037 this.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 3038 this.property.add(t); 3039 return this; 3040 } 3041 3042 /** 3043 * @return The first repetition of repeating field {@link #property}, creating 3044 * it if it does not already exist 3045 */ 3046 public DeviceDefinitionPropertyComponent getPropertyFirstRep() { 3047 if (getProperty().isEmpty()) { 3048 addProperty(); 3049 } 3050 return getProperty().get(0); 3051 } 3052 3053 /** 3054 * @return {@link #owner} (An organization that is responsible for the provision 3055 * and ongoing maintenance of the device.) 3056 */ 3057 public Reference getOwner() { 3058 if (this.owner == null) 3059 if (Configuration.errorOnAutoCreate()) 3060 throw new Error("Attempt to auto-create DeviceDefinition.owner"); 3061 else if (Configuration.doAutoCreate()) 3062 this.owner = new Reference(); // cc 3063 return this.owner; 3064 } 3065 3066 public boolean hasOwner() { 3067 return this.owner != null && !this.owner.isEmpty(); 3068 } 3069 3070 /** 3071 * @param value {@link #owner} (An organization that is responsible for the 3072 * provision and ongoing maintenance of the device.) 3073 */ 3074 public DeviceDefinition setOwner(Reference value) { 3075 this.owner = value; 3076 return this; 3077 } 3078 3079 /** 3080 * @return {@link #owner} The actual object that is the target of the reference. 3081 * The reference library doesn't populate this, but you can use it to 3082 * hold the resource if you resolve it. (An organization that is 3083 * responsible for the provision and ongoing maintenance of the device.) 3084 */ 3085 public Organization getOwnerTarget() { 3086 if (this.ownerTarget == null) 3087 if (Configuration.errorOnAutoCreate()) 3088 throw new Error("Attempt to auto-create DeviceDefinition.owner"); 3089 else if (Configuration.doAutoCreate()) 3090 this.ownerTarget = new Organization(); // aa 3091 return this.ownerTarget; 3092 } 3093 3094 /** 3095 * @param value {@link #owner} The actual object that is the target of the 3096 * reference. The reference library doesn't use these, but you can 3097 * use it to hold the resource if you resolve it. (An organization 3098 * that is responsible for the provision and ongoing maintenance of 3099 * the device.) 3100 */ 3101 public DeviceDefinition setOwnerTarget(Organization value) { 3102 this.ownerTarget = value; 3103 return this; 3104 } 3105 3106 /** 3107 * @return {@link #contact} (Contact details for an organization or a particular 3108 * human that is responsible for the device.) 3109 */ 3110 public List<ContactPoint> getContact() { 3111 if (this.contact == null) 3112 this.contact = new ArrayList<ContactPoint>(); 3113 return this.contact; 3114 } 3115 3116 /** 3117 * @return Returns a reference to <code>this</code> for easy method chaining 3118 */ 3119 public DeviceDefinition setContact(List<ContactPoint> theContact) { 3120 this.contact = theContact; 3121 return this; 3122 } 3123 3124 public boolean hasContact() { 3125 if (this.contact == null) 3126 return false; 3127 for (ContactPoint item : this.contact) 3128 if (!item.isEmpty()) 3129 return true; 3130 return false; 3131 } 3132 3133 public ContactPoint addContact() { // 3 3134 ContactPoint t = new ContactPoint(); 3135 if (this.contact == null) 3136 this.contact = new ArrayList<ContactPoint>(); 3137 this.contact.add(t); 3138 return t; 3139 } 3140 3141 public DeviceDefinition addContact(ContactPoint t) { // 3 3142 if (t == null) 3143 return this; 3144 if (this.contact == null) 3145 this.contact = new ArrayList<ContactPoint>(); 3146 this.contact.add(t); 3147 return this; 3148 } 3149 3150 /** 3151 * @return The first repetition of repeating field {@link #contact}, creating it 3152 * if it does not already exist 3153 */ 3154 public ContactPoint getContactFirstRep() { 3155 if (getContact().isEmpty()) { 3156 addContact(); 3157 } 3158 return getContact().get(0); 3159 } 3160 3161 /** 3162 * @return {@link #url} (A network address on which the device may be contacted 3163 * directly.). This is the underlying object with id, value and 3164 * extensions. The accessor "getUrl" gives direct access to the value 3165 */ 3166 public UriType getUrlElement() { 3167 if (this.url == null) 3168 if (Configuration.errorOnAutoCreate()) 3169 throw new Error("Attempt to auto-create DeviceDefinition.url"); 3170 else if (Configuration.doAutoCreate()) 3171 this.url = new UriType(); // bb 3172 return this.url; 3173 } 3174 3175 public boolean hasUrlElement() { 3176 return this.url != null && !this.url.isEmpty(); 3177 } 3178 3179 public boolean hasUrl() { 3180 return this.url != null && !this.url.isEmpty(); 3181 } 3182 3183 /** 3184 * @param value {@link #url} (A network address on which the device may be 3185 * contacted directly.). This is the underlying object with id, 3186 * value and extensions. The accessor "getUrl" gives direct access 3187 * to the value 3188 */ 3189 public DeviceDefinition setUrlElement(UriType value) { 3190 this.url = value; 3191 return this; 3192 } 3193 3194 /** 3195 * @return A network address on which the device may be contacted directly. 3196 */ 3197 public String getUrl() { 3198 return this.url == null ? null : this.url.getValue(); 3199 } 3200 3201 /** 3202 * @param value A network address on which the device may be contacted directly. 