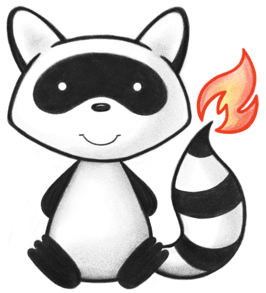
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * An association between a patient and an organization / healthcare provider(s) 047 * during which time encounters may occur. The managing organization assumes a 048 * level of responsibility for the patient during this time. 049 */ 050@ResourceDef(name = "EpisodeOfCare", profile = "http://hl7.org/fhir/StructureDefinition/EpisodeOfCare") 051public class EpisodeOfCare extends DomainResource { 052 053 public enum EpisodeOfCareStatus { 054 /** 055 * This episode of care is planned to start at the date specified in the 056 * period.start. During this status, an organization may perform assessments to 057 * determine if the patient is eligible to receive services, or be organizing to 058 * make resources available to provide care services. 059 */ 060 PLANNED, 061 /** 062 * This episode has been placed on a waitlist, pending the episode being made 063 * active (or cancelled). 064 */ 065 WAITLIST, 066 /** 067 * This episode of care is current. 068 */ 069 ACTIVE, 070 /** 071 * This episode of care is on hold; the organization has limited responsibility 072 * for the patient (such as while on respite). 073 */ 074 ONHOLD, 075 /** 076 * This episode of care is finished and the organization is not expecting to be 077 * providing further care to the patient. Can also be known as "closed", 078 * "completed" or other similar terms. 079 */ 080 FINISHED, 081 /** 082 * The episode of care was cancelled, or withdrawn from service, often selected 083 * during the planned stage as the patient may have gone elsewhere, or the 084 * circumstances have changed and the organization is unable to provide the 085 * care. It indicates that services terminated outside the planned/expected 086 * workflow. 087 */ 088 CANCELLED, 089 /** 090 * This instance should not have been part of this patient's medical record. 091 */ 092 ENTEREDINERROR, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 098 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 099 if (codeString == null || "".equals(codeString)) 100 return null; 101 if ("planned".equals(codeString)) 102 return PLANNED; 103 if ("waitlist".equals(codeString)) 104 return WAITLIST; 105 if ("active".equals(codeString)) 106 return ACTIVE; 107 if ("onhold".equals(codeString)) 108 return ONHOLD; 109 if ("finished".equals(codeString)) 110 return FINISHED; 111 if ("cancelled".equals(codeString)) 112 return CANCELLED; 113 if ("entered-in-error".equals(codeString)) 114 return ENTEREDINERROR; 115 if (Configuration.isAcceptInvalidEnums()) 116 return null; 117 else 118 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case PLANNED: 124 return "planned"; 125 case WAITLIST: 126 return "waitlist"; 127 case ACTIVE: 128 return "active"; 129 case ONHOLD: 130 return "onhold"; 131 case FINISHED: 132 return "finished"; 133 case CANCELLED: 134 return "cancelled"; 135 case ENTEREDINERROR: 136 return "entered-in-error"; 137 case NULL: 138 return null; 139 default: 140 return "?"; 141 } 142 } 143 144 public String getSystem() { 145 switch (this) { 146 case PLANNED: 147 return "http://hl7.org/fhir/episode-of-care-status"; 148 case WAITLIST: 149 return "http://hl7.org/fhir/episode-of-care-status"; 150 case ACTIVE: 151 return "http://hl7.org/fhir/episode-of-care-status"; 152 case ONHOLD: 153 return "http://hl7.org/fhir/episode-of-care-status"; 154 case FINISHED: 155 return "http://hl7.org/fhir/episode-of-care-status"; 156 case CANCELLED: 157 return "http://hl7.org/fhir/episode-of-care-status"; 158 case ENTEREDINERROR: 159 return "http://hl7.org/fhir/episode-of-care-status"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getDefinition() { 168 switch (this) { 169 case PLANNED: 170 return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 171 case WAITLIST: 172 return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 173 case ACTIVE: 174 return "This episode of care is current."; 175 case ONHOLD: 176 return "This episode of care is on hold; the organization has limited responsibility for the patient (such as while on respite)."; 177 case FINISHED: 178 return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 179 case CANCELLED: 180 return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 181 case ENTEREDINERROR: 182 return "This instance should not have been part of this patient's medical record."; 183 case NULL: 184 return null; 185 default: 186 return "?"; 187 } 188 } 189 190 public String getDisplay() { 191 switch (this) { 192 case PLANNED: 193 return "Planned"; 194 case WAITLIST: 195 return "Waitlist"; 196 case ACTIVE: 197 return "Active"; 198 case ONHOLD: 199 return "On Hold"; 200 case FINISHED: 201 return "Finished"; 202 case CANCELLED: 203 return "Cancelled"; 204 case ENTEREDINERROR: 205 return "Entered in Error"; 206 case NULL: 207 return null; 208 default: 209 return "?"; 210 } 211 } 212 } 213 214 public static class EpisodeOfCareStatusEnumFactory implements EnumFactory<EpisodeOfCareStatus> { 215 public EpisodeOfCareStatus fromCode(String codeString) throws IllegalArgumentException { 216 if (codeString == null || "".equals(codeString)) 217 if (codeString == null || "".equals(codeString)) 218 return null; 219 if ("planned".equals(codeString)) 220 return EpisodeOfCareStatus.PLANNED; 221 if ("waitlist".equals(codeString)) 222 return EpisodeOfCareStatus.WAITLIST; 223 if ("active".equals(codeString)) 224 return EpisodeOfCareStatus.ACTIVE; 225 if ("onhold".equals(codeString)) 226 return EpisodeOfCareStatus.ONHOLD; 227 if ("finished".equals(codeString)) 228 return EpisodeOfCareStatus.FINISHED; 229 if ("cancelled".equals(codeString)) 230 return EpisodeOfCareStatus.CANCELLED; 231 if ("entered-in-error".equals(codeString)) 232 return EpisodeOfCareStatus.ENTEREDINERROR; 233 throw new IllegalArgumentException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 234 } 235 236 public Enumeration<EpisodeOfCareStatus> fromType(PrimitiveType<?> code) throws FHIRException { 237 if (code == null) 238 return null; 239 if (code.isEmpty()) 240 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 241 String codeString = code.asStringValue(); 242 if (codeString == null || "".equals(codeString)) 243 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.NULL, code); 244 if ("planned".equals(codeString)) 245 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.PLANNED, code); 246 if ("waitlist".equals(codeString)) 247 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.WAITLIST, code); 248 if ("active".equals(codeString)) 249 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ACTIVE, code); 250 if ("onhold".equals(codeString)) 251 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ONHOLD, code); 252 if ("finished".equals(codeString)) 253 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.FINISHED, code); 254 if ("cancelled".equals(codeString)) 255 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.CANCELLED, code); 256 if ("entered-in-error".equals(codeString)) 257 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ENTEREDINERROR, code); 258 throw new FHIRException("Unknown EpisodeOfCareStatus code '" + codeString + "'"); 259 } 260 261 public String toCode(EpisodeOfCareStatus code) { 262 if (code == EpisodeOfCareStatus.NULL) 263 return null; 264 if (code == EpisodeOfCareStatus.PLANNED) 265 return "planned"; 266 if (code == EpisodeOfCareStatus.WAITLIST) 267 return "waitlist"; 268 if (code == EpisodeOfCareStatus.ACTIVE) 269 return "active"; 270 if (code == EpisodeOfCareStatus.ONHOLD) 271 return "onhold"; 272 if (code == EpisodeOfCareStatus.FINISHED) 273 return "finished"; 274 if (code == EpisodeOfCareStatus.CANCELLED) 275 return "cancelled"; 276 if (code == EpisodeOfCareStatus.ENTEREDINERROR) 277 return "entered-in-error"; 278 return "?"; 279 } 280 281 public String toSystem(EpisodeOfCareStatus code) { 282 return code.getSystem(); 283 } 284 } 285 286 @Block() 287 public static class EpisodeOfCareStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 288 /** 289 * planned | waitlist | active | onhold | finished | cancelled. 290 */ 291 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 292 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episode-of-care-status") 294 protected Enumeration<EpisodeOfCareStatus> status; 295 296 /** 297 * The period during this EpisodeOfCare that the specific status applied. 298 */ 299 @Child(name = "period", type = { Period.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 300 @Description(shortDefinition = "Duration the EpisodeOfCare was in the specified status", formalDefinition = "The period during this EpisodeOfCare that the specific status applied.") 301 protected Period period; 302 303 private static final long serialVersionUID = -1192432864L; 304 305 /** 306 * Constructor 307 */ 308 public EpisodeOfCareStatusHistoryComponent() { 309 super(); 310 } 311 312 /** 313 * Constructor 314 */ 315 public EpisodeOfCareStatusHistoryComponent(Enumeration<EpisodeOfCareStatus> status, Period period) { 316 super(); 317 this.status = status; 318 this.period = period; 319 } 320 321 /** 322 * @return {@link #status} (planned | waitlist | active | onhold | finished | 323 * cancelled.). This is the underlying object with id, value and 324 * extensions. The accessor "getStatus" gives direct access to the value 325 */ 326 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 327 if (this.status == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.status"); 330 else if (Configuration.doAutoCreate()) 331 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 332 return this.status; 333 } 334 335 public boolean hasStatusElement() { 336 return this.status != null && !this.status.isEmpty(); 337 } 338 339 public boolean hasStatus() { 340 return this.status != null && !this.status.isEmpty(); 341 } 342 343 /** 344 * @param value {@link #status} (planned | waitlist | active | onhold | finished 345 * | cancelled.). This is the underlying object with id, value and 346 * extensions. The accessor "getStatus" gives direct access to the 347 * value 348 */ 349 public EpisodeOfCareStatusHistoryComponent setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 350 this.status = value; 351 return this; 352 } 353 354 /** 355 * @return planned | waitlist | active | onhold | finished | cancelled. 