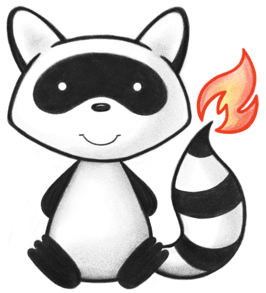
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseElement; 038import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * Base definition for all elements in a resource. 046 */ 047public abstract class Element extends Base implements IBaseHasExtensions, IBaseElement { 048 049 /** 050 * Unique id for the element within a resource (for internal references). This 051 * may be any string value that does not contain spaces. 052 */ 053 @Child(name = "id", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = false) 054 @Description(shortDefinition = "Unique id for inter-element referencing", formalDefinition = "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.") 055 protected StringType id; 056 057 /** 058 * May be used to represent additional information that is not part of the basic 059 * definition of the element. To make the use of extensions safe and manageable, 060 * there is a strict set of governance applied to the definition and use of 061 * extensions. Though any implementer can define an extension, there is a set of 062 * requirements that SHALL be met as part of the definition of the extension. 063 */ 064 @Child(name = "extension", type = { 065 Extension.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 066 @Description(shortDefinition = "Additional content defined by implementations", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.") 067 protected List<Extension> extension; 068 069 private static final long serialVersionUID = -1452745816L; 070 071 /** 072 * Constructor 073 */ 074 public Element() { 075 super(); 076 } 077 078 /** 079 * @return {@link #id} (Unique id for the element within a resource (for 080 * internal references). This may be any string value that does not 081 * contain spaces.). This is the underlying object with id, value and 082 * extensions. The accessor "getId" gives direct access to the value 083 */ 084 public StringType getIdElement() { 085 if (this.id == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create Element.id"); 088 else if (Configuration.doAutoCreate()) 089 this.id = new StringType(); // bb 090 return this.id; 091 } 092 093 public boolean hasIdElement() { 094 return this.id != null && !this.id.isEmpty(); 095 } 096 097 public boolean hasId() { 098 return this.id != null && !this.id.isEmpty(); 099 } 100 101 /** 102 * @param value {@link #id} (Unique id for the element within a resource (for 103 * internal references). This may be any string value that does not 104 * contain spaces.). This is the underlying object with id, value 105 * and extensions. The accessor "getId" gives direct access to the 106 * value 107 */ 108 public Element setIdElement(StringType value) { 109 this.id = value; 110 return this; 111 } 112 113 /** 114 * @return Unique id for the element within a resource (for internal 115 * references). This may be any string value that does not contain 116 * spaces. 117 */ 118 public String getId() { 119 return this.id == null ? null : this.id.getValue(); 120 } 121 122 /** 123 * @param value Unique id for the element within a resource (for internal 124 * references). This may be any string value that does not contain 125 * spaces. 126 */ 127 public Element setId(String value) { 128 if (Utilities.noString(value)) 129 this.id = null; 130 else { 131 if (this.id == null) 132 this.id = new StringType(); 133 this.id.setValue(value); 134 } 135 return this; 136 } 137 138 /** 139 * @return {@link #extension} (May be used to represent additional information 140 * that is not part of the basic definition of the element. To make the 141 * use of extensions safe and manageable, there is a strict set of 142 * governance applied to the definition and use of extensions. Though 143 * any implementer can define an extension, there is a set of 144 * requirements that SHALL be met as part of the definition of the 145 * extension.) 