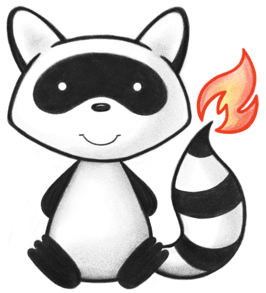
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus; 040import org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * A collection of documents compiled for a purpose together with metadata that 051 * applies to the collection. 052 */ 053@ResourceDef(name = "DocumentManifest", profile = "http://hl7.org/fhir/StructureDefinition/DocumentManifest") 054public class DocumentManifest extends DomainResource { 055 056 @Block() 057 public static class DocumentManifestRelatedComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Related identifier to this DocumentManifest. For example, Order numbers, 060 * accession numbers, XDW workflow numbers. 061 */ 062 @Child(name = "identifier", type = { 063 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 064 @Description(shortDefinition = "Identifiers of things that are related", formalDefinition = "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.") 065 protected Identifier identifier; 066 067 /** 068 * Related Resource to this DocumentManifest. For example, Order, 069 * ServiceRequest, Procedure, EligibilityRequest, etc. 070 */ 071 @Child(name = "ref", type = { Reference.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 072 @Description(shortDefinition = "Related Resource", formalDefinition = "Related Resource to this DocumentManifest. For example, Order, ServiceRequest, Procedure, EligibilityRequest, etc.") 073 protected Reference ref; 074 075 /** 076 * The actual object that is the target of the reference (Related Resource to 077 * this DocumentManifest. For example, Order, ServiceRequest, Procedure, 078 * EligibilityRequest, etc.) 079 */ 080 protected Resource refTarget; 081 082 private static final long serialVersionUID = -1670123330L; 083 084 /** 085 * Constructor 086 */ 087 public DocumentManifestRelatedComponent() { 088 super(); 089 } 090 091 /** 092 * @return {@link #identifier} (Related identifier to this DocumentManifest. For 093 * example, Order numbers, accession numbers, XDW workflow numbers.) 094 */ 095 public Identifier getIdentifier() { 096 if (this.identifier == null) 097 if (Configuration.errorOnAutoCreate()) 098 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.identifier"); 099 else if (Configuration.doAutoCreate()) 100 this.identifier = new Identifier(); // cc 101 return this.identifier; 102 } 103 104 public boolean hasIdentifier() { 105 return this.identifier != null && !this.identifier.isEmpty(); 106 } 107 108 /** 109 * @param value {@link #identifier} (Related identifier to this 110 * DocumentManifest. For example, Order numbers, accession numbers, 111 * XDW workflow numbers.) 112 */ 113 public DocumentManifestRelatedComponent setIdentifier(Identifier value) { 114 this.identifier = value; 115 return this; 116 } 117 118 /** 119 * @return {@link #ref} (Related Resource to this DocumentManifest. For example, 120 * Order, ServiceRequest, Procedure, EligibilityRequest, etc.) 121 */ 122 public Reference getRef() { 123 if (this.ref == null) 124 if (Configuration.errorOnAutoCreate()) 125 throw new Error("Attempt to auto-create DocumentManifestRelatedComponent.ref"); 126 else if (Configuration.doAutoCreate()) 127 this.ref = new Reference(); // cc 128 return this.ref; 129 } 130 131 public boolean hasRef() { 132 return this.ref != null && !this.ref.isEmpty(); 133 } 134 135 /** 136 * @param value {@link #ref} (Related Resource to this DocumentManifest. For 137 * example, Order, ServiceRequest, Procedure, EligibilityRequest, 138 * etc.) 139 */ 140 public DocumentManifestRelatedComponent setRef(Reference value) { 141 this.ref = value; 142 return this; 143 } 144 145 /** 146 * @return {@link #ref} The actual object that is the target of the reference. 147 * The reference library doesn't populate this, but you can use it to 148 * hold the resource if you resolve it. (Related Resource to this 149 * DocumentManifest. For example, Order, ServiceRequest, Procedure, 150 * EligibilityRequest, etc.) 151 */ 152 public Resource getRefTarget() { 153 return this.refTarget; 154 } 155 156 /** 157 * @param value {@link #ref} The actual object that is the target of the 158 * reference. The reference library doesn't use these, but you can 159 * use it to hold the resource if you resolve it. (Related Resource 160 * to this DocumentManifest. For example, Order, ServiceRequest, 161 * Procedure, EligibilityRequest, etc.) 162 */ 163 public DocumentManifestRelatedComponent setRefTarget(Resource value) { 164 this.refTarget = value; 165 return this; 166 } 167 168 protected void listChildren(List<Property> children) { 169 super.listChildren(children); 170 children.add(new Property("identifier", "Identifier", 171 "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.", 172 0, 1, identifier)); 173 children.add(new Property("ref", "Reference(Any)", 174 "Related Resource to this DocumentManifest. For example, Order, ServiceRequest, Procedure, EligibilityRequest, etc.", 175 0, 1, ref)); 176 } 177 178 @Override 179 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 180 switch (_hash) { 181 case -1618432855: 182 /* identifier */ return new Property("identifier", "Identifier", 183 "Related identifier to this DocumentManifest. For example, Order numbers, accession numbers, XDW workflow numbers.", 184 0, 1, identifier); 185 case 112787: 186 /* ref */ return new Property("ref", "Reference(Any)", 187 "Related Resource to this DocumentManifest. For example, Order, ServiceRequest, Procedure, EligibilityRequest, etc.", 188 0, 1, ref); 189 default: 190 return super.getNamedProperty(_hash, _name, _checkValid); 191 } 192 193 } 194 195 @Override 196 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 197 switch (hash) { 198 case -1618432855: 199 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 200 case 112787: 201 /* ref */ return this.ref == null ? new Base[0] : new Base[] { this.ref }; // Reference 202 default: 203 return super.getProperty(hash, name, checkValid); 204 } 205 206 } 207 208 @Override 209 public Base setProperty(int hash, String name, Base value) throws FHIRException { 210 switch (hash) { 211 case -1618432855: // identifier 212 this.identifier = castToIdentifier(value); // Identifier 213 return value; 214 case 112787: // ref 215 this.ref = castToReference(value); // Reference 216 return value; 217 default: 218 return super.setProperty(hash, name, value); 219 } 220 221 } 222 223 @Override 224 public Base setProperty(String name, Base value) throws FHIRException { 225 if (name.equals("identifier")) { 226 this.identifier = castToIdentifier(value); // Identifier 227 } else if (name.equals("ref")) { 228 this.ref = castToReference(value); // Reference 229 } else 230 return super.setProperty(name, value); 231 return value; 232 } 233 234 @Override 235 public void removeChild(String name, Base value) throws FHIRException { 236 if (name.equals("identifier")) { 237 this.identifier = null; 238 } else if (name.equals("ref")) { 239 this.ref = null; 240 } else 241 super.removeChild(name, value); 242 243 } 244 245 @Override 246 public Base makeProperty(int hash, String name) throws FHIRException { 247 switch (hash) { 248 case -1618432855: 249 return getIdentifier(); 250 case 112787: 251 return getRef(); 252 default: 253 return super.makeProperty(hash, name); 254 } 255 256 } 257 258 @Override 259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 260 switch (hash) { 261 case -1618432855: 262 /* identifier */ return new String[] { "Identifier" }; 263 case 112787: 264 /* ref */ return new String[] { "Reference" }; 265 default: 266 return super.getTypesForProperty(hash, name); 267 } 268 269 } 270 271 @Override 272 public Base addChild(String name) throws FHIRException { 273 if (name.equals("identifier")) { 274 this.identifier = new Identifier(); 275 return this.identifier; 276 } else if (name.equals("ref")) { 277 this.ref = new Reference(); 278 return this.ref; 279 } else 280 return super.addChild(name); 281 } 282 283 public DocumentManifestRelatedComponent copy() { 284 DocumentManifestRelatedComponent dst = new DocumentManifestRelatedComponent(); 285 copyValues(dst); 286 return dst; 287 } 288 289 public void copyValues(DocumentManifestRelatedComponent dst) { 290 super.copyValues(dst); 291 dst.identifier = identifier == null ? null : identifier.copy(); 292 dst.ref = ref == null ? null : ref.copy(); 293 } 294 295 @Override 296 public boolean equalsDeep(Base other_) { 297 if (!super.equalsDeep(other_)) 298 return false; 299 if (!