356 */ 357 public EpisodeOfCareStatus getStatus() { 358 return this.status == null ? null : this.status.getValue(); 359 } 360 361 /** 362 * @param value planned | waitlist | active | onhold | finished | cancelled. 363 */ 364 public EpisodeOfCareStatusHistoryComponent setStatus(EpisodeOfCareStatus value) { 365 if (this.status == null) 366 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 367 this.status.setValue(value); 368 return this; 369 } 370 371 /** 372 * @return {@link #period} (The period during this EpisodeOfCare that the 373 * specific status applied.) 374 */ 375 public Period getPeriod() { 376 if (this.period == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.period"); 379 else if (Configuration.doAutoCreate()) 380 this.period = new Period(); // cc 381 return this.period; 382 } 383 384 public boolean hasPeriod() { 385 return this.period != null && !this.period.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #period} (The period during this EpisodeOfCare that the 390 * specific status applied.) 391 */ 392 public EpisodeOfCareStatusHistoryComponent setPeriod(Period value) { 393 this.period = value; 394 return this; 395 } 396 397 protected void listChildren(List<Property> children) { 398 super.listChildren(children); 399 children.add( 400 new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 401 children.add(new Property("period", "Period", 402 "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period)); 403 } 404 405 @Override 406 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 407 switch (_hash) { 408 case -892481550: 409 /* status */ return new Property("status", "code", 410 "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 411 case -991726143: 412 /* period */ return new Property("period", "Period", 413 "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period); 414 default: 415 return super.getNamedProperty(_hash, _name, _checkValid); 416 } 417 418 } 419 420 @Override 421 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 422 switch (hash) { 423 case -892481550: 424 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EpisodeOfCareStatus> 425 case -991726143: 426 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 427 default: 428 return super.getProperty(hash, name, checkValid); 429 } 430 431 } 432 433 @Override 434 public Base setProperty(int hash, String name, Base value) throws FHIRException { 435 switch (hash) { 436 case -892481550: // status 437 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 438 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 439 return value; 440 case -991726143: // period 441 this.period = castToPeriod(value); // Period 442 return value; 443 default: 444 return super.setProperty(hash, name, value); 445 } 446 447 } 448 449 @Override 450 public Base setProperty(String name, Base value) throws FHIRException { 451 if (name.equals("status")) { 452 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 453 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 454 } else if (name.equals("period")) { 455 this.period = castToPeriod(value); // Period 456 } else 457 return super.setProperty(name, value); 458 return value; 459 } 460 461 @Override 462 public void removeChild(String name, Base value) throws FHIRException { 463 if (name.equals("status")) { 464 this.status = null; 465 } else if (name.equals("period")) { 466 this.period = null; 467 } else 468 super.removeChild(name, value); 469 470 } 471 472 @Override 473 public Base makeProperty(int hash, String name) throws FHIRException { 474 switch (hash) { 475 case -892481550: 476 return getStatusElement(); 477 case -991726143: 478 return getPeriod(); 479 default: 480 return super.makeProperty(hash, name); 481 } 482 483 } 484 485 @Override 486 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 487 switch (hash) { 488 case -892481550: 489 /* status */ return new String[] { "code" }; 490 case -991726143: 491 /* period */ return new String[] { "Period" }; 492 default: 493 return super.getTypesForProperty(hash, name); 494 } 495 496 } 497 498 @Override 499 public Base addChild(String name) throws FHIRException { 500 if (name.equals("status")) { 501 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 502 } else if (name.equals("period")) { 503 this.period = new Period(); 504 return this.period; 505 } else 506 return super.addChild(name); 507 } 508 509 public EpisodeOfCareStatusHistoryComponent copy() { 510 EpisodeOfCareStatusHistoryComponent dst = new EpisodeOfCareStatusHistoryComponent(); 511 copyValues(dst); 512 return dst; 513 } 514 515 public void copyValues(EpisodeOfCareStatusHistoryComponent dst) { 516 super.copyValues(dst); 517 dst.status = status == null ? null : status.copy(); 518 dst.period = period == null ? null : period.copy(); 519 } 520 521 @Override 522 public boolean equalsDeep(Base other_) { 523 if (!super.equalsDeep(other_)) 524 return false; 525 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 526 return false; 527 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 528 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 529 } 530 531 @Override 532 public boolean equalsShallow(Base other_) { 533 if (!super.equalsShallow(other_)) 534 return false; 535 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 536 return false; 537 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 538 return compareValues(status, o.status, true); 539 } 540 541 public boolean isEmpty() { 542 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 543 } 544 545 public String fhirType() { 546 return "EpisodeOfCare.statusHistory"; 547 548 } 549 550 } 551 552 @Block() 553 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 554 /** 555 * A list of conditions/problems/diagnoses that this episode of care is intended 556 * to be providing care for. 557 */ 558 @Child(name = "condition", type = { 559 Condition.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 560 @Description(shortDefinition = "Conditions/problems/diagnoses this episode of care is for", formalDefinition = "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.") 561 protected Reference condition; 562 563 /** 564 * The actual object that is the target of the reference (A list of 565 * conditions/problems/diagnoses that this episode of care is intended to be 566 * providing care for.) 567 */ 568 protected Condition conditionTarget; 569 570 /** 571 * Role that this diagnosis has within the episode of care (e.g. admission, 572 * billing, discharge ?). 573 */ 574 @Child(name = "role", type = { 575 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 576 @Description(shortDefinition = "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)", formalDefinition = "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).") 577 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/diagnosis-role") 578 protected CodeableConcept role; 579 580 /** 581 * Ranking of the diagnosis (for each role type). 582 */ 583 @Child(name = "rank", type = { 584 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 585 @Description(shortDefinition = "Ranking of the diagnosis (for each role type)", formalDefinition = "Ranking of the diagnosis (for each role type).") 586 protected PositiveIntType rank; 587 588 private static final long serialVersionUID = 249445632L; 589 590 /** 591 * Constructor 592 */ 593 public DiagnosisComponent() { 594 super(); 595 } 596 597 /** 598 * Constructor 599 */ 600 public DiagnosisComponent(Reference condition) { 601 super(); 602 this.condition = condition; 603 } 604 605 /** 606 * @return {@link #condition} (A list of conditions/problems/diagnoses that this 607 * episode of care is intended to be providing care for.) 608 */ 609 public Reference getCondition() { 610 if (this.condition == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 613 else if (Configuration.doAutoCreate()) 614 this.condition = new Reference(); // cc 615 return this.condition; 616 } 617 618 public boolean hasCondition() { 619 return this.condition != null && !this.condition.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #condition} (A list of conditions/problems/diagnoses that 624 * this episode of care is intended to be providing care for.) 625 */ 626 public DiagnosisComponent setCondition(Reference value) { 627 this.condition = value; 628 return this; 629 } 630 631 /** 632 * @return {@link #condition} The actual object that is the target of the 633 * reference. The reference library doesn't populate this, but you can 634 * use it to hold the resource if you resolve it. (A list of 635 * conditions/problems/diagnoses that this episode of care is intended 636 * to be providing care for.) 637 */ 638 public Condition getConditionTarget() { 639 if (this.conditionTarget == null) 640 if (Configuration.errorOnAutoCreate()) 641 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 642 else if (Configuration.doAutoCreate()) 643 this.conditionTarget = new Condition(); // aa 644 return this.conditionTarget; 645 } 646 647 /** 648 * @param value {@link #condition} The actual object that is the target of the 649 * reference. The reference library doesn't use these, but you can 650 * use it to hold the resource if you resolve it. (A list of 651 * conditions/problems/diagnoses that this episode of care is 652 * intended to be providing care for.) 653 */ 654 public DiagnosisComponent setConditionTarget(Condition value) { 655 this.conditionTarget = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #role} (Role that this diagnosis has within the episode of 661 * care (e.g. admission, billing, discharge ?).) 662 */ 663 public CodeableConcept getRole() { 664 if (this.role == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create DiagnosisComponent.role"); 667 else if (Configuration.doAutoCreate()) 668 this.role = new CodeableConcept(); // cc 669 return this.role; 670 } 671 672 public boolean hasRole() { 673 return this.role != null && !this.role.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #role} (Role that this diagnosis has within the episode 678 * of care (e.g. admission, billing, discharge ?).) 