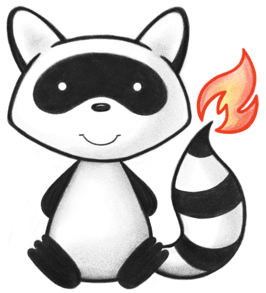
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A record of a device being used by a patient where the record is the result 046 * of a report from the patient or another clinician. 047 */ 048@ResourceDef(name = "DeviceUseStatement", profile = "http://hl7.org/fhir/StructureDefinition/DeviceUseStatement") 049public class DeviceUseStatement extends DomainResource { 050 051 public enum DeviceUseStatementStatus { 052 /** 053 * The device is still being used. 054 */ 055 ACTIVE, 056 /** 057 * The device is no longer being used. 058 */ 059 COMPLETED, 060 /** 061 * The statement was recorded incorrectly. 062 */ 063 ENTEREDINERROR, 064 /** 065 * The device may be used at some time in the future. 066 */ 067 INTENDED, 068 /** 069 * Actions implied by the statement have been permanently halted, before all of 070 * them occurred. 071 */ 072 STOPPED, 073 /** 074 * Actions implied by the statement have been temporarily halted, but are 075 * expected to continue later. May also be called "suspended". 076 */ 077 ONHOLD, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static DeviceUseStatementStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("completed".equals(codeString)) 089 return COMPLETED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("intended".equals(codeString)) 093 return INTENDED; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case ACTIVE: 107 return "active"; 108 case COMPLETED: 109 return "completed"; 110 case ENTEREDINERROR: 111 return "entered-in-error"; 112 case INTENDED: 113 return "intended"; 114 case STOPPED: 115 return "stopped"; 116 case ONHOLD: 117 return "on-hold"; 118 case NULL: 119 return null; 120 default: 121 return "?"; 122 } 123 } 124 125 public String getSystem() { 126 switch (this) { 127 case ACTIVE: 128 return "http://hl7.org/fhir/device-statement-status"; 129 case COMPLETED: 130 return "http://hl7.org/fhir/device-statement-status"; 131 case ENTEREDINERROR: 132 return "http://hl7.org/fhir/device-statement-status"; 133 case INTENDED: 134 return "http://hl7.org/fhir/device-statement-status"; 135 case STOPPED: 136 return "http://hl7.org/fhir/device-statement-status"; 137 case ONHOLD: 138 return "http://hl7.org/fhir/device-statement-status"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDefinition() { 147 switch (this) { 148 case ACTIVE: 149 return "The device is still being used."; 150 case COMPLETED: 151 return "The device is no longer being used."; 152 case ENTEREDINERROR: 153 return "The statement was recorded incorrectly."; 154 case INTENDED: 155 return "The device may be used at some time in the future."; 156 case STOPPED: 157 return "Actions implied by the statement have been permanently halted, before all of them occurred."; 158 case ONHOLD: 159 return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDisplay() { 168 switch (this) { 169 case ACTIVE: 170 return "Active"; 171 case COMPLETED: 172 return "Completed"; 173 case ENTEREDINERROR: 174 return "Entered in Error"; 175 case INTENDED: 176 return "Intended"; 177 case STOPPED: 178 return "Stopped"; 179 case ONHOLD: 180 return "On Hold"; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 } 188 189 public static class DeviceUseStatementStatusEnumFactory implements EnumFactory<DeviceUseStatementStatus> { 190 public DeviceUseStatementStatus fromCode(String codeString) throws IllegalArgumentException { 191 if (codeString == null || "".equals(codeString)) 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("active".equals(codeString)) 195 return DeviceUseStatementStatus.ACTIVE; 196 if ("completed".equals(codeString)) 197 return DeviceUseStatementStatus.COMPLETED; 198 if ("entered-in-error".equals(codeString)) 199 return DeviceUseStatementStatus.ENTEREDINERROR; 200 if ("intended".equals(codeString)) 201 return DeviceUseStatementStatus.INTENDED; 202 if ("stopped".equals(codeString)) 203 return DeviceUseStatementStatus.STOPPED; 204 if ("on-hold".equals(codeString)) 205 return DeviceUseStatementStatus.ONHOLD; 206 throw new IllegalArgumentException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 207 } 208 209 public Enumeration<DeviceUseStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 210 if (code == null) 211 return null; 212 if (code.isEmpty()) 213 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.NULL, code); 214 String codeString = code.asStringValue(); 215 if (codeString == null || "".equals(codeString)) 216 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.NULL, code); 217 if ("active".equals(codeString)) 218 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ACTIVE, code); 219 if ("completed".equals(codeString)) 220 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.COMPLETED, code); 221 if ("entered-in-error".equals(codeString)) 222 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ENTEREDINERROR, code); 223 if ("intended".equals(codeString)) 224 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.INTENDED, code); 225 if ("stopped".equals(codeString)) 226 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.STOPPED, code); 227 if ("on-hold".equals(codeString)) 228 return new Enumeration<DeviceUseStatementStatus>(this, DeviceUseStatementStatus.ONHOLD, code); 229 throw new FHIRException("Unknown DeviceUseStatementStatus code '" + codeString + "'"); 230 } 231 232 public String toCode(DeviceUseStatementStatus code) { 233 if (code == DeviceUseStatementStatus.NULL) 234 return null; 235 if (code == DeviceUseStatementStatus.ACTIVE) 236 return "active"; 237 if (code == DeviceUseStatementStatus.COMPLETED) 238 return "completed"; 239 if (code == DeviceUseStatementStatus.ENTEREDINERROR) 240 return "entered-in-error"; 241 if (code == DeviceUseStatementStatus.INTENDED) 242 return "intended"; 243 if (code == DeviceUseStatementStatus.STOPPED) 244 return "stopped"; 245 if (code == DeviceUseStatementStatus.ONHOLD) 246 return "on-hold"; 247 return "?"; 248 } 249 250 public String toSystem(DeviceUseStatementStatus code) { 251 return code.getSystem(); 252 } 253 } 254 255 /** 256 * An external identifier for this statement such as an IRI. 257 */ 258 @Child(name = "identifier", type = { 259 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 260 @Description(shortDefinition = "External identifier for this record", formalDefinition = "An external identifier for this statement such as an IRI.") 261 protected List<Identifier> identifier; 262 263 /** 264 * A plan, proposal or order that is fulfilled in whole or in part by this 265 * DeviceUseStatement. 266 */ 267 @Child(name = "basedOn", type = { 268 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 269 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.") 270 protected List<Reference> basedOn; 271 /** 272 * The actual objects that are the target of the reference (A plan, proposal or 273 * order that is fulfilled in whole or in part by this DeviceUseStatement.) 274 */ 275 protected List<ServiceRequest> basedOnTarget; 276 277 /** 278 * A code representing the patient or other source's judgment about the state of 279 * the device used that this statement is about. Generally this will be active 280 * or completed. 