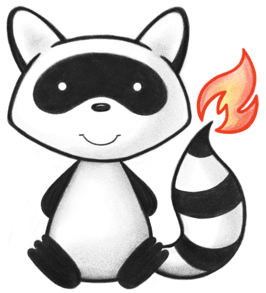
001package org.hl7.fhir.r4.model; 002 003import org.hl7.fhir.exceptions.FHIRException; 004 005public class Enumerations { 006 007// In here: 008// AbstractType: A list of the base types defined by this version of the FHIR specification - types that are defined, but for which only specializations actually are created. 009// AdministrativeGender: The gender of a person used for administrative purposes. 010// AgeUnits: A valueSet of UCUM codes for representing age value units. 011// BindingStrength: Indication of the degree of conformance expectations associated with a binding. 012// ConceptMapEquivalence: The degree of equivalence between concepts. 013// DataAbsentReason: Used to specify why the normally expected content of the data element is missing. 014// DataType: A version specific list of the data types defined by the FHIR specification for use as an element type (any of the FHIR defined data types). 015// DefinitionResourceType: A list of all the definition resource types defined in this version of the FHIR specification. 016// DocumentReferenceStatus: The status of the document reference. 017// EventResourceType: A list of all the event resource types defined in this version of the FHIR specification. 018// FHIRAllTypes: A list of all the concrete types defined in this version of the FHIR specification - Abstract Types, Data Types and Resource Types. 019// FHIRDefinedType: A list of all the concrete types defined in this version of the FHIR specification - Data Types and Resource Types. 020// FHIRVersion: All published FHIR Versions. 021// KnowledgeResourceType: A list of all the knowledge resource types defined in this version of the FHIR specification. 022// MessageEvent: One of the message events defined as part of this version of FHIR. 023// NoteType: The presentation types of notes. 024// PublicationStatus: The lifecycle status of an artifact. 025// RemittanceOutcome: The outcome of the processing. 026// RequestResourceType: A list of all the request resource types defined in this version of the FHIR specification. 027// ResourceType: One of the resource types defined as part of this version of FHIR. 028// SearchParamType: Data types allowed to be used for search parameters. 029// SpecialValues: A set of generally useful codes defined so they can be included in value sets. 030 031 public enum AbstractType { 032 /** 033 * A place holder that means any kind of data type 034 */ 035 TYPE, 036 /** 037 * A place holder that means any kind of resource 038 */ 039 ANY, 040 /** 041 * added to help the parsers 042 */ 043 NULL; 044 045 public static AbstractType fromCode(String codeString) throws FHIRException { 046 if (codeString == null || "".equals(codeString)) 047 return null; 048 if ("Type".equals(codeString)) 049 return TYPE; 050 if ("Any".equals(codeString)) 051 return ANY; 052 throw new FHIRException("Unknown AbstractType code '" + codeString + "'"); 053 } 054 055 public String toCode() { 056 switch (this) { 057 case TYPE: 058 return "Type"; 059 case ANY: 060 return "Any"; 061 case NULL: 062 return null; 063 default: 064 return "?"; 065 } 066 } 067 068 public String getSystem() { 069 switch (this) { 070 case TYPE: 071 return "http://hl7.org/fhir/abstract-types"; 072 case ANY: 073 return "http://hl7.org/fhir/abstract-types"; 074 case NULL: 075 return null; 076 default: 077 return "?"; 078 } 079 } 080 081 public String getDefinition() { 082 switch (this) { 083 case TYPE: 084 return "A place holder that means any kind of data type"; 085 case ANY: 086 return "A place holder that means any kind of resource"; 087 case NULL: 088 return null; 089 default: 090 return "?"; 091 } 092 } 093 094 public String getDisplay() { 095 switch (this) { 096 case TYPE: 097 return "Type"; 098 case ANY: 099 return "Any"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 } 107 108 public static class AbstractTypeEnumFactory implements EnumFactory<AbstractType> { 109 public AbstractType fromCode(String codeString) throws IllegalArgumentException { 110 if (codeString == null || "".equals(codeString)) 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("Type".equals(codeString)) 114 return AbstractType.TYPE; 115 if ("Any".equals(codeString)) 116 return AbstractType.ANY; 117 throw new IllegalArgumentException("Unknown AbstractType code '" + codeString + "'"); 118 } 119 120 public Enumeration<AbstractType> fromType(PrimitiveType<?> code) throws FHIRException { 121 if (code == null) 122 return null; 123 if (code.isEmpty()) 124 return new Enumeration<AbstractType>(this, AbstractType.NULL, code); 125 String codeString = code.asStringValue(); 126 if (codeString == null || "".equals(codeString)) 127 return new Enumeration<AbstractType>(this, AbstractType.NULL, code); 128 if ("Type".equals(codeString)) 129 return new Enumeration<AbstractType>(this, AbstractType.TYPE, code); 130 if ("Any".equals(codeString)) 131 return new Enumeration<AbstractType>(this, AbstractType.ANY, code); 132 throw new FHIRException("Unknown AbstractType code '" + codeString + "'"); 133 } 134 135 public String toCode(AbstractType code) { 136 if (code == AbstractType.TYPE) 137 return "Type"; 138 if (code == AbstractType.ANY) 139 return "Any"; 140 return "?"; 141 } 142 143 public String toSystem(AbstractType code) { 144 return code.getSystem(); 145 } 146 } 147 148 public enum AdministrativeGender { 149 /** 150 * Male. 151 */ 152 MALE, 153 /** 154 * Female. 155 */ 156 FEMALE, 157 /** 158 * Other. 159 */ 160 OTHER, 161 /** 162 * Unknown. 163 */ 164 UNKNOWN, 165 /** 166 * added to help the parsers 167 */ 168 NULL; 169 170 public static AdministrativeGender fromCode(String codeString) throws FHIRException { 171 if (codeString == null || "".equals(codeString)) 172 return null; 173 if ("male".equals(codeString)) 174 return MALE; 175 if ("female".equals(codeString)) 176 return FEMALE; 177 if ("other".equals(codeString)) 178 return OTHER; 179 if ("unknown".equals(codeString)) 180 return UNKNOWN; 181 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 182 } 183 184 public String toCode() { 185 switch (this) { 186 case MALE: 187 return "male"; 188 case FEMALE: 189 return "female"; 190 case OTHER: 191 return "other"; 192 case UNKNOWN: 193 return "unknown"; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 201 public String getSystem() { 202 switch (this) { 203 case MALE: 204 return "http://hl7.org/fhir/administrative-gender"; 205 case FEMALE: 206 return "http://hl7.org/fhir/administrative-gender"; 207 case OTHER: 208 return "http://hl7.org/fhir/administrative-gender"; 209 case UNKNOWN: 210 return "http://hl7.org/fhir/administrative-gender"; 211 case NULL: 212 return null; 213 default: 214 return "?"; 215 } 216 } 217 218 public String getDefinition() { 219 switch (this) { 220 case MALE: 221 return "Male."; 222 case FEMALE: 223 return "Female."; 224 case OTHER: 225 return "Other."; 226 case UNKNOWN: 227 return "Unknown."; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 235 public String getDisplay() { 236 switch (this) { 237 case MALE: 238 return "Male"; 239 case FEMALE: 240 return "Female"; 241 case OTHER: 242 return "Other"; 243 case UNKNOWN: 244 return "Unknown"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 } 252 253 public static class AdministrativeGenderEnumFactory implements EnumFactory<AdministrativeGender> { 254 public AdministrativeGender fromCode(String codeString) throws IllegalArgumentException { 255 if (codeString == null || "".equals(codeString)) 256 if (codeString == null || "".equals(codeString)) 257 return null; 258 if ("male".equals(codeString)) 259 return AdministrativeGender.MALE; 260 if ("female".equals(codeString)) 261 return AdministrativeGender.FEMALE; 262 if ("other".equals(codeString)) 263 return AdministrativeGender.OTHER; 264 if ("unknown".equals(codeString)) 265 return AdministrativeGender.UNKNOWN; 266 throw new IllegalArgumentException("Unknown AdministrativeGender code '" + codeString + "'"); 267 } 268 269 public Enumeration<AdministrativeGender> fromType(PrimitiveType<?> code) throws FHIRException { 270 if (code == null) 271 return null; 272 if (code.isEmpty()) 273 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.NULL, code); 274 String codeString = code.asStringValue(); 275 if (codeString == null || "".equals(codeString)) 276 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.NULL, code); 277 if ("male".equals(codeString)) 278 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.MALE, code); 279 if ("female".equals(codeString)) 280 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.FEMALE, code); 281 if ("other".equals(codeString)) 282 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.OTHER, code); 283 if ("unknown".equals(codeString)) 284 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.UNKNOWN, code); 285 throw new FHIRException("Unknown AdministrativeGender code '" + codeString + "'"); 286 } 287 288 public String toCode(AdministrativeGender code) { 289 if (code == AdministrativeGender.MALE) 290 return "male"; 291 if (code == AdministrativeGender.FEMALE) 292 return "female"; 293 if (code == AdministrativeGender.OTHER) 294 return "other"; 295 if (code == AdministrativeGender.UNKNOWN) 296 return "unknown"; 297 return "?"; 298 } 299 300 public String toSystem(AdministrativeGender code) { 301 return code.getSystem(); 302 } 303 } 304 305 public enum AgeUnits { 306 /** 307 * null 308 */ 309 MIN, 310 /** 311 * null 312 */ 313 H, 314 /** 315 * null 316 */ 317 D, 318 /** 319 * null 320 */ 321 WK, 322 /** 323 * null 324 */ 325 MO, 326 /** 327 * null 328 */ 329 A, 330 /** 331 * added to help the parsers 332 */ 333 NULL; 334 335 public static AgeUnits fromCode(String codeString) throws FHIRException { 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("min".equals(codeString)) 339 return MIN; 340 if ("h".equals(codeString)) 341 return H; 342 if ("d".equals(codeString)) 343 return D; 344 if ("wk".equals(codeString)) 345 return WK; 346 if ("mo".equals(codeString)) 347 return MO; 348 if ("a".equals(codeString)) 349 return A; 350 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 351 } 352 353 public String toCode() { 354 switch (this) { 355 case MIN: 356 return "min"; 357 case H: 358 return "h"; 359 case D: 360 return "d"; 361 case WK: 362 return "wk"; 363 case MO: 364 return "mo"; 365 case A: 366 return "a"; 367 case NULL: 368 return null; 369 default: 370 return "?"; 371 } 372 } 373 374 public String getSystem() { 375 switch (this) { 376 case MIN: 377 return "http://unitsofmeasure.org"; 378 case H: 379 return "http://unitsofmeasure.org"; 380 case D: 381 return "http://unitsofmeasure.org"; 382 case WK: 383 return "http://unitsofmeasure.org"; 384 case MO: 385 return "http://unitsofmeasure.org"; 386 case A: 387 return "http://unitsofmeasure.org"; 388 case NULL: 389 return null; 390 default: 391 return "?"; 392 } 393 } 394 395 public String getDefinition() { 396 switch (this) { 397 case MIN: 398 return ""; 399 case H: 400 return ""; 401 case D: 402 return ""; 403 case WK: 404 return ""; 405 case MO: 406 return ""; 407 case A: 408 return ""; 409 case NULL: 410 return null; 411 default: 412 return "?"; 413 } 414 } 415 416 public String getDisplay() { 417 switch (this) { 418 case MIN: 419 return "Minute"; 420 case H: 421 return "Hour"; 422 case D: 423 return "Day"; 424 case WK: 425 return "Week"; 426 case MO: 427 return "Month"; 428 case A: 429 return "Year"; 430 case NULL: 431 return null; 432 default: 433 return "?"; 434 } 435 } 436 } 437 438 public static class AgeUnitsEnumFactory implements EnumFactory<AgeUnits> { 439 public AgeUnits fromCode(String codeString) throws IllegalArgumentException { 440 if (codeString == null || "".equals(codeString)) 441 if (codeString == null || "".equals(codeString)) 442 return null; 443 if ("min".equals(codeString)) 444 return AgeUnits.MIN; 445 if ("h".equals(codeString)) 446 return AgeUnits.H; 447 if ("d".equals(codeString)) 448 return AgeUnits.D; 449 if ("wk".equals(codeString)) 450 return AgeUnits.WK; 451 if ("mo".equals(codeString)) 452 return AgeUnits.MO; 453 if ("a".equals(codeString)) 454 return AgeUnits.A; 455 throw new IllegalArgumentException("Unknown AgeUnits code '" + codeString + "'"); 456 } 457 458 public Enumeration<AgeUnits> fromType(PrimitiveType<?> code) throws FHIRException { 459 if (code == null) 460 return null; 461 if (code.isEmpty()) 462 return new Enumeration<AgeUnits>(this, AgeUnits.NULL, code); 463 String codeString = code.asStringValue(); 464 if (codeString == null || "".equals(codeString)) 465 return new Enumeration<AgeUnits>(this, AgeUnits.NULL, code); 466 if ("min".equals(codeString)) 467 return new Enumeration<AgeUnits>(this, AgeUnits.MIN, code); 468 if ("h".equals(codeString)) 469 return new Enumeration<AgeUnits>(this, AgeUnits.H, code); 470 if ("d".equals(codeString)) 471 return new Enumeration<AgeUnits>(this, AgeUnits.D, code); 472 if ("wk".equals(codeString)) 473 return new Enumeration<AgeUnits>(this, AgeUnits.WK, code); 474 if ("mo".equals(codeString)) 475 return new Enumeration<AgeUnits>(this, AgeUnits.MO, code); 476 if ("a".equals(codeString)) 477 return new Enumeration<AgeUnits>(this, AgeUnits.A, code); 478 throw new FHIRException("Unknown AgeUnits code '" + codeString + "'"); 479 } 480 481 public String toCode(AgeUnits code) { 482 if (code == AgeUnits.MIN) 483 return "min"; 484 if (code == AgeUnits.H) 485 return "h"; 486 if (code == AgeUnits.D) 487 return "d"; 488 if (code == AgeUnits.WK) 489 return "wk"; 490 if (code == AgeUnits.MO) 491 return "mo"; 492 if (code == AgeUnits.A) 493 return "a"; 494 return "?"; 495 } 496 497 public String toSystem(AgeUnits code) { 498 return code.getSystem(); 499 } 500 } 501 502 public enum BindingStrength { 503 /** 504 * To be conformant, the concept in this element SHALL be from the specified 505 * value set. 506 */ 507 REQUIRED, 508 /** 509 * To be conformant, the concept in this element SHALL be from the specified 510 * value set if any of the codes within the value set can apply to the concept 511 * being communicated. If the value set does not cover the concept (based on 512 * human review), alternate codings (or, data type allowing, text) may be 513 * included instead. 514 */ 515 EXTENSIBLE, 516 /** 517 * Instances are encouraged to draw from the specified codes for 518 * interoperability purposes but are not required to do so to be considered 519 * conformant. 520 */ 521 PREFERRED, 522 /** 523 * Instances are not expected or even encouraged to draw from the specified 524 * value set. The value set merely provides examples of the types of concepts 525 * intended to be included. 526 */ 527 EXAMPLE, 528 /** 529 * added to help the parsers 530 */ 531 NULL; 532 533 public static BindingStrength fromCode(String codeString) throws FHIRException { 534 if (codeString == null || "".equals(codeString)) 535 return null; 536 if ("required".equals(codeString)) 537 return REQUIRED; 538 if ("extensible".equals(codeString)) 539 return EXTENSIBLE; 540 if ("preferred".equals(codeString)) 541 return PREFERRED; 542 if ("example".equals(codeString)) 543 return EXAMPLE; 544 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 545 } 546 547 public String toCode() { 548 switch (this) { 549 case REQUIRED: 550 return "required"; 551 case EXTENSIBLE: 552 return "extensible"; 553 case PREFERRED: 554 return "preferred"; 555 case EXAMPLE: 556 return "example"; 557 case NULL: 558 return null; 559 default: 560 return "?"; 561 } 562 } 563 564 public String getSystem() { 565 switch (this) { 566 case REQUIRED: 567 return "http://hl7.org/fhir/binding-strength"; 568 case EXTENSIBLE: 569 return "http://hl7.org/fhir/binding-strength"; 570 case PREFERRED: 571 return "http://hl7.org/fhir/binding-strength"; 572 case EXAMPLE: 573 return "http://hl7.org/fhir/binding-strength"; 574 case NULL: 575 return null; 576 default: 577 return "?"; 578 } 579 } 580 581 public String getDefinition() { 582 switch (this) { 583 case REQUIRED: 584 return "To be conformant, the concept in this element SHALL be from the specified value set."; 585 case EXTENSIBLE: 586 return "To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead."; 587 case PREFERRED: 588 return "Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant."; 589 case EXAMPLE: 590 return "Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included."; 591 case NULL: 592 return null; 593 default: 594 return "?"; 595 } 596 } 597 598 public String getDisplay() { 599 switch (this) { 600 case REQUIRED: 601 return "Required"; 602 case EXTENSIBLE: 603 return "Extensible"; 604 case PREFERRED: 605 return "Preferred"; 606 case EXAMPLE: 607 return "Example"; 608 case NULL: 609 return null; 610 default: 611 return "?"; 612 } 613 } 614 } 615 616 public static class BindingStrengthEnumFactory implements EnumFactory<BindingStrength> { 617 public BindingStrength fromCode(String codeString) throws IllegalArgumentException { 618 if (codeString == null || "".equals(codeString)) 619 if (codeString == null || "".equals(codeString)) 620 return null; 621 if ("required".equals(codeString)) 622 return BindingStrength.REQUIRED; 623 if ("extensible".equals(codeString)) 624 return BindingStrength.EXTENSIBLE; 625 if ("preferred".equals(codeString)) 626 return BindingStrength.PREFERRED; 627 if ("example".equals(codeString)) 628 return BindingStrength.EXAMPLE; 629 throw new IllegalArgumentException("Unknown BindingStrength code '" + codeString + "'"); 630 } 631 632 public Enumeration<BindingStrength> fromType(PrimitiveType<?> code) throws FHIRException { 633 if (code == null) 634 return null; 635 if (code.isEmpty()) 636 return new Enumeration<BindingStrength>(this, BindingStrength.NULL, code); 637 String codeString = code.asStringValue(); 638 if (codeString == null || "".equals(codeString)) 639 return new Enumeration<BindingStrength>(this, BindingStrength.NULL, code); 640 if ("required".equals(codeString)) 641 return new Enumeration<BindingStrength>(this, BindingStrength.REQUIRED, code); 642 if ("extensible".equals(codeString)) 643 return new Enumeration<BindingStrength>(this, BindingStrength.EXTENSIBLE, code); 644 if ("preferred".equals(codeString)) 645 return new Enumeration<BindingStrength>(this, BindingStrength.PREFERRED, code); 646 if ("example".equals(codeString)) 647 return new Enumeration<BindingStrength>(this, BindingStrength.EXAMPLE, code); 648 throw new FHIRException("Unknown BindingStrength code '" + codeString + "'"); 649 } 650 651 public String toCode(BindingStrength code) { 652 if (code == BindingStrength.REQUIRED) 653 return "required"; 654 if (code == BindingStrength.EXTENSIBLE) 655 return "extensible"; 656 if (code == BindingStrength.PREFERRED) 657 return "preferred"; 658 if (code == BindingStrength.EXAMPLE) 659 return "example"; 660 return "?"; 661 } 662 663 public String toSystem(BindingStrength code) { 664 return code.getSystem(); 665 } 666 } 667 668 public enum ConceptMapEquivalence { 669 /** 670 * The concepts are related to each other, and have at least some overlap in 671 * meaning, but the exact relationship is not known. 672 */ 673 RELATEDTO, 674 /** 675 * The definitions of the concepts mean the same thing (including when 676 * structural implications of meaning are considered) (i.e. extensionally 677 * identical). 678 */ 679 EQUIVALENT, 680 /** 681 * The definitions of the concepts are exactly the same (i.e. only grammatical 682 * differences) and structural implications of meaning are identical or 683 * irrelevant (i.e. intentionally identical). 684 */ 685 EQUAL, 686 /** 687 * The target mapping is wider in meaning than the source concept. 688 */ 689 WIDER, 690 /** 691 * The target mapping subsumes the meaning of the source concept (e.g. the 692 * source is-a target). 693 */ 694 SUBSUMES, 695 /** 696 * The target mapping is narrower in meaning than the source concept. The sense 697 * in which the mapping is narrower SHALL be described in the comments in this 698 * case, and applications should be careful when attempting to use these 699 * mappings operationally. 700 */ 701 NARROWER, 702 /** 703 * The target mapping specializes the meaning of the source concept (e.g. the 704 * target is-a source). 705 */ 706 SPECIALIZES, 707 /** 708 * The target mapping overlaps with the source concept, but both source and 709 * target cover additional meaning, or the definitions are imprecise and it is 710 * uncertain whether they have the same boundaries to their meaning. The sense 711 * in which the mapping is inexact SHALL be described in the comments in this 712 * case, and applications should be careful when attempting to use these 713 * mappings operationally. 714 */ 715 INEXACT, 716 /** 717 * There is no match for this concept in the target code system. 718 */ 719 UNMATCHED, 720 /** 721 * This is an explicit assertion that there is no mapping between the source and 722 * target concept. 723 */ 724 DISJOINT, 725 /** 726 * added to help the parsers 727 */ 728 NULL; 729 730 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 731 if (codeString == null || "".equals(codeString)) 732 return null; 733 if ("relatedto".equals(codeString)) 734 return RELATEDTO; 735 if ("equivalent".equals(codeString)) 736 return EQUIVALENT; 737 if ("equal".equals(codeString)) 738 return EQUAL; 739 if ("wider".equals(codeString)) 740 return WIDER; 741 if ("subsumes".equals(codeString)) 742 return SUBSUMES; 743 if ("narrower".equals(codeString)) 744 return NARROWER; 745 if ("specializes".equals(codeString)) 746 return SPECIALIZES; 747 if ("inexact".equals(codeString)) 748 return INEXACT; 749 if ("unmatched".equals(codeString)) 750 return UNMATCHED; 751 if ("disjoint".equals(codeString)) 752 return DISJOINT; 753 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 754 } 755 756 public String toCode() { 757 switch (this) { 758 case RELATEDTO: 759 return "relatedto"; 760 case EQUIVALENT: 761 return "equivalent"; 762 case EQUAL: 763 return "equal"; 764 case WIDER: 765 return "wider"; 766 case SUBSUMES: 767 return "subsumes"; 768 case NARROWER: 769 return "narrower"; 770 case SPECIALIZES: 771 return "specializes"; 772 case INEXACT: 773 return "inexact"; 774 case UNMATCHED: 775 return "unmatched"; 776 case DISJOINT: 777 return "disjoint"; 778 case NULL: 779 return null; 780 default: 781 return "?"; 782 } 783 } 784 785 public String getSystem() { 786 switch (this) { 787 case RELATEDTO: 788 return "http://hl7.org/fhir/concept-map-equivalence"; 789 case EQUIVALENT: 790 return "http://hl7.org/fhir/concept-map-equivalence"; 791 case EQUAL: 792 return "http://hl7.org/fhir/concept-map-equivalence"; 793 case WIDER: 794 return "http://hl7.org/fhir/concept-map-equivalence"; 795 case SUBSUMES: 796 return "http://hl7.org/fhir/concept-map-equivalence"; 797 case NARROWER: 798 return "http://hl7.org/fhir/concept-map-equivalence"; 799 case SPECIALIZES: 800 return "http://hl7.org/fhir/concept-map-equivalence"; 801 case INEXACT: 802 return "http://hl7.org/fhir/concept-map-equivalence"; 803 case UNMATCHED: 804 return "http://hl7.org/fhir/concept-map-equivalence"; 805 case DISJOINT: 806 return "http://hl7.org/fhir/concept-map-equivalence"; 807 case NULL: 808 return null; 809 default: 810 return "?"; 811 } 812 } 813 814 public String getDefinition() { 815 switch (this) { 816 case RELATEDTO: 817 return "The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known."; 818 case EQUIVALENT: 819 return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 820 case EQUAL: 821 return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 822 case WIDER: 823 return "The target mapping is wider in meaning than the source concept."; 824 case SUBSUMES: 825 return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 826 case NARROWER: 827 return "The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 828 case SPECIALIZES: 829 return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 830 case INEXACT: 831 return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is inexact SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 832 case UNMATCHED: 833 return "There is no match for this concept in the target code system."; 834 case DISJOINT: 835 return "This is an explicit assertion that there is no mapping between the source and target concept."; 836 case NULL: 837 return null; 838 default: 839 return "?"; 840 } 841 } 842 843 public String getDisplay() { 844 switch (this) { 845 case RELATEDTO: 846 return "Related To"; 847 case EQUIVALENT: 848 return "Equivalent"; 849 case EQUAL: 850 return "Equal"; 851 case WIDER: 852 return "Wider"; 853 case SUBSUMES: 854 return "Subsumes"; 855 case NARROWER: 856 return "Narrower"; 857 case SPECIALIZES: 858 return "Specializes"; 859 case INEXACT: 860 return "Inexact"; 861 case UNMATCHED: 862 return "Unmatched"; 863 case DISJOINT: 864 return "Disjoint"; 865 case NULL: 866 return null; 867 default: 868 return "?"; 869 } 870 } 871 } 872 873 public static class ConceptMapEquivalenceEnumFactory implements EnumFactory<ConceptMapEquivalence> { 874 public ConceptMapEquivalence fromCode(String codeString) throws IllegalArgumentException { 875 if (codeString == null || "".equals(codeString)) 876 if (codeString == null || "".equals(codeString)) 877 return null; 878 if ("relatedto".equals(codeString)) 879 return ConceptMapEquivalence.RELATEDTO; 880 if ("equivalent".equals(codeString)) 881 return ConceptMapEquivalence.EQUIVALENT; 882 if ("equal".equals(codeString)) 883 return ConceptMapEquivalence.EQUAL; 884 if ("wider".equals(codeString)) 885 return ConceptMapEquivalence.WIDER; 886 if ("subsumes".equals(codeString)) 887 return ConceptMapEquivalence.SUBSUMES; 888 if ("narrower".equals(codeString)) 889 return ConceptMapEquivalence.NARROWER; 890 if ("specializes".equals(codeString)) 891 return ConceptMapEquivalence.SPECIALIZES; 892 if ("inexact".equals(codeString)) 893 return ConceptMapEquivalence.INEXACT; 894 if ("unmatched".equals(codeString)) 895 return ConceptMapEquivalence.UNMATCHED; 896 if ("disjoint".equals(codeString)) 897 return ConceptMapEquivalence.DISJOINT; 898 throw new IllegalArgumentException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 899 } 900 901 public Enumeration<ConceptMapEquivalence> fromType(PrimitiveType<?> code) throws FHIRException { 902 if (code == null) 903 return null; 904 if (code.isEmpty()) 905 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NULL, code); 906 String codeString = code.asStringValue(); 907 if (codeString == null || "".equals(codeString)) 908 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NULL, code); 909 if ("relatedto".equals(codeString)) 910 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.RELATEDTO, code); 911 if ("equivalent".equals(codeString)) 912 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUIVALENT, code); 913 if ("equal".equals(codeString)) 914 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUAL, code); 915 if ("wider".equals(codeString)) 916 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.WIDER, code); 917 if ("subsumes".equals(codeString)) 918 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SUBSUMES, code); 919 if ("narrower".equals(codeString)) 920 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NARROWER, code); 921 if ("specializes".equals(codeString)) 922 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SPECIALIZES, code); 923 if ("inexact".equals(codeString)) 924 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.INEXACT, code); 925 if ("unmatched".equals(codeString)) 926 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.UNMATCHED, code); 927 if ("disjoint".equals(codeString)) 928 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.DISJOINT, code); 929 throw new FHIRException("Unknown ConceptMapEquivalence code '" + codeString + "'"); 930 } 931 932 public String toCode(ConceptMapEquivalence code) { 933 if (code == ConceptMapEquivalence.RELATEDTO) 934 return "relatedto"; 935 if (code == ConceptMapEquivalence.EQUIVALENT) 936 return "equivalent"; 937 if (code == ConceptMapEquivalence.EQUAL) 938 return "equal"; 939 if (code == ConceptMapEquivalence.WIDER) 940 return "wider"; 941 if (code == ConceptMapEquivalence.SUBSUMES) 942 return "subsumes"; 943 if (code == ConceptMapEquivalence.NARROWER) 944 return "narrower"; 945 if (code == ConceptMapEquivalence.SPECIALIZES) 946 return "specializes"; 947 if (code == ConceptMapEquivalence.INEXACT) 948 return "inexact"; 949 if (code == ConceptMapEquivalence.UNMATCHED) 950 return "unmatched"; 951 if (code == ConceptMapEquivalence.DISJOINT) 952 return "disjoint"; 953 return "?"; 954 } 955 956 public String toSystem(ConceptMapEquivalence code) { 957 return code.getSystem(); 958 } 959 } 960 961 public enum DataAbsentReason { 962 /** 963 * The value is expected to exist but is not known. 964 */ 965 UNKNOWN, 966 /** 967 * The source was asked but does not know the value. 968 */ 969 ASKEDUNKNOWN, 970 /** 971 * There is reason to expect (from the workflow) that the value may become 972 * known. 973 */ 974 TEMPUNKNOWN, 975 /** 976 * The workflow didn't lead to this value being known. 977 */ 978 NOTASKED, 979 /** 980 * The source was asked but declined to answer. 981 */ 982 ASKEDDECLINED, 983 /** 984 * The information is not available due to security, privacy or related reasons. 985 */ 986 MASKED, 987 /** 988 * There is no proper value for this element (e.g. last menstrual period for a 989 * male). 990 */ 991 NOTAPPLICABLE, 992 /** 993 * The source system wasn't capable of supporting this element. 994 */ 995 UNSUPPORTED, 996 /** 997 * The content of the data is represented in the resource narrative. 998 */ 999 ASTEXT, 1000 /** 1001 * Some system or workflow process error means that the information is not 1002 * available. 1003 */ 1004 ERROR, 1005 /** 1006 * The numeric value is undefined or unrepresentable due to a floating point 1007 * processing error. 1008 */ 1009 NOTANUMBER, 1010 /** 1011 * The numeric value is excessively low and unrepresentable due to a floating 1012 * point processing error. 1013 */ 1014 NEGATIVEINFINITY, 1015 /** 1016 * The numeric value is excessively high and unrepresentable due to a floating 1017 * point processing error. 1018 */ 1019 POSITIVEINFINITY, 1020 /** 1021 * The value is not available because the observation procedure (test, etc.) was 1022 * not performed. 1023 */ 1024 NOTPERFORMED, 1025 /** 1026 * The value is not permitted in this context (e.g. due to profiles, or the base 1027 * data types). 1028 */ 1029 NOTPERMITTED, 1030 /** 1031 * added to help the parsers 1032 */ 1033 NULL; 1034 1035 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 1036 if (codeString == null || "".equals(codeString)) 1037 return null; 1038 if ("unknown".equals(codeString)) 1039 return UNKNOWN; 1040 if ("asked-unknown".equals(codeString)) 1041 return ASKEDUNKNOWN; 1042 if ("temp-unknown".equals(codeString)) 1043 return TEMPUNKNOWN; 1044 if ("not-asked".equals(codeString)) 1045 return NOTASKED; 1046 if ("asked-declined".equals(codeString)) 1047 return ASKEDDECLINED; 1048 if ("masked".equals(codeString)) 1049 return MASKED; 1050 if ("not-applicable".equals(codeString)) 1051 return NOTAPPLICABLE; 1052 if ("unsupported".equals(codeString)) 1053 return UNSUPPORTED; 1054 if ("as-text".equals(codeString)) 1055 return ASTEXT; 1056 if ("error".equals(codeString)) 1057 return ERROR; 1058 if ("not-a-number".equals(codeString)) 1059 return NOTANUMBER; 1060 if ("negative-infinity".equals(codeString)) 1061 return NEGATIVEINFINITY; 1062 if ("positive-infinity".equals(codeString)) 1063 return POSITIVEINFINITY; 1064 if ("not-performed".equals(codeString)) 1065 return NOTPERFORMED; 1066 if ("not-permitted".equals(codeString)) 1067 return NOTPERMITTED; 1068 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1069 } 1070 1071 public String toCode() { 1072 switch (this) { 1073 case UNKNOWN: 1074 return "unknown"; 1075 case ASKEDUNKNOWN: 1076 return "asked-unknown"; 1077 case TEMPUNKNOWN: 1078 return "temp-unknown"; 1079 case NOTASKED: 1080 return "not-asked"; 1081 case ASKEDDECLINED: 1082 return "asked-declined"; 1083 case MASKED: 1084 return "masked"; 1085 case NOTAPPLICABLE: 1086 return "not-applicable"; 1087 case UNSUPPORTED: 1088 return "unsupported"; 1089 case ASTEXT: 1090 return "as-text"; 1091 case ERROR: 1092 return "error"; 1093 case NOTANUMBER: 1094 return "not-a-number"; 1095 case NEGATIVEINFINITY: 1096 return "negative-infinity"; 1097 case POSITIVEINFINITY: 1098 return "positive-infinity"; 1099 case NOTPERFORMED: 1100 return "not-performed"; 1101 case NOTPERMITTED: 1102 return "not-permitted"; 1103 case NULL: 1104 return null; 1105 default: 1106 return "?"; 1107 } 1108 } 1109 1110 public String getSystem() { 1111 switch (this) { 1112 case UNKNOWN: 1113 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1114 case ASKEDUNKNOWN: 1115 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1116 case TEMPUNKNOWN: 1117 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1118 case NOTASKED: 1119 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1120 case ASKEDDECLINED: 1121 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1122 case MASKED: 1123 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1124 case NOTAPPLICABLE: 1125 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1126 case UNSUPPORTED: 1127 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1128 case ASTEXT: 1129 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1130 case ERROR: 1131 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1132 case NOTANUMBER: 1133 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1134 case NEGATIVEINFINITY: 1135 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1136 case POSITIVEINFINITY: 1137 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1138 case NOTPERFORMED: 1139 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1140 case NOTPERMITTED: 1141 return "http://terminology.hl7.org/CodeSystem/data-absent-reason"; 1142 case NULL: 1143 return null; 1144 default: 1145 return "?"; 1146 } 1147 } 1148 1149 public String getDefinition() { 1150 switch (this) { 1151 case UNKNOWN: 1152 return "The value is expected to exist but is not known."; 1153 case ASKEDUNKNOWN: 1154 return "The source was asked but does not know the value."; 1155 case TEMPUNKNOWN: 1156 return "There is reason to expect (from the workflow) that the value may become known."; 1157 case NOTASKED: 1158 return "The workflow didn't lead to this value being known."; 1159 case ASKEDDECLINED: 1160 return "The source was asked but declined to answer."; 1161 case MASKED: 1162 return "The information is not available due to security, privacy or related reasons."; 1163 case NOTAPPLICABLE: 1164 return "There is no proper value for this element (e.g. last menstrual period for a male)."; 1165 case UNSUPPORTED: 1166 return "The source system wasn't capable of supporting this element."; 1167 case ASTEXT: 1168 return "The content of the data is represented in the resource narrative."; 1169 case ERROR: 1170 return "Some system or workflow process error means that the information is not available."; 1171 case NOTANUMBER: 1172 return "The numeric value is undefined or unrepresentable due to a floating point processing error."; 1173 case NEGATIVEINFINITY: 1174 return "The numeric value is excessively low and unrepresentable due to a floating point processing error."; 1175 case POSITIVEINFINITY: 1176 return "The numeric value is excessively high and unrepresentable due to a floating point processing error."; 1177 case NOTPERFORMED: 1178 return "The value is not available because the observation procedure (test, etc.) was not performed."; 1179 case NOTPERMITTED: 1180 return "The value is not permitted in this context (e.g. due to profiles, or the base data types)."; 1181 case NULL: 1182 return null; 1183 default: 1184 return "?"; 1185 } 1186 } 1187 1188 public String getDisplay() { 1189 switch (this) { 1190 case UNKNOWN: 1191 return "Unknown"; 1192 case ASKEDUNKNOWN: 1193 return "Asked But Unknown"; 1194 case TEMPUNKNOWN: 1195 return "Temporarily Unknown"; 1196 case NOTASKED: 1197 return "Not Asked"; 1198 case ASKEDDECLINED: 1199 return "Asked But Declined"; 1200 case MASKED: 1201 return "Masked"; 1202 case NOTAPPLICABLE: 1203 return "Not Applicable"; 1204 case UNSUPPORTED: 1205 return "Unsupported"; 1206 case ASTEXT: 1207 return "As Text"; 1208 case ERROR: 1209 return "Error"; 1210 case NOTANUMBER: 1211 return "Not a Number (NaN)"; 1212 case NEGATIVEINFINITY: 1213 return "Negative Infinity (NINF)"; 1214 case POSITIVEINFINITY: 1215 return "Positive Infinity (PINF)"; 1216 case NOTPERFORMED: 1217 return "Not Performed"; 1218 case NOTPERMITTED: 1219 return "Not Permitted"; 1220 case NULL: 1221 return null; 1222 default: 1223 return "?"; 1224 } 1225 } 1226 } 1227 1228 public static class DataAbsentReasonEnumFactory implements EnumFactory<DataAbsentReason> { 1229 public DataAbsentReason fromCode(String codeString) throws IllegalArgumentException { 1230 if (codeString == null || "".equals(codeString)) 1231 if (codeString == null || "".equals(codeString)) 1232 return null; 1233 if ("unknown".equals(codeString)) 1234 return DataAbsentReason.UNKNOWN; 1235 if ("asked-unknown".equals(codeString)) 1236 return DataAbsentReason.ASKEDUNKNOWN; 1237 if ("temp-unknown".equals(codeString)) 1238 return DataAbsentReason.TEMPUNKNOWN; 1239 if ("not-asked".equals(codeString)) 1240 return DataAbsentReason.NOTASKED; 1241 if ("asked-declined".equals(codeString)) 1242 return DataAbsentReason.ASKEDDECLINED; 1243 if ("masked".equals(codeString)) 1244 return DataAbsentReason.MASKED; 1245 if ("not-applicable".equals(codeString)) 1246 return DataAbsentReason.NOTAPPLICABLE; 1247 if ("unsupported".equals(codeString)) 1248 return DataAbsentReason.UNSUPPORTED; 1249 if ("as-text".equals(codeString)) 1250 return DataAbsentReason.ASTEXT; 1251 if ("error".equals(codeString)) 1252 return DataAbsentReason.ERROR; 1253 if ("not-a-number".equals(codeString)) 1254 return DataAbsentReason.NOTANUMBER; 1255 if ("negative-infinity".equals(codeString)) 1256 return DataAbsentReason.NEGATIVEINFINITY; 1257 if ("positive-infinity".equals(codeString)) 1258 return DataAbsentReason.POSITIVEINFINITY; 1259 if ("not-performed".equals(codeString)) 1260 return DataAbsentReason.NOTPERFORMED; 1261 if ("not-permitted".equals(codeString)) 1262 return DataAbsentReason.NOTPERMITTED; 1263 throw new IllegalArgumentException("Unknown DataAbsentReason code '" + codeString + "'"); 1264 } 1265 1266 public Enumeration<DataAbsentReason> fromType(PrimitiveType<?> code) throws FHIRException { 1267 if (code == null) 1268 return null; 1269 if (code.isEmpty()) 1270 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NULL, code); 1271 String codeString = code.asStringValue(); 1272 if (codeString == null || "".equals(codeString)) 1273 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NULL, code); 1274 if ("unknown".equals(codeString)) 1275 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNKNOWN, code); 1276 if ("asked-unknown".equals(codeString)) 1277 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKEDUNKNOWN, code); 1278 if ("temp-unknown".equals(codeString)) 1279 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.TEMPUNKNOWN, code); 1280 if ("not-asked".equals(codeString)) 1281 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTASKED, code); 1282 if ("asked-declined".equals(codeString)) 1283 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKEDDECLINED, code); 1284 if ("masked".equals(codeString)) 1285 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.MASKED, code); 1286 if ("not-applicable".equals(codeString)) 1287 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTAPPLICABLE, code); 1288 if ("unsupported".equals(codeString)) 1289 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNSUPPORTED, code); 1290 if ("as-text".equals(codeString)) 1291 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASTEXT, code); 1292 if ("error".equals(codeString)) 1293 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ERROR, code); 1294 if ("not-a-number".equals(codeString)) 1295 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTANUMBER, code); 1296 if ("negative-infinity".equals(codeString)) 1297 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NEGATIVEINFINITY, code); 1298 if ("positive-infinity".equals(codeString)) 1299 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.POSITIVEINFINITY, code); 1300 if ("not-performed".equals(codeString)) 1301 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERFORMED, code); 1302 if ("not-permitted".equals(codeString)) 1303 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERMITTED, code); 1304 throw new FHIRException("Unknown DataAbsentReason code '" + codeString + "'"); 1305 } 1306 1307 public String toCode(DataAbsentReason code) { 1308 if (code == DataAbsentReason.UNKNOWN) 1309 return "unknown"; 1310 if (code == DataAbsentReason.ASKEDUNKNOWN) 1311 return "asked-unknown"; 1312 if (code == DataAbsentReason.TEMPUNKNOWN) 1313 return "temp-unknown"; 1314 if (code == DataAbsentReason.NOTASKED) 1315 return "not-asked"; 1316 if (code == DataAbsentReason.ASKEDDECLINED) 1317 return "asked-declined"; 1318 if (code == DataAbsentReason.MASKED) 1319 return "masked"; 1320 if (code == DataAbsentReason.NOTAPPLICABLE) 1321 return "not-applicable"; 1322 if (code == DataAbsentReason.UNSUPPORTED) 1323 return "unsupported"; 1324 if (code == DataAbsentReason.ASTEXT) 1325 return "as-text"; 1326 if (code == DataAbsentReason.ERROR) 1327 return "error"; 1328 if (code == DataAbsentReason.NOTANUMBER) 1329 return "not-a-number"; 1330 if (code == DataAbsentReason.NEGATIVEINFINITY) 1331 return "negative-infinity"; 1332 if (code == DataAbsentReason.POSITIVEINFINITY) 1333 return "positive-infinity"; 1334 if (code == DataAbsentReason.NOTPERFORMED) 1335 return "not-performed"; 1336 if (code == DataAbsentReason.NOTPERMITTED) 1337 return "not-permitted"; 1338 return "?"; 1339 } 1340 1341 public String toSystem(DataAbsentReason code) { 1342 return code.getSystem(); 1343 } 1344 } 1345 1346 public enum DataType { 1347 /** 1348 * An address expressed using postal conventions (as opposed to GPS or other 1349 * location definition formats). This data type may be used to convey addresses 1350 * for use in delivering mail as well as for visiting locations which might not 1351 * be valid for mail delivery. There are a variety of postal address formats 1352 * defined around the world. 1353 */ 1354 ADDRESS, 1355 /** 1356 * A duration of time during which an organism (or a process) has existed. 1357 */ 1358 AGE, 1359 /** 1360 * A text note which also contains information about who made the statement and 1361 * when. 1362 */ 1363 ANNOTATION, 1364 /** 1365 * For referring to data content defined in other formats. 1366 */ 1367 ATTACHMENT, 1368 /** 1369 * Base definition for all elements that are defined inside a resource - but not 1370 * those in a data type. 1371 */ 1372 BACKBONEELEMENT, 1373 /** 1374 * A concept that may be defined by a formal reference to a terminology or 1375 * ontology or may be provided by text. 1376 */ 1377 CODEABLECONCEPT, 1378 /** 1379 * A reference to a code defined by a terminology system. 1380 */ 1381 CODING, 1382 /** 1383 * Specifies contact information for a person or organization. 1384 */ 1385 CONTACTDETAIL, 1386 /** 1387 * Details for all kinds of technology mediated contact points for a person or 1388 * organization, including telephone, email, etc. 1389 */ 1390 CONTACTPOINT, 1391 /** 1392 * A contributor to the content of a knowledge asset, including authors, 1393 * editors, reviewers, and endorsers. 1394 */ 1395 CONTRIBUTOR, 1396 /** 1397 * A measured amount (or an amount that can potentially be measured). Note that 1398 * measured amounts include amounts that are not precisely quantified, including 1399 * amounts involving arbitrary units and floating currencies. 1400 */ 1401 COUNT, 1402 /** 1403 * Describes a required data item for evaluation in terms of the type of data, 1404 * and optional code or date-based filters of the data. 1405 */ 1406 DATAREQUIREMENT, 1407 /** 1408 * A length - a value with a unit that is a physical distance. 1409 */ 1410 DISTANCE, 1411 /** 1412 * Indicates how the medication is/was taken or should be taken by the patient. 1413 */ 1414 DOSAGE, 1415 /** 1416 * A length of time. 1417 */ 1418 DURATION, 1419 /** 1420 * Base definition for all elements in a resource. 1421 */ 1422 ELEMENT, 1423 /** 1424 * Captures constraints on each element within the resource, profile, or 1425 * extension. 1426 */ 1427 ELEMENTDEFINITION, 1428 /** 1429 * A expression that is evaluated in a specified context and returns a value. 1430 * The context of use of the expression must specify the context in which the 1431 * expression is evaluated, and how the result of the expression is used. 1432 */ 1433 EXPRESSION, 1434 /** 1435 * Optional Extension Element - found in all resources. 1436 */ 1437 EXTENSION, 1438 /** 1439 * A human's name with the ability to identify parts and usage. 1440 */ 1441 HUMANNAME, 1442 /** 1443 * An identifier - identifies some entity uniquely and unambiguously. Typically 1444 * this is used for business identifiers. 1445 */ 1446 IDENTIFIER, 1447 /** 1448 * The marketing status describes the date when a medicinal product is actually 1449 * put on the market or the date as of which it is no longer available. 1450 */ 1451 MARKETINGSTATUS, 1452 /** 1453 * The metadata about a resource. This is content in the resource that is 1454 * maintained by the infrastructure. Changes to the content might not always be 1455 * associated with version changes to the resource. 1456 */ 1457 META, 1458 /** 1459 * An amount of economic utility in some recognized currency. 1460 */ 1461 MONEY, 1462 /** 1463 * null 1464 */ 1465 MONEYQUANTITY, 1466 /** 1467 * A human-readable summary of the resource conveying the essential clinical and 1468 * business information for the resource. 1469 */ 1470 NARRATIVE, 1471 /** 1472 * The parameters to the module. This collection specifies both the input and 1473 * output parameters. Input parameters are provided by the caller as part of the 1474 * $evaluate operation. Output parameters are included in the GuidanceResponse. 1475 */ 1476 PARAMETERDEFINITION, 1477 /** 1478 * A time period defined by a start and end date and optionally time. 1479 */ 1480 PERIOD, 1481 /** 1482 * A populatioof people with some set of grouping criteria. 1483 */ 1484 POPULATION, 1485 /** 1486 * The marketing status describes the date when a medicinal product is actually 1487 * put on the market or the date as of which it is no longer available. 1488 */ 1489 PRODCHARACTERISTIC, 1490 /** 1491 * The shelf-life and storage information for a medicinal product item or 1492 * container can be described using this class. 1493 */ 1494 PRODUCTSHELFLIFE, 1495 /** 1496 * A measured amount (or an amount that can potentially be measured). Note that 1497 * measured amounts include amounts that are not precisely quantified, including 1498 * amounts involving arbitrary units and floating currencies. 1499 */ 1500 QUANTITY, 1501 /** 1502 * A set of ordered Quantities defined by a low and high limit. 1503 */ 1504 RANGE, 1505 /** 1506 * A relationship of two Quantity values - expressed as a numerator and a 1507 * denominator. 1508 */ 1509 RATIO, 1510 /** 1511 * A reference from one resource to another. 1512 */ 1513 REFERENCE, 1514 /** 1515 * Related artifacts such as additional documentation, justification, or 1516 * bibliographic references. 1517 */ 1518 RELATEDARTIFACT, 1519 /** 1520 * A series of measurements taken by a device, with upper and lower limits. 1521 * There may be more than one dimension in the data. 1522 */ 1523 SAMPLEDDATA, 1524 /** 1525 * A signature along with supporting context. The signature may be a digital 1526 * signature that is cryptographic in nature, or some other signature acceptable 1527 * to the domain. This other signature may be as simple as a graphical image 1528 * representing a hand-written signature, or a signature ceremony Different 1529 * signature approaches have different utilities. 1530 */ 1531 SIGNATURE, 1532 /** 1533 * null 1534 */ 1535 SIMPLEQUANTITY, 1536 /** 1537 * Chemical substances are a single substance type whose primary defining 1538 * element is the molecular structure. Chemical substances shall be defined on 1539 * the basis of their complete covalent molecular structure; the presence of a 1540 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 1541 * Purity, grade, physical form or particle size are not taken into account in 1542 * the definition of a chemical substance or in the assignment of a Substance 1543 * ID. 1544 */ 1545 SUBSTANCEAMOUNT, 1546 /** 1547 * Specifies an event that may occur multiple times. Timing schedules are used 1548 * to record when things are planned, expected or requested to occur. The most 1549 * common usage is in dosage instructions for medications. They are also used 1550 * when planning care of various kinds, and may be used for reporting the 1551 * schedule to which past regular activities were carried out. 1552 */ 1553 TIMING, 1554 /** 1555 * A description of a triggering event. Triggering events can be named events, 1556 * data events, or periodic, as determined by the type element. 1557 */ 1558 TRIGGERDEFINITION, 1559 /** 1560 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 1561 * and/or categorize an artifact. This metadata can either be specific to the 1562 * applicable population (e.g., age category, DRG) or the specific context of 1563 * care (e.g., venue, care setting, provider of care). 1564 */ 1565 USAGECONTEXT, 1566 /** 1567 * A stream of bytes 1568 */ 1569 BASE64BINARY, 1570 /** 1571 * Value of "true" or "false" 1572 */ 1573 BOOLEAN, 1574 /** 1575 * A URI that is a reference to a canonical URL on a FHIR resource 1576 */ 1577 CANONICAL, 1578 /** 1579 * A string which has at least one character and no leading or trailing 1580 * whitespace and where there is no whitespace other than single spaces in the 1581 * contents 1582 */ 1583 CODE, 1584 /** 1585 * A date or partial date (e.g. just year or year + month). There is no time 1586 * zone. The format is a union of the schema types gYear, gYearMonth and date. 1587 * Dates SHALL be valid dates. 1588 */ 1589 DATE, 1590 /** 1591 * A date, date-time or partial date (e.g. just year or year + month). If hours 1592 * and minutes are specified, a time zone SHALL be populated. The format is a 1593 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 1594 * be provided due to schema type constraints but may be zero-filled and may be 1595 * ignored. Dates SHALL be valid dates. 1596 */ 1597 DATETIME, 1598 /** 1599 * A rational number with implicit precision 1600 */ 1601 DECIMAL, 1602 /** 1603 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 1604 * characters. (This might be an integer, an unprefixed OID, UUID or any other 1605 * identifier pattern that meets these constraints.) Ids are case-insensitive. 1606 */ 1607 ID, 1608 /** 1609 * An instant in time - known at least to the second 1610 */ 1611 INSTANT, 1612 /** 1613 * A whole number 1614 */ 1615 INTEGER, 1616 /** 1617 * A string that may contain Github Flavored Markdown syntax for optional 1618 * processing by a mark down presentation engine 1619 */ 1620 MARKDOWN, 1621 /** 1622 * An OID represented as a URI 1623 */ 1624 OID, 1625 /** 1626 * An integer with a value that is positive (e.g. >0) 1627 */ 1628 POSITIVEINT, 1629 /** 1630 * A sequence of Unicode characters 1631 */ 1632 STRING, 1633 /** 1634 * A time during the day, with no date specified 1635 */ 1636 TIME, 1637 /** 1638 * An integer with a value that is not negative (e.g. >= 0) 1639 */ 1640 UNSIGNEDINT, 1641 /** 1642 * String of characters used to identify a name or a resource 1643 */ 1644 URI, 1645 /** 1646 * A URI that is a literal reference 1647 */ 1648 URL, 1649 /** 1650 * A UUID, represented as a URI 1651 */ 1652 UUID, 1653 /** 1654 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 1655 * content) 1656 */ 1657 XHTML, 1658 /** 1659 * added to help the parsers 1660 */ 1661 NULL; 1662 1663 public static DataType fromCode(String codeString) throws FHIRException { 1664 if (codeString == null || "".equals(codeString)) 1665 return null; 1666 if ("Address".equals(codeString)) 1667 return ADDRESS; 1668 if ("Age".equals(codeString)) 1669 return AGE; 1670 if ("Annotation".equals(codeString)) 1671 return ANNOTATION; 1672 if ("Attachment".equals(codeString)) 1673 return ATTACHMENT; 1674 if ("BackboneElement".equals(codeString)) 1675 return BACKBONEELEMENT; 1676 if ("CodeableConcept".equals(codeString)) 1677 return CODEABLECONCEPT; 1678 if ("Coding".equals(codeString)) 1679 return CODING; 1680 if ("ContactDetail".equals(codeString)) 1681 return CONTACTDETAIL; 1682 if ("ContactPoint".equals(codeString)) 1683 return CONTACTPOINT; 1684 if ("Contributor".equals(codeString)) 1685 return CONTRIBUTOR; 1686 if ("Count".equals(codeString)) 1687 return COUNT; 1688 if ("DataRequirement".equals(codeString)) 1689 return DATAREQUIREMENT; 1690 if ("Distance".equals(codeString)) 1691 return DISTANCE; 1692 if ("Dosage".equals(codeString)) 1693 return DOSAGE; 1694 if ("Duration".equals(codeString)) 1695 return DURATION; 1696 if ("Element".equals(codeString)) 1697 return ELEMENT; 1698 if ("ElementDefinition".equals(codeString)) 1699 return ELEMENTDEFINITION; 1700 if ("Expression".equals(codeString)) 1701 return EXPRESSION; 1702 if ("Extension".equals(codeString)) 1703 return EXTENSION; 1704 if ("HumanName".equals(codeString)) 1705 return HUMANNAME; 1706 if ("Identifier".equals(codeString)) 1707 return IDENTIFIER; 1708 if ("MarketingStatus".equals(codeString)) 1709 return MARKETINGSTATUS; 1710 if ("Meta".equals(codeString)) 1711 return META; 1712 if ("Money".equals(codeString)) 1713 return MONEY; 1714 if ("MoneyQuantity".equals(codeString)) 1715 return MONEYQUANTITY; 1716 if ("Narrative".equals(codeString)) 1717 return NARRATIVE; 1718 if ("ParameterDefinition".equals(codeString)) 1719 return PARAMETERDEFINITION; 1720 if ("Period".equals(codeString)) 1721 return PERIOD; 1722 if ("Population".equals(codeString)) 1723 return POPULATION; 1724 if ("ProdCharacteristic".equals(codeString)) 1725 return PRODCHARACTERISTIC; 1726 if ("ProductShelfLife".equals(codeString)) 1727 return PRODUCTSHELFLIFE; 1728 if ("Quantity".equals(codeString)) 1729 return QUANTITY; 1730 if ("Range".equals(codeString)) 1731 return RANGE; 1732 if ("Ratio".equals(codeString)) 1733 return RATIO; 1734 if ("Reference".equals(codeString)) 1735 return REFERENCE; 1736 if ("RelatedArtifact".equals(codeString)) 1737 return RELATEDARTIFACT; 1738 if ("SampledData".equals(codeString)) 1739 return SAMPLEDDATA; 1740 if ("Signature".equals(codeString)) 1741 return SIGNATURE; 1742 if ("SimpleQuantity".equals(codeString)) 1743 return SIMPLEQUANTITY; 1744 if ("SubstanceAmount".equals(codeString)) 1745 return SUBSTANCEAMOUNT; 1746 if ("Timing".equals(codeString)) 1747 return TIMING; 1748 if ("TriggerDefinition".equals(codeString)) 1749 return TRIGGERDEFINITION; 1750 if ("UsageContext".equals(codeString)) 1751 return USAGECONTEXT; 1752 if ("base64Binary".equals(codeString)) 1753 return BASE64BINARY; 1754 if ("boolean".equals(codeString)) 1755 return BOOLEAN; 1756 if ("canonical".equals(codeString)) 1757 return CANONICAL; 1758 if ("code".equals(codeString)) 1759 return CODE; 1760 if ("date".equals(codeString)) 1761 return DATE; 1762 if ("dateTime".equals(codeString)) 1763 return DATETIME; 1764 if ("decimal".equals(codeString)) 1765 return DECIMAL; 1766 if ("id".equals(codeString)) 1767 return ID; 1768 if ("instant".equals(codeString)) 1769 return INSTANT; 1770 if ("integer".equals(codeString)) 1771 return INTEGER; 1772 if ("markdown".equals(codeString)) 1773 return MARKDOWN; 1774 if ("oid".equals(codeString)) 1775 return OID; 1776 if ("positiveInt".equals(codeString)) 1777 return POSITIVEINT; 1778 if ("string".equals(codeString)) 1779 return STRING; 1780 if ("time".equals(codeString)) 1781 return TIME; 1782 if ("unsignedInt".equals(codeString)) 1783 return UNSIGNEDINT; 1784 if ("uri".equals(codeString)) 1785 return URI; 1786 if ("url".equals(codeString)) 1787 return URL; 1788 if ("uuid".equals(codeString)) 1789 return UUID; 1790 if ("xhtml".equals(codeString)) 1791 return XHTML; 1792 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 1793 } 1794 1795 public String toCode() { 1796 switch (this) { 1797 case ADDRESS: 1798 return "Address"; 1799 case AGE: 1800 return "Age"; 1801 case ANNOTATION: 1802 return "Annotation"; 1803 case ATTACHMENT: 1804 return "Attachment"; 1805 case BACKBONEELEMENT: 1806 return "BackboneElement"; 1807 case CODEABLECONCEPT: 1808 return "CodeableConcept"; 1809 case CODING: 1810 return "Coding"; 1811 case CONTACTDETAIL: 1812 return "ContactDetail"; 1813 case CONTACTPOINT: 1814 return "ContactPoint"; 1815 case CONTRIBUTOR: 1816 return "Contributor"; 1817 case COUNT: 1818 return "Count"; 1819 case DATAREQUIREMENT: 1820 return "DataRequirement"; 1821 case DISTANCE: 1822 return "Distance"; 1823 case DOSAGE: 1824 return "Dosage"; 1825 case DURATION: 1826 return "Duration"; 1827 case ELEMENT: 1828 return "Element"; 1829 case ELEMENTDEFINITION: 1830 return "ElementDefinition"; 1831 case EXPRESSION: 1832 return "Expression"; 1833 case EXTENSION: 1834 return "Extension"; 1835 case HUMANNAME: 1836 return "HumanName"; 1837 case IDENTIFIER: 1838 return "Identifier"; 1839 case MARKETINGSTATUS: 1840 return "MarketingStatus"; 1841 case META: 1842 return "Meta"; 1843 case MONEY: 1844 return "Money"; 1845 case MONEYQUANTITY: 1846 return "MoneyQuantity"; 1847 case NARRATIVE: 1848 return "Narrative"; 1849 case PARAMETERDEFINITION: 1850 return "ParameterDefinition"; 1851 case PERIOD: 1852 return "Period"; 1853 case POPULATION: 1854 return "Population"; 1855 case PRODCHARACTERISTIC: 1856 return "ProdCharacteristic"; 1857 case PRODUCTSHELFLIFE: 1858 return "ProductShelfLife"; 1859 case QUANTITY: 1860 return "Quantity"; 1861 case RANGE: 1862 return "Range"; 1863 case RATIO: 1864 return "Ratio"; 1865 case REFERENCE: 1866 return "Reference"; 1867 case RELATEDARTIFACT: 1868 return "RelatedArtifact"; 1869 case SAMPLEDDATA: 1870 return "SampledData"; 1871 case SIGNATURE: 1872 return "Signature"; 1873 case SIMPLEQUANTITY: 1874 return "SimpleQuantity"; 1875 case SUBSTANCEAMOUNT: 1876 return "SubstanceAmount"; 1877 case TIMING: 1878 return "Timing"; 1879 case TRIGGERDEFINITION: 1880 return "TriggerDefinition"; 1881 case USAGECONTEXT: 1882 return "UsageContext"; 1883 case BASE64BINARY: 1884 return "base64Binary"; 1885 case BOOLEAN: 1886 return "boolean"; 1887 case CANONICAL: 1888 return "canonical"; 1889 case CODE: 1890 return "code"; 1891 case DATE: 1892 return "date"; 1893 case DATETIME: 1894 return "dateTime"; 1895 case DECIMAL: 1896 return "decimal"; 1897 case ID: 1898 return "id"; 1899 case INSTANT: 1900 return "instant"; 1901 case INTEGER: 1902 return "integer"; 1903 case MARKDOWN: 1904 return "markdown"; 1905 case OID: 1906 return "oid"; 1907 case POSITIVEINT: 1908 return "positiveInt"; 1909 case STRING: 1910 return "string"; 1911 case TIME: 1912 return "time"; 1913 case UNSIGNEDINT: 1914 return "unsignedInt"; 1915 case URI: 1916 return "uri"; 1917 case URL: 1918 return "url"; 1919 case UUID: 1920 return "uuid"; 1921 case XHTML: 1922 return "xhtml"; 1923 case NULL: 1924 return null; 1925 default: 1926 return "?"; 1927 } 1928 } 1929 1930 public String getSystem() { 1931 switch (this) { 1932 case ADDRESS: 1933 return "http://hl7.org/fhir/data-types"; 1934 case AGE: 1935 return "http://hl7.org/fhir/data-types"; 1936 case ANNOTATION: 1937 return "http://hl7.org/fhir/data-types"; 1938 case ATTACHMENT: 1939 return "http://hl7.org/fhir/data-types"; 1940 case BACKBONEELEMENT: 1941 return "http://hl7.org/fhir/data-types"; 1942 case CODEABLECONCEPT: 1943 return "http://hl7.org/fhir/data-types"; 1944 case CODING: 1945 return "http://hl7.org/fhir/data-types"; 1946 case CONTACTDETAIL: 1947 return "http://hl7.org/fhir/data-types"; 1948 case CONTACTPOINT: 1949 return "http://hl7.org/fhir/data-types"; 1950 case CONTRIBUTOR: 1951 return "http://hl7.org/fhir/data-types"; 1952 case COUNT: 1953 return "http://hl7.org/fhir/data-types"; 1954 case DATAREQUIREMENT: 1955 return "http://hl7.org/fhir/data-types"; 1956 case DISTANCE: 1957 return "http://hl7.org/fhir/data-types"; 1958 case DOSAGE: 1959 return "http://hl7.org/fhir/data-types"; 1960 case DURATION: 1961 return "http://hl7.org/fhir/data-types"; 1962 case ELEMENT: 1963 return "http://hl7.org/fhir/data-types"; 1964 case ELEMENTDEFINITION: 1965 return "http://hl7.org/fhir/data-types"; 1966 case EXPRESSION: 1967 return "http://hl7.org/fhir/data-types"; 1968 case EXTENSION: 1969 return "http://hl7.org/fhir/data-types"; 1970 case HUMANNAME: 1971 return "http://hl7.org/fhir/data-types"; 1972 case IDENTIFIER: 1973 return "http://hl7.org/fhir/data-types"; 1974 case MARKETINGSTATUS: 1975 return "http://hl7.org/fhir/data-types"; 1976 case META: 1977 return "http://hl7.org/fhir/data-types"; 1978 case MONEY: 1979 return "http://hl7.org/fhir/data-types"; 1980 case MONEYQUANTITY: 1981 return "http://hl7.org/fhir/data-types"; 1982 case NARRATIVE: 1983 return "http://hl7.org/fhir/data-types"; 1984 case PARAMETERDEFINITION: 1985 return "http://hl7.org/fhir/data-types"; 1986 case PERIOD: 1987 return "http://hl7.org/fhir/data-types"; 1988 case POPULATION: 1989 return "http://hl7.org/fhir/data-types"; 1990 case PRODCHARACTERISTIC: 1991 return "http://hl7.org/fhir/data-types"; 1992 case PRODUCTSHELFLIFE: 1993 return "http://hl7.org/fhir/data-types"; 1994 case QUANTITY: 1995 return "http://hl7.org/fhir/data-types"; 1996 case RANGE: 1997 return "http://hl7.org/fhir/data-types"; 1998 case RATIO: 1999 return "http://hl7.org/fhir/data-types"; 2000 case REFERENCE: 2001 return "http://hl7.org/fhir/data-types"; 2002 case RELATEDARTIFACT: 2003 return "http://hl7.org/fhir/data-types"; 2004 case SAMPLEDDATA: 2005 return "http://hl7.org/fhir/data-types"; 2006 case SIGNATURE: 2007 return "http://hl7.org/fhir/data-types"; 2008 case SIMPLEQUANTITY: 2009 return "http://hl7.org/fhir/data-types"; 2010 case SUBSTANCEAMOUNT: 2011 return "http://hl7.org/fhir/data-types"; 2012 case TIMING: 2013 return "http://hl7.org/fhir/data-types"; 2014 case TRIGGERDEFINITION: 2015 return "http://hl7.org/fhir/data-types"; 2016 case USAGECONTEXT: 2017 return "http://hl7.org/fhir/data-types"; 2018 case BASE64BINARY: 2019 return "http://hl7.org/fhir/data-types"; 2020 case BOOLEAN: 2021 return "http://hl7.org/fhir/data-types"; 2022 case CANONICAL: 2023 return "http://hl7.org/fhir/data-types"; 2024 case CODE: 2025 return "http://hl7.org/fhir/data-types"; 2026 case DATE: 2027 return "http://hl7.org/fhir/data-types"; 2028 case DATETIME: 2029 return "http://hl7.org/fhir/data-types"; 2030 case DECIMAL: 2031 return "http://hl7.org/fhir/data-types"; 2032 case ID: 2033 return "http://hl7.org/fhir/data-types"; 2034 case INSTANT: 2035 return "http://hl7.org/fhir/data-types"; 2036 case INTEGER: 2037 return "http://hl7.org/fhir/data-types"; 2038 case MARKDOWN: 2039 return "http://hl7.org/fhir/data-types"; 2040 case OID: 2041 return "http://hl7.org/fhir/data-types"; 2042 case POSITIVEINT: 2043 return "http://hl7.org/fhir/data-types"; 2044 case STRING: 2045 return "http://hl7.org/fhir/data-types"; 2046 case TIME: 2047 return "http://hl7.org/fhir/data-types"; 2048 case UNSIGNEDINT: 2049 return "http://hl7.org/fhir/data-types"; 2050 case URI: 2051 return "http://hl7.org/fhir/data-types"; 2052 case URL: 2053 return "http://hl7.org/fhir/data-types"; 2054 case UUID: 2055 return "http://hl7.org/fhir/data-types"; 2056 case XHTML: 2057 return "http://hl7.org/fhir/data-types"; 2058 case NULL: 2059 return null; 2060 default: 2061 return "?"; 2062 } 2063 } 2064 2065 public String getDefinition() { 2066 switch (this) { 2067 case ADDRESS: 2068 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 2069 case AGE: 2070 return "A duration of time during which an organism (or a process) has existed."; 2071 case ANNOTATION: 2072 return "A text note which also contains information about who made the statement and when."; 2073 case ATTACHMENT: 2074 return "For referring to data content defined in other formats."; 2075 case BACKBONEELEMENT: 2076 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 2077 case CODEABLECONCEPT: 2078 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 2079 case CODING: 2080 return "A reference to a code defined by a terminology system."; 2081 case CONTACTDETAIL: 2082 return "Specifies contact information for a person or organization."; 2083 case CONTACTPOINT: 2084 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 2085 case CONTRIBUTOR: 2086 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 2087 case COUNT: 2088 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 2089 case DATAREQUIREMENT: 2090 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 2091 case DISTANCE: 2092 return "A length - a value with a unit that is a physical distance."; 2093 case DOSAGE: 2094 return "Indicates how the medication is/was taken or should be taken by the patient."; 2095 case DURATION: 2096 return "A length of time."; 2097 case ELEMENT: 2098 return "Base definition for all elements in a resource."; 2099 case ELEMENTDEFINITION: 2100 return "Captures constraints on each element within the resource, profile, or extension."; 2101 case EXPRESSION: 2102 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 2103 case EXTENSION: 2104 return "Optional Extension Element - found in all resources."; 2105 case HUMANNAME: 2106 return "A human's name with the ability to identify parts and usage."; 2107 case IDENTIFIER: 2108 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 2109 case MARKETINGSTATUS: 2110 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 2111 case META: 2112 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 2113 case MONEY: 2114 return "An amount of economic utility in some recognized currency."; 2115 case MONEYQUANTITY: 2116 return ""; 2117 case NARRATIVE: 2118 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 2119 case PARAMETERDEFINITION: 2120 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 2121 case PERIOD: 2122 return "A time period defined by a start and end date and optionally time."; 2123 case POPULATION: 2124 return "A populatioof people with some set of grouping criteria."; 2125 case PRODCHARACTERISTIC: 2126 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 2127 case PRODUCTSHELFLIFE: 2128 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 2129 case QUANTITY: 2130 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 2131 case RANGE: 2132 return "A set of ordered Quantities defined by a low and high limit."; 2133 case RATIO: 2134 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 2135 case REFERENCE: 2136 return "A reference from one resource to another."; 2137 case RELATEDARTIFACT: 2138 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 2139 case SAMPLEDDATA: 2140 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 2141 case SIGNATURE: 2142 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 2143 case SIMPLEQUANTITY: 2144 return ""; 2145 case SUBSTANCEAMOUNT: 2146 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 2147 case TIMING: 2148 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 2149 case TRIGGERDEFINITION: 2150 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 2151 case USAGECONTEXT: 2152 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 2153 case BASE64BINARY: 2154 return "A stream of bytes"; 2155 case BOOLEAN: 2156 return "Value of \"true\" or \"false\""; 2157 case CANONICAL: 2158 return "A URI that is a reference to a canonical URL on a FHIR resource"; 2159 case CODE: 2160 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 2161 case DATE: 2162 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 2163 case DATETIME: 2164 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 2165 case DECIMAL: 2166 return "A rational number with implicit precision"; 2167 case ID: 2168 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 2169 case INSTANT: 2170 return "An instant in time - known at least to the second"; 2171 case INTEGER: 2172 return "A whole number"; 2173 case MARKDOWN: 2174 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 2175 case OID: 2176 return "An OID represented as a URI"; 2177 case POSITIVEINT: 2178 return "An integer with a value that is positive (e.g. >0)"; 2179 case STRING: 2180 return "A sequence of Unicode characters"; 2181 case TIME: 2182 return "A time during the day, with no date specified"; 2183 case UNSIGNEDINT: 2184 return "An integer with a value that is not negative (e.g. >= 0)"; 2185 case URI: 2186 return "String of characters used to identify a name or a resource"; 2187 case URL: 2188 return "A URI that is a literal reference"; 2189 case UUID: 2190 return "A UUID, represented as a URI"; 2191 case XHTML: 2192 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 2193 case NULL: 2194 return null; 2195 default: 2196 return "?"; 2197 } 2198 } 2199 2200 public String getDisplay() { 2201 switch (this) { 2202 case ADDRESS: 2203 return "Address"; 2204 case AGE: 2205 return "Age"; 2206 case ANNOTATION: 2207 return "Annotation"; 2208 case ATTACHMENT: 2209 return "Attachment"; 2210 case BACKBONEELEMENT: 2211 return "BackboneElement"; 2212 case CODEABLECONCEPT: 2213 return "CodeableConcept"; 2214 case CODING: 2215 return "Coding"; 2216 case CONTACTDETAIL: 2217 return "ContactDetail"; 2218 case CONTACTPOINT: 2219 return "ContactPoint"; 2220 case CONTRIBUTOR: 2221 return "Contributor"; 2222 case COUNT: 2223 return "Count"; 2224 case DATAREQUIREMENT: 2225 return "DataRequirement"; 2226 case DISTANCE: 2227 return "Distance"; 2228 case DOSAGE: 2229 return "Dosage"; 2230 case DURATION: 2231 return "Duration"; 2232 case ELEMENT: 2233 return "Element"; 2234 case ELEMENTDEFINITION: 2235 return "ElementDefinition"; 2236 case EXPRESSION: 2237 return "Expression"; 2238 case EXTENSION: 2239 return "Extension"; 2240 case HUMANNAME: 2241 return "HumanName"; 2242 case IDENTIFIER: 2243 return "Identifier"; 2244 case MARKETINGSTATUS: 2245 return "MarketingStatus"; 2246 case META: 2247 return "Meta"; 2248 case MONEY: 2249 return "Money"; 2250 case MONEYQUANTITY: 2251 return "MoneyQuantity"; 2252 case NARRATIVE: 2253 return "Narrative"; 2254 case PARAMETERDEFINITION: 2255 return "ParameterDefinition"; 2256 case PERIOD: 2257 return "Period"; 2258 case POPULATION: 2259 return "Population"; 2260 case PRODCHARACTERISTIC: 2261 return "ProdCharacteristic"; 2262 case PRODUCTSHELFLIFE: 2263 return "ProductShelfLife"; 2264 case QUANTITY: 2265 return "Quantity"; 2266 case RANGE: 2267 return "Range"; 2268 case RATIO: 2269 return "Ratio"; 2270 case REFERENCE: 2271 return "Reference"; 2272 case RELATEDARTIFACT: 2273 return "RelatedArtifact"; 2274 case SAMPLEDDATA: 2275 return "SampledData"; 2276 case SIGNATURE: 2277 return "Signature"; 2278 case SIMPLEQUANTITY: 2279 return "SimpleQuantity"; 2280 case SUBSTANCEAMOUNT: 2281 return "SubstanceAmount"; 2282 case TIMING: 2283 return "Timing"; 2284 case TRIGGERDEFINITION: 2285 return "TriggerDefinition"; 2286 case USAGECONTEXT: 2287 return "UsageContext"; 2288 case BASE64BINARY: 2289 return "base64Binary"; 2290 case BOOLEAN: 2291 return "boolean"; 2292 case CANONICAL: 2293 return "canonical"; 2294 case CODE: 2295 return "code"; 2296 case DATE: 2297 return "date"; 2298 case DATETIME: 2299 return "dateTime"; 2300 case DECIMAL: 2301 return "decimal"; 2302 case ID: 2303 return "id"; 2304 case INSTANT: 2305 return "instant"; 2306 case INTEGER: 2307 return "integer"; 2308 case MARKDOWN: 2309 return "markdown"; 2310 case OID: 2311 return "oid"; 2312 case POSITIVEINT: 2313 return "positiveInt"; 2314 case STRING: 2315 return "string"; 2316 case TIME: 2317 return "time"; 2318 case UNSIGNEDINT: 2319 return "unsignedInt"; 2320 case URI: 2321 return "uri"; 2322 case URL: 2323 return "url"; 2324 case UUID: 2325 return "uuid"; 2326 case XHTML: 2327 return "XHTML"; 2328 case NULL: 2329 return null; 2330 default: 2331 return "?"; 2332 } 2333 } 2334 } 2335 2336 public static class DataTypeEnumFactory implements EnumFactory<DataType> { 2337 public DataType fromCode(String codeString) throws IllegalArgumentException { 2338 if (codeString == null || "".equals(codeString)) 2339 if (codeString == null || "".equals(codeString)) 2340 return null; 2341 if ("Address".equals(codeString)) 2342 return DataType.ADDRESS; 2343 if ("Age".equals(codeString)) 2344 return DataType.AGE; 2345 if ("Annotation".equals(codeString)) 2346 return DataType.ANNOTATION; 2347 if ("Attachment".equals(codeString)) 2348 return DataType.ATTACHMENT; 2349 if ("BackboneElement".equals(codeString)) 2350 return DataType.BACKBONEELEMENT; 2351 if ("CodeableConcept".equals(codeString)) 2352 return DataType.CODEABLECONCEPT; 2353 if ("Coding".equals(codeString)) 2354 return DataType.CODING; 2355 if ("ContactDetail".equals(codeString)) 2356 return DataType.CONTACTDETAIL; 2357 if ("ContactPoint".equals(codeString)) 2358 return DataType.CONTACTPOINT; 2359 if ("Contributor".equals(codeString)) 2360 return DataType.CONTRIBUTOR; 2361 if ("Count".equals(codeString)) 2362 return DataType.COUNT; 2363 if ("DataRequirement".equals(codeString)) 2364 return DataType.DATAREQUIREMENT; 2365 if ("Distance".equals(codeString)) 2366 return DataType.DISTANCE; 2367 if ("Dosage".equals(codeString)) 2368 return DataType.DOSAGE; 2369 if ("Duration".equals(codeString)) 2370 return DataType.DURATION; 2371 if ("Element".equals(codeString)) 2372 return DataType.ELEMENT; 2373 if ("ElementDefinition".equals(codeString)) 2374 return DataType.ELEMENTDEFINITION; 2375 if ("Expression".equals(codeString)) 2376 return DataType.EXPRESSION; 2377 if ("Extension".equals(codeString)) 2378 return DataType.EXTENSION; 2379 if ("HumanName".equals(codeString)) 2380 return DataType.HUMANNAME; 2381 if ("Identifier".equals(codeString)) 2382 return DataType.IDENTIFIER; 2383 if ("MarketingStatus".equals(codeString)) 2384 return DataType.MARKETINGSTATUS; 2385 if ("Meta".equals(codeString)) 2386 return DataType.META; 2387 if ("Money".equals(codeString)) 2388 return DataType.MONEY; 2389 if ("MoneyQuantity".equals(codeString)) 2390 return DataType.MONEYQUANTITY; 2391 if ("Narrative".equals(codeString)) 2392 return DataType.NARRATIVE; 2393 if ("ParameterDefinition".equals(codeString)) 2394 return DataType.PARAMETERDEFINITION; 2395 if ("Period".equals(codeString)) 2396 return DataType.PERIOD; 2397 if ("Population".equals(codeString)) 2398 return DataType.POPULATION; 2399 if ("ProdCharacteristic".equals(codeString)) 2400 return DataType.PRODCHARACTERISTIC; 2401 if ("ProductShelfLife".equals(codeString)) 2402 return DataType.PRODUCTSHELFLIFE; 2403 if ("Quantity".equals(codeString)) 2404 return DataType.QUANTITY; 2405 if ("Range".equals(codeString)) 2406 return DataType.RANGE; 2407 if ("Ratio".equals(codeString)) 2408 return DataType.RATIO; 2409 if ("Reference".equals(codeString)) 2410 return DataType.REFERENCE; 2411 if ("RelatedArtifact".equals(codeString)) 2412 return DataType.RELATEDARTIFACT; 2413 if ("SampledData".equals(codeString)) 2414 return DataType.SAMPLEDDATA; 2415 if ("Signature".equals(codeString)) 2416 return DataType.SIGNATURE; 2417 if ("SimpleQuantity".equals(codeString)) 2418 return DataType.SIMPLEQUANTITY; 2419 if ("SubstanceAmount".equals(codeString)) 2420 return DataType.SUBSTANCEAMOUNT; 2421 if ("Timing".equals(codeString)) 2422 return DataType.TIMING; 2423 if ("TriggerDefinition".equals(codeString)) 2424 return DataType.TRIGGERDEFINITION; 2425 if ("UsageContext".equals(codeString)) 2426 return DataType.USAGECONTEXT; 2427 if ("base64Binary".equals(codeString)) 2428 return DataType.BASE64BINARY; 2429 if ("boolean".equals(codeString)) 2430 return DataType.BOOLEAN; 2431 if ("canonical".equals(codeString)) 2432 return DataType.CANONICAL; 2433 if ("code".equals(codeString)) 2434 return DataType.CODE; 2435 if ("date".equals(codeString)) 2436 return DataType.DATE; 2437 if ("dateTime".equals(codeString)) 2438 return DataType.DATETIME; 2439 if ("decimal".equals(codeString)) 2440 return DataType.DECIMAL; 2441 if ("id".equals(codeString)) 2442 return DataType.ID; 2443 if ("instant".equals(codeString)) 2444 return DataType.INSTANT; 2445 if ("integer".equals(codeString)) 2446 return DataType.INTEGER; 2447 if ("markdown".equals(codeString)) 2448 return DataType.MARKDOWN; 2449 if ("oid".equals(codeString)) 2450 return DataType.OID; 2451 if ("positiveInt".equals(codeString)) 2452 return DataType.POSITIVEINT; 2453 if ("string".equals(codeString)) 2454 return DataType.STRING; 2455 if ("time".equals(codeString)) 2456 return DataType.TIME; 2457 if ("unsignedInt".equals(codeString)) 2458 return DataType.UNSIGNEDINT; 2459 if ("uri".equals(codeString)) 2460 return DataType.URI; 2461 if ("url".equals(codeString)) 2462 return DataType.URL; 2463 if ("uuid".equals(codeString)) 2464 return DataType.UUID; 2465 if ("xhtml".equals(codeString)) 2466 return DataType.XHTML; 2467 throw new IllegalArgumentException("Unknown DataType code '" + codeString + "'"); 2468 } 2469 2470 public Enumeration<DataType> fromType(PrimitiveType<?> code) throws FHIRException { 2471 if (code == null) 2472 return null; 2473 if (code.isEmpty()) 2474 return new Enumeration<DataType>(this, DataType.NULL, code); 2475 String codeString = code.asStringValue(); 2476 if (codeString == null || "".equals(codeString)) 2477 return new Enumeration<DataType>(this, DataType.NULL, code); 2478 if ("Address".equals(codeString)) 2479 return new Enumeration<DataType>(this, DataType.ADDRESS, code); 2480 if ("Age".equals(codeString)) 2481 return new Enumeration<DataType>(this, DataType.AGE, code); 2482 if ("Annotation".equals(codeString)) 2483 return new Enumeration<DataType>(this, DataType.ANNOTATION, code); 2484 if ("Attachment".equals(codeString)) 2485 return new Enumeration<DataType>(this, DataType.ATTACHMENT, code); 2486 if ("BackboneElement".equals(codeString)) 2487 return new Enumeration<DataType>(this, DataType.BACKBONEELEMENT, code); 2488 if ("CodeableConcept".equals(codeString)) 2489 return new Enumeration<DataType>(this, DataType.CODEABLECONCEPT, code); 2490 if ("Coding".equals(codeString)) 2491 return new Enumeration<DataType>(this, DataType.CODING, code); 2492 if ("ContactDetail".equals(codeString)) 2493 return new Enumeration<DataType>(this, DataType.CONTACTDETAIL, code); 2494 if ("ContactPoint".equals(codeString)) 2495 return new Enumeration<DataType>(this, DataType.CONTACTPOINT, code); 2496 if ("Contributor".equals(codeString)) 2497 return new Enumeration<DataType>(this, DataType.CONTRIBUTOR, code); 2498 if ("Count".equals(codeString)) 2499 return new Enumeration<DataType>(this, DataType.COUNT, code); 2500 if ("DataRequirement".equals(codeString)) 2501 return new Enumeration<DataType>(this, DataType.DATAREQUIREMENT, code); 2502 if ("Distance".equals(codeString)) 2503 return new Enumeration<DataType>(this, DataType.DISTANCE, code); 2504 if ("Dosage".equals(codeString)) 2505 return new Enumeration<DataType>(this, DataType.DOSAGE, code); 2506 if ("Duration".equals(codeString)) 2507 return new Enumeration<DataType>(this, DataType.DURATION, code); 2508 if ("Element".equals(codeString)) 2509 return new Enumeration<DataType>(this, DataType.ELEMENT, code); 2510 if ("ElementDefinition".equals(codeString)) 2511 return new Enumeration<DataType>(this, DataType.ELEMENTDEFINITION, code); 2512 if ("Expression".equals(codeString)) 2513 return new Enumeration<DataType>(this, DataType.EXPRESSION, code); 2514 if ("Extension".equals(codeString)) 2515 return new Enumeration<DataType>(this, DataType.EXTENSION, code); 2516 if ("HumanName".equals(codeString)) 2517 return new Enumeration<DataType>(this, DataType.HUMANNAME, code); 2518 if ("Identifier".equals(codeString)) 2519 return new Enumeration<DataType>(this, DataType.IDENTIFIER, code); 2520 if ("MarketingStatus".equals(codeString)) 2521 return new Enumeration<DataType>(this, DataType.MARKETINGSTATUS, code); 2522 if ("Meta".equals(codeString)) 2523 return new Enumeration<DataType>(this, DataType.META, code); 2524 if ("Money".equals(codeString)) 2525 return new Enumeration<DataType>(this, DataType.MONEY, code); 2526 if ("MoneyQuantity".equals(codeString)) 2527 return new Enumeration<DataType>(this, DataType.MONEYQUANTITY, code); 2528 if ("Narrative".equals(codeString)) 2529 return new Enumeration<DataType>(this, DataType.NARRATIVE, code); 2530 if ("ParameterDefinition".equals(codeString)) 2531 return new Enumeration<DataType>(this, DataType.PARAMETERDEFINITION, code); 2532 if ("Period".equals(codeString)) 2533 return new Enumeration<DataType>(this, DataType.PERIOD, code); 2534 if ("Population".equals(codeString)) 2535 return new Enumeration<DataType>(this, DataType.POPULATION, code); 2536 if ("ProdCharacteristic".equals(codeString)) 2537 return new Enumeration<DataType>(this, DataType.PRODCHARACTERISTIC, code); 2538 if ("ProductShelfLife".equals(codeString)) 2539 return new Enumeration<DataType>(this, DataType.PRODUCTSHELFLIFE, code); 2540 if ("Quantity".equals(codeString)) 2541 return new Enumeration<DataType>(this, DataType.QUANTITY, code); 2542 if ("Range".equals(codeString)) 2543 return new Enumeration<DataType>(this, DataType.RANGE, code); 2544 if ("Ratio".equals(codeString)) 2545 return new Enumeration<DataType>(this, DataType.RATIO, code); 2546 if ("Reference".equals(codeString)) 2547 return new Enumeration<DataType>(this, DataType.REFERENCE, code); 2548 if ("RelatedArtifact".equals(codeString)) 2549 return new Enumeration<DataType>(this, DataType.RELATEDARTIFACT, code); 2550 if ("SampledData".equals(codeString)) 2551 return new Enumeration<DataType>(this, DataType.SAMPLEDDATA, code); 2552 if ("Signature".equals(codeString)) 2553 return new Enumeration<DataType>(this, DataType.SIGNATURE, code); 2554 if ("SimpleQuantity".equals(codeString)) 2555 return new Enumeration<DataType>(this, DataType.SIMPLEQUANTITY, code); 2556 if ("SubstanceAmount".equals(codeString)) 2557 return new Enumeration<DataType>(this, DataType.SUBSTANCEAMOUNT, code); 2558 if ("Timing".equals(codeString)) 2559 return new Enumeration<DataType>(this, DataType.TIMING, code); 2560 if ("TriggerDefinition".equals(codeString)) 2561 return new Enumeration<DataType>(this, DataType.TRIGGERDEFINITION, code); 2562 if ("UsageContext".equals(codeString)) 2563 return new Enumeration<DataType>(this, DataType.USAGECONTEXT, code); 2564 if ("base64Binary".equals(codeString)) 2565 return new Enumeration<DataType>(this, DataType.BASE64BINARY, code); 2566 if ("boolean".equals(codeString)) 2567 return new Enumeration<DataType>(this, DataType.BOOLEAN, code); 2568 if ("canonical".equals(codeString)) 2569 return new Enumeration<DataType>(this, DataType.CANONICAL, code); 2570 if ("code".equals(codeString)) 2571 return new Enumeration<DataType>(this, DataType.CODE, code); 2572 if ("date".equals(codeString)) 2573 return new Enumeration<DataType>(this, DataType.DATE, code); 2574 if ("dateTime".equals(codeString)) 2575 return new Enumeration<DataType>(this, DataType.DATETIME, code); 2576 if ("decimal".equals(codeString)) 2577 return new Enumeration<DataType>(this, DataType.DECIMAL, code); 2578 if ("id".equals(codeString)) 2579 return new Enumeration<DataType>(this, DataType.ID, code); 2580 if ("instant".equals(codeString)) 2581 return new Enumeration<DataType>(this, DataType.INSTANT, code); 2582 if ("integer".equals(codeString)) 2583 return new Enumeration<DataType>(this, DataType.INTEGER, code); 2584 if ("markdown".equals(codeString)) 2585 return new Enumeration<DataType>(this, DataType.MARKDOWN, code); 2586 if ("oid".equals(codeString)) 2587 return new Enumeration<DataType>(this, DataType.OID, code); 2588 if ("positiveInt".equals(codeString)) 2589 return new Enumeration<DataType>(this, DataType.POSITIVEINT, code); 2590 if ("string".equals(codeString)) 2591 return new Enumeration<DataType>(this, DataType.STRING, code); 2592 if ("time".equals(codeString)) 2593 return new Enumeration<DataType>(this, DataType.TIME, code); 2594 if ("unsignedInt".equals(codeString)) 2595 return new Enumeration<DataType>(this, DataType.UNSIGNEDINT, code); 2596 if ("uri".equals(codeString)) 2597 return new Enumeration<DataType>(this, DataType.URI, code); 2598 if ("url".equals(codeString)) 2599 return new Enumeration<DataType>(this, DataType.URL, code); 2600 if ("uuid".equals(codeString)) 2601 return new Enumeration<DataType>(this, DataType.UUID, code); 2602 if ("xhtml".equals(codeString)) 2603 return new Enumeration<DataType>(this, DataType.XHTML, code); 2604 throw new FHIRException("Unknown DataType code '" + codeString + "'"); 2605 } 2606 2607 public String toCode(DataType code) { 2608 if (code == DataType.ADDRESS) 2609 return "Address"; 2610 if (code == DataType.AGE) 2611 return "Age"; 2612 if (code == DataType.ANNOTATION) 2613 return "Annotation"; 2614 if (code == DataType.ATTACHMENT) 2615 return "Attachment"; 2616 if (code == DataType.BACKBONEELEMENT) 2617 return "BackboneElement"; 2618 if (code == DataType.CODEABLECONCEPT) 2619 return "CodeableConcept"; 2620 if (code == DataType.CODING) 2621 return "Coding"; 2622 if (code == DataType.CONTACTDETAIL) 2623 return "ContactDetail"; 2624 if (code == DataType.CONTACTPOINT) 2625 return "ContactPoint"; 2626 if (code == DataType.CONTRIBUTOR) 2627 return "Contributor"; 2628 if (code == DataType.COUNT) 2629 return "Count"; 2630 if (code == DataType.DATAREQUIREMENT) 2631 return "DataRequirement"; 2632 if (code == DataType.DISTANCE) 2633 return "Distance"; 2634 if (code == DataType.DOSAGE) 2635 return "Dosage"; 2636 if (code == DataType.DURATION) 2637 return "Duration"; 2638 if (code == DataType.ELEMENT) 2639 return "Element"; 2640 if (code == DataType.ELEMENTDEFINITION) 2641 return "ElementDefinition"; 2642 if (code == DataType.EXPRESSION) 2643 return "Expression"; 2644 if (code == DataType.EXTENSION) 2645 return "Extension"; 2646 if (code == DataType.HUMANNAME) 2647 return "HumanName"; 2648 if (code == DataType.IDENTIFIER) 2649 return "Identifier"; 2650 if (code == DataType.MARKETINGSTATUS) 2651 return "MarketingStatus"; 2652 if (code == DataType.META) 2653 return "Meta"; 2654 if (code == DataType.MONEY) 2655 return "Money"; 2656 if (code == DataType.MONEYQUANTITY) 2657 return "MoneyQuantity"; 2658 if (code == DataType.NARRATIVE) 2659 return "Narrative"; 2660 if (code == DataType.PARAMETERDEFINITION) 2661 return "ParameterDefinition"; 2662 if (code == DataType.PERIOD) 2663 return "Period"; 2664 if (code == DataType.POPULATION) 2665 return "Population"; 2666 if (code == DataType.PRODCHARACTERISTIC) 2667 return "ProdCharacteristic"; 2668 if (code == DataType.PRODUCTSHELFLIFE) 2669 return "ProductShelfLife"; 2670 if (code == DataType.QUANTITY) 2671 return "Quantity"; 2672 if (code == DataType.RANGE) 2673 return "Range"; 2674 if (code == DataType.RATIO) 2675 return "Ratio"; 2676 if (code == DataType.REFERENCE) 2677 return "Reference"; 2678 if (code == DataType.RELATEDARTIFACT) 2679 return "RelatedArtifact"; 2680 if (code == DataType.SAMPLEDDATA) 2681 return "SampledData"; 2682 if (code == DataType.SIGNATURE) 2683 return "Signature"; 2684 if (code == DataType.SIMPLEQUANTITY) 2685 return "SimpleQuantity"; 2686 if (code == DataType.SUBSTANCEAMOUNT) 2687 return "SubstanceAmount"; 2688 if (code == DataType.TIMING) 2689 return "Timing"; 2690 if (code == DataType.TRIGGERDEFINITION) 2691 return "TriggerDefinition"; 2692 if (code == DataType.USAGECONTEXT) 2693 return "UsageContext"; 2694 if (code == DataType.BASE64BINARY) 2695 return "base64Binary"; 2696 if (code == DataType.BOOLEAN) 2697 return "boolean"; 2698 if (code == DataType.CANONICAL) 2699 return "canonical"; 2700 if (code == DataType.CODE) 2701 return "code"; 2702 if (code == DataType.DATE) 2703 return "date"; 2704 if (code == DataType.DATETIME) 2705 return "dateTime"; 2706 if (code == DataType.DECIMAL) 2707 return "decimal"; 2708 if (code == DataType.ID) 2709 return "id"; 2710 if (code == DataType.INSTANT) 2711 return "instant"; 2712 if (code == DataType.INTEGER) 2713 return "integer"; 2714 if (code == DataType.MARKDOWN) 2715 return "markdown"; 2716 if (code == DataType.OID) 2717 return "oid"; 2718 if (code == DataType.POSITIVEINT) 2719 return "positiveInt"; 2720 if (code == DataType.STRING) 2721 return "string"; 2722 if (code == DataType.TIME) 2723 return "time"; 2724 if (code == DataType.UNSIGNEDINT) 2725 return "unsignedInt"; 2726 if (code == DataType.URI) 2727 return "uri"; 2728 if (code == DataType.URL) 2729 return "url"; 2730 if (code == DataType.UUID) 2731 return "uuid"; 2732 if (code == DataType.XHTML) 2733 return "xhtml"; 2734 return "?"; 2735 } 2736 2737 public String toSystem(DataType code) { 2738 return code.getSystem(); 2739 } 2740 } 2741 2742 public enum DefinitionResourceType { 2743 /** 2744 * This resource allows for the definition of some activity to be performed, 2745 * independent of a particular patient, practitioner, or other performance 2746 * context. 2747 */ 2748 ACTIVITYDEFINITION, 2749 /** 2750 * The EventDefinition resource provides a reusable description of when a 2751 * particular event can occur. 2752 */ 2753 EVENTDEFINITION, 2754 /** 2755 * The Measure resource provides the definition of a quality measure. 2756 */ 2757 MEASURE, 2758 /** 2759 * A formal computable definition of an operation (on the RESTful interface) or 2760 * a named query (using the search interaction). 2761 */ 2762 OPERATIONDEFINITION, 2763 /** 2764 * This resource allows for the definition of various types of plans as a 2765 * sharable, consumable, and executable artifact. The resource is general enough 2766 * to support the description of a broad range of clinical artifacts such as 2767 * clinical decision support rules, order sets and protocols. 2768 */ 2769 PLANDEFINITION, 2770 /** 2771 * A structured set of questions intended to guide the collection of answers 2772 * from end-users. Questionnaires provide detailed control over order, 2773 * presentation, phraseology and grouping to allow coherent, consistent data 2774 * collection. 