3203 */ 3204 public DeviceDefinition setUrl(String value) { 3205 if (Utilities.noString(value)) 3206 this.url = null; 3207 else { 3208 if (this.url == null) 3209 this.url = new UriType(); 3210 this.url.setValue(value); 3211 } 3212 return this; 3213 } 3214 3215 /** 3216 * @return {@link #onlineInformation} (Access to on-line information about the 3217 * device.). This is the underlying object with id, value and 3218 * extensions. The accessor "getOnlineInformation" gives direct access 3219 * to the value 3220 */ 3221 public UriType getOnlineInformationElement() { 3222 if (this.onlineInformation == null) 3223 if (Configuration.errorOnAutoCreate()) 3224 throw new Error("Attempt to auto-create DeviceDefinition.onlineInformation"); 3225 else if (Configuration.doAutoCreate()) 3226 this.onlineInformation = new UriType(); // bb 3227 return this.onlineInformation; 3228 } 3229 3230 public boolean hasOnlineInformationElement() { 3231 return this.onlineInformation != null && !this.onlineInformation.isEmpty(); 3232 } 3233 3234 public boolean hasOnlineInformation() { 3235 return this.onlineInformation != null && !this.onlineInformation.isEmpty(); 3236 } 3237 3238 /** 3239 * @param value {@link #onlineInformation} (Access to on-line information about 3240 * the device.). This is the underlying object with id, value and 3241 * extensions. The accessor "getOnlineInformation" gives direct 3242 * access to the value 3243 */ 3244 public DeviceDefinition setOnlineInformationElement(UriType value) { 3245 this.onlineInformation = value; 3246 return this; 3247 } 3248 3249 /** 3250 * @return Access to on-line information about the device. 3251 */ 3252 public String getOnlineInformation() { 3253 return this.onlineInformation == null ? null : this.onlineInformation.getValue(); 3254 } 3255 3256 /** 3257 * @param value Access to on-line information about the device. 3258 */ 3259 public DeviceDefinition setOnlineInformation(String value) { 3260 if (Utilities.noString(value)) 3261 this.onlineInformation = null; 3262 else { 3263 if (this.onlineInformation == null) 3264 this.onlineInformation = new UriType(); 3265 this.onlineInformation.setValue(value); 3266 } 3267 return this; 3268 } 3269 3270 /** 3271 * @return {@link #note} (Descriptive information, usage information or 3272 * implantation information that is not captured in an existing 3273 * element.) 3274 */ 3275 public List<Annotation> getNote() { 3276 if (this.note == null) 3277 this.note = new ArrayList<Annotation>(); 3278 return this.note; 3279 } 3280 3281 /** 3282 * @return Returns a reference to <code>this</code> for easy method chaining 3283 */ 3284 public DeviceDefinition setNote(List<Annotation> theNote) { 3285 this.note = theNote; 3286 return this; 3287 } 3288 3289 public boolean hasNote() { 3290 if (this.note == null) 3291 return false; 3292 for (Annotation item : this.note) 3293 if (!item.isEmpty()) 3294 return true; 3295 return false; 3296 } 3297 3298 public Annotation addNote() { // 3 3299 Annotation t = new Annotation(); 3300 if (this.note == null) 3301 this.note = new ArrayList<Annotation>(); 3302 this.note.add(t); 3303 return t; 3304 } 3305 3306 public DeviceDefinition addNote(Annotation t) { // 3 3307 if (t == null) 3308 return this; 3309 if (this.note == null) 3310 this.note = new ArrayList<Annotation>(); 3311 this.note.add(t); 3312 return this; 3313 } 3314 3315 /** 3316 * @return The first repetition of repeating field {@link #note}, creating it if 3317 * it does not already exist 3318 */ 3319 public Annotation getNoteFirstRep() { 3320 if (getNote().isEmpty()) { 3321 addNote(); 3322 } 3323 return getNote().get(0); 3324 } 3325 3326 /** 3327 * @return {@link #quantity} (The quantity of the device present in the 3328 * packaging (e.g. the number of devices present in a pack, or the 3329 * number of devices in the same package of the medicinal product).) 3330 */ 3331 public Quantity getQuantity() { 3332 if (this.quantity == null) 3333 if (Configuration.errorOnAutoCreate()) 3334 throw new Error("Attempt to auto-create DeviceDefinition.quantity"); 3335 else if (Configuration.doAutoCreate()) 3336 this.quantity = new Quantity(); // cc 3337 return this.quantity; 3338 } 3339 3340 public boolean hasQuantity() { 3341 return this.quantity != null && !this.quantity.isEmpty(); 3342 } 3343 3344 /** 3345 * @param value {@link #quantity} (The quantity of the device present in the 3346 * packaging (e.g. the number of devices present in a pack, or the 3347 * number of devices in the same package of the medicinal 3348 * product).) 3349 */ 3350 public DeviceDefinition setQuantity(Quantity value) { 3351 this.quantity = value; 3352 return this; 3353 } 3354 3355 /** 3356 * @return {@link #parentDevice} (The parent device it can be part of.) 3357 */ 3358 public Reference getParentDevice() { 3359 if (this.parentDevice == null) 3360 if (Configuration.errorOnAutoCreate()) 3361 throw new Error("Attempt to auto-create DeviceDefinition.parentDevice"); 3362 else if (Configuration.doAutoCreate()) 3363 this.parentDevice = new Reference(); // cc 3364 return this.parentDevice; 3365 } 3366 3367 public boolean hasParentDevice() { 3368 return this.parentDevice != null && !this.parentDevice.isEmpty(); 3369 } 3370 3371 /** 3372 * @param value {@link #parentDevice} (The parent device it can be part of.) 3373 */ 3374 public DeviceDefinition setParentDevice(Reference value) { 3375 this.parentDevice = value; 3376 return this; 3377 } 3378 3379 /** 3380 * @return {@link #parentDevice} The actual object that is the target of the 3381 * reference. The reference library doesn't populate this, but you can 3382 * use it to hold the resource if you resolve it. (The parent device it 3383 * can be part of.) 3384 */ 3385 public DeviceDefinition getParentDeviceTarget() { 3386 if (this.parentDeviceTarget == null) 3387 if (Configuration.errorOnAutoCreate()) 3388 throw new Error("Attempt to auto-create DeviceDefinition.parentDevice"); 3389 else if (Configuration.doAutoCreate()) 3390 this.parentDeviceTarget = new DeviceDefinition(); // aa 3391 return this.parentDeviceTarget; 3392 } 3393 3394 /** 3395 * @param value {@link #parentDevice} The actual object that is the target