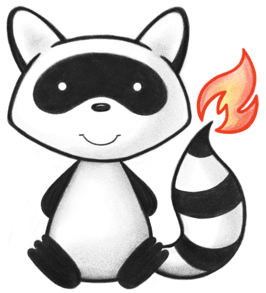
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * The EvidenceVariable resource describes a "PICO" element that knowledge 052 * (evidence, assertion, recommendation) is about. 053 */ 054@ResourceDef(name = "EvidenceVariable", profile = "http://hl7.org/fhir/StructureDefinition/EvidenceVariable") 055@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", "date", 056 "publisher", "contact", "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", 057 "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", "type", 058 "characteristic" }) 059public class EvidenceVariable extends MetadataResource { 060 061 public enum EvidenceVariableType { 062 /** 063 * The variable is dichotomous, such as present or absent. 064 */ 065 DICHOTOMOUS, 066 /** 067 * The variable is a continuous result such as a quantity. 068 */ 069 CONTINUOUS, 070 /** 071 * The variable is described narratively rather than quantitatively. 072 */ 073 DESCRIPTIVE, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static EvidenceVariableType fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("dichotomous".equals(codeString)) 083 return DICHOTOMOUS; 084 if ("continuous".equals(codeString)) 085 return CONTINUOUS; 086 if ("descriptive".equals(codeString)) 087 return DESCRIPTIVE; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown EvidenceVariableType code '" + codeString + "'"); 092 } 093 094 public String toCode() { 095 switch (this) { 096 case DICHOTOMOUS: 097 return "dichotomous"; 098 case CONTINUOUS: 099 return "continuous"; 100 case DESCRIPTIVE: 101 return "descriptive"; 102 case NULL: 103 return null; 104 default: 105 return "?"; 106 } 107 } 108 109 public String getSystem() { 110 switch (this) { 111 case DICHOTOMOUS: 112 return "http://hl7.org/fhir/variable-type"; 113 case CONTINUOUS: 114 return "http://hl7.org/fhir/variable-type"; 115 case DESCRIPTIVE: 116 return "http://hl7.org/fhir/variable-type"; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDefinition() { 125 switch (this) { 126 case DICHOTOMOUS: 127 return "The variable is dichotomous, such as present or absent."; 128 case CONTINUOUS: 129 return "The variable is a continuous result such as a quantity."; 130 case DESCRIPTIVE: 131 return "The variable is described narratively rather than quantitatively."; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDisplay() { 140 switch (this) { 141 case DICHOTOMOUS: 142 return "Dichotomous"; 143 case CONTINUOUS: 144 return "Continuous"; 145 case DESCRIPTIVE: 146 return "Descriptive"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 } 154 155 public static class EvidenceVariableTypeEnumFactory implements EnumFactory<EvidenceVariableType> { 156 public EvidenceVariableType fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("dichotomous".equals(codeString)) 161 return EvidenceVariableType.DICHOTOMOUS; 162 if ("continuous".equals(codeString)) 163 return EvidenceVariableType.CONTINUOUS; 164 if ("descriptive".equals(codeString)) 165 return EvidenceVariableType.DESCRIPTIVE; 166 throw new IllegalArgumentException("Unknown EvidenceVariableType code '" + codeString + "'"); 167 } 168 169 public Enumeration<EvidenceVariableType> fromType(PrimitiveType<?> code) throws FHIRException { 170 if (code == null) 171 return null; 172 if (code.isEmpty()) 173 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.NULL, code); 174 String codeString = code.asStringValue(); 175 if (codeString == null || "".equals(codeString)) 176 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.NULL, code); 177 if ("dichotomous".equals(codeString)) 178 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.DICHOTOMOUS, code); 179 if ("continuous".equals(codeString)) 180 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.CONTINUOUS, code); 181 if ("descriptive".equals(codeString)) 182 return new Enumeration<EvidenceVariableType>(this, EvidenceVariableType.DESCRIPTIVE, code); 183 throw new FHIRException("Unknown EvidenceVariableType code '" + codeString + "'"); 184 } 185 186 public String toCode(EvidenceVariableType code) { 187 if (code == EvidenceVariableType.NULL) 188 return null; 189 if (code == EvidenceVariableType.DICHOTOMOUS) 190 return "dichotomous"; 191 if (code == EvidenceVariableType.CONTINUOUS) 192 return "continuous"; 193 if (code == EvidenceVariableType.DESCRIPTIVE) 194 return "descriptive"; 195 return "?"; 196 } 197 198 public String toSystem(EvidenceVariableType code) { 199 return code.getSystem(); 200 } 201 } 202 203 public enum GroupMeasure { 204 /** 205 * Aggregated using Mean of participant values. 206 */ 207 MEAN, 208 /** 209 * Aggregated using Median of participant values. 210 */ 211 MEDIAN, 212 /** 213 * Aggregated using Mean of study mean values. 214 */ 215 MEANOFMEAN, 216 /** 217 * Aggregated using Mean of study median values. 218 */ 219 MEANOFMEDIAN, 220 /** 221 * Aggregated using Median of study mean values. 222 */ 223 MEDIANOFMEAN, 224 /** 225 * Aggregated using Median of study median values. 226 */ 227 MEDIANOFMEDIAN, 228 /** 229 * added to help the parsers with the generic types 230 */ 231 NULL; 232 233 public static GroupMeasure fromCode(String codeString) throws FHIRException { 234 if (codeString == null || "".equals(codeString)) 235 return null; 236 if ("mean".equals(codeString)) 237 return MEAN; 238 if ("median".equals(codeString)) 239 return MEDIAN; 240 if ("mean-of-mean".equals(codeString)) 241 return MEANOFMEAN; 242 if ("mean-of-median".equals(codeString)) 243 return MEANOFMEDIAN; 244 if ("median-of-mean".equals(codeString)) 245 return MEDIANOFMEAN; 246 if ("median-of-median".equals(codeString)) 247 return MEDIANOFMEDIAN; 248 if (Configuration.isAcceptInvalidEnums()) 249 return null; 250 else 251 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 252 } 253 254 public String toCode() { 255 switch (this) { 256 case MEAN: 257 return "mean"; 258 case MEDIAN: 259 return "median"; 260 case MEANOFMEAN: 261 return "mean-of-mean"; 262 case MEANOFMEDIAN: 263 return "mean-of-median"; 264 case MEDIANOFMEAN: 265 return "median-of-mean"; 266 case MEDIANOFMEDIAN: 267 return "median-of-median"; 268 case NULL: 269 return null; 270 default: 271 return "?"; 272 } 273 } 274 275 public String getSystem() { 276 switch (this) { 277 case MEAN: 278 return "http://hl7.org/fhir/group-measure"; 279 case MEDIAN: 280 return "http://hl7.org/fhir/group-measure"; 281 case MEANOFMEAN: 282 return "http://hl7.org/fhir/group-measure"; 283 case MEANOFMEDIAN: 284 return "http://hl7.org/fhir/group-measure"; 285 case MEDIANOFMEAN: 286 return "http://hl7.org/fhir/group-measure"; 287 case MEDIANOFMEDIAN: 288 return "http://hl7.org/fhir/group-measure"; 289 case NULL: 290 return null; 291 default: 292 return "?"; 293 } 294 } 295 296 public String getDefinition() { 297 switch (this) { 298 case MEAN: 299 return "Aggregated using Mean of participant values."; 300 case MEDIAN: 301 return "Aggregated using Median of participant values."; 302 case MEANOFMEAN: 303 return "Aggregated using Mean of study mean values."; 304 case MEANOFMEDIAN: 305 return "Aggregated using Mean of study median values."; 306 case MEDIANOFMEAN: 307 return "Aggregated using Median of study mean values."; 308 case MEDIANOFMEDIAN: 309 return "Aggregated using Median of study median values."; 310 case NULL: 311 return null; 312 default: 313 return "?"; 314 } 315 } 316 317 public String getDisplay() { 318 switch (this) { 319 case MEAN: 320 return "Mean"; 321 case MEDIAN: 322 return "Median"; 323 case MEANOFMEAN: 324 return "Mean of Study Means"; 325 case MEANOFMEDIAN: 326 return "Mean of Study Medins"; 327 case MEDIANOFMEAN: 328 return "Median of Study Means"; 329 case MEDIANOFMEDIAN: 330 return "Median of Study Medians"; 331 case NULL: 332 return null; 333 default: 334 return "?"; 335 } 336 } 337 } 338 339 public static class GroupMeasureEnumFactory implements EnumFactory<GroupMeasure> { 340 public GroupMeasure fromCode(String codeString) throws IllegalArgumentException { 341 if (codeString == null || "".equals(codeString)) 342 if (codeString == null || "".equals(codeString)) 343 return null; 344 if ("mean".equals(codeString)) 345 return GroupMeasure.MEAN; 346 if ("median".equals(codeString)) 347 return GroupMeasure.MEDIAN; 348 if ("mean-of-mean".equals(codeString)) 349 return GroupMeasure.MEANOFMEAN; 350 if ("mean-of-median".equals(codeString)) 351 return GroupMeasure.MEANOFMEDIAN; 352 if ("median-of-mean".equals(codeString)) 353 return GroupMeasure.MEDIANOFMEAN; 354 if ("median-of-median".equals(codeString)) 355 return GroupMeasure.MEDIANOFMEDIAN; 356 throw new IllegalArgumentException("Unknown GroupMeasure code '" + codeString + "'"); 357 } 358 359 public Enumeration<GroupMeasure> fromType(PrimitiveType<?> code) throws FHIRException { 360 if (code == null) 361 return null; 362 if (code.isEmpty()) 363 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 364 String codeString = code.asStringValue(); 365 if (codeString == null || "".equals(codeString)) 366 return new Enumeration<GroupMeasure>(this, GroupMeasure.NULL, code); 367 if ("mean".equals(codeString)) 368 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEAN, code); 369 if ("median".equals(codeString)) 370 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIAN, code); 371 if ("mean-of-mean".equals(codeString)) 372 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEAN, code); 373 if ("mean-of-median".equals(codeString)) 374 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEANOFMEDIAN, code); 375 if ("median-of-mean".equals(codeString)) 376 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEAN, code); 377 if ("median-of-median".equals(codeString)) 378 return new Enumeration<GroupMeasure>(this, GroupMeasure.MEDIANOFMEDIAN, code); 379 throw new FHIRException("Unknown GroupMeasure code '" + codeString + "'"); 380 } 381 382 public String toCode(GroupMeasure code) { 383 if (code == GroupMeasure.NULL) 384 return null; 385 if (code == GroupMeasure.MEAN) 386 return "mean"; 387 if (code == GroupMeasure.MEDIAN) 388 return "median"; 389 if (code == GroupMeasure.MEANOFMEAN) 390 return "mean-of-mean"; 391 if (code == GroupMeasure.MEANOFMEDIAN) 392 return "mean-of-median"; 393 if (code == GroupMeasure.MEDIANOFMEAN) 394 return "median-of-mean"; 395 if (code == GroupMeasure.MEDIANOFMEDIAN) 396 return "median-of-median"; 397 return "?"; 398 } 399 400 public String toSystem(GroupMeasure code) { 401 return code.getSystem(); 402 } 403 } 404 405 @Block() 406 public static class EvidenceVariableCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 407 /** 408 * A short, natural language description of the characteristic that could be 409 * used to communicate the criteria to an end-user. 410 */ 411 @Child(name = "description", type = { 412 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 413 @Description(shortDefinition = "Natural language description of the characteristic", formalDefinition = "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.") 414 protected StringType description; 415 416 /** 417 * Define members of the evidence element using Codes (such as condition, 418 * medication, or observation), Expressions ( using an expression language such 419 * as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in 420 * the last year). 421 */ 422 @Child(name = "definition", type = { Group.class, CanonicalType.class, CodeableConcept.class, Expression.class, 423 DataRequirement.class, TriggerDefinition.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 424 @Description(shortDefinition = "What code or expression defines members?", formalDefinition = "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).") 425 protected Type definition; 426 427 /** 428 * Use UsageContext to define the members of the population, such as Age Ranges, 429 * Genders, Settings. 430 */ 431 @Child(name = "usageContext", type = { 432 UsageContext.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 433 @Description(shortDefinition = "What code/value pairs define members?", formalDefinition = "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.") 434 protected List<UsageContext> usageContext; 435 436 /** 437 * When true, members with this characteristic are excluded from the element. 438 */ 439 @Child(name = "exclude", type = { 440 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 441 @Description(shortDefinition = "Whether the characteristic includes or excludes members", formalDefinition = "When true, members with this characteristic are excluded from the element.") 442 protected BooleanType exclude; 443 444 /** 445 * Indicates what effective period the study covers. 446 */ 447 @Child(name = "participantEffective", type = { DateTimeType.class, Period.class, Duration.class, 448 Timing.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 449 @Description(shortDefinition = "What time period do participants cover", formalDefinition = "Indicates what effective period the study covers.") 450 protected Type participantEffective; 451 452 /** 453 * Indicates duration from the participant's study entry. 454 */ 455 @Child(name = "timeFromStart", type = { 456 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 457 @Description(shortDefinition = "Observation time from study start", formalDefinition = "Indicates duration from the participant's study entry.") 458 protected Duration timeFromStart; 459 460 /** 461 * Indicates how elements are aggregated within the study effective period. 462 */ 463 @Child(name = "groupMeasure", type = { 464 CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 465 @Description(shortDefinition = "mean | median | mean-of-mean | mean-of-median | median-of-mean | median-of-median", formalDefinition = "Indicates how elements are aggregated within the study effective period.") 466 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-measure") 467 protected Enumeration<GroupMeasure> groupMeasure; 468 469 private static final long serialVersionUID = 1901961318L; 470 471 /** 472 * Constructor 473 */ 474 public EvidenceVariableCharacteristicComponent() { 475 super(); 476 } 477 478 /** 479 * Constructor 480 */ 481 public EvidenceVariableCharacteristicComponent(Type definition) { 482 super(); 483 this.definition = definition; 484 } 485 486 /** 487 * @return {@link #description} (A short, natural language description of the 488 * characteristic that could be used to communicate the criteria to an 489 * end-user.). This is the underlying object with id, value and 490 * extensions. The accessor "getDescription" gives direct access to the 491 * value 492 */ 493 public StringType getDescriptionElement() { 494 if (this.description == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.description"); 497 else if (Configuration.doAutoCreate()) 498 this.description = new StringType(); // bb 499 return this.description; 500 } 501 502 public boolean hasDescriptionElement() { 503 return this.description != null && !this.description.isEmpty(); 504 } 505 506 public boolean hasDescription() { 507 return this.description != null && !this.description.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #description} (A short, natural language description of 512 * the characteristic that could be used to communicate the 513 * criteria to an end-user.). This is the underlying object with 514 * id, value and extensions. The accessor "getDescription" gives 515 * direct access to the value 516 */ 517 public EvidenceVariableCharacteristicComponent setDescriptionElement(StringType value) { 518 this.description = value; 519 return this; 520 } 521 522 /** 523 * @return A short, natural language description of the characteristic that 524 * could be used to communicate the criteria to an end-user. 525 */ 526 public String getDescription() { 527 return this.description == null ? null : this.description.getValue(); 528 } 529 530 /** 531 * @param value A short, natural language description of the characteristic that 532 * could be used to communicate the criteria to an end-user. 533 */ 534 public EvidenceVariableCharacteristicComponent setDescription(String value) { 535 if (Utilities.noString(value)) 536 this.description = null; 537 else { 538 if (this.description == null) 539 this.description = new StringType(); 540 this.description.setValue(value); 541 } 542 return this; 543 } 544 545 /** 546 * @return {@link #definition} (Define members of the evidence element using 547 * Codes (such as condition, medication, or observation), Expressions ( 548 * using an expression language such as FHIRPath or CQL) or 549 * DataRequirements (such as Diabetes diagnosis onset in the last 550 * year).) 