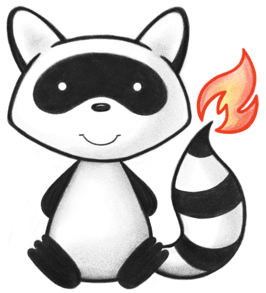
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * This resource provides the insurance enrollment details to the insurer 046 * regarding a specified coverage. 047 */ 048@ResourceDef(name = "EnrollmentRequest", profile = "http://hl7.org/fhir/StructureDefinition/EnrollmentRequest") 049public class EnrollmentRequest extends DomainResource { 050 051 public enum EnrollmentRequestStatus { 052 /** 053 * The instance is currently in-force. 054 */ 055 ACTIVE, 056 /** 057 * The instance is withdrawn, rescinded or reversed. 058 */ 059 CANCELLED, 060 /** 061 * A new instance the contents of which is not complete. 062 */ 063 DRAFT, 064 /** 065 * The instance was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 073 public static EnrollmentRequestStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("cancelled".equals(codeString)) 079 return CANCELLED; 080 if ("draft".equals(codeString)) 081 return DRAFT; 082 if ("entered-in-error".equals(codeString)) 083 return ENTEREDINERROR; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown EnrollmentRequestStatus code '" + codeString + "'"); 088 } 089 090 public String toCode() { 091 switch (this) { 092 case ACTIVE: 093 return "active"; 094 case CANCELLED: 095 return "cancelled"; 096 case DRAFT: 097 return "draft"; 098 case ENTEREDINERROR: 099 return "entered-in-error"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 switch (this) { 109 case ACTIVE: 110 return "http://hl7.org/fhir/fm-status"; 111 case CANCELLED: 112 return "http://hl7.org/fhir/fm-status"; 113 case DRAFT: 114 return "http://hl7.org/fhir/fm-status"; 115 case ENTEREDINERROR: 116 return "http://hl7.org/fhir/fm-status"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case ACTIVE: 127 return "The instance is currently in-force."; 128 case CANCELLED: 129 return "The instance is withdrawn, rescinded or reversed."; 130 case DRAFT: 131 return "A new instance the contents of which is not complete."; 132 case ENTEREDINERROR: 133 return "The instance was entered in error."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case ACTIVE: 144 return "Active"; 145 case CANCELLED: 146 return "Cancelled"; 147 case DRAFT: 148 return "Draft"; 149 case ENTEREDINERROR: 150 return "Entered in Error"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class EnrollmentRequestStatusEnumFactory implements EnumFactory<EnrollmentRequestStatus> { 160 public EnrollmentRequestStatus fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("active".equals(codeString)) 165 return EnrollmentRequestStatus.ACTIVE; 166 if ("cancelled".equals(codeString)) 167 return EnrollmentRequestStatus.CANCELLED; 168 if ("draft".equals(codeString)) 169 return EnrollmentRequestStatus.DRAFT; 170 if ("entered-in-error".equals(codeString)) 171 return EnrollmentRequestStatus.ENTEREDINERROR; 172 throw new IllegalArgumentException("Unknown EnrollmentRequestStatus code '" + codeString + "'"); 173 } 174 175 public Enumeration<EnrollmentRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 176 if (code == null) 177 return null; 178 if (code.isEmpty()) 179 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.NULL, code); 180 String codeString = code.asStringValue(); 181 if (codeString == null || "".equals(codeString)) 182 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.NULL, code); 183 if ("active".equals(codeString)) 184 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.ACTIVE, code); 185 if ("cancelled".equals(codeString)) 186 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.CANCELLED, code); 187 if ("draft".equals(codeString)) 188 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.DRAFT, code); 189 if ("entered-in-error".equals(codeString)) 190 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.ENTEREDINERROR, code); 191 throw new FHIRException("Unknown EnrollmentRequestStatus code '" + codeString + "'"); 192 } 193 194 public String toCode(EnrollmentRequestStatus code) { 195 if (code == EnrollmentRequestStatus.NULL) 196 return null; 197 if (code == EnrollmentRequestStatus.ACTIVE) 198 return "active"; 199 if (code == EnrollmentRequestStatus.CANCELLED) 200 return "cancelled"; 201 if (code == EnrollmentRequestStatus.DRAFT) 202 return "draft"; 203 if (code == EnrollmentRequestStatus.ENTEREDINERROR) 204 return "entered-in-error"; 205 return "?"; 206 } 207 208 public String toSystem(EnrollmentRequestStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 /** 214 * The Response business identifier. 