of 3396 * the reference. The reference library doesn't use these, but you 3397 * can use it to hold the resource if you resolve it. (The parent 3398 * device it can be part of.) 3399 */ 3400 public DeviceDefinition setParentDeviceTarget(DeviceDefinition value) { 3401 this.parentDeviceTarget = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return {@link #material} (A substance used to create the material(s) of 3407 * which the device is made.) 3408 */ 3409 public List<DeviceDefinitionMaterialComponent> getMaterial() { 3410 if (this.material == null) 3411 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 3412 return this.material; 3413 } 3414 3415 /** 3416 * @return Returns a reference to <code>this</code> for easy method chaining 3417 */ 3418 public DeviceDefinition setMaterial(List<DeviceDefinitionMaterialComponent> theMaterial) { 3419 this.material = theMaterial; 3420 return this; 3421 } 3422 3423 public boolean hasMaterial() { 3424 if (this.material == null) 3425 return false; 3426 for (DeviceDefinitionMaterialComponent item : this.material) 3427 if (!item.isEmpty()) 3428 return true; 3429 return false; 3430 } 3431 3432 public DeviceDefinitionMaterialComponent addMaterial() { // 3 3433 DeviceDefinitionMaterialComponent t = new DeviceDefinitionMaterialComponent(); 3434 if (this.material == null) 3435 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 3436 this.material.add(t); 3437 return t; 3438 } 3439 3440 public DeviceDefinition addMaterial(DeviceDefinitionMaterialComponent t) { // 3 3441 if (t == null) 3442 return this; 3443 if (this.material == null) 3444 this.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 3445 this.material.add(t); 3446 return this; 3447 } 3448 3449 /** 3450 * @return The first repetition of repeating field {@link #material}, creating 3451 * it if it does not already exist 3452 */ 3453 public DeviceDefinitionMaterialComponent getMaterialFirstRep() { 3454 if (getMaterial().isEmpty()) { 3455 addMaterial(); 3456 } 3457 return getMaterial().get(0); 3458 } 3459 3460 protected void listChildren(List<Property> children) { 3461 super.listChildren(children); 3462 children.add(new Property("identifier", "Identifier", 3463 "Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID.", 3464 0, java.lang.Integer.MAX_VALUE, identifier)); 3465 children.add(new Property("udiDeviceIdentifier", "", 3466 "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 3467 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier)); 3468 children.add(new Property("manufacturer[x]", "string|Reference(Organization)", "A name of the manufacturer.", 0, 1, 3469 manufacturer)); 3470 children.add(new Property("deviceName", "", "A name given to the device to identify it.", 0, 3471 java.lang.Integer.MAX_VALUE, deviceName)); 3472 children.add(new Property("modelNumber", "string", "The model number for the device.", 0, 1, modelNumber)); 3473 children.add(new Property("type", "CodeableConcept", "What kind of device or device system this is.", 0, 1, type)); 3474 children.add(new Property("specialization", "", 3475 "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.", 3476 0, java.lang.Integer.MAX_VALUE, specialization)); 3477 children.add(new Property("version", "string", "The available versions of the device, e.g., software versions.", 0, 3478 java.lang.Integer.MAX_VALUE, version)); 3479 children.add(new Property("safety", "CodeableConcept", "Safety characteristics of the device.", 0, 3480 java.lang.Integer.MAX_VALUE, safety)); 3481 children.add(new Property("shelfLifeStorage", "ProductShelfLife", "Shelf Life and storage information.", 0, 3482 java.lang.Integer.MAX_VALUE, shelfLifeStorage)); 3483 children.add(new Property("physicalCharacteristics", "ProdCharacteristic", "Dimensions, color etc.", 0, 1, 3484 physicalCharacteristics)); 3485 children.add(new Property("languageCode", "CodeableConcept", 3486 "Language code for the human-readable text strings produced by the device (all supported).", 0, 3487 java.lang.Integer.MAX_VALUE, languageCode)); 3488 children.add(new Property("capability", "", "Device capabilities.", 0, java.lang.Integer.MAX_VALUE, capability)); 3489 children.add(new Property("property", "", 3490 "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.", 3491 0, java.lang.Integer.MAX_VALUE, property)); 3492 children.add(new Property("owner", "Reference(Organization)", 3493 "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner)); 3494 children.add(new Property("contact", "ContactPoint", 3495 "Contact details for an organization or a particular human that is responsible for the device.", 0, 3496 java.lang.Integer.MAX_VALUE, contact)); 3497 children 3498 .add(new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 1, url)); 3499 children.add(new Property("onlineInformation", "uri", "Access to on-line information about the device.", 0, 1, 3500 onlineInformation)); 3501 children.add(new Property("note", "Annotation", 3502 "Descriptive information, usage information or implantation information that is not captured in an existing element.", 3503 0, java.lang.Integer.MAX_VALUE, note)); 3504 children.add(new Property("quantity", "Quantity", 3505 "The quantity of the device present in the packaging (e.g. the number of devices present in a pack, or the number of devices in the same package of the medicinal product).", 3506 0, 1, quantity)); 3507 children.add(new Property("parentDevice", "Reference(DeviceDefinition)", "The parent device it can be part of.", 0, 3508 1, parentDevice)); 3509 children.add(new Property("material", "", "A substance used to create the material(s) of which the device is made.", 3510 0, java.lang.Integer.MAX_VALUE, material)); 3511 } 3512 3513 @Override 3514 