2775 */ 2776 QUESTIONNAIRE, 2777 /** 2778 * added to help the parsers 2779 */ 2780 NULL; 2781 2782 public static DefinitionResourceType fromCode(String codeString) throws FHIRException { 2783 if (codeString == null || "".equals(codeString)) 2784 return null; 2785 if ("ActivityDefinition".equals(codeString)) 2786 return ACTIVITYDEFINITION; 2787 if ("EventDefinition".equals(codeString)) 2788 return EVENTDEFINITION; 2789 if ("Measure".equals(codeString)) 2790 return MEASURE; 2791 if ("OperationDefinition".equals(codeString)) 2792 return OPERATIONDEFINITION; 2793 if ("PlanDefinition".equals(codeString)) 2794 return PLANDEFINITION; 2795 if ("Questionnaire".equals(codeString)) 2796 return QUESTIONNAIRE; 2797 throw new FHIRException("Unknown DefinitionResourceType code '" + codeString + "'"); 2798 } 2799 2800 public String toCode() { 2801 switch (this) { 2802 case ACTIVITYDEFINITION: 2803 return "ActivityDefinition"; 2804 case EVENTDEFINITION: 2805 return "EventDefinition"; 2806 case MEASURE: 2807 return "Measure"; 2808 case OPERATIONDEFINITION: 2809 return "OperationDefinition"; 2810 case PLANDEFINITION: 2811 return "PlanDefinition"; 2812 case QUESTIONNAIRE: 2813 return "Questionnaire"; 2814 case NULL: 2815 return null; 2816 default: 2817 return "?"; 2818 } 2819 } 2820 2821 public String getSystem() { 2822 switch (this) { 2823 case ACTIVITYDEFINITION: 2824 return "http://hl7.org/fhir/definition-resource-types"; 2825 case EVENTDEFINITION: 2826 return "http://hl7.org/fhir/definition-resource-types"; 2827 case MEASURE: 2828 return "http://hl7.org/fhir/definition-resource-types"; 2829 case OPERATIONDEFINITION: 2830 return "http://hl7.org/fhir/definition-resource-types"; 2831 case PLANDEFINITION: 2832 return "http://hl7.org/fhir/definition-resource-types"; 2833 case QUESTIONNAIRE: 2834 return "http://hl7.org/fhir/definition-resource-types"; 2835 case NULL: 2836 return null; 2837 default: 2838 return "?"; 2839 } 2840 } 2841 2842 public String getDefinition() { 2843 switch (this) { 2844 case ACTIVITYDEFINITION: 2845 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 2846 case EVENTDEFINITION: 2847 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 2848 case MEASURE: 2849 return "The Measure resource provides the definition of a quality measure."; 2850 case OPERATIONDEFINITION: 2851 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 2852 case PLANDEFINITION: 2853 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 2854 case QUESTIONNAIRE: 2855 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 2856 case NULL: 2857 return null; 2858 default: 2859 return "?"; 2860 } 2861 } 2862 2863 public String getDisplay() { 2864 switch (this) { 2865 case ACTIVITYDEFINITION: 2866 return "ActivityDefinition"; 2867 case EVENTDEFINITION: 2868 return "EventDefinition"; 2869 case MEASURE: 2870 return "Measure"; 2871 case OPERATIONDEFINITION: 2872 return "OperationDefinition"; 2873 case PLANDEFINITION: 2874 return "PlanDefinition"; 2875 case QUESTIONNAIRE: 2876 return "Questionnaire"; 2877 case NULL: 2878 return null; 2879 default: 2880 return "?"; 2881 } 2882 } 2883 } 2884 2885 public static class DefinitionResourceTypeEnumFactory implements EnumFactory<DefinitionResourceType> { 2886 public DefinitionResourceType fromCode(String codeString) throws IllegalArgumentException { 2887 if (codeString == null || "".equals(codeString)) 2888 if (codeString == null || "".equals(codeString)) 2889 return null; 2890 if ("ActivityDefinition".equals(codeString)) 2891 return DefinitionResourceType.ACTIVITYDEFINITION; 2892 if ("EventDefinition".equals(codeString)) 2893 return DefinitionResourceType.EVENTDEFINITION; 2894 if ("Measure".equals(codeString)) 2895 return DefinitionResourceType.MEASURE; 2896 if ("OperationDefinition".equals(codeString)) 2897 return DefinitionResourceType.OPERATIONDEFINITION; 2898 if ("PlanDefinition".equals(codeString)) 2899 return DefinitionResourceType.PLANDEFINITION; 2900 if ("Questionnaire".equals(codeString)) 2901 return DefinitionResourceType.QUESTIONNAIRE; 2902 throw new IllegalArgumentException("Unknown DefinitionResourceType code '" + codeString + "'"); 2903 } 2904 2905 public Enumeration<DefinitionResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 2906 if (code == null) 2907 return null; 2908 if (code.isEmpty()) 2909 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.NULL, code); 2910 String codeString = code.asStringValue(); 2911 if (codeString == null || "".equals(codeString)) 2912 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.NULL, code); 2913 if ("ActivityDefinition".equals(codeString)) 2914 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.ACTIVITYDEFINITION, code); 2915 if ("EventDefinition".equals(codeString)) 2916 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.EVENTDEFINITION, code); 2917 if ("Measure".equals(codeString)) 2918 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.MEASURE, code); 2919 if ("OperationDefinition".equals(codeString)) 2920 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.OPERATIONDEFINITION, code); 2921 if ("PlanDefinition".equals(codeString)) 2922 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.PLANDEFINITION, code); 2923 if ("Questionnaire".equals(codeString)) 2924 return new Enumeration<DefinitionResourceType>(this, DefinitionResourceType.QUESTIONNAIRE, code); 2925 throw new FHIRException("Unknown DefinitionResourceType code '" + codeString + "'"); 2926 } 2927 2928 public String toCode(DefinitionResourceType code) { 2929 if (code == DefinitionResourceType.ACTIVITYDEFINITION) 2930 return "ActivityDefinition"; 2931 if (code == DefinitionResourceType.EVENTDEFINITION) 2932 return "EventDefinition"; 2933 if (code == DefinitionResourceType.MEASURE) 2934 return "Measure"; 2935 if (code == DefinitionResourceType.OPERATIONDEFINITION) 2936 return "OperationDefinition"; 2937 if (code == DefinitionResourceType.PLANDEFINITION) 2938 return "PlanDefinition"; 2939 if (code == DefinitionResourceType.QUESTIONNAIRE) 2940 return "Questionnaire"; 2941 return "?"; 2942 } 2943 2944 public String toSystem(DefinitionResourceType code) { 2945 return code.getSystem(); 2946 } 2947 } 2948 2949 public enum DocumentReferenceStatus { 2950 /** 2951 * This is the current reference for this document. 2952 */ 2953 CURRENT, 2954 /** 2955 * This reference has been superseded by another reference. 2956 */ 2957 SUPERSEDED, 2958 /** 2959 * This reference was created in error. 2960 */ 2961 ENTEREDINERROR, 2962 /** 2963 * added to help the parsers 2964 */ 2965 NULL; 2966 2967 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 2968 if (codeString == null || "".equals(codeString)) 2969 return null; 2970 if ("current".equals(codeString)) 2971 return CURRENT; 2972 if ("superseded".equals(codeString)) 2973 return SUPERSEDED; 2974 if ("entered-in-error".equals(codeString)) 2975 return ENTEREDINERROR; 2976 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 2977 } 2978 2979 public String toCode() { 2980 switch (this) { 2981 case CURRENT: 2982 return "current"; 2983 case SUPERSEDED: 2984 return "superseded"; 2985 case ENTEREDINERROR: 2986 return "entered-in-error"; 2987 case NULL: 2988 return null; 2989 default: 2990 return "?"; 2991 } 2992 } 2993 2994 public String getSystem() { 2995 switch (this) { 2996 case CURRENT: 2997 return "http://hl7.org/fhir/document-reference-status"; 2998 case SUPERSEDED: 2999 return "http://hl7.org/fhir/document-reference-status"; 3000 case ENTEREDINERROR: 3001 return "http://hl7.org/fhir/document-reference-status"; 3002 case NULL: 3003 return null; 3004 default: 3005 return "?"; 3006 } 3007 } 3008 3009 public String getDefinition() { 3010 switch (this) { 3011 case CURRENT: 3012 return "This is the current reference for this document."; 3013 case SUPERSEDED: 3014 return "This reference has been superseded by another reference."; 3015 case ENTEREDINERROR: 3016 return "This reference was created in error."; 3017 case NULL: 3018 return null; 3019 default: 3020 return "?"; 3021 } 3022 } 3023 3024 public String getDisplay() { 3025 switch (this) { 3026 case CURRENT: 3027 return "Current"; 3028 case SUPERSEDED: 3029 return "Superseded"; 3030 case ENTEREDINERROR: 3031 return "Entered in Error"; 3032 case NULL: 3033 return null; 3034 default: 3035 return "?"; 3036 } 3037 } 3038 } 3039 3040 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 3041 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 3042 if (codeString == null || "".equals(codeString)) 3043 if (codeString == null || "".equals(codeString)) 3044 return null; 3045 if ("current".equals(codeString)) 3046 return DocumentReferenceStatus.CURRENT; 3047 if ("superseded".equals(codeString)) 3048 return DocumentReferenceStatus.SUPERSEDED; 3049 if ("entered-in-error".equals(codeString)) 3050 return DocumentReferenceStatus.ENTEREDINERROR; 3051 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 3052 } 3053 3054 public Enumeration<DocumentReferenceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 3055 if (code == null) 3056 return null; 3057 if (code.isEmpty()) 3058 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 3059 String codeString = code.asStringValue(); 3060 if (codeString == null || "".equals(codeString)) 3061 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.NULL, code); 3062 if ("current".equals(codeString)) 3063 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT, code); 3064 if ("superseded".equals(codeString)) 3065 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED, code); 3066 if ("entered-in-error".equals(codeString)) 3067 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR, code); 3068 throw new FHIRException("Unknown DocumentReferenceStatus code '" + codeString + "'"); 3069 } 3070 3071 public String toCode(DocumentReferenceStatus code) { 3072 if (code == DocumentReferenceStatus.CURRENT) 3073 return "current"; 3074 if (code == DocumentReferenceStatus.SUPERSEDED) 3075 return "superseded"; 3076 if (code == DocumentReferenceStatus.ENTEREDINERROR) 3077 return "entered-in-error"; 3078 return "?"; 3079 } 3080 3081 public String toSystem(DocumentReferenceStatus code) { 3082 return code.getSystem(); 3083 } 3084 } 3085 3086 public enum EventResourceType { 3087 /** 3088 * Item containing charge code(s) associated with the provision of healthcare 3089 * provider products. 3090 */ 3091 CHARGEITEM, 3092 /** 3093 * Remittance resource. 3094 */ 3095 CLAIMRESPONSE, 3096 /** 3097 * A clinical assessment performed when planning treatments and management 3098 * strategies for a patient. 3099 */ 3100 CLINICALIMPRESSION, 3101 /** 3102 * A record of information transmitted from a sender to a receiver. 3103 */ 3104 COMMUNICATION, 3105 /** 3106 * A set of resources composed into a single coherent clinical statement with 3107 * clinical attestation. 3108 */ 3109 COMPOSITION, 3110 /** 3111 * Detailed information about conditions, problems or diagnoses. 3112 */ 3113 CONDITION, 3114 /** 3115 * A healthcare consumer's policy choices to permits or denies recipients or 3116 * roles to perform actions for specific purposes and periods of time. 3117 */ 3118 CONSENT, 3119 /** 3120 * Insurance or medical plan or a payment agreement. 3121 */ 3122 COVERAGE, 3123 /** 3124 * Record of use of a device. 3125 */ 3126 DEVICEUSESTATEMENT, 3127 /** 3128 * A Diagnostic report - a combination of request information, atomic results, 3129 * images, interpretation, as well as formatted reports. 3130 */ 3131 DIAGNOSTICREPORT, 3132 /** 3133 * A list that defines a set of documents. 3134 */ 3135 DOCUMENTMANIFEST, 3136 /** 3137 * A reference to a document. 3138 */ 3139 DOCUMENTREFERENCE, 3140 /** 3141 * An interaction during which services are provided to the patient. 3142 */ 3143 ENCOUNTER, 3144 /** 3145 * EnrollmentResponse resource. 3146 */ 3147 ENROLLMENTRESPONSE, 3148 /** 3149 * An association of a Patient with an Organization and Healthcare Provider(s) 3150 * for a period of time that the Organization assumes some level of 3151 * responsibility. 3152 */ 3153 EPISODEOFCARE, 3154 /** 3155 * Explanation of Benefit resource. 3156 */ 3157 EXPLANATIONOFBENEFIT, 3158 /** 3159 * Information about patient's relatives, relevant for patient. 3160 */ 3161 FAMILYMEMBERHISTORY, 3162 /** 3163 * The formal response to a guidance request. 3164 */ 3165 GUIDANCERESPONSE, 3166 /** 3167 * A set of images produced in single study (one or more series of references 3168 * images). 3169 */ 3170 IMAGINGSTUDY, 3171 /** 3172 * Immunization event information. 3173 */ 3174 IMMUNIZATION, 3175 /** 3176 * Results of a measure evaluation. 3177 */ 3178 MEASUREREPORT, 3179 /** 3180 * A photo, video, or audio recording acquired or used in healthcare. The actual 3181 * content may be inline or provided by direct reference. 3182 */ 3183 MEDIA, 3184 /** 3185 * Administration of medication to a patient. 3186 */ 3187 MEDICATIONADMINISTRATION, 3188 /** 3189 * Dispensing a medication to a named patient. 3190 */ 3191 MEDICATIONDISPENSE, 3192 /** 3193 * Record of medication being taken by a patient. 3194 */ 3195 MEDICATIONSTATEMENT, 3196 /** 3197 * Measurements and simple assertions. 3198 */ 3199 OBSERVATION, 3200 /** 3201 * PaymentNotice request. 3202 */ 3203 PAYMENTNOTICE, 3204 /** 3205 * PaymentReconciliation resource. 3206 */ 3207 PAYMENTRECONCILIATION, 3208 /** 3209 * An action that is being or was performed on a patient. 3210 */ 3211 PROCEDURE, 3212 /** 3213 * ProcessResponse resource. 3214 */ 3215 PROCESSRESPONSE, 3216 /** 3217 * A structured set of questions and their answers. 3218 */ 3219 QUESTIONNAIRERESPONSE, 3220 /** 3221 * Potential outcomes for a subject with likelihood. 3222 */ 3223 RISKASSESSMENT, 3224 /** 3225 * Delivery of bulk Supplies. 3226 */ 3227 SUPPLYDELIVERY, 3228 /** 3229 * A task to be performed. 3230 */ 3231 TASK, 3232 /** 3233 * added to help the parsers 3234 */ 3235 NULL; 3236 3237 public static EventResourceType fromCode(String codeString) throws FHIRException { 3238 if (codeString == null || "".equals(codeString)) 3239 return null; 3240 if ("ChargeItem".equals(codeString)) 3241 return CHARGEITEM; 3242 if ("ClaimResponse".equals(codeString)) 3243 return CLAIMRESPONSE; 3244 if ("ClinicalImpression".equals(codeString)) 3245 return CLINICALIMPRESSION; 3246 if ("Communication".equals(codeString)) 3247 return COMMUNICATION; 3248 if ("Composition".equals(codeString)) 3249 return COMPOSITION; 3250 if ("Condition".equals(codeString)) 3251 return CONDITION; 3252 if ("Consent".equals(codeString)) 3253 return CONSENT; 3254 if ("Coverage".equals(codeString)) 3255 return COVERAGE; 3256 if ("DeviceUseStatement".equals(codeString)) 3257 return DEVICEUSESTATEMENT; 3258 if ("DiagnosticReport".equals(codeString)) 3259 return DIAGNOSTICREPORT; 3260 if ("DocumentManifest".equals(codeString)) 3261 return DOCUMENTMANIFEST; 3262 if ("DocumentReference".equals(codeString)) 3263 return DOCUMENTREFERENCE; 3264 if ("Encounter".equals(codeString)) 3265 return ENCOUNTER; 3266 if ("EnrollmentResponse".equals(codeString)) 3267 return ENROLLMENTRESPONSE; 3268 if ("EpisodeOfCare".equals(codeString)) 3269 return EPISODEOFCARE; 3270 if ("ExplanationOfBenefit".equals(codeString)) 3271 return EXPLANATIONOFBENEFIT; 3272 if ("FamilyMemberHistory".equals(codeString)) 3273 return FAMILYMEMBERHISTORY; 3274 if ("GuidanceResponse".equals(codeString)) 3275 return GUIDANCERESPONSE; 3276 if ("ImagingStudy".equals(codeString)) 3277 return IMAGINGSTUDY; 3278 if ("Immunization".equals(codeString)) 3279 return IMMUNIZATION; 3280 if ("MeasureReport".equals(codeString)) 3281 return MEASUREREPORT; 3282 if ("Media".equals(codeString)) 3283 return MEDIA; 3284 if ("MedicationAdministration".equals(codeString)) 3285 return MEDICATIONADMINISTRATION; 3286 if ("MedicationDispense".equals(codeString)) 3287 return MEDICATIONDISPENSE; 3288 if ("MedicationStatement".equals(codeString)) 3289 return MEDICATIONSTATEMENT; 3290 if ("Observation".equals(codeString)) 3291 return OBSERVATION; 3292 if ("PaymentNotice".equals(codeString)) 3293 return PAYMENTNOTICE; 3294 if ("PaymentReconciliation".equals(codeString)) 3295 return PAYMENTRECONCILIATION; 3296 if ("Procedure".equals(codeString)) 3297 return PROCEDURE; 3298 if ("ProcessResponse".equals(codeString)) 3299 return PROCESSRESPONSE; 3300 if ("QuestionnaireResponse".equals(codeString)) 3301 return QUESTIONNAIRERESPONSE; 3302 if ("RiskAssessment".equals(codeString)) 3303 return RISKASSESSMENT; 3304 if ("SupplyDelivery".equals(codeString)) 3305 return SUPPLYDELIVERY; 3306 if ("Task".equals(codeString)) 3307 return TASK; 3308 throw new FHIRException("Unknown EventResourceType code '" + codeString + "'"); 3309 } 3310 3311 public String toCode() { 3312 switch (this) { 3313 case CHARGEITEM: 3314 return "ChargeItem"; 3315 case CLAIMRESPONSE: 3316 return "ClaimResponse"; 3317 case CLINICALIMPRESSION: 3318 return "ClinicalImpression"; 3319 case COMMUNICATION: 3320 return "Communication"; 3321 case COMPOSITION: 3322 return "Composition"; 3323 case CONDITION: 3324 return "Condition"; 3325 case CONSENT: 3326 return "Consent"; 3327 case COVERAGE: 3328 return "Coverage"; 3329 case DEVICEUSESTATEMENT: 3330 return "DeviceUseStatement"; 3331 case DIAGNOSTICREPORT: 3332 return "DiagnosticReport"; 3333 case DOCUMENTMANIFEST: 3334 return "DocumentManifest"; 3335 case DOCUMENTREFERENCE: 3336 return "DocumentReference"; 3337 case ENCOUNTER: 3338 return "Encounter"; 3339 case ENROLLMENTRESPONSE: 3340 return "EnrollmentResponse"; 3341 case EPISODEOFCARE: 3342 return "EpisodeOfCare"; 3343 case EXPLANATIONOFBENEFIT: 3344 return "ExplanationOfBenefit"; 3345 case FAMILYMEMBERHISTORY: 3346 return "FamilyMemberHistory"; 3347 case GUIDANCERESPONSE: 3348 return "GuidanceResponse"; 3349 case IMAGINGSTUDY: 3350 return "ImagingStudy"; 3351 case IMMUNIZATION: 3352 return "Immunization"; 3353 case MEASUREREPORT: 3354 return "MeasureReport"; 3355 case MEDIA: 3356 return "Media"; 3357 case MEDICATIONADMINISTRATION: 3358 return "MedicationAdministration"; 3359 case MEDICATIONDISPENSE: 3360 return "MedicationDispense"; 3361 case MEDICATIONSTATEMENT: 3362 return "MedicationStatement"; 3363 case OBSERVATION: 3364 return "Observation"; 3365 case PAYMENTNOTICE: 3366 return "PaymentNotice"; 3367 case PAYMENTRECONCILIATION: 3368 return "PaymentReconciliation"; 3369 case PROCEDURE: 3370 return "Procedure"; 3371 case PROCESSRESPONSE: 3372 return "ProcessResponse"; 3373 case QUESTIONNAIRERESPONSE: 3374 return "QuestionnaireResponse"; 3375 case RISKASSESSMENT: 3376 return "RiskAssessment"; 3377 case SUPPLYDELIVERY: 3378 return "SupplyDelivery"; 3379 case TASK: 3380 return "Task"; 3381 case NULL: 3382 return null; 3383 default: 3384 return "?"; 3385 } 3386 } 3387 3388 public String getSystem() { 3389 switch (this) { 3390 case CHARGEITEM: 3391 return "http://hl7.org/fhir/event-resource-types"; 3392 case CLAIMRESPONSE: 3393 return "http://hl7.org/fhir/event-resource-types"; 3394 case CLINICALIMPRESSION: 3395 return "http://hl7.org/fhir/event-resource-types"; 3396 case COMMUNICATION: 3397 return "http://hl7.org/fhir/event-resource-types"; 3398 case COMPOSITION: 3399 return "http://hl7.org/fhir/event-resource-types"; 3400 case CONDITION: 3401 return "http://hl7.org/fhir/event-resource-types"; 3402 case CONSENT: 3403 return "http://hl7.org/fhir/event-resource-types"; 3404 case COVERAGE: 3405 return "http://hl7.org/fhir/event-resource-types"; 3406 case DEVICEUSESTATEMENT: 3407 return "http://hl7.org/fhir/event-resource-types"; 3408 case DIAGNOSTICREPORT: 3409 return "http://hl7.org/fhir/event-resource-types"; 3410 case DOCUMENTMANIFEST: 3411 return "http://hl7.org/fhir/event-resource-types"; 3412 case DOCUMENTREFERENCE: 3413 return "http://hl7.org/fhir/event-resource-types"; 3414 case ENCOUNTER: 3415 return "http://hl7.org/fhir/event-resource-types"; 3416 case ENROLLMENTRESPONSE: 3417 return "http://hl7.org/fhir/event-resource-types"; 3418 case EPISODEOFCARE: 3419 return "http://hl7.org/fhir/event-resource-types"; 3420 case EXPLANATIONOFBENEFIT: 3421 return "http://hl7.org/fhir/event-resource-types"; 3422 case FAMILYMEMBERHISTORY: 3423 return "http://hl7.org/fhir/event-resource-types"; 3424 case GUIDANCERESPONSE: 3425 return "http://hl7.org/fhir/event-resource-types"; 3426 case IMAGINGSTUDY: 3427 return "http://hl7.org/fhir/event-resource-types"; 3428 case IMMUNIZATION: 3429 return "http://hl7.org/fhir/event-resource-types"; 3430 case MEASUREREPORT: 3431 return "http://hl7.org/fhir/event-resource-types"; 3432 case MEDIA: 3433 return "http://hl7.org/fhir/event-resource-types"; 3434 case MEDICATIONADMINISTRATION: 3435 return "http://hl7.org/fhir/event-resource-types"; 3436 case MEDICATIONDISPENSE: 3437 return "http://hl7.org/fhir/event-resource-types"; 3438 case MEDICATIONSTATEMENT: 3439 return "http://hl7.org/fhir/event-resource-types"; 3440 case OBSERVATION: 3441 return "http://hl7.org/fhir/event-resource-types"; 3442 case PAYMENTNOTICE: 3443 return "http://hl7.org/fhir/event-resource-types"; 3444 case PAYMENTRECONCILIATION: 3445 return "http://hl7.org/fhir/event-resource-types"; 3446 case PROCEDURE: 3447 return "http://hl7.org/fhir/event-resource-types"; 3448 case PROCESSRESPONSE: 3449 return "http://hl7.org/fhir/event-resource-types"; 3450 case QUESTIONNAIRERESPONSE: 3451 return "http://hl7.org/fhir/event-resource-types"; 3452 case RISKASSESSMENT: 3453 return "http://hl7.org/fhir/event-resource-types"; 3454 case SUPPLYDELIVERY: 3455 return "http://hl7.org/fhir/event-resource-types"; 3456 case TASK: 3457 return "http://hl7.org/fhir/event-resource-types"; 3458 case NULL: 3459 return null; 3460 default: 3461 return "?"; 3462 } 3463 } 3464 3465 public String getDefinition() { 3466 switch (this) { 3467 case CHARGEITEM: 3468 return "Item containing charge code(s) associated with the provision of healthcare provider products."; 3469 case CLAIMRESPONSE: 3470 return "Remittance resource."; 3471 case CLINICALIMPRESSION: 3472 return "A clinical assessment performed when planning treatments and management strategies for a patient."; 3473 case COMMUNICATION: 3474 return "A record of information transmitted from a sender to a receiver."; 3475 case COMPOSITION: 3476 return "A set of resources composed into a single coherent clinical statement with clinical attestation."; 3477 case CONDITION: 3478 return "Detailed information about conditions, problems or diagnoses."; 3479 case CONSENT: 3480 return "A healthcare consumer's policy choices to permits or denies recipients or roles to perform actions for specific purposes and periods of time."; 3481 case COVERAGE: 3482 return "Insurance or medical plan or a payment agreement."; 3483 case DEVICEUSESTATEMENT: 3484 return "Record of use of a device."; 3485 case DIAGNOSTICREPORT: 3486 return "A Diagnostic report - a combination of request information, atomic results, images, interpretation, as well as formatted reports."; 3487 case DOCUMENTMANIFEST: 3488 return "A list that defines a set of documents."; 3489 case DOCUMENTREFERENCE: 3490 return "A reference to a document."; 3491 case ENCOUNTER: 3492 return "An interaction during which services are provided to the patient."; 3493 case ENROLLMENTRESPONSE: 3494 return "EnrollmentResponse resource."; 3495 case EPISODEOFCARE: 3496 return "An association of a Patient with an Organization and Healthcare Provider(s) for a period of time that the Organization assumes some level of responsibility."; 3497 case EXPLANATIONOFBENEFIT: 3498 return "Explanation of Benefit resource."; 3499 case FAMILYMEMBERHISTORY: 3500 return "Information about patient's relatives, relevant for patient."; 3501 case GUIDANCERESPONSE: 3502 return "The formal response to a guidance request."; 3503 case IMAGINGSTUDY: 3504 return "A set of images produced in single study (one or more series of references images)."; 3505 case IMMUNIZATION: 3506 return "Immunization event information."; 3507 case MEASUREREPORT: 3508 return "Results of a measure evaluation."; 3509 case MEDIA: 3510 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 3511 case MEDICATIONADMINISTRATION: 3512 return "Administration of medication to a patient."; 3513 case MEDICATIONDISPENSE: 3514 return "Dispensing a medication to a named patient."; 3515 case MEDICATIONSTATEMENT: 3516 return "Record of medication being taken by a patient."; 3517 case OBSERVATION: 3518 return "Measurements and simple assertions."; 3519 case PAYMENTNOTICE: 3520 return "PaymentNotice request."; 3521 case PAYMENTRECONCILIATION: 3522 return "PaymentReconciliation resource."; 3523 case PROCEDURE: 3524 return "An action that is being or was performed on a patient."; 3525 case PROCESSRESPONSE: 3526 return "ProcessResponse resource."; 3527 case QUESTIONNAIRERESPONSE: 3528 return "A structured set of questions and their answers."; 3529 case RISKASSESSMENT: 3530 return "Potential outcomes for a subject with likelihood."; 3531 case SUPPLYDELIVERY: 3532 return "Delivery of bulk Supplies."; 3533 case TASK: 3534 return "A task to be performed."; 3535 case NULL: 3536 return null; 3537 default: 3538 return "?"; 3539 } 3540 } 3541 3542 public String getDisplay() { 3543 switch (this) { 3544 case CHARGEITEM: 3545 return "ChargeItem"; 3546 case CLAIMRESPONSE: 3547 return "ClaimResponse"; 3548 case CLINICALIMPRESSION: 3549 return "ClinicalImpression"; 3550 case COMMUNICATION: 3551 return "Communication"; 3552 case COMPOSITION: 3553 return "Composition"; 3554 case CONDITION: 3555 return "Condition"; 3556 case CONSENT: 3557 return "Consent"; 3558 case COVERAGE: 3559 return "Coverage"; 3560 case DEVICEUSESTATEMENT: 3561 return "DeviceUseStatement"; 3562 case DIAGNOSTICREPORT: 3563 return "DiagnosticReport"; 3564 case DOCUMENTMANIFEST: 3565 return "DocumentManifest"; 3566 case DOCUMENTREFERENCE: 3567 return "DocumentReference"; 3568 case ENCOUNTER: 3569 return "Encounter"; 3570 case ENROLLMENTRESPONSE: 3571 return "EnrollmentResponse"; 3572 case EPISODEOFCARE: 3573 return "EpisodeOfCare"; 3574 case EXPLANATIONOFBENEFIT: 3575 return "ExplanationOfBenefit"; 3576 case FAMILYMEMBERHISTORY: 3577 return "FamilyMemberHistory"; 3578 case GUIDANCERESPONSE: 3579 return "GuidanceResponse"; 3580 case IMAGINGSTUDY: 3581 return "ImagingStudy"; 3582 case IMMUNIZATION: 3583 return "Immunization"; 3584 case MEASUREREPORT: 3585 return "MeasureReport"; 3586 case MEDIA: 3587 return "Media"; 3588 case MEDICATIONADMINISTRATION: 3589 return "MedicationAdministration"; 3590 case MEDICATIONDISPENSE: 3591 return "MedicationDispense"; 3592 case MEDICATIONSTATEMENT: 3593 return "MedicationStatement"; 3594 case OBSERVATION: 3595 return "Observation"; 3596 case PAYMENTNOTICE: 3597 return "PaymentNotice"; 3598 case PAYMENTRECONCILIATION: 3599 return "PaymentReconciliation"; 3600 case PROCEDURE: 3601 return "Procedure"; 3602 case PROCESSRESPONSE: 3603 return "ProcessResponse"; 3604 case QUESTIONNAIRERESPONSE: 3605 return "QuestionnaireResponse"; 3606 case RISKASSESSMENT: 3607 return "RiskAssessment"; 3608 case SUPPLYDELIVERY: 3609 return "SupplyDelivery"; 3610 case TASK: 3611 return "Task"; 3612 case NULL: 3613 return null; 3614 default: 3615 return "?"; 3616 } 3617 } 3618 } 3619 3620 public static class EventResourceTypeEnumFactory implements EnumFactory<EventResourceType> { 3621 public EventResourceType fromCode(String codeString) throws IllegalArgumentException { 3622 if (codeString == null || "".equals(codeString)) 3623 if (codeString == null || "".equals(codeString)) 3624 return null; 3625 if ("ChargeItem".equals(codeString)) 3626 return EventResourceType.CHARGEITEM; 3627 if ("ClaimResponse".equals(codeString)) 3628 return EventResourceType.CLAIMRESPONSE; 3629 if ("ClinicalImpression".equals(codeString)) 3630 return EventResourceType.CLINICALIMPRESSION; 3631 if ("Communication".equals(codeString)) 3632 return EventResourceType.COMMUNICATION; 3633 if ("Composition".equals(codeString)) 3634 return EventResourceType.COMPOSITION; 3635 if ("Condition".equals(codeString)) 3636 return EventResourceType.CONDITION; 3637 if ("Consent".equals(codeString)) 3638 return EventResourceType.CONSENT; 3639 if ("Coverage".equals(codeString)) 3640 return EventResourceType.COVERAGE; 3641 if ("DeviceUseStatement".equals(codeString)) 3642 return EventResourceType.DEVICEUSESTATEMENT; 3643 if ("DiagnosticReport".equals(codeString)) 3644 return EventResourceType.DIAGNOSTICREPORT; 3645 if ("DocumentManifest".equals(codeString)) 3646 return EventResourceType.DOCUMENTMANIFEST; 3647 if ("DocumentReference".equals(codeString)) 3648 return EventResourceType.DOCUMENTREFERENCE; 3649 if ("Encounter".equals(codeString)) 3650 return EventResourceType.ENCOUNTER; 3651 if ("EnrollmentResponse".equals(codeString)) 3652 return EventResourceType.ENROLLMENTRESPONSE; 3653 if ("EpisodeOfCare".equals(codeString)) 3654 return EventResourceType.EPISODEOFCARE; 3655 if ("ExplanationOfBenefit".equals(codeString)) 3656 return EventResourceType.EXPLANATIONOFBENEFIT; 3657 if ("FamilyMemberHistory".equals(codeString)) 3658 return EventResourceType.FAMILYMEMBERHISTORY; 3659 if ("GuidanceResponse".equals(codeString)) 3660 return EventResourceType.GUIDANCERESPONSE; 3661 if ("ImagingStudy".equals(codeString)) 3662 return EventResourceType.IMAGINGSTUDY; 3663 if ("Immunization".equals(codeString)) 3664 return EventResourceType.IMMUNIZATION; 3665 if ("MeasureReport".equals(codeString)) 3666 return EventResourceType.MEASUREREPORT; 3667 if ("Media".equals(codeString)) 3668 return EventResourceType.MEDIA; 3669 if ("MedicationAdministration".equals(codeString)) 3670 return EventResourceType.MEDICATIONADMINISTRATION; 3671 if ("MedicationDispense".equals(codeString)) 3672 return EventResourceType.MEDICATIONDISPENSE; 3673 if ("MedicationStatement".equals(codeString)) 3674 return EventResourceType.MEDICATIONSTATEMENT; 3675 if ("Observation".equals(codeString)) 3676 return EventResourceType.OBSERVATION; 3677 if ("PaymentNotice".equals(codeString)) 3678 return EventResourceType.PAYMENTNOTICE; 3679 if ("PaymentReconciliation".equals(codeString)) 3680 return EventResourceType.PAYMENTRECONCILIATION; 3681 if ("Procedure".equals(codeString)) 3682 return EventResourceType.PROCEDURE; 3683 if ("ProcessResponse".equals(codeString)) 3684 return EventResourceType.PROCESSRESPONSE; 3685 if ("QuestionnaireResponse".equals(codeString)) 3686 return EventResourceType.QUESTIONNAIRERESPONSE; 3687 if ("RiskAssessment".equals(codeString)) 3688 return EventResourceType.RISKASSESSMENT; 3689 if ("SupplyDelivery".equals(codeString)) 3690 return EventResourceType.SUPPLYDELIVERY; 3691 if ("Task".equals(codeString)) 3692 return EventResourceType.TASK; 3693 throw new IllegalArgumentException("Unknown EventResourceType code '" + codeString + "'"); 3694 } 3695 3696 public Enumeration<EventResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 3697 if (code == null) 3698 return null; 3699 if (code.isEmpty()) 3700 return new Enumeration<EventResourceType>(this, EventResourceType.NULL, code); 3701 String codeString = code.asStringValue(); 3702 if (codeString == null || "".equals(codeString)) 3703 return new Enumeration<EventResourceType>(this, EventResourceType.NULL, code); 3704 if ("ChargeItem".equals(codeString)) 3705 return new Enumeration<EventResourceType>(this, EventResourceType.CHARGEITEM, code); 3706 if ("ClaimResponse".equals(codeString)) 3707 return new Enumeration<EventResourceType>(this, EventResourceType.CLAIMRESPONSE, code); 3708 if ("ClinicalImpression".equals(codeString)) 3709 return new Enumeration<EventResourceType>(this, EventResourceType.CLINICALIMPRESSION, code); 3710 if ("Communication".equals(codeString)) 3711 return new Enumeration<EventResourceType>(this, EventResourceType.COMMUNICATION, code); 3712 if ("Composition".equals(codeString)) 3713 return new Enumeration<EventResourceType>(this, EventResourceType.COMPOSITION, code); 3714 if ("Condition".equals(codeString)) 3715 return new Enumeration<EventResourceType>(this, EventResourceType.CONDITION, code); 3716 if ("Consent".equals(codeString)) 3717 return new Enumeration<EventResourceType>(this, EventResourceType.CONSENT, code); 3718 if ("Coverage".equals(codeString)) 3719 return new Enumeration<EventResourceType>(this, EventResourceType.COVERAGE, code); 3720 if ("DeviceUseStatement".equals(codeString)) 3721 return new Enumeration<EventResourceType>(this, EventResourceType.DEVICEUSESTATEMENT, code); 3722 if ("DiagnosticReport".equals(codeString)) 3723 return new Enumeration<EventResourceType>(this, EventResourceType.DIAGNOSTICREPORT, code); 3724 if ("DocumentManifest".equals(codeString)) 3725 return new Enumeration<EventResourceType>(this, EventResourceType.DOCUMENTMANIFEST, code); 3726 if ("DocumentReference".equals(codeString)) 3727 return new Enumeration<EventResourceType>(this, EventResourceType.DOCUMENTREFERENCE, code); 3728 if ("Encounter".equals(codeString)) 3729 return new Enumeration<EventResourceType>(this, EventResourceType.ENCOUNTER, code); 3730 if ("EnrollmentResponse".equals(codeString)) 3731 return new Enumeration<EventResourceType>(this, EventResourceType.ENROLLMENTRESPONSE, code); 3732 if ("EpisodeOfCare".equals(codeString)) 3733 return new Enumeration<EventResourceType>(this, EventResourceType.EPISODEOFCARE, code); 3734 if ("ExplanationOfBenefit".equals(codeString)) 3735 return new Enumeration<EventResourceType>(this, EventResourceType.EXPLANATIONOFBENEFIT, code); 3736 if ("FamilyMemberHistory".equals(codeString)) 3737 return new Enumeration<EventResourceType>(this, EventResourceType.FAMILYMEMBERHISTORY, code); 3738 if ("GuidanceResponse".equals(codeString)) 3739 return new Enumeration<EventResourceType>(this, EventResourceType.GUIDANCERESPONSE, code); 3740 if ("ImagingStudy".equals(codeString)) 3741 return new Enumeration<EventResourceType>(this, EventResourceType.IMAGINGSTUDY, code); 3742 if ("Immunization".equals(codeString)) 3743 return new Enumeration<EventResourceType>(this, EventResourceType.IMMUNIZATION, code); 3744 if ("MeasureReport".equals(codeString)) 3745 return new Enumeration<EventResourceType>(this, EventResourceType.MEASUREREPORT, code); 3746 if ("Media".equals(codeString)) 3747 return new Enumeration<EventResourceType>(this, EventResourceType.MEDIA, code); 3748 if ("MedicationAdministration".equals(codeString)) 3749 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONADMINISTRATION, code); 3750 if ("MedicationDispense".equals(codeString)) 3751 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONDISPENSE, code); 3752 if ("MedicationStatement".equals(codeString)) 3753 return new Enumeration<EventResourceType>(this, EventResourceType.MEDICATIONSTATEMENT, code); 3754 if ("Observation".equals(codeString)) 3755 return new Enumeration<EventResourceType>(this, EventResourceType.OBSERVATION, code); 3756 if ("PaymentNotice".equals(codeString)) 3757 return new Enumeration<EventResourceType>(this, EventResourceType.PAYMENTNOTICE, code); 3758 if ("PaymentReconciliation".equals(codeString)) 3759 return new Enumeration<EventResourceType>(this, EventResourceType.PAYMENTRECONCILIATION, code); 3760 if ("Procedure".equals(codeString)) 3761 return new Enumeration<EventResourceType>(this, EventResourceType.PROCEDURE, code); 3762 if ("ProcessResponse".equals(codeString)) 3763 return new Enumeration<EventResourceType>(this, EventResourceType.PROCESSRESPONSE, code); 3764 if ("QuestionnaireResponse".equals(codeString)) 3765 return new Enumeration<EventResourceType>(this, EventResourceType.QUESTIONNAIRERESPONSE, code); 3766 if ("RiskAssessment".equals(codeString)) 3767 return new Enumeration<EventResourceType>(this, EventResourceType.RISKASSESSMENT, code); 3768 if ("SupplyDelivery".equals(codeString)) 3769 return new Enumeration<EventResourceType>(this, EventResourceType.SUPPLYDELIVERY, code); 3770 if ("Task".equals(codeString)) 3771 return new Enumeration<EventResourceType>(this, EventResourceType.TASK, code); 3772 throw new FHIRException("Unknown EventResourceType code '" + codeString + "'"); 3773 } 3774 3775 public String toCode(EventResourceType code) { 3776 if (code == EventResourceType.CHARGEITEM) 3777 return "ChargeItem"; 3778 if (code == EventResourceType.CLAIMRESPONSE) 3779 return "ClaimResponse"; 3780 if (code == EventResourceType.CLINICALIMPRESSION) 3781 return "ClinicalImpression"; 3782 if (code == EventResourceType.COMMUNICATION) 3783 return "Communication"; 3784 if (code == EventResourceType.COMPOSITION) 3785 return "Composition"; 3786 if (code == EventResourceType.CONDITION) 3787 return "Condition"; 3788 if (code == EventResourceType.CONSENT) 3789 return "Consent"; 3790 if (code == EventResourceType.COVERAGE) 3791 return "Coverage"; 3792 if (code == EventResourceType.DEVICEUSESTATEMENT) 3793 return "DeviceUseStatement"; 3794 if (code == EventResourceType.DIAGNOSTICREPORT) 3795 return "DiagnosticReport"; 3796 if (code == EventResourceType.DOCUMENTMANIFEST) 3797 return "DocumentManifest"; 3798 if (code == EventResourceType.DOCUMENTREFERENCE) 3799 return "DocumentReference"; 3800 if (code == EventResourceType.ENCOUNTER) 3801 return "Encounter"; 3802 if (code == EventResourceType.ENROLLMENTRESPONSE) 3803 return "EnrollmentResponse"; 3804 if (code == EventResourceType.EPISODEOFCARE) 3805 return "EpisodeOfCare"; 3806 if (code == EventResourceType.EXPLANATIONOFBENEFIT) 3807 return "ExplanationOfBenefit"; 3808 if (code == EventResourceType.FAMILYMEMBERHISTORY) 3809 return "FamilyMemberHistory"; 3810 if (code == EventResourceType.GUIDANCERESPONSE) 3811 return "GuidanceResponse"; 3812 if (code == EventResourceType.IMAGINGSTUDY) 3813 return "ImagingStudy"; 3814 if (code == EventResourceType.IMMUNIZATION) 3815 return "Immunization"; 3816 if (code == EventResourceType.MEASUREREPORT) 3817 return "MeasureReport"; 3818 if (code == EventResourceType.MEDIA) 3819 return "Media"; 3820 if (code == EventResourceType.MEDICATIONADMINISTRATION) 3821 return "MedicationAdministration"; 3822 if (code == EventResourceType.MEDICATIONDISPENSE) 3823 return "MedicationDispense"; 3824 if (code == EventResourceType.MEDICATIONSTATEMENT) 3825 return "MedicationStatement"; 3826 if (code == EventResourceType.OBSERVATION) 3827 return "Observation"; 3828 if (code == EventResourceType.PAYMENTNOTICE) 3829 return "PaymentNotice"; 3830 if (code == EventResourceType.PAYMENTRECONCILIATION) 3831 return "PaymentReconciliation"; 3832 if (code == EventResourceType.PROCEDURE) 3833 return "Procedure"; 3834 if (code == EventResourceType.PROCESSRESPONSE) 3835 return "ProcessResponse"; 3836 if (code == EventResourceType.QUESTIONNAIRERESPONSE) 3837 return "QuestionnaireResponse"; 3838 if (code == EventResourceType.RISKASSESSMENT) 3839 return "RiskAssessment"; 3840 if (code == EventResourceType.SUPPLYDELIVERY) 3841 return "SupplyDelivery"; 3842 if (code == EventResourceType.TASK) 3843 return "Task"; 3844 return "?"; 3845 } 3846 3847 public String toSystem(EventResourceType code) { 3848 return code.getSystem(); 3849 } 3850 } 3851 3852 public enum FHIRAllTypes { 3853 /** 3854 * An address expressed using postal conventions (as opposed to GPS or other 3855 * location definition formats). This data type may be used to convey addresses 3856 * for use in delivering mail as well as for visiting locations which might not 3857 * be valid for mail delivery. There are a variety of postal address formats 3858 * defined around the world. 3859 */ 3860 ADDRESS, 3861 /** 3862 * A duration of time during which an organism (or a process) has existed. 3863 */ 3864 AGE, 3865 /** 3866 * A text note which also contains information about who made the statement and 3867 * when. 3868 */ 3869 ANNOTATION, 3870 /** 3871 * For referring to data content defined in other formats. 3872 */ 3873 ATTACHMENT, 3874 /** 3875 * Base definition for all elements that are defined inside a resource - but not 3876 * those in a data type. 3877 */ 3878 BACKBONEELEMENT, 3879 /** 3880 * A concept that may be defined by a formal reference to a terminology or 3881 * ontology or may be provided by text. 3882 */ 3883 CODEABLECONCEPT, 3884 /** 3885 * A reference to a code defined by a terminology system. 3886 */ 3887 CODING, 3888 /** 3889 * Specifies contact information for a person or organization. 3890 */ 3891 CONTACTDETAIL, 3892 /** 3893 * Details for all kinds of technology mediated contact points for a person or 3894 * organization, including telephone, email, etc. 3895 */ 3896 CONTACTPOINT, 3897 /** 3898 * A contributor to the content of a knowledge asset, including authors, 3899 * editors, reviewers, and endorsers. 3900 */ 3901 CONTRIBUTOR, 3902 /** 3903 * A measured amount (or an amount that can potentially be measured). Note that 3904 * measured amounts include amounts that are not precisely quantified, including 3905 * amounts involving arbitrary units and floating currencies. 3906 */ 3907 COUNT, 3908 /** 3909 * Describes a required data item for evaluation in terms of the type of data, 3910 * and optional code or date-based filters of the data. 3911 */ 3912 DATAREQUIREMENT, 3913 /** 3914 * A length - a value with a unit that is a physical distance. 3915 */ 3916 DISTANCE, 3917 /** 3918 * Indicates how the medication is/was taken or should be taken by the patient. 3919 */ 3920 DOSAGE, 3921 /** 3922 * A length of time. 3923 */ 3924 DURATION, 3925 /** 3926 * Base definition for all elements in a resource. 3927 */ 3928 ELEMENT, 3929 /** 3930 * Captures constraints on each element within the resource, profile, or 3931 * extension. 3932 */ 3933 ELEMENTDEFINITION, 3934 /** 3935 * A expression that is evaluated in a specified context and returns a value. 3936 * The context of use of the expression must specify the context in which the 3937 * expression is evaluated, and how the result of the expression is used. 3938 */ 3939 EXPRESSION, 3940 /** 3941 * Optional Extension Element - found in all resources. 3942 */ 3943 EXTENSION, 3944 /** 3945 * A human's name with the ability to identify parts and usage. 3946 */ 3947 HUMANNAME, 3948 /** 3949 * An identifier - identifies some entity uniquely and unambiguously. Typically 3950 * this is used for business identifiers. 3951 */ 3952 IDENTIFIER, 3953 /** 3954 * The marketing status describes the date when a medicinal product is actually 3955 * put on the market or the date as of which it is no longer available. 3956 */ 3957 MARKETINGSTATUS, 3958 /** 3959 * The metadata about a resource. This is content in the resource that is 3960 * maintained by the infrastructure. Changes to the content might not always be 3961 * associated with version changes to the resource. 3962 */ 3963 META, 3964 /** 3965 * An amount of economic utility in some recognized currency. 3966 */ 3967 MONEY, 3968 /** 3969 * null 3970 */ 3971 MONEYQUANTITY, 3972 /** 3973 * A human-readable summary of the resource conveying the essential clinical and 3974 * business information for the resource. 3975 */ 3976 NARRATIVE, 3977 /** 3978 * The parameters to the module. This collection specifies both the input and 3979 * output parameters. Input parameters are provided by the caller as part of the 3980 * $evaluate operation. Output parameters are included in the GuidanceResponse. 3981 */ 3982 PARAMETERDEFINITION, 3983 /** 3984 * A time period defined by a start and end date and optionally time. 3985 */ 3986 PERIOD, 3987 /** 3988 * A populatioof people with some set of grouping criteria. 3989 */ 3990 POPULATION, 3991 /** 3992 * The marketing status describes the date when a medicinal product is actually 3993 * put on the market or the date as of which it is no longer available. 3994 */ 3995 PRODCHARACTERISTIC, 3996 /** 3997 * The shelf-life and storage information for a medicinal product item or 3998 * container can be described using this class. 3999 */ 4000 PRODUCTSHELFLIFE, 4001 /** 4002 * A measured amount (or an amount that can potentially be measured). Note that 4003 * measured amounts include amounts that are not precisely quantified, including 4004 * amounts involving arbitrary units and floating currencies. 4005 */ 4006 QUANTITY, 4007 /** 4008 * A set of ordered Quantities defined by a low and high limit. 4009 */ 4010 RANGE, 4011 /** 4012 * A relationship of two Quantity values - expressed as a numerator and a 4013 * denominator. 4014 */ 4015 RATIO, 4016 /** 4017 * A reference from one resource to another. 4018 */ 4019 REFERENCE, 4020 /** 4021 * Related artifacts such as additional documentation, justification, or 4022 * bibliographic references. 4023 */ 4024 RELATEDARTIFACT, 4025 /** 4026 * A series of measurements taken by a device, with upper and lower limits. 4027 * There may be more than one dimension in the data. 4028 */ 4029 SAMPLEDDATA, 4030 /** 4031 * A signature along with supporting context. The signature may be a digital 4032 * signature that is cryptographic in nature, or some other signature acceptable 4033 * to the domain. This other signature may be as simple as a graphical image 4034 * representing a hand-written signature, or a signature ceremony Different 4035 * signature approaches have different utilities. 4036 */ 4037 SIGNATURE, 4038 /** 4039 * null 4040 */ 4041 SIMPLEQUANTITY, 4042 /** 4043 * Chemical substances are a single substance type whose primary defining 4044 * element is the molecular structure. Chemical substances shall be defined on 4045 * the basis of their complete covalent molecular structure; the presence of a 4046 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 4047 * Purity, grade, physical form or particle size are not taken into account in 4048 * the definition of a chemical substance or in the assignment of a Substance 4049 * ID. 4050 */ 4051 SUBSTANCEAMOUNT, 4052 /** 4053 * Specifies an event that may occur multiple times. Timing schedules are used 4054 * to record when things are planned, expected or requested to occur. The most 4055 * common usage is in dosage instructions for medications. They are also used 4056 * when planning care of various kinds, and may be used for reporting the 4057 * schedule to which past regular activities were carried out. 4058 */ 4059 TIMING, 4060 /** 4061 * A description of a triggering event. Triggering events can be named events, 4062 * data events, or periodic, as determined by the type element. 4063 */ 4064 TRIGGERDEFINITION, 4065 /** 4066 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 4067 * and/or categorize an artifact. This metadata can either be specific to the 4068 * applicable population (e.g., age category, DRG) or the specific context of 4069 * care (e.g., venue, care setting, provider of care). 4070 */ 4071 USAGECONTEXT, 4072 /** 4073 * A stream of bytes 4074 */ 4075 BASE64BINARY, 4076 /** 4077 * Value of "true" or "false" 4078 */ 4079 BOOLEAN, 4080 /** 4081 * A URI that is a reference to a canonical URL on a FHIR resource 4082 */ 4083 CANONICAL, 4084 /** 4085 * A string which has at least one character and no leading or trailing 4086 * whitespace and where there is no whitespace other than single spaces in the 4087 * contents 4088 */ 4089 CODE, 4090 /** 4091 * A date or partial date (e.g. just year or year + month). There is no time 4092 * zone. The format is a union of the schema types gYear, gYearMonth and date. 4093 * Dates SHALL be valid dates. 4094 */ 4095 DATE, 4096 /** 4097 * A date, date-time or partial date (e.g. just year or year + month). If hours 4098 * and minutes are specified, a time zone SHALL be populated. The format is a 4099 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 4100 * be provided due to schema type constraints but may be zero-filled and may be 4101 * ignored. Dates SHALL be valid dates. 4102 */ 4103 DATETIME, 4104 /** 4105 * A rational number with implicit precision 4106 */ 4107 DECIMAL, 4108 /** 4109 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 4110 * characters. (This might be an integer, an unprefixed OID, UUID or any other 4111 * identifier pattern that meets these constraints.) Ids are case-insensitive. 4112 */ 4113 ID, 4114 /** 4115 * An instant in time - known at least to the second 4116 */ 4117 INSTANT, 4118 /** 4119 * A whole number 4120 */ 4121 INTEGER, 4122 /** 4123 * A string that may contain Github Flavored Markdown syntax for optional 4124 * processing by a mark down presentation engine 4125 */ 4126 MARKDOWN, 4127 /** 4128 * An OID represented as a URI 4129 */ 4130 OID, 4131 /** 4132 * An integer with a value that is positive (e.g. >0) 4133 */ 4134 POSITIVEINT, 4135 /** 4136 * A sequence of Unicode characters 4137 */ 4138 STRING, 4139 /** 4140 * A time during the day, with no date specified 4141 */ 4142 TIME, 4143 /** 4144 * An integer with a value that is not negative (e.g. >= 0) 4145 */ 4146 UNSIGNEDINT, 4147 /** 4148 * String of characters used to identify a name or a resource 4149 */ 4150 URI, 4151 /** 4152 * A URI that is a literal reference 4153 */ 4154 URL, 4155 /** 4156 * A UUID, represented as a URI 4157 */ 4158 UUID, 4159 /** 4160 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 4161 * content) 4162 */ 4163 XHTML, 4164 /** 4165 * A financial tool for tracking value accrued for a particular purpose. In the 4166 * healthcare field, used to track charges for a patient, cost centers, etc. 4167 */ 4168 ACCOUNT, 4169 /** 4170 * This resource allows for the definition of some activity to be performed, 4171 * independent of a particular patient, practitioner, or other performance 4172 * context. 4173 */ 4174 ACTIVITYDEFINITION, 4175 /** 4176 * Actual or potential/avoided event causing unintended physical injury 4177 * resulting from or contributed to by medical care, a research study or other 4178 * healthcare setting factors that requires additional monitoring, treatment, or 4179 * hospitalization, or that results in death. 4180 */ 4181 ADVERSEEVENT, 4182 /** 4183 * Risk of harmful or undesirable, physiological response which is unique to an 4184 * individual and associated with exposure to a substance. 4185 */ 4186 ALLERGYINTOLERANCE, 4187 /** 4188 * A booking of a healthcare event among patient(s), practitioner(s), related 4189 * person(s) and/or device(s) for a specific date/time. This may result in one 4190 * or more Encounter(s). 4191 */ 4192 APPOINTMENT, 4193 /** 4194 * A reply to an appointment request for a patient and/or practitioner(s), such 4195 * as a confirmation or rejection. 4196 */ 4197 APPOINTMENTRESPONSE, 4198 /** 4199 * A record of an event made for purposes of maintaining a security log. Typical 4200 * uses include detection of intrusion attempts and monitoring for inappropriate 4201 * usage. 4202 */ 4203 AUDITEVENT, 4204 /** 4205 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 4206 * resources that don't map to an existing resource, and custom resources not 4207 * appropriate for inclusion in the FHIR specification. 4208 */ 4209 BASIC, 4210 /** 4211 * A resource that represents the data of a single raw artifact as digital 4212 * content accessible in its native format. A Binary resource can contain any 4213 * content, whether text, image, pdf, zip archive, etc. 4214 */ 4215 BINARY, 4216 /** 4217 * A material substance originating from a biological entity intended to be 4218 * transplanted or infused into another (possibly the same) biological entity. 4219 */ 4220 BIOLOGICALLYDERIVEDPRODUCT, 4221 /** 4222 * Record details about an anatomical structure. This resource may be used when 4223 * a coded concept does not provide the necessary detail needed for the use 4224 * case. 4225 */ 4226 BODYSTRUCTURE, 4227 /** 4228 * A container for a collection of resources. 4229 */ 4230 BUNDLE, 4231 /** 4232 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 4233 * Server for a particular version of FHIR that may be used as a statement of 4234 * actual server functionality or a statement of required or desired server 4235 * implementation. 4236 */ 4237 CAPABILITYSTATEMENT, 4238 /** 4239 * Describes the intention of how one or more practitioners intend to deliver 4240 * care for a particular patient, group or community for a period of time, 4241 * possibly limited to care for a specific condition or set of conditions. 4242 */ 4243 CAREPLAN, 4244 /** 4245 * The Care Team includes all the people and organizations who plan to 4246 * participate in the coordination and delivery of care for a patient. 4247 */ 4248 CARETEAM, 4249 /** 4250 * Catalog entries are wrappers that contextualize items included in a catalog. 4251 */ 4252 CATALOGENTRY, 4253 /** 4254 * The resource ChargeItem describes the provision of healthcare provider 4255 * products for a certain patient, therefore referring not only to the product, 4256 * but containing in addition details of the provision, like date, time, amounts 4257 * and participating organizations and persons. Main Usage of the ChargeItem is 4258 * to enable the billing process and internal cost allocation. 4259 */ 4260 CHARGEITEM, 4261 /** 4262 * The ChargeItemDefinition resource provides the properties that apply to the 4263 * (billing) codes necessary to calculate costs and prices. The properties may 4264 * differ largely depending on type and realm, therefore this resource gives 4265 * only a rough structure and requires profiling for each type of billing code 4266 * system. 4267 */ 4268 CHARGEITEMDEFINITION, 4269 /** 4270 * A provider issued list of professional services and products which have been 4271 * provided, or are to be provided, to a patient which is sent to an insurer for 4272 * reimbursement. 4273 */ 4274 CLAIM, 4275 /** 4276 * This resource provides the adjudication details from the processing of a 4277 * Claim resource. 4278 */ 4279 CLAIMRESPONSE, 4280 /** 4281 * A record of a clinical assessment performed to determine what problem(s) may 4282 * affect the patient and before planning the treatments or management 4283 * strategies that are best to manage a patient's condition. Assessments are 4284 * often 1:1 with a clinical consultation / encounter, but this varies greatly 4285 * depending on the clinical workflow. This resource is called 4286 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 4287 * the recording of assessment tools such as Apgar score. 4288 */ 4289 CLINICALIMPRESSION, 4290 /** 4291 * The CodeSystem resource is used to declare the existence of and describe a 4292 * code system or code system supplement and its key properties, and optionally 4293 * define a part or all of its content. 4294 */ 4295 CODESYSTEM, 4296 /** 4297 * An occurrence of information being transmitted; e.g. an alert that was sent 4298 * to a responsible provider, a public health agency that was notified about a 4299 * reportable condition. 4300 */ 4301 COMMUNICATION, 4302 /** 4303 * A request to convey information; e.g. the CDS system proposes that an alert 4304 * be sent to a responsible provider, the CDS system proposes that the public 4305 * health agency be notified about a reportable condition. 4306 */ 4307 COMMUNICATIONREQUEST, 4308 /** 4309 * A compartment definition that defines how resources are accessed on a server. 4310 */ 4311 COMPARTMENTDEFINITION, 4312 /** 4313 * A set of healthcare-related information that is assembled together into a 4314 * single logical package that provides a single coherent statement of meaning, 4315 * establishes its own context and that has clinical attestation with regard to 4316 * who is making the statement. A Composition defines the structure and 4317 * narrative content necessary for a document. However, a Composition alone does 4318 * not constitute a document. Rather, the Composition must be the first entry in 4319 * a Bundle where Bundle.type=document, and any other resources referenced from 4320 * Composition must be included as subsequent entries in the Bundle (for example 4321 * Patient, Practitioner, Encounter, etc.). 4322 */ 4323 COMPOSITION, 4324 /** 4325 * A statement of relationships from one set of concepts to one or more other 4326 * concepts - either concepts in code systems, or data element/data element 4327 * concepts, or classes in class models. 4328 */ 4329 CONCEPTMAP, 4330 /** 4331 * A clinical condition, problem, diagnosis, or other event, situation, issue, 4332 * or clinical concept that has risen to a level of concern. 4333 */ 4334 CONDITION, 4335 /** 4336 * A record of a healthcare consumer?s choices, which permits or denies 4337 * identified recipient(s) or recipient role(s) to perform one or more actions 4338 * within a given policy context, for specific purposes and periods of time. 4339 */ 4340 CONSENT, 4341 /** 4342 * Legally enforceable, formally recorded unilateral or bilateral directive 4343 * i.e., a policy or agreement. 4344 */ 4345 CONTRACT, 4346 /** 4347 * Financial instrument which may be used to reimburse or pay for health care 4348 * products and services. Includes both insurance and self-payment. 4349 */ 4350 COVERAGE, 4351 /** 4352 * The CoverageEligibilityRequest provides patient and insurance coverage 4353 * information to an insurer for them to respond, in the form of an 4354 * CoverageEligibilityResponse, with information regarding whether the stated 4355 * coverage is valid and in-force and optionally to provide the insurance 4356 * details of the policy. 4357 */ 4358 COVERAGEELIGIBILITYREQUEST, 4359 /** 4360 * This resource provides eligibility and plan details from the processing of an 4361 * CoverageEligibilityRequest resource. 4362 */ 4363 COVERAGEELIGIBILITYRESPONSE, 4364 /** 4365 * Indicates an actual or potential clinical issue with or between one or more 4366 * active or proposed clinical actions for a patient; e.g. Drug-drug 4367 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 4368 * etc. 4369 */ 4370 DETECTEDISSUE, 4371 /** 4372 * A type of a manufactured item that is used in the provision of healthcare 4373 * without being substantially changed through that activity. The device may be 4374 * a medical or non-medical device. 4375 */ 4376 DEVICE, 4377 /** 4378 * The characteristics, operational status and capabilities of a medical-related 4379 * component of a medical device. 4380 */ 4381 DEVICEDEFINITION, 4382 /** 4383 * Describes a measurement, calculation or setting capability of a medical 4384 * device. 4385 */ 4386 DEVICEMETRIC, 4387 /** 4388 * Represents a request for a patient to employ a medical device. The device may 4389 * be an implantable device, or an external assistive device, such as a walker. 4390 */ 4391 DEVICEREQUEST, 4392 /** 4393 * A record of a device being used by a patient where the record is the result 4394 * of a report from the patient or another clinician. 4395 */ 4396 DEVICEUSESTATEMENT, 4397 /** 4398 * The findings and interpretation of diagnostic tests performed on patients, 4399 * groups of patients, devices, and locations, and/or specimens derived from 4400 * these. The report includes clinical context such as requesting and provider 4401 * information, and some mix of atomic results, images, textual and coded 4402 * interpretations, and formatted representation of diagnostic reports. 4403 */ 4404 DIAGNOSTICREPORT, 4405 /** 4406 * A collection of documents compiled for a purpose together with metadata that 4407 * applies to the collection. 4408 */ 4409 DOCUMENTMANIFEST, 4410 /** 4411 * A reference to a document of any kind for any purpose. Provides metadata 4412 * about the document so that the document can be discovered and managed. The 4413 * scope of a document is any seralized object with a mime-type, so includes 4414 * formal patient centric documents (CDA), cliical notes, scanned paper, and 4415 * non-patient specific documents like policy text. 4416 */ 4417 DOCUMENTREFERENCE, 4418 /** 4419 * A resource that includes narrative, extensions, and contained resources. 4420 */ 4421 DOMAINRESOURCE, 4422 /** 4423 * The EffectEvidenceSynthesis resource describes the difference in an outcome 4424 * between exposures states in a population where the effect estimate is derived 4425 * from a combination of research studies. 4426 */ 4427 EFFECTEVIDENCESYNTHESIS, 4428 /** 4429 * An interaction between a patient and healthcare provider(s) for the purpose 4430 * of providing healthcare service(s) or assessing the health status of a 4431 * patient. 4432 */ 4433 ENCOUNTER, 4434 /** 4435 * The technical details of an endpoint that can be used for electronic 4436 * services, such as for web services providing XDS.b or a REST endpoint for 4437 * another FHIR server. This may include any security context information. 4438 */ 4439 ENDPOINT, 4440 /** 4441 * This resource provides the insurance enrollment details to the insurer 4442 * regarding a specified coverage. 4443 */ 4444 ENROLLMENTREQUEST, 4445 /** 4446 * This resource provides enrollment and plan details from the processing of an 4447 * EnrollmentRequest resource. 4448 */ 4449 ENROLLMENTRESPONSE, 4450 /** 4451 * An association between a patient and an organization / healthcare provider(s) 4452 * during which time encounters may occur. The managing organization assumes a 4453 * level of responsibility for the patient during this time. 4454 */ 4455 EPISODEOFCARE, 4456 /** 4457 * The EventDefinition resource provides a reusable description of when a 4458 * particular event can occur. 4459 */ 4460 EVENTDEFINITION, 4461 /** 4462 * The Evidence resource describes the conditional state (population and any 4463 * exposures being compared within the population) and outcome (if specified) 4464 * that the knowledge (evidence, assertion, recommendation) is about. 4465 */ 4466 EVIDENCE, 4467 /** 4468 * The EvidenceVariable resource describes a "PICO" element that knowledge 4469 * (evidence, assertion, recommendation) is about. 4470 */ 4471 EVIDENCEVARIABLE, 4472 /** 4473 * Example of workflow instance. 4474 */ 4475 EXAMPLESCENARIO, 4476 /** 4477 * This resource provides: the claim details; adjudication details from the 4478 * processing of a Claim; and optionally account balance information, for 4479 * informing the subscriber of the benefits provided. 4480 */ 4481 EXPLANATIONOFBENEFIT, 4482 /** 4483 * Significant health conditions for a person related to the patient relevant in 4484 * the context of care for the patient. 4485 */ 4486 FAMILYMEMBERHISTORY, 4487 /** 4488 * Prospective warnings of potential issues when providing care to the patient. 4489 */ 4490 FLAG, 4491 /** 4492 * Describes the intended objective(s) for a patient, group or organization 4493 * care, for example, weight loss, restoring an activity of daily living, 4494 * obtaining herd immunity via immunization, meeting a process improvement 4495 * objective, etc. 4496 */ 4497 GOAL, 4498 /** 4499 * A formal computable definition of a graph of resources - that is, a coherent 4500 * set of resources that form a graph by following references. The Graph 4501 * Definition resource defines a set and makes rules about the set. 4502 */ 4503 GRAPHDEFINITION, 4504 /** 4505 * Represents a defined collection of entities that may be discussed or acted 4506 * upon collectively but which are not expected to act collectively, and are not 4507 * formally or legally recognized; i.e. a collection of entities that isn't an 4508 * Organization. 4509 */ 4510 GROUP, 4511 /** 4512 * A guidance response is the formal response to a guidance request, including 4513 * any output parameters returned by the evaluation, as well as the description 4514 * of any proposed actions to be taken. 4515 */ 4516 GUIDANCERESPONSE, 4517 /** 4518 * The details of a healthcare service available at a location. 4519 */ 4520 HEALTHCARESERVICE, 4521 /** 4522 * Representation of the content produced in a DICOM imaging study. A study 4523 * comprises a set of series, each of which includes a set of Service-Object 4524 * Pair Instances (SOP Instances - images or other data) acquired or produced in 4525 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 4526 * ultrasound), but a study may have multiple series of different modalities. 4527 */ 4528 IMAGINGSTUDY, 4529 /** 4530 * Describes the event of a patient being administered a vaccine or a record of 4531 * an immunization as reported by a patient, a clinician or another party. 4532 */ 4533 IMMUNIZATION, 4534 /** 4535 * Describes a comparison of an immunization event against published 4536 * recommendations to determine if the administration is "valid" in relation to 4537 * those recommendations. 4538 */ 4539 IMMUNIZATIONEVALUATION, 4540 /** 4541 * A patient's point-in-time set of recommendations (i.e. forecasting) according 4542 * to a published schedule with optional supporting justification. 4543 */ 4544 IMMUNIZATIONRECOMMENDATION, 4545 /** 4546 * A set of rules of how a particular interoperability or standards problem is 4547 * solved - typically through the use of FHIR resources. This resource is used 4548 * to gather all the parts of an implementation guide into a logical whole and 4549 * to publish a computable definition of all the parts. 4550 */ 4551 IMPLEMENTATIONGUIDE, 4552 /** 4553 * Details of a Health Insurance product/plan provided by an organization. 4554 */ 4555 INSURANCEPLAN, 4556 /** 4557 * Invoice containing collected ChargeItems from an Account with calculated 4558 * individual and total price for Billing purpose. 4559 */ 4560 INVOICE, 4561 /** 4562 * The Library resource is a general-purpose container for knowledge asset 4563 * definitions. It can be used to describe and expose existing knowledge assets 4564 * such as logic libraries and information model descriptions, as well as to 4565 * describe a collection of knowledge assets. 4566 */ 4567 LIBRARY, 4568 /** 4569 * Identifies two or more records (resource instances) that refer to the same 4570 * real-world "occurrence". 4571 */ 4572 LINKAGE, 4573 /** 4574 * A list is a curated collection of resources. 4575 */ 4576 LIST, 4577 /** 4578 * Details and position information for a physical place where services are 4579 * provided and resources and participants may be stored, found, contained, or 4580 * accommodated. 4581 */ 4582 LOCATION, 4583 /** 4584 * The Measure resource provides the definition of a quality measure. 4585 */ 4586 MEASURE, 4587 /** 4588 * The MeasureReport resource contains the results of the calculation of a 4589 * measure; and optionally a reference to the resources involved in that 4590 * calculation. 4591 */ 4592 MEASUREREPORT, 4593 /** 4594 * A photo, video, or audio recording acquired or used in healthcare. The actual 4595 * content may be inline or provided by direct reference. 4596 */ 4597 MEDIA, 4598 /** 4599 * This resource is primarily used for the identification and definition of a 4600 * medication for the purposes of prescribing, dispensing, and administering a 4601 * medication as well as for making statements about medication use. 4602 */ 4603 MEDICATION, 4604 /** 4605 * Describes the event of a patient consuming or otherwise being administered a 4606 * medication. This may be as simple as swallowing a tablet or it may be a long 4607 * running infusion. Related resources tie this event to the authorizing 4608 * prescription, and the specific encounter between patient and health care 4609 * practitioner. 4610 */ 4611 MEDICATIONADMINISTRATION, 4612 /** 4613 * Indicates that a medication product is to be or has been dispensed for a 4614 * named person/patient. This includes a description of the medication product 4615 * (supply) provided and the instructions for administering the medication. The 4616 * medication dispense is the result of a pharmacy system responding to a 4617 * medication order. 4618 */ 4619 MEDICATIONDISPENSE, 4620 /** 4621 * Information about a medication that is used to support knowledge. 4622 */ 4623 MEDICATIONKNOWLEDGE, 4624 /** 4625 * An order or request for both supply of the medication and the instructions 4626 * for administration of the medication to a patient. The resource is called 4627 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 4628 * to generalize the use across inpatient and outpatient settings, including 4629 * care plans, etc., and to harmonize with workflow patterns. 4630 */ 4631 MEDICATIONREQUEST, 4632 /** 4633 * A record of a medication that is being consumed by a patient. A 4634 * MedicationStatement may indicate that the patient may be taking the 4635 * medication now or has taken the medication in the past or will be taking the 4636 * medication in the future. The source of this information can be the patient, 4637 * significant other (such as a family member or spouse), or a clinician. A 4638 * common scenario where this information is captured is during the history 4639 * taking process during a patient visit or stay. The medication information may 4640 * come from sources such as the patient's memory, from a prescription bottle, 4641 * or from a list of medications the patient, clinician or other party 4642 * maintains. 4643 * 4644 * The primary difference between a medication statement and a medication 4645 * administration is that the medication administration has complete 4646 * administration information and is based on actual administration information 4647 * from the person who administered the medication. A medication statement is 4648 * often, if not always, less specific. There is no required date/time when the 4649 * medication was administered, in fact we only know that a source has reported 4650 * the patient is taking this medication, where details such as time, quantity, 4651 * or rate or even medication product may be incomplete or missing or less 4652 * precise. As stated earlier, the medication statement information may come 4653 * from the patient's memory, from a prescription bottle or from a list of 4654 * medications the patient, clinician or other party maintains. Medication 4655 * administration is more formal and is not missing detailed information. 4656 */ 4657 MEDICATIONSTATEMENT, 4658 /** 4659 * Detailed definition of a medicinal product, typically for uses other than 4660 * direct patient care (e.g. regulatory use). 4661 */ 4662 MEDICINALPRODUCT, 4663 /** 4664 * The regulatory authorization of a medicinal product. 4665 */ 4666 MEDICINALPRODUCTAUTHORIZATION, 4667 /** 4668 * The clinical particulars - indications, contraindications etc. of a medicinal 4669 * product, including for regulatory purposes. 4670 */ 4671 MEDICINALPRODUCTCONTRAINDICATION, 4672 /** 4673 * Indication for the Medicinal Product. 4674 */ 4675 MEDICINALPRODUCTINDICATION, 4676 /** 4677 * An ingredient of a manufactured item or pharmaceutical product. 4678 */ 4679 MEDICINALPRODUCTINGREDIENT, 4680 /** 4681 * The interactions of the medicinal product with other medicinal products, or 4682 * other forms of interactions. 4683 */ 4684 MEDICINALPRODUCTINTERACTION, 4685 /** 4686 * The manufactured item as contained in the packaged medicinal product. 4687 */ 4688 MEDICINALPRODUCTMANUFACTURED, 4689 /** 4690 * A medicinal product in a container or package. 4691 */ 4692 MEDICINALPRODUCTPACKAGED, 4693 /** 4694 * A pharmaceutical product described in terms of its composition and dose form. 4695 */ 4696 MEDICINALPRODUCTPHARMACEUTICAL, 4697 /** 4698 * Describe the undesirable effects of the medicinal product. 4699 */ 4700 MEDICINALPRODUCTUNDESIRABLEEFFECT, 4701 /** 4702 * Defines the characteristics of a message that can be shared between systems, 4703 * including the type of event that initiates the message, the content to be 4704 * transmitted and what response(s), if any, are permitted. 4705 */ 4706 MESSAGEDEFINITION, 4707 /** 4708 * The header for a message exchange that is either requesting or responding to 4709 * an action. The reference(s) that are the subject of the action as well as 4710 * other information related to the action are typically transmitted in a bundle 4711 * in which the MessageHeader resource instance is the first resource in the 4712 * bundle. 4713 */ 4714 MESSAGEHEADER, 4715 /** 4716 * Raw data describing a biological sequence. 4717 */ 4718 MOLECULARSEQUENCE, 4719 /** 4720 * A curated namespace that issues unique symbols within that namespace for the 4721 * identification of concepts, people, devices, etc. Represents a "System" used 4722 * within the Identifier and Coding data types. 4723 */ 4724 NAMINGSYSTEM, 4725 /** 4726 * A request to supply a diet, formula feeding (enteral) or oral nutritional 4727 * supplement to a patient/resident. 4728 */ 4729 NUTRITIONORDER, 4730 /** 4731 * Measurements and simple assertions made about a patient, device or other 4732 * subject. 4733 */ 4734 OBSERVATION, 4735 /** 4736 * Set of definitional characteristics for a kind of observation or measurement 4737 * produced or consumed by an orderable health care service. 4738 */ 4739 OBSERVATIONDEFINITION, 4740 /** 4741 * A formal computable definition of an operation (on the RESTful interface) or 4742 * a named query (using the search interaction). 4743 */ 4744 OPERATIONDEFINITION, 4745 /** 4746 * A collection of error, warning, or information messages that result from a 4747 * system action. 4748 */ 4749 OPERATIONOUTCOME, 4750 /** 4751 * A formally or informally recognized grouping of people or organizations 4752 * formed for the purpose of achieving some form of collective action. Includes 4753 * companies, institutions, corporations, departments, community groups, 4754 * healthcare practice groups, payer/insurer, etc. 4755 */ 4756 ORGANIZATION, 4757 /** 4758 * Defines an affiliation/assotiation/relationship between 2 distinct 4759 * oganizations, that is not a part-of relationship/sub-division relationship. 4760 */ 4761 ORGANIZATIONAFFILIATION, 4762 /** 4763 * This resource is a non-persisted resource used to pass information into and 4764 * back from an [operation](operations.html). It has no other use, and there is 4765 * no RESTful endpoint associated with it. 4766 */ 4767 PARAMETERS, 4768 /** 4769 * Demographics and other administrative information about an individual or 4770 * animal receiving care or other health-related services. 4771 */ 4772 PATIENT, 4773 /** 4774 * This resource provides the status of the payment for goods and services 4775 * rendered, and the request and response resource references. 4776 */ 4777 PAYMENTNOTICE, 4778 /** 4779 * This resource provides the details including amount of a payment and 4780 * allocates the payment items being paid. 4781 */ 4782 PAYMENTRECONCILIATION, 4783 /** 4784 * Demographics and administrative information about a person independent of a 4785 * specific health-related context. 4786 */ 4787 PERSON, 4788 /** 4789 * This resource allows for the definition of various types of plans as a 4790 * sharable, consumable, and executable artifact. The resource is general enough 4791 * to support the description of a broad range of clinical artifacts such as 4792 * clinical decision support rules, order sets and protocols. 4793 */ 4794 PLANDEFINITION, 4795 /** 4796 * A person who is directly or indirectly involved in the provisioning of 4797 * healthcare. 4798 */ 4799 PRACTITIONER, 4800 /** 4801 * A specific set of Roles/Locations/specialties/services that a practitioner 4802 * may perform at an organization for a period of time. 4803 */ 4804 PRACTITIONERROLE, 4805 /** 4806 * An action that is or was performed on or for a patient. This can be a 4807 * physical intervention like an operation, or less invasive like long term 4808 * services, counseling, or hypnotherapy. 4809 */ 4810 PROCEDURE, 4811 /** 4812 * Provenance of a resource is a record that describes entities and processes 4813 * involved in producing and delivering or otherwise influencing that resource. 4814 * Provenance provides a critical foundation for assessing authenticity, 4815 * enabling trust, and allowing reproducibility. Provenance assertions are a 4816 * form of contextual metadata and can themselves become important records with 4817 * their own provenance. Provenance statement indicates clinical significance in 4818 * terms of confidence in authenticity, reliability, and trustworthiness, 4819 * integrity, and stage in lifecycle (e.g. Document Completion - has the 4820 * artifact been legally authenticated), all of which may impact security, 4821 * privacy, and trust policies. 4822 */ 4823 PROVENANCE, 4824 /** 4825 * A structured set of questions intended to guide the collection of answers 4826 * from end-users. Questionnaires provide detailed control over order, 4827 * presentation, phraseology and grouping to allow coherent, consistent data 4828 * collection. 4829 */ 4830 QUESTIONNAIRE, 4831 /** 4832 * A structured set of questions and their answers. The questions are ordered 4833 * and grouped into coherent subsets, corresponding to the structure of the 4834 * grouping of the questionnaire being responded to. 4835 */ 4836 QUESTIONNAIRERESPONSE, 4837 /** 4838 * Information about a person that is involved in the care for a patient, but 4839 * who is not the target of healthcare, nor has a formal responsibility in the 4840 * care process. 4841 */ 4842 RELATEDPERSON, 4843 /** 4844 * A group of related requests that can be used to capture intended activities 4845 * that have inter-dependencies such as "give this medication after that one". 4846 */ 4847 REQUESTGROUP, 4848 /** 4849 * The ResearchDefinition resource describes the conditional state (population 4850 * and any exposures being compared within the population) and outcome (if 4851 * specified) that the knowledge (evidence, assertion, recommendation) is about. 4852 */ 4853 RESEARCHDEFINITION, 4854 /** 4855 * The ResearchElementDefinition resource describes a "PICO" element that 4856 * knowledge (evidence, assertion, recommendation) is about. 4857 */ 4858 RESEARCHELEMENTDEFINITION, 4859 /** 4860 * A process where a researcher or organization plans and then executes a series 4861 * of steps intended to increase the field of healthcare-related knowledge. This 4862 * includes studies of safety, efficacy, comparative effectiveness and other 4863 * information about medications, devices, therapies and other interventional 4864 * and investigative techniques. A ResearchStudy involves the gathering of 4865 * information about human or animal subjects. 4866 */ 4867 RESEARCHSTUDY, 4868 /** 4869 * A physical entity which is the primary unit of operational and/or 4870 * administrative interest in a study. 4871 */ 4872 RESEARCHSUBJECT, 4873 /** 4874 * This is the base resource type for everything. 4875 */ 4876 RESOURCE, 4877 /** 4878 * An assessment of the likely outcome(s) for a patient or other subject as well 4879 * as the likelihood of each outcome. 4880 */ 4881 RISKASSESSMENT, 4882 /** 4883 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 4884 * a population plus exposure state where the risk estimate is derived from a 4885 * combination of research studies. 4886 */ 4887 RISKEVIDENCESYNTHESIS, 4888 /** 4889 * A container for slots of time that may be available for booking appointments. 4890 */ 4891 SCHEDULE, 4892 /** 4893 * A search parameter that defines a named search item that can be used to 4894 * search/filter on a resource. 4895 */ 4896 SEARCHPARAMETER, 4897 /** 4898 * A record of a request for service such as diagnostic investigations, 4899 * treatments, or operations to be performed. 4900 */ 4901 SERVICEREQUEST, 4902 /** 4903 * A slot of time on a schedule that may be available for booking appointments. 4904 */ 4905 SLOT, 4906 /** 4907 * A sample to be used for analysis. 4908 */ 4909 SPECIMEN, 4910 /** 4911 * A kind of specimen with associated set of requirements. 4912 */ 4913 SPECIMENDEFINITION, 4914 /** 4915 * A definition of a FHIR structure. This resource is used to describe the 4916 * underlying resources, data types defined in FHIR, and also for describing 4917 * extensions and constraints on resources and data types. 4918 */ 4919 STRUCTUREDEFINITION, 4920 /** 4921 * A Map of relationships between 2 structures that can be used to transform 4922 * data. 4923 */ 4924 STRUCTUREMAP, 4925 /** 4926 * The subscription resource is used to define a push-based subscription from a 4927 * server to another system. Once a subscription is registered with the server, 4928 * the server checks every resource that is created or updated, and if the 4929 * resource matches the given criteria, it sends a message on the defined 4930 * "channel" so that another system can take an appropriate action. 4931 */ 4932 SUBSCRIPTION, 4933 /** 4934 * A homogeneous material with a definite composition. 4935 */ 4936 SUBSTANCE, 4937 /** 4938 * Nucleic acids are defined by three distinct elements: the base, sugar and 4939 * linkage. Individual substance/moiety IDs will be created for each of these 4940 * elements. The nucleotide sequence will be always entered in the 5?-3? 4941 * direction. 4942 */ 4943 SUBSTANCENUCLEICACID, 4944 /** 4945 * Todo. 4946 */ 4947 SUBSTANCEPOLYMER, 4948 /** 4949 * A SubstanceProtein is defined as a single unit of a linear amino acid 4950 * sequence, or a combination of subunits that are either covalently linked or 4951 * have a defined invariant stoichiometric relationship. This includes all 4952 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 4953 * whether the use is therapeutic or prophylactic. This set of elements will be 4954 * used to describe albumins, coagulation factors, cytokines, growth factors, 4955 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 4956 * vaccines, and immunomodulators. 4957 */ 4958 SUBSTANCEPROTEIN, 4959 /** 4960 * Todo. 4961 */ 4962 SUBSTANCEREFERENCEINFORMATION, 4963 /** 4964 * Source material shall capture information on the taxonomic and anatomical 4965 * origins as well as the fraction of a material that can result in or can be 4966 * modified to form a substance. This set of data elements shall be used to 4967 * define polymer substances isolated from biological matrices. Taxonomic and 4968 * anatomical origins shall be described using a controlled vocabulary as 4969 * required. This information is captured for naturally derived polymers ( . 4970 * starch) and structurally diverse substances. For Organisms belonging to the 4971 * Kingdom Plantae the Substance level defines the fresh material of a single 4972 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 4973 * Herbal preparations, the fraction information will be captured at the 4974 * Substance information level and additional information for herbal extracts 4975 * will be captured at the Specified Substance Group 1 information level. See 4976 * for further explanation the Substance Class: Structurally Diverse and the 4977 * herbal annex. 4978 */ 4979 SUBSTANCESOURCEMATERIAL, 4980 /** 4981 * The detailed description of a substance, typically at a level beyond what is 4982 * used for prescribing. 4983 */ 4984 SUBSTANCESPECIFICATION, 4985 /** 4986 * Record of delivery of what is supplied. 4987 */ 4988 SUPPLYDELIVERY, 4989 /** 4990 * A record of a request for a medication, substance or device used in the 4991 * healthcare setting. 4992 */ 4993 SUPPLYREQUEST, 4994 /** 4995 * A task to be performed. 4996 */ 4997 TASK, 4998 /** 4999 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 5000 * FHIR Server that may be used as a statement of actual server functionality or 5001 * a statement of required or desired server implementation. 5002 */ 5003 TERMINOLOGYCAPABILITIES, 5004 /** 5005 * A summary of information based on the results of executing a TestScript. 5006 */ 5007 TESTREPORT, 5008 /** 5009 * A structured set of tests against a FHIR server or client implementation to 5010 * determine compliance against the FHIR specification. 5011 */ 5012 TESTSCRIPT, 5013 /** 5014 * A ValueSet resource instance specifies a set of codes drawn from one or more 5015 * code systems, intended for use in a particular context. Value sets link 5016 * between [[[CodeSystem]]] definitions and their use in [coded 5017 * elements](terminologies.html). 5018 */ 5019 VALUESET, 5020 /** 5021 * Describes validation requirements, source(s), status and dates for one or 5022 * more elements. 5023 */ 5024 VERIFICATIONRESULT, 5025 /** 5026 * An authorization for the provision of glasses and/or contact lenses to a 5027 * patient. 