551 */ 552 public Type getDefinition() { 553 return this.definition; 554 } 555 556 /** 557 * @return {@link #definition} (Define members of the evidence element using 558 * Codes (such as condition, medication, or observation), Expressions ( 559 * using an expression language such as FHIRPath or CQL) or 560 * DataRequirements (such as Diabetes diagnosis onset in the last 561 * year).) 562 */ 563 public Reference getDefinitionReference() throws FHIRException { 564 if (this.definition == null) 565 this.definition = new Reference(); 566 if (!(this.definition instanceof Reference)) 567 throw new FHIRException("Type mismatch: the type Reference was expected, but " 568 + this.definition.getClass().getName() + " was encountered"); 569 return (Reference) this.definition; 570 } 571 572 public boolean hasDefinitionReference() { 573 return this != null && this.definition instanceof Reference; 574 } 575 576 /** 577 * @return {@link #definition} (Define members of the evidence element using 578 * Codes (such as condition, medication, or observation), Expressions ( 579 * using an expression language such as FHIRPath or CQL) or 580 * DataRequirements (such as Diabetes diagnosis onset in the last 581 * year).) 582 */ 583 public CanonicalType getDefinitionCanonicalType() throws FHIRException { 584 if (this.definition == null) 585 this.definition = new CanonicalType(); 586 if (!(this.definition instanceof CanonicalType)) 587 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 588 + this.definition.getClass().getName() + " was encountered"); 589 return (CanonicalType) this.definition; 590 } 591 592 public boolean hasDefinitionCanonicalType() { 593 return this != null && this.definition instanceof CanonicalType; 594 } 595 596 /** 597 * @return {@link #definition} (Define members of the evidence element using 598 * Codes (such as condition, medication, or observation), Expressions ( 599 * using an expression language such as FHIRPath or CQL) or 600 * DataRequirements (such as Diabetes diagnosis onset in the last 601 * year).) 602 */ 603 public CodeableConcept getDefinitionCodeableConcept() throws FHIRException { 604 if (this.definition == null) 605 this.definition = new CodeableConcept(); 606 if (!(this.definition instanceof CodeableConcept)) 607 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 608 + this.definition.getClass().getName() + " was encountered"); 609 return (CodeableConcept) this.definition; 610 } 611 612 public boolean hasDefinitionCodeableConcept() { 613 return this != null && this.definition instanceof CodeableConcept; 614 } 615 616 /** 617 * @return {@link #definition} (Define members of the evidence element using 618 * Codes (such as condition, medication, or observation), Expressions ( 619 * using an expression language such as FHIRPath or CQL) or 620 * DataRequirements (such as Diabetes diagnosis onset in the last 621 * year).) 622 */ 623 public Expression getDefinitionExpression() throws FHIRException { 624 if (this.definition == null) 625 this.definition = new Expression(); 626 if (!(this.definition instanceof Expression)) 627 throw new FHIRException("Type mismatch: the type Expression was expected, but " 628 + this.definition.getClass().getName() + " was encountered"); 629 return (Expression) this.definition; 630 } 631 632 public boolean hasDefinitionExpression() { 633 return this != null && this.definition instanceof Expression; 634 } 635 636 /** 637 * @return {@link #definition} (Define members of the evidence element using 638 * Codes (such as condition, medication, or observation), Expressions ( 639 * using an expression language such as FHIRPath or CQL) or 640 * DataRequirements (such as Diabetes diagnosis onset in the last 641 * year).) 642 */ 643 public DataRequirement getDefinitionDataRequirement() throws FHIRException { 644 if (this.definition == null) 645 this.definition = new DataRequirement(); 646 if (!(this.definition instanceof DataRequirement)) 647 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but " 648 + this.definition.getClass().getName() + " was encountered"); 649 return (DataRequirement) this.definition; 650 } 651 652 public boolean hasDefinitionDataRequirement() { 653 return this != null && this.definition instanceof DataRequirement; 654 } 655 656 /** 657 * @return {@link #definition} (Define members of the evidence element using 658 * Codes (such as condition, medication, or observation), Expressions ( 659 * using an expression language such as FHIRPath or CQL) or 660 * DataRequirements (such as Diabetes diagnosis onset in the last 661 * year).) 662 */ 663 public TriggerDefinition getDefinitionTriggerDefinition() throws FHIRException { 664 if (this.definition == null) 665 this.definition = new TriggerDefinition(); 666 if (!(this.definition instanceof TriggerDefinition)) 667 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but " 668 + this.definition.getClass().getName() + " was encountered"); 669 return (TriggerDefinition) this.definition; 670 } 671 672 public boolean hasDefinitionTriggerDefinition() { 673 return this != null && this.definition instanceof TriggerDefinition; 674 } 675 676 public boolean hasDefinition() { 677 return this.definition != null && !this.definition.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #definition} (Define members of the evidence element 682 * using Codes (such as condition, medication, or observation), 683 * Expressions ( using an expression language such as FHIRPath or 684 * CQL) or DataRequirements (such as Diabetes diagnosis onset in 685 * the last year).) 686 */ 687 public EvidenceVariableCharacteristicComponent setDefinition(Type value) { 688 if (value != null 689 && !(value instanceof Reference || value instanceof CanonicalType || value instanceof CodeableConcept 690 || value instanceof Expression || value instanceof DataRequirement || value instanceof TriggerDefinition)) 691 throw new Error("Not the right type for EvidenceVariable.characteristic.definition[x]: " + value.fhirType()); 692 this.definition = value; 693 return this; 694 } 695 696 /** 697 * @return {@link #usageContext} (Use UsageContext to define the members of the 698 * population, such as Age Ranges, Genders, Settings.) 699 */ 700 public List<UsageContext> getUsageContext() { 701 if (this.usageContext == null) 702 this.usageContext = new ArrayList<UsageContext>(); 703 return this.usageContext; 704 } 705 706 /** 707 * @return Returns a reference to <code>this</code> for easy method chaining 708 */ 709 public EvidenceVariableCharacteristicComponent setUsageContext(List<UsageContext> theUsageContext) { 710 this.usageContext = theUsageContext; 711 return this; 712 } 713 714 public boolean hasUsageContext() { 715 if (this.usageContext == null) 716 return false; 717 for (UsageContext item : this.usageContext) 718 if (!item.isEmpty()) 719 return true; 720 return false; 721 } 722 723 public UsageContext addUsageContext() { // 3 724 UsageContext t = new UsageContext(); 725 if (this.usageContext == null) 726 this.usageContext = new ArrayList<UsageContext>(); 727 this.usageContext.add(t); 728 return t; 729 } 730 731 public EvidenceVariableCharacteristicComponent addUsageContext(UsageContext t) { // 3 732 if (t == null) 733 return this; 734 if (this.usageContext == null) 735 this.usageContext = new ArrayList<UsageContext>(); 736 this.usageContext.add(t); 737 return this; 738 } 739 740 /** 741 * @return The first repetition of repeating field {@link #usageContext}, 742 * creating it if it does not already exist 743 */ 744 public UsageContext getUsageContextFirstRep() { 745 if (getUsageContext().isEmpty()) { 746 addUsageContext(); 747 } 748 return getUsageContext().get(0); 749 } 750 751 /** 752 * @return {@link #exclude} (When true, members with this characteristic are 753 * excluded from the element.). This is the underlying object with id, 754 * value and extensions. The accessor "getExclude" gives direct access 755 * to the value 756 */ 757 public BooleanType getExcludeElement() { 758 if (this.exclude == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.exclude"); 761 else if (Configuration.doAutoCreate()) 762 this.exclude = new BooleanType(); // bb 763 return this.exclude; 764 } 765 766 public boolean hasExcludeElement() { 767 return this.exclude != null && !this.exclude.isEmpty(); 768 } 769 770 public boolean hasExclude() { 771 return this.exclude != null && !this.exclude.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #exclude} (When true, members with this characteristic 776 * are excluded from the element.). This is the underlying object 777 * with id, value and extensions. The accessor "getExclude" gives 778 * direct access to the value 779 */ 780 public EvidenceVariableCharacteristicComponent setExcludeElement(BooleanType value) { 781 this.exclude = value; 782 return this; 783 } 784 785 /** 786 * @return When true, members with this characteristic are excluded from the 787 * element. 788 */ 789 public boolean getExclude() { 790 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 791 } 792 793 /** 794 * @param value When true, members with this characteristic are excluded from 795 * the element. 796 */ 797 public EvidenceVariableCharacteristicComponent setExclude(boolean value) { 798 if (this.exclude == null) 799 this.exclude = new BooleanType(); 800 this.exclude.setValue(value); 801 return this; 802 } 803 804 /** 805 * @return {@link #participantEffective} (Indicates what effective period the 806 * study covers.) 807 */ 808 public Type getParticipantEffective() { 809 return this.participantEffective; 810 } 811 812 /** 813 * @return {@link #participantEffective} (Indicates what effective period the 814 * study covers.) 815 */ 816 public DateTimeType getParticipantEffectiveDateTimeType() throws FHIRException { 817 if (this.participantEffective == null) 818 this.participantEffective = new DateTimeType(); 819 if (!(this.participantEffective instanceof DateTimeType)) 820 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 821 + this.participantEffective.getClass().getName() + " was encountered"); 822 return (DateTimeType) this.participantEffective; 823 } 824 825 public boolean hasParticipantEffectiveDateTimeType() { 826 return this != null && this.participantEffective instanceof DateTimeType; 827 } 828 829 /** 830 * @return {@link #participantEffective} (Indicates what effective period the 831 * study covers.) 832 */ 833 public Period getParticipantEffectivePeriod() throws FHIRException { 834 if (this.participantEffective == null) 835 this.participantEffective = new Period(); 836 if (!(this.participantEffective instanceof Period)) 837 throw new FHIRException("Type mismatch: the type Period was expected, but " 838 + this.participantEffective.getClass().getName() + " was encountered"); 839 return (Period) this.participantEffective; 840 } 841 842 public boolean hasParticipantEffectivePeriod() { 843 return this != null && this.participantEffective instanceof Period; 844 } 845 846 /** 847 * @return {@link #participantEffective} (Indicates what effective period the 848 * study covers.) 849 */ 850 public Duration getParticipantEffectiveDuration() throws FHIRException { 851 if (this.participantEffective == null) 852 this.participantEffective = new Duration(); 853 if (!(this.participantEffective instanceof Duration)) 854 throw new FHIRException("Type mismatch: the type Duration was expected, but " 855 + this.participantEffective.getClass().getName() + " was encountered"); 856 return (Duration) this.participantEffective; 857 } 858 859 public boolean hasParticipantEffectiveDuration() { 860 return this != null && this.participantEffective instanceof Duration; 861 } 862 863 /** 864 * @return {@link #participantEffective} (Indicates what effective period the 865 * study covers.) 866 */ 867 public Timing getParticipantEffectiveTiming() throws FHIRException { 868 if (this.participantEffective == null) 869 this.participantEffective = new Timing(); 870 if (!(this.participantEffective instanceof Timing)) 871 throw new FHIRException("Type mismatch: the type Timing was expected, but " 872 + this.participantEffective.getClass().getName() + " was encountered"); 873 return (Timing) this.participantEffective; 874 } 875 876 public boolean hasParticipantEffectiveTiming() { 877 return this != null && this.participantEffective instanceof Timing; 878 } 879 880 public boolean hasParticipantEffective() { 881 return this.participantEffective != null && !this.participantEffective.isEmpty(); 882 } 883 884 /** 885 * @param value {@link #participantEffective} (Indicates what effective period 886 * the study covers.) 887 */ 888 public EvidenceVariableCharacteristicComponent setParticipantEffective(Type value) { 889 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Duration 890 || value instanceof Timing)) 891 throw new Error( 892 "Not the right type for EvidenceVariable.characteristic.participantEffective[x]: " + value.fhirType()); 893 this.participantEffective = value; 894 return this; 895 } 896 897 /** 898 * @return {@link #timeFromStart} (Indicates duration from the participant's 899 * study entry.) 900 */ 901 public Duration getTimeFromStart() { 902 if (this.timeFromStart == null) 903 if (Configuration.errorOnAutoCreate()) 904 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.timeFromStart"); 905 else if (Configuration.doAutoCreate()) 906 this.timeFromStart = new Duration(); // cc 907 return this.timeFromStart; 908 } 909 910 public boolean hasTimeFromStart() { 911 return this.timeFromStart != null && !this.timeFromStart.isEmpty(); 912 } 913 914 /** 915 * @param value {@link #timeFromStart} (Indicates duration from the 916 * participant's study entry.) 917 */ 918 public EvidenceVariableCharacteristicComponent setTimeFromStart(Duration value) { 919 this.timeFromStart = value; 920 return this; 921 } 922 923 /** 924 * @return {@link #groupMeasure} (Indicates how elements are aggregated within 925 * the study effective period.). This is the underlying object with id, 926 * value and extensions. The accessor "getGroupMeasure" gives direct 927 * access to the value 928 */ 929 public Enumeration<GroupMeasure> getGroupMeasureElement() { 930 if (this.groupMeasure == null) 931 if (Configuration.errorOnAutoCreate()) 932 throw new Error("Attempt to auto-create EvidenceVariableCharacteristicComponent.groupMeasure"); 933 else if (Configuration.doAutoCreate()) 934 this.groupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); // bb 935 return this.groupMeasure; 936 } 937 938 public boolean hasGroupMeasureElement() { 939 return this.groupMeasure != null && !this.groupMeasure.isEmpty(); 940 } 941 942 public boolean hasGroupMeasure() { 943 return this.groupMeasure != null && !this.groupMeasure.isEmpty(); 944 } 945 946 /** 947 * @param value {@link #groupMeasure} (Indicates how elements are aggregated 948 * within the study effective period.). This is the underlying 949 * object with id, value and extensions. The accessor 950 * "getGroupMeasure" gives direct access to the value 951 */ 952 public EvidenceVariableCharacteristicComponent setGroupMeasureElement(Enumeration<GroupMeasure> value) { 953 this.groupMeasure = value; 954 return this; 955 } 956 957 /** 958 * @return Indicates how elements are aggregated within the study effective 959 * period. 960 */ 961 public GroupMeasure getGroupMeasure() { 962 return this.groupMeasure == null ? null : this.groupMeasure.getValue(); 963 } 964 965 /** 966 * @param value Indicates how elements are aggregated within the study effective 967 * period. 