(other_ instanceof DocumentManifestRelatedComponent)) 300 return false; 301 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other_; 302 return compareDeep(identifier, o.identifier, true) && compareDeep(ref, o.ref, true); 303 } 304 305 @Override 306 public boolean equalsShallow(Base other_) { 307 if (!super.equalsShallow(other_)) 308 return false; 309 if (!(other_ instanceof DocumentManifestRelatedComponent)) 310 return false; 311 DocumentManifestRelatedComponent o = (DocumentManifestRelatedComponent) other_; 312 return true; 313 } 314 315 public boolean isEmpty() { 316 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, ref); 317 } 318 319 public String fhirType() { 320 return "DocumentManifest.related"; 321 322 } 323 324 } 325 326 /** 327 * A single identifier that uniquely identifies this manifest. Principally used 328 * to refer to the manifest in non-FHIR contexts. 329 */ 330 @Child(name = "masterIdentifier", type = { 331 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 332 @Description(shortDefinition = "Unique Identifier for the set of documents", formalDefinition = "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.") 333 protected Identifier masterIdentifier; 334 335 /** 336 * Other identifiers associated with the document manifest, including version 337 * independent identifiers. 338 */ 339 @Child(name = "identifier", type = { 340 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 341 @Description(shortDefinition = "Other identifiers for the manifest", formalDefinition = "Other identifiers associated with the document manifest, including version independent identifiers.") 342 protected List<Identifier> identifier; 343 344 /** 345 * The status of this document manifest. 346 */ 347 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 348 @Description(shortDefinition = "current | superseded | entered-in-error", formalDefinition = "The status of this document manifest.") 349 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/document-reference-status") 350 protected Enumeration<DocumentReferenceStatus> status; 351 352 /** 353 * The code specifying the type of clinical activity that resulted in placing 354 * the associated content into the DocumentManifest. 355 */ 356 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 357 @Description(shortDefinition = "Kind of document set", formalDefinition = "The code specifying the type of clinical activity that resulted in placing the associated content into the DocumentManifest.") 358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActCode") 359 protected CodeableConcept type; 360 361 /** 362 * Who or what the set of documents is about. The documents can be about a 363 * person, (patient or healthcare practitioner), a device (i.e. machine) or even 364 * a group of subjects (such as a document about a herd of farm animals, or a 365 * set of patients that share a common exposure). If the documents cross more 366 * than one subject, then more than one subject is allowed here (unusual use 367 * case). 368 */ 369 @Child(name = "subject", type = { Patient.class, Practitioner.class, Group.class, 370 Device.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 371 @Description(shortDefinition = "The subject of the set of documents", formalDefinition = "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).") 372 protected Reference subject; 373 374 /** 375 * The actual object that is the target of the reference (Who or what the set of 376 * documents is about. The documents can be about a person, (patient or 377 * healthcare practitioner), a device (i.e. machine) or even a group of subjects 378 * (such as a document about a herd of farm animals, or a set of patients that 379 * share a common exposure). If the documents cross more than one subject, then 380 * more than one subject is allowed here (unusual use case).) 381 */ 382 protected Resource subjectTarget; 383 384 /** 385 * When the document manifest was created for submission to the server (not 386 * necessarily the same thing as the actual resource last modified time, since 387 * it may be modified, replicated, etc.). 388 */ 389 @Child(name = "created", type = { 390 DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 391 @Description(shortDefinition = "When this document manifest created", formalDefinition = "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).") 392 protected DateTimeType created; 393 394 /** 395 * Identifies who is the author of the manifest. Manifest author is not 396 * necessarly the author of the references included. 397 */ 398 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Organization.class, Device.class, 399 Patient.class, 400 RelatedPerson.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 401 @Description(shortDefinition = "Who and/or what authored the DocumentManifest", formalDefinition = "Identifies who is the author of the manifest. Manifest author is not necessarly the author of the references included.") 402 protected List<Reference> author; 403 /** 404 * The actual objects that are the target of the reference (Identifies who is 405 * the author of the manifest. Manifest author is not necessarly the author of 406 * the references included.) 407 */ 408 protected List<Resource> authorTarget; 409 410 /** 411 * A patient, practitioner, or organization for which this set of documents is 412 * intended. 413 */ 414 @Child(name = "recipient", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 415 Organization.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 416 @Description(shortDefinition = "Intended to get notified about this set of documents", formalDefinition = "A patient, practitioner, or organization for which this set of documents is intended.") 417 protected List<Reference> recipient; 418 /** 419 * The actual objects that are the target of the reference (A patient, 420 * practitioner, or organization for which this set of documents is intended.) 421 */ 422 protected List<Resource> recipientTarget; 423 424 /** 425 * Identifies the source system, application, or software that produced the 426 * document manifest. 427 */ 428 @Child(name = "source", type = { UriType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 429 @Description(shortDefinition = "The source system/application/software", formalDefinition = "Identifies the source system, application, or software that produced the document manifest.") 430 protected UriType source; 431 432 /** 433 * Human-readable description of the source document. This is sometimes known as 434 * the "title". 435 */ 436 @Child(name = "description", type = { 437 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 438 @Description(shortDefinition = "Human-readable description (title)", formalDefinition = "Human-readable description of the source document. This is sometimes known as the \"title\".") 439 protected StringType description; 440 441 /** 442 * The list of Resources that consist of the parts of this manifest. 443 */ 444 @Child(name = "content", type = { 445 Reference.class }, order = 10, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 446 @Description(shortDefinition = "Items in manifest", formalDefinition = "The list of Resources that consist of the parts of this manifest.") 447 protected List<Reference> content; 448 /** 449 * The actual objects that are the target of the reference (The list of 450 * Resources that consist of the parts of this manifest.) 451 */ 452 protected List<Resource> contentTarget; 453 454 /** 455 * Related identifiers or resources associated with the DocumentManifest. 456 */ 457 @Child(name = "related", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 458 @Description(shortDefinition = "Related things", formalDefinition = "Related identifiers or resources associated with the DocumentManifest.") 459 protected List<DocumentManifestRelatedComponent> related; 460 461 private static final long serialVersionUID = 432971934L; 462 463 /** 464 * Constructor 465 */ 466 public DocumentManifest() { 467 super(); 468 } 469 470 /** 471 * Constructor 472 */ 473 public DocumentManifest(Enumeration<DocumentReferenceStatus> status) { 474 super(); 475 this.status = status; 476 } 477 478 /** 479 * @return {@link #masterIdentifier} (A single identifier that uniquely 480 * identifies this manifest. Principally used to refer to the manifest 481 * in non-FHIR contexts.) 