215 */ 216 @Child(name = "identifier", type = { 217 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 218 @Description(shortDefinition = "Business Identifier", formalDefinition = "The Response business identifier.") 219 protected List<Identifier> identifier; 220 221 /** 222 * The status of the resource instance. 223 */ 224 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 225 @Description(shortDefinition = "active | cancelled | draft | entered-in-error", formalDefinition = "The status of the resource instance.") 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/fm-status") 227 protected Enumeration<EnrollmentRequestStatus> status; 228 229 /** 230 * The date when this resource was created. 231 */ 232 @Child(name = "created", type = { 233 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 234 @Description(shortDefinition = "Creation date", formalDefinition = "The date when this resource was created.") 235 protected DateTimeType created; 236 237 /** 238 * The Insurer who is target of the request. 239 */ 240 @Child(name = "insurer", type = { 241 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 242 @Description(shortDefinition = "Target", formalDefinition = "The Insurer who is target of the request.") 243 protected Reference insurer; 244 245 /** 246 * The actual object that is the target of the reference (The Insurer who is 247 * target of the request.) 248 */ 249 protected Organization insurerTarget; 250 251 /** 252 * The practitioner who is responsible for the services rendered to the patient. 253 */ 254 @Child(name = "provider", type = { Practitioner.class, PractitionerRole.class, 255 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 256 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 257 protected Reference provider; 258 259 /** 260 * The actual object that is the target of the reference (The practitioner who 261 * is responsible for the services rendered to the patient.) 262 */ 263 protected Resource providerTarget; 264 265 /** 266 * Patient Resource. 267 */ 268 @Child(name = "candidate", type = { Patient.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 269 @Description(shortDefinition = "The subject to be enrolled", formalDefinition = "Patient Resource.") 270 protected Reference candidate; 271 272 /** 273 * The actual object that is the target of the reference (Patient Resource.) 274 */ 275 protected Patient candidateTarget; 276 277 /** 278 * Reference to the program or plan identification, underwriter or payor. 279 */ 280 @Child(name = "coverage", type = { Coverage.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the program or plan identification, underwriter or payor.") 282 protected Reference coverage; 283 284 /** 285 * The actual object that is the target of the reference (Reference to the 286 * program or plan identification, underwriter or payor.) 287 */ 288 protected Coverage coverageTarget; 289 290 private static final long serialVersionUID = 631501951L; 291 292 /** 293 * Constructor 294 */ 295 public EnrollmentRequest() { 296 super(); 297 } 298 299 /** 300 * @return {@link #identifier} (The Response business identifier.) 301 */ 302 public List<Identifier> getIdentifier() { 303 if (this.identifier == null) 304 this.identifier = new ArrayList<Identifier>(); 305 return this.identifier; 306 } 307 308 /** 309 * @return Returns a reference to <code>this</code> for easy method chaining 310 */ 311 public EnrollmentRequest setIdentifier(List<Identifier> theIdentifier) { 312 this.identifier = theIdentifier; 313 return this; 314 } 315 316 public boolean hasIdentifier() { 317 if (this.identifier == null) 318 return false; 319 for (Identifier item : this.identifier) 320 if (!item.isEmpty()) 321 return true; 322 return false; 323 } 324 325 public Identifier addIdentifier() { // 3 326 Identifier t = new Identifier(); 327 if (this.identifier == null) 328 this.identifier = new ArrayList<Identifier>(); 329 this.identifier.add(t); 330 return t; 331 } 332 333 public EnrollmentRequest addIdentifier(Identifier t) { // 3 334 if (t == null) 335 return this; 336 if (this.identifier == null) 337 this.identifier = new ArrayList<Identifier>(); 338 this.identifier.add(t); 339 return this; 340 } 341 342 /** 343 * @return The first repetition of repeating field {@link #identifier}, creating 344 * it if it does not already exist 345 */ 346 public Identifier getIdentifierFirstRep() { 347 if (getIdentifier().isEmpty()) { 348 addIdentifier(); 349 } 350 return getIdentifier().get(0); 351 } 352 353 /** 354 * @return {@link #status} (The status of the resource instance.). This is the 355 * underlying object with id, value and extensions. The accessor 356 * "getStatus" gives direct