5028 */ 5029 VISIONPRESCRIPTION, 5030 /** 5031 * A place holder that means any kind of data type 5032 */ 5033 TYPE, 5034 /** 5035 * A place holder that means any kind of resource 5036 */ 5037 ANY, 5038 /** 5039 * added to help the parsers 5040 */ 5041 NULL; 5042 5043 public static FHIRAllTypes fromCode(String codeString) throws FHIRException { 5044 if (codeString == null || "".equals(codeString)) 5045 return null; 5046 if ("Address".equals(codeString)) 5047 return ADDRESS; 5048 if ("Age".equals(codeString)) 5049 return AGE; 5050 if ("Annotation".equals(codeString)) 5051 return ANNOTATION; 5052 if ("Attachment".equals(codeString)) 5053 return ATTACHMENT; 5054 if ("BackboneElement".equals(codeString)) 5055 return BACKBONEELEMENT; 5056 if ("CodeableConcept".equals(codeString)) 5057 return CODEABLECONCEPT; 5058 if ("Coding".equals(codeString)) 5059 return CODING; 5060 if ("ContactDetail".equals(codeString)) 5061 return CONTACTDETAIL; 5062 if ("ContactPoint".equals(codeString)) 5063 return CONTACTPOINT; 5064 if ("Contributor".equals(codeString)) 5065 return CONTRIBUTOR; 5066 if ("Count".equals(codeString)) 5067 return COUNT; 5068 if ("DataRequirement".equals(codeString)) 5069 return DATAREQUIREMENT; 5070 if ("Distance".equals(codeString)) 5071 return DISTANCE; 5072 if ("Dosage".equals(codeString)) 5073 return DOSAGE; 5074 if ("Duration".equals(codeString)) 5075 return DURATION; 5076 if ("Element".equals(codeString)) 5077 return ELEMENT; 5078 if ("ElementDefinition".equals(codeString)) 5079 return ELEMENTDEFINITION; 5080 if ("Expression".equals(codeString)) 5081 return EXPRESSION; 5082 if ("Extension".equals(codeString)) 5083 return EXTENSION; 5084 if ("HumanName".equals(codeString)) 5085 return HUMANNAME; 5086 if ("Identifier".equals(codeString)) 5087 return IDENTIFIER; 5088 if ("MarketingStatus".equals(codeString)) 5089 return MARKETINGSTATUS; 5090 if ("Meta".equals(codeString)) 5091 return META; 5092 if ("Money".equals(codeString)) 5093 return MONEY; 5094 if ("MoneyQuantity".equals(codeString)) 5095 return MONEYQUANTITY; 5096 if ("Narrative".equals(codeString)) 5097 return NARRATIVE; 5098 if ("ParameterDefinition".equals(codeString)) 5099 return PARAMETERDEFINITION; 5100 if ("Period".equals(codeString)) 5101 return PERIOD; 5102 if ("Population".equals(codeString)) 5103 return POPULATION; 5104 if ("ProdCharacteristic".equals(codeString)) 5105 return PRODCHARACTERISTIC; 5106 if ("ProductShelfLife".equals(codeString)) 5107 return PRODUCTSHELFLIFE; 5108 if ("Quantity".equals(codeString)) 5109 return QUANTITY; 5110 if ("Range".equals(codeString)) 5111 return RANGE; 5112 if ("Ratio".equals(codeString)) 5113 return RATIO; 5114 if ("Reference".equals(codeString)) 5115 return REFERENCE; 5116 if ("RelatedArtifact".equals(codeString)) 5117 return RELATEDARTIFACT; 5118 if ("SampledData".equals(codeString)) 5119 return SAMPLEDDATA; 5120 if ("Signature".equals(codeString)) 5121 return SIGNATURE; 5122 if ("SimpleQuantity".equals(codeString)) 5123 return SIMPLEQUANTITY; 5124 if ("SubstanceAmount".equals(codeString)) 5125 return SUBSTANCEAMOUNT; 5126 if ("Timing".equals(codeString)) 5127 return TIMING; 5128 if ("TriggerDefinition".equals(codeString)) 5129 return TRIGGERDEFINITION; 5130 if ("UsageContext".equals(codeString)) 5131 return USAGECONTEXT; 5132 if ("base64Binary".equals(codeString)) 5133 return BASE64BINARY; 5134 if ("boolean".equals(codeString)) 5135 return BOOLEAN; 5136 if ("canonical".equals(codeString)) 5137 return CANONICAL; 5138 if ("code".equals(codeString)) 5139 return CODE; 5140 if ("date".equals(codeString)) 5141 return DATE; 5142 if ("dateTime".equals(codeString)) 5143 return DATETIME; 5144 if ("decimal".equals(codeString)) 5145 return DECIMAL; 5146 if ("id".equals(codeString)) 5147 return ID; 5148 if ("instant".equals(codeString)) 5149 return INSTANT; 5150 if ("integer".equals(codeString)) 5151 return INTEGER; 5152 if ("markdown".equals(codeString)) 5153 return MARKDOWN; 5154 if ("oid".equals(codeString)) 5155 return OID; 5156 if ("positiveInt".equals(codeString)) 5157 return POSITIVEINT; 5158 if ("string".equals(codeString)) 5159 return STRING; 5160 if ("time".equals(codeString)) 5161 return TIME; 5162 if ("unsignedInt".equals(codeString)) 5163 return UNSIGNEDINT; 5164 if ("uri".equals(codeString)) 5165 return URI; 5166 if ("url".equals(codeString)) 5167 return URL; 5168 if ("uuid".equals(codeString)) 5169 return UUID; 5170 if ("xhtml".equals(codeString)) 5171 return XHTML; 5172 if ("Account".equals(codeString)) 5173 return ACCOUNT; 5174 if ("ActivityDefinition".equals(codeString)) 5175 return ACTIVITYDEFINITION; 5176 if ("AdverseEvent".equals(codeString)) 5177 return ADVERSEEVENT; 5178 if ("AllergyIntolerance".equals(codeString)) 5179 return ALLERGYINTOLERANCE; 5180 if ("Appointment".equals(codeString)) 5181 return APPOINTMENT; 5182 if ("AppointmentResponse".equals(codeString)) 5183 return APPOINTMENTRESPONSE; 5184 if ("AuditEvent".equals(codeString)) 5185 return AUDITEVENT; 5186 if ("Basic".equals(codeString)) 5187 return BASIC; 5188 if ("Binary".equals(codeString)) 5189 return BINARY; 5190 if ("BiologicallyDerivedProduct".equals(codeString)) 5191 return BIOLOGICALLYDERIVEDPRODUCT; 5192 if ("BodyStructure".equals(codeString)) 5193 return BODYSTRUCTURE; 5194 if ("Bundle".equals(codeString)) 5195 return BUNDLE; 5196 if ("CapabilityStatement".equals(codeString)) 5197 return CAPABILITYSTATEMENT; 5198 if ("CarePlan".equals(codeString)) 5199 return CAREPLAN; 5200 if ("CareTeam".equals(codeString)) 5201 return CARETEAM; 5202 if ("CatalogEntry".equals(codeString)) 5203 return CATALOGENTRY; 5204 if ("ChargeItem".equals(codeString)) 5205 return CHARGEITEM; 5206 if ("ChargeItemDefinition".equals(codeString)) 5207 return CHARGEITEMDEFINITION; 5208 if ("Claim".equals(codeString)) 5209 return CLAIM; 5210 if ("ClaimResponse".equals(codeString)) 5211 return CLAIMRESPONSE; 5212 if ("ClinicalImpression".equals(codeString)) 5213 return CLINICALIMPRESSION; 5214 if ("CodeSystem".equals(codeString)) 5215 return CODESYSTEM; 5216 if ("Communication".equals(codeString)) 5217 return COMMUNICATION; 5218 if ("CommunicationRequest".equals(codeString)) 5219 return COMMUNICATIONREQUEST; 5220 if ("CompartmentDefinition".equals(codeString)) 5221 return COMPARTMENTDEFINITION; 5222 if ("Composition".equals(codeString)) 5223 return COMPOSITION; 5224 if ("ConceptMap".equals(codeString)) 5225 return CONCEPTMAP; 5226 if ("Condition".equals(codeString)) 5227 return CONDITION; 5228 if ("Consent".equals(codeString)) 5229 return CONSENT; 5230 if ("Contract".equals(codeString)) 5231 return CONTRACT; 5232 if ("Coverage".equals(codeString)) 5233 return COVERAGE; 5234 if ("CoverageEligibilityRequest".equals(codeString)) 5235 return COVERAGEELIGIBILITYREQUEST; 5236 if ("CoverageEligibilityResponse".equals(codeString)) 5237 return COVERAGEELIGIBILITYRESPONSE; 5238 if ("DetectedIssue".equals(codeString)) 5239 return DETECTEDISSUE; 5240 if ("Device".equals(codeString)) 5241 return DEVICE; 5242 if ("DeviceDefinition".equals(codeString)) 5243 return DEVICEDEFINITION; 5244 if ("DeviceMetric".equals(codeString)) 5245 return DEVICEMETRIC; 5246 if ("DeviceRequest".equals(codeString)) 5247 return DEVICEREQUEST; 5248 if ("DeviceUseStatement".equals(codeString)) 5249 return DEVICEUSESTATEMENT; 5250 if ("DiagnosticReport".equals(codeString)) 5251 return DIAGNOSTICREPORT; 5252 if ("DocumentManifest".equals(codeString)) 5253 return DOCUMENTMANIFEST; 5254 if ("DocumentReference".equals(codeString)) 5255 return DOCUMENTREFERENCE; 5256 if ("DomainResource".equals(codeString)) 5257 return DOMAINRESOURCE; 5258 if ("EffectEvidenceSynthesis".equals(codeString)) 5259 return EFFECTEVIDENCESYNTHESIS; 5260 if ("Encounter".equals(codeString)) 5261 return ENCOUNTER; 5262 if ("Endpoint".equals(codeString)) 5263 return ENDPOINT; 5264 if ("EnrollmentRequest".equals(codeString)) 5265 return ENROLLMENTREQUEST; 5266 if ("EnrollmentResponse".equals(codeString)) 5267 return ENROLLMENTRESPONSE; 5268 if ("EpisodeOfCare".equals(codeString)) 5269 return EPISODEOFCARE; 5270 if ("EventDefinition".equals(codeString)) 5271 return EVENTDEFINITION; 5272 if ("Evidence".equals(codeString)) 5273 return EVIDENCE; 5274 if ("EvidenceVariable".equals(codeString)) 5275 return EVIDENCEVARIABLE; 5276 if ("ExampleScenario".equals(codeString)) 5277 return EXAMPLESCENARIO; 5278 if ("ExplanationOfBenefit".equals(codeString)) 5279 return EXPLANATIONOFBENEFIT; 5280 if ("FamilyMemberHistory".equals(codeString)) 5281 return FAMILYMEMBERHISTORY; 5282 if ("Flag".equals(codeString)) 5283 return FLAG; 5284 if ("Goal".equals(codeString)) 5285 return GOAL; 5286 if ("GraphDefinition".equals(codeString)) 5287 return GRAPHDEFINITION; 5288 if ("Group".equals(codeString)) 5289 return GROUP; 5290 if ("GuidanceResponse".equals(codeString)) 5291 return GUIDANCERESPONSE; 5292 if ("HealthcareService".equals(codeString)) 5293 return HEALTHCARESERVICE; 5294 if ("ImagingStudy".equals(codeString)) 5295 return IMAGINGSTUDY; 5296 if ("Immunization".equals(codeString)) 5297 return IMMUNIZATION; 5298 if ("ImmunizationEvaluation".equals(codeString)) 5299 return IMMUNIZATIONEVALUATION; 5300 if ("ImmunizationRecommendation".equals(codeString)) 5301 return IMMUNIZATIONRECOMMENDATION; 5302 if ("ImplementationGuide".equals(codeString)) 5303 return IMPLEMENTATIONGUIDE; 5304 if ("InsurancePlan".equals(codeString)) 5305 return INSURANCEPLAN; 5306 if ("Invoice".equals(codeString)) 5307 return INVOICE; 5308 if ("Library".equals(codeString)) 5309 return LIBRARY; 5310 if ("Linkage".equals(codeString)) 5311 return LINKAGE; 5312 if ("List".equals(codeString)) 5313 return LIST; 5314 if ("Location".equals(codeString)) 5315 return LOCATION; 5316 if ("Measure".equals(codeString)) 5317 return MEASURE; 5318 if ("MeasureReport".equals(codeString)) 5319 return MEASUREREPORT; 5320 if ("Media".equals(codeString)) 5321 return MEDIA; 5322 if ("Medication".equals(codeString)) 5323 return MEDICATION; 5324 if ("MedicationAdministration".equals(codeString)) 5325 return MEDICATIONADMINISTRATION; 5326 if ("MedicationDispense".equals(codeString)) 5327 return MEDICATIONDISPENSE; 5328 if ("MedicationKnowledge".equals(codeString)) 5329 return MEDICATIONKNOWLEDGE; 5330 if ("MedicationRequest".equals(codeString)) 5331 return MEDICATIONREQUEST; 5332 if ("MedicationStatement".equals(codeString)) 5333 return MEDICATIONSTATEMENT; 5334 if ("MedicinalProduct".equals(codeString)) 5335 return MEDICINALPRODUCT; 5336 if ("MedicinalProductAuthorization".equals(codeString)) 5337 return MEDICINALPRODUCTAUTHORIZATION; 5338 if ("MedicinalProductContraindication".equals(codeString)) 5339 return MEDICINALPRODUCTCONTRAINDICATION; 5340 if ("MedicinalProductIndication".equals(codeString)) 5341 return MEDICINALPRODUCTINDICATION; 5342 if ("MedicinalProductIngredient".equals(codeString)) 5343 return MEDICINALPRODUCTINGREDIENT; 5344 if ("MedicinalProductInteraction".equals(codeString)) 5345 return MEDICINALPRODUCTINTERACTION; 5346 if ("MedicinalProductManufactured".equals(codeString)) 5347 return MEDICINALPRODUCTMANUFACTURED; 5348 if ("MedicinalProductPackaged".equals(codeString)) 5349 return MEDICINALPRODUCTPACKAGED; 5350 if ("MedicinalProductPharmaceutical".equals(codeString)) 5351 return MEDICINALPRODUCTPHARMACEUTICAL; 5352 if ("MedicinalProductUndesirableEffect".equals(codeString)) 5353 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 5354 if ("MessageDefinition".equals(codeString)) 5355 return MESSAGEDEFINITION; 5356 if ("MessageHeader".equals(codeString)) 5357 return MESSAGEHEADER; 5358 if ("MolecularSequence".equals(codeString)) 5359 return MOLECULARSEQUENCE; 5360 if ("NamingSystem".equals(codeString)) 5361 return NAMINGSYSTEM; 5362 if ("NutritionOrder".equals(codeString)) 5363 return NUTRITIONORDER; 5364 if ("Observation".equals(codeString)) 5365 return OBSERVATION; 5366 if ("ObservationDefinition".equals(codeString)) 5367 return OBSERVATIONDEFINITION; 5368 if ("OperationDefinition".equals(codeString)) 5369 return OPERATIONDEFINITION; 5370 if ("OperationOutcome".equals(codeString)) 5371 return OPERATIONOUTCOME; 5372 if ("Organization".equals(codeString)) 5373 return ORGANIZATION; 5374 if ("OrganizationAffiliation".equals(codeString)) 5375 return ORGANIZATIONAFFILIATION; 5376 if ("Parameters".equals(codeString)) 5377 return PARAMETERS; 5378 if ("Patient".equals(codeString)) 5379 return PATIENT; 5380 if ("PaymentNotice".equals(codeString)) 5381 return PAYMENTNOTICE; 5382 if ("PaymentReconciliation".equals(codeString)) 5383 return PAYMENTRECONCILIATION; 5384 if ("Person".equals(codeString)) 5385 return PERSON; 5386 if ("PlanDefinition".equals(codeString)) 5387 return PLANDEFINITION; 5388 if ("Practitioner".equals(codeString)) 5389 return PRACTITIONER; 5390 if ("PractitionerRole".equals(codeString)) 5391 return PRACTITIONERROLE; 5392 if ("Procedure".equals(codeString)) 5393 return PROCEDURE; 5394 if ("Provenance".equals(codeString)) 5395 return PROVENANCE; 5396 if ("Questionnaire".equals(codeString)) 5397 return QUESTIONNAIRE; 5398 if ("QuestionnaireResponse".equals(codeString)) 5399 return QUESTIONNAIRERESPONSE; 5400 if ("RelatedPerson".equals(codeString)) 5401 return RELATEDPERSON; 5402 if ("RequestGroup".equals(codeString)) 5403 return REQUESTGROUP; 5404 if ("ResearchDefinition".equals(codeString)) 5405 return RESEARCHDEFINITION; 5406 if ("ResearchElementDefinition".equals(codeString)) 5407 return RESEARCHELEMENTDEFINITION; 5408 if ("ResearchStudy".equals(codeString)) 5409 return RESEARCHSTUDY; 5410 if ("ResearchSubject".equals(codeString)) 5411 return RESEARCHSUBJECT; 5412 if ("Resource".equals(codeString)) 5413 return RESOURCE; 5414 if ("RiskAssessment".equals(codeString)) 5415 return RISKASSESSMENT; 5416 if ("RiskEvidenceSynthesis".equals(codeString)) 5417 return RISKEVIDENCESYNTHESIS; 5418 if ("Schedule".equals(codeString)) 5419 return SCHEDULE; 5420 if ("SearchParameter".equals(codeString)) 5421 return SEARCHPARAMETER; 5422 if ("ServiceRequest".equals(codeString)) 5423 return SERVICEREQUEST; 5424 if ("Slot".equals(codeString)) 5425 return SLOT; 5426 if ("Specimen".equals(codeString)) 5427 return SPECIMEN; 5428 if ("SpecimenDefinition".equals(codeString)) 5429 return SPECIMENDEFINITION; 5430 if ("StructureDefinition".equals(codeString)) 5431 return STRUCTUREDEFINITION; 5432 if ("StructureMap".equals(codeString)) 5433 return STRUCTUREMAP; 5434 if ("Subscription".equals(codeString)) 5435 return SUBSCRIPTION; 5436 if ("Substance".equals(codeString)) 5437 return SUBSTANCE; 5438 if ("SubstanceNucleicAcid".equals(codeString)) 5439 return SUBSTANCENUCLEICACID; 5440 if ("SubstancePolymer".equals(codeString)) 5441 return SUBSTANCEPOLYMER; 5442 if ("SubstanceProtein".equals(codeString)) 5443 return SUBSTANCEPROTEIN; 5444 if ("SubstanceReferenceInformation".equals(codeString)) 5445 return SUBSTANCEREFERENCEINFORMATION; 5446 if ("SubstanceSourceMaterial".equals(codeString)) 5447 return SUBSTANCESOURCEMATERIAL; 5448 if ("SubstanceSpecification".equals(codeString)) 5449 return SUBSTANCESPECIFICATION; 5450 if ("SupplyDelivery".equals(codeString)) 5451 return SUPPLYDELIVERY; 5452 if ("SupplyRequest".equals(codeString)) 5453 return SUPPLYREQUEST; 5454 if ("Task".equals(codeString)) 5455 return TASK; 5456 if ("TerminologyCapabilities".equals(codeString)) 5457 return TERMINOLOGYCAPABILITIES; 5458 if ("TestReport".equals(codeString)) 5459 return TESTREPORT; 5460 if ("TestScript".equals(codeString)) 5461 return TESTSCRIPT; 5462 if ("ValueSet".equals(codeString)) 5463 return VALUESET; 5464 if ("VerificationResult".equals(codeString)) 5465 return VERIFICATIONRESULT; 5466 if ("VisionPrescription".equals(codeString)) 5467 return VISIONPRESCRIPTION; 5468 if ("Type".equals(codeString)) 5469 return TYPE; 5470 if ("Any".equals(codeString)) 5471 return ANY; 5472 throw new FHIRException("Unknown FHIRAllTypes code '" + codeString + "'"); 5473 } 5474 5475 public String toCode() { 5476 switch (this) { 5477 case ADDRESS: 5478 return "Address"; 5479 case AGE: 5480 return "Age"; 5481 case ANNOTATION: 5482 return "Annotation"; 5483 case ATTACHMENT: 5484 return "Attachment"; 5485 case BACKBONEELEMENT: 5486 return "BackboneElement"; 5487 case CODEABLECONCEPT: 5488 return "CodeableConcept"; 5489 case CODING: 5490 return "Coding"; 5491 case CONTACTDETAIL: 5492 return "ContactDetail"; 5493 case CONTACTPOINT: 5494 return "ContactPoint"; 5495 case CONTRIBUTOR: 5496 return "Contributor"; 5497 case COUNT: 5498 return "Count"; 5499 case DATAREQUIREMENT: 5500 return "DataRequirement"; 5501 case DISTANCE: 5502 return "Distance"; 5503 case DOSAGE: 5504 return "Dosage"; 5505 case DURATION: 5506 return "Duration"; 5507 case ELEMENT: 5508 return "Element"; 5509 case ELEMENTDEFINITION: 5510 return "ElementDefinition"; 5511 case EXPRESSION: 5512 return "Expression"; 5513 case EXTENSION: 5514 return "Extension"; 5515 case HUMANNAME: 5516 return "HumanName"; 5517 case IDENTIFIER: 5518 return "Identifier"; 5519 case MARKETINGSTATUS: 5520 return "MarketingStatus"; 5521 case META: 5522 return "Meta"; 5523 case MONEY: 5524 return "Money"; 5525 case MONEYQUANTITY: 5526 return "MoneyQuantity"; 5527 case NARRATIVE: 5528 return "Narrative"; 5529 case PARAMETERDEFINITION: 5530 return "ParameterDefinition"; 5531 case PERIOD: 5532 return "Period"; 5533 case POPULATION: 5534 return "Population"; 5535 case PRODCHARACTERISTIC: 5536 return "ProdCharacteristic"; 5537 case PRODUCTSHELFLIFE: 5538 return "ProductShelfLife"; 5539 case QUANTITY: 5540 return "Quantity"; 5541 case RANGE: 5542 return "Range"; 5543 case RATIO: 5544 return "Ratio"; 5545 case REFERENCE: 5546 return "Reference"; 5547 case RELATEDARTIFACT: 5548 return "RelatedArtifact"; 5549 case SAMPLEDDATA: 5550 return "SampledData"; 5551 case SIGNATURE: 5552 return "Signature"; 5553 case SIMPLEQUANTITY: 5554 return "SimpleQuantity"; 5555 case SUBSTANCEAMOUNT: 5556 return "SubstanceAmount"; 5557 case TIMING: 5558 return "Timing"; 5559 case TRIGGERDEFINITION: 5560 return "TriggerDefinition"; 5561 case USAGECONTEXT: 5562 return "UsageContext"; 5563 case BASE64BINARY: 5564 return "base64Binary"; 5565 case BOOLEAN: 5566 return "boolean"; 5567 case CANONICAL: 5568 return "canonical"; 5569 case CODE: 5570 return "code"; 5571 case DATE: 5572 return "date"; 5573 case DATETIME: 5574 return "dateTime"; 5575 case DECIMAL: 5576 return "decimal"; 5577 case ID: 5578 return "id"; 5579 case INSTANT: 5580 return "instant"; 5581 case INTEGER: 5582 return "integer"; 5583 case MARKDOWN: 5584 return "markdown"; 5585 case OID: 5586 return "oid"; 5587 case POSITIVEINT: 5588 return "positiveInt"; 5589 case STRING: 5590 return "string"; 5591 case TIME: 5592 return "time"; 5593 case UNSIGNEDINT: 5594 return "unsignedInt"; 5595 case URI: 5596 return "uri"; 5597 case URL: 5598 return "url"; 5599 case UUID: 5600 return "uuid"; 5601 case XHTML: 5602 return "xhtml"; 5603 case ACCOUNT: 5604 return "Account"; 5605 case ACTIVITYDEFINITION: 5606 return "ActivityDefinition"; 5607 case ADVERSEEVENT: 5608 return "AdverseEvent"; 5609 case ALLERGYINTOLERANCE: 5610 return "AllergyIntolerance"; 5611 case APPOINTMENT: 5612 return "Appointment"; 5613 case APPOINTMENTRESPONSE: 5614 return "AppointmentResponse"; 5615 case AUDITEVENT: 5616 return "AuditEvent"; 5617 case BASIC: 5618 return "Basic"; 5619 case BINARY: 5620 return "Binary"; 5621 case BIOLOGICALLYDERIVEDPRODUCT: 5622 return "BiologicallyDerivedProduct"; 5623 case BODYSTRUCTURE: 5624 return "BodyStructure"; 5625 case BUNDLE: 5626 return "Bundle"; 5627 case CAPABILITYSTATEMENT: 5628 return "CapabilityStatement"; 5629 case CAREPLAN: 5630 return "CarePlan"; 5631 case CARETEAM: 5632 return "CareTeam"; 5633 case CATALOGENTRY: 5634 return "CatalogEntry"; 5635 case CHARGEITEM: 5636 return "ChargeItem"; 5637 case CHARGEITEMDEFINITION: 5638 return "ChargeItemDefinition"; 5639 case CLAIM: 5640 return "Claim"; 5641 case CLAIMRESPONSE: 5642 return "ClaimResponse"; 5643 case CLINICALIMPRESSION: 5644 return "ClinicalImpression"; 5645 case CODESYSTEM: 5646 return "CodeSystem"; 5647 case COMMUNICATION: 5648 return "Communication"; 5649 case COMMUNICATIONREQUEST: 5650 return "CommunicationRequest"; 5651 case COMPARTMENTDEFINITION: 5652 return "CompartmentDefinition"; 5653 case COMPOSITION: 5654 return "Composition"; 5655 case CONCEPTMAP: 5656 return "ConceptMap"; 5657 case CONDITION: 5658 return "Condition"; 5659 case CONSENT: 5660 return "Consent"; 5661 case CONTRACT: 5662 return "Contract"; 5663 case COVERAGE: 5664 return "Coverage"; 5665 case COVERAGEELIGIBILITYREQUEST: 5666 return "CoverageEligibilityRequest"; 5667 case COVERAGEELIGIBILITYRESPONSE: 5668 return "CoverageEligibilityResponse"; 5669 case DETECTEDISSUE: 5670 return "DetectedIssue"; 5671 case DEVICE: 5672 return "Device"; 5673 case DEVICEDEFINITION: 5674 return "DeviceDefinition"; 5675 case DEVICEMETRIC: 5676 return "DeviceMetric"; 5677 case DEVICEREQUEST: 5678 return "DeviceRequest"; 5679 case DEVICEUSESTATEMENT: 5680 return "DeviceUseStatement"; 5681 case DIAGNOSTICREPORT: 5682 return "DiagnosticReport"; 5683 case DOCUMENTMANIFEST: 5684 return "DocumentManifest"; 5685 case DOCUMENTREFERENCE: 5686 return "DocumentReference"; 5687 case DOMAINRESOURCE: 5688 return "DomainResource"; 5689 case EFFECTEVIDENCESYNTHESIS: 5690 return "EffectEvidenceSynthesis"; 5691 case ENCOUNTER: 5692 return "Encounter"; 5693 case ENDPOINT: 5694 return "Endpoint"; 5695 case ENROLLMENTREQUEST: 5696 return "EnrollmentRequest"; 5697 case ENROLLMENTRESPONSE: 5698 return "EnrollmentResponse"; 5699 case EPISODEOFCARE: 5700 return "EpisodeOfCare"; 5701 case EVENTDEFINITION: 5702 return "EventDefinition"; 5703 case EVIDENCE: 5704 return "Evidence"; 5705 case EVIDENCEVARIABLE: 5706 return "EvidenceVariable"; 5707 case EXAMPLESCENARIO: 5708 return "ExampleScenario"; 5709 case EXPLANATIONOFBENEFIT: 5710 return "ExplanationOfBenefit"; 5711 case FAMILYMEMBERHISTORY: 5712 return "FamilyMemberHistory"; 5713 case FLAG: 5714 return "Flag"; 5715 case GOAL: 5716 return "Goal"; 5717 case GRAPHDEFINITION: 5718 return "GraphDefinition"; 5719 case GROUP: 5720 return "Group"; 5721 case GUIDANCERESPONSE: 5722 return "GuidanceResponse"; 5723 case HEALTHCARESERVICE: 5724 return "HealthcareService"; 5725 case IMAGINGSTUDY: 5726 return "ImagingStudy"; 5727 case IMMUNIZATION: 5728 return "Immunization"; 5729 case IMMUNIZATIONEVALUATION: 5730 return "ImmunizationEvaluation"; 5731 case IMMUNIZATIONRECOMMENDATION: 5732 return "ImmunizationRecommendation"; 5733 case IMPLEMENTATIONGUIDE: 5734 return "ImplementationGuide"; 5735 case INSURANCEPLAN: 5736 return "InsurancePlan"; 5737 case INVOICE: 5738 return "Invoice"; 5739 case LIBRARY: 5740 return "Library"; 5741 case LINKAGE: 5742 return "Linkage"; 5743 case LIST: 5744 return "List"; 5745 case LOCATION: 5746 return "Location"; 5747 case MEASURE: 5748 return "Measure"; 5749 case MEASUREREPORT: 5750 return "MeasureReport"; 5751 case MEDIA: 5752 return "Media"; 5753 case MEDICATION: 5754 return "Medication"; 5755 case MEDICATIONADMINISTRATION: 5756 return "MedicationAdministration"; 5757 case MEDICATIONDISPENSE: 5758 return "MedicationDispense"; 5759 case MEDICATIONKNOWLEDGE: 5760 return "MedicationKnowledge"; 5761 case MEDICATIONREQUEST: 5762 return "MedicationRequest"; 5763 case MEDICATIONSTATEMENT: 5764 return "MedicationStatement"; 5765 case MEDICINALPRODUCT: 5766 return "MedicinalProduct"; 5767 case MEDICINALPRODUCTAUTHORIZATION: 5768 return "MedicinalProductAuthorization"; 5769 case MEDICINALPRODUCTCONTRAINDICATION: 5770 return "MedicinalProductContraindication"; 5771 case MEDICINALPRODUCTINDICATION: 5772 return "MedicinalProductIndication"; 5773 case MEDICINALPRODUCTINGREDIENT: 5774 return "MedicinalProductIngredient"; 5775 case MEDICINALPRODUCTINTERACTION: 5776 return "MedicinalProductInteraction"; 5777 case MEDICINALPRODUCTMANUFACTURED: 5778 return "MedicinalProductManufactured"; 5779 case MEDICINALPRODUCTPACKAGED: 5780 return "MedicinalProductPackaged"; 5781 case MEDICINALPRODUCTPHARMACEUTICAL: 5782 return "MedicinalProductPharmaceutical"; 5783 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 5784 return "MedicinalProductUndesirableEffect"; 5785 case MESSAGEDEFINITION: 5786 return "MessageDefinition"; 5787 case MESSAGEHEADER: 5788 return "MessageHeader"; 5789 case MOLECULARSEQUENCE: 5790 return "MolecularSequence"; 5791 case NAMINGSYSTEM: 5792 return "NamingSystem"; 5793 case NUTRITIONORDER: 5794 return "NutritionOrder"; 5795 case OBSERVATION: 5796 return "Observation"; 5797 case OBSERVATIONDEFINITION: 5798 return "ObservationDefinition"; 5799 case OPERATIONDEFINITION: 5800 return "OperationDefinition"; 5801 case OPERATIONOUTCOME: 5802 return "OperationOutcome"; 5803 case ORGANIZATION: 5804 return "Organization"; 5805 case ORGANIZATIONAFFILIATION: 5806 return "OrganizationAffiliation"; 5807 case PARAMETERS: 5808 return "Parameters"; 5809 case PATIENT: 5810 return "Patient"; 5811 case PAYMENTNOTICE: 5812 return "PaymentNotice"; 5813 case PAYMENTRECONCILIATION: 5814 return "PaymentReconciliation"; 5815 case PERSON: 5816 return "Person"; 5817 case PLANDEFINITION: 5818 return "PlanDefinition"; 5819 case PRACTITIONER: 5820 return "Practitioner"; 5821 case PRACTITIONERROLE: 5822 return "PractitionerRole"; 5823 case PROCEDURE: 5824 return "Procedure"; 5825 case PROVENANCE: 5826 return "Provenance"; 5827 case QUESTIONNAIRE: 5828 return "Questionnaire"; 5829 case QUESTIONNAIRERESPONSE: 5830 return "QuestionnaireResponse"; 5831 case RELATEDPERSON: 5832 return "RelatedPerson"; 5833 case REQUESTGROUP: 5834 return "RequestGroup"; 5835 case RESEARCHDEFINITION: 5836 return "ResearchDefinition"; 5837 case RESEARCHELEMENTDEFINITION: 5838 return "ResearchElementDefinition"; 5839 case RESEARCHSTUDY: 5840 return "ResearchStudy"; 5841 case RESEARCHSUBJECT: 5842 return "ResearchSubject"; 5843 case RESOURCE: 5844 return "Resource"; 5845 case RISKASSESSMENT: 5846 return "RiskAssessment"; 5847 case RISKEVIDENCESYNTHESIS: 5848 return "RiskEvidenceSynthesis"; 5849 case SCHEDULE: 5850 return "Schedule"; 5851 case SEARCHPARAMETER: 5852 return "SearchParameter"; 5853 case SERVICEREQUEST: 5854 return "ServiceRequest"; 5855 case SLOT: 5856 return "Slot"; 5857 case SPECIMEN: 5858 return "Specimen"; 5859 case SPECIMENDEFINITION: 5860 return "SpecimenDefinition"; 5861 case STRUCTUREDEFINITION: 5862 return "StructureDefinition"; 5863 case STRUCTUREMAP: 5864 return "StructureMap"; 5865 case SUBSCRIPTION: 5866 return "Subscription"; 5867 case SUBSTANCE: 5868 return "Substance"; 5869 case SUBSTANCENUCLEICACID: 5870 return "SubstanceNucleicAcid"; 5871 case SUBSTANCEPOLYMER: 5872 return "SubstancePolymer"; 5873 case SUBSTANCEPROTEIN: 5874 return "SubstanceProtein"; 5875 case SUBSTANCEREFERENCEINFORMATION: 5876 return "SubstanceReferenceInformation"; 5877 case SUBSTANCESOURCEMATERIAL: 5878 return "SubstanceSourceMaterial"; 5879 case SUBSTANCESPECIFICATION: 5880 return "SubstanceSpecification"; 5881 case SUPPLYDELIVERY: 5882 return "SupplyDelivery"; 5883 case SUPPLYREQUEST: 5884 return "SupplyRequest"; 5885 case TASK: 5886 return "Task"; 5887 case TERMINOLOGYCAPABILITIES: 5888 return "TerminologyCapabilities"; 5889 case TESTREPORT: 5890 return "TestReport"; 5891 case TESTSCRIPT: 5892 return "TestScript"; 5893 case VALUESET: 5894 return "ValueSet"; 5895 case VERIFICATIONRESULT: 5896 return "VerificationResult"; 5897 case VISIONPRESCRIPTION: 5898 return "VisionPrescription"; 5899 case TYPE: 5900 return "Type"; 5901 case ANY: 5902 return "Any"; 5903 case NULL: 5904 return null; 5905 default: 5906 return "?"; 5907 } 5908 } 5909 5910 public String getSystem() { 5911 switch (this) { 5912 case ADDRESS: 5913 return "http://hl7.org/fhir/data-types"; 5914 case AGE: 5915 return "http://hl7.org/fhir/data-types"; 5916 case ANNOTATION: 5917 return "http://hl7.org/fhir/data-types"; 5918 case ATTACHMENT: 5919 return "http://hl7.org/fhir/data-types"; 5920 case BACKBONEELEMENT: 5921 return "http://hl7.org/fhir/data-types"; 5922 case CODEABLECONCEPT: 5923 return "http://hl7.org/fhir/data-types"; 5924 case CODING: 5925 return "http://hl7.org/fhir/data-types"; 5926 case CONTACTDETAIL: 5927 return "http://hl7.org/fhir/data-types"; 5928 case CONTACTPOINT: 5929 return "http://hl7.org/fhir/data-types"; 5930 case CONTRIBUTOR: 5931 return "http://hl7.org/fhir/data-types"; 5932 case COUNT: 5933 return "http://hl7.org/fhir/data-types"; 5934 case DATAREQUIREMENT: 5935 return "http://hl7.org/fhir/data-types"; 5936 case DISTANCE: 5937 return "http://hl7.org/fhir/data-types"; 5938 case DOSAGE: 5939 return "http://hl7.org/fhir/data-types"; 5940 case DURATION: 5941 return "http://hl7.org/fhir/data-types"; 5942 case ELEMENT: 5943 return "http://hl7.org/fhir/data-types"; 5944 case ELEMENTDEFINITION: 5945 return "http://hl7.org/fhir/data-types"; 5946 case EXPRESSION: 5947 return "http://hl7.org/fhir/data-types"; 5948 case EXTENSION: 5949 return "http://hl7.org/fhir/data-types"; 5950 case HUMANNAME: 5951 return "http://hl7.org/fhir/data-types"; 5952 case IDENTIFIER: 5953 return "http://hl7.org/fhir/data-types"; 5954 case MARKETINGSTATUS: 5955 return "http://hl7.org/fhir/data-types"; 5956 case META: 5957 return "http://hl7.org/fhir/data-types"; 5958 case MONEY: 5959 return "http://hl7.org/fhir/data-types"; 5960 case MONEYQUANTITY: 5961 return "http://hl7.org/fhir/data-types"; 5962 case NARRATIVE: 5963 return "http://hl7.org/fhir/data-types"; 5964 case PARAMETERDEFINITION: 5965 return "http://hl7.org/fhir/data-types"; 5966 case PERIOD: 5967 return "http://hl7.org/fhir/data-types"; 5968 case POPULATION: 5969 return "http://hl7.org/fhir/data-types"; 5970 case PRODCHARACTERISTIC: 5971 return "http://hl7.org/fhir/data-types"; 5972 case PRODUCTSHELFLIFE: 5973 return "http://hl7.org/fhir/data-types"; 5974 case QUANTITY: 5975 return "http://hl7.org/fhir/data-types"; 5976 case RANGE: 5977 return "http://hl7.org/fhir/data-types"; 5978 case RATIO: 5979 return "http://hl7.org/fhir/data-types"; 5980 case REFERENCE: 5981 return "http://hl7.org/fhir/data-types"; 5982 case RELATEDARTIFACT: 5983 return "http://hl7.org/fhir/data-types"; 5984 case SAMPLEDDATA: 5985 return "http://hl7.org/fhir/data-types"; 5986 case SIGNATURE: 5987 return "http://hl7.org/fhir/data-types"; 5988 case SIMPLEQUANTITY: 5989 return "http://hl7.org/fhir/data-types"; 5990 case SUBSTANCEAMOUNT: 5991 return "http://hl7.org/fhir/data-types"; 5992 case TIMING: 5993 return "http://hl7.org/fhir/data-types"; 5994 case TRIGGERDEFINITION: 5995 return "http://hl7.org/fhir/data-types"; 5996 case USAGECONTEXT: 5997 return "http://hl7.org/fhir/data-types"; 5998 case BASE64BINARY: 5999 return "http://hl7.org/fhir/data-types"; 6000 case BOOLEAN: 6001 return "http://hl7.org/fhir/data-types"; 6002 case CANONICAL: 6003 return "http://hl7.org/fhir/data-types"; 6004 case CODE: 6005 return "http://hl7.org/fhir/data-types"; 6006 case DATE: 6007 return "http://hl7.org/fhir/data-types"; 6008 case DATETIME: 6009 return "http://hl7.org/fhir/data-types"; 6010 case DECIMAL: 6011 return "http://hl7.org/fhir/data-types"; 6012 case ID: 6013 return "http://hl7.org/fhir/data-types"; 6014 case INSTANT: 6015 return "http://hl7.org/fhir/data-types"; 6016 case INTEGER: 6017 return "http://hl7.org/fhir/data-types"; 6018 case MARKDOWN: 6019 return "http://hl7.org/fhir/data-types"; 6020 case OID: 6021 return "http://hl7.org/fhir/data-types"; 6022 case POSITIVEINT: 6023 return "http://hl7.org/fhir/data-types"; 6024 case STRING: 6025 return "http://hl7.org/fhir/data-types"; 6026 case TIME: 6027 return "http://hl7.org/fhir/data-types"; 6028 case UNSIGNEDINT: 6029 return "http://hl7.org/fhir/data-types"; 6030 case URI: 6031 return "http://hl7.org/fhir/data-types"; 6032 case URL: 6033 return "http://hl7.org/fhir/data-types"; 6034 case UUID: 6035 return "http://hl7.org/fhir/data-types"; 6036 case XHTML: 6037 return "http://hl7.org/fhir/data-types"; 6038 case ACCOUNT: 6039 return "http://hl7.org/fhir/resource-types"; 6040 case ACTIVITYDEFINITION: 6041 return "http://hl7.org/fhir/resource-types"; 6042 case ADVERSEEVENT: 6043 return "http://hl7.org/fhir/resource-types"; 6044 case ALLERGYINTOLERANCE: 6045 return "http://hl7.org/fhir/resource-types"; 6046 case APPOINTMENT: 6047 return "http://hl7.org/fhir/resource-types"; 6048 case APPOINTMENTRESPONSE: 6049 return "http://hl7.org/fhir/resource-types"; 6050 case AUDITEVENT: 6051 return "http://hl7.org/fhir/resource-types"; 6052 case BASIC: 6053 return "http://hl7.org/fhir/resource-types"; 6054 case BINARY: 6055 return "http://hl7.org/fhir/resource-types"; 6056 case BIOLOGICALLYDERIVEDPRODUCT: 6057 return "http://hl7.org/fhir/resource-types"; 6058 case BODYSTRUCTURE: 6059 return "http://hl7.org/fhir/resource-types"; 6060 case BUNDLE: 6061 return "http://hl7.org/fhir/resource-types"; 6062 case CAPABILITYSTATEMENT: 6063 return "http://hl7.org/fhir/resource-types"; 6064 case CAREPLAN: 6065 return "http://hl7.org/fhir/resource-types"; 6066 case CARETEAM: 6067 return "http://hl7.org/fhir/resource-types"; 6068 case CATALOGENTRY: 6069 return "http://hl7.org/fhir/resource-types"; 6070 case CHARGEITEM: 6071 return "http://hl7.org/fhir/resource-types"; 6072 case CHARGEITEMDEFINITION: 6073 return "http://hl7.org/fhir/resource-types"; 6074 case CLAIM: 6075 return "http://hl7.org/fhir/resource-types"; 6076 case CLAIMRESPONSE: 6077 return "http://hl7.org/fhir/resource-types"; 6078 case CLINICALIMPRESSION: 6079 return "http://hl7.org/fhir/resource-types"; 6080 case CODESYSTEM: 6081 return "http://hl7.org/fhir/resource-types"; 6082 case COMMUNICATION: 6083 return "http://hl7.org/fhir/resource-types"; 6084 case COMMUNICATIONREQUEST: 6085 return "http://hl7.org/fhir/resource-types"; 6086 case COMPARTMENTDEFINITION: 6087 return "http://hl7.org/fhir/resource-types"; 6088 case COMPOSITION: 6089 return "http://hl7.org/fhir/resource-types"; 6090 case CONCEPTMAP: 6091 return "http://hl7.org/fhir/resource-types"; 6092 case CONDITION: 6093 return "http://hl7.org/fhir/resource-types"; 6094 case CONSENT: 6095 return "http://hl7.org/fhir/resource-types"; 6096 case CONTRACT: 6097 return "http://hl7.org/fhir/resource-types"; 6098 case COVERAGE: 6099 return "http://hl7.org/fhir/resource-types"; 6100 case COVERAGEELIGIBILITYREQUEST: 6101 return "http://hl7.org/fhir/resource-types"; 6102 case COVERAGEELIGIBILITYRESPONSE: 6103 return "http://hl7.org/fhir/resource-types"; 6104 case DETECTEDISSUE: 6105 return "http://hl7.org/fhir/resource-types"; 6106 case DEVICE: 6107 return "http://hl7.org/fhir/resource-types"; 6108 case DEVICEDEFINITION: 6109 return "http://hl7.org/fhir/resource-types"; 6110 case DEVICEMETRIC: 6111 return "http://hl7.org/fhir/resource-types"; 6112 case DEVICEREQUEST: 6113 return "http://hl7.org/fhir/resource-types"; 6114 case DEVICEUSESTATEMENT: 6115 return "http://hl7.org/fhir/resource-types"; 6116 case DIAGNOSTICREPORT: 6117 return "http://hl7.org/fhir/resource-types"; 6118 case DOCUMENTMANIFEST: 6119 return "http://hl7.org/fhir/resource-types"; 6120 case DOCUMENTREFERENCE: 6121 return "http://hl7.org/fhir/resource-types"; 6122 case DOMAINRESOURCE: 6123 return "http://hl7.org/fhir/resource-types"; 6124 case EFFECTEVIDENCESYNTHESIS: 6125 return "http://hl7.org/fhir/resource-types"; 6126 case ENCOUNTER: 6127 return "http://hl7.org/fhir/resource-types"; 6128 case ENDPOINT: 6129 return "http://hl7.org/fhir/resource-types"; 6130 case ENROLLMENTREQUEST: 6131 return "http://hl7.org/fhir/resource-types"; 6132 case ENROLLMENTRESPONSE: 6133 return "http://hl7.org/fhir/resource-types"; 6134 case EPISODEOFCARE: 6135 return "http://hl7.org/fhir/resource-types"; 6136 case EVENTDEFINITION: 6137 return "http://hl7.org/fhir/resource-types"; 6138 case EVIDENCE: 6139 return "http://hl7.org/fhir/resource-types"; 6140 case EVIDENCEVARIABLE: 6141 return "http://hl7.org/fhir/resource-types"; 6142 case EXAMPLESCENARIO: 6143 return "http://hl7.org/fhir/resource-types"; 6144 case EXPLANATIONOFBENEFIT: 6145 return "http://hl7.org/fhir/resource-types"; 6146 case FAMILYMEMBERHISTORY: 6147 return "http://hl7.org/fhir/resource-types"; 6148 case FLAG: 6149 return "http://hl7.org/fhir/resource-types"; 6150 case GOAL: 6151 return "http://hl7.org/fhir/resource-types"; 6152 case GRAPHDEFINITION: 6153 return "http://hl7.org/fhir/resource-types"; 6154 case GROUP: 6155 return "http://hl7.org/fhir/resource-types"; 6156 case GUIDANCERESPONSE: 6157 return "http://hl7.org/fhir/resource-types"; 6158 case HEALTHCARESERVICE: 6159 return "http://hl7.org/fhir/resource-types"; 6160 case IMAGINGSTUDY: 6161 return "http://hl7.org/fhir/resource-types"; 6162 case IMMUNIZATION: 6163 return "http://hl7.org/fhir/resource-types"; 6164 case IMMUNIZATIONEVALUATION: 6165 return "http://hl7.org/fhir/resource-types"; 6166 case IMMUNIZATIONRECOMMENDATION: 6167 return "http://hl7.org/fhir/resource-types"; 6168 case IMPLEMENTATIONGUIDE: 6169 return "http://hl7.org/fhir/resource-types"; 6170 case INSURANCEPLAN: 6171 return "http://hl7.org/fhir/resource-types"; 6172 case INVOICE: 6173 return "http://hl7.org/fhir/resource-types"; 6174 case LIBRARY: 6175 return "http://hl7.org/fhir/resource-types"; 6176 case LINKAGE: 6177 return "http://hl7.org/fhir/resource-types"; 6178 case LIST: 6179 return "http://hl7.org/fhir/resource-types"; 6180 case LOCATION: 6181 return "http://hl7.org/fhir/resource-types"; 6182 case MEASURE: 6183 return "http://hl7.org/fhir/resource-types"; 6184 case MEASUREREPORT: 6185 return "http://hl7.org/fhir/resource-types"; 6186 case MEDIA: 6187 return "http://hl7.org/fhir/resource-types"; 6188 case MEDICATION: 6189 return "http://hl7.org/fhir/resource-types"; 6190 case MEDICATIONADMINISTRATION: 6191 return "http://hl7.org/fhir/resource-types"; 6192 case MEDICATIONDISPENSE: 6193 return "http://hl7.org/fhir/resource-types"; 6194 case MEDICATIONKNOWLEDGE: 6195 return "http://hl7.org/fhir/resource-types"; 6196 case MEDICATIONREQUEST: 6197 return "http://hl7.org/fhir/resource-types"; 6198 case MEDICATIONSTATEMENT: 6199 return "http://hl7.org/fhir/resource-types"; 6200 case MEDICINALPRODUCT: 6201 return "http://hl7.org/fhir/resource-types"; 6202 case MEDICINALPRODUCTAUTHORIZATION: 6203 return "http://hl7.org/fhir/resource-types"; 6204 case MEDICINALPRODUCTCONTRAINDICATION: 6205 return "http://hl7.org/fhir/resource-types"; 6206 case MEDICINALPRODUCTINDICATION: 6207 return "http://hl7.org/fhir/resource-types"; 6208 case MEDICINALPRODUCTINGREDIENT: 6209 return "http://hl7.org/fhir/resource-types"; 6210 case MEDICINALPRODUCTINTERACTION: 6211 return "http://hl7.org/fhir/resource-types"; 6212 case MEDICINALPRODUCTMANUFACTURED: 6213 return "http://hl7.org/fhir/resource-types"; 6214 case MEDICINALPRODUCTPACKAGED: 6215 return "http://hl7.org/fhir/resource-types"; 6216 case MEDICINALPRODUCTPHARMACEUTICAL: 6217 return "http://hl7.org/fhir/resource-types"; 6218 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 6219 return "http://hl7.org/fhir/resource-types"; 6220 case MESSAGEDEFINITION: 6221 return "http://hl7.org/fhir/resource-types"; 6222 case MESSAGEHEADER: 6223 return "http://hl7.org/fhir/resource-types"; 6224 case MOLECULARSEQUENCE: 6225 return "http://hl7.org/fhir/resource-types"; 6226 case NAMINGSYSTEM: 6227 return "http://hl7.org/fhir/resource-types"; 6228 case NUTRITIONORDER: 6229 return "http://hl7.org/fhir/resource-types"; 6230 case OBSERVATION: 6231 return "http://hl7.org/fhir/resource-types"; 6232 case OBSERVATIONDEFINITION: 6233 return "http://hl7.org/fhir/resource-types"; 6234 case OPERATIONDEFINITION: 6235 return "http://hl7.org/fhir/resource-types"; 6236 case OPERATIONOUTCOME: 6237 return "http://hl7.org/fhir/resource-types"; 6238 case ORGANIZATION: 6239 return "http://hl7.org/fhir/resource-types"; 6240 case ORGANIZATIONAFFILIATION: 6241 return "http://hl7.org/fhir/resource-types"; 6242 case PARAMETERS: 6243 return "http://hl7.org/fhir/resource-types"; 6244 case PATIENT: 6245 return "http://hl7.org/fhir/resource-types"; 6246 case PAYMENTNOTICE: 6247 return "http://hl7.org/fhir/resource-types"; 6248 case PAYMENTRECONCILIATION: 6249 return "http://hl7.org/fhir/resource-types"; 6250 case PERSON: 6251 return "http://hl7.org/fhir/resource-types"; 6252 case PLANDEFINITION: 6253 return "http://hl7.org/fhir/resource-types"; 6254 case PRACTITIONER: 6255 return "http://hl7.org/fhir/resource-types"; 6256 case PRACTITIONERROLE: 6257 return "http://hl7.org/fhir/resource-types"; 6258 case PROCEDURE: 6259 return "http://hl7.org/fhir/resource-types"; 6260 case PROVENANCE: 6261 return "http://hl7.org/fhir/resource-types"; 6262 case QUESTIONNAIRE: 6263 return "http://hl7.org/fhir/resource-types"; 6264 case QUESTIONNAIRERESPONSE: 6265 return "http://hl7.org/fhir/resource-types"; 6266 case RELATEDPERSON: 6267 return "http://hl7.org/fhir/resource-types"; 6268 case REQUESTGROUP: 6269 return "http://hl7.org/fhir/resource-types"; 6270 case RESEARCHDEFINITION: 6271 return "http://hl7.org/fhir/resource-types"; 6272 case RESEARCHELEMENTDEFINITION: 6273 return "http://hl7.org/fhir/resource-types"; 6274 case RESEARCHSTUDY: 6275 return "http://hl7.org/fhir/resource-types"; 6276 case RESEARCHSUBJECT: 6277 return "http://hl7.org/fhir/resource-types"; 6278 case RESOURCE: 6279 return "http://hl7.org/fhir/resource-types"; 6280 case RISKASSESSMENT: 6281 return "http://hl7.org/fhir/resource-types"; 6282 case RISKEVIDENCESYNTHESIS: 6283 return "http://hl7.org/fhir/resource-types"; 6284 case SCHEDULE: 6285 return "http://hl7.org/fhir/resource-types"; 6286 case SEARCHPARAMETER: 6287 return "http://hl7.org/fhir/resource-types"; 6288 case SERVICEREQUEST: 6289 return "http://hl7.org/fhir/resource-types"; 6290 case SLOT: 6291 return "http://hl7.org/fhir/resource-types"; 6292 case SPECIMEN: 6293 return "http://hl7.org/fhir/resource-types"; 6294 case SPECIMENDEFINITION: 6295 return "http://hl7.org/fhir/resource-types"; 6296 case STRUCTUREDEFINITION: 6297 return "http://hl7.org/fhir/resource-types"; 6298 case STRUCTUREMAP: 6299 return "http://hl7.org/fhir/resource-types"; 6300 case SUBSCRIPTION: 6301 return "http://hl7.org/fhir/resource-types"; 6302 case SUBSTANCE: 6303 return "http://hl7.org/fhir/resource-types"; 6304 case SUBSTANCENUCLEICACID: 6305 return "http://hl7.org/fhir/resource-types"; 6306 case SUBSTANCEPOLYMER: 6307 return "http://hl7.org/fhir/resource-types"; 6308 case SUBSTANCEPROTEIN: 6309 return "http://hl7.org/fhir/resource-types"; 6310 case SUBSTANCEREFERENCEINFORMATION: 6311 return "http://hl7.org/fhir/resource-types"; 6312 case SUBSTANCESOURCEMATERIAL: 6313 return "http://hl7.org/fhir/resource-types"; 6314 case SUBSTANCESPECIFICATION: 6315 return "http://hl7.org/fhir/resource-types"; 6316 case SUPPLYDELIVERY: 6317 return "http://hl7.org/fhir/resource-types"; 6318 case SUPPLYREQUEST: 6319 return "http://hl7.org/fhir/resource-types"; 6320 case TASK: 6321 return "http://hl7.org/fhir/resource-types"; 6322 case TERMINOLOGYCAPABILITIES: 6323 return "http://hl7.org/fhir/resource-types"; 6324 case TESTREPORT: 6325 return "http://hl7.org/fhir/resource-types"; 6326 case TESTSCRIPT: 6327 return "http://hl7.org/fhir/resource-types"; 6328 case VALUESET: 6329 return "http://hl7.org/fhir/resource-types"; 6330 case VERIFICATIONRESULT: 6331 return "http://hl7.org/fhir/resource-types"; 6332 case VISIONPRESCRIPTION: 6333 return "http://hl7.org/fhir/resource-types"; 6334 case TYPE: 6335 return "http://hl7.org/fhir/abstract-types"; 6336 case ANY: 6337 return "http://hl7.org/fhir/abstract-types"; 6338 case NULL: 6339 return null; 6340 default: 6341 return "?"; 6342 } 6343 } 6344 6345 public String getDefinition() { 6346 switch (this) { 6347 case ADDRESS: 6348 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 6349 case AGE: 6350 return "A duration of time during which an organism (or a process) has existed."; 6351 case ANNOTATION: 6352 return "A text note which also contains information about who made the statement and when."; 6353 case ATTACHMENT: 6354 return "For referring to data content defined in other formats."; 6355 case BACKBONEELEMENT: 6356 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 6357 case CODEABLECONCEPT: 6358 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 6359 case CODING: 6360 return "A reference to a code defined by a terminology system."; 6361 case CONTACTDETAIL: 6362 return "Specifies contact information for a person or organization."; 6363 case CONTACTPOINT: 6364 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 6365 case CONTRIBUTOR: 6366 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 6367 case COUNT: 6368 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6369 case DATAREQUIREMENT: 6370 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 6371 case DISTANCE: 6372 return "A length - a value with a unit that is a physical distance."; 6373 case DOSAGE: 6374 return "Indicates how the medication is/was taken or should be taken by the patient."; 6375 case DURATION: 6376 return "A length of time."; 6377 case ELEMENT: 6378 return "Base definition for all elements in a resource."; 6379 case ELEMENTDEFINITION: 6380 return "Captures constraints on each element within the resource, profile, or extension."; 6381 case EXPRESSION: 6382 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 6383 case EXTENSION: 6384 return "Optional Extension Element - found in all resources."; 6385 case HUMANNAME: 6386 return "A human's name with the ability to identify parts and usage."; 6387 case IDENTIFIER: 6388 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 6389 case MARKETINGSTATUS: 6390 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 6391 case META: 6392 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 6393 case MONEY: 6394 return "An amount of economic utility in some recognized currency."; 6395 case MONEYQUANTITY: 6396 return ""; 6397 case NARRATIVE: 6398 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 6399 case PARAMETERDEFINITION: 6400 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 6401 case PERIOD: 6402 return "A time period defined by a start and end date and optionally time."; 6403 case POPULATION: 6404 return "A populatioof people with some set of grouping criteria."; 6405 case PRODCHARACTERISTIC: 6406 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 6407 case PRODUCTSHELFLIFE: 6408 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 6409 case QUANTITY: 6410 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6411 case RANGE: 6412 return "A set of ordered Quantities defined by a low and high limit."; 6413 case RATIO: 6414 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 6415 case REFERENCE: 6416 return "A reference from one resource to another."; 6417 case RELATEDARTIFACT: 6418 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 6419 case SAMPLEDDATA: 6420 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 6421 case SIGNATURE: 6422 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 6423 case SIMPLEQUANTITY: 6424 return ""; 6425 case SUBSTANCEAMOUNT: 6426 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 6427 case TIMING: 6428 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 6429 case TRIGGERDEFINITION: 6430 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 6431 case USAGECONTEXT: 6432 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 6433 case BASE64BINARY: 6434 return "A stream of bytes"; 6435 case BOOLEAN: 6436 return "Value of \"true\" or \"false\""; 6437 case CANONICAL: 6438 return "A URI that is a reference to a canonical URL on a FHIR resource"; 6439 case CODE: 6440 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 6441 case DATE: 6442 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 6443 case DATETIME: 6444 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 6445 case DECIMAL: 6446 return "A rational number with implicit precision"; 6447 case ID: 6448 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 6449 case INSTANT: 6450 return "An instant in time - known at least to the second"; 6451 case INTEGER: 6452 return "A whole number"; 6453 case MARKDOWN: 6454 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 6455 case OID: 6456 return "An OID represented as a URI"; 6457 case POSITIVEINT: 6458 return "An integer with a value that is positive (e.g. >0)"; 6459 case STRING: 6460 return "A sequence of Unicode characters"; 6461 case TIME: 6462 return "A time during the day, with no date specified"; 6463 case UNSIGNEDINT: 6464 return "An integer with a value that is not negative (e.g. >= 0)"; 6465 case URI: 6466 return "String of characters used to identify a name or a resource"; 6467 case URL: 6468 return "A URI that is a literal reference"; 6469 case UUID: 6470 return "A UUID, represented as a URI"; 6471 case XHTML: 6472 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 6473 case ACCOUNT: 6474 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 6475 case ACTIVITYDEFINITION: 6476 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 6477 case ADVERSEEVENT: 6478 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 6479 case ALLERGYINTOLERANCE: 6480 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 6481 case APPOINTMENT: 6482 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 6483 case APPOINTMENTRESPONSE: 6484 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 6485 case AUDITEVENT: 6486 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 6487 case BASIC: 6488 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 6489 case BINARY: 6490 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 6491 case BIOLOGICALLYDERIVEDPRODUCT: 6492 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 6493 case BODYSTRUCTURE: 6494 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 6495 case BUNDLE: 6496 return "A container for a collection of resources."; 6497 case CAPABILITYSTATEMENT: 6498 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6499 case CAREPLAN: 6500 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 6501 case CARETEAM: 6502 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 6503 case CATALOGENTRY: 6504 return "Catalog entries are wrappers that contextualize items included in a catalog."; 6505 case CHARGEITEM: 6506 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 6507 case CHARGEITEMDEFINITION: 6508 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 6509 case CLAIM: 6510 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 6511 case CLAIMRESPONSE: 6512 return "This resource provides the adjudication details from the processing of a Claim resource."; 6513 case CLINICALIMPRESSION: 6514 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 6515 case CODESYSTEM: 6516 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 6517 case COMMUNICATION: 6518 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 6519 case COMMUNICATIONREQUEST: 6520 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 6521 case COMPARTMENTDEFINITION: 6522 return "A compartment definition that defines how resources are accessed on a server."; 6523 case COMPOSITION: 6524 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 6525 case CONCEPTMAP: 6526 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 6527 case CONDITION: 6528 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 6529 case CONSENT: 6530 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 6531 case CONTRACT: 6532 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 6533 case COVERAGE: 6534 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 6535 case COVERAGEELIGIBILITYREQUEST: 6536 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 6537 case COVERAGEELIGIBILITYRESPONSE: 6538 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 6539 case DETECTEDISSUE: 6540 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 6541 case DEVICE: 6542 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 6543 case DEVICEDEFINITION: 6544 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 6545 case DEVICEMETRIC: 6546 return "Describes a measurement, calculation or setting capability of a medical device."; 6547 case DEVICEREQUEST: 6548 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 6549 case DEVICEUSESTATEMENT: 6550 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 6551 case DIAGNOSTICREPORT: 6552 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 6553 case DOCUMENTMANIFEST: 6554 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 6555 case DOCUMENTREFERENCE: 6556 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 6557 case DOMAINRESOURCE: 6558 return "A resource that includes narrative, extensions, and contained resources."; 6559 case EFFECTEVIDENCESYNTHESIS: 6560 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 6561 case ENCOUNTER: 6562 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 6563 case ENDPOINT: 6564 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 6565 case ENROLLMENTREQUEST: 6566 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 6567 case ENROLLMENTRESPONSE: 6568 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 6569 case EPISODEOFCARE: 6570 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 6571 case EVENTDEFINITION: 6572 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 6573 case EVIDENCE: 6574 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 6575 case EVIDENCEVARIABLE: 6576 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 6577 case EXAMPLESCENARIO: 6578 return "Example of workflow instance."; 6579 case EXPLANATIONOFBENEFIT: 6580 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 6581 case FAMILYMEMBERHISTORY: 6582 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 6583 case FLAG: 6584 return "Prospective warnings of potential issues when providing care to the patient."; 6585 case GOAL: 6586 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 6587 case GRAPHDEFINITION: 6588 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 6589 case GROUP: 6590 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 6591 case GUIDANCERESPONSE: 6592 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 6593 case HEALTHCARESERVICE: 6594 return "The details of a healthcare service available at a location."; 6595 case IMAGINGSTUDY: 6596 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 6597 case IMMUNIZATION: 6598 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 6599 case IMMUNIZATIONEVALUATION: 6600 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 6601 case IMMUNIZATIONRECOMMENDATION: 6602 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 6603 case IMPLEMENTATIONGUIDE: 6604 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 6605 case INSURANCEPLAN: 6606 return "Details of a Health Insurance product/plan provided by an organization."; 6607 case INVOICE: 6608 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 6609 case LIBRARY: 6610 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 6611 case LINKAGE: 6612 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 6613 case LIST: 6614 return "A list is a curated collection of resources."; 6615 case LOCATION: 6616 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 6617 case MEASURE: 6618 return "The Measure resource provides the definition of a quality measure."; 6619 case MEASUREREPORT: 6620 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 6621 case MEDIA: 6622 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 6623 case MEDICATION: 6624 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 6625 case MEDICATIONADMINISTRATION: 6626 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 6627 case MEDICATIONDISPENSE: 6628 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 6629 case MEDICATIONKNOWLEDGE: 6630 return "Information about a medication that is used to support knowledge."; 6631 case MEDICATIONREQUEST: 6632 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 6633 case MEDICATIONSTATEMENT: 6634 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 6635 case MEDICINALPRODUCT: 6636 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 6637 case MEDICINALPRODUCTAUTHORIZATION: 6638 return "The regulatory authorization of a medicinal product."; 6639 case MEDICINALPRODUCTCONTRAINDICATION: 6640 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 6641 case MEDICINALPRODUCTINDICATION: 6642 return "Indication for the Medicinal Product."; 6643 case MEDICINALPRODUCTINGREDIENT: 6644 return "An ingredient of a manufactured item or pharmaceutical product."; 6645 case MEDICINALPRODUCTINTERACTION: 6646 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 6647 case MEDICINALPRODUCTMANUFACTURED: 6648 return "The manufactured item as contained in the packaged medicinal product."; 6649 case MEDICINALPRODUCTPACKAGED: 6650 return "A medicinal product in a container or package."; 6651 case MEDICINALPRODUCTPHARMACEUTICAL: 6652 return "A pharmaceutical product described in terms of its composition and dose form."; 6653 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 6654 return "Describe the undesirable effects of the medicinal product."; 6655 case MESSAGEDEFINITION: 6656 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 6657 case MESSAGEHEADER: 6658 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 6659 case MOLECULARSEQUENCE: 6660 return "Raw data describing a biological sequence."; 6661 case NAMINGSYSTEM: 6662 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 6663 case NUTRITIONORDER: 6664 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 6665 case OBSERVATION: 6666 return "Measurements and simple assertions made about a patient, device or other subject."; 6667 case OBSERVATIONDEFINITION: 6668 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 6669 case OPERATIONDEFINITION: 6670 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 6671 case OPERATIONOUTCOME: 6672 return "A collection of error, warning, or information messages that result from a system action."; 6673 case ORGANIZATION: 6674 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 6675 case ORGANIZATIONAFFILIATION: 6676 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 6677 case PARAMETERS: 6678 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 6679 case PATIENT: 6680 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 6681 case PAYMENTNOTICE: 6682 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 6683 case PAYMENTRECONCILIATION: 6684 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 6685 case PERSON: 6686 return "Demographics and administrative information about a person independent of a specific health-related context."; 6687 case PLANDEFINITION: 6688 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 6689 case PRACTITIONER: 6690 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 6691 case PRACTITIONERROLE: 6692 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 6693 case PROCEDURE: 6694 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 6695 case PROVENANCE: 6696 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 6697 case QUESTIONNAIRE: 6698 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 6699 case QUESTIONNAIRERESPONSE: 6700 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 6701 case RELATEDPERSON: 6702 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 6703 case REQUESTGROUP: 6704 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 6705 case RESEARCHDEFINITION: 6706 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 6707 case RESEARCHELEMENTDEFINITION: 6708 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 6709 case RESEARCHSTUDY: 6710 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6711 case RESEARCHSUBJECT: 6712 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 6713 case RESOURCE: 6714 return "This is the base resource type for everything."; 6715 case RISKASSESSMENT: 6716 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 6717 case RISKEVIDENCESYNTHESIS: 6718 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 6719 case SCHEDULE: 6720 return "A container for slots of time that may be available for booking appointments."; 6721 case SEARCHPARAMETER: 6722 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 6723 case SERVICEREQUEST: 6724 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 6725 case SLOT: 6726 return "A slot of time on a schedule that may be available for booking appointments."; 6727 case SPECIMEN: 6728 return "A sample to be used for analysis."; 6729 case SPECIMENDEFINITION: 6730 return "A kind of specimen with associated set of requirements."; 6731 case STRUCTUREDEFINITION: 6732 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 6733 case STRUCTUREMAP: 6734 return "A Map of relationships between 2 structures that can be used to transform data."; 6735 case SUBSCRIPTION: 6736 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 6737 case SUBSTANCE: 6738 return "A homogeneous material with a definite composition."; 6739 case SUBSTANCENUCLEICACID: 6740 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 6741 case SUBSTANCEPOLYMER: 6742 return "Todo."; 6743 case SUBSTANCEPROTEIN: 6744 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 6745 case SUBSTANCEREFERENCEINFORMATION: 6746 return "Todo."; 6747 case SUBSTANCESOURCEMATERIAL: 6748 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 6749 case SUBSTANCESPECIFICATION: 6750 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 6751 case SUPPLYDELIVERY: 6752 return "Record of delivery of what is supplied."; 6753 case SUPPLYREQUEST: 6754 return "A record of a request for a medication, substance or device used in the healthcare setting."; 6755 case TASK: 6756 return "A task to be performed."; 6757 case TERMINOLOGYCAPABILITIES: 6758 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6759 case TESTREPORT: 6760 return "A summary of information based on the results of executing a TestScript."; 6761 case TESTSCRIPT: 6762 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 6763 case VALUESET: 6764 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 6765 case VERIFICATIONRESULT: 6766 return "Describes validation requirements, source(s), status and dates for one or more elements."; 6767 case VISIONPRESCRIPTION: 6768 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 6769 case TYPE: 6770 return "A place holder that means any kind of data type"; 6771 case ANY: 6772 return "A place holder that means any kind of resource"; 6773 case NULL: 6774 return null; 6775 default: 6776 return "?"; 6777 } 6778 } 6779 6780 public String getDisplay() { 6781 switch (this) { 6782 case ADDRESS: 6783 return "Address"; 6784 case AGE: 6785 return "Age"; 6786 case ANNOTATION: 6787 return "Annotation"; 6788 case ATTACHMENT: 6789 return "Attachment"; 6790 case BACKBONEELEMENT: 6791 return "BackboneElement"; 6792 case CODEABLECONCEPT: 6793 return "CodeableConcept"; 6794 case CODING: 6795 return "Coding"; 6796 case CONTACTDETAIL: 6797 return "ContactDetail"; 6798 case CONTACTPOINT: 6799 return "ContactPoint"; 6800 case CONTRIBUTOR: 6801 return "Contributor"; 6802 case COUNT: 6803 return "Count"; 6804 case DATAREQUIREMENT: 6805 return "DataRequirement"; 6806 case DISTANCE: 6807 return "Distance"; 6808 case DOSAGE: 6809 return "Dosage"; 6810 case DURATION: 6811 return "Duration"; 6812 case ELEMENT: 6813 return "Element"; 6814 case ELEMENTDEFINITION: 6815 return "ElementDefinition"; 6816 case EXPRESSION: 6817 return "Expression"; 6818 case EXTENSION: 6819 return "Extension"; 6820 case HUMANNAME: 6821 return "HumanName"; 6822 case IDENTIFIER: 6823 return "Identifier"; 6824 case MARKETINGSTATUS: 6825 return "MarketingStatus"; 6826 case META: 6827 return "Meta"; 6828 case MONEY: 6829 return "Money"; 6830 case MONEYQUANTITY: 6831 return "MoneyQuantity"; 6832 case NARRATIVE: 6833 return "Narrative"; 6834 case PARAMETERDEFINITION: 6835 return "ParameterDefinition"; 6836 case PERIOD: 6837 return "Period"; 6838 case POPULATION: 6839 return "Population"; 6840 case PRODCHARACTERISTIC: 6841 return "ProdCharacteristic"; 6842 case PRODUCTSHELFLIFE: 6843 return "ProductShelfLife"; 6844 case QUANTITY: 6845 return "Quantity"; 6846 case RANGE: 6847 return "Range"; 6848 case RATIO: 6849 return "Ratio"; 6850 case REFERENCE: 6851 return "Reference"; 6852 case RELATEDARTIFACT: 6853 return "RelatedArtifact"; 6854 case SAMPLEDDATA: 6855 return "SampledData"; 6856 case SIGNATURE: 6857 return "Signature"; 6858 case SIMPLEQUANTITY: 6859 return "SimpleQuantity"; 6860 case SUBSTANCEAMOUNT: 6861 return "SubstanceAmount"; 6862 case TIMING: 6863 return "Timing"; 6864 case TRIGGERDEFINITION: 6865 return "TriggerDefinition"; 6866 case USAGECONTEXT: 6867 return "UsageContext"; 6868 case BASE64BINARY: 6869 return "base64Binary"; 6870 case BOOLEAN: 6871 return "boolean"; 6872 case CANONICAL: 6873 return "canonical"; 6874 case CODE: 6875 return "code"; 6876 case DATE: 6877 return "date"; 6878 case DATETIME: 6879 return "dateTime"; 6880 case DECIMAL: 6881 return "decimal"; 6882 case ID: 6883 return "id"; 6884 case INSTANT: 6885 return "instant"; 6886 case INTEGER: 6887 return "integer"; 6888 case MARKDOWN: 6889 return "markdown"; 6890 case OID: 6891 return "oid"; 6892 case POSITIVEINT: 6893 return "positiveInt"; 6894 case STRING: 6895 return "string"; 6896 case TIME: 6897 return "time"; 6898 case UNSIGNEDINT: 6899 return "unsignedInt"; 6900 case URI: 6901 return "uri"; 6902 case URL: 6903 return "url"; 6904 case UUID: 6905 return "uuid"; 6906 case XHTML: 6907 return "XHTML"; 6908 case ACCOUNT: 6909 return "Account"; 6910 case ACTIVITYDEFINITION: 6911 return "ActivityDefinition"; 6912 case ADVERSEEVENT: 6913 return "AdverseEvent"; 6914 case ALLERGYINTOLERANCE: 6915 return "AllergyIntolerance"; 6916 case APPOINTMENT: 6917 return "Appointment"; 6918 case APPOINTMENTRESPONSE: 6919 return "AppointmentResponse"; 6920 case AUDITEVENT: 6921 return "AuditEvent"; 6922 case BASIC: 6923 return "Basic"; 6924 case BINARY: 6925 return "Binary"; 6926 case BIOLOGICALLYDERIVEDPRODUCT: 6927 return "BiologicallyDerivedProduct"; 6928 case BODYSTRUCTURE: 6929 return "BodyStructure"; 6930 case BUNDLE: 6931 return "Bundle"; 6932 case CAPABILITYSTATEMENT: 6933 return "CapabilityStatement"; 6934 case CAREPLAN: 6935 return "CarePlan"; 6936 case CARETEAM: 6937 return "CareTeam"; 6938 case CATALOGENTRY: 6939 return "CatalogEntry"; 6940 case CHARGEITEM: 6941 return "ChargeItem"; 6942 case CHARGEITEMDEFINITION: 6943 return "ChargeItemDefinition"; 6944 case CLAIM: 6945 return "Claim"; 6946 case CLAIMRESPONSE: 6947 return "ClaimResponse"; 6948 case CLINICALIMPRESSION: 6949 return "ClinicalImpression"; 6950 case CODESYSTEM: 6951 return "CodeSystem"; 6952 case COMMUNICATION: 6953 return "Communication"; 6954 case COMMUNICATIONREQUEST: 6955 return "CommunicationRequest"; 6956 case COMPARTMENTDEFINITION: 6957 return "CompartmentDefinition"; 6958 case COMPOSITION: 6959 return "Composition"; 6960 case CONCEPTMAP: 6961 return "ConceptMap"; 6962 case CONDITION: 6963 return "Condition"; 6964 case CONSENT: 6965 return "Consent"; 6966 case CONTRACT: 6967 return "Contract"; 6968 case COVERAGE: 6969 return "Coverage"; 6970 case COVERAGEELIGIBILITYREQUEST: 6971 return "CoverageEligibilityRequest"; 6972 case COVERAGEELIGIBILITYRESPONSE: 6973 return "CoverageEligibilityResponse"; 6974 case DETECTEDISSUE: 6975 return "DetectedIssue"; 6976 case DEVICE: 6977 return "Device"; 6978 case DEVICEDEFINITION: 6979 return "DeviceDefinition"; 6980 case DEVICEMETRIC: 6981 return "DeviceMetric"; 6982 case DEVICEREQUEST: 6983 return "DeviceRequest"; 6984 case DEVICEUSESTATEMENT: 6985 return "DeviceUseStatement"; 6986 case DIAGNOSTICREPORT: 6987 return "DiagnosticReport"; 6988 case DOCUMENTMANIFEST: 6989 return "DocumentManifest"; 6990 case DOCUMENTREFERENCE: 6991 return "DocumentReference"; 6992 case DOMAINRESOURCE: 6993 return "DomainResource"; 6994 case EFFECTEVIDENCESYNTHESIS: 6995 return "EffectEvidenceSynthesis"; 6996 case ENCOUNTER: 6997 return "Encounter"; 6998 case ENDPOINT: 6999 return "Endpoint"; 7000 case ENROLLMENTREQUEST: 7001 return "EnrollmentRequest"; 7002 case ENROLLMENTRESPONSE: 7003 return "EnrollmentResponse"; 7004 case EPISODEOFCARE: 7005 return "EpisodeOfCare"; 7006 case EVENTDEFINITION: 7007 return "EventDefinition"; 7008 case EVIDENCE: 7009 return "Evidence"; 7010 case EVIDENCEVARIABLE: 7011 return "EvidenceVariable"; 7012 case EXAMPLESCENARIO: 7013 return "ExampleScenario"; 7014 case EXPLANATIONOFBENEFIT: 7015 return "ExplanationOfBenefit"; 7016 case FAMILYMEMBERHISTORY: 7017 return "FamilyMemberHistory"; 7018 case FLAG: 7019 return "Flag"; 7020 case GOAL: 7021 return "Goal"; 7022 case GRAPHDEFINITION: 7023 return "GraphDefinition"; 7024 case GROUP: 7025 return "Group"; 7026 case GUIDANCERESPONSE: 7027 return "GuidanceResponse"; 7028 case HEALTHCARESERVICE: 7029 return "HealthcareService"; 7030 case IMAGINGSTUDY: 7031 return "ImagingStudy"; 7032 case IMMUNIZATION: 7033 return "Immunization"; 7034 case IMMUNIZATIONEVALUATION: 7035 return "ImmunizationEvaluation"; 7036 case IMMUNIZATIONRECOMMENDATION: 7037 return "ImmunizationRecommendation"; 7038 case IMPLEMENTATIONGUIDE: 7039 return "ImplementationGuide"; 7040 case INSURANCEPLAN: 7041 return "InsurancePlan"; 7042 case INVOICE: 7043 return "Invoice"; 7044 case LIBRARY: 7045 return "Library"; 7046 case LINKAGE: 7047 return "Linkage"; 7048 case LIST: 7049 return "List"; 7050 case LOCATION: 7051 return "Location"; 7052 case MEASURE: 7053 return "Measure"; 7054 case MEASUREREPORT: 7055 return "MeasureReport"; 7056 case MEDIA: 7057 return "Media"; 7058 case MEDICATION: 7059 return "Medication"; 7060 case MEDICATIONADMINISTRATION: 7061 return "MedicationAdministration"; 7062 case MEDICATIONDISPENSE: 7063 return "MedicationDispense"; 7064 case MEDICATIONKNOWLEDGE: 7065 return "MedicationKnowledge"; 7066 case MEDICATIONREQUEST: 7067 return "MedicationRequest"; 7068 case MEDICATIONSTATEMENT: 7069 return "MedicationStatement"; 7070 case MEDICINALPRODUCT: 7071 return "MedicinalProduct"; 7072 case MEDICINALPRODUCTAUTHORIZATION: 7073 return "MedicinalProductAuthorization"; 7074 case MEDICINALPRODUCTCONTRAINDICATION: 7075 return "MedicinalProductContraindication"; 7076 case MEDICINALPRODUCTINDICATION: 7077 return "MedicinalProductIndication"; 7078 case MEDICINALPRODUCTINGREDIENT: 7079 return "MedicinalProductIngredient"; 7080 case MEDICINALPRODUCTINTERACTION: 7081 return "MedicinalProductInteraction"; 7082 case MEDICINALPRODUCTMANUFACTURED: 7083 return "MedicinalProductManufactured"; 7084 case MEDICINALPRODUCTPACKAGED: 7085 return "MedicinalProductPackaged"; 7086 case MEDICINALPRODUCTPHARMACEUTICAL: 7087 return "MedicinalProductPharmaceutical"; 7088 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 7089 return "MedicinalProductUndesirableEffect"; 7090 case MESSAGEDEFINITION: 7091 return "MessageDefinition"; 7092 case MESSAGEHEADER: 7093 return "MessageHeader"; 7094 case MOLECULARSEQUENCE: 7095 return "MolecularSequence"; 7096 case NAMINGSYSTEM: 7097 return "NamingSystem"; 7098 case NUTRITIONORDER: 7099 return "NutritionOrder"; 7100 case OBSERVATION: 7101 return "Observation"; 7102 case OBSERVATIONDEFINITION: 7103 return "ObservationDefinition"; 7104 case OPERATIONDEFINITION: 7105 return "OperationDefinition"; 7106 case OPERATIONOUTCOME: 7107 return "OperationOutcome"; 7108 case ORGANIZATION: 7109 return "Organization"; 7110 case ORGANIZATIONAFFILIATION: 7111 return "OrganizationAffiliation"; 7112 case PARAMETERS: 7113 return "Parameters"; 7114 case PATIENT: 7115 return "Patient"; 7116 case PAYMENTNOTICE: 7117 return "PaymentNotice"; 7118 case PAYMENTRECONCILIATION: 7119 return "PaymentReconciliation"; 7120 case PERSON: 7121 return "Person"; 7122 case PLANDEFINITION: 7123 return "PlanDefinition"; 7124 case PRACTITIONER: 7125 return "Practitioner"; 7126 case PRACTITIONERROLE: 7127 return "PractitionerRole"; 7128 case PROCEDURE: 7129 return "Procedure"; 7130 case PROVENANCE: 7131 return "Provenance"; 7132 case QUESTIONNAIRE: 7133 return "Questionnaire"; 7134 case QUESTIONNAIRERESPONSE: 7135 return "QuestionnaireResponse"; 7136 case RELATEDPERSON: 7137 return "RelatedPerson"; 7138 case REQUESTGROUP: 7139 return "RequestGroup"; 7140 case RESEARCHDEFINITION: 7141 return "ResearchDefinition"; 7142 case RESEARCHELEMENTDEFINITION: 7143 return "ResearchElementDefinition"; 7144 case RESEARCHSTUDY: 7145 return "ResearchStudy"; 7146 case RESEARCHSUBJECT: 7147 return "ResearchSubject"; 7148 case RESOURCE: 7149 return "Resource"; 7150 case RISKASSESSMENT: 7151 return "RiskAssessment"; 7152 case RISKEVIDENCESYNTHESIS: 7153 return "RiskEvidenceSynthesis"; 7154 case SCHEDULE: 7155 return "Schedule"; 7156 case SEARCHPARAMETER: 7157 return "SearchParameter"; 7158 case SERVICEREQUEST: 7159 return "ServiceRequest"; 7160 case SLOT: 7161 return "Slot"; 7162 case SPECIMEN: 7163 return "Specimen"; 7164 case SPECIMENDEFINITION: 7165 return "SpecimenDefinition"; 7166 case STRUCTUREDEFINITION: 7167 return "StructureDefinition"; 7168 case STRUCTUREMAP: 7169 return "StructureMap"; 7170 case SUBSCRIPTION: 7171 return "Subscription"; 7172 case SUBSTANCE: 7173 return "Substance"; 7174 case SUBSTANCENUCLEICACID: 7175 return "SubstanceNucleicAcid"; 7176 case SUBSTANCEPOLYMER: 7177 return "SubstancePolymer"; 7178 case SUBSTANCEPROTEIN: 7179 return "SubstanceProtein"; 7180 case SUBSTANCEREFERENCEINFORMATION: 7181 return "SubstanceReferenceInformation"; 7182 case SUBSTANCESOURCEMATERIAL: 7183 return "SubstanceSourceMaterial"; 7184 case SUBSTANCESPECIFICATION: 7185 return "SubstanceSpecification"; 7186 case SUPPLYDELIVERY: 7187 return "SupplyDelivery"; 7188 case SUPPLYREQUEST: 7189 return "SupplyRequest"; 7190 case TASK: 7191 return "Task"; 7192 case TERMINOLOGYCAPABILITIES: 7193 return "TerminologyCapabilities"; 7194 case TESTREPORT: 7195 return "TestReport"; 7196 case TESTSCRIPT: 7197 return "TestScript"; 7198 case VALUESET: 7199 return "ValueSet"; 7200 case VERIFICATIONRESULT: 7201 return "VerificationResult"; 7202 case VISIONPRESCRIPTION: 7203 return "VisionPrescription"; 7204 case TYPE: 7205 return "Type"; 7206 case ANY: 7207 return "Any"; 7208 case NULL: 7209 return null; 7210 default: 7211 return "?"; 7212 } 7213 } 7214 } 7215 7216 public static class FHIRAllTypesEnumFactory implements EnumFactory<FHIRAllTypes> { 7217 public FHIRAllTypes fromCode(String codeString) throws IllegalArgumentException { 7218 if (codeString == null || "".equals(codeString)) 7219 if (codeString == null || "".equals(codeString)) 7220 return null; 7221 if ("Address".equals(codeString)) 7222 return FHIRAllTypes.ADDRESS; 7223 if ("Age".equals(codeString)) 7224 return FHIRAllTypes.AGE; 7225 if ("Annotation".equals(codeString)) 7226 return FHIRAllTypes.ANNOTATION; 7227 if ("Attachment".equals(codeString)) 7228 return FHIRAllTypes.ATTACHMENT; 7229 if ("BackboneElement".equals(codeString)) 7230 return FHIRAllTypes.BACKBONEELEMENT; 7231 if ("CodeableConcept".equals(codeString)) 7232 return FHIRAllTypes.CODEABLECONCEPT; 7233 if ("Coding".equals(codeString)) 7234 return FHIRAllTypes.CODING; 7235 if ("ContactDetail".equals(codeString)) 7236 return FHIRAllTypes.CONTACTDETAIL; 7237 if ("ContactPoint".equals(codeString)) 7238 return FHIRAllTypes.CONTACTPOINT; 7239 if ("Contributor".equals(codeString)) 7240 return FHIRAllTypes.CONTRIBUTOR; 7241 if ("Count".equals(codeString)) 7242 return FHIRAllTypes.COUNT; 7243 if ("DataRequirement".equals(codeString)) 7244 return FHIRAllTypes.DATAREQUIREMENT; 7245 if ("Distance".equals(codeString)) 7246 return FHIRAllTypes.DISTANCE; 7247 if ("Dosage".equals(codeString)) 7248 return FHIRAllTypes.DOSAGE; 7249 if ("Duration".equals(codeString)) 7250 return FHIRAllTypes.DURATION; 7251 if ("Element".equals(codeString)) 7252 return FHIRAllTypes.ELEMENT; 7253 if ("ElementDefinition".equals(codeString)) 7254 return FHIRAllTypes.ELEMENTDEFINITION; 7255 if ("Expression".equals(codeString)) 7256 return FHIRAllTypes.EXPRESSION; 7257 if ("Extension".equals(codeString)) 7258 return FHIRAllTypes.EXTENSION; 7259 if ("HumanName".equals(codeString)) 7260 return FHIRAllTypes.HUMANNAME; 7261 if ("Identifier".equals(codeString)) 7262 return FHIRAllTypes.IDENTIFIER; 7263 if ("MarketingStatus".equals(codeString)) 7264 return FHIRAllTypes.MARKETINGSTATUS; 7265 if ("Meta".equals(codeString)) 7266 return FHIRAllTypes.META; 7267 if ("Money".equals(codeString)) 7268 return FHIRAllTypes.MONEY; 7269 if ("MoneyQuantity".equals(codeString)) 7270 return FHIRAllTypes.MONEYQUANTITY; 7271 if ("Narrative".equals(codeString)) 7272 return FHIRAllTypes.NARRATIVE; 7273 if ("ParameterDefinition".equals(codeString)) 7274 return FHIRAllTypes.PARAMETERDEFINITION; 7275 if ("Period".equals(codeString)) 7276 return FHIRAllTypes.PERIOD; 7277 if ("Population".equals(codeString)) 7278 return FHIRAllTypes.POPULATION; 7279 if ("ProdCharacteristic".equals(codeString)) 7280 return FHIRAllTypes.PRODCHARACTERISTIC; 7281 if ("ProductShelfLife".equals(codeString)) 7282 return FHIRAllTypes.PRODUCTSHELFLIFE; 7283 if ("Quantity".equals(codeString)) 7284 return FHIRAllTypes.QUANTITY; 7285 if ("Range".equals(codeString)) 7286 return FHIRAllTypes.RANGE; 7287 if ("Ratio".equals(codeString)) 7288 return FHIRAllTypes.RATIO; 7289 if ("Reference".equals(codeString)) 7290 return FHIRAllTypes.REFERENCE; 7291 if ("RelatedArtifact".equals(codeString)) 7292 return FHIRAllTypes.RELATEDARTIFACT; 7293 if ("SampledData".equals(codeString)) 7294 return FHIRAllTypes.SAMPLEDDATA; 7295 if ("Signature".equals(codeString)) 7296 return FHIRAllTypes.SIGNATURE; 7297 if ("SimpleQuantity".equals(codeString)) 7298 return FHIRAllTypes.SIMPLEQUANTITY; 7299 if ("SubstanceAmount".equals(codeString)) 7300 return FHIRAllTypes.SUBSTANCEAMOUNT; 7301 if ("Timing".equals(codeString)) 7302 return FHIRAllTypes.TIMING; 7303 if ("TriggerDefinition".equals(codeString)) 7304 return FHIRAllTypes.TRIGGERDEFINITION; 7305 if ("UsageContext".equals(codeString)) 7306 return FHIRAllTypes.USAGECONTEXT; 7307 if ("base64Binary".equals(codeString)) 7308 return FHIRAllTypes.BASE64BINARY; 7309 if ("boolean".equals(codeString)) 7310 return FHIRAllTypes.BOOLEAN; 7311 if ("canonical".equals(codeString)) 7312 return FHIRAllTypes.CANONICAL; 7313 if ("code".equals(codeString)) 7314 return FHIRAllTypes.CODE; 7315 if ("date".equals(codeString)) 7316 return FHIRAllTypes.DATE; 7317 if ("dateTime".equals(codeString)) 7318 return FHIRAllTypes.DATETIME; 7319 if ("decimal".equals(codeString)) 7320 return FHIRAllTypes.DECIMAL; 7321 if ("id".equals(codeString)) 7322 return FHIRAllTypes.ID; 7323 if ("instant".equals(codeString)) 7324 return FHIRAllTypes.INSTANT; 7325 if ("integer".equals(codeString)) 7326 return FHIRAllTypes.INTEGER; 7327 if ("markdown".equals(codeString)) 7328 return FHIRAllTypes.MARKDOWN; 7329 if ("oid".equals(codeString)) 7330 return FHIRAllTypes.OID; 7331 if ("positiveInt".equals(codeString)) 7332 return FHIRAllTypes.POSITIVEINT; 7333 if ("string".equals(codeString)) 7334 return FHIRAllTypes.STRING; 7335 if ("time".equals(codeString)) 7336 return FHIRAllTypes.TIME; 7337 if ("unsignedInt".equals(codeString)) 7338 return FHIRAllTypes.UNSIGNEDINT; 7339 if ("uri".equals(codeString)) 7340 return FHIRAllTypes.URI; 7341 if ("url".equals(codeString)) 7342 return FHIRAllTypes.URL; 7343 if ("uuid".equals(codeString)) 7344 return FHIRAllTypes.UUID; 7345 if ("xhtml".equals(codeString)) 7346 return FHIRAllTypes.XHTML; 7347 if ("Account".equals(codeString)) 7348 return FHIRAllTypes.ACCOUNT; 7349 if ("ActivityDefinition".equals(codeString)) 7350 return FHIRAllTypes.ACTIVITYDEFINITION; 7351 if ("AdverseEvent".equals(codeString)) 7352 return FHIRAllTypes.ADVERSEEVENT; 7353 if ("AllergyIntolerance".equals(codeString)) 7354 return FHIRAllTypes.ALLERGYINTOLERANCE; 7355 if ("Appointment".equals(codeString)) 7356 return FHIRAllTypes.APPOINTMENT; 7357 if ("AppointmentResponse".equals(codeString)) 7358 return FHIRAllTypes.APPOINTMENTRESPONSE; 7359 if ("AuditEvent".equals(codeString)) 7360 return FHIRAllTypes.AUDITEVENT; 7361 if ("Basic".equals(codeString)) 7362 return FHIRAllTypes.BASIC; 7363 if ("Binary".equals(codeString)) 7364 return FHIRAllTypes.BINARY; 7365 if ("BiologicallyDerivedProduct".equals(codeString)) 7366 return FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT; 7367 if ("BodyStructure".equals(codeString)) 7368 return FHIRAllTypes.BODYSTRUCTURE; 7369 if ("Bundle".equals(codeString)) 7370 return FHIRAllTypes.BUNDLE; 7371 if ("CapabilityStatement".equals(codeString)) 7372 return FHIRAllTypes.CAPABILITYSTATEMENT; 7373 if ("CarePlan".equals(codeString)) 7374 return FHIRAllTypes.CAREPLAN; 7375 if ("CareTeam".equals(codeString)) 7376 return FHIRAllTypes.CARETEAM; 7377 if ("CatalogEntry".equals(codeString)) 7378 return FHIRAllTypes.CATALOGENTRY; 7379 if ("ChargeItem".equals(codeString)) 7380 return FHIRAllTypes.CHARGEITEM; 7381 if ("ChargeItemDefinition".equals(codeString)) 7382 return FHIRAllTypes.CHARGEITEMDEFINITION; 7383 if ("Claim".equals(codeString)) 7384 return FHIRAllTypes.CLAIM; 7385 if ("ClaimResponse".equals(codeString)) 7386 return FHIRAllTypes.CLAIMRESPONSE; 7387 if ("ClinicalImpression".equals(codeString)) 7388 return FHIRAllTypes.CLINICALIMPRESSION; 7389 if ("CodeSystem".equals(codeString)) 7390 return FHIRAllTypes.CODESYSTEM; 7391 if ("Communication".equals(codeString)) 7392 return FHIRAllTypes.COMMUNICATION; 7393 if ("CommunicationRequest".equals(codeString)) 7394 return FHIRAllTypes.COMMUNICATIONREQUEST; 7395 if ("CompartmentDefinition".equals(codeString)) 7396 return FHIRAllTypes.COMPARTMENTDEFINITION; 7397 if ("Composition".equals(codeString)) 7398 return FHIRAllTypes.COMPOSITION; 7399 if ("ConceptMap".equals(codeString)) 7400 return FHIRAllTypes.CONCEPTMAP; 7401 if ("Condition".equals(codeString)) 7402 return FHIRAllTypes.CONDITION; 7403 if ("Consent".equals(codeString)) 7404 return FHIRAllTypes.CONSENT; 7405 if ("Contract".equals(codeString)) 7406 return FHIRAllTypes.CONTRACT; 7407 if ("Coverage".equals(codeString)) 7408 return FHIRAllTypes.COVERAGE; 7409 if ("CoverageEligibilityRequest".equals(codeString)) 7410 return FHIRAllTypes.COVERAGEELIGIBILITYREQUEST; 7411 if ("CoverageEligibilityResponse".equals(codeString)) 7412 return FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE; 7413 if ("DetectedIssue".equals(codeString)) 7414 return FHIRAllTypes.DETECTEDISSUE; 7415 if ("Device".equals(codeString)) 7416 return FHIRAllTypes.DEVICE; 7417 if ("DeviceDefinition".equals(codeString)) 7418 return FHIRAllTypes.DEVICEDEFINITION; 7419 if ("DeviceMetric".equals(codeString)) 7420 return FHIRAllTypes.DEVICEMETRIC; 7421 if ("DeviceRequest".equals(codeString)) 7422 return FHIRAllTypes.DEVICEREQUEST; 7423 if ("DeviceUseStatement".equals(codeString)) 7424 return FHIRAllTypes.DEVICEUSESTATEMENT; 7425 if ("DiagnosticReport".equals(codeString)) 7426 return FHIRAllTypes.DIAGNOSTICREPORT; 7427 if ("DocumentManifest".equals(codeString)) 7428 return FHIRAllTypes.DOCUMENTMANIFEST; 7429 if ("DocumentReference".equals(codeString)) 7430 return FHIRAllTypes.DOCUMENTREFERENCE; 7431 if ("DomainResource".equals(codeString)) 7432 return FHIRAllTypes.DOMAINRESOURCE; 7433 if ("EffectEvidenceSynthesis".equals(codeString)) 7434 return FHIRAllTypes.EFFECTEVIDENCESYNTHESIS; 7435 if ("Encounter".equals(codeString)) 7436 return FHIRAllTypes.ENCOUNTER; 7437 if ("Endpoint".equals(codeString)) 7438 return FHIRAllTypes.ENDPOINT; 7439 if ("EnrollmentRequest".equals(codeString)) 7440 return FHIRAllTypes.ENROLLMENTREQUEST; 7441 if ("EnrollmentResponse".equals(codeString)) 7442 return FHIRAllTypes.ENROLLMENTRESPONSE; 7443 if ("EpisodeOfCare".equals(codeString)) 7444 return FHIRAllTypes.EPISODEOFCARE; 7445 if ("EventDefinition".equals(codeString)) 7446 return FHIRAllTypes.EVENTDEFINITION; 7447 if ("Evidence".equals(codeString)) 7448 return FHIRAllTypes.EVIDENCE; 7449 if ("EvidenceVariable".equals(codeString)) 7450 return FHIRAllTypes.EVIDENCEVARIABLE; 7451 if ("ExampleScenario".equals(codeString)) 7452 return FHIRAllTypes.EXAMPLESCENARIO; 7453 if ("ExplanationOfBenefit".equals(codeString)) 7454 return FHIRAllTypes.EXPLANATIONOFBENEFIT; 7455 if ("FamilyMemberHistory".equals(codeString)) 7456 return FHIRAllTypes.FAMILYMEMBERHISTORY; 7457 if ("Flag".equals(codeString)) 7458 return FHIRAllTypes.FLAG; 7459 if ("Goal".equals(codeString)) 7460 return FHIRAllTypes.GOAL; 7461 if ("GraphDefinition".equals(codeString)) 7462 return FHIRAllTypes.GRAPHDEFINITION; 7463 if ("Group".equals(codeString)) 7464 return FHIRAllTypes.GROUP; 7465 if ("GuidanceResponse".equals(codeString)) 7466 return FHIRAllTypes.GUIDANCERESPONSE; 7467 if ("HealthcareService".equals(codeString)) 7468 return FHIRAllTypes.HEALTHCARESERVICE; 7469 if ("ImagingStudy".equals(codeString)) 7470 return FHIRAllTypes.IMAGINGSTUDY; 7471 if ("Immunization".equals(codeString)) 7472 return FHIRAllTypes.IMMUNIZATION; 7473 if ("ImmunizationEvaluation".equals(codeString)) 7474 return FHIRAllTypes.IMMUNIZATIONEVALUATION; 7475 if ("ImmunizationRecommendation".equals(codeString)) 7476 return FHIRAllTypes.IMMUNIZATIONRECOMMENDATION; 7477 if ("ImplementationGuide".equals(codeString)) 7478 return FHIRAllTypes.IMPLEMENTATIONGUIDE; 7479 if ("InsurancePlan".equals(codeString)) 7480 return FHIRAllTypes.INSURANCEPLAN; 7481 if ("Invoice".equals(codeString)) 7482 return FHIRAllTypes.INVOICE; 7483 if ("Library".equals(codeString)) 7484 return FHIRAllTypes.LIBRARY; 7485 if ("Linkage".equals(codeString)) 7486 return FHIRAllTypes.LINKAGE; 7487 if ("List".equals(codeString)) 7488 return FHIRAllTypes.LIST; 7489 if ("Location".equals(codeString)) 7490 return FHIRAllTypes.LOCATION; 7491 if ("Measure".equals(codeString)) 7492 return FHIRAllTypes.MEASURE; 7493 if ("MeasureReport".equals(codeString)) 7494 return FHIRAllTypes.MEASUREREPORT; 7495 if ("Media".equals(codeString)) 7496 return FHIRAllTypes.MEDIA; 7497 if ("Medication".equals(codeString)) 7498 return FHIRAllTypes.MEDICATION; 7499 if ("MedicationAdministration".equals(codeString)) 7500 return FHIRAllTypes.MEDICATIONADMINISTRATION; 7501 if ("MedicationDispense".equals(codeString)) 7502 return FHIRAllTypes.MEDICATIONDISPENSE; 7503 if ("MedicationKnowledge".equals(codeString)) 7504 return FHIRAllTypes.MEDICATIONKNOWLEDGE; 7505 if ("MedicationRequest".equals(codeString)) 7506 return FHIRAllTypes.MEDICATIONREQUEST; 7507 if ("MedicationStatement".equals(codeString)) 7508 return FHIRAllTypes.MEDICATIONSTATEMENT; 7509 if ("MedicinalProduct".equals(codeString)) 7510 return FHIRAllTypes.MEDICINALPRODUCT; 7511 if ("MedicinalProductAuthorization".equals(codeString)) 7512 return FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION; 7513 if ("MedicinalProductContraindication".equals(codeString)) 7514 return FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION; 7515 if ("MedicinalProductIndication".equals(codeString)) 7516 return FHIRAllTypes.MEDICINALPRODUCTINDICATION; 7517 if ("MedicinalProductIngredient".equals(codeString)) 7518 return FHIRAllTypes.MEDICINALPRODUCTINGREDIENT; 7519 if ("MedicinalProductInteraction".equals(codeString)) 7520 return FHIRAllTypes.MEDICINALPRODUCTINTERACTION; 7521 if ("MedicinalProductManufactured".equals(codeString)) 7522 return FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED; 7523 if ("MedicinalProductPackaged".equals(codeString)) 7524 return FHIRAllTypes.MEDICINALPRODUCTPACKAGED; 7525 if ("MedicinalProductPharmaceutical".equals(codeString)) 7526 return FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL; 7527 if ("MedicinalProductUndesirableEffect".equals(codeString)) 7528 return FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT; 7529 if ("MessageDefinition".equals(codeString)) 7530 return FHIRAllTypes.MESSAGEDEFINITION; 7531 if ("MessageHeader".equals(codeString)) 7532 return FHIRAllTypes.MESSAGEHEADER; 7533 if ("MolecularSequence".equals(codeString)) 7534 return FHIRAllTypes.MOLECULARSEQUENCE; 7535 if ("NamingSystem".equals(codeString)) 7536 return FHIRAllTypes.NAMINGSYSTEM; 7537 if ("NutritionOrder".equals(codeString)) 7538 return FHIRAllTypes.NUTRITIONORDER; 7539 if ("Observation".equals(codeString)) 7540 return FHIRAllTypes.OBSERVATION; 7541 if ("ObservationDefinition".equals(codeString)) 7542 return FHIRAllTypes.OBSERVATIONDEFINITION; 7543 if ("OperationDefinition".equals(codeString)) 7544 return FHIRAllTypes.OPERATIONDEFINITION; 7545 if ("OperationOutcome".equals(codeString)) 7546 return FHIRAllTypes.OPERATIONOUTCOME; 7547 if ("Organization".equals(codeString)) 7548 return FHIRAllTypes.ORGANIZATION; 7549 if ("OrganizationAffiliation".equals(codeString)) 7550 return FHIRAllTypes.ORGANIZATIONAFFILIATION; 7551 if ("Parameters".equals(codeString)) 7552 return FHIRAllTypes.PARAMETERS; 7553 if ("Patient".equals(codeString)) 7554 return FHIRAllTypes.PATIENT; 7555 if ("PaymentNotice".equals(codeString)) 7556 return FHIRAllTypes.PAYMENTNOTICE; 7557 if ("PaymentReconciliation".equals(codeString)) 7558 return FHIRAllTypes.PAYMENTRECONCILIATION; 7559 if ("Person".equals(codeString)) 7560 return FHIRAllTypes.PERSON; 7561 if ("PlanDefinition".equals(codeString)) 7562 return FHIRAllTypes.PLANDEFINITION; 7563 if ("Practitioner".equals(codeString)) 7564 return FHIRAllTypes.PRACTITIONER; 7565 if ("PractitionerRole".equals(codeString)) 7566 return FHIRAllTypes.PRACTITIONERROLE; 7567 if ("Procedure".equals(codeString)) 7568 return FHIRAllTypes.PROCEDURE; 7569 if ("Provenance".equals(codeString)) 7570 return FHIRAllTypes.PROVENANCE; 7571 if ("Questionnaire".equals(codeString)) 7572 return FHIRAllTypes.QUESTIONNAIRE; 7573 if ("QuestionnaireResponse".equals(codeString)) 7574 return FHIRAllTypes.QUESTIONNAIRERESPONSE; 7575 if ("RelatedPerson".equals(codeString)) 7576 return FHIRAllTypes.RELATEDPERSON; 7577 if ("RequestGroup".equals(codeString)) 7578 return FHIRAllTypes.REQUESTGROUP; 7579 if ("ResearchDefinition".equals(codeString)) 7580 return FHIRAllTypes.RESEARCHDEFINITION; 7581 if ("ResearchElementDefinition".equals(codeString)) 7582 return FHIRAllTypes.RESEARCHELEMENTDEFINITION; 7583 if ("ResearchStudy".equals(codeString)) 7584 return FHIRAllTypes.RESEARCHSTUDY; 7585 if ("ResearchSubject".equals(codeString)) 7586 return FHIRAllTypes.RESEARCHSUBJECT; 7587 if ("Resource".equals(codeString)) 7588 return FHIRAllTypes.RESOURCE; 7589 if ("RiskAssessment".equals(codeString)) 7590 return FHIRAllTypes.RISKASSESSMENT; 7591 if ("RiskEvidenceSynthesis".equals(codeString)) 7592 return FHIRAllTypes.RISKEVIDENCESYNTHESIS; 7593 if ("Schedule".equals(codeString)) 7594 return FHIRAllTypes.SCHEDULE; 7595 if ("SearchParameter".equals(codeString)) 7596 return FHIRAllTypes.SEARCHPARAMETER; 7597 if ("ServiceRequest".equals(codeString)) 7598 return FHIRAllTypes.SERVICEREQUEST; 7599 if ("Slot".equals(codeString)) 7600 return FHIRAllTypes.SLOT; 7601 if ("Specimen".equals(codeString)) 7602 return FHIRAllTypes.SPECIMEN; 7603 if ("SpecimenDefinition".equals(codeString)) 7604 return FHIRAllTypes.SPECIMENDEFINITION; 7605 if ("StructureDefinition".equals(codeString)) 7606 return FHIRAllTypes.STRUCTUREDEFINITION; 7607 if ("StructureMap".equals(codeString)) 7608 return FHIRAllTypes.STRUCTUREMAP; 7609 if ("Subscription".equals(codeString)) 7610 return FHIRAllTypes.SUBSCRIPTION; 7611 if ("Substance".equals(codeString)) 7612 return FHIRAllTypes.SUBSTANCE; 7613 if ("SubstanceNucleicAcid".equals(codeString)) 7614 return FHIRAllTypes.SUBSTANCENUCLEICACID; 7615 if ("SubstancePolymer".equals(codeString)) 7616 return FHIRAllTypes.SUBSTANCEPOLYMER; 7617 if ("SubstanceProtein".equals(codeString)) 7618 return FHIRAllTypes.SUBSTANCEPROTEIN; 7619 if ("SubstanceReferenceInformation".equals(codeString)) 7620 return FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION; 7621 if ("SubstanceSourceMaterial".equals(codeString)) 7622 return FHIRAllTypes.SUBSTANCESOURCEMATERIAL; 7623 if ("SubstanceSpecification".equals(codeString)) 7624 return FHIRAllTypes.SUBSTANCESPECIFICATION; 7625 if ("SupplyDelivery".equals(codeString)) 7626 return FHIRAllTypes.SUPPLYDELIVERY; 7627 if ("SupplyRequest".equals(codeString)) 7628 return FHIRAllTypes.SUPPLYREQUEST; 7629 if ("Task".equals(codeString)) 7630 return FHIRAllTypes.TASK; 7631 if ("TerminologyCapabilities".equals(codeString)) 7632 return FHIRAllTypes.TERMINOLOGYCAPABILITIES; 7633 if ("TestReport".equals(codeString)) 7634 return FHIRAllTypes.TESTREPORT; 7635 if ("TestScript".equals(codeString)) 7636 return FHIRAllTypes.TESTSCRIPT; 7637 if ("ValueSet".equals(codeString)) 7638 return FHIRAllTypes.VALUESET; 7639 if ("VerificationResult".equals(codeString)) 7640 return FHIRAllTypes.VERIFICATIONRESULT; 7641 if ("VisionPrescription".equals(codeString)) 7642 return FHIRAllTypes.VISIONPRESCRIPTION; 7643 if ("Type".equals(codeString)) 7644 return FHIRAllTypes.TYPE; 7645 if ("Any".equals(codeString)) 7646 return FHIRAllTypes.ANY; 7647 throw new IllegalArgumentException("Unknown FHIRAllTypes code '" + codeString + "'"); 7648 } 7649 7650 public Enumeration<FHIRAllTypes> fromType(PrimitiveType<?