968 */ 969 public EvidenceVariableCharacteristicComponent setGroupMeasure(GroupMeasure value) { 970 if (value == null) 971 this.groupMeasure = null; 972 else { 973 if (this.groupMeasure == null) 974 this.groupMeasure = new Enumeration<GroupMeasure>(new GroupMeasureEnumFactory()); 975 this.groupMeasure.setValue(value); 976 } 977 return this; 978 } 979 980 protected void listChildren(List<Property> children) { 981 super.listChildren(children); 982 children.add(new Property("description", "string", 983 "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 984 0, 1, description)); 985 children.add(new Property("definition[x]", 986 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 987 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 988 0, 1, definition)); 989 children.add(new Property("usageContext", "UsageContext", 990 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 991 java.lang.Integer.MAX_VALUE, usageContext)); 992 children.add(new Property("exclude", "boolean", 993 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude)); 994 children.add(new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 995 "Indicates what effective period the study covers.", 0, 1, participantEffective)); 996 children.add(new Property("timeFromStart", "Duration", "Indicates duration from the participant's study entry.", 997 0, 1, timeFromStart)); 998 children.add(new Property("groupMeasure", "code", 999 "Indicates how elements are aggregated within the study effective period.", 0, 1, groupMeasure)); 1000 } 1001 1002 @Override 1003 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1004 switch (_hash) { 1005 case -1724546052: 1006 /* description */ return new Property("description", "string", 1007 "A short, natural language description of the characteristic that could be used to communicate the criteria to an end-user.", 1008 0, 1, description); 1009 case -1139422643: 1010 /* definition[x] */ return new Property("definition[x]", 1011 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1012 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1013 0, 1, definition); 1014 case -1014418093: 1015 /* definition */ return new Property("definition[x]", 1016 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1017 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1018 0, 1, definition); 1019 case -820021448: 1020 /* definitionReference */ return new Property("definition[x]", 1021 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1022 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1023 0, 1, definition); 1024 case 933485793: 1025 /* definitionCanonical */ return new Property("definition[x]", 1026 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1027 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1028 0, 1, definition); 1029 case -1446002226: 1030 /* definitionCodeableConcept */ return new Property("definition[x]", 1031 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1032 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1033 0, 1, definition); 1034 case 1463703627: 1035 /* definitionExpression */ return new Property("definition[x]", 1036 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1037 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1038 0, 1, definition); 1039 case -660350874: 1040 /* definitionDataRequirement */ return new Property("definition[x]", 1041 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1042 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1043 0, 1, definition); 1044 case -1130324968: 1045 /* definitionTriggerDefinition */ return new Property("definition[x]", 1046 "Reference(Group)|canonical(ActivityDefinition)|CodeableConcept|Expression|DataRequirement|TriggerDefinition", 1047 "Define members of the evidence element using Codes (such as condition, medication, or observation), Expressions ( using an expression language such as FHIRPath or CQL) or DataRequirements (such as Diabetes diagnosis onset in the last year).", 1048 0, 1, definition); 1049 case 907012302: 1050 /* usageContext */ return new Property("usageContext", "UsageContext", 1051 "Use UsageContext to define the members of the population, such as Age Ranges, Genders, Settings.", 0, 1052 java.lang.Integer.MAX_VALUE, usageContext); 1053 case -1321148966: 1054 /* exclude */ return new Property("exclude", "boolean", 1055 "When true, members with this characteristic are excluded from the element.", 0, 1, exclude); 1056 case 1777308748: 1057 /* participantEffective[x] */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1058 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1059 case 1376306100: 1060 /* participantEffective */ return new Property("participantEffective[x]", "dateTime|Period|Duration|Timing", 1061 "Indicates what effective period the study covers.", 0, 1, participantEffective); 1062 case -1721146513: 1063 /* participantEffectiveDateTime */ return new Property("participantEffective[x]", 1064 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1065 participantEffective); 1066 case -883650923: 1067 /* participantEffectivePeriod */ return new Property("participantEffective[x]", 1068 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1069 participantEffective); 1070 case -1210941080: 1071 /* participantEffectiveDuration */ return new Property("participantEffective[x]", 1072 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1073 participantEffective); 1074 case -765589218: 1075 /* participantEffectiveTiming */ return new Property("participantEffective[x]", 1076 "dateTime|Period|Duration|Timing", "Indicates what effective period the study covers.", 0, 1, 1077 participantEffective); 1078 case 2100140683: 1079 /* timeFromStart */ return new Property("timeFromStart", "Duration", 1080 "Indicates duration from the participant's study entry.", 0, 1, timeFromStart); 1081 case 588892639: 1082 /* groupMeasure */ return new Property("groupMeasure", "code", 1083 "Indicates how elements are aggregated within the study effective period.", 0, 1, groupMeasure); 1084 default: 1085 return super.getNamedProperty(_hash, _name, _checkValid); 1086 } 1087 1088 } 1089 1090 @Override 1091 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1092 switch (hash) { 1093 case -1724546052: 1094 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1095 case -1014418093: 1096 /* definition */ return this.definition == null ? new Base[0] : new Base[] { this.definition }; // Type 1097 case 907012302: 1098 /* usageContext */ return this.usageContext == null ? new Base[0] 1099 : this.usageContext.toArray(new Base[this.usageContext.size()]); // UsageContext 1100 case -1321148966: 1101 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 1102 case 1376306100: 1103 /* participantEffective */ return this.participantEffective == null ? new Base[0] 1104 : new Base[] { this.participantEffective }; // Type 1105 case 2100140683: 1106 /* timeFromStart */ return this.timeFromStart == null ? new Base[0] : new Base[] { this.timeFromStart }; // Duration 1107 case 588892639: 1108 /* groupMeasure */ return this.groupMeasure == null ? new Base[0] : new Base[] { this.groupMeasure }; // Enumeration<GroupMeasure> 1109 default: 1110 return super.getProperty(hash, name, checkValid); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1117 switch (hash) { 1118 case -1724546052: // description 1119 this.description = castToString(value); // StringType 1120 return value; 1121 case -1014418093: // definition 1122 this.definition = castToType(value); // Type 1123 return value; 1124 case 907012302: // usageContext 1125 this.getUsageContext().add(castToUsageContext(value)); // UsageContext 1126 return value; 1127 case -1321148966: // exclude 1128 this.exclude = castToBoolean(value); // BooleanType 1129 return value; 1130 case 1376306100: // participantEffective 1131 this.participantEffective = castToType(value); // Type 1132 return value; 1133 case 2100140683: // timeFromStart 1134 this.timeFromStart = castToDuration(value); // Duration 1135 return value; 1136 case 588892639: // groupMeasure 1137 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1138 this.groupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1139 return value; 1140 default: 1141 return super.setProperty(hash, name, value); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base setProperty(String name, Base value) throws FHIRException { 1148 if (name.equals("description")) { 1149 this.description = castToString(value); // StringType 1150 } else if (name.equals("definition[x]")) { 1151 this.definition = castToType(value); // Type 1152 } else if (name.equals("usageContext")) { 1153 this.getUsageContext().add(castToUsageContext(value)); 1154 } else if (name.equals("exclude")) { 1155 this.exclude = castToBoolean(value); // BooleanType 1156 } else if (name.equals("participantEffective[x]")) { 1157 this.participantEffective = castToType(value); // Type 1158 } else if (name.equals("timeFromStart")) { 1159 this.timeFromStart = castToDuration(value); // Duration 1160 } else if (name.equals("groupMeasure")) { 1161 value = new GroupMeasureEnumFactory().fromType(castToCode(value)); 1162 this.groupMeasure = (Enumeration) value; // Enumeration<GroupMeasure> 1163 } else 1164 return super.setProperty(name, value); 1165 return value; 1166 } 1167 1168 @Override 1169 public void removeChild(String name, Base value) throws FHIRException { 1170 if (name.equals("description")) { 1171 this.description = null; 1172 } else if (name.equals("definition[x]")) { 1173 this.definition = null; 1174 } else if (name.equals("usageContext")) { 1175 this.getUsageContext().remove(castToUsageContext(value)); 1176 } else if (name.equals("exclude")) { 1177 this.exclude = null; 1178 } else if (name.equals("participantEffective[x]")) { 1179 this.participantEffective = null; 1180 } else if (name.equals("timeFromStart")) { 1181 this.timeFromStart = null; 1182 } else if (name.equals("groupMeasure")) { 1183 this.groupMeasure = null; 1184 } else 1185 super.removeChild(name, value); 1186 1187 } 1188 1189 @Override 1190 public Base makeProperty(int hash, String name) throws FHIRException { 1191 switch (hash) { 1192 case -1724546052: 1193 return getDescriptionElement(); 1194 case -1139422643: 1195 return getDefinition(); 1196 case -1014418093: 1197 return getDefinition(); 1198 case 907012302: 1199 return addUsageContext(); 1200 case -1321148966: 1201 return getExcludeElement(); 1202 case 1777308748: 1203 return getParticipantEffective(); 1204 case 1376306100: 1205 return getParticipantEffective(); 1206 case 2100140683: 1207 return getTimeFromStart(); 1208 case 588892639: 1209 return getGroupMeasureElement(); 1210 default: 1211 return super.makeProperty(hash, name); 1212 } 1213 1214 } 1215 1216 @Override 1217 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1218 switch (hash) { 1219 case -1724546052: 1220 /* description */ return new String[] { "string" }; 1221 case -1014418093: 1222 /* definition */ return new String[] { "Reference", "canonical", "CodeableConcept", "Expression", 1223 "DataRequirement", "TriggerDefinition" }; 1224 case 907012302: 1225 /* usageContext */ return new String[] { "UsageContext" }; 1226 case -1321148966: 1227 /* exclude */ return new String[] { "boolean" }; 1228 case 1376306100: 1229 /* participantEffective */ return new String[] { "dateTime", "Period", "Duration", "Timing" }; 1230 case 2100140683: 1231 /* timeFromStart */ return new String[] { "Duration" }; 1232 case 588892639: 1233 /* groupMeasure */ return new String[] { "code" }; 1234 default: 1235 return super.getTypesForProperty(hash, name); 1236 } 1237 1238 } 1239 1240 @Override 1241 public Base addChild(String name) throws FHIRException { 1242 if (name.equals("description")) { 1243 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 1244 } else if (name.equals("definitionReference")) { 1245 this.definition = new Reference(); 1246 return this.definition; 1247 } else if (name.equals("definitionCanonical")) { 1248 this.definition = new CanonicalType(); 1249 return this.definition; 1250 } else if (name.equals("definitionCodeableConcept")) { 1251 this.definition = new CodeableConcept(); 1252 return this.definition; 1253 } else if (name.equals("definitionExpression")) { 1254 this.definition = new Expression(); 1255 return this.definition; 1256 } else if (name.equals("definitionDataRequirement")) { 1257 this.definition = new DataRequirement(); 1258 return this.definition; 1259 } else if (name.equals("definitionTriggerDefinition")) { 1260 this.definition = new TriggerDefinition(); 1261 return this.definition; 1262 } else if (name.equals("usageContext")) { 1263 return addUsageContext(); 1264 } else if (name.equals("exclude")) { 1265 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.exclude"); 1266 } else if (name.equals("participantEffectiveDateTime")) { 1267 this.participantEffective = new DateTimeType(); 1268 return this.participantEffective; 1269 } else if (name.equals("participantEffectivePeriod")) { 1270 this.participantEffective = new Period(); 1271 return this.participantEffective; 1272 } else if (name.equals("participantEffectiveDuration")) { 1273 this.participantEffective = new Duration(); 1274 return this.participantEffective; 1275 } else if (name.equals("participantEffectiveTiming")) { 1276 this.participantEffective = new Timing(); 1277 return this.participantEffective; 1278 } else if (name.equals("timeFromStart")) { 1279 this.timeFromStart = new Duration(); 1280 return this.timeFromStart; 1281 } else if (name.equals("groupMeasure")) { 1282 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.groupMeasure"); 1283 } else 1284 return super.addChild(name); 1285 } 1286 1287 public EvidenceVariableCharacteristicComponent copy() { 1288 EvidenceVariableCharacteristicComponent dst = new EvidenceVariableCharacteristicComponent(); 1289 copyValues(dst); 1290 return dst; 1291 } 1292 1293 public void copyValues(EvidenceVariableCharacteristicComponent dst) { 1294 super.copyValues(dst); 1295 dst.description = description == null ? null : description.copy(); 1296 dst.definition = definition == null ? null : definition.copy(); 1297 if (usageContext != null) { 1298 dst.usageContext = new ArrayList<UsageContext>(); 1299 for (UsageContext i : usageContext) 1300 dst.usageContext.add(i.copy()); 1301 } 1302 ; 1303 dst.exclude = exclude == null ? null : exclude.copy(); 1304 dst.participantEffective = participantEffective == null ? null : participantEffective.copy(); 1305 dst.timeFromStart = timeFromStart == null ? null : timeFromStart.copy(); 1306 dst.groupMeasure = groupMeasure == null ? null : groupMeasure.copy(); 1307 } 1308 1309 @Override 1310 public boolean equalsDeep(Base other_) { 1311 if (!super.equalsDeep(other_)) 1312 return false; 1313 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1314 return false; 1315 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1316 return compareDeep(description, o.description, true) && compareDeep(definition, o.definition, true) 1317 && compareDeep(usageContext, o.usageContext, true) && compareDeep(exclude, o.exclude, true) 1318 && compareDeep(participantEffective, o.participantEffective, true) 1319 && compareDeep(timeFromStart, o.timeFromStart, true) && compareDeep(groupMeasure, o.groupMeasure, true); 1320 } 1321 1322 @Override 1323 public boolean equalsShallow(Base other_) { 1324 if (!super.equalsShallow(other_)) 1325 return false; 1326 if (!(other_ instanceof EvidenceVariableCharacteristicComponent)) 1327 return false; 1328 EvidenceVariableCharacteristicComponent o = (EvidenceVariableCharacteristicComponent) other_; 1329 return compareValues(description, o.description, true) && compareValues(exclude, o.exclude, true) 1330 && compareValues(groupMeasure, o.groupMeasure, true); 1331 } 1332 1333 public boolean isEmpty() { 1334 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, definition, usageContext, exclude, 1335 participantEffective, timeFromStart, groupMeasure); 1336 } 1337 1338 public String fhirType() { 1339 return "EvidenceVariable.characteristic"; 1340 1341 } 1342 1343 } 1344 1345 /** 1346 * A formal identifier that is used to identify this evidence variable when it 1347 * is represented in other formats, or referenced in a specification, model, 1348 * design or an instance. 