access to the value 357 */ 358 public Enumeration<EnrollmentRequestStatus> getStatusElement() { 359 if (this.status == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create EnrollmentRequest.status"); 362 else if (Configuration.doAutoCreate()) 363 this.status = new Enumeration<EnrollmentRequestStatus>(new EnrollmentRequestStatusEnumFactory()); // bb 364 return this.status; 365 } 366 367 public boolean hasStatusElement() { 368 return this.status != null && !this.status.isEmpty(); 369 } 370 371 public boolean hasStatus() { 372 return this.status != null && !this.status.isEmpty(); 373 } 374 375 /** 376 * @param value {@link #status} (The status of the resource instance.). This is 377 * the underlying object with id, value and extensions. The 378 * accessor "getStatus" gives direct access to the value 379 */ 380 public EnrollmentRequest setStatusElement(Enumeration<EnrollmentRequestStatus> value) { 381 this.status = value; 382 return this; 383 } 384 385 /** 386 * @return The status of the resource instance. 387 */ 388 public EnrollmentRequestStatus getStatus() { 389 return this.status == null ? null : this.status.getValue(); 390 } 391 392 /** 393 * @param value The status of the resource instance. 394 */ 395 public EnrollmentRequest setStatus(EnrollmentRequestStatus value) { 396 if (value == null) 397 this.status = null; 398 else { 399 if (this.status == null) 400 this.status = new Enumeration<EnrollmentRequestStatus>(new EnrollmentRequestStatusEnumFactory()); 401 this.status.setValue(value); 402 } 403 return this; 404 } 405 406 /** 407 * @return {@link #created} (The date when this resource was created.). This is 408 * the underlying object with id, value and extensions. The accessor 409 * "getCreated" gives direct access to the value 410 */ 411 public DateTimeType getCreatedElement() { 412 if (this.created == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create EnrollmentRequest.created"); 415 else if (Configuration.doAutoCreate()) 416 this.created = new DateTimeType(); // bb 417 return this.created; 418 } 419 420 public boolean hasCreatedElement() { 421 return this.created != null && !this.created.isEmpty(); 422 } 423 424 public boolean hasCreated() { 425 return this.created != null && !this.created.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #created} (The date when this resource was created.). 430 * This is the underlying object with id, value and extensions. The 431 * accessor "getCreated" gives direct access to the value 432 */ 433 public EnrollmentRequest setCreatedElement(DateTimeType value) { 434 this.created = value; 435 return this; 436 } 437 438 /** 439 * @return The date when this resource was created. 440 */ 441 public Date getCreated() { 442 return this.created == null ? null : this.created.getValue(); 443 } 444 445 /** 446 * @param value The date when this resource was created. 447 */ 448 public EnrollmentRequest setCreated(Date value) { 449 if (value == null) 450 this.created = null; 451 else { 452 if (this.created == null) 453 this.created = new DateTimeType(); 454 this.created.setValue(value); 455 } 456 return this; 457 } 458 459 /** 460 * @return {@link #insurer} (The Insurer who is target of the request.) 461 */ 462 public Reference getInsurer() { 463 if (this.insurer == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create EnrollmentRequest.insurer"); 466 else if (Configuration.doAutoCreate()) 467 this.insurer = new Reference(); // cc 468 return this.insurer; 469 } 470 471 public boolean hasInsurer() { 472 return this.insurer != null && !this.insurer.isEmpty(); 473 } 474 475 /** 476 * @param value {@link #insurer} (The Insurer who is target of the request.) 477 */ 478 public EnrollmentRequest setInsurer(Reference value) { 479 this.insurer = value; 480 return this; 481 } 482 483 /** 484 * @return {@link #insurer} The actual object that is the target of the 485 * reference. The reference library doesn't populate this, but you can 486 * use it to hold the resource if you resolve it. (The Insurer who is 487 * target of the request.) 488 */ 489 public Organization getInsurerTarget() { 490 if (this.insurerTarget == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create EnrollmentRequest.insurer"); 493 else if (Configuration.doAutoCreate()) 494 this.insurerTarget = new Organization(); // aa 495 return this.insurerTarget; 496 } 497 498 /** 499 * @param value {@link #insurer} The actual object that is the target of the 500 * reference. The reference library doesn't use these, but you can 501 * use it to hold the resource if you resolve it. (The Insurer who 502 * is target of the request.) 