> code) throws FHIRException { 7651 if (code == null) 7652 return null; 7653 if (code.isEmpty()) 7654 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NULL, code); 7655 String codeString = code.asStringValue(); 7656 if (codeString == null || "".equals(codeString)) 7657 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NULL, code); 7658 if ("Address".equals(codeString)) 7659 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADDRESS, code); 7660 if ("Age".equals(codeString)) 7661 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AGE, code); 7662 if ("Annotation".equals(codeString)) 7663 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANNOTATION, code); 7664 if ("Attachment".equals(codeString)) 7665 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ATTACHMENT, code); 7666 if ("BackboneElement".equals(codeString)) 7667 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BACKBONEELEMENT, code); 7668 if ("CodeableConcept".equals(codeString)) 7669 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODEABLECONCEPT, code); 7670 if ("Coding".equals(codeString)) 7671 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODING, code); 7672 if ("ContactDetail".equals(codeString)) 7673 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTDETAIL, code); 7674 if ("ContactPoint".equals(codeString)) 7675 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTPOINT, code); 7676 if ("Contributor".equals(codeString)) 7677 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRIBUTOR, code); 7678 if ("Count".equals(codeString)) 7679 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COUNT, code); 7680 if ("DataRequirement".equals(codeString)) 7681 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAREQUIREMENT, code); 7682 if ("Distance".equals(codeString)) 7683 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DISTANCE, code); 7684 if ("Dosage".equals(codeString)) 7685 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOSAGE, code); 7686 if ("Duration".equals(codeString)) 7687 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DURATION, code); 7688 if ("Element".equals(codeString)) 7689 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENT, code); 7690 if ("ElementDefinition".equals(codeString)) 7691 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENTDEFINITION, code); 7692 if ("Expression".equals(codeString)) 7693 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPRESSION, code); 7694 if ("Extension".equals(codeString)) 7695 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXTENSION, code); 7696 if ("HumanName".equals(codeString)) 7697 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HUMANNAME, code); 7698 if ("Identifier".equals(codeString)) 7699 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IDENTIFIER, code); 7700 if ("MarketingStatus".equals(codeString)) 7701 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKETINGSTATUS, code); 7702 if ("Meta".equals(codeString)) 7703 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.META, code); 7704 if ("Money".equals(codeString)) 7705 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEY, code); 7706 if ("MoneyQuantity".equals(codeString)) 7707 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEYQUANTITY, code); 7708 if ("Narrative".equals(codeString)) 7709 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NARRATIVE, code); 7710 if ("ParameterDefinition".equals(codeString)) 7711 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERDEFINITION, code); 7712 if ("Period".equals(codeString)) 7713 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERIOD, code); 7714 if ("Population".equals(codeString)) 7715 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POPULATION, code); 7716 if ("ProdCharacteristic".equals(codeString)) 7717 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRODCHARACTERISTIC, code); 7718 if ("ProductShelfLife".equals(codeString)) 7719 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRODUCTSHELFLIFE, code); 7720 if ("Quantity".equals(codeString)) 7721 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUANTITY, code); 7722 if ("Range".equals(codeString)) 7723 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RANGE, code); 7724 if ("Ratio".equals(codeString)) 7725 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RATIO, code); 7726 if ("Reference".equals(codeString)) 7727 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERENCE, code); 7728 if ("RelatedArtifact".equals(codeString)) 7729 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDARTIFACT, code); 7730 if ("SampledData".equals(codeString)) 7731 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SAMPLEDDATA, code); 7732 if ("Signature".equals(codeString)) 7733 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIGNATURE, code); 7734 if ("SimpleQuantity".equals(codeString)) 7735 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIMPLEQUANTITY, code); 7736 if ("SubstanceAmount".equals(codeString)) 7737 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEAMOUNT, code); 7738 if ("Timing".equals(codeString)) 7739 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIMING, code); 7740 if ("TriggerDefinition".equals(codeString)) 7741 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TRIGGERDEFINITION, code); 7742 if ("UsageContext".equals(codeString)) 7743 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.USAGECONTEXT, code); 7744 if ("base64Binary".equals(codeString)) 7745 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASE64BINARY, code); 7746 if ("boolean".equals(codeString)) 7747 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BOOLEAN, code); 7748 if ("canonical".equals(codeString)) 7749 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CANONICAL, code); 7750 if ("code".equals(codeString)) 7751 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODE, code); 7752 if ("date".equals(codeString)) 7753 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATE, code); 7754 if ("dateTime".equals(codeString)) 7755 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATETIME, code); 7756 if ("decimal".equals(codeString)) 7757 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DECIMAL, code); 7758 if ("id".equals(codeString)) 7759 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ID, code); 7760 if ("instant".equals(codeString)) 7761 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSTANT, code); 7762 if ("integer".equals(codeString)) 7763 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INTEGER, code); 7764 if ("markdown".equals(codeString)) 7765 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKDOWN, code); 7766 if ("oid".equals(codeString)) 7767 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OID, code); 7768 if ("positiveInt".equals(codeString)) 7769 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POSITIVEINT, code); 7770 if ("string".equals(codeString)) 7771 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRING, code); 7772 if ("time".equals(codeString)) 7773 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIME, code); 7774 if ("unsignedInt".equals(codeString)) 7775 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UNSIGNEDINT, code); 7776 if ("uri".equals(codeString)) 7777 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URI, code); 7778 if ("url".equals(codeString)) 7779 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URL, code); 7780 if ("uuid".equals(codeString)) 7781 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UUID, code); 7782 if ("xhtml".equals(codeString)) 7783 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.XHTML, code); 7784 if ("Account".equals(codeString)) 7785 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACCOUNT, code); 7786 if ("ActivityDefinition".equals(codeString)) 7787 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACTIVITYDEFINITION, code); 7788 if ("AdverseEvent".equals(codeString)) 7789 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADVERSEEVENT, code); 7790 if ("AllergyIntolerance".equals(codeString)) 7791 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ALLERGYINTOLERANCE, code); 7792 if ("Appointment".equals(codeString)) 7793 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENT, code); 7794 if ("AppointmentResponse".equals(codeString)) 7795 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENTRESPONSE, code); 7796 if ("AuditEvent".equals(codeString)) 7797 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AUDITEVENT, code); 7798 if ("Basic".equals(codeString)) 7799 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASIC, code); 7800 if ("Binary".equals(codeString)) 7801 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BINARY, code); 7802 if ("BiologicallyDerivedProduct".equals(codeString)) 7803 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT, code); 7804 if ("BodyStructure".equals(codeString)) 7805 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BODYSTRUCTURE, code); 7806 if ("Bundle".equals(codeString)) 7807 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BUNDLE, code); 7808 if ("CapabilityStatement".equals(codeString)) 7809 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAPABILITYSTATEMENT, code); 7810 if ("CarePlan".equals(codeString)) 7811 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAREPLAN, code); 7812 if ("CareTeam".equals(codeString)) 7813 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CARETEAM, code); 7814 if ("CatalogEntry".equals(codeString)) 7815 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CATALOGENTRY, code); 7816 if ("ChargeItem".equals(codeString)) 7817 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEM, code); 7818 if ("ChargeItemDefinition".equals(codeString)) 7819 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEMDEFINITION, code); 7820 if ("Claim".equals(codeString)) 7821 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIM, code); 7822 if ("ClaimResponse".equals(codeString)) 7823 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIMRESPONSE, code); 7824 if ("ClinicalImpression".equals(codeString)) 7825 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLINICALIMPRESSION, code); 7826 if ("CodeSystem".equals(codeString)) 7827 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODESYSTEM, code); 7828 if ("Communication".equals(codeString)) 7829 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATION, code); 7830 if ("CommunicationRequest".equals(codeString)) 7831 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATIONREQUEST, code); 7832 if ("CompartmentDefinition".equals(codeString)) 7833 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPARTMENTDEFINITION, code); 7834 if ("Composition".equals(codeString)) 7835 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPOSITION, code); 7836 if ("ConceptMap".equals(codeString)) 7837 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONCEPTMAP, code); 7838 if ("Condition".equals(codeString)) 7839 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONDITION, code); 7840 if ("Consent".equals(codeString)) 7841 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONSENT, code); 7842 if ("Contract".equals(codeString)) 7843 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRACT, code); 7844 if ("Coverage".equals(codeString)) 7845 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGE, code); 7846 if ("CoverageEligibilityRequest".equals(codeString)) 7847 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGEELIGIBILITYREQUEST, code); 7848 if ("CoverageEligibilityResponse".equals(codeString)) 7849 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE, code); 7850 if ("DetectedIssue".equals(codeString)) 7851 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DETECTEDISSUE, code); 7852 if ("Device".equals(codeString)) 7853 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICE, code); 7854 if ("DeviceDefinition".equals(codeString)) 7855 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEDEFINITION, code); 7856 if ("DeviceMetric".equals(codeString)) 7857 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEMETRIC, code); 7858 if ("DeviceRequest".equals(codeString)) 7859 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEREQUEST, code); 7860 if ("DeviceUseStatement".equals(codeString)) 7861 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEUSESTATEMENT, code); 7862 if ("DiagnosticReport".equals(codeString)) 7863 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DIAGNOSTICREPORT, code); 7864 if ("DocumentManifest".equals(codeString)) 7865 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTMANIFEST, code); 7866 if ("DocumentReference".equals(codeString)) 7867 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTREFERENCE, code); 7868 if ("DomainResource".equals(codeString)) 7869 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOMAINRESOURCE, code); 7870 if ("EffectEvidenceSynthesis".equals(codeString)) 7871 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EFFECTEVIDENCESYNTHESIS, code); 7872 if ("Encounter".equals(codeString)) 7873 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENCOUNTER, code); 7874 if ("Endpoint".equals(codeString)) 7875 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENDPOINT, code); 7876 if ("EnrollmentRequest".equals(codeString)) 7877 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTREQUEST, code); 7878 if ("EnrollmentResponse".equals(codeString)) 7879 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTRESPONSE, code); 7880 if ("EpisodeOfCare".equals(codeString)) 7881 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EPISODEOFCARE, code); 7882 if ("EventDefinition".equals(codeString)) 7883 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVENTDEFINITION, code); 7884 if ("Evidence".equals(codeString)) 7885 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVIDENCE, code); 7886 if ("EvidenceVariable".equals(codeString)) 7887 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EVIDENCEVARIABLE, code); 7888 if ("ExampleScenario".equals(codeString)) 7889 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXAMPLESCENARIO, code); 7890 if ("ExplanationOfBenefit".equals(codeString)) 7891 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPLANATIONOFBENEFIT, code); 7892 if ("FamilyMemberHistory".equals(codeString)) 7893 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FAMILYMEMBERHISTORY, code); 7894 if ("Flag".equals(codeString)) 7895 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FLAG, code); 7896 if ("Goal".equals(codeString)) 7897 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GOAL, code); 7898 if ("GraphDefinition".equals(codeString)) 7899 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GRAPHDEFINITION, code); 7900 if ("Group".equals(codeString)) 7901 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GROUP, code); 7902 if ("GuidanceResponse".equals(codeString)) 7903 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GUIDANCERESPONSE, code); 7904 if ("HealthcareService".equals(codeString)) 7905 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HEALTHCARESERVICE, code); 7906 if ("ImagingStudy".equals(codeString)) 7907 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGSTUDY, code); 7908 if ("Immunization".equals(codeString)) 7909 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATION, code); 7910 if ("ImmunizationEvaluation".equals(codeString)) 7911 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONEVALUATION, code); 7912 if ("ImmunizationRecommendation".equals(codeString)) 7913 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONRECOMMENDATION, code); 7914 if ("ImplementationGuide".equals(codeString)) 7915 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMPLEMENTATIONGUIDE, code); 7916 if ("InsurancePlan".equals(codeString)) 7917 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSURANCEPLAN, code); 7918 if ("Invoice".equals(codeString)) 7919 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INVOICE, code); 7920 if ("Library".equals(codeString)) 7921 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIBRARY, code); 7922 if ("Linkage".equals(codeString)) 7923 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LINKAGE, code); 7924 if ("List".equals(codeString)) 7925 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIST, code); 7926 if ("Location".equals(codeString)) 7927 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LOCATION, code); 7928 if ("Measure".equals(codeString)) 7929 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASURE, code); 7930 if ("MeasureReport".equals(codeString)) 7931 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASUREREPORT, code); 7932 if ("Media".equals(codeString)) 7933 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDIA, code); 7934 if ("Medication".equals(codeString)) 7935 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATION, code); 7936 if ("MedicationAdministration".equals(codeString)) 7937 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONADMINISTRATION, code); 7938 if ("MedicationDispense".equals(codeString)) 7939 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONDISPENSE, code); 7940 if ("MedicationKnowledge".equals(codeString)) 7941 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONKNOWLEDGE, code); 7942 if ("MedicationRequest".equals(codeString)) 7943 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONREQUEST, code); 7944 if ("MedicationStatement".equals(codeString)) 7945 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONSTATEMENT, code); 7946 if ("MedicinalProduct".equals(codeString)) 7947 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCT, code); 7948 if ("MedicinalProductAuthorization".equals(codeString)) 7949 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION, code); 7950 if ("MedicinalProductContraindication".equals(codeString)) 7951 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION, code); 7952 if ("MedicinalProductIndication".equals(codeString)) 7953 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINDICATION, code); 7954 if ("MedicinalProductIngredient".equals(codeString)) 7955 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINGREDIENT, code); 7956 if ("MedicinalProductInteraction".equals(codeString)) 7957 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTINTERACTION, code); 7958 if ("MedicinalProductManufactured".equals(codeString)) 7959 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED, code); 7960 if ("MedicinalProductPackaged".equals(codeString)) 7961 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTPACKAGED, code); 7962 if ("MedicinalProductPharmaceutical".equals(codeString)) 7963 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL, code); 7964 if ("MedicinalProductUndesirableEffect".equals(codeString)) 7965 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 7966 if ("MessageDefinition".equals(codeString)) 7967 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEDEFINITION, code); 7968 if ("MessageHeader".equals(codeString)) 7969 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEHEADER, code); 7970 if ("MolecularSequence".equals(codeString)) 7971 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MOLECULARSEQUENCE, code); 7972 if ("NamingSystem".equals(codeString)) 7973 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NAMINGSYSTEM, code); 7974 if ("NutritionOrder".equals(codeString)) 7975 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NUTRITIONORDER, code); 7976 if ("Observation".equals(codeString)) 7977 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATION, code); 7978 if ("ObservationDefinition".equals(codeString)) 7979 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATIONDEFINITION, code); 7980 if ("OperationDefinition".equals(codeString)) 7981 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONDEFINITION, code); 7982 if ("OperationOutcome".equals(codeString)) 7983 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONOUTCOME, code); 7984 if ("Organization".equals(codeString)) 7985 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATION, code); 7986 if ("OrganizationAffiliation".equals(codeString)) 7987 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATIONAFFILIATION, code); 7988 if ("Parameters".equals(codeString)) 7989 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERS, code); 7990 if ("Patient".equals(codeString)) 7991 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PATIENT, code); 7992 if ("PaymentNotice".equals(codeString)) 7993 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTNOTICE, code); 7994 if ("PaymentReconciliation".equals(codeString)) 7995 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTRECONCILIATION, code); 7996 if ("Person".equals(codeString)) 7997 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERSON, code); 7998 if ("PlanDefinition".equals(codeString)) 7999 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PLANDEFINITION, code); 8000 if ("Practitioner".equals(codeString)) 8001 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONER, code); 8002 if ("PractitionerRole".equals(codeString)) 8003 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONERROLE, code); 8004 if ("Procedure".equals(codeString)) 8005 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDURE, code); 8006 if ("Provenance".equals(codeString)) 8007 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROVENANCE, code); 8008 if ("Questionnaire".equals(codeString)) 8009 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRE, code); 8010 if ("QuestionnaireResponse".equals(codeString)) 8011 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRERESPONSE, code); 8012 if ("RelatedPerson".equals(codeString)) 8013 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDPERSON, code); 8014 if ("RequestGroup".equals(codeString)) 8015 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REQUESTGROUP, code); 8016 if ("ResearchDefinition".equals(codeString)) 8017 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHDEFINITION, code); 8018 if ("ResearchElementDefinition".equals(codeString)) 8019 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHELEMENTDEFINITION, code); 8020 if ("ResearchStudy".equals(codeString)) 8021 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSTUDY, code); 8022 if ("ResearchSubject".equals(codeString)) 8023 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSUBJECT, code); 8024 if ("Resource".equals(codeString)) 8025 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESOURCE, code); 8026 if ("RiskAssessment".equals(codeString)) 8027 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKASSESSMENT, code); 8028 if ("RiskEvidenceSynthesis".equals(codeString)) 8029 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKEVIDENCESYNTHESIS, code); 8030 if ("Schedule".equals(codeString)) 8031 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SCHEDULE, code); 8032 if ("SearchParameter".equals(codeString)) 8033 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEARCHPARAMETER, code); 8034 if ("ServiceRequest".equals(codeString)) 8035 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SERVICEREQUEST, code); 8036 if ("Slot".equals(codeString)) 8037 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SLOT, code); 8038 if ("Specimen".equals(codeString)) 8039 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMEN, code); 8040 if ("SpecimenDefinition".equals(codeString)) 8041 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMENDEFINITION, code); 8042 if ("StructureDefinition".equals(codeString)) 8043 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREDEFINITION, code); 8044 if ("StructureMap".equals(codeString)) 8045 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREMAP, code); 8046 if ("Subscription".equals(codeString)) 8047 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSCRIPTION, code); 8048 if ("Substance".equals(codeString)) 8049 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCE, code); 8050 if ("SubstanceNucleicAcid".equals(codeString)) 8051 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCENUCLEICACID, code); 8052 if ("SubstancePolymer".equals(codeString)) 8053 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEPOLYMER, code); 8054 if ("SubstanceProtein".equals(codeString)) 8055 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEPROTEIN, code); 8056 if ("SubstanceReferenceInformation".equals(codeString)) 8057 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION, code); 8058 if ("SubstanceSourceMaterial".equals(codeString)) 8059 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCESOURCEMATERIAL, code); 8060 if ("SubstanceSpecification".equals(codeString)) 8061 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCESPECIFICATION, code); 8062 if ("SupplyDelivery".equals(codeString)) 8063 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYDELIVERY, code); 8064 if ("SupplyRequest".equals(codeString)) 8065 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYREQUEST, code); 8066 if ("Task".equals(codeString)) 8067 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TASK, code); 8068 if ("TerminologyCapabilities".equals(codeString)) 8069 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TERMINOLOGYCAPABILITIES, code); 8070 if ("TestReport".equals(codeString)) 8071 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTREPORT, code); 8072 if ("TestScript".equals(codeString)) 8073 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTSCRIPT, code); 8074 if ("ValueSet".equals(codeString)) 8075 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VALUESET, code); 8076 if ("VerificationResult".equals(codeString)) 8077 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VERIFICATIONRESULT, code); 8078 if ("VisionPrescription".equals(codeString)) 8079 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VISIONPRESCRIPTION, code); 8080 if ("Type".equals(codeString)) 8081 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TYPE, code); 8082 if ("Any".equals(codeString)) 8083 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANY, code); 8084 throw new FHIRException("Unknown FHIRAllTypes code '" + codeString + "'"); 8085 } 8086 8087 public String toCode(FHIRAllTypes code) { 8088 if (code == FHIRAllTypes.ADDRESS) 8089 return "Address"; 8090 if (code == FHIRAllTypes.AGE) 8091 return "Age"; 8092 if (code == FHIRAllTypes.ANNOTATION) 8093 return "Annotation"; 8094 if (code == FHIRAllTypes.ATTACHMENT) 8095 return "Attachment"; 8096 if (code == FHIRAllTypes.BACKBONEELEMENT) 8097 return "BackboneElement"; 8098 if (code == FHIRAllTypes.CODEABLECONCEPT) 8099 return "CodeableConcept"; 8100 if (code == FHIRAllTypes.CODING) 8101 return "Coding"; 8102 if (code == FHIRAllTypes.CONTACTDETAIL) 8103 return "ContactDetail"; 8104 if (code == FHIRAllTypes.CONTACTPOINT) 8105 return "ContactPoint"; 8106 if (code == FHIRAllTypes.CONTRIBUTOR) 8107 return "Contributor"; 8108 if (code == FHIRAllTypes.COUNT) 8109 return "Count"; 8110 if (code == FHIRAllTypes.DATAREQUIREMENT) 8111 return "DataRequirement"; 8112 if (code == FHIRAllTypes.DISTANCE) 8113 return "Distance"; 8114 if (code == FHIRAllTypes.DOSAGE) 8115 return "Dosage"; 8116 if (code == FHIRAllTypes.DURATION) 8117 return "Duration"; 8118 if (code == FHIRAllTypes.ELEMENT) 8119 return "Element"; 8120 if (code == FHIRAllTypes.ELEMENTDEFINITION) 8121 return "ElementDefinition"; 8122 if (code == FHIRAllTypes.EXPRESSION) 8123 return "Expression"; 8124 if (code == FHIRAllTypes.EXTENSION) 8125 return "Extension"; 8126 if (code == FHIRAllTypes.HUMANNAME) 8127 return "HumanName"; 8128 if (code == FHIRAllTypes.IDENTIFIER) 8129 return "Identifier"; 8130 if (code == FHIRAllTypes.MARKETINGSTATUS) 8131 return "MarketingStatus"; 8132 if (code == FHIRAllTypes.META) 8133 return "Meta"; 8134 if (code == FHIRAllTypes.MONEY) 8135 return "Money"; 8136 if (code == FHIRAllTypes.MONEYQUANTITY) 8137 return "MoneyQuantity"; 8138 if (code == FHIRAllTypes.NARRATIVE) 8139 return "Narrative"; 8140 if (code == FHIRAllTypes.PARAMETERDEFINITION) 8141 return "ParameterDefinition"; 8142 if (code == FHIRAllTypes.PERIOD) 8143 return "Period"; 8144 if (code == FHIRAllTypes.POPULATION) 8145 return "Population"; 8146 if (code == FHIRAllTypes.PRODCHARACTERISTIC) 8147 return "ProdCharacteristic"; 8148 if (code == FHIRAllTypes.PRODUCTSHELFLIFE) 8149 return "ProductShelfLife"; 8150 if (code == FHIRAllTypes.QUANTITY) 8151 return "Quantity"; 8152 if (code == FHIRAllTypes.RANGE) 8153 return "Range"; 8154 if (code == FHIRAllTypes.RATIO) 8155 return "Ratio"; 8156 if (code == FHIRAllTypes.REFERENCE) 8157 return "Reference"; 8158 if (code == FHIRAllTypes.RELATEDARTIFACT) 8159 return "RelatedArtifact"; 8160 if (code == FHIRAllTypes.SAMPLEDDATA) 8161 return "SampledData"; 8162 if (code == FHIRAllTypes.SIGNATURE) 8163 return "Signature"; 8164 if (code == FHIRAllTypes.SIMPLEQUANTITY) 8165 return "SimpleQuantity"; 8166 if (code == FHIRAllTypes.SUBSTANCEAMOUNT) 8167 return "SubstanceAmount"; 8168 if (code == FHIRAllTypes.TIMING) 8169 return "Timing"; 8170 if (code == FHIRAllTypes.TRIGGERDEFINITION) 8171 return "TriggerDefinition"; 8172 if (code == FHIRAllTypes.USAGECONTEXT) 8173 return "UsageContext"; 8174 if (code == FHIRAllTypes.BASE64BINARY) 8175 return "base64Binary"; 8176 if (code == FHIRAllTypes.BOOLEAN) 8177 return "boolean"; 8178 if (code == FHIRAllTypes.CANONICAL) 8179 return "canonical"; 8180 if (code == FHIRAllTypes.CODE) 8181 return "code"; 8182 if (code == FHIRAllTypes.DATE) 8183 return "date"; 8184 if (code == FHIRAllTypes.DATETIME) 8185 return "dateTime"; 8186 if (code == FHIRAllTypes.DECIMAL) 8187 return "decimal"; 8188 if (code == FHIRAllTypes.ID) 8189 return "id"; 8190 if (code == FHIRAllTypes.INSTANT) 8191 return "instant"; 8192 if (code == FHIRAllTypes.INTEGER) 8193 return "integer"; 8194 if (code == FHIRAllTypes.MARKDOWN) 8195 return "markdown"; 8196 if (code == FHIRAllTypes.OID) 8197 return "oid"; 8198 if (code == FHIRAllTypes.POSITIVEINT) 8199 return "positiveInt"; 8200 if (code == FHIRAllTypes.STRING) 8201 return "string"; 8202 if (code == FHIRAllTypes.TIME) 8203 return "time"; 8204 if (code == FHIRAllTypes.UNSIGNEDINT) 8205 return "unsignedInt"; 8206 if (code == FHIRAllTypes.URI) 8207 return "uri"; 8208 if (code == FHIRAllTypes.URL) 8209 return "url"; 8210 if (code == FHIRAllTypes.UUID) 8211 return "uuid"; 8212 if (code == FHIRAllTypes.XHTML) 8213 return "xhtml"; 8214 if (code == FHIRAllTypes.ACCOUNT) 8215 return "Account"; 8216 if (code == FHIRAllTypes.ACTIVITYDEFINITION) 8217 return "ActivityDefinition"; 8218 if (code == FHIRAllTypes.ADVERSEEVENT) 8219 return "AdverseEvent"; 8220 if (code == FHIRAllTypes.ALLERGYINTOLERANCE) 8221 return "AllergyIntolerance"; 8222 if (code == FHIRAllTypes.APPOINTMENT) 8223 return "Appointment"; 8224 if (code == FHIRAllTypes.APPOINTMENTRESPONSE) 8225 return "AppointmentResponse"; 8226 if (code == FHIRAllTypes.AUDITEVENT) 8227 return "AuditEvent"; 8228 if (code == FHIRAllTypes.BASIC) 8229 return "Basic"; 8230 if (code == FHIRAllTypes.BINARY) 8231 return "Binary"; 8232 if (code == FHIRAllTypes.BIOLOGICALLYDERIVEDPRODUCT) 8233 return "BiologicallyDerivedProduct"; 8234 if (code == FHIRAllTypes.BODYSTRUCTURE) 8235 return "BodyStructure"; 8236 if (code == FHIRAllTypes.BUNDLE) 8237 return "Bundle"; 8238 if (code == FHIRAllTypes.CAPABILITYSTATEMENT) 8239 return "CapabilityStatement"; 8240 if (code == FHIRAllTypes.CAREPLAN) 8241 return "CarePlan"; 8242 if (code == FHIRAllTypes.CARETEAM) 8243 return "CareTeam"; 8244 if (code == FHIRAllTypes.CATALOGENTRY) 8245 return "CatalogEntry"; 8246 if (code == FHIRAllTypes.CHARGEITEM) 8247 return "ChargeItem"; 8248 if (code == FHIRAllTypes.CHARGEITEMDEFINITION) 8249 return "ChargeItemDefinition"; 8250 if (code == FHIRAllTypes.CLAIM) 8251 return "Claim"; 8252 if (code == FHIRAllTypes.CLAIMRESPONSE) 8253 return "ClaimResponse"; 8254 if (code == FHIRAllTypes.CLINICALIMPRESSION) 8255 return "ClinicalImpression"; 8256 if (code == FHIRAllTypes.CODESYSTEM) 8257 return "CodeSystem"; 8258 if (code == FHIRAllTypes.COMMUNICATION) 8259 return "Communication"; 8260 if (code == FHIRAllTypes.COMMUNICATIONREQUEST) 8261 return "CommunicationRequest"; 8262 if (code == FHIRAllTypes.COMPARTMENTDEFINITION) 8263 return "CompartmentDefinition"; 8264 if (code == FHIRAllTypes.COMPOSITION) 8265 return "Composition"; 8266 if (code == FHIRAllTypes.CONCEPTMAP) 8267 return "ConceptMap"; 8268 if (code == FHIRAllTypes.CONDITION) 8269 return "Condition"; 8270 if (code == FHIRAllTypes.CONSENT) 8271 return "Consent"; 8272 if (code == FHIRAllTypes.CONTRACT) 8273 return "Contract"; 8274 if (code == FHIRAllTypes.COVERAGE) 8275 return "Coverage"; 8276 if (code == FHIRAllTypes.COVERAGEELIGIBILITYREQUEST) 8277 return "CoverageEligibilityRequest"; 8278 if (code == FHIRAllTypes.COVERAGEELIGIBILITYRESPONSE) 8279 return "CoverageEligibilityResponse"; 8280 if (code == FHIRAllTypes.DETECTEDISSUE) 8281 return "DetectedIssue"; 8282 if (code == FHIRAllTypes.DEVICE) 8283 return "Device"; 8284 if (code == FHIRAllTypes.DEVICEDEFINITION) 8285 return "DeviceDefinition"; 8286 if (code == FHIRAllTypes.DEVICEMETRIC) 8287 return "DeviceMetric"; 8288 if (code == FHIRAllTypes.DEVICEREQUEST) 8289 return "DeviceRequest"; 8290 if (code == FHIRAllTypes.DEVICEUSESTATEMENT) 8291 return "DeviceUseStatement"; 8292 if (code == FHIRAllTypes.DIAGNOSTICREPORT) 8293 return "DiagnosticReport"; 8294 if (code == FHIRAllTypes.DOCUMENTMANIFEST) 8295 return "DocumentManifest"; 8296 if (code == FHIRAllTypes.DOCUMENTREFERENCE) 8297 return "DocumentReference"; 8298 if (code == FHIRAllTypes.DOMAINRESOURCE) 8299 return "DomainResource"; 8300 if (code == FHIRAllTypes.EFFECTEVIDENCESYNTHESIS) 8301 return "EffectEvidenceSynthesis"; 8302 if (code == FHIRAllTypes.ENCOUNTER) 8303 return "Encounter"; 8304 if (code == FHIRAllTypes.ENDPOINT) 8305 return "Endpoint"; 8306 if (code == FHIRAllTypes.ENROLLMENTREQUEST) 8307 return "EnrollmentRequest"; 8308 if (code == FHIRAllTypes.ENROLLMENTRESPONSE) 8309 return "EnrollmentResponse"; 8310 if (code == FHIRAllTypes.EPISODEOFCARE) 8311 return "EpisodeOfCare"; 8312 if (code == FHIRAllTypes.EVENTDEFINITION) 8313 return "EventDefinition"; 8314 if (code == FHIRAllTypes.EVIDENCE) 8315 return "Evidence"; 8316 if (code == FHIRAllTypes.EVIDENCEVARIABLE) 8317 return "EvidenceVariable"; 8318 if (code == FHIRAllTypes.EXAMPLESCENARIO) 8319 return "ExampleScenario"; 8320 if (code == FHIRAllTypes.EXPLANATIONOFBENEFIT) 8321 return "ExplanationOfBenefit"; 8322 if (code == FHIRAllTypes.FAMILYMEMBERHISTORY) 8323 return "FamilyMemberHistory"; 8324 if (code == FHIRAllTypes.FLAG) 8325 return "Flag"; 8326 if (code == FHIRAllTypes.GOAL) 8327 return "Goal"; 8328 if (code == FHIRAllTypes.GRAPHDEFINITION) 8329 return "GraphDefinition"; 8330 if (code == FHIRAllTypes.GROUP) 8331 return "Group"; 8332 if (code == FHIRAllTypes.GUIDANCERESPONSE) 8333 return "GuidanceResponse"; 8334 if (code == FHIRAllTypes.HEALTHCARESERVICE) 8335 return "HealthcareService"; 8336 if (code == FHIRAllTypes.IMAGINGSTUDY) 8337 return "ImagingStudy"; 8338 if (code == FHIRAllTypes.IMMUNIZATION) 8339 return "Immunization"; 8340 if (code == FHIRAllTypes.IMMUNIZATIONEVALUATION) 8341 return "ImmunizationEvaluation"; 8342 if (code == FHIRAllTypes.IMMUNIZATIONRECOMMENDATION) 8343 return "ImmunizationRecommendation"; 8344 if (code == FHIRAllTypes.IMPLEMENTATIONGUIDE) 8345 return "ImplementationGuide"; 8346 if (code == FHIRAllTypes.INSURANCEPLAN) 8347 return "InsurancePlan"; 8348 if (code == FHIRAllTypes.INVOICE) 8349 return "Invoice"; 8350 if (code == FHIRAllTypes.LIBRARY) 8351 return "Library"; 8352 if (code == FHIRAllTypes.LINKAGE) 8353 return "Linkage"; 8354 if (code == FHIRAllTypes.LIST) 8355 return "List"; 8356 if (code == FHIRAllTypes.LOCATION) 8357 return "Location"; 8358 if (code == FHIRAllTypes.MEASURE) 8359 return "Measure"; 8360 if (code == FHIRAllTypes.MEASUREREPORT) 8361 return "MeasureReport"; 8362 if (code == FHIRAllTypes.MEDIA) 8363 return "Media"; 8364 if (code == FHIRAllTypes.MEDICATION) 8365 return "Medication"; 8366 if (code == FHIRAllTypes.MEDICATIONADMINISTRATION) 8367 return "MedicationAdministration"; 8368 if (code == FHIRAllTypes.MEDICATIONDISPENSE) 8369 return "MedicationDispense"; 8370 if (code == FHIRAllTypes.MEDICATIONKNOWLEDGE) 8371 return "MedicationKnowledge"; 8372 if (code == FHIRAllTypes.MEDICATIONREQUEST) 8373 return "MedicationRequest"; 8374 if (code == FHIRAllTypes.MEDICATIONSTATEMENT) 8375 return "MedicationStatement"; 8376 if (code == FHIRAllTypes.MEDICINALPRODUCT) 8377 return "MedicinalProduct"; 8378 if (code == FHIRAllTypes.MEDICINALPRODUCTAUTHORIZATION) 8379 return "MedicinalProductAuthorization"; 8380 if (code == FHIRAllTypes.MEDICINALPRODUCTCONTRAINDICATION) 8381 return "MedicinalProductContraindication"; 8382 if (code == FHIRAllTypes.MEDICINALPRODUCTINDICATION) 8383 return "MedicinalProductIndication"; 8384 if (code == FHIRAllTypes.MEDICINALPRODUCTINGREDIENT) 8385 return "MedicinalProductIngredient"; 8386 if (code == FHIRAllTypes.MEDICINALPRODUCTINTERACTION) 8387 return "MedicinalProductInteraction"; 8388 if (code == FHIRAllTypes.MEDICINALPRODUCTMANUFACTURED) 8389 return "MedicinalProductManufactured"; 8390 if (code == FHIRAllTypes.MEDICINALPRODUCTPACKAGED) 8391 return "MedicinalProductPackaged"; 8392 if (code == FHIRAllTypes.MEDICINALPRODUCTPHARMACEUTICAL) 8393 return "MedicinalProductPharmaceutical"; 8394 if (code == FHIRAllTypes.MEDICINALPRODUCTUNDESIRABLEEFFECT) 8395 return "MedicinalProductUndesirableEffect"; 8396 if (code == FHIRAllTypes.MESSAGEDEFINITION) 8397 return "MessageDefinition"; 8398 if (code == FHIRAllTypes.MESSAGEHEADER) 8399 return "MessageHeader"; 8400 if (code == FHIRAllTypes.MOLECULARSEQUENCE) 8401 return "MolecularSequence"; 8402 if (code == FHIRAllTypes.NAMINGSYSTEM) 8403 return "NamingSystem"; 8404 if (code == FHIRAllTypes.NUTRITIONORDER) 8405 return "NutritionOrder"; 8406 if (code == FHIRAllTypes.OBSERVATION) 8407 return "Observation"; 8408 if (code == FHIRAllTypes.OBSERVATIONDEFINITION) 8409 return "ObservationDefinition"; 8410 if (code == FHIRAllTypes.OPERATIONDEFINITION) 8411 return "OperationDefinition"; 8412 if (code == FHIRAllTypes.OPERATIONOUTCOME) 8413 return "OperationOutcome"; 8414 if (code == FHIRAllTypes.ORGANIZATION) 8415 return "Organization"; 8416 if (code == FHIRAllTypes.ORGANIZATIONAFFILIATION) 8417 return "OrganizationAffiliation"; 8418 if (code == FHIRAllTypes.PARAMETERS) 8419 return "Parameters"; 8420 if (code == FHIRAllTypes.PATIENT) 8421 return "Patient"; 8422 if (code == FHIRAllTypes.PAYMENTNOTICE) 8423 return "PaymentNotice"; 8424 if (code == FHIRAllTypes.PAYMENTRECONCILIATION) 8425 return "PaymentReconciliation"; 8426 if (code == FHIRAllTypes.PERSON) 8427 return "Person"; 8428 if (code == FHIRAllTypes.PLANDEFINITION) 8429 return "PlanDefinition"; 8430 if (code == FHIRAllTypes.PRACTITIONER) 8431 return "Practitioner"; 8432 if (code == FHIRAllTypes.PRACTITIONERROLE) 8433 return "PractitionerRole"; 8434 if (code == FHIRAllTypes.PROCEDURE) 8435 return "Procedure"; 8436 if (code == FHIRAllTypes.PROVENANCE) 8437 return "Provenance"; 8438 if (code == FHIRAllTypes.QUESTIONNAIRE) 8439 return "Questionnaire"; 8440 if (code == FHIRAllTypes.QUESTIONNAIRERESPONSE) 8441 return "QuestionnaireResponse"; 8442 if (code == FHIRAllTypes.RELATEDPERSON) 8443 return "RelatedPerson"; 8444 if (code == FHIRAllTypes.REQUESTGROUP) 8445 return "RequestGroup"; 8446 if (code == FHIRAllTypes.RESEARCHDEFINITION) 8447 return "ResearchDefinition"; 8448 if (code == FHIRAllTypes.RESEARCHELEMENTDEFINITION) 8449 return "ResearchElementDefinition"; 8450 if (code == FHIRAllTypes.RESEARCHSTUDY) 8451 return "ResearchStudy"; 8452 if (code == FHIRAllTypes.RESEARCHSUBJECT) 8453 return "ResearchSubject"; 8454 if (code == FHIRAllTypes.RESOURCE) 8455 return "Resource"; 8456 if (code == FHIRAllTypes.RISKASSESSMENT) 8457 return "RiskAssessment"; 8458 if (code == FHIRAllTypes.RISKEVIDENCESYNTHESIS) 8459 return "RiskEvidenceSynthesis"; 8460 if (code == FHIRAllTypes.SCHEDULE) 8461 return "Schedule"; 8462 if (code == FHIRAllTypes.SEARCHPARAMETER) 8463 return "SearchParameter"; 8464 if (code == FHIRAllTypes.SERVICEREQUEST) 8465 return "ServiceRequest"; 8466 if (code == FHIRAllTypes.SLOT) 8467 return "Slot"; 8468 if (code == FHIRAllTypes.SPECIMEN) 8469 return "Specimen"; 8470 if (code == FHIRAllTypes.SPECIMENDEFINITION) 8471 return "SpecimenDefinition"; 8472 if (code == FHIRAllTypes.STRUCTUREDEFINITION) 8473 return "StructureDefinition"; 8474 if (code == FHIRAllTypes.STRUCTUREMAP) 8475 return "StructureMap"; 8476 if (code == FHIRAllTypes.SUBSCRIPTION) 8477 return "Subscription"; 8478 if (code == FHIRAllTypes.SUBSTANCE) 8479 return "Substance"; 8480 if (code == FHIRAllTypes.SUBSTANCENUCLEICACID) 8481 return "SubstanceNucleicAcid"; 8482 if (code == FHIRAllTypes.SUBSTANCEPOLYMER) 8483 return "SubstancePolymer"; 8484 if (code == FHIRAllTypes.SUBSTANCEPROTEIN) 8485 return "SubstanceProtein"; 8486 if (code == FHIRAllTypes.SUBSTANCEREFERENCEINFORMATION) 8487 return "SubstanceReferenceInformation"; 8488 if (code == FHIRAllTypes.SUBSTANCESOURCEMATERIAL) 8489 return "SubstanceSourceMaterial"; 8490 if (code == FHIRAllTypes.SUBSTANCESPECIFICATION) 8491 return "SubstanceSpecification"; 8492 if (code == FHIRAllTypes.SUPPLYDELIVERY) 8493 return "SupplyDelivery"; 8494 if (code == FHIRAllTypes.SUPPLYREQUEST) 8495 return "SupplyRequest"; 8496 if (code == FHIRAllTypes.TASK) 8497 return "Task"; 8498 if (code == FHIRAllTypes.TERMINOLOGYCAPABILITIES) 8499 return "TerminologyCapabilities"; 8500 if (code == FHIRAllTypes.TESTREPORT) 8501 return "TestReport"; 8502 if (code == FHIRAllTypes.TESTSCRIPT) 8503 return "TestScript"; 8504 if (code == FHIRAllTypes.VALUESET) 8505 return "ValueSet"; 8506 if (code == FHIRAllTypes.VERIFICATIONRESULT) 8507 return "VerificationResult"; 8508 if (code == FHIRAllTypes.VISIONPRESCRIPTION) 8509 return "VisionPrescription"; 8510 if (code == FHIRAllTypes.TYPE) 8511 return "Type"; 8512 if (code == FHIRAllTypes.ANY) 8513 return "Any"; 8514 return "?"; 8515 } 8516 8517 public String toSystem(FHIRAllTypes code) { 8518 return code.getSystem(); 8519 } 8520 } 8521 8522 public enum FHIRDefinedType { 8523 /** 8524 * An address expressed using postal conventions (as opposed to GPS or other 8525 * location definition formats). This data type may be used to convey addresses 8526 * for use in delivering mail as well as for visiting locations which might not 8527 * be valid for mail delivery. There are a variety of postal address formats 8528 * defined around the world. 8529 */ 8530 ADDRESS, 8531 /** 8532 * A duration of time during which an organism (or a process) has existed. 8533 */ 8534 AGE, 8535 /** 8536 * A text note which also contains information about who made the statement and 8537 * when. 8538 */ 8539 ANNOTATION, 8540 /** 8541 * For referring to data content defined in other formats. 8542 */ 8543 ATTACHMENT, 8544 /** 8545 * Base definition for all elements that are defined inside a resource - but not 8546 * those in a data type. 8547 */ 8548 BACKBONEELEMENT, 8549 /** 8550 * A concept that may be defined by a formal reference to a terminology or 8551 * ontology or may be provided by text. 8552 */ 8553 CODEABLECONCEPT, 8554 /** 8555 * A reference to a code defined by a terminology system. 8556 */ 8557 CODING, 8558 /** 8559 * Specifies contact information for a person or organization. 8560 */ 8561 CONTACTDETAIL, 8562 /** 8563 * Details for all kinds of technology mediated contact points for a person or 8564 * organization, including telephone, email, etc. 8565 */ 8566 CONTACTPOINT, 8567 /** 8568 * A contributor to the content of a knowledge asset, including authors, 8569 * editors, reviewers, and endorsers. 8570 */ 8571 CONTRIBUTOR, 8572 /** 8573 * A measured amount (or an amount that can potentially be measured). Note that 8574 * measured amounts include amounts that are not precisely quantified, including 8575 * amounts involving arbitrary units and floating currencies. 8576 */ 8577 COUNT, 8578 /** 8579 * Describes a required data item for evaluation in terms of the type of data, 8580 * and optional code or date-based filters of the data. 8581 */ 8582 DATAREQUIREMENT, 8583 /** 8584 * A length - a value with a unit that is a physical distance. 8585 */ 8586 DISTANCE, 8587 /** 8588 * Indicates how the medication is/was taken or should be taken by the patient. 8589 */ 8590 DOSAGE, 8591 /** 8592 * A length of time. 8593 */ 8594 DURATION, 8595 /** 8596 * Base definition for all elements in a resource. 8597 */ 8598 ELEMENT, 8599 /** 8600 * Captures constraints on each element within the resource, profile, or 8601 * extension. 8602 */ 8603 ELEMENTDEFINITION, 8604 /** 8605 * A expression that is evaluated in a specified context and returns a value. 8606 * The context of use of the expression must specify the context in which the 8607 * expression is evaluated, and how the result of the expression is used. 8608 */ 8609 EXPRESSION, 8610 /** 8611 * Optional Extension Element - found in all resources. 8612 */ 8613 EXTENSION, 8614 /** 8615 * A human's name with the ability to identify parts and usage. 8616 */ 8617 HUMANNAME, 8618 /** 8619 * An identifier - identifies some entity uniquely and unambiguously. Typically 8620 * this is used for business identifiers. 8621 */ 8622 IDENTIFIER, 8623 /** 8624 * The marketing status describes the date when a medicinal product is actually 8625 * put on the market or the date as of which it is no longer available. 8626 */ 8627 MARKETINGSTATUS, 8628 /** 8629 * The metadata about a resource. This is content in the resource that is 8630 * maintained by the infrastructure. Changes to the content might not always be 8631 * associated with version changes to the resource. 8632 */ 8633 META, 8634 /** 8635 * An amount of economic utility in some recognized currency. 8636 */ 8637 MONEY, 8638 /** 8639 * null 8640 */ 8641 MONEYQUANTITY, 8642 /** 8643 * A human-readable summary of the resource conveying the essential clinical and 8644 * business information for the resource. 8645 */ 8646 NARRATIVE, 8647 /** 8648 * The parameters to the module. This collection specifies both the input and 8649 * output parameters. Input parameters are provided by the caller as part of the 8650 * $evaluate operation. Output parameters are included in the GuidanceResponse. 8651 */ 8652 PARAMETERDEFINITION, 8653 /** 8654 * A time period defined by a start and end date and optionally time. 8655 */ 8656 PERIOD, 8657 /** 8658 * A populatioof people with some set of grouping criteria. 8659 */ 8660 POPULATION, 8661 /** 8662 * The marketing status describes the date when a medicinal product is actually 8663 * put on the market or the date as of which it is no longer available. 8664 */ 8665 PRODCHARACTERISTIC, 8666 /** 8667 * The shelf-life and storage information for a medicinal product item or 8668 * container can be described using this class. 8669 */ 8670 PRODUCTSHELFLIFE, 8671 /** 8672 * A measured amount (or an amount that can potentially be measured). Note that 8673 * measured amounts include amounts that are not precisely quantified, including 8674 * amounts involving arbitrary units and floating currencies. 8675 */ 8676 QUANTITY, 8677 /** 8678 * A set of ordered Quantities defined by a low and high limit. 8679 */ 8680 RANGE, 8681 /** 8682 * A relationship of two Quantity values - expressed as a numerator and a 8683 * denominator. 8684 */ 8685 RATIO, 8686 /** 8687 * A reference from one resource to another. 8688 */ 8689 REFERENCE, 8690 /** 8691 * Related artifacts such as additional documentation, justification, or 8692 * bibliographic references. 8693 */ 8694 RELATEDARTIFACT, 8695 /** 8696 * A series of measurements taken by a device, with upper and lower limits. 8697 * There may be more than one dimension in the data. 8698 */ 8699 SAMPLEDDATA, 8700 /** 8701 * A signature along with supporting context. The signature may be a digital 8702 * signature that is cryptographic in nature, or some other signature acceptable 8703 * to the domain. This other signature may be as simple as a graphical image 8704 * representing a hand-written signature, or a signature ceremony Different 8705 * signature approaches have different utilities. 8706 */ 8707 SIGNATURE, 8708 /** 8709 * null 8710 */ 8711 SIMPLEQUANTITY, 8712 /** 8713 * Chemical substances are a single substance type whose primary defining 8714 * element is the molecular structure. Chemical substances shall be defined on 8715 * the basis of their complete covalent molecular structure; the presence of a 8716 * salt (counter-ion) and/or solvates (water, alcohols) is also captured. 8717 * Purity, grade, physical form or particle size are not taken into account in 8718 * the definition of a chemical substance or in the assignment of a Substance 8719 * ID. 8720 */ 8721 SUBSTANCEAMOUNT, 8722 /** 8723 * Specifies an event that may occur multiple times. Timing schedules are used 8724 * to record when things are planned, expected or requested to occur. The most 8725 * common usage is in dosage instructions for medications. They are also used 8726 * when planning care of various kinds, and may be used for reporting the 8727 * schedule to which past regular activities were carried out. 8728 */ 8729 TIMING, 8730 /** 8731 * A description of a triggering event. Triggering events can be named events, 8732 * data events, or periodic, as determined by the type element. 8733 */ 8734 TRIGGERDEFINITION, 8735 /** 8736 * Specifies clinical/business/etc. metadata that can be used to retrieve, index 8737 * and/or categorize an artifact. This metadata can either be specific to the 8738 * applicable population (e.g., age category, DRG) or the specific context of 8739 * care (e.g., venue, care setting, provider of care). 8740 */ 8741 USAGECONTEXT, 8742 /** 8743 * A stream of bytes 8744 */ 8745 BASE64BINARY, 8746 /** 8747 * Value of "true" or "false" 8748 */ 8749 BOOLEAN, 8750 /** 8751 * A URI that is a reference to a canonical URL on a FHIR resource 8752 */ 8753 CANONICAL, 8754 /** 8755 * A string which has at least one character and no leading or trailing 8756 * whitespace and where there is no whitespace other than single spaces in the 8757 * contents 8758 */ 8759 CODE, 8760 /** 8761 * A date or partial date (e.g. just year or year + month). There is no time 8762 * zone. The format is a union of the schema types gYear, gYearMonth and date. 8763 * Dates SHALL be valid dates. 8764 */ 8765 DATE, 8766 /** 8767 * A date, date-time or partial date (e.g. just year or year + month). If hours 8768 * and minutes are specified, a time zone SHALL be populated. The format is a 8769 * union of the schema types gYear, gYearMonth, date and dateTime. Seconds must 8770 * be provided due to schema type constraints but may be zero-filled and may be 8771 * ignored. Dates SHALL be valid dates. 8772 */ 8773 DATETIME, 8774 /** 8775 * A rational number with implicit precision 8776 */ 8777 DECIMAL, 8778 /** 8779 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 8780 * characters. (This might be an integer, an unprefixed OID, UUID or any other 8781 * identifier pattern that meets these constraints.) Ids are case-insensitive. 8782 */ 8783 ID, 8784 /** 8785 * An instant in time - known at least to the second 8786 */ 8787 INSTANT, 8788 /** 8789 * A whole number 8790 */ 8791 INTEGER, 8792 /** 8793 * A string that may contain Github Flavored Markdown syntax for optional 8794 * processing by a mark down presentation engine 8795 */ 8796 MARKDOWN, 8797 /** 8798 * An OID represented as a URI 8799 */ 8800 OID, 8801 /** 8802 * An integer with a value that is positive (e.g. >0) 8803 */ 8804 POSITIVEINT, 8805 /** 8806 * A sequence of Unicode characters 8807 */ 8808 STRING, 8809 /** 8810 * A time during the day, with no date specified 8811 */ 8812 TIME, 8813 /** 8814 * An integer with a value that is not negative (e.g. >= 0) 8815 */ 8816 UNSIGNEDINT, 8817 /** 8818 * String of characters used to identify a name or a resource 8819 */ 8820 URI, 8821 /** 8822 * A URI that is a literal reference 8823 */ 8824 URL, 8825 /** 8826 * A UUID, represented as a URI 8827 */ 8828 UUID, 8829 /** 8830 * XHTML format, as defined by W3C, but restricted usage (mainly, no active 8831 * content) 8832 */ 8833 XHTML, 8834 /** 8835 * A financial tool for tracking value accrued for a particular purpose. In the 8836 * healthcare field, used to track charges for a patient, cost centers, etc. 8837 */ 8838 ACCOUNT, 8839 /** 8840 * This resource allows for the definition of some activity to be performed, 8841 * independent of a particular patient, practitioner, or other performance 8842 * context. 8843 */ 8844 ACTIVITYDEFINITION, 8845 /** 8846 * Actual or potential/avoided event causing unintended physical injury 8847 * resulting from or contributed to by medical care, a research study or other 8848 * healthcare setting factors that requires additional monitoring, treatment, or 8849 * hospitalization, or that results in death. 8850 */ 8851 ADVERSEEVENT, 8852 /** 8853 * Risk of harmful or undesirable, physiological response which is unique to an 8854 * individual and associated with exposure to a substance. 8855 */ 8856 ALLERGYINTOLERANCE, 8857 /** 8858 * A booking of a healthcare event among patient(s), practitioner(s), related 8859 * person(s) and/or device(s) for a specific date/time. This may result in one 8860 * or more Encounter(s). 8861 */ 8862 APPOINTMENT, 8863 /** 8864 * A reply to an appointment request for a patient and/or practitioner(s), such 8865 * as a confirmation or rejection. 8866 */ 8867 APPOINTMENTRESPONSE, 8868 /** 8869 * A record of an event made for purposes of maintaining a security log. Typical 8870 * uses include detection of intrusion attempts and monitoring for inappropriate 8871 * usage. 8872 */ 8873 AUDITEVENT, 8874 /** 8875 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 8876 * resources that don't map to an existing resource, and custom resources not 8877 * appropriate for inclusion in the FHIR specification. 8878 */ 8879 BASIC, 8880 /** 8881 * A resource that represents the data of a single raw artifact as digital 8882 * content accessible in its native format. A Binary resource can contain any 8883 * content, whether text, image, pdf, zip archive, etc. 8884 */ 8885 BINARY, 8886 /** 8887 * A material substance originating from a biological entity intended to be 8888 * transplanted or infused into another (possibly the same) biological entity. 8889 */ 8890 BIOLOGICALLYDERIVEDPRODUCT, 8891 /** 8892 * Record details about an anatomical structure. This resource may be used when 8893 * a coded concept does not provide the necessary detail needed for the use 8894 * case. 8895 */ 8896 BODYSTRUCTURE, 8897 /** 8898 * A container for a collection of resources. 8899 */ 8900 BUNDLE, 8901 /** 8902 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 8903 * Server for a particular version of FHIR that may be used as a statement of 8904 * actual server functionality or a statement of required or desired server 8905 * implementation. 8906 */ 8907 CAPABILITYSTATEMENT, 8908 /** 8909 * Describes the intention of how one or more practitioners intend to deliver 8910 * care for a particular patient, group or community for a period of time, 8911 * possibly limited to care for a specific condition or set of conditions. 8912 */ 8913 CAREPLAN, 8914 /** 8915 * The Care Team includes all the people and organizations who plan to 8916 * participate in the coordination and delivery of care for a patient. 8917 */ 8918 CARETEAM, 8919 /** 8920 * Catalog entries are wrappers that contextualize items included in a catalog. 8921 */ 8922 CATALOGENTRY, 8923 /** 8924 * The resource ChargeItem describes the provision of healthcare provider 8925 * products for a certain patient, therefore referring not only to the product, 8926 * but containing in addition details of the provision, like date, time, amounts 8927 * and participating organizations and persons. Main Usage of the ChargeItem is 8928 * to enable the billing process and internal cost allocation. 8929 */ 8930 CHARGEITEM, 8931 /** 8932 * The ChargeItemDefinition resource provides the properties that apply to the 8933 * (billing) codes necessary to calculate costs and prices. The properties may 8934 * differ largely depending on type and realm, therefore this resource gives 8935 * only a rough structure and requires profiling for each type of billing code 8936 * system. 8937 */ 8938 CHARGEITEMDEFINITION, 8939 /** 8940 * A provider issued list of professional services and products which have been 8941 * provided, or are to be provided, to a patient which is sent to an insurer for 8942 * reimbursement. 8943 */ 8944 CLAIM, 8945 /** 8946 * This resource provides the adjudication details from the processing of a 8947 * Claim resource. 8948 */ 8949 CLAIMRESPONSE, 8950 /** 8951 * A record of a clinical assessment performed to determine what problem(s) may 8952 * affect the patient and before planning the treatments or management 8953 * strategies that are best to manage a patient's condition. Assessments are 8954 * often 1:1 with a clinical consultation / encounter, but this varies greatly 8955 * depending on the clinical workflow. This resource is called 8956 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 8957 * the recording of assessment tools such as Apgar score. 8958 */ 8959 CLINICALIMPRESSION, 8960 /** 8961 * The CodeSystem resource is used to declare the existence of and describe a 8962 * code system or code system supplement and its key properties, and optionally 8963 * define a part or all of its content. 8964 */ 8965 CODESYSTEM, 8966 /** 8967 * An occurrence of information being transmitted; e.g. an alert that was sent 8968 * to a responsible provider, a public health agency that was notified about a 8969 * reportable condition. 8970 */ 8971 COMMUNICATION, 8972 /** 8973 * A request to convey information; e.g. the CDS system proposes that an alert 8974 * be sent to a responsible provider, the CDS system proposes that the public 8975 * health agency be notified about a reportable condition. 8976 */ 8977 COMMUNICATIONREQUEST, 8978 /** 8979 * A compartment definition that defines how resources are accessed on a server. 8980 */ 8981 COMPARTMENTDEFINITION, 8982 /** 8983 * A set of healthcare-related information that is assembled together into a 8984 * single logical package that provides a single coherent statement of meaning, 8985 * establishes its own context and that has clinical attestation with regard to 8986 * who is making the statement. A Composition defines the structure and 8987 * narrative content necessary for a document. However, a Composition alone does 8988 * not constitute a document. Rather, the Composition must be the first entry in 8989 * a Bundle where Bundle.type=document, and any other resources referenced from 8990 * Composition must be included as subsequent entries in the Bundle (for example 8991 * Patient, Practitioner, Encounter, etc.). 8992 */ 8993 COMPOSITION, 8994 /** 8995 * A statement of relationships from one set of concepts to one or more other 8996 * concepts - either concepts in code systems, or data element/data element 8997 * concepts, or classes in class models. 8998 */ 8999 CONCEPTMAP, 9000 /** 9001 * A clinical condition, problem, diagnosis, or other event, situation, issue, 9002 * or clinical concept that has risen to a level of concern. 9003 */ 9004 CONDITION, 9005 /** 9006 * A record of a healthcare consumer?s choices, which permits or denies 9007 * identified recipient(s) or recipient role(s) to perform one or more actions 9008 * within a given policy context, for specific purposes and periods of time. 9009 */ 9010 CONSENT, 9011 /** 9012 * Legally enforceable, formally recorded unilateral or bilateral directive 9013 * i.e., a policy or agreement. 9014 */ 9015 CONTRACT, 9016 /** 9017 * Financial instrument which may be used to reimburse or pay for health care 9018 * products and services. Includes both insurance and self-payment. 9019 */ 9020 COVERAGE, 9021 /** 9022 * The CoverageEligibilityRequest provides patient and insurance coverage 9023 * information to an insurer for them to respond, in the form of an 9024 * CoverageEligibilityResponse, with information regarding whether the stated 9025 * coverage is valid and in-force and optionally to provide the insurance 9026 * details of the policy. 9027 */ 9028 COVERAGEELIGIBILITYREQUEST, 9029 /** 9030 * This resource provides eligibility and plan details from the processing of an 9031 * CoverageEligibilityRequest resource. 9032 */ 9033 COVERAGEELIGIBILITYRESPONSE, 9034 /** 9035 * Indicates an actual or potential clinical issue with or between one or more 9036 * active or proposed clinical actions for a patient; e.g. Drug-drug 9037 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 9038 * etc. 9039 */ 9040 DETECTEDISSUE, 9041 /** 9042 * A type of a manufactured item that is used in the provision of healthcare 9043 * without being substantially changed through that activity. The device may be 9044 * a medical or non-medical device. 9045 */ 9046 DEVICE, 9047 /** 9048 * The characteristics, operational status and capabilities of a medical-related 9049 * component of a medical device. 9050 */ 9051 DEVICEDEFINITION, 9052 /** 9053 * Describes a measurement, calculation or setting capability of a medical 9054 * device. 9055 */ 9056 DEVICEMETRIC, 9057 /** 9058 * Represents a request for a patient to employ a medical device. The device may 9059 * be an implantable device, or an external assistive device, such as a walker. 9060 */ 9061 DEVICEREQUEST, 9062 /** 9063 * A record of a device being used by a patient where the record is the result 9064 * of a report from the patient or another clinician. 9065 */ 9066 DEVICEUSESTATEMENT, 9067 /** 9068 * The findings and interpretation of diagnostic tests performed on patients, 9069 * groups of patients, devices, and locations, and/or specimens derived from 9070 * these. The report includes clinical context such as requesting and provider 9071 * information, and some mix of atomic results, images, textual and coded 9072 * interpretations, and formatted representation of diagnostic reports. 9073 */ 9074 DIAGNOSTICREPORT, 9075 /** 9076 * A collection of documents compiled for a purpose together with metadata that 9077 * applies to the collection. 9078 */ 9079 DOCUMENTMANIFEST, 9080 /** 9081 * A reference to a document of any kind for any purpose. Provides metadata 9082 * about the document so that the document can be discovered and managed. The 9083 * scope of a document is any seralized object with a mime-type, so includes 9084 * formal patient centric documents (CDA), cliical notes, scanned paper, and 9085 * non-patient specific documents like policy text. 9086 */ 9087 DOCUMENTREFERENCE, 9088 /** 9089 * A resource that includes narrative, extensions, and contained resources. 9090 */ 9091 DOMAINRESOURCE, 9092 /** 9093 * The EffectEvidenceSynthesis resource describes the difference in an outcome 9094 * between exposures states in a population where the effect estimate is derived 9095 * from a combination of research studies. 9096 */ 9097 EFFECTEVIDENCESYNTHESIS, 9098 /** 9099 * An interaction between a patient and healthcare provider(s) for the purpose 9100 * of providing healthcare service(s) or assessing the health status of a 9101 * patient. 9102 */ 9103 ENCOUNTER, 9104 /** 9105 * The technical details of an endpoint that can be used for electronic 9106 * services, such as for web services providing XDS.b or a REST endpoint for 9107 * another FHIR server. This may include any security context information. 9108 */ 9109 ENDPOINT, 9110 /** 9111 * This resource provides the insurance enrollment details to the insurer 9112 * regarding a specified coverage. 9113 */ 9114 ENROLLMENTREQUEST, 9115 /** 9116 * This resource provides enrollment and plan details from the processing of an 9117 * EnrollmentRequest resource. 9118 */ 9119 ENROLLMENTRESPONSE, 9120 /** 9121 * An association between a patient and an organization / healthcare provider(s) 9122 * during which time encounters may occur. The managing organization assumes a 9123 * level of responsibility for the patient during this time. 9124 */ 9125 EPISODEOFCARE, 9126 /** 9127 * The EventDefinition resource provides a reusable description of when a 9128 * particular event can occur. 9129 */ 9130 EVENTDEFINITION, 9131 /** 9132 * The Evidence resource describes the conditional state (population and any 9133 * exposures being compared within the population) and outcome (if specified) 9134 * that the knowledge (evidence, assertion, recommendation) is about. 9135 */ 9136 EVIDENCE, 9137 /** 9138 * The EvidenceVariable resource describes a "PICO" element that knowledge 9139 * (evidence, assertion, recommendation) is about. 9140 */ 9141 EVIDENCEVARIABLE, 9142 /** 9143 * Example of workflow instance. 9144 */ 9145 EXAMPLESCENARIO, 9146 /** 9147 * This resource provides: the claim details; adjudication details from the 9148 * processing of a Claim; and optionally account balance information, for 9149 * informing the subscriber of the benefits provided. 9150 */ 9151 EXPLANATIONOFBENEFIT, 9152 /** 9153 * Significant health conditions for a person related to the patient relevant in 9154 * the context of care for the patient. 9155 */ 9156 FAMILYMEMBERHISTORY, 9157 /** 9158 * Prospective warnings of potential issues when providing care to the patient. 9159 */ 9160 FLAG, 9161 /** 9162 * Describes the intended objective(s) for a patient, group or organization 9163 * care, for example, weight loss, restoring an activity of daily living, 9164 * obtaining herd immunity via immunization, meeting a process improvement 9165 * objective, etc. 9166 */ 9167 GOAL, 9168 /** 9169 * A formal computable definition of a graph of resources - that is, a coherent 9170 * set of resources that form a graph by following references. The Graph 9171 * Definition resource defines a set and makes rules about the set. 9172 */ 9173 GRAPHDEFINITION, 9174 /** 9175 * Represents a defined collection of entities that may be discussed or acted 9176 * upon collectively but which are not expected to act collectively, and are not 9177 * formally or legally recognized; i.e. a collection of entities that isn't an 9178 * Organization. 9179 */ 9180 GROUP, 9181 /** 9182 * A guidance response is the formal response to a guidance request, including 9183 * any output parameters returned by the evaluation, as well as the description 9184 * of any proposed actions to be taken. 9185 */ 9186 GUIDANCERESPONSE, 9187 /** 9188 * The details of a healthcare service available at a location. 9189 */ 9190 HEALTHCARESERVICE, 9191 /** 9192 * Representation of the content produced in a DICOM imaging study. A study 9193 * comprises a set of series, each of which includes a set of Service-Object 9194 * Pair Instances (SOP Instances - images or other data) acquired or produced in 9195 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 9196 * ultrasound), but a study may have multiple series of different modalities. 9197 */ 9198 IMAGINGSTUDY, 9199 /** 9200 * Describes the event of a patient being administered a vaccine or a record of 9201 * an immunization as reported by a patient, a clinician or another party. 9202 */ 9203 IMMUNIZATION, 9204 /** 9205 * Describes a comparison of an immunization event against published 9206 * recommendations to determine if the administration is "valid" in relation to 9207 * those recommendations. 9208 */ 9209 IMMUNIZATIONEVALUATION, 9210 /** 9211 * A patient's point-in-time set of recommendations (i.e. forecasting) according 9212 * to a published schedule with optional supporting justification. 9213 */ 9214 IMMUNIZATIONRECOMMENDATION, 9215 /** 9216 * A set of rules of how a particular interoperability or standards problem is 9217 * solved - typically through the use of FHIR resources. This resource is used 9218 * to gather all the parts of an implementation guide into a logical whole and 9219 * to publish a computable definition of all the parts. 9220 */ 9221 IMPLEMENTATIONGUIDE, 9222 /** 9223 * Details of a Health Insurance product/plan provided by an organization. 9224 */ 9225 INSURANCEPLAN, 9226 /** 9227 * Invoice containing collected ChargeItems from an Account with calculated 9228 * individual and total price for Billing purpose. 9229 */ 9230 INVOICE, 9231 /** 9232 * The Library resource is a general-purpose container for knowledge asset 9233 * definitions. It can be used to describe and expose existing knowledge assets 9234 * such as logic libraries and information model descriptions, as well as to 9235 * describe a collection of knowledge assets. 9236 */ 9237 LIBRARY, 9238 /** 9239 * Identifies two or more records (resource instances) that refer to the same 9240 * real-world "occurrence". 9241 */ 9242 LINKAGE, 9243 /** 9244 * A list is a curated collection of resources. 9245 */ 9246 LIST, 9247 /** 9248 * Details and position information for a physical place where services are 9249 * provided and resources and participants may be stored, found, contained, or 9250 * accommodated. 9251 */ 9252 LOCATION, 9253 /** 9254 * The Measure resource provides the definition of a quality measure. 9255 */ 9256 MEASURE, 9257 /** 9258 * The MeasureReport resource contains the results of the calculation of a 9259 * measure; and optionally a reference to the resources involved in that 9260 * calculation. 9261 */ 9262 MEASUREREPORT, 9263 /** 9264 * A photo, video, or audio recording acquired or used in healthcare. The actual 9265 * content may be inline or provided by direct reference. 9266 */ 9267 MEDIA, 9268 /** 9269 * This resource is primarily used for the identification and definition of a 9270 * medication for the purposes of prescribing, dispensing, and administering a 9271 * medication as well as for making statements about medication use. 9272 */ 9273 MEDICATION, 9274 /** 9275 * Describes the event of a patient consuming or otherwise being administered a 9276 * medication. This may be as simple as swallowing a tablet or it may be a long 9277 * running infusion. Related resources tie this event to the authorizing 9278 * prescription, and the specific encounter between patient and health care 9279 * practitioner. 9280 */ 9281 MEDICATIONADMINISTRATION, 9282 /** 9283 * Indicates that a medication product is to be or has been dispensed for a 9284 * named person/patient. This includes a description of the medication product 9285 * (supply) provided and the instructions for administering the medication. The 9286 * medication dispense is the result of a pharmacy system responding to a 9287 * medication order. 9288 */ 9289 MEDICATIONDISPENSE, 9290 /** 9291 * Information about a medication that is used to support knowledge. 9292 */ 9293 MEDICATIONKNOWLEDGE, 9294 /** 9295 * An order or request for both supply of the medication and the instructions 9296 * for administration of the medication to a patient. The resource is called 9297 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 9298 * to generalize the use across inpatient and outpatient settings, including 9299 * care plans, etc., and to harmonize with workflow patterns. 9300 */ 9301 MEDICATIONREQUEST, 9302 /** 9303 * A record of a medication that is being consumed by a patient. A 9304 * MedicationStatement may indicate that the patient may be taking the 9305 * medication now or has taken the medication in the past or will be taking the 9306 * medication in the future. The source of this information can be the patient, 9307 * significant other (such as a family member or spouse), or a clinician. A 9308 * common scenario where this information is captured is during the history 9309 * taking process during a patient visit or stay. The medication information may 9310 * come from sources such as the patient's memory, from a prescription bottle, 9311 * or from a list of medications the patient, clinician or other party 9312 * maintains. 9313 * 9314 * The primary difference between a medication statement and a medication 9315 * administration is that the medication administration has complete 9316 * administration information and is based on actual administration information 9317 * from the person who administered the medication. A medication statement is 9318 * often, if not always, less specific. There is no required date/time when the 9319 * medication was administered, in fact we only know that a source has reported 9320 * the patient is taking this medication, where details such as time, quantity, 9321 * or rate or even medication product may be incomplete or missing or less 9322 * precise. As stated earlier, the medication statement information may come 9323 * from the patient's memory, from a prescription bottle or from a list of 9324 * medications the patient, clinician or other party maintains. Medication 9325 * administration is more formal and is not missing detailed information. 9326 */ 9327 MEDICATIONSTATEMENT, 9328 /** 9329 * Detailed definition of a medicinal product, typically for uses other than 9330 * direct patient care (e.g. regulatory use). 9331 */ 9332 MEDICINALPRODUCT, 9333 /** 9334 * The regulatory authorization of a medicinal product. 9335 */ 9336 MEDICINALPRODUCTAUTHORIZATION, 9337 /** 9338 * The clinical particulars - indications, contraindications etc. of a medicinal 9339 * product, including for regulatory purposes. 9340 */ 9341 MEDICINALPRODUCTCONTRAINDICATION, 9342 /** 9343 * Indication for the Medicinal Product. 9344 */ 9345 MEDICINALPRODUCTINDICATION, 9346 /** 9347 * An ingredient of a manufactured item or pharmaceutical product. 9348 */ 9349 MEDICINALPRODUCTINGREDIENT, 9350 /** 9351 * The interactions of the medicinal product with other medicinal products, or 9352 * other forms of interactions. 9353 */ 9354 MEDICINALPRODUCTINTERACTION, 9355 /** 9356 * The manufactured item as contained in the packaged medicinal product. 9357 */ 9358 MEDICINALPRODUCTMANUFACTURED, 9359 /** 9360 * A medicinal product in a container or package. 9361 */ 9362 MEDICINALPRODUCTPACKAGED, 9363 /** 9364 * A pharmaceutical product described in terms of its composition and dose form. 9365 */ 9366 MEDICINALPRODUCTPHARMACEUTICAL, 9367 /** 9368 * Describe the undesirable effects of the medicinal product. 9369 */ 9370 MEDICINALPRODUCTUNDESIRABLEEFFECT, 9371 /** 9372 * Defines the characteristics of a message that can be shared between systems, 9373 * including the type of event that initiates the message, the content to be 9374 * transmitted and what response(s), if any, are permitted. 9375 */ 9376 MESSAGEDEFINITION, 9377 /** 9378 * The header for a message exchange that is either requesting or responding to 9379 * an action. The reference(s) that are the subject of the action as well as 9380 * other information related to the action are typically transmitted in a bundle 9381 * in which the MessageHeader resource instance is the first resource in the 9382 * bundle. 9383 */ 9384 MESSAGEHEADER, 9385 /** 9386 * Raw data describing a biological sequence. 9387 */ 9388 MOLECULARSEQUENCE, 9389 /** 9390 * A curated namespace that issues unique symbols within that namespace for the 9391 * identification of concepts, people, devices, etc. Represents a "System" used 9392 * within the Identifier and Coding data types. 9393 */ 9394 NAMINGSYSTEM, 9395 /** 9396 * A request to supply a diet, formula feeding (enteral) or oral nutritional 9397 * supplement to a patient/resident. 9398 */ 9399 NUTRITIONORDER, 9400 /** 9401 * Measurements and simple assertions made about a patient, device or other 9402 * subject. 9403 */ 9404 OBSERVATION, 9405 /** 9406 * Set of definitional characteristics for a kind of observation or measurement 9407 * produced or consumed by an orderable health care service. 9408 */ 9409 OBSERVATIONDEFINITION, 9410 /** 9411 * A formal computable definition of an operation (on the RESTful interface) or 9412 * a named query (using the search interaction). 9413 */ 9414 OPERATIONDEFINITION, 9415 /** 9416 * A collection of error, warning, or information messages that result from a 9417 * system action. 9418 */ 9419 OPERATIONOUTCOME, 9420 /** 9421 * A formally or informally recognized grouping of people or organizations 9422 * formed for the purpose of achieving some form of collective action. Includes 9423 * companies, institutions, corporations, departments, community groups, 9424 * healthcare practice groups, payer/insurer, etc. 9425 */ 9426 ORGANIZATION, 9427 /** 9428 * Defines an affiliation/assotiation/relationship between 2 distinct 9429 * oganizations, that is not a part-of relationship/sub-division relationship. 9430 */ 9431 ORGANIZATIONAFFILIATION, 9432 /** 9433 * This resource is a non-persisted resource used to pass information into and 9434 * back from an [operation](operations.html). It has no other use, and there is 9435 * no RESTful endpoint associated with it. 9436 */ 9437 PARAMETERS, 9438 /** 9439 * Demographics and other administrative information about an individual or 9440 * animal receiving care or other health-related services. 9441 */ 9442 PATIENT, 9443 /** 9444 * This resource provides the status of the payment for goods and services 9445 * rendered, and the request and response resource references. 9446 */ 9447 PAYMENTNOTICE, 9448 /** 9449 * This resource provides the details including amount of a payment and 9450 * allocates the payment items being paid. 9451 */ 9452 PAYMENTRECONCILIATION, 9453 /** 9454 * Demographics and administrative information about a person independent of a 9455 * specific health-related context. 9456 */ 9457 PERSON, 9458 /** 9459 * This resource allows for the definition of various types of plans as a 9460 * sharable, consumable, and executable artifact. The resource is general enough 9461 * to support the description of a broad range of clinical artifacts such as 9462 * clinical decision support rules, order sets and protocols. 9463 */ 9464 PLANDEFINITION, 9465 /** 9466 * A person who is directly or indirectly involved in the provisioning of 9467 * healthcare. 9468 */ 9469 PRACTITIONER, 9470 /** 9471 * A specific set of Roles/Locations/specialties/services that a practitioner 9472 * may perform at an organization for a period of time. 9473 */ 9474 PRACTITIONERROLE, 9475 /** 9476 * An action that is or was performed on or for a patient. This can be a 9477 * physical intervention like an operation, or less invasive like long term 9478 * services, counseling, or hypnotherapy. 9479 */ 9480 PROCEDURE, 9481 /** 9482 * Provenance of a resource is a record that describes entities and processes 9483 * involved in producing and delivering or otherwise influencing that resource. 9484 * Provenance provides a critical foundation for assessing authenticity, 9485 * enabling trust, and allowing reproducibility. Provenance assertions are a 9486 * form of contextual metadata and can themselves become important records with 9487 * their own provenance. Provenance statement indicates clinical significance in 9488 * terms of confidence in authenticity, reliability, and trustworthiness, 9489 * integrity, and stage in lifecycle (e.g. Document Completion - has the 9490 * artifact been legally authenticated), all of which may impact security, 9491 * privacy, and trust policies. 9492 */ 9493 PROVENANCE, 9494 /** 9495 * A structured set of questions intended to guide the collection of answers 9496 * from end-users. Questionnaires provide detailed control over order, 9497 * presentation, phraseology and grouping to allow coherent, consistent data 9498 * collection. 9499 */ 9500 QUESTIONNAIRE, 9501 /** 9502 * A structured set of questions and their answers. The questions are ordered 9503 * and grouped into coherent subsets, corresponding to the structure of the 9504 * grouping of the questionnaire being responded to. 9505 */ 9506 QUESTIONNAIRERESPONSE, 9507 /** 9508 * Information about a person that is involved in the care for a patient, but 9509 * who is not the target of healthcare, nor has a formal responsibility in the 9510 * care process. 9511 */ 9512 RELATEDPERSON, 9513 /** 9514 * A group of related requests that can be used to capture intended activities 9515 * that have inter-dependencies such as "give this medication after that one". 9516 */ 9517 REQUESTGROUP, 9518 /** 9519 * The ResearchDefinition resource describes the conditional state (population 9520 * and any exposures being compared within the population) and outcome (if 9521 * specified) that the knowledge (evidence, assertion, recommendation) is about. 9522 */ 9523 RESEARCHDEFINITION, 9524 /** 9525 * The ResearchElementDefinition resource describes a "PICO" element that 9526 * knowledge (evidence, assertion, recommendation) is about. 9527 */ 9528 RESEARCHELEMENTDEFINITION, 9529 /** 9530 * A process where a researcher or organization plans and then executes a series 9531 * of steps intended to increase the field of healthcare-related knowledge. This 9532 * includes studies of safety, efficacy, comparative effectiveness and other 9533 * information about medications, devices, therapies and other interventional 9534 * and investigative techniques. A ResearchStudy involves the gathering of 9535 * information about human or animal subjects. 9536 */ 9537 RESEARCHSTUDY, 9538 /** 9539 * A physical entity which is the primary unit of operational and/or 9540 * administrative interest in a study. 9541 */ 9542 RESEARCHSUBJECT, 9543 /** 9544 * This is the base resource type for everything. 9545 */ 9546 RESOURCE, 9547 /** 9548 * An assessment of the likely outcome(s) for a patient or other subject as well 9549 * as the likelihood of each outcome. 9550 */ 9551 RISKASSESSMENT, 9552 /** 9553 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 9554 * a population plus exposure state where the risk estimate is derived from a 9555 * combination of research studies. 9556 */ 9557 RISKEVIDENCESYNTHESIS, 9558 /** 9559 * A container for slots of time that may be available for booking appointments. 9560 */ 9561 SCHEDULE, 9562 /** 9563 * A search parameter that defines a named search item that can be used to 9564 * search/filter on a resource. 9565 */ 9566 SEARCHPARAMETER, 9567 /** 9568 * A record of a request for service such as diagnostic investigations, 9569 * treatments, or operations to be performed. 9570 */ 9571 SERVICEREQUEST, 9572 /** 9573 * A slot of time on a schedule that may be available for booking appointments. 9574 */ 9575 SLOT, 9576 /** 9577 * A sample to be used for analysis. 9578 */ 9579 SPECIMEN, 9580 /** 9581 * A kind of specimen with associated set of requirements. 9582 */ 9583 SPECIMENDEFINITION, 9584 /** 9585 * A definition of a FHIR structure. This resource is used to describe the 9586 * underlying resources, data types defined in FHIR, and also for describing 9587 * extensions and constraints on resources and data types. 9588 */ 9589 STRUCTUREDEFINITION, 9590 /** 9591 * A Map of relationships between 2 structures that can be used to transform 9592 * data. 9593 */ 9594 STRUCTUREMAP, 9595 /** 9596 * The subscription resource is used to define a push-based subscription from a 9597 * server to another system. Once a subscription is registered with the server, 9598 * the server checks every resource that is created or updated, and if the 9599 * resource matches the given criteria, it sends a message on the defined 9600 * "channel" so that another system can take an appropriate action. 9601 */ 9602 SUBSCRIPTION, 9603 /** 9604 * A homogeneous material with a definite composition. 9605 */ 9606 SUBSTANCE, 9607 /** 9608 * Nucleic acids are defined by three distinct elements: the base, sugar and 9609 * linkage. Individual substance/moiety IDs will be created for each of these 9610 * elements. The nucleotide sequence will be always entered in the 5?-3? 9611 * direction. 9612 */ 9613 SUBSTANCENUCLEICACID, 9614 /** 9615 * Todo. 9616 */ 9617 SUBSTANCEPOLYMER, 9618 /** 9619 * A SubstanceProtein is defined as a single unit of a linear amino acid 9620 * sequence, or a combination of subunits that are either covalently linked or 9621 * have a defined invariant stoichiometric relationship. This includes all 9622 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 9623 * whether the use is therapeutic or prophylactic. This set of elements will be 9624 * used to describe albumins, coagulation factors, cytokines, growth factors, 9625 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 9626 * vaccines, and immunomodulators. 9627 */ 9628 SUBSTANCEPROTEIN, 9629 /** 9630 * Todo. 9631 */ 9632 SUBSTANCEREFERENCEINFORMATION, 9633 /** 9634 * Source material shall capture information on the taxonomic and anatomical 9635 * origins as well as the fraction of a material that can result in or can be 9636 * modified to form a substance. This set of data elements shall be used to 9637 * define polymer substances isolated from biological matrices. Taxonomic and 9638 * anatomical origins shall be described using a controlled vocabulary as 9639 * required. This information is captured for naturally derived polymers ( . 9640 * starch) and structurally diverse substances. For Organisms belonging to the 9641 * Kingdom Plantae the Substance level defines the fresh material of a single 9642 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 9643 * Herbal preparations, the fraction information will be captured at the 9644 * Substance information level and additional information for herbal extracts 9645 * will be captured at the Specified Substance Group 1 information level. See 9646 * for further explanation the Substance Class: Structurally Diverse and the 9647 * herbal annex. 9648 */ 9649 SUBSTANCESOURCEMATERIAL, 9650 /** 9651 * The detailed description of a substance, typically at a level beyond what is 9652 * used for prescribing. 9653 */ 9654 SUBSTANCESPECIFICATION, 9655 /** 9656 * Record of delivery of what is supplied. 9657 */ 9658 SUPPLYDELIVERY, 9659 /** 9660 * A record of a request for a medication, substance or device used in the 9661 * healthcare setting. 9662 */ 9663 SUPPLYREQUEST, 9664 /** 9665 * A task to be performed. 9666 */ 9667 TASK, 9668 /** 9669 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 9670 * FHIR Server that may be used as a statement of actual server functionality or 9671 * a statement of required or desired server implementation. 9672 */ 9673 TERMINOLOGYCAPABILITIES, 9674 /** 9675 * A summary of information based on the results of executing a TestScript. 9676 */ 9677 TESTREPORT, 9678 /** 9679 * A structured set of tests against a FHIR server or client implementation to 9680 * determine compliance against the FHIR specification. 9681 */ 9682 TESTSCRIPT, 9683 /** 9684 * A ValueSet resource instance specifies a set of codes drawn from one or more 9685 * code systems, intended for use in a particular context. Value sets link 9686 * between [[[CodeSystem]]] definitions and their use in [coded 9687 * elements](terminologies.html). 9688 */ 9689 VALUESET, 9690 /** 9691 * Describes validation requirements, source(s), status and dates for one or 9692 * more elements. 9693 */ 9694 VERIFICATIONRESULT, 9695 /** 9696 * An authorization for the provision of glasses and/or contact lenses to a 9697 * patient. 