1349 */ 1350 @Child(name = "identifier", type = { 1351 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1352 @Description(shortDefinition = "Additional identifier for the evidence variable", formalDefinition = "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1353 protected List<Identifier> identifier; 1354 1355 /** 1356 * The short title provides an alternate title for use in informal descriptive 1357 * contexts where the full, formal title is not necessary. 1358 */ 1359 @Child(name = "shortTitle", type = { 1360 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1361 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 1362 protected StringType shortTitle; 1363 1364 /** 1365 * An explanatory or alternate title for the EvidenceVariable giving additional 1366 * information about its content. 1367 */ 1368 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1369 @Description(shortDefinition = "Subordinate title of the EvidenceVariable", formalDefinition = "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.") 1370 protected StringType subtitle; 1371 1372 /** 1373 * A human-readable string to clarify or explain concepts about the resource. 1374 */ 1375 @Child(name = "note", type = { 1376 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1377 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 1378 protected List<Annotation> note; 1379 1380 /** 1381 * A copyright statement relating to the evidence variable and/or its contents. 1382 * Copyright statements are generally legal restrictions on the use and 1383 * publishing of the evidence variable. 1384 */ 1385 @Child(name = "copyright", type = { 1386 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1387 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.") 1388 protected MarkdownType copyright; 1389 1390 /** 1391 * The date on which the resource content was approved by the publisher. 1392 * Approval happens once when the content is officially approved for usage. 1393 */ 1394 @Child(name = "approvalDate", type = { 1395 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1396 @Description(shortDefinition = "When the evidence variable was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 1397 protected DateType approvalDate; 1398 1399 /** 1400 * The date on which the resource content was last reviewed. Review happens 1401 * periodically after approval but does not change the original approval date. 1402 */ 1403 @Child(name = "lastReviewDate", type = { 1404 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 1405 @Description(shortDefinition = "When the evidence variable was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 1406 protected DateType lastReviewDate; 1407 1408 /** 1409 * The period during which the evidence variable content was or is planned to be 1410 * in active use. 1411 */ 1412 @Child(name = "effectivePeriod", type = { 1413 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1414 @Description(shortDefinition = "When the evidence variable is expected to be used", formalDefinition = "The period during which the evidence variable content was or is planned to be in active use.") 1415 protected Period effectivePeriod; 1416 1417 /** 1418 * Descriptive topics related to the content of the EvidenceVariable. Topics 1419 * provide a high-level categorization grouping types of EvidenceVariables that 1420 * can be useful for filtering and searching. 1421 */ 1422 @Child(name = "topic", type = { 1423 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1424 @Description(shortDefinition = "The category of the EvidenceVariable, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.") 1425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 1426 protected List<CodeableConcept> topic; 1427 1428 /** 1429 * An individiual or organization primarily involved in the creation and 1430 * maintenance of the content. 1431 */ 1432 @Child(name = "author", type = { 1433 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1434 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 1435 protected List<ContactDetail> author; 1436 1437 /** 1438 * An individual or organization primarily responsible for internal coherence of 1439 * the content. 1440 */ 1441 @Child(name = "editor", type = { 1442 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1443 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 1444 protected List<ContactDetail> editor; 1445 1446 /** 1447 * An individual or organization primarily responsible for review of some aspect 1448 * of the content. 1449 */ 1450 @Child(name = "reviewer", type = { 1451 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1452 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 1453 protected List<ContactDetail> reviewer; 1454 1455 /** 1456 * An individual or organization responsible for officially endorsing the 1457 * content for use in some setting. 1458 */ 1459 @Child(name = "endorser", type = { 1460 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1461 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 1462 protected List<ContactDetail> endorser; 1463 1464 /** 1465 * Related artifacts such as additional documentation, justification, or 1466 * bibliographic references. 1467 */ 1468 @Child(name = "relatedArtifact", type = { 1469 RelatedArtifact.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1470 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 1471 protected List<RelatedArtifact> relatedArtifact; 1472 1473 /** 1474 * The type of evidence element, a population, an exposure, or an outcome. 1475 */ 1476 @Child(name = "type", type = { CodeType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 1477 @Description(shortDefinition = "dichotomous | continuous | descriptive", formalDefinition = "The type of evidence element, a population, an exposure, or an outcome.") 1478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/variable-type") 1479 protected Enumeration<EvidenceVariableType> type; 1480 1481 /** 1482 * A characteristic that defines the members of the evidence element. Multiple 1483 * characteristics are applied with "and" semantics. 1484 */ 1485 @Child(name = "characteristic", type = {}, order = 15, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1486 @Description(shortDefinition = "What defines the members of the evidence element", formalDefinition = "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.") 1487 protected List<EvidenceVariableCharacteristicComponent> characteristic; 1488 1489 private static final long serialVersionUID = -317280154L; 1490 1491 /** 1492 * Constructor 1493 */ 1494 public EvidenceVariable() { 1495 super(); 1496 } 1497 1498 /** 1499 * Constructor 1500 */ 1501 public EvidenceVariable(Enumeration<PublicationStatus> status) { 1502 super(); 1503 this.status = status; 1504 } 1505 1506 /** 1507 * @return {@link #url} (An absolute URI that is used to identify this evidence 1508 * variable when it is referenced in a specification, model, design or 1509 * an instance; also called its canonical identifier. This SHOULD be 1510 * globally unique and SHOULD be a literal address at which at which an 1511 * authoritative instance of this evidence variable is (or will be) 1512 * published. This URL can be the target of a canonical reference. It 1513 * SHALL remain the same when the evidence variable is stored on 1514 * different servers.). This is the underlying object with id, value and 1515 * extensions. The accessor "getUrl" gives direct access to the value 1516 */ 1517 public UriType getUrlElement() { 1518 if (this.url == null) 1519 if (Configuration.errorOnAutoCreate()) 1520 throw new Error("Attempt to auto-create EvidenceVariable.url"); 1521 else if (Configuration.doAutoCreate()) 1522 this.url = new UriType(); // bb 1523 return this.url; 1524 } 1525 1526 public boolean hasUrlElement() { 1527 return this.url != null && !this.url.isEmpty(); 1528 } 1529 1530 public boolean hasUrl() { 1531 return this.url != null && !this.url.isEmpty(); 1532 } 1533 1534 /** 1535 * @param value {@link #url} (An absolute URI that is used to identify this 1536 * evidence variable when it is referenced in a specification, 1537 * model, design or an instance; also called its canonical 1538 * identifier. This SHOULD be globally unique and SHOULD be a 1539 * literal address at which at which an authoritative instance of 1540 * this evidence variable is (or will be) published. This URL can 1541 * be the target of a canonical reference. It SHALL remain the same 1542 * when the evidence variable is stored on different servers.). 1543 * This is the underlying object with id, value and extensions. The 1544 * accessor "getUrl" gives direct access to the value 1545 */ 1546 public EvidenceVariable setUrlElement(UriType value) { 1547 this.url = value; 1548 return this; 1549 } 1550 1551 /** 1552 * @return An absolute URI that is used to identify this evidence variable when 1553 * it is referenced in a specification, model, design or an instance; 1554 * also called its canonical identifier. This SHOULD be globally unique 1555 * and SHOULD be a literal address at which at which an authoritative 1556 * instance of this evidence variable is (or will be) published. This 1557 * URL can be the target of a canonical reference. It SHALL remain the 1558 * same when the evidence variable is stored on different servers. 1559 */ 1560 public String getUrl() { 1561 return this.url == null ? null : this.url.getValue(); 1562 } 1563 1564 /** 1565 * @param value An absolute URI that is used to identify this evidence variable 1566 * when it is referenced in a specification, model, design or an 1567 * instance; also called its canonical identifier. This SHOULD be 1568 * globally unique and SHOULD be a literal address at which at 1569 * which an authoritative instance of this evidence variable is (or 1570 * will be) published. This URL can be the target of a canonical 1571 * reference. It SHALL remain the same when the evidence variable 1572 * is stored on different servers. 1573 */ 1574 public EvidenceVariable setUrl(String value) { 1575 if (Utilities.noString(value)) 1576 this.url = null; 1577 else { 1578 if (this.url == null) 1579 this.url = new UriType(); 1580 this.url.setValue(value); 1581 } 1582 return this; 1583 } 1584 1585 /** 1586 * @return {@link #identifier} (A formal identifier that is used to identify 1587 * this evidence variable when it is represented in other formats, or 1588 * referenced in a specification, model, design or an instance.) 1589 */ 1590 public List<Identifier> getIdentifier() { 1591 if (this.identifier == null) 1592 this.identifier = new ArrayList<Identifier>(); 1593 return this.identifier; 1594 } 1595 1596 /** 1597 * @return Returns a reference to <code>this</code> for easy method chaining 1598 */ 1599 public EvidenceVariable setIdentifier(List<Identifier> theIdentifier) { 1600 this.identifier = theIdentifier; 1601 return this; 1602 } 1603 1604 public boolean hasIdentifier() { 1605 if (this.identifier == null) 1606 return false; 1607 for (Identifier item : this.identifier) 1608 if (!item.isEmpty()) 1609 return true; 1610 return false; 1611 } 1612 1613 public Identifier addIdentifier() { // 3 1614 Identifier t = new Identifier(); 1615 if (this.identifier == null) 1616 this.identifier = new ArrayList<Identifier>(); 1617 this.identifier.add(t); 1618 return t; 1619 } 1620 1621 public EvidenceVariable addIdentifier(Identifier t) { // 3 1622 if (t == null) 1623 return this; 1624 if (this.identifier == null) 1625 this.identifier = new ArrayList<Identifier>(); 1626 this.identifier.add(t); 1627 return this; 1628 } 1629 1630 /** 1631 * @return The first repetition of repeating field {@link #identifier}, creating 1632 * it if it does not already exist 1633 */ 1634 public Identifier getIdentifierFirstRep() { 1635 if (getIdentifier().isEmpty()) { 1636 addIdentifier(); 1637 } 1638 return getIdentifier().get(0); 1639 } 1640 1641 /** 1642 * @return {@link #version} (The identifier that is used to identify this 1643 * version of the evidence variable when it is referenced in a 1644 * specification, model, design or instance. This is an arbitrary value 1645 * managed by the evidence variable author and is not expected to be 1646 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1647 * if a managed version is not available. There is also no expectation 1648 * that versions can be placed in a lexicographical sequence. To provide 1649 * a version consistent with the Decision Support Service specification, 1650 * use the format Major.Minor.Revision (e.g. 1.0.0). For more 1651 * information on versioning knowledge assets, refer to the Decision 1652 * Support Service specification. Note that a version is required for 1653 * non-experimental active artifacts.). This is the underlying object 1654 * with id, value and extensions. The accessor "getVersion" gives direct 1655 * access to the value 1656 */ 1657 public StringType getVersionElement() { 1658 if (this.version == null) 1659 if (Configuration.errorOnAutoCreate()) 1660 throw new Error("Attempt to auto-create EvidenceVariable.version"); 1661 else if (Configuration.doAutoCreate()) 1662 this.version = new StringType(); // bb 1663 return this.version; 1664 } 1665 1666 public boolean hasVersionElement() { 1667 return this.version != null && !this.version.isEmpty(); 1668 } 1669 1670 public boolean hasVersion() { 1671 return this.version != null && !this.version.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #version} (The identifier that is used to identify this 1676 * version of the evidence variable when it is referenced in a 1677 * specification, model, design or instance. This is an arbitrary 1678 * value managed by the evidence variable author and is not 1679 * expected to be globally unique. For example, it might be a 1680 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1681 * There is also no expectation that versions can be placed in a 1682 * lexicographical sequence. To provide a version consistent with 1683 * the Decision Support Service specification, use the format 1684 * Major.Minor.Revision (e.g. 1.0.0). For more information on 1685 * versioning knowledge assets, refer to the Decision Support 1686 * Service specification. Note that a version is required for 1687 * non-experimental active artifacts.). This is the underlying 1688 * object with id, value and extensions. The accessor "getVersion" 1689 * gives direct access to the value 1690 */ 1691 public EvidenceVariable setVersionElement(StringType value) { 1692 this.version = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return The identifier that is used to identify this version of the evidence 1698 * variable when it is referenced in a specification, model, design or 1699 * instance. This is an arbitrary value managed by the evidence variable 1700 * author and is not expected to be globally unique. For example, it 1701 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 1702 * available. There is also no expectation that versions can be placed 1703 * in a lexicographical sequence. To provide a version consistent with 1704 * the Decision Support Service specification, use the format 1705 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 1706 * knowledge assets, refer to the Decision Support Service 1707 * specification. Note that a version is required for non-experimental 1708 * active artifacts. 1709 */ 1710 public String getVersion() { 1711 return this.version == null ? null : this.version.getValue(); 1712 } 1713 1714 /** 1715 * @param value The identifier that is used to identify this version of the 1716 * evidence variable when it is referenced in a specification, 1717 * model, design or instance. This is an arbitrary value managed by 1718 * the evidence variable author and is not expected to be globally 1719 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1720 * a managed version is not available. There is also no expectation 1721 * that versions can be placed in a lexicographical sequence. To 1722 * provide a version consistent with the Decision Support Service 1723 * specification, use the format Major.Minor.Revision (e.g. 1.0.0). 