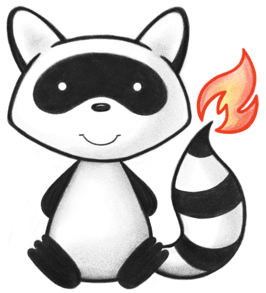
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * An interaction between a patient and healthcare provider(s) for the purpose 047 * of providing healthcare service(s) or assessing the health status of a 048 * patient. 049 */ 050@ResourceDef(name = "Encounter", profile = "http://hl7.org/fhir/StructureDefinition/Encounter") 051public class Encounter extends DomainResource { 052 053 public enum EncounterStatus { 054 /** 055 * The Encounter has not yet started. 056 */ 057 PLANNED, 058 /** 059 * The Patient is present for the encounter, however is not currently meeting 060 * with a practitioner. 061 */ 062 ARRIVED, 063 /** 064 * The patient has been assessed for the priority of their treatment based on 065 * the severity of their condition. 066 */ 067 TRIAGED, 068 /** 069 * The Encounter has begun and the patient is present / the practitioner and the 070 * patient are meeting. 071 */ 072 INPROGRESS, 073 /** 074 * The Encounter has begun, but the patient is temporarily on leave. 075 */ 076 ONLEAVE, 077 /** 078 * The Encounter has ended. 079 */ 080 FINISHED, 081 /** 082 * The Encounter has ended before it has begun. 083 */ 084 CANCELLED, 085 /** 086 * This instance should not have been part of this patient's medical record. 087 */ 088 ENTEREDINERROR, 089 /** 090 * The encounter status is unknown. Note that "unknown" is a value of last 091 * resort and every attempt should be made to provide a meaningful value other 092 * than "unknown". 093 */ 094 UNKNOWN, 095 /** 096 * added to help the parsers with the generic types 097 */ 098 NULL; 099 100 public static EncounterStatus fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("planned".equals(codeString)) 104 return PLANNED; 105 if ("arrived".equals(codeString)) 106 return ARRIVED; 107 if ("triaged".equals(codeString)) 108 return TRIAGED; 109 if ("in-progress".equals(codeString)) 110 return INPROGRESS; 111 if ("onleave".equals(codeString)) 112 return ONLEAVE; 113 if ("finished".equals(codeString)) 114 return FINISHED; 115 if ("cancelled".equals(codeString)) 116 return CANCELLED; 117 if ("entered-in-error".equals(codeString)) 118 return ENTEREDINERROR; 119 if ("unknown".equals(codeString)) 120 return UNKNOWN; 121 if (Configuration.isAcceptInvalidEnums()) 122 return null; 123 else 124 throw new FHIRException("Unknown EncounterStatus code '" + codeString + "'"); 125 } 126 127 public String toCode() { 128 switch (this) { 129 case PLANNED: 130 return "planned"; 131 case ARRIVED: 132 return "arrived"; 133 case TRIAGED: 134 return "triaged"; 135 case INPROGRESS: 136 return "in-progress"; 137 case ONLEAVE: 138 return "onleave"; 139 case FINISHED: 140 return "finished"; 141 case CANCELLED: 142 return "cancelled"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case UNKNOWN: 146 return "unknown"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PLANNED: 157 return "http://hl7.org/fhir/encounter-status"; 158 case ARRIVED: 159 return "http://hl7.org/fhir/encounter-status"; 160 case TRIAGED: 161 return "http://hl7.org/fhir/encounter-status"; 162 case INPROGRESS: 163 return "http://hl7.org/fhir/encounter-status"; 164 case ONLEAVE: 165 return "http://hl7.org/fhir/encounter-status"; 166 case FINISHED: 167 return "http://hl7.org/fhir/encounter-status"; 168 case CANCELLED: 169 return "http://hl7.org/fhir/encounter-status"; 170 case ENTEREDINERROR: 171 return "http://hl7.org/fhir/encounter-status"; 172 case UNKNOWN: 173 return "http://hl7.org/fhir/encounter-status"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDefinition() { 182 switch (this) { 183 case PLANNED: 184 return "The Encounter has not yet started."; 185 case ARRIVED: 186 return "The Patient is present for the encounter, however is not currently meeting with a practitioner."; 187 case TRIAGED: 188 return "The patient has been assessed for the priority of their treatment based on the severity of their condition."; 189 case INPROGRESS: 190 return "The Encounter has begun and the patient is present / the practitioner and the patient are meeting."; 191 case ONLEAVE: 192 return "The Encounter has begun, but the patient is temporarily on leave."; 193 case FINISHED: 194 return "The Encounter has ended."; 195 case CANCELLED: 196 return "The Encounter has ended before it has begun."; 197 case ENTEREDINERROR: 198 return "This instance should not have been part of this patient's medical record."; 199 case UNKNOWN: 200 return "The encounter status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getDisplay() { 209 switch (this) { 210 case PLANNED: 211 return "Planned"; 212 case ARRIVED: 213 return "Arrived"; 214 case TRIAGED: 215 return "Triaged"; 216 case INPROGRESS: 217 return "In Progress"; 218 case ONLEAVE: 219 return "On Leave"; 220 case FINISHED: 221 return "Finished"; 222 case CANCELLED: 223 return "Cancelled"; 224 case ENTEREDINERROR: 225 return "Entered in Error"; 226 case UNKNOWN: 227 return "Unknown"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class EncounterStatusEnumFactory implements EnumFactory<EncounterStatus> { 237 public EncounterStatus fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("planned".equals(codeString)) 242 return EncounterStatus.PLANNED; 243 if ("arrived".equals(codeString)) 244 return EncounterStatus.ARRIVED; 245 if ("triaged".equals(codeString)) 246 return EncounterStatus.TRIAGED; 247 if ("in-progress".equals(codeString)) 248 return EncounterStatus.INPROGRESS; 249 if ("onleave".equals(codeString)) 250 return EncounterStatus.ONLEAVE; 251 if ("finished".equals(codeString)) 252 return EncounterStatus.FINISHED; 253 if ("cancelled".equals(codeString)) 254 return EncounterStatus.CANCELLED; 255 if ("entered-in-error".equals(codeString)) 256 return EncounterStatus.ENTEREDINERROR; 257 if ("unknown".equals(codeString)) 258 return EncounterStatus.UNKNOWN; 259 throw new IllegalArgumentException("Unknown EncounterStatus code '" + codeString + "'"); 260 } 261 262 public Enumeration<EncounterStatus> fromType(PrimitiveType<?> code) throws FHIRException { 263 if (code == null) 264 return null; 265 if (code.isEmpty()) 266 return new Enumeration<EncounterStatus>(this, EncounterStatus.NULL, code); 267 String codeString = code.asStringValue(); 268 if (codeString == null || "".equals(codeString)) 269 return new Enumeration<EncounterStatus>(this, EncounterStatus.NULL, code); 270 if ("planned".equals(codeString)) 271 return new Enumeration<EncounterStatus>(this, EncounterStatus.PLANNED, code); 272 if ("arrived".equals(codeString)) 273 return new Enumeration<EncounterStatus>(this, EncounterStatus.ARRIVED, code); 274 if ("triaged".equals(codeString)) 275 return new Enumeration<EncounterStatus>(this, EncounterStatus.TRIAGED, code); 276 if ("in-progress".equals(codeString)) 277 return new Enumeration<EncounterStatus>(this, EncounterStatus.INPROGRESS, code); 278 if ("onleave".equals(codeString)) 279 return new Enumeration<EncounterStatus>(this, EncounterStatus.ONLEAVE, code); 280 if ("finished".equals(codeString)) 281 return new Enumeration<EncounterStatus>(this, EncounterStatus.FINISHED, code); 282 if ("cancelled".equals(codeString)) 283 return new Enumeration<EncounterStatus>(this, EncounterStatus.CANCELLED, code); 284 if ("entered-in-error".equals(codeString)) 285 return new Enumeration<EncounterStatus>(this, EncounterStatus.ENTEREDINERROR, code); 286 if ("unknown".equals(codeString)) 287 return new Enumeration<EncounterStatus>(this, EncounterStatus.UNKNOWN, code); 288 throw new FHIRException("Unknown EncounterStatus code '" + codeString + "'"); 289 } 290 291 public String toCode(EncounterStatus code) { 292 if (code == EncounterStatus.PLANNED) 293 return "planned"; 294 if (code == EncounterStatus.ARRIVED) 295 return "arrived"; 296 if (code == EncounterStatus.TRIAGED) 297 return "triaged"; 298 if (code == EncounterStatus.INPROGRESS) 299 return "in-progress"; 300 if (code == EncounterStatus.ONLEAVE) 301 return "onleave"; 302 if (code == EncounterStatus.FINISHED) 303 return "finished"; 304 if (code == EncounterStatus.CANCELLED) 305 return "cancelled"; 306 if (code == EncounterStatus.ENTEREDINERROR) 307 return "entered-in-error"; 308 if (code == EncounterStatus.UNKNOWN) 309 return "unknown"; 310 return "?"; 311 } 312 313 public String toSystem(EncounterStatus code) { 314 return code.getSystem(); 315 } 316 } 317 318 public enum EncounterLocationStatus { 319 /** 320 * The patient is planned to be moved to this location at some point in the 321 * future. 322 */ 323 PLANNED, 324 /** 325 * The patient is currently at this location, or was between the period 326 * specified. 327 * 328 * A system may update these records when the patient leaves the location to 329 * either reserved, or completed. 330 */ 331 ACTIVE, 332 /** 333 * This location is held empty for this patient. 334 */ 335 RESERVED, 336 /** 337 * The patient was at this location during the period specified. 338 * 339 * Not to be used when the patient is currently at the location. 340 */ 341 COMPLETED, 342 /** 343 * added to help the parsers with the generic types 344 */ 345 NULL; 346 347 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 348 if (codeString == null || "".equals(codeString)) 349 return null; 350 if ("planned".equals(codeString)) 351 return PLANNED; 352 if ("active".equals(codeString)) 353 return ACTIVE; 354 if ("reserved".equals(codeString)) 355 return RESERVED; 356 if ("completed".equals(codeString)) 357 return COMPLETED; 358 if (Configuration.isAcceptInvalidEnums()) 359 return null; 360 else 361 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 362 } 363 364 public String toCode() { 365 switch (this) { 366 case PLANNED: 367 return "planned"; 368 case ACTIVE: 369 return "active"; 370 case RESERVED: 371 return "reserved"; 372 case COMPLETED: 373 return "completed"; 374 case NULL: 375 return null; 376 default: 377 return "?"; 378 } 379 } 380 381 public String getSystem() { 382 switch (this) { 383 case PLANNED: 384 return "http://hl7.org/fhir/encounter-location-status"; 385 case ACTIVE: 386 return "http://hl7.org/fhir/encounter-location-status"; 387 case RESERVED: 388 return "http://hl7.org/fhir/encounter-location-status"; 389 case COMPLETED: 390 return "http://hl7.org/fhir/encounter-location-status"; 391 case NULL: 392 return null; 393 default: 394 return "?"; 395 } 396 } 397 398 public String getDefinition() { 399 switch (this) { 400 case PLANNED: 401 return "The patient is planned to be moved to this location at some point in the future."; 402 case ACTIVE: 403 return "The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed."; 404 case RESERVED: 405 return "This location is held empty for this patient."; 406 case COMPLETED: 407 return "The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location."; 408 case NULL: 409 return null; 410 default: 411 return "?"; 412 } 413 } 414 415 public String getDisplay() { 416 switch (this) { 417 case PLANNED: 418 return "Planned"; 419 case ACTIVE: 420 return "Active"; 421 case RESERVED: 422 return "Reserved"; 423 case COMPLETED: 424 return "Completed"; 425 case NULL: 426 return null; 427 default: 428 return "?"; 429 } 430 } 431 } 432 433 public static class EncounterLocationStatusEnumFactory implements EnumFactory<EncounterLocationStatus> { 434 public EncounterLocationStatus fromCode(String codeString) throws IllegalArgumentException { 435 if (codeString == null || "".equals(codeString)) 436 if (codeString == null || "".equals(codeString)) 437 return null; 438 if ("planned".equals(codeString)) 439 return EncounterLocationStatus.PLANNED; 440 if ("active".equals(codeString)) 441 return EncounterLocationStatus.ACTIVE; 442 if ("reserved".equals(codeString)) 443 return EncounterLocationStatus.RESERVED; 444 if ("completed".equals(codeString)) 445 return EncounterLocationStatus.COMPLETED; 446 throw new IllegalArgumentException("Unknown EncounterLocationStatus code '" + codeString + "'"); 447 } 448 449 public Enumeration<EncounterLocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 450 if (code == null) 451 return null; 452 if (code.isEmpty()) 453 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 454 String codeString = code.asStringValue(); 455 if (codeString == null || "".equals(codeString)) 456 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.NULL, code); 457 if ("planned".equals(codeString)) 458 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.PLANNED, code); 459 if ("active".equals(codeString)) 460 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.ACTIVE, code); 461 if ("reserved".equals(codeString)) 462 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.RESERVED, code); 463 if ("completed".equals(codeString)) 464 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.COMPLETED, code); 465 throw new FHIRException("Unknown EncounterLocationStatus code '" + codeString + "'"); 466 } 467 468 public String toCode(EncounterLocationStatus code) { 469 if (code == EncounterLocationStatus.PLANNED) 470 return "planned"; 471 if (code == EncounterLocationStatus.ACTIVE) 472 return "active"; 473 if (code == EncounterLocationStatus.RESERVED) 474 return "reserved"; 475 if (code == EncounterLocationStatus.COMPLETED) 476 return "completed"; 477 return "?"; 478 } 479 480 public String toSystem(EncounterLocationStatus code) { 481 return code.getSystem(); 482 } 483 } 484 485 @Block() 486 public static class StatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 487 /** 488 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 489 */ 490 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 491 @Description(shortDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.") 492 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-status") 493 protected Enumeration<EncounterStatus> status; 494 495 /** 496 * The time that the episode was in the specified status. 497 */ 498 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 499 @Description(shortDefinition = "The time that the episode was in the specified status", formalDefinition = "The time that the episode was in the specified status.") 500 protected Period period; 501 502 private static final long serialVersionUID = -1893906736L; 503 504 /** 505 * Constructor 506 */ 507 public StatusHistoryComponent() { 508 super(); 509 } 510 511 /** 512 * Constructor 513 */ 514 public StatusHistoryComponent(Enumeration<EncounterStatus> status, Period period) { 515 super(); 516 this.status = status; 517 this.period = period; 518 } 519 520 /** 521 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave 522 * | finished | cancelled +.). This is the underlying object with id, 523 * value and extensions. The accessor "getStatus" gives direct access to 524 * the value 525 */ 526 public Enumeration<EncounterStatus> getStatusElement() { 527 if (this.status == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create StatusHistoryComponent.status"); 530 else if (Configuration.doAutoCreate()) 531 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 532 return this.status; 533 } 534 535 public boolean hasStatusElement() { 536 return this.status != null && !this.status.isEmpty(); 537 } 538 539 public boolean hasStatus() { 540 return this.status != null && !this.status.isEmpty(); 541 } 542 543 /** 544 * @param value {@link #status} (planned | arrived | triaged | in-progress | 545 * onleave | finished | cancelled +.). This is the underlying 546 * object with id, value and extensions. The accessor "getStatus" 547 * gives direct access to the value 548 */ 549 public StatusHistoryComponent setStatusElement(Enumeration<EncounterStatus> value) { 550 this.status = value; 551 return this; 552 } 553 554 /** 555 * @return planned | arrived | triaged | in-progress | onleave | finished | 556 * cancelled +. 557 */ 558 public EncounterStatus getStatus() { 559 return this.status == null ? null : this.status.getValue(); 560 } 561 562 /** 563 * @param value planned | arrived | triaged | in-progress | onleave | finished | 564 * cancelled +. 565 */ 566 public StatusHistoryComponent setStatus(EncounterStatus value) { 567 if (this.status == null) 568 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 569 this.status.setValue(value); 570 return this; 571 } 572 573 /** 574 * @return {@link #period} (The time that the episode was in the specified 575 * status.) 576 */ 577 public Period getPeriod() { 578 if (this.period == null) 579 if (Configuration.errorOnAutoCreate()) 580 throw new Error("Attempt to auto-create StatusHistoryComponent.period"); 581 else if (Configuration.doAutoCreate()) 582 this.period = new Period(); // cc 583 return this.period; 584 } 585 586 public boolean hasPeriod() { 587 return this.period != null && !this.period.isEmpty(); 588 } 589 590 /** 591 * @param value {@link #period} (The time that the episode was in the specified 592 * status.) 593 */ 594 public StatusHistoryComponent setPeriod(Period value) { 595 this.period = value; 596 return this; 597 } 598 599 protected void listChildren(List<Property> children) { 600 super.listChildren(children); 601 children.add(new Property("status", "code", 602 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 603 children.add( 604 new Property("period", "Period", "The time that the episode was in the specified status.", 0, 1, period)); 605 } 606 607 @Override 608 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 609 switch (_hash) { 610 case -892481550: 611 /* status */ return new Property("status", "code", 612 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 613 case -991726143: 614 /* period */ return new Property("period", "Period", "The time that the episode was in the specified status.", 615 0, 1, period); 616 default: 617 return super.getNamedProperty(_hash, _name, _checkValid); 618 } 619 620 } 621 622 @Override 623 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 624 switch (hash) { 625 case -892481550: 626 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterStatus> 627 case -991726143: 628 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 629 default: 630 return super.getProperty(hash, name, checkValid); 631 } 632 633 } 634 635 @Override 636 public Base setProperty(int hash, String name, Base value) throws FHIRException { 637 switch (hash) { 638 case -892481550: // status 639 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 640 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 641 return value; 642 case -991726143: // period 643 this.period = castToPeriod(value); // Period 644 return value; 645 default: 646 return super.setProperty(hash, name, value); 647 } 648 649 } 650 651 @Override 652 public Base setProperty(String name, Base value) throws FHIRException { 653 if (name.equals("status")) { 654 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 655 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 656 } else if (name.equals("period")) { 657 this.period = castToPeriod(value); // Period 658 } else 659 return super.setProperty(name, value); 660 return value; 661 } 662 663 @Override 664 public Base makeProperty(int hash, String name) throws FHIRException { 665 switch (hash) { 666 case -892481550: 667 return getStatusElement(); 668 case -991726143: 669 return getPeriod(); 670 default: 671 return super.makeProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 678 switch (hash) { 679 case -892481550: 680 /* status */ return new String[] { "code" }; 681 case -991726143: 682 /* period */ return new String[] { "Period" }; 683 default: 684 return super.getTypesForProperty(hash, name); 685 } 686 687 } 688 689 @Override 690 public Base addChild(String name) throws FHIRException { 691 if (name.equals("status")) { 692 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 693 } else if (name.equals("period")) { 694 this.period = new Period(); 695 return this.period; 696 } else 697 return super.addChild(name); 698 } 699 700 public StatusHistoryComponent copy() { 701 StatusHistoryComponent dst = new StatusHistoryComponent(); 702 copyValues(dst); 703 return dst; 704 } 705 706 public void copyValues(StatusHistoryComponent dst) { 707 super.copyValues(dst); 708 dst.status = status == null ? null : status.copy(); 709 dst.period = period == null ? null : period.copy(); 710 } 711 712 @Override 713 public boolean equalsDeep(Base other_) { 714 if (!super.equalsDeep(other_)) 715 return false; 716 if (!(other_ instanceof StatusHistoryComponent)) 717 return false; 718 StatusHistoryComponent o = (StatusHistoryComponent) other_; 719 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 720 } 721 722 @Override 723 public boolean equalsShallow(Base other_) { 724 if (!super.equalsShallow(other_)) 725 return false; 726 if (!(other_ instanceof StatusHistoryComponent)) 727 return false; 728 StatusHistoryComponent o = (StatusHistoryComponent) other_; 729 return compareValues(status, o.status, true); 730 } 731 732 public boolean isEmpty() { 733 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 734 } 735 736 public String fhirType() { 737 return "Encounter.statusHistory"; 738 739 } 740 741 } 742 743 @Block() 744 public static class ClassHistoryComponent extends BackboneElement implements IBaseBackboneElement { 745 /** 746 * inpatient | outpatient | ambulatory | emergency +. 747 */ 748 @Child(name = "class", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 749 @Description(shortDefinition = "inpatient | outpatient | ambulatory | emergency +", formalDefinition = "inpatient | outpatient | ambulatory | emergency +.") 750 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActEncounterCode") 751 protected Coding class_; 752 753 /** 754 * The time that the episode was in the specified class. 755 */ 756 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 757 @Description(shortDefinition = "The time that the episode was in the specified class", formalDefinition = "The time that the episode was in the specified class.") 758 protected Period period; 759 760 private static final long serialVersionUID = 1331020311L; 761 762 /** 763 * Constructor 764 */ 765 public ClassHistoryComponent() { 766 super(); 767 } 768 769 /** 770 * Constructor 771 */ 772 public ClassHistoryComponent(Coding class_, Period period) { 773 super(); 774 this.class_ = class_; 775 this.period = period; 776 } 777 778 /** 779 * @return {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 780 */ 781 public Coding getClass_() { 782 if (this.class_ == null) 783 if (Configuration.errorOnAutoCreate()) 784 throw new Error("Attempt to auto-create ClassHistoryComponent.class_"); 785 else if (Configuration.doAutoCreate()) 786 this.class_ = new Coding(); // cc 787 return this.class_; 788 } 789 790 public boolean hasClass_() { 791 return this.class_ != null && !this.class_.isEmpty(); 792 } 793 794 /** 795 * @param value {@link #class_} (inpatient | outpatient | ambulatory | emergency 796 * +.) 797 */ 798 public ClassHistoryComponent setClass_(Coding value) { 799 this.class_ = value; 800 return this; 801 } 802 803 /** 804 * @return {@link #period} (The time that the episode was in the specified 805 * class.) 806 */ 807 public Period getPeriod() { 808 if (this.period == null) 809 if (Configuration.errorOnAutoCreate()) 810 throw new Error("Attempt to auto-create ClassHistoryComponent.period"); 811 else if (Configuration.doAutoCreate()) 812 this.period = new Period(); // cc 813 return this.period; 814 } 815 816 public boolean hasPeriod() { 817 return this.period != null && !this.period.isEmpty(); 818 } 819 820 /** 821 * @param value {@link #period} (The time that the episode was in the specified 822 * class.) 