281 */ 282 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 283 @Description(shortDefinition = "active | completed | entered-in-error +", formalDefinition = "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.") 284 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/device-statement-status") 285 protected Enumeration<DeviceUseStatementStatus> status; 286 287 /** 288 * The patient who used the device. 289 */ 290 @Child(name = "subject", type = { Patient.class, 291 Group.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 292 @Description(shortDefinition = "Patient using device", formalDefinition = "The patient who used the device.") 293 protected Reference subject; 294 295 /** 296 * The actual object that is the target of the reference (The patient who used 297 * the device.) 298 */ 299 protected Resource subjectTarget; 300 301 /** 302 * Allows linking the DeviceUseStatement to the underlying Request, or to other 303 * information that supports or is used to derive the DeviceUseStatement. 304 */ 305 @Child(name = "derivedFrom", type = { ServiceRequest.class, Procedure.class, Claim.class, Observation.class, 306 QuestionnaireResponse.class, 307 DocumentReference.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 308 @Description(shortDefinition = "Supporting information", formalDefinition = "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.") 309 protected List<Reference> derivedFrom; 310 /** 311 * The actual objects that are the target of the reference (Allows linking the 312 * DeviceUseStatement to the underlying Request, or to other information that 313 * supports or is used to derive the DeviceUseStatement.) 314 */ 315 protected List<Resource> derivedFromTarget; 316 317 /** 318 * How often the device was used. 319 */ 320 @Child(name = "timing", type = { Timing.class, Period.class, 321 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 322 @Description(shortDefinition = "How often the device was used", formalDefinition = "How often the device was used.") 323 protected Type timing; 324 325 /** 326 * The time at which the statement was made/recorded. 327 */ 328 @Child(name = "recordedOn", type = { 329 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 330 @Description(shortDefinition = "When statement was recorded", formalDefinition = "The time at which the statement was made/recorded.") 331 protected DateTimeType recordedOn; 332 333 /** 334 * Who reported the device was being used by the patient. 335 */ 336 @Child(name = "source", type = { Patient.class, Practitioner.class, PractitionerRole.class, 337 RelatedPerson.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 338 @Description(shortDefinition = "Who made the statement", formalDefinition = "Who reported the device was being used by the patient.") 339 protected Reference source; 340 341 /** 342 * The actual object that is the target of the reference (Who reported the 343 * device was being used by the patient.) 344 */ 345 protected Resource sourceTarget; 346 347 /** 348 * The details of the device used. 349 */ 350 @Child(name = "device", type = { Device.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 351 @Description(shortDefinition = "Reference to device used", formalDefinition = "The details of the device used.") 352 protected Reference device; 353 354 /** 355 * The actual object that is the target of the reference (The details of the 356 * device used.) 357 */ 358 protected Device deviceTarget; 359 360 /** 361 * Reason or justification for the use of the device. 362 */ 363 @Child(name = "reasonCode", type = { 364 CodeableConcept.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 365 @Description(shortDefinition = "Why device was used", formalDefinition = "Reason or justification for the use of the device.") 366 protected List<CodeableConcept> reasonCode; 367 368 /** 369 * Indicates another resource whose existence justifies this DeviceUseStatement. 370 */ 371 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 372 DocumentReference.class, 373 Media.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 374 @Description(shortDefinition = "Why was DeviceUseStatement performed?", formalDefinition = "Indicates another resource whose existence justifies this DeviceUseStatement.") 375 protected List<Reference> reasonReference; 376 /** 377 * The actual objects that are the target of the reference (Indicates another 378 * resource whose existence justifies this DeviceUseStatement.) 379 */ 380 protected List<Resource> reasonReferenceTarget; 381 382 /** 383 * Indicates the anotomic location on the subject's body where the device was 384 * used ( i.e. the target). 385 */ 386 @Child(name = "bodySite", type = { 387 CodeableConcept.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 388 @Description(shortDefinition = "Target body site", formalDefinition = "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).") 389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 390 protected CodeableConcept bodySite; 391 392 /** 393 * Details about the device statement that were not represented at all or 394 * sufficiently in one of the attributes provided in a class. These may include 395 * for example a comment, an instruction, or a note associated with the 396 * statement. 397 */ 398 @Child(name = "note", type = { 399 Annotation.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 400 @Description(shortDefinition = "Addition details (comments, instructions)", formalDefinition = "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.") 401 protected List<Annotation> note; 402 403 private static final long serialVersionUID = -968330048L; 404 405 /** 406 * Constructor 407 */ 408 public DeviceUseStatement() { 409 super(); 410 } 411 412 /** 413 * Constructor 414 */ 415 public DeviceUseStatement(Enumeration<DeviceUseStatementStatus> status, Reference subject, Reference device) { 416 super(); 417 this.status = status; 418 this.subject = subject; 419 this.device = device; 420 } 421 422 /** 423 * @return {@link #identifier} (An external identifier for this statement such 424 * as an IRI.) 425 */ 426 public List<Identifier> getIdentifier() { 427 if (this.identifier == null) 428 this.identifier = new ArrayList<Identifier>(); 429 return this.identifier; 430 } 431 432 /** 433 * @return Returns a reference to <code>this</code> for easy method chaining 434 */ 435 public DeviceUseStatement setIdentifier(List<Identifier> theIdentifier) { 436 this.identifier = theIdentifier; 437 return this; 438 } 439 440 public boolean hasIdentifier() { 441 if (this.identifier == null) 442 return false; 443 for (Identifier item : this.identifier) 444 if (!item.isEmpty()) 445 return true; 446 return false; 447 } 448 449 public Identifier addIdentifier() { // 3 450 Identifier t = new Identifier(); 451 if (this.identifier == null) 452 this.identifier = new ArrayList<Identifier>(); 453 this.identifier.add(t); 454 return t; 455 } 456 457 public DeviceUseStatement addIdentifier(Identifier t) { // 3 458 if (t == null) 459 return this; 460 if (this.identifier == null) 461 this.identifier = new ArrayList<Identifier>(); 462 this.identifier.add(t); 463 return this; 464 } 465 466 /** 467 * @return The first repetition of repeating field {@link #identifier}, creating 468 * it if it does not already exist 469 */ 470 public Identifier getIdentifierFirstRep() { 471 if (getIdentifier().isEmpty()) { 472 addIdentifier(); 473 } 474 return getIdentifier().get(0); 475 } 476 477 /** 478 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 479 * whole or in part by this DeviceUseStatement.) 