1724 * For more information on versioning knowledge assets, refer to 1725 * the Decision Support Service specification. Note that a version 1726 * is required for non-experimental active artifacts. 1727 */ 1728 public EvidenceVariable setVersion(String value) { 1729 if (Utilities.noString(value)) 1730 this.version = null; 1731 else { 1732 if (this.version == null) 1733 this.version = new StringType(); 1734 this.version.setValue(value); 1735 } 1736 return this; 1737 } 1738 1739 /** 1740 * @return {@link #name} (A natural language name identifying the evidence 1741 * variable. This name should be usable as an identifier for the module 1742 * by machine processing applications such as code generation.). This is 1743 * the underlying object with id, value and extensions. The accessor 1744 * "getName" gives direct access to the value 1745 */ 1746 public StringType getNameElement() { 1747 if (this.name == null) 1748 if (Configuration.errorOnAutoCreate()) 1749 throw new Error("Attempt to auto-create EvidenceVariable.name"); 1750 else if (Configuration.doAutoCreate()) 1751 this.name = new StringType(); // bb 1752 return this.name; 1753 } 1754 1755 public boolean hasNameElement() { 1756 return this.name != null && !this.name.isEmpty(); 1757 } 1758 1759 public boolean hasName() { 1760 return this.name != null && !this.name.isEmpty(); 1761 } 1762 1763 /** 1764 * @param value {@link #name} (A natural language name identifying the evidence 1765 * variable. This name should be usable as an identifier for the 1766 * module by machine processing applications such as code 1767 * generation.). This is the underlying object with id, value and 1768 * extensions. The accessor "getName" gives direct access to the 1769 * value 1770 */ 1771 public EvidenceVariable setNameElement(StringType value) { 1772 this.name = value; 1773 return this; 1774 } 1775 1776 /** 1777 * @return A natural language name identifying the evidence variable. This name 1778 * should be usable as an identifier for the module by machine 1779 * processing applications such as code generation. 1780 */ 1781 public String getName() { 1782 return this.name == null ? null : this.name.getValue(); 1783 } 1784 1785 /** 1786 * @param value A natural language name identifying the evidence variable. This 1787 * name should be usable as an identifier for the module by machine 1788 * processing applications such as code generation. 1789 */ 1790 public EvidenceVariable setName(String value) { 1791 if (Utilities.noString(value)) 1792 this.name = null; 1793 else { 1794 if (this.name == null) 1795 this.name = new StringType(); 1796 this.name.setValue(value); 1797 } 1798 return this; 1799 } 1800 1801 /** 1802 * @return {@link #title} (A short, descriptive, user-friendly title for the 1803 * evidence variable.). This is the underlying object with id, value and 1804 * extensions. The accessor "getTitle" gives direct access to the value 1805 */ 1806 public StringType getTitleElement() { 1807 if (this.title == null) 1808 if (Configuration.errorOnAutoCreate()) 1809 throw new Error("Attempt to auto-create EvidenceVariable.title"); 1810 else if (Configuration.doAutoCreate()) 1811 this.title = new StringType(); // bb 1812 return this.title; 1813 } 1814 1815 public boolean hasTitleElement() { 1816 return this.title != null && !this.title.isEmpty(); 1817 } 1818 1819 public boolean hasTitle() { 1820 return this.title != null && !this.title.isEmpty(); 1821 } 1822 1823 /** 1824 * @param value {@link #title} (A short, descriptive, user-friendly title for 1825 * the evidence variable.). This is the underlying object with id, 1826 * value and extensions. The accessor "getTitle" gives direct 1827 * access to the value 1828 */ 1829 public EvidenceVariable setTitleElement(StringType value) { 1830 this.title = value; 1831 return this; 1832 } 1833 1834 /** 1835 * @return A short, descriptive, user-friendly title for the evidence variable. 1836 */ 1837 public String getTitle() { 1838 return this.title == null ? null : this.title.getValue(); 1839 } 1840 1841 /** 1842 * @param value A short, descriptive, user-friendly title for the evidence 1843 * variable. 1844 */ 1845 public EvidenceVariable setTitle(String value) { 1846 if (Utilities.noString(value)) 1847 this.title = null; 1848 else { 1849 if (this.title == null) 1850 this.title = new StringType(); 1851 this.title.setValue(value); 1852 } 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #shortTitle} (The short title provides an alternate title for 1858 * use in informal descriptive contexts where the full, formal title is 1859 * not necessary.). This is the underlying object with id, value and 1860 * extensions. The accessor "getShortTitle" gives direct access to the 1861 * value 1862 */ 1863 public StringType getShortTitleElement() { 1864 if (this.shortTitle == null) 1865 if (Configuration.errorOnAutoCreate()) 1866 throw new Error("Attempt to auto-create EvidenceVariable.shortTitle"); 1867 else if (Configuration.doAutoCreate()) 1868 this.shortTitle = new StringType(); // bb 1869 return this.shortTitle; 1870 } 1871 1872 public boolean hasShortTitleElement() { 1873 return this.shortTitle != null && !this.shortTitle.isEmpty(); 1874 } 1875 1876 public boolean hasShortTitle() { 1877 return this.shortTitle != null && !this.shortTitle.isEmpty(); 1878 } 1879 1880 /** 1881 * @param value {@link #shortTitle} (The short title provides an alternate title 1882 * for use in informal descriptive contexts where the full, formal 1883 * title is not necessary.). This is the underlying object with id, 1884 * value and extensions. The accessor "getShortTitle" gives direct 1885 * access to the value 1886 */ 1887 public EvidenceVariable setShortTitleElement(StringType value) { 1888 this.shortTitle = value; 1889 return this; 1890 } 1891 1892 /** 1893 * @return The short title provides an alternate title for use in informal 1894 * descriptive contexts where the full, formal title is not necessary. 1895 */ 1896 public String getShortTitle() { 1897 return this.shortTitle == null ? null : this.shortTitle.getValue(); 1898 } 1899 1900 /** 1901 * @param value The short title provides an alternate title for use in informal 1902 * descriptive contexts where the full, formal title is not 1903 * necessary. 1904 */ 1905 public EvidenceVariable setShortTitle(String value) { 1906 if (Utilities.noString(value)) 1907 this.shortTitle = null; 1908 else { 1909 if (this.shortTitle == null) 1910 this.shortTitle = new StringType(); 1911 this.shortTitle.setValue(value); 1912 } 1913 return this; 1914 } 1915 1916 /** 1917 * @return {@link #subtitle} (An explanatory or alternate title for the 1918 * EvidenceVariable giving additional information about its content.). 1919 * This is the underlying object with id, value and extensions. The 1920 * accessor "getSubtitle" gives direct access to the value 1921 */ 1922 public StringType getSubtitleElement() { 1923 if (this.subtitle == null) 1924 if (Configuration.errorOnAutoCreate()) 1925 throw new Error("Attempt to auto-create EvidenceVariable.subtitle"); 1926 else if (Configuration.doAutoCreate()) 1927 this.subtitle = new StringType(); // bb 1928 return this.subtitle; 1929 } 1930 1931 public boolean hasSubtitleElement() { 1932 return this.subtitle != null && !this.subtitle.isEmpty(); 1933 } 1934 1935 public boolean hasSubtitle() { 1936 return this.subtitle != null && !this.subtitle.isEmpty(); 1937 } 1938 1939 /** 1940 * @param value {@link #subtitle} (An explanatory or alternate title for the 1941 * EvidenceVariable giving additional information about its 1942 * content.). This is the underlying object with id, value and 1943 * extensions. The accessor "getSubtitle" gives direct access to 1944 * the value 1945 */ 1946 public EvidenceVariable setSubtitleElement(StringType value) { 1947 this.subtitle = value; 1948 return this; 1949 } 1950 1951 /** 1952 * @return An explanatory or alternate title for the EvidenceVariable giving 1953 * additional information about its content. 1954 */ 1955 public String getSubtitle() { 1956 return this.subtitle == null ? null : this.subtitle.getValue(); 1957 } 1958 1959 /** 1960 * @param value An explanatory or alternate title for the EvidenceVariable 1961 * giving additional information about its content. 1962 */ 1963 public EvidenceVariable setSubtitle(String value) { 1964 if (Utilities.noString(value)) 1965 this.subtitle = null; 1966 else { 1967 if (this.subtitle == null) 1968 this.subtitle = new StringType(); 1969 this.subtitle.setValue(value); 1970 } 1971 return this; 1972 } 1973 1974 /** 1975 * @return {@link #status} (The status of this evidence variable. Enables 1976 * tracking the life-cycle of the content.). This is the underlying 1977 * object with id, value and extensions. The accessor "getStatus" gives 1978 * direct access to the value 1979 */ 1980 public Enumeration<PublicationStatus> getStatusElement() { 1981 if (this.status == null) 1982 if (Configuration.errorOnAutoCreate()) 1983 throw new Error("Attempt to auto-create EvidenceVariable.status"); 1984 else if (Configuration.doAutoCreate()) 1985 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1986 return this.status; 1987 } 1988 1989 public boolean hasStatusElement() { 1990 return this.status != null && !this.status.isEmpty(); 1991 } 1992 1993 public boolean hasStatus() { 1994 return this.status != null && !this.status.isEmpty(); 1995 } 1996 1997 /** 1998 * @param value {@link #status} (The status of this evidence variable. Enables 1999 * tracking the life-cycle of the content.). This is the underlying 2000 * object with id, value and extensions. The accessor "getStatus" 2001 * gives direct access to the value 2002 */ 2003 public EvidenceVariable setStatusElement(Enumeration<PublicationStatus> value) { 2004 this.status = value; 2005 return this; 2006 } 2007 2008 /** 2009 * @return The status of this evidence variable. Enables tracking the life-cycle 2010 * of the content. 2011 */ 2012 public PublicationStatus getStatus() { 2013 return this.status == null ? null : this.status.getValue(); 2014 } 2015 2016 /** 2017 * @param value The status of this evidence variable. Enables tracking the 2018 * life-cycle of the content. 2019 */ 2020 public EvidenceVariable setStatus(PublicationStatus value) { 2021 if (this.status == null) 2022 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2023 this.status.setValue(value); 2024 return this; 2025 } 2026 2027 /** 2028 * @return {@link #date} (The date (and optionally time) when the evidence 2029 * variable was published. The date must change when the business 2030 * version changes and it must change if the status code changes. In 2031 * addition, it should change when the substantive content of the 2032 * evidence variable changes.). This is the underlying object with id, 2033 * value and extensions. The accessor "getDate" gives direct access to 2034 * the value 2035 */ 2036 public DateTimeType getDateElement() { 2037 if (this.date == null) 2038 if (Configuration.errorOnAutoCreate()) 2039 throw new Error("Attempt to auto-create EvidenceVariable.date"); 2040 else if (Configuration.doAutoCreate()) 2041 this.date = new DateTimeType(); // bb 2042 return this.date; 2043 } 2044 2045 public boolean hasDateElement() { 2046 return this.date != null && !this.date.isEmpty(); 2047 } 2048 2049 public boolean hasDate() { 2050 return this.date != null && !this.date.isEmpty(); 2051 } 2052 2053 /** 2054 * @param value {@link #date} (The date (and optionally time) when the evidence 2055 * variable was published. The date must change when the business 2056 * version changes and it must change if the status code changes. 2057 * In addition, it should change when the substantive content of 2058 * the evidence variable changes.). This is the underlying object 2059 * with id, value and extensions. The accessor "getDate" gives 2060 * direct access to the value 2061 */ 2062 public EvidenceVariable setDateElement(DateTimeType value) { 2063 this.date = value; 2064 return this; 2065 } 2066 2067 /** 2068 * @return The date (and optionally time) when the evidence variable was 2069 * published. The date must change when the business version changes and 2070 * it must change if the status code changes. In addition, it should 2071 * change when the substantive content of the evidence variable changes. 2072 */ 2073 public Date getDate() { 2074 return this.date == null ? null : this.date.getValue(); 2075 } 2076 2077 /** 2078 * @param value The date (and optionally time) when the evidence variable was 2079 * published. The date must change when the business version 2080 * changes and it must change if the status code changes. In 2081 * addition, it should change when the substantive content of the 2082 * evidence variable changes. 2083 */ 2084 public EvidenceVariable setDate(Date value) { 2085 if (value == null) 2086 this.date = null; 2087 else { 2088 if (this.date == null) 2089 this.date = new DateTimeType(); 2090 this.date.setValue(value); 2091 } 2092 return this; 2093 } 2094 2095 /** 2096 * @return {@link #publisher} (The name of the organization or individual that 2097 * published the evidence variable.). This is the underlying object with 2098 * id, value and extensions. The accessor "getPublisher" gives direct 2099 * access to the value 2100 */ 2101 public StringType getPublisherElement() { 2102 if (this.publisher == null) 2103 if (Configuration.errorOnAutoCreate()) 2104 throw new Error("Attempt to auto-create EvidenceVariable.publisher"); 2105 else if (Configuration.doAutoCreate()) 2106 this.publisher = new StringType(); // bb 2107 return this.publisher; 2108 } 2109 2110 public boolean hasPublisherElement() { 2111 return this.publisher != null && !this.publisher.isEmpty(); 2112 } 2113 2114 public boolean hasPublisher() { 2115 return this.publisher != null && !this.publisher.isEmpty(); 2116 } 2117 2118 /** 2119 * @param value {@link #publisher} (The name of the organization or individual 2120 * that published the evidence variable.). This is the underlying 2121 * object with id, value and extensions. The accessor 2122 * "getPublisher" gives direct access to the value 2123 */ 2124 public EvidenceVariable setPublisherElement(StringType value) { 2125 this.publisher = value; 2126 return this; 2127 } 2128 2129 /** 2130 * @return The name of the organization or individual that published the 2131 * evidence variable. 2132 */ 2133 public String getPublisher() { 2134 return this.publisher == null ? null : this.publisher.getValue(); 2135 } 2136 2137 /** 2138 * @param value The name of the organization or individual that published the 2139 * evidence variable. 2140 */ 2141 public EvidenceVariable setPublisher(String value) { 2142 if (Utilities.noString(value)) 2143 this.publisher = null; 2144 else { 2145 if (this.publisher == null) 2146 this.publisher = new StringType(); 2147 this.publisher.setValue(value); 2148 } 2149 return this; 2150 } 2151 2152 /** 2153 * @return {@link #contact} (Contact details to assist a user in finding and 2154 * communicating with the publisher.) 