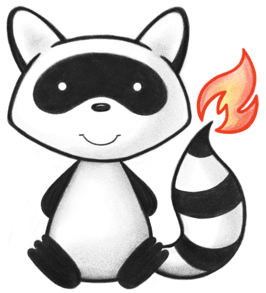
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Collections; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.instance.model.api.IBaseHasModifierExtensions; 040import org.hl7.fhir.instance.model.api.IDomainResource; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * A resource that includes narrative, extensions, and contained resources. 047 */ 048public abstract class DomainResource extends Resource 049 implements IBaseHasExtensions, IBaseHasModifierExtensions, IDomainResource { 050 051 /** 052 * A human-readable narrative that contains a summary of the resource and can be 053 * used to represent the content of the resource to a human. The narrative need 054 * not encode all the structured data, but is required to contain sufficient 055 * detail to make it "clinically safe" for a human to just read the narrative. 056 * Resource definitions may define what content should be represented in the 057 * narrative to ensure clinical safety. 058 */ 059 @Child(name = "text", type = { Narrative.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 060 @Description(shortDefinition = "Text summary of the resource, for human interpretation", formalDefinition = "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.") 061 protected Narrative text; 062 063 /** 064 * These resources do not have an independent existence apart from the resource 065 * that contains them - they cannot be identified independently, and nor can 066 * they have their own independent transaction scope. 067 */ 068 @Child(name = "contained", type = { 069 Resource.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 070 @Description(shortDefinition = "Contained, inline Resources", formalDefinition = "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.") 071 protected List<Resource> contained; 072 073 /** 074 * May be used to represent additional information that is not part of the basic 075 * definition of the resource. To make the use of extensions safe and 076 * manageable, there is a strict set of governance applied to the definition and 077 * use of extensions. Though any implementer can define an extension, there is a 078 * set of requirements that SHALL be met as part of the definition of the 079 * extension. 080 */ 081 @Child(name = "extension", type = { 082 Extension.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 083 @Description(shortDefinition = "Additional content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 084 protected List<Extension> extension; 085 086 /** 087 * May be used to represent additional information that is not part of the basic 088 * definition of the resource and that modifies the understanding of the element 089 * that contains it and/or the understanding of the containing element's 090 * descendants. Usually modifier elements provide negation or qualification. To 091 * make the use of extensions safe and manageable, there is a strict set of 092 * governance applied to the definition and use of extensions. Though any 093 * implementer is allowed to define an extension, there is a set of requirements 094 * that SHALL be met as part of the definition of the extension. Applications 095 * processing a resource are required to check for modifier extensions. 096 * 097 * Modifier extensions SHALL NOT change the meaning of any elements on Resource 098 * or DomainResource (including cannot change the meaning of modifierExtension 099 * itself). 100 */ 101 @Child(name = "modifierExtension", type = { 102 Extension.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 103 @Description(shortDefinition = "Extensions that cannot be ignored", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).") 104 protected List<Extension> modifierExtension; 105 106 private static final long serialVersionUID = -970285559L; 107 108 /** 109 * Constructor 110 */ 111 public DomainResource() { 112 super(); 113 } 114 115 /** 116 * @return {@link #text} (A human-readable narrative that contains a summary of 117 * the resource and can be used to represent the content of the resource 118 * to a human. The narrative need not encode all the structured data, 119 * but is required to contain sufficient detail to make it "clinically 120 * safe" for a human to just read the narrative. Resource definitions 121 * may define what content should be represented in the narrative to 122 * ensure clinical safety.) 123 */ 124 public Narrative getText() { 125 if (this.text == null) 126 if (Configuration.errorOnAutoCreate()) 127 throw new Error("Attempt to auto-create DomainResource.text"); 128 else if (Configuration.doAutoCreate()) 129 this.text = new Narrative(); // cc 130 return this.text; 131 } 132 133 public boolean hasText() { 134 return this.text != null && !this.text.isEmpty(); 135 } 136 137 /** 138 * @param value {@link #text} (A human-readable narrative that contains a 139 * summary of the resource and can be used to represent the content 140 * of the resource to a human. The narrative need not encode all 141 * the structured data, but is required to contain sufficient 142 * detail to make it "clinically safe" for a human to just read the 143 * narrative. Resource definitions may define what content should 144 * be represented in the narrative to ensure clinical safety.) 