482 */ 483 public Identifier getMasterIdentifier() { 484 if (this.masterIdentifier == null) 485 if (Configuration.errorOnAutoCreate()) 486 throw new Error("Attempt to auto-create DocumentManifest.masterIdentifier"); 487 else if (Configuration.doAutoCreate()) 488 this.masterIdentifier = new Identifier(); // cc 489 return this.masterIdentifier; 490 } 491 492 public boolean hasMasterIdentifier() { 493 return this.masterIdentifier != null && !this.masterIdentifier.isEmpty(); 494 } 495 496 /** 497 * @param value {@link #masterIdentifier} (A single identifier that uniquely 498 * identifies this manifest. Principally used to refer to the 499 * manifest in non-FHIR contexts.) 500 */ 501 public DocumentManifest setMasterIdentifier(Identifier value) { 502 this.masterIdentifier = value; 503 return this; 504 } 505 506 /** 507 * @return {@link #identifier} (Other identifiers associated with the document 508 * manifest, including version independent identifiers.) 509 */ 510 public List<Identifier> getIdentifier() { 511 if (this.identifier == null) 512 this.identifier = new ArrayList<Identifier>(); 513 return this.identifier; 514 } 515 516 /** 517 * @return Returns a reference to <code>this</code> for easy method chaining 518 */ 519 public DocumentManifest setIdentifier(List<Identifier> theIdentifier) { 520 this.identifier = theIdentifier; 521 return this; 522 } 523 524 public boolean hasIdentifier() { 525 if (this.identifier == null) 526 return false; 527 for (Identifier item : this.identifier) 528 if (!item.isEmpty()) 529 return true; 530 return false; 531 } 532 533 public Identifier addIdentifier() { // 3 534 Identifier t = new Identifier(); 535 if (this.identifier == null) 536 this.identifier = new ArrayList<Identifier>(); 537 this.identifier.add(t); 538 return t; 539 } 540 541 public DocumentManifest addIdentifier(Identifier t) { // 3 542 if (t == null) 543 return this; 544 if (this.identifier == null) 545 this.identifier = new ArrayList<Identifier>(); 546 this.identifier.add(t); 547 return this; 548 } 549 550 /** 551 * @return The first repetition of repeating field {@link #identifier}, creating 552 * it if it does not already exist 553 */ 554 public Identifier getIdentifierFirstRep() { 555 if (getIdentifier().isEmpty()) { 556 addIdentifier(); 557 } 558 return getIdentifier().get(0); 559 } 560 561 /** 562 * @return {@link #status} (The status of this document manifest.). This is the 563 * underlying object with id, value and extensions. The accessor 564 * "getStatus" gives direct access to the value 565 */ 566 public Enumeration<DocumentReferenceStatus> getStatusElement() { 567 if (this.status == null) 568 if (Configuration.errorOnAutoCreate()) 569 throw new Error("Attempt to auto-create DocumentManifest.status"); 570 else if (Configuration.doAutoCreate()) 571 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); // bb 572 return this.status; 573 } 574 575 public boolean hasStatusElement() { 576 return this.status != null && !this.status.isEmpty(); 577 } 578 579 public boolean hasStatus() { 580 return this.status != null && !this.status.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #status} (The status of this document manifest.). This is 585 * the underlying object with id, value and extensions. The 586 * accessor "getStatus" gives direct access to the value 587 */ 588 public DocumentManifest setStatusElement(Enumeration<DocumentReferenceStatus> value) { 589 this.status = value; 590 return this; 591 } 592 593 /** 594 * @return The status of this document manifest. 595 */ 596 public DocumentReferenceStatus getStatus() { 597 return this.status == null ? null : this.status.getValue(); 598 } 599 600 /** 601 * @param value The status of this document manifest. 602 */ 603 public DocumentManifest setStatus(DocumentReferenceStatus value) { 604 if (this.status == null) 605 this.status = new Enumeration<DocumentReferenceStatus>(new DocumentReferenceStatusEnumFactory()); 606 this.status.setValue(value); 607 return this; 608 } 609 610 /** 611 * @return {@link #type} (The code specifying the type of clinical activity that 612 * resulted in placing the associated content into the 613 * DocumentManifest.) 614 */ 615 public CodeableConcept getType() { 616 if (this.type == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create DocumentManifest.type"); 619 else if (Configuration.doAutoCreate()) 620 this.type = new CodeableConcept(); // cc 621 return this.type; 622 } 623 624 public boolean hasType() { 625 return this.type != null && !this.type.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #type} (The code specifying the type of clinical activity 630 * that resulted in placing the associated content into the 631 * DocumentManifest.) 632 */ 633 public DocumentManifest setType(CodeableConcept value) { 634 this.type = value; 635 return this; 636 } 637 638 /** 639 * @return {@link #subject} (Who or what the set of documents is about. The 640 * documents can be about a person, (patient or healthcare 641 * practitioner), a device (i.e. machine) or even a group of subjects 642 * (such as a document about a herd of farm animals, or a set of 643 * patients that share a common exposure). If the documents cross more 644 * than one subject, then more than one subject is allowed here (unusual 645 * use case).) 646 */ 647 public Reference getSubject() { 648 if (this.subject == null) 649 if (Configuration.errorOnAutoCreate()) 650 throw new Error("Attempt to auto-create DocumentManifest.subject"); 651 else if (Configuration.doAutoCreate()) 652 this.subject = new Reference(); // cc 653 return this.subject; 654 } 655 656 public boolean hasSubject() { 657 return this.subject != null && !this.subject.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #subject} (Who or what the set of documents is about. The 662 * documents can be about a person, (patient or healthcare 663 * practitioner), a device (i.e. machine) or even a group of 664 * subjects (such as a document about a herd of farm animals, or a 665 * set of patients that share a common exposure). If the documents 666 * cross more than one subject, then more than one subject is 667 * allowed here (unusual use case).) 668 */ 669 public DocumentManifest setSubject(Reference value) { 670 this.subject = value; 671 return this; 672 } 673 674 /** 675 * @return {@link #subject} The actual object that is the target of the 676 * reference. The reference library doesn't populate this, but you can 677 * use it to hold the resource if you resolve it. (Who or what the set 678 * of documents is about. The documents can be about a person, (patient 679 * or healthcare practitioner), a device (i.e. machine) or even a group 680 * of subjects (such as a document about a herd of farm animals, or a 681 * set of patients that share a common exposure). If the documents cross 682 * more than one subject, then more than one subject is allowed here 683 * (unusual use case).) 684 */ 685 public Resource getSubjectTarget() { 686 return this.subjectTarget; 687 } 688 689 /** 690 * @param value {@link #subject} The actual object that is the target of the 691 * reference. The reference library doesn't use these, but you can 692 * use it to hold the resource if you resolve it. (Who or what the 693 * set of documents is about. The documents can be about a person, 694 * (patient or healthcare practitioner), a device (i.e. machine) or 695 * even a group of subjects (such as a document about a herd of 696 * farm animals, or a set of patients that share a common 697 * exposure). If the documents cross more than one subject, then 698 * more than one subject is allowed here (unusual use case).) 