9698 */ 9699 VISIONPRESCRIPTION, 9700 /** 9701 * added to help the parsers 9702 */ 9703 NULL; 9704 9705 public static FHIRDefinedType fromCode(String codeString) throws FHIRException { 9706 if (codeString == null || "".equals(codeString)) 9707 return null; 9708 if ("Address".equals(codeString)) 9709 return ADDRESS; 9710 if ("Age".equals(codeString)) 9711 return AGE; 9712 if ("Annotation".equals(codeString)) 9713 return ANNOTATION; 9714 if ("Attachment".equals(codeString)) 9715 return ATTACHMENT; 9716 if ("BackboneElement".equals(codeString)) 9717 return BACKBONEELEMENT; 9718 if ("CodeableConcept".equals(codeString)) 9719 return CODEABLECONCEPT; 9720 if ("Coding".equals(codeString)) 9721 return CODING; 9722 if ("ContactDetail".equals(codeString)) 9723 return CONTACTDETAIL; 9724 if ("ContactPoint".equals(codeString)) 9725 return CONTACTPOINT; 9726 if ("Contributor".equals(codeString)) 9727 return CONTRIBUTOR; 9728 if ("Count".equals(codeString)) 9729 return COUNT; 9730 if ("DataRequirement".equals(codeString)) 9731 return DATAREQUIREMENT; 9732 if ("Distance".equals(codeString)) 9733 return DISTANCE; 9734 if ("Dosage".equals(codeString)) 9735 return DOSAGE; 9736 if ("Duration".equals(codeString)) 9737 return DURATION; 9738 if ("Element".equals(codeString)) 9739 return ELEMENT; 9740 if ("ElementDefinition".equals(codeString)) 9741 return ELEMENTDEFINITION; 9742 if ("Expression".equals(codeString)) 9743 return EXPRESSION; 9744 if ("Extension".equals(codeString)) 9745 return EXTENSION; 9746 if ("HumanName".equals(codeString)) 9747 return HUMANNAME; 9748 if ("Identifier".equals(codeString)) 9749 return IDENTIFIER; 9750 if ("MarketingStatus".equals(codeString)) 9751 return MARKETINGSTATUS; 9752 if ("Meta".equals(codeString)) 9753 return META; 9754 if ("Money".equals(codeString)) 9755 return MONEY; 9756 if ("MoneyQuantity".equals(codeString)) 9757 return MONEYQUANTITY; 9758 if ("Narrative".equals(codeString)) 9759 return NARRATIVE; 9760 if ("ParameterDefinition".equals(codeString)) 9761 return PARAMETERDEFINITION; 9762 if ("Period".equals(codeString)) 9763 return PERIOD; 9764 if ("Population".equals(codeString)) 9765 return POPULATION; 9766 if ("ProdCharacteristic".equals(codeString)) 9767 return PRODCHARACTERISTIC; 9768 if ("ProductShelfLife".equals(codeString)) 9769 return PRODUCTSHELFLIFE; 9770 if ("Quantity".equals(codeString)) 9771 return QUANTITY; 9772 if ("Range".equals(codeString)) 9773 return RANGE; 9774 if ("Ratio".equals(codeString)) 9775 return RATIO; 9776 if ("Reference".equals(codeString)) 9777 return REFERENCE; 9778 if ("RelatedArtifact".equals(codeString)) 9779 return RELATEDARTIFACT; 9780 if ("SampledData".equals(codeString)) 9781 return SAMPLEDDATA; 9782 if ("Signature".equals(codeString)) 9783 return SIGNATURE; 9784 if ("SimpleQuantity".equals(codeString)) 9785 return SIMPLEQUANTITY; 9786 if ("SubstanceAmount".equals(codeString)) 9787 return SUBSTANCEAMOUNT; 9788 if ("Timing".equals(codeString)) 9789 return TIMING; 9790 if ("TriggerDefinition".equals(codeString)) 9791 return TRIGGERDEFINITION; 9792 if ("UsageContext".equals(codeString)) 9793 return USAGECONTEXT; 9794 if ("base64Binary".equals(codeString)) 9795 return BASE64BINARY; 9796 if ("boolean".equals(codeString)) 9797 return BOOLEAN; 9798 if ("canonical".equals(codeString)) 9799 return CANONICAL; 9800 if ("code".equals(codeString)) 9801 return CODE; 9802 if ("date".equals(codeString)) 9803 return DATE; 9804 if ("dateTime".equals(codeString)) 9805 return DATETIME; 9806 if ("decimal".equals(codeString)) 9807 return DECIMAL; 9808 if ("id".equals(codeString)) 9809 return ID; 9810 if ("instant".equals(codeString)) 9811 return INSTANT; 9812 if ("integer".equals(codeString)) 9813 return INTEGER; 9814 if ("markdown".equals(codeString)) 9815 return MARKDOWN; 9816 if ("oid".equals(codeString)) 9817 return OID; 9818 if ("positiveInt".equals(codeString)) 9819 return POSITIVEINT; 9820 if ("string".equals(codeString)) 9821 return STRING; 9822 if ("time".equals(codeString)) 9823 return TIME; 9824 if ("unsignedInt".equals(codeString)) 9825 return UNSIGNEDINT; 9826 if ("uri".equals(codeString)) 9827 return URI; 9828 if ("url".equals(codeString)) 9829 return URL; 9830 if ("uuid".equals(codeString)) 9831 return UUID; 9832 if ("xhtml".equals(codeString)) 9833 return XHTML; 9834 if ("Account".equals(codeString)) 9835 return ACCOUNT; 9836 if ("ActivityDefinition".equals(codeString)) 9837 return ACTIVITYDEFINITION; 9838 if ("AdverseEvent".equals(codeString)) 9839 return ADVERSEEVENT; 9840 if ("AllergyIntolerance".equals(codeString)) 9841 return ALLERGYINTOLERANCE; 9842 if ("Appointment".equals(codeString)) 9843 return APPOINTMENT; 9844 if ("AppointmentResponse".equals(codeString)) 9845 return APPOINTMENTRESPONSE; 9846 if ("AuditEvent".equals(codeString)) 9847 return AUDITEVENT; 9848 if ("Basic".equals(codeString)) 9849 return BASIC; 9850 if ("Binary".equals(codeString)) 9851 return BINARY; 9852 if ("BiologicallyDerivedProduct".equals(codeString)) 9853 return BIOLOGICALLYDERIVEDPRODUCT; 9854 if ("BodyStructure".equals(codeString)) 9855 return BODYSTRUCTURE; 9856 if ("Bundle".equals(codeString)) 9857 return BUNDLE; 9858 if ("CapabilityStatement".equals(codeString)) 9859 return CAPABILITYSTATEMENT; 9860 if ("CarePlan".equals(codeString)) 9861 return CAREPLAN; 9862 if ("CareTeam".equals(codeString)) 9863 return CARETEAM; 9864 if ("CatalogEntry".equals(codeString)) 9865 return CATALOGENTRY; 9866 if ("ChargeItem".equals(codeString)) 9867 return CHARGEITEM; 9868 if ("ChargeItemDefinition".equals(codeString)) 9869 return CHARGEITEMDEFINITION; 9870 if ("Claim".equals(codeString)) 9871 return CLAIM; 9872 if ("ClaimResponse".equals(codeString)) 9873 return CLAIMRESPONSE; 9874 if ("ClinicalImpression".equals(codeString)) 9875 return CLINICALIMPRESSION; 9876 if ("CodeSystem".equals(codeString)) 9877 return CODESYSTEM; 9878 if ("Communication".equals(codeString)) 9879 return COMMUNICATION; 9880 if ("CommunicationRequest".equals(codeString)) 9881 return COMMUNICATIONREQUEST; 9882 if ("CompartmentDefinition".equals(codeString)) 9883 return COMPARTMENTDEFINITION; 9884 if ("Composition".equals(codeString)) 9885 return COMPOSITION; 9886 if ("ConceptMap".equals(codeString)) 9887 return CONCEPTMAP; 9888 if ("Condition".equals(codeString)) 9889 return CONDITION; 9890 if ("Consent".equals(codeString)) 9891 return CONSENT; 9892 if ("Contract".equals(codeString)) 9893 return CONTRACT; 9894 if ("Coverage".equals(codeString)) 9895 return COVERAGE; 9896 if ("CoverageEligibilityRequest".equals(codeString)) 9897 return COVERAGEELIGIBILITYREQUEST; 9898 if ("CoverageEligibilityResponse".equals(codeString)) 9899 return COVERAGEELIGIBILITYRESPONSE; 9900 if ("DetectedIssue".equals(codeString)) 9901 return DETECTEDISSUE; 9902 if ("Device".equals(codeString)) 9903 return DEVICE; 9904 if ("DeviceDefinition".equals(codeString)) 9905 return DEVICEDEFINITION; 9906 if ("DeviceMetric".equals(codeString)) 9907 return DEVICEMETRIC; 9908 if ("DeviceRequest".equals(codeString)) 9909 return DEVICEREQUEST; 9910 if ("DeviceUseStatement".equals(codeString)) 9911 return DEVICEUSESTATEMENT; 9912 if ("DiagnosticReport".equals(codeString)) 9913 return DIAGNOSTICREPORT; 9914 if ("DocumentManifest".equals(codeString)) 9915 return DOCUMENTMANIFEST; 9916 if ("DocumentReference".equals(codeString)) 9917 return DOCUMENTREFERENCE; 9918 if ("DomainResource".equals(codeString)) 9919 return DOMAINRESOURCE; 9920 if ("EffectEvidenceSynthesis".equals(codeString)) 9921 return EFFECTEVIDENCESYNTHESIS; 9922 if ("Encounter".equals(codeString)) 9923 return ENCOUNTER; 9924 if ("Endpoint".equals(codeString)) 9925 return ENDPOINT; 9926 if ("EnrollmentRequest".equals(codeString)) 9927 return ENROLLMENTREQUEST; 9928 if ("EnrollmentResponse".equals(codeString)) 9929 return ENROLLMENTRESPONSE; 9930 if ("EpisodeOfCare".equals(codeString)) 9931 return EPISODEOFCARE; 9932 if ("EventDefinition".equals(codeString)) 9933 return EVENTDEFINITION; 9934 if ("Evidence".equals(codeString)) 9935 return EVIDENCE; 9936 if ("EvidenceVariable".equals(codeString)) 9937 return EVIDENCEVARIABLE; 9938 if ("ExampleScenario".equals(codeString)) 9939 return EXAMPLESCENARIO; 9940 if ("ExplanationOfBenefit".equals(codeString)) 9941 return EXPLANATIONOFBENEFIT; 9942 if ("FamilyMemberHistory".equals(codeString)) 9943 return FAMILYMEMBERHISTORY; 9944 if ("Flag".equals(codeString)) 9945 return FLAG; 9946 if ("Goal".equals(codeString)) 9947 return GOAL; 9948 if ("GraphDefinition".equals(codeString)) 9949 return GRAPHDEFINITION; 9950 if ("Group".equals(codeString)) 9951 return GROUP; 9952 if ("GuidanceResponse".equals(codeString)) 9953 return GUIDANCERESPONSE; 9954 if ("HealthcareService".equals(codeString)) 9955 return HEALTHCARESERVICE; 9956 if ("ImagingStudy".equals(codeString)) 9957 return IMAGINGSTUDY; 9958 if ("Immunization".equals(codeString)) 9959 return IMMUNIZATION; 9960 if ("ImmunizationEvaluation".equals(codeString)) 9961 return IMMUNIZATIONEVALUATION; 9962 if ("ImmunizationRecommendation".equals(codeString)) 9963 return IMMUNIZATIONRECOMMENDATION; 9964 if ("ImplementationGuide".equals(codeString)) 9965 return IMPLEMENTATIONGUIDE; 9966 if ("InsurancePlan".equals(codeString)) 9967 return INSURANCEPLAN; 9968 if ("Invoice".equals(codeString)) 9969 return INVOICE; 9970 if ("Library".equals(codeString)) 9971 return LIBRARY; 9972 if ("Linkage".equals(codeString)) 9973 return LINKAGE; 9974 if ("List".equals(codeString)) 9975 return LIST; 9976 if ("Location".equals(codeString)) 9977 return LOCATION; 9978 if ("Measure".equals(codeString)) 9979 return MEASURE; 9980 if ("MeasureReport".equals(codeString)) 9981 return MEASUREREPORT; 9982 if ("Media".equals(codeString)) 9983 return MEDIA; 9984 if ("Medication".equals(codeString)) 9985 return MEDICATION; 9986 if ("MedicationAdministration".equals(codeString)) 9987 return MEDICATIONADMINISTRATION; 9988 if ("MedicationDispense".equals(codeString)) 9989 return MEDICATIONDISPENSE; 9990 if ("MedicationKnowledge".equals(codeString)) 9991 return MEDICATIONKNOWLEDGE; 9992 if ("MedicationRequest".equals(codeString)) 9993 return MEDICATIONREQUEST; 9994 if ("MedicationStatement".equals(codeString)) 9995 return MEDICATIONSTATEMENT; 9996 if ("MedicinalProduct".equals(codeString)) 9997 return MEDICINALPRODUCT; 9998 if ("MedicinalProductAuthorization".equals(codeString)) 9999 return MEDICINALPRODUCTAUTHORIZATION; 10000 if ("MedicinalProductContraindication".equals(codeString)) 10001 return MEDICINALPRODUCTCONTRAINDICATION; 10002 if ("MedicinalProductIndication".equals(codeString)) 10003 return MEDICINALPRODUCTINDICATION; 10004 if ("MedicinalProductIngredient".equals(codeString)) 10005 return MEDICINALPRODUCTINGREDIENT; 10006 if ("MedicinalProductInteraction".equals(codeString)) 10007 return MEDICINALPRODUCTINTERACTION; 10008 if ("MedicinalProductManufactured".equals(codeString)) 10009 return MEDICINALPRODUCTMANUFACTURED; 10010 if ("MedicinalProductPackaged".equals(codeString)) 10011 return MEDICINALPRODUCTPACKAGED; 10012 if ("MedicinalProductPharmaceutical".equals(codeString)) 10013 return MEDICINALPRODUCTPHARMACEUTICAL; 10014 if ("MedicinalProductUndesirableEffect".equals(codeString)) 10015 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 10016 if ("MessageDefinition".equals(codeString)) 10017 return MESSAGEDEFINITION; 10018 if ("MessageHeader".equals(codeString)) 10019 return MESSAGEHEADER; 10020 if ("MolecularSequence".equals(codeString)) 10021 return MOLECULARSEQUENCE; 10022 if ("NamingSystem".equals(codeString)) 10023 return NAMINGSYSTEM; 10024 if ("NutritionOrder".equals(codeString)) 10025 return NUTRITIONORDER; 10026 if ("Observation".equals(codeString)) 10027 return OBSERVATION; 10028 if ("ObservationDefinition".equals(codeString)) 10029 return OBSERVATIONDEFINITION; 10030 if ("OperationDefinition".equals(codeString)) 10031 return OPERATIONDEFINITION; 10032 if ("OperationOutcome".equals(codeString)) 10033 return OPERATIONOUTCOME; 10034 if ("Organization".equals(codeString)) 10035 return ORGANIZATION; 10036 if ("OrganizationAffiliation".equals(codeString)) 10037 return ORGANIZATIONAFFILIATION; 10038 if ("Parameters".equals(codeString)) 10039 return PARAMETERS; 10040 if ("Patient".equals(codeString)) 10041 return PATIENT; 10042 if ("PaymentNotice".equals(codeString)) 10043 return PAYMENTNOTICE; 10044 if ("PaymentReconciliation".equals(codeString)) 10045 return PAYMENTRECONCILIATION; 10046 if ("Person".equals(codeString)) 10047 return PERSON; 10048 if ("PlanDefinition".equals(codeString)) 10049 return PLANDEFINITION; 10050 if ("Practitioner".equals(codeString)) 10051 return PRACTITIONER; 10052 if ("PractitionerRole".equals(codeString)) 10053 return PRACTITIONERROLE; 10054 if ("Procedure".equals(codeString)) 10055 return PROCEDURE; 10056 if ("Provenance".equals(codeString)) 10057 return PROVENANCE; 10058 if ("Questionnaire".equals(codeString)) 10059 return QUESTIONNAIRE; 10060 if ("QuestionnaireResponse".equals(codeString)) 10061 return QUESTIONNAIRERESPONSE; 10062 if ("RelatedPerson".equals(codeString)) 10063 return RELATEDPERSON; 10064 if ("RequestGroup".equals(codeString)) 10065 return REQUESTGROUP; 10066 if ("ResearchDefinition".equals(codeString)) 10067 return RESEARCHDEFINITION; 10068 if ("ResearchElementDefinition".equals(codeString)) 10069 return RESEARCHELEMENTDEFINITION; 10070 if ("ResearchStudy".equals(codeString)) 10071 return RESEARCHSTUDY; 10072 if ("ResearchSubject".equals(codeString)) 10073 return RESEARCHSUBJECT; 10074 if ("Resource".equals(codeString)) 10075 return RESOURCE; 10076 if ("RiskAssessment".equals(codeString)) 10077 return RISKASSESSMENT; 10078 if ("RiskEvidenceSynthesis".equals(codeString)) 10079 return RISKEVIDENCESYNTHESIS; 10080 if ("Schedule".equals(codeString)) 10081 return SCHEDULE; 10082 if ("SearchParameter".equals(codeString)) 10083 return SEARCHPARAMETER; 10084 if ("ServiceRequest".equals(codeString)) 10085 return SERVICEREQUEST; 10086 if ("Slot".equals(codeString)) 10087 return SLOT; 10088 if ("Specimen".equals(codeString)) 10089 return SPECIMEN; 10090 if ("SpecimenDefinition".equals(codeString)) 10091 return SPECIMENDEFINITION; 10092 if ("StructureDefinition".equals(codeString)) 10093 return STRUCTUREDEFINITION; 10094 if ("StructureMap".equals(codeString)) 10095 return STRUCTUREMAP; 10096 if ("Subscription".equals(codeString)) 10097 return SUBSCRIPTION; 10098 if ("Substance".equals(codeString)) 10099 return SUBSTANCE; 10100 if ("SubstanceNucleicAcid".equals(codeString)) 10101 return SUBSTANCENUCLEICACID; 10102 if ("SubstancePolymer".equals(codeString)) 10103 return SUBSTANCEPOLYMER; 10104 if ("SubstanceProtein".equals(codeString)) 10105 return SUBSTANCEPROTEIN; 10106 if ("SubstanceReferenceInformation".equals(codeString)) 10107 return SUBSTANCEREFERENCEINFORMATION; 10108 if ("SubstanceSourceMaterial".equals(codeString)) 10109 return SUBSTANCESOURCEMATERIAL; 10110 if ("SubstanceSpecification".equals(codeString)) 10111 return SUBSTANCESPECIFICATION; 10112 if ("SupplyDelivery".equals(codeString)) 10113 return SUPPLYDELIVERY; 10114 if ("SupplyRequest".equals(codeString)) 10115 return SUPPLYREQUEST; 10116 if ("Task".equals(codeString)) 10117 return TASK; 10118 if ("TerminologyCapabilities".equals(codeString)) 10119 return TERMINOLOGYCAPABILITIES; 10120 if ("TestReport".equals(codeString)) 10121 return TESTREPORT; 10122 if ("TestScript".equals(codeString)) 10123 return TESTSCRIPT; 10124 if ("ValueSet".equals(codeString)) 10125 return VALUESET; 10126 if ("VerificationResult".equals(codeString)) 10127 return VERIFICATIONRESULT; 10128 if ("VisionPrescription".equals(codeString)) 10129 return VISIONPRESCRIPTION; 10130 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 10131 } 10132 10133 public String toCode() { 10134 switch (this) { 10135 case ADDRESS: 10136 return "Address"; 10137 case AGE: 10138 return "Age"; 10139 case ANNOTATION: 10140 return "Annotation"; 10141 case ATTACHMENT: 10142 return "Attachment"; 10143 case BACKBONEELEMENT: 10144 return "BackboneElement"; 10145 case CODEABLECONCEPT: 10146 return "CodeableConcept"; 10147 case CODING: 10148 return "Coding"; 10149 case CONTACTDETAIL: 10150 return "ContactDetail"; 10151 case CONTACTPOINT: 10152 return "ContactPoint"; 10153 case CONTRIBUTOR: 10154 return "Contributor"; 10155 case COUNT: 10156 return "Count"; 10157 case DATAREQUIREMENT: 10158 return "DataRequirement"; 10159 case DISTANCE: 10160 return "Distance"; 10161 case DOSAGE: 10162 return "Dosage"; 10163 case DURATION: 10164 return "Duration"; 10165 case ELEMENT: 10166 return "Element"; 10167 case ELEMENTDEFINITION: 10168 return "ElementDefinition"; 10169 case EXPRESSION: 10170 return "Expression"; 10171 case EXTENSION: 10172 return "Extension"; 10173 case HUMANNAME: 10174 return "HumanName"; 10175 case IDENTIFIER: 10176 return "Identifier"; 10177 case MARKETINGSTATUS: 10178 return "MarketingStatus"; 10179 case META: 10180 return "Meta"; 10181 case MONEY: 10182 return "Money"; 10183 case MONEYQUANTITY: 10184 return "MoneyQuantity"; 10185 case NARRATIVE: 10186 return "Narrative"; 10187 case PARAMETERDEFINITION: 10188 return "ParameterDefinition"; 10189 case PERIOD: 10190 return "Period"; 10191 case POPULATION: 10192 return "Population"; 10193 case PRODCHARACTERISTIC: 10194 return "ProdCharacteristic"; 10195 case PRODUCTSHELFLIFE: 10196 return "ProductShelfLife"; 10197 case QUANTITY: 10198 return "Quantity"; 10199 case RANGE: 10200 return "Range"; 10201 case RATIO: 10202 return "Ratio"; 10203 case REFERENCE: 10204 return "Reference"; 10205 case RELATEDARTIFACT: 10206 return "RelatedArtifact"; 10207 case SAMPLEDDATA: 10208 return "SampledData"; 10209 case SIGNATURE: 10210 return "Signature"; 10211 case SIMPLEQUANTITY: 10212 return "SimpleQuantity"; 10213 case SUBSTANCEAMOUNT: 10214 return "SubstanceAmount"; 10215 case TIMING: 10216 return "Timing"; 10217 case TRIGGERDEFINITION: 10218 return "TriggerDefinition"; 10219 case USAGECONTEXT: 10220 return "UsageContext"; 10221 case BASE64BINARY: 10222 return "base64Binary"; 10223 case BOOLEAN: 10224 return "boolean"; 10225 case CANONICAL: 10226 return "canonical"; 10227 case CODE: 10228 return "code"; 10229 case DATE: 10230 return "date"; 10231 case DATETIME: 10232 return "dateTime"; 10233 case DECIMAL: 10234 return "decimal"; 10235 case ID: 10236 return "id"; 10237 case INSTANT: 10238 return "instant"; 10239 case INTEGER: 10240 return "integer"; 10241 case MARKDOWN: 10242 return "markdown"; 10243 case OID: 10244 return "oid"; 10245 case POSITIVEINT: 10246 return "positiveInt"; 10247 case STRING: 10248 return "string"; 10249 case TIME: 10250 return "time"; 10251 case UNSIGNEDINT: 10252 return "unsignedInt"; 10253 case URI: 10254 return "uri"; 10255 case URL: 10256 return "url"; 10257 case UUID: 10258 return "uuid"; 10259 case XHTML: 10260 return "xhtml"; 10261 case ACCOUNT: 10262 return "Account"; 10263 case ACTIVITYDEFINITION: 10264 return "ActivityDefinition"; 10265 case ADVERSEEVENT: 10266 return "AdverseEvent"; 10267 case ALLERGYINTOLERANCE: 10268 return "AllergyIntolerance"; 10269 case APPOINTMENT: 10270 return "Appointment"; 10271 case APPOINTMENTRESPONSE: 10272 return "AppointmentResponse"; 10273 case AUDITEVENT: 10274 return "AuditEvent"; 10275 case BASIC: 10276 return "Basic"; 10277 case BINARY: 10278 return "Binary"; 10279 case BIOLOGICALLYDERIVEDPRODUCT: 10280 return "BiologicallyDerivedProduct"; 10281 case BODYSTRUCTURE: 10282 return "BodyStructure"; 10283 case BUNDLE: 10284 return "Bundle"; 10285 case CAPABILITYSTATEMENT: 10286 return "CapabilityStatement"; 10287 case CAREPLAN: 10288 return "CarePlan"; 10289 case CARETEAM: 10290 return "CareTeam"; 10291 case CATALOGENTRY: 10292 return "CatalogEntry"; 10293 case CHARGEITEM: 10294 return "ChargeItem"; 10295 case CHARGEITEMDEFINITION: 10296 return "ChargeItemDefinition"; 10297 case CLAIM: 10298 return "Claim"; 10299 case CLAIMRESPONSE: 10300 return "ClaimResponse"; 10301 case CLINICALIMPRESSION: 10302 return "ClinicalImpression"; 10303 case CODESYSTEM: 10304 return "CodeSystem"; 10305 case COMMUNICATION: 10306 return "Communication"; 10307 case COMMUNICATIONREQUEST: 10308 return "CommunicationRequest"; 10309 case COMPARTMENTDEFINITION: 10310 return "CompartmentDefinition"; 10311 case COMPOSITION: 10312 return "Composition"; 10313 case CONCEPTMAP: 10314 return "ConceptMap"; 10315 case CONDITION: 10316 return "Condition"; 10317 case CONSENT: 10318 return "Consent"; 10319 case CONTRACT: 10320 return "Contract"; 10321 case COVERAGE: 10322 return "Coverage"; 10323 case COVERAGEELIGIBILITYREQUEST: 10324 return "CoverageEligibilityRequest"; 10325 case COVERAGEELIGIBILITYRESPONSE: 10326 return "CoverageEligibilityResponse"; 10327 case DETECTEDISSUE: 10328 return "DetectedIssue"; 10329 case DEVICE: 10330 return "Device"; 10331 case DEVICEDEFINITION: 10332 return "DeviceDefinition"; 10333 case DEVICEMETRIC: 10334 return "DeviceMetric"; 10335 case DEVICEREQUEST: 10336 return "DeviceRequest"; 10337 case DEVICEUSESTATEMENT: 10338 return "DeviceUseStatement"; 10339 case DIAGNOSTICREPORT: 10340 return "DiagnosticReport"; 10341 case DOCUMENTMANIFEST: 10342 return "DocumentManifest"; 10343 case DOCUMENTREFERENCE: 10344 return "DocumentReference"; 10345 case DOMAINRESOURCE: 10346 return "DomainResource"; 10347 case EFFECTEVIDENCESYNTHESIS: 10348 return "EffectEvidenceSynthesis"; 10349 case ENCOUNTER: 10350 return "Encounter"; 10351 case ENDPOINT: 10352 return "Endpoint"; 10353 case ENROLLMENTREQUEST: 10354 return "EnrollmentRequest"; 10355 case ENROLLMENTRESPONSE: 10356 return "EnrollmentResponse"; 10357 case EPISODEOFCARE: 10358 return "EpisodeOfCare"; 10359 case EVENTDEFINITION: 10360 return "EventDefinition"; 10361 case EVIDENCE: 10362 return "Evidence"; 10363 case EVIDENCEVARIABLE: 10364 return "EvidenceVariable"; 10365 case EXAMPLESCENARIO: 10366 return "ExampleScenario"; 10367 case EXPLANATIONOFBENEFIT: 10368 return "ExplanationOfBenefit"; 10369 case FAMILYMEMBERHISTORY: 10370 return "FamilyMemberHistory"; 10371 case FLAG: 10372 return "Flag"; 10373 case GOAL: 10374 return "Goal"; 10375 case GRAPHDEFINITION: 10376 return "GraphDefinition"; 10377 case GROUP: 10378 return "Group"; 10379 case GUIDANCERESPONSE: 10380 return "GuidanceResponse"; 10381 case HEALTHCARESERVICE: 10382 return "HealthcareService"; 10383 case IMAGINGSTUDY: 10384 return "ImagingStudy"; 10385 case IMMUNIZATION: 10386 return "Immunization"; 10387 case IMMUNIZATIONEVALUATION: 10388 return "ImmunizationEvaluation"; 10389 case IMMUNIZATIONRECOMMENDATION: 10390 return "ImmunizationRecommendation"; 10391 case IMPLEMENTATIONGUIDE: 10392 return "ImplementationGuide"; 10393 case INSURANCEPLAN: 10394 return "InsurancePlan"; 10395 case INVOICE: 10396 return "Invoice"; 10397 case LIBRARY: 10398 return "Library"; 10399 case LINKAGE: 10400 return "Linkage"; 10401 case LIST: 10402 return "List"; 10403 case LOCATION: 10404 return "Location"; 10405 case MEASURE: 10406 return "Measure"; 10407 case MEASUREREPORT: 10408 return "MeasureReport"; 10409 case MEDIA: 10410 return "Media"; 10411 case MEDICATION: 10412 return "Medication"; 10413 case MEDICATIONADMINISTRATION: 10414 return "MedicationAdministration"; 10415 case MEDICATIONDISPENSE: 10416 return "MedicationDispense"; 10417 case MEDICATIONKNOWLEDGE: 10418 return "MedicationKnowledge"; 10419 case MEDICATIONREQUEST: 10420 return "MedicationRequest"; 10421 case MEDICATIONSTATEMENT: 10422 return "MedicationStatement"; 10423 case MEDICINALPRODUCT: 10424 return "MedicinalProduct"; 10425 case MEDICINALPRODUCTAUTHORIZATION: 10426 return "MedicinalProductAuthorization"; 10427 case MEDICINALPRODUCTCONTRAINDICATION: 10428 return "MedicinalProductContraindication"; 10429 case MEDICINALPRODUCTINDICATION: 10430 return "MedicinalProductIndication"; 10431 case MEDICINALPRODUCTINGREDIENT: 10432 return "MedicinalProductIngredient"; 10433 case MEDICINALPRODUCTINTERACTION: 10434 return "MedicinalProductInteraction"; 10435 case MEDICINALPRODUCTMANUFACTURED: 10436 return "MedicinalProductManufactured"; 10437 case MEDICINALPRODUCTPACKAGED: 10438 return "MedicinalProductPackaged"; 10439 case MEDICINALPRODUCTPHARMACEUTICAL: 10440 return "MedicinalProductPharmaceutical"; 10441 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 10442 return "MedicinalProductUndesirableEffect"; 10443 case MESSAGEDEFINITION: 10444 return "MessageDefinition"; 10445 case MESSAGEHEADER: 10446 return "MessageHeader"; 10447 case MOLECULARSEQUENCE: 10448 return "MolecularSequence"; 10449 case NAMINGSYSTEM: 10450 return "NamingSystem"; 10451 case NUTRITIONORDER: 10452 return "NutritionOrder"; 10453 case OBSERVATION: 10454 return "Observation"; 10455 case OBSERVATIONDEFINITION: 10456 return "ObservationDefinition"; 10457 case OPERATIONDEFINITION: 10458 return "OperationDefinition"; 10459 case OPERATIONOUTCOME: 10460 return "OperationOutcome"; 10461 case ORGANIZATION: 10462 return "Organization"; 10463 case ORGANIZATIONAFFILIATION: 10464 return "OrganizationAffiliation"; 10465 case PARAMETERS: 10466 return "Parameters"; 10467 case PATIENT: 10468 return "Patient"; 10469 case PAYMENTNOTICE: 10470 return "PaymentNotice"; 10471 case PAYMENTRECONCILIATION: 10472 return "PaymentReconciliation"; 10473 case PERSON: 10474 return "Person"; 10475 case PLANDEFINITION: 10476 return "PlanDefinition"; 10477 case PRACTITIONER: 10478 return "Practitioner"; 10479 case PRACTITIONERROLE: 10480 return "PractitionerRole"; 10481 case PROCEDURE: 10482 return "Procedure"; 10483 case PROVENANCE: 10484 return "Provenance"; 10485 case QUESTIONNAIRE: 10486 return "Questionnaire"; 10487 case QUESTIONNAIRERESPONSE: 10488 return "QuestionnaireResponse"; 10489 case RELATEDPERSON: 10490 return "RelatedPerson"; 10491 case REQUESTGROUP: 10492 return "RequestGroup"; 10493 case RESEARCHDEFINITION: 10494 return "ResearchDefinition"; 10495 case RESEARCHELEMENTDEFINITION: 10496 return "ResearchElementDefinition"; 10497 case RESEARCHSTUDY: 10498 return "ResearchStudy"; 10499 case RESEARCHSUBJECT: 10500 return "ResearchSubject"; 10501 case RESOURCE: 10502 return "Resource"; 10503 case RISKASSESSMENT: 10504 return "RiskAssessment"; 10505 case RISKEVIDENCESYNTHESIS: 10506 return "RiskEvidenceSynthesis"; 10507 case SCHEDULE: 10508 return "Schedule"; 10509 case SEARCHPARAMETER: 10510 return "SearchParameter"; 10511 case SERVICEREQUEST: 10512 return "ServiceRequest"; 10513 case SLOT: 10514 return "Slot"; 10515 case SPECIMEN: 10516 return "Specimen"; 10517 case SPECIMENDEFINITION: 10518 return "SpecimenDefinition"; 10519 case STRUCTUREDEFINITION: 10520 return "StructureDefinition"; 10521 case STRUCTUREMAP: 10522 return "StructureMap"; 10523 case SUBSCRIPTION: 10524 return "Subscription"; 10525 case SUBSTANCE: 10526 return "Substance"; 10527 case SUBSTANCENUCLEICACID: 10528 return "SubstanceNucleicAcid"; 10529 case SUBSTANCEPOLYMER: 10530 return "SubstancePolymer"; 10531 case SUBSTANCEPROTEIN: 10532 return "SubstanceProtein"; 10533 case SUBSTANCEREFERENCEINFORMATION: 10534 return "SubstanceReferenceInformation"; 10535 case SUBSTANCESOURCEMATERIAL: 10536 return "SubstanceSourceMaterial"; 10537 case SUBSTANCESPECIFICATION: 10538 return "SubstanceSpecification"; 10539 case SUPPLYDELIVERY: 10540 return "SupplyDelivery"; 10541 case SUPPLYREQUEST: 10542 return "SupplyRequest"; 10543 case TASK: 10544 return "Task"; 10545 case TERMINOLOGYCAPABILITIES: 10546 return "TerminologyCapabilities"; 10547 case TESTREPORT: 10548 return "TestReport"; 10549 case TESTSCRIPT: 10550 return "TestScript"; 10551 case VALUESET: 10552 return "ValueSet"; 10553 case VERIFICATIONRESULT: 10554 return "VerificationResult"; 10555 case VISIONPRESCRIPTION: 10556 return "VisionPrescription"; 10557 case NULL: 10558 return null; 10559 default: 10560 return "?"; 10561 } 10562 } 10563 10564 public String getSystem() { 10565 switch (this) { 10566 case ADDRESS: 10567 return "http://hl7.org/fhir/data-types"; 10568 case AGE: 10569 return "http://hl7.org/fhir/data-types"; 10570 case ANNOTATION: 10571 return "http://hl7.org/fhir/data-types"; 10572 case ATTACHMENT: 10573 return "http://hl7.org/fhir/data-types"; 10574 case BACKBONEELEMENT: 10575 return "http://hl7.org/fhir/data-types"; 10576 case CODEABLECONCEPT: 10577 return "http://hl7.org/fhir/data-types"; 10578 case CODING: 10579 return "http://hl7.org/fhir/data-types"; 10580 case CONTACTDETAIL: 10581 return "http://hl7.org/fhir/data-types"; 10582 case CONTACTPOINT: 10583 return "http://hl7.org/fhir/data-types"; 10584 case CONTRIBUTOR: 10585 return "http://hl7.org/fhir/data-types"; 10586 case COUNT: 10587 return "http://hl7.org/fhir/data-types"; 10588 case DATAREQUIREMENT: 10589 return "http://hl7.org/fhir/data-types"; 10590 case DISTANCE: 10591 return "http://hl7.org/fhir/data-types"; 10592 case DOSAGE: 10593 return "http://hl7.org/fhir/data-types"; 10594 case DURATION: 10595 return "http://hl7.org/fhir/data-types"; 10596 case ELEMENT: 10597 return "http://hl7.org/fhir/data-types"; 10598 case ELEMENTDEFINITION: 10599 return "http://hl7.org/fhir/data-types"; 10600 case EXPRESSION: 10601 return "http://hl7.org/fhir/data-types"; 10602 case EXTENSION: 10603 return "http://hl7.org/fhir/data-types"; 10604 case HUMANNAME: 10605 return "http://hl7.org/fhir/data-types"; 10606 case IDENTIFIER: 10607 return "http://hl7.org/fhir/data-types"; 10608 case MARKETINGSTATUS: 10609 return "http://hl7.org/fhir/data-types"; 10610 case META: 10611 return "http://hl7.org/fhir/data-types"; 10612 case MONEY: 10613 return "http://hl7.org/fhir/data-types"; 10614 case MONEYQUANTITY: 10615 return "http://hl7.org/fhir/data-types"; 10616 case NARRATIVE: 10617 return "http://hl7.org/fhir/data-types"; 10618 case PARAMETERDEFINITION: 10619 return "http://hl7.org/fhir/data-types"; 10620 case PERIOD: 10621 return "http://hl7.org/fhir/data-types"; 10622 case POPULATION: 10623 return "http://hl7.org/fhir/data-types"; 10624 case PRODCHARACTERISTIC: 10625 return "http://hl7.org/fhir/data-types"; 10626 case PRODUCTSHELFLIFE: 10627 return "http://hl7.org/fhir/data-types"; 10628 case QUANTITY: 10629 return "http://hl7.org/fhir/data-types"; 10630 case RANGE: 10631 return "http://hl7.org/fhir/data-types"; 10632 case RATIO: 10633 return "http://hl7.org/fhir/data-types"; 10634 case REFERENCE: 10635 return "http://hl7.org/fhir/data-types"; 10636 case RELATEDARTIFACT: 10637 return "http://hl7.org/fhir/data-types"; 10638 case SAMPLEDDATA: 10639 return "http://hl7.org/fhir/data-types"; 10640 case SIGNATURE: 10641 return "http://hl7.org/fhir/data-types"; 10642 case SIMPLEQUANTITY: 10643 return "http://hl7.org/fhir/data-types"; 10644 case SUBSTANCEAMOUNT: 10645 return "http://hl7.org/fhir/data-types"; 10646 case TIMING: 10647 return "http://hl7.org/fhir/data-types"; 10648 case TRIGGERDEFINITION: 10649 return "http://hl7.org/fhir/data-types"; 10650 case USAGECONTEXT: 10651 return "http://hl7.org/fhir/data-types"; 10652 case BASE64BINARY: 10653 return "http://hl7.org/fhir/data-types"; 10654 case BOOLEAN: 10655 return "http://hl7.org/fhir/data-types"; 10656 case CANONICAL: 10657 return "http://hl7.org/fhir/data-types"; 10658 case CODE: 10659 return "http://hl7.org/fhir/data-types"; 10660 case DATE: 10661 return "http://hl7.org/fhir/data-types"; 10662 case DATETIME: 10663 return "http://hl7.org/fhir/data-types"; 10664 case DECIMAL: 10665 return "http://hl7.org/fhir/data-types"; 10666 case ID: 10667 return "http://hl7.org/fhir/data-types"; 10668 case INSTANT: 10669 return "http://hl7.org/fhir/data-types"; 10670 case INTEGER: 10671 return "http://hl7.org/fhir/data-types"; 10672 case MARKDOWN: 10673 return "http://hl7.org/fhir/data-types"; 10674 case OID: 10675 return "http://hl7.org/fhir/data-types"; 10676 case POSITIVEINT: 10677 return "http://hl7.org/fhir/data-types"; 10678 case STRING: 10679 return "http://hl7.org/fhir/data-types"; 10680 case TIME: 10681 return "http://hl7.org/fhir/data-types"; 10682 case UNSIGNEDINT: 10683 return "http://hl7.org/fhir/data-types"; 10684 case URI: 10685 return "http://hl7.org/fhir/data-types"; 10686 case URL: 10687 return "http://hl7.org/fhir/data-types"; 10688 case UUID: 10689 return "http://hl7.org/fhir/data-types"; 10690 case XHTML: 10691 return "http://hl7.org/fhir/data-types"; 10692 case ACCOUNT: 10693 return "http://hl7.org/fhir/resource-types"; 10694 case ACTIVITYDEFINITION: 10695 return "http://hl7.org/fhir/resource-types"; 10696 case ADVERSEEVENT: 10697 return "http://hl7.org/fhir/resource-types"; 10698 case ALLERGYINTOLERANCE: 10699 return "http://hl7.org/fhir/resource-types"; 10700 case APPOINTMENT: 10701 return "http://hl7.org/fhir/resource-types"; 10702 case APPOINTMENTRESPONSE: 10703 return "http://hl7.org/fhir/resource-types"; 10704 case AUDITEVENT: 10705 return "http://hl7.org/fhir/resource-types"; 10706 case BASIC: 10707 return "http://hl7.org/fhir/resource-types"; 10708 case BINARY: 10709 return "http://hl7.org/fhir/resource-types"; 10710 case BIOLOGICALLYDERIVEDPRODUCT: 10711 return "http://hl7.org/fhir/resource-types"; 10712 case BODYSTRUCTURE: 10713 return "http://hl7.org/fhir/resource-types"; 10714 case BUNDLE: 10715 return "http://hl7.org/fhir/resource-types"; 10716 case CAPABILITYSTATEMENT: 10717 return "http://hl7.org/fhir/resource-types"; 10718 case CAREPLAN: 10719 return "http://hl7.org/fhir/resource-types"; 10720 case CARETEAM: 10721 return "http://hl7.org/fhir/resource-types"; 10722 case CATALOGENTRY: 10723 return "http://hl7.org/fhir/resource-types"; 10724 case CHARGEITEM: 10725 return "http://hl7.org/fhir/resource-types"; 10726 case CHARGEITEMDEFINITION: 10727 return "http://hl7.org/fhir/resource-types"; 10728 case CLAIM: 10729 return "http://hl7.org/fhir/resource-types"; 10730 case CLAIMRESPONSE: 10731 return "http://hl7.org/fhir/resource-types"; 10732 case CLINICALIMPRESSION: 10733 return "http://hl7.org/fhir/resource-types"; 10734 case CODESYSTEM: 10735 return "http://hl7.org/fhir/resource-types"; 10736 case COMMUNICATION: 10737 return "http://hl7.org/fhir/resource-types"; 10738 case COMMUNICATIONREQUEST: 10739 return "http://hl7.org/fhir/resource-types"; 10740 case COMPARTMENTDEFINITION: 10741 return "http://hl7.org/fhir/resource-types"; 10742 case COMPOSITION: 10743 return "http://hl7.org/fhir/resource-types"; 10744 case CONCEPTMAP: 10745 return "http://hl7.org/fhir/resource-types"; 10746 case CONDITION: 10747 return "http://hl7.org/fhir/resource-types"; 10748 case CONSENT: 10749 return "http://hl7.org/fhir/resource-types"; 10750 case CONTRACT: 10751 return "http://hl7.org/fhir/resource-types"; 10752 case COVERAGE: 10753 return "http://hl7.org/fhir/resource-types"; 10754 case COVERAGEELIGIBILITYREQUEST: 10755 return "http://hl7.org/fhir/resource-types"; 10756 case COVERAGEELIGIBILITYRESPONSE: 10757 return "http://hl7.org/fhir/resource-types"; 10758 case DETECTEDISSUE: 10759 return "http://hl7.org/fhir/resource-types"; 10760 case DEVICE: 10761 return "http://hl7.org/fhir/resource-types"; 10762 case DEVICEDEFINITION: 10763 return "http://hl7.org/fhir/resource-types"; 10764 case DEVICEMETRIC: 10765 return "http://hl7.org/fhir/resource-types"; 10766 case DEVICEREQUEST: 10767 return "http://hl7.org/fhir/resource-types"; 10768 case DEVICEUSESTATEMENT: 10769 return "http://hl7.org/fhir/resource-types"; 10770 case DIAGNOSTICREPORT: 10771 return "http://hl7.org/fhir/resource-types"; 10772 case DOCUMENTMANIFEST: 10773 return "http://hl7.org/fhir/resource-types"; 10774 case DOCUMENTREFERENCE: 10775 return "http://hl7.org/fhir/resource-types"; 10776 case DOMAINRESOURCE: 10777 return "http://hl7.org/fhir/resource-types"; 10778 case EFFECTEVIDENCESYNTHESIS: 10779 return "http://hl7.org/fhir/resource-types"; 10780 case ENCOUNTER: 10781 return "http://hl7.org/fhir/resource-types"; 10782 case ENDPOINT: 10783 return "http://hl7.org/fhir/resource-types"; 10784 case ENROLLMENTREQUEST: 10785 return "http://hl7.org/fhir/resource-types"; 10786 case ENROLLMENTRESPONSE: 10787 return "http://hl7.org/fhir/resource-types"; 10788 case EPISODEOFCARE: 10789 return "http://hl7.org/fhir/resource-types"; 10790 case EVENTDEFINITION: 10791 return "http://hl7.org/fhir/resource-types"; 10792 case EVIDENCE: 10793 return "http://hl7.org/fhir/resource-types"; 10794 case EVIDENCEVARIABLE: 10795 return "http://hl7.org/fhir/resource-types"; 10796 case EXAMPLESCENARIO: 10797 return "http://hl7.org/fhir/resource-types"; 10798 case EXPLANATIONOFBENEFIT: 10799 return "http://hl7.org/fhir/resource-types"; 10800 case FAMILYMEMBERHISTORY: 10801 return "http://hl7.org/fhir/resource-types"; 10802 case FLAG: 10803 return "http://hl7.org/fhir/resource-types"; 10804 case GOAL: 10805 return "http://hl7.org/fhir/resource-types"; 10806 case GRAPHDEFINITION: 10807 return "http://hl7.org/fhir/resource-types"; 10808 case GROUP: 10809 return "http://hl7.org/fhir/resource-types"; 10810 case GUIDANCERESPONSE: 10811 return "http://hl7.org/fhir/resource-types"; 10812 case HEALTHCARESERVICE: 10813 return "http://hl7.org/fhir/resource-types"; 10814 case IMAGINGSTUDY: 10815 return "http://hl7.org/fhir/resource-types"; 10816 case IMMUNIZATION: 10817 return "http://hl7.org/fhir/resource-types"; 10818 case IMMUNIZATIONEVALUATION: 10819 return "http://hl7.org/fhir/resource-types"; 10820 case IMMUNIZATIONRECOMMENDATION: 10821 return "http://hl7.org/fhir/resource-types"; 10822 case IMPLEMENTATIONGUIDE: 10823 return "http://hl7.org/fhir/resource-types"; 10824 case INSURANCEPLAN: 10825 return "http://hl7.org/fhir/resource-types"; 10826 case INVOICE: 10827 return "http://hl7.org/fhir/resource-types"; 10828 case LIBRARY: 10829 return "http://hl7.org/fhir/resource-types"; 10830 case LINKAGE: 10831 return "http://hl7.org/fhir/resource-types"; 10832 case LIST: 10833 return "http://hl7.org/fhir/resource-types"; 10834 case LOCATION: 10835 return "http://hl7.org/fhir/resource-types"; 10836 case MEASURE: 10837 return "http://hl7.org/fhir/resource-types"; 10838 case MEASUREREPORT: 10839 return "http://hl7.org/fhir/resource-types"; 10840 case MEDIA: 10841 return "http://hl7.org/fhir/resource-types"; 10842 case MEDICATION: 10843 return "http://hl7.org/fhir/resource-types"; 10844 case MEDICATIONADMINISTRATION: 10845 return "http://hl7.org/fhir/resource-types"; 10846 case MEDICATIONDISPENSE: 10847 return "http://hl7.org/fhir/resource-types"; 10848 case MEDICATIONKNOWLEDGE: 10849 return "http://hl7.org/fhir/resource-types"; 10850 case MEDICATIONREQUEST: 10851 return "http://hl7.org/fhir/resource-types"; 10852 case MEDICATIONSTATEMENT: 10853 return "http://hl7.org/fhir/resource-types"; 10854 case MEDICINALPRODUCT: 10855 return "http://hl7.org/fhir/resource-types"; 10856 case MEDICINALPRODUCTAUTHORIZATION: 10857 return "http://hl7.org/fhir/resource-types"; 10858 case MEDICINALPRODUCTCONTRAINDICATION: 10859 return "http://hl7.org/fhir/resource-types"; 10860 case MEDICINALPRODUCTINDICATION: 10861 return "http://hl7.org/fhir/resource-types"; 10862 case MEDICINALPRODUCTINGREDIENT: 10863 return "http://hl7.org/fhir/resource-types"; 10864 case MEDICINALPRODUCTINTERACTION: 10865 return "http://hl7.org/fhir/resource-types"; 10866 case MEDICINALPRODUCTMANUFACTURED: 10867 return "http://hl7.org/fhir/resource-types"; 10868 case MEDICINALPRODUCTPACKAGED: 10869 return "http://hl7.org/fhir/resource-types"; 10870 case MEDICINALPRODUCTPHARMACEUTICAL: 10871 return "http://hl7.org/fhir/resource-types"; 10872 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 10873 return "http://hl7.org/fhir/resource-types"; 10874 case MESSAGEDEFINITION: 10875 return "http://hl7.org/fhir/resource-types"; 10876 case MESSAGEHEADER: 10877 return "http://hl7.org/fhir/resource-types"; 10878 case MOLECULARSEQUENCE: 10879 return "http://hl7.org/fhir/resource-types"; 10880 case NAMINGSYSTEM: 10881 return "http://hl7.org/fhir/resource-types"; 10882 case NUTRITIONORDER: 10883 return "http://hl7.org/fhir/resource-types"; 10884 case OBSERVATION: 10885 return "http://hl7.org/fhir/resource-types"; 10886 case OBSERVATIONDEFINITION: 10887 return "http://hl7.org/fhir/resource-types"; 10888 case OPERATIONDEFINITION: 10889 return "http://hl7.org/fhir/resource-types"; 10890 case OPERATIONOUTCOME: 10891 return "http://hl7.org/fhir/resource-types"; 10892 case ORGANIZATION: 10893 return "http://hl7.org/fhir/resource-types"; 10894 case ORGANIZATIONAFFILIATION: 10895 return "http://hl7.org/fhir/resource-types"; 10896 case PARAMETERS: 10897 return "http://hl7.org/fhir/resource-types"; 10898 case PATIENT: 10899 return "http://hl7.org/fhir/resource-types"; 10900 case PAYMENTNOTICE: 10901 return "http://hl7.org/fhir/resource-types"; 10902 case PAYMENTRECONCILIATION: 10903 return "http://hl7.org/fhir/resource-types"; 10904 case PERSON: 10905 return "http://hl7.org/fhir/resource-types"; 10906 case PLANDEFINITION: 10907 return "http://hl7.org/fhir/resource-types"; 10908 case PRACTITIONER: 10909 return "http://hl7.org/fhir/resource-types"; 10910 case PRACTITIONERROLE: 10911 return "http://hl7.org/fhir/resource-types"; 10912 case PROCEDURE: 10913 return "http://hl7.org/fhir/resource-types"; 10914 case PROVENANCE: 10915 return "http://hl7.org/fhir/resource-types"; 10916 case QUESTIONNAIRE: 10917 return "http://hl7.org/fhir/resource-types"; 10918 case QUESTIONNAIRERESPONSE: 10919 return "http://hl7.org/fhir/resource-types"; 10920 case RELATEDPERSON: 10921 return "http://hl7.org/fhir/resource-types"; 10922 case REQUESTGROUP: 10923 return "http://hl7.org/fhir/resource-types"; 10924 case RESEARCHDEFINITION: 10925 return "http://hl7.org/fhir/resource-types"; 10926 case RESEARCHELEMENTDEFINITION: 10927 return "http://hl7.org/fhir/resource-types"; 10928 case RESEARCHSTUDY: 10929 return "http://hl7.org/fhir/resource-types"; 10930 case RESEARCHSUBJECT: 10931 return "http://hl7.org/fhir/resource-types"; 10932 case RESOURCE: 10933 return "http://hl7.org/fhir/resource-types"; 10934 case RISKASSESSMENT: 10935 return "http://hl7.org/fhir/resource-types"; 10936 case RISKEVIDENCESYNTHESIS: 10937 return "http://hl7.org/fhir/resource-types"; 10938 case SCHEDULE: 10939 return "http://hl7.org/fhir/resource-types"; 10940 case SEARCHPARAMETER: 10941 return "http://hl7.org/fhir/resource-types"; 10942 case SERVICEREQUEST: 10943 return "http://hl7.org/fhir/resource-types"; 10944 case SLOT: 10945 return "http://hl7.org/fhir/resource-types"; 10946 case SPECIMEN: 10947 return "http://hl7.org/fhir/resource-types"; 10948 case SPECIMENDEFINITION: 10949 return "http://hl7.org/fhir/resource-types"; 10950 case STRUCTUREDEFINITION: 10951 return "http://hl7.org/fhir/resource-types"; 10952 case STRUCTUREMAP: 10953 return "http://hl7.org/fhir/resource-types"; 10954 case SUBSCRIPTION: 10955 return "http://hl7.org/fhir/resource-types"; 10956 case SUBSTANCE: 10957 return "http://hl7.org/fhir/resource-types"; 10958 case SUBSTANCENUCLEICACID: 10959 return "http://hl7.org/fhir/resource-types"; 10960 case SUBSTANCEPOLYMER: 10961 return "http://hl7.org/fhir/resource-types"; 10962 case SUBSTANCEPROTEIN: 10963 return "http://hl7.org/fhir/resource-types"; 10964 case SUBSTANCEREFERENCEINFORMATION: 10965 return "http://hl7.org/fhir/resource-types"; 10966 case SUBSTANCESOURCEMATERIAL: 10967 return "http://hl7.org/fhir/resource-types"; 10968 case SUBSTANCESPECIFICATION: 10969 return "http://hl7.org/fhir/resource-types"; 10970 case SUPPLYDELIVERY: 10971 return "http://hl7.org/fhir/resource-types"; 10972 case SUPPLYREQUEST: 10973 return "http://hl7.org/fhir/resource-types"; 10974 case TASK: 10975 return "http://hl7.org/fhir/resource-types"; 10976 case TERMINOLOGYCAPABILITIES: 10977 return "http://hl7.org/fhir/resource-types"; 10978 case TESTREPORT: 10979 return "http://hl7.org/fhir/resource-types"; 10980 case TESTSCRIPT: 10981 return "http://hl7.org/fhir/resource-types"; 10982 case VALUESET: 10983 return "http://hl7.org/fhir/resource-types"; 10984 case VERIFICATIONRESULT: 10985 return "http://hl7.org/fhir/resource-types"; 10986 case VISIONPRESCRIPTION: 10987 return "http://hl7.org/fhir/resource-types"; 10988 case NULL: 10989 return null; 10990 default: 10991 return "?"; 10992 } 10993 } 10994 10995 public String getDefinition() { 10996 switch (this) { 10997 case ADDRESS: 10998 return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 10999 case AGE: 11000 return "A duration of time during which an organism (or a process) has existed."; 11001 case ANNOTATION: 11002 return "A text note which also contains information about who made the statement and when."; 11003 case ATTACHMENT: 11004 return "For referring to data content defined in other formats."; 11005 case BACKBONEELEMENT: 11006 return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 11007 case CODEABLECONCEPT: 11008 return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 11009 case CODING: 11010 return "A reference to a code defined by a terminology system."; 11011 case CONTACTDETAIL: 11012 return "Specifies contact information for a person or organization."; 11013 case CONTACTPOINT: 11014 return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 11015 case CONTRIBUTOR: 11016 return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 11017 case COUNT: 11018 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 11019 case DATAREQUIREMENT: 11020 return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 11021 case DISTANCE: 11022 return "A length - a value with a unit that is a physical distance."; 11023 case DOSAGE: 11024 return "Indicates how the medication is/was taken or should be taken by the patient."; 11025 case DURATION: 11026 return "A length of time."; 11027 case ELEMENT: 11028 return "Base definition for all elements in a resource."; 11029 case ELEMENTDEFINITION: 11030 return "Captures constraints on each element within the resource, profile, or extension."; 11031 case EXPRESSION: 11032 return "A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used."; 11033 case EXTENSION: 11034 return "Optional Extension Element - found in all resources."; 11035 case HUMANNAME: 11036 return "A human's name with the ability to identify parts and usage."; 11037 case IDENTIFIER: 11038 return "An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers."; 11039 case MARKETINGSTATUS: 11040 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 11041 case META: 11042 return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content might not always be associated with version changes to the resource."; 11043 case MONEY: 11044 return "An amount of economic utility in some recognized currency."; 11045 case MONEYQUANTITY: 11046 return ""; 11047 case NARRATIVE: 11048 return "A human-readable summary of the resource conveying the essential clinical and business information for the resource."; 11049 case PARAMETERDEFINITION: 11050 return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 11051 case PERIOD: 11052 return "A time period defined by a start and end date and optionally time."; 11053 case POPULATION: 11054 return "A populatioof people with some set of grouping criteria."; 11055 case PRODCHARACTERISTIC: 11056 return "The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available."; 11057 case PRODUCTSHELFLIFE: 11058 return "The shelf-life and storage information for a medicinal product item or container can be described using this class."; 11059 case QUANTITY: 11060 return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 11061 case RANGE: 11062 return "A set of ordered Quantities defined by a low and high limit."; 11063 case RATIO: 11064 return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 11065 case REFERENCE: 11066 return "A reference from one resource to another."; 11067 case RELATEDARTIFACT: 11068 return "Related artifacts such as additional documentation, justification, or bibliographic references."; 11069 case SAMPLEDDATA: 11070 return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 11071 case SIGNATURE: 11072 return "A signature along with supporting context. The signature may be a digital signature that is cryptographic in nature, or some other signature acceptable to the domain. This other signature may be as simple as a graphical image representing a hand-written signature, or a signature ceremony Different signature approaches have different utilities."; 11073 case SIMPLEQUANTITY: 11074 return ""; 11075 case SUBSTANCEAMOUNT: 11076 return "Chemical substances are a single substance type whose primary defining element is the molecular structure. Chemical substances shall be defined on the basis of their complete covalent molecular structure; the presence of a salt (counter-ion) and/or solvates (water, alcohols) is also captured. Purity, grade, physical form or particle size are not taken into account in the definition of a chemical substance or in the assignment of a Substance ID."; 11077 case TIMING: 11078 return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 11079 case TRIGGERDEFINITION: 11080 return "A description of a triggering event. Triggering events can be named events, data events, or periodic, as determined by the type element."; 11081 case USAGECONTEXT: 11082 return "Specifies clinical/business/etc. metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 11083 case BASE64BINARY: 11084 return "A stream of bytes"; 11085 case BOOLEAN: 11086 return "Value of \"true\" or \"false\""; 11087 case CANONICAL: 11088 return "A URI that is a reference to a canonical URL on a FHIR resource"; 11089 case CODE: 11090 return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 11091 case DATE: 11092 return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 11093 case DATETIME: 11094 return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 11095 case DECIMAL: 11096 return "A rational number with implicit precision"; 11097 case ID: 11098 return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 11099 case INSTANT: 11100 return "An instant in time - known at least to the second"; 11101 case INTEGER: 11102 return "A whole number"; 11103 case MARKDOWN: 11104 return "A string that may contain Github Flavored Markdown syntax for optional processing by a mark down presentation engine"; 11105 case OID: 11106 return "An OID represented as a URI"; 11107 case POSITIVEINT: 11108 return "An integer with a value that is positive (e.g. >0)"; 11109 case STRING: 11110 return "A sequence of Unicode characters"; 11111 case TIME: 11112 return "A time during the day, with no date specified"; 11113 case UNSIGNEDINT: 11114 return "An integer with a value that is not negative (e.g. >= 0)"; 11115 case URI: 11116 return "String of characters used to identify a name or a resource"; 11117 case URL: 11118 return "A URI that is a literal reference"; 11119 case UUID: 11120 return "A UUID, represented as a URI"; 11121 case XHTML: 11122 return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 11123 case ACCOUNT: 11124 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 11125 case ACTIVITYDEFINITION: 11126 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 11127 case ADVERSEEVENT: 11128 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 11129 case ALLERGYINTOLERANCE: 11130 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 11131 case APPOINTMENT: 11132 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 11133 case APPOINTMENTRESPONSE: 11134 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 11135 case AUDITEVENT: 11136 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 11137 case BASIC: 11138 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 11139 case BINARY: 11140 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 11141 case BIOLOGICALLYDERIVEDPRODUCT: 11142 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 11143 case BODYSTRUCTURE: 11144 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 11145 case BUNDLE: 11146 return "A container for a collection of resources."; 11147 case CAPABILITYSTATEMENT: 11148 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 11149 case CAREPLAN: 11150 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 11151 case CARETEAM: 11152 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 11153 case CATALOGENTRY: 11154 return "Catalog entries are wrappers that contextualize items included in a catalog."; 11155 case CHARGEITEM: 11156 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 11157 case CHARGEITEMDEFINITION: 11158 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 11159 case CLAIM: 11160 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 11161 case CLAIMRESPONSE: 11162 return "This resource provides the adjudication details from the processing of a Claim resource."; 11163 case CLINICALIMPRESSION: 11164 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 11165 case CODESYSTEM: 11166 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 11167 case COMMUNICATION: 11168 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 11169 case COMMUNICATIONREQUEST: 11170 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 11171 case COMPARTMENTDEFINITION: 11172 return "A compartment definition that defines how resources are accessed on a server."; 11173 case COMPOSITION: 11174 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 11175 case CONCEPTMAP: 11176 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 11177 case CONDITION: 11178 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 11179 case CONSENT: 11180 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 11181 case CONTRACT: 11182 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 11183 case COVERAGE: 11184 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 11185 case COVERAGEELIGIBILITYREQUEST: 11186 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 11187 case COVERAGEELIGIBILITYRESPONSE: 11188 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 11189 case DETECTEDISSUE: 11190 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 11191 case DEVICE: 11192 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 11193 case DEVICEDEFINITION: 11194 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 11195 case DEVICEMETRIC: 11196 return "Describes a measurement, calculation or setting capability of a medical device."; 11197 case DEVICEREQUEST: 11198 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 11199 case DEVICEUSESTATEMENT: 11200 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 11201 case DIAGNOSTICREPORT: 11202 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 11203 case DOCUMENTMANIFEST: 11204 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 11205 case DOCUMENTREFERENCE: 11206 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 11207 case DOMAINRESOURCE: 11208 return "A resource that includes narrative, extensions, and contained resources."; 11209 case EFFECTEVIDENCESYNTHESIS: 11210 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 11211 case ENCOUNTER: 11212 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 11213 case ENDPOINT: 11214 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 11215 case ENROLLMENTREQUEST: 11216 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 11217 case ENROLLMENTRESPONSE: 11218 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 11219 case EPISODEOFCARE: 11220 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 11221 case EVENTDEFINITION: 11222 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 11223 case EVIDENCE: 11224 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 11225 case EVIDENCEVARIABLE: 11226 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 11227 case EXAMPLESCENARIO: 11228 return "Example of workflow instance."; 11229 case EXPLANATIONOFBENEFIT: 11230 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 11231 case FAMILYMEMBERHISTORY: 11232 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 11233 case FLAG: 11234 return "Prospective warnings of potential issues when providing care to the patient."; 11235 case GOAL: 11236 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 11237 case GRAPHDEFINITION: 11238 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 11239 case GROUP: 11240 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 11241 case GUIDANCERESPONSE: 11242 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 11243 case HEALTHCARESERVICE: 11244 return "The details of a healthcare service available at a location."; 11245 case IMAGINGSTUDY: 11246 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 11247 case IMMUNIZATION: 11248 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 11249 case IMMUNIZATIONEVALUATION: 11250 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 11251 case IMMUNIZATIONRECOMMENDATION: 11252 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 11253 case IMPLEMENTATIONGUIDE: 11254 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 11255 case INSURANCEPLAN: 11256 return "Details of a Health Insurance product/plan provided by an organization."; 11257 case INVOICE: 11258 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 11259 case LIBRARY: 11260 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 11261 case LINKAGE: 11262 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 11263 case LIST: 11264 return "A list is a curated collection of resources."; 11265 case LOCATION: 11266 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 11267 case MEASURE: 11268 return "The Measure resource provides the definition of a quality measure."; 11269 case MEASUREREPORT: 11270 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 11271 case MEDIA: 11272 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 11273 case MEDICATION: 11274 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 11275 case MEDICATIONADMINISTRATION: 11276 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 11277 case MEDICATIONDISPENSE: 11278 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 11279 case MEDICATIONKNOWLEDGE: 11280 return "Information about a medication that is used to support knowledge."; 11281 case MEDICATIONREQUEST: 11282 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 11283 case MEDICATIONSTATEMENT: 11284 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 11285 case MEDICINALPRODUCT: 11286 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 11287 case MEDICINALPRODUCTAUTHORIZATION: 11288 return "The regulatory authorization of a medicinal product."; 11289 case MEDICINALPRODUCTCONTRAINDICATION: 11290 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 11291 case MEDICINALPRODUCTINDICATION: 11292 return "Indication for the Medicinal Product."; 11293 case MEDICINALPRODUCTINGREDIENT: 11294 return "An ingredient of a manufactured item or pharmaceutical product."; 11295 case MEDICINALPRODUCTINTERACTION: 11296 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 11297 case MEDICINALPRODUCTMANUFACTURED: 11298 return "The manufactured item as contained in the packaged medicinal product."; 11299 case MEDICINALPRODUCTPACKAGED: 11300 return "A medicinal product in a container or package."; 11301 case MEDICINALPRODUCTPHARMACEUTICAL: 11302 return "A pharmaceutical product described in terms of its composition and dose form."; 11303 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 11304 return "Describe the undesirable effects of the medicinal product."; 11305 case MESSAGEDEFINITION: 11306 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 11307 case MESSAGEHEADER: 11308 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 11309 case MOLECULARSEQUENCE: 11310 return "Raw data describing a biological sequence."; 11311 case NAMINGSYSTEM: 11312 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 11313 case NUTRITIONORDER: 11314 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 11315 case OBSERVATION: 11316 return "Measurements and simple assertions made about a patient, device or other subject."; 11317 case OBSERVATIONDEFINITION: 11318 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 11319 case OPERATIONDEFINITION: 11320 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 11321 case OPERATIONOUTCOME: 11322 return "A collection of error, warning, or information messages that result from a system action."; 11323 case ORGANIZATION: 11324 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 11325 case ORGANIZATIONAFFILIATION: 11326 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 11327 case PARAMETERS: 11328 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 11329 case PATIENT: 11330 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 11331 case PAYMENTNOTICE: 11332 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 11333 case PAYMENTRECONCILIATION: 11334 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 11335 case PERSON: 11336 return "Demographics and administrative information about a person independent of a specific health-related context."; 11337 case PLANDEFINITION: 11338 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 11339 case PRACTITIONER: 11340 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 11341 case PRACTITIONERROLE: 11342 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 11343 case PROCEDURE: 11344 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 11345 case PROVENANCE: 11346 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 11347 case QUESTIONNAIRE: 11348 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 11349 case QUESTIONNAIRERESPONSE: 11350 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 11351 case RELATEDPERSON: 11352 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 11353 case REQUESTGROUP: 11354 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 11355 case RESEARCHDEFINITION: 11356 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 11357 case RESEARCHELEMENTDEFINITION: 11358 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 11359 case RESEARCHSTUDY: 11360 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 11361 case RESEARCHSUBJECT: 11362 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 11363 case RESOURCE: 11364 return "This is the base resource type for everything."; 11365 case RISKASSESSMENT: 11366 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 11367 case RISKEVIDENCESYNTHESIS: 11368 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 11369 case SCHEDULE: 11370 return "A container for slots of time that may be available for booking appointments."; 11371 case SEARCHPARAMETER: 11372 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 11373 case SERVICEREQUEST: 11374 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 11375 case SLOT: 11376 return "A slot of time on a schedule that may be available for booking appointments."; 11377 case SPECIMEN: 11378 return "A sample to be used for analysis."; 11379 case SPECIMENDEFINITION: 11380 return "A kind of specimen with associated set of requirements."; 11381 case STRUCTUREDEFINITION: 11382 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 11383 case STRUCTUREMAP: 11384 return "A Map of relationships between 2 structures that can be used to transform data."; 11385 case SUBSCRIPTION: 11386 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 11387 case SUBSTANCE: 11388 return "A homogeneous material with a definite composition."; 11389 case SUBSTANCENUCLEICACID: 11390 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 11391 case SUBSTANCEPOLYMER: 11392 return "Todo."; 11393 case SUBSTANCEPROTEIN: 11394 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 11395 case SUBSTANCEREFERENCEINFORMATION: 11396 return "Todo."; 11397 case SUBSTANCESOURCEMATERIAL: 11398 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 11399 case SUBSTANCESPECIFICATION: 11400 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 11401 case SUPPLYDELIVERY: 11402 return "Record of delivery of what is supplied."; 11403 case SUPPLYREQUEST: 11404 return "A record of a request for a medication, substance or device used in the healthcare setting."; 11405 case TASK: 11406 return "A task to be performed."; 11407 case TERMINOLOGYCAPABILITIES: 11408 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 11409 case TESTREPORT: 11410 return "A summary of information based on the results of executing a TestScript."; 11411 case TESTSCRIPT: 11412 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 11413 case VALUESET: 11414 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 11415 case VERIFICATIONRESULT: 11416 return "Describes validation requirements, source(s), status and dates for one or more elements."; 11417 case VISIONPRESCRIPTION: 11418 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 11419 case NULL: 11420 return null; 11421 default: 11422 return "?"; 11423 } 11424 } 11425 11426 public String getDisplay() { 11427 switch (this) { 11428 case ADDRESS: 11429 return "Address"; 11430 case AGE: 11431 return "Age"; 11432 case ANNOTATION: 11433 return "Annotation"; 11434 case ATTACHMENT: 11435 return "Attachment"; 11436 case BACKBONEELEMENT: 11437 return "BackboneElement"; 11438 case CODEABLECONCEPT: 11439 return "CodeableConcept"; 11440 case CODING: 11441 return "Coding"; 11442 case CONTACTDETAIL: 11443 return "ContactDetail"; 11444 case CONTACTPOINT: 11445 return "ContactPoint"; 11446 case CONTRIBUTOR: 11447 return "Contributor"; 11448 case COUNT: 11449 return "Count"; 11450 case DATAREQUIREMENT: 11451 return "DataRequirement"; 11452 case DISTANCE: 11453 return "Distance"; 11454 case DOSAGE: 11455 return "Dosage"; 11456 case DURATION: 11457 return "Duration"; 11458 case ELEMENT: 11459 return "Element"; 11460 case ELEMENTDEFINITION: 11461 return "ElementDefinition"; 11462 case EXPRESSION: 11463 return "Expression"; 11464 case EXTENSION: 11465 return "Extension"; 11466 case HUMANNAME: 11467 return "HumanName"; 11468 case IDENTIFIER: 11469 return "Identifier"; 11470 case MARKETINGSTATUS: 11471 return "MarketingStatus"; 11472 case META: 11473 return "Meta"; 11474 case MONEY: 11475 return "Money"; 11476 case MONEYQUANTITY: 11477 return "MoneyQuantity"; 11478 case NARRATIVE: 11479 return "Narrative"; 11480 case PARAMETERDEFINITION: 11481 return "ParameterDefinition"; 11482 case PERIOD: 11483 return "Period"; 11484 case POPULATION: 11485 return "Population"; 11486 case PRODCHARACTERISTIC: 11487 return "ProdCharacteristic"; 11488 case PRODUCTSHELFLIFE: 11489 return "ProductShelfLife"; 11490 case QUANTITY: 11491 return "Quantity"; 11492 case RANGE: 11493 return "Range"; 11494 case RATIO: 11495 return "Ratio"; 11496 case REFERENCE: 11497 return "Reference"; 11498 case RELATEDARTIFACT: 11499 return "RelatedArtifact"; 11500 case SAMPLEDDATA: 11501 return "SampledData"; 11502 case SIGNATURE: 11503 return "Signature"; 11504 case SIMPLEQUANTITY: 11505 return "SimpleQuantity"; 11506 case SUBSTANCEAMOUNT: 11507 return "SubstanceAmount"; 11508 case TIMING: 11509 return "Timing"; 11510 case TRIGGERDEFINITION: 11511 return "TriggerDefinition"; 11512 case USAGECONTEXT: 11513 return "UsageContext"; 11514 case BASE64BINARY: 11515 return "base64Binary"; 11516 case BOOLEAN: 11517 return "boolean"; 11518 case CANONICAL: 11519 return "canonical"; 11520 case CODE: 11521 return "code"; 11522 case DATE: 11523 return "date"; 11524 case DATETIME: 11525 return "dateTime"; 11526 case DECIMAL: 11527 return "decimal"; 11528 case ID: 11529 return "id"; 11530 case INSTANT: 11531 return "instant"; 11532 case INTEGER: 11533 return "integer"; 11534 case MARKDOWN: 11535 return "markdown"; 11536 case OID: 11537 return "oid"; 11538 case POSITIVEINT: 11539 return "positiveInt"; 11540 case STRING: 11541 return "string"; 11542 case TIME: 11543 return "time"; 11544 case UNSIGNEDINT: 11545 return "unsignedInt"; 11546 case URI: 11547 return "uri"; 11548 case URL: 11549 return "url"; 11550 case UUID: 11551 return "uuid"; 11552 case XHTML: 11553 return "XHTML"; 11554 case ACCOUNT: 11555 return "Account"; 11556 case ACTIVITYDEFINITION: 11557 return "ActivityDefinition"; 11558 case ADVERSEEVENT: 11559 return "AdverseEvent"; 11560 case ALLERGYINTOLERANCE: 11561 return "AllergyIntolerance"; 11562 case APPOINTMENT: 11563 return "Appointment"; 11564 case APPOINTMENTRESPONSE: 11565 return "AppointmentResponse"; 11566 case AUDITEVENT: 11567 return "AuditEvent"; 11568 case BASIC: 11569 return "Basic"; 11570 case BINARY: 11571 return "Binary"; 11572 case BIOLOGICALLYDERIVEDPRODUCT: 11573 return "BiologicallyDerivedProduct"; 11574 case BODYSTRUCTURE: 11575 return "BodyStructure"; 11576 case BUNDLE: 11577 return "Bundle"; 11578 case CAPABILITYSTATEMENT: 11579 return "CapabilityStatement"; 11580 case CAREPLAN: 11581 return "CarePlan"; 11582 case CARETEAM: 11583 return "CareTeam"; 11584 case CATALOGENTRY: 11585 return "CatalogEntry"; 11586 case CHARGEITEM: 11587 return "ChargeItem"; 11588 case CHARGEITEMDEFINITION: 11589 return "ChargeItemDefinition"; 11590 case CLAIM: 11591 return "Claim"; 11592 case CLAIMRESPONSE: 11593 return "ClaimResponse"; 11594 case CLINICALIMPRESSION: 11595 return "ClinicalImpression"; 11596 case CODESYSTEM: 11597 return "CodeSystem"; 11598 case COMMUNICATION: 11599 return "Communication"; 11600 case COMMUNICATIONREQUEST: 11601 return "CommunicationRequest"; 11602 case COMPARTMENTDEFINITION: 11603 return "CompartmentDefinition"; 11604 case COMPOSITION: 11605 return "Composition"; 11606 case CONCEPTMAP: 11607 return "ConceptMap"; 11608 case CONDITION: 11609 return "Condition"; 11610 case CONSENT: 11611 return "Consent"; 11612 case CONTRACT: 11613 return "Contract"; 11614 case COVERAGE: 11615 return "Coverage"; 11616 case COVERAGEELIGIBILITYREQUEST: 11617 return "CoverageEligibilityRequest"; 11618 case COVERAGEELIGIBILITYRESPONSE: 11619 return "CoverageEligibilityResponse"; 11620 case DETECTEDISSUE: 11621 return "DetectedIssue"; 11622 case DEVICE: 11623 return "Device"; 11624 case DEVICEDEFINITION: 11625 return "DeviceDefinition"; 11626 case DEVICEMETRIC: 11627 return "DeviceMetric"; 11628 case DEVICEREQUEST: 11629 return "DeviceRequest"; 11630 case DEVICEUSESTATEMENT: 11631 return "DeviceUseStatement"; 11632 case DIAGNOSTICREPORT: 11633 return "DiagnosticReport"; 11634 case DOCUMENTMANIFEST: 11635 return "DocumentManifest"; 11636 case DOCUMENTREFERENCE: 11637 return "DocumentReference"; 11638 case DOMAINRESOURCE: 11639 return "DomainResource"; 11640 case EFFECTEVIDENCESYNTHESIS: 11641 return "EffectEvidenceSynthesis"; 11642 case ENCOUNTER: 11643 return "Encounter"; 11644 case ENDPOINT: 11645 return "Endpoint"; 11646 case ENROLLMENTREQUEST: 11647 return "EnrollmentRequest"; 11648 case ENROLLMENTRESPONSE: 11649 return "EnrollmentResponse"; 11650 case EPISODEOFCARE: 11651 return "EpisodeOfCare"; 11652 case EVENTDEFINITION: 11653 return "EventDefinition"; 11654 case EVIDENCE: 11655 return "Evidence"; 11656 case EVIDENCEVARIABLE: 11657 return "EvidenceVariable"; 11658 case EXAMPLESCENARIO: 11659 return "ExampleScenario"; 11660 case EXPLANATIONOFBENEFIT: 11661 return "ExplanationOfBenefit"; 11662 case FAMILYMEMBERHISTORY: 11663 return "FamilyMemberHistory"; 11664 case FLAG: 11665 return "Flag"; 11666 case GOAL: 11667 return "Goal"; 11668 case GRAPHDEFINITION: 11669 return "GraphDefinition"; 11670 case GROUP: 11671 return "Group"; 11672 case GUIDANCERESPONSE: 11673 return "GuidanceResponse"; 11674 case HEALTHCARESERVICE: 11675 return "HealthcareService"; 11676 case IMAGINGSTUDY: 11677 return "ImagingStudy"; 11678 case IMMUNIZATION: 11679 return "Immunization"; 11680 case IMMUNIZATIONEVALUATION: 11681 return "ImmunizationEvaluation"; 11682 case IMMUNIZATIONRECOMMENDATION: 11683 return "ImmunizationRecommendation"; 11684 case IMPLEMENTATIONGUIDE: 11685 return "ImplementationGuide"; 11686 case INSURANCEPLAN: 11687 return "InsurancePlan"; 11688 case INVOICE: 11689 return "Invoice"; 11690 case LIBRARY: 11691 return "Library"; 11692 case LINKAGE: 11693 return "Linkage"; 11694 case LIST: 11695 return "List"; 11696 case LOCATION: 11697 return "Location"; 11698 case MEASURE: 11699 return "Measure"; 11700 case MEASUREREPORT: 11701 return "MeasureReport"; 11702 case MEDIA: 11703 return "Media"; 11704 case MEDICATION: 11705 return "Medication"; 11706 case MEDICATIONADMINISTRATION: 11707 return "MedicationAdministration"; 11708 case MEDICATIONDISPENSE: 11709 return "MedicationDispense"; 11710 case MEDICATIONKNOWLEDGE: 11711 return "MedicationKnowledge"; 11712 case MEDICATIONREQUEST: 11713 return "MedicationRequest"; 11714 case MEDICATIONSTATEMENT: 11715 return "MedicationStatement"; 11716 case MEDICINALPRODUCT: 11717 return "MedicinalProduct"; 11718 case MEDICINALPRODUCTAUTHORIZATION: 11719 return "MedicinalProductAuthorization"; 11720 case MEDICINALPRODUCTCONTRAINDICATION: 11721 return "MedicinalProductContraindication"; 11722 case MEDICINALPRODUCTINDICATION: 11723 return "MedicinalProductIndication"; 11724 case MEDICINALPRODUCTINGREDIENT: 11725 return "MedicinalProductIngredient"; 11726 case MEDICINALPRODUCTINTERACTION: 11727 return "MedicinalProductInteraction"; 11728 case MEDICINALPRODUCTMANUFACTURED: 11729 return "MedicinalProductManufactured"; 11730 case MEDICINALPRODUCTPACKAGED: 11731 return "MedicinalProductPackaged"; 11732 case MEDICINALPRODUCTPHARMACEUTICAL: 11733 return "MedicinalProductPharmaceutical"; 11734 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 11735 return "MedicinalProductUndesirableEffect"; 11736 case MESSAGEDEFINITION: 11737 return "MessageDefinition"; 11738 case MESSAGEHEADER: 11739 return "MessageHeader"; 11740 case MOLECULARSEQUENCE: 11741 return "MolecularSequence"; 11742 case NAMINGSYSTEM: 11743 return "NamingSystem"; 11744 case NUTRITIONORDER: 11745 return "NutritionOrder"; 11746 case OBSERVATION: 11747 return "Observation"; 11748 case OBSERVATIONDEFINITION: 11749 return "ObservationDefinition"; 11750 case OPERATIONDEFINITION: 11751 return "OperationDefinition"; 11752 case OPERATIONOUTCOME: 11753 return "OperationOutcome"; 11754 case ORGANIZATION: 11755 return "Organization"; 11756 case ORGANIZATIONAFFILIATION: 11757 return "OrganizationAffiliation"; 11758 case PARAMETERS: 11759 return "Parameters"; 11760 case PATIENT: 11761 return "Patient"; 11762 case PAYMENTNOTICE: 11763 return "PaymentNotice"; 11764 case PAYMENTRECONCILIATION: 11765 return "PaymentReconciliation"; 11766 case PERSON: 11767 return "Person"; 11768 case PLANDEFINITION: 11769 return "PlanDefinition"; 11770 case PRACTITIONER: 11771 return "Practitioner"; 11772 case PRACTITIONERROLE: 11773 return "PractitionerRole"; 11774 case PROCEDURE: 11775 return "Procedure"; 11776 case PROVENANCE: 11777 return "Provenance"; 11778 case QUESTIONNAIRE: 11779 return "Questionnaire"; 11780 case QUESTIONNAIRERESPONSE: 11781 return "QuestionnaireResponse"; 11782 case RELATEDPERSON: 11783 return "RelatedPerson"; 11784 case REQUESTGROUP: 11785 return "RequestGroup"; 11786 case RESEARCHDEFINITION: 11787 return "ResearchDefinition"; 11788 case RESEARCHELEMENTDEFINITION: 11789 return "ResearchElementDefinition"; 11790 case RESEARCHSTUDY: 11791 return "ResearchStudy"; 11792 case RESEARCHSUBJECT: 11793 return "ResearchSubject"; 11794 case RESOURCE: 11795 return "Resource"; 11796 case RISKASSESSMENT: 11797 return "RiskAssessment"; 11798 case RISKEVIDENCESYNTHESIS: 11799 return "RiskEvidenceSynthesis"; 11800 case SCHEDULE: 11801 return "Schedule"; 11802 case SEARCHPARAMETER: 11803 return "SearchParameter"; 11804 case SERVICEREQUEST: 11805 return "ServiceRequest"; 11806 case SLOT: 11807 return "Slot"; 11808 case SPECIMEN: 11809 return "Specimen"; 11810 case SPECIMENDEFINITION: 11811 return "SpecimenDefinition"; 11812 case STRUCTUREDEFINITION: 11813 return "StructureDefinition"; 11814 case STRUCTUREMAP: 11815 return "StructureMap"; 11816 case SUBSCRIPTION: 11817 return "Subscription"; 11818 case SUBSTANCE: 11819 return "Substance"; 11820 case SUBSTANCENUCLEICACID: 11821 return "SubstanceNucleicAcid"; 11822 case SUBSTANCEPOLYMER: 11823 return "SubstancePolymer"; 11824 case SUBSTANCEPROTEIN: 11825 return "SubstanceProtein"; 11826 case SUBSTANCEREFERENCEINFORMATION: 11827 return "SubstanceReferenceInformation"; 11828 case SUBSTANCESOURCEMATERIAL: 11829 return "SubstanceSourceMaterial"; 11830 case SUBSTANCESPECIFICATION: 11831 return "SubstanceSpecification"; 11832 case SUPPLYDELIVERY: 11833 return "SupplyDelivery"; 11834 case SUPPLYREQUEST: 11835 return "SupplyRequest"; 11836 case TASK: 11837 return "Task"; 11838 case TERMINOLOGYCAPABILITIES: 11839 return "TerminologyCapabilities"; 11840 case TESTREPORT: 11841 return "TestReport"; 11842 case TESTSCRIPT: 11843 return "TestScript"; 11844 case VALUESET: 11845 return "ValueSet"; 11846 case VERIFICATIONRESULT: 11847 return "VerificationResult"; 11848 case VISIONPRESCRIPTION: 11849 return "VisionPrescription"; 11850 case NULL: 11851 return null; 11852 default: 11853 return "?"; 11854 } 11855 } 11856 } 11857 11858 public static class FHIRDefinedTypeEnumFactory implements EnumFactory<FHIRDefinedType> { 11859 public FHIRDefinedType fromCode(String codeString) throws IllegalArgumentException { 11860 if (codeString == null || "".equals(codeString)) 11861 if (codeString == null || "".equals(codeString)) 11862 return null; 11863 if ("Address".equals(codeString)) 11864 return FHIRDefinedType.ADDRESS; 11865 if ("Age".equals(codeString)) 11866 return FHIRDefinedType.AGE; 11867 if ("Annotation".equals(codeString)) 11868 return FHIRDefinedType.ANNOTATION; 11869 if ("Attachment".equals(codeString)) 11870 return FHIRDefinedType.ATTACHMENT; 11871 if ("BackboneElement".equals(codeString)) 11872 return FHIRDefinedType.BACKBONEELEMENT; 11873 if ("CodeableConcept".equals(codeString)) 11874 return FHIRDefinedType.CODEABLECONCEPT; 11875 if ("Coding".equals(codeString)) 11876 return FHIRDefinedType.CODING; 11877 if ("ContactDetail".equals(codeString)) 11878 return FHIRDefinedType.CONTACTDETAIL; 11879 if ("ContactPoint".equals(codeString)) 11880 return FHIRDefinedType.CONTACTPOINT; 11881 if ("Contributor".equals(codeString)) 11882 return FHIRDefinedType.CONTRIBUTOR; 11883 if ("Count".equals(codeString)) 11884 return FHIRDefinedType.COUNT; 11885 if ("DataRequirement".equals(codeString)) 11886 return FHIRDefinedType.DATAREQUIREMENT; 11887 if ("Distance".equals(codeString)) 11888 return FHIRDefinedType.DISTANCE; 11889 if ("Dosage".equals(codeString)) 11890 return FHIRDefinedType.DOSAGE; 11891 if ("Duration".equals(codeString)) 11892 return FHIRDefinedType.DURATION; 11893 if ("Element".equals(codeString)) 11894 return FHIRDefinedType.ELEMENT; 11895 if ("ElementDefinition".equals(codeString)) 11896 return FHIRDefinedType.ELEMENTDEFINITION; 11897 if ("Expression".equals(codeString)) 11898 return FHIRDefinedType.EXPRESSION; 11899 if ("Extension".equals(codeString)) 11900 return FHIRDefinedType.EXTENSION; 11901 if ("HumanName".equals(codeString)) 11902 return FHIRDefinedType.HUMANNAME; 11903 if ("Identifier".equals(codeString)) 11904 return FHIRDefinedType.IDENTIFIER; 11905 if ("MarketingStatus".equals(codeString)) 11906 return FHIRDefinedType.MARKETINGSTATUS; 11907 if ("Meta".equals(codeString)) 11908 return FHIRDefinedType.META; 11909 if ("Money".equals(codeString)) 11910 return FHIRDefinedType.MONEY; 11911 if ("MoneyQuantity".equals(codeString)) 11912 return FHIRDefinedType.MONEYQUANTITY; 11913 if ("Narrative".equals(codeString)) 11914 return FHIRDefinedType.NARRATIVE; 11915 if ("ParameterDefinition".equals(codeString)) 11916 return FHIRDefinedType.PARAMETERDEFINITION; 11917 if ("Period".equals(codeString)) 11918 return FHIRDefinedType.PERIOD; 11919 if ("Population".equals(codeString)) 11920 return FHIRDefinedType.POPULATION; 11921 if ("ProdCharacteristic".equals(codeString)) 11922 return FHIRDefinedType.PRODCHARACTERISTIC; 11923 if ("ProductShelfLife".equals(codeString)) 11924 return FHIRDefinedType.PRODUCTSHELFLIFE; 11925 if ("Quantity".equals(codeString)) 11926 return FHIRDefinedType.QUANTITY; 11927 if ("Range".equals(codeString)) 11928 return FHIRDefinedType.RANGE; 11929 if ("Ratio".equals(codeString)) 11930 return FHIRDefinedType.RATIO; 11931 if ("Reference".equals(codeString)) 11932 return FHIRDefinedType.REFERENCE; 11933 if ("RelatedArtifact".equals(codeString)) 11934 return FHIRDefinedType.RELATEDARTIFACT; 11935 if ("SampledData".equals(codeString)) 11936 return FHIRDefinedType.SAMPLEDDATA; 11937 if ("Signature".equals(codeString)) 11938 return FHIRDefinedType.SIGNATURE; 11939 if ("SimpleQuantity".equals(codeString)) 11940 return FHIRDefinedType.SIMPLEQUANTITY; 11941 if ("SubstanceAmount".equals(codeString)) 11942 return FHIRDefinedType.SUBSTANCEAMOUNT; 11943 if ("Timing".equals(codeString)) 11944 return FHIRDefinedType.TIMING; 11945 if ("TriggerDefinition".equals(codeString)) 11946 return FHIRDefinedType.TRIGGERDEFINITION; 11947 if ("UsageContext".equals(codeString)) 11948 return FHIRDefinedType.USAGECONTEXT; 11949 if ("base64Binary".equals(codeString)) 11950 return FHIRDefinedType.BASE64BINARY; 11951 if ("boolean".equals(codeString)) 11952 return FHIRDefinedType.BOOLEAN; 11953 if ("canonical".equals(codeString)) 11954 return FHIRDefinedType.CANONICAL; 11955 if ("code".equals(codeString)) 11956 return FHIRDefinedType.CODE; 11957 if ("date".equals(codeString)) 11958 return FHIRDefinedType.DATE; 11959 if ("dateTime".equals(codeString)) 11960 return FHIRDefinedType.DATETIME; 11961 if ("decimal".equals(codeString)) 11962 return FHIRDefinedType.DECIMAL; 11963 if ("id".equals(codeString)) 11964 return FHIRDefinedType.ID; 11965 if ("instant".equals(codeString)) 11966 return FHIRDefinedType.INSTANT; 11967 if ("integer".equals(codeString)) 11968 return FHIRDefinedType.INTEGER; 11969 if ("markdown".equals(codeString)) 11970 return FHIRDefinedType.MARKDOWN; 11971 if ("oid".equals(codeString)) 11972 return FHIRDefinedType.OID; 11973 if ("positiveInt".equals(codeString)) 11974 return FHIRDefinedType.POSITIVEINT; 11975 if ("string".equals(codeString)) 11976 return FHIRDefinedType.STRING; 11977 if ("time".equals(codeString)) 11978 return FHIRDefinedType.TIME; 11979 if ("unsignedInt".equals(codeString)) 11980 return FHIRDefinedType.UNSIGNEDINT; 11981 if ("uri".equals(codeString)) 11982 return FHIRDefinedType.URI; 11983 if ("url".equals(codeString)) 11984 return FHIRDefinedType.URL; 11985 if ("uuid".equals(codeString)) 11986 return FHIRDefinedType.UUID; 11987 if ("xhtml".equals(codeString)) 11988 return FHIRDefinedType.XHTML; 11989 if ("Account".equals(codeString)) 11990 return FHIRDefinedType.ACCOUNT; 11991 if ("ActivityDefinition".equals(codeString)) 11992 return FHIRDefinedType.ACTIVITYDEFINITION; 11993 if ("AdverseEvent".equals(codeString)) 11994 return FHIRDefinedType.ADVERSEEVENT; 11995 if ("AllergyIntolerance".equals(codeString)) 11996 return FHIRDefinedType.ALLERGYINTOLERANCE; 11997 if ("Appointment".equals(codeString)) 11998 return FHIRDefinedType.APPOINTMENT; 11999 if ("AppointmentResponse".equals(codeString)) 12000 return FHIRDefinedType.APPOINTMENTRESPONSE; 12001 if ("AuditEvent".equals(codeString)) 12002 return FHIRDefinedType.AUDITEVENT; 12003 if ("Basic".equals(codeString)) 12004 return FHIRDefinedType.BASIC; 12005 if ("Binary".equals(codeString)) 12006 return FHIRDefinedType.BINARY; 12007 if ("BiologicallyDerivedProduct".equals(codeString)) 12008 return FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT; 12009 if ("BodyStructure".equals(codeString)) 12010 return FHIRDefinedType.BODYSTRUCTURE; 12011 if ("Bundle".equals(codeString)) 12012 return FHIRDefinedType.BUNDLE; 12013 if ("CapabilityStatement".equals(codeString)) 12014 return FHIRDefinedType.CAPABILITYSTATEMENT; 12015 if ("CarePlan".equals(codeString)) 12016 return FHIRDefinedType.CAREPLAN; 12017 if ("CareTeam".equals(codeString)) 12018 return FHIRDefinedType.CARETEAM; 12019 if ("CatalogEntry".equals(codeString)) 12020 return FHIRDefinedType.CATALOGENTRY; 12021 if ("ChargeItem".equals(codeString)) 12022 return FHIRDefinedType.CHARGEITEM; 12023 if ("ChargeItemDefinition".equals(codeString)) 12024 return FHIRDefinedType.CHARGEITEMDEFINITION; 12025 if ("Claim".equals(codeString)) 12026 return FHIRDefinedType.CLAIM; 12027 if ("ClaimResponse".equals(codeString)) 12028 return FHIRDefinedType.CLAIMRESPONSE; 12029 if ("ClinicalImpression".equals(codeString)) 12030 return FHIRDefinedType.CLINICALIMPRESSION; 12031 if ("CodeSystem".equals(codeString)) 12032 return FHIRDefinedType.CODESYSTEM; 12033 if ("Communication".equals(codeString)) 12034 return FHIRDefinedType.COMMUNICATION; 12035 if ("CommunicationRequest".equals(codeString)) 12036 return FHIRDefinedType.COMMUNICATIONREQUEST; 12037 if ("CompartmentDefinition".equals(codeString)) 12038 return FHIRDefinedType.COMPARTMENTDEFINITION; 12039 if ("Composition".equals(codeString)) 12040 return FHIRDefinedType.COMPOSITION; 12041 if ("ConceptMap".equals(codeString)) 12042 return FHIRDefinedType.CONCEPTMAP; 12043 if ("Condition".equals(codeString)) 12044 return FHIRDefinedType.CONDITION; 12045 if ("Consent".equals(codeString)) 12046 return FHIRDefinedType.CONSENT; 12047 if ("Contract".equals(codeString)) 12048 return FHIRDefinedType.CONTRACT; 12049 if ("Coverage".equals(codeString)) 12050 return FHIRDefinedType.COVERAGE; 12051 if ("CoverageEligibilityRequest".equals(codeString)) 12052 return FHIRDefinedType.COVERAGEELIGIBILITYREQUEST; 12053 if ("CoverageEligibilityResponse".equals(codeString)) 12054 return FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE; 12055 if ("DetectedIssue".equals(codeString)) 12056 return FHIRDefinedType.DETECTEDISSUE; 12057 if ("Device".equals(codeString)) 12058 return FHIRDefinedType.DEVICE; 12059 if ("DeviceDefinition".equals(codeString)) 12060 return FHIRDefinedType.DEVICEDEFINITION; 12061 if ("DeviceMetric".equals(codeString)) 12062 return FHIRDefinedType.DEVICEMETRIC; 12063 if ("DeviceRequest".equals(codeString)) 12064 return FHIRDefinedType.DEVICEREQUEST; 12065 if ("DeviceUseStatement".equals(codeString)) 12066 return FHIRDefinedType.DEVICEUSESTATEMENT; 12067 if ("DiagnosticReport".equals(codeString)) 12068 return FHIRDefinedType.DIAGNOSTICREPORT; 12069 if ("DocumentManifest".equals(codeString)) 12070 return FHIRDefinedType.DOCUMENTMANIFEST; 12071 if ("DocumentReference".equals(codeString)) 12072 return FHIRDefinedType.DOCUMENTREFERENCE; 12073 if ("DomainResource".equals(codeString)) 12074 return FHIRDefinedType.DOMAINRESOURCE; 12075 if ("EffectEvidenceSynthesis".equals(codeString)) 12076 return FHIRDefinedType.EFFECTEVIDENCESYNTHESIS; 12077 if ("Encounter".equals(codeString)) 12078 return FHIRDefinedType.ENCOUNTER; 12079 if ("Endpoint".equals(codeString)) 12080 return FHIRDefinedType.ENDPOINT; 12081 if ("EnrollmentRequest".equals(codeString)) 12082 return FHIRDefinedType.ENROLLMENTREQUEST; 12083 if ("EnrollmentResponse".equals(codeString)) 12084 return FHIRDefinedType.ENROLLMENTRESPONSE; 12085 if ("EpisodeOfCare".equals(codeString)) 12086 return FHIRDefinedType.EPISODEOFCARE; 12087 if ("EventDefinition".equals(codeString)) 12088 return FHIRDefinedType.EVENTDEFINITION; 12089 if ("Evidence".equals(codeString)) 12090 return FHIRDefinedType.EVIDENCE; 12091 if ("EvidenceVariable".equals(codeString)) 12092 return FHIRDefinedType.EVIDENCEVARIABLE; 12093 if ("ExampleScenario".equals(codeString)) 12094 return FHIRDefinedType.EXAMPLESCENARIO; 12095 if ("ExplanationOfBenefit".equals(codeString)) 12096 return FHIRDefinedType.EXPLANATIONOFBENEFIT; 12097 if ("FamilyMemberHistory".equals(codeString)) 12098 return FHIRDefinedType.FAMILYMEMBERHISTORY; 12099 if ("Flag".equals(codeString)) 12100 return FHIRDefinedType.FLAG; 12101 if ("Goal".equals(codeString)) 12102 return FHIRDefinedType.GOAL; 12103 if ("GraphDefinition".equals(codeString)) 12104 return FHIRDefinedType.GRAPHDEFINITION; 12105 if ("Group".equals(codeString)) 12106 return FHIRDefinedType.GROUP; 12107 if ("GuidanceResponse".equals(codeString)) 12108 return FHIRDefinedType.GUIDANCERESPONSE; 12109 if ("HealthcareService".equals(codeString)) 12110 return FHIRDefinedType.HEALTHCARESERVICE; 12111 if ("ImagingStudy".equals(codeString)) 12112 return FHIRDefinedType.IMAGINGSTUDY; 12113 if ("Immunization".equals(codeString)) 12114 return FHIRDefinedType.IMMUNIZATION; 12115 if ("ImmunizationEvaluation".equals(codeString)) 12116 return FHIRDefinedType.IMMUNIZATIONEVALUATION; 12117 if ("ImmunizationRecommendation".equals(codeString)) 12118 return FHIRDefinedType.IMMUNIZATIONRECOMMENDATION; 12119 if ("ImplementationGuide".equals(codeString)) 12120 return FHIRDefinedType.IMPLEMENTATIONGUIDE; 12121 if ("InsurancePlan".equals(codeString)) 12122 return FHIRDefinedType.INSURANCEPLAN; 12123 if ("Invoice".equals(codeString)) 12124 return FHIRDefinedType.INVOICE; 12125 if ("Library".equals(codeString)) 12126 return FHIRDefinedType.LIBRARY; 12127 if ("Linkage".equals(codeString)) 12128 return FHIRDefinedType.LINKAGE; 12129 if ("List".equals(codeString)) 12130 return FHIRDefinedType.LIST; 12131 if ("Location".equals(codeString)) 12132 return FHIRDefinedType.LOCATION; 12133 if ("Measure".equals(codeString)) 12134 return FHIRDefinedType.MEASURE; 12135 if ("MeasureReport".equals(codeString)) 12136 return FHIRDefinedType.MEASUREREPORT; 12137 if ("Media".equals(codeString)) 12138 return FHIRDefinedType.MEDIA; 12139 if ("Medication".equals(codeString)) 12140 return FHIRDefinedType.MEDICATION; 12141 if ("MedicationAdministration".equals(codeString)) 12142 return FHIRDefinedType.MEDICATIONADMINISTRATION; 12143 if ("MedicationDispense".equals(codeString)) 12144 return FHIRDefinedType.MEDICATIONDISPENSE; 12145 if ("MedicationKnowledge".equals(codeString)) 12146 return FHIRDefinedType.MEDICATIONKNOWLEDGE; 12147 if ("MedicationRequest".equals(codeString)) 12148 return FHIRDefinedType.MEDICATIONREQUEST; 12149 if ("MedicationStatement".equals(codeString)) 12150 return FHIRDefinedType.MEDICATIONSTATEMENT; 12151 if ("MedicinalProduct".equals(codeString)) 12152 return FHIRDefinedType.MEDICINALPRODUCT; 12153 if ("MedicinalProductAuthorization".equals(codeString)) 12154 return FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION; 12155 if ("MedicinalProductContraindication".equals(codeString)) 12156 return FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION; 12157 if ("MedicinalProductIndication".equals(codeString)) 12158 return FHIRDefinedType.MEDICINALPRODUCTINDICATION; 12159 if ("MedicinalProductIngredient".equals(codeString)) 12160 return FHIRDefinedType.MEDICINALPRODUCTINGREDIENT; 12161 if ("MedicinalProductInteraction".equals(codeString)) 12162 return FHIRDefinedType.MEDICINALPRODUCTINTERACTION; 12163 if ("MedicinalProductManufactured".equals(codeString)) 12164 return FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED; 12165 if ("MedicinalProductPackaged".equals(codeString)) 12166 return FHIRDefinedType.MEDICINALPRODUCTPACKAGED; 12167 if ("MedicinalProductPharmaceutical".equals(codeString)) 12168 return FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL; 12169 if ("MedicinalProductUndesirableEffect".equals(codeString)) 12170 return FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 12171 if ("MessageDefinition".equals(codeString)) 12172 return FHIRDefinedType.MESSAGEDEFINITION; 12173 if ("MessageHeader".equals(codeString)) 12174 return FHIRDefinedType.MESSAGEHEADER; 12175 if ("MolecularSequence".equals(codeString)) 12176 return FHIRDefinedType.MOLECULARSEQUENCE; 12177 if ("NamingSystem".equals(codeString)) 12178 return FHIRDefinedType.NAMINGSYSTEM; 12179 if ("NutritionOrder".equals(codeString)) 12180 return FHIRDefinedType.NUTRITIONORDER; 12181 if ("Observation".equals(codeString)) 12182 return FHIRDefinedType.OBSERVATION; 12183 if ("ObservationDefinition".equals(codeString)) 12184 return FHIRDefinedType.OBSERVATIONDEFINITION; 12185 if ("OperationDefinition".equals(codeString)) 12186 return FHIRDefinedType.OPERATIONDEFINITION; 12187 if ("OperationOutcome".equals(codeString)) 12188 return FHIRDefinedType.OPERATIONOUTCOME; 12189 if ("Organization".equals(codeString)) 12190 return FHIRDefinedType.ORGANIZATION; 12191 if ("OrganizationAffiliation".equals(codeString)) 12192 return FHIRDefinedType.ORGANIZATIONAFFILIATION; 12193 if ("Parameters".equals(codeString)) 12194 return FHIRDefinedType.PARAMETERS; 12195 if ("Patient".equals(codeString)) 12196 return FHIRDefinedType.PATIENT; 12197 if ("PaymentNotice".equals(codeString)) 12198 return FHIRDefinedType.PAYMENTNOTICE; 12199 if ("PaymentReconciliation".equals(codeString)) 12200 return FHIRDefinedType.PAYMENTRECONCILIATION; 12201 if ("Person".equals(codeString)) 12202 return FHIRDefinedType.PERSON; 12203 if ("PlanDefinition".equals(codeString)) 12204 return FHIRDefinedType.PLANDEFINITION; 12205 if ("Practitioner".equals(codeString)) 12206 return FHIRDefinedType.PRACTITIONER; 12207 if ("PractitionerRole".equals(codeString)) 12208 return FHIRDefinedType.PRACTITIONERROLE; 12209 if ("Procedure".equals(codeString)) 12210 return FHIRDefinedType.PROCEDURE; 12211 if ("Provenance".equals(codeString)) 12212 return FHIRDefinedType.PROVENANCE; 12213 if ("Questionnaire".equals(codeString)) 12214 return FHIRDefinedType.QUESTIONNAIRE; 12215 if ("QuestionnaireResponse".equals(codeString)) 12216 return FHIRDefinedType.QUESTIONNAIRERESPONSE; 12217 if ("RelatedPerson".equals(codeString)) 12218 return FHIRDefinedType.RELATEDPERSON; 12219 if ("RequestGroup".equals(codeString)) 12220 return FHIRDefinedType.REQUESTGROUP; 12221 if ("ResearchDefinition".equals(codeString)) 12222 return FHIRDefinedType.RESEARCHDEFINITION; 12223 if ("ResearchElementDefinition".equals(codeString)) 12224 return FHIRDefinedType.RESEARCHELEMENTDEFINITION; 12225 if ("ResearchStudy".equals(codeString)) 12226 return FHIRDefinedType.RESEARCHSTUDY; 12227 if ("ResearchSubject".equals(codeString)) 12228 return FHIRDefinedType.RESEARCHSUBJECT; 12229 if ("Resource".equals(codeString)) 12230 return FHIRDefinedType.RESOURCE; 12231 if ("RiskAssessment".equals(codeString)) 12232 return FHIRDefinedType.RISKASSESSMENT; 12233 if ("RiskEvidenceSynthesis".equals(codeString)) 12234 return FHIRDefinedType.RISKEVIDENCESYNTHESIS; 12235 if ("Schedule".equals(codeString)) 12236 return FHIRDefinedType.SCHEDULE; 12237 if ("SearchParameter".equals(codeString)) 12238 return FHIRDefinedType.SEARCHPARAMETER; 12239 if ("ServiceRequest".equals(codeString)) 12240 return FHIRDefinedType.SERVICEREQUEST; 12241 if ("Slot".equals(codeString)) 12242 return FHIRDefinedType.SLOT; 12243 if ("Specimen".equals(codeString)) 12244 return FHIRDefinedType.SPECIMEN; 12245 if ("SpecimenDefinition".equals(codeString)) 12246 return FHIRDefinedType.SPECIMENDEFINITION; 12247 if ("StructureDefinition".equals(codeString)) 12248 return FHIRDefinedType.STRUCTUREDEFINITION; 12249 if ("StructureMap".equals(codeString)) 12250 return FHIRDefinedType.STRUCTUREMAP; 12251 if ("Subscription".equals(codeString)) 12252 return FHIRDefinedType.SUBSCRIPTION; 12253 if ("Substance".equals(codeString)) 12254 return FHIRDefinedType.SUBSTANCE; 12255 if ("SubstanceNucleicAcid".equals(codeString)) 12256 return FHIRDefinedType.SUBSTANCENUCLEICACID; 12257 if ("SubstancePolymer".equals(codeString)) 12258 return FHIRDefinedType.SUBSTANCEPOLYMER; 12259 if ("SubstanceProtein".equals(codeString)) 12260 return FHIRDefinedType.SUBSTANCEPROTEIN; 12261 if ("SubstanceReferenceInformation".equals(codeString)) 12262 return FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION; 12263 if ("SubstanceSourceMaterial".equals(codeString)) 12264 return FHIRDefinedType.SUBSTANCESOURCEMATERIAL; 12265 if ("SubstanceSpecification".equals(codeString)) 12266 return FHIRDefinedType.SUBSTANCESPECIFICATION; 12267 if ("SupplyDelivery".equals(codeString)) 12268 return FHIRDefinedType.SUPPLYDELIVERY; 12269 if ("SupplyRequest".equals(codeString)) 12270 return FHIRDefinedType.SUPPLYREQUEST; 12271 if ("Task".equals(codeString)) 12272 return FHIRDefinedType.TASK; 12273 if ("TerminologyCapabilities".equals(codeString)) 12274 return FHIRDefinedType.TERMINOLOGYCAPABILITIES; 12275 if ("TestReport".equals(codeString)) 12276 return FHIRDefinedType.TESTREPORT; 12277 if ("TestScript".equals(codeString)) 12278 return FHIRDefinedType.TESTSCRIPT; 12279 if ("ValueSet".equals(codeString)) 12280 return FHIRDefinedType.VALUESET; 12281 if ("VerificationResult".equals(codeString)) 12282 return FHIRDefinedType.VERIFICATIONRESULT; 12283 if ("VisionPrescription".equals(codeString)) 12284 return FHIRDefinedType.VISIONPRESCRIPTION; 12285 throw new IllegalArgumentException("Unknown FHIRDefinedType code '" + codeString + "'"); 12286 } 12287 12288 public Enumeration<FHIRDefinedType> fromType(PrimitiveType<?