823 */ 824 public ClassHistoryComponent setPeriod(Period value) { 825 this.period = value; 826 return this; 827 } 828 829 protected void listChildren(List<Property> children) { 830 super.listChildren(children); 831 children.add(new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_)); 832 children 833 .add(new Property("period", "Period", "The time that the episode was in the specified class.", 0, 1, period)); 834 } 835 836 @Override 837 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 838 switch (_hash) { 839 case 94742904: 840 /* class */ return new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, 841 class_); 842 case -991726143: 843 /* period */ return new Property("period", "Period", "The time that the episode was in the specified class.", 0, 844 1, period); 845 default: 846 return super.getNamedProperty(_hash, _name, _checkValid); 847 } 848 849 } 850 851 @Override 852 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 853 switch (hash) { 854 case 94742904: 855 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // Coding 856 case -991726143: 857 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 858 default: 859 return super.getProperty(hash, name, checkValid); 860 } 861 862 } 863 864 @Override 865 public Base setProperty(int hash, String name, Base value) throws FHIRException { 866 switch (hash) { 867 case 94742904: // class 868 this.class_ = castToCoding(value); // Coding 869 return value; 870 case -991726143: // period 871 this.period = castToPeriod(value); // Period 872 return value; 873 default: 874 return super.setProperty(hash, name, value); 875 } 876 877 } 878 879 @Override 880 public Base setProperty(String name, Base value) throws FHIRException { 881 if (name.equals("class")) { 882 this.class_ = castToCoding(value); // Coding 883 } else if (name.equals("period")) { 884 this.period = castToPeriod(value); // Period 885 } else 886 return super.setProperty(name, value); 887 return value; 888 } 889 890 @Override 891 public Base makeProperty(int hash, String name) throws FHIRException { 892 switch (hash) { 893 case 94742904: 894 return getClass_(); 895 case -991726143: 896 return getPeriod(); 897 default: 898 return super.makeProperty(hash, name); 899 } 900 901 } 902 903 @Override 904 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 905 switch (hash) { 906 case 94742904: 907 /* class */ return new String[] { "Coding" }; 908 case -991726143: 909 /* period */ return new String[] { "Period" }; 910 default: 911 return super.getTypesForProperty(hash, name); 912 } 913 914 } 915 916 @Override 917 public Base addChild(String name) throws FHIRException { 918 if (name.equals("class")) { 919 this.class_ = new Coding(); 920 return this.class_; 921 } else if (name.equals("period")) { 922 this.period = new Period(); 923 return this.period; 924 } else 925 return super.addChild(name); 926 } 927 928 public ClassHistoryComponent copy() { 929 ClassHistoryComponent dst = new ClassHistoryComponent(); 930 copyValues(dst); 931 return dst; 932 } 933 934 public void copyValues(ClassHistoryComponent dst) { 935 super.copyValues(dst); 936 dst.class_ = class_ == null ? null : class_.copy(); 937 dst.period = period == null ? null : period.copy(); 938 } 939 940 @Override 941 public boolean equalsDeep(Base other_) { 942 if (!super.equalsDeep(other_)) 943 return false; 944 if (!(other_ instanceof ClassHistoryComponent)) 945 return false; 946 ClassHistoryComponent o = (ClassHistoryComponent) other_; 947 return compareDeep(class_, o.class_, true) && compareDeep(period, o.period, true); 948 } 949 950 @Override 951 public boolean equalsShallow(Base other_) { 952 if (!super.equalsShallow(other_)) 953 return false; 954 if (!(other_ instanceof ClassHistoryComponent)) 955 return false; 956 ClassHistoryComponent o = (ClassHistoryComponent) other_; 957 return true; 958 } 959 960 public boolean isEmpty() { 961 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(class_, period); 962 } 963 964 public String fhirType() { 965 return "Encounter.classHistory"; 966 967 } 968 969 } 970 971 @Block() 972 public static class EncounterParticipantComponent extends BackboneElement implements IBaseBackboneElement { 973 /** 974 * Role of participant in encounter. 975 */ 976 @Child(name = "type", type = { 977 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 978 @Description(shortDefinition = "Role of participant in encounter", formalDefinition = "Role of participant in encounter.") 979 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-participant-type") 980 protected List<CodeableConcept> type; 981 982 /** 983 * The period of time that the specified participant participated in the 984 * encounter. These can overlap or be sub-sets of the overall encounter's 985 * period. 986 */ 987 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 988 @Description(shortDefinition = "Period of time during the encounter that the participant participated", formalDefinition = "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.") 989 protected Period period; 990 991 /** 992 * Persons involved in the encounter other than the patient. 993 */ 994 @Child(name = "individual", type = { Practitioner.class, PractitionerRole.class, 995 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 996 @Description(shortDefinition = "Persons involved in the encounter other than the patient", formalDefinition = "Persons involved in the encounter other than the patient.") 997 protected Reference individual; 998 999 /** 1000 * The actual object that is the target of the reference (Persons involved in 1001 * the encounter other than the patient.) 1002 */ 1003 protected Resource individualTarget; 1004 1005 private static final long serialVersionUID = 317095765L; 1006 1007 /** 1008 * Constructor 1009 */ 1010 public EncounterParticipantComponent() { 1011 super(); 1012 } 1013 1014 /** 1015 * @return {@link #type} (Role of participant in encounter.) 1016 */ 1017 public List<CodeableConcept> getType() { 1018 if (this.type == null) 1019 this.type = new ArrayList<CodeableConcept>(); 1020 return this.type; 1021 } 1022 1023 /** 1024 * @return Returns a reference to <code>this</code> for easy method chaining 1025 */ 1026 public EncounterParticipantComponent setType(List<CodeableConcept> theType) { 1027 this.type = theType; 1028 return this; 1029 } 1030 1031 public boolean hasType() { 1032 if (this.type == null) 1033 return false; 1034 for (CodeableConcept item : this.type) 1035 if (!item.isEmpty()) 1036 return true; 1037 return false; 1038 } 1039 1040 public CodeableConcept addType() { // 3 1041 CodeableConcept t = new CodeableConcept(); 1042 if (this.type == null) 1043 this.type = new ArrayList<CodeableConcept>(); 1044 this.type.add(t); 1045 return t; 1046 } 1047 1048 public EncounterParticipantComponent addType(CodeableConcept t) { // 3 1049 if (t == null) 1050 return this; 1051 if (this.type == null) 1052 this.type = new ArrayList<CodeableConcept>(); 1053 this.type.add(t); 1054 return this; 1055 } 1056 1057 /** 1058 * @return The first repetition of repeating field {@link #type}, creating it if 1059 * it does not already exist 1060 */ 1061 public CodeableConcept getTypeFirstRep() { 1062 if (getType().isEmpty()) { 1063 addType(); 1064 } 1065 return getType().get(0); 1066 } 1067 1068 /** 1069 * @return {@link #period} (The period of time that the specified participant 1070 * participated in the encounter. These can overlap or be sub-sets of 1071 * the overall encounter's period.) 1072 */ 1073 public Period getPeriod() { 1074 if (this.period == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create EncounterParticipantComponent.period"); 1077 else if (Configuration.doAutoCreate()) 1078 this.period = new Period(); // cc 1079 return this.period; 1080 } 1081 1082 public boolean hasPeriod() { 1083 return this.period != null && !this.period.isEmpty(); 1084 } 1085 1086 /** 1087 * @param value {@link #period} (The period of time that the specified 1088 * participant participated in the encounter. These can overlap or 1089 * be sub-sets of the overall encounter's period.) 1090 */ 1091 public EncounterParticipantComponent setPeriod(Period value) { 1092 this.period = value; 1093 return this; 1094 } 1095 1096 /** 1097 * @return {@link #individual} (Persons involved in the encounter other than the 1098 * patient.) 1099 */ 1100 public Reference getIndividual() { 1101 if (this.individual == null) 1102 if (Configuration.errorOnAutoCreate()) 1103 throw new Error("Attempt to auto-create EncounterParticipantComponent.individual"); 1104 else if (Configuration.doAutoCreate()) 1105 this.individual = new Reference(); // cc 1106 return this.individual; 1107 } 1108 1109 public boolean hasIndividual() { 1110 return this.individual != null && !this.individual.isEmpty(); 1111 } 1112 1113 /** 1114 * @param value {@link #individual} (Persons involved in the encounter other 1115 * than the patient.) 1116 */ 1117 public EncounterParticipantComponent setIndividual(Reference value) { 1118 this.individual = value; 1119 return this; 1120 } 1121 1122 /** 1123 * @return {@link #individual} The actual object that is the target of the 1124 * reference. The reference library doesn't populate this, but you can 1125 * use it to hold the resource if you resolve it. (Persons involved in 1126 * the encounter other than the patient.) 1127 */ 1128 public Resource getIndividualTarget() { 1129 return this.individualTarget; 1130 } 1131 1132 /** 1133 * @param value {@link #individual} The actual object that is the target of the 1134 * reference. The reference library doesn't use these, but you can 1135 * use it to hold the resource if you resolve it. (Persons involved 1136 * in the encounter other than the patient.) 1137 */ 1138 public EncounterParticipantComponent setIndividualTarget(Resource value) { 1139 this.individualTarget = value; 1140 return this; 1141 } 1142 1143 protected void listChildren(List<Property> children) { 1144 super.listChildren(children); 1145 children.add(new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, 1146 java.lang.Integer.MAX_VALUE, type)); 1147 children.add(new Property("period", "Period", 1148 "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 1149 0, 1, period)); 1150 children.add(new Property("individual", "Reference(Practitioner|PractitionerRole|RelatedPerson)", 1151 "Persons involved in the encounter other than the patient.", 0, 1, individual)); 1152 } 1153 1154 @Override 1155 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1156 switch (_hash) { 1157 case 3575610: 1158 /* type */ return new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, 1159 java.lang.Integer.MAX_VALUE, type); 1160 case -991726143: 1161 /* period */ return new Property("period", "Period", 1162 "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 1163 0, 1, period); 1164 case -46292327: 1165 /* individual */ return new Property("individual", "Reference(Practitioner|PractitionerRole|RelatedPerson)", 1166 "Persons involved in the encounter other than the patient.", 0, 1, individual); 1167 default: 1168 return super.getNamedProperty(_hash, _name, _checkValid); 1169 } 1170 1171 } 1172 1173 @Override 1174 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1175 switch (hash) { 1176 case 3575610: 1177 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1178 case -991726143: 1179 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1180 case -46292327: 1181 /* individual */ return this.individual == null ? new Base[0] : new Base[] { this.individual }; // Reference 1182 default: 1183 return super.getProperty(hash, name, checkValid); 1184 } 1185 1186 } 1187 1188 @Override 1189 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1190 switch (hash) { 1191 case 3575610: // type 1192 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1193 return value; 1194 case -991726143: // period 1195 this.period = castToPeriod(value); // Period 1196 return value; 1197 case -46292327: // individual 1198 this.individual = castToReference(value); // Reference 1199 return value; 1200 default: 1201 return super.setProperty(hash, name, value); 1202 } 1203 1204 } 1205 1206 @Override 1207 public Base setProperty(String name, Base value) throws FHIRException { 1208 if (name.equals("type")) { 1209 this.getType().add(castToCodeableConcept(value)); 1210 } else if (name.equals("period")) { 1211 this.period = castToPeriod(value); // Period 1212 } else if (name.equals("individual")) { 1213 this.individual = castToReference(value); // Reference 1214 } else 1215 return super.setProperty(name, value); 1216 return value; 1217 } 1218 1219 @Override 1220 public Base makeProperty(int hash, String name) throws FHIRException { 1221 switch (hash) { 1222 case 3575610: 1223 return addType(); 1224 case -991726143: 1225 return getPeriod(); 1226 case -46292327: 1227 return getIndividual(); 1228 default: 1229 return super.makeProperty(hash, name); 1230 } 1231 1232 } 1233 1234 @Override 1235 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1236 switch (hash) { 1237 case 3575610: 1238 /* type */ return new String[] { "CodeableConcept" }; 1239 case -991726143: 1240 /* period */ return new String[] { "Period" }; 1241 case -46292327: 1242 /* individual */ return new String[] { "Reference" }; 1243 default: 1244 return super.getTypesForProperty(hash, name); 1245 } 1246 1247 } 1248 1249 @Override 1250 public Base addChild(String name) throws FHIRException { 1251 if (name.equals("type")) { 1252 return addType(); 1253 } else if (name.equals("period")) { 1254 this.period = new Period(); 1255 return this.period; 1256 } else if (name.equals("individual")) { 1257 this.individual = new Reference(); 1258 return this.individual; 1259 } else 1260 return super.addChild(name); 1261 } 1262 1263 public EncounterParticipantComponent copy() { 1264 EncounterParticipantComponent dst = new EncounterParticipantComponent(); 1265 copyValues(dst); 1266 return dst; 1267 } 1268 1269 public void copyValues(EncounterParticipantComponent dst) { 1270 super.copyValues(dst); 1271 if (type != null) { 1272 dst.type = new ArrayList<CodeableConcept>(); 1273 for (CodeableConcept i : type) 1274 dst.type.add(i.copy()); 1275 } 1276 ; 1277 dst.period = period == null ? null : period.copy(); 1278 dst.individual = individual == null ? null : individual.copy(); 1279 } 1280 1281 @Override 1282 public boolean equalsDeep(Base other_) { 1283 if (!super.equalsDeep(other_)) 1284 return false; 1285 if (!(other_ instanceof EncounterParticipantComponent)) 1286 return false; 1287 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1288 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) 1289 && compareDeep(individual, o.individual, true); 1290 } 1291 1292 @Override 1293 public boolean equalsShallow(Base other_) { 1294 if (!super.equalsShallow(other_)) 1295 return false; 1296 if (!(other_ instanceof EncounterParticipantComponent)) 1297 return false; 1298 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1299 return true; 1300 } 1301 1302 public boolean isEmpty() { 1303 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, individual); 1304 } 1305 1306 public String fhirType() { 1307 return "Encounter.participant"; 1308 1309 } 1310 1311 } 1312 1313 @Block() 1314 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1315 /** 1316 * Reason the encounter takes place, as specified using information from another 1317 * resource. For admissions, this is the admission diagnosis. The indication 1318 * will typically be a Condition (with other resources referenced in the 1319 * evidence.detail), or a Procedure. 1320 */ 1321 @Child(name = "condition", type = { Condition.class, 1322 Procedure.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1323 @Description(shortDefinition = "The diagnosis or procedure relevant to the encounter", formalDefinition = "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.") 1324 protected Reference condition; 1325 1326 /** 1327 * The actual object that is the target of the reference (Reason the encounter 1328 * takes place, as specified using information from another resource. For 1329 * admissions, this is the admission diagnosis. The indication will typically be 1330 * a Condition (with other resources referenced in the evidence.detail), or a 1331 * Procedure.) 1332 */ 1333 protected Resource conditionTarget; 1334 1335 /** 1336 * Role that this diagnosis has within the encounter (e.g. admission, billing, 1337 * discharge ?). 1338 */ 1339 @Child(name = "use", type = { 1340 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1341 @Description(shortDefinition = "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)", formalDefinition = "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).") 1342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnosis-role") 1343 protected CodeableConcept use; 1344 1345 /** 1346 * Ranking of the diagnosis (for each role type). 1347 */ 1348 @Child(name = "rank", type = { 1349 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1350 @Description(shortDefinition = "Ranking of the diagnosis (for each role type)", formalDefinition = "Ranking of the diagnosis (for each role type).") 1351 protected PositiveIntType rank; 1352 1353 private static final long serialVersionUID = 128213376L; 1354 1355 /** 1356 * Constructor 1357 */ 1358 public DiagnosisComponent() { 1359 super(); 1360 } 1361 1362 /** 1363 * Constructor 1364 */ 1365 public DiagnosisComponent(Reference condition) { 1366 super(); 1367 this.condition = condition; 1368 } 1369 1370 /** 1371 * @return {@link #condition} (Reason the encounter takes place, as specified 1372 * using information from another resource. For admissions, this is the 1373 * admission diagnosis. The indication will typically be a Condition 1374 * (with other resources referenced in the evidence.detail), or a 1375 * Procedure.) 1376 */ 1377 public Reference getCondition() { 1378 if (this.condition == null) 1379 if (Configuration.errorOnAutoCreate()) 1380 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 1381 else if (Configuration.doAutoCreate()) 1382 this.condition = new Reference(); // cc 1383 return this.condition; 1384 } 1385 1386 public boolean hasCondition() { 1387 return this.condition != null && !this.condition.isEmpty(); 1388 } 1389 1390 /** 1391 * @param value {@link #condition} (Reason the encounter takes place, as 1392 * specified using information from another resource. For 1393 * admissions, this is the admission diagnosis. The indication will 1394 * typically be a Condition (with other resources referenced in the 1395 * evidence.detail), or a Procedure.) 1396 */ 1397 public DiagnosisComponent setCondition(Reference value) { 1398 this.condition = value; 1399 return this; 1400 } 1401 1402 /** 1403 * @return {@link #condition} The actual object that is the target of the 1404 * reference. The reference library doesn't populate this, but you can 1405 * use it to hold the resource if you resolve it. (Reason the encounter 1406 * takes place, as specified using information from another resource. 1407 * For admissions, this is the admission diagnosis. The indication will 1408 * typically be a Condition (with other resources referenced in the 1409 * evidence.detail), or a Procedure.) 1410 */ 1411 public Resource getConditionTarget() { 1412 return this.conditionTarget; 1413 } 1414 1415 /** 1416 * @param value {@link #condition} The actual object that is the target of the 1417 * reference. The reference library doesn't use these, but you can 1418 * use it to hold the resource if you resolve it. (Reason the 1419 * encounter takes place, as specified using information from 1420 * another resource. For admissions, this is the admission 1421 * diagnosis. The indication will typically be a Condition (with 1422 * other resources referenced in the evidence.detail), or a 1423 * Procedure.) 1424 */ 1425 public DiagnosisComponent setConditionTarget(Resource value) { 1426 this.conditionTarget = value; 1427 return this; 1428 } 1429 1430 /** 1431 * @return {@link #use} (Role that this diagnosis has within the encounter (e.g. 1432 * admission, billing, discharge ?).) 1433 */ 1434 public CodeableConcept getUse() { 1435 if (this.use == null) 1436 if (Configuration.errorOnAutoCreate()) 1437 throw new Error("Attempt to auto-create DiagnosisComponent.use"); 1438 else if (Configuration.doAutoCreate()) 1439 this.use = new CodeableConcept(); // cc 1440 return this.use; 1441 } 1442 1443 public boolean hasUse() { 1444 return this.use != null && !this.use.isEmpty(); 1445 } 1446 1447 /** 1448 * @param value {@link #use} (Role that this diagnosis has within the encounter 1449 * (e.g. admission, billing, discharge ?).) 1450 */ 1451 public DiagnosisComponent setUse(CodeableConcept value) { 1452 this.use = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This 1458 * is the underlying object with id, value and extensions. The accessor 1459 * "getRank" gives direct access to the value 1460 */ 1461 public PositiveIntType getRankElement() { 1462 if (this.rank == null) 1463 if (Configuration.errorOnAutoCreate()) 1464 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 1465 else if (Configuration.doAutoCreate()) 1466 this.rank = new PositiveIntType(); // bb 1467 return this.rank; 1468 } 1469 1470 public boolean hasRankElement() { 1471 return this.rank != null && !this.rank.isEmpty(); 1472 } 1473 1474 public boolean hasRank() { 1475 return this.rank != null && !this.rank.isEmpty(); 1476 } 1477 1478 /** 1479 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). 1480 * This is the underlying object with id, value and extensions. The 1481 * accessor "getRank" gives direct access to the value 1482 */ 1483 public DiagnosisComponent setRankElement(PositiveIntType value) { 1484 this.rank = value; 1485 return this; 1486 } 1487 1488 /** 1489 * @return Ranking of the diagnosis (for each role type). 1490 */ 1491 public int getRank() { 1492 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 1493 } 1494 1495 /** 1496 * @param value Ranking of the diagnosis (for each role type). 1497 */ 1498 public DiagnosisComponent setRank(int value) { 1499 if (this.rank == null) 1500 this.rank = new PositiveIntType(); 1501 this.rank.setValue(value); 1502 return this; 1503 } 1504 1505 protected void listChildren(List<Property> children) { 1506 super.listChildren(children); 1507 children.add(new Property("condition", "Reference(Condition|Procedure)", 1508 "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 1509 0, 1, condition)); 1510 children.add(new Property("use", "CodeableConcept", 1511 "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, use)); 1512 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 1513 } 1514 1515 @Override 1516 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1517 switch (_hash) { 1518 case -861311717: 1519 /* condition */ return new Property("condition", "Reference(Condition|Procedure)", 1520 "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 1521 0, 1, condition); 1522 case 116103: 1523 /* use */ return new Property("use", "CodeableConcept", 1524 "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, use); 1525 case 3492908: 1526 /* rank */ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, 1527 rank); 1528 default: 1529 return super.getNamedProperty(_hash, _name, _checkValid); 1530 } 1531 1532 } 1533 1534 @Override 1535 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1536 switch (hash) { 1537 case -861311717: 1538 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 1539 case 116103: 1540 /* use */ return this.use == null ? new Base[0] : new Base[] { this.use }; // CodeableConcept 1541 case 3492908: 1542 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 1543 default: 1544 return super.getProperty(hash, name, checkValid); 1545 } 1546 1547 } 1548 1549 @Override 1550 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1551 switch (hash) { 1552 case -861311717: // condition 1553 this.condition = castToReference(value); // Reference 1554 return value; 1555 case 116103: // use 1556 this.use = castToCodeableConcept(value); // CodeableConcept 1557 return value; 1558 case 3492908: // rank 1559 this.rank = castToPositiveInt(value); // PositiveIntType 1560 return value; 1561 default: 1562 return super.setProperty(hash, name, value); 1563 } 1564 1565 } 1566 1567 @Override 1568 public Base setProperty(String name, Base value) throws FHIRException { 1569 if (name.equals("condition")) { 1570 this.condition = castToReference(value); // Reference 1571 } else if (name.equals("use")) { 1572 this.use = castToCodeableConcept(value); // CodeableConcept 1573 } else if (name.equals("rank")) { 1574 this.rank = castToPositiveInt(value); // PositiveIntType 1575 } else 1576 return super.setProperty(name, value); 1577 return value; 1578 } 1579 1580 @Override 1581 public Base makeProperty(int hash, String name) throws FHIRException { 1582 switch (hash) { 1583 case -861311717: 1584 return getCondition(); 1585 case 116103: 1586 return getUse(); 1587 case 3492908: 1588 return getRankElement(); 1589 default: 1590 return super.makeProperty(hash, name); 1591 } 1592 1593 } 1594 1595 @Override 1596 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1597 switch (hash) { 1598 case -861311717: 1599 /* condition */ return new String[] { "Reference" }; 1600 case 116103: 1601 /* use */ return new String[] { "CodeableConcept" }; 1602 case 3492908: 1603 /* rank */ return new String[] { "positiveInt" }; 1604 default: 1605 return super.getTypesForProperty(hash, name); 1606 } 1607 1608 } 1609 1610 @Override 1611 public Base addChild(String name) throws FHIRException { 1612 if (name.equals("condition")) { 1613 this.condition = new Reference(); 1614 return this.condition; 1615 } else if (name.equals("use")) { 1616 this.use = new CodeableConcept(); 1617 return this.use; 1618 } else if (name.equals("rank")) { 1619 throw new FHIRException("Cannot call addChild on a singleton property Encounter.rank"); 1620 } else 1621 return super.addChild(name); 1622 } 1623 1624 public DiagnosisComponent copy() { 1625 DiagnosisComponent dst = new DiagnosisComponent(); 1626 copyValues(dst); 1627 return dst; 1628 } 1629 1630 public void copyValues(DiagnosisComponent dst) { 1631 super.copyValues(dst); 1632 dst.condition = condition == null ? null : condition.copy(); 1633 dst.use = use == null ? null : use.copy(); 1634 dst.rank = rank == null ? null : rank.copy(); 1635 } 1636 1637 @Override 1638 public boolean equalsDeep(Base other_) { 1639 if (!super.equalsDeep(other_)) 1640 return false; 1641 if (!