699 */ 700 public DocumentManifest setSubjectTarget(Resource value) { 701 this.subjectTarget = value; 702 return this; 703 } 704 705 /** 706 * @return {@link #created} (When the document manifest was created for 707 * submission to the server (not necessarily the same thing as the 708 * actual resource last modified time, since it may be modified, 709 * replicated, etc.).). This is the underlying object with id, value and 710 * extensions. The accessor "getCreated" gives direct access to the 711 * value 712 */ 713 public DateTimeType getCreatedElement() { 714 if (this.created == null) 715 if (Configuration.errorOnAutoCreate()) 716 throw new Error("Attempt to auto-create DocumentManifest.created"); 717 else if (Configuration.doAutoCreate()) 718 this.created = new DateTimeType(); // bb 719 return this.created; 720 } 721 722 public boolean hasCreatedElement() { 723 return this.created != null && !this.created.isEmpty(); 724 } 725 726 public boolean hasCreated() { 727 return this.created != null && !this.created.isEmpty(); 728 } 729 730 /** 731 * @param value {@link #created} (When the document manifest was created for 732 * submission to the server (not necessarily the same thing as the 733 * actual resource last modified time, since it may be modified, 734 * replicated, etc.).). This is the underlying object with id, 735 * value and extensions. The accessor "getCreated" gives direct 736 * access to the value 737 */ 738 public DocumentManifest setCreatedElement(DateTimeType value) { 739 this.created = value; 740 return this; 741 } 742 743 /** 744 * @return When the document manifest was created for submission to the server 745 * (not necessarily the same thing as the actual resource last modified 746 * time, since it may be modified, replicated, etc.). 747 */ 748 public Date getCreated() { 749 return this.created == null ? null : this.created.getValue(); 750 } 751 752 /** 753 * @param value When the document manifest was created for submission to the 754 * server (not necessarily the same thing as the actual resource 755 * last modified time, since it may be modified, replicated, etc.). 756 */ 757 public DocumentManifest setCreated(Date value) { 758 if (value == null) 759 this.created = null; 760 else { 761 if (this.created == null) 762 this.created = new DateTimeType(); 763 this.created.setValue(value); 764 } 765 return this; 766 } 767 768 /** 769 * @return {@link #author} (Identifies who is the author of the manifest. 770 * Manifest author is not necessarly the author of the references 771 * included.) 772 */ 773 public List<Reference> getAuthor() { 774 if (this.author == null) 775 this.author = new ArrayList<Reference>(); 776 return this.author; 777 } 778 779 /** 780 * @return Returns a reference to <code>this</code> for easy method chaining 781 */ 782 public DocumentManifest setAuthor(List<Reference> theAuthor) { 783 this.author = theAuthor; 784 return this; 785 } 786 787 public boolean hasAuthor() { 788 if (this.author == null) 789 return false; 790 for (Reference item : this.author) 791 if (!item.isEmpty()) 792 return true; 793 return false; 794 } 795 796 public Reference addAuthor() { // 3 797 Reference t = new Reference(); 798 if (this.author == null) 799 this.author = new ArrayList<Reference>(); 800 this.author.add(t); 801 return t; 802 } 803 804 public DocumentManifest addAuthor(Reference t) { // 3 805 if (t == null) 806 return this; 807 if (this.author == null) 808 this.author = new ArrayList<Reference>(); 809 this.author.add(t); 810 return this; 811 } 812 813 /** 814 * @return The first repetition of repeating field {@link #author}, creating it 815 * if it does not already exist 816 */ 817 public Reference getAuthorFirstRep() { 818 if (getAuthor().isEmpty()) { 819 addAuthor(); 820 } 821 return getAuthor().get(0); 822 } 823 824 /** 825 * @return {@link #recipient} (A patient, practitioner, or organization for 826 * which this set of documents is intended.) 827 */ 828 public List<Reference> getRecipient() { 829 if (this.recipient == null) 830 this.recipient = new ArrayList<Reference>(); 831 return this.recipient; 832 } 833 834 /** 835 * @return Returns a reference to <code>this</code> for easy method chaining 836 */ 837 public DocumentManifest setRecipient(List<Reference> theRecipient) { 838 this.recipient = theRecipient; 839 return this; 840 } 841 842 public boolean hasRecipient() { 843 if (this.recipient == null) 844 return false; 845 for (Reference item : this.recipient) 846 if (!item.isEmpty()) 847 return true; 848 return false; 849 } 850 851 public Reference addRecipient() { // 3 852 Reference t = new Reference(); 853 if (this.recipient == null) 854 this.recipient = new ArrayList<Reference>(); 855 this.recipient.add(t); 856 return t; 857 } 858 859 public DocumentManifest addRecipient(Reference t) { // 3 860 if (t == null) 861 return this; 862 if (this.recipient == null) 863 this.recipient = new ArrayList<Reference>(); 864 this.recipient.add(t); 865 return this; 866 } 867 868 /** 869 * @return The first repetition of repeating field {@link #recipient}, creating 870 * it if it does not already exist 871 */ 872 public Reference getRecipientFirstRep() { 873 if (getRecipient().isEmpty()) { 874 addRecipient(); 875 } 876 return getRecipient().get(0); 877 } 878 879 /** 880 * @return {@link #source} (Identifies the source system, application, or 881 * software that produced the document manifest.). This is the 882 * underlying object with id, value and extensions. The accessor 883 * "getSource" gives direct access to the value 884 */ 885 public UriType getSourceElement() { 886 if (this.source == null) 887 if (Configuration.errorOnAutoCreate()) 888 throw new Error("Attempt to auto-create DocumentManifest.source"); 889 else if (Configuration.doAutoCreate()) 890 this.source = new UriType(); // bb 891 return this.source; 892 } 893 894 public boolean hasSourceElement() { 895 return this.source != null && !this.source.isEmpty(); 896 } 897 898 public boolean hasSource() { 899 return this.source != null && !this.source.isEmpty(); 900 } 901 902 /** 903 * @param value {@link #source} (Identifies the source system, application, or 904 * software that produced the document manifest.). This is the 905 * underlying object with id, value and extensions. The accessor 906 * "getSource" gives direct access to the value 907 */ 908 public DocumentManifest setSourceElement(UriType value) { 909 this.source = value; 910 return this; 911 } 912 913 /** 914 * @return Identifies the source system, application, or software that produced 915 * the document manifest. 916 */ 917 public String getSource() { 918 return this.source == null ? null : this.source.getValue(); 919 } 920 921 /** 922 * @param value Identifies the source system, application, or software that 923 * produced the document manifest. 924 */ 925 public DocumentManifest setSource(String value) { 926 if (Utilities.noString(value)) 927 this.source = null; 928 else { 929 if (this.source == null) 930 this.source = new UriType(); 931 this.source.setValue(value); 932 } 933 return this; 934 } 935 936 /** 937 * @return {@link #description} (Human-readable description of the source 938 * document. This is sometimes known as the "title".). This is the 939 * underlying object with id, value and extensions. The accessor 940 * "getDescription" gives direct access to the value 941 */ 942 public StringType getDescriptionElement() { 943 if (this.description == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create DocumentManifest.description"); 946 else if (Configuration.doAutoCreate()) 947 this.description = new StringType(); // bb 948 return this.description; 949 } 950 951 public boolean hasDescriptionElement() { 952 return this.description != null && !this.description.isEmpty(); 953 } 954 955 public boolean hasDescription() { 956 return this.description != null && !this.description.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #description} (Human-readable description of the source 961 * document. This is sometimes known as the "title".). This is the 962 * underlying object with id, value and extensions. The accessor 963 * "getDescription" gives direct access to the value 964 */ 965 public DocumentManifest setDescriptionElement(StringType value) { 966 this.description = value; 967 return this; 968 } 969 970 /** 971 * @return Human-readable description of the source document. This is sometimes 972 * known as the "title". 973 */ 974 public String getDescription() { 975 return this.description == null ? null : this.description.getValue(); 976 } 977 978 /** 979 * @param value Human-readable description of the source document. This is 980 * sometimes known as the "title". 981 */ 982 public DocumentManifest setDescription(String value) { 983 if (Utilities.noString(value)) 984 this.description = null; 985 else { 986 if (this.description == null) 987 this.description = new StringType(); 988 this.description.setValue(value); 989 } 990 return this; 991 } 992 993 /** 994 * @return {@link #content} (The list of Resources that consist of the parts of 995 * this manifest.) 