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3515 switch (_hash) { 3516 case -1618432855: 3517 /* identifier */ return new Property("identifier", "Identifier", 3518 "Unique instance identifiers assigned to a device by the software, manufacturers, other organizations or owners. For example: handle ID.", 3519 0, java.lang.Integer.MAX_VALUE, identifier); 3520 case -99121287: 3521 /* udiDeviceIdentifier */ return new Property("udiDeviceIdentifier", "", 3522 "Unique device identifier (UDI) assigned to device label or package. Note that the Device may include multiple udiCarriers as it either may include just the udiCarrier for the jurisdiction it is sold, or for multiple jurisdictions it could have been sold.", 3523 0, java.lang.Integer.MAX_VALUE, udiDeviceIdentifier); 3524 case 418079503: 3525 /* manufacturer[x] */ return new Property("manufacturer[x]", "string|Reference(Organization)", 3526 "A name of the manufacturer.", 0, 1, manufacturer); 3527 case -1969347631: 3528 /* manufacturer */ return new Property("manufacturer[x]", "string|Reference(Organization)", 3529 "A name of the manufacturer.", 0, 1, manufacturer); 3530 case -630681790: 3531 /* manufacturerString */ return new Property("manufacturer[x]", "string|Reference(Organization)", 3532 "A name of the manufacturer.", 0, 1, manufacturer); 3533 case 1104934522: 3534 /* manufacturerReference */ return new Property("manufacturer[x]", "string|Reference(Organization)", 3535 "A name of the manufacturer.", 0, 1, manufacturer); 3536 case 780988929: 3537 /* deviceName */ return new Property("deviceName", "", "A name given to the device to identify it.", 0, 3538 java.lang.Integer.MAX_VALUE, deviceName); 3539 case 346619858: 3540 /* modelNumber */ return new Property("modelNumber", "string", "The model number for the device.", 0, 1, 3541 modelNumber); 3542 case 3575610: 3543 /* type */ return new Property("type", "CodeableConcept", "What kind of device or device system this is.", 0, 1, 3544 type); 3545 case 682815883: 3546 /* specialization */ return new Property("specialization", "", 3547 "The capabilities supported on a device, the standards to which the device conforms for a particular purpose, and used for the communication.", 3548 0, java.lang.Integer.MAX_VALUE, specialization); 3549 case 351608024: 3550 /* version */ return new Property("version", "string", 3551 "The available versions of the device, e.g., software versions.", 0, java.lang.Integer.MAX_VALUE, version); 3552 case -909893934: 3553 /* safety */ return new Property("safety", "CodeableConcept", "Safety characteristics of the device.", 0, 3554 java.lang.Integer.MAX_VALUE, safety); 3555 case 172049237: 3556 /* shelfLifeStorage */ return new Property("shelfLifeStorage", "ProductShelfLife", 3557 "Shelf Life and storage information.", 0, java.lang.Integer.MAX_VALUE, shelfLifeStorage); 3558 case -1599676319: 3559 /* physicalCharacteristics */ return new Property("physicalCharacteristics", "ProdCharacteristic", 3560 "Dimensions, color etc.", 0, 1, physicalCharacteristics); 3561 case -2092349083: 3562 /* languageCode */ return new Property("languageCode", "CodeableConcept", 3563 "Language code for the human-readable text strings produced by the device (all supported).", 0, 3564 java.lang.Integer.MAX_VALUE, languageCode); 3565 case -783669992: 3566 /* capability */ return new Property("capability", "", "Device capabilities.", 0, java.lang.Integer.MAX_VALUE, 3567 capability); 3568 case -993141291: 3569 /* property */ return new Property("property", "", 3570 "The actual configuration settings of a device as it actually operates, e.g., regulation status, time properties.", 3571 0, java.lang.Integer.MAX_VALUE, property); 3572 case 106164915: 3573 /* owner */ return new Property("owner", "Reference(Organization)", 3574 "An organization that is responsible for the provision and ongoing maintenance of the device.", 0, 1, owner); 3575 case 951526432: 3576 /* contact */ return new Property("contact", "ContactPoint", 3577 "Contact details for an organization or a particular human that is responsible for the device.", 0, 3578 java.lang.Integer.MAX_VALUE, contact); 3579 case 116079: 3580 /* url */ return new Property("url", "uri", "A network address on which the device may be contacted directly.", 0, 3581 1, url); 3582 case -788511527: 3583 /* onlineInformation */ return new Property("onlineInformation", "uri", 3584 "Access to on-line information about the device.", 0, 1, onlineInformation); 3585 case 3387378: 3586 /* note */ return new Property("note", "Annotation", 3587 "Descriptive information, usage information or implantation information that is not captured in an existing element.", 3588 0, java.lang.Integer.MAX_VALUE, note); 3589 case -1285004149: 3590 /* quantity */ return new Property("quantity", "Quantity", 3591 "The quantity of the device present in the packaging (e.g. the number of devices present in a pack, or the number of devices in the same package of the medicinal product).", 3592 0, 1, quantity); 3593 case 620260256: 3594 /* parentDevice */ return new Property("parentDevice", "Reference(DeviceDefinition)", 3595 "The parent device it can be part of.", 0, 1, parentDevice); 3596 case 299066663: 3597 /* material */ return new Property("material", "", 3598 "A substance used to create the material(s) of which the device is made.", 0, java.lang.Integer.MAX_VALUE, 3599 material); 3600 default: 3601 return super.getNamedProperty(_hash, _name, _checkValid); 3602 } 3603 3604 } 3605 3606 @Override 3607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3608 switch (hash) { 3609 case -1618432855: 3610 /* identifier */ return this.identifier == null ? new Base[0] 3611 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3612 case -99121287: 3613 /* udiDeviceIdentifier */ return this.udiDeviceIdentifier == null ? new Base[0] 3614 : this.udiDeviceIdentifier.toArray(new Base[this.udiDeviceIdentifier.size()]); // DeviceDefinitionUdiDeviceIdentifierComponent 3615 case -1969347631: 3616 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Type 3617 case 780988929: 3618 /* deviceName */ return this.deviceName == null ? new Base[0] 3619 : this.deviceName.toArray(new Base[this.deviceName.size()]); // DeviceDefinitionDeviceNameComponent 3620 case 346619858: 3621 /* modelNumber */ return this.modelNumber == null ? new Base[0] : new Base[] { this.modelNumber }; // StringType 3622 case 3575610: 3623 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3624 case 682815883: 3625 /* specialization */ return this.specialization == null ? new Base[0] 3626 : this.specialization.toArray(new Base[this.specialization.size()]); // DeviceDefinitionSpecializationComponent 3627 case 351608024: 3628 /* version */ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // StringType 3629 case -909893934: 3630 /* safety */ return this.safety == null ? new Base[0] : this.safety.toArray(new Base[this.safety.size()]); // CodeableConcept 3631 case 172049237: 3632 /* shelfLifeStorage */ return this.shelfLifeStorage == null ? new Base[0] 3633 : this.shelfLifeStorage.toArray(new Base[this.shelfLifeStorage.size()]); // ProductShelfLife 3634 case -1599676319: 3635 /* physicalCharacteristics */ return this.physicalCharacteristics == null ? new Base[0] 3636 : new Base[] { this.physicalCharacteristics }; // ProdCharacteristic 3637 case -2092349083: 3638 /* languageCode */ return this.languageCode == null ? new Base[0] 3639 : this.languageCode.toArray(new Base[this.languageCode.size()]); // CodeableConcept 3640 case -783669992: 3641 /* capability */ return this.capability == null ? new Base[0] 3642 : this.capability.toArray(new Base[this.capability.size()]); // DeviceDefinitionCapabilityComponent 3643 case -993141291: 3644 /* property */ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // DeviceDefinitionPropertyComponent 3645 case 106164915: 3646 /* owner */ return this.owner == null ? new Base[0] : new Base[] { this.owner }; // Reference 3647 case 951526432: 3648 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 3649 case 116079: 3650 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3651 case -788511527: 3652 /* onlineInformation */ return this.onlineInformation == null ? new Base[0] 3653 : new Base[] { this.onlineInformation }; // UriType 3654 case 3387378: 3655 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3656 case -1285004149: 3657 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 3658 case 620260256: 3659 /* parentDevice */ return this.parentDevice == null ? new Base[0] : new Base[] { this.parentDevice }; // Reference 3660 case 299066663: 3661 /* material */ return this.material == null ? new Base[0] : this.material.toArray(new Base[this.material.size()]); // DeviceDefinitionMaterialComponent 3662 default: 3663 return super.getProperty(hash, name, checkValid); 3664 } 3665 3666 } 3667 3668 @Override 3669 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3670 switch (hash) { 3671 case -1618432855: // identifier 3672 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3673 return value; 3674 case -99121287: // udiDeviceIdentifier 3675 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); // DeviceDefinitionUdiDeviceIdentifierComponent 3676 return value; 3677 case -1969347631: // manufacturer 3678 this.manufacturer = castToType(value); // Type 3679 return value; 3680 case 780988929: // deviceName 3681 this.getDeviceName().add((DeviceDefinitionDeviceNameComponent) value); // DeviceDefinitionDeviceNameComponent 3682 return value; 3683 case 346619858: // modelNumber 3684 this.modelNumber = castToString(value); // StringType 3685 return value; 3686 case 3575610: // type 3687 this.type = castToCodeableConcept(value); // CodeableConcept 3688 return value; 3689 case 682815883: // specialization 3690 this.getSpecialization().add((DeviceDefinitionSpecializationComponent) value); // DeviceDefinitionSpecializationComponent 3691 return value; 3692 case 351608024: // version 3693 this.getVersion().add(castToString(value)); // StringType 3694 return value; 3695 case -909893934: // safety 3696 this.getSafety().add(castToCodeableConcept(value)); // CodeableConcept 3697 return value; 3698 case 172049237: // shelfLifeStorage 3699 this.getShelfLifeStorage().add(castToProductShelfLife(value)); // ProductShelfLife 3700 return value; 3701 case -1599676319: // physicalCharacteristics 3702 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 3703 return value; 3704 case -2092349083: // languageCode 3705 this.getLanguageCode().add(castToCodeableConcept(value)); // CodeableConcept 3706 return value; 3707 case -783669992: // capability 3708 this.getCapability().add((DeviceDefinitionCapabilityComponent) value); // DeviceDefinitionCapabilityComponent 3709 return value; 3710 case -993141291: // property 3711 this.getProperty().add((DeviceDefinitionPropertyComponent) value); // DeviceDefinitionPropertyComponent 3712 return value; 3713 case 106164915: // owner 3714 this.owner = castToReference(value); // Reference 3715 return value; 3716 case 951526432: // contact 3717 this.getContact().add(castToContactPoint(value)); // ContactPoint 3718 return value; 3719 case 116079: // url 3720 this.url = castToUri(value); // UriType 3721 return value; 3722 case -788511527: // onlineInformation 3723 this.onlineInformation = castToUri(value); // UriType 3724 return value; 3725 case 3387378: // note 3726 this.getNote().add(castToAnnotation(value)); // Annotation 3727 return value; 3728 case -1285004149: // quantity 3729 this.quantity = castToQuantity(value); // Quantity 3730 return value; 3731 case 620260256: // parentDevice 3732 this.parentDevice = castToReference(value); // Reference 3733 return value; 3734 case 299066663: // material 3735 this.getMaterial().add((DeviceDefinitionMaterialComponent) value); // DeviceDefinitionMaterialComponent 3736 return value; 3737 default: 3738 return super.setProperty(hash, name, value); 3739 } 3740 3741 } 3742 3743 @Override 3744 public Base setProperty(String name, Base value) throws FHIRException { 3745 if (name.equals("identifier")) { 3746 this.getIdentifier().add(castToIdentifier(value)); 3747 } else if (name.equals("udiDeviceIdentifier")) { 3748 this.getUdiDeviceIdentifier().add((DeviceDefinitionUdiDeviceIdentifierComponent) value); 3749 } else if (name.equals("manufacturer[x]")) { 3750 this.manufacturer = castToType(value); // Type 3751 } else if (name.equals("deviceName")) { 3752 this.getDeviceName().add((DeviceDefinitionDeviceNameComponent) value); 3753 } else if (name.equals("modelNumber")) { 3754 this.modelNumber = castToString(value); // StringType 3755 } else if (name.equals("type")) { 3756 this.type = castToCodeableConcept(value); // CodeableConcept 3757 } else if (name.equals("specialization")) { 3758 this.getSpecialization().add((DeviceDefinitionSpecializationComponent) value); 3759 } else if (name.equals("version")) { 3760 this.getVersion().add(castToString(value)); 3761 } else if (name.equals("safety")) { 3762 this.getSafety().add(castToCodeableConcept(value)); 3763 } else if (name.equals("shelfLifeStorage")) { 3764 this.getShelfLifeStorage().add(castToProductShelfLife(value)); 3765 } else if (name.equals("physicalCharacteristics")) { 3766 this.physicalCharacteristics = castToProdCharacteristic(value); // ProdCharacteristic 3767 } else if (name.equals("languageCode")) { 3768 this.getLanguageCode().add(castToCodeableConcept(value)); 3769 } else if (name.equals("capability")) { 3770 this.getCapability().add((DeviceDefinitionCapabilityComponent) value); 3771 } else if (name.equals("property")) { 3772 this.getProperty().add((DeviceDefinitionPropertyComponent) value); 3773 } else if (name.equals("owner")) { 3774 this.owner = castToReference(value); // Reference 3775 } else if (name.equals("contact")) { 3776 this.getContact().add(castToContactPoint(value)); 3777 } else if (name.equals("url")) { 3778 this.url = castToUri(value); // UriType 3779 } else if (name.equals("onlineInformation")) { 3780 this.onlineInformation = castToUri(value); // UriType 3781 } else if (name.equals("note")) { 3782 this.getNote().add(castToAnnotation(value)); 3783 } else if (name.equals("quantity")) { 3784 this.quantity = castToQuantity(value); // Quantity 3785 } else if (name.equals("parentDevice")) { 3786 this.parentDevice = castToReference(value); // Reference 3787 } else if (name.equals("material")) { 3788 this.getMaterial().add((DeviceDefinitionMaterialComponent) value); 3789 } else 3790 return super.setProperty(name, value); 3791 return value; 3792 } 3793 3794 @Override 3795 public Base makeProperty(int hash, String name) throws FHIRException { 3796 switch (hash) { 3797 case -1618432855: 3798 return addIdentifier(); 3799 case -99121287: 3800 return addUdiDeviceIdentifier(); 3801 case 418079503: 3802 return getManufacturer(); 3803 case -1969347631: 3804 return getManufacturer(); 3805 case 780988929: 3806 return addDeviceName(); 3807 case 346619858: 3808 return getModelNumberElement(); 3809 case 3575610: 3810 return getType(); 3811 case 682815883: 3812 return addSpecialization(); 3813 case 351608024: 3814 return addVersionElement(); 3815 case -909893934: 3816 return addSafety(); 3817 case 172049237: 3818 return addShelfLifeStorage(); 3819 case -1599676319: 3820 return getPhysicalCharacteristics(); 3821 case -2092349083: 3822 return addLanguageCode(); 3823 case -783669992: 3824 return addCapability(); 3825 case -993141291: 3826 return addProperty(); 3827 case 106164915: 3828 return getOwner(); 3829 case 951526432: 3830 return addContact(); 3831 case 116079: 3832 return getUrlElement(); 3833 case -788511527: 3834 return getOnlineInformationElement(); 3835 case 3387378: 3836 return addNote(); 3837 case -1285004149: 3838 return getQuantity(); 3839 case 620260256: 3840 return getParentDevice(); 3841 case 299066663: 3842 return addMaterial(); 3843 default: 3844 return super.makeProperty(hash, name); 3845 } 3846 3847 } 3848 3849 @Override 3850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3851 switch (hash) { 3852 case -1618432855: 3853 /* identifier */ return new String[] { "Identifier" }; 3854 case -99121287: 3855 /* udiDeviceIdentifier */ return new String[] {}; 3856 case -1969347631: 3857 /* manufacturer */ return new String[] { "string", "Reference" }; 3858 case 780988929: 3859 /* deviceName */ return new String[] {}; 3860 case 346619858: 3861 /* modelNumber */ return new String[] { "string" }; 3862 case 3575610: 3863 /* type */ return new String[] { "CodeableConcept" }; 3864 case 682815883: 3865 /* specialization */ return new String[] {}; 3866 case 351608024: 3867 /* version */ return new String[] { "string" }; 3868 case -909893934: 3869 /* safety */ return new String[] { "CodeableConcept" }; 3870 case 172049237: 3871 /* shelfLifeStorage */ return new String[] { "ProductShelfLife" }; 3872 case -1599676319: 3873 /* physicalCharacteristics */ return new String[] { "ProdCharacteristic" }; 3874 case -2092349083: 3875 /* languageCode */ return new String[] { "CodeableConcept" }; 3876 case -783669992: 3877 /* capability */ return new String[] {}; 3878 case -993141291: 3879 /* property */ return new String[] {}; 3880 case 106164915: 3881 /* owner */ return new String[] { "Reference" }; 