(other_ instanceof DiagnosisComponent)) 1642 return false; 1643 DiagnosisComponent o = (DiagnosisComponent) other_; 1644 return compareDeep(condition, o.condition, true) && compareDeep(use, o.use, true) 1645 && compareDeep(rank, o.rank, true); 1646 } 1647 1648 @Override 1649 public boolean equalsShallow(Base other_) { 1650 if (!super.equalsShallow(other_)) 1651 return false; 1652 if (!(other_ instanceof DiagnosisComponent)) 1653 return false; 1654 DiagnosisComponent o = (DiagnosisComponent) other_; 1655 return compareValues(rank, o.rank, true); 1656 } 1657 1658 public boolean isEmpty() { 1659 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, use, rank); 1660 } 1661 1662 public String fhirType() { 1663 return "Encounter.diagnosis"; 1664 1665 } 1666 1667 } 1668 1669 @Block() 1670 public static class EncounterHospitalizationComponent extends BackboneElement implements IBaseBackboneElement { 1671 /** 1672 * Pre-admission identifier. 1673 */ 1674 @Child(name = "preAdmissionIdentifier", type = { 1675 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1676 @Description(shortDefinition = "Pre-admission identifier", formalDefinition = "Pre-admission identifier.") 1677 protected Identifier preAdmissionIdentifier; 1678 1679 /** 1680 * The location/organization from which the patient came before admission. 1681 */ 1682 @Child(name = "origin", type = { Location.class, 1683 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1684 @Description(shortDefinition = "The location/organization from which the patient came before admission", formalDefinition = "The location/organization from which the patient came before admission.") 1685 protected Reference origin; 1686 1687 /** 1688 * The actual object that is the target of the reference (The 1689 * location/organization from which the patient came before admission.) 1690 */ 1691 protected Resource originTarget; 1692 1693 /** 1694 * From where patient was admitted (physician referral, transfer). 1695 */ 1696 @Child(name = "admitSource", type = { 1697 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1698 @Description(shortDefinition = "From where patient was admitted (physician referral, transfer)", formalDefinition = "From where patient was admitted (physician referral, transfer).") 1699 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-admit-source") 1700 protected CodeableConcept admitSource; 1701 1702 /** 1703 * Whether this hospitalization is a readmission and why if known. 1704 */ 1705 @Child(name = "reAdmission", type = { 1706 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1707 @Description(shortDefinition = "The type of hospital re-admission that has occurred (if any). If the value is absent, then this is not identified as a readmission", formalDefinition = "Whether this hospitalization is a readmission and why if known.") 1708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0092") 1709 protected CodeableConcept reAdmission; 1710 1711 /** 1712 * Diet preferences reported by the patient. 1713 */ 1714 @Child(name = "dietPreference", type = { 1715 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1716 @Description(shortDefinition = "Diet preferences reported by the patient", formalDefinition = "Diet preferences reported by the patient.") 1717 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-diet") 1718 protected List<CodeableConcept> dietPreference; 1719 1720 /** 1721 * Special courtesies (VIP, board member). 1722 */ 1723 @Child(name = "specialCourtesy", type = { 1724 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1725 @Description(shortDefinition = "Special courtesies (VIP, board member)", formalDefinition = "Special courtesies (VIP, board member).") 1726 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-special-courtesy") 1727 protected List<CodeableConcept> specialCourtesy; 1728 1729 /** 1730 * Any special requests that have been made for this hospitalization encounter, 1731 * such as the provision of specific equipment or other things. 1732 */ 1733 @Child(name = "specialArrangement", type = { 1734 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1735 @Description(shortDefinition = "Wheelchair, translator, stretcher, etc.", formalDefinition = "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.") 1736 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-special-arrangements") 1737 protected List<CodeableConcept> specialArrangement; 1738 1739 /** 1740 * Location/organization to which the patient is discharged. 1741 */ 1742 @Child(name = "destination", type = { Location.class, 1743 Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1744 @Description(shortDefinition = "Location/organization to which the patient is discharged", formalDefinition = "Location/organization to which the patient is discharged.") 1745 protected Reference destination; 1746 1747 /** 1748 * The actual object that is the target of the reference (Location/organization 1749 * to which the patient is discharged.) 1750 */ 1751 protected Resource destinationTarget; 1752 1753 /** 1754 * Category or kind of location after discharge. 1755 */ 1756 @Child(name = "dischargeDisposition", type = { 1757 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1758 @Description(shortDefinition = "Category or kind of location after discharge", formalDefinition = "Category or kind of location after discharge.") 1759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-discharge-disposition") 1760 protected CodeableConcept dischargeDisposition; 1761 1762 private static final long serialVersionUID = 1350555270L; 1763 1764 /** 1765 * Constructor 1766 */ 1767 public EncounterHospitalizationComponent() { 1768 super(); 1769 } 1770 1771 /** 1772 * @return {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1773 */ 1774 public Identifier getPreAdmissionIdentifier() { 1775 if (this.preAdmissionIdentifier == null) 1776 if (Configuration.errorOnAutoCreate()) 1777 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.preAdmissionIdentifier"); 1778 else if (Configuration.doAutoCreate()) 1779 this.preAdmissionIdentifier = new Identifier(); // cc 1780 return this.preAdmissionIdentifier; 1781 } 1782 1783 public boolean hasPreAdmissionIdentifier() { 1784 return this.preAdmissionIdentifier != null && !this.preAdmissionIdentifier.isEmpty(); 1785 } 1786 1787 /** 1788 * @param value {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1789 */ 1790 public EncounterHospitalizationComponent setPreAdmissionIdentifier(Identifier value) { 1791 this.preAdmissionIdentifier = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #origin} (The location/organization from which the patient 1797 * came before admission.) 1798 */ 1799 public Reference getOrigin() { 1800 if (this.origin == null) 1801 if (Configuration.errorOnAutoCreate()) 1802 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1803 else if (Configuration.doAutoCreate()) 1804 this.origin = new Reference(); // cc 1805 return this.origin; 1806 } 1807 1808 public boolean hasOrigin() { 1809 return this.origin != null && !this.origin.isEmpty(); 1810 } 1811 1812 /** 1813 * @param value {@link #origin} (The location/organization from which the 1814 * patient came before admission.) 1815 */ 1816 public EncounterHospitalizationComponent setOrigin(Reference value) { 1817 this.origin = value; 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #origin} The actual object that is the target of the 1823 * reference. The reference library doesn't populate this, but you can 1824 * use it to hold the resource if you resolve it. (The 1825 * location/organization from which the patient came before admission.) 1826 */ 1827 public Resource getOriginTarget() { 1828 return this.originTarget; 1829 } 1830 1831 /** 1832 * @param value {@link #origin} The actual object that is the target of the 1833 * reference. The reference library doesn't use these, but you can 1834 * use it to hold the resource if you resolve it. (The 1835 * location/organization from which the patient came before 1836 * admission.) 1837 */ 1838 public EncounterHospitalizationComponent setOriginTarget(Resource value) { 1839 this.originTarget = value; 1840 return this; 1841 } 1842 1843 /** 1844 * @return {@link #admitSource} (From where patient was admitted (physician 1845 * referral, transfer).) 1846 */ 1847 public CodeableConcept getAdmitSource() { 1848 if (this.admitSource == null) 1849 if (Configuration.errorOnAutoCreate()) 1850 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.admitSource"); 1851 else if (Configuration.doAutoCreate()) 1852 this.admitSource = new CodeableConcept(); // cc 1853 return this.admitSource; 1854 } 1855 1856 public boolean hasAdmitSource() { 1857 return this.admitSource != null && !this.admitSource.isEmpty(); 1858 } 1859 1860 /** 1861 * @param value {@link #admitSource} (From where patient was admitted (physician 1862 * referral, transfer).) 1863 */ 1864 public EncounterHospitalizationComponent setAdmitSource(CodeableConcept value) { 1865 this.admitSource = value; 1866 return this; 1867 } 1868 1869 /** 1870 * @return {@link #reAdmission} (Whether this hospitalization is a readmission 1871 * and why if known.) 1872 */ 1873 public CodeableConcept getReAdmission() { 1874 if (this.reAdmission == null) 1875 if (Configuration.errorOnAutoCreate()) 1876 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.reAdmission"); 1877 else if (Configuration.doAutoCreate()) 1878 this.reAdmission = new CodeableConcept(); // cc 1879 return this.reAdmission; 1880 } 1881 1882 public boolean hasReAdmission() { 1883 return this.reAdmission != null && !this.reAdmission.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #reAdmission} (Whether this hospitalization is a 1888 * readmission and why if known.) 1889 */ 1890 public EncounterHospitalizationComponent setReAdmission(CodeableConcept value) { 1891 this.reAdmission = value; 1892 return this; 1893 } 1894 1895 /** 1896 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 1897 */ 1898 public List<CodeableConcept> getDietPreference() { 1899 if (this.dietPreference == null) 1900 this.dietPreference = new ArrayList<CodeableConcept>(); 1901 return this.dietPreference; 1902 } 1903 1904 /** 1905 * @return Returns a reference to <code>this</code> for easy method chaining 1906 */ 1907 public EncounterHospitalizationComponent setDietPreference(List<CodeableConcept> theDietPreference) { 1908 this.dietPreference = theDietPreference; 1909 return this; 1910 } 1911 1912 public boolean hasDietPreference() { 1913 if (this.dietPreference == null) 1914 return false; 1915 for (CodeableConcept item : this.dietPreference) 1916 if (!item.isEmpty()) 1917 return true; 1918 return false; 1919 } 1920 1921 public CodeableConcept addDietPreference() { // 3 1922 CodeableConcept t = new CodeableConcept(); 1923 if (this.dietPreference == null) 1924 this.dietPreference = new ArrayList<CodeableConcept>(); 1925 this.dietPreference.add(t); 1926 return t; 1927 } 1928 1929 public EncounterHospitalizationComponent addDietPreference(CodeableConcept t) { // 3 1930 if (t == null) 1931 return this; 1932 if (this.dietPreference == null) 1933 this.dietPreference = new ArrayList<CodeableConcept>(); 1934 this.dietPreference.add(t); 1935 return this; 1936 } 1937 1938 /** 1939 * @return The first repetition of repeating field {@link #dietPreference}, 1940 * creating it if it does not already exist 1941 */ 1942 public CodeableConcept getDietPreferenceFirstRep() { 1943 if (getDietPreference().isEmpty()) { 1944 addDietPreference(); 1945 } 1946 return getDietPreference().get(0); 1947 } 1948 1949 /** 1950 * @return {@link #specialCourtesy} (Special courtesies (VIP, board member).) 1951 */ 1952 public List<CodeableConcept> getSpecialCourtesy() { 1953 if (this.specialCourtesy == null) 1954 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1955 return this.specialCourtesy; 1956 } 1957 1958 /** 1959 * @return Returns a reference to <code>this</code> for easy method chaining 1960 */ 1961 public EncounterHospitalizationComponent setSpecialCourtesy(List<CodeableConcept> theSpecialCourtesy) { 1962 this.specialCourtesy = theSpecialCourtesy; 1963 return this; 1964 } 1965 1966 public boolean hasSpecialCourtesy() { 1967 if (this.specialCourtesy == null) 1968 return false; 1969 for (CodeableConcept item : this.specialCourtesy) 1970 if (!item.isEmpty()) 1971 return true; 1972 return false; 1973 } 1974 1975 public CodeableConcept addSpecialCourtesy() { // 3 1976 CodeableConcept t = new CodeableConcept(); 1977 if (this.specialCourtesy == null) 1978 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1979 this.specialCourtesy.add(t); 1980 return t; 1981 } 1982 1983 public EncounterHospitalizationComponent addSpecialCourtesy(CodeableConcept t) { // 3 1984 if (t == null) 1985 return this; 1986 if (this.specialCourtesy == null) 1987 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1988 this.specialCourtesy.add(t); 1989 return this; 1990 } 1991 1992 /** 1993 * @return The first repetition of repeating field {@link #specialCourtesy}, 1994 * creating it if it does not already exist 1995 */ 1996 public CodeableConcept getSpecialCourtesyFirstRep() { 1997 if (getSpecialCourtesy().isEmpty()) { 1998 addSpecialCourtesy(); 1999 } 2000 return getSpecialCourtesy().get(0); 2001 } 2002 2003 /** 2004 * @return {@link #specialArrangement} (Any special requests that have been made 2005 * for this hospitalization encounter, such as the provision of specific 2006 * equipment or other things.) 2007 */ 2008 public List<CodeableConcept> getSpecialArrangement() { 2009 if (this.specialArrangement == null) 2010 this.specialArrangement = new ArrayList<CodeableConcept>(); 2011 return this.specialArrangement; 2012 } 2013 2014 /** 2015 * @return Returns a reference to <code>this</code> for easy method chaining 2016 */ 2017 public EncounterHospitalizationComponent setSpecialArrangement(List<CodeableConcept> theSpecialArrangement) { 2018 this.specialArrangement = theSpecialArrangement; 2019 return this; 2020 } 2021 2022 public boolean hasSpecialArrangement() { 2023 if (this.specialArrangement == null) 2024 return false; 2025 for (CodeableConcept item : this.specialArrangement) 2026 if (!item.isEmpty()) 2027 return true; 2028 return false; 2029 } 2030 2031 public CodeableConcept addSpecialArrangement() { // 3 2032 CodeableConcept t = new CodeableConcept(); 2033 if (this.specialArrangement == null) 2034 this.specialArrangement = new ArrayList<CodeableConcept>(); 2035 this.specialArrangement.add(t); 2036 return t; 2037 } 2038 2039 public EncounterHospitalizationComponent addSpecialArrangement(CodeableConcept t) { // 3 2040 if (t == null) 2041 return this; 2042 if (this.specialArrangement == null) 2043 this.specialArrangement = new ArrayList<CodeableConcept>(); 2044 this.specialArrangement.add(t); 2045 return this; 2046 } 2047 2048 /** 2049 * @return The first repetition of repeating field {@link #specialArrangement}, 2050 * creating it if it does not already exist 2051 */ 2052 public CodeableConcept getSpecialArrangementFirstRep() { 2053 if (getSpecialArrangement().isEmpty()) { 2054 addSpecialArrangement(); 2055 } 2056 return getSpecialArrangement().get(0); 2057 } 2058 2059 /** 2060 * @return {@link #destination} (Location/organization to which the patient is 2061 * discharged.) 2062 */ 2063 public Reference getDestination() { 2064 if (this.destination == null) 2065 if (Configuration.errorOnAutoCreate()) 2066 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 2067 else if (Configuration.doAutoCreate()) 2068 this.destination = new Reference(); // cc 2069 return this.destination; 2070 } 2071 2072 public boolean hasDestination() { 2073 return this.destination != null && !this.destination.isEmpty(); 2074 } 2075 2076 /** 2077 * @param value {@link #destination} (Location/organization to which the patient 2078 * is discharged.) 2079 */ 2080 public EncounterHospitalizationComponent setDestination(Reference value) { 2081 this.destination = value; 2082 return this; 2083 } 2084 2085 /** 2086 * @return {@link #destination} The actual object that is the target of the 2087 * reference. The reference library doesn't populate this, but you can 2088 * use it to hold the resource if you resolve it. (Location/organization 2089 * to which the patient is discharged.) 2090 */ 2091 public Resource getDestinationTarget() { 2092 return this.destinationTarget; 2093 } 2094 2095 /** 2096 * @param value {@link #destination} The actual object that is the target of the 2097 * reference. The reference library doesn't use these, but you can 2098 * use it to hold the resource if you resolve it. 2099 * (Location/organization to which the patient is discharged.) 2100 */ 2101 public EncounterHospitalizationComponent setDestinationTarget(Resource value) { 2102 this.destinationTarget = value; 2103 return this; 2104 } 2105 2106 /** 2107 * @return {@link #dischargeDisposition} (Category or kind of location after 2108 * discharge.) 2109 */ 2110 public CodeableConcept getDischargeDisposition() { 2111 if (this.dischargeDisposition == null) 2112 if (Configuration.errorOnAutoCreate()) 2113 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.dischargeDisposition"); 2114 else if (Configuration.doAutoCreate()) 2115 this.dischargeDisposition = new CodeableConcept(); // cc 2116 return this.dischargeDisposition; 2117 } 2118 2119 public boolean hasDischargeDisposition() { 2120 return this.dischargeDisposition != null && !this.dischargeDisposition.isEmpty(); 2121 } 2122 2123 /** 2124 * @param value {@link #dischargeDisposition} (Category or kind of location 2125 * after discharge.) 2126 */ 2127 public EncounterHospitalizationComponent setDischargeDisposition(CodeableConcept value) { 2128 this.dischargeDisposition = value; 2129 return this; 2130 } 2131 2132 protected void listChildren(List<Property> children) { 2133 super.listChildren(children); 2134 children.add(new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, 2135 preAdmissionIdentifier)); 2136 children.add(new Property("origin", "Reference(Location|Organization)", 2137 "The location/organization from which the patient came before admission.", 0, 1, origin)); 2138 children.add(new Property("admitSource", "CodeableConcept", 2139 "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource)); 2140 children.add(new Property("reAdmission", "CodeableConcept", 2141 "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission)); 2142 children.add(new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, 2143 java.lang.Integer.MAX_VALUE, dietPreference)); 2144 children.add(new Property("specialCourtesy", "CodeableConcept", "Special courtesies (VIP, board member).", 0, 2145 java.lang.Integer.MAX_VALUE, specialCourtesy)); 2146 children.add(new Property("specialArrangement", "CodeableConcept", 2147 "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 2148 0, java.lang.Integer.MAX_VALUE, specialArrangement)); 2149 children.add(new Property("destination", "Reference(Location|Organization)", 2150 "Location/organization to which the patient is discharged.", 0, 1, destination)); 2151 children.add(new Property("dischargeDisposition", "CodeableConcept", 2152 "Category or kind of location after discharge.", 0, 1, dischargeDisposition)); 2153 } 2154 2155 @Override 2156 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2157 switch (_hash) { 2158 case -965394961: 2159 /* preAdmissionIdentifier */ return new Property("preAdmissionIdentifier", "Identifier", 2160 "Pre-admission identifier.", 0, 1, preAdmissionIdentifier); 2161 case -1008619738: 2162 /* origin */ return new Property("origin", "Reference(Location|Organization)", 2163 "The location/organization from which the patient came before admission.", 0, 1, origin); 2164 case 538887120: 2165 /* admitSource */ return new Property("admitSource", "CodeableConcept", 2166 "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource); 2167 case 669348630: 2168 /* reAdmission */ return new Property("reAdmission", "CodeableConcept", 2169 "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission); 2170 case -1360641041: 2171 /* dietPreference */ return new Property("dietPreference", "CodeableConcept", 2172 "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference); 2173 case 1583588345: 2174 /* specialCourtesy */ return new Property("specialCourtesy", "CodeableConcept", 2175 "Special courtesies (VIP, board member).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy); 2176 case 47410321: 2177 /* specialArrangement */ return new Property("specialArrangement", "CodeableConcept", 2178 "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 2179 0, java.lang.Integer.MAX_VALUE, specialArrangement); 2180 case -1429847026: 2181 /* destination */ return new Property("destination", "Reference(Location|Organization)", 2182 "Location/organization to which the patient is discharged.", 0, 1, destination); 2183 case 528065941: 2184 /* dischargeDisposition */ return new Property("dischargeDisposition", "CodeableConcept", 2185 "Category or kind of location after discharge.", 0, 1, dischargeDisposition); 2186 default: 2187 return super.getNamedProperty(_hash, _name, _checkValid); 2188 } 2189 2190 } 2191 2192 @Override 2193 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2194 switch (hash) { 2195 case -965394961: 2196 /* preAdmissionIdentifier */ return this.preAdmissionIdentifier == null ? new Base[0] 2197 : new Base[] { this.preAdmissionIdentifier }; // Identifier 2198 case -1008619738: 2199 /* origin */ return this.origin == null ? new Base[0] : new Base[] { this.origin }; // Reference 2200 case 538887120: 2201 /* admitSource */ return this.admitSource == null ? new Base[0] : new Base[] { this.admitSource }; // CodeableConcept 2202 case 669348630: 2203 /* reAdmission */ return this.reAdmission == null ? new Base[0] : new Base[] { this.reAdmission }; // CodeableConcept 2204 case -1360641041: 2205 /* dietPreference */ return this.dietPreference == null ? new Base[0] 2206 : this.dietPreference.toArray(new Base[this.dietPreference.size()]); // CodeableConcept 2207 case 1583588345: 2208 /* specialCourtesy */ return this.specialCourtesy == null ? new Base[0] 2209 : this.specialCourtesy.toArray(new Base[this.specialCourtesy.size()]); // CodeableConcept 2210 case 47410321: 2211 /* specialArrangement */ return this.specialArrangement == null ? new Base[0] 2212 : this.specialArrangement.toArray(new Base[this.specialArrangement.size()]); // CodeableConcept 2213 case -1429847026: 2214 /* destination */ return this.destination == null ? new Base[0] : new Base[] { this.destination }; // Reference 2215 case 528065941: 2216 /* dischargeDisposition */ return this.dischargeDisposition == null ? new Base[0] 2217 : new Base[] { this.dischargeDisposition }; // CodeableConcept 2218 default: 2219 return super.getProperty(hash, name, checkValid); 2220 } 2221 2222 } 2223 2224 @Override 2225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2226 switch (hash) { 2227 case -965394961: // preAdmissionIdentifier 2228 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 2229 return value; 2230 case -1008619738: // origin 2231 this.origin = castToReference(value); // Reference 2232 return value; 2233 case 538887120: // admitSource 2234 this.admitSource = castToCodeableConcept(value); // CodeableConcept 2235 return value; 2236 case 669348630: // reAdmission 2237 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 2238 return value; 2239 case -1360641041: // dietPreference 2240 this.getDietPreference().add(castToCodeableConcept(value)); // CodeableConcept 2241 return value; 2242 case 1583588345: // specialCourtesy 2243 this.getSpecialCourtesy().add(castToCodeableConcept(value)); // CodeableConcept 2244 return value; 2245 case 47410321: // specialArrangement 2246 this.getSpecialArrangement().add(castToCodeableConcept(value)); // CodeableConcept 2247 return value; 2248 case -1429847026: // destination 2249 this.destination = castToReference(value); // Reference 2250 return value; 2251 case 528065941: // dischargeDisposition 2252 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 2253 return value; 2254 default: 2255 return super.setProperty(hash, name, value); 2256 } 2257 2258 } 2259 2260 @Override 2261 public Base setProperty(String name, Base value) throws FHIRException { 2262 if (name.equals("preAdmissionIdentifier")) { 2263 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 2264 } else if (name.equals("origin")) { 2265 this.origin = castToReference(value); // Reference 2266 } else if (name.equals("admitSource")) { 2267 this.admitSource = castToCodeableConcept(value); // CodeableConcept 2268 } else if (name.equals("reAdmission")) { 2269 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 2270 } else if (name.equals("dietPreference")) { 2271 this.getDietPreference().add(castToCodeableConcept(value)); 2272 } else if (name.equals("specialCourtesy")) { 2273 this.getSpecialCourtesy().add(castToCodeableConcept(value)); 2274 } else if (name.equals("specialArrangement")) { 2275 this.getSpecialArrangement().add(castToCodeableConcept(value)); 2276 } else if (name.equals("destination")) { 2277 this.destination = castToReference(value); // Reference 2278 } else if (name.equals("dischargeDisposition")) { 2279 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 