480 */ 481 public List<Reference> getBasedOn() { 482 if (this.basedOn == null) 483 this.basedOn = new ArrayList<Reference>(); 484 return this.basedOn; 485 } 486 487 /** 488 * @return Returns a reference to <code>this</code> for easy method chaining 489 */ 490 public DeviceUseStatement setBasedOn(List<Reference> theBasedOn) { 491 this.basedOn = theBasedOn; 492 return this; 493 } 494 495 public boolean hasBasedOn() { 496 if (this.basedOn == null) 497 return false; 498 for (Reference item : this.basedOn) 499 if (!item.isEmpty()) 500 return true; 501 return false; 502 } 503 504 public Reference addBasedOn() { // 3 505 Reference t = new Reference(); 506 if (this.basedOn == null) 507 this.basedOn = new ArrayList<Reference>(); 508 this.basedOn.add(t); 509 return t; 510 } 511 512 public DeviceUseStatement addBasedOn(Reference t) { // 3 513 if (t == null) 514 return this; 515 if (this.basedOn == null) 516 this.basedOn = new ArrayList<Reference>(); 517 this.basedOn.add(t); 518 return this; 519 } 520 521 /** 522 * @return The first repetition of repeating field {@link #basedOn}, creating it 523 * if it does not already exist 524 */ 525 public Reference getBasedOnFirstRep() { 526 if (getBasedOn().isEmpty()) { 527 addBasedOn(); 528 } 529 return getBasedOn().get(0); 530 } 531 532 /** 533 * @deprecated Use Reference#setResource(IBaseResource) instead 534 */ 535 @Deprecated 536 public List<ServiceRequest> getBasedOnTarget() { 537 if (this.basedOnTarget == null) 538 this.basedOnTarget = new ArrayList<ServiceRequest>(); 539 return this.basedOnTarget; 540 } 541 542 /** 543 * @deprecated Use Reference#setResource(IBaseResource) instead 544 */ 545 @Deprecated 546 public ServiceRequest addBasedOnTarget() { 547 ServiceRequest r = new ServiceRequest(); 548 if (this.basedOnTarget == null) 549 this.basedOnTarget = new ArrayList<ServiceRequest>(); 550 this.basedOnTarget.add(r); 551 return r; 552 } 553 554 /** 555 * @return {@link #status} (A code representing the patient or other source's 556 * judgment about the state of the device used that this statement is 557 * about. Generally this will be active or completed.). This is the 558 * underlying object with id, value and extensions. The accessor 559 * "getStatus" gives direct access to the value 560 */ 561 public Enumeration<DeviceUseStatementStatus> getStatusElement() { 562 if (this.status == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create DeviceUseStatement.status"); 565 else if (Configuration.doAutoCreate()) 566 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); // bb 567 return this.status; 568 } 569 570 public boolean hasStatusElement() { 571 return this.status != null && !this.status.isEmpty(); 572 } 573 574 public boolean hasStatus() { 575 return this.status != null && !this.status.isEmpty(); 576 } 577 578 /** 579 * @param value {@link #status} (A code representing the patient or other 580 * source's judgment about the state of the device used that this 581 * statement is about. Generally this will be active or 582 * completed.). This is the underlying object with id, value and 583 * extensions. The accessor "getStatus" gives direct access to the 584 * value 585 */ 586 public DeviceUseStatement setStatusElement(Enumeration<DeviceUseStatementStatus> value) { 587 this.status = value; 588 return this; 589 } 590 591 /** 592 * @return A code representing the patient or other source's judgment about the 593 * state of the device used that this statement is about. Generally this 594 * will be active or completed. 595 */ 596 public DeviceUseStatementStatus getStatus() { 597 return this.status == null ? null : this.status.getValue(); 598 } 599 600 /** 601 * @param value A code representing the patient or other source's judgment about 602 * the state of the device used that this statement is about. 603 * Generally this will be active or completed. 604 */ 605 public DeviceUseStatement setStatus(DeviceUseStatementStatus value) { 606 if (this.status == null) 607 this.status = new Enumeration<DeviceUseStatementStatus>(new DeviceUseStatementStatusEnumFactory()); 608 this.status.setValue(value); 609 return this; 610 } 611 612 /** 613 * @return {@link #subject} (The patient who used the device.) 614 */ 615 public Reference getSubject() { 616 if (this.subject == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create DeviceUseStatement.subject"); 619 else if (Configuration.doAutoCreate()) 620 this.subject = new Reference(); // cc 621 return this.subject; 622 } 623 624 public boolean hasSubject() { 625 return this.subject != null && !this.subject.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #subject} (The patient who used the device.) 630 */ 631 public DeviceUseStatement setSubject(Reference value) { 632 this.subject = value; 633 return this; 634 } 635 636 /** 637 * @return {@link #subject} The actual object that is the target of the 638 * reference. The reference library doesn't populate this, but you can 639 * use it to hold the resource if you resolve it. (The patient who used 640 * the device.) 641 */ 642 public Resource getSubjectTarget() { 643 return this.subjectTarget; 644 } 645 646 /** 647 * @param value {@link #subject} The actual object that is the target of the 648 * reference. The reference library doesn't use these, but you can 649 * use it to hold the resource if you resolve it. (The patient who 650 * used the device.) 651 */ 652 public DeviceUseStatement setSubjectTarget(Resource value) { 653 this.subjectTarget = value; 654 return this; 655 } 656 657 /** 658 * @return {@link #derivedFrom} (Allows linking the DeviceUseStatement to the 659 * underlying Request, or to other information that supports or is used 660 * to derive the DeviceUseStatement.) 661 */ 662 public List<Reference> getDerivedFrom() { 663 if (this.derivedFrom == null) 664 this.derivedFrom = new ArrayList<Reference>(); 665 return this.derivedFrom; 666 } 667 668 /** 669 * @return Returns a reference to <code>this</code> for easy method chaining 670 */ 671 public DeviceUseStatement setDerivedFrom(List<Reference> theDerivedFrom) { 672 this.derivedFrom = theDerivedFrom; 673 return this; 674 } 675 676 public boolean hasDerivedFrom() { 677 if (this.derivedFrom == null) 678 return false; 679 for (Reference item : this.derivedFrom) 680 if (!item.isEmpty()) 681 return true; 682 return false; 683 } 684 685 public Reference addDerivedFrom() { // 3 686 Reference t = new Reference(); 687 if (this.derivedFrom == null) 688 this.derivedFrom = new ArrayList<Reference>(); 689 this.derivedFrom.add(t); 690 return t; 691 } 692 693 public DeviceUseStatement addDerivedFrom(Reference t) { // 3 694 if (t == null) 695 return this; 696 if (this.derivedFrom == null) 697 this.derivedFrom = new ArrayList<Reference>(); 698 this.derivedFrom.add(t); 699 return this; 700 } 701 702 /** 703 * @return The first repetition of repeating field {@link #derivedFrom}, 704 * creating it if it does not already exist 705 */ 706 public Reference getDerivedFromFirstRep() { 707 if (getDerivedFrom().isEmpty()) { 708 addDerivedFrom(); 709 } 710 return getDerivedFrom().get(0); 711 } 712 713 /** 714 * @deprecated Use Reference#setResource(IBaseResource) instead 715 */ 716 @Deprecated 717 public List<Resource> getDerivedFromTarget() { 718 if (this.derivedFromTarget == null) 719 this.derivedFromTarget = new ArrayList<Resource>(); 720 return this.derivedFromTarget; 721 } 722 723 /** 724 * @return {@link #timing} (How often the device was used.) 725 */ 726 public Type getTiming() { 727 return this.timing; 728 } 729 730 /** 731 * @return {@link #timing} (How often the device was used.) 732 */ 733 public Timing getTimingTiming() throws FHIRException { 734 if (this.timing == null) 735 this.timing = new Timing(); 736 if (!