996 */ 997 public List<Reference> getContent() { 998 if (this.content == null) 999 this.content = new ArrayList<Reference>(); 1000 return this.content; 1001 } 1002 1003 /** 1004 * @return Returns a reference to <code>this</code> for easy method chaining 1005 */ 1006 public DocumentManifest setContent(List<Reference> theContent) { 1007 this.content = theContent; 1008 return this; 1009 } 1010 1011 public boolean hasContent() { 1012 if (this.content == null) 1013 return false; 1014 for (Reference item : this.content) 1015 if (!item.isEmpty()) 1016 return true; 1017 return false; 1018 } 1019 1020 public Reference addContent() { // 3 1021 Reference t = new Reference(); 1022 if (this.content == null) 1023 this.content = new ArrayList<Reference>(); 1024 this.content.add(t); 1025 return t; 1026 } 1027 1028 public DocumentManifest addContent(Reference t) { // 3 1029 if (t == null) 1030 return this; 1031 if (this.content == null) 1032 this.content = new ArrayList<Reference>(); 1033 this.content.add(t); 1034 return this; 1035 } 1036 1037 /** 1038 * @return The first repetition of repeating field {@link #content}, creating it 1039 * if it does not already exist 1040 */ 1041 public Reference getContentFirstRep() { 1042 if (getContent().isEmpty()) { 1043 addContent(); 1044 } 1045 return getContent().get(0); 1046 } 1047 1048 /** 1049 * @return {@link #related} (Related identifiers or resources associated with 1050 * the DocumentManifest.) 1051 */ 1052 public List<DocumentManifestRelatedComponent> getRelated() { 1053 if (this.related == null) 1054 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1055 return this.related; 1056 } 1057 1058 /** 1059 * @return Returns a reference to <code>this</code> for easy method chaining 1060 */ 1061 public DocumentManifest setRelated(List<DocumentManifestRelatedComponent> theRelated) { 1062 this.related = theRelated; 1063 return this; 1064 } 1065 1066 public boolean hasRelated() { 1067 if (this.related == null) 1068 return false; 1069 for (DocumentManifestRelatedComponent item : this.related) 1070 if (!item.isEmpty()) 1071 return true; 1072 return false; 1073 } 1074 1075 public DocumentManifestRelatedComponent addRelated() { // 3 1076 DocumentManifestRelatedComponent t = new DocumentManifestRelatedComponent(); 1077 if (this.related == null) 1078 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1079 this.related.add(t); 1080 return t; 1081 } 1082 1083 public DocumentManifest addRelated(DocumentManifestRelatedComponent t) { // 3 1084 if (t == null) 1085 return this; 1086 if (this.related == null) 1087 this.related = new ArrayList<DocumentManifestRelatedComponent>(); 1088 this.related.add(t); 1089 return this; 1090 } 1091 1092 /** 1093 * @return The first repetition of repeating field {@link #related}, creating it 1094 * if it does not already exist 1095 */ 1096 public DocumentManifestRelatedComponent getRelatedFirstRep() { 1097 if (getRelated().isEmpty()) { 1098 addRelated(); 1099 } 1100 return getRelated().get(0); 1101 } 1102 1103 protected void listChildren(List<Property> children) { 1104 super.listChildren(children); 1105 children.add(new Property("masterIdentifier", "Identifier", 1106 "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.", 1107 0, 1, masterIdentifier)); 1108 children.add(new Property("identifier", "Identifier", 1109 "Other identifiers associated with the document manifest, including version independent identifiers.", 0, 1110 java.lang.Integer.MAX_VALUE, identifier)); 1111 children.add(new Property("status", "code", "The status of this document manifest.", 0, 1, status)); 1112 children.add(new Property("type", "CodeableConcept", 1113 "The code specifying the type of clinical activity that resulted in placing the associated content into the DocumentManifest.", 1114 0, 1, type)); 1115 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 1116 "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).", 1117 0, 1, subject)); 1118 children.add(new Property("created", "dateTime", 1119 "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).", 1120 0, 1, created)); 1121 children.add(new Property("author", 1122 "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 1123 "Identifies who is the author of the manifest. Manifest author is not necessarly the author of the references included.", 1124 0, java.lang.Integer.MAX_VALUE, author)); 1125 children 1126 .add(new Property("recipient", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 1127 "A patient, practitioner, or organization for which this set of documents is intended.", 0, 1128 java.lang.Integer.MAX_VALUE, recipient)); 1129 children.add(new Property("source", "uri", 1130 "Identifies the source system, application, or software that produced the document manifest.", 0, 1, source)); 1131 children.add(new Property("description", "string", 1132 "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, 1133 description)); 1134 children.add(new Property("content", "Reference(Any)", 1135 "The list of Resources that consist of the parts of this manifest.", 0, java.lang.Integer.MAX_VALUE, content)); 1136 children.add(new Property("related", "", "Related identifiers or resources associated with the DocumentManifest.", 1137 0, java.lang.Integer.MAX_VALUE, related)); 1138 } 1139 1140 @Override 1141 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1142 switch (_hash) { 1143 case 243769515: 1144 /* masterIdentifier */ return new Property("masterIdentifier", "Identifier", 1145 "A single identifier that uniquely identifies this manifest. Principally used to refer to the manifest in non-FHIR contexts.", 1146 0, 1, masterIdentifier); 1147 case -1618432855: 1148 /* identifier */ return new Property("identifier", "Identifier", 1149 "Other identifiers associated with the document manifest, including version independent identifiers.", 0, 1150 java.lang.Integer.MAX_VALUE, identifier); 1151 case -892481550: 1152 /* status */ return new Property("status", "code", "The status of this document manifest.", 0, 1, status); 1153 case 3575610: 1154 /* type */ return new Property("type", "CodeableConcept", 1155 "The code specifying the type of clinical activity that resulted in placing the associated content into the DocumentManifest.", 1156 0, 1, type); 1157 case -1867885268: 1158 /* subject */ return new Property("subject", "Reference(Patient|Practitioner|Group|Device)", 1159 "Who or what the set of documents is about. The documents can be about a person, (patient or healthcare practitioner), a device (i.e. machine) or even a group of subjects (such as a document about a herd of farm animals, or a set of patients that share a common exposure). If the documents cross more than one subject, then more than one subject is allowed here (unusual use case).", 1160 0, 1, subject); 1161 case 1028554472: 1162 /* created */ return new Property("created", "dateTime", 1163 "When the document manifest was created for submission to the server (not necessarily the same thing as the actual resource last modified time, since it may be modified, replicated, etc.).", 1164 0, 1, created); 1165 case -1406328437: 1166 /* author */ return new Property("author", 1167 "Reference(Practitioner|PractitionerRole|Organization|Device|Patient|RelatedPerson)", 1168 "Identifies who is the author of the manifest. Manifest author is not necessarly the author of the references included.", 1169 0, java.lang.Integer.MAX_VALUE, author); 1170 case 820081177: 1171 /* recipient */ return new Property("recipient", 1172 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 1173 "A patient, practitioner, or organization for which this set of documents is intended.", 0, 1174 java.lang.Integer.MAX_VALUE, recipient); 1175 case -896505829: 1176 /* source */ return new Property("source", "uri", 1177 "Identifies the source system, application, or software that produced the document manifest.", 0, 1, source); 1178 case -1724546052: 1179 /* description */ return new Property("description", "string", 1180 "Human-readable description of the source document. This is sometimes known as the \"title\".", 0, 1, 1181 description); 1182 case 951530617: 1183 /* content */ return new Property("content", "Reference(Any)", 1184 "The list of Resources that consist of the parts of this manifest.", 0, java.lang.Integer.MAX_VALUE, content); 1185 case 1090493483: 1186 /* related */ return new Property("related", "", 1187 "Related identifiers or resources associated with the DocumentManifest.", 0, java.lang.Integer.MAX_VALUE, 1188 related); 1189 default: 1190 return super.getNamedProperty(_hash, _name, _checkValid); 1191 } 