679 */ 680 public DiagnosisComponent setRole(CodeableConcept value) { 681 this.role = value; 682 return this; 683 } 684 685 /** 686 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This 687 * is the underlying object with id, value and extensions. The accessor 688 * "getRank" gives direct access to the value 689 */ 690 public PositiveIntType getRankElement() { 691 if (this.rank == null) 692 if (Configuration.errorOnAutoCreate()) 693 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 694 else if (Configuration.doAutoCreate()) 695 this.rank = new PositiveIntType(); // bb 696 return this.rank; 697 } 698 699 public boolean hasRankElement() { 700 return this.rank != null && !this.rank.isEmpty(); 701 } 702 703 public boolean hasRank() { 704 return this.rank != null && !this.rank.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). 709 * This is the underlying object with id, value and extensions. The 710 * accessor "getRank" gives direct access to the value 711 */ 712 public DiagnosisComponent setRankElement(PositiveIntType value) { 713 this.rank = value; 714 return this; 715 } 716 717 /** 718 * @return Ranking of the diagnosis (for each role type). 719 */ 720 public int getRank() { 721 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 722 } 723 724 /** 725 * @param value Ranking of the diagnosis (for each role type). 726 */ 727 public DiagnosisComponent setRank(int value) { 728 if (this.rank == null) 729 this.rank = new PositiveIntType(); 730 this.rank.setValue(value); 731 return this; 732 } 733 734 protected void listChildren(List<Property> children) { 735 super.listChildren(children); 736 children.add(new Property("condition", "Reference(Condition)", 737 "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 0, 738 1, condition)); 739 children.add(new Property("role", "CodeableConcept", 740 "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, 741 role)); 742 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 743 } 744 745 @Override 746 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 747 switch (_hash) { 748 case -861311717: 749 /* condition */ return new Property("condition", "Reference(Condition)", 750 "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 751 0, 1, condition); 752 case 3506294: 753 /* role */ return new Property("role", "CodeableConcept", 754 "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, 755 role); 756 case 3492908: 757 /* rank */ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, 758 rank); 759 default: 760 return super.getNamedProperty(_hash, _name, _checkValid); 761 } 762 763 } 764 765 @Override 766 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 767 switch (hash) { 768 case -861311717: 769 /* condition */ return this.condition == null ? new Base[0] : new Base[] { this.condition }; // Reference 770 case 3506294: 771 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 772 case 3492908: 773 /* rank */ return this.rank == null ? new Base[0] : new Base[] { this.rank }; // PositiveIntType 774 default: 775 return super.getProperty(hash, name, checkValid); 776 } 777 778 } 779 780 @Override 781 public Base setProperty(int hash, String name, Base value) throws FHIRException { 782 switch (hash) { 783 case -861311717: // condition 784 this.condition = castToReference(value); // Reference 785 return value; 786 case 3506294: // role 787 this.role = castToCodeableConcept(value); // CodeableConcept 788 return value; 789 case 3492908: // rank 790 this.rank = castToPositiveInt(value); // PositiveIntType 791 return value; 792 default: 793 return super.setProperty(hash, name, value); 794 } 795 796 } 797 798 @Override 799 public Base setProperty(String name, Base value) throws FHIRException { 800 if (name.equals("condition")) { 801 this.condition = castToReference(value); // Reference 802 } else if (name.equals("role")) { 803 this.role = castToCodeableConcept(value); // CodeableConcept 804 } else if (name.equals("rank")) { 805 this.rank = castToPositiveInt(value); // PositiveIntType 806 } else 807 return super.setProperty(name, value); 808 return value; 809 } 810 811 @Override 812 public void removeChild(String name, Base value) throws FHIRException { 813 if (name.equals("condition")) { 814 this.condition = null; 815 } else if (name.equals("role")) { 816 this.role = null; 817 } else if (name.equals("rank")) { 818 this.rank = null; 819 } else 820 super.removeChild(name, value); 821 822 } 823 824 @Override 825 public Base makeProperty(int hash, String name) throws FHIRException { 826 switch (hash) { 827 case -861311717: 828 return getCondition(); 829 case 3506294: 830 return getRole(); 831 case 3492908: 832 return getRankElement(); 833 default: 834 return super.makeProperty(hash, name); 835 } 836 837 } 838 839 @Override 840 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 841 switch (hash) { 842 case -861311717: 843 /* condition */ return new String[] { "Reference" }; 844 case 3506294: 845 /* role */ return new String[] { "CodeableConcept" }; 846 case 3492908: 847 /* rank */ return new String[] { "positiveInt" }; 848 default: 849 return super.getTypesForProperty(hash, name); 850 } 851 852 } 853 854 @Override 855 public Base addChild(String name) throws FHIRException { 856 if (name.equals("condition")) { 857 this.condition = new Reference(); 858 return this.condition; 859 } else if (name.equals("role")) { 860 this.role = new CodeableConcept(); 861 return this.role; 862 } else if (name.equals("rank")) { 863 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.rank"); 864 } else 865 return super.addChild(name); 866 } 867 868 public DiagnosisComponent copy() { 869 DiagnosisComponent dst = new DiagnosisComponent(); 870 copyValues(dst); 871 return dst; 872 } 873 874 public void copyValues(DiagnosisComponent dst) { 875 super.copyValues(dst); 876 dst.condition = condition == null ? null : condition.copy(); 877 dst.role = role == null ? null : role.copy(); 878 dst.rank = rank == null ? null : rank.copy(); 879 } 880 881 @Override 882 public boolean equalsDeep(Base other_) { 883 if (!super.equalsDeep(other_)) 884 return false; 885 if (!(other_ instanceof DiagnosisComponent)) 886 return false; 887 DiagnosisComponent o = (DiagnosisComponent) other_; 888 return compareDeep(condition, o.condition, true) && compareDeep(role, o.role, true) 889 && compareDeep(rank, o.rank, true); 890 } 891 892 @Override 893 public boolean equalsShallow(Base other_) { 894 if (!super.equalsShallow(other_)) 895 return false; 896 if (!(other_ instanceof DiagnosisComponent)) 897 return false; 898 DiagnosisComponent o = (DiagnosisComponent) other_; 899 return compareValues(rank, o.rank, true); 900 } 901 902 public boolean isEmpty() { 903 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, role, rank); 904 } 905 906 public String fhirType() { 907 return "EpisodeOfCare.diagnosis"; 908 909 } 910 911 } 912 913 /** 914 * The EpisodeOfCare may be known by different identifiers for different 915 * contexts of use, such as when an external agency is tracking the Episode for 916 * funding purposes. 917 */ 918 @Child(name = "identifier", type = { 919 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 920 @Description(shortDefinition = "Business Identifier(s) relevant for this EpisodeOfCare", formalDefinition = "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.") 921 protected List<Identifier> identifier; 922 923 /** 924 * planned | waitlist | active | onhold | finished | cancelled. 925 */ 926 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 927 @Description(shortDefinition = "planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition = "planned | waitlist | active | onhold | finished | cancelled.") 928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episode-of-care-status") 929 protected Enumeration<EpisodeOfCareStatus> status; 930 931 /** 932 * The history of statuses that the EpisodeOfCare has been through (without 933 * requiring processing the history of the resource). 934 */ 935 @Child(name = "statusHistory", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 936 @Description(shortDefinition = "Past list of status codes (the current status may be included to cover the start date of the status)", formalDefinition = "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).") 937 protected List<EpisodeOfCareStatusHistoryComponent> statusHistory; 938 939 /** 940 * A classification of the type of episode of care; e.g. specialist referral, 941 * disease management, type of funded care. 942 */ 943 @Child(name = "type", type = { 944 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 945 @Description(shortDefinition = "Type/class - e.g. specialist referral, disease management", formalDefinition = "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.") 946 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/episodeofcare-type") 947 protected List<CodeableConcept> type; 948 949 /** 950 * The list of diagnosis relevant to this episode of care. 951 */ 952 @Child(name = "diagnosis", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 953 @Description(shortDefinition = "The list of diagnosis relevant to this episode of care", formalDefinition = "The list of diagnosis relevant to this episode of care.") 954 protected List<DiagnosisComponent> diagnosis; 955 956 /** 957 * The patient who is the focus of this episode of care. 958 */ 959 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 960 @Description(shortDefinition = "The patient who is the focus of this episode of care", formalDefinition = "The patient who is the focus of this episode of care.") 961 protected Reference patient; 962 963 /** 964 * The actual object that is the target of the reference (The patient who is the 965 * focus of this episode of care.) 966 */ 967 protected Patient patientTarget; 968 969 /** 970 * The organization that has assumed the specific responsibilities for the 971 * specified duration. 972 */ 973 @Child(name = "managingOrganization", type = { 974 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 975 @Description(shortDefinition = "Organization that assumes care", formalDefinition = "The organization that has assumed the specific responsibilities for the specified duration.") 