(this.timing instanceof Timing)) 737 throw new FHIRException( 738 "Type mismatch: the type Timing was expected, but " + this.timing.getClass().getName() + " was encountered"); 739 return (Timing) this.timing; 740 } 741 742 public boolean hasTimingTiming() { 743 return this.timing instanceof Timing; 744 } 745 746 /** 747 * @return {@link #timing} (How often the device was used.) 748 */ 749 public Period getTimingPeriod() throws FHIRException { 750 if (this.timing == null) 751 this.timing = new Period(); 752 if (!(this.timing instanceof Period)) 753 throw new FHIRException( 754 "Type mismatch: the type Period was expected, but " + this.timing.getClass().getName() + " was encountered"); 755 return (Period) this.timing; 756 } 757 758 public boolean hasTimingPeriod() { 759 return this.timing instanceof Period; 760 } 761 762 /** 763 * @return {@link #timing} (How often the device was used.) 764 */ 765 public DateTimeType getTimingDateTimeType() throws FHIRException { 766 if (this.timing == null) 767 this.timing = new DateTimeType(); 768 if (!(this.timing instanceof DateTimeType)) 769 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 770 + this.timing.getClass().getName() + " was encountered"); 771 return (DateTimeType) this.timing; 772 } 773 774 public boolean hasTimingDateTimeType() { 775 return this.timing instanceof DateTimeType; 776 } 777 778 public boolean hasTiming() { 779 return this.timing != null && !this.timing.isEmpty(); 780 } 781 782 /** 783 * @param value {@link #timing} (How often the device was used.) 784 */ 785 public DeviceUseStatement setTiming(Type value) { 786 if (value != null && !(value instanceof Timing || value instanceof Period || value instanceof DateTimeType)) 787 throw new Error("Not the right type for DeviceUseStatement.timing[x]: " + value.fhirType()); 788 this.timing = value; 789 return this; 790 } 791 792 /** 793 * @return {@link #recordedOn} (The time at which the statement was 794 * made/recorded.). This is the underlying object with id, value and 795 * extensions. The accessor "getRecordedOn" gives direct access to the 796 * value 797 */ 798 public DateTimeType getRecordedOnElement() { 799 if (this.recordedOn == null) 800 if (Configuration.errorOnAutoCreate()) 801 throw new Error("Attempt to auto-create DeviceUseStatement.recordedOn"); 802 else if (Configuration.doAutoCreate()) 803 this.recordedOn = new DateTimeType(); // bb 804 return this.recordedOn; 805 } 806 807 public boolean hasRecordedOnElement() { 808 return this.recordedOn != null && !this.recordedOn.isEmpty(); 809 } 810 811 public boolean hasRecordedOn() { 812 return this.recordedOn != null && !this.recordedOn.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #recordedOn} (The time at which the statement was 817 * made/recorded.). This is the underlying object with id, value 818 * and extensions. The accessor "getRecordedOn" gives direct access 819 * to the value 820 */ 821 public DeviceUseStatement setRecordedOnElement(DateTimeType value) { 822 this.recordedOn = value; 823 return this; 824 } 825 826 /** 827 * @return The time at which the statement was made/recorded. 828 */ 829 public Date getRecordedOn() { 830 return this.recordedOn == null ? null : this.recordedOn.getValue(); 831 } 832 833 /** 834 * @param value The time at which the statement was made/recorded. 835 */ 836 public DeviceUseStatement setRecordedOn(Date value) { 837 if (value == null) 838 this.recordedOn = null; 839 else { 840 if (this.recordedOn == null) 841 this.recordedOn = new DateTimeType(); 842 this.recordedOn.setValue(value); 843 } 844 return this; 845 } 846 847 /** 848 * @return {@link #source} (Who reported the device was being used by the 849 * patient.) 850 */ 851 public Reference getSource() { 852 if (this.source == null) 853 if (Configuration.errorOnAutoCreate()) 854 throw new Error("Attempt to auto-create DeviceUseStatement.source"); 855 else if (Configuration.doAutoCreate()) 856 this.source = new Reference(); // cc 857 return this.source; 858 } 859 860 public boolean hasSource() { 861 return this.source != null && !this.source.isEmpty(); 862 } 863 864 /** 865 * @param value {@link #source} (Who reported the device was being used by the 866 * patient.) 867 */ 868 public DeviceUseStatement setSource(Reference value) { 869 this.source = value; 870 return this; 871 } 872 873 /** 874 * @return {@link #source} The actual object that is the target of the 875 * reference. The reference library doesn't populate this, but you can 876 * use it to hold the resource if you resolve it. (Who reported the 877 * device was being used by the patient.) 878 */ 879 public Resource getSourceTarget() { 880 return this.sourceTarget; 881 } 882 883 /** 884 * @param value {@link #source} The actual object that is the target of the 885 * reference. The reference library doesn't use these, but you can 886 * use it to hold the resource if you resolve it. (Who reported the 887 * device was being used by the patient.) 888 */ 889 public DeviceUseStatement setSourceTarget(Resource value) { 890 this.sourceTarget = value; 891 return this; 892 } 893 894 /** 895 * @return {@link #device} (The details of the device used.) 896 */ 897 public Reference getDevice() { 898 if (this.device == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 901 else if (Configuration.doAutoCreate()) 902 this.device = new Reference(); // cc 903 return this.device; 904 } 905 906 public boolean hasDevice() { 907 return this.device != null && !this.device.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #device} (The details of the device used.) 912 */ 913 public DeviceUseStatement setDevice(Reference value) { 914 this.device = value; 915 return this; 916 } 917 918 /** 919 * @return {@link #device} The actual object that is the target of the 920 * reference. The reference library doesn't populate this, but you can 921 * use it to hold the resource if you resolve it. (The details of the 922 * device used.) 923 */ 924 public Device getDeviceTarget() { 925 if (this.deviceTarget == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create DeviceUseStatement.device"); 928 else if (Configuration.doAutoCreate()) 929 this.deviceTarget = new Device(); // aa 930 return this.deviceTarget; 931 } 932 933 /** 934 * @param value {@link #device} The actual object that is the target of the 935 * reference. The reference library doesn't use these, but you can 936 * use it to hold the resource if you resolve it. (The details of 937 * the device used.) 938 */ 939 public DeviceUseStatement setDeviceTarget(Device value) { 940 this.deviceTarget = value; 941 return this; 942 } 943 944 /** 945 * @return {@link #reasonCode} (Reason or justification for the use of the 946 * device.) 