146 */ 147 public List<Extension> getExtension() { 148 if (this.extension == null) 149 this.extension = new ArrayList<Extension>(); 150 return this.extension; 151 } 152 153 /** 154 * @return Returns a reference to <code>this</code> for easy method chaining 155 */ 156 public Element setExtension(List<Extension> theExtension) { 157 this.extension = theExtension; 158 return this; 159 } 160 161 public boolean hasExtension() { 162 if (this.extension == null) 163 return false; 164 for (Extension item : this.extension) 165 if (!item.isEmpty()) 166 return true; 167 return false; 168 } 169 170 public Extension addExtension() { // 3 171 Extension t = new Extension(); 172 if (this.extension == null) 173 this.extension = new ArrayList<Extension>(); 174 this.extension.add(t); 175 return t; 176 } 177 178 public Element addExtension(Extension t) { // 3 179 if (t == null) 180 return this; 181 if (this.extension == null) 182 this.extension = new ArrayList<Extension>(); 183 this.extension.add(t); 184 return this; 185 } 186 187 /** 188 * @return The first repetition of repeating field {@link #extension}, creating 189 * it if it does not already exist 190 */ 191 public Extension getExtensionFirstRep() { 192 if (getExtension().isEmpty()) { 193 addExtension(); 194 } 195 return getExtension().get(0); 196 } 197 198 /** 199 * Returns an unmodifiable list containing all extensions on this element which 200 * match the given URL. 201 * 202 * @param theUrl The URL. Must not be blank or null. 203 * @return an unmodifiable list containing all extensions on this element which 204 * match the given URL 205 */ 206 public List<Extension> getExtensionsByUrl(String theUrl) { 207 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 208 ArrayList<Extension> retVal = new ArrayList<Extension>(); 209 for (Extension next : getExtension()) { 210 if (theUrl.equals(next.getUrl())) { 211 retVal.add(next); 212 } 213 } 214 return java.util.Collections.unmodifiableList(retVal); 215 } 216 217 public boolean hasExtension(String theUrl) { 218 return !getExtensionsByUrl(theUrl).isEmpty(); 219 } 220 221 public String getExtensionString(String theUrl) throws FHIRException { 222 List<Extension> ext = getExtensionsByUrl(theUrl); 223 if (ext.isEmpty()) 224 return null; 225 if (ext.size() > 1) 226 throw new FHIRException("Multiple matching extensions found"); 227 if (!ext.get(0).getValue().isPrimitive()) 228 throw new FHIRException("Extension could not be converted to a string"); 229 return ext.get(0).getValue().primitiveValue(); 230 } 231 232 protected void listChildren(List<Property> children) { 233 children.add(new Property("id", "string", 234 "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 235 0, 1, id)); 236 children.add(new Property("extension", "Extension", 237 "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 238 0, java.lang.Integer.MAX_VALUE, extension)); 239 } 240 241 @Override 242 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 243 switch (_hash) { 244 case 3355: 245 /* id */ return new Property("id", "string", 246 "Unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 247 0, 1, id); 248 case -612557761: 249 /* extension */ return new Property("extension", "Extension", 250 "May be used to represent additional information that is not part of the basic definition of the element. To make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 251 0, java.lang.Integer.MAX_VALUE, extension); 252 default: 253 return super.getNamedProperty(_hash, _name, _checkValid); 254 } 255 256 } 257 258 @Override 259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 260 switch (hash) { 261 case 3355: 262 /* id */ return this.id == null ? new Base[0] : new Base[] { this.id }; // StringType 263 case -612557761: 264 /* extension */ return this.extension == null ? new Base[0] 265 : this.extension.toArray(new Base[this.extension.size()]); // Extension 266 default: 267 return super.getProperty(hash, name, checkValid); 268 } 269 270 } 271 272 @Override 273 public Base setProperty(int hash, String name, Base value) throws FHIRException { 274 switch (hash) { 275 case 3355: // id 276 this.id = castToString(value); // StringType 277 return value; 278 case -612557761: // extension 279 this.getExtension().add(castToExtension(value)); // Extension 280 return value; 281 default: 