3882 case 951526432: 3883 /* contact */ return new String[] { "ContactPoint" }; 3884 case 116079: 3885 /* url */ return new String[] { "uri" }; 3886 case -788511527: 3887 /* onlineInformation */ return new String[] { "uri" }; 3888 case 3387378: 3889 /* note */ return new String[] { "Annotation" }; 3890 case -1285004149: 3891 /* quantity */ return new String[] { "Quantity" }; 3892 case 620260256: 3893 /* parentDevice */ return new String[] { "Reference" }; 3894 case 299066663: 3895 /* material */ return new String[] {}; 3896 default: 3897 return super.getTypesForProperty(hash, name); 3898 } 3899 3900 } 3901 3902 @Override 3903 public Base addChild(String name) throws FHIRException { 3904 if (name.equals("identifier")) { 3905 return addIdentifier(); 3906 } else if (name.equals("udiDeviceIdentifier")) { 3907 return addUdiDeviceIdentifier(); 3908 } else if (name.equals("manufacturerString")) { 3909 this.manufacturer = new StringType(); 3910 return this.manufacturer; 3911 } else if (name.equals("manufacturerReference")) { 3912 this.manufacturer = new Reference(); 3913 return this.manufacturer; 3914 } else if (name.equals("deviceName")) { 3915 return addDeviceName(); 3916 } else if (name.equals("modelNumber")) { 3917 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.modelNumber"); 3918 } else if (name.equals("type")) { 3919 this.type = new CodeableConcept(); 3920 return this.type; 3921 } else if (name.equals("specialization")) { 3922 return addSpecialization(); 3923 } else if (name.equals("version")) { 3924 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.version"); 3925 } else if (name.equals("safety")) { 3926 return addSafety(); 3927 } else if (name.equals("shelfLifeStorage")) { 3928 return addShelfLifeStorage(); 3929 } else if (name.equals("physicalCharacteristics")) { 3930 this.physicalCharacteristics = new ProdCharacteristic(); 3931 return this.physicalCharacteristics; 3932 } else if (name.equals("languageCode")) { 3933 return addLanguageCode(); 3934 } else if (name.equals("capability")) { 3935 return addCapability(); 3936 } else if (name.equals("property")) { 3937 return addProperty(); 3938 } else if (name.equals("owner")) { 3939 this.owner = new Reference(); 3940 return this.owner; 3941 } else if (name.equals("contact")) { 3942 return addContact(); 3943 } else if (name.equals("url")) { 3944 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.url"); 3945 } else if (name.equals("onlineInformation")) { 3946 throw new FHIRException("Cannot call addChild on a singleton property DeviceDefinition.onlineInformation"); 3947 } else if (name.equals("note")) { 3948 return addNote(); 3949 } else if (name.equals("quantity")) { 3950 this.quantity = new Quantity(); 3951 return this.quantity; 3952 } else if (name.equals("parentDevice")) { 3953 this.parentDevice = new Reference(); 3954 return this.parentDevice; 3955 } else if (name.equals("material")) { 3956 return addMaterial(); 3957 } else 3958 return super.addChild(name); 3959 } 3960 3961 public String fhirType() { 3962 return "DeviceDefinition"; 3963 3964 } 3965 3966 public DeviceDefinition copy() { 3967 DeviceDefinition dst = new DeviceDefinition(); 3968 copyValues(dst); 3969 return dst; 3970 } 3971 3972 public void copyValues(DeviceDefinition dst) { 3973 super.copyValues(dst); 3974 if (identifier != null) { 3975 dst.identifier = new ArrayList<Identifier>(); 3976 for (Identifier i : identifier) 3977 dst.identifier.add(i.copy()); 3978 } 3979 ; 3980 if (udiDeviceIdentifier != null) { 3981 dst.udiDeviceIdentifier = new ArrayList<DeviceDefinitionUdiDeviceIdentifierComponent>(); 3982 for (DeviceDefinitionUdiDeviceIdentifierComponent i : udiDeviceIdentifier) 3983 dst.udiDeviceIdentifier.add(i.copy()); 3984 } 3985 ; 3986 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 3987 if (deviceName != null) { 3988 dst.deviceName = new ArrayList<DeviceDefinitionDeviceNameComponent>(); 3989 for (DeviceDefinitionDeviceNameComponent i : deviceName) 3990 dst.deviceName.add(i.copy()); 3991 } 3992 ; 3993 dst.modelNumber = modelNumber == null ? null : modelNumber.copy(); 3994 dst.type = type == null ? null : type.copy(); 3995 if (specialization != null) { 3996 dst.specialization = new ArrayList<DeviceDefinitionSpecializationComponent>(); 3997 for (DeviceDefinitionSpecializationComponent i : specialization) 3998 dst.specialization.add(i.copy()); 3999 } 4000 ; 4001 if (version != null) { 4002 dst.version = new ArrayList<StringType>(); 4003 for (StringType i : version) 4004 dst.version.add(i.copy()); 4005 } 4006 ; 4007 if (safety != null) { 4008 dst.safety = new ArrayList<CodeableConcept>(); 4009 for (CodeableConcept i : safety) 4010 dst.safety.add(i.copy()); 4011 } 4012 ; 4013 if (shelfLifeStorage != null) { 4014 dst.shelfLifeStorage = new ArrayList<ProductShelfLife>(); 4015 for (ProductShelfLife i : shelfLifeStorage) 4016 dst.shelfLifeStorage.add(i.copy()); 4017 } 4018 ; 4019 dst.physicalCharacteristics = physicalCharacteristics == null ? null : physicalCharacteristics.copy(); 4020 if (languageCode != null) { 4021 dst.languageCode = new ArrayList<CodeableConcept>(); 4022 for (CodeableConcept i : languageCode) 4023 dst.languageCode.add(i.copy()); 4024 } 4025 ; 4026 if (capability != null) { 4027 dst.capability = new ArrayList<DeviceDefinitionCapabilityComponent>(); 4028 for (DeviceDefinitionCapabilityComponent i : capability) 4029 dst.capability.add(i.copy()); 4030 } 4031 ; 4032 if (property != null) { 4033 dst.property = new ArrayList<DeviceDefinitionPropertyComponent>(); 4034 for (DeviceDefinitionPropertyComponent i : property) 4035 dst.property.add(i.copy()); 4036 } 4037 ; 4038 dst.owner = owner == null ? null : owner.copy(); 4039 if (contact != null) { 4040 dst.contact = new