976 protected Reference managingOrganization; 977 978 /** 979 * The actual object that is the target of the reference (The organization that 980 * has assumed the specific responsibilities for the specified duration.) 981 */ 982 protected Organization managingOrganizationTarget; 983 984 /** 985 * The interval during which the managing organization assumes the defined 986 * responsibility. 987 */ 988 @Child(name = "period", type = { Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 989 @Description(shortDefinition = "Interval during responsibility is assumed", formalDefinition = "The interval during which the managing organization assumes the defined responsibility.") 990 protected Period period; 991 992 /** 993 * Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming 994 * referrals. 995 */ 996 @Child(name = "referralRequest", type = { 997 ServiceRequest.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 998 @Description(shortDefinition = "Originating Referral Request(s)", formalDefinition = "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.") 999 protected List<Reference> referralRequest; 1000 /** 1001 * The actual objects that are the target of the reference (Referral Request(s) 1002 * that are fulfilled by this EpisodeOfCare, incoming referrals.) 1003 */ 1004 protected List<ServiceRequest> referralRequestTarget; 1005 1006 /** 1007 * The practitioner that is the care manager/care coordinator for this patient. 1008 */ 1009 @Child(name = "careManager", type = { Practitioner.class, 1010 PractitionerRole.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1011 @Description(shortDefinition = "Care manager/care coordinator for the patient", formalDefinition = "The practitioner that is the care manager/care coordinator for this patient.") 1012 protected Reference careManager; 1013 1014 /** 1015 * The actual object that is the target of the reference (The practitioner that 1016 * is the care manager/care coordinator for this patient.) 1017 */ 1018 protected Resource careManagerTarget; 1019 1020 /** 1021 * The list of practitioners that may be facilitating this episode of care for 1022 * specific purposes. 1023 */ 1024 @Child(name = "team", type = { 1025 CareTeam.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1026 @Description(shortDefinition = "Other practitioners facilitating this episode of care", formalDefinition = "The list of practitioners that may be facilitating this episode of care for specific purposes.") 1027 protected List<Reference> team; 1028 /** 1029 * The actual objects that are the target of the reference (The list of 1030 * practitioners that may be facilitating this episode of care for specific 1031 * purposes.) 1032 */ 1033 protected List<CareTeam> teamTarget; 1034 1035 /** 1036 * The set of accounts that may be used for billing for this EpisodeOfCare. 1037 */ 1038 @Child(name = "account", type = { 1039 Account.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1040 @Description(shortDefinition = "The set of accounts that may be used for billing for this EpisodeOfCare", formalDefinition = "The set of accounts that may be used for billing for this EpisodeOfCare.") 1041 protected List<Reference> account; 1042 /** 1043 * The actual objects that are the target of the reference (The set of accounts 1044 * that may be used for billing for this EpisodeOfCare.) 1045 */ 1046 protected List<Account> accountTarget; 1047 1048 private static final long serialVersionUID = 548033949L; 1049 1050 /** 1051 * Constructor 1052 */ 1053 public EpisodeOfCare() { 1054 super(); 1055 } 1056 1057 /** 1058 * Constructor 1059 */ 1060 public EpisodeOfCare(Enumeration<EpisodeOfCareStatus> status, Reference patient) { 1061 super(); 1062 this.status = status; 1063 this.patient = patient; 1064 } 1065 1066 /** 1067 * @return {@link #identifier} (The EpisodeOfCare may be known by different 1068 * identifiers for different contexts of use, such as when an external 1069 * agency is tracking the Episode for funding purposes.) 1070 */ 1071 public List<Identifier> getIdentifier() { 1072 if (this.identifier == null) 1073 this.identifier = new ArrayList<Identifier>(); 1074 return this.identifier; 1075 } 1076 1077 /** 1078 * @return Returns a reference to <code>this</code> for easy method chaining 1079 */ 1080 public EpisodeOfCare setIdentifier(List<Identifier> theIdentifier) { 1081 this.identifier = theIdentifier; 1082 return this; 1083 } 1084 1085 public boolean hasIdentifier() { 1086 if (this.identifier == null) 1087 return false; 1088 for (Identifier item : this.identifier) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 public Identifier addIdentifier() { // 3 1095 Identifier t = new Identifier(); 1096 if (this.identifier == null) 1097 this.identifier = new ArrayList<Identifier>(); 1098 this.identifier.add(t); 1099 return t; 1100 } 1101 1102 public EpisodeOfCare addIdentifier(Identifier t) { // 3 1103 if (t == null) 1104 return this; 1105 if (this.identifier == null) 1106 this.identifier = new ArrayList<Identifier>(); 1107 this.identifier.add(t); 1108 return this; 1109 } 1110 1111 /** 1112 * @return The first repetition of repeating field {@link #identifier}, creating 1113 * it if it does not already exist 1114 */ 1115 public Identifier getIdentifierFirstRep() { 1116 if (getIdentifier().isEmpty()) { 1117 addIdentifier(); 1118 } 1119 return getIdentifier().get(0); 1120 } 1121 1122 /** 1123 * @return {@link #status} (planned | waitlist | active | onhold | finished | 1124 * cancelled.). This is the underlying object with id, value and 1125 * extensions. The accessor "getStatus" gives direct access to the value 1126 */ 1127 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 1128 if (this.status == null) 1129 if (Configuration.errorOnAutoCreate()) 1130 throw new Error("Attempt to auto-create EpisodeOfCare.status"); 1131 else if (Configuration.doAutoCreate()) 1132 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 1133 return this.status; 1134 } 1135 1136 public boolean hasStatusElement() { 1137 return this.status != null && !this.status.isEmpty(); 1138 } 1139 1140 public boolean hasStatus() { 1141 return this.status != null && !this.status.isEmpty(); 1142 } 1143 1144 /** 1145 * @param value {@link #status} (planned | waitlist | active | onhold | finished 1146 * | cancelled.). This is the underlying object with id, value and 1147 * extensions. The accessor "getStatus" gives direct access to the 1148 * value 1149 */ 1150 public EpisodeOfCare setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 1151 this.status = value; 1152 return this; 1153 } 1154 1155 /** 1156 * @return planned | waitlist | active | onhold | finished | cancelled. 1157 */ 1158 public EpisodeOfCareStatus getStatus() { 1159 return this.status == null ? null : this.status.getValue(); 1160 } 1161 1162 /** 1163 * @param value planned | waitlist | active | onhold | finished | cancelled. 1164 */ 1165 public EpisodeOfCare setStatus(EpisodeOfCareStatus value) { 1166 if (this.status == null) 1167 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 1168 this.status.setValue(value); 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #statusHistory} (The history of statuses that the 1174 * EpisodeOfCare has been through (without requiring processing the 1175 * history of the resource).) 1176 */ 1177 public List<EpisodeOfCareStatusHistoryComponent> getStatusHistory() { 1178 if (this.statusHistory == null) 1179 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1180 return this.statusHistory; 1181 } 1182 1183 /** 1184 * @return Returns a reference to <code>this</code> for easy method chaining 1185 */ 1186 public EpisodeOfCare setStatusHistory(List<EpisodeOfCareStatusHistoryComponent> theStatusHistory) { 1187 this.statusHistory = theStatusHistory; 1188 return this; 1189 } 1190 1191 public boolean hasStatusHistory() { 1192 if (this.statusHistory == null) 1193 return false; 1194 for (EpisodeOfCareStatusHistoryComponent item : this.statusHistory) 1195 if (!item.isEmpty()) 1196 return true; 1197 return false; 1198 } 1199 1200 public EpisodeOfCareStatusHistoryComponent addStatusHistory() { // 3 1201 EpisodeOfCareStatusHistoryComponent t = new EpisodeOfCareStatusHistoryComponent(); 1202 if (this.statusHistory == null) 1203 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1204 this.statusHistory.add(t); 1205 return t; 1206 } 1207 1208 public EpisodeOfCare addStatusHistory(EpisodeOfCareStatusHistoryComponent t) { // 3 1209 if (t == null) 1210 return this; 1211 if (this.statusHistory == null) 1212 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1213 this.statusHistory.add(t); 1214 return this; 1215 } 1216 1217 /** 1218 * @return The first repetition of repeating field {@link #statusHistory}, 1219 * creating it if it does not already exist 1220 */ 1221 public EpisodeOfCareStatusHistoryComponent getStatusHistoryFirstRep() { 1222 if (getStatusHistory().isEmpty()) { 1223 addStatusHistory(); 1224 } 1225 return getStatusHistory().get(0); 1226 } 1227 1228 /** 1229 * @return {@link #type} (A classification of the type of episode of care; e.g. 1230 * specialist referral, disease management, type of funded care.) 1231 */ 1232 public List<CodeableConcept> getType() { 1233 if (this.type == null) 1234 this.type = new ArrayList<CodeableConcept>(); 1235 return this.type; 1236 } 1237 1238 /** 1239 * @return Returns a reference to <code>this</code> for easy method chaining 1240 */ 1241 public EpisodeOfCare setType(List<CodeableConcept> theType) { 1242 this.type = theType; 1243 return this; 1244 } 1245 1246 public boolean hasType() { 1247 if (this.type == null) 1248 return false; 1249 for (CodeableConcept item : this.type) 1250 if (!item.isEmpty()) 1251 return true; 1252 return false; 1253 } 1254 1255 public CodeableConcept addType() { // 3 1256 CodeableConcept t = new CodeableConcept(); 1257 if (this.type == null) 1258 this.type = new ArrayList<CodeableConcept>(); 1259 this.type.add(t); 1260 return t; 1261 } 1262 1263 public EpisodeOfCare addType(CodeableConcept t) { // 3 1264 if (t == null) 1265 return this; 1266 if (this.type == null) 1267 this.type = new ArrayList<CodeableConcept>(); 1268 this.type.add(t); 1269 return this; 1270 } 1271 1272 /** 1273 * @return The first repetition