2155 */ 2156 public List<ContactDetail> getContact() { 2157 if (this.contact == null) 2158 this.contact = new ArrayList<ContactDetail>(); 2159 return this.contact; 2160 } 2161 2162 /** 2163 * @return Returns a reference to <code>this</code> for easy method chaining 2164 */ 2165 public EvidenceVariable setContact(List<ContactDetail> theContact) { 2166 this.contact = theContact; 2167 return this; 2168 } 2169 2170 public boolean hasContact() { 2171 if (this.contact == null) 2172 return false; 2173 for (ContactDetail item : this.contact) 2174 if (!item.isEmpty()) 2175 return true; 2176 return false; 2177 } 2178 2179 public ContactDetail addContact() { // 3 2180 ContactDetail t = new ContactDetail(); 2181 if (this.contact == null) 2182 this.contact = new ArrayList<ContactDetail>(); 2183 this.contact.add(t); 2184 return t; 2185 } 2186 2187 public EvidenceVariable addContact(ContactDetail t) { // 3 2188 if (t == null) 2189 return this; 2190 if (this.contact == null) 2191 this.contact = new ArrayList<ContactDetail>(); 2192 this.contact.add(t); 2193 return this; 2194 } 2195 2196 /** 2197 * @return The first repetition of repeating field {@link #contact}, creating it 2198 * if it does not already exist 2199 */ 2200 public ContactDetail getContactFirstRep() { 2201 if (getContact().isEmpty()) { 2202 addContact(); 2203 } 2204 return getContact().get(0); 2205 } 2206 2207 /** 2208 * @return {@link #description} (A free text natural language description of the 2209 * evidence variable from a consumer's perspective.). This is the 2210 * underlying object with id, value and extensions. The accessor 2211 * "getDescription" gives direct access to the value 2212 */ 2213 public MarkdownType getDescriptionElement() { 2214 if (this.description == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create EvidenceVariable.description"); 2217 else if (Configuration.doAutoCreate()) 2218 this.description = new MarkdownType(); // bb 2219 return this.description; 2220 } 2221 2222 public boolean hasDescriptionElement() { 2223 return this.description != null && !this.description.isEmpty(); 2224 } 2225 2226 public boolean hasDescription() { 2227 return this.description != null && !this.description.isEmpty(); 2228 } 2229 2230 /** 2231 * @param value {@link #description} (A free text natural language description 2232 * of the evidence variable from a consumer's perspective.). This 2233 * is the underlying object with id, value and extensions. The 2234 * accessor "getDescription" gives direct access to the value 2235 */ 2236 public EvidenceVariable setDescriptionElement(MarkdownType value) { 2237 this.description = value; 2238 return this; 2239 } 2240 2241 /** 2242 * @return A free text natural language description of the evidence variable 2243 * from a consumer's perspective. 2244 */ 2245 public String getDescription() { 2246 return this.description == null ? null : this.description.getValue(); 2247 } 2248 2249 /** 2250 * @param value A free text natural language description of the evidence 2251 * variable from a consumer's perspective. 2252 */ 2253 public EvidenceVariable setDescription(String value) { 2254 if (value == null) 2255 this.description = null; 2256 else { 2257 if (this.description == null) 2258 this.description = new MarkdownType(); 2259 this.description.setValue(value); 2260 } 2261 return this; 2262 } 2263 2264 /** 2265 * @return {@link #note} (A human-readable string to clarify or explain concepts 2266 * about the resource.) 2267 */ 2268 public List<Annotation> getNote() { 2269 if (this.note == null) 2270 this.note = new ArrayList<Annotation>(); 2271 return this.note; 2272 } 2273 2274 /** 2275 * @return Returns a reference to <code>this</code> for easy method chaining 2276 */ 2277 public EvidenceVariable setNote(List<Annotation> theNote) { 2278 this.note = theNote; 2279 return this; 2280 } 2281 2282 public boolean hasNote() { 2283 if (this.note == null) 2284 return false; 2285 for (Annotation item : this.note) 2286 if (!item.isEmpty()) 2287 return true; 2288 return false; 2289 } 2290 2291 public Annotation addNote() { // 3 2292 Annotation t = new Annotation(); 2293 if (this.note == null) 2294 this.note = new ArrayList<Annotation>(); 2295 this.note.add(t); 2296 return t; 2297 } 2298 2299 public EvidenceVariable addNote(Annotation t) { // 3 2300 if (t == null) 2301 return this; 2302 if (this.note == null) 2303 this.note = new ArrayList<Annotation>(); 2304 this.note.add(t); 2305 return this; 2306 } 2307 2308 /** 2309 * @return The first repetition of repeating field {@link #note}, creating it if 2310 * it does not already exist 2311 */ 2312 public Annotation getNoteFirstRep() { 2313 if (getNote().isEmpty()) { 2314 addNote(); 2315 } 2316 return getNote().get(0); 2317 } 2318 2319 /** 2320 * @return {@link #useContext} (The content was developed with a focus and 2321 * intent of supporting the contexts that are listed. These contexts may 2322 * be general categories (gender, age, ...) or may be references to 2323 * specific programs (insurance plans, studies, ...) and may be used to 2324 * assist with indexing and searching for appropriate evidence variable 2325 * instances.) 2326 */ 2327 public List<UsageContext> getUseContext() { 2328 if (this.useContext == null) 2329 this.useContext = new ArrayList<UsageContext>(); 2330 return this.useContext; 2331 } 2332 2333 /** 2334 * @return Returns a reference to <code>this</code> for easy method chaining 2335 */ 2336 public EvidenceVariable setUseContext(List<UsageContext> theUseContext) { 2337 this.useContext = theUseContext; 2338 return this; 2339 } 2340 2341 public boolean hasUseContext() { 2342 if (this.useContext == null) 2343 return false; 2344 for (UsageContext item : this.useContext) 2345 if (!item.isEmpty()) 2346 return true; 2347 return false; 2348 } 2349 2350 public UsageContext addUseContext() { // 3 2351 UsageContext t = new UsageContext(); 2352 if (this.useContext == null) 2353 this.useContext = new ArrayList<UsageContext>(); 2354 this.useContext.add(t); 2355 return t; 2356 } 2357 2358 public EvidenceVariable addUseContext(UsageContext t) { // 3 2359 if (t == null) 2360 return this; 2361 if (this.useContext == null) 2362 this.useContext = new ArrayList<UsageContext>(); 2363 this.useContext.add(t); 2364 return this; 2365 } 2366 2367 /** 2368 * @return The first repetition of repeating field {@link #useContext}, creating 2369 * it if it does not already exist 2370 */ 2371 public UsageContext getUseContextFirstRep() { 2372 if (getUseContext().isEmpty()) { 2373 addUseContext(); 2374 } 2375 return getUseContext().get(0); 2376 } 2377 2378 /** 2379 * @return {@link #jurisdiction} (A legal or geographic region in which the 2380 * evidence variable is intended to be used.) 2381 */ 2382 public List<CodeableConcept> getJurisdiction() { 2383 if (this.jurisdiction == null) 2384 this.jurisdiction = new ArrayList<CodeableConcept>(); 2385 return this.jurisdiction; 2386 } 2387 2388 /** 2389 * @return Returns a reference to <code>this</code> for easy method chaining 2390 */ 2391 public EvidenceVariable setJurisdiction(List<CodeableConcept> theJurisdiction) { 2392 this.jurisdiction = theJurisdiction; 2393 return this; 2394 } 2395 2396 public boolean hasJurisdiction() { 2397 if (this.jurisdiction == null) 2398 return false; 2399 for (CodeableConcept item : this.jurisdiction) 2400 if (!item.isEmpty()) 2401 return true; 2402 return false; 2403 } 2404 2405 public CodeableConcept addJurisdiction() { // 3 2406 CodeableConcept t = new CodeableConcept(); 2407 if (this.jurisdiction == null) 2408 this.jurisdiction = new ArrayList<CodeableConcept>(); 2409 this.jurisdiction.add(t); 2410 return t; 2411 } 2412 2413 public EvidenceVariable addJurisdiction(CodeableConcept t) { // 3 2414 if (t == null) 2415 return this; 2416 if (this.jurisdiction == null) 2417 this.jurisdiction = new ArrayList<CodeableConcept>(); 2418 this.jurisdiction.add(t); 2419 return this; 2420 } 2421 2422 /** 2423 * @return The first repetition of repeating field {@link #jurisdiction}, 2424 * creating it if it does not already exist 2425 */ 2426 public CodeableConcept getJurisdictionFirstRep() { 2427 if (getJurisdiction().isEmpty()) { 2428 addJurisdiction(); 2429 } 2430 return getJurisdiction().get(0); 2431 } 2432 2433 /** 2434 * @return {@link #copyright} (A copyright statement relating to the evidence 2435 * variable and/or its contents. Copyright statements are generally 2436 * legal restrictions on the use and publishing of the evidence 2437 * variable.). This is the underlying object with id, value and 2438 * extensions. The accessor "getCopyright" gives direct access to the 2439 * value 2440 */ 2441 public MarkdownType getCopyrightElement() { 2442 if (this.copyright == null) 2443 if (Configuration.errorOnAutoCreate()) 2444 throw new Error("Attempt to auto-create EvidenceVariable.copyright"); 2445 else if (Configuration.doAutoCreate()) 2446 this.copyright = new MarkdownType(); // bb 2447 return this.copyright; 2448 } 2449 2450 public boolean hasCopyrightElement() { 2451 return this.copyright != null && !this.copyright.isEmpty(); 2452 } 2453 2454 public boolean hasCopyright() { 2455 return this.copyright != null && !this.copyright.isEmpty(); 2456 } 2457 2458 /** 2459 * @param value {@link #copyright} (A copyright statement relating to the 2460 * evidence variable and/or its contents. Copyright statements are 2461 * generally legal restrictions on the use and publishing of the 2462 * evidence variable.). This is the underlying object with id, 2463 * value and extensions. The accessor "getCopyright" gives direct 2464 * access to the value 2465 */ 2466 public EvidenceVariable setCopyrightElement(MarkdownType value) { 2467 this.copyright = value; 2468 return this; 2469 } 2470 2471 /** 2472 * @return A copyright statement relating to the evidence variable and/or its 2473 * contents. Copyright statements are generally legal restrictions on 2474 * the use and publishing of the evidence variable. 2475 */ 2476 public String getCopyright() { 2477 return this.copyright == null ? null : this.copyright.getValue(); 2478 } 2479 2480 /** 2481 * @param value A copyright statement relating to the evidence variable and/or 2482 * its contents. Copyright statements are generally legal 2483 * restrictions on the use and publishing of the evidence variable. 2484 */ 2485 public EvidenceVariable setCopyright(String value) { 2486 if (value == null) 2487 this.copyright = null; 2488 else { 2489 if (this.copyright == null) 2490 this.copyright = new MarkdownType(); 2491 this.copyright.setValue(value); 2492 } 2493 return this; 2494 } 2495 2496 /** 2497 * @return {@link #approvalDate} (The date on which the resource content was 2498 * approved by the publisher. Approval happens once when the content is 2499 * officially approved for usage.). This is the underlying object with 2500 * id, value and extensions. The accessor "getApprovalDate" gives direct 2501 * access to the value 2502 */ 2503 public DateType getApprovalDateElement() { 2504 if (this.approvalDate == null) 2505 if (Configuration.errorOnAutoCreate()) 2506 throw new Error("Attempt to auto-create EvidenceVariable.approvalDate"); 2507 else if (Configuration.doAutoCreate()) 2508 this.approvalDate = new DateType(); // bb 2509 return this.approvalDate; 2510 } 2511 2512 public boolean hasApprovalDateElement() { 2513 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2514 } 2515 2516 public boolean hasApprovalDate() { 2517 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2518 } 2519 2520 /** 2521 * @param value {@link #approvalDate} (The date on which the resource content 2522 * was approved by the publisher. Approval happens once when the 2523 * content is officially approved for usage.). This is the 2524 * underlying object with id, value and extensions. The accessor 2525 * "getApprovalDate" gives direct access to the value 2526 */ 2527 public EvidenceVariable setApprovalDateElement(DateType value) { 2528 this.approvalDate = value; 2529 return this; 2530 } 2531 2532 /** 2533 * @return The date on which the resource content was approved by the publisher. 2534 * Approval happens once when the content is officially approved for 2535 * usage. 2536 */ 2537 public Date getApprovalDate() { 2538 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2539 } 2540 2541 /** 2542 * @param value The date on which the resource content was approved by the 2543 * publisher. Approval happens once when the content is officially 2544 * approved for usage. 2545 */ 2546 public EvidenceVariable setApprovalDate(Date value) { 2547 if (value == null) 2548 this.approvalDate = null; 2549 else { 2550 if (this.approvalDate == null) 2551 this.approvalDate = new DateType(); 2552 this.approvalDate.setValue(value); 2553 } 2554 return this; 2555 } 2556 2557 /** 2558 * @return {@link #lastReviewDate} (The date on which the resource content was 2559 * last reviewed. Review happens periodically after approval but does 2560 * not change the original approval date.). This is the underlying 2561 * object with id, value and extensions. The accessor 2562 * "getLastReviewDate" gives direct access to the value 2563 */ 2564 public DateType getLastReviewDateElement() { 2565 if (this.lastReviewDate == null) 2566 if (Configuration.errorOnAutoCreate()) 2567 throw new Error("Attempt to auto-create EvidenceVariable.lastReviewDate"); 2568 else if (Configuration.doAutoCreate()) 2569 this.lastReviewDate = new DateType(); // bb 2570 return this.lastReviewDate; 2571 } 2572 2573 public boolean hasLastReviewDateElement() { 2574 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2575 } 2576 2577 public boolean hasLastReviewDate() { 2578 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #lastReviewDate} (The date on which the resource content 2583 * was last reviewed. Review happens periodically after approval 2584 * but does not change the original approval date.). This is the 2585 * underlying object with id, value and extensions. The accessor 2586 * "getLastReviewDate" gives direct access to the value 2587 */ 2588 public EvidenceVariable setLastReviewDateElement(DateType value) { 2589 this.lastReviewDate = value; 2590 return this; 2591 } 2592 2593 /** 2594 * @return The date on which the resource content was last reviewed. Review 2595 * happens periodically after approval but does not change the original 2596 * approval date. 2597 */ 2598 public Date getLastReviewDate() { 2599 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2600 } 2601 2602 /** 2603 * @param value The date on which the resource content was last reviewed. Review 2604 * happens periodically after approval but does not change the 2605 * original approval date. 2606 */ 2607 public EvidenceVariable setLastReviewDate(Date value) { 2608 if (value == null) 2609 this.lastReviewDate = null; 2610 else { 2611 if (this.lastReviewDate == null) 2612 this.lastReviewDate = new DateType(); 2613 this.lastReviewDate.setValue(value); 2614 } 2615 return this; 2616 } 2617 2618 /** 2619 * @return {@link #effectivePeriod} (The period during which the evidence 2620 * variable content was or is planned to be in active use.) 2621 */ 2622 public Period getEffectivePeriod() { 2623 if (this.effectivePeriod == null) 2624 if (Configuration.errorOnAutoCreate()) 2625 throw new Error("Attempt to auto-create EvidenceVariable.effectivePeriod"); 2626 else if (Configuration.doAutoCreate()) 2627 this.effectivePeriod = new Period(); // cc 2628 return this.effectivePeriod; 2629 } 2630 2631 public boolean hasEffectivePeriod() { 2632 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2633 } 2634 2635 /** 2636 * @param value {@link #effectivePeriod} (The period during which the evidence 2637 * variable content was or is planned to be in active use.) 2638 */ 2639 public EvidenceVariable setEffectivePeriod(Period value) { 2640 this.effectivePeriod = value; 2641 return this; 2642 } 2643 2644 /** 2645 * @return {@link #topic} (Descriptive topics related to the content of the 2646 * EvidenceVariable. Topics provide a high-level categorization grouping 2647 * types of EvidenceVariables that can be useful for filtering and 2648 * searching.) 2649 */ 2650 public List<CodeableConcept> getTopic() { 2651 if (this.topic == null) 2652 this.topic = new ArrayList<CodeableConcept>(); 2653 return this.topic; 2654 } 2655 2656 /** 2657 * @return Returns a reference to <code>this</code> for easy method chaining 2658 */ 2659 public EvidenceVariable setTopic(List<CodeableConcept> theTopic) { 2660 this.topic = theTopic; 2661 return this; 2662 } 2663 2664 public boolean hasTopic() { 2665 if (this.topic == null) 2666 return false; 2667 for (CodeableConcept item : this.topic) 2668 if (!item.isEmpty()) 2669 return true; 2670 return false; 2671 } 2672 2673 public CodeableConcept addTopic() { // 3 2674 CodeableConcept t = new CodeableConcept(); 2675 if (this.topic == null) 2676 this.topic = new ArrayList<CodeableConcept>(); 2677 this.topic.add(t); 2678 return t; 2679 } 2680 2681 public EvidenceVariable addTopic(CodeableConcept t) { // 3 2682 if (t == null) 2683 return this; 2684 if (this.topic == null) 2685 this.topic = new ArrayList<CodeableConcept>(); 2686 this.topic.add(t); 2687 return this; 2688 } 2689 2690 /** 2691 * @return The first repetition of repeating field {@link #topic}, creating it 2692 * if it does not already exist 2693 */ 2694 public CodeableConcept getTopicFirstRep() { 2695 if (getTopic().isEmpty()) { 2696 addTopic(); 2697 } 2698 return getTopic().get(0); 2699 } 2700 2701 /** 2702 * @return {@link #author} (An individiual or organization primarily involved in 2703 * the creation and maintenance of the content.) 