ArrayList<ContactPoint>(); 4041 for (ContactPoint i : contact) 4042 dst.contact.add(i.copy()); 4043 } 4044 ; 4045 dst.url = url == null ? null : url.copy(); 4046 dst.onlineInformation = onlineInformation == null ? null : onlineInformation.copy(); 4047 if (note != null) { 4048 dst.note = new ArrayList<Annotation>(); 4049 for (Annotation i : note) 4050 dst.note.add(i.copy()); 4051 } 4052 ; 4053 dst.quantity = quantity == null ? null : quantity.copy(); 4054 dst.parentDevice = parentDevice == null ? null : parentDevice.copy(); 4055 if (material != null) { 4056 dst.material = new ArrayList<DeviceDefinitionMaterialComponent>(); 4057 for (DeviceDefinitionMaterialComponent i : material) 4058 dst.material.add(i.copy()); 4059 } 4060 ; 4061 } 4062 4063 protected DeviceDefinition typedCopy() { 4064 return copy(); 4065 } 4066 4067 @Override 4068 public boolean equalsDeep(Base other_) { 4069 if (!super.equalsDeep(other_)) 4070 return false; 4071 if (!(other_ instanceof DeviceDefinition)) 4072 return false; 4073 DeviceDefinition o = (DeviceDefinition) other_; 4074 return compareDeep(identifier, o.identifier, true) && compareDeep(udiDeviceIdentifier, o.udiDeviceIdentifier, true) 4075 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(deviceName, o.deviceName, true) 4076 && compareDeep(modelNumber, o.modelNumber, true) && compareDeep(type, o.type, true) 4077 && compareDeep(specialization, o.specialization, true) && compareDeep(version, o.version, true) 4078 && compareDeep(safety, o.safety, true) && compareDeep(shelfLifeStorage, o.shelfLifeStorage, true) 4079 && compareDeep(physicalCharacteristics, o.physicalCharacteristics, true) 4080 && compareDeep(languageCode, o.languageCode, true) && compareDeep(capability, o.capability, true) 4081 && compareDeep(property, o.property, true) && compareDeep(owner, o.owner, true) 4082 && compareDeep(contact, o.contact, true) && compareDeep(url, o.url, true) 4083 && compareDeep(onlineInformation, o.onlineInformation, true) && compareDeep(note, o.note, true) 4084 && compareDeep(quantity, o.quantity, true) && compareDeep(parentDevice, o.parentDevice, true) 4085 && compareDeep(material, o.material, true); 4086 } 4087 4088 @Override 4089 public boolean equalsShallow(Base other_) { 4090 if (!super.equalsShallow(other_)) 4091 return false; 4092 if (!(other_ instanceof DeviceDefinition)) 4093 return false; 4094 DeviceDefinition o = (DeviceDefinition) other_; 4095 return compareValues(modelNumber, o.modelNumber, true) && compareValues(version, o.version, true) 4096 && compareValues(url, o.url, true) && compareValues(onlineInformation, o.onlineInformation, true); 4097 } 4098 4099 public boolean isEmpty() { 4100 return super.isEmpty() 4101 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, udiDeviceIdentifier, manufacturer, deviceName, modelNumber, 4102 type, specialization, version, safety, shelfLifeStorage, physicalCharacteristics, languageCode, capability, 4103 property, owner, contact, url, onlineInformation, note, quantity, parentDevice, material); 4104 } 4105 4106 @Override 4107 public ResourceType getResourceType() { 4108 return ResourceType.DeviceDefinition; 4109 } 4110 4111 /** 4112 * Search parameter: <b>parent</b> 4113 * <p> 4114 * Description: <b>The parent DeviceDefinition resource</b><br> 4115 * Type: <b>reference</b><br> 4116 * Path: <b>DeviceDefinition.parentDevice</b><br> 4117 * </p> 4118 */ 4119 @SearchParamDefinition(name = "parent", path = "DeviceDefinition.parentDevice", description = "The parent DeviceDefinition resource", type = "reference", target = { 4120 DeviceDefinition.class }) 4121 public static final String SP_PARENT = "parent"; 4122 /** 4123 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 4124 * <p> 4125 * Description: <b>The parent DeviceDefinition resource</b><br> 4126 * Type: <b>reference</b><br> 4127 * Path: <b>DeviceDefinition.parentDevice</b><br> 4128 * </p> 4129 */ 4130 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4131 SP_PARENT); 4132 4133 /** 4134 * Constant for fluent queries to be used to add include statements. Specifies 4135 * the path value of "<b>DeviceDefinition:parent</b>". 4136 */ 4137 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 4138 "DeviceDefinition:parent").toLocked(); 4139 4140 /** 4141 * Search parameter: <b>identifier</b> 4142 * <p> 4143 * Description: <b>The identifier of the component</b><br> 4144 * Type: <b>token</b><br> 4145 * Path: <b>DeviceDefinition.identifier</b><br> 4146 * </p> 4147 */ 4148 @SearchParamDefinition(name = "identifier", path = "DeviceDefinition.identifier", description = "The identifier of the component", type = "token") 4149 public static final String SP_IDENTIFIER = "identifier"; 4150 /** 4151 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4152 * <p> 4153 * Description: <b>The identifier of the component</b><br> 4154 * Type: <b>token</b><br> 4155 * Path: <b>DeviceDefinition.identifier</b><br> 4156 * </p> 4157 */ 4158 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4159 SP_IDENTIFIER); 4160 4161 /** 4162 * Search parameter: <b>type</b> 4163 * <p> 4164 * Description: <b>The device component type</b><br> 4165 * Type: <b>token</b><br> 4166 * Path: <b>DeviceDefinition.type</b><br> 4167 * </p> 4168 */ 4169 @SearchParamDefinition(name = "type", path = "DeviceDefinition.type", description = "The device component type", type = "token") 4170 public static final String SP_TYPE = "type"; 4171 /** 4172 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4173 * <p> 4174 * Description: <b>The device component type</b><br> 4175 * Type: <b>token</b><br> 4176 * Path: <b>DeviceDefinition.type</b><br> 4177 * </p> 4178 */ 4179 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4180 SP_TYPE); 4181 4182}