of repeating field {@link #type}, creating it if 1274 * it does not already exist 1275 */ 1276 public CodeableConcept getTypeFirstRep() { 1277 if (getType().isEmpty()) { 1278 addType(); 1279 } 1280 return getType().get(0); 1281 } 1282 1283 /** 1284 * @return {@link #diagnosis} (The list of diagnosis relevant to this episode of 1285 * care.) 1286 */ 1287 public List<DiagnosisComponent> getDiagnosis() { 1288 if (this.diagnosis == null) 1289 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1290 return this.diagnosis; 1291 } 1292 1293 /** 1294 * @return Returns a reference to <code>this</code> for easy method chaining 1295 */ 1296 public EpisodeOfCare setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1297 this.diagnosis = theDiagnosis; 1298 return this; 1299 } 1300 1301 public boolean hasDiagnosis() { 1302 if (this.diagnosis == null) 1303 return false; 1304 for (DiagnosisComponent item : this.diagnosis) 1305 if (!item.isEmpty()) 1306 return true; 1307 return false; 1308 } 1309 1310 public DiagnosisComponent addDiagnosis() { // 3 1311 DiagnosisComponent t = new DiagnosisComponent(); 1312 if (this.diagnosis == null) 1313 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1314 this.diagnosis.add(t); 1315 return t; 1316 } 1317 1318 public EpisodeOfCare addDiagnosis(DiagnosisComponent t) { // 3 1319 if (t == null) 1320 return this; 1321 if (this.diagnosis == null) 1322 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1323 this.diagnosis.add(t); 1324 return this; 1325 } 1326 1327 /** 1328 * @return The first repetition of repeating field {@link #diagnosis}, creating 1329 * it if it does not already exist 1330 */ 1331 public DiagnosisComponent getDiagnosisFirstRep() { 1332 if (getDiagnosis().isEmpty()) { 1333 addDiagnosis(); 1334 } 1335 return getDiagnosis().get(0); 1336 } 1337 1338 /** 1339 * @return {@link #patient} (The patient who is the focus of this episode of 1340 * care.) 1341 */ 1342 public Reference getPatient() { 1343 if (this.patient == null) 1344 if (Configuration.errorOnAutoCreate()) 1345 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1346 else if (Configuration.doAutoCreate()) 1347 this.patient = new Reference(); // cc 1348 return this.patient; 1349 } 1350 1351 public boolean hasPatient() { 1352 return this.patient != null && !this.patient.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #patient} (The patient who is the focus of this episode 1357 * of care.) 1358 */ 1359 public EpisodeOfCare setPatient(Reference value) { 1360 this.patient = value; 1361 return this; 1362 } 1363 1364 /** 1365 * @return {@link #patient} The actual object that is the target of the 1366 * reference. The reference library doesn't populate this, but you can 1367 * use it to hold the resource if you resolve it. (The patient who is 1368 * the focus of this episode of care.) 1369 */ 1370 public Patient getPatientTarget() { 1371 if (this.patientTarget == null) 1372 if (Configuration.errorOnAutoCreate()) 1373 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1374 else if (Configuration.doAutoCreate()) 1375 this.patientTarget = new Patient(); // aa 1376 return this.patientTarget; 1377 } 1378 1379 /** 1380 * @param value {@link #patient} The actual object that is the target of the 1381 * reference. The reference library doesn't use these, but you can 1382 * use it to hold the resource if you resolve it. (The patient who 1383 * is the focus of this episode of care.) 1384 */ 1385 public EpisodeOfCare setPatientTarget(Patient value) { 1386 this.patientTarget = value; 1387 return this; 1388 } 1389 1390 /** 1391 * @return {@link #managingOrganization} (The organization that has assumed the 1392 * specific responsibilities for the specified duration.) 1393 */ 1394 public Reference getManagingOrganization() { 1395 if (this.managingOrganization == null) 1396 if (Configuration.errorOnAutoCreate()) 1397 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1398 else if (Configuration.doAutoCreate()) 1399 this.managingOrganization = new Reference(); // cc 1400 return this.managingOrganization; 1401 } 1402 1403 public boolean hasManagingOrganization() { 1404 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1405 } 1406 1407 /** 1408 * @param value {@link #managingOrganization} (The organization that has assumed 1409 * the specific responsibilities for the specified duration.) 1410 */ 1411 public EpisodeOfCare setManagingOrganization(Reference value) { 1412 this.managingOrganization = value; 1413 return this; 1414 } 1415 1416 /** 1417 * @return {@link #managingOrganization} The actual object that is the target of 1418 * the reference. The reference library doesn't populate this, but you 1419 * can use it to hold the resource if you resolve it. (The organization 1420 * that has assumed the specific responsibilities for the specified 1421 * duration.) 1422 */ 1423 public Organization getManagingOrganizationTarget() { 1424 if (this.managingOrganizationTarget == null) 1425 if (Configuration.errorOnAutoCreate()) 1426 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1427 else if (Configuration.doAutoCreate()) 1428 this.managingOrganizationTarget = new Organization(); // aa 1429 return this.managingOrganizationTarget; 1430 } 1431 1432 /** 1433 * @param value {@link #managingOrganization} The actual object that is the 1434 * target of the reference. The reference library doesn't use 1435 * these, but you can use it to hold the resource if you resolve 1436 * it. (The organization that has assumed the specific 1437 * responsibilities for the specified duration.) 1438 */ 1439 public EpisodeOfCare setManagingOrganizationTarget(Organization value) { 1440 this.managingOrganizationTarget = value; 1441 return this; 1442 } 1443 1444 /** 1445 * @return {@link #period} (The interval during which the managing organization 1446 * assumes the defined responsibility.) 1447 */ 1448 public Period getPeriod() { 1449 if (this.period == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create EpisodeOfCare.period"); 1452 else if (Configuration.doAutoCreate()) 1453 this.period = new Period(); // cc 1454 return this.period; 1455 } 1456 1457 public boolean hasPeriod() { 1458 return this.period != null && !this.period.isEmpty(); 1459 } 1460 1461 /** 1462 * @param value {@link #period} (The interval during which the managing 1463 * organization assumes the defined responsibility.) 1464 */ 1465 public EpisodeOfCare setPeriod(Period value) { 1466 this.period = value; 1467 return this; 1468 } 1469 1470 /** 1471 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by 1472 * this EpisodeOfCare, incoming referrals.) 1473 */ 1474 public List<Reference> getReferralRequest() { 1475 if (this.referralRequest == null) 1476 this.referralRequest = new ArrayList<Reference>(); 1477 return this.referralRequest; 1478 } 1479 1480 /** 1481 * @return Returns a reference to <code>this</code> for easy method chaining 1482 */ 1483 public EpisodeOfCare setReferralRequest(List<Reference> theReferralRequest) { 1484 this.referralRequest = theReferralRequest; 1485 return this; 1486 } 1487 1488 public boolean hasReferralRequest() { 1489 if (this.referralRequest == null) 1490 return false; 1491 for (Reference item : this.referralRequest) 1492 if (!item.isEmpty()) 1493 return true; 1494 return false; 1495 } 1496 1497 public Reference addReferralRequest() { // 3 1498 Reference t = new Reference(); 1499 if (this.referralRequest == null) 1500 this.referralRequest = new ArrayList<Reference>(); 1501 this.referralRequest.add(t); 1502 return t; 1503 } 1504 1505 public EpisodeOfCare addReferralRequest(Reference t) { // 3 1506 if (t == null) 1507 return this; 1508 if (this.referralRequest == null) 1509 this.referralRequest = new ArrayList<Reference>(); 1510 this.referralRequest.add(t); 1511 return this; 1512 } 1513 1514 /** 1515 * @return The first repetition of repeating field {@link #referralRequest}, 1516 * creating it if it does not already exist 1517 */ 1518 public Reference getReferralRequestFirstRep() { 1519 if (getReferralRequest().isEmpty()) { 1520 addReferralRequest(); 1521 } 1522 return getReferralRequest().get(0); 1523 } 1524 1525 /** 1526 * @deprecated Use Reference#setResource(IBaseResource) instead 1527 */ 1528 @Deprecated 1529 public List<ServiceRequest> getReferralRequestTarget() { 1530 if (this.referralRequestTarget == null) 1531 this.referralRequestTarget = new ArrayList<ServiceRequest>(); 1532 return this.referralRequestTarget; 1533 } 1534 1535 /** 1536 * @deprecated Use Reference#setResource(IBaseResource) instead 1537 */ 1538 @Deprecated 1539 public ServiceRequest addReferralRequestTarget() { 1540 ServiceRequest r = new ServiceRequest(); 1541 if (this.referralRequestTarget == null) 1542 this.referralRequestTarget = new ArrayList<ServiceRequest>(); 1543 this.referralRequestTarget.add(r); 1544 return r; 1545 } 1546 1547 /** 1548 * @return {@link #careManager} (The practitioner that is the care manager/care 1549 * coordinator for this patient.) 1550 */ 1551 public Reference getCareManager() { 1552 if (this.careManager == null) 1553 if (Configuration.errorOnAutoCreate()) 1554 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1555 else if (Configuration.doAutoCreate()) 1556 this.careManager = new Reference(); // cc 1557 return this.careManager; 1558 } 1559 1560 public boolean hasCareManager() { 1561 return this.careManager != null && !this.careManager.isEmpty(); 1562 } 1563 1564 /** 1565 * @param value {@link #careManager} (The practitioner that is the care 1566 * manager/care coordinator for this patient.) 1567 */ 1568 public EpisodeOfCare setCareManager(Reference value) { 1569 this.careManager = value; 1570 return this; 1571 } 1572 1573 /** 1574 * @return {@link #careManager} The actual object that is the target of the 1575 * reference. The reference library doesn't populate this, but you can 1576 * use it to hold the resource if you resolve it. (The practitioner that 1577 * is the care manager/care coordinator for this patient.) 1578 */ 1579 public Resource getCareManagerTarget() { 1580 return this.careManagerTarget; 1581 } 1582 1583 /** 1584 * @param value {@link #careManager} The actual object that is the target of the 1585 * reference. The reference library doesn't use these, but you can 1586 * use it to hold the resource if you resolve it. (The practitioner 1587 * that is the care manager/care coordinator for this patient.) 