947 */ 948 public List<CodeableConcept> getReasonCode() { 949 if (this.reasonCode == null) 950 this.reasonCode = new ArrayList<CodeableConcept>(); 951 return this.reasonCode; 952 } 953 954 /** 955 * @return Returns a reference to <code>this</code> for easy method chaining 956 */ 957 public DeviceUseStatement setReasonCode(List<CodeableConcept> theReasonCode) { 958 this.reasonCode = theReasonCode; 959 return this; 960 } 961 962 public boolean hasReasonCode() { 963 if (this.reasonCode == null) 964 return false; 965 for (CodeableConcept item : this.reasonCode) 966 if (!item.isEmpty()) 967 return true; 968 return false; 969 } 970 971 public CodeableConcept addReasonCode() { // 3 972 CodeableConcept t = new CodeableConcept(); 973 if (this.reasonCode == null) 974 this.reasonCode = new ArrayList<CodeableConcept>(); 975 this.reasonCode.add(t); 976 return t; 977 } 978 979 public DeviceUseStatement addReasonCode(CodeableConcept t) { // 3 980 if (t == null) 981 return this; 982 if (this.reasonCode == null) 983 this.reasonCode = new ArrayList<CodeableConcept>(); 984 this.reasonCode.add(t); 985 return this; 986 } 987 988 /** 989 * @return The first repetition of repeating field {@link #reasonCode}, creating 990 * it if it does not already exist 991 */ 992 public CodeableConcept getReasonCodeFirstRep() { 993 if (getReasonCode().isEmpty()) { 994 addReasonCode(); 995 } 996 return getReasonCode().get(0); 997 } 998 999 /** 1000 * @return {@link #reasonReference} (Indicates another resource whose existence 1001 * justifies this DeviceUseStatement.) 1002 */ 1003 public List<Reference> getReasonReference() { 1004 if (this.reasonReference == null) 1005 this.reasonReference = new ArrayList<Reference>(); 1006 return this.reasonReference; 1007 } 1008 1009 /** 1010 * @return Returns a reference to <code>this</code> for easy method chaining 1011 */ 1012 public DeviceUseStatement setReasonReference(List<Reference> theReasonReference) { 1013 this.reasonReference = theReasonReference; 1014 return this; 1015 } 1016 1017 public boolean hasReasonReference() { 1018 if (this.reasonReference == null) 1019 return false; 1020 for (Reference item : this.reasonReference) 1021 if (!item.isEmpty()) 1022 return true; 1023 return false; 1024 } 1025 1026 public Reference addReasonReference() { // 3 1027 Reference t = new Reference(); 1028 if (this.reasonReference == null) 1029 this.reasonReference = new ArrayList<Reference>(); 1030 this.reasonReference.add(t); 1031 return t; 1032 } 1033 1034 public DeviceUseStatement addReasonReference(Reference t) { // 3 1035 if (t == null) 1036 return this; 1037 if (this.reasonReference == null) 1038 this.reasonReference = new ArrayList<Reference>(); 1039 this.reasonReference.add(t); 1040 return this; 1041 } 1042 1043 /** 1044 * @return The first repetition of repeating field {@link #reasonReference}, 1045 * creating it if it does not already exist 1046 */ 1047 public Reference getReasonReferenceFirstRep() { 1048 if (getReasonReference().isEmpty()) { 1049 addReasonReference(); 1050 } 1051 return getReasonReference().get(0); 1052 } 1053 1054 /** 1055 * @deprecated Use Reference#setResource(IBaseResource) instead 1056 */ 1057 @Deprecated 1058 public List<Resource> getReasonReferenceTarget() { 1059 if (this.reasonReferenceTarget == null) 1060 this.reasonReferenceTarget = new ArrayList<Resource>(); 1061 return this.reasonReferenceTarget; 1062 } 1063 1064 /** 1065 * @return {@link #bodySite} (Indicates the anotomic location on the subject's 1066 * body where the device was used ( i.e. the target).) 1067 */ 1068 public CodeableConcept getBodySite() { 1069 if (this.bodySite == null) 1070 if (Configuration.errorOnAutoCreate()) 1071 throw new Error("Attempt to auto-create DeviceUseStatement.bodySite"); 1072 else if (Configuration.doAutoCreate()) 1073 this.bodySite = new CodeableConcept(); // cc 1074 return this.bodySite; 1075 } 1076 1077 public boolean hasBodySite() { 1078 return this.bodySite != null && !this.bodySite.isEmpty(); 1079 } 1080 1081 /** 1082 * @param value {@link #bodySite} (Indicates the anotomic location on the 1083 * subject's body where the device was used ( i.e. the target).) 1084 */ 1085 public DeviceUseStatement setBodySite(CodeableConcept value) { 1086 this.bodySite = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #note} (Details about the device statement that were not 1092 * represented at all or sufficiently in one of the attributes provided 1093 * in a class. These may include for example a comment, an instruction, 1094 * or a note associated with the statement.) 1095 */ 1096 public List<Annotation> getNote() { 1097 if (this.note == null) 1098 this.note = new ArrayList<Annotation>(); 1099 return this.note; 1100 } 1101 1102 /** 1103 * @return Returns a reference to <code>this</code> for easy method chaining 1104 */ 1105 public DeviceUseStatement setNote(List<Annotation> theNote) { 1106 this.note = theNote; 1107 return this; 1108 } 1109 1110 public boolean hasNote() { 1111 if (this.note == null) 1112 return false; 1113 for (Annotation item : this.note) 1114 if (!item.isEmpty()) 1115 return true; 1116 return false; 1117 } 1118 1119 public Annotation addNote() { // 3 1120 Annotation t = new Annotation(); 1121 if (this.note == null) 1122 this.note = new ArrayList<Annotation>(); 1123 this.note.add(t); 1124 return t; 1125 } 1126 1127 public DeviceUseStatement addNote(Annotation t) { // 3 1128 if (t == null) 1129 return this; 1130 if (this.note == null) 1131 this.note = new ArrayList<Annotation>(); 1132 this.note.add(t); 1133 return this; 1134 } 1135 1136 /** 1137 * @return The first repetition of repeating field {@link #note}, creating it if 1138 * it does not already exist 1139 */ 1140 public Annotation getNoteFirstRep() { 1141 if (getNote().isEmpty()) { 1142 addNote(); 1143 } 1144 return getNote().get(0); 1145 } 1146 1147 protected void listChildren(List<Property> children) { 1148 super.listChildren(children); 1149 children.add(new Property("identifier", "Identifier", "An external identifier for this statement such as an IRI.", 1150 0, java.lang.Integer.MAX_VALUE, identifier)); 1151 children.add(new Property("basedOn", "Reference(ServiceRequest)", 1152 "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.", 0, 1153 java.lang.Integer.MAX_VALUE, basedOn)); 1154 children.add(new Property("status", "code", 1155 "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 1156 0, 1, status)); 1157 children 1158 .add(new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, subject)); 1159 children.add(new Property("derivedFrom", 1160 "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", 1161 "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.", 1162 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1163 children.add(new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, timing)); 1164 children.add( 1165 new Property("recordedOn", "dateTime", "The time at which the statement was made/recorded.", 0, 1, recordedOn)); 1166 