1192 1193 } 1194 1195 @Override 1196 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1197 switch (hash) { 1198 case 243769515: 1199 /* masterIdentifier */ return this.masterIdentifier == null ? new Base[0] : new Base[] { this.masterIdentifier }; // Identifier 1200 case -1618432855: 1201 /* identifier */ return this.identifier == null ? new Base[0] 1202 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1203 case -892481550: 1204 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<DocumentReferenceStatus> 1205 case 3575610: 1206 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1207 case -1867885268: 1208 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1209 case 1028554472: 1210 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 1211 case -1406328437: 1212 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // Reference 1213 case 820081177: 1214 /* recipient */ return this.recipient == null ? new Base[0] 1215 : this.recipient.toArray(new Base[this.recipient.size()]); // Reference 1216 case -896505829: 1217 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // UriType 1218 case -1724546052: 1219 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1220 case 951530617: 1221 /* content */ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // Reference 1222 case 1090493483: 1223 /* related */ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // DocumentManifestRelatedComponent 1224 default: 1225 return super.getProperty(hash, name, checkValid); 1226 } 1227 1228 } 1229 1230 @Override 1231 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1232 switch (hash) { 1233 case 243769515: // masterIdentifier 1234 this.masterIdentifier = castToIdentifier(value); // Identifier 1235 return value; 1236 case -1618432855: // identifier 1237 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1238 return value; 1239 case -892481550: // status 1240 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 1241 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 1242 return value; 1243 case 3575610: // type 1244 this.type = castToCodeableConcept(value); // CodeableConcept 1245 return value; 1246 case -1867885268: // subject 1247 this.subject = castToReference(value); // Reference 1248 return value; 1249 case 1028554472: // created 1250 this.created = castToDateTime(value); // DateTimeType 1251 return value; 1252 case -1406328437: // author 1253 this.getAuthor().add(castToReference(value)); // Reference 1254 return value; 1255 case 820081177: // recipient 1256 this.getRecipient().add(castToReference(value)); // Reference 1257 return value; 1258 case -896505829: // source 1259 this.source = castToUri(value); // UriType 1260 return value; 1261 case -1724546052: // description 1262 this.description = castToString(value); // StringType 1263 return value; 1264 case 951530617: // content 1265 this.getContent().add(castToReference(value)); // Reference 1266 return value; 1267 case 1090493483: // related 1268 this.getRelated().add((DocumentManifestRelatedComponent) value); // DocumentManifestRelatedComponent 1269 return value; 1270 default: 1271 return super.setProperty(hash, name, value); 1272 } 1273 1274 } 1275 1276 @Override 1277 public Base setProperty(String name, Base value) throws FHIRException { 1278 if (name.equals("masterIdentifier")) { 1279 this.masterIdentifier = castToIdentifier(value); // Identifier 1280 } else if (name.equals("identifier")) { 1281 this.getIdentifier().add(castToIdentifier(value)); 1282 } else if (name.equals("status")) { 1283 value = new DocumentReferenceStatusEnumFactory().fromType(castToCode(value)); 1284 this.status = (Enumeration) value; // Enumeration<DocumentReferenceStatus> 1285 } else if (name.equals("type")) { 1286 this.type = castToCodeableConcept(value); // CodeableConcept 1287 } else if (name.equals("subject")) { 1288 this.subject = castToReference(value); // Reference 1289 } else if (name.equals("created")) { 1290 this.created = castToDateTime(value); // DateTimeType 1291 } else if (name.equals("author")) { 1292 this.getAuthor().add(castToReference(value)); 1293 } else if (name.equals("recipient")) { 1294 this.getRecipient().add(castToReference(value)); 1295 } else if (name.equals("source")) { 1296 this.source = castToUri(value); // UriType 1297 } else if (name.equals("description")) { 1298 this.description = castToString(value); // StringType 1299 } else if (name.equals("content")) { 1300 this.getContent().add(castToReference(value)); 1301 } else if (name.equals("related")) { 1302 this.getRelated().add((DocumentManifestRelatedComponent) value); 1303 } else 1304 return super.setProperty(name, value); 1305 return value; 1306 } 1307 1308 @Override 1309 public void removeChild(String name, Base value) throws FHIRException { 1310 if (name.equals("masterIdentifier")) { 1311 this.masterIdentifier = null; 1312 } else if (name.equals("identifier")) { 1313 this.getIdentifier().remove(castToIdentifier(value)); 1314 } else if (name.equals("status")) { 1315 this.status = null; 1316 } else if (name.equals("type")) { 1317 this.type = null; 1318 } else if (name.equals("subject")) { 1319 this.subject = null; 1320 } else if (name.equals("created")) { 1321 this.created = null; 1322 } else if (name.equals("author")) { 1323 this.getAuthor().remove(castToReference(value)); 1324 } else if (name.equals("recipient")) { 1325 this.getRecipient().remove(castToReference(value)); 1326 } else if (name.equals("source")) { 1327 this.source = null; 1328 } else if (name.equals("description")) { 1329 this.description = null; 1330 } else if (name.equals("content")) { 1331 this.getContent().remove(castToReference(value)); 1332 } else if (name.equals("related")) { 1333 this.getRelated().remove((DocumentManifestRelatedComponent) value); 1334 } else 1335 super.removeChild(name, value); 1336 1337 } 1338 1339 @Override 1340 public Base makeProperty(int hash, String name) throws FHIRException { 1341 switch (hash) { 1342 case 243769515: 1343 return getMasterIdentifier(); 1344 case -1618432855: 1345 return addIdentifier(); 1346 case -892481550: 1347 return getStatusElement(); 1348 case 3575610: 1349 return getType(); 1350 case -1867885268: 1351 return getSubject(); 1352 case 1028554472: 1353 return getCreatedElement(); 1354 case -1406328437: 1355 return addAuthor(); 1356 case 820081177: 1357 return addRecipient(); 1358 case -896505829: 1359 return getSourceElement(); 1360 case -1724546052: 1361 return getDescriptionElement(); 1362 case 951530617: 1363 return addContent(); 1364 case 1090493483: 1365 return addRelated(); 1366 default: 1367 return super.makeProperty(hash, name); 1368 } 1369 1370 } 1371 1372 @Override 1373 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1374 switch (hash) { 1375 case 243769515: 1376 /* masterIdentifier */ return new String[] { "Identifier" }; 1377 case -1618432855: 1378 /* identifier */ return new String[] { "Identifier" }; 1379 case -892481550: 1380 /* status */ return new String[] { "code" }; 1381 case 3575610: 1382 /* type */ return new String[] { "CodeableConcept" }; 1383 case -1867885268: 1384 /* subject */ return new String[] { "Reference" }; 1385 case 1028554472: 1386 /* created */ return new String[] { "dateTime" }; 1387 case -1406328437: 1388 /* author */ return new String[] { "Reference" }; 1389 case 820081177: 1390 /* recipient */ return new String[] { "Reference" }; 1391 case -896505829: 1392 /* source */ return new String[] { "uri" }; 1393 case -1724546052: 1394 /* description */ return new String[] { "string" }; 1395 case 951530617: 1396 /* content */ return new String[] { "Reference" }; 1397 case 1090493483: 1398 /* related */ return new String[] {}; 1399 default: 1400 return super.getTypesForProperty(hash, name); 1401 } 1402 1403 } 1404 1405 @Override 1406 public Base addChild(String name) throws FHIRException { 1407 if (name.equals("masterIdentifier")) { 1408 this.masterIdentifier = new Identifier(); 1409 return this.masterIdentifier; 1410 } else if (name.equals("identifier")) { 1411 return addIdentifier(); 1412 } else if (name.equals("status")) { 1413 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.status"); 1414 } else if (name.equals("type")) { 1415 this.type = new CodeableConcept(); 1416 return this.type; 1417 } else if (name.equals("subject")) { 1418 this.subject = new Reference(); 1419 return this.subject; 1420 } else if (name.equals("created")) { 1421 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.created"); 