2280 } else 2281 return super.setProperty(name, value); 2282 return value; 2283 } 2284 2285 @Override 2286 public Base makeProperty(int hash, String name) throws FHIRException { 2287 switch (hash) { 2288 case -965394961: 2289 return getPreAdmissionIdentifier(); 2290 case -1008619738: 2291 return getOrigin(); 2292 case 538887120: 2293 return getAdmitSource(); 2294 case 669348630: 2295 return getReAdmission(); 2296 case -1360641041: 2297 return addDietPreference(); 2298 case 1583588345: 2299 return addSpecialCourtesy(); 2300 case 47410321: 2301 return addSpecialArrangement(); 2302 case -1429847026: 2303 return getDestination(); 2304 case 528065941: 2305 return getDischargeDisposition(); 2306 default: 2307 return super.makeProperty(hash, name); 2308 } 2309 2310 } 2311 2312 @Override 2313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2314 switch (hash) { 2315 case -965394961: 2316 /* preAdmissionIdentifier */ return new String[] { "Identifier" }; 2317 case -1008619738: 2318 /* origin */ return new String[] { "Reference" }; 2319 case 538887120: 2320 /* admitSource */ return new String[] { "CodeableConcept" }; 2321 case 669348630: 2322 /* reAdmission */ return new String[] { "CodeableConcept" }; 2323 case -1360641041: 2324 /* dietPreference */ return new String[] { "CodeableConcept" }; 2325 case 1583588345: 2326 /* specialCourtesy */ return new String[] { "CodeableConcept" }; 2327 case 47410321: 2328 /* specialArrangement */ return new String[] { "CodeableConcept" }; 2329 case -1429847026: 2330 /* destination */ return new String[] { "Reference" }; 2331 case 528065941: 2332 /* dischargeDisposition */ return new String[] { "CodeableConcept" }; 2333 default: 2334 return super.getTypesForProperty(hash, name); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base addChild(String name) throws FHIRException { 2341 if (name.equals("preAdmissionIdentifier")) { 2342 this.preAdmissionIdentifier = new Identifier(); 2343 return this.preAdmissionIdentifier; 2344 } else if (name.equals("origin")) { 2345 this.origin = new Reference(); 2346 return this.origin; 2347 } else if (name.equals("admitSource")) { 2348 this.admitSource = new CodeableConcept(); 2349 return this.admitSource; 2350 } else if (name.equals("reAdmission")) { 2351 this.reAdmission = new CodeableConcept(); 2352 return this.reAdmission; 2353 } else if (name.equals("dietPreference")) { 2354 return addDietPreference(); 2355 } else if (name.equals("specialCourtesy")) { 2356 return addSpecialCourtesy(); 2357 } else if (name.equals("specialArrangement")) { 2358 return addSpecialArrangement(); 2359 } else if (name.equals("destination")) { 2360 this.destination = new Reference(); 2361 return this.destination; 2362 } else if (name.equals("dischargeDisposition")) { 2363 this.dischargeDisposition = new CodeableConcept(); 2364 return this.dischargeDisposition; 2365 } else 2366 return super.addChild(name); 2367 } 2368 2369 public EncounterHospitalizationComponent copy() { 2370 EncounterHospitalizationComponent dst = new EncounterHospitalizationComponent(); 2371 copyValues(dst); 2372 return dst; 2373 } 2374 2375 public void copyValues(EncounterHospitalizationComponent dst) { 2376 super.copyValues(dst); 2377 dst.preAdmissionIdentifier = preAdmissionIdentifier == null ? null : preAdmissionIdentifier.copy(); 2378 dst.origin = origin == null ? null : origin.copy(); 2379 dst.admitSource = admitSource == null ? null : admitSource.copy(); 2380 dst.reAdmission = reAdmission == null ? null : reAdmission.copy(); 2381 if (dietPreference != null) { 2382 dst.dietPreference = new ArrayList<CodeableConcept>(); 2383 for (CodeableConcept i : dietPreference) 2384 dst.dietPreference.add(i.copy()); 2385 } 2386 ; 2387 if (specialCourtesy != null) { 2388 dst.specialCourtesy = new ArrayList<CodeableConcept>(); 2389 for (CodeableConcept i : specialCourtesy) 2390 dst.specialCourtesy.add(i.copy()); 2391 } 2392 ; 2393 if (specialArrangement != null) { 2394 dst.specialArrangement = new ArrayList<CodeableConcept>(); 2395 for (CodeableConcept i : specialArrangement) 2396 dst.specialArrangement.add(i.copy()); 2397 } 2398 ; 2399 dst.destination = destination == null ? null : destination.copy(); 2400 dst.dischargeDisposition = dischargeDisposition == null ? null : dischargeDisposition.copy(); 2401 } 2402 2403 @Override 2404 public boolean equalsDeep(Base other_) { 2405 if (!super.equalsDeep(other_)) 2406 return false; 2407 if (!(other_ instanceof EncounterHospitalizationComponent)) 2408 return false; 2409 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2410 return compareDeep(preAdmissionIdentifier, o.preAdmissionIdentifier, true) && compareDeep(origin, o.origin, true) 2411 && compareDeep(admitSource, o.admitSource, true) && compareDeep(reAdmission, o.reAdmission, true) 2412 && compareDeep(dietPreference, o.dietPreference, true) 2413 && compareDeep(specialCourtesy, o.specialCourtesy, true) 2414 && compareDeep(specialArrangement, o.specialArrangement, true) 2415 && compareDeep(destination, o.destination, true) 2416 && compareDeep(dischargeDisposition, o.dischargeDisposition, true); 2417 } 2418 2419 @Override 2420 public boolean equalsShallow(Base other_) { 2421 if (!super.equalsShallow(other_)) 2422 return false; 2423 if (!(other_ instanceof EncounterHospitalizationComponent)) 2424 return false; 2425 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2426 return true; 2427 } 2428 2429 public boolean isEmpty() { 2430 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(preAdmissionIdentifier, origin, admitSource, 2431 reAdmission, dietPreference, specialCourtesy, specialArrangement, destination, dischargeDisposition); 2432 } 2433 2434 public String fhirType() { 2435 return "Encounter.hospitalization"; 2436 2437 } 2438 2439 } 2440 2441 @Block() 2442 public static class EncounterLocationComponent extends BackboneElement implements IBaseBackboneElement { 2443 /** 2444 * The location where the encounter takes place. 2445 */ 2446 @Child(name = "location", type = { Location.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2447 @Description(shortDefinition = "Location the encounter takes place", formalDefinition = "The location where the encounter takes place.") 2448 protected Reference location; 2449 2450 /** 2451 * The actual object that is the target of the reference (The location where the 2452 * encounter takes place.) 2453 */ 2454 protected Location locationTarget; 2455 2456 /** 2457 * The status of the participants' presence at the specified location during the 2458 * period specified. If the participant is no longer at the location, then the 2459 * period will have an end date/time. 2460 */ 2461 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2462 @Description(shortDefinition = "planned | active | reserved | completed", formalDefinition = "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.") 2463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-location-status") 2464 protected Enumeration<EncounterLocationStatus> status; 2465 2466 /** 2467 * This will be used to specify the required levels (bed/ward/room/etc.) desired 2468 * to be recorded to simplify either messaging or query. 2469 */ 2470 @Child(name = "physicalType", type = { 2471 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2472 @Description(shortDefinition = "The physical type of the location (usually the level in the location hierachy - bed room ward etc.)", formalDefinition = "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.") 2473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-physical-type") 2474 protected CodeableConcept physicalType; 2475 2476 /** 2477 * Time period during which the patient was present at the location. 2478 */ 2479 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2480 @Description(shortDefinition = "Time period during which the patient was present at the location", formalDefinition = "Time period during which the patient was present at the location.") 2481 protected Period period; 2482 2483 private static final long serialVersionUID = -755081862L; 2484 2485 /** 2486 * Constructor 2487 */ 2488 public EncounterLocationComponent() { 2489 super(); 2490 } 2491 2492 /** 2493 * Constructor 2494 */ 2495 public EncounterLocationComponent(Reference location) { 2496 super(); 2497 this.location = location; 2498 } 2499 2500 /** 2501 * @return {@link #location} (The location where the encounter takes place.) 2502 */ 2503 public Reference getLocation() { 2504 if (this.location == null) 2505 if (Configuration.errorOnAutoCreate()) 2506 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2507 else if (Configuration.doAutoCreate()) 2508 this.location = new Reference(); // cc 2509 return this.location; 2510 } 2511 2512 public boolean hasLocation() { 2513 return this.location != null && !this.location.isEmpty(); 2514 } 2515 2516 /** 2517 * @param value {@link #location} (The location where the encounter takes 2518 * place.) 2519 */ 2520 public EncounterLocationComponent setLocation(Reference value) { 2521 this.location = value; 2522 return this; 2523 } 2524 2525 /** 2526 * @return {@link #location} The actual object that is the target of the 2527 * reference. The reference library doesn't populate this, but you can 2528 * use it to hold the resource if you resolve it. (The location where 2529 * the encounter takes place.) 2530 */ 2531 public Location getLocationTarget() { 2532 if (this.locationTarget == null) 2533 if (Configuration.errorOnAutoCreate()) 2534 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2535 else if (Configuration.doAutoCreate()) 2536 this.locationTarget = new Location(); // aa 2537 return this.locationTarget; 2538 } 2539 2540 /** 2541 * @param value {@link #location} The actual object that is the target of the 2542 * reference. The reference library doesn't use these, but you can 2543 * use it to hold the resource if you resolve it. (The location 2544 * where the encounter takes place.) 2545 */ 2546 public EncounterLocationComponent setLocationTarget(Location value) { 2547 this.locationTarget = value; 2548 return this; 2549 } 2550 2551 /** 2552 * @return {@link #status} (The status of the participants' presence at the 2553 * specified location during the period specified. If the participant is 2554 * no longer at the location, then the period will have an end 2555 * date/time.). This is the underlying object with id, value and 2556 * extensions. The accessor "getStatus" gives direct access to the value 2557 */ 2558 public Enumeration<EncounterLocationStatus> getStatusElement() { 2559 if (this.status == null) 2560 if (Configuration.errorOnAutoCreate()) 2561 throw new Error("Attempt to auto-create EncounterLocationComponent.status"); 2562 else if (Configuration.doAutoCreate()) 2563 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); // bb 2564 return this.status; 2565 } 2566 2567 public boolean hasStatusElement() { 2568 return this.status != null && !this.status.isEmpty(); 2569 } 2570 2571 public boolean hasStatus() { 2572 return this.status != null && !this.status.isEmpty(); 2573 } 2574 2575 /** 2576 * @param value {@link #status} (The status of the participants' presence at the 2577 * specified location during the period specified. If the 2578 * participant is no longer at the location, then the period will 2579 * have an end date/time.). This is the underlying object with id, 2580 * value and extensions. The accessor "getStatus" gives direct 2581 * access to the value 2582 */ 2583 public EncounterLocationComponent setStatusElement(Enumeration<EncounterLocationStatus> value) { 2584 this.status = value; 2585 return this; 2586 } 2587 2588 /** 2589 * @return The status of the participants' presence at the specified location 2590 * during the period specified. If the participant is no longer at the 2591 * location, then the period will have an end date/time. 2592 */ 2593 public EncounterLocationStatus getStatus() { 2594 return this.status == null ? null : this.status.getValue(); 2595 } 2596 2597 /** 2598 * @param value The status of the participants' presence at the specified 2599 * location during the period specified. If the participant is no 2600 * longer at the location, then the period will have an end 2601 * date/time. 2602 */ 2603 public EncounterLocationComponent setStatus(EncounterLocationStatus value) { 2604 if (value == null) 2605 this.status = null; 2606 else { 2607 if (this.status == null) 2608 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); 2609 this.status.setValue(value); 2610 } 2611 return this; 2612 } 2613 2614 /** 2615 * @return {@link #physicalType} (This will be used to specify the required 2616 * levels (bed/ward/room/etc.) desired to be recorded to simplify either 2617 * messaging or query.) 2618 */ 2619 public CodeableConcept getPhysicalType() { 2620 if (this.physicalType == null) 2621 if (Configuration.errorOnAutoCreate()) 2622 throw new Error("Attempt to auto-create EncounterLocationComponent.physicalType"); 2623 else if (Configuration.doAutoCreate()) 2624 this.physicalType = new CodeableConcept(); // cc 2625 return this.physicalType; 2626 } 2627 2628 public boolean hasPhysicalType() { 2629 return this.physicalType != null && !this.physicalType.isEmpty(); 2630 } 2631 2632 /** 2633 * @param value {@link #physicalType} (This will be used to specify the required 2634 * levels (bed/ward/room/etc.) desired to be recorded to simplify 2635 * either messaging or query.) 2636 */ 2637 public EncounterLocationComponent setPhysicalType(CodeableConcept value) { 2638 this.physicalType = value; 2639 return this; 2640 } 2641 2642 /** 2643 * @return {@link #period} (Time period during which the patient was present at 2644 * the location.) 2645 */ 2646 public Period getPeriod() { 2647 if (this.period == null) 2648 if (Configuration.errorOnAutoCreate()) 2649 throw new Error("Attempt to auto-create EncounterLocationComponent.period"); 2650 else if (Configuration.doAutoCreate()) 2651 this.period = new Period(); // cc 2652 return this.period; 2653 } 2654 2655 public boolean hasPeriod() { 2656 return this.period != null && !this.period.isEmpty(); 2657 } 2658 2659 /** 2660 * @param value {@link #period} (Time period during which the patient was 2661 * present at the location.) 2662 */ 2663 public EncounterLocationComponent setPeriod(Period value) { 2664 this.period = value; 2665 return this; 2666 } 2667 2668 protected void listChildren(List<Property> children) { 2669 super.listChildren(children); 2670 children.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 2671 1, location)); 2672 children.add(new Property("status", "code", 2673 "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 2674 0, 1, status)); 2675 children.add(new Property("physicalType", "CodeableConcept", 2676 "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 2677 0, 1, physicalType)); 2678 children.add(new Property("period", "Period", "Time period during which the patient was present at the location.", 2679 0, 1, period)); 2680 } 2681 2682 @Override 2683 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2684 switch (_hash) { 2685 case 1901043637: 2686 /* location */ return new Property("location", "Reference(Location)", 2687 "The location where the encounter takes place.", 0, 1, location); 2688 case -892481550: 2689 /* status */ return new Property("status", "code", 2690 "The status of the participants' presence at the specified location during the period specified. If the participant is no longer at the location, then the period will have an end date/time.", 2691 0, 1, status); 2692 case -1474715471: 2693 /* physicalType */ return new Property("physicalType", "CodeableConcept", 2694 "This will be used to specify the required levels (bed/ward/room/etc.) desired to be recorded to simplify either messaging or query.", 2695 0, 1, physicalType); 2696 case -991726143: 2697 /* period */ return new Property("period", "Period", 2698 "Time period during which the patient was present at the location.", 0, 1, period); 2699 default: 2700 return super.getNamedProperty(_hash, _name, _checkValid); 2701 } 2702 2703 } 2704 2705 @Override 2706 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2707 switch (hash) { 2708 case 1901043637: 2709 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 2710 case -892481550: 2711 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterLocationStatus> 2712 case -1474715471: 2713 /* physicalType */ return this.physicalType == null ? new Base[0] : new Base[] { this.physicalType }; // CodeableConcept 2714 case -991726143: 2715 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 2716 default: 2717 return super.getProperty(hash, name, checkValid); 2718 } 2719 2720 } 2721 2722 @Override 2723 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2724 switch (hash) { 2725 case 1901043637: // location 2726 this.location = castToReference(value); // Reference 2727 return value; 2728 case -892481550: // status 2729 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2730 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2731 return value; 2732 case -1474715471: // physicalType 2733 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2734 return value; 2735 case -991726143: // period 2736 this.period = castToPeriod(value); // Period 2737 return value; 2738 default: 2739 return super.setProperty(hash, name, value); 2740 } 2741 2742 } 2743 2744 @Override 2745 public Base setProperty(String name, Base value) throws FHIRException { 2746 if (name.equals("location")) { 2747 this.location = castToReference(value); // Reference 2748 } else if (name.equals("status")) { 2749 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2750 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2751 } else if (name.equals("physicalType")) { 2752 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2753 } else if (name.equals("period")) { 2754 this.period = castToPeriod(value); // Period 2755 } else 2756 return super.setProperty(name, value); 2757 return value; 2758 } 2759 2760 @Override 2761 public Base makeProperty(int hash, String name) throws FHIRException { 2762 switch (hash) { 2763 case 1901043637: 2764 return getLocation(); 2765 case -892481550: 2766 return getStatusElement(); 2767 case -1474715471: 2768 return getPhysicalType(); 2769 case -991726143: 2770 return getPeriod(); 2771 default: 2772 return super.makeProperty(hash, name); 2773 } 2774 2775 } 2776 2777 @Override 2778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2779 switch (hash) { 2780 case 1901043637: 2781 /* location */ return new String[] { "Reference" }; 2782 case -892481550: 2783 /* status */ return new String[] { "code" }; 2784 case -1474715471: 2785 /* physicalType */ return new String[] { "CodeableConcept" }; 2786 case -991726143: 2787 /* period */ return new String[] { "Period" }; 2788 default: 2789 return super.getTypesForProperty(hash, name); 2790 } 2791 2792 } 2793 2794 @Override 2795 public Base addChild(String name) throws FHIRException { 2796 if (name.equals("location")) { 2797 this.location = new Reference(); 2798 return this.location; 2799 } else if (name.equals("status")) { 2800 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 2801 } else if (name.equals("physicalType")) { 2802 this.physicalType = new CodeableConcept(); 2803 return this.physicalType; 2804 } else if (name.equals("period")) { 2805 this.period = new Period(); 2806 return this.period; 2807 } else 2808 return super.addChild(name); 2809 } 2810 2811 public EncounterLocationComponent copy() { 2812 EncounterLocationComponent dst = new EncounterLocationComponent(); 2813 copyValues(dst); 2814 return dst; 2815 } 2816 2817 public void copyValues(EncounterLocationComponent dst) { 2818 super.copyValues(dst); 2819 dst.location = location == null ? null : location.copy(); 2820 dst.status = status == null ? null : status.copy(); 2821 dst.physicalType = physicalType == null ? null : physicalType.copy(); 2822 dst.period = period == null ? null : period.copy(); 2823 } 2824 2825 @Override 2826 public boolean equalsDeep(Base other_) { 2827 if (!super.equalsDeep(other_)) 2828 return false; 2829 if (!(other_ instanceof EncounterLocationComponent)) 2830 return false; 2831 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2832 return compareDeep(location, o.location, true) && compareDeep(status, o.status, true) 2833 && compareDeep(physicalType, o.physicalType, true) && compareDeep(period, o.period, true); 2834 } 2835 2836 @Override 2837 public boolean equalsShallow(Base other_) { 2838 if (!super.equalsShallow(other_)) 2839 return false; 2840 if (!(other_ instanceof EncounterLocationComponent)) 2841 return false; 2842 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2843 return compareValues(status, o.status, true); 2844 } 2845 2846 public boolean isEmpty() { 2847 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, status, physicalType, period); 2848 } 2849 2850 public String fhirType() { 2851 return "Encounter.location"; 2852 2853 } 2854 2855 } 2856 2857 /** 2858 * Identifier(s) by which this encounter is known. 2859 */ 2860 @Child(name = "identifier", type = { 2861 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2862 @Description(shortDefinition = "Identifier(s) by which this encounter is known", formalDefinition = "Identifier(s) by which this encounter is known.") 2863 protected List<Identifier> identifier; 2864 2865 /** 2866 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 2867 */ 2868 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 2869 @Description(shortDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.") 2870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-status") 2871 protected Enumeration<EncounterStatus> status; 2872 2873 /** 2874 * The status history permits the encounter resource to contain the status 2875 * history without needing to read through the historical versions of the 2876 * resource, or even have the server store them. 2877 */ 2878 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2879 @Description(shortDefinition = "List of past encounter statuses", formalDefinition = "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.") 2880 protected List<StatusHistoryComponent> statusHistory; 2881 2882 /** 2883 * Concepts representing classification of patient encounter such as ambulatory 2884 * (outpatient), inpatient, emergency, home health or others due to local 2885 * variations. 2886 */ 2887 @Child(name = "class", type = { Coding.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2888 @Description(shortDefinition = "Classification of patient encounter", formalDefinition = "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.") 2889 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActEncounterCode") 2890 protected Coding class_; 2891 2892 /** 2893 * The class history permits the tracking of the encounters transitions without 2894 * needing to go through the resource history. This would be used for a case 2895 * where an admission starts of as an emergency encounter, then transitions into 2896 * an inpatient scenario. Doing this and not restarting a new encounter ensures 2897 * that any lab/diagnostic results can more easily follow the patient and not 2898 * require re-processing and not get lost or cancelled during a kind of 2899 * discharge from emergency to inpatient. 2900 */ 2901 @Child(name = "classHistory", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2902 @Description(shortDefinition = "List of past encounter classes", formalDefinition = "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.") 2903 protected List<ClassHistoryComponent> classHistory; 2904 2905 /** 2906 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, 2907 * skilled nursing, rehabilitation). 2908 */ 2909 @Child(name = "type", type = { 2910 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2911 @Description(shortDefinition = "Specific type of encounter", formalDefinition = "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).") 2912 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-type") 2913 protected List<CodeableConcept> type; 2914 2915 /** 2916 * Broad categorization of the service that is to be provided (e.g. cardiology). 2917 */ 2918 @Child(name = "serviceType", type = { 2919 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2920 @Description(shortDefinition = "Specific type of service", formalDefinition = "Broad categorization of the service that is to be provided (e.g. cardiology).") 2921 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 2922 protected CodeableConcept serviceType; 2923 2924 /** 2925 * Indicates the urgency of the encounter. 2926 */ 2927 @Child(name = "priority", type = { 2928 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 2929 @Description(shortDefinition = "Indicates the urgency of the encounter", formalDefinition = "Indicates the urgency of the encounter.") 2930 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActPriority") 2931 protected CodeableConcept priority; 2932 2933 /** 2934 * The patient or group present at the encounter. 2935 */ 2936 @Child(name = "subject", type = { Patient.class, 2937 Group.