children.add(new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1167 "Who reported the device was being used by the patient.", 0, 1, source)); 1168 children.add(new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device)); 1169 children.add(new Property("reasonCode", "CodeableConcept", "Reason or justification for the use of the device.", 0, 1170 java.lang.Integer.MAX_VALUE, reasonCode)); 1171 children.add( 1172 new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Media)", 1173 "Indicates another resource whose existence justifies this DeviceUseStatement.", 0, 1174 java.lang.Integer.MAX_VALUE, reasonReference)); 1175 children.add(new Property("bodySite", "CodeableConcept", 1176 "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, 1177 bodySite)); 1178 children.add(new Property("note", "Annotation", 1179 "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 1180 0, java.lang.Integer.MAX_VALUE, note)); 1181 } 1182 1183 @Override 1184 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1185 switch (_hash) { 1186 case -1618432855: 1187 /* identifier */ return new Property("identifier", "Identifier", 1188 "An external identifier for this statement such as an IRI.", 0, java.lang.Integer.MAX_VALUE, identifier); 1189 case -332612366: 1190 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 1191 "A plan, proposal or order that is fulfilled in whole or in part by this DeviceUseStatement.", 0, 1192 java.lang.Integer.MAX_VALUE, basedOn); 1193 case -892481550: 1194 /* status */ return new Property("status", "code", 1195 "A code representing the patient or other source's judgment about the state of the device used that this statement is about. Generally this will be active or completed.", 1196 0, 1, status); 1197 case -1867885268: 1198 /* subject */ return new Property("subject", "Reference(Patient|Group)", "The patient who used the device.", 0, 1, 1199 subject); 1200 case 1077922663: 1201 /* derivedFrom */ return new Property("derivedFrom", 1202 "Reference(ServiceRequest|Procedure|Claim|Observation|QuestionnaireResponse|DocumentReference)", 1203 "Allows linking the DeviceUseStatement to the underlying Request, or to other information that supports or is used to derive the DeviceUseStatement.", 1204 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1205 case 164632566: 1206 /* timing[x] */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, 1207 timing); 1208 case -873664438: 1209 /* timing */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1, 1210 timing); 1211 case -497554124: 1212 /* timingTiming */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1213 1, timing); 1214 case -615615829: 1215 /* timingPeriod */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 0, 1216 1, timing); 1217 case -1837458939: 1218 /* timingDateTime */ return new Property("timing[x]", "Timing|Period|dateTime", "How often the device was used.", 1219 0, 1, timing); 1220 case 735397551: 1221 /* recordedOn */ return new Property("recordedOn", "dateTime", 1222 "The time at which the statement was made/recorded.", 0, 1, recordedOn); 1223 case -896505829: 1224 /* source */ return new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson)", 1225 "Who reported the device was being used by the patient.", 0, 1, source); 1226 case -1335157162: 1227 /* device */ return new Property("device", "Reference(Device)", "The details of the device used.", 0, 1, device); 1228 case 722137681: 1229 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1230 "Reason or justification for the use of the device.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1231 case -1146218137: 1232 /* reasonReference */ return new Property("reasonReference", 1233 "Reference(Condition|Observation|DiagnosticReport|DocumentReference|Media)", 1234 "Indicates another resource whose existence justifies this DeviceUseStatement.", 0, 1235 java.lang.Integer.MAX_VALUE, reasonReference); 1236 case 1702620169: 1237 /* bodySite */ return new Property("bodySite", "CodeableConcept", 1238 "Indicates the anotomic location on the subject's body where the device was used ( i.e. the target).", 0, 1, 1239 bodySite); 1240 case 3387378: 1241 /* note */ return new Property("note", "Annotation", 1242 "Details about the device statement that were not represented at all or sufficiently in one of the attributes provided in a class. These may include for example a comment, an instruction, or a note associated with the statement.", 1243 0, java.lang.Integer.MAX_VALUE, note); 1244 default: 1245 return super.getNamedProperty(_hash, _name, _checkValid); 1246 } 1247 1248 } 1249 1250 @Override 1251 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1252 switch (hash) { 1253 case -1618432855: 1254 /* identifier */ return this.identifier == null ? new Base[0] 1255 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1256 case -332612366: 1257 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1258 case -892481550: 1259 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DeviceUseStatementStatus> 1260 case -1867885268: 1261 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1262 case 1077922663: 1263 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 1264 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1265 case -873664438: 1266 /* timing */ return this.timing == null ? new Base[0] : new Base[] { this.timing }; // Type 1267 case 735397551: 1268 /* recordedOn */ return this.recordedOn == null ? new Base[0] : new Base[] { this.recordedOn }; // DateTimeType 1269 case -896505829: 1270 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 1271 case -1335157162: 1272 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 1273 case 722137681: 1274 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1275 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1276 case -1146218137: 1277 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1278 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1279 case 1702620169: 1280 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 1281 case 3387378: 1282 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1283 default: 1284 return super.getProperty(hash, name, checkValid); 1285 } 1286 1287 } 1288 1289 @Override 1290 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1291 switch (hash) { 1292 case -1618432855: // identifier 1293 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1294 return value; 1295 case -332612366: // basedOn 1296 this.getBasedOn().add(castToReference(value)); // Reference 1297 return value; 1298 case -892481550: // status 1299 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 1300 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 1301 return value; 1302 case -1867885268: // subject 1303 this.subject = castToReference(value); // Reference 1304 return value; 1305 case 1077922663: // derivedFrom 1306 this.getDerivedFrom().add(castToReference(value)); // Reference 1307 return value; 1308 case -873664438: // timing 1309 this.timing = castToType(value); // Type 1310 return value; 1311 case 735397551: // recordedOn 1312 this.recordedOn = castToDateTime(value); // DateTimeType 1313 return value; 1314 case -896505829: // source 1315 this.source = castToReference(value); // Reference 1316 return