503 */ 504 public EnrollmentRequest setInsurerTarget(Organization value) { 505 this.insurerTarget = value; 506 return this; 507 } 508 509 /** 510 * @return {@link #provider} (The practitioner who is responsible for the 511 * services rendered to the patient.) 512 */ 513 public Reference getProvider() { 514 if (this.provider == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 517 else if (Configuration.doAutoCreate()) 518 this.provider = new Reference(); // cc 519 return this.provider; 520 } 521 522 public boolean hasProvider() { 523 return this.provider != null && !this.provider.isEmpty(); 524 } 525 526 /** 527 * @param value {@link #provider} (The practitioner who is responsible for the 528 * services rendered to the patient.) 529 */ 530 public EnrollmentRequest setProvider(Reference value) { 531 this.provider = value; 532 return this; 533 } 534 535 /** 536 * @return {@link #provider} The actual object that is the target of the 537 * reference. The reference library doesn't populate this, but you can 538 * use it to hold the resource if you resolve it. (The practitioner who 539 * is responsible for the services rendered to the patient.) 540 */ 541 public Resource getProviderTarget() { 542 return this.providerTarget; 543 } 544 545 /** 546 * @param value {@link #provider} The actual object that is the target of the 547 * reference. The reference library doesn't use these, but you can 548 * use it to hold the resource if you resolve it. (The practitioner 549 * who is responsible for the services rendered to the patient.) 550 */ 551 public EnrollmentRequest setProviderTarget(Resource value) { 552 this.providerTarget = value; 553 return this; 554 } 555 556 /** 557 * @return {@link #candidate} (Patient Resource.) 558 */ 559 public Reference getCandidate() { 560 if (this.candidate == null) 561 if (Configuration.errorOnAutoCreate()) 562 throw new Error("Attempt to auto-create EnrollmentRequest.candidate"); 563 else if (Configuration.doAutoCreate()) 564 this.candidate = new Reference(); // cc 565 return this.candidate; 566 } 567 568 public boolean hasCandidate() { 569 return this.candidate != null && !this.candidate.isEmpty(); 570 } 571 572 /** 573 * @param value {@link #candidate} (Patient Resource.) 574 */ 575 public EnrollmentRequest setCandidate(Reference value) { 576 this.candidate = value; 577 return this; 578 } 579 580 /** 581 * @return {@link #candidate} The actual object that is the target of the 582 * reference. The reference library doesn't populate this, but you can 583 * use it to hold the resource if you resolve it. (Patient Resource.) 584 */ 585 public Patient getCandidateTarget() { 586 if (this.candidateTarget == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create EnrollmentRequest.candidate"); 589 else if (Configuration.doAutoCreate()) 590 this.candidateTarget = new Patient(); // aa 591 return this.candidateTarget; 592 } 593 594 /** 595 * @param value {@link #candidate} The actual object that is the target of the 596 * reference. The reference library doesn't use these, but you can 597 * use it to hold the resource if you resolve it. (Patient 598 * Resource.) 599 */ 600 public EnrollmentRequest setCandidateTarget(Patient value) { 601 this.candidateTarget = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #coverage} (Reference to the program or plan identification, 607 * underwriter or payor.) 608 */ 609 public Reference getCoverage() { 610 if (this.coverage == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 613 else if (Configuration.doAutoCreate()) 614 this.coverage = new Reference(); // cc 615 return this.coverage; 616 } 617 618 public boolean hasCoverage() { 619 return this.coverage != null && !this.coverage.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #coverage} (Reference to the program or plan 624 * identification, underwriter or payor.) 625 */ 626 public EnrollmentRequest setCoverage(Reference value) { 627 this.coverage = value; 628 return this; 629 } 630 631 /** 632 * @return {@link #coverage} The actual object that is the target of the 633 * reference. The reference library doesn't populate this, but you can 634 * use it to hold the resource if you resolve it. (Reference to the 635 * program or plan identification, underwriter or payor.) 636 */ 637 public Coverage getCoverageTarget() { 638 if (this.coverageTarget == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 641 else if (Configuration.doAutoCreate()) 642 this.coverageTarget = new Coverage(); // aa 643 return this.coverageTarget; 644 } 645 646 /** 647 * @param value {@link #coverage} The actual object that is the target of the 648 * reference. The reference library doesn't use these, but you can 649 * use it to hold the resource if you resolve it. (Reference to the 650 * program or plan identification, underwriter or payor.) 