1588 */ 1589 public EpisodeOfCare setCareManagerTarget(Resource value) { 1590 this.careManagerTarget = value; 1591 return this; 1592 } 1593 1594 /** 1595 * @return {@link #team} (The list of practitioners that may be facilitating 1596 * this episode of care for specific purposes.) 1597 */ 1598 public List<Reference> getTeam() { 1599 if (this.team == null) 1600 this.team = new ArrayList<Reference>(); 1601 return this.team; 1602 } 1603 1604 /** 1605 * @return Returns a reference to <code>this</code> for easy method chaining 1606 */ 1607 public EpisodeOfCare setTeam(List<Reference> theTeam) { 1608 this.team = theTeam; 1609 return this; 1610 } 1611 1612 public boolean hasTeam() { 1613 if (this.team == null) 1614 return false; 1615 for (Reference item : this.team) 1616 if (!item.isEmpty()) 1617 return true; 1618 return false; 1619 } 1620 1621 public Reference addTeam() { // 3 1622 Reference t = new Reference(); 1623 if (this.team == null) 1624 this.team = new ArrayList<Reference>(); 1625 this.team.add(t); 1626 return t; 1627 } 1628 1629 public EpisodeOfCare addTeam(Reference t) { // 3 1630 if (t == null) 1631 return this; 1632 if (this.team == null) 1633 this.team = new ArrayList<Reference>(); 1634 this.team.add(t); 1635 return this; 1636 } 1637 1638 /** 1639 * @return The first repetition of repeating field {@link #team}, creating it if 1640 * it does not already exist 1641 */ 1642 public Reference getTeamFirstRep() { 1643 if (getTeam().isEmpty()) { 1644 addTeam(); 1645 } 1646 return getTeam().get(0); 1647 } 1648 1649 /** 1650 * @deprecated Use Reference#setResource(IBaseResource) instead 1651 */ 1652 @Deprecated 1653 public List<CareTeam> getTeamTarget() { 1654 if (this.teamTarget == null) 1655 this.teamTarget = new ArrayList<CareTeam>(); 1656 return this.teamTarget; 1657 } 1658 1659 /** 1660 * @deprecated Use Reference#setResource(IBaseResource) instead 1661 */ 1662 @Deprecated 1663 public CareTeam addTeamTarget() { 1664 CareTeam r = new CareTeam(); 1665 if (this.teamTarget == null) 1666 this.teamTarget = new ArrayList<CareTeam>(); 1667 this.teamTarget.add(r); 1668 return r; 1669 } 1670 1671 /** 1672 * @return {@link #account} (The set of accounts that may be used for billing 1673 * for this EpisodeOfCare.) 1674 */ 1675 public List<Reference> getAccount() { 1676 if (this.account == null) 1677 this.account = new ArrayList<Reference>(); 1678 return this.account; 1679 } 1680 1681 /** 1682 * @return Returns a reference to <code>this</code> for easy method chaining 1683 */ 1684 public EpisodeOfCare setAccount(List<Reference> theAccount) { 1685 this.account = theAccount; 1686 return this; 1687 } 1688 1689 public boolean hasAccount() { 1690 if (this.account == null) 1691 return false; 1692 for (Reference item : this.account) 1693 if (!item.isEmpty()) 1694 return true; 1695 return false; 1696 } 1697 1698 public Reference addAccount() { // 3 1699 Reference t = new Reference(); 1700 if (this.account == null) 1701 this.account = new ArrayList<Reference>(); 1702 this.account.add(t); 1703 return t; 1704 } 1705 1706 public EpisodeOfCare addAccount(Reference t) { // 3 1707 if (t == null) 1708 return this; 1709 if (this.account == null) 1710 this.account = new ArrayList<Reference>(); 1711 this.account.add(t); 1712 return this; 1713 } 1714 1715 /** 1716 * @return The first repetition of repeating field {@link #account}, creating it 1717 * if it does not already exist 1718 */ 1719 public Reference getAccountFirstRep() { 1720 if (getAccount().isEmpty()) { 1721 addAccount(); 1722 } 1723 return getAccount().get(0); 1724 } 1725 1726 /** 1727 * @deprecated Use Reference#setResource(IBaseResource) instead 1728 */ 1729 @Deprecated 1730 public List<Account> getAccountTarget() { 1731 if (this.accountTarget == null) 1732 this.accountTarget = new ArrayList<Account>(); 1733 return this.accountTarget; 1734 } 1735 1736 /** 1737 * @deprecated Use Reference#setResource(IBaseResource) instead 1738 */ 1739 @Deprecated 1740 public Account addAccountTarget() { 1741 Account r = new Account(); 1742 if (this.accountTarget == null) 1743 this.accountTarget = new ArrayList<Account>(); 1744 this.accountTarget.add(r); 1745 return r; 1746 } 1747 1748 protected void listChildren(List<Property> children) { 1749 super.listChildren(children); 1750 children.add(new Property("identifier", "Identifier", 1751 "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 1752 0, java.lang.Integer.MAX_VALUE, identifier)); 1753 children.add( 1754 new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 1755 children.add(new Property("statusHistory", "", 1756 "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 1757 0, java.lang.Integer.MAX_VALUE, statusHistory)); 1758 children.add(new Property("type", "CodeableConcept", 1759 "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 1760 0, java.lang.Integer.MAX_VALUE, type)); 1761 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, 1762 java.lang.Integer.MAX_VALUE, diagnosis)); 1763 children.add(new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 1764 0, 1, patient)); 1765 children.add(new Property("managingOrganization", "Reference(Organization)", 1766 "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, 1767 managingOrganization)); 1768 children.add(new Property("period", "Period", 1769 "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period)); 1770 children.add(new Property("referralRequest", "Reference(ServiceRequest)", 1771 "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, 1772 java.lang.Integer.MAX_VALUE, referralRequest)); 1773 children.add(new Property("careManager", "Reference(Practitioner|PractitionerRole)", 1774 "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager)); 1775 children.add(new Property("team", "Reference(CareTeam)", 1776 "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, 1777 java.lang.Integer.MAX_VALUE, team)); 1778 children.add(new Property("account", "Reference(Account)", 1779 "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, 1780 account)); 1781 } 1782 1783 @Override 1784 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1785 switch (_hash) { 1786 case -1618432855: 1787 /* identifier */ return new Property("identifier", "Identifier", 1788 "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 1789 0, java.lang.Integer.MAX_VALUE, identifier); 1790 case -892481550: 1791 /* status */ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 1792 0, 1, status); 1793 case -986695614: 1794 /* statusHistory */ return new Property("statusHistory", "", 1795 "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 1796 0, java.lang.Integer.MAX_VALUE, statusHistory); 1797 case 3575610: 1798 /* type */ return new Property("type", "CodeableConcept", 1799 "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 1800 0, java.lang.Integer.MAX_VALUE, type); 1801 case 1196993265: 1802 /* diagnosis */ return new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, 1803 java.lang.Integer.MAX_VALUE, diagnosis); 1804 case -791418107: 1805 /* patient */ return new Property("patient", "Reference(Patient)", 1806 "The patient who is the focus of this episode of care.", 0, 1, patient); 1807 case -2058947787: 1808 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 1809 "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, 1810 managingOrganization); 1811 case -991726143: 1812 /* period */ return new Property("period", "Period", 1813 "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period); 1814 case -310299598: 1815 /* referralRequest */ return new Property("referralRequest", "Reference(ServiceRequest)", 1816 "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, 1817 java.lang.Integer.MAX_VALUE, referralRequest); 1818 case -1147746468: 1819 /* careManager */ return new Property("careManager", "Reference(Practitioner|PractitionerRole)", 1820 "The practitioner that is the care manager/care coordinator for this patient.", 0, 1, careManager); 1821 case 3555933: 1822 /* team */ return new Property("team", "Reference(CareTeam)", 1823 "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, 1824 java.lang.Integer.MAX_VALUE, team); 1825 case -1177318867: 1826 /* account */ return new Property("account", "Reference(Account)", 1827 "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, 1828 account); 1829 default: 1830 return super.getNamedProperty(_hash, _name, _checkValid); 1831 } 1832 1833 } 1834 1835 @Override 1836 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1837 switch (hash) { 1838 case -1618432855: 1839 /* identifier */ return this.identifier == null ? new Base[0] 1840 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1841 case -892481550: 1842 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<EpisodeOfCareStatus> 1843 case -986695614: 1844 /* statusHistory */ return this.statusHistory == null ? new Base[0] 1845 : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // EpisodeOfCareStatusHistoryComponent 1846 case 3575610: 1847 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1848 case 1196993265: 1849 /* diagnosis */ return this.diagnosis == null ? new Base[0] 1850 : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1851 case -791418107: 1852 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1853 case -2058947787: 1854 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 1855 : new Base[] { this.managingOrganization }; // Reference 1856 case -991726143: 1857 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 1858 case -310299598: 1859 /* referralRequest */ return this.referralRequest == null ? new Base[0] 1860 : this.referralRequest.toArray(new Base[this.referralRequest.size()]); // Reference 1861 case -1147746468: 1862 /* careManager */ return this.careManager == null ? new Base[0] : new Base[] { this.careManager }; // Reference 1863 case 3555933: 1864 /* team */ return this.team == null ? new Base[0] : this.team.toArray(new Base[this.team.size()]); // Reference 1865 case -1177318867: 1866 /* account */ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1867 