1422 } else if (name.equals("author")) { 1423 return addAuthor(); 1424 } else if (name.equals("recipient")) { 1425 return addRecipient(); 1426 } else if (name.equals("source")) { 1427 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.source"); 1428 } else if (name.equals("description")) { 1429 throw new FHIRException("Cannot call addChild on a singleton property DocumentManifest.description"); 1430 } else if (name.equals("content")) { 1431 return addContent(); 1432 } else if (name.equals("related")) { 1433 return addRelated(); 1434 } else 1435 return super.addChild(name); 1436 } 1437 1438 public String fhirType() { 1439 return "DocumentManifest"; 1440 1441 } 1442 1443 public DocumentManifest copy() { 1444 DocumentManifest dst = new DocumentManifest(); 1445 copyValues(dst); 1446 return dst; 1447 } 1448 1449 public void copyValues(DocumentManifest dst) { 1450 super.copyValues(dst); 1451 dst.masterIdentifier = masterIdentifier == null ? null : masterIdentifier.copy(); 1452 if (identifier != null) { 1453 dst.identifier = new ArrayList<Identifier>(); 1454 for (Identifier i : identifier) 1455 dst.identifier.add(i.copy()); 1456 } 1457 ; 1458 dst.status = status == null ? null : status.copy(); 1459 dst.type = type == null ? null : type.copy(); 1460 dst.subject = subject == null ? null : subject.copy(); 1461 dst.created = created == null ? null : created.copy(); 1462 if (author != null) { 1463 dst.author = new ArrayList<Reference>(); 1464 for (Reference i : author) 1465 dst.author.add(i.copy()); 1466 } 1467 ; 1468 if (recipient != null) { 1469 dst.recipient = new ArrayList<Reference>(); 1470 for (Reference i : recipient) 1471 dst.recipient.add(i.copy()); 1472 } 1473 ; 1474 dst.source = source == null ? null : source.copy(); 1475 dst.description = description == null ? null : description.copy(); 1476 if (content != null) { 1477 dst.content = new ArrayList<Reference>(); 1478 for (Reference i : content) 1479 dst.content.add(i.copy()); 1480 } 1481 ; 1482 if (related != null) { 1483 dst.related = new ArrayList<DocumentManifestRelatedComponent>(); 1484 for (DocumentManifestRelatedComponent i : related) 1485 dst.related.add(i.copy()); 1486 } 1487 ; 1488 } 1489 1490 protected DocumentManifest typedCopy() { 1491 return copy(); 1492 } 1493 1494 @Override 1495 public boolean equalsDeep(Base other_) { 1496 if (!super.equalsDeep(other_)) 1497 return false; 1498 if (!(other_ instanceof DocumentManifest)) 1499 return false; 1500 DocumentManifest o = (DocumentManifest) other_; 1501 return compareDeep(masterIdentifier, o.masterIdentifier, true) && compareDeep(identifier, o.identifier, true) 1502 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 1503 && compareDeep(subject, o.subject, true) && compareDeep(created, o.created, true) 1504 && compareDeep(author, o.author, true) && compareDeep(recipient, o.recipient, true) 1505 && compareDeep(source, o.source, true) && compareDeep(description, o.description, true) 1506 && compareDeep(content, o.content, true) && compareDeep(related, o.related, true); 1507 } 1508 1509 @Override 1510 public boolean equalsShallow(Base other_) { 1511 if (!super.equalsShallow(other_)) 1512 return false; 1513 if (!(other_ instanceof DocumentManifest)) 1514 return false; 1515 DocumentManifest o = (DocumentManifest) other_; 1516 return compareValues(status, o.status, true) && compareValues(created, o.created, true) 1517 && compareValues(source, o.source, true) && compareValues(description, o.description, true); 1518 } 1519 1520 public boolean isEmpty() { 1521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(masterIdentifier, identifier, status, type, subject, 1522 created, author, recipient, source, description, content, related); 1523 } 1524 1525 @Override 1526 public ResourceType getResourceType() { 1527 return ResourceType.DocumentManifest; 1528 } 1529 1530 /** 1531 * Search parameter: <b>identifier</b> 1532 * <p> 1533 * Description: <b>Unique Identifier for the set of documents</b><br> 1534 * Type: <b>token</b><br> 1535 * Path: <b>DocumentManifest.masterIdentifier, 1536 * DocumentManifest.identifier</b><br> 1537 * </p> 1538 */ 1539 @SearchParamDefinition(name = "identifier", path = "DocumentManifest.masterIdentifier | DocumentManifest.identifier", description = "Unique Identifier for the set of documents", type = "token") 1540 public static final String SP_IDENTIFIER = "identifier"; 1541 /** 1542 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1543 * <p> 1544 * Description: <b>Unique Identifier for the set of documents</b><br> 1545 * Type: <b>token</b><br> 1546 * Path: <b>DocumentManifest.masterIdentifier, 1547 * DocumentManifest.identifier</b><br> 1548 * </p> 1549 */ 1550 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1551 SP_IDENTIFIER); 1552 1553 /** 1554 * Search parameter: <b>item</b> 1555 * <p> 1556 * Description: <b>Items in manifest</b><br> 1557 * Type: <b>reference</b><br> 1558 * Path: <b>DocumentManifest.content</b><br> 1559 * </p> 1560 */ 1561 @SearchParamDefinition(name = "item", path = "DocumentManifest.content", description = "Items in manifest", type = "reference") 1562 public static final String SP_ITEM = "item"; 1563 /** 1564 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1565 * <p> 1566 * Description: <b>Items in manifest</b><br> 1567 * Type: <b>reference</b><br> 1568 * Path: <b>DocumentManifest.content</b><br> 1569 * </p> 1570 */ 1571 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1572 SP_ITEM); 1573 1574 /** 1575 * Constant for fluent queries to be used to add include statements. Specifies 1576 * the path value of "<b>DocumentManifest:item</b>". 1577 */ 1578 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include( 1579 "DocumentManifest:item").toLocked(); 1580 1581 /** 1582 * Search parameter: <b>related-id</b> 1583 * <p> 1584 * Description: <b>Identifiers of things that are related</b><br> 1585 * Type: <b>token</b><br> 1586 * Path: <b>DocumentManifest.related.identifier</b><br> 1587 * </p> 1588 */ 1589 @SearchParamDefinition(name = "related-id", path = "DocumentManifest.related.identifier", description = "Identifiers of things that are related", type = "token") 1590 public static final String SP_RELATED_ID = "related-id"; 1591 /** 1592 * <b>Fluent Client</b> search parameter constant for <b>related-id</b> 1593 * <p> 1594 * Description: <b>Identifiers of things that are related</b><br> 1595 * Type: <b>token</b><br> 1596 * Path: <b>DocumentManifest.related.identifier</b><br> 1597 * </p> 1598 */ 1599 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATED_ID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1600 SP_RELATED_ID); 1601 1602 /** 1603 * Search parameter: <b>subject</b> 1604 * <p> 1605 * Description: <b>The subject of the set of documents</b><br> 1606 * Type: <b>reference</b><br> 1607 * Path: <b>DocumentManifest.subject</b><br> 1608 * </p> 1609 */ 1610 @SearchParamDefinition(name = "subject", path = "DocumentManifest.subject", description = "The subject of the set of documents", type = "reference", providesMembershipIn = { 1611 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 1612 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 1613 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 1614 Patient.class, Practitioner.class }) 1615 public static final String SP_SUBJECT = "subject"; 1616 /** 1617 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1618 * <p> 1619 * Description: <b>The subject of the set of documents</b><br> 1620 * Type: <b>reference</b><br> 1621 * Path: <b>DocumentManifest.subject</b><br> 1622 * </p> 1623 */ 1624 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1625 SP_SUBJECT); 1626 1627 /** 1628 * Constant for fluent queries to be used to add include statements. Specifies 1629 * the path value of "<b>DocumentManifest:subject</b>". 