651 */ 652 public EnrollmentRequest setCoverageTarget(Coverage value) { 653 this.coverageTarget = value; 654 return this; 655 } 656 657 protected void listChildren(List<Property> children) { 658 super.listChildren(children); 659 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 660 java.lang.Integer.MAX_VALUE, identifier)); 661 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 662 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 663 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, 664 insurer)); 665 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 666 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 667 children.add(new Property("candidate", "Reference(Patient)", "Patient Resource.", 0, 1, candidate)); 668 children.add(new Property("coverage", "Reference(Coverage)", 669 "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 670 } 671 672 @Override 673 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 674 switch (_hash) { 675 case -1618432855: 676 /* identifier */ return new Property("identifier", "Identifier", "The Response business identifier.", 0, 677 java.lang.Integer.MAX_VALUE, identifier); 678 case -892481550: 679 /* status */ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 680 case 1028554472: 681 /* created */ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, 682 created); 683 case 1957615864: 684 /* insurer */ return new Property("insurer", "Reference(Organization)", 685 "The Insurer who is target of the request.", 0, 1, insurer); 686 case -987494927: 687 /* provider */ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", 688 "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 689 case 508663171: 690 /* candidate */ return new Property("candidate", "Reference(Patient)", "Patient Resource.", 0, 1, candidate); 691 case -351767064: 692 /* coverage */ return new Property("coverage", "Reference(Coverage)", 693 "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 694 default: 695 return super.getNamedProperty(_hash, _name, _checkValid); 696 } 697 698 } 699 700 @Override 701 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 702 switch (hash) { 703 case -1618432855: 704 /* identifier */ return this.identifier == null ? new Base[0] 705 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 706 case -892481550: 707 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EnrollmentRequestStatus> 708 case 1028554472: 709 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateTimeType 710 case 1957615864: 711 /* insurer */ return this.insurer == null ? new Base[0] : new Base[] { this.insurer }; // Reference 712 case -987494927: 713 /* provider */ return this.provider == null ? new Base[0] : new Base[] { this.provider }; // Reference 714 case 508663171: 715 /* candidate */ return this.candidate == null ? new Base[0] : new Base[] { this.candidate }; // Reference 716 case -351767064: 717 /* coverage */ return this.coverage == null ? new Base[0] : new Base[] { this.coverage }; // Reference 718 default: 719 return super.getProperty(hash, name, checkValid); 720 } 721 722 } 723 724 @Override 725 public Base setProperty(int hash, String name, Base value) throws FHIRException { 726 switch (hash) { 727 case -1618432855: // identifier 728 this.getIdentifier().add(castToIdentifier(value)); // Identifier 729 return value; 730 case -892481550: // status 731 value = new EnrollmentRequestStatusEnumFactory().fromType(castToCode(value)); 732 this.status = (Enumeration) value; // Enumeration<EnrollmentRequestStatus> 733 return value; 734 case 1028554472: // created 735 this.created = castToDateTime(value); // DateTimeType 736 return value; 737 case 1957615864: // insurer 738 this.insurer = castToReference(value); // Reference 739 return value; 740 case -987494927: // provider 741 this.provider = castToReference(value); // Reference 742 return value; 743 case 508663171: // candidate 744 this.candidate = castToReference(value); // Reference 745 return value; 746 case -351767064: // coverage 747 this.coverage = castToReference(value); // Reference 748 return value; 749 default: 750 return super.setProperty(hash, name, value); 751 } 752 753 } 754 755 @Override 756 public Base setProperty(String name, Base value) throws FHIRException { 757 if (name.equals("identifier")) { 758 this.getIdentifier().add(castToIdentifier(value)); 