value; 1317 case -1335157162: // device 1318 this.device = castToReference(value); // Reference 1319 return value; 1320 case 722137681: // reasonCode 1321 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1322 return value; 1323 case -1146218137: // reasonReference 1324 this.getReasonReference().add(castToReference(value)); // Reference 1325 return value; 1326 case 1702620169: // bodySite 1327 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1328 return value; 1329 case 3387378: // note 1330 this.getNote().add(castToAnnotation(value)); // Annotation 1331 return value; 1332 default: 1333 return super.setProperty(hash, name, value); 1334 } 1335 1336 } 1337 1338 @Override 1339 public Base setProperty(String name, Base value) throws FHIRException { 1340 if (name.equals("identifier")) { 1341 this.getIdentifier().add(castToIdentifier(value)); 1342 } else if (name.equals("basedOn")) { 1343 this.getBasedOn().add(castToReference(value)); 1344 } else if (name.equals("status")) { 1345 value = new DeviceUseStatementStatusEnumFactory().fromType(castToCode(value)); 1346 this.status = (Enumeration) value; // Enumeration<DeviceUseStatementStatus> 1347 } else if (name.equals("subject")) { 1348 this.subject = castToReference(value); // Reference 1349 } else if (name.equals("derivedFrom")) { 1350 this.getDerivedFrom().add(castToReference(value)); 1351 } else if (name.equals("timing[x]")) { 1352 this.timing = castToType(value); // Type 1353 } else if (name.equals("recordedOn")) { 1354 this.recordedOn = castToDateTime(value); // DateTimeType 1355 } else if (name.equals("source")) { 1356 this.source = castToReference(value); // Reference 1357 } else if (name.equals("device")) { 1358 this.device = castToReference(value); // Reference 1359 } else if (name.equals("reasonCode")) { 1360 this.getReasonCode().add(castToCodeableConcept(value)); 1361 } else if (name.equals("reasonReference")) { 1362 this.getReasonReference().add(castToReference(value)); 1363 } else if (name.equals("bodySite")) { 1364 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1365 } else if (name.equals("note")) { 1366 this.getNote().add(castToAnnotation(value)); 1367 } else 1368 return super.setProperty(name, value); 1369 return value; 1370 } 1371 1372 @Override 1373 public void removeChild(String name, Base value) throws FHIRException { 1374 if (name.equals("identifier")) { 1375 this.getIdentifier().remove(castToIdentifier(value)); 1376 } else if (name.equals("basedOn")) { 1377 this.getBasedOn().remove(castToReference(value)); 1378 } else if (name.equals("status")) { 1379 this.status = null; 1380 } else if (name.equals("subject")) { 1381 this.subject = null; 1382 } else if (name.equals("derivedFrom")) { 1383 this.getDerivedFrom().remove(castToReference(value)); 1384 } else if (name.equals("timing[x]")) { 1385 this.timing = null; 1386 } else if (name.equals("recordedOn")) { 1387 this.recordedOn = null; 1388 } else if (name.equals("source")) { 1389 this.source = null; 1390 } else if (name.equals("device")) { 1391 this.device = null; 1392 } else if (name.equals("reasonCode")) { 1393 this.getReasonCode().remove(castToCodeableConcept(value)); 1394 } else if (name.equals("reasonReference")) { 1395 this.getReasonReference().remove(castToReference(value)); 1396 } else if (name.equals("bodySite")) { 1397 this.bodySite = null; 1398 } else if (name.equals("note")) { 1399 this.getNote().remove(castToAnnotation(value)); 1400 } else 1401 super.removeChild(name, value); 1402 1403 } 1404 1405 @Override 1406 public Base makeProperty(int hash, String name) throws FHIRException { 1407 switch (hash) { 1408 case -1618432855: 1409 return addIdentifier(); 1410 case -332612366: 1411 return addBasedOn(); 1412 case -892481550: 1413 return getStatusElement(); 1414 case -1867885268: 1415 return getSubject(); 1416 case 1077922663: 1417 return addDerivedFrom(); 1418 case 164632566: 1419 return getTiming(); 1420 case -873664438: 1421 return getTiming(); 1422 case 735397551: 1423 return getRecordedOnElement(); 1424 case -896505829: 1425 return getSource(); 1426 case -1335157162: 1427 return getDevice(); 1428 case 722137681: 1429 return addReasonCode(); 1430 case -1146218137: 1431 return addReasonReference(); 1432 case 1702620169: 1433 return getBodySite(); 1434 case 3387378: 1435 return addNote(); 1436 default: 1437 return super.makeProperty(hash, name); 1438 } 1439 1440 } 1441 1442 @Override 1443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1444 switch (hash) { 1445 case -1618432855: 1446 /* identifier */ return new String[] { "Identifier" }; 1447 case -332612366: 1448 /* basedOn */ return new String[] { "Reference" }; 1449 case -892481550: 1450 /* status */ return new String[] { "code" }; 1451 case -1867885268: 1452 /* subject */ return new String[] { "Reference" }; 1453 case 1077922663: 1454 /* derivedFrom */ return new String[] { "Reference" }; 1455 case -873664438: 1456 /* timing */ return new String[] { "Timing", "Period", "dateTime" }; 1457 case 735397551: 1458 /* recordedOn */ return new String[] { "dateTime" }; 1459 case -896505829: 1460 /* source */ return new String[] { "Reference" }; 1461 case -1335157162: 1462 /* device */ return new String[] { "Reference" }; 1463 case 722137681: 1464 /* reasonCode */ return new String[] { "CodeableConcept" }; 1465 case -1146218137: 1466 /* reasonReference */ return new String[] { "Reference" }; 1467 case 1702620169: 1468 /* bodySite */ return new String[] { "CodeableConcept" }; 1469 case 3387378: 1470 /* note */ return new String[] { "Annotation" }; 1471 default: 1472 return super.getTypesForProperty(hash, name); 1473 } 1474 1475 } 1476 1477 @Override 1478 public Base addChild(String name) throws FHIRException { 1479 if (name.equals("identifier")) { 1480 return addIdentifier(); 1481 } else if (name.equals("basedOn")) { 1482 return addBasedOn(); 1483 } else if (name.equals("status")) { 1484 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.status"); 1485 } else if (name.equals("subject")) { 1486 this.subject = new Reference(); 1487 return this.subject; 1488 } else if (name.equals("derivedFrom")) { 1489 return addDerivedFrom(); 1490 } else if (name.equals("timingTiming")) { 1491 this.timing = new Timing(); 1492 return this.timing; 1493 } else if (name.equals("timingPeriod")) { 1494 this.timing = new Period(); 1495 return this.timing; 1496 } else if (name.equals("timingDateTime")) { 1497 this.timing = new DateTimeType(); 1498 return this.timing; 1499 } else if (name.equals("recordedOn")) { 1500 throw new FHIRException("Cannot call addChild on a singleton property DeviceUseStatement.recordedOn"); 1501 } else if (name.equals("source")) { 1502 this.source = new Reference(); 1503 return this.source; 1504 } else if (name.equals("device")) { 1505 this.device = new Reference(); 1506 return this.device; 1507 } else if (name.equals("reasonCode")) { 1508 return addReasonCode(); 1509 } else if (name.equals("reasonReference")) { 1510 return addReasonReference(); 1511 } else if (name.equals("bodySite")) { 1512 this.bodySite = new CodeableConcept(); 1513 return this.bodySite; 1514 } else if (name.equals("note")) { 1515 return addNote(); 1516 } else 1517 return super.addChild(name); 1518 } 1519 1520 public String