282 return super.setProperty(hash, name, value); 283 } 284 285 } 286 287 @Override 288 public Base setProperty(String name, Base value) throws FHIRException { 289 if (name.equals("id")) { 290 this.id = castToString(value); // StringType 291 } else if (name.equals("extension")) { 292 this.getExtension().add(castToExtension(value)); 293 } else 294 return super.setProperty(name, value); 295 return value; 296 } 297 298 @Override 299 public Base makeProperty(int hash, String name) throws FHIRException { 300 switch (hash) { 301 case 3355: 302 return getIdElement(); 303 case -612557761: 304 return addExtension(); 305 default: 306 return super.makeProperty(hash, name); 307 } 308 309 } 310 311 @Override 312 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 313 switch (hash) { 314 case 3355: 315 /* id */ return new String[] { "string" }; 316 case -612557761: 317 /* extension */ return new String[] { "Extension" }; 318 default: 319 return super.getTypesForProperty(hash, name); 320 } 321 322 } 323 324 @Override 325 public Base addChild(String name) throws FHIRException { 326 if (name.equals("id")) { 327 throw new FHIRException("Cannot call addChild on a singleton property Element.id"); 328 } else if (name.equals("extension")) { 329 return addExtension(); 330 } else 331 return super.addChild(name); 332 } 333 334 public String fhirType() { 335 return "Element"; 336 337 } 338 339 public abstract Element copy(); 340 341 public void copyValues(Element dst) { 342 dst.id = id == null ? null : id.copy(); 343 if (extension != null) { 344 dst.extension = new ArrayList<Extension>(); 345 for (Extension i : extension) 346 dst.extension.add(i.copy()); 347 } 348 ; 349 } 350 351 @Override 352 public boolean equalsDeep(Base other_) { 353 if (!super.equalsDeep(other_)) 354 return false; 355 if (!(other_ instanceof Element)) 356 return false; 357 Element o = (Element) other_; 358 return compareDeep(id, o.id, true) && compareDeep(extension, o.extension, true); 359 } 360 361 @Override 362 public boolean equalsShallow(Base other_) { 363 if (!super.equalsShallow(other_)) 364 return false; 365 if (!(other_ instanceof Element)) 366 return false; 367 Element o = (Element) other_; 368 return compareValues(id, o.id, true); 369 } 370 371 public boolean isEmpty() { 372 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension); 373 } 374 375 @Override 376 public String getIdBase() { 377 return getId(); 378 } 379 380 @Override 381 public void setIdBase(String value) { 382 setId(value); 383 } 384 385// added from java-adornments.txt: 386 public void addExtension(String url, Type value) { 387 if (disallowExtensions) 388 throw new Error("Extensions are not allowed in this context"); 389 Extension ex = new Extension(); 390 ex.setUrl(url); 391 ex.setValue(value); 392 getExtension().add(ex); 393 } 394 395 public Extension getExtensionByUrl(String theUrl) { 396 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 397 ArrayList<Extension> retVal = new ArrayList<Extension>(); 398 for (Extension next : getExtension()) { 399 if (theUrl.equals(next.getUrl())) { 400 retVal.add(next); 401 } 402 } 403 if (retVal.size() == 0) 404 return null; 405 else { 406 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url " + theUrl + " must have only one match"); 407 return retVal.get(0); 408 } 409 } 410 411 public void removeExtension(String theUrl) { 412 for (int i = getExtension().size() - 1; i >= 0; i--) { 413 if (theUrl.equals(getExtension().get(i).getUrl())) 414 getExtension().remove(i); 415 } 416 } 417 418 /** 419 * This is used in the FHIRPath engine to record that no extensions are allowed 420 * for this item in the context in which it is used. todo: enforce this.... 421 */ 422 private boolean disallowExtensions; 423 424 public boolean isDisallowExtensions() { 425 return disallowExtensions; 426 } 427 428 public Element setDisallowExtensions(boolean disallowExtensions) { 429 this.disallowExtensions = disallowExtensions; 430 return this; 431 } 432 433 public Element noExtensions() { 434 this.disallowExtensions = true; 435 return this; 436 } 437 438// end addition 439 440}