759 } else if (name.equals("status")) { 760 value = new EnrollmentRequestStatusEnumFactory().fromType(castToCode(value)); 761 this.status = (Enumeration) value; // Enumeration<EnrollmentRequestStatus> 762 } else if (name.equals("created")) { 763 this.created = castToDateTime(value); // DateTimeType 764 } else if (name.equals("insurer")) { 765 this.insurer = castToReference(value); // Reference 766 } else if (name.equals("provider")) { 767 this.provider = castToReference(value); // Reference 768 } else if (name.equals("candidate")) { 769 this.candidate = castToReference(value); // Reference 770 } else if (name.equals("coverage")) { 771 this.coverage = castToReference(value); // Reference 772 } else 773 return super.setProperty(name, value); 774 return value; 775 } 776 777 @Override 778 public void removeChild(String name, Base value) throws FHIRException { 779 if (name.equals("identifier")) { 780 this.getIdentifier().remove(castToIdentifier(value)); 781 } else if (name.equals("status")) { 782 this.status = null; 783 } else if (name.equals("created")) { 784 this.created = null; 785 } else if (name.equals("insurer")) { 786 this.insurer = null; 787 } else if (name.equals("provider")) { 788 this.provider = null; 789 } else if (name.equals("candidate")) { 790 this.candidate = null; 791 } else if (name.equals("coverage")) { 792 this.coverage = null; 793 } else 794 super.removeChild(name, value); 795 796 } 797 798 @Override 799 public Base makeProperty(int hash, String name) throws FHIRException { 800 switch (hash) { 801 case -1618432855: 802 return addIdentifier(); 803 case -892481550: 804 return getStatusElement(); 805 case 1028554472: 806 return getCreatedElement(); 807 case 1957615864: 808 return getInsurer(); 809 case -987494927: 810 return getProvider(); 811 case 508663171: 812 return getCandidate(); 813 case -351767064: 814 return getCoverage(); 815 default: 816 return super.makeProperty(hash, name); 817 } 818 819 } 820 821 @Override 822 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 823 switch (hash) { 824 case -1618432855: 825 /* identifier */ return new String[] { "Identifier" }; 826 case -892481550: 827 /* status */ return new String[] { "code" }; 828 case 1028554472: 829 /* created */ return new String[] { "dateTime" }; 830 case 1957615864: 831 /* insurer */ return new String[] { "Reference" }; 832 case -987494927: 833 /* provider */ return new String[] { "Reference" }; 834 case 508663171: 835 /* candidate */ return new String[] { "Reference" }; 836 case -351767064: 837 /* coverage */ return new String[] { "Reference" }; 838 default: 839 return super.getTypesForProperty(hash, name); 840 } 841 842 } 843 844 @Override 845 public Base addChild(String name) throws FHIRException { 846 if (name.equals("identifier")) { 847 return addIdentifier(); 848 } else if (name.equals("status")) { 849 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.status"); 850 } else if (name.equals("created")) { 851 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.created"); 852 } else if (name.equals("insurer")) { 853 this.insurer = new Reference(); 854 return this.insurer; 855 } else if (name.equals("provider")) { 856 this.provider = new Reference(); 857 return this.provider; 858 } else if (name.equals("candidate")) { 859 this.candidate = new Reference(); 860 return this.candidate; 861 } else if (name.equals("coverage")) { 862 this.coverage = new Reference(); 863 return this.coverage; 864 } else 865 return super.addChild(name); 866 } 867 868 public String fhirType() { 869 return "EnrollmentRequest"; 870 871 } 872 873 public EnrollmentRequest copy() { 874 EnrollmentRequest dst = new EnrollmentRequest(); 875 copyValues(dst); 876 return dst; 877 } 878 879 public void copyValues(EnrollmentRequest dst) { 880 super.copyValues(dst); 881 if (identifier != null) { 882 dst.identifier = new ArrayList<Identifier>(); 883 for (Identifier i : identifier) 884 dst.identifier.add(i.copy()); 885 } 886 ; 887 dst.status = status == null ? null : status.copy(); 888 dst.created = created == null ? null : created.copy(); 889 dst.insurer = insurer == null ? null : insurer.copy(); 890 dst.provider = provider == null ? null : provider.copy(); 891 dst.candidate = candidate == null ? null : candidate.copy(); 892 dst.coverage = coverage == null ? null : coverage.copy(); 893 } 894 895 protected EnrollmentRequest typedCopy() { 896 return copy(); 897 } 898 899 @Override 900 public boolean equalsDeep(Base other_) { 901 if (!super.equalsDeep(other_)) 902 return false; 903 if (!