fhirType() { 1521 return "DeviceUseStatement"; 1522 1523 } 1524 1525 public DeviceUseStatement copy() { 1526 DeviceUseStatement dst = new DeviceUseStatement(); 1527 copyValues(dst); 1528 return dst; 1529 } 1530 1531 public void copyValues(DeviceUseStatement dst) { 1532 super.copyValues(dst); 1533 if (identifier != null) { 1534 dst.identifier = new ArrayList<Identifier>(); 1535 for (Identifier i : identifier) 1536 dst.identifier.add(i.copy()); 1537 } 1538 ; 1539 if (basedOn != null) { 1540 dst.basedOn = new ArrayList<Reference>(); 1541 for (Reference i : basedOn) 1542 dst.basedOn.add(i.copy()); 1543 } 1544 ; 1545 dst.status = status == null ? null : status.copy(); 1546 dst.subject = subject == null ? null : subject.copy(); 1547 if (derivedFrom != null) { 1548 dst.derivedFrom = new ArrayList<Reference>(); 1549 for (Reference i : derivedFrom) 1550 dst.derivedFrom.add(i.copy()); 1551 } 1552 ; 1553 dst.timing = timing == null ? null : timing.copy(); 1554 dst.recordedOn = recordedOn == null ? null : recordedOn.copy(); 1555 dst.source = source == null ? null : source.copy(); 1556 dst.device = device == null ? null : device.copy(); 1557 if (reasonCode != null) { 1558 dst.reasonCode = new ArrayList<CodeableConcept>(); 1559 for (CodeableConcept i : reasonCode) 1560 dst.reasonCode.add(i.copy()); 1561 } 1562 ; 1563 if (reasonReference != null) { 1564 dst.reasonReference = new ArrayList<Reference>(); 1565 for (Reference i : reasonReference) 1566 dst.reasonReference.add(i.copy()); 1567 } 1568 ; 1569 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1570 if (note != null) { 1571 dst.note = new ArrayList<Annotation>(); 1572 for (Annotation i : note) 1573 dst.note.add(i.copy()); 1574 } 1575 ; 1576 } 1577 1578 protected DeviceUseStatement typedCopy() { 1579 return copy(); 1580 } 1581 1582 @Override 1583 public boolean equalsDeep(Base other_) { 1584 if (!super.equalsDeep(other_)) 1585 return false; 1586 if (!(other_ instanceof DeviceUseStatement)) 1587 return false; 1588 DeviceUseStatement o = (DeviceUseStatement) other_; 1589 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 1590 && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1591 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(timing, o.timing, true) 1592 && compareDeep(recordedOn, o.recordedOn, true) && compareDeep(source, o.source, true) 1593 && compareDeep(device, o.device, true) && compareDeep(reasonCode, o.reasonCode, true) 1594 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(bodySite, o.bodySite, true) 1595 && compareDeep(note, o.note, true); 1596 } 1597 1598 @Override 1599 public boolean equalsShallow(Base other_) { 1600 if (!super.equalsShallow(other_)) 1601 return false; 1602 if (!(other_ instanceof DeviceUseStatement)) 1603 return false; 1604 DeviceUseStatement o = (DeviceUseStatement) other_; 1605 return compareValues(status, o.status, true) && compareValues(recordedOn, o.recordedOn, true); 1606 } 1607 1608 public boolean isEmpty() { 1609 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status, subject, derivedFrom, 1610 timing, recordedOn, source, device, reasonCode, reasonReference, bodySite, note); 1611 } 1612 1613 @Override 1614 public ResourceType getResourceType() { 1615 return ResourceType.DeviceUseStatement; 1616 } 1617 1618 /** 1619 * Search parameter: <b>identifier</b> 1620 * <p> 1621 * Description: <b>Search by identifier</b><br> 1622 * Type: <b>token</b><br> 1623 * Path: <b>DeviceUseStatement.identifier</b><br> 1624 * </p> 1625 */ 1626 @SearchParamDefinition(name = "identifier", path = "DeviceUseStatement.identifier", description = "Search by identifier", type = "token") 1627 public static final String SP_IDENTIFIER = "identifier"; 1628 /** 1629 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1630 * <p> 1631 * Description: <b>Search by identifier</b><br> 1632 * Type: <b>token</b><br> 1633 * Path: <b>DeviceUseStatement.identifier</b><br> 1634 * </p> 1635 */ 1636 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1637 SP_IDENTIFIER); 1638 1639 /** 1640 * Search parameter: <b>subject</b> 1641 * <p> 1642 * Description: <b>Search by subject</b><br> 1643 * Type: <b>reference</b><br> 1644 * Path: <b>DeviceUseStatement.subject</b><br> 1645 * </p> 1646 */ 1647 @SearchParamDefinition(name = "subject", path = "DeviceUseStatement.subject", description = "Search by subject", type = "reference", providesMembershipIn = { 1648 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 1649 public static final String SP_SUBJECT = "subject"; 1650 /** 1651 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1652 * <p> 1653 * Description: <b>Search by subject</b><br> 1654 * Type: <b>reference</b><br> 1655 * Path: <b>DeviceUseStatement.subject</b><br> 1656 * </p> 1657 */ 1658 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1659 SP_SUBJECT); 1660 1661 /** 1662 * Constant for fluent queries to be used to add include statements. Specifies 1663 * the path value of "<b>DeviceUseStatement:subject</b>". 1664 */ 1665 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1666 "DeviceUseStatement:subject").toLocked(); 1667 1668 /** 1669 * Search parameter: <b>patient</b> 1670 * <p> 1671 * Description: <b>Search by subject - a patient</b><br> 1672 * Type: <b>reference</b><br> 1673 * Path: <b>DeviceUseStatement.subject</b><br> 1674 * </p> 1675 */ 1676 @SearchParamDefinition(name = "patient", path = "DeviceUseStatement.subject", description = "Search by subject - a patient", type = "reference", target = { 1677 Group.class, Patient.class }) 1678 public static final String SP_PATIENT = "patient"; 1679 /** 1680 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1681 * <p> 1682 * Description: <b>Search by subject - a patient</b><br> 1683 * Type: <b>reference</b><br> 1684 * Path: <b>DeviceUseStatement.subject</b><br> 1685 * </p> 1686 */ 1687 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1688 SP_PATIENT); 1689 1690 /** 1691 * Constant for fluent queries to be used to add include statements. Specifies 1692 * the path value of "<b>DeviceUseStatement:patient</b>". 1693 */ 1694 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1695 "DeviceUseStatement:patient").toLocked(); 1696 1697 /** 1698 * Search parameter: <b>device</b> 1699 * <p> 1700 * Description: <b>Search by device</b><br> 1701 * Type: <b>reference</b><br> 1702 * Path: <b>DeviceUseStatement.device</b><br> 1703 * </p> 1704 */ 1705 @SearchParamDefinition(name = "device", path = "DeviceUseStatement.device", description = "Search by device", type = "reference", providesMembershipIn = { 1706 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 1707 public static final String SP_DEVICE = "device"; 1708 /** 1709 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1710 * <p> 1711 * Description: <b>Search by device</b><br> 1712 * Type: <b>reference</b><br> 1713 * Path: <b>DeviceUseStatement.device</b><br> 1714 * </p> 1715 */ 1716 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1717 SP_DEVICE); 1718 1719 /** 1720 * Constant for fluent queries to be used to add include statements. Specifies 1721 * the path value of "<b>DeviceUseStatement:device</b>". 1722 */ 1723 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 1724 "DeviceUseStatement:device").toLocked(); 1725 1726}