> code) throws FHIRException { 12289 if (code == null) 12290 return null; 12291 if (code.isEmpty()) 12292 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NULL, code); 12293 String codeString = code.asStringValue(); 12294 if (codeString == null || "".equals(codeString)) 12295 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NULL, code); 12296 if ("Address".equals(codeString)) 12297 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADDRESS, code); 12298 if ("Age".equals(codeString)) 12299 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AGE, code); 12300 if ("Annotation".equals(codeString)) 12301 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ANNOTATION, code); 12302 if ("Attachment".equals(codeString)) 12303 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ATTACHMENT, code); 12304 if ("BackboneElement".equals(codeString)) 12305 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BACKBONEELEMENT, code); 12306 if ("CodeableConcept".equals(codeString)) 12307 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODEABLECONCEPT, code); 12308 if ("Coding".equals(codeString)) 12309 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODING, code); 12310 if ("ContactDetail".equals(codeString)) 12311 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTDETAIL, code); 12312 if ("ContactPoint".equals(codeString)) 12313 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTPOINT, code); 12314 if ("Contributor".equals(codeString)) 12315 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRIBUTOR, code); 12316 if ("Count".equals(codeString)) 12317 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COUNT, code); 12318 if ("DataRequirement".equals(codeString)) 12319 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAREQUIREMENT, code); 12320 if ("Distance".equals(codeString)) 12321 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DISTANCE, code); 12322 if ("Dosage".equals(codeString)) 12323 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOSAGE, code); 12324 if ("Duration".equals(codeString)) 12325 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DURATION, code); 12326 if ("Element".equals(codeString)) 12327 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENT, code); 12328 if ("ElementDefinition".equals(codeString)) 12329 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENTDEFINITION, code); 12330 if ("Expression".equals(codeString)) 12331 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPRESSION, code); 12332 if ("Extension".equals(codeString)) 12333 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXTENSION, code); 12334 if ("HumanName".equals(codeString)) 12335 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HUMANNAME, code); 12336 if ("Identifier".equals(codeString)) 12337 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IDENTIFIER, code); 12338 if ("MarketingStatus".equals(codeString)) 12339 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKETINGSTATUS, code); 12340 if ("Meta".equals(codeString)) 12341 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.META, code); 12342 if ("Money".equals(codeString)) 12343 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEY, code); 12344 if ("MoneyQuantity".equals(codeString)) 12345 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEYQUANTITY, code); 12346 if ("Narrative".equals(codeString)) 12347 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NARRATIVE, code); 12348 if ("ParameterDefinition".equals(codeString)) 12349 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERDEFINITION, code); 12350 if ("Period".equals(codeString)) 12351 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERIOD, code); 12352 if ("Population".equals(codeString)) 12353 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POPULATION, code); 12354 if ("ProdCharacteristic".equals(codeString)) 12355 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRODCHARACTERISTIC, code); 12356 if ("ProductShelfLife".equals(codeString)) 12357 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRODUCTSHELFLIFE, code); 12358 if ("Quantity".equals(codeString)) 12359 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUANTITY, code); 12360 if ("Range".equals(codeString)) 12361 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RANGE, code); 12362 if ("Ratio".equals(codeString)) 12363 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RATIO, code); 12364 if ("Reference".equals(codeString)) 12365 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERENCE, code); 12366 if ("RelatedArtifact".equals(codeString)) 12367 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDARTIFACT, code); 12368 if ("SampledData".equals(codeString)) 12369 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SAMPLEDDATA, code); 12370 if ("Signature".equals(codeString)) 12371 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIGNATURE, code); 12372 if ("SimpleQuantity".equals(codeString)) 12373 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIMPLEQUANTITY, code); 12374 if ("SubstanceAmount".equals(codeString)) 12375 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEAMOUNT, code); 12376 if ("Timing".equals(codeString)) 12377 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIMING, code); 12378 if ("TriggerDefinition".equals(codeString)) 12379 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TRIGGERDEFINITION, code); 12380 if ("UsageContext".equals(codeString)) 12381 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.USAGECONTEXT, code); 12382 if ("base64Binary".equals(codeString)) 12383 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASE64BINARY, code); 12384 if ("boolean".equals(codeString)) 12385 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BOOLEAN, code); 12386 if ("canonical".equals(codeString)) 12387 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CANONICAL, code); 12388 if ("code".equals(codeString)) 12389 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODE, code); 12390 if ("date".equals(codeString)) 12391 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATE, code); 12392 if ("dateTime".equals(codeString)) 12393 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATETIME, code); 12394 if ("decimal".equals(codeString)) 12395 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DECIMAL, code); 12396 if ("id".equals(codeString)) 12397 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ID, code); 12398 if ("instant".equals(codeString)) 12399 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSTANT, code); 12400 if ("integer".equals(codeString)) 12401 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INTEGER, code); 12402 if ("markdown".equals(codeString)) 12403 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKDOWN, code); 12404 if ("oid".equals(codeString)) 12405 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OID, code); 12406 if ("positiveInt".equals(codeString)) 12407 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POSITIVEINT, code); 12408 if ("string".equals(codeString)) 12409 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRING, code); 12410 if ("time".equals(codeString)) 12411 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIME, code); 12412 if ("unsignedInt".equals(codeString)) 12413 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UNSIGNEDINT, code); 12414 if ("uri".equals(codeString)) 12415 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URI, code); 12416 if ("url".equals(codeString)) 12417 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URL, code); 12418 if ("uuid".equals(codeString)) 12419 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UUID, code); 12420 if ("xhtml".equals(codeString)) 12421 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.XHTML, code); 12422 if ("Account".equals(codeString)) 12423 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACCOUNT, code); 12424 if ("ActivityDefinition".equals(codeString)) 12425 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACTIVITYDEFINITION, code); 12426 if ("AdverseEvent".equals(codeString)) 12427 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADVERSEEVENT, code); 12428 if ("AllergyIntolerance".equals(codeString)) 12429 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ALLERGYINTOLERANCE, code); 12430 if ("Appointment".equals(codeString)) 12431 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENT, code); 12432 if ("AppointmentResponse".equals(codeString)) 12433 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENTRESPONSE, code); 12434 if ("AuditEvent".equals(codeString)) 12435 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AUDITEVENT, code); 12436 if ("Basic".equals(codeString)) 12437 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASIC, code); 12438 if ("Binary".equals(codeString)) 12439 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BINARY, code); 12440 if ("BiologicallyDerivedProduct".equals(codeString)) 12441 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT, code); 12442 if ("BodyStructure".equals(codeString)) 12443 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BODYSTRUCTURE, code); 12444 if ("Bundle".equals(codeString)) 12445 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BUNDLE, code); 12446 if ("CapabilityStatement".equals(codeString)) 12447 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAPABILITYSTATEMENT, code); 12448 if ("CarePlan".equals(codeString)) 12449 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAREPLAN, code); 12450 if ("CareTeam".equals(codeString)) 12451 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CARETEAM, code); 12452 if ("CatalogEntry".equals(codeString)) 12453 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CATALOGENTRY, code); 12454 if ("ChargeItem".equals(codeString)) 12455 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEM, code); 12456 if ("ChargeItemDefinition".equals(codeString)) 12457 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEMDEFINITION, code); 12458 if ("Claim".equals(codeString)) 12459 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIM, code); 12460 if ("ClaimResponse".equals(codeString)) 12461 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIMRESPONSE, code); 12462 if ("ClinicalImpression".equals(codeString)) 12463 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLINICALIMPRESSION, code); 12464 if ("CodeSystem".equals(codeString)) 12465 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODESYSTEM, code); 12466 if ("Communication".equals(codeString)) 12467 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATION, code); 12468 if ("CommunicationRequest".equals(codeString)) 12469 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATIONREQUEST, code); 12470 if ("CompartmentDefinition".equals(codeString)) 12471 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPARTMENTDEFINITION, code); 12472 if ("Composition".equals(codeString)) 12473 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPOSITION, code); 12474 if ("ConceptMap".equals(codeString)) 12475 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONCEPTMAP, code); 12476 if ("Condition".equals(codeString)) 12477 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONDITION, code); 12478 if ("Consent".equals(codeString)) 12479 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONSENT, code); 12480 if ("Contract".equals(codeString)) 12481 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRACT, code); 12482 if ("Coverage".equals(codeString)) 12483 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGE, code); 12484 if ("CoverageEligibilityRequest".equals(codeString)) 12485 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGEELIGIBILITYREQUEST, code); 12486 if ("CoverageEligibilityResponse".equals(codeString)) 12487 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE, code); 12488 if ("DetectedIssue".equals(codeString)) 12489 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DETECTEDISSUE, code); 12490 if ("Device".equals(codeString)) 12491 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICE, code); 12492 if ("DeviceDefinition".equals(codeString)) 12493 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEDEFINITION, code); 12494 if ("DeviceMetric".equals(codeString)) 12495 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEMETRIC, code); 12496 if ("DeviceRequest".equals(codeString)) 12497 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEREQUEST, code); 12498 if ("DeviceUseStatement".equals(codeString)) 12499 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSESTATEMENT, code); 12500 if ("DiagnosticReport".equals(codeString)) 12501 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICREPORT, code); 12502 if ("DocumentManifest".equals(codeString)) 12503 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTMANIFEST, code); 12504 if ("DocumentReference".equals(codeString)) 12505 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTREFERENCE, code); 12506 if ("DomainResource".equals(codeString)) 12507 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOMAINRESOURCE, code); 12508 if ("EffectEvidenceSynthesis".equals(codeString)) 12509 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EFFECTEVIDENCESYNTHESIS, code); 12510 if ("Encounter".equals(codeString)) 12511 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENCOUNTER, code); 12512 if ("Endpoint".equals(codeString)) 12513 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENDPOINT, code); 12514 if ("EnrollmentRequest".equals(codeString)) 12515 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTREQUEST, code); 12516 if ("EnrollmentResponse".equals(codeString)) 12517 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTRESPONSE, code); 12518 if ("EpisodeOfCare".equals(codeString)) 12519 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EPISODEOFCARE, code); 12520 if ("EventDefinition".equals(codeString)) 12521 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVENTDEFINITION, code); 12522 if ("Evidence".equals(codeString)) 12523 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVIDENCE, code); 12524 if ("EvidenceVariable".equals(codeString)) 12525 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EVIDENCEVARIABLE, code); 12526 if ("ExampleScenario".equals(codeString)) 12527 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXAMPLESCENARIO, code); 12528 if ("ExplanationOfBenefit".equals(codeString)) 12529 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPLANATIONOFBENEFIT, code); 12530 if ("FamilyMemberHistory".equals(codeString)) 12531 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FAMILYMEMBERHISTORY, code); 12532 if ("Flag".equals(codeString)) 12533 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FLAG, code); 12534 if ("Goal".equals(codeString)) 12535 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GOAL, code); 12536 if ("GraphDefinition".equals(codeString)) 12537 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GRAPHDEFINITION, code); 12538 if ("Group".equals(codeString)) 12539 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GROUP, code); 12540 if ("GuidanceResponse".equals(codeString)) 12541 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GUIDANCERESPONSE, code); 12542 if ("HealthcareService".equals(codeString)) 12543 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HEALTHCARESERVICE, code); 12544 if ("ImagingStudy".equals(codeString)) 12545 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGSTUDY, code); 12546 if ("Immunization".equals(codeString)) 12547 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATION, code); 12548 if ("ImmunizationEvaluation".equals(codeString)) 12549 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONEVALUATION, code); 12550 if ("ImmunizationRecommendation".equals(codeString)) 12551 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONRECOMMENDATION, code); 12552 if ("ImplementationGuide".equals(codeString)) 12553 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMPLEMENTATIONGUIDE, code); 12554 if ("InsurancePlan".equals(codeString)) 12555 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSURANCEPLAN, code); 12556 if ("Invoice".equals(codeString)) 12557 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INVOICE, code); 12558 if ("Library".equals(codeString)) 12559 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIBRARY, code); 12560 if ("Linkage".equals(codeString)) 12561 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LINKAGE, code); 12562 if ("List".equals(codeString)) 12563 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIST, code); 12564 if ("Location".equals(codeString)) 12565 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LOCATION, code); 12566 if ("Measure".equals(codeString)) 12567 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASURE, code); 12568 if ("MeasureReport".equals(codeString)) 12569 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASUREREPORT, code); 12570 if ("Media".equals(codeString)) 12571 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDIA, code); 12572 if ("Medication".equals(codeString)) 12573 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATION, code); 12574 if ("MedicationAdministration".equals(codeString)) 12575 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONADMINISTRATION, code); 12576 if ("MedicationDispense".equals(codeString)) 12577 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONDISPENSE, code); 12578 if ("MedicationKnowledge".equals(codeString)) 12579 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONKNOWLEDGE, code); 12580 if ("MedicationRequest".equals(codeString)) 12581 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONREQUEST, code); 12582 if ("MedicationStatement".equals(codeString)) 12583 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONSTATEMENT, code); 12584 if ("MedicinalProduct".equals(codeString)) 12585 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCT, code); 12586 if ("MedicinalProductAuthorization".equals(codeString)) 12587 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION, code); 12588 if ("MedicinalProductContraindication".equals(codeString)) 12589 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION, code); 12590 if ("MedicinalProductIndication".equals(codeString)) 12591 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINDICATION, code); 12592 if ("MedicinalProductIngredient".equals(codeString)) 12593 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINGREDIENT, code); 12594 if ("MedicinalProductInteraction".equals(codeString)) 12595 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTINTERACTION, code); 12596 if ("MedicinalProductManufactured".equals(codeString)) 12597 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED, code); 12598 if ("MedicinalProductPackaged".equals(codeString)) 12599 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTPACKAGED, code); 12600 if ("MedicinalProductPharmaceutical".equals(codeString)) 12601 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL, code); 12602 if ("MedicinalProductUndesirableEffect".equals(codeString)) 12603 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 12604 if ("MessageDefinition".equals(codeString)) 12605 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEDEFINITION, code); 12606 if ("MessageHeader".equals(codeString)) 12607 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEHEADER, code); 12608 if ("MolecularSequence".equals(codeString)) 12609 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MOLECULARSEQUENCE, code); 12610 if ("NamingSystem".equals(codeString)) 12611 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NAMINGSYSTEM, code); 12612 if ("NutritionOrder".equals(codeString)) 12613 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NUTRITIONORDER, code); 12614 if ("Observation".equals(codeString)) 12615 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATION, code); 12616 if ("ObservationDefinition".equals(codeString)) 12617 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATIONDEFINITION, code); 12618 if ("OperationDefinition".equals(codeString)) 12619 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONDEFINITION, code); 12620 if ("OperationOutcome".equals(codeString)) 12621 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONOUTCOME, code); 12622 if ("Organization".equals(codeString)) 12623 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATION, code); 12624 if ("OrganizationAffiliation".equals(codeString)) 12625 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATIONAFFILIATION, code); 12626 if ("Parameters".equals(codeString)) 12627 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERS, code); 12628 if ("Patient".equals(codeString)) 12629 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PATIENT, code); 12630 if ("PaymentNotice".equals(codeString)) 12631 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTNOTICE, code); 12632 if ("PaymentReconciliation".equals(codeString)) 12633 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTRECONCILIATION, code); 12634 if ("Person".equals(codeString)) 12635 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERSON, code); 12636 if ("PlanDefinition".equals(codeString)) 12637 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PLANDEFINITION, code); 12638 if ("Practitioner".equals(codeString)) 12639 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONER, code); 12640 if ("PractitionerRole".equals(codeString)) 12641 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONERROLE, code); 12642 if ("Procedure".equals(codeString)) 12643 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDURE, code); 12644 if ("Provenance".equals(codeString)) 12645 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROVENANCE, code); 12646 if ("Questionnaire".equals(codeString)) 12647 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRE, code); 12648 if ("QuestionnaireResponse".equals(codeString)) 12649 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRERESPONSE, code); 12650 if ("RelatedPerson".equals(codeString)) 12651 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDPERSON, code); 12652 if ("RequestGroup".equals(codeString)) 12653 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REQUESTGROUP, code); 12654 if ("ResearchDefinition".equals(codeString)) 12655 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHDEFINITION, code); 12656 if ("ResearchElementDefinition".equals(codeString)) 12657 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHELEMENTDEFINITION, code); 12658 if ("ResearchStudy".equals(codeString)) 12659 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSTUDY, code); 12660 if ("ResearchSubject".equals(codeString)) 12661 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSUBJECT, code); 12662 if ("Resource".equals(codeString)) 12663 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESOURCE, code); 12664 if ("RiskAssessment".equals(codeString)) 12665 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKASSESSMENT, code); 12666 if ("RiskEvidenceSynthesis".equals(codeString)) 12667 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKEVIDENCESYNTHESIS, code); 12668 if ("Schedule".equals(codeString)) 12669 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SCHEDULE, code); 12670 if ("SearchParameter".equals(codeString)) 12671 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEARCHPARAMETER, code); 12672 if ("ServiceRequest".equals(codeString)) 12673 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SERVICEREQUEST, code); 12674 if ("Slot".equals(codeString)) 12675 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SLOT, code); 12676 if ("Specimen".equals(codeString)) 12677 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMEN, code); 12678 if ("SpecimenDefinition".equals(codeString)) 12679 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMENDEFINITION, code); 12680 if ("StructureDefinition".equals(codeString)) 12681 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREDEFINITION, code); 12682 if ("StructureMap".equals(codeString)) 12683 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREMAP, code); 12684 if ("Subscription".equals(codeString)) 12685 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSCRIPTION, code); 12686 if ("Substance".equals(codeString)) 12687 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCE, code); 12688 if ("SubstanceNucleicAcid".equals(codeString)) 12689 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCENUCLEICACID, code); 12690 if ("SubstancePolymer".equals(codeString)) 12691 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEPOLYMER, code); 12692 if ("SubstanceProtein".equals(codeString)) 12693 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEPROTEIN, code); 12694 if ("SubstanceReferenceInformation".equals(codeString)) 12695 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION, code); 12696 if ("SubstanceSourceMaterial".equals(codeString)) 12697 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCESOURCEMATERIAL, code); 12698 if ("SubstanceSpecification".equals(codeString)) 12699 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCESPECIFICATION, code); 12700 if ("SupplyDelivery".equals(codeString)) 12701 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYDELIVERY, code); 12702 if ("SupplyRequest".equals(codeString)) 12703 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYREQUEST, code); 12704 if ("Task".equals(codeString)) 12705 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TASK, code); 12706 if ("TerminologyCapabilities".equals(codeString)) 12707 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TERMINOLOGYCAPABILITIES, code); 12708 if ("TestReport".equals(codeString)) 12709 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTREPORT, code); 12710 if ("TestScript".equals(codeString)) 12711 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTSCRIPT, code); 12712 if ("ValueSet".equals(codeString)) 12713 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VALUESET, code); 12714 if ("VerificationResult".equals(codeString)) 12715 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VERIFICATIONRESULT, code); 12716 if ("VisionPrescription".equals(codeString)) 12717 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VISIONPRESCRIPTION, code); 12718 throw new FHIRException("Unknown FHIRDefinedType code '" + codeString + "'"); 12719 } 12720 12721 public String toCode(FHIRDefinedType code) { 12722 if (code == FHIRDefinedType.ADDRESS) 12723 return "Address"; 12724 if (code == FHIRDefinedType.AGE) 12725 return "Age"; 12726 if (code == FHIRDefinedType.ANNOTATION) 12727 return "Annotation"; 12728 if (code == FHIRDefinedType.ATTACHMENT) 12729 return "Attachment"; 12730 if (code == FHIRDefinedType.BACKBONEELEMENT) 12731 return "BackboneElement"; 12732 if (code == FHIRDefinedType.CODEABLECONCEPT) 12733 return "CodeableConcept"; 12734 if (code == FHIRDefinedType.CODING) 12735 return "Coding"; 12736 if (code == FHIRDefinedType.CONTACTDETAIL) 12737 return "ContactDetail"; 12738 if (code == FHIRDefinedType.CONTACTPOINT) 12739 return "ContactPoint"; 12740 if (code == FHIRDefinedType.CONTRIBUTOR) 12741 return "Contributor"; 12742 if (code == FHIRDefinedType.COUNT) 12743 return "Count"; 12744 if (code == FHIRDefinedType.DATAREQUIREMENT) 12745 return "DataRequirement"; 12746 if (code == FHIRDefinedType.DISTANCE) 12747 return "Distance"; 12748 if (code == FHIRDefinedType.DOSAGE) 12749 return "Dosage"; 12750 if (code == FHIRDefinedType.DURATION) 12751 return "Duration"; 12752 if (code == FHIRDefinedType.ELEMENT) 12753 return "Element"; 12754 if (code == FHIRDefinedType.ELEMENTDEFINITION) 12755 return "ElementDefinition"; 12756 if (code == FHIRDefinedType.EXPRESSION) 12757 return "Expression"; 12758 if (code == FHIRDefinedType.EXTENSION) 12759 return "Extension"; 12760 if (code == FHIRDefinedType.HUMANNAME) 12761 return "HumanName"; 12762 if (code == FHIRDefinedType.IDENTIFIER) 12763 return "Identifier"; 12764 if (code == FHIRDefinedType.MARKETINGSTATUS) 12765 return "MarketingStatus"; 12766 if (code == FHIRDefinedType.META) 12767 return "Meta"; 12768 if (code == FHIRDefinedType.MONEY) 12769 return "Money"; 12770 if (code == FHIRDefinedType.MONEYQUANTITY) 12771 return "MoneyQuantity"; 12772 if (code == FHIRDefinedType.NARRATIVE) 12773 return "Narrative"; 12774 if (code == FHIRDefinedType.PARAMETERDEFINITION) 12775 return "ParameterDefinition"; 12776 if (code == FHIRDefinedType.PERIOD) 12777 return "Period"; 12778 if (code == FHIRDefinedType.POPULATION) 12779 return "Population"; 12780 if (code == FHIRDefinedType.PRODCHARACTERISTIC) 12781 return "ProdCharacteristic"; 12782 if (code == FHIRDefinedType.PRODUCTSHELFLIFE) 12783 return "ProductShelfLife"; 12784 if (code == FHIRDefinedType.QUANTITY) 12785 return "Quantity"; 12786 if (code == FHIRDefinedType.RANGE) 12787 return "Range"; 12788 if (code == FHIRDefinedType.RATIO) 12789 return "Ratio"; 12790 if (code == FHIRDefinedType.REFERENCE) 12791 return "Reference"; 12792 if (code == FHIRDefinedType.RELATEDARTIFACT) 12793 return "RelatedArtifact"; 12794 if (code == FHIRDefinedType.SAMPLEDDATA) 12795 return "SampledData"; 12796 if (code == FHIRDefinedType.SIGNATURE) 12797 return "Signature"; 12798 if (code == FHIRDefinedType.SIMPLEQUANTITY) 12799 return "SimpleQuantity"; 12800 if (code == FHIRDefinedType.SUBSTANCEAMOUNT) 12801 return "SubstanceAmount"; 12802 if (code == FHIRDefinedType.TIMING) 12803 return "Timing"; 12804 if (code == FHIRDefinedType.TRIGGERDEFINITION) 12805 return "TriggerDefinition"; 12806 if (code == FHIRDefinedType.USAGECONTEXT) 12807 return "UsageContext"; 12808 if (code == FHIRDefinedType.BASE64BINARY) 12809 return "base64Binary"; 12810 if (code == FHIRDefinedType.BOOLEAN) 12811 return "boolean"; 12812 if (code == FHIRDefinedType.CANONICAL) 12813 return "canonical"; 12814 if (code == FHIRDefinedType.CODE) 12815 return "code"; 12816 if (code == FHIRDefinedType.DATE) 12817 return "date"; 12818 if (code == FHIRDefinedType.DATETIME) 12819 return "dateTime"; 12820 if (code == FHIRDefinedType.DECIMAL) 12821 return "decimal"; 12822 if (code == FHIRDefinedType.ID) 12823 return "id"; 12824 if (code == FHIRDefinedType.INSTANT) 12825 return "instant"; 12826 if (code == FHIRDefinedType.INTEGER) 12827 return "integer"; 12828 if (code == FHIRDefinedType.MARKDOWN) 12829 return "markdown"; 12830 if (code == FHIRDefinedType.OID) 12831 return "oid"; 12832 if (code == FHIRDefinedType.POSITIVEINT) 12833 return "positiveInt"; 12834 if (code == FHIRDefinedType.STRING) 12835 return "string"; 12836 if (code == FHIRDefinedType.TIME) 12837 return "time"; 12838 if (code == FHIRDefinedType.UNSIGNEDINT) 12839 return "unsignedInt"; 12840 if (code == FHIRDefinedType.URI) 12841 return "uri"; 12842 if (code == FHIRDefinedType.URL) 12843 return "url"; 12844 if (code == FHIRDefinedType.UUID) 12845 return "uuid"; 12846 if (code == FHIRDefinedType.XHTML) 12847 return "xhtml"; 12848 if (code == FHIRDefinedType.ACCOUNT) 12849 return "Account"; 12850 if (code == FHIRDefinedType.ACTIVITYDEFINITION) 12851 return "ActivityDefinition"; 12852 if (code == FHIRDefinedType.ADVERSEEVENT) 12853 return "AdverseEvent"; 12854 if (code == FHIRDefinedType.ALLERGYINTOLERANCE) 12855 return "AllergyIntolerance"; 12856 if (code == FHIRDefinedType.APPOINTMENT) 12857 return "Appointment"; 12858 if (code == FHIRDefinedType.APPOINTMENTRESPONSE) 12859 return "AppointmentResponse"; 12860 if (code == FHIRDefinedType.AUDITEVENT) 12861 return "AuditEvent"; 12862 if (code == FHIRDefinedType.BASIC) 12863 return "Basic"; 12864 if (code == FHIRDefinedType.BINARY) 12865 return "Binary"; 12866 if (code == FHIRDefinedType.BIOLOGICALLYDERIVEDPRODUCT) 12867 return "BiologicallyDerivedProduct"; 12868 if (code == FHIRDefinedType.BODYSTRUCTURE) 12869 return "BodyStructure"; 12870 if (code == FHIRDefinedType.BUNDLE) 12871 return "Bundle"; 12872 if (code == FHIRDefinedType.CAPABILITYSTATEMENT) 12873 return "CapabilityStatement"; 12874 if (code == FHIRDefinedType.CAREPLAN) 12875 return "CarePlan"; 12876 if (code == FHIRDefinedType.CARETEAM) 12877 return "CareTeam"; 12878 if (code == FHIRDefinedType.CATALOGENTRY) 12879 return "CatalogEntry"; 12880 if (code == FHIRDefinedType.CHARGEITEM) 12881 return "ChargeItem"; 12882 if (code == FHIRDefinedType.CHARGEITEMDEFINITION) 12883 return "ChargeItemDefinition"; 12884 if (code == FHIRDefinedType.CLAIM) 12885 return "Claim"; 12886 if (code == FHIRDefinedType.CLAIMRESPONSE) 12887 return "ClaimResponse"; 12888 if (code == FHIRDefinedType.CLINICALIMPRESSION) 12889 return "ClinicalImpression"; 12890 if (code == FHIRDefinedType.CODESYSTEM) 12891 return "CodeSystem"; 12892 if (code == FHIRDefinedType.COMMUNICATION) 12893 return "Communication"; 12894 if (code == FHIRDefinedType.COMMUNICATIONREQUEST) 12895 return "CommunicationRequest"; 12896 if (code == FHIRDefinedType.COMPARTMENTDEFINITION) 12897 return "CompartmentDefinition"; 12898 if (code == FHIRDefinedType.COMPOSITION) 12899 return "Composition"; 12900 if (code == FHIRDefinedType.CONCEPTMAP) 12901 return "ConceptMap"; 12902 if (code == FHIRDefinedType.CONDITION) 12903 return "Condition"; 12904 if (code == FHIRDefinedType.CONSENT) 12905 return "Consent"; 12906 if (code == FHIRDefinedType.CONTRACT) 12907 return "Contract"; 12908 if (code == FHIRDefinedType.COVERAGE) 12909 return "Coverage"; 12910 if (code == FHIRDefinedType.COVERAGEELIGIBILITYREQUEST) 12911 return "CoverageEligibilityRequest"; 12912 if (code == FHIRDefinedType.COVERAGEELIGIBILITYRESPONSE) 12913 return "CoverageEligibilityResponse"; 12914 if (code == FHIRDefinedType.DETECTEDISSUE) 12915 return "DetectedIssue"; 12916 if (code == FHIRDefinedType.DEVICE) 12917 return "Device"; 12918 if (code == FHIRDefinedType.DEVICEDEFINITION) 12919 return "DeviceDefinition"; 12920 if (code == FHIRDefinedType.DEVICEMETRIC) 12921 return "DeviceMetric"; 12922 if (code == FHIRDefinedType.DEVICEREQUEST) 12923 return "DeviceRequest"; 12924 if (code == FHIRDefinedType.DEVICEUSESTATEMENT) 12925 return "DeviceUseStatement"; 12926 if (code == FHIRDefinedType.DIAGNOSTICREPORT) 12927 return "DiagnosticReport"; 12928 if (code == FHIRDefinedType.DOCUMENTMANIFEST) 12929 return "DocumentManifest"; 12930 if (code == FHIRDefinedType.DOCUMENTREFERENCE) 12931 return "DocumentReference"; 12932 if (code == FHIRDefinedType.DOMAINRESOURCE) 12933 return "DomainResource"; 12934 if (code == FHIRDefinedType.EFFECTEVIDENCESYNTHESIS) 12935 return "EffectEvidenceSynthesis"; 12936 if (code == FHIRDefinedType.ENCOUNTER) 12937 return "Encounter"; 12938 if (code == FHIRDefinedType.ENDPOINT) 12939 return "Endpoint"; 12940 if (code == FHIRDefinedType.ENROLLMENTREQUEST) 12941 return "EnrollmentRequest"; 12942 if (code == FHIRDefinedType.ENROLLMENTRESPONSE) 12943 return "EnrollmentResponse"; 12944 if (code == FHIRDefinedType.EPISODEOFCARE) 12945 return "EpisodeOfCare"; 12946 if (code == FHIRDefinedType.EVENTDEFINITION) 12947 return "EventDefinition"; 12948 if (code == FHIRDefinedType.EVIDENCE) 12949 return "Evidence"; 12950 if (code == FHIRDefinedType.EVIDENCEVARIABLE) 12951 return "EvidenceVariable"; 12952 if (code == FHIRDefinedType.EXAMPLESCENARIO) 12953 return "ExampleScenario"; 12954 if (code == FHIRDefinedType.EXPLANATIONOFBENEFIT) 12955 return "ExplanationOfBenefit"; 12956 if (code == FHIRDefinedType.FAMILYMEMBERHISTORY) 12957 return "FamilyMemberHistory"; 12958 if (code == FHIRDefinedType.FLAG) 12959 return "Flag"; 12960 if (code == FHIRDefinedType.GOAL) 12961 return "Goal"; 12962 if (code == FHIRDefinedType.GRAPHDEFINITION) 12963 return "GraphDefinition"; 12964 if (code == FHIRDefinedType.GROUP) 12965 return "Group"; 12966 if (code == FHIRDefinedType.GUIDANCERESPONSE) 12967 return "GuidanceResponse"; 12968 if (code == FHIRDefinedType.HEALTHCARESERVICE) 12969 return "HealthcareService"; 12970 if (code == FHIRDefinedType.IMAGINGSTUDY) 12971 return "ImagingStudy"; 12972 if (code == FHIRDefinedType.IMMUNIZATION) 12973 return "Immunization"; 12974 if (code == FHIRDefinedType.IMMUNIZATIONEVALUATION) 12975 return "ImmunizationEvaluation"; 12976 if (code == FHIRDefinedType.IMMUNIZATIONRECOMMENDATION) 12977 return "ImmunizationRecommendation"; 12978 if (code == FHIRDefinedType.IMPLEMENTATIONGUIDE) 12979 return "ImplementationGuide"; 12980 if (code == FHIRDefinedType.INSURANCEPLAN) 12981 return "InsurancePlan"; 12982 if (code == FHIRDefinedType.INVOICE) 12983 return "Invoice"; 12984 if (code == FHIRDefinedType.LIBRARY) 12985 return "Library"; 12986 if (code == FHIRDefinedType.LINKAGE) 12987 return "Linkage"; 12988 if (code == FHIRDefinedType.LIST) 12989 return "List"; 12990 if (code == FHIRDefinedType.LOCATION) 12991 return "Location"; 12992 if (code == FHIRDefinedType.MEASURE) 12993 return "Measure"; 12994 if (code == FHIRDefinedType.MEASUREREPORT) 12995 return "MeasureReport"; 12996 if (code == FHIRDefinedType.MEDIA) 12997 return "Media"; 12998 if (code == FHIRDefinedType.MEDICATION) 12999 return "Medication"; 13000 if (code == FHIRDefinedType.MEDICATIONADMINISTRATION) 13001 return "MedicationAdministration"; 13002 if (code == FHIRDefinedType.MEDICATIONDISPENSE) 13003 return "MedicationDispense"; 13004 if (code == FHIRDefinedType.MEDICATIONKNOWLEDGE) 13005 return "MedicationKnowledge"; 13006 if (code == FHIRDefinedType.MEDICATIONREQUEST) 13007 return "MedicationRequest"; 13008 if (code == FHIRDefinedType.MEDICATIONSTATEMENT) 13009 return "MedicationStatement"; 13010 if (code == FHIRDefinedType.MEDICINALPRODUCT) 13011 return "MedicinalProduct"; 13012 if (code == FHIRDefinedType.MEDICINALPRODUCTAUTHORIZATION) 13013 return "MedicinalProductAuthorization"; 13014 if (code == FHIRDefinedType.MEDICINALPRODUCTCONTRAINDICATION) 13015 return "MedicinalProductContraindication"; 13016 if (code == FHIRDefinedType.MEDICINALPRODUCTINDICATION) 13017 return "MedicinalProductIndication"; 13018 if (code == FHIRDefinedType.MEDICINALPRODUCTINGREDIENT) 13019 return "MedicinalProductIngredient"; 13020 if (code == FHIRDefinedType.MEDICINALPRODUCTINTERACTION) 13021 return "MedicinalProductInteraction"; 13022 if (code == FHIRDefinedType.MEDICINALPRODUCTMANUFACTURED) 13023 return "MedicinalProductManufactured"; 13024 if (code == FHIRDefinedType.MEDICINALPRODUCTPACKAGED) 13025 return "MedicinalProductPackaged"; 13026 if (code == FHIRDefinedType.MEDICINALPRODUCTPHARMACEUTICAL) 13027 return "MedicinalProductPharmaceutical"; 13028 if (code == FHIRDefinedType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 13029 return "MedicinalProductUndesirableEffect"; 13030 if (code == FHIRDefinedType.MESSAGEDEFINITION) 13031 return "MessageDefinition"; 13032 if (code == FHIRDefinedType.MESSAGEHEADER) 13033 return "MessageHeader"; 13034 if (code == FHIRDefinedType.MOLECULARSEQUENCE) 13035 return "MolecularSequence"; 13036 if (code == FHIRDefinedType.NAMINGSYSTEM) 13037 return "NamingSystem"; 13038 if (code == FHIRDefinedType.NUTRITIONORDER) 13039 return "NutritionOrder"; 13040 if (code == FHIRDefinedType.OBSERVATION) 13041 return "Observation"; 13042 if (code == FHIRDefinedType.OBSERVATIONDEFINITION) 13043 return "ObservationDefinition"; 13044 if (code == FHIRDefinedType.OPERATIONDEFINITION) 13045 return "OperationDefinition"; 13046 if (code == FHIRDefinedType.OPERATIONOUTCOME) 13047 return "OperationOutcome"; 13048 if (code == FHIRDefinedType.ORGANIZATION) 13049 return "Organization"; 13050 if (code == FHIRDefinedType.ORGANIZATIONAFFILIATION) 13051 return "OrganizationAffiliation"; 13052 if (code == FHIRDefinedType.PARAMETERS) 13053 return "Parameters"; 13054 if (code == FHIRDefinedType.PATIENT) 13055 return "Patient"; 13056 if (code == FHIRDefinedType.PAYMENTNOTICE) 13057 return "PaymentNotice"; 13058 if (code == FHIRDefinedType.PAYMENTRECONCILIATION) 13059 return "PaymentReconciliation"; 13060 if (code == FHIRDefinedType.PERSON) 13061 return "Person"; 13062 if (code == FHIRDefinedType.PLANDEFINITION) 13063 return "PlanDefinition"; 13064 if (code == FHIRDefinedType.PRACTITIONER) 13065 return "Practitioner"; 13066 if (code == FHIRDefinedType.PRACTITIONERROLE) 13067 return "PractitionerRole"; 13068 if (code == FHIRDefinedType.PROCEDURE) 13069 return "Procedure"; 13070 if (code == FHIRDefinedType.PROVENANCE) 13071 return "Provenance"; 13072 if (code == FHIRDefinedType.QUESTIONNAIRE) 13073 return "Questionnaire"; 13074 if (code == FHIRDefinedType.QUESTIONNAIRERESPONSE) 13075 return "QuestionnaireResponse"; 13076 if (code == FHIRDefinedType.RELATEDPERSON) 13077 return "RelatedPerson"; 13078 if (code == FHIRDefinedType.REQUESTGROUP) 13079 return "RequestGroup"; 13080 if (code == FHIRDefinedType.RESEARCHDEFINITION) 13081 return "ResearchDefinition"; 13082 if (code == FHIRDefinedType.RESEARCHELEMENTDEFINITION) 13083 return "ResearchElementDefinition"; 13084 if (code == FHIRDefinedType.RESEARCHSTUDY) 13085 return "ResearchStudy"; 13086 if (code == FHIRDefinedType.RESEARCHSUBJECT) 13087 return "ResearchSubject"; 13088 if (code == FHIRDefinedType.RESOURCE) 13089 return "Resource"; 13090 if (code == FHIRDefinedType.RISKASSESSMENT) 13091 return "RiskAssessment"; 13092 if (code == FHIRDefinedType.RISKEVIDENCESYNTHESIS) 13093 return "RiskEvidenceSynthesis"; 13094 if (code == FHIRDefinedType.SCHEDULE) 13095 return "Schedule"; 13096 if (code == FHIRDefinedType.SEARCHPARAMETER) 13097 return "SearchParameter"; 13098 if (code == FHIRDefinedType.SERVICEREQUEST) 13099 return "ServiceRequest"; 13100 if (code == FHIRDefinedType.SLOT) 13101 return "Slot"; 13102 if (code == FHIRDefinedType.SPECIMEN) 13103 return "Specimen"; 13104 if (code == FHIRDefinedType.SPECIMENDEFINITION) 13105 return "SpecimenDefinition"; 13106 if (code == FHIRDefinedType.STRUCTUREDEFINITION) 13107 return "StructureDefinition"; 13108 if (code == FHIRDefinedType.STRUCTUREMAP) 13109 return "StructureMap"; 13110 if (code == FHIRDefinedType.SUBSCRIPTION) 13111 return "Subscription"; 13112 if (code == FHIRDefinedType.SUBSTANCE) 13113 return "Substance"; 13114 if (code == FHIRDefinedType.SUBSTANCENUCLEICACID) 13115 return "SubstanceNucleicAcid"; 13116 if (code == FHIRDefinedType.SUBSTANCEPOLYMER) 13117 return "SubstancePolymer"; 13118 if (code == FHIRDefinedType.SUBSTANCEPROTEIN) 13119 return "SubstanceProtein"; 13120 if (code == FHIRDefinedType.SUBSTANCEREFERENCEINFORMATION) 13121 return "SubstanceReferenceInformation"; 13122 if (code == FHIRDefinedType.SUBSTANCESOURCEMATERIAL) 13123 return "SubstanceSourceMaterial"; 13124 if (code == FHIRDefinedType.SUBSTANCESPECIFICATION) 13125 return "SubstanceSpecification"; 13126 if (code == FHIRDefinedType.SUPPLYDELIVERY) 13127 return "SupplyDelivery"; 13128 if (code == FHIRDefinedType.SUPPLYREQUEST) 13129 return "SupplyRequest"; 13130 if (code == FHIRDefinedType.TASK) 13131 return "Task"; 13132 if (code == FHIRDefinedType.TERMINOLOGYCAPABILITIES) 13133 return "TerminologyCapabilities"; 13134 if (code == FHIRDefinedType.TESTREPORT) 13135 return "TestReport"; 13136 if (code == FHIRDefinedType.TESTSCRIPT) 13137 return "TestScript"; 13138 if (code == FHIRDefinedType.VALUESET) 13139 return "ValueSet"; 13140 if (code == FHIRDefinedType.VERIFICATIONRESULT) 13141 return "VerificationResult"; 13142 if (code == FHIRDefinedType.VISIONPRESCRIPTION) 13143 return "VisionPrescription"; 13144 return "?"; 13145 } 13146 13147 public String toSystem(FHIRDefinedType code) { 13148 return code.getSystem(); 13149 } 13150 } 13151 13152 public enum FHIRVersion { 13153 /** 13154 * Oldest archived version of FHIR. 13155 */ 13156 _0_01, 13157 /** 13158 * 1st Draft for Comment (Sept 2012 Ballot). 13159 */ 13160 _0_05, 13161 /** 13162 * 2nd Draft for Comment (January 2013 Ballot). 13163 */ 13164 _0_06, 13165 /** 13166 * DSTU 1 Ballot version. 13167 */ 13168 _0_11, 13169 /** 13170 * DSTU 1 version. 13171 */ 13172 _0_0, 13173 /** 13174 * DSTU 1 Official version. 13175 */ 13176 _0_0_80, 13177 /** 13178 * DSTU 1 Official version Technical Errata #1. 13179 */ 13180 _0_0_81, 13181 /** 13182 * DSTU 1 Official version Technical Errata #2. 13183 */ 13184 _0_0_82, 13185 /** 13186 * January 2015 Ballot. 13187 */ 13188 _0_4, 13189 /** 13190 * Draft For Comment (January 2015 Ballot). 13191 */ 13192 _0_4_0, 13193 /** 13194 * May 2015 Ballot. 13195 */ 13196 _0_5, 13197 /** 13198 * DSTU 2 Ballot version (May 2015 Ballot). 13199 */ 13200 _0_5_0, 13201 /** 13202 * DSTU 2 version. 13203 */ 13204 _1_0, 13205 /** 13206 * DSTU 2 QA Preview + CQIF Ballot (Sep 2015). 13207 */ 13208 _1_0_0, 13209 /** 13210 * DSTU 2 (Official version). 13211 */ 13212 _1_0_1, 13213 /** 13214 * DSTU 2 (Official version) with 1 technical errata. 13215 */ 13216 _1_0_2, 13217 /** 13218 * GAO Ballot version. 13219 */ 13220 _1_1, 13221 /** 13222 * GAO Ballot + draft changes to main FHIR standard. 13223 */ 13224 _1_1_0, 13225 /** 13226 * Connectathon 12 (Montreal) version. 13227 */ 13228 _1_4, 13229 /** 13230 * CQF on FHIR Ballot + Connectathon 12 (Montreal). 13231 */ 13232 _1_4_0, 13233 /** 13234 * Connectathon 13 (Baltimore) version. 13235 */ 13236 _1_6, 13237 /** 13238 * FHIR STU3 Ballot + Connectathon 13 (Baltimore). 13239 */ 13240 _1_6_0, 13241 /** 13242 * Connectathon 14 (San Antonio) version. 13243 */ 13244 _1_8, 13245 /** 13246 * FHIR STU3 Candidate + Connectathon 14 (San Antonio). 13247 */ 13248 _1_8_0, 13249 /** 13250 * STU3 version. 13251 */ 13252 _3_0, 13253 /** 13254 * FHIR Release 3 (STU). 13255 */ 13256 _3_0_0, 13257 /** 13258 * FHIR Release 3 (STU) with 1 technical errata. 13259 */ 13260 _3_0_1, 13261 /** 13262 * FHIR Release 3 (STU) with 2 technical errata. 13263 */ 13264 _3_0_2, 13265 /** 13266 * R4 Ballot #1 version. 13267 */ 13268 _3_3, 13269 /** 13270 * R4 Ballot #1 + Connectaton 18 (Cologne). 13271 */ 13272 _3_3_0, 13273 /** 13274 * R4 Ballot #2 version. 13275 */ 13276 _3_5, 13277 /** 13278 * R4 Ballot #2 + Connectathon 19 (Baltimore). 13279 */ 13280 _3_5_0, 13281 /** 13282 * R4 version. 13283 */ 13284 _4_0, 13285 /** 13286 * FHIR Release 4 (Normative + STU). 13287 */ 13288 _4_0_0, 13289 /** 13290 * FHIR Release 4 (Normative + STU) with 1 technical errata. 13291 */ 13292 _4_0_1, 13293 /** 13294 * R4B Ballot #1 version. 13295 */ 13296 _4_1, 13297 /** 13298 * R4B Ballot #1 + Connectathon 27 (Virtual). 13299 */ 13300 _4_1_0, _4_3_0_SNAPSHOT1, _4_3_0_CIBUILD, 13301 /** 13302 * R5 Preview #1 version. 13303 */ 13304 _4_2, 13305 /** 13306 * R5 Preview #1 + Connectathon 23 (Sydney). 13307 */ 13308 _4_2_0, 13309 /** 13310 * R4B version. 13311 */ 13312 _4_3, 13313 /** 13314 * FHIR Release 4B (Normative + STU). 13315 */ 13316 _4_3_0, 13317 /** 13318 * R5 Preview #2 version. 13319 */ 13320 _4_4, 13321 /** 13322 * R5 Preview #2 + Connectathon 24 (Virtual). 13323 */ 13324 _4_4_0, 13325 /** 13326 * R5 Preview #3 version. 13327 */ 13328 _4_5, 13329 /** 13330 * R5 Preview #3 + Connectathon 25 (Virtual). 13331 */ 13332 _4_5_0, 13333 /** 13334 * R5 Draft Ballot version. 13335 */ 13336 _4_6, 13337 /** 13338 * R5 Draft Ballot + Connectathon 27 (Virtual). 13339 */ 13340 _4_6_0, 13341 /** 13342 * R5 Versions. 13343 */ 13344 _5_0, 13345 /** 13346 * R5 Final Version. 13347 */ 13348 _5_0_0, 13349 /** 13350 * R5 Rolling ci-build. 13351 */ 13352 _5_0_0CIBUILD, 13353 /** 13354 * R5 Preview #2. 13355 */ 13356 _5_0_0SNAPSHOT1, 13357 /** 13358 * R5 Interim tooling stage. 13359 */ 13360 _5_0_0SNAPSHOT2, 13361 /** 13362 * R5 Ballot. 13363 */ 13364 _5_0_0BALLOT, 13365 /** 13366 * R5Connectathon 32 release. 13367 */ 13368 _5_0_0SNAPSHOT3, _5_0_0DRAFTFINAL, 13369 /** 13370 * added to help the parsers 13371 */ 13372 NULL; 13373 13374 public static FHIRVersion fromCode(String codeString) throws FHIRException { 13375 if (codeString == null || "".equals(codeString)) 13376 return null; 13377 if ("0.01".equals(codeString)) 13378 return _0_01; 13379 if ("0.05".equals(codeString)) 13380 return _0_05; 13381 if ("0.06".equals(codeString)) 13382 return _0_06; 13383 if ("0.11".equals(codeString)) 13384 return _0_11; 13385 if ("0.0".equals(codeString)) 13386 return _0_0; 13387 if ("0.0.80".equals(codeString)) 13388 return _0_0_80; 13389 if ("0.0.81".equals(codeString)) 13390 return _0_0_81; 13391 if ("0.0.82".equals(codeString)) 13392 return _0_0_82; 13393 if ("0.4".equals(codeString)) 13394 return _0_4; 13395 if ("0.4.0".equals(codeString)) 13396 return _0_4_0; 13397 if ("0.5".equals(codeString)) 13398 return _0_5; 13399 if ("0.5.0".equals(codeString)) 13400 return _0_5_0; 13401 if ("1.0".equals(codeString)) 13402 return _1_0; 13403 if ("1.0.0".equals(codeString)) 13404 return _1_0_0; 13405 if ("1.0.1".equals(codeString)) 13406 return _1_0_1; 13407 if ("1.0.2".equals(codeString)) 13408 return _1_0_2; 13409 if ("1.1".equals(codeString)) 13410 return _1_1; 13411 if ("1.1.0".equals(codeString)) 13412 return _1_1_0; 13413 if ("1.4".equals(codeString)) 13414 return _1_4; 13415 if ("1.4.0".equals(codeString)) 13416 return _1_4_0; 13417 if ("1.6".equals(codeString)) 13418 return _1_6; 13419 if ("1.6.0".equals(codeString)) 13420 return _1_6_0; 13421 if ("1.8".equals(codeString)) 13422 return _1_8; 13423 if ("1.8.0".equals(codeString)) 13424 return _1_8_0; 13425 if ("3.0".equals(codeString)) 13426 return _3_0; 13427 if ("3.0.0".equals(codeString)) 13428 return _3_0_0; 13429 if ("3.0.1".equals(codeString)) 13430 return _3_0_1; 13431 if ("3.0.2".equals(codeString)) 13432 return _3_0_2; 13433 if ("3.3".equals(codeString)) 13434 return _3_3; 13435 if ("3.3.0".equals(codeString)) 13436 return _3_3_0; 13437 if ("3.5".equals(codeString)) 13438 return _3_5; 13439 if ("3.5.0".equals(codeString)) 13440 return _3_5_0; 13441 if ("4.0".equals(codeString)) 13442 return _4_0; 13443 if ("4.0.0".equals(codeString)) 13444 return _4_0_0; 13445 if ("4.0.1".equals(codeString)) 13446 return _4_0_1; 13447 if ("4.1".equals(codeString)) 13448 return _4_1; 13449 if ("4.1.0".equals(codeString)) 13450 return _4_1_0; 13451 if ("4.3.0-snapshot1".equals(codeString)) 13452 return _4_3_0_SNAPSHOT1; 13453 if ("4.3.0-cibuild".equals(codeString)) 13454 return _4_3_0_CIBUILD; 13455 if ("4.2".equals(codeString)) 13456 return _4_2; 13457 if ("4.2.0".equals(codeString)) 13458 return _4_2_0; 13459 if ("4.3".equals(codeString)) 13460 return _4_3; 13461 if ("4.3.0".equals(codeString)) 13462 return _4_3_0; 13463 if ("4.4".equals(codeString)) 13464 return _4_4; 13465 if ("4.4.0".equals(codeString)) 13466 return _4_4_0; 13467 if ("4.5".equals(codeString)) 13468 return _4_5; 13469 if ("4.5.0".equals(codeString)) 13470 return _4_5_0; 13471 if ("4.6".equals(codeString)) 13472 return _4_6; 13473 if ("4.6.0".equals(codeString)) 13474 return _4_6_0; 13475 if ("5.0".equals(codeString)) 13476 return _5_0; 13477 if ("5.0.0".equals(codeString)) 13478 return _5_0_0; 13479 if ("5.0.0-cibuild".equals(codeString)) 13480 return _5_0_0CIBUILD; 13481 if ("5.0.0-snapshot1".equals(codeString)) 13482 return _5_0_0SNAPSHOT1; 13483 if ("5.0.0-snapshot2".equals(codeString)) 13484 return _5_0_0SNAPSHOT2; 13485 if ("5.0.0-ballot".equals(codeString)) 13486 return _5_0_0BALLOT; 13487 if ("5.0.0-snapshot3".equals(codeString)) 13488 return _5_0_0SNAPSHOT3; 13489 if ("5.0.0-draft-final".equals(codeString)) 13490 return _5_0_0DRAFTFINAL; 13491 throw new FHIRException("Unknown FHIRVersion code '" + codeString + "'"); 13492 } 13493 13494 public static boolean isValidCode(String codeString) { 13495 if (codeString == null || "".equals(codeString)) 13496 return false; 13497 if ("0.01".equals(codeString)) 13498 return true; 13499 if ("0.05".equals(codeString)) 13500 return true; 13501 if ("0.06".equals(codeString)) 13502 return true; 13503 if ("0.11".equals(codeString)) 13504 return true; 13505 if ("0.0".equals(codeString)) 13506 return true; 13507 if ("0.0.80".equals(codeString)) 13508 return true; 13509 if ("0.0.81".equals(codeString)) 13510 return true; 13511 if ("0.0.82".equals(codeString)) 13512 return true; 13513 if ("0.4".equals(codeString)) 13514 return true; 13515 if ("0.4.0".equals(codeString)) 13516 return true; 13517 if ("0.5".equals(codeString)) 13518 return true; 13519 if ("0.5.0".equals(codeString)) 13520 return true; 13521 if ("1.0".equals(codeString)) 13522 return true; 13523 if ("1.0.0".equals(codeString)) 13524 return true; 13525 if ("1.0.1".equals(codeString)) 13526 return true; 13527 if ("1.0.2".equals(codeString)) 13528 return true; 13529 if ("1.1".equals(codeString)) 13530 return true; 13531 if ("1.1.0".equals(codeString)) 13532 return true; 13533 if ("1.4".equals(codeString)) 13534 return true; 13535 if ("1.4.0".equals(codeString)) 13536 return true; 13537 if ("1.6".equals(codeString)) 13538 return true; 13539 if ("1.6.0".equals(codeString)) 13540 return true; 13541 if ("1.8".equals(codeString)) 13542 return true; 13543 if ("1.8.0".equals(codeString)) 13544 return true; 13545 if ("3.0".equals(codeString)) 13546 return true; 13547 if ("3.0.0".equals(codeString)) 13548 return true; 13549 if ("3.0.1".equals(codeString)) 13550 return true; 13551 if ("3.0.2".equals(codeString)) 13552 return true; 13553 if ("3.3".equals(codeString)) 13554 return true; 13555 if ("3.3.0".equals(codeString)) 13556 return true; 13557 if ("3.5".equals(codeString)) 13558 return true; 13559 if ("3.5.0".equals(codeString)) 13560 return true; 13561 if ("4.0".equals(codeString)) 13562 return true; 13563 if ("4.0.0".equals(codeString)) 13564 return true; 13565 if ("4.0.1".equals(codeString)) 13566 return true; 13567 if ("4.1".equals(codeString)) 13568 return true; 13569 if ("4.1.0".equals(codeString)) 13570 return true; 13571 if ("4.2".equals(codeString)) 13572 return true; 13573 if ("4.2.0".equals(codeString)) 13574 return true; 13575 if ("4.3".equals(codeString)) 13576 return true; 13577 if ("4.3.0".equals(codeString)) 13578 return true; 13579 if ("4.4".equals(codeString)) 13580 return true; 13581 if ("4.4.0".equals(codeString)) 13582 return true; 13583 if ("4.5".equals(codeString)) 13584 return true; 13585 if ("4.5.0".equals(codeString)) 13586 return true; 13587 if ("4.6".equals(codeString)) 13588 return true; 13589 if ("4.6.0".equals(codeString)) 13590 return true; 13591 if ("5.0".equals(codeString)) 13592 return true; 13593 if ("5.0.0".equals(codeString)) 13594 return true; 13595 if ("5.0.0-cibuild".equals(codeString)) 13596 return true; 13597 if ("5.0.0-snapshot1".equals(codeString)) 13598 return true; 13599 if ("5.0.0-snapshot2".equals(codeString)) 13600 return true; 13601 if ("5.0.0-ballot".equals(codeString)) 13602 return true; 13603 return false; 13604 } 13605 13606 public String toCode() { 13607 switch (this) { 13608 case _0_01: 13609 return "0.01"; 13610 case _0_05: 13611 return "0.05"; 13612 case _0_06: 13613 return "0.06"; 13614 case _0_11: 13615 return "0.11"; 13616 case _0_0: 13617 return "0.0"; 13618 case _0_0_80: 13619 return "0.0.80"; 13620 case _0_0_81: 13621 return "0.0.81"; 13622 case _0_0_82: 13623 return "0.0.82"; 13624 case _0_4: 13625 return "0.4"; 13626 case _0_4_0: 13627 return "0.4.0"; 13628 case _0_5: 13629 return "0.5"; 13630 case _0_5_0: 13631 return "0.5.0"; 13632 case _1_0: 13633 return "1.0"; 13634 case _1_0_0: 13635 return "1.0.0"; 13636 case _1_0_1: 13637 return "1.0.1"; 13638 case _1_0_2: 13639 return "1.0.2"; 13640 case _1_1: 13641 return "1.1"; 13642 case _1_1_0: 13643 return "1.1.0"; 13644 case _1_4: 13645 return "1.4"; 13646 case _1_4_0: 13647 return "1.4.0"; 13648 case _1_6: 13649 return "1.6"; 13650 case _1_6_0: 13651 return "1.6.0"; 13652 case _1_8: 13653 return "1.8"; 13654 case _1_8_0: 13655 return "1.8.0"; 13656 case _3_0: 13657 return "3.0"; 13658 case _3_0_0: 13659 return "3.0.0"; 13660 case _3_0_1: 13661 return "3.0.1"; 13662 case _3_0_2: 13663 return "3.0.2"; 13664 case _3_3: 13665 return "3.3"; 13666 case _3_3_0: 13667 return "3.3.0"; 13668 case _3_5: 13669 return "3.5"; 13670 case _3_5_0: 13671 return "3.5.0"; 13672 case _4_0: 13673 return "4.0"; 13674 case _4_0_0: 13675 return "4.0.0"; 13676 case _4_0_1: 13677 return "4.0.1"; 13678 case _4_1: 13679 return "4.1"; 13680 case _4_1_0: 13681 return "4.1.0"; 13682 case _4_2: 13683 return "4.2"; 13684 case _4_2_0: 13685 return "4.2.0"; 13686 case _4_3: 13687 return "4.3"; 13688 case _4_3_0: 13689 return "4.3.0"; 13690 case _4_3_0_SNAPSHOT1: 13691 return "4.3.0-snapshot1"; 13692 case _4_3_0_CIBUILD: 13693 return "4.3.0-cibuild"; 13694 case _4_4: 13695 return "4.4"; 13696 case _4_4_0: 13697 return "4.4.0"; 13698 case _4_5: 13699 return "4.5"; 13700 case _4_5_0: 13701 return "4.5.0"; 13702 case _4_6: 13703 return "4.6"; 13704 case _4_6_0: 13705 return "4.6.0"; 13706 case _5_0: 13707 return "5.0"; 13708 case _5_0_0: 13709 return "5.0.0"; 13710 case _5_0_0CIBUILD: 13711 return "5.0.0-cibuild"; 13712 case _5_0_0SNAPSHOT1: 13713 return "5.0.0-snapshot1"; 13714 case _5_0_0SNAPSHOT2: 13715 return "5.0.0-snapshot2"; 13716 case _5_0_0BALLOT: 13717 return "5.0.0-ballot"; 13718 case _5_0_0SNAPSHOT3: 13719 return "5.0.0-snapshot3"; 13720 case _5_0_0DRAFTFINAL: 13721 return "5.0.0-draft-final"; 13722 case NULL: 13723 return null; 13724 default: 13725 return "?"; 13726 } 13727 } 13728 13729 public String getSystem() { 13730 switch (this) { 13731 case _0_01: 13732 return "http://hl7.org/fhir/FHIR-version"; 13733 case _0_05: 13734 return "http://hl7.org/fhir/FHIR-version"; 13735 case _0_06: 13736 return "http://hl7.org/fhir/FHIR-version"; 13737 case _0_11: 13738 return "http://hl7.org/fhir/FHIR-version"; 13739 case _0_0: 13740 return "http://hl7.org/fhir/FHIR-version"; 13741 case _0_0_80: 13742 return "http://hl7.org/fhir/FHIR-version"; 13743 case _0_0_81: 13744 return "http://hl7.org/fhir/FHIR-version"; 13745 case _0_0_82: 13746 return "http://hl7.org/fhir/FHIR-version"; 13747 case _0_4: 13748 return "http://hl7.org/fhir/FHIR-version"; 13749 case _0_4_0: 13750 return "http://hl7.org/fhir/FHIR-version"; 13751 case _0_5: 13752 return "http://hl7.org/fhir/FHIR-version"; 13753 case _0_5_0: 13754 return "http://hl7.org/fhir/FHIR-version"; 13755 case _1_0: 13756 return "http://hl7.org/fhir/FHIR-version"; 13757 case _1_0_0: 13758 return "http://hl7.org/fhir/FHIR-version"; 13759 case _1_0_1: 13760 return "http://hl7.org/fhir/FHIR-version"; 13761 case _1_0_2: 13762 return "http://hl7.org/fhir/FHIR-version"; 13763 case _1_1: 13764 return "http://hl7.org/fhir/FHIR-version"; 13765 case _1_1_0: 13766 return "http://hl7.org/fhir/FHIR-version"; 13767 case _1_4: 13768 return "http://hl7.org/fhir/FHIR-version"; 13769 case _1_4_0: 13770 return "http://hl7.org/fhir/FHIR-version"; 13771 case _1_6: 13772 return "http://hl7.org/fhir/FHIR-version"; 13773 case _1_6_0: 13774 return "http://hl7.org/fhir/FHIR-version"; 13775 case _1_8: 13776 return "http://hl7.org/fhir/FHIR-version"; 13777 case _1_8_0: 13778 return "http://hl7.org/fhir/FHIR-version"; 13779 case _3_0: 13780 return "http://hl7.org/fhir/FHIR-version"; 13781 case _3_0_0: 13782 return "http://hl7.org/fhir/FHIR-version"; 13783 case _3_0_1: 13784 return "http://hl7.org/fhir/FHIR-version"; 13785 case _3_0_2: 13786 return "http://hl7.org/fhir/FHIR-version"; 13787 case _3_3: 13788 return "http://hl7.org/fhir/FHIR-version"; 13789 case _3_3_0: 13790 return "http://hl7.org/fhir/FHIR-version"; 13791 case _3_5: 13792 return "http://hl7.org/fhir/FHIR-version"; 13793 case _3_5_0: 13794 return "http://hl7.org/fhir/FHIR-version"; 13795 case _4_0: 13796 return "http://hl7.org/fhir/FHIR-version"; 13797 case _4_0_0: 13798 return "http://hl7.org/fhir/FHIR-version"; 13799 case _4_0_1: 13800 return "http://hl7.org/fhir/FHIR-version"; 13801 case _4_1: 13802 return "http://hl7.org/fhir/FHIR-version"; 13803 case _4_1_0: 13804 return "http://hl7.org/fhir/FHIR-version"; 13805 case _4_2: 13806 return "http://hl7.org/fhir/FHIR-version"; 13807 case _4_2_0: 13808 return "http://hl7.org/fhir/FHIR-version"; 13809 case _4_3: 13810 return "http://hl7.org/fhir/FHIR-version"; 13811 case _4_3_0: 13812 return "http://hl7.org/fhir/FHIR-version"; 13813 case _4_3_0_SNAPSHOT1: 13814 return "http://hl7.org/fhir/FHIR-version"; 13815 case _4_3_0_CIBUILD: 13816 return "http://hl7.org/fhir/FHIR-version"; 13817 case _4_4: 13818 return "http://hl7.org/fhir/FHIR-version"; 13819 case _4_4_0: 13820 return "http://hl7.org/fhir/FHIR-version"; 13821 case _4_5: 13822 return "http://hl7.org/fhir/FHIR-version"; 13823 case _4_5_0: 13824 return "http://hl7.org/fhir/FHIR-version"; 13825 case _4_6: 13826 return "http://hl7.org/fhir/FHIR-version"; 13827 case _4_6_0: 13828 return "http://hl7.org/fhir/FHIR-version"; 13829 case _5_0: 13830 return "http://hl7.org/fhir/FHIR-version"; 13831 case _5_0_0: 13832 return "http://hl7.org/fhir/FHIR-version"; 13833 case _5_0_0CIBUILD: 13834 return "http://hl7.org/fhir/FHIR-version"; 13835 case _5_0_0SNAPSHOT1: 13836 return "http://hl7.org/fhir/FHIR-version"; 13837 case _5_0_0SNAPSHOT2: 13838 return "http://hl7.org/fhir/FHIR-version"; 13839 case _5_0_0BALLOT: 13840 return "http://hl7.org/fhir/FHIR-version"; 13841 case _5_0_0SNAPSHOT3: 13842 return "http://hl7.org/fhir/FHIR-version"; 13843 case _5_0_0DRAFTFINAL: 13844 return "http://hl7.org/fhir/FHIR-version"; 13845 case NULL: 13846 return null; 13847 default: 13848 return "?"; 13849 } 13850 } 13851 13852 public String getDefinition() { 13853 switch (this) { 13854 case _0_01: 13855 return "Oldest archived version of FHIR."; 13856 case _0_05: 13857 return "1st Draft for Comment (Sept 2012 Ballot)."; 13858 case _0_06: 13859 return "2nd Draft for Comment (January 2013 Ballot)."; 13860 case _0_11: 13861 return "DSTU 1 Ballot version."; 13862 case _0_0: 13863 return "DSTU 1 version."; 13864 case _0_0_80: 13865 return "DSTU 1 Official version."; 13866 case _0_0_81: 13867 return "DSTU 1 Official version Technical Errata #1."; 13868 case _0_0_82: 13869 return "DSTU 1 Official version Technical Errata #2."; 13870 case _0_4: 13871 return "January 2015 Ballot."; 13872 case _0_4_0: 13873 return "Draft For Comment (January 2015 Ballot)."; 13874 case _0_5: 13875 return "May 2015 Ballot."; 13876 case _0_5_0: 13877 return "DSTU 2 Ballot version (May 2015 Ballot)."; 13878 case _1_0: 13879 return "DSTU 2 version."; 13880 case _1_0_0: 13881 return "DSTU 2 QA Preview + CQIF Ballot (Sep 2015)."; 13882 case _1_0_1: 13883 return "DSTU 2 (Official version)."; 13884 case _1_0_2: 13885 return "DSTU 2 (Official version) with 1 technical errata."; 13886 case _1_1: 13887 return "GAO Ballot version."; 13888 case _1_1_0: 13889 return "GAO Ballot + draft changes to main FHIR standard."; 13890 case _1_4: 13891 return "Connectathon 12 (Montreal) version."; 13892 case _1_4_0: 13893 return "CQF on FHIR Ballot + Connectathon 12 (Montreal)."; 13894 case _1_6: 13895 return "Connectathon 13 (Baltimore) version."; 13896 case _1_6_0: 13897 return "FHIR STU3 Ballot + Connectathon 13 (Baltimore)."; 13898 case _1_8: 13899 return "Connectathon 14 (San Antonio) version."; 13900 case _1_8_0: 13901 return "FHIR STU3 Candidate + Connectathon 14 (San Antonio)."; 13902 case _3_0: 13903 return "STU3 version."; 13904 case _3_0_0: 13905 return "FHIR Release 3 (STU)."; 13906 case _3_0_1: 13907 return "FHIR Release 3 (STU) with 1 technical errata."; 13908 case _3_0_2: 13909 return "FHIR Release 3 (STU) with 2 technical errata."; 13910 case _3_3: 13911 return "R4 Ballot #1 version."; 13912 case _3_3_0: 13913 return "R4 Ballot #1 + Connectaton 18 (Cologne)."; 13914 case _3_5: 13915 return "R4 Ballot #2 version."; 13916 case _3_5_0: 13917 return "R4 Ballot #2 + Connectathon 19 (Baltimore)."; 13918 case _4_0: 13919 return "R4 version."; 13920 case _4_0_0: 13921 return "FHIR Release 4 (Normative + STU)."; 13922 case _4_0_1: 13923 return "FHIR Release 4 (Normative + STU) with 1 technical errata."; 13924 case _4_1: 13925 return "R4B Ballot #1 version."; 13926 case _4_1_0: 13927 return "R4B Ballot #1 + Connectathon 27 (Virtual)."; 13928 case _4_2: 13929 return "R5 Preview #1 version."; 13930 case _4_2_0: 13931 return "R5 Preview #1 + Connectathon 23 (Sydney)."; 13932 case _4_3_0_SNAPSHOT1: 13933 return "FHIR Release 4B Snapshot #1"; 13934 case _4_3_0_CIBUILD: 13935 return "FHIR Release 4B CI-Builld"; 13936 case _4_3: 13937 return "R4B version."; 13938 case _4_3_0: 13939 return "FHIR Release 4B (Normative + STU)."; 13940 case _4_4: 13941 return "R5 Preview #2 version."; 13942 case _4_4_0: 13943 return "R5 Preview #2 + Connectathon 24 (Virtual)."; 13944 case _4_5: 13945 return "R5 Preview #3 version."; 13946 case _4_5_0: 13947 return "R5 Preview #3 + Connectathon 25 (Virtual)."; 13948 case _4_6: 13949 return "R5 Draft Ballot version."; 13950 case _4_6_0: 13951 return "R5 Draft Ballot + Connectathon 27 (Virtual)."; 13952 case _5_0: 13953 return "R5 Versions."; 13954 case _5_0_0: 13955 return "R5 Final Version."; 13956 case _5_0_0CIBUILD: 13957 return "R5 Rolling ci-build."; 13958 case _5_0_0SNAPSHOT1: 13959 return "R5 Preview #2."; 13960 case _5_0_0SNAPSHOT2: 13961 return "R5 Interim tooling stage."; 13962 case _5_0_0BALLOT: 13963 return "R5 Ballot."; 13964 case _5_0_0SNAPSHOT3: 13965 return "R5 Connectathon 32 release."; 13966 case _5_0_0DRAFTFINAL: 13967 return "R5 Final QA."; 13968 case NULL: 13969 return null; 13970 default: 13971 return "?"; 13972 } 13973 } 13974 13975 public String getDisplay() { 13976 switch (this) { 13977 case _0_01: 13978 return "0.01"; 13979 case _0_05: 13980 return "0.05"; 13981 case _0_06: 13982 return "0.06"; 13983 case _0_11: 13984 return "0.11"; 13985 case _0_0: 13986 return "0.0"; 13987 case _0_0_80: 13988 return "0.0.80"; 13989 case _0_0_81: 13990 return "0.0.81"; 13991 case _0_0_82: 13992 return "0.0.82"; 13993 case _0_4: 13994 return "0.4"; 13995 case _0_4_0: 13996 return "0.4.0"; 13997 case _0_5: 13998 return "0.5"; 13999 case _0_5_0: 14000 return "0.5.0"; 14001 case _1_0: 14002 return "1.0"; 14003 case _1_0_0: 14004 return "1.0.0"; 14005 case _1_0_1: 14006 return "1.0.1"; 14007 case _1_0_2: 14008 return "1.0.2"; 14009 case _1_1: 14010 return "1.1"; 14011 case _1_1_0: 14012 return "1.1.0"; 14013 case _1_4: 14014 return "1.4"; 14015 case _1_4_0: 14016 return "1.4.0"; 14017 case _1_6: 14018 return "1.6"; 14019 case _1_6_0: 14020 return "1.6.0"; 14021 case _1_8: 14022 return "1.8"; 14023 case _1_8_0: 14024 return "1.8.0"; 14025 case _3_0: 14026 return "3.0"; 14027 case _3_0_0: 14028 return "3.0.0"; 14029 case _3_0_1: 14030 return "3.0.1"; 14031 case _3_0_2: 14032 return "3.0.2"; 14033 case _3_3: 14034 return "3.3"; 14035 case _3_3_0: 14036 return "3.3.0"; 14037 case _3_5: 14038 return "3.5"; 14039 case _3_5_0: 14040 return "3.5.0"; 14041 case _4_0: 14042 return "4.0"; 14043 case _4_0_0: 14044 return "4.0.0"; 14045 case _4_0_1: 14046 return "4.0.1"; 14047 case _4_1: 14048 return "4.1"; 14049 case _4_1_0: 14050 return "4.1.0"; 14051 case _4_2: 14052 return "4.2"; 14053 case _4_2_0: 14054 return "4.2.0"; 14055 case _4_3: 14056 return "4.3"; 14057 case _4_3_0: 14058 return "4.3.0"; 14059 case _4_3_0_SNAPSHOT1: 14060 return "4.3.0-snapshot"; 14061 case _4_3_0_CIBUILD: 14062 return "4.3.0-cibuild"; 14063 case _4_4: 14064 return "4.4"; 14065 case _4_4_0: 14066 return "4.4.0"; 14067 case _4_5: 14068 return "4.5"; 14069 case _4_5_0: 14070 return "4.5.0"; 14071 case _4_6: 14072 return "4.6"; 14073 case _4_6_0: 14074 return "4.6.0"; 14075 case _5_0: 14076 return "5.0"; 14077 case _5_0_0: 14078 return "5.0.0"; 14079 case _5_0_0CIBUILD: 14080 return "5.0.0-cibuild"; 14081 case _5_0_0SNAPSHOT1: 14082 return "5.0.0-snapshot1"; 14083 case _5_0_0SNAPSHOT2: 14084 return "5.0.0-snapshot2"; 14085 case _5_0_0BALLOT: 14086 return "5.0.0-ballot"; 14087 case _5_0_0SNAPSHOT3: 14088 return "5.0.0-snapshot3"; 14089 case _5_0_0DRAFTFINAL: 14090 return "5.0.0-draft-final"; 14091 case NULL: 14092 return null; 14093 default: 14094 return "?"; 14095 } 14096 } 14097 } 14098 14099 public static class FHIRVersionEnumFactory implements EnumFactory<FHIRVersion> { 14100 public FHIRVersion fromCode(String codeString) throws IllegalArgumentException { 14101 if (codeString == null || "".equals(codeString)) 14102 if (codeString == null || "".equals(codeString)) 14103 return null; 14104 if ("0.01".equals(codeString)) 14105 return FHIRVersion._0_01; 14106 if ("0.05".equals(codeString)) 14107 return FHIRVersion._0_05; 14108 if ("0.06".equals(codeString)) 14109 return FHIRVersion._0_06; 14110 if ("0.11".equals(codeString)) 14111 return FHIRVersion._0_11; 14112 if ("0.0.80".equals(codeString)) 14113 return FHIRVersion._0_0_80; 14114 if ("0.0.81".equals(codeString)) 14115 return FHIRVersion._0_0_81; 14116 if ("0.0.82".equals(codeString)) 14117 return FHIRVersion._0_0_82; 14118 if ("0.4.0".equals(codeString)) 14119 return FHIRVersion._0_4_0; 14120 if ("0.5".equals(codeString)) 14121 return FHIRVersion._0_5; 14122 if ("0.5.0".equals(codeString)) 14123 return FHIRVersion._0_5_0; 14124 if ("1.0".equals(codeString)) 14125 return FHIRVersion._1_0; 14126 if ("1.0.0".equals(codeString)) 14127 return FHIRVersion._1_0_0; 14128 if ("1.0.1".equals(codeString)) 14129 return FHIRVersion._1_0_1; 14130 if ("1.0.2".equals(codeString)) 14131 return FHIRVersion._1_0_2; 14132 if ("1.1".equals(codeString)) 14133 return FHIRVersion._1_1; 14134 if ("1.1.0".equals(codeString)) 14135 return FHIRVersion._1_1_0; 14136 if ("1.4".equals(codeString)) 14137 return FHIRVersion._1_4; 14138 if ("1.4.0".equals(codeString)) 14139 return FHIRVersion._1_4_0; 14140 if ("1.6".equals(codeString)) 14141 return FHIRVersion._1_6; 14142 if ("1.6.0".equals(codeString)) 14143 return FHIRVersion._1_6_0; 14144 if ("1.8".equals(codeString)) 14145 return FHIRVersion._1_8; 14146 if ("1.8.0".equals(codeString)) 14147 return FHIRVersion._1_8_0; 14148 if ("3.0".equals(codeString)) 14149 return FHIRVersion._3_0; 14150 if ("3.0.0".equals(codeString)) 14151 return FHIRVersion._3_0_0; 14152 if ("3.0.1".equals(codeString)) 14153 return FHIRVersion._3_0_1; 14154 if ("3.0.2".equals(codeString)) 14155 return FHIRVersion._3_0_2; 14156 if ("3.3".equals(codeString)) 14157 return FHIRVersion._3_3; 14158 if ("3.3.0".equals(codeString)) 14159 return FHIRVersion._3_3_0; 14160 if ("3.5".equals(codeString)) 14161 return FHIRVersion._3_5; 14162 if ("3.5.0".equals(codeString)) 14163 return FHIRVersion._3_5_0; 14164 if ("4.0".equals(codeString)) 14165 return FHIRVersion._4_0; 14166 if ("4.0.0".equals(codeString)) 14167 return FHIRVersion._4_0_0; 14168 if ("4.0.1".equals(codeString)) 14169 return FHIRVersion._4_0_1; 14170 if ("4.1".equals(codeString)) 14171 return FHIRVersion._4_1; 14172 if ("4.1.0".equals(codeString)) 14173 return FHIRVersion._4_1_0; 14174 if ("4.2".equals(codeString)) 14175 return FHIRVersion._4_2; 14176 if ("4.2.0".equals(codeString)) 14177 return FHIRVersion._4_2_0; 14178 if ("4.3".equals(codeString)) 14179 return FHIRVersion._4_3; 14180 if ("4.3.0".equals(codeString)) 14181 return FHIRVersion._4_3_0; 14182 if ("4.3.0-snapshot1".equalsIgnoreCase(codeString)) 14183 return FHIRVersion._4_3_0_SNAPSHOT1; 14184 if ("4.3.0-cibuild".equalsIgnoreCase(codeString)) 14185 return FHIRVersion._4_3_0_CIBUILD; 14186 if ("4.4".equals(codeString)) 14187 return FHIRVersion._4_4; 14188 if ("4.4.0".equals(codeString)) 14189 return FHIRVersion._4_4_0; 14190 if ("4.5".equals(codeString)) 14191 return FHIRVersion._4_5; 14192 if ("4.5.0".equals(codeString)) 14193 return FHIRVersion._4_5_0; 14194 if ("4.6".equals(codeString)) 14195 return FHIRVersion._4_6; 14196 if ("4.6.0".equals(codeString)) 14197 return FHIRVersion._4_6_0; 14198 if ("5.0".equals(codeString)) 14199 return FHIRVersion._5_0; 14200 if ("5.0.0".equals(codeString)) 14201 return FHIRVersion._5_0_0; 14202 if ("5.0.0-cibuild".equals(codeString)) 14203 return FHIRVersion._5_0_0CIBUILD; 14204 if ("5.0.0-snapshot1".equals(codeString)) 14205 return FHIRVersion._5_0_0SNAPSHOT1; 14206 if ("5.0.0-snapshot2".equals(codeString)) 14207 return FHIRVersion._5_0_0SNAPSHOT2; 14208 if ("5.0.0-ballot".equals(codeString)) 14209 return FHIRVersion._5_0_0BALLOT; 14210 if ("5.0.0-snapshot3".equals(codeString)) 14211 return FHIRVersion._5_0_0SNAPSHOT3; 14212 if ("5.0.0-draft-final".equals(codeString)) 14213 return FHIRVersion._5_0_0DRAFTFINAL; 14214 throw new IllegalArgumentException("Unknown FHIRVersion code '" + codeString + "'"); 14215 } 14216 14217 public Enumeration<FHIRVersion> fromType(PrimitiveType<?> code) throws FHIRException { 14218 if (code == null) 14219 return null; 14220 if (code.isEmpty()) 14221 return new Enumeration<FHIRVersion>(this, FHIRVersion.NULL, code); 14222 String codeString = code.asStringValue(); 14223 if (codeString == null || "".equals(codeString)) 14224 return new Enumeration<FHIRVersion>(this, FHIRVersion.NULL, code); 14225 if ("0.01".equals(codeString)) 14226 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_01, code); 14227 if ("0.05".equals(codeString)) 14228 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_05, code); 14229 if ("0.06".equals(codeString)) 14230 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_06, code); 14231 if ("0.11".equals(codeString)) 14232 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_11, code); 14233 if ("0.0".equals(codeString)) 14234 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0, code); 14235 if ("0.0.80".equals(codeString)) 14236 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_80, code); 14237 if ("0.0.81".equals(codeString)) 14238 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_81, code); 14239 if ("0.0.82".equals(codeString)) 14240 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_0_82, code); 14241 if ("0.4".equals(codeString)) 14242 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_4, code); 14243 if ("0.4.0".equals(codeString)) 14244 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_4_0, code); 14245 if ("0.5".equals(codeString)) 14246 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_5, code); 14247 if ("0.5.0".equals(codeString)) 14248 return new Enumeration<FHIRVersion>(this, FHIRVersion._0_5_0, code); 14249 if ("1.0".equals(codeString)) 14250 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0, code); 14251 if ("1.0.0".equals(codeString)) 14252 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_0, code); 14253 if ("1.0.1".equals(codeString)) 14254 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_1, code); 14255 if ("1.0.2".equals(codeString)) 14256 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_0_2, code); 14257 if ("1.1".equals(codeString)) 14258 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_1, code); 14259 if ("1.1.0".equals(codeString)) 14260 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_1_0, code); 14261 if ("1.4".equals(codeString)) 14262 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_4, code); 14263 if ("1.4.0".equals(codeString)) 14264 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_4_0, code); 14265 if ("1.6".equals(codeString)) 14266 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_6, code); 14267 if ("1.6.0".equals(codeString)) 14268 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_6_0, code); 14269 if ("1.8".equals(codeString)) 14270 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_8, code); 14271 if ("1.8.0".equals(codeString)) 14272 return new Enumeration<FHIRVersion>(this, FHIRVersion._1_8_0, code); 14273 if ("3.0".equals(codeString)) 14274 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0, code); 14275 if ("3.0.0".equals(codeString)) 14276 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_0, code); 14277 if ("3.0.1".equals(codeString)) 14278 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_1, code); 14279 if ("3.0.2".equals(codeString)) 14280 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_0_2, code); 14281 if ("3.3".equals(codeString)) 14282 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_3, code); 14283 if ("3.3.0".equals(codeString)) 14284 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_3_0, code); 14285 if ("3.5".equals(codeString)) 14286 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_5, code); 14287 if ("3.5.0".equals(codeString)) 14288 return new Enumeration<FHIRVersion>(this, FHIRVersion._3_5_0, code); 14289 if ("4.0".equals(codeString)) 14290 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0, code); 14291 if ("4.0.0".equals(codeString)) 14292 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0_0, code); 14293 if ("4.0.1".equals(codeString)) 14294 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_0_1, code); 14295 if ("4.1".equals(codeString)) 14296 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_1, code); 14297 if ("4.1.0".equals(codeString)) 14298 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_1_0, code); 14299 if ("4.2".equals(codeString)) 14300 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_2, code); 14301 if ("4.2.0".equals(codeString)) 14302 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_2_0, code); 14303 if ("4.3".equals(codeString)) 14304 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3, code); 14305 if ("4.3.0".equals(codeString)) 14306 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0, code); 14307 if ("4.3.0-snapshot1".equals(codeString)) 14308 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0_SNAPSHOT1, code); 14309 if ("4.3.0-cibuild".equals(codeString)) 14310 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_3_0_CIBUILD, code); 14311 if ("4.4".equals(codeString)) 14312 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_4, code); 14313 if ("4.4.0".equals(codeString)) 14314 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_4_0, code); 14315 if ("4.5".equals(codeString)) 14316 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_5, code); 14317 if ("4.5.0".equals(codeString)) 14318 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_5_0, code); 14319 if ("4.6".equals(codeString)) 14320 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_6, code); 14321 if ("4.6.0".equals(codeString)) 14322 return new Enumeration<FHIRVersion>(this, FHIRVersion._4_6_0, code); 14323 if ("5.0".equals(codeString)) 14324 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0, code); 14325 if ("5.0.0".equals(codeString)) 14326 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0, code); 14327 if ("5.0.0-cibuild".equals(codeString)) 14328 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0CIBUILD, code); 14329 if ("5.0.0-snapshot1".equals(codeString)) 14330 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT1, code); 14331 if ("5.0.0-snapshot2".equals(codeString)) 14332 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT2, code); 14333 if ("5.0.0-ballot".equals(codeString)) 14334 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0BALLOT, code); 14335 if ("5.0.0-snapshot3".equals(codeString)) 14336 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0SNAPSHOT3, code); 14337 if ("5.0.0-draft-final".equals(codeString)) 14338 return new Enumeration<FHIRVersion>(this, FHIRVersion._5_0_0DRAFTFINAL, code); 14339 throw new FHIRException("Unknown FHIRVersion code '" + codeString + "'"); 14340 } 14341 14342 public String toCode(FHIRVersion code) { 14343 if (code == FHIRVersion._0_01) 14344 return "0.01"; 14345 if (code == FHIRVersion._0_05) 14346 return "0.05"; 14347 if (code == FHIRVersion._0_06) 14348 return "0.06"; 14349 if (code == FHIRVersion._0_11) 14350 return "0.11"; 14351 if (code == FHIRVersion._0_0) 14352 return "0.0"; 14353 if (code == FHIRVersion._0_0_80) 14354 return "0.0.80"; 14355 if (code == FHIRVersion._0_0_81) 14356 return "0.0.81"; 14357 if (code == FHIRVersion._0_0_82) 14358 return "0.0.82"; 14359 if (code == FHIRVersion._0_4) 14360 return "0.4"; 14361 if (code == FHIRVersion._0_4_0) 14362 return "0.4.0"; 14363 if (code == FHIRVersion._0_5) 14364 return "0.5"; 14365 if (code == FHIRVersion._0_5_0) 14366 return "0.5.0"; 14367 if (code == FHIRVersion._1_0) 14368 return "1.0"; 14369 if (code == FHIRVersion._1_0_0) 14370 return "1.0.0"; 14371 if (code == FHIRVersion._1_0_1) 14372 return "1.0.1"; 14373 if (code == FHIRVersion._1_0_2) 14374 return "1.0.2"; 14375 if (code == FHIRVersion._1_1) 14376 return "1.1"; 14377 if (code == FHIRVersion._1_1_0) 14378 return "1.1.0"; 14379 if (code == FHIRVersion._1_4) 14380 return "1.4"; 14381 if (code == FHIRVersion._1_4_0) 14382 return "1.4.0"; 14383 if (code == FHIRVersion._1_6) 14384 return "1.6"; 14385 if (code == FHIRVersion._1_6_0) 14386 return "1.6.0"; 14387 if (code == FHIRVersion._1_8) 14388 return "1.8"; 14389 if (code == FHIRVersion._1_8_0) 14390 return "1.8.0"; 14391 if (code == FHIRVersion._3_0) 14392 return "3.0"; 14393 if (code == FHIRVersion._3_0_0) 14394 return "3.0.0"; 14395 if (code == FHIRVersion._3_0_1) 14396 return "3.0.1"; 14397 if (code == FHIRVersion._3_0_2) 14398 return "3.0.2"; 14399 if (code == FHIRVersion._3_3) 14400 return "3.3"; 14401 if (code == FHIRVersion._3_3_0) 14402 return "3.3.0"; 14403 if (code == FHIRVersion._3_5) 14404 return "3.5"; 14405 if (code == FHIRVersion._3_5_0) 14406 return "3.5.0"; 14407 if (code == FHIRVersion._4_0) 14408 return "4.0"; 14409 if (code == FHIRVersion._4_0_0) 14410 return "4.0.0"; 14411 if (code == FHIRVersion._4_0_1) 14412 return "4.0.1"; 14413 if (code == FHIRVersion._4_1) 14414 return "4.1"; 14415 if (code == FHIRVersion._4_1_0) 14416 return "4.1.0"; 14417 if (code == FHIRVersion._4_2) 14418 return "4.2"; 14419 if (code == FHIRVersion._4_2_0) 14420 return "4.2.0"; 14421 if (code == FHIRVersion._4_3) 14422 return "4.3"; 14423 if (code == FHIRVersion._4_3_0) 14424 return "4.3.0"; 14425 if (code == FHIRVersion._4_3_0_SNAPSHOT1) 14426 return "4.3.0-snapshot1"; 14427 if (code == FHIRVersion._4_3_0_CIBUILD) 14428 return "4.3.0-cibuild"; 14429 if (code == FHIRVersion._4_4) 14430 return "4.4"; 14431 if (code == FHIRVersion._4_4_0) 14432 return "4.4.0"; 14433 if (code == FHIRVersion._4_5) 14434 return "4.5"; 14435 if (code == FHIRVersion._4_5_0) 14436 return "4.5.0"; 14437 if (code == FHIRVersion._4_6) 14438 return "4.6"; 14439 if (code == FHIRVersion._4_6_0) 14440 return "4.6.0"; 14441 if (code == FHIRVersion._5_0) 14442 return "5.0"; 14443 if (code == FHIRVersion._5_0_0) 14444 return "5.0.0"; 14445 if (code == FHIRVersion._5_0_0CIBUILD) 14446 return "5.0.0-cibuild"; 14447 if (code == FHIRVersion._5_0_0SNAPSHOT1) 14448 return "5.0.0-snapshot1"; 14449 if (code == FHIRVersion._5_0_0SNAPSHOT2) 14450 return "5.0.0-snapshot2"; 14451 if (code == FHIRVersion._5_0_0BALLOT) 14452 return "5.0.0-ballot"; 14453 if (code == FHIRVersion._5_0_0SNAPSHOT3) 14454 return "5.0.0-snapshot3"; 14455 if (code == FHIRVersion._5_0_0DRAFTFINAL) 14456 return "5.0.0-draft-final"; 14457 return "?"; 14458 } 14459 14460 public String toSystem(FHIRVersion code) { 14461 return code.getSystem(); 14462 } 14463 } 14464 14465 public enum KnowledgeResourceType { 14466 /** 14467 * The definition of a specific activity to be taken, independent of any 14468 * particular patient or context. 14469 */ 14470 ACTIVITYDEFINITION, 14471 /** 14472 * A set of codes drawn from one or more code systems. 14473 */ 14474 CODESYSTEM, 14475 /** 14476 * A map from one set of concepts to one or more other concepts. 14477 */ 14478 CONCEPTMAP, 14479 /** 14480 * Represents a library of quality improvement components. 14481 */ 14482 LIBRARY, 14483 /** 14484 * A quality measure definition. 14485 */ 14486 MEASURE, 14487 /** 14488 * The definition of a plan for a series of actions, independent of any specific 14489 * patient or context. 14490 */ 14491 PLANDEFINITION, 14492 /** 14493 * Structural Definition. 14494 */ 14495 STRUCTUREDEFINITION, 14496 /** 14497 * A Map of relationships between 2 structures that can be used to transform 14498 * data. 14499 */ 14500 STRUCTUREMAP, 14501 /** 14502 * A set of codes drawn from one or more code systems. 14503 */ 14504 VALUESET, 14505 /** 14506 * added to help the parsers 14507 */ 14508 NULL; 14509 14510 public static KnowledgeResourceType fromCode(String codeString) throws FHIRException { 14511 if (codeString == null || "".equals(codeString)) 14512 return null; 14513 if ("ActivityDefinition".equals(codeString)) 14514 return ACTIVITYDEFINITION; 14515 if ("CodeSystem".equals(codeString)) 14516 return CODESYSTEM; 14517 if ("ConceptMap".equals(codeString)) 14518 return CONCEPTMAP; 14519 if ("Library".equals(codeString)) 14520 return LIBRARY; 14521 if ("Measure".equals(codeString)) 14522 return MEASURE; 14523 if ("PlanDefinition".equals(codeString)) 14524 return PLANDEFINITION; 14525 if ("StructureDefinition".equals(codeString)) 14526 return STRUCTUREDEFINITION; 14527 if ("StructureMap".equals(codeString)) 14528 return STRUCTUREMAP; 14529 if ("ValueSet".equals(codeString)) 14530 return VALUESET; 14531 throw new FHIRException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14532 } 14533 14534 public String toCode() { 14535 switch (this) { 14536 case ACTIVITYDEFINITION: 14537 return "ActivityDefinition"; 14538 case CODESYSTEM: 14539 return "CodeSystem"; 14540 case CONCEPTMAP: 14541 return "ConceptMap"; 14542 case LIBRARY: 14543 return "Library"; 14544 case MEASURE: 14545 return "Measure"; 14546 case PLANDEFINITION: 14547 return "PlanDefinition"; 14548 case STRUCTUREDEFINITION: 14549 return "StructureDefinition"; 14550 case STRUCTUREMAP: 14551 return "StructureMap"; 14552 case VALUESET: 14553 return "ValueSet"; 14554 case NULL: 14555 return null; 14556 default: 14557 return "?"; 14558 } 14559 } 14560 14561 public String getSystem() { 14562 switch (this) { 14563 case ACTIVITYDEFINITION: 14564 return "http://hl7.org/fhir/knowledge-resource-types"; 14565 case CODESYSTEM: 14566 return "http://hl7.org/fhir/knowledge-resource-types"; 14567 case CONCEPTMAP: 14568 return "http://hl7.org/fhir/knowledge-resource-types"; 14569 case LIBRARY: 14570 return "http://hl7.org/fhir/knowledge-resource-types"; 14571 case MEASURE: 14572 return "http://hl7.org/fhir/knowledge-resource-types"; 14573 case PLANDEFINITION: 14574 return "http://hl7.org/fhir/knowledge-resource-types"; 14575 case STRUCTUREDEFINITION: 14576 return "http://hl7.org/fhir/knowledge-resource-types"; 14577 case STRUCTUREMAP: 14578 return "http://hl7.org/fhir/knowledge-resource-types"; 14579 case VALUESET: 14580 return "http://hl7.org/fhir/knowledge-resource-types"; 14581 case NULL: 14582 return null; 14583 default: 14584 return "?"; 14585 } 14586 } 14587 14588 public String getDefinition() { 14589 switch (this) { 14590 case ACTIVITYDEFINITION: 14591 return "The definition of a specific activity to be taken, independent of any particular patient or context."; 14592 case CODESYSTEM: 14593 return "A set of codes drawn from one or more code systems."; 14594 case CONCEPTMAP: 14595 return "A map from one set of concepts to one or more other concepts."; 14596 case LIBRARY: 14597 return "Represents a library of quality improvement components."; 14598 case MEASURE: 14599 return "A quality measure definition."; 14600 case PLANDEFINITION: 14601 return "The definition of a plan for a series of actions, independent of any specific patient or context."; 14602 case STRUCTUREDEFINITION: 14603 return "Structural Definition."; 14604 case STRUCTUREMAP: 14605 return "A Map of relationships between 2 structures that can be used to transform data."; 14606 case VALUESET: 14607 return "A set of codes drawn from one or more code systems."; 14608 case NULL: 14609 return null; 14610 default: 14611 return "?"; 14612 } 14613 } 14614 14615 public String getDisplay() { 14616 switch (this) { 14617 case ACTIVITYDEFINITION: 14618 return "ActivityDefinition"; 14619 case CODESYSTEM: 14620 return "CodeSystem"; 14621 case CONCEPTMAP: 14622 return "ConceptMap"; 14623 case LIBRARY: 14624 return "Library"; 14625 case MEASURE: 14626 return "Measure"; 14627 case PLANDEFINITION: 14628 return "PlanDefinition"; 14629 case STRUCTUREDEFINITION: 14630 return "StructureDefinition"; 14631 case STRUCTUREMAP: 14632 return "StructureMap"; 14633 case VALUESET: 14634 return "ValueSet"; 14635 case NULL: 14636 return null; 14637 default: 14638 return "?"; 14639 } 14640 } 14641 } 14642 14643 public static class KnowledgeResourceTypeEnumFactory implements EnumFactory<KnowledgeResourceType> { 14644 public KnowledgeResourceType fromCode(String codeString) throws IllegalArgumentException { 14645 if (codeString == null || "".equals(codeString)) 14646 if (codeString == null || "".equals(codeString)) 14647 return null; 14648 if ("ActivityDefinition".equals(codeString)) 14649 return KnowledgeResourceType.ACTIVITYDEFINITION; 14650 if ("CodeSystem".equals(codeString)) 14651 return KnowledgeResourceType.CODESYSTEM; 14652 if ("ConceptMap".equals(codeString)) 14653 return KnowledgeResourceType.CONCEPTMAP; 14654 if ("Library".equals(codeString)) 14655 return KnowledgeResourceType.LIBRARY; 14656 if ("Measure".equals(codeString)) 14657 return KnowledgeResourceType.MEASURE; 14658 if ("PlanDefinition".equals(codeString)) 14659 return KnowledgeResourceType.PLANDEFINITION; 14660 if ("StructureDefinition".equals(codeString)) 14661 return KnowledgeResourceType.STRUCTUREDEFINITION; 14662 if ("StructureMap".equals(codeString)) 14663 return KnowledgeResourceType.STRUCTUREMAP; 14664 if ("ValueSet".equals(codeString)) 14665 return KnowledgeResourceType.VALUESET; 14666 throw new IllegalArgumentException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14667 } 14668 14669 public Enumeration<KnowledgeResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 14670 if (code == null) 14671 return null; 14672 if (code.isEmpty()) 14673 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.NULL, code); 14674 String codeString = code.asStringValue(); 14675 if (codeString == null || "".equals(codeString)) 14676 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.NULL, code); 14677 if ("ActivityDefinition".equals(codeString)) 14678 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.ACTIVITYDEFINITION, code); 14679 if ("CodeSystem".equals(codeString)) 14680 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.CODESYSTEM, code); 14681 if ("ConceptMap".equals(codeString)) 14682 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.CONCEPTMAP, code); 14683 if ("Library".equals(codeString)) 14684 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.LIBRARY, code); 14685 if ("Measure".equals(codeString)) 14686 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.MEASURE, code); 14687 if ("PlanDefinition".equals(codeString)) 14688 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.PLANDEFINITION, code); 14689 if ("StructureDefinition".equals(codeString)) 14690 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.STRUCTUREDEFINITION, code); 14691 if ("StructureMap".equals(codeString)) 14692 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.STRUCTUREMAP, code); 14693 if ("ValueSet".equals(codeString)) 14694 return new Enumeration<KnowledgeResourceType>(this, KnowledgeResourceType.VALUESET, code); 14695 throw new FHIRException("Unknown KnowledgeResourceType code '" + codeString + "'"); 14696 } 14697 14698 public String toCode(KnowledgeResourceType code) { 14699 if (code == KnowledgeResourceType.ACTIVITYDEFINITION) 14700 return "ActivityDefinition"; 14701 if (code == KnowledgeResourceType.CODESYSTEM) 14702 return "CodeSystem"; 14703 if (code == KnowledgeResourceType.CONCEPTMAP) 14704 return "ConceptMap"; 14705 if (code == KnowledgeResourceType.LIBRARY) 14706 return "Library"; 14707 if (code == KnowledgeResourceType.MEASURE) 14708 return "Measure"; 14709 if (code == KnowledgeResourceType.PLANDEFINITION) 14710 return "PlanDefinition"; 14711 if (code == KnowledgeResourceType.STRUCTUREDEFINITION) 14712 return "StructureDefinition"; 14713 if (code == KnowledgeResourceType.STRUCTUREMAP) 14714 return "StructureMap"; 14715 if (code == KnowledgeResourceType.VALUESET) 14716 return "ValueSet"; 14717 return "?"; 14718 } 14719 14720 public String toSystem(KnowledgeResourceType code) { 14721 return code.getSystem(); 14722 } 14723 } 14724 14725 public enum MessageEvent { 14726 /** 14727 * added to help the parsers 14728 */ 14729 NULL; 14730 14731 public static MessageEvent fromCode(String codeString) throws FHIRException { 14732 if (codeString == null || "".equals(codeString)) 14733 return null; 14734 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 14735 } 14736 14737 public String toCode() { 14738 switch (this) { 14739 case NULL: 14740 return null; 14741 default: 14742 return "?"; 14743 } 14744 } 14745 14746 public String getSystem() { 14747 switch (this) { 14748 case NULL: 14749 return null; 14750 default: 14751 return "?"; 14752 } 14753 } 14754 14755 public String getDefinition() { 14756 switch (this) { 14757 case NULL: 14758 return null; 14759 default: 14760 return "?"; 14761 } 14762 } 14763 14764 public String getDisplay() { 14765 switch (this) { 14766 case NULL: 14767 return null; 14768 default: 14769 return "?"; 14770 } 14771 } 14772 } 14773 14774 public static class MessageEventEnumFactory implements EnumFactory<MessageEvent> { 14775 public MessageEvent fromCode(String codeString) throws IllegalArgumentException { 14776 if (codeString == null || "".equals(codeString)) 14777 if (codeString == null || "".equals(codeString)) 14778 return null; 14779 throw new IllegalArgumentException("Unknown MessageEvent code '" + codeString + "'"); 14780 } 14781 14782 public Enumeration<MessageEvent> fromType(PrimitiveType<?