(other_ instanceof EnrollmentRequest)) 904 return false; 905 EnrollmentRequest o = (EnrollmentRequest) other_; 906 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 907 && compareDeep(created, o.created, true) && compareDeep(insurer, o.insurer, true) 908 && compareDeep(provider, o.provider, true) && compareDeep(candidate, o.candidate, true) 909 && compareDeep(coverage, o.coverage, true); 910 } 911 912 @Override 913 public boolean equalsShallow(Base other_) { 914 if (!super.equalsShallow(other_)) 915 return false; 916 if (!(other_ instanceof EnrollmentRequest)) 917 return false; 918 EnrollmentRequest o = (EnrollmentRequest) other_; 919 return compareValues(status, o.status, true) && compareValues(created, o.created, true); 920 } 921 922 public boolean isEmpty() { 923 return super.isEmpty() 924 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created, insurer, provider, candidate, coverage); 925 } 926 927 @Override 928 public ResourceType getResourceType() { 929 return ResourceType.EnrollmentRequest; 930 } 931 932 /** 933 * Search parameter: <b>identifier</b> 934 * <p> 935 * Description: <b>The business identifier of the Enrollment</b><br> 936 * Type: <b>token</b><br> 937 * Path: <b>EnrollmentRequest.identifier</b><br> 938 * </p> 939 */ 940 @SearchParamDefinition(name = "identifier", path = "EnrollmentRequest.identifier", description = "The business identifier of the Enrollment", type = "token") 941 public static final String SP_IDENTIFIER = "identifier"; 942 /** 943 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 944 * <p> 945 * Description: <b>The business identifier of the Enrollment</b><br> 946 * Type: <b>token</b><br> 947 * Path: <b>EnrollmentRequest.identifier</b><br> 948 * </p> 949 */ 950 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 951 SP_IDENTIFIER); 952 953 /** 954 * Search parameter: <b>subject</b> 955 * <p> 956 * Description: <b>The party to be enrolled</b><br> 957 * Type: <b>reference</b><br> 958 * Path: <b>EnrollmentRequest.candidate</b><br> 959 * </p> 960 */ 961 @SearchParamDefinition(name = "subject", path = "EnrollmentRequest.candidate", description = "The party to be enrolled", type = "reference", providesMembershipIn = { 962 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 963 public static final String SP_SUBJECT = "subject"; 964 /** 965 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 966 * <p> 967 * Description: <b>The party to be enrolled</b><br> 968 * Type: <b>reference</b><br> 969 * Path: <b>EnrollmentRequest.candidate</b><br> 970 * </p> 971 */ 972 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 973 SP_SUBJECT); 974 975 /** 976 * Constant for fluent queries to be used to add include statements. Specifies 977 * the path value of "<b>EnrollmentRequest:subject</b>". 978 */ 979 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 980 "EnrollmentRequest:subject").toLocked(); 981 982 /** 983 * Search parameter: <b>patient</b> 984 * <p> 985 * Description: <b>The party to be enrolled</b><br> 986 * Type: <b>reference</b><br> 987 * Path: <b>EnrollmentRequest.candidate</b><br> 988 * </p> 989 */ 990 @SearchParamDefinition(name = "patient", path = "EnrollmentRequest.candidate", description = "The party to be enrolled", type = "reference", target = { 991 Patient.class }) 992 public static final String SP_PATIENT = "patient"; 993 /** 994 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 995 * <p> 996 * Description: <b>The party to be enrolled</b><br> 997 * Type: <b>reference</b><br> 998 * Path: <b>EnrollmentRequest.candidate</b><br> 999 * </p> 1000 */ 1001 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1002 SP_PATIENT); 1003 1004 /** 1005 * Constant for fluent queries to be used to add include statements. Specifies 1006 * the path value of "<b>EnrollmentRequest:patient</b>". 1007 */ 1008 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1009 "EnrollmentRequest:patient").toLocked(); 1010 1011 /** 1012 * Search parameter: <b>status</b> 1013 * <p> 1014 * Description: <b>The status of the enrollment</b><br> 1015 * Type: <b>token</b><br> 1016 * Path: <b>EnrollmentRequest.status</b><br> 1017 * </p> 1018 */ 1019 @SearchParamDefinition(name = "status", path = "EnrollmentRequest.status", description = "The status of the enrollment", type = "token") 1020 public static final String SP_STATUS = "status"; 1021 /** 1022 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1023 * <p> 1024 * Description: <b>The status of the enrollment</b><br> 1025 * Type: <b>token</b><br> 1026 * Path: <b>EnrollmentRequest.status</b><br> 1027 * </p> 1028 */ 1029 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1030 SP_STATUS); 1031 1032}