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2938 @Description(shortDefinition = "The patient or group present at the encounter", formalDefinition = "The patient or group present at the encounter.") 2939 protected Reference subject; 2940 2941 /** 2942 * The actual object that is the target of the reference (The patient or group 2943 * present at the encounter.) 2944 */ 2945 protected Resource subjectTarget; 2946 2947 /** 2948 * Where a specific encounter should be classified as a part of a specific 2949 * episode(s) of care this field should be used. This association can facilitate 2950 * grouping of related encounters together for a specific purpose, such as 2951 * government reporting, issue tracking, association via a common problem. The 2952 * association is recorded on the encounter as these are typically created after 2953 * the episode of care and grouped on entry rather than editing the episode of 2954 * care to append another encounter to it (the episode of care could span 2955 * years). 2956 */ 2957 @Child(name = "episodeOfCare", type = { 2958 EpisodeOfCare.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2959 @Description(shortDefinition = "Episode(s) of care that this encounter should be recorded against", formalDefinition = "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).") 2960 protected List<Reference> episodeOfCare; 2961 /** 2962 * The actual objects that are the target of the reference (Where a specific 2963 * encounter should be classified as a part of a specific episode(s) of care 2964 * this field should be used. This association can facilitate grouping of 2965 * related encounters together for a specific purpose, such as government 2966 * reporting, issue tracking, association via a common problem. The association 2967 * is recorded on the encounter as these are typically created after the episode 2968 * of care and grouped on entry rather than editing the episode of care to 2969 * append another encounter to it (the episode of care could span years).) 2970 */ 2971 protected List<EpisodeOfCare> episodeOfCareTarget; 2972 2973 /** 2974 * The request this encounter satisfies (e.g. incoming referral or procedure 2975 * request). 2976 */ 2977 @Child(name = "basedOn", type = { 2978 ServiceRequest.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2979 @Description(shortDefinition = "The ServiceRequest that initiated this encounter", formalDefinition = "The request this encounter satisfies (e.g. incoming referral or procedure request).") 2980 protected List<Reference> basedOn; 2981 /** 2982 * The actual objects that are the target of the reference (The request this 2983 * encounter satisfies (e.g. incoming referral or procedure request).) 2984 */ 2985 protected List<ServiceRequest> basedOnTarget; 2986 2987 /** 2988 * The list of people responsible for providing the service. 2989 */ 2990 @Child(name = "participant", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2991 @Description(shortDefinition = "List of participants involved in the encounter", formalDefinition = "The list of people responsible for providing the service.") 2992 protected List<EncounterParticipantComponent> participant; 2993 2994 /** 2995 * The appointment that scheduled this encounter. 2996 */ 2997 @Child(name = "appointment", type = { 2998 Appointment.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2999 @Description(shortDefinition = "The appointment that scheduled this encounter", formalDefinition = "The appointment that scheduled this encounter.") 3000 protected List<Reference> appointment; 3001 /** 3002 * The actual objects that are the target of the reference (The appointment that 3003 * scheduled this encounter.) 3004 */ 3005 protected List<Appointment> appointmentTarget; 3006 3007 /** 3008 * The start and end time of the encounter. 3009 */ 3010 @Child(name = "period", type = { Period.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 3011 @Description(shortDefinition = "The start and end time of the encounter", formalDefinition = "The start and end time of the encounter.") 3012 protected Period period; 3013 3014 /** 3015 * Quantity of time the encounter lasted. This excludes the time during leaves 3016 * of absence. 3017 */ 3018 @Child(name = "length", type = { Duration.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 3019 @Description(shortDefinition = "Quantity of time the encounter lasted (less time absent)", formalDefinition = "Quantity of time the encounter lasted. This excludes the time during leaves of absence.") 3020 protected Duration length; 3021 3022 /** 3023 * Reason the encounter takes place, expressed as a code. For admissions, this 3024 * can be used for a coded admission diagnosis. 3025 */ 3026 @Child(name = "reasonCode", type = { 3027 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3028 @Description(shortDefinition = "Coded reason the encounter takes place", formalDefinition = "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.") 3029 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/encounter-reason") 3030 protected List<CodeableConcept> reasonCode; 3031 3032 /** 3033 * Reason the encounter takes place, expressed as a code. For admissions, this 3034 * can be used for a coded admission diagnosis. 3035 */ 3036 @Child(name = "reasonReference", type = { Condition.class, Procedure.class, Observation.class, 3037 ImmunizationRecommendation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3038 @Description(shortDefinition = "Reason the encounter takes place (reference)", formalDefinition = "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.") 3039 protected List<Reference> reasonReference; 3040 /** 3041 * The actual objects that are the target of the reference (Reason the encounter 3042 * takes place, expressed as a code. For admissions, this can be used for a 3043 * coded admission diagnosis.) 3044 */ 3045 protected List<Resource> reasonReferenceTarget; 3046 3047 /** 3048 * The list of diagnosis relevant to this encounter. 3049 */ 3050 @Child(name = "diagnosis", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3051 @Description(shortDefinition = "The list of diagnosis relevant to this encounter", formalDefinition = "The list of diagnosis relevant to this encounter.") 3052 protected List<DiagnosisComponent> diagnosis; 3053 3054 /** 3055 * The set of accounts that may be used for billing for this Encounter. 3056 */ 3057 @Child(name = "account", type = { 3058 Account.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3059 @Description(shortDefinition = "The set of accounts that may be used for billing for this Encounter", formalDefinition = "The set of accounts that may be used for billing for this Encounter.") 3060 protected List<Reference> account; 3061 /** 3062 * The actual objects that are the target of the reference (The set of accounts 3063 * that may be used for billing for this Encounter.) 3064 */ 3065 protected List<Account> accountTarget; 3066 3067 /** 3068 * Details about the admission to a healthcare service. 3069 */ 3070 @Child(name = "hospitalization", type = {}, order = 19, min = 0, max = 1, modifier = false, summary = false) 3071 @Description(shortDefinition = "Details about the admission to a healthcare service", formalDefinition = "Details about the admission to a healthcare service.") 3072 protected EncounterHospitalizationComponent hospitalization; 3073 3074 /** 3075 * List of locations where the patient has been during this encounter. 3076 */ 3077 @Child(name = "location", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3078 @Description(shortDefinition = "List of locations where the patient has been", formalDefinition = "List of locations where the patient has been during this encounter.") 3079 protected List<EncounterLocationComponent> location; 3080 3081 /** 3082 * The organization that is primarily responsible for this Encounter's services. 3083 * This MAY be the same as the organization on the Patient record, however it 3084 * could be different, such as if the actor performing the services was from an 3085 * external organization (which may be billed seperately) for an external 3086 * consultation. Refer to the example bundle showing an abbreviated set of 3087 * Encounters for a colonoscopy. 3088 */ 3089 @Child(name = "serviceProvider", type = { 3090 Organization.class }, order = 21, min = 0, max = 1, modifier = false, summary = false) 3091 @Description(shortDefinition = "The organization (facility) responsible for this encounter", formalDefinition = "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.") 3092 protected Reference serviceProvider; 3093 3094 /** 3095 * The actual object that is the target of the reference (The organization that 3096 * is primarily responsible for this Encounter's services. This MAY be the same 3097 * as the organization on the Patient record, however it could be different, 3098 * such as if the actor performing the services was from an external 3099 * organization (which may be billed seperately) for an external consultation. 3100 * Refer to the example bundle showing an abbreviated set of Encounters for a 3101 * colonoscopy.) 3102 */ 3103 protected Organization serviceProviderTarget; 3104 3105 /** 3106 * Another Encounter of which this encounter is a part of (administratively or 3107 * in time). 3108 */ 3109 @Child(name = "partOf", type = { Encounter.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 3110 @Description(shortDefinition = "Another Encounter this encounter is part of", formalDefinition = "Another Encounter of which this encounter is a part of (administratively or in time).") 3111 protected Reference partOf; 3112 3113 /** 3114 * The actual object that is the target of the reference (Another Encounter of 3115 * which this encounter is a part of (administratively or in time).) 3116 */ 3117 protected Encounter partOfTarget; 3118 3119 private static final long serialVersionUID = 1358318037L; 3120 3121 /** 3122 * Constructor 3123 */ 3124 public Encounter() { 3125 super(); 3126 } 3127 3128 /** 3129 * Constructor 3130 */ 3131 public Encounter(Enumeration<EncounterStatus> status, Coding class_) { 3132 super(); 3133 this.status = status; 3134 this.class_ = class_; 3135 } 3136 3137 /** 3138 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 3139 */ 3140 public List<Identifier> getIdentifier() { 3141 if (this.identifier == null) 3142 this.identifier = new ArrayList<Identifier>(); 3143 return this.identifier; 3144 } 3145 3146 /** 3147 * @return Returns a reference to <code>this</code> for easy method chaining 3148 */ 3149 public Encounter setIdentifier(List<Identifier> theIdentifier) { 3150 this.identifier = theIdentifier; 3151 return this; 3152 } 3153 3154 public boolean hasIdentifier() { 3155 if (this.identifier == null) 3156 return false; 3157 for (Identifier item : this.identifier) 3158 if (!item.isEmpty()) 3159 return true; 3160 return false; 3161 } 3162 3163 public Identifier addIdentifier() { // 3 3164 Identifier t = new Identifier(); 3165 if (this.identifier == null) 3166 this.identifier = new ArrayList<Identifier>(); 3167 this.identifier.add(t); 3168 return t; 3169 } 3170 3171 public Encounter addIdentifier(Identifier t) { // 3 3172 if (t == null) 3173 return this; 3174 if (this.identifier == null) 3175 this.identifier = new ArrayList<Identifier>(); 3176 this.identifier.add(t); 3177 return this; 3178 } 3179 3180 /** 3181 * @return The first repetition of repeating field {@link #identifier}, creating 3182 * it if it does not already exist 3183 */ 3184 public Identifier getIdentifierFirstRep() { 3185 if (getIdentifier().isEmpty()) { 3186 addIdentifier(); 3187 } 3188 return getIdentifier().get(0); 3189 } 3190 3191 /** 3192 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave 3193 * | finished | cancelled +.). This is the underlying object with id, 3194 * value and extensions. The accessor "getStatus" gives direct access to 3195 * the value 3196 */ 3197 public Enumeration<EncounterStatus> getStatusElement() { 3198 if (this.status == null) 3199 if (Configuration.errorOnAutoCreate()) 3200 throw new Error("Attempt to auto-create Encounter.status"); 3201 else if (Configuration.doAutoCreate()) 3202 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 3203 return this.status; 3204 } 3205 3206 public boolean hasStatusElement() { 3207 return this.status != null && !this.status.isEmpty(); 3208 } 3209 3210 public boolean hasStatus() { 3211 return this.status != null && !this.status.isEmpty(); 3212 } 3213 3214 /** 3215 * @param value {@link #status} (planned | arrived | triaged | in-progress | 3216 * onleave | finished | cancelled +.). This is the underlying 3217 * object with id, value and extensions. The accessor "getStatus" 3218 * gives direct access to the value 3219 */ 3220 public Encounter setStatusElement(Enumeration<EncounterStatus> value) { 3221 this.status = value; 3222 return this; 3223 } 3224 3225 /** 3226 * @return planned | arrived | triaged | in-progress | onleave | finished | 3227 * cancelled +. 3228 */ 3229 public EncounterStatus getStatus() { 3230 return this.status == null ? null : this.status.getValue(); 3231 } 3232 3233 /** 3234 * @param value planned | arrived | triaged | in-progress | onleave | finished | 3235 * cancelled +. 3236 */ 3237 public Encounter setStatus(EncounterStatus value) { 3238 if (this.status == null) 3239 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 3240 this.status.setValue(value); 3241 return this; 3242 } 3243 3244 /** 3245 * @return {@link #statusHistory} (The status history permits the encounter 3246 * resource to contain the status history without needing to read 3247 * through the historical versions of the resource, or even have the 3248 * server store them.) 3249 */ 3250 public List<StatusHistoryComponent> getStatusHistory() { 3251 if (this.statusHistory == null) 3252 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3253 return this.statusHistory; 3254 } 3255 3256 /** 3257 * @return Returns a reference to <code>this</code> for easy method chaining 3258 */ 3259 public Encounter setStatusHistory(List<StatusHistoryComponent> theStatusHistory) { 3260 this.statusHistory = theStatusHistory; 3261 return this; 3262 } 3263 3264 public boolean hasStatusHistory() { 3265 if (this.statusHistory == null) 3266 return false; 3267 for (StatusHistoryComponent item : this.statusHistory) 3268 if (!item.isEmpty()) 3269 return true; 3270 return false; 3271 } 3272 3273 public StatusHistoryComponent addStatusHistory() { // 3 3274 StatusHistoryComponent t = new StatusHistoryComponent(); 3275 if (this.statusHistory == null) 3276 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3277 this.statusHistory.add(t); 3278 return t; 3279 } 3280 3281 public Encounter addStatusHistory(StatusHistoryComponent t) { // 3 3282 if (t == null) 3283 return this; 3284 if (this.statusHistory == null) 3285 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 3286 this.statusHistory.add(t); 3287 return this; 3288 } 3289 3290 /** 3291 * @return The first repetition of repeating field {@link #statusHistory}, 3292 * creating it if it does not already exist 3293 */ 3294 public StatusHistoryComponent getStatusHistoryFirstRep() { 3295 if (getStatusHistory().isEmpty()) { 3296 addStatusHistory(); 3297 } 3298 return getStatusHistory().get(0); 3299 } 3300 3301 /** 3302 * @return {@link #class_} (Concepts representing classification of patient 3303 * encounter such as ambulatory (outpatient), inpatient, emergency, home 3304 * health or others due to local variations.) 3305 */ 3306 public Coding getClass_() { 3307 if (this.class_ == null) 3308 if (Configuration.errorOnAutoCreate()) 3309 throw new Error("Attempt to auto-create Encounter.class_"); 3310 else if (Configuration.doAutoCreate()) 3311 this.class_ = new Coding(); // cc 3312 return this.class_; 3313 } 3314 3315 public boolean hasClass_() { 3316 return this.class_ != null && !this.class_.isEmpty(); 3317 } 3318 3319 /** 3320 * @param value {@link #class_} (Concepts representing classification of patient 3321 * encounter such as ambulatory (outpatient), inpatient, emergency, 3322 * home health or others due to local variations.) 3323 */ 3324 public Encounter setClass_(Coding value) { 3325 this.class_ = value; 3326 return this; 3327 } 3328 3329 /** 3330 * @return {@link #classHistory} (The class history permits the tracking of the 3331 * encounters transitions without needing to go through the resource 3332 * history. This would be used for a case where an admission starts of 3333 * as an emergency encounter, then transitions into an inpatient 3334 * scenario. Doing this and not restarting a new encounter ensures that 3335 * any lab/diagnostic results can more easily follow the patient and not 3336 * require re-processing and not get lost or cancelled during a kind of 3337 * discharge from emergency to inpatient.) 3338 */ 3339 public List<ClassHistoryComponent> getClassHistory() { 3340 if (this.classHistory == null) 3341 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3342 return this.classHistory; 3343 } 3344 3345 /** 3346 * @return Returns a reference to <code>this</code> for easy method chaining 3347 */ 3348 public Encounter setClassHistory(List<ClassHistoryComponent> theClassHistory) { 3349 this.classHistory = theClassHistory; 3350 return this; 3351 } 3352 3353 public boolean hasClassHistory() { 3354 if (this.classHistory == null) 3355 return false; 3356 for (ClassHistoryComponent item : this.classHistory) 3357 if (!item.isEmpty()) 3358 return true; 3359 return false; 3360 } 3361 3362 public ClassHistoryComponent addClassHistory() { // 3 3363 ClassHistoryComponent t = new ClassHistoryComponent(); 3364 if (this.classHistory == null) 3365 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3366 this.classHistory.add(t); 3367 return t; 3368 } 3369 3370 public Encounter addClassHistory(ClassHistoryComponent t) { // 3 3371 if (t == null) 3372 return this; 3373 if (this.classHistory == null) 3374 this.classHistory = new ArrayList<ClassHistoryComponent>(); 3375 this.classHistory.add(t); 3376 return this; 3377 } 3378 3379 /** 3380 * @return The first repetition of repeating field {@link #classHistory}, 3381 * creating it if it does not already exist 3382 */ 3383 public ClassHistoryComponent getClassHistoryFirstRep() { 3384 if (getClassHistory().isEmpty()) { 3385 addClassHistory(); 3386 } 3387 return getClassHistory().get(0); 3388 } 3389 3390 /** 3391 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, 3392 * surgical day-care, skilled nursing, rehabilitation).) 3393 */ 3394 public List<CodeableConcept> getType() { 3395 if (this.type == null) 3396 this.type = new ArrayList<CodeableConcept>(); 3397 return this.type; 3398 } 3399 3400 /** 3401 * @return Returns a reference to <code>this</code> for easy method chaining 3402 */ 3403 public Encounter setType(List<CodeableConcept> theType) { 3404 this.type = theType; 3405 return this; 3406 } 3407 3408 public boolean hasType() { 3409 if (this.type == null) 3410 return false; 3411 for (CodeableConcept item : this.type) 3412 if (!item.isEmpty()) 3413 return true; 3414 return false; 3415 } 3416 3417 public CodeableConcept addType() { // 3 3418 CodeableConcept t = new CodeableConcept(); 3419 if (this.type == null) 3420 this.type = new ArrayList<CodeableConcept>(); 3421 this.type.add(t); 3422 return t; 3423 } 3424 3425 public Encounter addType(CodeableConcept t) { // 3 3426 if (t == null) 3427 return this; 3428 if (this.type == null) 3429 this.type = new ArrayList<CodeableConcept>(); 3430 this.type.add(t); 3431 return this; 3432 } 3433 3434 /** 3435 * @return The first repetition of repeating field {@link #type}, creating it if 3436 * it does not already exist 3437 */ 3438 public CodeableConcept getTypeFirstRep() { 3439 if (getType().isEmpty()) { 3440 addType(); 3441 } 3442 return getType().get(0); 3443 } 3444 3445 /** 3446 * @return {@link #serviceType} (Broad categorization of the service that is to 3447 * be provided (e.g. cardiology).) 3448 */ 3449 public CodeableConcept getServiceType() { 3450 if (this.serviceType == null) 3451 if (Configuration.errorOnAutoCreate()) 3452 throw new Error("Attempt to auto-create Encounter.serviceType"); 3453 else if (Configuration.doAutoCreate()) 3454 this.serviceType = new CodeableConcept(); // cc 3455 return this.serviceType; 3456 } 3457 3458 public boolean hasServiceType() { 3459 return this.serviceType != null && !this.serviceType.isEmpty(); 3460 } 3461 3462 /** 3463 * @param value {@link #serviceType} (Broad categorization of the service that 3464 * is to be provided (e.g. cardiology).) 3465 */ 3466 public Encounter setServiceType(CodeableConcept value) { 3467 this.serviceType = value; 3468 return this; 3469 } 3470 3471 /** 3472 * @return {@link #priority} (Indicates the urgency of the encounter.) 3473 */ 3474 public CodeableConcept getPriority() { 3475 if (this.priority == null) 3476 if (Configuration.errorOnAutoCreate()) 3477 throw new Error("Attempt to auto-create Encounter.priority"); 3478 else if (Configuration.doAutoCreate()) 3479 this.priority = new CodeableConcept(); // cc 3480 return this.priority; 3481 } 3482 3483 public boolean hasPriority() { 3484 return this.priority != null && !this.priority.isEmpty(); 3485 } 3486 3487 /** 3488 * @param value {@link #priority} (Indicates the urgency of the encounter.) 3489 */ 3490 public Encounter setPriority(CodeableConcept value) { 3491 this.priority = value; 3492 return this; 3493 } 3494 3495 /** 3496 * @return {@link #subject} (The patient or group present at the encounter.) 3497 */ 3498 public Reference getSubject() { 3499 if (this.subject == null) 3500 if (Configuration.errorOnAutoCreate()) 3501 throw new Error("Attempt to auto-create Encounter.subject"); 3502 else if (Configuration.doAutoCreate()) 3503 this.subject = new Reference(); // cc 3504 return this.subject; 3505 } 3506 3507 public boolean hasSubject() { 3508 return this.subject != null && !this.subject.isEmpty(); 3509 } 3510 3511 /** 3512 * @param value {@link #subject} (The patient or group present at the 3513 * encounter.) 3514 */ 3515 public Encounter setSubject(Reference value) { 3516 this.subject = value; 3517 return this; 3518 } 3519 3520 /** 3521 * @return {@link #subject} The actual object that is the target of the 3522 * reference. The reference library doesn't populate this, but you can 3523 * use it to hold the resource if you resolve it. (The patient or group 3524 * present at the encounter.) 3525 */ 3526 public Resource getSubjectTarget() { 3527 return this.subjectTarget; 3528 } 3529 3530 /** 3531 * @param value {@link #subject} The actual object that is the target of the 3532 * reference. The reference library doesn't use these, but you can 3533 * use it to hold the resource if you resolve it. (The patient or 3534 * group present at the encounter.) 3535 */ 3536 public Encounter setSubjectTarget(Resource value) { 3537 this.subjectTarget = value; 3538 return this; 3539 } 3540 3541 /** 3542 * @return {@link #episodeOfCare} (Where a specific encounter should be 3543 * classified as a part of a specific episode(s) of care this field 3544 * should be used. This association can facilitate grouping of related 3545 * encounters together for a specific purpose, such as government 3546 * reporting, issue tracking, association via a common problem. The 3547 * association is recorded on the encounter as these are typically 3548 * created after the episode of care and grouped on entry rather than 3549 * editing the episode of care to append another encounter to it (the 3550 * episode of care could span years).) 3551 */ 3552 public List<Reference> getEpisodeOfCare() { 3553 if (this.episodeOfCare == null) 3554 this.episodeOfCare = new ArrayList<Reference>(); 3555 return this.episodeOfCare; 3556 } 3557 3558 /** 3559 * @return Returns a reference to <code>this</code> for easy method chaining 3560 */ 3561 public Encounter setEpisodeOfCare(List<Reference> theEpisodeOfCare) { 3562 this.episodeOfCare = theEpisodeOfCare; 3563 return this; 3564 } 3565 3566 public boolean hasEpisodeOfCare() { 3567 if (this.episodeOfCare == null) 3568 return false; 3569 for (Reference item : this.episodeOfCare) 3570 if (!item.isEmpty()) 3571 return true; 3572 return false; 3573 } 3574 3575 public Reference addEpisodeOfCare() { // 3 3576 Reference t = new Reference(); 3577 if (this.episodeOfCare == null) 3578 this.episodeOfCare = new ArrayList<Reference>(); 3579 this.episodeOfCare.add(t); 3580 return t; 3581 } 3582 3583 public Encounter addEpisodeOfCare(Reference t) { // 3 3584 if (t == null) 3585 return this; 3586 if (this.episodeOfCare == null) 3587 this.episodeOfCare = new ArrayList<Reference>(); 3588 this.episodeOfCare.add(t); 3589 return this; 3590 } 3591 3592 /** 3593 * @return The first repetition of repeating field {@link #episodeOfCare}, 3594 * creating it if it does not already exist 3595 */ 3596 public Reference getEpisodeOfCareFirstRep() { 3597 if (getEpisodeOfCare().isEmpty()) { 3598 addEpisodeOfCare(); 3599 } 3600 return getEpisodeOfCare().get(0); 3601 } 3602 3603 /** 3604 * @deprecated Use Reference#setResource(IBaseResource) instead 3605 */ 3606 @Deprecated 3607 public List<EpisodeOfCare> getEpisodeOfCareTarget() { 3608 if (this.episodeOfCareTarget == null) 3609 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3610 return this.episodeOfCareTarget; 3611 } 3612 3613 /** 3614 * @deprecated Use Reference#setResource(IBaseResource) instead 3615 */ 3616 @Deprecated 3617 public EpisodeOfCare addEpisodeOfCareTarget() { 3618 EpisodeOfCare r = new EpisodeOfCare(); 3619 if (this.episodeOfCareTarget == null) 3620 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3621 this.episodeOfCareTarget.add(r); 3622 return r; 3623 } 3624 3625 /** 3626 * @return {@link #basedOn} (The request this encounter satisfies (e.g. incoming 3627 * referral or procedure request).) 