default: 1868 return super.getProperty(hash, name, checkValid); 1869 } 1870 1871 } 1872 1873 @Override 1874 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1875 switch (hash) { 1876 case -1618432855: // identifier 1877 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1878 return value; 1879 case -892481550: // status 1880 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1881 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1882 return value; 1883 case -986695614: // statusHistory 1884 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); // EpisodeOfCareStatusHistoryComponent 1885 return value; 1886 case 3575610: // type 1887 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1888 return value; 1889 case 1196993265: // diagnosis 1890 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1891 return value; 1892 case -791418107: // patient 1893 this.patient = castToReference(value); // Reference 1894 return value; 1895 case -2058947787: // managingOrganization 1896 this.managingOrganization = castToReference(value); // Reference 1897 return value; 1898 case -991726143: // period 1899 this.period = castToPeriod(value); // Period 1900 return value; 1901 case -310299598: // referralRequest 1902 this.getReferralRequest().add(castToReference(value)); // Reference 1903 return value; 1904 case -1147746468: // careManager 1905 this.careManager = castToReference(value); // Reference 1906 return value; 1907 case 3555933: // team 1908 this.getTeam().add(castToReference(value)); // Reference 1909 return value; 1910 case -1177318867: // account 1911 this.getAccount().add(castToReference(value)); // Reference 1912 return value; 1913 default: 1914 return super.setProperty(hash, name, value); 1915 } 1916 1917 } 1918 1919 @Override 1920 public Base setProperty(String name, Base value) throws FHIRException { 1921 if (name.equals("identifier")) { 1922 this.getIdentifier().add(castToIdentifier(value)); 1923 } else if (name.equals("status")) { 1924 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1925 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1926 } else if (name.equals("statusHistory")) { 1927 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); 1928 } else if (name.equals("type")) { 1929 this.getType().add(castToCodeableConcept(value)); 1930 } else if (name.equals("diagnosis")) { 1931 this.getDiagnosis().add((DiagnosisComponent) value); 1932 } else if (name.equals("patient")) { 1933 this.patient = castToReference(value); // Reference 1934 } else if (name.equals("managingOrganization")) { 1935 this.managingOrganization = castToReference(value); // Reference 1936 } else if (name.equals("period")) { 1937 this.period = castToPeriod(value); // Period 1938 } else if (name.equals("referralRequest")) { 1939 this.getReferralRequest().add(castToReference(value)); 1940 } else if (name.equals("careManager")) { 1941 this.careManager = castToReference(value); // Reference 1942 } else if (name.equals("team")) { 1943 this.getTeam().add(castToReference(value)); 1944 } else if (name.equals("account")) { 1945 this.getAccount().add(castToReference(value)); 1946 } else 1947 return super.setProperty(name, value); 1948 return value; 1949 } 1950 1951 @Override 1952 public void removeChild(String name, Base value) throws FHIRException { 1953 if (name.equals("identifier")) { 1954 this.getIdentifier().remove(castToIdentifier(value)); 1955 } else if (name.equals("status")) { 1956 this.status = null; 1957 } else if (name.equals("statusHistory")) { 1958 this.getStatusHistory().remove((EpisodeOfCareStatusHistoryComponent) value); 1959 } else if (name.equals("type")) { 1960 this.getType().remove(castToCodeableConcept(value)); 1961 } else if (name.equals("diagnosis")) { 1962 this.getDiagnosis().remove((DiagnosisComponent) value); 1963 } else if (name.equals("patient")) { 1964 this.patient = null; 1965 } else if (name.equals("managingOrganization")) { 1966 this.managingOrganization = null; 1967 } else if (name.equals("period")) { 1968 this.period = null; 1969 } else if (name.equals("referralRequest")) { 1970 this.getReferralRequest().remove(castToReference(value)); 1971 } else if (name.equals("careManager")) { 1972 this.careManager = null; 1973 } else if (name.equals("team")) { 1974 this.getTeam().remove(castToReference(value)); 1975 } else if (name.equals("account")) { 1976 this.getAccount().remove(castToReference(value)); 1977 } else 1978 super.removeChild(name, value); 1979 1980 } 1981 1982 @Override 1983 public Base makeProperty(int hash, String name) throws FHIRException { 1984 switch (hash) { 1985 case -1618432855: 1986 return addIdentifier(); 1987 case -892481550: 1988 return getStatusElement(); 1989 case -986695614: 1990 return addStatusHistory(); 1991 case 3575610: 1992 return addType(); 1993 case 1196993265: 1994 return addDiagnosis(); 1995 case -791418107: 1996 return getPatient(); 1997 case -2058947787: 1998 return getManagingOrganization(); 1999 case -991726143: 2000 return getPeriod(); 2001 case -310299598: 2002 return addReferralRequest(); 2003 case -1147746468: 2004 return getCareManager(); 2005 case 3555933: 2006 return addTeam(); 2007 case -1177318867: 2008 return addAccount(); 2009 default: 2010 return super.makeProperty(hash, name); 2011 } 2012 2013 } 2014 2015 @Override 2016 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2017 switch (hash) { 2018 case -1618432855: 2019 /* identifier */ return new String[] { "Identifier" }; 2020 case -892481550: 2021 /* status */ return new String[] { "code" }; 2022 case -986695614: 2023 /* statusHistory */ return new String[] {}; 2024 case 3575610: 2025 /* type */ return new String[] { "CodeableConcept" }; 2026 case 1196993265: 2027 /* diagnosis */ return new String[] {}; 2028 case -791418107: 2029 /* patient */ return new String[] { "Reference" }; 2030 case -2058947787: 2031 /* managingOrganization */ return new String[] { "Reference" }; 2032 case -991726143: 2033 /* period */ return new String[] { "Period" }; 2034 case -310299598: 2035 /* referralRequest */ return new String[] { "Reference" }; 2036 case -1147746468: 2037 /* careManager */ return new String[] { "Reference" }; 2038 case 3555933: 2039 /* team */ return new String[] { "Reference" }; 2040 case -1177318867: 2041 /* account */ return new String[] { "Reference" }; 2042 default: 2043 return super.getTypesForProperty(hash, name); 2044 } 2045 2046 } 2047 2048 @Override 2049 public Base addChild(String name) throws FHIRException { 2050 if (name.equals("identifier")) { 2051 return addIdentifier(); 2052 } else if (name.equals("status")) { 2053 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 2054 } else if (name.equals("statusHistory")) { 2055 return addStatusHistory(); 2056 } else if (name.equals("type")) { 2057 return addType(); 2058 } else if (name.equals("diagnosis")) { 2059 return addDiagnosis(); 2060 } else if (name.equals("patient")) { 2061 this.patient = new Reference(); 2062 return this.patient; 2063 } else if (name.equals("managingOrganization")) { 2064 this.managingOrganization = new Reference(); 2065 return this.managingOrganization; 2066 } else if (name.equals("period")) { 2067 this.period = new Period(); 2068 return this.period; 2069 } else if (name.equals("referralRequest")) { 2070 return addReferralRequest(); 2071 } else if (name.equals("careManager")) { 2072 this.careManager = new Reference(); 2073 return this.careManager; 2074 } else if (name.equals("team")) { 2075 return addTeam(); 2076 } else if (name.equals("account")) { 2077 return addAccount(); 2078 } else 2079 return super.addChild(name); 2080 } 2081 2082 public String fhirType() { 2083 return "EpisodeOfCare"; 2084 2085 } 2086 2087 public EpisodeOfCare copy() { 2088 EpisodeOfCare dst = new EpisodeOfCare(); 2089 copyValues(dst); 2090 return dst; 2091 } 2092 2093 public void copyValues(EpisodeOfCare dst) { 2094 super.copyValues(dst); 2095 if (identifier != null) { 2096 dst.identifier = new ArrayList<Identifier>(); 2097 for (Identifier i : identifier) 2098 dst.identifier.add(i.copy()); 2099 } 2100 ; 2101 dst.status = status == null ? null : status.copy(); 2102 if (statusHistory != null) { 2103 dst.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 2104 for (EpisodeOfCareStatusHistoryComponent i : statusHistory) 2105 dst.statusHistory.add(i.copy()); 2106 } 2107 ; 2108 if (type != null) { 2109 dst.type = new ArrayList<CodeableConcept>(); 2110 for (CodeableConcept i : type) 2111 dst.type.add(i.copy()); 2112 } 2113 ; 2114 if (diagnosis != null) { 2115 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 2116 for (DiagnosisComponent i : diagnosis) 2117 dst.diagnosis.add(i.copy()); 2118 } 2119 ; 2120 dst.patient = patient == null ? null : patient.copy(); 2121 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2122 dst.period = period == null ? null : period.copy(); 2123 if (referralRequest != null) { 2124 dst.referralRequest = new ArrayList<Reference>(); 2125 for (Reference i : referralRequest) 2126 dst.referralRequest.add(i.copy()); 2127 } 2128 ; 2129 dst.careManager = careManager == null ? null : careManager.copy(); 2130 if (team != null) { 2131 dst.team = new ArrayList<Reference>(); 2132 for (Reference i : team) 2133 dst.team.add(i.copy()); 2134 } 2135 ; 2136 if (account != null) { 2137 dst.account = new ArrayList<Reference>(); 2138 for (Reference i : account) 2139 dst.account.add(i.copy()); 2140 } 2141 ; 2142 } 2143 2144 protected EpisodeOfCare typedCopy() { 2145 return copy(); 2146 } 2147 2148 @Override 2149 public boolean equalsDeep(Base other_) { 2150 if (!super.equalsDeep(other_)) 2151 return false; 2152 if (!(other_ instanceof EpisodeOfCare)) 2153 return false; 2154 EpisodeOfCare o = (EpisodeOfCare) other_; 2155 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2156 && compareDeep(statusHistory, o.statusHistory, true) && compareDeep(type, o.type, true) 2157 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(patient, o.patient, true) 2158 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(period, o.period, true) 2159 && compareDeep(referralRequest, o.referralRequest, true) && compareDeep(careManager, o.careManager, true) 2160 && compareDeep(team, o.team, true) && compareDeep(account, o.account, true); 2161 } 2162 2163 @Override 2164 public boolean equalsShallow(Base other_) { 2165 if (!super.equalsShallow(other_)) 2166 return false; 2167 if (!