145 */ 146 public DomainResource setText(Narrative value) { 147 this.text = value; 148 return this; 149 } 150 151 /** 152 * @return {@link #contained} (These resources do not have an independent 153 * existence apart from the resource that contains them - they cannot be 154 * identified independently, and nor can they have their own independent 155 * transaction scope.) 156 */ 157 public List<Resource> getContained() { 158 if (this.contained == null) 159 this.contained = new ArrayList<Resource>(); 160 return this.contained; 161 } 162 163 /** 164 * @return Returns a reference to <code>this</code> for easy method chaining 165 */ 166 public DomainResource setContained(List<Resource> theContained) { 167 this.contained = theContained; 168 return this; 169 } 170 171 public boolean hasContained() { 172 if (this.contained == null) 173 return false; 174 for (Resource item : this.contained) 175 if (!item.isEmpty()) 176 return true; 177 return false; 178 } 179 180 public DomainResource addContained(Resource t) { // 3 181 if (t == null) 182 return this; 183 if (this.contained == null) 184 this.contained = new ArrayList<Resource>(); 185 this.contained.add(t); 186 return this; 187 } 188 189 /** 190 * @return {@link #extension} (May be used to represent additional information 191 * that is not part of the basic definition of the resource. To make the 192 * use of extensions safe and manageable, there is a strict set of 193 * governance applied to the definition and use of extensions. Though 194 * any implementer can define an extension, there is a set of 195 * requirements that SHALL be met as part of the definition of the 196 * extension.) 197 */ 198 public List<Extension> getExtension() { 199 if (this.extension == null) 200 this.extension = new ArrayList<Extension>(); 201 return this.extension; 202 } 203 204 /** 205 * @return Returns a reference to <code>this</code> for easy method chaining 206 */ 207 public DomainResource setExtension(List<Extension> theExtension) { 208 this.extension = theExtension; 209 return this; 210 } 211 212 public boolean hasExtension() { 213 if (this.extension == null) 214 return false; 215 for (Extension item : this.extension) 216 if (!item.isEmpty()) 217 return true; 218 return false; 219 } 220 221 public Extension addExtension() { // 3 222 Extension t = new Extension(); 223 if (this.extension == null) 224 this.extension = new ArrayList<Extension>(); 225 this.extension.add(t); 226 return t; 227 } 228 229 public DomainResource addExtension(Extension t) { // 3 230 if (t == null) 231 return this; 232 if (this.extension == null) 233 this.extension = new ArrayList<Extension>(); 234 this.extension.add(t); 235 return this; 236 } 237 238 /** 239 * @return {@link #modifierExtension} (May be used to represent additional 240 * information that is not part of the basic definition of the resource 241 * and that modifies the understanding of the element that contains it 242 * and/or the understanding of the containing element's descendants. 243 * Usually modifier elements provide negation or qualification. To make 244 * the use of extensions safe and manageable, there is a strict set of 245 * governance applied to the definition and use of extensions. Though 246 * any implementer is allowed to define an extension, there is a set of 247 * requirements that SHALL be met as part of the definition of the 248 * extension. Applications processing a resource are required to check 249 * for modifier extensions. 250 * 251 * Modifier extensions SHALL NOT change the meaning of any elements on 252 * Resource or DomainResource (including cannot change the meaning of 253 * modifierExtension itself).) 254 */ 255 public List<Extension> getModifierExtension() { 256 if (this.modifierExtension == null) 257 this.modifierExtension = new ArrayList<Extension>(); 258 return this.modifierExtension; 259 } 260 261 /** 262 * @return Returns a reference to <code>this</code> for easy method chaining 263 */ 264 public DomainResource setModifierExtension(List<Extension> theModifierExtension) { 265 this.modifierExtension = theModifierExtension; 266 return this; 267 } 268 269 public boolean hasModifierExtension() { 270 if (this.modifierExtension == null) 271 return false; 272 for (Extension item : this.modifierExtension) 273 if (!item.isEmpty()) 274 return true; 275 return false; 276 } 277 278 public Extension addModifierExtension() { // 3 279 Extension t = new Extension(); 280 if (this.modifierExtension == null) 281 this.modifierExtension = new ArrayList<Extension>(); 282 this.modifierExtension.add(t); 283 return t; 284 } 285 286 public DomainResource addModifierExtension(Extension t) { // 3 287 if (t == null) 288 return this; 289 if (this.modifierExtension == null) 290 this.modifierExtension = new ArrayList<Extension>(); 291 this.modifierExtension.add(t); 292 return this; 293 } 294 295 /** 296 * Returns a list of extensions from this element which have the given URL. Note 297 * that this list may not be modified (you can not add or remove elements from 298 * it) 299 */ 300 public List<Extension> getExtensionsByUrl(String theUrl) { 301 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 302 ArrayList<Extension> retVal = new ArrayList<Extension>(); 303 for (Extension next : getExtension()) { 304 if (theUrl.equals(next.getUrl())) { 305 retVal.add(next); 306 } 307 } 308 for (Extension next : getModifierExtension()) { 309 if (theUrl.equals(next.getUrl())) { 310 retVal.add(next); 311 } 312 } 313 return Collections.unmodifiableList(retVal); 314 } 315 316 /** 317 * Returns a list of modifier extensions from this element which have the given 318 * URL. Note that this list may not be modified (you can not add or remove 319 * elements from it) 320 */ 321 public List<Extension> getModifierExtensionsByUrl(String theUrl) { 322 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must be provided with a value"); 323 ArrayList<Extension> retVal = new ArrayList<Extension>(); 324 for (Extension next : getModifierExtension()) { 325 if (theUrl.equals(next.getUrl())) { 326 retVal.add(next); 327 } 328 } 329 return Collections.unmodifiableList(retVal); 330 } 331 332 protected void listChildren(List<Property> children) { 333 super.listChildren(children); 334 children.add(new Property("text", "Narrative", 335 "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 336 0, 1, text)); 337 children.add(new Property("contained", "Resource", 338 "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 339 0, java.lang.Integer.MAX_VALUE, contained)); 340 children.add(new Property("extension", "Extension", 341 "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 342 0, java.lang.Integer.MAX_VALUE, extension)); 343 children.add(new Property("modifierExtension", "Extension", 344 "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 345 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 346 } 347 348 @Override 349 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 350 switch (_hash) { 351 case 3556653: 352 /* text */ return new Property("text", "Narrative", 353 "A human-readable narrative that contains a summary of the resource and can be used to represent the content of the resource to a human. The narrative need not encode all the structured data, but is required to contain sufficient detail to make it \"clinically safe\" for a human to just read the narrative. Resource definitions may define what content should be represented in the narrative to ensure clinical safety.", 354 0, 1, text); 355 case -410956685: 356 /* contained */ return new Property("contained", "Resource", 357 "These resources do not have an independent existence apart from the resource that contains them - they cannot be identified independently, and nor can they have their own independent transaction scope.", 358 0, java.lang.Integer.MAX_VALUE, contained); 359 case -612557761: 360 /* extension */ return new Property("extension", "Extension", 361 "May be used to represent additional information that is not part of the basic definition of the resource. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 362 0, java.lang.Integer.MAX_VALUE, extension); 363 case -298878168: 364 /* modifierExtension */ return new Property("modifierExtension", "Extension", 365 "May be used to represent additional information that is not part of the basic definition of the resource and that modifies the understanding of the element that contains it and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 366 0, java.lang.Integer.MAX_VALUE, modifierExtension); 367 default: 368 return super.getNamedProperty(_hash, _name, _checkValid); 369 } 370 371 } 372 373 @Override 374 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 375 switch (hash) { 376 case 3556653: 377 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // Narrative 378 case -410956685: 379 /* contained */ return this.contained == null ? new Base[0] 380 : this.contained.toArray(new Base[this.contained.size()]); // Resource 381 case -612557761: 382 /* extension */ return this.extension == null ? new Base[0] 383 : this.extension.toArray(new Base[this.extension.size()]); // Extension 384 case -298878168: 385 /* modifierExtension */ return this.modifierExtension == null ? new Base[0] 386 : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 387 default: 388 return super.getProperty(hash, name, checkValid); 389 } 390 391 } 392 393 @Override 394 public Base setProperty(int hash, String name, Base value) throws FHIRException { 395 switch (hash) { 396 case 3556653: // text 397 this.text = castToNarrative(value); // Narrative 398 return value; 399 case -410956685: // contained 400 this.getContained().add(castToResource(value)); // Resource 401 return value; 402 case -612557761: // extension 403 this.getExtension().add(castToExtension(value)); // Extension 404 return value; 405 case -298878168: // modifierExtension 406 this.getModifierExtension().add(castToExtension(value)); // Extension 407 return value; 408 default: 409 return super.setProperty(hash, name, value); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(String name, Base value) throws FHIRException { 416 if (name.equals("text")) { 417 this.text = castToNarrative(value); // Narrative 418 } else if (name.equals("contained")) { 419 this.getContained().add(castToResource(value)); 420 } else if (name.equals("extension")) { 421 this.getExtension().add(castToExtension(value)); 422 } else if (name.equals("modifierExtension")) { 