3628 */ 3629 public List<Reference> getBasedOn() { 3630 if (this.basedOn == null) 3631 this.basedOn = new ArrayList<Reference>(); 3632 return this.basedOn; 3633 } 3634 3635 /** 3636 * @return Returns a reference to <code>this</code> for easy method chaining 3637 */ 3638 public Encounter setBasedOn(List<Reference> theBasedOn) { 3639 this.basedOn = theBasedOn; 3640 return this; 3641 } 3642 3643 public boolean hasBasedOn() { 3644 if (this.basedOn == null) 3645 return false; 3646 for (Reference item : this.basedOn) 3647 if (!item.isEmpty()) 3648 return true; 3649 return false; 3650 } 3651 3652 public Reference addBasedOn() { // 3 3653 Reference t = new Reference(); 3654 if (this.basedOn == null) 3655 this.basedOn = new ArrayList<Reference>(); 3656 this.basedOn.add(t); 3657 return t; 3658 } 3659 3660 public Encounter addBasedOn(Reference t) { // 3 3661 if (t == null) 3662 return this; 3663 if (this.basedOn == null) 3664 this.basedOn = new ArrayList<Reference>(); 3665 this.basedOn.add(t); 3666 return this; 3667 } 3668 3669 /** 3670 * @return The first repetition of repeating field {@link #basedOn}, creating it 3671 * if it does not already exist 3672 */ 3673 public Reference getBasedOnFirstRep() { 3674 if (getBasedOn().isEmpty()) { 3675 addBasedOn(); 3676 } 3677 return getBasedOn().get(0); 3678 } 3679 3680 /** 3681 * @deprecated Use Reference#setResource(IBaseResource) instead 3682 */ 3683 @Deprecated 3684 public List<ServiceRequest> getBasedOnTarget() { 3685 if (this.basedOnTarget == null) 3686 this.basedOnTarget = new ArrayList<ServiceRequest>(); 3687 return this.basedOnTarget; 3688 } 3689 3690 /** 3691 * @deprecated Use Reference#setResource(IBaseResource) instead 3692 */ 3693 @Deprecated 3694 public ServiceRequest addBasedOnTarget() { 3695 ServiceRequest r = new ServiceRequest(); 3696 if (this.basedOnTarget == null) 3697 this.basedOnTarget = new ArrayList<ServiceRequest>(); 3698 this.basedOnTarget.add(r); 3699 return r; 3700 } 3701 3702 /** 3703 * @return {@link #participant} (The list of people responsible for providing 3704 * the service.) 3705 */ 3706 public List<EncounterParticipantComponent> getParticipant() { 3707 if (this.participant == null) 3708 this.participant = new ArrayList<EncounterParticipantComponent>(); 3709 return this.participant; 3710 } 3711 3712 /** 3713 * @return Returns a reference to <code>this</code> for easy method chaining 3714 */ 3715 public Encounter setParticipant(List<EncounterParticipantComponent> theParticipant) { 3716 this.participant = theParticipant; 3717 return this; 3718 } 3719 3720 public boolean hasParticipant() { 3721 if (this.participant == null) 3722 return false; 3723 for (EncounterParticipantComponent item : this.participant) 3724 if (!item.isEmpty()) 3725 return true; 3726 return false; 3727 } 3728 3729 public EncounterParticipantComponent addParticipant() { // 3 3730 EncounterParticipantComponent t = new EncounterParticipantComponent(); 3731 if (this.participant == null) 3732 this.participant = new ArrayList<EncounterParticipantComponent>(); 3733 this.participant.add(t); 3734 return t; 3735 } 3736 3737 public Encounter addParticipant(EncounterParticipantComponent t) { // 3 3738 if (t == null) 3739 return this; 3740 if (this.participant == null) 3741 this.participant = new ArrayList<EncounterParticipantComponent>(); 3742 this.participant.add(t); 3743 return this; 3744 } 3745 3746 /** 3747 * @return The first repetition of repeating field {@link #participant}, 3748 * creating it if it does not already exist 3749 */ 3750 public EncounterParticipantComponent getParticipantFirstRep() { 3751 if (getParticipant().isEmpty()) { 3752 addParticipant(); 3753 } 3754 return getParticipant().get(0); 3755 } 3756 3757 /** 3758 * @return {@link #appointment} (The appointment that scheduled this encounter.) 3759 */ 3760 public List<Reference> getAppointment() { 3761 if (this.appointment == null) 3762 this.appointment = new ArrayList<Reference>(); 3763 return this.appointment; 3764 } 3765 3766 /** 3767 * @return Returns a reference to <code>this</code> for easy method chaining 3768 */ 3769 public Encounter setAppointment(List<Reference> theAppointment) { 3770 this.appointment = theAppointment; 3771 return this; 3772 } 3773 3774 public boolean hasAppointment() { 3775 if (this.appointment == null) 3776 return false; 3777 for (Reference item : this.appointment) 3778 if (!item.isEmpty()) 3779 return true; 3780 return false; 3781 } 3782 3783 public Reference addAppointment() { // 3 3784 Reference t = new Reference(); 3785 if (this.appointment == null) 3786 this.appointment = new ArrayList<Reference>(); 3787 this.appointment.add(t); 3788 return t; 3789 } 3790 3791 public Encounter addAppointment(Reference t) { // 3 3792 if (t == null) 3793 return this; 3794 if (this.appointment == null) 3795 this.appointment = new ArrayList<Reference>(); 3796 this.appointment.add(t); 3797 return this; 3798 } 3799 3800 /** 3801 * @return The first repetition of repeating field {@link #appointment}, 3802 * creating it if it does not already exist 3803 */ 3804 public Reference getAppointmentFirstRep() { 3805 if (getAppointment().isEmpty()) { 3806 addAppointment(); 3807 } 3808 return getAppointment().get(0); 3809 } 3810 3811 /** 3812 * @deprecated Use Reference#setResource(IBaseResource) instead 3813 */ 3814 @Deprecated 3815 public List<Appointment> getAppointmentTarget() { 3816 if (this.appointmentTarget == null) 3817 this.appointmentTarget = new ArrayList<Appointment>(); 3818 return this.appointmentTarget; 3819 } 3820 3821 /** 3822 * @deprecated Use Reference#setResource(IBaseResource) instead 3823 */ 3824 @Deprecated 3825 public Appointment addAppointmentTarget() { 3826 Appointment r = new Appointment(); 3827 if (this.appointmentTarget == null) 3828 this.appointmentTarget = new ArrayList<Appointment>(); 3829 this.appointmentTarget.add(r); 3830 return r; 3831 } 3832 3833 /** 3834 * @return {@link #period} (The start and end time of the encounter.) 3835 */ 3836 public Period getPeriod() { 3837 if (this.period == null) 3838 if (Configuration.errorOnAutoCreate()) 3839 throw new Error("Attempt to auto-create Encounter.period"); 3840 else if (Configuration.doAutoCreate()) 3841 this.period = new Period(); // cc 3842 return this.period; 3843 } 3844 3845 public boolean hasPeriod() { 3846 return this.period != null && !this.period.isEmpty(); 3847 } 3848 3849 /** 3850 * @param value {@link #period} (The start and end time of the encounter.) 3851 */ 3852 public Encounter setPeriod(Period value) { 3853 this.period = value; 3854 return this; 3855 } 3856 3857 /** 3858 * @return {@link #length} (Quantity of time the encounter lasted. This excludes 3859 * the time during leaves of absence.) 3860 */ 3861 public Duration getLength() { 3862 if (this.length == null) 3863 if (Configuration.errorOnAutoCreate()) 3864 throw new Error("Attempt to auto-create Encounter.length"); 3865 else if (Configuration.doAutoCreate()) 3866 this.length = new Duration(); // cc 3867 return this.length; 3868 } 3869 3870 public boolean hasLength() { 3871 return this.length != null && !this.length.isEmpty(); 3872 } 3873 3874 /** 3875 * @param value {@link #length} (Quantity of time the encounter lasted. This 3876 * excludes the time during leaves of absence.) 3877 */ 3878 public Encounter setLength(Duration value) { 3879 this.length = value; 3880 return this; 3881 } 3882 3883 /** 3884 * @return {@link #reasonCode} (Reason the encounter takes place, expressed as a 3885 * code. For admissions, this can be used for a coded admission 3886 * diagnosis.) 3887 */ 3888 public List<CodeableConcept> getReasonCode() { 3889 if (this.reasonCode == null) 3890 this.reasonCode = new ArrayList<CodeableConcept>(); 3891 return this.reasonCode; 3892 } 3893 3894 /** 3895 * @return Returns a reference to <code>this</code> for easy method chaining 3896 */ 3897 public Encounter setReasonCode(List<CodeableConcept> theReasonCode) { 3898 this.reasonCode = theReasonCode; 3899 return this; 3900 } 3901 3902 public boolean hasReasonCode() { 3903 if (this.reasonCode == null) 3904 return false; 3905 for (CodeableConcept item : this.reasonCode) 3906 if (!item.isEmpty()) 3907 return true; 3908 return false; 3909 } 3910 3911 public CodeableConcept addReasonCode() { // 3 3912 CodeableConcept t = new CodeableConcept(); 3913 if (this.reasonCode == null) 3914 this.reasonCode = new ArrayList<CodeableConcept>(); 3915 this.reasonCode.add(t); 3916 return t; 3917 } 3918 3919 public Encounter addReasonCode(CodeableConcept t) { // 3 3920 if (t == null) 3921 return this; 3922 if (this.reasonCode == null) 3923 this.reasonCode = new ArrayList<CodeableConcept>(); 3924 this.reasonCode.add(t); 3925 return this; 3926 } 3927 3928 /** 3929 * @return The first repetition of repeating field {@link #reasonCode}, creating 3930 * it if it does not already exist 3931 */ 3932 public CodeableConcept getReasonCodeFirstRep() { 3933 if (getReasonCode().isEmpty()) { 3934 addReasonCode(); 3935 } 3936 return getReasonCode().get(0); 3937 } 3938 3939 /** 3940 * @return {@link #reasonReference} (Reason the encounter takes place, expressed 3941 * as a code. For admissions, this can be used for a coded admission 3942 * diagnosis.) 3943 */ 3944 public List<Reference> getReasonReference() { 3945 if (this.reasonReference == null) 3946 this.reasonReference = new ArrayList<Reference>(); 3947 return this.reasonReference; 3948 } 3949 3950 /** 3951 * @return Returns a reference to <code>this</code> for easy method chaining 3952 */ 3953 public Encounter setReasonReference(List<Reference> theReasonReference) { 3954 this.reasonReference = theReasonReference; 3955 return this; 3956 } 3957 3958 public boolean hasReasonReference() { 3959 if (this.reasonReference == null) 3960 return false; 3961 for (Reference item : this.reasonReference) 3962 if (!item.isEmpty()) 3963 return true; 3964 return false; 3965 } 3966 3967 public Reference addReasonReference() { // 3 3968 Reference t = new Reference(); 3969 if (this.reasonReference == null) 3970 this.reasonReference = new ArrayList<Reference>(); 3971 this.reasonReference.add(t); 3972 return t; 3973 } 3974 3975 public Encounter addReasonReference(Reference t) { // 3 3976 if (t == null) 3977 return this; 3978 if (this.reasonReference == null) 3979 this.reasonReference = new ArrayList<Reference>(); 3980 this.reasonReference.add(t); 3981 return this; 3982 } 3983 3984 /** 3985 * @return The first repetition of repeating field {@link #reasonReference}, 3986 * creating it if it does not already exist 3987 */ 3988 public Reference getReasonReferenceFirstRep() { 3989 if (getReasonReference().isEmpty()) { 3990 addReasonReference(); 3991 } 3992 return getReasonReference().get(0); 3993 } 3994 3995 /** 3996 * @deprecated Use Reference#setResource(IBaseResource) instead 3997 */ 3998 @Deprecated 3999 public List<Resource> getReasonReferenceTarget() { 4000 if (this.reasonReferenceTarget == null) 4001 this.reasonReferenceTarget = new ArrayList<Resource>(); 4002 return this.reasonReferenceTarget; 4003 } 4004 4005 /** 4006 * @return {@link #diagnosis} (The list of diagnosis relevant to this 4007 * encounter.) 4008 */ 4009 public List<DiagnosisComponent> getDiagnosis() { 4010 if (this.diagnosis == null) 4011 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4012 return this.diagnosis; 4013 } 4014 4015 /** 4016 * @return Returns a reference to <code>this</code> for easy method chaining 4017 */ 4018 public Encounter setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 4019 this.diagnosis = theDiagnosis; 4020 return this; 4021 } 4022 4023 public boolean hasDiagnosis() { 4024 if (this.diagnosis == null) 4025 return false; 4026 for (DiagnosisComponent item : this.diagnosis) 4027 if (!item.isEmpty()) 4028 return true; 4029 return false; 4030 } 4031 4032 public DiagnosisComponent addDiagnosis() { // 3 4033 DiagnosisComponent t = new DiagnosisComponent(); 4034 if (this.diagnosis == null) 4035 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4036 this.diagnosis.add(t); 4037 return t; 4038 } 4039 4040 public Encounter addDiagnosis(DiagnosisComponent t) { // 3 4041 if (t == null) 4042 return this; 4043 if (this.diagnosis == null) 4044 this.diagnosis = new ArrayList<DiagnosisComponent>(); 4045 this.diagnosis.add(t); 4046 return this; 4047 } 4048 4049 /** 4050 * @return The first repetition of repeating field {@link #diagnosis}, creating 4051 * it if it does not already exist 4052 */ 4053 public DiagnosisComponent getDiagnosisFirstRep() { 4054 if (getDiagnosis().isEmpty()) { 4055 addDiagnosis(); 4056 } 4057 return getDiagnosis().get(0); 4058 } 4059 4060 /** 4061 * @return {@link #account} (The set of accounts that may be used for billing 4062 * for this Encounter.) 4063 */ 4064 public List<Reference> getAccount() { 4065 if (this.account == null) 4066 this.account = new ArrayList<Reference>(); 4067 return this.account; 4068 } 4069 4070 /** 4071 * @return Returns a reference to <code>this</code> for easy method chaining 4072 */ 4073 public Encounter setAccount(List<Reference> theAccount) { 4074 this.account = theAccount; 4075 return this; 4076 } 4077 4078 public boolean hasAccount() { 4079 if (this.account == null) 4080 return false; 4081 for (Reference item : this.account) 4082 if (!item.isEmpty()) 4083 return true; 4084 return false; 4085 } 4086 4087 public Reference addAccount() { // 3 4088 Reference t = new Reference(); 4089 if (this.account == null) 4090 this.account = new ArrayList<Reference>(); 4091 this.account.add(t); 4092 return t; 4093 } 4094 4095 public Encounter addAccount(Reference t) { // 3 4096 if (t == null) 4097 return this; 4098 if (this.account == null) 4099 this.account = new ArrayList<Reference>(); 4100 this.account.add(t); 4101 return this; 4102 } 4103 4104 /** 4105 * @return The first repetition of repeating field {@link #account}, creating it 4106 * if it does not already exist 4107 */ 4108 public Reference getAccountFirstRep() { 4109 if (getAccount().isEmpty()) { 4110 addAccount(); 4111 } 4112 return getAccount().get(0); 4113 } 4114 4115 /** 4116 * @deprecated Use Reference#setResource(IBaseResource) instead 4117 */ 4118 @Deprecated 4119 public List<Account> getAccountTarget() { 4120 if (this.accountTarget == null) 4121 this.accountTarget = new ArrayList<Account>(); 4122 return this.accountTarget; 4123 } 4124 4125 /** 4126 * @deprecated Use Reference#setResource(IBaseResource) instead 4127 */ 4128 @Deprecated 4129 public Account addAccountTarget() { 4130 Account r = new Account(); 4131 if (this.accountTarget == null) 4132 this.accountTarget = new ArrayList<Account>(); 4133 this.accountTarget.add(r); 4134 return r; 4135 } 4136 4137 /** 4138 * @return {@link #hospitalization} (Details about the admission to a healthcare 4139 * service.) 4140 */ 4141 public EncounterHospitalizationComponent getHospitalization() { 4142 if (this.hospitalization == null) 4143 if (Configuration.errorOnAutoCreate()) 4144 throw new Error("Attempt to auto-create Encounter.hospitalization"); 4145 else if (Configuration.doAutoCreate()) 4146 this.hospitalization = new EncounterHospitalizationComponent(); // cc 4147 return this.hospitalization; 4148 } 4149 4150 public boolean hasHospitalization() { 4151 return this.hospitalization != null && !this.hospitalization.isEmpty(); 4152 } 4153 4154 /** 4155 * @param value {@link #hospitalization} (Details about the admission to a 4156 * healthcare service.) 4157 */ 4158 public Encounter setHospitalization(EncounterHospitalizationComponent value) { 4159 this.hospitalization = value; 4160 return this; 4161 } 4162 4163 /** 4164 * @return {@link #location} (List of locations where the patient has been 4165 * during this encounter.) 4166 */ 4167 public List<EncounterLocationComponent> getLocation() { 4168 if (this.location == null) 4169 this.location = new ArrayList<EncounterLocationComponent>(); 4170 return this.location; 4171 } 4172 4173 /** 4174 * @return Returns a reference to <code>this</code> for easy method chaining 4175 */ 4176 public Encounter setLocation(List<EncounterLocationComponent> theLocation) { 4177 this.location = theLocation; 4178 return this; 4179 } 4180 4181 public boolean hasLocation() { 4182 if (this.location == null) 4183 return false; 4184 for (EncounterLocationComponent item : this.location) 4185 if (!item.isEmpty()) 4186 return true; 4187 return false; 4188 } 4189 4190 public EncounterLocationComponent addLocation() { // 3 4191 EncounterLocationComponent t = new EncounterLocationComponent(); 4192 if (this.location == null) 4193 this.location = new ArrayList<EncounterLocationComponent>(); 4194 this.location.add(t); 4195 return t; 4196 } 4197 4198 public Encounter addLocation(EncounterLocationComponent t) { // 3 4199 if (t == null) 4200 return this; 4201 if (this.location == null) 4202 this.location = new ArrayList<EncounterLocationComponent>(); 4203 this.location.add(t); 4204 return this; 4205 } 4206 4207 /** 4208 * @return The first repetition of repeating field {@link #location}, creating 4209 * it if it does not already exist 4210 */ 4211 public EncounterLocationComponent getLocationFirstRep() { 4212 if (getLocation().isEmpty()) { 4213 addLocation(); 4214 } 4215 return getLocation().get(0); 4216 } 4217 4218 /** 4219 * @return {@link #serviceProvider} (The organization that is primarily 4220 * responsible for this Encounter's services. This MAY be the same as 4221 * the organization on the Patient record, however it could be 4222 * different, such as if the actor performing the services was from an 4223 * external organization (which may be billed seperately) for an 4224 * external consultation. Refer to the example bundle showing an 4225 * abbreviated set of Encounters for a colonoscopy.) 4226 */ 4227 public Reference getServiceProvider() { 4228 if (this.serviceProvider == null) 4229 if (Configuration.errorOnAutoCreate()) 4230 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 4231 else if (Configuration.doAutoCreate()) 4232 this.serviceProvider = new Reference(); // cc 4233 return this.serviceProvider; 4234 } 4235 4236 public boolean hasServiceProvider() { 4237 return this.serviceProvider != null && !this.serviceProvider.isEmpty(); 4238 } 4239 4240 /** 4241 * @param value {@link #serviceProvider} (The organization that is primarily 4242 * responsible for this Encounter's services. This MAY be the same 4243 * as the organization on the Patient record, however it could be 4244 * different, such as if the actor performing the services was from 4245 * an external organization (which may be billed seperately) for an 4246 * external consultation. Refer to the example bundle showing an 4247 * abbreviated set of Encounters for a colonoscopy.) 4248 */ 4249 public Encounter setServiceProvider(Reference value) { 4250 this.serviceProvider = value; 4251 return this; 4252 } 4253 4254 /** 4255 * @return {@link #serviceProvider} The actual object that is the target of the 4256 * reference. The reference library doesn't populate this, but you can 4257 * use it to hold the resource if you resolve it. (The organization that 4258 * is primarily responsible for this Encounter's services. This MAY be 4259 * the same as the organization on the Patient record, however it could 4260 * be different, such as if the actor performing the services was from 4261 * an external organization (which may be billed seperately) for an 4262 * external consultation. Refer to the example bundle showing an 4263 * abbreviated set of Encounters for a colonoscopy.) 4264 */ 4265 public Organization getServiceProviderTarget() { 4266 if (this.serviceProviderTarget == null) 4267 if (Configuration.errorOnAutoCreate()) 4268 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 4269 else if (Configuration.doAutoCreate()) 4270 this.serviceProviderTarget = new Organization(); // aa 4271 return this.serviceProviderTarget; 4272 } 4273 4274 /** 4275 * @param value {@link #serviceProvider} The actual object that is the target of 4276 * the reference. The reference library doesn't use these, but you 4277 * can use it to hold the resource if you resolve it. (The 4278 * organization that is primarily responsible for this Encounter's 4279 * services. This MAY be the same as the organization on the 4280 * Patient record, however it could be different, such as if the 4281 * actor performing the services was from an external organization 4282 * (which may be billed seperately) for an external consultation. 4283 * Refer to the example bundle showing an abbreviated set of 4284 * Encounters for a colonoscopy.) 4285 */ 4286 public Encounter setServiceProviderTarget(Organization value) { 4287 this.serviceProviderTarget = value; 4288 return this; 4289 } 4290 4291 /** 4292 * @return {@link #partOf} (Another Encounter of which this encounter is a part 4293 * of (administratively or in time).) 4294 */ 4295 public Reference getPartOf() { 4296 if (this.partOf == null) 4297 if (Configuration.errorOnAutoCreate()) 4298 throw new Error("Attempt to auto-create Encounter.partOf"); 4299 else if (Configuration.doAutoCreate()) 4300 this.partOf = new Reference(); // cc 4301 return this.partOf; 4302 } 4303 4304 public boolean hasPartOf() { 4305 return this.partOf != null && !this.partOf.isEmpty(); 4306 } 4307 4308 /** 4309 * @param value {@link #partOf} (Another Encounter of which this encounter is a 4310 * part of (administratively or in time).) 4311 */ 4312 public Encounter setPartOf(Reference value) { 4313 this.partOf = value; 4314 return this; 4315 } 4316 4317 /** 4318 * @return {@link #partOf} The actual object that is the target of the 4319 * reference. The reference library doesn't populate this, but you can 4320 * use it to hold the resource if you resolve it. (Another Encounter of 4321 * which this encounter is a part of (administratively or in time).) 4322 */ 4323 public Encounter getPartOfTarget() { 4324 if (this.partOfTarget == null) 4325 if (Configuration.errorOnAutoCreate()) 4326 throw new Error("Attempt to auto-create Encounter.partOf"); 4327 else if (Configuration.doAutoCreate()) 4328 this.partOfTarget = new Encounter(); // aa 4329 return this.partOfTarget; 4330 } 4331 4332 /** 4333 * @param value {@link #partOf} The actual object that is the target of the 4334 * reference. The reference library doesn't use these, but you can 4335 * use it to hold the resource if you resolve it. (Another 4336 * Encounter of which this encounter is a part of (administratively 4337 * or in time).) 4338 */ 4339 public Encounter setPartOfTarget(Encounter value) { 4340 this.partOfTarget = value; 4341 return this; 4342 } 4343 4344 protected void listChildren(List<Property> children) { 4345 super.listChildren(children); 4346 children.