1630 */ 1631 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1632 "DocumentManifest:subject").toLocked(); 1633 1634 /** 1635 * Search parameter: <b>author</b> 1636 * <p> 1637 * Description: <b>Who and/or what authored the DocumentManifest</b><br> 1638 * Type: <b>reference</b><br> 1639 * Path: <b>DocumentManifest.author</b><br> 1640 * </p> 1641 */ 1642 @SearchParamDefinition(name = "author", path = "DocumentManifest.author", description = "Who and/or what authored the DocumentManifest", type = "reference", providesMembershipIn = { 1643 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 1644 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 1645 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 1646 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 1647 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 1648 public static final String SP_AUTHOR = "author"; 1649 /** 1650 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1651 * <p> 1652 * Description: <b>Who and/or what authored the DocumentManifest</b><br> 1653 * Type: <b>reference</b><br> 1654 * Path: <b>DocumentManifest.author</b><br> 1655 * </p> 1656 */ 1657 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1658 SP_AUTHOR); 1659 1660 /** 1661 * Constant for fluent queries to be used to add include statements. Specifies 1662 * the path value of "<b>DocumentManifest:author</b>". 1663 */ 1664 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include( 1665 "DocumentManifest:author").toLocked(); 1666 1667 /** 1668 * Search parameter: <b>created</b> 1669 * <p> 1670 * Description: <b>When this document manifest created</b><br> 1671 * Type: <b>date</b><br> 1672 * Path: <b>DocumentManifest.created</b><br> 1673 * </p> 1674 */ 1675 @SearchParamDefinition(name = "created", path = "DocumentManifest.created", description = "When this document manifest created", type = "date") 1676 public static final String SP_CREATED = "created"; 1677 /** 1678 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1679 * <p> 1680 * Description: <b>When this document manifest created</b><br> 1681 * Type: <b>date</b><br> 1682 * Path: <b>DocumentManifest.created</b><br> 1683 * </p> 1684 */ 1685 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 1686 SP_CREATED); 1687 1688 /** 1689 * Search parameter: <b>description</b> 1690 * <p> 1691 * Description: <b>Human-readable description (title)</b><br> 1692 * Type: <b>string</b><br> 1693 * Path: <b>DocumentManifest.description</b><br> 1694 * </p> 1695 */ 1696 @SearchParamDefinition(name = "description", path = "DocumentManifest.description", description = "Human-readable description (title)", type = "string") 1697 public static final String SP_DESCRIPTION = "description"; 1698 /** 1699 * <b>Fluent Client</b> search parameter constant for <b>description</b> 1700 * <p> 1701 * Description: <b>Human-readable description (title)</b><br> 1702 * Type: <b>string</b><br> 1703 * Path: <b>DocumentManifest.description</b><br> 1704 * </p> 1705 */ 1706 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 1707 SP_DESCRIPTION); 1708 1709 /** 1710 * Search parameter: <b>source</b> 1711 * <p> 1712 * Description: <b>The source system/application/software</b><br> 1713 * Type: <b>uri</b><br> 1714 * Path: <b>DocumentManifest.source</b><br> 1715 * </p> 1716 */ 1717 @SearchParamDefinition(name = "source", path = "DocumentManifest.source", description = "The source system/application/software", type = "uri") 1718 public static final String SP_SOURCE = "source"; 1719 /** 1720 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1721 * <p> 1722 * Description: <b>The source system/application/software</b><br> 1723 * Type: <b>uri</b><br> 1724 * Path: <b>DocumentManifest.source</b><br> 1725 * </p> 1726 */ 1727 public static final ca.uhn.fhir.rest.gclient.UriClientParam SOURCE = new ca.uhn.fhir.rest.gclient.UriClientParam( 1728 SP_SOURCE); 1729 1730 /** 1731 * Search parameter: <b>type</b> 1732 * <p> 1733 * Description: <b>Kind of document set</b><br> 1734 * Type: <b>token</b><br> 1735 * Path: <b>DocumentManifest.type</b><br> 1736 * </p> 1737 */ 1738 @SearchParamDefinition(name = "type", path = "DocumentManifest.type", description = "Kind of document set", type = "token") 1739 public static final String SP_TYPE = "type"; 1740 /** 1741 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1742 * <p> 1743 * Description: <b>Kind of document set</b><br> 1744 * Type: <b>token</b><br> 1745 * Path: <b>DocumentManifest.type</b><br> 1746 * </p> 1747 */ 1748 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1749 SP_TYPE); 1750 1751 /** 1752 * Search parameter: <b>related-ref</b> 1753 * <p> 1754 * Description: <b>Related Resource</b><br> 1755 * Type: <b>reference</b><br> 1756 * Path: <b>DocumentManifest.related.ref</b><br> 1757 * </p> 1758 */ 1759 @SearchParamDefinition(name = "related-ref", path = "DocumentManifest.related.ref", description = "Related Resource", type = "reference", providesMembershipIn = { 1760 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }) 1761 public static final String SP_RELATED_REF = "related-ref"; 1762 /** 1763 * <b>Fluent Client</b> search parameter constant for <b>related-ref</b> 1764 * <p> 1765 * Description: <b>Related Resource</b><br> 1766 * Type: <b>reference</b><br> 1767 * Path: <b>DocumentManifest.related.ref</b><br> 1768 * </p> 1769 */ 1770 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RELATED_REF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1771 SP_RELATED_REF); 1772 1773 /** 1774 * Constant for fluent queries to be used to add include statements. Specifies 1775 * the path value of "<b>DocumentManifest:related-ref</b>". 1776 */ 1777 public static final ca.uhn.fhir.model.api.Include INCLUDE_RELATED_REF = new ca.uhn.fhir.model.api.Include( 1778 "DocumentManifest:related-ref").toLocked(); 1779 1780 /** 1781 * Search parameter: <b>patient</b> 1782 * <p> 1783 * Description: <b>The subject of the set of documents</b><br> 1784 * Type: <b>reference</b><br> 1785 * Path: <b>DocumentManifest.subject</b><br> 1786 * </p> 1787 */ 1788 @SearchParamDefinition(name = "patient", path = "DocumentManifest.subject.where(resolve() is Patient)", description = "The subject of the set of documents", type = "reference", target = { 1789 Patient.class }) 1790 public static final String SP_PATIENT = "patient"; 1791 /** 1792 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1793 * <p> 1794 * Description: <b>The subject of the set of documents</b><br> 1795 * Type: <b>reference</b><br> 1796 * Path: <b>DocumentManifest.subject</b><br> 1797 * </p> 1798 */ 1799 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1800 SP_PATIENT); 1801 1802 /** 1803 * Constant for fluent queries to be used to add include statements. Specifies 1804 * the path value of "<b>DocumentManifest:patient</b>". 1805 */ 1806 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1807 "DocumentManifest:patient").toLocked(); 1808 1809 /** 1810 * Search parameter: <b>recipient</b> 1811 * <p> 1812 * Description: <b>Intended to get notified about this set of documents</b><br> 1813 * Type: <b>reference</b><br> 1814 * Path: <b>DocumentManifest.recipient</b><br> 1815 * </p> 1816 */ 1817 @SearchParamDefinition(name = "recipient", path = "DocumentManifest.recipient", description = "Intended to get notified about this set of documents", type = "reference", providesMembershipIn = { 1818 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 1819 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 1820 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 1821 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 1822 public static final String SP_RECIPIENT = "recipient"; 1823 /** 1824 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 1825 * <p> 1826 * Description: <b>Intended to get notified about this set of documents</b><br> 1827 * Type: <b>reference</b><br> 1828 * Path: <b>DocumentManifest.recipient</b><br> 1829 * </p> 1830 */ 1831 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1832 SP_RECIPIENT); 1833 1834 /** 1835 * Constant for fluent queries to be used to add include statements. Specifies 1836 * the path value of "<b>DocumentManifest:recipient</b>". 1837 */ 1838 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 1839 "DocumentManifest:recipient").toLocked(); 1840 1841 /** 1842 * Search parameter: <b>status</b> 1843 * <p> 1844 * Description: <b>current | superseded | entered-in-error</b><br> 1845 * Type: <b>token</b><br> 1846 * Path: <b>DocumentManifest.status</b><br> 1847 * </p> 1848 */ 1849 @SearchParamDefinition(name = "status", path = "DocumentManifest.status", description = "current | superseded | entered-in-error", type = "token") 1850 public static final String SP_STATUS = "status"; 1851 /** 1852 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1853 * <p> 1854 * Description: <b>current | superseded | entered-in-error</b><br> 1855 * Type: <b>token</b><br> 1856 * Path: <b>DocumentManifest.status</b><br> 1857 * </p> 1858 */ 1859 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1860 SP_STATUS); 1861 1862}