423 this.getModifierExtension().add(castToExtension(value)); 424 } else 425 return super.setProperty(name, value); 426 return value; 427 } 428 429 @Override 430 public void removeChild(String name, Base value) throws FHIRException { 431 if (name.equals("text")) { 432 this.text = null; 433 } else if (name.equals("contained")) { 434 this.getContained().remove(castToResource(value)); 435 } else if (name.equals("extension")) { 436 this.getExtension().remove(castToExtension(value)); 437 } else if (name.equals("modifierExtension")) { 438 this.getModifierExtension().remove(castToExtension(value)); 439 } else 440 super.removeChild(name, value); 441 442 } 443 444 @Override 445 public Base makeProperty(int hash, String name) throws FHIRException { 446 switch (hash) { 447 case 3556653: 448 return getText(); 449 case -410956685: 450 throw new FHIRException("Cannot make property contained as it is not a complex type"); // Resource 451 case -612557761: 452 return addExtension(); 453 case -298878168: 454 return addModifierExtension(); 455 default: 456 return super.makeProperty(hash, name); 457 } 458 459 } 460 461 @Override 462 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 463 switch (hash) { 464 case 3556653: 465 /* text */ return new String[] { "Narrative" }; 466 case -410956685: 467 /* contained */ return new String[] { "Resource" }; 468 case -612557761: 469 /* extension */ return new String[] { "Extension" }; 470 case -298878168: 471 /* modifierExtension */ return new String[] { "Extension" }; 472 default: 473 return super.getTypesForProperty(hash, name); 474 } 475 476 } 477 478 @Override 479 public Base addChild(String name) throws FHIRException { 480 if (name.equals("text")) { 481 this.text = new Narrative(); 482 return this.text; 483 } else if (name.equals("contained")) { 484 throw new FHIRException("Cannot call addChild on an abstract type DomainResource.contained"); 485 } else if (name.equals("extension")) { 486 return addExtension(); 487 } else if (name.equals("modifierExtension")) { 488 return addModifierExtension(); 489 } else 490 return super.addChild(name); 491 } 492 493 public String fhirType() { 494 return "DomainResource"; 495 496 } 497 498 public abstract DomainResource copy(); 499 500 public void copyValues(DomainResource dst) { 501 super.copyValues(dst); 502 dst.text = text == null ? null : text.copy(); 503 if (contained != null) { 504 dst.contained = new ArrayList<Resource>(); 505 for (Resource i : contained) 506 dst.contained.add(i.copy()); 507 } 508 ; 509 if (extension != null) { 510 dst.extension = new ArrayList<Extension>(); 511 for (Extension i : extension) 512 dst.extension.add(i.copy()); 513 } 514 ; 515 if (modifierExtension != null) { 516 dst.modifierExtension = new ArrayList<Extension>(); 517 for (Extension i : modifierExtension) 518 dst.modifierExtension.add(i.copy()); 519 } 520 ; 521 } 522 523 @Override 524 public boolean equalsDeep(Base other_) { 525 if (!super.equalsDeep(other_)) 526 return false; 527 if (!(other_ instanceof DomainResource)) 528 return false; 529 DomainResource o = (DomainResource) other_; 530 return compareDeep(text, o.text, true) && compareDeep(contained, o.contained, true) 531 && compareDeep(extension, o.extension, true) && compareDeep(modifierExtension, o.modifierExtension, true); 532 } 533 534 @Override 535 public boolean equalsShallow(Base other_) { 536 if (!super.equalsShallow(other_)) 537 return false; 538 if (!(other_ instanceof DomainResource)) 539 return false; 540 DomainResource o = (DomainResource) other_; 541 return true; 542 } 543 544 public boolean isEmpty() { 545 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, contained, extension, modifierExtension); 546 } 547 548// added from java-adornments.txt: 549 550 public void checkNoModifiers(String noun, String verb) throws FHIRException { 551 if (hasModifierExtension()) { 552 throw new FHIRException("Found unknown Modifier Exceptions on " + noun + " doing " + verb); 553 } 554 555 } 556 557 public void addExtension(String url, Type value) { 558 Extension ex = new Extension(); 559 ex.setUrl(url); 560 ex.setValue(value); 561 getExtension().add(ex); 562 } 563 564 public boolean hasExtension(String url) { 565 for (Extension next : getModifierExtension()) { 566 if (url.equals(next.getUrl())) { 567 return true; 568 } 569 } 570 for (Extension e : getExtension()) 571 if (url.equals(e.getUrl())) 572 return true; 573 return false; 574 } 575 576 public Extension getExtensionByUrl(String theUrl) { 577 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 578 ArrayList<Extension> retVal = new ArrayList<Extension>(); 579 for (Extension next : getExtension()) { 580 if (theUrl.equals(next.getUrl())) { 581 retVal.add(next); 582 } 583 } 584 585 for (Extension next : getModifierExtension()) { 586 if (theUrl.equals(next.getUrl())) { 587 retVal.add(next); 588 } 589 } 590 if (retVal.size() == 0) 591 return null; 592 else { 593 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 594 return retVal.get(0); 595 } 596 } 597 598 public Resource getContained(String reference) { 599 for (Resource c : getContained()) { 600 if (reference.equals("#"+c.getId())) { 601 return c; 602 } 603 } 604 return null; 605 } 606 607// end addition 608 609}