> code) throws FHIRException { 14783 if (code == null) 14784 return null; 14785 if (code.isEmpty()) 14786 return new Enumeration<MessageEvent>(this, MessageEvent.NULL, code); 14787 String codeString = code.asStringValue(); 14788 if (codeString == null || "".equals(codeString)) 14789 return new Enumeration<MessageEvent>(this, MessageEvent.NULL, code); 14790 throw new FHIRException("Unknown MessageEvent code '" + codeString + "'"); 14791 } 14792 14793 public String toCode(MessageEvent code) { 14794 return "?"; 14795 } 14796 14797 public String toSystem(MessageEvent code) { 14798 return code.getSystem(); 14799 } 14800 } 14801 14802 public enum NoteType { 14803 /** 14804 * Display the note. 14805 */ 14806 DISPLAY, 14807 /** 14808 * Print the note on the form. 14809 */ 14810 PRINT, 14811 /** 14812 * Print the note for the operator. 14813 */ 14814 PRINTOPER, 14815 /** 14816 * added to help the parsers 14817 */ 14818 NULL; 14819 14820 public static NoteType fromCode(String codeString) throws FHIRException { 14821 if (codeString == null || "".equals(codeString)) 14822 return null; 14823 if ("display".equals(codeString)) 14824 return DISPLAY; 14825 if ("print".equals(codeString)) 14826 return PRINT; 14827 if ("printoper".equals(codeString)) 14828 return PRINTOPER; 14829 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 14830 } 14831 14832 public String toCode() { 14833 switch (this) { 14834 case DISPLAY: 14835 return "display"; 14836 case PRINT: 14837 return "print"; 14838 case PRINTOPER: 14839 return "printoper"; 14840 case NULL: 14841 return null; 14842 default: 14843 return "?"; 14844 } 14845 } 14846 14847 public String getSystem() { 14848 switch (this) { 14849 case DISPLAY: 14850 return "http://hl7.org/fhir/note-type"; 14851 case PRINT: 14852 return "http://hl7.org/fhir/note-type"; 14853 case PRINTOPER: 14854 return "http://hl7.org/fhir/note-type"; 14855 case NULL: 14856 return null; 14857 default: 14858 return "?"; 14859 } 14860 } 14861 14862 public String getDefinition() { 14863 switch (this) { 14864 case DISPLAY: 14865 return "Display the note."; 14866 case PRINT: 14867 return "Print the note on the form."; 14868 case PRINTOPER: 14869 return "Print the note for the operator."; 14870 case NULL: 14871 return null; 14872 default: 14873 return "?"; 14874 } 14875 } 14876 14877 public String getDisplay() { 14878 switch (this) { 14879 case DISPLAY: 14880 return "Display"; 14881 case PRINT: 14882 return "Print (Form)"; 14883 case PRINTOPER: 14884 return "Print (Operator)"; 14885 case NULL: 14886 return null; 14887 default: 14888 return "?"; 14889 } 14890 } 14891 } 14892 14893 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 14894 public NoteType fromCode(String codeString) throws IllegalArgumentException { 14895 if (codeString == null || "".equals(codeString)) 14896 if (codeString == null || "".equals(codeString)) 14897 return null; 14898 if ("display".equals(codeString)) 14899 return NoteType.DISPLAY; 14900 if ("print".equals(codeString)) 14901 return NoteType.PRINT; 14902 if ("printoper".equals(codeString)) 14903 return NoteType.PRINTOPER; 14904 throw new IllegalArgumentException("Unknown NoteType code '" + codeString + "'"); 14905 } 14906 14907 public Enumeration<NoteType> fromType(PrimitiveType<?> code) throws FHIRException { 14908 if (code == null) 14909 return null; 14910 if (code.isEmpty()) 14911 return new Enumeration<NoteType>(this, NoteType.NULL, code); 14912 String codeString = code.asStringValue(); 14913 if (codeString == null || "".equals(codeString)) 14914 return new Enumeration<NoteType>(this, NoteType.NULL, code); 14915 if ("display".equals(codeString)) 14916 return new Enumeration<NoteType>(this, NoteType.DISPLAY, code); 14917 if ("print".equals(codeString)) 14918 return new Enumeration<NoteType>(this, NoteType.PRINT, code); 14919 if ("printoper".equals(codeString)) 14920 return new Enumeration<NoteType>(this, NoteType.PRINTOPER, code); 14921 throw new FHIRException("Unknown NoteType code '" + codeString + "'"); 14922 } 14923 14924 public String toCode(NoteType code) { 14925 if (code == NoteType.DISPLAY) 14926 return "display"; 14927 if (code == NoteType.PRINT) 14928 return "print"; 14929 if (code == NoteType.PRINTOPER) 14930 return "printoper"; 14931 return "?"; 14932 } 14933 14934 public String toSystem(NoteType code) { 14935 return code.getSystem(); 14936 } 14937 } 14938 14939 public enum PublicationStatus { 14940 /** 14941 * This resource is still under development and is not yet considered to be 14942 * ready for normal use. 14943 */ 14944 DRAFT, 14945 /** 14946 * This resource is ready for normal use. 14947 */ 14948 ACTIVE, 14949 /** 14950 * This resource has been withdrawn or superseded and should no longer be used. 14951 */ 14952 RETIRED, 14953 /** 14954 * The authoring system does not know which of the status values currently 14955 * applies for this resource. Note: This concept is not to be used for "other" - 14956 * one of the listed statuses is presumed to apply, it's just not known which 14957 * one. 14958 */ 14959 UNKNOWN, 14960 /** 14961 * added to help the parsers 14962 */ 14963 NULL; 14964 14965 public static PublicationStatus fromCode(String codeString) throws FHIRException { 14966 if (codeString == null || "".equals(codeString)) 14967 return null; 14968 if ("draft".equals(codeString)) 14969 return DRAFT; 14970 if ("active".equals(codeString)) 14971 return ACTIVE; 14972 if ("retired".equals(codeString)) 14973 return RETIRED; 14974 if ("unknown".equals(codeString)) 14975 return UNKNOWN; 14976 throw new FHIRException("Unknown PublicationStatus code '" + codeString + "'"); 14977 } 14978 14979 public String toCode() { 14980 switch (this) { 14981 case DRAFT: 14982 return "draft"; 14983 case ACTIVE: 14984 return "active"; 14985 case RETIRED: 14986 return "retired"; 14987 case UNKNOWN: 14988 return "unknown"; 14989 case NULL: 14990 return null; 14991 default: 14992 return "?"; 14993 } 14994 } 14995 14996 public String getSystem() { 14997 switch (this) { 14998 case DRAFT: 14999 return "http://hl7.org/fhir/publication-status"; 15000 case ACTIVE: 15001 return "http://hl7.org/fhir/publication-status"; 15002 case RETIRED: 15003 return "http://hl7.org/fhir/publication-status"; 15004 case UNKNOWN: 15005 return "http://hl7.org/fhir/publication-status"; 15006 case NULL: 15007 return null; 15008 default: 15009 return "?"; 15010 } 15011 } 15012 15013 public String getDefinition() { 15014 switch (this) { 15015 case DRAFT: 15016 return "This resource is still under development and is not yet considered to be ready for normal use."; 15017 case ACTIVE: 15018 return "This resource is ready for normal use."; 15019 case RETIRED: 15020 return "This resource has been withdrawn or superseded and should no longer be used."; 15021 case UNKNOWN: 15022 return "The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 15023 case NULL: 15024 return null; 15025 default: 15026 return "?"; 15027 } 15028 } 15029 15030 public String getDisplay() { 15031 switch (this) { 15032 case DRAFT: 15033 return "Draft"; 15034 case ACTIVE: 15035 return "Active"; 15036 case RETIRED: 15037 return "Retired"; 15038 case UNKNOWN: 15039 return "Unknown"; 15040 case NULL: 15041 return null; 15042 default: 15043 return "?"; 15044 } 15045 } 15046 } 15047 15048 public static class PublicationStatusEnumFactory implements EnumFactory<PublicationStatus> { 15049 public PublicationStatus fromCode(String codeString) throws IllegalArgumentException { 15050 if (codeString == null || "".equals(codeString)) 15051 if (codeString == null || "".equals(codeString)) 15052 return null; 15053 if ("draft".equals(codeString)) 15054 return PublicationStatus.DRAFT; 15055 if ("active".equals(codeString)) 15056 return PublicationStatus.ACTIVE; 15057 if ("retired".equals(codeString)) 15058 return PublicationStatus.RETIRED; 15059 if ("unknown".equals(codeString)) 15060 return PublicationStatus.UNKNOWN; 15061 throw new IllegalArgumentException("Unknown PublicationStatus code '" + codeString + "'"); 15062 } 15063 15064 public Enumeration<PublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 15065 if (code == null) 15066 return null; 15067 if (code.isEmpty()) 15068 return new Enumeration<PublicationStatus>(this, PublicationStatus.NULL, code); 15069 String codeString = code.asStringValue(); 15070 if (codeString == null || "".equals(codeString)) 15071 return new Enumeration<PublicationStatus>(this, PublicationStatus.NULL, code); 15072 if ("draft".equals(codeString)) 15073 return new Enumeration<PublicationStatus>(this, PublicationStatus.DRAFT, code); 15074 if ("active".equals(codeString)) 15075 return new Enumeration<PublicationStatus>(this, PublicationStatus.ACTIVE, code); 15076 if ("retired".equals(codeString)) 15077 return new Enumeration<PublicationStatus>(this, PublicationStatus.RETIRED, code); 15078 if ("unknown".equals(codeString)) 15079 return new Enumeration<PublicationStatus>(this, PublicationStatus.UNKNOWN, code); 15080 throw new FHIRException("Unknown PublicationStatus code '" + codeString + "'"); 15081 } 15082 15083 public String toCode(PublicationStatus code) { 15084 if (code == PublicationStatus.DRAFT) 15085 return "draft"; 15086 if (code == PublicationStatus.ACTIVE) 15087 return "active"; 15088 if (code == PublicationStatus.RETIRED) 15089 return "retired"; 15090 if (code == PublicationStatus.UNKNOWN) 15091 return "unknown"; 15092 return "?"; 15093 } 15094 15095 public String toSystem(PublicationStatus code) { 15096 return code.getSystem(); 15097 } 15098 } 15099 15100 public enum RemittanceOutcome { 15101 /** 15102 * The Claim/Pre-authorization/Pre-determination has been received but 15103 * processing has not begun. 15104 */ 15105 QUEUED, 15106 /** 15107 * The processing has completed without errors 15108 */ 15109 COMPLETE, 15110 /** 15111 * One or more errors have been detected in the Claim 15112 */ 15113 ERROR, 15114 /** 15115 * No errors have been detected in the Claim and some of the adjudication has 15116 * been performed. 15117 */ 15118 PARTIAL, 15119 /** 15120 * added to help the parsers 15121 */ 15122 NULL; 15123 15124 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 15125 if (codeString == null || "".equals(codeString)) 15126 return null; 15127 if ("queued".equals(codeString)) 15128 return QUEUED; 15129 if ("complete".equals(codeString)) 15130 return COMPLETE; 15131 if ("error".equals(codeString)) 15132 return ERROR; 15133 if ("partial".equals(codeString)) 15134 return PARTIAL; 15135 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 15136 } 15137 15138 public String toCode() { 15139 switch (this) { 15140 case QUEUED: 15141 return "queued"; 15142 case COMPLETE: 15143 return "complete"; 15144 case ERROR: 15145 return "error"; 15146 case PARTIAL: 15147 return "partial"; 15148 case NULL: 15149 return null; 15150 default: 15151 return "?"; 15152 } 15153 } 15154 15155 public String getSystem() { 15156 switch (this) { 15157 case QUEUED: 15158 return "http://hl7.org/fhir/remittance-outcome"; 15159 case COMPLETE: 15160 return "http://hl7.org/fhir/remittance-outcome"; 15161 case ERROR: 15162 return "http://hl7.org/fhir/remittance-outcome"; 15163 case PARTIAL: 15164 return "http://hl7.org/fhir/remittance-outcome"; 15165 case NULL: 15166 return null; 15167 default: 15168 return "?"; 15169 } 15170 } 15171 15172 public String getDefinition() { 15173 switch (this) { 15174 case QUEUED: 15175 return "The Claim/Pre-authorization/Pre-determination has been received but processing has not begun."; 15176 case COMPLETE: 15177 return "The processing has completed without errors"; 15178 case ERROR: 15179 return "One or more errors have been detected in the Claim"; 15180 case PARTIAL: 15181 return "No errors have been detected in the Claim and some of the adjudication has been performed."; 15182 case NULL: 15183 return null; 15184 default: 15185 return "?"; 15186 } 15187 } 15188 15189 public String getDisplay() { 15190 switch (this) { 15191 case QUEUED: 15192 return "Queued"; 15193 case COMPLETE: 15194 return "Processing Complete"; 15195 case ERROR: 15196 return "Error"; 15197 case PARTIAL: 15198 return "Partial Processing"; 15199 case NULL: 15200 return null; 15201 default: 15202 return "?"; 15203 } 15204 } 15205 } 15206 15207 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 15208 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 15209 if (codeString == null || "".equals(codeString)) 15210 if (codeString == null || "".equals(codeString)) 15211 return null; 15212 if ("queued".equals(codeString)) 15213 return RemittanceOutcome.QUEUED; 15214 if ("complete".equals(codeString)) 15215 return RemittanceOutcome.COMPLETE; 15216 if ("error".equals(codeString)) 15217 return RemittanceOutcome.ERROR; 15218 if ("partial".equals(codeString)) 15219 return RemittanceOutcome.PARTIAL; 15220 throw new IllegalArgumentException("Unknown RemittanceOutcome code '" + codeString + "'"); 15221 } 15222 15223 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 15224 if (code == null) 15225 return null; 15226 if (code.isEmpty()) 15227 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 15228 String codeString = code.asStringValue(); 15229 if (codeString == null || "".equals(codeString)) 15230 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.NULL, code); 15231 if ("queued".equals(codeString)) 15232 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.QUEUED, code); 15233 if ("complete".equals(codeString)) 15234 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE, code); 15235 if ("error".equals(codeString)) 15236 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR, code); 15237 if ("partial".equals(codeString)) 15238 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL, code); 15239 throw new FHIRException("Unknown RemittanceOutcome code '" + codeString + "'"); 15240 } 15241 15242 public String toCode(RemittanceOutcome code) { 15243 if (code == RemittanceOutcome.QUEUED) 15244 return "queued"; 15245 if (code == RemittanceOutcome.COMPLETE) 15246 return "complete"; 15247 if (code == RemittanceOutcome.ERROR) 15248 return "error"; 15249 if (code == RemittanceOutcome.PARTIAL) 15250 return "partial"; 15251 return "?"; 15252 } 15253 15254 public String toSystem(RemittanceOutcome code) { 15255 return code.getSystem(); 15256 } 15257 } 15258 15259 public enum RequestResourceType { 15260 /** 15261 * A booking of a healthcare event among patient(s), practitioner(s), related 15262 * person(s) and/or device(s) for a specific date/time. This may result in one 15263 * or more Encounter(s). 15264 */ 15265 APPOINTMENT, 15266 /** 15267 * A reply to an appointment request for a patient and/or practitioner(s), such 15268 * as a confirmation or rejection. 15269 */ 15270 APPOINTMENTRESPONSE, 15271 /** 15272 * Healthcare plan for patient or group. 15273 */ 15274 CAREPLAN, 15275 /** 15276 * Claim, Pre-determination or Pre-authorization. 15277 */ 15278 CLAIM, 15279 /** 15280 * A request for information to be sent to a receiver. 15281 */ 15282 COMMUNICATIONREQUEST, 15283 /** 15284 * Legal Agreement. 15285 */ 15286 CONTRACT, 15287 /** 15288 * Medical device request. 15289 */ 15290 DEVICEREQUEST, 15291 /** 15292 * Enrollment request. 15293 */ 15294 ENROLLMENTREQUEST, 15295 /** 15296 * Guidance or advice relating to an immunization. 15297 */ 15298 IMMUNIZATIONRECOMMENDATION, 15299 /** 15300 * Ordering of medication for patient or group. 15301 */ 15302 MEDICATIONREQUEST, 15303 /** 15304 * Diet, formula or nutritional supplement request. 15305 */ 15306 NUTRITIONORDER, 15307 /** 15308 * A record of a request for service such as diagnostic investigations, 15309 * treatments, or operations to be performed. 15310 */ 15311 SERVICEREQUEST, 15312 /** 15313 * Request for a medication, substance or device. 15314 */ 15315 SUPPLYREQUEST, 15316 /** 15317 * A task to be performed. 15318 */ 15319 TASK, 15320 /** 15321 * Prescription for vision correction products for a patient. 15322 */ 15323 VISIONPRESCRIPTION, 15324 /** 15325 * added to help the parsers 15326 */ 15327 NULL; 15328 15329 public static RequestResourceType fromCode(String codeString) throws FHIRException { 15330 if (codeString == null || "".equals(codeString)) 15331 return null; 15332 if ("Appointment".equals(codeString)) 15333 return APPOINTMENT; 15334 if ("AppointmentResponse".equals(codeString)) 15335 return APPOINTMENTRESPONSE; 15336 if ("CarePlan".equals(codeString)) 15337 return CAREPLAN; 15338 if ("Claim".equals(codeString)) 15339 return CLAIM; 15340 if ("CommunicationRequest".equals(codeString)) 15341 return COMMUNICATIONREQUEST; 15342 if ("Contract".equals(codeString)) 15343 return CONTRACT; 15344 if ("DeviceRequest".equals(codeString)) 15345 return DEVICEREQUEST; 15346 if ("EnrollmentRequest".equals(codeString)) 15347 return ENROLLMENTREQUEST; 15348 if ("ImmunizationRecommendation".equals(codeString)) 15349 return IMMUNIZATIONRECOMMENDATION; 15350 if ("MedicationRequest".equals(codeString)) 15351 return MEDICATIONREQUEST; 15352 if ("NutritionOrder".equals(codeString)) 15353 return NUTRITIONORDER; 15354 if ("ServiceRequest".equals(codeString)) 15355 return SERVICEREQUEST; 15356 if ("SupplyRequest".equals(codeString)) 15357 return SUPPLYREQUEST; 15358 if ("Task".equals(codeString)) 15359 return TASK; 15360 if ("VisionPrescription".equals(codeString)) 15361 return VISIONPRESCRIPTION; 15362 throw new FHIRException("Unknown RequestResourceType code '" + codeString + "'"); 15363 } 15364 15365 public String toCode() { 15366 switch (this) { 15367 case APPOINTMENT: 15368 return "Appointment"; 15369 case APPOINTMENTRESPONSE: 15370 return "AppointmentResponse"; 15371 case CAREPLAN: 15372 return "CarePlan"; 15373 case CLAIM: 15374 return "Claim"; 15375 case COMMUNICATIONREQUEST: 15376 return "CommunicationRequest"; 15377 case CONTRACT: 15378 return "Contract"; 15379 case DEVICEREQUEST: 15380 return "DeviceRequest"; 15381 case ENROLLMENTREQUEST: 15382 return "EnrollmentRequest"; 15383 case IMMUNIZATIONRECOMMENDATION: 15384 return "ImmunizationRecommendation"; 15385 case MEDICATIONREQUEST: 15386 return "MedicationRequest"; 15387 case NUTRITIONORDER: 15388 return "NutritionOrder"; 15389 case SERVICEREQUEST: 15390 return "ServiceRequest"; 15391 case SUPPLYREQUEST: 15392 return "SupplyRequest"; 15393 case TASK: 15394 return "Task"; 15395 case VISIONPRESCRIPTION: 15396 return "VisionPrescription"; 15397 case NULL: 15398 return null; 15399 default: 15400 return "?"; 15401 } 15402 } 15403 15404 public String getSystem() { 15405 switch (this) { 15406 case APPOINTMENT: 15407 return "http://hl7.org/fhir/request-resource-types"; 15408 case APPOINTMENTRESPONSE: 15409 return "http://hl7.org/fhir/request-resource-types"; 15410 case CAREPLAN: 15411 return "http://hl7.org/fhir/request-resource-types"; 15412 case CLAIM: 15413 return "http://hl7.org/fhir/request-resource-types"; 15414 case COMMUNICATIONREQUEST: 15415 return "http://hl7.org/fhir/request-resource-types"; 15416 case CONTRACT: 15417 return "http://hl7.org/fhir/request-resource-types"; 15418 case DEVICEREQUEST: 15419 return "http://hl7.org/fhir/request-resource-types"; 15420 case ENROLLMENTREQUEST: 15421 return "http://hl7.org/fhir/request-resource-types"; 15422 case IMMUNIZATIONRECOMMENDATION: 15423 return "http://hl7.org/fhir/request-resource-types"; 15424 case MEDICATIONREQUEST: 15425 return "http://hl7.org/fhir/request-resource-types"; 15426 case NUTRITIONORDER: 15427 return "http://hl7.org/fhir/request-resource-types"; 15428 case SERVICEREQUEST: 15429 return "http://hl7.org/fhir/request-resource-types"; 15430 case SUPPLYREQUEST: 15431 return "http://hl7.org/fhir/request-resource-types"; 15432 case TASK: 15433 return "http://hl7.org/fhir/request-resource-types"; 15434 case VISIONPRESCRIPTION: 15435 return "http://hl7.org/fhir/request-resource-types"; 15436 case NULL: 15437 return null; 15438 default: 15439 return "?"; 15440 } 15441 } 15442 15443 public String getDefinition() { 15444 switch (this) { 15445 case APPOINTMENT: 15446 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 15447 case APPOINTMENTRESPONSE: 15448 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 15449 case CAREPLAN: 15450 return "Healthcare plan for patient or group."; 15451 case CLAIM: 15452 return "Claim, Pre-determination or Pre-authorization."; 15453 case COMMUNICATIONREQUEST: 15454 return "A request for information to be sent to a receiver."; 15455 case CONTRACT: 15456 return "Legal Agreement."; 15457 case DEVICEREQUEST: 15458 return "Medical device request."; 15459 case ENROLLMENTREQUEST: 15460 return "Enrollment request."; 15461 case IMMUNIZATIONRECOMMENDATION: 15462 return "Guidance or advice relating to an immunization."; 15463 case MEDICATIONREQUEST: 15464 return "Ordering of medication for patient or group."; 15465 case NUTRITIONORDER: 15466 return "Diet, formula or nutritional supplement request."; 15467 case SERVICEREQUEST: 15468 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 15469 case SUPPLYREQUEST: 15470 return "Request for a medication, substance or device."; 15471 case TASK: 15472 return "A task to be performed."; 15473 case VISIONPRESCRIPTION: 15474 return "Prescription for vision correction products for a patient."; 15475 case NULL: 15476 return null; 15477 default: 15478 return "?"; 15479 } 15480 } 15481 15482 public String getDisplay() { 15483 switch (this) { 15484 case APPOINTMENT: 15485 return "Appointment"; 15486 case APPOINTMENTRESPONSE: 15487 return "AppointmentResponse"; 15488 case CAREPLAN: 15489 return "CarePlan"; 15490 case CLAIM: 15491 return "Claim"; 15492 case COMMUNICATIONREQUEST: 15493 return "CommunicationRequest"; 15494 case CONTRACT: 15495 return "Contract"; 15496 case DEVICEREQUEST: 15497 return "DeviceRequest"; 15498 case ENROLLMENTREQUEST: 15499 return "EnrollmentRequest"; 15500 case IMMUNIZATIONRECOMMENDATION: 15501 return "ImmunizationRecommendation"; 15502 case MEDICATIONREQUEST: 15503 return "MedicationRequest"; 15504 case NUTRITIONORDER: 15505 return "NutritionOrder"; 15506 case SERVICEREQUEST: 15507 return "ServiceRequest"; 15508 case SUPPLYREQUEST: 15509 return "SupplyRequest"; 15510 case TASK: 15511 return "Task"; 15512 case VISIONPRESCRIPTION: 15513 return "VisionPrescription"; 15514 case NULL: 15515 return null; 15516 default: 15517 return "?"; 15518 } 15519 } 15520 } 15521 15522 public static class RequestResourceTypeEnumFactory implements EnumFactory<RequestResourceType> { 15523 public RequestResourceType fromCode(String codeString) throws IllegalArgumentException { 15524 if (codeString == null || "".equals(codeString)) 15525 if (codeString == null || "".equals(codeString)) 15526 return null; 15527 if ("Appointment".equals(codeString)) 15528 return RequestResourceType.APPOINTMENT; 15529 if ("AppointmentResponse".equals(codeString)) 15530 return RequestResourceType.APPOINTMENTRESPONSE; 15531 if ("CarePlan".equals(codeString)) 15532 return RequestResourceType.CAREPLAN; 15533 if ("Claim".equals(codeString)) 15534 return RequestResourceType.CLAIM; 15535 if ("CommunicationRequest".equals(codeString)) 15536 return RequestResourceType.COMMUNICATIONREQUEST; 15537 if ("Contract".equals(codeString)) 15538 return RequestResourceType.CONTRACT; 15539 if ("DeviceRequest".equals(codeString)) 15540 return RequestResourceType.DEVICEREQUEST; 15541 if ("EnrollmentRequest".equals(codeString)) 15542 return RequestResourceType.ENROLLMENTREQUEST; 15543 if ("ImmunizationRecommendation".equals(codeString)) 15544 return RequestResourceType.IMMUNIZATIONRECOMMENDATION; 15545 if ("MedicationRequest".equals(codeString)) 15546 return RequestResourceType.MEDICATIONREQUEST; 15547 if ("NutritionOrder".equals(codeString)) 15548 return RequestResourceType.NUTRITIONORDER; 15549 if ("ServiceRequest".equals(codeString)) 15550 return RequestResourceType.SERVICEREQUEST; 15551 if ("SupplyRequest".equals(codeString)) 15552 return RequestResourceType.SUPPLYREQUEST; 15553 if ("Task".equals(codeString)) 15554 return RequestResourceType.TASK; 15555 if ("VisionPrescription".equals(codeString)) 15556 return RequestResourceType.VISIONPRESCRIPTION; 15557 throw new IllegalArgumentException("Unknown RequestResourceType code '" + codeString + "'"); 15558 } 15559 15560 public Enumeration<RequestResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 15561 if (code == null) 15562 return null; 15563 if (code.isEmpty()) 15564 return new Enumeration<RequestResourceType>(this, RequestResourceType.NULL, code); 15565 String codeString = code.asStringValue(); 15566 if (codeString == null || "".equals(codeString)) 15567 return new Enumeration<RequestResourceType>(this, RequestResourceType.NULL, code); 15568 if ("Appointment".equals(codeString)) 15569 return new Enumeration<RequestResourceType>(this, RequestResourceType.APPOINTMENT, code); 15570 if ("AppointmentResponse".equals(codeString)) 15571 return new Enumeration<RequestResourceType>(this, RequestResourceType.APPOINTMENTRESPONSE, code); 15572 if ("CarePlan".equals(codeString)) 15573 return new Enumeration<RequestResourceType>(this, RequestResourceType.CAREPLAN, code); 15574 if ("Claim".equals(codeString)) 15575 return new Enumeration<RequestResourceType>(this, RequestResourceType.CLAIM, code); 15576 if ("CommunicationRequest".equals(codeString)) 15577 return new Enumeration<RequestResourceType>(this, RequestResourceType.COMMUNICATIONREQUEST, code); 15578 if ("Contract".equals(codeString)) 15579 return new Enumeration<RequestResourceType>(this, RequestResourceType.CONTRACT, code); 15580 if ("DeviceRequest".equals(codeString)) 15581 return new Enumeration<RequestResourceType>(this, RequestResourceType.DEVICEREQUEST, code); 15582 if ("EnrollmentRequest".equals(codeString)) 15583 return new Enumeration<RequestResourceType>(this, RequestResourceType.ENROLLMENTREQUEST, code); 15584 if ("ImmunizationRecommendation".equals(codeString)) 15585 return new Enumeration<RequestResourceType>(this, RequestResourceType.IMMUNIZATIONRECOMMENDATION, code); 15586 if ("MedicationRequest".equals(codeString)) 15587 return new Enumeration<RequestResourceType>(this, RequestResourceType.MEDICATIONREQUEST, code); 15588 if ("NutritionOrder".equals(codeString)) 15589 return new Enumeration<RequestResourceType>(this, RequestResourceType.NUTRITIONORDER, code); 15590 if ("ServiceRequest".equals(codeString)) 15591 return new Enumeration<RequestResourceType>(this, RequestResourceType.SERVICEREQUEST, code); 15592 if ("SupplyRequest".equals(codeString)) 15593 return new Enumeration<RequestResourceType>(this, RequestResourceType.SUPPLYREQUEST, code); 15594 if ("Task".equals(codeString)) 15595 return new Enumeration<RequestResourceType>(this, RequestResourceType.TASK, code); 15596 if ("VisionPrescription".equals(codeString)) 15597 return new Enumeration<RequestResourceType>(this, RequestResourceType.VISIONPRESCRIPTION, code); 15598 throw new FHIRException("Unknown RequestResourceType code '" + codeString + "'"); 15599 } 15600 15601 public String toCode(RequestResourceType code) { 15602 if (code == RequestResourceType.APPOINTMENT) 15603 return "Appointment"; 15604 if (code == RequestResourceType.APPOINTMENTRESPONSE) 15605 return "AppointmentResponse"; 15606 if (code == RequestResourceType.CAREPLAN) 15607 return "CarePlan"; 15608 if (code == RequestResourceType.CLAIM) 15609 return "Claim"; 15610 if (code == RequestResourceType.COMMUNICATIONREQUEST) 15611 return "CommunicationRequest"; 15612 if (code == RequestResourceType.CONTRACT) 15613 return "Contract"; 15614 if (code == RequestResourceType.DEVICEREQUEST) 15615 return "DeviceRequest"; 15616 if (code == RequestResourceType.ENROLLMENTREQUEST) 15617 return "EnrollmentRequest"; 15618 if (code == RequestResourceType.IMMUNIZATIONRECOMMENDATION) 15619 return "ImmunizationRecommendation"; 15620 if (code == RequestResourceType.MEDICATIONREQUEST) 15621 return "MedicationRequest"; 15622 if (code == RequestResourceType.NUTRITIONORDER) 15623 return "NutritionOrder"; 15624 if (code == RequestResourceType.SERVICEREQUEST) 15625 return "ServiceRequest"; 15626 if (code == RequestResourceType.SUPPLYREQUEST) 15627 return "SupplyRequest"; 15628 if (code == RequestResourceType.TASK) 15629 return "Task"; 15630 if (code == RequestResourceType.VISIONPRESCRIPTION) 15631 return "VisionPrescription"; 15632 return "?"; 15633 } 15634 15635 public String toSystem(RequestResourceType code) { 15636 return code.getSystem(); 15637 } 15638 } 15639 15640 public enum ResourceType { 15641 /** 15642 * A financial tool for tracking value accrued for a particular purpose. In the 15643 * healthcare field, used to track charges for a patient, cost centers, etc. 15644 */ 15645 ACCOUNT, 15646 /** 15647 * This resource allows for the definition of some activity to be performed, 15648 * independent of a particular patient, practitioner, or other performance 15649 * context. 15650 */ 15651 ACTIVITYDEFINITION, 15652 /** 15653 * Actual or potential/avoided event causing unintended physical injury 15654 * resulting from or contributed to by medical care, a research study or other 15655 * healthcare setting factors that requires additional monitoring, treatment, or 15656 * hospitalization, or that results in death. 15657 */ 15658 ADVERSEEVENT, 15659 /** 15660 * Risk of harmful or undesirable, physiological response which is unique to an 15661 * individual and associated with exposure to a substance. 15662 */ 15663 ALLERGYINTOLERANCE, 15664 /** 15665 * A booking of a healthcare event among patient(s), practitioner(s), related 15666 * person(s) and/or device(s) for a specific date/time. This may result in one 15667 * or more Encounter(s). 15668 */ 15669 APPOINTMENT, 15670 /** 15671 * A reply to an appointment request for a patient and/or practitioner(s), such 15672 * as a confirmation or rejection. 15673 */ 15674 APPOINTMENTRESPONSE, 15675 /** 15676 * A record of an event made for purposes of maintaining a security log. Typical 15677 * uses include detection of intrusion attempts and monitoring for inappropriate 15678 * usage. 15679 */ 15680 AUDITEVENT, 15681 /** 15682 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 15683 * resources that don't map to an existing resource, and custom resources not 15684 * appropriate for inclusion in the FHIR specification. 15685 */ 15686 BASIC, 15687 /** 15688 * A resource that represents the data of a single raw artifact as digital 15689 * content accessible in its native format. A Binary resource can contain any 15690 * content, whether text, image, pdf, zip archive, etc. 15691 */ 15692 BINARY, 15693 /** 15694 * A material substance originating from a biological entity intended to be 15695 * transplanted or infused into another (possibly the same) biological entity. 15696 */ 15697 BIOLOGICALLYDERIVEDPRODUCT, 15698 /** 15699 * Record details about an anatomical structure. This resource may be used when 15700 * a coded concept does not provide the necessary detail needed for the use 15701 * case. 15702 */ 15703 BODYSTRUCTURE, 15704 /** 15705 * A container for a collection of resources. 15706 */ 15707 BUNDLE, 15708 /** 15709 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 15710 * Server for a particular version of FHIR that may be used as a statement of 15711 * actual server functionality or a statement of required or desired server 15712 * implementation. 15713 */ 15714 CAPABILITYSTATEMENT, 15715 /** 15716 * Describes the intention of how one or more practitioners intend to deliver 15717 * care for a particular patient, group or community for a period of time, 15718 * possibly limited to care for a specific condition or set of conditions. 15719 */ 15720 CAREPLAN, 15721 /** 15722 * The Care Team includes all the people and organizations who plan to 15723 * participate in the coordination and delivery of care for a patient. 15724 */ 15725 CARETEAM, 15726 /** 15727 * Catalog entries are wrappers that contextualize items included in a catalog. 15728 */ 15729 CATALOGENTRY, 15730 /** 15731 * The resource ChargeItem describes the provision of healthcare provider 15732 * products for a certain patient, therefore referring not only to the product, 15733 * but containing in addition details of the provision, like date, time, amounts 15734 * and participating organizations and persons. Main Usage of the ChargeItem is 15735 * to enable the billing process and internal cost allocation. 15736 */ 15737 CHARGEITEM, 15738 /** 15739 * The ChargeItemDefinition resource provides the properties that apply to the 15740 * (billing) codes necessary to calculate costs and prices. The properties may 15741 * differ largely depending on type and realm, therefore this resource gives 15742 * only a rough structure and requires profiling for each type of billing code 15743 * system. 15744 */ 15745 CHARGEITEMDEFINITION, 15746 /** 15747 * A provider issued list of professional services and products which have been 15748 * provided, or are to be provided, to a patient which is sent to an insurer for 15749 * reimbursement. 15750 */ 15751 CLAIM, 15752 /** 15753 * This resource provides the adjudication details from the processing of a 15754 * Claim resource. 15755 */ 15756 CLAIMRESPONSE, 15757 /** 15758 * A record of a clinical assessment performed to determine what problem(s) may 15759 * affect the patient and before planning the treatments or management 15760 * strategies that are best to manage a patient's condition. Assessments are 15761 * often 1:1 with a clinical consultation / encounter, but this varies greatly 15762 * depending on the clinical workflow. This resource is called 15763 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 15764 * the recording of assessment tools such as Apgar score. 15765 */ 15766 CLINICALIMPRESSION, 15767 /** 15768 * The CodeSystem resource is used to declare the existence of and describe a 15769 * code system or code system supplement and its key properties, and optionally 15770 * define a part or all of its content. 15771 */ 15772 CODESYSTEM, 15773 /** 15774 * An occurrence of information being transmitted; e.g. an alert that was sent 15775 * to a responsible provider, a public health agency that was notified about a 15776 * reportable condition. 15777 */ 15778 COMMUNICATION, 15779 /** 15780 * A request to convey information; e.g. the CDS system proposes that an alert 15781 * be sent to a responsible provider, the CDS system proposes that the public 15782 * health agency be notified about a reportable condition. 15783 */ 15784 COMMUNICATIONREQUEST, 15785 /** 15786 * A compartment definition that defines how resources are accessed on a server. 15787 */ 15788 COMPARTMENTDEFINITION, 15789 /** 15790 * A set of healthcare-related information that is assembled together into a 15791 * single logical package that provides a single coherent statement of meaning, 15792 * establishes its own context and that has clinical attestation with regard to 15793 * who is making the statement. A Composition defines the structure and 15794 * narrative content necessary for a document. However, a Composition alone does 15795 * not constitute a document. Rather, the Composition must be the first entry in 15796 * a Bundle where Bundle.type=document, and any other resources referenced from 15797 * Composition must be included as subsequent entries in the Bundle (for example 15798 * Patient, Practitioner, Encounter, etc.). 15799 */ 15800 COMPOSITION, 15801 /** 15802 * A statement of relationships from one set of concepts to one or more other 15803 * concepts - either concepts in code systems, or data element/data element 15804 * concepts, or classes in class models. 15805 */ 15806 CONCEPTMAP, 15807 /** 15808 * A clinical condition, problem, diagnosis, or other event, situation, issue, 15809 * or clinical concept that has risen to a level of concern. 15810 */ 15811 CONDITION, 15812 /** 15813 * A record of a healthcare consumer?s choices, which permits or denies 15814 * identified recipient(s) or recipient role(s) to perform one or more actions 15815 * within a given policy context, for specific purposes and periods of time. 15816 */ 15817 CONSENT, 15818 /** 15819 * Legally enforceable, formally recorded unilateral or bilateral directive 15820 * i.e., a policy or agreement. 15821 */ 15822 CONTRACT, 15823 /** 15824 * Financial instrument which may be used to reimburse or pay for health care 15825 * products and services. Includes both insurance and self-payment. 15826 */ 15827 COVERAGE, 15828 /** 15829 * The CoverageEligibilityRequest provides patient and insurance coverage 15830 * information to an insurer for them to respond, in the form of an 15831 * CoverageEligibilityResponse, with information regarding whether the stated 15832 * coverage is valid and in-force and optionally to provide the insurance 15833 * details of the policy. 15834 */ 15835 COVERAGEELIGIBILITYREQUEST, 15836 /** 15837 * This resource provides eligibility and plan details from the processing of an 15838 * CoverageEligibilityRequest resource. 15839 */ 15840 COVERAGEELIGIBILITYRESPONSE, 15841 /** 15842 * Indicates an actual or potential clinical issue with or between one or more 15843 * active or proposed clinical actions for a patient; e.g. Drug-drug 15844 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 15845 * etc. 15846 */ 15847 DETECTEDISSUE, 15848 /** 15849 * A type of a manufactured item that is used in the provision of healthcare 15850 * without being substantially changed through that activity. The device may be 15851 * a medical or non-medical device. 15852 */ 15853 DEVICE, 15854 /** 15855 * The characteristics, operational status and capabilities of a medical-related 15856 * component of a medical device. 15857 */ 15858 DEVICEDEFINITION, 15859 /** 15860 * Describes a measurement, calculation or setting capability of a medical 15861 * device. 15862 */ 15863 DEVICEMETRIC, 15864 /** 15865 * Represents a request for a patient to employ a medical device. The device may 15866 * be an implantable device, or an external assistive device, such as a walker. 15867 */ 15868 DEVICEREQUEST, 15869 /** 15870 * A record of a device being used by a patient where the record is the result 15871 * of a report from the patient or another clinician. 15872 */ 15873 DEVICEUSESTATEMENT, 15874 /** 15875 * The findings and interpretation of diagnostic tests performed on patients, 15876 * groups of patients, devices, and locations, and/or specimens derived from 15877 * these. The report includes clinical context such as requesting and provider 15878 * information, and some mix of atomic results, images, textual and coded 15879 * interpretations, and formatted representation of diagnostic reports. 15880 */ 15881 DIAGNOSTICREPORT, 15882 /** 15883 * A collection of documents compiled for a purpose together with metadata that 15884 * applies to the collection. 15885 */ 15886 DOCUMENTMANIFEST, 15887 /** 15888 * A reference to a document of any kind for any purpose. Provides metadata 15889 * about the document so that the document can be discovered and managed. The 15890 * scope of a document is any seralized object with a mime-type, so includes 15891 * formal patient centric documents (CDA), cliical notes, scanned paper, and 15892 * non-patient specific documents like policy text. 15893 */ 15894 DOCUMENTREFERENCE, 15895 /** 15896 * A resource that includes narrative, extensions, and contained resources. 15897 */ 15898 DOMAINRESOURCE, 15899 /** 15900 * The EffectEvidenceSynthesis resource describes the difference in an outcome 15901 * between exposures states in a population where the effect estimate is derived 15902 * from a combination of research studies. 15903 */ 15904 EFFECTEVIDENCESYNTHESIS, 15905 /** 15906 * An interaction between a patient and healthcare provider(s) for the purpose 15907 * of providing healthcare service(s) or assessing the health status of a 15908 * patient. 15909 */ 15910 ENCOUNTER, 15911 /** 15912 * The technical details of an endpoint that can be used for electronic 15913 * services, such as for web services providing XDS.b or a REST endpoint for 15914 * another FHIR server. This may include any security context information. 15915 */ 15916 ENDPOINT, 15917 /** 15918 * This resource provides the insurance enrollment details to the insurer 15919 * regarding a specified coverage. 15920 */ 15921 ENROLLMENTREQUEST, 15922 /** 15923 * This resource provides enrollment and plan details from the processing of an 15924 * EnrollmentRequest resource. 15925 */ 15926 ENROLLMENTRESPONSE, 15927 /** 15928 * An association between a patient and an organization / healthcare provider(s) 15929 * during which time encounters may occur. The managing organization assumes a 15930 * level of responsibility for the patient during this time. 15931 */ 15932 EPISODEOFCARE, 15933 /** 15934 * The EventDefinition resource provides a reusable description of when a 15935 * particular event can occur. 15936 */ 15937 EVENTDEFINITION, 15938 /** 15939 * The Evidence resource describes the conditional state (population and any 15940 * exposures being compared within the population) and outcome (if specified) 15941 * that the knowledge (evidence, assertion, recommendation) is about. 15942 */ 15943 EVIDENCE, 15944 /** 15945 * The EvidenceVariable resource describes a "PICO" element that knowledge 15946 * (evidence, assertion, recommendation) is about. 15947 */ 15948 EVIDENCEVARIABLE, 15949 /** 15950 * Example of workflow instance. 15951 */ 15952 EXAMPLESCENARIO, 15953 /** 15954 * This resource provides: the claim details; adjudication details from the 15955 * processing of a Claim; and optionally account balance information, for 15956 * informing the subscriber of the benefits provided. 15957 */ 15958 EXPLANATIONOFBENEFIT, 15959 /** 15960 * Significant health conditions for a person related to the patient relevant in 15961 * the context of care for the patient. 15962 */ 15963 FAMILYMEMBERHISTORY, 15964 /** 15965 * Prospective warnings of potential issues when providing care to the patient. 15966 */ 15967 FLAG, 15968 /** 15969 * Describes the intended objective(s) for a patient, group or organization 15970 * care, for example, weight loss, restoring an activity of daily living, 15971 * obtaining herd immunity via immunization, meeting a process improvement 15972 * objective, etc. 15973 */ 15974 GOAL, 15975 /** 15976 * A formal computable definition of a graph of resources - that is, a coherent 15977 * set of resources that form a graph by following references. The Graph 15978 * Definition resource defines a set and makes rules about the set. 15979 */ 15980 GRAPHDEFINITION, 15981 /** 15982 * Represents a defined collection of entities that may be discussed or acted 15983 * upon collectively but which are not expected to act collectively, and are not 15984 * formally or legally recognized; i.e. a collection of entities that isn't an 15985 * Organization. 15986 */ 15987 GROUP, 15988 /** 15989 * A guidance response is the formal response to a guidance request, including 15990 * any output parameters returned by the evaluation, as well as the description 15991 * of any proposed actions to be taken. 15992 */ 15993 GUIDANCERESPONSE, 15994 /** 15995 * The details of a healthcare service available at a location. 15996 */ 15997 HEALTHCARESERVICE, 15998 /** 15999 * Representation of the content produced in a DICOM imaging study. A study 16000 * comprises a set of series, each of which includes a set of Service-Object 16001 * Pair Instances (SOP Instances - images or other data) acquired or produced in 16002 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 16003 * ultrasound), but a study may have multiple series of different modalities. 16004 */ 16005 IMAGINGSTUDY, 16006 /** 16007 * Describes the event of a patient being administered a vaccine or a record of 16008 * an immunization as reported by a patient, a clinician or another party. 16009 */ 16010 IMMUNIZATION, 16011 /** 16012 * Describes a comparison of an immunization event against published 16013 * recommendations to determine if the administration is "valid" in relation to 16014 * those recommendations. 16015 */ 16016 IMMUNIZATIONEVALUATION, 16017 /** 16018 * A patient's point-in-time set of recommendations (i.e. forecasting) according 16019 * to a published schedule with optional supporting justification. 16020 */ 16021 IMMUNIZATIONRECOMMENDATION, 16022 /** 16023 * A set of rules of how a particular interoperability or standards problem is 16024 * solved - typically through the use of FHIR resources. This resource is used 16025 * to gather all the parts of an implementation guide into a logical whole and 16026 * to publish a computable definition of all the parts. 16027 */ 16028 IMPLEMENTATIONGUIDE, 16029 /** 16030 * Details of a Health Insurance product/plan provided by an organization. 16031 */ 16032 INSURANCEPLAN, 16033 /** 16034 * Invoice containing collected ChargeItems from an Account with calculated 16035 * individual and total price for Billing purpose. 16036 */ 16037 INVOICE, 16038 /** 16039 * The Library resource is a general-purpose container for knowledge asset 16040 * definitions. It can be used to describe and expose existing knowledge assets 16041 * such as logic libraries and information model descriptions, as well as to 16042 * describe a collection of knowledge assets. 16043 */ 16044 LIBRARY, 16045 /** 16046 * Identifies two or more records (resource instances) that refer to the same 16047 * real-world "occurrence". 16048 */ 16049 LINKAGE, 16050 /** 16051 * A list is a curated collection of resources. 16052 */ 16053 LIST, 16054 /** 16055 * Details and position information for a physical place where services are 16056 * provided and resources and participants may be stored, found, contained, or 16057 * accommodated. 16058 */ 16059 LOCATION, 16060 /** 16061 * The Measure resource provides the definition of a quality measure. 16062 */ 16063 MEASURE, 16064 /** 16065 * The MeasureReport resource contains the results of the calculation of a 16066 * measure; and optionally a reference to the resources involved in that 16067 * calculation. 16068 */ 16069 MEASUREREPORT, 16070 /** 16071 * A photo, video, or audio recording acquired or used in healthcare. The actual 16072 * content may be inline or provided by direct reference. 16073 */ 16074 MEDIA, 16075 /** 16076 * This resource is primarily used for the identification and definition of a 16077 * medication for the purposes of prescribing, dispensing, and administering a 16078 * medication as well as for making statements about medication use. 16079 */ 16080 MEDICATION, 16081 /** 16082 * Describes the event of a patient consuming or otherwise being administered a 16083 * medication. This may be as simple as swallowing a tablet or it may be a long 16084 * running infusion. Related resources tie this event to the authorizing 16085 * prescription, and the specific encounter between patient and health care 16086 * practitioner. 16087 */ 16088 MEDICATIONADMINISTRATION, 16089 /** 16090 * Indicates that a medication product is to be or has been dispensed for a 16091 * named person/patient. This includes a description of the medication product 16092 * (supply) provided and the instructions for administering the medication. The 16093 * medication dispense is the result of a pharmacy system responding to a 16094 * medication order. 16095 */ 16096 MEDICATIONDISPENSE, 16097 /** 16098 * Information about a medication that is used to support knowledge. 16099 */ 16100 MEDICATIONKNOWLEDGE, 16101 /** 16102 * An order or request for both supply of the medication and the instructions 16103 * for administration of the medication to a patient. The resource is called 16104 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 16105 * to generalize the use across inpatient and outpatient settings, including 16106 * care plans, etc., and to harmonize with workflow patterns. 16107 */ 16108 MEDICATIONREQUEST, 16109 /** 16110 * A record of a medication that is being consumed by a patient. A 16111 * MedicationStatement may indicate that the patient may be taking the 16112 * medication now or has taken the medication in the past or will be taking the 16113 * medication in the future. The source of this information can be the patient, 16114 * significant other (such as a family member or spouse), or a clinician. A 16115 * common scenario where this information is captured is during the history 16116 * taking process during a patient visit or stay. The medication information may 16117 * come from sources such as the patient's memory, from a prescription bottle, 16118 * or from a list of medications the patient, clinician or other party 16119 * maintains. 16120 * 16121 * The primary difference between a medication statement and a medication 16122 * administration is that the medication administration has complete 16123 * administration information and is based on actual administration information 16124 * from the person who administered the medication. A medication statement is 16125 * often, if not always, less specific. There is no required date/time when the 16126 * medication was administered, in fact we only know that a source has reported 16127 * the patient is taking this medication, where details such as time, quantity, 16128 * or rate or even medication product may be incomplete or missing or less 16129 * precise. As stated earlier, the medication statement information may come 16130 * from the patient's memory, from a prescription bottle or from a list of 16131 * medications the patient, clinician or other party maintains. Medication 16132 * administration is more formal and is not missing detailed information. 16133 */ 16134 MEDICATIONSTATEMENT, 16135 /** 16136 * Detailed definition of a medicinal product, typically for uses other than 16137 * direct patient care (e.g. regulatory use). 16138 */ 16139 MEDICINALPRODUCT, 16140 /** 16141 * The regulatory authorization of a medicinal product. 16142 */ 16143 MEDICINALPRODUCTAUTHORIZATION, 16144 /** 16145 * The clinical particulars - indications, contraindications etc. of a medicinal 16146 * product, including for regulatory purposes. 16147 */ 16148 MEDICINALPRODUCTCONTRAINDICATION, 16149 /** 16150 * Indication for the Medicinal Product. 16151 */ 16152 MEDICINALPRODUCTINDICATION, 16153 /** 16154 * An ingredient of a manufactured item or pharmaceutical product. 16155 */ 16156 MEDICINALPRODUCTINGREDIENT, 16157 /** 16158 * The interactions of the medicinal product with other medicinal products, or 16159 * other forms of interactions. 16160 */ 16161 MEDICINALPRODUCTINTERACTION, 16162 /** 16163 * The manufactured item as contained in the packaged medicinal product. 16164 */ 16165 MEDICINALPRODUCTMANUFACTURED, 16166 /** 16167 * A medicinal product in a container or package. 16168 */ 16169 MEDICINALPRODUCTPACKAGED, 16170 /** 16171 * A pharmaceutical product described in terms of its composition and dose form. 16172 */ 16173 MEDICINALPRODUCTPHARMACEUTICAL, 16174 /** 16175 * Describe the undesirable effects of the medicinal product. 16176 */ 16177 MEDICINALPRODUCTUNDESIRABLEEFFECT, 16178 /** 16179 * Defines the characteristics of a message that can be shared between systems, 16180 * including the type of event that initiates the message, the content to be 16181 * transmitted and what response(s), if any, are permitted. 16182 */ 16183 MESSAGEDEFINITION, 16184 /** 16185 * The header for a message exchange that is either requesting or responding to 16186 * an action. The reference(s) that are the subject of the action as well as 16187 * other information related to the action are typically transmitted in a bundle 16188 * in which the MessageHeader resource instance is the first resource in the 16189 * bundle. 16190 */ 16191 MESSAGEHEADER, 16192 /** 16193 * Raw data describing a biological sequence. 16194 */ 16195 MOLECULARSEQUENCE, 16196 /** 16197 * A curated namespace that issues unique symbols within that namespace for the 16198 * identification of concepts, people, devices, etc. Represents a "System" used 16199 * within the Identifier and Coding data types. 16200 */ 16201 NAMINGSYSTEM, 16202 /** 16203 * A request to supply a diet, formula feeding (enteral) or oral nutritional 16204 * supplement to a patient/resident. 16205 */ 16206 NUTRITIONORDER, 16207 /** 16208 * Measurements and simple assertions made about a patient, device or other 16209 * subject. 16210 */ 16211 OBSERVATION, 16212 /** 16213 * Set of definitional characteristics for a kind of observation or measurement 16214 * produced or consumed by an orderable health care service. 16215 */ 16216 OBSERVATIONDEFINITION, 16217 /** 16218 * A formal computable definition of an operation (on the RESTful interface) or 16219 * a named query (using the search interaction). 16220 */ 16221 OPERATIONDEFINITION, 16222 /** 16223 * A collection of error, warning, or information messages that result from a 16224 * system action. 16225 */ 16226 OPERATIONOUTCOME, 16227 /** 16228 * A formally or informally recognized grouping of people or organizations 16229 * formed for the purpose of achieving some form of collective action. Includes 16230 * companies, institutions, corporations, departments, community groups, 16231 * healthcare practice groups, payer/insurer, etc. 16232 */ 16233 ORGANIZATION, 16234 /** 16235 * Defines an affiliation/assotiation/relationship between 2 distinct 16236 * oganizations, that is not a part-of relationship/sub-division relationship. 16237 */ 16238 ORGANIZATIONAFFILIATION, 16239 /** 16240 * This resource is a non-persisted resource used to pass information into and 16241 * back from an [operation](operations.html). It has no other use, and there is 16242 * no RESTful endpoint associated with it. 16243 */ 16244 PARAMETERS, 16245 /** 16246 * Demographics and other administrative information about an individual or 16247 * animal receiving care or other health-related services. 16248 */ 16249 PATIENT, 16250 /** 16251 * This resource provides the status of the payment for goods and services 16252 * rendered, and the request and response resource references. 16253 */ 16254 PAYMENTNOTICE, 16255 /** 16256 * This resource provides the details including amount of a payment and 16257 * allocates the payment items being paid. 16258 */ 16259 PAYMENTRECONCILIATION, 16260 /** 16261 * Demographics and administrative information about a person independent of a 16262 * specific health-related context. 16263 */ 16264 PERSON, 16265 /** 16266 * This resource allows for the definition of various types of plans as a 16267 * sharable, consumable, and executable artifact. The resource is general enough 16268 * to support the description of a broad range of clinical artifacts such as 16269 * clinical decision support rules, order sets and protocols. 16270 */ 16271 PLANDEFINITION, 16272 /** 16273 * A person who is directly or indirectly involved in the provisioning of 16274 * healthcare. 16275 */ 16276 PRACTITIONER, 16277 /** 16278 * A specific set of Roles/Locations/specialties/services that a practitioner 16279 * may perform at an organization for a period of time. 16280 */ 16281 PRACTITIONERROLE, 16282 /** 16283 * An action that is or was performed on or for a patient. This can be a 16284 * physical intervention like an operation, or less invasive like long term 16285 * services, counseling, or hypnotherapy. 16286 */ 16287 PROCEDURE, 16288 /** 16289 * Provenance of a resource is a record that describes entities and processes 16290 * involved in producing and delivering or otherwise influencing that resource. 16291 * Provenance provides a critical foundation for assessing authenticity, 16292 * enabling trust, and allowing reproducibility. Provenance assertions are a 16293 * form of contextual metadata and can themselves become important records with 16294 * their own provenance. Provenance statement indicates clinical significance in 16295 * terms of confidence in authenticity, reliability, and trustworthiness, 16296 * integrity, and stage in lifecycle (e.g. Document Completion - has the 16297 * artifact been legally authenticated), all of which may impact security, 16298 * privacy, and trust policies. 16299 */ 16300 PROVENANCE, 16301 /** 16302 * A structured set of questions intended to guide the collection of answers 16303 * from end-users. Questionnaires provide detailed control over order, 16304 * presentation, phraseology and grouping to allow coherent, consistent data 16305 * collection. 16306 */ 16307 QUESTIONNAIRE, 16308 /** 16309 * A structured set of questions and their answers. The questions are ordered 16310 * and grouped into coherent subsets, corresponding to the structure of the 16311 * grouping of the questionnaire being responded to. 16312 */ 16313 QUESTIONNAIRERESPONSE, 16314 /** 16315 * Information about a person that is involved in the care for a patient, but 16316 * who is not the target of healthcare, nor has a formal responsibility in the 16317 * care process. 16318 */ 16319 RELATEDPERSON, 16320 /** 16321 * A group of related requests that can be used to capture intended activities 16322 * that have inter-dependencies such as "give this medication after that one". 16323 */ 16324 REQUESTGROUP, 16325 /** 16326 * The ResearchDefinition resource describes the conditional state (population 16327 * and any exposures being compared within the population) and outcome (if 16328 * specified) that the knowledge (evidence, assertion, recommendation) is about. 16329 */ 16330 RESEARCHDEFINITION, 16331 /** 16332 * The ResearchElementDefinition resource describes a "PICO" element that 16333 * knowledge (evidence, assertion, recommendation) is about. 16334 */ 16335 RESEARCHELEMENTDEFINITION, 16336 /** 16337 * A process where a researcher or organization plans and then executes a series 16338 * of steps intended to increase the field of healthcare-related knowledge. This 16339 * includes studies of safety, efficacy, comparative effectiveness and other 16340 * information about medications, devices, therapies and other interventional 16341 * and investigative techniques. A ResearchStudy involves the gathering of 16342 * information about human or animal subjects. 16343 */ 16344 RESEARCHSTUDY, 16345 /** 16346 * A physical entity which is the primary unit of operational and/or 16347 * administrative interest in a study. 16348 */ 16349 RESEARCHSUBJECT, 16350 /** 16351 * This is the base resource type for everything. 16352 */ 16353 RESOURCE, 16354 /** 16355 * An assessment of the likely outcome(s) for a patient or other subject as well 16356 * as the likelihood of each outcome. 16357 */ 16358 RISKASSESSMENT, 16359 /** 16360 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 16361 * a population plus exposure state where the risk estimate is derived from a 16362 * combination of research studies. 16363 */ 16364 RISKEVIDENCESYNTHESIS, 16365 /** 16366 * A container for slots of time that may be available for booking appointments. 16367 */ 16368 SCHEDULE, 16369 /** 16370 * A search parameter that defines a named search item that can be used to 16371 * search/filter on a resource. 16372 */ 16373 SEARCHPARAMETER, 16374 /** 16375 * A record of a request for service such as diagnostic investigations, 16376 * treatments, or operations to be performed. 16377 */ 16378 SERVICEREQUEST, 16379 /** 16380 * A slot of time on a schedule that may be available for booking appointments. 16381 */ 16382 SLOT, 16383 /** 16384 * A sample to be used for analysis. 16385 */ 16386 SPECIMEN, 16387 /** 16388 * A kind of specimen with associated set of requirements. 16389 */ 16390 SPECIMENDEFINITION, 16391 /** 16392 * A definition of a FHIR structure. This resource is used to describe the 16393 * underlying resources, data types defined in FHIR, and also for describing 16394 * extensions and constraints on resources and data types. 16395 */ 16396 STRUCTUREDEFINITION, 16397 /** 16398 * A Map of relationships between 2 structures that can be used to transform 16399 * data. 16400 */ 16401 STRUCTUREMAP, 16402 /** 16403 * The subscription resource is used to define a push-based subscription from a 16404 * server to another system. Once a subscription is registered with the server, 16405 * the server checks every resource that is created or updated, and if the 16406 * resource matches the given criteria, it sends a message on the defined 16407 * "channel" so that another system can take an appropriate action. 16408 */ 16409 SUBSCRIPTION, 16410 /** 16411 * A homogeneous material with a definite composition. 16412 */ 16413 SUBSTANCE, 16414 /** 16415 * Nucleic acids are defined by three distinct elements: the base, sugar and 16416 * linkage. Individual substance/moiety IDs will be created for each of these 16417 * elements. The nucleotide sequence will be always entered in the 5?-3? 16418 * direction. 16419 */ 16420 SUBSTANCENUCLEICACID, 16421 /** 16422 * Todo. 16423 */ 16424 SUBSTANCEPOLYMER, 16425 /** 16426 * A SubstanceProtein is defined as a single unit of a linear amino acid 16427 * sequence, or a combination of subunits that are either covalently linked or 16428 * have a defined invariant stoichiometric relationship. This includes all 16429 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 16430 * whether the use is therapeutic or prophylactic. This set of elements will be 16431 * used to describe albumins, coagulation factors, cytokines, growth factors, 16432 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 16433 * vaccines, and immunomodulators. 16434 */ 16435 SUBSTANCEPROTEIN, 16436 /** 16437 * Todo. 16438 */ 16439 SUBSTANCEREFERENCEINFORMATION, 16440 /** 16441 * Source material shall capture information on the taxonomic and anatomical 16442 * origins as well as the fraction of a material that can result in or can be 16443 * modified to form a substance. This set of data elements shall be used to 16444 * define polymer substances isolated from biological matrices. Taxonomic and 16445 * anatomical origins shall be described using a controlled vocabulary as 16446 * required. This information is captured for naturally derived polymers ( . 16447 * starch) and structurally diverse substances. For Organisms belonging to the 16448 * Kingdom Plantae the Substance level defines the fresh material of a single 16449 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 16450 * Herbal preparations, the fraction information will be captured at the 16451 * Substance information level and additional information for herbal extracts 16452 * will be captured at the Specified Substance Group 1 information level. See 16453 * for further explanation the Substance Class: Structurally Diverse and the 16454 * herbal annex. 16455 */ 16456 SUBSTANCESOURCEMATERIAL, 16457 /** 16458 * The detailed description of a substance, typically at a level beyond what is 16459 * used for prescribing. 16460 */ 16461 SUBSTANCESPECIFICATION, 16462 /** 16463 * Record of delivery of what is supplied. 16464 */ 16465 SUPPLYDELIVERY, 16466 /** 16467 * A record of a request for a medication, substance or device used in the 16468 * healthcare setting. 16469 */ 16470 SUPPLYREQUEST, 16471 /** 16472 * A task to be performed. 16473 */ 16474 TASK, 16475 /** 16476 * A Terminology Capabilities documents a set of capabilities (behaviors) of a 16477 * FHIR Server that may be used as a statement of actual server functionality or 16478 * a statement of required or desired server implementation. 16479 */ 16480 TERMINOLOGYCAPABILITIES, 16481 /** 16482 * A summary of information based on the results of executing a TestScript. 16483 */ 16484 TESTREPORT, 16485 /** 16486 * A structured set of tests against a FHIR server or client implementation to 16487 * determine compliance against the FHIR specification. 16488 */ 16489 TESTSCRIPT, 16490 /** 16491 * A ValueSet resource instance specifies a set of codes drawn from one or more 16492 * code systems, intended for use in a particular context. Value sets link 16493 * between [[[CodeSystem]]] definitions and their use in [coded 16494 * elements](terminologies.html). 16495 */ 16496 VALUESET, 16497 /** 16498 * Describes validation requirements, source(s), status and dates for one or 16499 * more elements. 16500 */ 16501 VERIFICATIONRESULT, 16502 /** 16503 * An authorization for the provision of glasses and/or contact lenses to a 16504 * patient. 16505 */ 16506 VISIONPRESCRIPTION, 16507 /** 16508 * added to help the parsers 16509 */ 16510 NULL; 16511 16512 public static ResourceType fromCode(String codeString) throws FHIRException { 16513 if (codeString == null || "".equals(codeString)) 16514 return null; 16515 if ("Account".equals(codeString)) 16516 return ACCOUNT; 16517 if ("ActivityDefinition".equals(codeString)) 16518 return ACTIVITYDEFINITION; 16519 if ("AdverseEvent".equals(codeString)) 16520 return ADVERSEEVENT; 16521 if ("AllergyIntolerance".equals(codeString)) 16522 return ALLERGYINTOLERANCE; 16523 if ("Appointment".equals(codeString)) 16524 return APPOINTMENT; 16525 if ("AppointmentResponse".equals(codeString)) 16526 return APPOINTMENTRESPONSE; 16527 if ("AuditEvent".equals(codeString)) 16528 return AUDITEVENT; 16529 if ("Basic".equals(codeString)) 16530 return BASIC; 16531 if ("Binary".equals(codeString)) 16532 return BINARY; 16533 if ("BiologicallyDerivedProduct".equals(codeString)) 16534 return BIOLOGICALLYDERIVEDPRODUCT; 16535 if ("BodyStructure".equals(codeString)) 16536 return BODYSTRUCTURE; 16537 if ("Bundle".equals(codeString)) 16538 return BUNDLE; 16539 if ("CapabilityStatement".equals(codeString)) 16540 return CAPABILITYSTATEMENT; 16541 if ("CarePlan".equals(codeString)) 16542 return CAREPLAN; 16543 if ("CareTeam".equals(codeString)) 16544 return CARETEAM; 16545 if ("CatalogEntry".equals(codeString)) 16546 return CATALOGENTRY; 16547 if ("ChargeItem".equals(codeString)) 16548 return CHARGEITEM; 16549 if ("ChargeItemDefinition".equals(codeString)) 16550 return CHARGEITEMDEFINITION; 16551 if ("Claim".equals(codeString)) 16552 return CLAIM; 16553 if ("ClaimResponse".equals(codeString)) 16554 return CLAIMRESPONSE; 16555 if ("ClinicalImpression".equals(codeString)) 16556 return CLINICALIMPRESSION; 16557 if ("CodeSystem".equals(codeString)) 16558 return CODESYSTEM; 16559 if ("Communication".equals(codeString)) 16560 return COMMUNICATION; 16561 if ("CommunicationRequest".equals(codeString)) 16562 return COMMUNICATIONREQUEST; 16563 if ("CompartmentDefinition".equals(codeString)) 16564 return COMPARTMENTDEFINITION; 16565 if ("Composition".equals(codeString)) 16566 return COMPOSITION; 16567 if ("ConceptMap".equals(codeString)) 16568 return CONCEPTMAP; 16569 if ("Condition".equals(codeString)) 16570 return CONDITION; 16571 if ("Consent".equals(codeString)) 16572 return CONSENT; 16573 if ("Contract".equals(codeString)) 16574 return CONTRACT; 16575 if ("Coverage".equals(codeString)) 16576 return COVERAGE; 16577 if ("CoverageEligibilityRequest".equals(codeString)) 16578 return COVERAGEELIGIBILITYREQUEST; 16579 if ("CoverageEligibilityResponse".equals(codeString)) 16580 return COVERAGEELIGIBILITYRESPONSE; 16581 if ("DetectedIssue".equals(codeString)) 16582 return DETECTEDISSUE; 16583 if ("Device".equals(codeString)) 16584 return DEVICE; 16585 if ("DeviceDefinition".equals(codeString)) 16586 return DEVICEDEFINITION; 16587 if ("DeviceMetric".equals(codeString)) 16588 return DEVICEMETRIC; 16589 if ("DeviceRequest".equals(codeString)) 16590 return DEVICEREQUEST; 16591 if ("DeviceUseStatement".equals(codeString)) 16592 return DEVICEUSESTATEMENT; 16593 if ("DiagnosticReport".equals(codeString)) 16594 return DIAGNOSTICREPORT; 16595 if ("DocumentManifest".equals(codeString)) 16596 return DOCUMENTMANIFEST; 16597 if ("DocumentReference".equals(codeString)) 16598 return DOCUMENTREFERENCE; 16599 if ("DomainResource".equals(codeString)) 16600 return DOMAINRESOURCE; 16601 if ("EffectEvidenceSynthesis".equals(codeString)) 16602 return EFFECTEVIDENCESYNTHESIS; 16603 if ("Encounter".equals(codeString)) 16604 return ENCOUNTER; 16605 if ("Endpoint".equals(codeString)) 16606 return ENDPOINT; 16607 if ("EnrollmentRequest".equals(codeString)) 16608 return ENROLLMENTREQUEST; 16609 if ("EnrollmentResponse".equals(codeString)) 16610 return ENROLLMENTRESPONSE; 16611 if ("EpisodeOfCare".equals(codeString)) 16612 return EPISODEOFCARE; 16613 if ("EventDefinition".equals(codeString)) 16614 return EVENTDEFINITION; 16615 if ("Evidence".equals(codeString)) 16616 return EVIDENCE; 16617 if ("EvidenceVariable".equals(codeString)) 16618 return EVIDENCEVARIABLE; 16619 if ("ExampleScenario".equals(codeString)) 16620 return EXAMPLESCENARIO; 16621 if ("ExplanationOfBenefit".equals(codeString)) 16622 return EXPLANATIONOFBENEFIT; 16623 if ("FamilyMemberHistory".equals(codeString)) 16624 return FAMILYMEMBERHISTORY; 16625 if ("Flag".equals(codeString)) 16626 return FLAG; 16627 if ("Goal".equals(codeString)) 16628 return GOAL; 16629 if ("GraphDefinition".equals(codeString)) 16630 return GRAPHDEFINITION; 16631 if ("Group".equals(codeString)) 16632 return GROUP; 16633 if ("GuidanceResponse".equals(codeString)) 16634 return GUIDANCERESPONSE; 16635 if ("HealthcareService".equals(codeString)) 16636 return HEALTHCARESERVICE; 16637 if ("ImagingStudy".equals(codeString)) 16638 return IMAGINGSTUDY; 16639 if ("Immunization".equals(codeString)) 16640 return IMMUNIZATION; 16641 if ("ImmunizationEvaluation".equals(codeString)) 16642 return IMMUNIZATIONEVALUATION; 16643 if ("ImmunizationRecommendation".equals(codeString)) 16644 return IMMUNIZATIONRECOMMENDATION; 16645 if ("ImplementationGuide".equals(codeString)) 16646 return IMPLEMENTATIONGUIDE; 16647 if ("InsurancePlan".equals(codeString)) 16648 return INSURANCEPLAN; 16649 if ("Invoice".equals(codeString)) 16650 return INVOICE; 16651 if ("Library".equals(codeString)) 16652 return LIBRARY; 16653 if ("Linkage".equals(codeString)) 16654 return LINKAGE; 16655 if ("List".equals(codeString)) 16656 return LIST; 16657 if ("Location".equals(codeString)) 16658 return LOCATION; 16659 if ("Measure".equals(codeString)) 16660 return MEASURE; 16661 if ("MeasureReport".equals(codeString)) 16662 return MEASUREREPORT; 16663 if ("Media".equals(codeString)) 16664 return MEDIA; 16665 if ("Medication".equals(codeString)) 16666 return MEDICATION; 16667 if ("MedicationAdministration".equals(codeString)) 16668 return MEDICATIONADMINISTRATION; 16669 if ("MedicationDispense".equals(codeString)) 16670 return MEDICATIONDISPENSE; 16671 if ("MedicationKnowledge".equals(codeString)) 16672 return MEDICATIONKNOWLEDGE; 16673 if ("MedicationRequest".equals(codeString)) 16674 return MEDICATIONREQUEST; 16675 if ("MedicationStatement".equals(codeString)) 16676 return MEDICATIONSTATEMENT; 16677 if ("MedicinalProduct".equals(codeString)) 16678 return MEDICINALPRODUCT; 16679 if ("MedicinalProductAuthorization".equals(codeString)) 16680 return MEDICINALPRODUCTAUTHORIZATION; 16681 if ("MedicinalProductContraindication".equals(codeString)) 16682 return MEDICINALPRODUCTCONTRAINDICATION; 16683 if ("MedicinalProductIndication".equals(codeString)) 16684 return MEDICINALPRODUCTINDICATION; 16685 if ("MedicinalProductIngredient".equals(codeString)) 16686 return MEDICINALPRODUCTINGREDIENT; 16687 if ("MedicinalProductInteraction".equals(codeString)) 16688 return MEDICINALPRODUCTINTERACTION; 16689 if ("MedicinalProductManufactured".equals(codeString)) 16690 return MEDICINALPRODUCTMANUFACTURED; 16691 if ("MedicinalProductPackaged".equals(codeString)) 16692 return MEDICINALPRODUCTPACKAGED; 16693 if ("MedicinalProductPharmaceutical".equals(codeString)) 16694 return MEDICINALPRODUCTPHARMACEUTICAL; 16695 if ("MedicinalProductUndesirableEffect".equals(codeString)) 16696 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 16697 if ("MessageDefinition".equals(codeString)) 16698 return MESSAGEDEFINITION; 16699 if ("MessageHeader".equals(codeString)) 16700 return MESSAGEHEADER; 16701 if ("MolecularSequence".equals(codeString)) 16702 return MOLECULARSEQUENCE; 16703 if ("NamingSystem".equals(codeString)) 16704 return NAMINGSYSTEM; 16705 if ("NutritionOrder".equals(codeString)) 16706 return NUTRITIONORDER; 16707 if ("Observation".equals(codeString)) 16708 return OBSERVATION; 16709 if ("ObservationDefinition".equals(codeString)) 16710 return OBSERVATIONDEFINITION; 16711 if ("OperationDefinition".equals(codeString)) 16712 return OPERATIONDEFINITION; 16713 if ("OperationOutcome".equals(codeString)) 16714 return OPERATIONOUTCOME; 16715 if ("Organization".equals(codeString)) 16716 return ORGANIZATION; 16717 if ("OrganizationAffiliation".equals(codeString)) 16718 return ORGANIZATIONAFFILIATION; 16719 if ("Parameters".equals(codeString)) 16720 return PARAMETERS; 16721 if ("Patient".equals(codeString)) 16722 return PATIENT; 16723 if ("PaymentNotice".equals(codeString)) 16724 return PAYMENTNOTICE; 16725 if ("PaymentReconciliation".equals(codeString)) 16726 return PAYMENTRECONCILIATION; 16727 if ("Person".equals(codeString)) 16728 return PERSON; 16729 if ("PlanDefinition".equals(codeString)) 16730 return PLANDEFINITION; 16731 if ("Practitioner".equals(codeString)) 16732 return PRACTITIONER; 16733 if ("PractitionerRole".equals(codeString)) 16734 return PRACTITIONERROLE; 16735 if ("Procedure".equals(codeString)) 16736 return PROCEDURE; 16737 if ("Provenance".equals(codeString)) 16738 return PROVENANCE; 16739 if ("Questionnaire".equals(codeString)) 16740 return QUESTIONNAIRE; 16741 if ("QuestionnaireResponse".equals(codeString)) 16742 return QUESTIONNAIRERESPONSE; 16743 if ("RelatedPerson".equals(codeString)) 16744 return RELATEDPERSON; 16745 if ("RequestGroup".equals(codeString)) 16746 return REQUESTGROUP; 16747 if ("ResearchDefinition".equals(codeString)) 16748 return RESEARCHDEFINITION; 16749 if ("ResearchElementDefinition".equals(codeString)) 16750 return RESEARCHELEMENTDEFINITION; 16751 if ("ResearchStudy".equals(codeString)) 16752 return RESEARCHSTUDY; 16753 if ("ResearchSubject".equals(codeString)) 16754 return RESEARCHSUBJECT; 16755 if ("Resource".equals(codeString)) 16756 return RESOURCE; 16757 if ("RiskAssessment".equals(codeString)) 16758 return RISKASSESSMENT; 16759 if ("RiskEvidenceSynthesis".equals(codeString)) 16760 return RISKEVIDENCESYNTHESIS; 16761 if ("Schedule".equals(codeString)) 16762 return SCHEDULE; 16763 if ("SearchParameter".equals(codeString)) 16764 return SEARCHPARAMETER; 16765 if ("ServiceRequest".equals(codeString)) 16766 return SERVICEREQUEST; 16767 if ("Slot".equals(codeString)) 16768 return SLOT; 16769 if ("Specimen".equals(codeString)) 16770 return SPECIMEN; 16771 if ("SpecimenDefinition".equals(codeString)) 16772 return SPECIMENDEFINITION; 16773 if ("StructureDefinition".equals(codeString)) 16774 return STRUCTUREDEFINITION; 16775 if ("StructureMap".equals(codeString)) 16776 return STRUCTUREMAP; 16777 if ("Subscription".equals(codeString)) 16778 return SUBSCRIPTION; 16779 if ("Substance".equals(codeString)) 16780 return SUBSTANCE; 16781 if ("SubstanceNucleicAcid".equals(codeString)) 16782 return SUBSTANCENUCLEICACID; 16783 if ("SubstancePolymer".equals(codeString)) 16784 return SUBSTANCEPOLYMER; 16785 if ("SubstanceProtein".equals(codeString)) 16786 return SUBSTANCEPROTEIN; 16787 if ("SubstanceReferenceInformation".equals(codeString)) 16788 return SUBSTANCEREFERENCEINFORMATION; 16789 if ("SubstanceSourceMaterial".equals(codeString)) 16790 return SUBSTANCESOURCEMATERIAL; 16791 if ("SubstanceSpecification".equals(codeString)) 16792 return SUBSTANCESPECIFICATION; 16793 if ("SupplyDelivery".equals(codeString)) 16794 return SUPPLYDELIVERY; 16795 if ("SupplyRequest".equals(codeString)) 16796 return SUPPLYREQUEST; 16797 if ("Task".equals(codeString)) 16798 return TASK; 16799 if ("TerminologyCapabilities".equals(codeString)) 16800 return TERMINOLOGYCAPABILITIES; 16801 if ("TestReport".equals(codeString)) 16802 return TESTREPORT; 16803 if ("TestScript".equals(codeString)) 16804 return TESTSCRIPT; 16805 if ("ValueSet".equals(codeString)) 16806 return VALUESET; 16807 if ("VerificationResult".equals(codeString)) 16808 return VERIFICATIONRESULT; 16809 if ("VisionPrescription".equals(codeString)) 16810 return VISIONPRESCRIPTION; 16811 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 16812 } 16813 16814 public String toCode() { 16815 switch (this) { 16816 case ACCOUNT: 16817 return "Account"; 16818 case ACTIVITYDEFINITION: 16819 return "ActivityDefinition"; 16820 case ADVERSEEVENT: 16821 return "AdverseEvent"; 16822 case ALLERGYINTOLERANCE: 16823 return "AllergyIntolerance"; 16824 case APPOINTMENT: 16825 return "Appointment"; 16826 case APPOINTMENTRESPONSE: 16827 return "AppointmentResponse"; 16828 case AUDITEVENT: 16829 return "AuditEvent"; 16830 case BASIC: 16831 return "Basic"; 16832 case BINARY: 16833 return "Binary"; 16834 case BIOLOGICALLYDERIVEDPRODUCT: 16835 return "BiologicallyDerivedProduct"; 16836 case BODYSTRUCTURE: 16837 return "BodyStructure"; 16838 case BUNDLE: 16839 return "Bundle"; 16840 case CAPABILITYSTATEMENT: 16841 return "CapabilityStatement"; 16842 case CAREPLAN: 16843 return "CarePlan"; 16844 case CARETEAM: 16845 return "CareTeam"; 16846 case CATALOGENTRY: 16847 return "CatalogEntry"; 16848 case CHARGEITEM: 16849 return "ChargeItem"; 16850 case CHARGEITEMDEFINITION: 16851 return "ChargeItemDefinition"; 16852 case CLAIM: 16853 return "Claim"; 16854 case CLAIMRESPONSE: 16855 return "ClaimResponse"; 16856 case CLINICALIMPRESSION: 16857 return "ClinicalImpression"; 16858 case CODESYSTEM: 16859 return "CodeSystem"; 16860 case COMMUNICATION: 16861 return "Communication"; 16862 case COMMUNICATIONREQUEST: 16863 return "CommunicationRequest"; 16864 case COMPARTMENTDEFINITION: 16865 return "CompartmentDefinition"; 16866 case COMPOSITION: 16867 return "Composition"; 16868 case CONCEPTMAP: 16869 return "ConceptMap"; 16870 case CONDITION: 16871 return "Condition"; 16872 case CONSENT: 16873 return "Consent"; 16874 case CONTRACT: 16875 return "Contract"; 16876 case COVERAGE: 16877 return "Coverage"; 16878 case COVERAGEELIGIBILITYREQUEST: 16879 return "CoverageEligibilityRequest"; 16880 case COVERAGEELIGIBILITYRESPONSE: 16881 return "CoverageEligibilityResponse"; 16882 case DETECTEDISSUE: 16883 return "DetectedIssue"; 16884 case DEVICE: 16885 return "Device"; 16886 case DEVICEDEFINITION: 16887 return "DeviceDefinition"; 16888 case DEVICEMETRIC: 16889 return "DeviceMetric"; 16890 case DEVICEREQUEST: 16891 return "DeviceRequest"; 16892 case DEVICEUSESTATEMENT: 16893 return "DeviceUseStatement"; 16894 case DIAGNOSTICREPORT: 16895 return "DiagnosticReport"; 16896 case DOCUMENTMANIFEST: 16897 return "DocumentManifest"; 16898 case DOCUMENTREFERENCE: 16899 return "DocumentReference"; 16900 case DOMAINRESOURCE: 16901 return "DomainResource"; 16902 case EFFECTEVIDENCESYNTHESIS: 16903 return "EffectEvidenceSynthesis"; 16904 case ENCOUNTER: 16905 return "Encounter"; 16906 case ENDPOINT: 16907 return "Endpoint"; 16908 case ENROLLMENTREQUEST: 16909 return "EnrollmentRequest"; 16910 case ENROLLMENTRESPONSE: 16911 return "EnrollmentResponse"; 16912 case EPISODEOFCARE: 16913 return "EpisodeOfCare"; 16914 case EVENTDEFINITION: 16915 return "EventDefinition"; 16916 case EVIDENCE: 16917 return "Evidence"; 16918 case EVIDENCEVARIABLE: 16919 return "EvidenceVariable"; 16920 case EXAMPLESCENARIO: 16921 return "ExampleScenario"; 16922 case EXPLANATIONOFBENEFIT: 16923 return "ExplanationOfBenefit"; 16924 case FAMILYMEMBERHISTORY: 16925 return "FamilyMemberHistory"; 16926 case FLAG: 16927 return "Flag"; 16928 case GOAL: 16929 return "Goal"; 16930 case GRAPHDEFINITION: 16931 return "GraphDefinition"; 16932 case GROUP: 16933 return "Group"; 16934 case GUIDANCERESPONSE: 16935 return "GuidanceResponse"; 16936 case HEALTHCARESERVICE: 16937 return "HealthcareService"; 16938 case IMAGINGSTUDY: 16939 return "ImagingStudy"; 16940 case IMMUNIZATION: 16941 return "Immunization"; 16942 case IMMUNIZATIONEVALUATION: 16943 return "ImmunizationEvaluation"; 16944 case IMMUNIZATIONRECOMMENDATION: 16945 return "ImmunizationRecommendation"; 16946 case IMPLEMENTATIONGUIDE: 16947 return "ImplementationGuide"; 16948 case INSURANCEPLAN: 16949 return "InsurancePlan"; 16950 case INVOICE: 16951 return "Invoice"; 16952 case LIBRARY: 16953 return "Library"; 16954 case LINKAGE: 16955 return "Linkage"; 16956 case LIST: 16957 return "List"; 16958 case LOCATION: 16959 return "Location"; 16960 case MEASURE: 16961 return "Measure"; 16962 case MEASUREREPORT: 16963 return "MeasureReport"; 16964 case MEDIA: 16965 return "Media"; 16966 case MEDICATION: 16967 return "Medication"; 16968 case MEDICATIONADMINISTRATION: 16969 return "MedicationAdministration"; 16970 case MEDICATIONDISPENSE: 16971 return "MedicationDispense"; 16972 case MEDICATIONKNOWLEDGE: 16973 return "MedicationKnowledge"; 16974 case MEDICATIONREQUEST: 16975 return "MedicationRequest"; 16976 case MEDICATIONSTATEMENT: 16977 return "MedicationStatement"; 16978 case MEDICINALPRODUCT: 16979 return "MedicinalProduct"; 16980 case MEDICINALPRODUCTAUTHORIZATION: 16981 return "MedicinalProductAuthorization"; 16982 case MEDICINALPRODUCTCONTRAINDICATION: 16983 return "MedicinalProductContraindication"; 16984 case MEDICINALPRODUCTINDICATION: 16985 return "MedicinalProductIndication"; 16986 case MEDICINALPRODUCTINGREDIENT: 16987 return "MedicinalProductIngredient"; 16988 case MEDICINALPRODUCTINTERACTION: 16989 return "MedicinalProductInteraction"; 16990 case MEDICINALPRODUCTMANUFACTURED: 16991 return "MedicinalProductManufactured"; 16992 case MEDICINALPRODUCTPACKAGED: 16993 return "MedicinalProductPackaged"; 16994 case MEDICINALPRODUCTPHARMACEUTICAL: 16995 return "MedicinalProductPharmaceutical"; 16996 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 16997 return "MedicinalProductUndesirableEffect"; 16998 case MESSAGEDEFINITION: 16999 return "MessageDefinition"; 17000 case MESSAGEHEADER: 17001 return "MessageHeader"; 17002 case MOLECULARSEQUENCE: 17003 return "MolecularSequence"; 17004 case NAMINGSYSTEM: 17005 return "NamingSystem"; 17006 case NUTRITIONORDER: 17007 return "NutritionOrder"; 17008 case OBSERVATION: 17009 return "Observation"; 17010 case OBSERVATIONDEFINITION: 17011 return "ObservationDefinition"; 17012 case OPERATIONDEFINITION: 17013 return "OperationDefinition"; 17014 case OPERATIONOUTCOME: 17015 return "OperationOutcome"; 17016 case ORGANIZATION: 17017 return "Organization"; 17018 case ORGANIZATIONAFFILIATION: 17019 return "OrganizationAffiliation"; 17020 case PARAMETERS: 17021 return "Parameters"; 17022 case PATIENT: 17023 return "Patient"; 17024 case PAYMENTNOTICE: 17025 return "PaymentNotice"; 17026 case PAYMENTRECONCILIATION: 17027 return "PaymentReconciliation"; 17028 case PERSON: 17029 return "Person"; 17030 case PLANDEFINITION: 17031 return "PlanDefinition"; 17032 case PRACTITIONER: 17033 return "Practitioner"; 17034 case PRACTITIONERROLE: 17035 return "PractitionerRole"; 17036 case PROCEDURE: 17037 return "Procedure"; 17038 case PROVENANCE: 17039 return "Provenance"; 17040 case QUESTIONNAIRE: 17041 return "Questionnaire"; 17042 case QUESTIONNAIRERESPONSE: 17043 return "QuestionnaireResponse"; 17044 case RELATEDPERSON: 17045 return "RelatedPerson"; 17046 case REQUESTGROUP: 17047 return "RequestGroup"; 17048 case RESEARCHDEFINITION: 17049 return "ResearchDefinition"; 17050 case RESEARCHELEMENTDEFINITION: 17051 return "ResearchElementDefinition"; 17052 case RESEARCHSTUDY: 17053 return "ResearchStudy"; 17054 case RESEARCHSUBJECT: 17055 return "ResearchSubject"; 17056 case RESOURCE: 17057 return "Resource"; 17058 case RISKASSESSMENT: 17059 return "RiskAssessment"; 17060 case RISKEVIDENCESYNTHESIS: 17061 return "RiskEvidenceSynthesis"; 17062 case SCHEDULE: 17063 return "Schedule"; 17064 case SEARCHPARAMETER: 17065 return "SearchParameter"; 17066 case SERVICEREQUEST: 17067 return "ServiceRequest"; 17068 case SLOT: 17069 return "Slot"; 17070 case SPECIMEN: 17071 return "Specimen"; 17072 case SPECIMENDEFINITION: 17073 return "SpecimenDefinition"; 17074 case STRUCTUREDEFINITION: 17075 return "StructureDefinition"; 17076 case STRUCTUREMAP: 17077 return "StructureMap"; 17078 case SUBSCRIPTION: 17079 return "Subscription"; 17080 case SUBSTANCE: 17081 return "Substance"; 17082 case SUBSTANCENUCLEICACID: 17083 return "SubstanceNucleicAcid"; 17084 case SUBSTANCEPOLYMER: 17085 return "SubstancePolymer"; 17086 case SUBSTANCEPROTEIN: 17087 return "SubstanceProtein"; 17088 case SUBSTANCEREFERENCEINFORMATION: 17089 return "SubstanceReferenceInformation"; 17090 case SUBSTANCESOURCEMATERIAL: 17091 return "SubstanceSourceMaterial"; 17092 case SUBSTANCESPECIFICATION: 17093 return "SubstanceSpecification"; 17094 case SUPPLYDELIVERY: 17095 return "SupplyDelivery"; 17096 case SUPPLYREQUEST: 17097 return "SupplyRequest"; 17098 case TASK: 17099 return "Task"; 17100 case TERMINOLOGYCAPABILITIES: 17101 return "TerminologyCapabilities"; 17102 case TESTREPORT: 17103 return "TestReport"; 17104 case TESTSCRIPT: 17105 return "TestScript"; 17106 case VALUESET: 17107 return "ValueSet"; 17108 case VERIFICATIONRESULT: 17109 return "VerificationResult"; 17110 case VISIONPRESCRIPTION: 17111 return "VisionPrescription"; 17112 case NULL: 17113 return null; 17114 default: 17115 return "?"; 17116 } 17117 } 17118 17119 public String getSystem() { 17120 switch (this) { 17121 case ACCOUNT: 17122 return "http://hl7.org/fhir/resource-types"; 17123 case ACTIVITYDEFINITION: 17124 return "http://hl7.org/fhir/resource-types"; 17125 case ADVERSEEVENT: 17126 return "http://hl7.org/fhir/resource-types"; 17127 case ALLERGYINTOLERANCE: 17128 return "http://hl7.org/fhir/resource-types"; 17129 case APPOINTMENT: 17130 return "http://hl7.org/fhir/resource-types"; 17131 case APPOINTMENTRESPONSE: 17132 return "http://hl7.org/fhir/resource-types"; 17133 case AUDITEVENT: 17134 return "http://hl7.org/fhir/resource-types"; 17135 case BASIC: 17136 return "http://hl7.org/fhir/resource-types"; 17137 case BINARY: 17138 return "http://hl7.org/fhir/resource-types"; 17139 case BIOLOGICALLYDERIVEDPRODUCT: 17140 return "http://hl7.org/fhir/resource-types"; 17141 case BODYSTRUCTURE: 17142 return "http://hl7.org/fhir/resource-types"; 17143 case BUNDLE: 17144 return "http://hl7.org/fhir/resource-types"; 17145 case CAPABILITYSTATEMENT: 17146 return "http://hl7.org/fhir/resource-types"; 17147 case CAREPLAN: 17148 return "http://hl7.org/fhir/resource-types"; 17149 case CARETEAM: 17150 return "http://hl7.org/fhir/resource-types"; 17151 case CATALOGENTRY: 17152 return "http://hl7.org/fhir/resource-types"; 17153 case CHARGEITEM: 17154 return "http://hl7.org/fhir/resource-types"; 17155 case CHARGEITEMDEFINITION: 17156 return "http://hl7.org/fhir/resource-types"; 17157 case CLAIM: 17158 return "http://hl7.org/fhir/resource-types"; 17159 case CLAIMRESPONSE: 17160 return "http://hl7.org/fhir/resource-types"; 17161 case CLINICALIMPRESSION: 17162 return "http://hl7.org/fhir/resource-types"; 17163 case CODESYSTEM: 17164 return "http://hl7.org/fhir/resource-types"; 17165 case COMMUNICATION: 17166 return "http://hl7.org/fhir/resource-types"; 17167 case COMMUNICATIONREQUEST: 17168 return "http://hl7.org/fhir/resource-types"; 17169 case COMPARTMENTDEFINITION: 17170 return "http://hl7.org/fhir/resource-types"; 17171 case COMPOSITION: 17172 return "http://hl7.org/fhir/resource-types"; 17173 case CONCEPTMAP: 17174 return "http://hl7.org/fhir/resource-types"; 17175 case CONDITION: 17176 return "http://hl7.org/fhir/resource-types"; 17177 case CONSENT: 17178 return "http://hl7.org/fhir/resource-types"; 17179 case CONTRACT: 17180 return "http://hl7.org/fhir/resource-types"; 17181 case COVERAGE: 17182 return "http://hl7.org/fhir/resource-types"; 17183 case COVERAGEELIGIBILITYREQUEST: 17184 return "http://hl7.org/fhir/resource-types"; 17185 case COVERAGEELIGIBILITYRESPONSE: 17186 return "http://hl7.org/fhir/resource-types"; 17187 case DETECTEDISSUE: 17188 return "http://hl7.org/fhir/resource-types"; 17189 case DEVICE: 17190 return "http://hl7.org/fhir/resource-types"; 17191 case DEVICEDEFINITION: 17192 return "http://hl7.org/fhir/resource-types"; 17193 case DEVICEMETRIC: 17194 return "http://hl7.org/fhir/resource-types"; 17195 case DEVICEREQUEST: 17196 return "http://hl7.org/fhir/resource-types"; 17197 case DEVICEUSESTATEMENT: 17198 return "http://hl7.org/fhir/resource-types"; 17199 case DIAGNOSTICREPORT: 17200 return "http://hl7.org/fhir/resource-types"; 17201 case DOCUMENTMANIFEST: 17202 return "http://hl7.org/fhir/resource-types"; 17203 case DOCUMENTREFERENCE: 17204 return "http://hl7.org/fhir/resource-types"; 17205 case DOMAINRESOURCE: 17206 return "http://hl7.org/fhir/resource-types"; 17207 case EFFECTEVIDENCESYNTHESIS: 17208 return "http://hl7.org/fhir/resource-types"; 17209 case ENCOUNTER: 17210 return "http://hl7.org/fhir/resource-types"; 17211 case ENDPOINT: 17212 return "http://hl7.org/fhir/resource-types"; 17213 case ENROLLMENTREQUEST: 17214 return "http://hl7.org/fhir/resource-types"; 17215 case ENROLLMENTRESPONSE: 17216 return "http://hl7.org/fhir/resource-types"; 17217 case EPISODEOFCARE: 17218 return "http://hl7.org/fhir/resource-types"; 17219 case EVENTDEFINITION: 17220 return "http://hl7.org/fhir/resource-types"; 17221 case EVIDENCE: 17222 return "http://hl7.org/fhir/resource-types"; 17223 case EVIDENCEVARIABLE: 17224 return "http://hl7.org/fhir/resource-types"; 17225 case EXAMPLESCENARIO: 17226 return "http://hl7.org/fhir/resource-types"; 17227 case EXPLANATIONOFBENEFIT: 17228 return "http://hl7.org/fhir/resource-types"; 17229 case FAMILYMEMBERHISTORY: 17230 return "http://hl7.org/fhir/resource-types"; 17231 case FLAG: 17232 return "http://hl7.org/fhir/resource-types"; 17233 case GOAL: 17234 return "http://hl7.org/fhir/resource-types"; 17235 case GRAPHDEFINITION: 17236 return "http://hl7.org/fhir/resource-types"; 17237 case GROUP: 17238 return "http://hl7.org/fhir/resource-types"; 17239 case GUIDANCERESPONSE: 17240 return "http://hl7.org/fhir/resource-types"; 17241 case HEALTHCARESERVICE: 17242 return "http://hl7.org/fhir/resource-types"; 17243 case IMAGINGSTUDY: 17244 return "http://hl7.org/fhir/resource-types"; 17245 case IMMUNIZATION: 17246 return "http://hl7.org/fhir/resource-types"; 17247 case IMMUNIZATIONEVALUATION: 17248 return "http://hl7.org/fhir/resource-types"; 17249 case IMMUNIZATIONRECOMMENDATION: 17250 return "http://hl7.org/fhir/resource-types"; 17251 case IMPLEMENTATIONGUIDE: 17252 return "http://hl7.org/fhir/resource-types"; 17253 case INSURANCEPLAN: 17254 return "http://hl7.org/fhir/resource-types"; 17255 case INVOICE: 17256 return "http://hl7.org/fhir/resource-types"; 17257 case LIBRARY: 17258 return "http://hl7.org/fhir/resource-types"; 17259 case LINKAGE: 17260 return "http://hl7.org/fhir/resource-types"; 17261 case LIST: 17262 return "http://hl7.org/fhir/resource-types"; 17263 case LOCATION: 17264 return "http://hl7.org/fhir/resource-types"; 17265 case MEASURE: 17266 return "http://hl7.org/fhir/resource-types"; 17267 case MEASUREREPORT: 17268 return "http://hl7.org/fhir/resource-types"; 17269 case MEDIA: 17270 return "http://hl7.org/fhir/resource-types"; 17271 case MEDICATION: 17272 return "http://hl7.org/fhir/resource-types"; 17273 case MEDICATIONADMINISTRATION: 17274 return "http://hl7.org/fhir/resource-types"; 17275 case MEDICATIONDISPENSE: 17276 return "http://hl7.org/fhir/resource-types"; 17277 case MEDICATIONKNOWLEDGE: 17278 return "http://hl7.org/fhir/resource-types"; 17279 case MEDICATIONREQUEST: 17280 return "http://hl7.org/fhir/resource-types"; 17281 case MEDICATIONSTATEMENT: 17282 return "http://hl7.org/fhir/resource-types"; 17283 case MEDICINALPRODUCT: 17284 return "http://hl7.org/fhir/resource-types"; 17285 case MEDICINALPRODUCTAUTHORIZATION: 17286 return "http://hl7.org/fhir/resource-types"; 17287 case MEDICINALPRODUCTCONTRAINDICATION: 17288 return "http://hl7.org/fhir/resource-types"; 17289 case MEDICINALPRODUCTINDICATION: 17290 return "http://hl7.org/fhir/resource-types"; 17291 case MEDICINALPRODUCTINGREDIENT: 17292 return "http://hl7.org/fhir/resource-types"; 17293 case MEDICINALPRODUCTINTERACTION: 17294 return "http://hl7.org/fhir/resource-types"; 17295 case MEDICINALPRODUCTMANUFACTURED: 17296 return "http://hl7.org/fhir/resource-types"; 17297 case MEDICINALPRODUCTPACKAGED: 17298 return "http://hl7.org/fhir/resource-types"; 17299 case MEDICINALPRODUCTPHARMACEUTICAL: 17300 return "http://hl7.org/fhir/resource-types"; 17301 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17302 return "http://hl7.org/fhir/resource-types"; 17303 case MESSAGEDEFINITION: 17304 return "http://hl7.org/fhir/resource-types"; 17305 case MESSAGEHEADER: 17306 return "http://hl7.org/fhir/resource-types"; 17307 case MOLECULARSEQUENCE: 17308 return "http://hl7.org/fhir/resource-types"; 17309 case NAMINGSYSTEM: 17310 return "http://hl7.org/fhir/resource-types"; 17311 case NUTRITIONORDER: 17312 return "http://hl7.org/fhir/resource-types"; 17313 case OBSERVATION: 17314 return "http://hl7.org/fhir/resource-types"; 17315 case OBSERVATIONDEFINITION: 17316 return "http://hl7.org/fhir/resource-types"; 17317 case OPERATIONDEFINITION: 17318 return "http://hl7.org/fhir/resource-types"; 17319 case OPERATIONOUTCOME: 17320 return "http://hl7.org/fhir/resource-types"; 17321 case ORGANIZATION: 17322 return "http://hl7.org/fhir/resource-types"; 17323 case ORGANIZATIONAFFILIATION: 17324 return "http://hl7.org/fhir/resource-types"; 17325 case PARAMETERS: 17326 return "http://hl7.org/fhir/resource-types"; 17327 case PATIENT: 17328 return "http://hl7.org/fhir/resource-types"; 17329 case PAYMENTNOTICE: 17330 return "http://hl7.org/fhir/resource-types"; 17331 case PAYMENTRECONCILIATION: 17332 return "http://hl7.org/fhir/resource-types"; 17333 case PERSON: 17334 return "http://hl7.org/fhir/resource-types"; 17335 case PLANDEFINITION: 17336 return "http://hl7.org/fhir/resource-types"; 17337 case PRACTITIONER: 17338 return "http://hl7.org/fhir/resource-types"; 17339 case PRACTITIONERROLE: 17340 return "http://hl7.org/fhir/resource-types"; 17341 case PROCEDURE: 17342 return "http://hl7.org/fhir/resource-types"; 17343 case PROVENANCE: 17344 return "http://hl7.org/fhir/resource-types"; 17345 case QUESTIONNAIRE: 17346 return "http://hl7.org/fhir/resource-types"; 17347 case QUESTIONNAIRERESPONSE: 17348 return "http://hl7.org/fhir/resource-types"; 17349 case RELATEDPERSON: 17350 return "http://hl7.org/fhir/resource-types"; 17351 case REQUESTGROUP: 17352 return "http://hl7.org/fhir/resource-types"; 17353 case RESEARCHDEFINITION: 17354 return "http://hl7.org/fhir/resource-types"; 17355 case RESEARCHELEMENTDEFINITION: 17356 return "http://hl7.org/fhir/resource-types"; 17357 case RESEARCHSTUDY: 17358 return "http://hl7.org/fhir/resource-types"; 17359 case RESEARCHSUBJECT: 17360 return "http://hl7.org/fhir/resource-types"; 17361 case RESOURCE: 17362 return "http://hl7.org/fhir/resource-types"; 17363 case RISKASSESSMENT: 17364 return "http://hl7.org/fhir/resource-types"; 17365 case RISKEVIDENCESYNTHESIS: 17366 return "http://hl7.org/fhir/resource-types"; 17367 case SCHEDULE: 17368 return "http://hl7.org/fhir/resource-types"; 17369 case SEARCHPARAMETER: 17370 return "http://hl7.org/fhir/resource-types"; 17371 case SERVICEREQUEST: 17372 return "http://hl7.org/fhir/resource-types"; 17373 case SLOT: 17374 return "http://hl7.org/fhir/resource-types"; 17375 case SPECIMEN: 17376 return "http://hl7.org/fhir/resource-types"; 17377 case SPECIMENDEFINITION: 17378 return "http://hl7.org/fhir/resource-types"; 17379 case STRUCTUREDEFINITION: 17380 return "http://hl7.org/fhir/resource-types"; 17381 case STRUCTUREMAP: 17382 return "http://hl7.org/fhir/resource-types"; 17383 case SUBSCRIPTION: 17384 return "http://hl7.org/fhir/resource-types"; 17385 case SUBSTANCE: 17386 return "http://hl7.org/fhir/resource-types"; 17387 case SUBSTANCENUCLEICACID: 17388 return "http://hl7.org/fhir/resource-types"; 17389 case SUBSTANCEPOLYMER: 17390 return "http://hl7.org/fhir/resource-types"; 17391 case SUBSTANCEPROTEIN: 17392 return "http://hl7.org/fhir/resource-types"; 17393 case SUBSTANCEREFERENCEINFORMATION: 17394 return "http://hl7.org/fhir/resource-types"; 17395 case SUBSTANCESOURCEMATERIAL: 17396 return "http://hl7.org/fhir/resource-types"; 17397 case SUBSTANCESPECIFICATION: 17398 return "http://hl7.org/fhir/resource-types"; 17399 case SUPPLYDELIVERY: 17400 return "http://hl7.org/fhir/resource-types"; 17401 case SUPPLYREQUEST: 17402 return "http://hl7.org/fhir/resource-types"; 17403 case TASK: 17404 return "http://hl7.org/fhir/resource-types"; 17405 case TERMINOLOGYCAPABILITIES: 17406 return "http://hl7.org/fhir/resource-types"; 17407 case TESTREPORT: 17408 return "http://hl7.org/fhir/resource-types"; 17409 case TESTSCRIPT: 17410 return "http://hl7.org/fhir/resource-types"; 17411 case VALUESET: 17412 return "http://hl7.org/fhir/resource-types"; 17413 case VERIFICATIONRESULT: 17414 return "http://hl7.org/fhir/resource-types"; 17415 case VISIONPRESCRIPTION: 17416 return "http://hl7.org/fhir/resource-types"; 17417 case NULL: 17418 return null; 17419 default: 17420 return "?"; 17421 } 17422 } 17423 17424 public String getDefinition() { 17425 switch (this) { 17426 case ACCOUNT: 17427 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 17428 case ACTIVITYDEFINITION: 17429 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 17430 case ADVERSEEVENT: 17431 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 17432 case ALLERGYINTOLERANCE: 17433 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 17434 case APPOINTMENT: 17435 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 17436 case APPOINTMENTRESPONSE: 17437 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 17438 case AUDITEVENT: 17439 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 17440 case BASIC: 17441 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 17442 case BINARY: 17443 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 17444 case BIOLOGICALLYDERIVEDPRODUCT: 17445 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 17446 case BODYSTRUCTURE: 17447 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 17448 case BUNDLE: 17449 return "A container for a collection of resources."; 17450 case CAPABILITYSTATEMENT: 17451 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 17452 case CAREPLAN: 17453 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 17454 case CARETEAM: 17455 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 17456 case CATALOGENTRY: 17457 return "Catalog entries are wrappers that contextualize items included in a catalog."; 17458 case CHARGEITEM: 17459 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 17460 case CHARGEITEMDEFINITION: 17461 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 17462 case CLAIM: 17463 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 17464 case CLAIMRESPONSE: 17465 return "This resource provides the adjudication details from the processing of a Claim resource."; 17466 case CLINICALIMPRESSION: 17467 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 17468 case CODESYSTEM: 17469 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 17470 case COMMUNICATION: 17471 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 17472 case COMMUNICATIONREQUEST: 17473 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 17474 case COMPARTMENTDEFINITION: 17475 return "A compartment definition that defines how resources are accessed on a server."; 17476 case COMPOSITION: 17477 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 17478 case CONCEPTMAP: 17479 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 17480 case CONDITION: 17481 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 17482 case CONSENT: 17483 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 17484 case CONTRACT: 17485 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 17486 case COVERAGE: 17487 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 17488 case COVERAGEELIGIBILITYREQUEST: 17489 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 17490 case COVERAGEELIGIBILITYRESPONSE: 17491 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 17492 case DETECTEDISSUE: 17493 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 17494 case DEVICE: 17495 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 17496 case DEVICEDEFINITION: 17497 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 17498 case DEVICEMETRIC: 17499 return "Describes a measurement, calculation or setting capability of a medical device."; 17500 case DEVICEREQUEST: 17501 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 17502 case DEVICEUSESTATEMENT: 17503 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 17504 case DIAGNOSTICREPORT: 17505 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 17506 case DOCUMENTMANIFEST: 17507 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 17508 case DOCUMENTREFERENCE: 17509 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 17510 case DOMAINRESOURCE: 17511 return "A resource that includes narrative, extensions, and contained resources."; 17512 case EFFECTEVIDENCESYNTHESIS: 17513 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 17514 case ENCOUNTER: 17515 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 17516 case ENDPOINT: 17517 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 17518 case ENROLLMENTREQUEST: 17519 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 17520 case ENROLLMENTRESPONSE: 17521 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 17522 case EPISODEOFCARE: 17523 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 17524 case EVENTDEFINITION: 17525 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 17526 case EVIDENCE: 17527 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 17528 case EVIDENCEVARIABLE: 17529 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 17530 case EXAMPLESCENARIO: 17531 return "Example of workflow instance."; 17532 case EXPLANATIONOFBENEFIT: 17533 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 17534 case FAMILYMEMBERHISTORY: 17535 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 17536 case FLAG: 17537 return "Prospective warnings of potential issues when providing care to the patient."; 17538 case GOAL: 17539 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 17540 case GRAPHDEFINITION: 17541 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 17542 case GROUP: 17543 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 17544 case GUIDANCERESPONSE: 17545 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 17546 case HEALTHCARESERVICE: 17547 return "The details of a healthcare service available at a location."; 17548 case IMAGINGSTUDY: 17549 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 17550 case IMMUNIZATION: 17551 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 17552 case IMMUNIZATIONEVALUATION: 17553 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 17554 case IMMUNIZATIONRECOMMENDATION: 17555 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 17556 case IMPLEMENTATIONGUIDE: 17557 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 17558 case INSURANCEPLAN: 17559 return "Details of a Health Insurance product/plan provided by an organization."; 17560 case INVOICE: 17561 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 17562 case LIBRARY: 17563 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 17564 case LINKAGE: 17565 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 17566 case LIST: 17567 return "A list is a curated collection of resources."; 17568 case LOCATION: 17569 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 17570 case MEASURE: 17571 return "The Measure resource provides the definition of a quality measure."; 17572 case MEASUREREPORT: 17573 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 17574 case MEDIA: 17575 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 17576 case MEDICATION: 17577 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 17578 case MEDICATIONADMINISTRATION: 17579 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 17580 case MEDICATIONDISPENSE: 17581 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 17582 case MEDICATIONKNOWLEDGE: 17583 return "Information about a medication that is used to support knowledge."; 17584 case MEDICATIONREQUEST: 17585 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 17586 case MEDICATIONSTATEMENT: 17587 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 17588 case MEDICINALPRODUCT: 17589 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 17590 case MEDICINALPRODUCTAUTHORIZATION: 17591 return "The regulatory authorization of a medicinal product."; 17592 case MEDICINALPRODUCTCONTRAINDICATION: 17593 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 17594 case MEDICINALPRODUCTINDICATION: 17595 return "Indication for the Medicinal Product."; 17596 case MEDICINALPRODUCTINGREDIENT: 17597 return "An ingredient of a manufactured item or pharmaceutical product."; 17598 case MEDICINALPRODUCTINTERACTION: 17599 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 17600 case MEDICINALPRODUCTMANUFACTURED: 17601 return "The manufactured item as contained in the packaged medicinal product."; 17602 case MEDICINALPRODUCTPACKAGED: 17603 return "A medicinal product in a container or package."; 17604 case MEDICINALPRODUCTPHARMACEUTICAL: 17605 return "A pharmaceutical product described in terms of its composition and dose form."; 17606 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17607 return "Describe the undesirable effects of the medicinal product."; 17608 case MESSAGEDEFINITION: 17609 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 17610 case MESSAGEHEADER: 17611 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 17612 case MOLECULARSEQUENCE: 17613 return "Raw data describing a biological sequence."; 17614 case NAMINGSYSTEM: 17615 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 17616 case NUTRITIONORDER: 17617 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 17618 case OBSERVATION: 17619 return "Measurements and simple assertions made about a patient, device or other subject."; 17620 case OBSERVATIONDEFINITION: 17621 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 17622 case OPERATIONDEFINITION: 17623 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 17624 case OPERATIONOUTCOME: 17625 return "A collection of error, warning, or information messages that result from a system action."; 17626 case ORGANIZATION: 17627 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 17628 case ORGANIZATIONAFFILIATION: 17629 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 17630 case PARAMETERS: 17631 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 17632 case PATIENT: 17633 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 17634 case PAYMENTNOTICE: 17635 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 17636 case PAYMENTRECONCILIATION: 17637 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 17638 case PERSON: 17639 return "Demographics and administrative information about a person independent of a specific health-related context."; 17640 case PLANDEFINITION: 17641 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 17642 case PRACTITIONER: 17643 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 17644 case PRACTITIONERROLE: 17645 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 17646 case PROCEDURE: 17647 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 17648 case PROVENANCE: 17649 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 17650 case QUESTIONNAIRE: 17651 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 17652 case QUESTIONNAIRERESPONSE: 17653 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 17654 case RELATEDPERSON: 17655 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 17656 case REQUESTGROUP: 17657 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 17658 case RESEARCHDEFINITION: 17659 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 17660 case RESEARCHELEMENTDEFINITION: 17661 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 17662 case RESEARCHSTUDY: 17663 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 17664 case RESEARCHSUBJECT: 17665 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 17666 case RESOURCE: 17667 return "This is the base resource type for everything."; 17668 case RISKASSESSMENT: 17669 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 17670 case RISKEVIDENCESYNTHESIS: 17671 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 17672 case SCHEDULE: 17673 return "A container for slots of time that may be available for booking appointments."; 17674 case SEARCHPARAMETER: 17675 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 17676 case SERVICEREQUEST: 17677 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 17678 case SLOT: 17679 return "A slot of time on a schedule that may be available for booking appointments."; 17680 case SPECIMEN: 17681 return "A sample to be used for analysis."; 17682 case SPECIMENDEFINITION: 17683 return "A kind of specimen with associated set of requirements."; 17684 case STRUCTUREDEFINITION: 17685 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 17686 case STRUCTUREMAP: 17687 return "A Map of relationships between 2 structures that can be used to transform data."; 17688 case SUBSCRIPTION: 17689 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 17690 case SUBSTANCE: 17691 return "A homogeneous material with a definite composition."; 17692 case SUBSTANCENUCLEICACID: 17693 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 17694 case SUBSTANCEPOLYMER: 17695 return "Todo."; 17696 case SUBSTANCEPROTEIN: 17697 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 17698 case SUBSTANCEREFERENCEINFORMATION: 17699 return "Todo."; 17700 case SUBSTANCESOURCEMATERIAL: 17701 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 17702 case SUBSTANCESPECIFICATION: 17703 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 17704 case SUPPLYDELIVERY: 17705 return "Record of delivery of what is supplied."; 17706 case SUPPLYREQUEST: 17707 return "A record of a request for a medication, substance or device used in the healthcare setting."; 17708 case TASK: 17709 return "A task to be performed."; 17710 case TERMINOLOGYCAPABILITIES: 17711 return "A Terminology Capabilities documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 17712 case TESTREPORT: 17713 return "A summary of information based on the results of executing a TestScript."; 17714 case TESTSCRIPT: 17715 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 17716 case VALUESET: 17717 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 17718 case VERIFICATIONRESULT: 17719 return "Describes validation requirements, source(s), status and dates for one or more elements."; 17720 case VISIONPRESCRIPTION: 17721 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 17722 case NULL: 17723 return null; 17724 default: 17725 return "?"; 17726 } 17727 } 17728 17729 public String getDisplay() { 17730 switch (this) { 17731 case ACCOUNT: 17732 return "Account"; 17733 case ACTIVITYDEFINITION: 17734 return "ActivityDefinition"; 17735 case ADVERSEEVENT: 17736 return "AdverseEvent"; 17737 case ALLERGYINTOLERANCE: 17738 return "AllergyIntolerance"; 17739 case APPOINTMENT: 17740 return "Appointment"; 17741 case APPOINTMENTRESPONSE: 17742 return "AppointmentResponse"; 17743 case AUDITEVENT: 17744 return "AuditEvent"; 17745 case BASIC: 17746 return "Basic"; 17747 case BINARY: 17748 return "Binary"; 17749 case BIOLOGICALLYDERIVEDPRODUCT: 17750 return "BiologicallyDerivedProduct"; 17751 case BODYSTRUCTURE: 17752 return "BodyStructure"; 17753 case BUNDLE: 17754 return "Bundle"; 17755 case CAPABILITYSTATEMENT: 17756 return "CapabilityStatement"; 17757 case CAREPLAN: 17758 return "CarePlan"; 17759 case CARETEAM: 17760 return "CareTeam"; 17761 case CATALOGENTRY: 17762 return "CatalogEntry"; 17763 case CHARGEITEM: 17764 return "ChargeItem"; 17765 case CHARGEITEMDEFINITION: 17766 return "ChargeItemDefinition"; 17767 case CLAIM: 17768 return "Claim"; 17769 case CLAIMRESPONSE: 17770 return "ClaimResponse"; 17771 case CLINICALIMPRESSION: 17772 return "ClinicalImpression"; 17773 case CODESYSTEM: 17774 return "CodeSystem"; 17775 case COMMUNICATION: 17776 return "Communication"; 17777 case COMMUNICATIONREQUEST: 17778 return "CommunicationRequest"; 17779 case COMPARTMENTDEFINITION: 17780 return "CompartmentDefinition"; 17781 case COMPOSITION: 17782 return "Composition"; 17783 case CONCEPTMAP: 17784 return "ConceptMap"; 17785 case CONDITION: 17786 return "Condition"; 17787 case CONSENT: 17788 return "Consent"; 17789 case CONTRACT: 17790 return "Contract"; 17791 case COVERAGE: 17792 return "Coverage"; 17793 case COVERAGEELIGIBILITYREQUEST: 17794 return "CoverageEligibilityRequest"; 17795 case COVERAGEELIGIBILITYRESPONSE: 17796 return "CoverageEligibilityResponse"; 17797 case DETECTEDISSUE: 17798 return "DetectedIssue"; 17799 case DEVICE: 17800 return "Device"; 17801 case DEVICEDEFINITION: 17802 return "DeviceDefinition"; 17803 case DEVICEMETRIC: 17804 return "DeviceMetric"; 17805 case DEVICEREQUEST: 17806 return "DeviceRequest"; 17807 case DEVICEUSESTATEMENT: 17808 return "DeviceUseStatement"; 17809 case DIAGNOSTICREPORT: 17810 return "DiagnosticReport"; 17811 case DOCUMENTMANIFEST: 17812 return "DocumentManifest"; 17813 case DOCUMENTREFERENCE: 17814 return "DocumentReference"; 17815 case DOMAINRESOURCE: 17816 return "DomainResource"; 17817 case EFFECTEVIDENCESYNTHESIS: 17818 return "EffectEvidenceSynthesis"; 17819 case ENCOUNTER: 17820 return "Encounter"; 17821 case ENDPOINT: 17822 return "Endpoint"; 17823 case ENROLLMENTREQUEST: 17824 return "EnrollmentRequest"; 17825 case ENROLLMENTRESPONSE: 17826 return "EnrollmentResponse"; 17827 case EPISODEOFCARE: 17828 return "EpisodeOfCare"; 17829 case EVENTDEFINITION: 17830 return "EventDefinition"; 17831 case EVIDENCE: 17832 return "Evidence"; 17833 case EVIDENCEVARIABLE: 17834 return "EvidenceVariable"; 17835 case EXAMPLESCENARIO: 17836 return "ExampleScenario"; 17837 case EXPLANATIONOFBENEFIT: 17838 return "ExplanationOfBenefit"; 17839 case FAMILYMEMBERHISTORY: 17840 return "FamilyMemberHistory"; 17841 case FLAG: 17842 return "Flag"; 17843 case GOAL: 17844 return "Goal"; 17845 case GRAPHDEFINITION: 17846 return "GraphDefinition"; 17847 case GROUP: 17848 return "Group"; 17849 case GUIDANCERESPONSE: 17850 return "GuidanceResponse"; 17851 case HEALTHCARESERVICE: 17852 return "HealthcareService"; 17853 case IMAGINGSTUDY: 17854 return "ImagingStudy"; 17855 case IMMUNIZATION: 17856 return "Immunization"; 17857 case IMMUNIZATIONEVALUATION: 17858 return "ImmunizationEvaluation"; 17859 case IMMUNIZATIONRECOMMENDATION: 17860 return "ImmunizationRecommendation"; 17861 case IMPLEMENTATIONGUIDE: 17862 return "ImplementationGuide"; 17863 case INSURANCEPLAN: 17864 return "InsurancePlan"; 17865 case INVOICE: 17866 return "Invoice"; 17867 case LIBRARY: 17868 return "Library"; 17869 case LINKAGE: 17870 return "Linkage"; 17871 case LIST: 17872 return "List"; 17873 case LOCATION: 17874 return "Location"; 17875 case MEASURE: 17876 return "Measure"; 17877 case MEASUREREPORT: 17878 return "MeasureReport"; 17879 case MEDIA: 17880 return "Media"; 17881 case MEDICATION: 17882 return "Medication"; 17883 case MEDICATIONADMINISTRATION: 17884 return "MedicationAdministration"; 17885 case MEDICATIONDISPENSE: 17886 return "MedicationDispense"; 17887 case MEDICATIONKNOWLEDGE: 17888 return "MedicationKnowledge"; 17889 case MEDICATIONREQUEST: 17890 return "MedicationRequest"; 17891 case MEDICATIONSTATEMENT: 17892 return "MedicationStatement"; 17893 case MEDICINALPRODUCT: 17894 return "MedicinalProduct"; 17895 case MEDICINALPRODUCTAUTHORIZATION: 17896 return "MedicinalProductAuthorization"; 17897 case MEDICINALPRODUCTCONTRAINDICATION: 17898 return "MedicinalProductContraindication"; 17899 case MEDICINALPRODUCTINDICATION: 17900 return "MedicinalProductIndication"; 17901 case MEDICINALPRODUCTINGREDIENT: 17902 return "MedicinalProductIngredient"; 17903 case MEDICINALPRODUCTINTERACTION: 17904 return "MedicinalProductInteraction"; 17905 case MEDICINALPRODUCTMANUFACTURED: 17906 return "MedicinalProductManufactured"; 17907 case MEDICINALPRODUCTPACKAGED: 17908 return "MedicinalProductPackaged"; 17909 case MEDICINALPRODUCTPHARMACEUTICAL: 17910 return "MedicinalProductPharmaceutical"; 17911 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 17912 return "MedicinalProductUndesirableEffect"; 17913 case MESSAGEDEFINITION: 17914 return "MessageDefinition"; 17915 case MESSAGEHEADER: 17916 return "MessageHeader"; 17917 case MOLECULARSEQUENCE: 17918 return "MolecularSequence"; 17919 case NAMINGSYSTEM: 17920 return "NamingSystem"; 17921 case NUTRITIONORDER: 17922 return "NutritionOrder"; 17923 case OBSERVATION: 17924 return "Observation"; 17925 case OBSERVATIONDEFINITION: 17926 return "ObservationDefinition"; 17927 case OPERATIONDEFINITION: 17928 return "OperationDefinition"; 17929 case OPERATIONOUTCOME: 17930 return "OperationOutcome"; 17931 case ORGANIZATION: 17932 return "Organization"; 17933 case ORGANIZATIONAFFILIATION: 17934 return "OrganizationAffiliation"; 17935 case PARAMETERS: 17936 return "Parameters"; 17937 case PATIENT: 17938 return "Patient"; 17939 case PAYMENTNOTICE: 17940 return "PaymentNotice"; 17941 case PAYMENTRECONCILIATION: 17942 return "PaymentReconciliation"; 17943 case PERSON: 17944 return "Person"; 17945 case PLANDEFINITION: 17946 return "PlanDefinition"; 17947 case PRACTITIONER: 17948 return "Practitioner"; 17949 case PRACTITIONERROLE: 17950 return "PractitionerRole"; 17951 case PROCEDURE: 17952 return "Procedure"; 17953 case PROVENANCE: 17954 return "Provenance"; 17955 case QUESTIONNAIRE: 17956 return "Questionnaire"; 17957 case QUESTIONNAIRERESPONSE: 17958 return "QuestionnaireResponse"; 17959 case RELATEDPERSON: 17960 return "RelatedPerson"; 17961 case REQUESTGROUP: 17962 return "RequestGroup"; 17963 case RESEARCHDEFINITION: 17964 return "ResearchDefinition"; 17965 case RESEARCHELEMENTDEFINITION: 17966 return "ResearchElementDefinition"; 17967 case RESEARCHSTUDY: 17968 return "ResearchStudy"; 17969 case RESEARCHSUBJECT: 17970 return "ResearchSubject"; 17971 case RESOURCE: 17972 return "Resource"; 17973 case RISKASSESSMENT: 17974 return "RiskAssessment"; 17975 case RISKEVIDENCESYNTHESIS: 17976 return "RiskEvidenceSynthesis"; 17977 case SCHEDULE: 17978 return "Schedule"; 17979 case SEARCHPARAMETER: 17980 return "SearchParameter"; 17981 case SERVICEREQUEST: 17982 return "ServiceRequest"; 17983 case SLOT: 17984 return "Slot"; 17985 case SPECIMEN: 17986 return "Specimen"; 17987 case SPECIMENDEFINITION: 17988 return "SpecimenDefinition"; 17989 case STRUCTUREDEFINITION: 17990 return "StructureDefinition"; 17991 case STRUCTUREMAP: 17992 return "StructureMap"; 17993 case SUBSCRIPTION: 17994 return "Subscription"; 17995 case SUBSTANCE: 17996 return "Substance"; 17997 case SUBSTANCENUCLEICACID: 17998 return "SubstanceNucleicAcid"; 17999 case SUBSTANCEPOLYMER: 18000 return "SubstancePolymer"; 18001 case SUBSTANCEPROTEIN: 18002 return "SubstanceProtein"; 18003 case SUBSTANCEREFERENCEINFORMATION: 18004 return "SubstanceReferenceInformation"; 18005 case SUBSTANCESOURCEMATERIAL: 18006 return "SubstanceSourceMaterial"; 18007 case SUBSTANCESPECIFICATION: 18008 return "SubstanceSpecification"; 18009 case SUPPLYDELIVERY: 18010 return "SupplyDelivery"; 18011 case SUPPLYREQUEST: 18012 return "SupplyRequest"; 18013 case TASK: 18014 return "Task"; 18015 case TERMINOLOGYCAPABILITIES: 18016 return "TerminologyCapabilities"; 18017 case TESTREPORT: 18018 return "TestReport"; 18019 case TESTSCRIPT: 18020 return "TestScript"; 18021 case VALUESET: 18022 return "ValueSet"; 18023 case VERIFICATIONRESULT: 18024 return "VerificationResult"; 18025 case VISIONPRESCRIPTION: 18026 return "VisionPrescription"; 18027 case NULL: 18028 return null; 18029 default: 18030 return "?"; 18031 } 18032 } 18033 } 18034 18035 public static class ResourceTypeEnumFactory implements EnumFactory<ResourceType> { 18036 public ResourceType fromCode(String codeString) throws IllegalArgumentException { 18037 if (codeString == null || "".equals(codeString)) 18038 if (codeString == null || "".equals(codeString)) 18039 return null; 18040 if ("Account".equals(codeString)) 18041 return ResourceType.ACCOUNT; 18042 if ("ActivityDefinition".equals(codeString)) 18043 return ResourceType.ACTIVITYDEFINITION; 18044 if ("AdverseEvent".equals(codeString)) 18045 return ResourceType.ADVERSEEVENT; 18046 if ("AllergyIntolerance".equals(codeString)) 18047 return ResourceType.ALLERGYINTOLERANCE; 18048 if ("Appointment".equals(codeString)) 18049 return ResourceType.APPOINTMENT; 18050 if ("AppointmentResponse".equals(codeString)) 18051 return ResourceType.APPOINTMENTRESPONSE; 18052 if ("AuditEvent".equals(codeString)) 18053 return ResourceType.AUDITEVENT; 18054 if ("Basic".equals(codeString)) 18055 return ResourceType.BASIC; 18056 if ("Binary".equals(codeString)) 18057 return ResourceType.BINARY; 18058 if ("BiologicallyDerivedProduct".equals(codeString)) 18059 return ResourceType.BIOLOGICALLYDERIVEDPRODUCT; 18060 if ("BodyStructure".equals(codeString)) 18061 return ResourceType.BODYSTRUCTURE; 18062 if ("Bundle".equals(codeString)) 18063 return ResourceType.BUNDLE; 18064 if ("CapabilityStatement".equals(codeString)) 18065 return ResourceType.CAPABILITYSTATEMENT; 18066 if ("CarePlan".equals(codeString)) 18067 return ResourceType.CAREPLAN; 18068 if ("CareTeam".equals(codeString)) 18069 return ResourceType.CARETEAM; 18070 if ("CatalogEntry".equals(codeString)) 18071 return ResourceType.CATALOGENTRY; 18072 if ("ChargeItem".equals(codeString)) 18073 return ResourceType.CHARGEITEM; 18074 if ("ChargeItemDefinition".equals(codeString)) 18075 return ResourceType.CHARGEITEMDEFINITION; 18076 if ("Claim".equals(codeString)) 18077 return ResourceType.CLAIM; 18078 if ("ClaimResponse".equals(codeString)) 18079 return ResourceType.CLAIMRESPONSE; 18080 if ("ClinicalImpression".equals(codeString)) 18081 return ResourceType.CLINICALIMPRESSION; 18082 if ("CodeSystem".equals(codeString)) 18083 return ResourceType.CODESYSTEM; 18084 if ("Communication".equals(codeString)) 18085 return ResourceType.COMMUNICATION; 18086 if ("CommunicationRequest".equals(codeString)) 18087 return ResourceType.COMMUNICATIONREQUEST; 18088 if ("CompartmentDefinition".equals(codeString)) 18089 return ResourceType.COMPARTMENTDEFINITION; 18090 if ("Composition".equals(codeString)) 18091 return ResourceType.COMPOSITION; 18092 if ("ConceptMap".equals(codeString)) 18093 return ResourceType.CONCEPTMAP; 18094 if ("Condition".equals(codeString)) 18095 return ResourceType.CONDITION; 18096 if ("Consent".equals(codeString)) 18097 return ResourceType.CONSENT; 18098 if ("Contract".equals(codeString)) 18099 return ResourceType.CONTRACT; 18100 if ("Coverage".equals(codeString)) 18101 return ResourceType.COVERAGE; 18102 if ("CoverageEligibilityRequest".equals(codeString)) 18103 return ResourceType.COVERAGEELIGIBILITYREQUEST; 18104 if ("CoverageEligibilityResponse".equals(codeString)) 18105 return ResourceType.COVERAGEELIGIBILITYRESPONSE; 18106 if ("DetectedIssue".equals(codeString)) 18107 return ResourceType.DETECTEDISSUE; 18108 if ("Device".equals(codeString)) 18109 return ResourceType.DEVICE; 18110 if ("DeviceDefinition".equals(codeString)) 18111 return ResourceType.DEVICEDEFINITION; 18112 if ("DeviceMetric".equals(codeString)) 18113 return ResourceType.DEVICEMETRIC; 18114 if ("DeviceRequest".equals(codeString)) 18115 return ResourceType.DEVICEREQUEST; 18116 if ("DeviceUseStatement".equals(codeString)) 18117 return ResourceType.DEVICEUSESTATEMENT; 18118 if ("DiagnosticReport".equals(codeString)) 18119 return ResourceType.DIAGNOSTICREPORT; 18120 if ("DocumentManifest".equals(codeString)) 18121 return ResourceType.DOCUMENTMANIFEST; 18122 if ("DocumentReference".equals(codeString)) 18123 return ResourceType.DOCUMENTREFERENCE; 18124 if ("DomainResource".equals(codeString)) 18125 return ResourceType.DOMAINRESOURCE; 18126 if ("EffectEvidenceSynthesis".equals(codeString)) 18127 return ResourceType.EFFECTEVIDENCESYNTHESIS; 18128 if ("Encounter".equals(codeString)) 18129 return ResourceType.ENCOUNTER; 18130 if ("Endpoint".equals(codeString)) 18131 return ResourceType.ENDPOINT; 18132 if ("EnrollmentRequest".equals(codeString)) 18133 return ResourceType.ENROLLMENTREQUEST; 18134 if ("EnrollmentResponse".equals(codeString)) 18135 return ResourceType.ENROLLMENTRESPONSE; 18136 if ("EpisodeOfCare".equals(codeString)) 18137 return ResourceType.EPISODEOFCARE; 18138 if ("EventDefinition".equals(codeString)) 18139 return ResourceType.EVENTDEFINITION; 18140 if ("Evidence".equals(codeString)) 18141 return ResourceType.EVIDENCE; 18142 if ("EvidenceVariable".equals(codeString)) 18143 return ResourceType.EVIDENCEVARIABLE; 18144 if ("ExampleScenario".equals(codeString)) 18145 return ResourceType.EXAMPLESCENARIO; 18146 if ("ExplanationOfBenefit".equals(codeString)) 18147 return ResourceType.EXPLANATIONOFBENEFIT; 18148 if ("FamilyMemberHistory".equals(codeString)) 18149 return ResourceType.FAMILYMEMBERHISTORY; 18150 if ("Flag".equals(codeString)) 18151 return ResourceType.FLAG; 18152 if ("Goal".equals(codeString)) 18153 return ResourceType.GOAL; 18154 if ("GraphDefinition".equals(codeString)) 18155 return ResourceType.GRAPHDEFINITION; 18156 if ("Group".equals(codeString)) 18157 return ResourceType.GROUP; 18158 if ("GuidanceResponse".equals(codeString)) 18159 return ResourceType.GUIDANCERESPONSE; 18160 if ("HealthcareService".equals(codeString)) 18161 return ResourceType.HEALTHCARESERVICE; 18162 if ("ImagingStudy".equals(codeString)) 18163 return ResourceType.IMAGINGSTUDY; 18164 if ("Immunization".equals(codeString)) 18165 return ResourceType.IMMUNIZATION; 18166 if ("ImmunizationEvaluation".equals(codeString)) 18167 return ResourceType.IMMUNIZATIONEVALUATION; 18168 if ("ImmunizationRecommendation".equals(codeString)) 18169 return ResourceType.IMMUNIZATIONRECOMMENDATION; 18170 if ("ImplementationGuide".equals(codeString)) 18171 return ResourceType.IMPLEMENTATIONGUIDE; 18172 if ("InsurancePlan".equals(codeString)) 18173 return ResourceType.INSURANCEPLAN; 18174 if ("Invoice".equals(codeString)) 18175 return ResourceType.INVOICE; 18176 if ("Library".equals(codeString)) 18177 return ResourceType.LIBRARY; 18178 if ("Linkage".equals(codeString)) 18179 return ResourceType.LINKAGE; 18180 if ("List".equals(codeString)) 18181 return ResourceType.LIST; 18182 if ("Location".equals(codeString)) 18183 return ResourceType.LOCATION; 18184 if ("Measure".equals(codeString)) 18185 return ResourceType.MEASURE; 18186 if ("MeasureReport".equals(codeString)) 18187 return ResourceType.MEASUREREPORT; 18188 if ("Media".equals(codeString)) 18189 return ResourceType.MEDIA; 18190 if ("Medication".equals(codeString)) 18191 return ResourceType.MEDICATION; 18192 if ("MedicationAdministration".equals(codeString)) 18193 return ResourceType.MEDICATIONADMINISTRATION; 18194 if ("MedicationDispense".equals(codeString)) 18195 return ResourceType.MEDICATIONDISPENSE; 18196 if ("MedicationKnowledge".equals(codeString)) 18197 return ResourceType.MEDICATIONKNOWLEDGE; 18198 if ("MedicationRequest".equals(codeString)) 18199 return ResourceType.MEDICATIONREQUEST; 18200 if ("MedicationStatement".equals(codeString)) 18201 return ResourceType.MEDICATIONSTATEMENT; 18202 if ("MedicinalProduct".equals(codeString)) 18203 return ResourceType.MEDICINALPRODUCT; 18204 if ("MedicinalProductAuthorization".equals(codeString)) 18205 return ResourceType.MEDICINALPRODUCTAUTHORIZATION; 18206 if ("MedicinalProductContraindication".equals(codeString)) 18207 return ResourceType.MEDICINALPRODUCTCONTRAINDICATION; 18208 if ("MedicinalProductIndication".equals(codeString)) 18209 return ResourceType.MEDICINALPRODUCTINDICATION; 18210 if ("MedicinalProductIngredient".equals(codeString)) 18211 return ResourceType.MEDICINALPRODUCTINGREDIENT; 18212 if ("MedicinalProductInteraction".equals(codeString)) 18213 return ResourceType.MEDICINALPRODUCTINTERACTION; 18214 if ("MedicinalProductManufactured".equals(codeString)) 18215 return ResourceType.MEDICINALPRODUCTMANUFACTURED; 18216 if ("MedicinalProductPackaged".equals(codeString)) 18217 return ResourceType.MEDICINALPRODUCTPACKAGED; 18218 if ("MedicinalProductPharmaceutical".equals(codeString)) 18219 return ResourceType.MEDICINALPRODUCTPHARMACEUTICAL; 18220 if ("MedicinalProductUndesirableEffect".equals(codeString)) 18221 return ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 18222 if ("MessageDefinition".equals(codeString)) 18223 return ResourceType.MESSAGEDEFINITION; 18224 if ("MessageHeader".equals(codeString)) 18225 return ResourceType.MESSAGEHEADER; 18226 if ("MolecularSequence".equals(codeString)) 18227 return ResourceType.MOLECULARSEQUENCE; 18228 if ("NamingSystem".equals(codeString)) 18229 return ResourceType.NAMINGSYSTEM; 18230 if ("NutritionOrder".equals(codeString)) 18231 return ResourceType.NUTRITIONORDER; 18232 if ("Observation".equals(codeString)) 18233 return ResourceType.OBSERVATION; 18234 if ("ObservationDefinition".equals(codeString)) 18235 return ResourceType.OBSERVATIONDEFINITION; 18236 if ("OperationDefinition".equals(codeString)) 18237 return ResourceType.OPERATIONDEFINITION; 18238 if ("OperationOutcome".equals(codeString)) 18239 return ResourceType.OPERATIONOUTCOME; 18240 if ("Organization".equals(codeString)) 18241 return ResourceType.ORGANIZATION; 18242 if ("OrganizationAffiliation".equals(codeString)) 18243 return ResourceType.ORGANIZATIONAFFILIATION; 18244 if ("Parameters".equals(codeString)) 18245 return ResourceType.PARAMETERS; 18246 if ("Patient".equals(codeString)) 18247 return ResourceType.PATIENT; 18248 if ("PaymentNotice".equals(codeString)) 18249 return ResourceType.PAYMENTNOTICE; 18250 if ("PaymentReconciliation".equals(codeString)) 18251 return ResourceType.PAYMENTRECONCILIATION; 18252 if ("Person".equals(codeString)) 18253 return ResourceType.PERSON; 18254 if ("PlanDefinition".equals(codeString)) 18255 return ResourceType.PLANDEFINITION; 18256 if ("Practitioner".equals(codeString)) 18257 return ResourceType.PRACTITIONER; 18258 if ("PractitionerRole".equals(codeString)) 18259 return ResourceType.PRACTITIONERROLE; 18260 if ("Procedure".equals(codeString)) 18261 return ResourceType.PROCEDURE; 18262 if ("Provenance".equals(codeString)) 18263 return ResourceType.PROVENANCE; 18264 if ("Questionnaire".equals(codeString)) 18265 return ResourceType.QUESTIONNAIRE; 18266 if ("QuestionnaireResponse".equals(codeString)) 18267 return ResourceType.QUESTIONNAIRERESPONSE; 18268 if ("RelatedPerson".equals(codeString)) 18269 return ResourceType.RELATEDPERSON; 18270 if ("RequestGroup".equals(codeString)) 18271 return ResourceType.REQUESTGROUP; 18272 if ("ResearchDefinition".equals(codeString)) 18273 return ResourceType.RESEARCHDEFINITION; 18274 if ("ResearchElementDefinition".equals(codeString)) 18275 return ResourceType.RESEARCHELEMENTDEFINITION; 18276 if ("ResearchStudy".equals(codeString)) 18277 return ResourceType.RESEARCHSTUDY; 18278 if ("ResearchSubject".equals(codeString)) 18279 return ResourceType.RESEARCHSUBJECT; 18280 if ("Resource".equals(codeString)) 18281 return ResourceType.RESOURCE; 18282 if ("RiskAssessment".equals(codeString)) 18283 return ResourceType.RISKASSESSMENT; 18284 if ("RiskEvidenceSynthesis".equals(codeString)) 18285 return ResourceType.RISKEVIDENCESYNTHESIS; 18286 if ("Schedule".equals(codeString)) 18287 return ResourceType.SCHEDULE; 18288 if ("SearchParameter".equals(codeString)) 18289 return ResourceType.SEARCHPARAMETER; 18290 if ("ServiceRequest".equals(codeString)) 18291 return ResourceType.SERVICEREQUEST; 18292 if ("Slot".equals(codeString)) 18293 return ResourceType.SLOT; 18294 if ("Specimen".equals(codeString)) 18295 return ResourceType.SPECIMEN; 18296 if ("SpecimenDefinition".equals(codeString)) 18297 return ResourceType.SPECIMENDEFINITION; 18298 if ("StructureDefinition".equals(codeString)) 18299 return ResourceType.STRUCTUREDEFINITION; 18300 if ("StructureMap".equals(codeString)) 18301 return ResourceType.STRUCTUREMAP; 18302 if ("Subscription".equals(codeString)) 18303 return ResourceType.SUBSCRIPTION; 18304 if ("Substance".equals(codeString)) 18305 return ResourceType.SUBSTANCE; 18306 if ("SubstanceNucleicAcid".equals(codeString)) 18307 return ResourceType.SUBSTANCENUCLEICACID; 18308 if ("SubstancePolymer".equals(codeString)) 18309 return ResourceType.SUBSTANCEPOLYMER; 18310 if ("SubstanceProtein".equals(codeString)) 18311 return ResourceType.SUBSTANCEPROTEIN; 18312 if ("SubstanceReferenceInformation".equals(codeString)) 18313 return ResourceType.SUBSTANCEREFERENCEINFORMATION; 18314 if ("SubstanceSourceMaterial".equals(codeString)) 18315 return ResourceType.SUBSTANCESOURCEMATERIAL; 18316 if ("SubstanceSpecification".equals(codeString)) 18317 return ResourceType.SUBSTANCESPECIFICATION; 18318 if ("SupplyDelivery".equals(codeString)) 18319 return ResourceType.SUPPLYDELIVERY; 18320 if ("SupplyRequest".equals(codeString)) 18321 return ResourceType.SUPPLYREQUEST; 18322 if ("Task".equals(codeString)) 18323 return ResourceType.TASK; 18324 if ("TerminologyCapabilities".equals(codeString)) 18325 return ResourceType.TERMINOLOGYCAPABILITIES; 18326 if ("TestReport".equals(codeString)) 18327 return ResourceType.TESTREPORT; 18328 if ("TestScript".equals(codeString)) 18329 return ResourceType.TESTSCRIPT; 18330 if ("ValueSet".equals(codeString)) 18331 return ResourceType.VALUESET; 18332 if ("VerificationResult".equals(codeString)) 18333 return ResourceType.VERIFICATIONRESULT; 18334 if ("VisionPrescription".equals(codeString)) 18335 return ResourceType.VISIONPRESCRIPTION; 18336 throw new IllegalArgumentException("Unknown ResourceType code '" + codeString + "'"); 18337 } 18338 18339 public Enumeration<ResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 18340 if (code == null) 18341 return null; 18342 if (code.isEmpty()) 18343 return new Enumeration<ResourceType>(this, ResourceType.NULL, code); 18344 String codeString = code.asStringValue(); 18345 if (codeString == null || "".equals(codeString)) 18346 return new Enumeration<ResourceType>(this, ResourceType.NULL, code); 18347 if ("Account".equals(codeString)) 18348 return new Enumeration<ResourceType>(this, ResourceType.ACCOUNT, code); 18349 if ("ActivityDefinition".equals(codeString)) 18350 return new Enumeration<ResourceType>(this, ResourceType.ACTIVITYDEFINITION, code); 18351 if ("AdverseEvent".equals(codeString)) 18352 return new Enumeration<ResourceType>(this, ResourceType.ADVERSEEVENT, code); 18353 if ("AllergyIntolerance".equals(codeString)) 18354 return new Enumeration<ResourceType>(this, ResourceType.ALLERGYINTOLERANCE, code); 18355 if ("Appointment".equals(codeString)) 18356 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENT, code); 18357 if ("AppointmentResponse".equals(codeString)) 18358 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENTRESPONSE, code); 18359 if ("AuditEvent".equals(codeString)) 18360 return new Enumeration<ResourceType>(this, ResourceType.AUDITEVENT, code); 18361 if ("Basic".equals(codeString)) 18362 return new Enumeration<ResourceType>(this, ResourceType.BASIC, code); 18363 if ("Binary".equals(codeString)) 18364 return new Enumeration<ResourceType>(this, ResourceType.BINARY, code); 18365 if ("BiologicallyDerivedProduct".equals(codeString)) 18366 return new Enumeration<ResourceType>(this, ResourceType.BIOLOGICALLYDERIVEDPRODUCT, code); 18367 if ("BodyStructure".equals(codeString)) 18368 return new Enumeration<ResourceType>(this, ResourceType.BODYSTRUCTURE, code); 18369 if ("Bundle".equals(codeString)) 18370 return new Enumeration<ResourceType>(this, ResourceType.BUNDLE, code); 18371 if ("CapabilityStatement".equals(codeString)) 18372 return new Enumeration<ResourceType>(this, ResourceType.CAPABILITYSTATEMENT, code); 18373 if ("CarePlan".equals(codeString)) 18374 return new Enumeration<ResourceType>(this, ResourceType.CAREPLAN, code); 18375 if ("CareTeam".equals(codeString)) 18376 return new Enumeration<ResourceType>(this, ResourceType.CARETEAM, code); 18377 if ("CatalogEntry".equals(codeString)) 18378 return new Enumeration<ResourceType>(this, ResourceType.CATALOGENTRY, code); 18379 if ("ChargeItem".equals(codeString)) 18380 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEM, code); 18381 if ("ChargeItemDefinition".equals(codeString)) 18382 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEMDEFINITION, code); 18383 if ("Claim".equals(codeString)) 18384 return new Enumeration<ResourceType>(this, ResourceType.CLAIM, code); 18385 if ("ClaimResponse".equals(codeString)) 18386 return new Enumeration<ResourceType>(this, ResourceType.CLAIMRESPONSE, code); 18387 if ("ClinicalImpression".equals(codeString)) 18388 return new Enumeration<ResourceType>(this, ResourceType.CLINICALIMPRESSION, code); 18389 if ("CodeSystem".equals(codeString)) 18390 return new Enumeration<ResourceType>(this, ResourceType.CODESYSTEM, code); 18391 if ("Communication".equals(codeString)) 18392 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATION, code); 18393 if ("CommunicationRequest".equals(codeString)) 18394 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATIONREQUEST, code); 18395 if ("CompartmentDefinition".equals(codeString)) 18396 return new Enumeration<ResourceType>(this, ResourceType.COMPARTMENTDEFINITION, code); 18397 if ("Composition".equals(codeString)) 18398 return new Enumeration<ResourceType>(this, ResourceType.COMPOSITION, code); 18399 if ("ConceptMap".equals(codeString)) 18400 return new Enumeration<ResourceType>(this, ResourceType.CONCEPTMAP, code); 18401 if ("Condition".equals(codeString)) 18402 return new Enumeration<ResourceType>(this, ResourceType.CONDITION, code); 18403 if ("Consent".equals(codeString)) 18404 return new Enumeration<ResourceType>(this, ResourceType.CONSENT, code); 18405 if ("Contract".equals(codeString)) 18406 return new Enumeration<ResourceType>(this, ResourceType.CONTRACT, code); 18407 if ("Coverage".equals(codeString)) 18408 return new Enumeration<ResourceType>(this, ResourceType.COVERAGE, code); 18409 if ("CoverageEligibilityRequest".equals(codeString)) 18410 return new Enumeration<ResourceType>(this, ResourceType.COVERAGEELIGIBILITYREQUEST, code); 18411 if ("CoverageEligibilityResponse".equals(codeString)) 18412 return new Enumeration<ResourceType>(this, ResourceType.COVERAGEELIGIBILITYRESPONSE, code); 18413 if ("DetectedIssue".equals(codeString)) 18414 return new Enumeration<ResourceType>(this, ResourceType.DETECTEDISSUE, code); 18415 if ("Device".equals(codeString)) 18416 return new Enumeration<ResourceType>(this, ResourceType.DEVICE, code); 18417 if ("DeviceDefinition".equals(codeString)) 18418 return new Enumeration<ResourceType>(this, ResourceType.DEVICEDEFINITION, code); 18419 if ("DeviceMetric".equals(codeString)) 18420 return new Enumeration<ResourceType>(this, ResourceType.DEVICEMETRIC, code); 18421 if ("DeviceRequest".equals(codeString)) 18422 return new Enumeration<ResourceType>(this, ResourceType.DEVICEREQUEST, code); 18423 if ("DeviceUseStatement".equals(codeString)) 18424 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSESTATEMENT, code); 18425 if ("DiagnosticReport".equals(codeString)) 18426 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICREPORT, code); 18427 if ("DocumentManifest".equals(codeString)) 18428 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTMANIFEST, code); 18429 if ("DocumentReference".equals(codeString)) 18430 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTREFERENCE, code); 18431 if ("DomainResource".equals(codeString)) 18432 return new Enumeration<ResourceType>(this, ResourceType.DOMAINRESOURCE, code); 18433 if ("EffectEvidenceSynthesis".equals(codeString)) 18434 return new Enumeration<ResourceType>(this, ResourceType.EFFECTEVIDENCESYNTHESIS, code); 18435 if ("Encounter".equals(codeString)) 18436 return new Enumeration<ResourceType>(this, ResourceType.ENCOUNTER, code); 18437 if ("Endpoint".equals(codeString)) 18438 return new Enumeration<ResourceType>(this, ResourceType.ENDPOINT, code); 18439 if ("EnrollmentRequest".equals(codeString)) 18440 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTREQUEST, code); 18441 if ("EnrollmentResponse".equals(codeString)) 18442 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTRESPONSE, code); 18443 if ("EpisodeOfCare".equals(codeString)) 18444 return new Enumeration<ResourceType>(this, ResourceType.EPISODEOFCARE, code); 18445 if ("EventDefinition".equals(codeString)) 18446 return new Enumeration<ResourceType>(this, ResourceType.EVENTDEFINITION, code); 18447 if ("Evidence".equals(codeString)) 18448 return new Enumeration<ResourceType>(this, ResourceType.EVIDENCE, code); 18449 if ("EvidenceVariable".equals(codeString)) 18450 return new Enumeration<ResourceType>(this, ResourceType.EVIDENCEVARIABLE, code); 18451 if ("ExampleScenario".equals(codeString)) 18452 return new Enumeration<ResourceType>(this, ResourceType.EXAMPLESCENARIO, code); 18453 if ("ExplanationOfBenefit".equals(codeString)) 18454 return new Enumeration<ResourceType>(this, ResourceType.EXPLANATIONOFBENEFIT, code); 18455 if ("FamilyMemberHistory".equals(codeString)) 18456 return new Enumeration<ResourceType>(this, ResourceType.FAMILYMEMBERHISTORY, code); 18457 if ("Flag".equals(codeString)) 18458 return new Enumeration<ResourceType>(this, ResourceType.FLAG, code); 18459 if ("Goal".equals(codeString)) 18460 return new Enumeration<ResourceType>(this, ResourceType.GOAL, code); 18461 if ("GraphDefinition".equals(codeString)) 18462 return new Enumeration<ResourceType>(this, ResourceType.GRAPHDEFINITION, code); 18463 if ("Group".equals(codeString)) 18464 return new Enumeration<ResourceType>(this, ResourceType.GROUP, code); 18465 if ("GuidanceResponse".equals(codeString)) 18466 return new Enumeration<ResourceType>(this, ResourceType.GUIDANCERESPONSE, code); 18467 if ("HealthcareService".equals(codeString)) 18468 return new Enumeration<ResourceType>(this, ResourceType.HEALTHCARESERVICE, code); 18469 if ("ImagingStudy".equals(codeString)) 18470 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGSTUDY, code); 18471 if ("Immunization".equals(codeString)) 18472 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATION, code); 18473 if ("ImmunizationEvaluation".equals(codeString)) 18474 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONEVALUATION, code); 18475 if ("ImmunizationRecommendation".equals(codeString)) 18476 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONRECOMMENDATION, code); 18477 if ("ImplementationGuide".equals(codeString)) 18478 return new Enumeration<ResourceType>(this, ResourceType.IMPLEMENTATIONGUIDE, code); 18479 if ("InsurancePlan".equals(codeString)) 18480 return new Enumeration<ResourceType>(this, ResourceType.INSURANCEPLAN, code); 18481 if ("Invoice".equals(codeString)) 18482 return new Enumeration<ResourceType>(this, ResourceType.INVOICE, code); 18483 if ("Library".equals(codeString)) 18484 return new Enumeration<ResourceType>(this, ResourceType.LIBRARY, code); 18485 if ("Linkage".equals(codeString)) 18486 return new Enumeration<ResourceType>(this, ResourceType.LINKAGE, code); 18487 if ("List".equals(codeString)) 18488 return new Enumeration<ResourceType>(this, ResourceType.LIST, code); 18489 if ("Location".equals(codeString)) 18490 return new Enumeration<ResourceType>(this, ResourceType.LOCATION, code); 18491 if ("Measure".equals(codeString)) 18492 return new Enumeration<ResourceType>(this, ResourceType.MEASURE, code); 18493 if ("MeasureReport".equals(codeString)) 18494 return new Enumeration<ResourceType>(this, ResourceType.MEASUREREPORT, code); 18495 if ("Media".equals(codeString)) 18496 return new Enumeration<ResourceType>(this, ResourceType.MEDIA, code); 18497 if ("Medication".equals(codeString)) 18498 return new Enumeration<ResourceType>(this, ResourceType.MEDICATION, code); 18499 if ("MedicationAdministration".equals(codeString)) 18500 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONADMINISTRATION, code); 18501 if ("MedicationDispense".equals(codeString)) 18502 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONDISPENSE, code); 18503 if ("MedicationKnowledge".equals(codeString)) 18504 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONKNOWLEDGE, code); 18505 if ("MedicationRequest".equals(codeString)) 18506 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONREQUEST, code); 18507 if ("MedicationStatement".equals(codeString)) 18508 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONSTATEMENT, code); 18509 if ("MedicinalProduct".equals(codeString)) 18510 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCT, code); 18511 if ("MedicinalProductAuthorization".equals(codeString)) 18512 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTAUTHORIZATION, code); 18513 if ("MedicinalProductContraindication".equals(codeString)) 18514 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTCONTRAINDICATION, code); 18515 if ("MedicinalProductIndication".equals(codeString)) 18516 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINDICATION, code); 18517 if ("MedicinalProductIngredient".equals(codeString)) 18518 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINGREDIENT, code); 18519 if ("MedicinalProductInteraction".equals(codeString)) 18520 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTINTERACTION, code); 18521 if ("MedicinalProductManufactured".equals(codeString)) 18522 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTMANUFACTURED, code); 18523 if ("MedicinalProductPackaged".equals(codeString)) 18524 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTPACKAGED, code); 18525 if ("MedicinalProductPharmaceutical".equals(codeString)) 18526 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTPHARMACEUTICAL, code); 18527 if ("MedicinalProductUndesirableEffect".equals(codeString)) 18528 return new Enumeration<ResourceType>(this, ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 18529 if ("MessageDefinition".equals(codeString)) 18530 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEDEFINITION, code); 18531 if ("MessageHeader".equals(codeString)) 18532 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEHEADER, code); 18533 if ("MolecularSequence".equals(codeString)) 18534 return new Enumeration<ResourceType>(this, ResourceType.MOLECULARSEQUENCE, code); 18535 if ("NamingSystem".equals(codeString)) 18536 return new Enumeration<ResourceType>(this, ResourceType.NAMINGSYSTEM, code); 18537 if ("NutritionOrder".equals(codeString)) 18538 return new Enumeration<ResourceType>(this, ResourceType.NUTRITIONORDER, code); 18539 if ("Observation".equals(codeString)) 18540 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATION, code); 18541 if ("ObservationDefinition".equals(codeString)) 18542 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATIONDEFINITION, code); 18543 if ("OperationDefinition".equals(codeString)) 18544 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONDEFINITION, code); 18545 if ("OperationOutcome".equals(codeString)) 18546 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONOUTCOME, code); 18547 if ("Organization".equals(codeString)) 18548 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATION, code); 18549 if ("OrganizationAffiliation".equals(codeString)) 18550 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATIONAFFILIATION, code); 18551 if ("Parameters".equals(codeString)) 18552 return new Enumeration<ResourceType>(this, ResourceType.PARAMETERS, code); 18553 if ("Patient".equals(codeString)) 18554 return new Enumeration<ResourceType>(this, ResourceType.PATIENT, code); 18555 if ("PaymentNotice".equals(codeString)) 18556 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTNOTICE, code); 18557 if ("PaymentReconciliation".equals(codeString)) 18558 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTRECONCILIATION, code); 18559 if ("Person".equals(codeString)) 18560 return new Enumeration<ResourceType>(this, ResourceType.PERSON, code); 18561 if ("PlanDefinition".equals(codeString)) 18562 return new Enumeration<ResourceType>(this, ResourceType.PLANDEFINITION, code); 18563 if ("Practitioner".equals(codeString)) 18564 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONER, code); 18565 if ("PractitionerRole".equals(codeString)) 18566 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONERROLE, code); 18567 if ("Procedure".equals(codeString)) 18568 return new Enumeration<ResourceType>(this, ResourceType.PROCEDURE, code); 18569 if ("Provenance".equals(codeString)) 18570 return new Enumeration<ResourceType>(this, ResourceType.PROVENANCE, code); 18571 if ("Questionnaire".equals(codeString)) 18572 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRE, code); 18573 if ("QuestionnaireResponse".equals(codeString)) 18574 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRERESPONSE, code); 18575 if ("RelatedPerson".equals(codeString)) 18576 return new Enumeration<ResourceType>(this, ResourceType.RELATEDPERSON, code); 18577 if ("RequestGroup".equals(codeString)) 18578 return new Enumeration<ResourceType>(this, ResourceType.REQUESTGROUP, code); 18579 if ("ResearchDefinition".equals(codeString)) 18580 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHDEFINITION, code); 18581 if ("ResearchElementDefinition".equals(codeString)) 18582 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHELEMENTDEFINITION, code); 18583 if ("ResearchStudy".equals(codeString)) 18584 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSTUDY, code); 18585 if ("ResearchSubject".equals(codeString)) 18586 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSUBJECT, code); 18587 if ("Resource".equals(codeString)) 18588 return new Enumeration<ResourceType>(this, ResourceType.RESOURCE, code); 18589 if ("RiskAssessment".equals(codeString)) 18590 return new Enumeration<ResourceType>(this, ResourceType.RISKASSESSMENT, code); 18591 if ("RiskEvidenceSynthesis".equals(codeString)) 18592 return new Enumeration<ResourceType>(this, ResourceType.RISKEVIDENCESYNTHESIS, code); 18593 if ("Schedule".equals(codeString)) 18594 return new Enumeration<ResourceType>(this, ResourceType.SCHEDULE, code); 18595 if ("SearchParameter".equals(codeString)) 18596 return new Enumeration<ResourceType>(this, ResourceType.SEARCHPARAMETER, code); 18597 if ("ServiceRequest".equals(codeString)) 18598 return new Enumeration<ResourceType>(this, ResourceType.SERVICEREQUEST, code); 18599 if ("Slot".equals(codeString)) 18600 return new Enumeration<ResourceType>(this, ResourceType.SLOT, code); 18601 if ("Specimen".equals(codeString)) 18602 return new Enumeration<ResourceType>(this, ResourceType.SPECIMEN, code); 18603 if ("SpecimenDefinition".equals(codeString)) 18604 return new Enumeration<ResourceType>(this, ResourceType.SPECIMENDEFINITION, code); 18605 if ("StructureDefinition".equals(codeString)) 18606 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREDEFINITION, code); 18607 if ("StructureMap".equals(codeString)) 18608 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREMAP, code); 18609 if ("Subscription".equals(codeString)) 18610 return new Enumeration<ResourceType>(this, ResourceType.SUBSCRIPTION, code); 18611 if ("Substance".equals(codeString)) 18612 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCE, code); 18613 if ("SubstanceNucleicAcid".equals(codeString)) 18614 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCENUCLEICACID, code); 18615 if ("SubstancePolymer".equals(codeString)) 18616 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEPOLYMER, code); 18617 if ("SubstanceProtein".equals(codeString)) 18618 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEPROTEIN, code); 18619 if ("SubstanceReferenceInformation".equals(codeString)) 18620 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCEREFERENCEINFORMATION, code); 18621 if ("SubstanceSourceMaterial".equals(codeString)) 18622 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCESOURCEMATERIAL, code); 18623 if ("SubstanceSpecification".equals(codeString)) 18624 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCESPECIFICATION, code); 18625 if ("SupplyDelivery".equals(codeString)) 18626 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYDELIVERY, code); 18627 if ("SupplyRequest".equals(codeString)) 18628 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYREQUEST, code); 18629 if ("Task".equals(codeString)) 18630 return new Enumeration<ResourceType>(this, ResourceType.TASK, code); 18631 if ("TerminologyCapabilities".equals(codeString)) 18632 return new Enumeration<ResourceType>(this, ResourceType.TERMINOLOGYCAPABILITIES, code); 18633 if ("TestReport".equals(codeString)) 18634 return new Enumeration<ResourceType>(this, ResourceType.TESTREPORT, code); 18635 if ("TestScript".equals(codeString)) 18636 return new Enumeration<ResourceType>(this, ResourceType.TESTSCRIPT, code); 18637 if ("ValueSet".equals(codeString)) 18638 return new Enumeration<ResourceType>(this, ResourceType.VALUESET, code); 18639 if ("VerificationResult".equals(codeString)) 18640 return new Enumeration<ResourceType>(this, ResourceType.VERIFICATIONRESULT, code); 18641 if ("VisionPrescription".equals(codeString)) 18642 return new Enumeration<ResourceType>(this, ResourceType.VISIONPRESCRIPTION, code); 18643 throw new FHIRException("Unknown ResourceType code '" + codeString + "'"); 18644 } 18645 18646 public String toCode(ResourceType code) { 18647 if (code == ResourceType.ACCOUNT) 18648 return "Account"; 18649 if (code == ResourceType.ACTIVITYDEFINITION) 18650 return "ActivityDefinition"; 18651 if (code == ResourceType.ADVERSEEVENT) 18652 return "AdverseEvent"; 18653 if (code == ResourceType.ALLERGYINTOLERANCE) 18654 return "AllergyIntolerance"; 18655 if (code == ResourceType.APPOINTMENT) 18656 return "Appointment"; 18657 if (code == ResourceType.APPOINTMENTRESPONSE) 18658 return "AppointmentResponse"; 18659 if (code == ResourceType.AUDITEVENT) 18660 return "AuditEvent"; 18661 if (code == ResourceType.BASIC) 18662 return "Basic"; 18663 if (code == ResourceType.BINARY) 18664 return "Binary"; 18665 if (code == ResourceType.BIOLOGICALLYDERIVEDPRODUCT) 18666 return "BiologicallyDerivedProduct"; 18667 if (code == ResourceType.BODYSTRUCTURE) 18668 return "BodyStructure"; 18669 if (code == ResourceType.BUNDLE) 18670 return "Bundle"; 18671 if (code == ResourceType.CAPABILITYSTATEMENT) 18672 return "CapabilityStatement"; 18673 if (code == ResourceType.CAREPLAN) 18674 return "CarePlan"; 18675 if (code == ResourceType.CARETEAM) 18676 return "CareTeam"; 18677 if (code == ResourceType.CATALOGENTRY) 18678 return "CatalogEntry"; 18679 if (code == ResourceType.CHARGEITEM) 18680 return "ChargeItem"; 18681 if (code == ResourceType.CHARGEITEMDEFINITION) 18682 return "ChargeItemDefinition"; 18683 if (code == ResourceType.CLAIM) 18684 return "Claim"; 18685 if (code == ResourceType.CLAIMRESPONSE) 18686 return "ClaimResponse"; 18687 if (code == ResourceType.CLINICALIMPRESSION) 18688 return "ClinicalImpression"; 18689 if (code == ResourceType.CODESYSTEM) 18690 return "CodeSystem"; 18691 if (code == ResourceType.COMMUNICATION) 18692 return "Communication"; 18693 if (code == ResourceType.COMMUNICATIONREQUEST) 18694 return "CommunicationRequest"; 18695 if (code == ResourceType.COMPARTMENTDEFINITION) 18696 return "CompartmentDefinition"; 18697 if (code == ResourceType.COMPOSITION) 18698 return "Composition"; 18699 if (code == ResourceType.CONCEPTMAP) 18700 return "ConceptMap"; 18701 if (code == ResourceType.CONDITION) 18702 return "Condition"; 18703 if (code == ResourceType.CONSENT) 18704 return "Consent"; 18705 if (code == ResourceType.CONTRACT) 18706 return "Contract"; 18707 if (code == ResourceType.COVERAGE) 18708 return "Coverage"; 18709 if (code == ResourceType.COVERAGEELIGIBILITYREQUEST) 18710 return "CoverageEligibilityRequest"; 18711 if (code == ResourceType.COVERAGEELIGIBILITYRESPONSE) 18712 return "CoverageEligibilityResponse"; 18713 if (code == ResourceType.DETECTEDISSUE) 18714 return "DetectedIssue"; 18715 if (code == ResourceType.DEVICE) 18716 return "Device"; 18717 if (code == ResourceType.DEVICEDEFINITION) 18718 return "DeviceDefinition"; 18719 if (code == ResourceType.DEVICEMETRIC) 18720 return "DeviceMetric"; 18721 if (code == ResourceType.DEVICEREQUEST) 18722 return "DeviceRequest"; 18723 if (code == ResourceType.DEVICEUSESTATEMENT) 18724 return "DeviceUseStatement"; 18725 if (code == ResourceType.DIAGNOSTICREPORT) 18726 return "DiagnosticReport"; 18727 if (code == ResourceType.DOCUMENTMANIFEST) 18728 return "DocumentManifest"; 18729 if (code == ResourceType.DOCUMENTREFERENCE) 18730 return "DocumentReference"; 18731 if (code == ResourceType.DOMAINRESOURCE) 18732 return "DomainResource"; 18733 if (code == ResourceType.EFFECTEVIDENCESYNTHESIS) 18734 return "EffectEvidenceSynthesis"; 18735 if (code == ResourceType.ENCOUNTER) 18736 return "Encounter"; 18737 if (code == ResourceType.ENDPOINT) 18738 return "Endpoint"; 18739 if (code == ResourceType.ENROLLMENTREQUEST) 18740 return "EnrollmentRequest"; 18741 if (code == ResourceType.ENROLLMENTRESPONSE) 18742 return "EnrollmentResponse"; 18743 if (code == ResourceType.EPISODEOFCARE) 18744 return "EpisodeOfCare"; 18745 if (code == ResourceType.EVENTDEFINITION) 18746 return "EventDefinition"; 18747 if (code == ResourceType.EVIDENCE) 18748 return "Evidence"; 18749 if (code == ResourceType.EVIDENCEVARIABLE) 18750 return "EvidenceVariable"; 18751 if (code == ResourceType.EXAMPLESCENARIO) 18752 return "ExampleScenario"; 18753 if (code == ResourceType.EXPLANATIONOFBENEFIT) 18754 return "ExplanationOfBenefit"; 18755 if (code == ResourceType.FAMILYMEMBERHISTORY) 18756 return "FamilyMemberHistory"; 18757 if (code == ResourceType.FLAG) 18758 return "Flag"; 18759 if (code == ResourceType.GOAL) 18760 return "Goal"; 18761 if (code == ResourceType.GRAPHDEFINITION) 18762 return "GraphDefinition"; 18763 if (code == ResourceType.GROUP) 18764 return "Group"; 18765 if (code == ResourceType.GUIDANCERESPONSE) 18766 return "GuidanceResponse"; 18767 if (code == ResourceType.HEALTHCARESERVICE) 18768 return "HealthcareService"; 18769 if (code == ResourceType.IMAGINGSTUDY) 18770 return "ImagingStudy"; 18771 if (code == ResourceType.IMMUNIZATION) 18772 return "Immunization"; 18773 if (code == ResourceType.IMMUNIZATIONEVALUATION) 18774 return "ImmunizationEvaluation"; 18775 if (code == ResourceType.IMMUNIZATIONRECOMMENDATION) 18776 return "ImmunizationRecommendation"; 18777 if (code == ResourceType.IMPLEMENTATIONGUIDE) 18778 return "ImplementationGuide"; 18779 if (code == ResourceType.INSURANCEPLAN) 18780 return "InsurancePlan"; 18781 if (code == ResourceType.INVOICE) 18782 return "Invoice"; 18783 if (code == ResourceType.LIBRARY) 18784 return "Library"; 18785 if (code == ResourceType.LINKAGE) 18786 return "Linkage"; 18787 if (code == ResourceType.LIST) 18788 return "List"; 18789 if (code == ResourceType.LOCATION) 18790 return "Location"; 18791 if (code == ResourceType.MEASURE) 18792 return "Measure"; 18793 if (code == ResourceType.MEASUREREPORT) 18794 return "MeasureReport"; 18795 if (code == ResourceType.MEDIA) 18796 return "Media"; 18797 if (code == ResourceType.MEDICATION) 18798 return "Medication"; 18799 if (code == ResourceType.MEDICATIONADMINISTRATION) 18800 return "MedicationAdministration"; 18801 if (code == ResourceType.MEDICATIONDISPENSE) 18802 return "MedicationDispense"; 18803 if (code == ResourceType.MEDICATIONKNOWLEDGE) 18804 return "MedicationKnowledge"; 18805 if (code == ResourceType.MEDICATIONREQUEST) 18806 return "MedicationRequest"; 18807 if (code == ResourceType.MEDICATIONSTATEMENT) 18808 return "MedicationStatement"; 18809 if (code == ResourceType.MEDICINALPRODUCT) 18810 return "MedicinalProduct"; 18811 if (code == ResourceType.MEDICINALPRODUCTAUTHORIZATION) 18812 return "MedicinalProductAuthorization"; 18813 if (code == ResourceType.MEDICINALPRODUCTCONTRAINDICATION) 18814 return "MedicinalProductContraindication"; 18815 if (code == ResourceType.MEDICINALPRODUCTINDICATION) 18816 return "MedicinalProductIndication"; 18817 if (code == ResourceType.MEDICINALPRODUCTINGREDIENT) 18818 return "MedicinalProductIngredient"; 18819 if (code == ResourceType.MEDICINALPRODUCTINTERACTION) 18820 return "MedicinalProductInteraction"; 18821 if (code == ResourceType.MEDICINALPRODUCTMANUFACTURED) 18822 return "MedicinalProductManufactured"; 18823 if (code == ResourceType.MEDICINALPRODUCTPACKAGED) 18824 return "MedicinalProductPackaged"; 18825 if (code == ResourceType.MEDICINALPRODUCTPHARMACEUTICAL) 18826 return "MedicinalProductPharmaceutical"; 18827 if (code == ResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 18828 return "MedicinalProductUndesirableEffect"; 18829 if (code == ResourceType.MESSAGEDEFINITION) 18830 return "MessageDefinition"; 18831 if (code == ResourceType.MESSAGEHEADER) 18832 return "MessageHeader"; 18833 if (code == ResourceType.MOLECULARSEQUENCE) 18834 return "MolecularSequence"; 18835 if (code == ResourceType.NAMINGSYSTEM) 18836 return "NamingSystem"; 18837 if (code == ResourceType.NUTRITIONORDER) 18838 return "NutritionOrder"; 18839 if (code == ResourceType.OBSERVATION) 18840 return "Observation"; 18841 if (code == ResourceType.OBSERVATIONDEFINITION) 18842 return "ObservationDefinition"; 18843 if (code == ResourceType.OPERATIONDEFINITION) 18844 return "OperationDefinition"; 18845 if (code == ResourceType.OPERATIONOUTCOME) 18846 return "OperationOutcome"; 18847 if (code == ResourceType.ORGANIZATION) 18848 return "Organization"; 18849 if (code == ResourceType.ORGANIZATIONAFFILIATION) 18850 return "OrganizationAffiliation"; 18851 if (code == ResourceType.PARAMETERS) 18852 return "Parameters"; 18853 if (code == ResourceType.PATIENT) 18854 return "Patient"; 18855 if (code == ResourceType.PAYMENTNOTICE) 18856 return "PaymentNotice"; 18857 if (code == ResourceType.PAYMENTRECONCILIATION) 18858 return "PaymentReconciliation"; 18859 if (code == ResourceType.PERSON) 18860 return "Person"; 18861 if (code == ResourceType.PLANDEFINITION) 18862 return "PlanDefinition"; 18863 if (code == ResourceType.PRACTITIONER) 18864 return "Practitioner"; 18865 if (code == ResourceType.PRACTITIONERROLE) 18866 return "PractitionerRole"; 18867 if (code == ResourceType.PROCEDURE) 18868 return "Procedure"; 18869 if (code == ResourceType.PROVENANCE) 18870 return "Provenance"; 18871 if (code == ResourceType.QUESTIONNAIRE) 18872 return "Questionnaire"; 18873 if (code == ResourceType.QUESTIONNAIRERESPONSE) 18874 return "QuestionnaireResponse"; 18875 if (code == ResourceType.RELATEDPERSON) 18876 return "RelatedPerson"; 18877 if (code == ResourceType.REQUESTGROUP) 18878 return "RequestGroup"; 18879 if (code == ResourceType.RESEARCHDEFINITION) 18880 return "ResearchDefinition"; 18881 if (code == ResourceType.RESEARCHELEMENTDEFINITION) 18882 return "ResearchElementDefinition"; 18883 if (code == ResourceType.RESEARCHSTUDY) 18884 return "ResearchStudy"; 18885 if (code == ResourceType.RESEARCHSUBJECT) 18886 return "ResearchSubject"; 18887 if (code == ResourceType.RESOURCE) 18888 return "Resource"; 18889 if (code == ResourceType.RISKASSESSMENT) 18890 return "RiskAssessment"; 18891 if (code == ResourceType.RISKEVIDENCESYNTHESIS) 18892 return "RiskEvidenceSynthesis"; 18893 if (code == ResourceType.SCHEDULE) 18894 return "Schedule"; 18895 if (code == ResourceType.SEARCHPARAMETER) 18896 return "SearchParameter"; 18897 if (code == ResourceType.SERVICEREQUEST) 18898 return "ServiceRequest"; 18899 if (code == ResourceType.SLOT) 18900 return "Slot"; 18901 if (code == ResourceType.SPECIMEN) 18902 return "Specimen"; 18903 if (code == ResourceType.SPECIMENDEFINITION) 18904 return "SpecimenDefinition"; 18905 if (code == ResourceType.STRUCTUREDEFINITION) 18906 return "StructureDefinition"; 18907 if (code == ResourceType.STRUCTUREMAP) 18908 return "StructureMap"; 18909 if (code == ResourceType.SUBSCRIPTION) 18910 return "Subscription"; 18911 if (code == ResourceType.SUBSTANCE) 18912 return "Substance"; 18913 if (code == ResourceType.SUBSTANCENUCLEICACID) 18914 return "SubstanceNucleicAcid"; 18915 if (code == ResourceType.SUBSTANCEPOLYMER) 18916 return "SubstancePolymer"; 18917 if (code == ResourceType.SUBSTANCEPROTEIN) 18918 return "SubstanceProtein"; 18919 if (code == ResourceType.SUBSTANCEREFERENCEINFORMATION) 18920 return "SubstanceReferenceInformation"; 18921 if (code == ResourceType.SUBSTANCESOURCEMATERIAL) 18922 return "SubstanceSourceMaterial"; 18923 if (code == ResourceType.SUBSTANCESPECIFICATION) 18924 return "SubstanceSpecification"; 18925 if (code == ResourceType.SUPPLYDELIVERY) 18926 return "SupplyDelivery"; 18927 if (code == ResourceType.SUPPLYREQUEST) 18928 return "SupplyRequest"; 18929 if (code == ResourceType.TASK) 18930 return "Task"; 18931 if (code == ResourceType.TERMINOLOGYCAPABILITIES) 18932 return "TerminologyCapabilities"; 18933 if (code == ResourceType.TESTREPORT) 18934 return "TestReport"; 18935 if (code == ResourceType.TESTSCRIPT) 18936 return "TestScript"; 18937 if (code == ResourceType.VALUESET) 18938 return "ValueSet"; 18939 if (code == ResourceType.VERIFICATIONRESULT) 18940 return "VerificationResult"; 18941 if (code == ResourceType.VISIONPRESCRIPTION) 18942 return "VisionPrescription"; 18943 return "?"; 18944 } 18945 18946 public String toSystem(ResourceType code) { 18947 return code.getSystem(); 18948 } 18949 } 18950 18951 public enum SearchParamType { 18952 /** 18953 * Search parameter SHALL be a number (a whole number, or a decimal). 18954 */ 18955 NUMBER, 18956 /** 18957 * Search parameter is on a date/time. The date format is the standard XML 18958 * format, though other formats may be supported. 18959 */ 18960 DATE, 18961 /** 18962 * Search parameter is a simple string, like a name part. Search is 18963 * case-insensitive and accent-insensitive. May match just the start of a 18964 * string. String parameters may contain spaces. 18965 */ 18966 STRING, 18967 /** 18968 * Search parameter on a coded element or identifier. May be used to search 18969 * through the text, display, code and code/codesystem (for codes) and label, 18970 * system and key (for identifier). Its value is either a string or a pair of 18971 * namespace and value, separated by a "|", depending on the modifier used. 18972 */ 18973 TOKEN, 18974 /** 18975 * A reference to another resource (Reference or canonical). 18976 */ 18977 REFERENCE, 18978 /** 18979 * A composite search parameter that combines a search on two values together. 18980 */ 18981 COMPOSITE, 18982 /** 18983 * A search parameter that searches on a quantity. 18984 */ 18985 QUANTITY, 18986 /** 18987 * A search parameter that searches on a URI (RFC 3986). 18988 */ 18989 URI, 18990 /** 18991 * Special logic applies to this parameter per the description of the search 18992 * parameter. 18993 */ 18994 SPECIAL, 18995 /** 18996 * added to help the parsers 18997 */ 18998 NULL; 18999 19000 public static SearchParamType fromCode(String codeString) throws FHIRException { 19001 if (codeString == null || "".equals(codeString)) 19002 return null; 19003 if ("number".equals(codeString)) 19004 return NUMBER; 19005 if ("date".equals(codeString)) 19006 return DATE; 19007 if ("string".equals(codeString)) 19008 return STRING; 19009 if ("token".equals(codeString)) 19010 return TOKEN; 19011 if ("reference".equals(codeString)) 19012 return REFERENCE; 19013 if ("composite".equals(codeString)) 19014 return COMPOSITE; 19015 if ("quantity".equals(codeString)) 19016 return QUANTITY; 19017 if ("uri".equals(codeString)) 19018 return URI; 19019 if ("special".equals(codeString)) 19020 return SPECIAL; 19021 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 19022 } 19023 19024 public String toCode() { 19025 switch (this) { 19026 case NUMBER: 19027 return "number"; 19028 case DATE: 19029 return "date"; 19030 case STRING: 19031 return "string"; 19032 case TOKEN: 19033 return "token"; 19034 case REFERENCE: 19035 return "reference"; 19036 case COMPOSITE: 19037 return "composite"; 19038 case QUANTITY: 19039 return "quantity"; 19040 case URI: 19041 return "uri"; 19042 case SPECIAL: 19043 return "special"; 19044 case NULL: 19045 return null; 19046 default: 19047 return "?"; 19048 } 19049 } 19050 19051 public String getSystem() { 19052 switch (this) { 19053 case NUMBER: 19054 return "http://hl7.org/fhir/search-param-type"; 19055 case DATE: 19056 return "http://hl7.org/fhir/search-param-type"; 19057 case STRING: 19058 return "http://hl7.org/fhir/search-param-type"; 19059 case TOKEN: 19060 return "http://hl7.org/fhir/search-param-type"; 19061 case REFERENCE: 19062 return "http://hl7.org/fhir/search-param-type"; 19063 case COMPOSITE: 19064 return "http://hl7.org/fhir/search-param-type"; 19065 case QUANTITY: 19066 return "http://hl7.org/fhir/search-param-type"; 19067 case URI: 19068 return "http://hl7.org/fhir/search-param-type"; 19069 case SPECIAL: 19070 return "http://hl7.org/fhir/search-param-type"; 19071 case NULL: 19072 return null; 19073 default: 19074 return "?"; 19075 } 19076 } 19077 19078 public String getDefinition() { 19079 switch (this) { 19080 case NUMBER: 19081 return "Search parameter SHALL be a number (a whole number, or a decimal)."; 19082 case DATE: 19083 return "Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported."; 19084 case STRING: 19085 return "Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces."; 19086 case TOKEN: 19087 return "Search parameter on a coded element or identifier. May be used to search through the text, display, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a \"|\", depending on the modifier used."; 19088 case REFERENCE: 19089 return "A reference to another resource (Reference or canonical)."; 19090 case COMPOSITE: 19091 return "A composite search parameter that combines a search on two values together."; 19092 case QUANTITY: 19093 return "A search parameter that searches on a quantity."; 19094 case URI: 19095 return "A search parameter that searches on a URI (RFC 3986)."; 19096 case SPECIAL: 19097 return "Special logic applies to this parameter per the description of the search parameter."; 19098 case NULL: 19099 return null; 19100 default: 19101 return "?"; 19102 } 19103 } 19104 19105 public String getDisplay() { 19106 switch (this) { 19107 case NUMBER: 19108 return "Number"; 19109 case DATE: 19110 return "Date/DateTime"; 19111 case STRING: 19112 return "String"; 19113 case TOKEN: 19114 return "Token"; 19115 case REFERENCE: 19116 return "Reference"; 19117 case COMPOSITE: 19118 return "Composite"; 19119 case QUANTITY: 19120 return "Quantity"; 19121 case URI: 19122 return "URI"; 19123 case SPECIAL: 19124 return "Special"; 19125 case NULL: 19126 return null; 19127 default: 19128 return "?"; 19129 } 19130 } 19131 } 19132 19133 public static class SearchParamTypeEnumFactory implements EnumFactory<SearchParamType> { 19134 public SearchParamType fromCode(String codeString) throws IllegalArgumentException { 19135 if (codeString == null || "".equals(codeString)) 19136 if (codeString == null || "".equals(codeString)) 19137 return null; 19138 if ("number".equals(codeString)) 19139 return SearchParamType.NUMBER; 19140 if ("date".equals(codeString)) 19141 return SearchParamType.DATE; 19142 if ("string".equals(codeString)) 19143 return SearchParamType.STRING; 19144 if ("token".equals(codeString)) 19145 return SearchParamType.TOKEN; 19146 if ("reference".equals(codeString)) 19147 return SearchParamType.REFERENCE; 19148 if ("composite".equals(codeString)) 19149 return SearchParamType.COMPOSITE; 19150 if ("quantity".equals(codeString)) 19151 return SearchParamType.QUANTITY; 19152 if ("uri".equals(codeString)) 19153 return SearchParamType.URI; 19154 if ("special".equals(codeString)) 19155 return SearchParamType.SPECIAL; 19156 throw new IllegalArgumentException("Unknown SearchParamType code '" + codeString + "'"); 19157 } 19158 19159 public Enumeration<SearchParamType> fromType(PrimitiveType<?> code) throws FHIRException { 19160 if (code == null) 19161 return null; 19162 if (code.isEmpty()) 19163 return new Enumeration<SearchParamType>(this, SearchParamType.NULL, code); 19164 String codeString = code.asStringValue(); 19165 if (codeString == null || "".equals(codeString)) 19166 return new Enumeration<SearchParamType>(this, SearchParamType.NULL, code); 19167 if ("number".equals(codeString)) 19168 return new Enumeration<SearchParamType>(this, SearchParamType.NUMBER, code); 19169 if ("date".equals(codeString)) 19170 return new Enumeration<SearchParamType>(this, SearchParamType.DATE, code); 19171 if ("string".equals(codeString)) 19172 return new Enumeration<SearchParamType>(this, SearchParamType.STRING, code); 19173 if ("token".equals(codeString)) 19174 return new Enumeration<SearchParamType>(this, SearchParamType.TOKEN, code); 19175 if ("reference".equals(codeString)) 19176 return new Enumeration<SearchParamType>(this, SearchParamType.REFERENCE, code); 19177 if ("composite".equals(codeString)) 19178 return new Enumeration<SearchParamType>(this, SearchParamType.COMPOSITE, code); 19179 if ("quantity".equals(codeString)) 19180 return new Enumeration<SearchParamType>(this, SearchParamType.QUANTITY, code); 19181 if ("uri".equals(codeString)) 19182 return new Enumeration<SearchParamType>(this, SearchParamType.URI, code); 19183 if ("special".equals(codeString)) 19184 return new Enumeration<SearchParamType>(this, SearchParamType.SPECIAL, code); 19185 throw new FHIRException("Unknown SearchParamType code '" + codeString + "'"); 19186 } 19187 19188 public String toCode(SearchParamType code) { 19189 if (code == SearchParamType.NUMBER) 19190 return "number"; 19191 if (code == SearchParamType.DATE) 19192 return "date"; 19193 if (code == SearchParamType.STRING) 19194 return "string"; 19195 if (code == SearchParamType.TOKEN) 19196 return "token"; 19197 if (code == SearchParamType.REFERENCE) 19198 return "reference"; 19199 if (code == SearchParamType.COMPOSITE) 19200 return "composite"; 19201 if (code == SearchParamType.QUANTITY) 19202 return "quantity"; 19203 if (code == SearchParamType.URI) 19204 return "uri"; 19205 if (code == SearchParamType.SPECIAL) 19206 return "special"; 19207 return "?"; 19208 } 19209 19210 public String toSystem(SearchParamType code) { 19211 return code.getSystem(); 19212 } 19213 } 19214 19215 public enum SpecialValues { 19216 /** 19217 * Boolean true. 19218 */ 19219 TRUE, 19220 /** 19221 * Boolean false. 19222 */ 19223 FALSE, 19224 /** 19225 * The content is greater than zero, but too small to be quantified. 19226 */ 19227 TRACE, 19228 /** 19229 * The specific quantity is not known, but is known to be non-zero and is not 19230 * specified because it makes up the bulk of the material. 19231 */ 19232 SUFFICIENT, 19233 /** 19234 * The value is no longer available. 19235 */ 19236 WITHDRAWN, 19237 /** 19238 * The are no known applicable values in this context. 19239 */ 19240 NILKNOWN, 19241 /** 19242 * added to help the parsers 19243 */ 19244 NULL; 19245 19246 public static SpecialValues fromCode(String codeString) throws FHIRException { 19247 if (codeString == null || "".equals(codeString)) 19248 return null; 19249 if ("true".equals(codeString)) 19250 return TRUE; 19251 if ("false".equals(codeString)) 19252 return FALSE; 19253 if ("trace".equals(codeString)) 19254 return TRACE; 19255 if ("sufficient".equals(codeString)) 19256 return SUFFICIENT; 19257 if ("withdrawn".equals(codeString)) 19258 return WITHDRAWN; 19259 if ("nil-known".equals(codeString)) 19260 return NILKNOWN; 19261 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 19262 } 19263 19264 public String toCode() { 19265 switch (this) { 19266 case TRUE: 19267 return "true"; 19268 case FALSE: 19269 return "false"; 19270 case TRACE: 19271 return "trace"; 19272 case SUFFICIENT: 19273 return "sufficient"; 19274 case WITHDRAWN: 19275 return "withdrawn"; 19276 case NILKNOWN: 19277 return "nil-known"; 19278 case NULL: 19279 return null; 19280 default: 19281 return "?"; 19282 } 19283 } 19284 19285 public String getSystem() { 19286 switch (this) { 19287 case TRUE: 19288 return "http://terminology.hl7.org/CodeSystem/special-values"; 19289 case FALSE: 19290 return "http://terminology.hl7.org/CodeSystem/special-values"; 19291 case TRACE: 19292 return "http://terminology.hl7.org/CodeSystem/special-values"; 19293 case SUFFICIENT: 19294 return "http://terminology.hl7.org/CodeSystem/special-values"; 19295 case WITHDRAWN: 19296 return "http://terminology.hl7.org/CodeSystem/special-values"; 19297 case NILKNOWN: 19298 return "http://terminology.hl7.org/CodeSystem/special-values"; 19299 case NULL: 19300 return null; 19301 default: 19302 return "?"; 19303 } 19304 } 19305 19306 public String getDefinition() { 19307 switch (this) { 19308 case TRUE: 19309 return "Boolean true."; 19310 case FALSE: 19311 return "Boolean false."; 19312 case TRACE: 19313 return "The content is greater than zero, but too small to be quantified."; 19314 case SUFFICIENT: 19315 return "The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material."; 19316 case WITHDRAWN: 19317 return "The value is no longer available."; 19318 case NILKNOWN: 19319 return "The are no known applicable values in this context."; 19320 case NULL: 19321 return null; 19322 default: 19323 return "?"; 19324 } 19325 } 19326 19327 public String getDisplay() { 19328 switch (this) { 19329 case TRUE: 19330 return "true"; 19331 case FALSE: 19332 return "false"; 19333 case TRACE: 19334 return "Trace Amount Detected"; 19335 case SUFFICIENT: 19336 return "Sufficient Quantity"; 19337 case WITHDRAWN: 19338 return "Value Withdrawn"; 19339 case NILKNOWN: 19340 return "Nil Known"; 19341 case NULL: 19342 return null; 19343 default: 19344 return "?"; 19345 } 19346 } 19347 } 19348 19349 public static class SpecialValuesEnumFactory implements EnumFactory<SpecialValues> { 19350 public SpecialValues fromCode(String codeString) throws IllegalArgumentException { 19351 if (codeString == null || "".equals(codeString)) 19352 if (codeString == null || "".equals(codeString)) 19353 return null; 19354 if ("true".equals(codeString)) 19355 return SpecialValues.TRUE; 19356 if ("false".equals(codeString)) 19357 return SpecialValues.FALSE; 19358 if ("trace".equals(codeString)) 19359 return SpecialValues.TRACE; 19360 if ("sufficient".equals(codeString)) 19361 return SpecialValues.SUFFICIENT; 19362 if ("withdrawn".equals(codeString)) 19363 return SpecialValues.WITHDRAWN; 19364 if ("nil-known".equals(codeString)) 19365 return SpecialValues.NILKNOWN; 19366 throw new IllegalArgumentException("Unknown SpecialValues code '" + codeString + "'"); 19367 } 19368 19369 public Enumeration<SpecialValues> fromType(PrimitiveType<?> code) throws FHIRException { 19370 if (code == null) 19371 return null; 19372 if (code.isEmpty()) 19373 return new Enumeration<SpecialValues>(this, SpecialValues.NULL, code); 19374 String codeString = code.asStringValue(); 19375 if (codeString == null || "".equals(codeString)) 19376 return new Enumeration<SpecialValues>(this, SpecialValues.NULL, code); 19377 if ("true".equals(codeString)) 19378 return new Enumeration<SpecialValues>(this, SpecialValues.TRUE, code); 19379 if ("false".equals(codeString)) 19380 return new Enumeration<SpecialValues>(this, SpecialValues.FALSE, code); 19381 if ("trace".equals(codeString)) 19382 return new Enumeration<SpecialValues>(this, SpecialValues.TRACE, code); 19383 if ("sufficient".equals(codeString)) 19384 return new Enumeration<SpecialValues>(this, SpecialValues.SUFFICIENT, code); 19385 if ("withdrawn".equals(codeString)) 19386 return new Enumeration<SpecialValues>(this, SpecialValues.WITHDRAWN, code); 19387 if ("nil-known".equals(codeString)) 19388 return new Enumeration<SpecialValues>(this, SpecialValues.NILKNOWN, code); 19389 throw new FHIRException("Unknown SpecialValues code '" + codeString + "'"); 19390 } 19391 19392 public String toCode(SpecialValues code) { 19393 if (code == SpecialValues.TRUE) 19394 return "true"; 19395 if (code == SpecialValues.FALSE) 19396 return "false"; 19397 if (code == SpecialValues.TRACE) 19398 return "trace"; 19399 if (code == SpecialValues.SUFFICIENT) 19400 return "sufficient"; 19401 if (code == SpecialValues.WITHDRAWN) 19402 return "withdrawn"; 19403 if (code == SpecialValues.NILKNOWN) 19404 return "nil-known"; 19405 return "?"; 19406 } 19407 19408 public String toSystem(SpecialValues code) { 19409 return code.getSystem(); 19410 } 19411 } 19412 19413}