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, 4347 java.lang.Integer.MAX_VALUE, identifier)); 4348 children.add(new Property("status", "code", 4349 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 4350 children.add(new Property("statusHistory", "", 4351 "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 4352 0, java.lang.Integer.MAX_VALUE, statusHistory)); 4353 children.add(new Property("class", "Coding", 4354 "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 4355 0, 1, class_)); 4356 children.add(new Property("classHistory", "", 4357 "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.", 4358 0, java.lang.Integer.MAX_VALUE, classHistory)); 4359 children.add(new Property("type", "CodeableConcept", 4360 "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, 4361 java.lang.Integer.MAX_VALUE, type)); 4362 children.add(new Property("serviceType", "CodeableConcept", 4363 "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, 1, serviceType)); 4364 children 4365 .add(new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority)); 4366 children.add(new Property("subject", "Reference(Patient|Group)", "The patient or group present at the encounter.", 4367 0, 1, subject)); 4368 children.add(new Property("episodeOfCare", "Reference(EpisodeOfCare)", 4369 "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 4370 0, java.lang.Integer.MAX_VALUE, episodeOfCare)); 4371 children.add(new Property("basedOn", "Reference(ServiceRequest)", 4372 "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, 4373 java.lang.Integer.MAX_VALUE, basedOn)); 4374 children.add(new Property("participant", "", "The list of people responsible for providing the service.", 0, 4375 java.lang.Integer.MAX_VALUE, participant)); 4376 children.add(new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 4377 0, java.lang.Integer.MAX_VALUE, appointment)); 4378 children.add(new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period)); 4379 children.add(new Property("length", "Duration", 4380 "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length)); 4381 children.add(new Property("reasonCode", "CodeableConcept", 4382 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4383 0, java.lang.Integer.MAX_VALUE, reasonCode)); 4384 children.add(new Property("reasonReference", 4385 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 4386 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4387 0, java.lang.Integer.MAX_VALUE, reasonReference)); 4388 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, 4389 java.lang.Integer.MAX_VALUE, diagnosis)); 4390 children.add(new Property("account", "Reference(Account)", 4391 "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, 4392 account)); 4393 children.add(new Property("hospitalization", "", "Details about the admission to a healthcare service.", 0, 1, 4394 hospitalization)); 4395 children.add(new Property("location", "", "List of locations where the patient has been during this encounter.", 0, 4396 java.lang.Integer.MAX_VALUE, location)); 4397 children.add(new Property("serviceProvider", "Reference(Organization)", 4398 "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.", 4399 0, 1, serviceProvider)); 4400 children.add(new Property("partOf", "Reference(Encounter)", 4401 "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf)); 4402 } 4403 4404 @Override 4405 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4406 switch (_hash) { 4407 case -1618432855: 4408 /* identifier */ return new Property("identifier", "Identifier", 4409 "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 4410 case -892481550: 4411 /* status */ return new Property("status", "code", 4412 "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 4413 case -986695614: 4414 /* statusHistory */ return new Property("statusHistory", "", 4415 "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 4416 0, java.lang.Integer.MAX_VALUE, statusHistory); 4417 case 94742904: 4418 /* class */ return new Property("class", "Coding", 4419 "Concepts representing classification of patient encounter such as ambulatory (outpatient), inpatient, emergency, home health or others due to local variations.", 4420 0, 1, class_); 4421 case 962575356: 4422 /* classHistory */ return new Property("classHistory", "", 4423 "The class history permits the tracking of the encounters transitions without needing to go through the resource history. This would be used for a case where an admission starts of as an emergency encounter, then transitions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kind of discharge from emergency to inpatient.", 4424 0, java.lang.Integer.MAX_VALUE, classHistory); 4425 case 3575610: 4426 /* type */ return new Property("type", "CodeableConcept", 4427 "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 4428 0, java.lang.Integer.MAX_VALUE, type); 4429 case -1928370289: 4430 /* serviceType */ return new Property("serviceType", "CodeableConcept", 4431 "Broad categorization of the service that is to be provided (e.g. cardiology).", 0, 1, serviceType); 4432 case -1165461084: 4433 /* priority */ return new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, 4434 priority); 4435 case -1867885268: 4436 /* subject */ return new Property("subject", "Reference(Patient|Group)", 4437 "The patient or group present at the encounter.", 0, 1, subject); 4438 case -1892140189: 4439 /* episodeOfCare */ return new Property("episodeOfCare", "Reference(EpisodeOfCare)", 4440 "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 4441 0, java.lang.Integer.MAX_VALUE, episodeOfCare); 4442 case -332612366: 4443 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest)", 4444 "The request this encounter satisfies (e.g. incoming referral or procedure request).", 0, 4445 java.lang.Integer.MAX_VALUE, basedOn); 4446 case 767422259: 4447 /* participant */ return new Property("participant", "", 4448 "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant); 4449 case -1474995297: 4450 /* appointment */ return new Property("appointment", "Reference(Appointment)", 4451 "The appointment that scheduled this encounter.", 0, java.lang.Integer.MAX_VALUE, appointment); 4452 case -991726143: 4453 /* period */ return new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period); 4454 case -1106363674: 4455 /* length */ return new Property("length", "Duration", 4456 "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length); 4457 case 722137681: 4458 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 4459 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4460 0, java.lang.Integer.MAX_VALUE, reasonCode); 4461 case -1146218137: 4462 /* reasonReference */ return new Property("reasonReference", 4463 "Reference(Condition|Procedure|Observation|ImmunizationRecommendation)", 4464 "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 4465 0, java.lang.Integer.MAX_VALUE, reasonReference); 4466 case 1196993265: 4467 /* diagnosis */ return new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, 4468 java.lang.Integer.MAX_VALUE, diagnosis); 4469 case -1177318867: 4470 /* account */ return new Property("account", "Reference(Account)", 4471 "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, 4472 account); 4473 case 1057894634: 4474 /* hospitalization */ return new Property("hospitalization", "", 4475 "Details about the admission to a healthcare service.", 0, 1, hospitalization); 4476 case 1901043637: 4477 /* location */ return new Property("location", "", 4478 "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, 4479 location); 4480 case 243182534: 4481 /* serviceProvider */ return new Property("serviceProvider", "Reference(Organization)", 4482 "The organization that is primarily responsible for this Encounter's services. This MAY be the same as the organization on the Patient record, however it could be different, such as if the actor performing the services was from an external organization (which may be billed seperately) for an external consultation. Refer to the example bundle showing an abbreviated set of Encounters for a colonoscopy.", 4483 0, 1, serviceProvider); 4484 case -995410646: 4485 /* partOf */ return new Property("partOf", "Reference(Encounter)", 4486 "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf); 4487 default: 4488 return super.getNamedProperty(_hash, _name, _checkValid); 4489 } 4490 4491 } 4492 4493 @Override 4494 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4495 switch (hash) { 4496 case -1618432855: 4497 /* identifier */ return this.identifier == null ? new Base[0] 4498 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4499 case -892481550: 4500 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EncounterStatus> 4501 case -986695614: 4502 /* statusHistory */ return this.statusHistory == null ? new Base[0] 4503 : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // StatusHistoryComponent 4504 case 94742904: 4505 /* class */ return this.class_ == null ? new Base[0] : new Base[] { this.class_ }; // Coding 4506 case 962575356: 4507 /* classHistory */ return this.classHistory == null ? new Base[0] 4508 : this.classHistory.toArray(new Base[this.classHistory.size()]); // ClassHistoryComponent 4509 case 3575610: 4510 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4511 case -1928370289: 4512 /* serviceType */ return this.serviceType == null ? new Base[0] : new Base[] { this.serviceType }; // CodeableConcept 4513 case -1165461084: 4514 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // CodeableConcept 4515 case -1867885268: 4516 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 4517 case -1892140189: 4518 /* episodeOfCare */ return this.episodeOfCare == null ? new Base[0] 4519 : this.episodeOfCare.toArray(new Base[this.episodeOfCare.size()]); // Reference 4520 case -332612366: 4521 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4522 case 767422259: 4523 /* participant */ return this.participant == null ? new Base[0] 4524 : this.participant.toArray(new Base[this.participant.size()]); // EncounterParticipantComponent 4525 case -1474995297: 4526 /* appointment */ return this.appointment == null ? new Base[0] 4527 : this.appointment.toArray(new Base[this.appointment.size()]); // Reference 4528 case -991726143: 4529 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4530 case -1106363674: 4531 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // Duration 4532 case 722137681: 4533 /* reasonCode */ return this.reasonCode == null ? new Base[0] 4534 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 4535 case -1146218137: 4536 /* reasonReference */ return this.reasonReference == null ? new Base[0] 4537 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 4538 case 1196993265: 4539 /* diagnosis */ return this.diagnosis == null ? new Base[0] 4540 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 4541 case -1177318867: 4542 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 4543 case 1057894634: 4544 /* hospitalization */ return this.hospitalization == null ? new Base[0] : new Base[] { this.hospitalization }; // EncounterHospitalizationComponent 4545 case 1901043637: 4546 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // EncounterLocationComponent 4547 case 243182534: 4548 /* serviceProvider */ return this.serviceProvider == null ? new Base[0] : new Base[] { this.serviceProvider }; // Reference 4549 case -995410646: 4550 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 4551 default: 4552 return super.getProperty(hash, name, checkValid); 4553 } 4554 4555 } 4556 4557 @Override 4558 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4559 switch (hash) { 4560 case -1618432855: // identifier 4561 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4562 return value; 4563 case -892481550: // status 4564 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 4565 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 4566 return value; 4567 case -986695614: // statusHistory 4568 this.getStatusHistory().add((StatusHistoryComponent) value); // StatusHistoryComponent 4569 return value; 4570 case 94742904: // class 4571 this.class_ = castToCoding(value); // Coding 4572 return value; 4573 case 962575356: // classHistory 4574 this.getClassHistory().add((ClassHistoryComponent) value); // ClassHistoryComponent 4575 return value; 4576 case 3575610: // type 4577 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 4578 return value; 4579 case -1928370289: // serviceType 4580 this.serviceType = castToCodeableConcept(value); // CodeableConcept 4581 return value; 4582 case -1165461084: // priority 4583 this.priority = castToCodeableConcept(value); // CodeableConcept 4584 return value; 4585 case -1867885268: // subject 4586 this.subject = castToReference(value); // Reference 4587 return value; 4588 case -1892140189: // episodeOfCare 4589 this.getEpisodeOfCare().add(castToReference(value)); // Reference 4590 return value; 4591 case -332612366: // basedOn 4592 this.getBasedOn().add(castToReference(value)); // Reference 4593 return value; 4594 case 767422259: // participant 4595 this.getParticipant().add((EncounterParticipantComponent) value); // EncounterParticipantComponent 4596 return value; 4597 case -1474995297: // appointment 4598 this.getAppointment().add(castToReference(value)); // Reference 4599 return value; 4600 case -991726143: // period 4601 this.period = castToPeriod(value); // Period 4602 return value; 4603 case -1106363674: // length 4604 this.length = castToDuration(value); // Duration 4605 return value; 4606 case 722137681: // reasonCode 4607 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 4608 return value; 4609 case -1146218137: // reasonReference 4610 this.getReasonReference().add(castToReference(value)); // Reference 4611 return value; 4612 case 1196993265: // diagnosis 4613 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 4614 return value; 4615 case -1177318867: // account 4616 this.getAccount().add(castToReference(value)); // Reference 4617 return value; 4618 case 1057894634: // hospitalization 4619 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 4620 return value; 4621 case 1901043637: // location 4622 this.getLocation().add((EncounterLocationComponent) value); // EncounterLocationComponent 4623 return value; 4624 case 243182534: // serviceProvider 4625 this.serviceProvider = castToReference(value); // Reference 4626 return value; 4627 case -995410646: // partOf 4628 this.partOf = castToReference(value); // Reference 4629 return value; 4630 default: 4631 return super.setProperty(hash, name, value); 4632 } 4633 4634 } 4635 4636 @Override 4637 public Base setProperty(String name, Base value) throws FHIRException { 4638 if (name.equals("identifier")) { 4639 this.getIdentifier().add(castToIdentifier(value)); 4640 } else if (name.equals("status")) { 4641 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 4642 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 4643 } else if (name.equals("statusHistory")) { 4644 this.getStatusHistory().add((StatusHistoryComponent) value); 4645 } else if (name.equals("class")) { 4646 this.class_ = castToCoding(value); // Coding 4647 } else if (name.equals("classHistory")) { 4648 this.getClassHistory().add((ClassHistoryComponent) value); 4649 } else if (name.equals("type")) { 4650 this.getType().add(castToCodeableConcept(value)); 4651 } else if (name.equals("serviceType")) { 4652 this.serviceType = castToCodeableConcept(value); // CodeableConcept 4653 } else if (name.equals("priority")) { 4654 this.priority = castToCodeableConcept(value); // CodeableConcept 4655 } else if (name.equals("subject")) { 4656 this.subject = castToReference(value); // Reference 4657 } else if (name.equals("episodeOfCare")) { 4658 this.getEpisodeOfCare().add(castToReference(value)); 4659 } else if (name.equals("basedOn")) { 4660 this.getBasedOn().add(castToReference(value)); 4661 } else if (name.equals("participant")) { 4662 this.getParticipant().add((EncounterParticipantComponent) value); 4663 } else if (name.equals("appointment")) { 4664 this.getAppointment().add(castToReference(value)); 4665 } else if (name.equals("period")) { 4666 this.period = castToPeriod(value); // Period 4667 } else if (name.equals("length")) { 4668 this.length = castToDuration(value); // Duration 4669 } else if (name.equals("reasonCode")) { 4670 this.getReasonCode().add(castToCodeableConcept(value)); 4671 } else if (name.equals("reasonReference")) { 4672 this.getReasonReference().add(castToReference(value)); 4673 } else if (name.equals("diagnosis")) { 4674 this.getDiagnosis().add((DiagnosisComponent) value); 4675 } else if (name.equals("account")) { 4676 this.getAccount().add(castToReference(value)); 4677 } else if (name.equals("hospitalization")) { 4678 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 4679 } else if (name.equals("location")) { 4680 this.getLocation().add((EncounterLocationComponent) value); 4681 } else if (name.equals("serviceProvider")) { 4682 this.serviceProvider = castToReference(value); // Reference 4683 } else if (name.equals("partOf")) { 4684 this.partOf = castToReference(value); // Reference 4685 } else 4686 return super.setProperty(name, value); 4687 return value; 4688 } 4689 4690 @Override 4691 public Base makeProperty(int hash, String name) throws FHIRException { 4692 switch (hash) { 4693 case -1618432855: 4694 return addIdentifier(); 4695 case -892481550: 4696 return getStatusElement(); 4697 case -986695614: 4698 return addStatusHistory(); 4699 case 94742904: 4700 return getClass_(); 4701 case 962575356: 4702 return addClassHistory(); 4703 case 3575610: 4704 return addType(); 4705 case -1928370289: 4706 return getServiceType(); 4707 case -1165461084: 4708 return getPriority(); 4709 case -1867885268: 4710 return getSubject(); 4711 case -1892140189: 4712 return addEpisodeOfCare(); 4713 case -332612366: 4714 return addBasedOn(); 4715 case 767422259: 4716 return addParticipant(); 4717 case -1474995297: 4718 return addAppointment(); 4719 case -991726143: 4720 return getPeriod(); 4721 case -1106363674: 4722 return getLength(); 4723 case 722137681: 4724 return addReasonCode(); 4725 case -1146218137: 4726 return addReasonReference(); 4727 case 1196993265: 4728 return addDiagnosis(); 4729 case -1177318867: 4730 return addAccount(); 4731 case 1057894634: 4732 return getHospitalization(); 4733 case 1901043637: 4734 return addLocation(); 4735 case 243182534: 4736 return getServiceProvider(); 4737 case -995410646: 4738 return getPartOf(); 4739 default: 4740 return super.makeProperty(hash, name); 4741 } 4742 4743 } 4744 4745 @Override 4746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4747 switch (hash) { 4748 case -1618432855: 4749 /* identifier */ return new String[] { "Identifier" }; 4750 case -892481550: 4751 /* status */ return new String[] { "code" }; 4752 case -986695614: 4753 /* statusHistory */ return new String[] {}; 4754 case 94742904: 4755 /* class */ return new String[] { "Coding" }; 4756 case 962575356: 4757 /* classHistory */ return new String[] {}; 4758 case 3575610: 4759 /* type */ return new String[] { "CodeableConcept" }; 4760 case -1928370289: 4761 /* serviceType */ return new String[] { "CodeableConcept" }; 4762 case -1165461084: 4763 /* priority */ return new String[] { "CodeableConcept" }; 4764 case -1867885268: 4765 /* subject */ return new String[] { "Reference" }; 4766 case -1892140189: 4767 /* episodeOfCare */ return new String[] { "Reference" }; 4768 case -332612366: 4769 /* basedOn */ return new String[] { "Reference" }; 4770 case 767422259: 4771 /* participant */ return new String[] {}; 4772 case -1474995297: 4773 /* appointment */ return new String[] { "Reference" }; 4774 case -991726143: 4775 /* period */ return new String[] { "Period" }; 4776 case -1106363674: 4777 /* length */ return new String[] { "Duration" }; 4778 case 722137681: 4779 /* reasonCode */ return new String[] { "CodeableConcept" }; 4780 case -1146218137: 4781 /* reasonReference */ return new String[] { "Reference" }; 4782 case 1196993265: 4783 /* diagnosis */ return new String[] {}; 4784 case -1177318867: 4785 /* account */ return new String[] { "Reference" }; 4786 case 1057894634: 4787 /* hospitalization */ return new String[] {}; 4788 case 1901043637: 4789 /* location */ return new String[] {}; 4790 case 243182534: 4791 /* serviceProvider */ return new String[] { "Reference" }; 4792 case -995410646: 4793 /* partOf */ return new String[] { "Reference" }; 4794 default: 4795 return super.getTypesForProperty(hash, name); 4796 } 4797 4798 } 4799 4800 @Override 4801 public Base addChild(String name) throws FHIRException { 4802 if (name.equals("identifier")) { 4803 return addIdentifier(); 4804 } else if (name.equals("status")) { 4805 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 4806 } else if (name.equals("statusHistory")) { 4807 return addStatusHistory(); 4808 } else if (name.equals("class")) { 4809 this.class_ = new Coding(); 4810 return this.class_; 4811 } else if (name.equals("classHistory")) { 4812 return addClassHistory(); 4813 } else if (name.equals("type")) { 4814 return addType(); 4815 } else if (name.equals("serviceType")) { 4816 this.serviceType = new CodeableConcept(); 4817 return this.serviceType; 4818 } else if (name.equals("priority")) { 4819 this.priority = new CodeableConcept(); 4820 return this.priority; 4821 } else if (name.equals("subject")) { 4822 this.subject = new Reference(); 4823 return this.subject; 4824 } else if (name.equals("episodeOfCare")) { 4825 return addEpisodeOfCare(); 4826 } else if (name.equals("basedOn")) { 4827 return addBasedOn(); 4828 } else if (name.equals("participant")) { 4829 return addParticipant(); 4830 } else if (name.equals("appointment")) { 4831 return addAppointment(); 4832 } else if (name.equals("period")) { 4833 this.period = new Period(); 4834 return this.period; 4835 } else if (name.equals("length")) { 4836 this.length = new Duration(); 4837 return this.length; 4838 } else if (name.equals("reasonCode")) { 4839 return addReasonCode(); 4840 } else if (name.equals("reasonReference")) { 4841 return addReasonReference(); 4842 } else if (name.equals("diagnosis")) { 4843 return addDiagnosis(); 4844 } else if (name.equals("account")) { 4845 return addAccount(); 4846 } else if (name.equals("hospitalization")) { 4847 this.hospitalization = new EncounterHospitalizationComponent(); 4848 return this.hospitalization; 4849 } else if (name.equals("location")) { 4850 return addLocation(); 4851 } else if (name.equals("serviceProvider")) { 4852 this.serviceProvider = new Reference(); 4853 return this.serviceProvider; 4854 } else if (name.equals("partOf")) { 4855 this.partOf = new Reference(); 4856 return this.partOf; 4857 } else 4858 return super.addChild(name); 4859 } 4860 4861 public String fhirType() { 4862 return "Encounter"; 4863 4864 } 4865 4866 public Encounter copy() { 4867 Encounter dst = new Encounter(); 4868 copyValues(dst); 4869 return dst; 4870 } 4871 4872 public void copyValues(Encounter dst) { 4873 super.copyValues(dst); 4874 if (identifier != null) { 4875 dst.identifier = new ArrayList<Identifier>(); 4876 for (Identifier i : identifier) 4877 dst.identifier.add(i.copy()); 4878 } 4879 ; 4880 dst.status = status == null ? null : status.copy(); 4881 if (statusHistory != null) { 4882 dst.statusHistory = new ArrayList<StatusHistoryComponent>(); 4883 for (StatusHistoryComponent i : statusHistory) 4884 dst.statusHistory.add(i.copy()); 4885 } 4886 ; 4887 dst.class_ = class_ == null ? null : class_.copy(); 4888 if (classHistory != null) { 4889 dst.classHistory = new ArrayList<ClassHistoryComponent>(); 4890 for (ClassHistoryComponent i : classHistory) 4891 dst.classHistory.add(i.copy()); 4892 } 4893 ; 4894 if (type != null) { 4895 dst.type = new ArrayList<CodeableConcept>(); 4896 for (CodeableConcept i : type) 4897 dst.type.add(i.copy()); 4898 } 4899 ; 4900 dst.serviceType = serviceType == null ? null : serviceType.copy(); 4901 dst.priority = priority == null ? null : priority.copy(); 4902 dst.subject = subject == null ? null : subject.copy(); 4903 if (episodeOfCare != null) { 4904 dst.episodeOfCare = new ArrayList<Reference>(); 4905 for (Reference i : episodeOfCare) 4906 dst.episodeOfCare.add(i.copy()); 4907 } 4908 ; 4909 if (basedOn != null) { 4910 dst.basedOn = new ArrayList<Reference>(); 4911 for (Reference i : basedOn) 4912 dst.basedOn.add(i.copy()); 4913 } 4914 ; 4915 if (participant != null) { 4916 dst.participant = new ArrayList<EncounterParticipantComponent>(); 4917 for (EncounterParticipantComponent i : participant) 4918 dst.participant.add(i.copy()); 4919 } 4920 ; 4921 if (appointment != null) { 4922 dst.appointment = new ArrayList<Reference>(); 4923 for (Reference i : appointment) 4924 dst.appointment.add(i.copy()); 4925 } 4926 ; 4927 dst.period = period == null ? null : period.copy(); 4928 dst.length = length == null ? null : length.copy(); 4929 if (reasonCode != null) { 4930 dst.reasonCode = new ArrayList<CodeableConcept>(); 4931 for (CodeableConcept i : reasonCode) 4932 dst.reasonCode.add(i.copy()); 4933 } 4934 ; 4935 if (reasonReference != null) { 4936 dst.reasonReference = new ArrayList<Reference>(); 4937 for (Reference i : reasonReference) 4938 dst.reasonReference.add(i.copy()); 4939 } 4940 ; 4941 if (diagnosis != null) { 4942 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 4943 for (DiagnosisComponent i : diagnosis) 4944 dst.diagnosis.add(i.copy()); 4945 } 4946 ; 4947 if (account != null) { 4948 dst.account = new ArrayList<Reference>(); 4949 for (Reference i : account) 4950 dst.account.add(i.copy()); 4951 } 4952 ; 4953 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 4954 if (location != null) { 4955 dst.location = new ArrayList<EncounterLocationComponent>(); 4956 for (EncounterLocationComponent i : location) 4957 dst.location.add(i.copy()); 4958 } 4959 ; 4960 dst.serviceProvider = serviceProvider == null ? null : serviceProvider.copy(); 4961 dst.partOf = partOf == null ? null : partOf.copy(); 4962 } 4963 4964 protected Encounter typedCopy() { 4965 return copy(); 4966 } 4967 4968 @Override 4969 public boolean equalsDeep(Base other_) { 4970 if (!super.equalsDeep(other_)) 4971 return false; 4972 if (!