(other_ instanceof EpisodeOfCare)) 2168 return false; 2169 EpisodeOfCare o = (EpisodeOfCare) other_; 2170 return compareValues(status, o.status, true); 2171 } 2172 2173 public boolean isEmpty() { 2174 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory, type, diagnosis, 2175 patient, managingOrganization, period, referralRequest, careManager, team, account); 2176 } 2177 2178 @Override 2179 public ResourceType getResourceType() { 2180 return ResourceType.EpisodeOfCare; 2181 } 2182 2183 /** 2184 * Search parameter: <b>date</b> 2185 * <p> 2186 * Description: <b>The provided date search value falls within the episode of 2187 * care's period</b><br> 2188 * Type: <b>date</b><br> 2189 * Path: <b>EpisodeOfCare.period</b><br> 2190 * </p> 2191 */ 2192 @SearchParamDefinition(name = "date", path = "EpisodeOfCare.period", description = "The provided date search value falls within the episode of care's period", type = "date") 2193 public static final String SP_DATE = "date"; 2194 /** 2195 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2196 * <p> 2197 * Description: <b>The provided date search value falls within the episode of 2198 * care's period</b><br> 2199 * Type: <b>date</b><br> 2200 * Path: <b>EpisodeOfCare.period</b><br> 2201 * </p> 2202 */ 2203 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2204 SP_DATE); 2205 2206 /** 2207 * Search parameter: <b>identifier</b> 2208 * <p> 2209 * Description: <b>Business Identifier(s) relevant for this 2210 * EpisodeOfCare</b><br> 2211 * Type: <b>token</b><br> 2212 * Path: <b>EpisodeOfCare.identifier</b><br> 2213 * </p> 2214 */ 2215 @SearchParamDefinition(name = "identifier", path = "EpisodeOfCare.identifier", description = "Business Identifier(s) relevant for this EpisodeOfCare", type = "token") 2216 public static final String SP_IDENTIFIER = "identifier"; 2217 /** 2218 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2219 * <p> 2220 * Description: <b>Business Identifier(s) relevant for this 2221 * EpisodeOfCare</b><br> 2222 * Type: <b>token</b><br> 2223 * Path: <b>EpisodeOfCare.identifier</b><br> 2224 * </p> 2225 */ 2226 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2227 SP_IDENTIFIER); 2228 2229 /** 2230 * Search parameter: <b>condition</b> 2231 * <p> 2232 * Description: <b>Conditions/problems/diagnoses this episode of care is 2233 * for</b><br> 2234 * Type: <b>reference</b><br> 2235 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name = "condition", path = "EpisodeOfCare.diagnosis.condition", description = "Conditions/problems/diagnoses this episode of care is for", type = "reference", target = { 2239 Condition.class }) 2240 public static final String SP_CONDITION = "condition"; 2241 /** 2242 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 2243 * <p> 2244 * Description: <b>Conditions/problems/diagnoses this episode of care is 2245 * for</b><br> 2246 * Type: <b>reference</b><br> 2247 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2251 SP_CONDITION); 2252 2253 /** 2254 * Constant for fluent queries to be used to add include statements. Specifies 2255 * the path value of "<b>EpisodeOfCare:condition</b>". 2256 */ 2257 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include( 2258 "EpisodeOfCare:condition").toLocked(); 2259 2260 /** 2261 * Search parameter: <b>patient</b> 2262 * <p> 2263 * Description: <b>The patient who is the focus of this episode of care</b><br> 2264 * Type: <b>reference</b><br> 2265 * Path: <b>EpisodeOfCare.patient</b><br> 2266 * </p> 2267 */ 2268 @SearchParamDefinition(name = "patient", path = "EpisodeOfCare.patient", description = "The patient who is the focus of this episode of care", type = "reference", providesMembershipIn = { 2269 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2270 public static final String SP_PATIENT = "patient"; 2271 /** 2272 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2273 * <p> 2274 * Description: <b>The patient who is the focus of this episode of care</b><br> 2275 * Type: <b>reference</b><br> 2276 * Path: <b>EpisodeOfCare.patient</b><br> 2277 * </p> 2278 */ 2279 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2280 SP_PATIENT); 2281 2282 /** 2283 * Constant for fluent queries to be used to add include statements. Specifies 2284 * the path value of "<b>EpisodeOfCare:patient</b>". 2285 */ 2286 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2287 "EpisodeOfCare:patient").toLocked(); 2288 2289 /** 2290 * Search parameter: <b>organization</b> 2291 * <p> 2292 * Description: <b>The organization that has assumed the specific 2293 * responsibilities of this EpisodeOfCare</b><br> 2294 * Type: <b>reference</b><br> 2295 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2296 * </p> 2297 */ 2298 @SearchParamDefinition(name = "organization", path = "EpisodeOfCare.managingOrganization", description = "The organization that has assumed the specific responsibilities of this EpisodeOfCare", type = "reference", target = { 2299 Organization.class }) 2300 public static final String SP_ORGANIZATION = "organization"; 2301 /** 2302 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2303 * <p> 2304 * Description: <b>The organization that has assumed the specific 2305 * responsibilities of this EpisodeOfCare</b><br> 2306 * Type: <b>reference</b><br> 2307 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 2308 * </p> 2309 */ 2310 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2311 SP_ORGANIZATION); 2312 2313 /** 2314 * Constant for fluent queries to be used to add include statements. Specifies 2315 * the path value of "<b>EpisodeOfCare:organization</b>". 2316 */ 2317 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 2318 "EpisodeOfCare:organization").toLocked(); 2319 2320 /** 2321 * Search parameter: <b>type</b> 2322 * <p> 2323 * Description: <b>Type/class - e.g. specialist referral, disease 2324 * management</b><br> 2325 * Type: <b>token</b><br> 2326 * Path: <b>EpisodeOfCare.type</b><br> 2327 * </p> 2328 */ 2329 @SearchParamDefinition(name = "type", path = "EpisodeOfCare.type", description = "Type/class - e.g. specialist referral, disease management", type = "token") 2330 public static final String SP_TYPE = "type"; 2331 /** 2332 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2333 * <p> 2334 * Description: <b>Type/class - e.g. specialist referral, disease 2335 * management</b><br> 2336 * Type: <b>token</b><br> 2337 * Path: <b>EpisodeOfCare.type</b><br> 2338 * </p> 2339 */ 2340 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2341 SP_TYPE); 2342 2343 /** 2344 * Search parameter: <b>care-manager</b> 2345 * <p> 2346 * Description: <b>Care manager/care coordinator for the patient</b><br> 2347 * Type: <b>reference</b><br> 2348 * Path: <b>EpisodeOfCare.careManager</b><br> 2349 * </p> 2350 */ 2351 @SearchParamDefinition(name = "care-manager", path = "EpisodeOfCare.careManager.where(resolve() is Practitioner)", description = "Care manager/care coordinator for the patient", type = "reference", providesMembershipIn = { 2352 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Practitioner.class }) 2353 public static final String SP_CARE_MANAGER = "care-manager"; 2354 /** 2355 * <b>Fluent Client</b> search parameter constant for <b>care-manager</b> 2356 * <p> 2357 * Description: <b>Care manager/care coordinator for the patient</b><br> 2358 * Type: <b>reference</b><br> 2359 * Path: <b>EpisodeOfCare.careManager</b><br> 2360 * </p> 2361 */ 2362 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2363 SP_CARE_MANAGER); 2364 2365 /** 2366 * Constant for fluent queries to be used to add include statements. Specifies 2367 * the path value of "<b>EpisodeOfCare:care-manager</b>". 2368 */ 2369 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_MANAGER = new ca.uhn.fhir.model.api.Include( 2370 "EpisodeOfCare:care-manager").toLocked(); 2371 2372 /** 2373 * Search parameter: <b>status</b> 2374 * <p> 2375 * Description: <b>The current status of the Episode of Care as provided (does 2376 * not check the status history collection)</b><br> 2377 * Type: <b>token</b><br> 2378 * Path: <b>EpisodeOfCare.status</b><br> 2379 * </p> 2380 */ 2381 @SearchParamDefinition(name = "status", path = "EpisodeOfCare.status", description = "The current status of the Episode of Care as provided (does not check the status history collection)", type = "token") 2382 public static final String SP_STATUS = "status"; 2383 /** 2384 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2385 * <p> 2386 * Description: <b>The current status of the Episode of Care as provided (does 2387 * not check the status history collection)</b><br> 2388 * Type: <b>token</b><br> 2389 * Path: <b>EpisodeOfCare.status</b><br> 2390 * </p> 2391 */ 2392 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2393 SP_STATUS); 2394 2395 /** 2396 * Search parameter: <b>incoming-referral</b> 2397 * <p> 2398 * Description: <b>Incoming Referral Request</b><br> 2399 * Type: <b>reference</b><br> 2400 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2401 * </p> 2402 */ 2403 @SearchParamDefinition(name = "incoming-referral", path = "EpisodeOfCare.referralRequest", description = "Incoming Referral Request", type = "reference", target = { 2404 ServiceRequest.class }) 2405 public static final String SP_INCOMING_REFERRAL = "incoming-referral"; 2406 /** 2407 * <b>Fluent Client</b> search parameter constant for <b>incoming-referral</b> 2408 * <p> 2409 * Description: <b>Incoming Referral Request</b><br> 2410 * Type: <b>reference</b><br> 2411 * Path: <b>EpisodeOfCare.referralRequest</b><br> 2412 * </p> 2413 */ 2414 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMING_REFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2415 SP_INCOMING_REFERRAL); 2416 2417 /** 2418 * Constant for fluent queries to be used to add include statements. Specifies 2419 * the path value of "<b>EpisodeOfCare:incoming-referral</b>". 2420 */ 2421 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMING_REFERRAL = new ca.uhn.fhir.model.api.Include( 2422 "EpisodeOfCare:incoming-referral").toLocked(); 2423 2424}