(other_ instanceof Encounter)) 4973 return false; 4974 Encounter o = (Encounter) other_; 4975 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4976 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(class_, o.class_, true) 4977 && compareDeep(classHistory, o.classHistory, true) && compareDeep(type, o.type, true) 4978 && compareDeep(serviceType, o.serviceType, true) && compareDeep(priority, o.priority, true) 4979 && compareDeep(subject, o.subject, true) && compareDeep(episodeOfCare, o.episodeOfCare, true) 4980 && compareDeep(basedOn, o.basedOn, true) && compareDeep(participant, o.participant, true) 4981 && compareDeep(appointment, o.appointment, true) && compareDeep(period, o.period, true) 4982 && compareDeep(length, o.length, true) && compareDeep(reasonCode, o.reasonCode, true) 4983 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(diagnosis, o.diagnosis, true) 4984 && compareDeep(account, o.account, true) && compareDeep(hospitalization, o.hospitalization, true) 4985 && compareDeep(location, o.location, true) && compareDeep(serviceProvider, o.serviceProvider, true) 4986 && compareDeep(partOf, o.partOf, true); 4987 } 4988 4989 @Override 4990 public boolean equalsShallow(Base other_) { 4991 if (!super.equalsShallow(other_)) 4992 return false; 4993 if (!(other_ instanceof Encounter)) 4994 return false; 4995 Encounter o = (Encounter) other_; 4996 return compareValues(status, o.status, true); 4997 } 4998 4999 public boolean isEmpty() { 5000 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory, class_, 5001 classHistory, type, serviceType, priority, subject, episodeOfCare, basedOn, participant, appointment, period, 5002 length, reasonCode, reasonReference, diagnosis, account, hospitalization, location, serviceProvider, partOf); 5003 } 5004 5005 @Override 5006 public ResourceType getResourceType() { 5007 return ResourceType.Encounter; 5008 } 5009 5010 /** 5011 * Search parameter: <b>date</b> 5012 * <p> 5013 * Description: <b>A date within the period the Encounter lasted</b><br> 5014 * Type: <b>date</b><br> 5015 * Path: <b>Encounter.period</b><br> 5016 * </p> 5017 */ 5018 @SearchParamDefinition(name = "date", path = "Encounter.period", description = "A date within the period the Encounter lasted", type = "date") 5019 public static final String SP_DATE = "date"; 5020 /** 5021 * <b>Fluent Client</b> search parameter constant for <b>date</b> 5022 * <p> 5023 * Description: <b>A date within the period the Encounter lasted</b><br> 5024 * Type: <b>date</b><br> 5025 * Path: <b>Encounter.period</b><br> 5026 * </p> 5027 */ 5028 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 5029 SP_DATE); 5030 5031 /** 5032 * Search parameter: <b>identifier</b> 5033 * <p> 5034 * Description: <b>Identifier(s) by which this encounter is known</b><br> 5035 * Type: <b>token</b><br> 5036 * Path: <b>Encounter.identifier</b><br> 5037 * </p> 5038 */ 5039 @SearchParamDefinition(name = "identifier", path = "Encounter.identifier", description = "Identifier(s) by which this encounter is known", type = "token") 5040 public static final String SP_IDENTIFIER = "identifier"; 5041 /** 5042 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 5043 * <p> 5044 * Description: <b>Identifier(s) by which this encounter is known</b><br> 5045 * Type: <b>token</b><br> 5046 * Path: <b>Encounter.identifier</b><br> 5047 * </p> 5048 */ 5049 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5050 SP_IDENTIFIER); 5051 5052 /** 5053 * Search parameter: <b>participant-type</b> 5054 * <p> 5055 * Description: <b>Role of participant in encounter</b><br> 5056 * Type: <b>token</b><br> 5057 * Path: <b>Encounter.participant.type</b><br> 5058 * </p> 5059 */ 5060 @SearchParamDefinition(name = "participant-type", path = "Encounter.participant.type", description = "Role of participant in encounter", type = "token") 5061 public static final String SP_PARTICIPANT_TYPE = "participant-type"; 5062 /** 5063 * <b>Fluent Client</b> search parameter constant for <b>participant-type</b> 5064 * <p> 5065 * Description: <b>Role of participant in encounter</b><br> 5066 * Type: <b>token</b><br> 5067 * Path: <b>Encounter.participant.type</b><br> 5068 * </p> 5069 */ 5070 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5071 SP_PARTICIPANT_TYPE); 5072 5073 /** 5074 * Search parameter: <b>practitioner</b> 5075 * <p> 5076 * Description: <b>Persons involved in the encounter other than the 5077 * patient</b><br> 5078 * Type: <b>reference</b><br> 5079 * Path: <b>Encounter.participant.individual</b><br> 5080 * </p> 5081 */ 5082 @SearchParamDefinition(name = "practitioner", path = "Encounter.participant.individual.where(resolve() is Practitioner)", description = "Persons involved in the encounter other than the patient", type = "reference", providesMembershipIn = { 5083 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 5084 public static final String SP_PRACTITIONER = "practitioner"; 5085 /** 5086 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 5087 * <p> 5088 * Description: <b>Persons involved in the encounter other than the 5089 * patient</b><br> 5090 * Type: <b>reference</b><br> 5091 * Path: <b>Encounter.participant.individual</b><br> 5092 * </p> 5093 */ 5094 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5095 SP_PRACTITIONER); 5096 5097 /** 5098 * Constant for fluent queries to be used to add include statements. Specifies 5099 * the path value of "<b>Encounter:practitioner</b>". 5100 */ 5101 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include( 5102 "Encounter:practitioner").toLocked(); 5103 5104 /** 5105 * Search parameter: <b>subject</b> 5106 * <p> 5107 * Description: <b>The patient or group present at the encounter</b><br> 5108 * Type: <b>reference</b><br> 5109 * Path: <b>Encounter.subject</b><br> 5110 * </p> 5111 */ 5112 @SearchParamDefinition(name = "subject", path = "Encounter.subject", description = "The patient or group present at the encounter", type = "reference", target = { 5113 Group.class, Patient.class }) 5114 public static final String SP_SUBJECT = "subject"; 5115 /** 5116 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5117 * <p> 5118 * Description: <b>The patient or group present at the encounter</b><br> 5119 * Type: <b>reference</b><br> 5120 * Path: <b>Encounter.subject</b><br> 5121 * </p> 5122 */ 5123 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5124 SP_SUBJECT); 5125 5126 /** 5127 * Constant for fluent queries to be used to add include statements. Specifies 5128 * the path value of "<b>Encounter:subject</b>". 5129 */ 5130 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 5131 "Encounter:subject").toLocked(); 5132 5133 /** 5134 * Search parameter: <b>length</b> 5135 * <p> 5136 * Description: <b>Length of encounter in days</b><br> 5137 * Type: <b>quantity</b><br> 5138 * Path: <b>Encounter.length</b><br> 5139 * </p> 5140 */ 5141 @SearchParamDefinition(name = "length", path = "Encounter.length", description = "Length of encounter in days", type = "quantity") 5142 public static final String SP_LENGTH = "length"; 5143 /** 5144 * <b>Fluent Client</b> search parameter constant for <b>length</b> 5145 * <p> 5146 * Description: <b>Length of encounter in days</b><br> 5147 * Type: <b>quantity</b><br> 5148 * Path: <b>Encounter.length</b><br> 5149 * </p> 5150 */ 5151 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam LENGTH = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5152 SP_LENGTH); 5153 5154 /** 5155 * Search parameter: <b>episode-of-care</b> 5156 * <p> 5157 * Description: <b>Episode(s) of care that this encounter should be recorded 5158 * against</b><br> 5159 * Type: <b>reference</b><br> 5160 * Path: <b>Encounter.episodeOfCare</b><br> 5161 * </p> 5162 */ 5163 @SearchParamDefinition(name = "episode-of-care", path = "Encounter.episodeOfCare", description = "Episode(s) of care that this encounter should be recorded against", type = "reference", target = { 5164 EpisodeOfCare.class }) 5165 public static final String SP_EPISODE_OF_CARE = "episode-of-care"; 5166 /** 5167 * <b>Fluent Client</b> search parameter constant for <b>episode-of-care</b> 5168 * <p> 5169 * Description: <b>Episode(s) of care that this encounter should be recorded 5170 * against</b><br> 5171 * Type: <b>reference</b><br> 5172 * Path: <b>Encounter.episodeOfCare</b><br> 5173 * </p> 5174 */ 5175 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EPISODE_OF_CARE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5176 SP_EPISODE_OF_CARE); 5177 5178 /** 5179 * Constant for fluent queries to be used to add include statements. Specifies 5180 * the path value of "<b>Encounter:episode-of-care</b>". 5181 */ 5182 public static final ca.uhn.fhir.model.api.Include INCLUDE_EPISODE_OF_CARE = new ca.uhn.fhir.model.api.Include( 5183 "Encounter:episode-of-care").toLocked(); 5184 5185 /** 5186 * Search parameter: <b>diagnosis</b> 5187 * <p> 5188 * Description: <b>The diagnosis or procedure relevant to the encounter</b><br> 5189 * Type: <b>reference</b><br> 5190 * Path: <b>Encounter.diagnosis.condition</b><br> 5191 * </p> 5192 */ 5193 @SearchParamDefinition(name = "diagnosis", path = "Encounter.diagnosis.condition", description = "The diagnosis or procedure relevant to the encounter", type = "reference", target = { 5194 Condition.class, Procedure.class }) 5195 public static final String SP_DIAGNOSIS = "diagnosis"; 5196 /** 5197 * <b>Fluent Client</b> search parameter constant for <b>diagnosis</b> 5198 * <p> 5199 * Description: <b>The diagnosis or procedure relevant to the encounter</b><br> 5200 * Type: <b>reference</b><br> 5201 * Path: <b>Encounter.diagnosis.condition</b><br> 5202 * </p> 5203 */ 5204 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DIAGNOSIS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5205 SP_DIAGNOSIS); 5206 5207 /** 5208 * Constant for fluent queries to be used to add include statements. Specifies 5209 * the path value of "<b>Encounter:diagnosis</b>". 5210 */ 5211 public static final ca.uhn.fhir.model.api.Include INCLUDE_DIAGNOSIS = new ca.uhn.fhir.model.api.Include( 5212 "Encounter:diagnosis").toLocked(); 5213 5214 /** 5215 * Search parameter: <b>appointment</b> 5216 * <p> 5217 * Description: <b>The appointment that scheduled this encounter</b><br> 5218 * Type: <b>reference</b><br> 5219 * Path: <b>Encounter.appointment</b><br> 5220 * </p> 5221 */ 5222 @SearchParamDefinition(name = "appointment", path = "Encounter.appointment", description = "The appointment that scheduled this encounter", type = "reference", target = { 5223 Appointment.class }) 5224 public static final String SP_APPOINTMENT = "appointment"; 5225 /** 5226 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 5227 * <p> 5228 * Description: <b>The appointment that scheduled this encounter</b><br> 5229 * Type: <b>reference</b><br> 5230 * Path: <b>Encounter.appointment</b><br> 5231 * </p> 5232 */ 5233 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5234 SP_APPOINTMENT); 5235 5236 /** 5237 * Constant for fluent queries to be used to add include statements. Specifies 5238 * the path value of "<b>Encounter:appointment</b>". 5239 */ 5240 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include( 5241 "Encounter:appointment").toLocked(); 5242 5243 /** 5244 * Search parameter: <b>part-of</b> 5245 * <p> 5246 * Description: <b>Another Encounter this encounter is part of</b><br> 5247 * Type: <b>reference</b><br> 5248 * Path: <b>Encounter.partOf</b><br> 5249 * </p> 5250 */ 5251 @SearchParamDefinition(name = "part-of", path = "Encounter.partOf", description = "Another Encounter this encounter is part of", type = "reference", target = { 5252 Encounter.class }) 5253 public static final String SP_PART_OF = "part-of"; 5254 /** 5255 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 5256 * <p> 5257 * Description: <b>Another Encounter this encounter is part of</b><br> 5258 * Type: <b>reference</b><br> 5259 * Path: <b>Encounter.partOf</b><br> 5260 * </p> 5261 */ 5262 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5263 SP_PART_OF); 5264 5265 /** 5266 * Constant for fluent queries to be used to add include statements. Specifies 5267 * the path value of "<b>Encounter:part-of</b>". 5268 */ 5269 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 5270 "Encounter:part-of").toLocked(); 5271 5272 /** 5273 * Search parameter: <b>type</b> 5274 * <p> 5275 * Description: <b>Specific type of encounter</b><br> 5276 * Type: <b>token</b><br> 5277 * Path: <b>Encounter.type</b><br> 5278 * </p> 5279 */ 5280 @SearchParamDefinition(name = "type", path = "Encounter.type", description = "Specific type of encounter", type = "token") 5281 public static final String SP_TYPE = "type"; 5282 /** 5283 * <b>Fluent Client</b> search parameter constant for <b>type</b> 5284 * <p> 5285 * Description: <b>Specific type of encounter</b><br> 5286 * Type: <b>token</b><br> 5287 * Path: <b>Encounter.type</b><br> 5288 * </p> 5289 */ 5290 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5291 SP_TYPE); 5292 5293 /** 5294 * Search parameter: <b>reason-code</b> 5295 * <p> 5296 * Description: <b>Coded reason the encounter takes place</b><br> 5297 * Type: <b>token</b><br> 5298 * Path: <b>Encounter.reasonCode</b><br> 5299 * </p> 5300 */ 5301 @SearchParamDefinition(name = "reason-code", path = "Encounter.reasonCode", description = "Coded reason the encounter takes place", type = "token") 5302 public static final String SP_REASON_CODE = "reason-code"; 5303 /** 5304 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 5305 * <p> 5306 * Description: <b>Coded reason the encounter takes place</b><br> 5307 * Type: <b>token</b><br> 5308 * Path: <b>Encounter.reasonCode</b><br> 5309 * </p> 5310 */ 5311 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5312 SP_REASON_CODE); 5313 5314 /** 5315 * Search parameter: <b>participant</b> 5316 * <p> 5317 * Description: <b>Persons involved in the encounter other than the 5318 * patient</b><br> 5319 * Type: <b>reference</b><br> 5320 * Path: <b>Encounter.participant.individual</b><br> 5321 * </p> 5322 */ 5323 @SearchParamDefinition(name = "participant", path = "Encounter.participant.individual", description = "Persons involved in the encounter other than the patient", type = "reference", providesMembershipIn = { 5324 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 5325 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Practitioner.class, 5326 PractitionerRole.class, RelatedPerson.class }) 5327 public static final String SP_PARTICIPANT = "participant"; 5328 /** 5329 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 5330 * <p> 5331 * Description: <b>Persons involved in the encounter other than the 5332 * patient</b><br> 5333 * Type: <b>reference</b><br> 5334 * Path: <b>Encounter.participant.individual</b><br> 5335 * </p> 5336 */ 5337 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5338 SP_PARTICIPANT); 5339 5340 /** 5341 * Constant for fluent queries to be used to add include statements. Specifies 5342 * the path value of "<b>Encounter:participant</b>". 5343 */ 5344 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 5345 "Encounter:participant").toLocked(); 5346 5347 /** 5348 * Search parameter: <b>based-on</b> 5349 * <p> 5350 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 5351 * Type: <b>reference</b><br> 5352 * Path: <b>Encounter.basedOn</b><br> 5353 * </p> 5354 */ 5355 @SearchParamDefinition(name = "based-on", path = "Encounter.basedOn", description = "The ServiceRequest that initiated this encounter", type = "reference", target = { 5356 ServiceRequest.class }) 5357 public static final String SP_BASED_ON = "based-on"; 5358 /** 5359 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 5360 * <p> 5361 * Description: <b>The ServiceRequest that initiated this encounter</b><br> 5362 * Type: <b>reference</b><br> 5363 * Path: <b>Encounter.basedOn</b><br> 5364 * </p> 5365 */ 5366 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5367 SP_BASED_ON); 5368 5369 /** 5370 * Constant for fluent queries to be used to add include statements. Specifies 5371 * the path value of "<b>Encounter:based-on</b>". 5372 */ 5373 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 5374 "Encounter:based-on").toLocked(); 5375 5376 /** 5377 * Search parameter: <b>patient</b> 5378 * <p> 5379 * Description: <b>The patient or group present at the encounter</b><br> 5380 * Type: <b>reference</b><br> 5381 * Path: <b>Encounter.subject</b><br> 5382 * </p> 5383 */ 5384 @SearchParamDefinition(name = "patient", path = "Encounter.subject.where(resolve() is Patient)", description = "The patient or group present at the encounter", type = "reference", providesMembershipIn = { 5385 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 5386 public static final String SP_PATIENT = "patient"; 5387 /** 5388 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5389 * <p> 5390 * Description: <b>The patient or group present at the encounter</b><br> 5391 * Type: <b>reference</b><br> 5392 * Path: <b>Encounter.subject</b><br> 5393 * </p> 5394 */ 5395 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5396 SP_PATIENT); 5397 5398 /** 5399 * Constant for fluent queries to be used to add include statements. Specifies 5400 * the path value of "<b>Encounter:patient</b>". 5401 */ 5402 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 5403 "Encounter:patient").toLocked(); 5404 5405 /** 5406 * Search parameter: <b>reason-reference</b> 5407 * <p> 5408 * Description: <b>Reason the encounter takes place (reference)</b><br> 5409 * Type: <b>reference</b><br> 5410 * Path: <b>Encounter.reasonReference</b><br> 5411 * </p> 5412 */ 5413 @SearchParamDefinition(name = "reason-reference", path = "Encounter.reasonReference", description = "Reason the encounter takes place (reference)", type = "reference", target = { 5414 Condition.class, ImmunizationRecommendation.class, Observation.class, Procedure.class }) 5415 public static final String SP_REASON_REFERENCE = "reason-reference"; 5416 /** 5417 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 5418 * <p> 5419 * Description: <b>Reason the encounter takes place (reference)</b><br> 5420 * Type: <b>reference</b><br> 5421 * Path: <b>Encounter.reasonReference</b><br> 5422 * </p> 5423 */ 5424 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5425 SP_REASON_REFERENCE); 5426 5427 /** 5428 * Constant for fluent queries to be used to add include statements. Specifies 5429 * the path value of "<b>Encounter:reason-reference</b>". 5430 */ 5431 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 5432 "Encounter:reason-reference").toLocked(); 5433 5434 /** 5435 * Search parameter: <b>location-period</b> 5436 * <p> 5437 * Description: <b>Time period during which the patient was present at the 5438 * location</b><br> 5439 * Type: <b>date</b><br> 5440 * Path: <b>Encounter.location.period</b><br> 5441 * </p> 5442 */ 5443 @SearchParamDefinition(name = "location-period", path = "Encounter.location.period", description = "Time period during which the patient was present at the location", type = "date") 5444 public static final String SP_LOCATION_PERIOD = "location-period"; 5445 /** 5446 * <b>Fluent Client</b> search parameter constant for <b>location-period</b> 5447 * <p> 5448 * Description: <b>Time period during which the patient was present at the 5449 * location</b><br> 5450 * Type: <b>date</b><br> 5451 * Path: <b>Encounter.location.period</b><br> 5452 * </p> 5453 */ 5454 public static final ca.uhn.fhir.rest.gclient.DateClientParam LOCATION_PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 5455 SP_LOCATION_PERIOD); 5456 5457 /** 5458 * Search parameter: <b>location</b> 5459 * <p> 5460 * Description: <b>Location the encounter takes place</b><br> 5461 * Type: <b>reference</b><br> 5462 * Path: <b>Encounter.location.location</b><br> 5463 * </p> 5464 */ 5465 @SearchParamDefinition(name = "location", path = "Encounter.location.location", description = "Location the encounter takes place", type = "reference", target = { 5466 Location.class }) 5467 public static final String SP_LOCATION = "location"; 5468 /** 5469 * <b>Fluent Client</b> search parameter constant for <b>location</b> 5470 * <p> 5471 * Description: <b>Location the encounter takes place</b><br> 5472 * Type: <b>reference</b><br> 5473 * Path: <b>Encounter.location.location</b><br> 5474 * </p> 5475 */ 5476 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5477 SP_LOCATION); 5478 5479 /** 5480 * Constant for fluent queries to be used to add include statements. Specifies 5481 * the path value of "<b>Encounter:location</b>". 5482 */ 5483 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 5484 "Encounter:location").toLocked(); 5485 5486 /** 5487 * Search parameter: <b>service-provider</b> 5488 * <p> 5489 * Description: <b>The organization (facility) responsible for this 5490 * encounter</b><br> 5491 * Type: <b>reference</b><br> 5492 * Path: <b>Encounter.serviceProvider</b><br> 5493 * </p> 5494 */ 5495 @SearchParamDefinition(name = "service-provider", path = "Encounter.serviceProvider", description = "The organization (facility) responsible for this encounter", type = "reference", target = { 5496 Organization.class }) 5497 public static final String SP_SERVICE_PROVIDER = "service-provider"; 5498 /** 5499 * <b>Fluent Client</b> search parameter constant for <b>service-provider</b> 5500 * <p> 5501 * Description: <b>The organization (facility) responsible for this 5502 * encounter</b><br> 5503 * Type: <b>reference</b><br> 5504 * Path: <b>Encounter.serviceProvider</b><br> 5505 * </p> 5506 */ 5507 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5508 SP_SERVICE_PROVIDER); 5509 5510 /** 5511 * Constant for fluent queries to be used to add include statements. Specifies 5512 * the path value of "<b>Encounter:service-provider</b>". 5513 */ 5514 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_PROVIDER = new ca.uhn.fhir.model.api.Include( 5515 "Encounter:service-provider").toLocked(); 5516 5517 /** 5518 * Search parameter: <b>special-arrangement</b> 5519 * <p> 5520 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 5521 * Type: <b>token</b><br> 5522 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 5523 * </p> 5524 */ 5525 @SearchParamDefinition(name = "special-arrangement", path = "Encounter.hospitalization.specialArrangement", description = "Wheelchair, translator, stretcher, etc.", type = "token") 5526 public static final String SP_SPECIAL_ARRANGEMENT = "special-arrangement"; 5527 /** 5528 * <b>Fluent Client</b> search parameter constant for <b>special-arrangement</b> 5529 * <p> 5530 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 5531 * Type: <b>token</b><br> 5532 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 5533 * </p> 5534 */ 5535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIAL_ARRANGEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5536 SP_SPECIAL_ARRANGEMENT); 5537 5538 /** 5539 * Search parameter: <b>class</b> 5540 * <p> 5541 * Description: <b>Classification of patient encounter</b><br> 5542 * Type: <b>token</b><br> 5543 * Path: <b>Encounter.class</b><br> 5544 * </p> 5545 */ 5546 @SearchParamDefinition(name = "class", path = "Encounter.class", description = "Classification of patient encounter", type = "token") 5547 public static final String SP_CLASS = "class"; 5548 /** 5549 * <b>Fluent Client</b> search parameter constant for <b>class</b> 5550 * <p> 5551 * Description: <b>Classification of patient encounter</b><br> 5552 * Type: <b>token</b><br> 5553 * Path: <b>Encounter.class</b><br> 5554 * </p> 5555 */ 5556 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5557 SP_CLASS); 5558 5559 /** 5560 * Search parameter: <b>account</b> 5561 * <p> 5562 * Description: <b>The set of accounts that may be used for billing for this 5563 * Encounter</b><br> 5564 * Type: <b>reference</b><br> 5565 * Path: <b>Encounter.account</b><br> 5566 * </p> 5567 */ 5568 @SearchParamDefinition(name = "account", path = "Encounter.account", description = "The set of accounts that may be used for billing for this Encounter", type = "reference", target = { 5569 Account.class }) 5570 public static final String SP_ACCOUNT = "account"; 5571 /** 5572 * <b>Fluent Client</b> search parameter constant for <b>account</b> 5573 * <p> 5574 * Description: <b>The set of accounts that may be used for billing for this 5575 * Encounter</b><br> 5576 * Type: <b>reference</b><br> 5577 * Path: <b>Encounter.account</b><br> 5578 * </p> 5579 */ 5580 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5581 SP_ACCOUNT); 5582 5583 /** 5584 * Constant for fluent queries to be used to add include statements. Specifies 5585 * the path value of "<b>Encounter:account</b>". 5586 */ 5587 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include( 5588 "Encounter:account").toLocked(); 5589 5590 /** 5591 * Search parameter: <b>status</b> 5592 * <p> 5593 * Description: <b>planned | arrived | triaged | in-progress | onleave | 5594 * finished | cancelled +</b><br> 5595 * Type: <b>token</b><br> 5596 * Path: <b>Encounter.status</b><br> 5597 * </p> 5598 */ 5599 @SearchParamDefinition(name = "status", path = "Encounter.status", description = "planned | arrived | triaged | in-progress | onleave | finished | cancelled +", type = "token") 5600 public static final String SP_STATUS = "status"; 5601 /** 5602 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5603 * <p> 5604 * Description: <b>planned | arrived | triaged | in-progress | onleave | 5605 * finished | cancelled +</b><br> 5606 * Type: <b>token</b><br> 5607 * Path: <b>Encounter.status</b><br> 5608 * </p> 5609 */ 5610 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5611 SP_STATUS); 5612 5613}