2704 */ 2705 public List<ContactDetail> getAuthor() { 2706 if (this.author == null) 2707 this.author = new ArrayList<ContactDetail>(); 2708 return this.author; 2709 } 2710 2711 /** 2712 * @return Returns a reference to <code>this</code> for easy method chaining 2713 */ 2714 public EvidenceVariable setAuthor(List<ContactDetail> theAuthor) { 2715 this.author = theAuthor; 2716 return this; 2717 } 2718 2719 public boolean hasAuthor() { 2720 if (this.author == null) 2721 return false; 2722 for (ContactDetail item : this.author) 2723 if (!item.isEmpty()) 2724 return true; 2725 return false; 2726 } 2727 2728 public ContactDetail addAuthor() { // 3 2729 ContactDetail t = new ContactDetail(); 2730 if (this.author == null) 2731 this.author = new ArrayList<ContactDetail>(); 2732 this.author.add(t); 2733 return t; 2734 } 2735 2736 public EvidenceVariable addAuthor(ContactDetail t) { // 3 2737 if (t == null) 2738 return this; 2739 if (this.author == null) 2740 this.author = new ArrayList<ContactDetail>(); 2741 this.author.add(t); 2742 return this; 2743 } 2744 2745 /** 2746 * @return The first repetition of repeating field {@link #author}, creating it 2747 * if it does not already exist 2748 */ 2749 public ContactDetail getAuthorFirstRep() { 2750 if (getAuthor().isEmpty()) { 2751 addAuthor(); 2752 } 2753 return getAuthor().get(0); 2754 } 2755 2756 /** 2757 * @return {@link #editor} (An individual or organization primarily responsible 2758 * for internal coherence of the content.) 2759 */ 2760 public List<ContactDetail> getEditor() { 2761 if (this.editor == null) 2762 this.editor = new ArrayList<ContactDetail>(); 2763 return this.editor; 2764 } 2765 2766 /** 2767 * @return Returns a reference to <code>this</code> for easy method chaining 2768 */ 2769 public EvidenceVariable setEditor(List<ContactDetail> theEditor) { 2770 this.editor = theEditor; 2771 return this; 2772 } 2773 2774 public boolean hasEditor() { 2775 if (this.editor == null) 2776 return false; 2777 for (ContactDetail item : this.editor) 2778 if (!item.isEmpty()) 2779 return true; 2780 return false; 2781 } 2782 2783 public ContactDetail addEditor() { // 3 2784 ContactDetail t = new ContactDetail(); 2785 if (this.editor == null) 2786 this.editor = new ArrayList<ContactDetail>(); 2787 this.editor.add(t); 2788 return t; 2789 } 2790 2791 public EvidenceVariable addEditor(ContactDetail t) { // 3 2792 if (t == null) 2793 return this; 2794 if (this.editor == null) 2795 this.editor = new ArrayList<ContactDetail>(); 2796 this.editor.add(t); 2797 return this; 2798 } 2799 2800 /** 2801 * @return The first repetition of repeating field {@link #editor}, creating it 2802 * if it does not already exist 2803 */ 2804 public ContactDetail getEditorFirstRep() { 2805 if (getEditor().isEmpty()) { 2806 addEditor(); 2807 } 2808 return getEditor().get(0); 2809 } 2810 2811 /** 2812 * @return {@link #reviewer} (An individual or organization primarily 2813 * responsible for review of some aspect of the content.) 2814 */ 2815 public List<ContactDetail> getReviewer() { 2816 if (this.reviewer == null) 2817 this.reviewer = new ArrayList<ContactDetail>(); 2818 return this.reviewer; 2819 } 2820 2821 /** 2822 * @return Returns a reference to <code>this</code> for easy method chaining 2823 */ 2824 public EvidenceVariable setReviewer(List<ContactDetail> theReviewer) { 2825 this.reviewer = theReviewer; 2826 return this; 2827 } 2828 2829 public boolean hasReviewer() { 2830 if (this.reviewer == null) 2831 return false; 2832 for (ContactDetail item : this.reviewer) 2833 if (!item.isEmpty()) 2834 return true; 2835 return false; 2836 } 2837 2838 public ContactDetail addReviewer() { // 3 2839 ContactDetail t = new ContactDetail(); 2840 if (this.reviewer == null) 2841 this.reviewer = new ArrayList<ContactDetail>(); 2842 this.reviewer.add(t); 2843 return t; 2844 } 2845 2846 public EvidenceVariable addReviewer(ContactDetail t) { // 3 2847 if (t == null) 2848 return this; 2849 if (this.reviewer == null) 2850 this.reviewer = new ArrayList<ContactDetail>(); 2851 this.reviewer.add(t); 2852 return this; 2853 } 2854 2855 /** 2856 * @return The first repetition of repeating field {@link #reviewer}, creating 2857 * it if it does not already exist 2858 */ 2859 public ContactDetail getReviewerFirstRep() { 2860 if (getReviewer().isEmpty()) { 2861 addReviewer(); 2862 } 2863 return getReviewer().get(0); 2864 } 2865 2866 /** 2867 * @return {@link #endorser} (An individual or organization responsible for 2868 * officially endorsing the content for use in some setting.) 2869 */ 2870 public List<ContactDetail> getEndorser() { 2871 if (this.endorser == null) 2872 this.endorser = new ArrayList<ContactDetail>(); 2873 return this.endorser; 2874 } 2875 2876 /** 2877 * @return Returns a reference to <code>this</code> for easy method chaining 2878 */ 2879 public EvidenceVariable setEndorser(List<ContactDetail> theEndorser) { 2880 this.endorser = theEndorser; 2881 return this; 2882 } 2883 2884 public boolean hasEndorser() { 2885 if (this.endorser == null) 2886 return false; 2887 for (ContactDetail item : this.endorser) 2888 if (!item.isEmpty()) 2889 return true; 2890 return false; 2891 } 2892 2893 public ContactDetail addEndorser() { // 3 2894 ContactDetail t = new ContactDetail(); 2895 if (this.endorser == null) 2896 this.endorser = new ArrayList<ContactDetail>(); 2897 this.endorser.add(t); 2898 return t; 2899 } 2900 2901 public EvidenceVariable addEndorser(ContactDetail t) { // 3 2902 if (t == null) 2903 return this; 2904 if (this.endorser == null) 2905 this.endorser = new ArrayList<ContactDetail>(); 2906 this.endorser.add(t); 2907 return this; 2908 } 2909 2910 /** 2911 * @return The first repetition of repeating field {@link #endorser}, creating 2912 * it if it does not already exist 2913 */ 2914 public ContactDetail getEndorserFirstRep() { 2915 if (getEndorser().isEmpty()) { 2916 addEndorser(); 2917 } 2918 return getEndorser().get(0); 2919 } 2920 2921 /** 2922 * @return {@link #relatedArtifact} (Related artifacts such as additional 2923 * documentation, justification, or bibliographic references.) 2924 */ 2925 public List<RelatedArtifact> getRelatedArtifact() { 2926 if (this.relatedArtifact == null) 2927 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2928 return this.relatedArtifact; 2929 } 2930 2931 /** 2932 * @return Returns a reference to <code>this</code> for easy method chaining 2933 */ 2934 public EvidenceVariable setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2935 this.relatedArtifact = theRelatedArtifact; 2936 return this; 2937 } 2938 2939 public boolean hasRelatedArtifact() { 2940 if (this.relatedArtifact == null) 2941 return false; 2942 for (RelatedArtifact item : this.relatedArtifact) 2943 if (!item.isEmpty()) 2944 return true; 2945 return false; 2946 } 2947 2948 public RelatedArtifact addRelatedArtifact() { // 3 2949 RelatedArtifact t = new RelatedArtifact(); 2950 if (this.relatedArtifact == null) 2951 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2952 this.relatedArtifact.add(t); 2953 return t; 2954 } 2955 2956 public EvidenceVariable addRelatedArtifact(RelatedArtifact t) { // 3 2957 if (t == null) 2958 return this; 2959 if (this.relatedArtifact == null) 2960 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2961 this.relatedArtifact.add(t); 2962 return this; 2963 } 2964 2965 /** 2966 * @return The first repetition of repeating field {@link #relatedArtifact}, 2967 * creating it if it does not already exist 2968 */ 2969 public RelatedArtifact getRelatedArtifactFirstRep() { 2970 if (getRelatedArtifact().isEmpty()) { 2971 addRelatedArtifact(); 2972 } 2973 return getRelatedArtifact().get(0); 2974 } 2975 2976 /** 2977 * @return {@link #type} (The type of evidence element, a population, an 2978 * exposure, or an outcome.). This is the underlying object with id, 2979 * value and extensions. The accessor "getType" gives direct access to 2980 * the value 2981 */ 2982 public Enumeration<EvidenceVariableType> getTypeElement() { 2983 if (this.type == null) 2984 if (Configuration.errorOnAutoCreate()) 2985 throw new Error("Attempt to auto-create EvidenceVariable.type"); 2986 else if (Configuration.doAutoCreate()) 2987 this.type = new Enumeration<EvidenceVariableType>(new EvidenceVariableTypeEnumFactory()); // bb 2988 return this.type; 2989 } 2990 2991 public boolean hasTypeElement() { 2992 return this.type != null && !this.type.isEmpty(); 2993 } 2994 2995 public boolean hasType() { 2996 return this.type != null && !this.type.isEmpty(); 2997 } 2998 2999 /** 3000 * @param value {@link #type} (The type of evidence element, a population, an 3001 * exposure, or an outcome.). This is the underlying object with 3002 * id, value and extensions. The accessor "getType" gives direct 3003 * access to the value 3004 */ 3005 public EvidenceVariable setTypeElement(Enumeration<EvidenceVariableType> value) { 3006 this.type = value; 3007 return this; 3008 } 3009 3010 /** 3011 * @return The type of evidence element, a population, an exposure, or an 3012 * outcome. 3013 */ 3014 public EvidenceVariableType getType() { 3015 return this.type == null ? null : this.type.getValue(); 3016 } 3017 3018 /** 3019 * @param value The type of evidence element, a population, an exposure, or an 3020 * outcome. 3021 */ 3022 public EvidenceVariable setType(EvidenceVariableType value) { 3023 if (value == null) 3024 this.type = null; 3025 else { 3026 if (this.type == null) 3027 this.type = new Enumeration<EvidenceVariableType>(new EvidenceVariableTypeEnumFactory()); 3028 this.type.setValue(value); 3029 } 3030 return this; 3031 } 3032 3033 /** 3034 * @return {@link #characteristic} (A characteristic that defines the members of 3035 * the evidence element. Multiple characteristics are applied with "and" 3036 * semantics.) 3037 */ 3038 public List<EvidenceVariableCharacteristicComponent> getCharacteristic() { 3039 if (this.characteristic == null) 3040 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3041 return this.characteristic; 3042 } 3043 3044 /** 3045 * @return Returns a reference to <code>this</code> for easy method chaining 3046 */ 3047 public EvidenceVariable setCharacteristic(List<EvidenceVariableCharacteristicComponent> theCharacteristic) { 3048 this.characteristic = theCharacteristic; 3049 return this; 3050 } 3051 3052 public boolean hasCharacteristic() { 3053 if (this.characteristic == null) 3054 return false; 3055 for (EvidenceVariableCharacteristicComponent item : this.characteristic) 3056 if (!item.isEmpty()) 3057 return true; 3058 return false; 3059 } 3060 3061 public EvidenceVariableCharacteristicComponent addCharacteristic() { // 3 3062 EvidenceVariableCharacteristicComponent t = new EvidenceVariableCharacteristicComponent(); 3063 if (this.characteristic == null) 3064 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3065 this.characteristic.add(t); 3066 return t; 3067 } 3068 3069 public EvidenceVariable addCharacteristic(EvidenceVariableCharacteristicComponent t) { // 3 3070 if (t == null) 3071 return this; 3072 if (this.characteristic == null) 3073 this.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3074 this.characteristic.add(t); 3075 return this; 3076 } 3077 3078 /** 3079 * @return The first repetition of repeating field {@link #characteristic}, 3080 * creating it if it does not already exist 3081 */ 3082 public EvidenceVariableCharacteristicComponent getCharacteristicFirstRep() { 3083 if (getCharacteristic().isEmpty()) { 3084 addCharacteristic(); 3085 } 3086 return getCharacteristic().get(0); 3087 } 3088 3089 protected void listChildren(List<Property> children) { 3090 super.listChildren(children); 3091 children.add(new Property("url", "uri", 3092 "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 3093 0, 1, url)); 3094 children.add(new Property("identifier", "Identifier", 3095 "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3096 0, java.lang.Integer.MAX_VALUE, identifier)); 3097 children.add(new Property("version", "string", 3098 "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 3099 0, 1, version)); 3100 children.add(new Property("name", "string", 3101 "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3102 0, 1, name)); 3103 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the evidence variable.", 3104 0, 1, title)); 3105 children.add(new Property("shortTitle", "string", 3106 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 3107 0, 1, shortTitle)); 3108 children.add(new Property("subtitle", "string", 3109 "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.", 3110 0, 1, subtitle)); 3111 children.add(new Property("status", "code", 3112 "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status)); 3113 children.add(new Property("date", "dateTime", 3114 "The date (and optionally time) when the evidence variable was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 3115 0, 1, date)); 3116 children.add(new Property("publisher", "string", 3117 "The name of the organization or individual that published the evidence variable.", 0, 1, publisher)); 3118 children.add(new Property("contact", "ContactDetail", 3119 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3120 java.lang.Integer.MAX_VALUE, contact)); 3121 children.add(new Property("description", "markdown", 3122 "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, 3123 description)); 3124 children.add( 3125 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 3126 0, java.lang.Integer.MAX_VALUE, note)); 3127 children.add(new Property("useContext", "UsageContext", 3128 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 3129 0, java.lang.Integer.MAX_VALUE, useContext)); 3130 children.add(new Property("jurisdiction", "CodeableConcept", 3131 "A legal or geographic region in which the evidence variable is intended to be used.", 0, 3132 java.lang.Integer.MAX_VALUE, jurisdiction)); 3133 children.add(new Property("copyright", "markdown", 3134 "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.", 3135 0, 1, copyright)); 3136 children.add(new Property("approvalDate", "date", 3137 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3138 0, 1, approvalDate)); 3139 children.add(new Property("lastReviewDate", "date", 3140 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3141 0, 1, lastReviewDate)); 3142 children.add(new Property("effectivePeriod", "Period", 3143 "The period during which the evidence variable content was or is planned to be in active use.", 0, 1, 3144 effectivePeriod)); 3145 children.add(new Property("topic", "CodeableConcept", 3146 "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.", 3147 0, java.lang.Integer.MAX_VALUE, topic)); 3148 children.add(new Property("author", "ContactDetail", 3149 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 3150 java.lang.Integer.MAX_VALUE, author)); 3151 children.add(new Property("editor", "ContactDetail", 3152 "An individual or organization primarily responsible for internal coherence of the content.", 0, 3153 java.lang.Integer.MAX_VALUE, editor)); 3154 children.add(new Property("reviewer", "ContactDetail", 3155 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 3156 java.lang.Integer.MAX_VALUE, reviewer)); 3157 children.add(new Property("endorser", "ContactDetail", 3158 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 3159 java.lang.Integer.MAX_VALUE, endorser)); 3160 children.add(new Property("relatedArtifact", "RelatedArtifact", 3161 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 3162 java.lang.Integer.MAX_VALUE, relatedArtifact)); 3163 children.add(new Property("type", "code", "The type of evidence element, a population, an exposure, or an outcome.", 3164 0, 1, type)); 3165 children.add(new Property("characteristic", "", 3166 "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.", 3167 0, java.lang.Integer.MAX_VALUE, characteristic)); 3168 } 3169 3170 @Override 3171 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3172 switch (_hash) { 3173 case 116079: 3174 /* url */ return new Property("url", "uri", 3175 "An absolute URI that is used to identify this evidence variable when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence variable is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence variable is stored on different servers.", 3176 0, 1, url); 3177 case -1618432855: 3178 /* identifier */ return new Property("identifier", "Identifier", 3179 "A formal identifier that is used to identify this evidence variable when it is represented in other formats, or referenced in a specification, model, design or an instance.", 3180 0, java.lang.Integer.MAX_VALUE, identifier); 3181 case 351608024: 3182 /* version */ return new Property("version", "string", 3183 "The identifier that is used to identify this version of the evidence variable when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence variable author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 3184 0, 1, version); 3185 case 3373707: 3186 /* name */ return new Property("name", "string", 3187 "A natural language name identifying the evidence variable. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 3188 0, 1, name); 3189 case 110371416: 3190 /* title */ return new Property("title", "string", 3191 "A short, descriptive, user-friendly title for the evidence variable.", 0, 1, title); 3192 case 1555503932: 3193 /* shortTitle */ return new Property("shortTitle", "string", 3194 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 3195 0, 1, shortTitle); 3196 case -2060497896: 3197 /* subtitle */ return new Property("subtitle", "string", 3198 "An explanatory or alternate title for the EvidenceVariable giving additional information about its content.", 3199 0, 1, subtitle); 3200 case -892481550: 3201 /* status */ return new Property("status", "code", 3202 "The status of this evidence variable. Enables tracking the life-cycle of the content.", 0, 1, status); 3203 case 3076014: 3204 /* date */ return new Property("date", "dateTime", 3205 "The date (and optionally time) when the evidence variable was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence variable changes.", 3206 0, 1, date); 3207 case 1447404028: 3208 /* publisher */ return new Property("publisher", "string", 3209 "The name of the organization or individual that published the evidence variable.", 0, 1, publisher); 3210 case 951526432: 3211 /* contact */ return new Property("contact", "ContactDetail", 3212 "Contact details to assist a user in finding and communicating with the publisher.", 0, 3213 java.lang.Integer.MAX_VALUE, contact); 3214 case -1724546052: 3215 /* description */ return new Property("description", "markdown", 3216 "A free text natural language description of the evidence variable from a consumer's perspective.", 0, 1, 3217 description); 3218 case 3387378: 3219 /* note */ return new Property("note", "Annotation", 3220 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 3221 note); 3222 case -669707736: 3223 /* useContext */ return new Property("useContext", "UsageContext", 3224 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence variable instances.", 3225 0, java.lang.Integer.MAX_VALUE, useContext); 3226 case -507075711: 3227 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 3228 "A legal or geographic region in which the evidence variable is intended to be used.", 0, 3229 java.lang.Integer.MAX_VALUE, jurisdiction); 3230 case 1522889671: 3231 /* copyright */ return new Property("copyright", "markdown", 3232 "A copyright statement relating to the evidence variable and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence variable.", 3233 0, 1, copyright); 3234 case 223539345: 3235 /* approvalDate */ return new Property("approvalDate", "date", 3236 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 3237 0, 1, approvalDate); 3238 case -1687512484: 3239 /* lastReviewDate */ return new Property("lastReviewDate", "date", 3240 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 3241 0, 1, lastReviewDate); 3242 case -403934648: 3243 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 3244 "The period during which the evidence variable content was or is planned to be in active use.", 0, 1, 3245 effectivePeriod); 3246 case 110546223: 3247 /* topic */ return new Property("topic", "CodeableConcept", 3248 "Descriptive topics related to the content of the EvidenceVariable. Topics provide a high-level categorization grouping types of EvidenceVariables that can be useful for filtering and searching.", 3249 0, java.lang.Integer.MAX_VALUE, topic); 3250 case -1406328437: 3251 /* author */ return new Property("author", "ContactDetail", 3252 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 3253 java.lang.Integer.MAX_VALUE, author); 3254 case -1307827859: 3255 /* editor */ return new Property("editor", "ContactDetail", 3256 "An individual or organization primarily responsible for internal coherence of the content.", 0, 3257 java.lang.Integer.MAX_VALUE, editor); 3258 case -261190139: 3259 /* reviewer */ return new Property("reviewer", "ContactDetail", 3260 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 3261 java.lang.Integer.MAX_VALUE, reviewer); 3262 case 1740277666: 3263 /* endorser */ return new Property("endorser", "ContactDetail", 3264 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 3265 java.lang.Integer.MAX_VALUE, endorser); 3266 case 666807069: 3267 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 3268 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 3269 java.lang.Integer.MAX_VALUE, relatedArtifact); 3270 case 3575610: 3271 /* type */ return new Property("type", "code", 3272 "The type of evidence element, a population, an exposure, or an outcome.", 0, 1, type); 3273 case 366313883: 3274 /* characteristic */ return new Property("characteristic", "", 3275 "A characteristic that defines the members of the evidence element. Multiple characteristics are applied with \"and\" semantics.", 3276 0, java.lang.Integer.MAX_VALUE, characteristic); 3277 default: 3278 return super.getNamedProperty(_hash, _name, _checkValid); 3279 } 3280 3281 } 3282 3283 @Override 3284 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3285 switch (hash) { 3286 case 116079: 3287 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 3288 case -1618432855: 3289 /* identifier */ return this.identifier == null ? new Base[0] 3290 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3291 case 351608024: 3292 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 3293 case 3373707: 3294 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3295 case 110371416: 3296 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 3297 case 1555503932: 3298 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 3299 case -2060497896: 3300 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 3301 case -892481550: 3302 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 3303 case 3076014: 3304 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 3305 case 1447404028: 3306 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 3307 case 951526432: 3308 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3309 case -1724546052: 3310 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3311 case 3387378: 3312 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3313 case -669707736: 3314 /* useContext */ return this.useContext == null ? new Base[0] 3315 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3316 case -507075711: 3317 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 3318 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3319 case 1522889671: 3320 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 3321 case 223539345: 3322 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 3323 case -1687512484: 3324 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 3325 case -403934648: 3326 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 3327 case 110546223: 3328 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 3329 case -1406328437: 3330 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 3331 case -1307827859: 3332 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 3333 case -261190139: 3334 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 3335 case 1740277666: 3336 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 3337 case 666807069: 3338 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 3339 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 3340 case 3575610: 3341 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<EvidenceVariableType> 3342 case 366313883: 3343 /* characteristic */ return this.characteristic == null ? new Base[0] 3344 : this.characteristic.toArray(new Base[this.characteristic.size()]); // EvidenceVariableCharacteristicComponent 3345 default: 3346 return super.getProperty(hash, name, checkValid); 3347 } 3348 3349 } 3350 3351 @Override 3352 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3353 switch (hash) { 3354 case 116079: // url 3355 this.url = castToUri(value); // UriType 3356 return value; 3357 case -1618432855: // identifier 3358 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3359 return value; 3360 case 351608024: // version 3361 this.version = castToString(value); // StringType 3362 return value; 3363 case 3373707: // name 3364 this.name = castToString(value); // StringType 3365 return value; 3366 case 110371416: // title 3367 this.title = castToString(value); // StringType 3368 return value; 3369 case 1555503932: // shortTitle 3370 this.shortTitle = castToString(value); // StringType 3371 return value; 3372 case -2060497896: // subtitle 3373 this.subtitle = castToString(value); // StringType 3374 return value; 3375 case -892481550: // status 3376 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3377 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3378 return value; 3379 case 3076014: // date 3380 this.date = castToDateTime(value); // DateTimeType 3381 return value; 3382 case 1447404028: // publisher 3383 this.publisher = castToString(value); // StringType 3384 return value; 3385 case 951526432: // contact 3386 this.getContact().add(castToContactDetail(value)); // ContactDetail 3387 return value; 3388 case -1724546052: // description 3389 this.description = castToMarkdown(value); // MarkdownType 3390 return value; 3391 case 3387378: // note 3392 this.getNote().add(castToAnnotation(value)); // Annotation 3393 return value; 3394 case -669707736: // useContext 3395 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3396 return value; 3397 case -507075711: // jurisdiction 3398 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3399 return value; 3400 case 1522889671: // copyright 3401 this.copyright = castToMarkdown(value); // MarkdownType 3402 return value; 3403 case 223539345: // approvalDate 3404 this.approvalDate = castToDate(value); // DateType 3405 return value; 3406 case -1687512484: // lastReviewDate 3407 this.lastReviewDate = castToDate(value); // DateType 3408 return value; 3409 case -403934648: // effectivePeriod 3410 this.effectivePeriod = castToPeriod(value); // Period 3411 return value; 3412 case 110546223: // topic 3413 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 3414 return value; 3415 case -1406328437: // author 3416 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 3417 return value; 3418 case -1307827859: // editor 3419 this.getEditor().add(castToContactDetail(value)); // ContactDetail 3420 return value; 3421 case -261190139: // reviewer 3422 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 3423 return value; 3424 case 1740277666: // endorser 3425 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 3426 return value; 3427 case 666807069: // relatedArtifact 3428 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 3429 return value; 3430 case 3575610: // type 3431 value = new EvidenceVariableTypeEnumFactory().fromType(castToCode(value)); 3432 this.type = (Enumeration) value; // Enumeration<EvidenceVariableType> 3433 return value; 3434 case 366313883: // characteristic 3435 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); // EvidenceVariableCharacteristicComponent 3436 return value; 3437 default: 3438 return super.setProperty(hash, name, value); 3439 } 3440 3441 } 3442 3443 @Override 3444 public Base setProperty(String name, Base value) throws FHIRException { 3445 if (name.equals("url")) { 3446 this.url = castToUri(value); // UriType 3447 } else if (name.equals("identifier")) { 3448 this.getIdentifier().add(castToIdentifier(value)); 3449 } else if (name.equals("version")) { 3450 this.version = castToString(value); // StringType 3451 } else if (name.equals("name")) { 3452 this.name = castToString(value); // StringType 3453 } else if (name.equals("title")) { 3454 this.title = castToString(value); // StringType 3455 } else if (name.equals("shortTitle")) { 3456 this.shortTitle = castToString(value); // StringType 3457 } else if (name.equals("subtitle")) { 3458 this.subtitle = castToString(value); // StringType 3459 } else if (name.equals("status")) { 3460 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3461 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3462 } else if (name.equals("date")) { 3463 this.date = castToDateTime(value); // DateTimeType 3464 } else if (name.equals("publisher")) { 3465 this.publisher = castToString(value); // StringType 3466 } else if (name.equals("contact")) { 3467 this.getContact().add(castToContactDetail(value)); 3468 } else if (name.equals("description")) { 3469 this.description = castToMarkdown(value); // MarkdownType 3470 } else if (name.equals("note")) { 3471 this.getNote().add(castToAnnotation(value)); 3472 } else if (name.equals("useContext")) { 3473 this.getUseContext().add(castToUsageContext(value)); 3474 } else if (name.equals("jurisdiction")) { 3475 this.getJurisdiction().add(castToCodeableConcept(value)); 3476 } else if (name.equals("copyright")) { 3477 this.copyright = castToMarkdown(value); // MarkdownType 3478 } else if (name.equals("approvalDate")) { 3479 this.approvalDate = castToDate(value); // DateType 3480 } else if (name.equals("lastReviewDate")) { 3481 this.lastReviewDate = castToDate(value); // DateType 3482 } else if (name.equals("effectivePeriod")) { 3483 this.effectivePeriod = castToPeriod(value); // Period 3484 } else if (name.equals("topic")) { 3485 this.getTopic().add(castToCodeableConcept(value)); 3486 } else if (name.equals("author")) { 3487 this.getAuthor().add(castToContactDetail(value)); 3488 } else if (name.equals("editor")) { 3489 this.getEditor().add(castToContactDetail(value)); 3490 } else if (name.equals("reviewer")) { 3491 this.getReviewer().add(castToContactDetail(value)); 3492 } else if (name.equals("endorser")) { 3493 this.getEndorser().add(castToContactDetail(value)); 3494 } else if (name.equals("relatedArtifact")) { 3495 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 3496 } else if (name.equals("type")) { 3497 value = new EvidenceVariableTypeEnumFactory().fromType(castToCode(value)); 3498 this.type = (Enumeration) value; // Enumeration<EvidenceVariableType> 3499 } else if (name.equals("characteristic")) { 3500 this.getCharacteristic().add((EvidenceVariableCharacteristicComponent) value); 3501 } else 3502 return super.setProperty(name, value); 3503 return value; 3504 } 3505 3506 @Override 3507 public void removeChild(String name, Base value) throws FHIRException { 3508 if (name.equals("url")) { 3509 this.url = null; 3510 } else if (name.equals("identifier")) { 3511 this.getIdentifier().remove(castToIdentifier(value)); 3512 } else if (name.equals("version")) { 3513 this.version = null; 3514 } else if (name.equals("name")) { 3515 this.name = null; 3516 } else if (name.equals("title")) { 3517 this.title = null; 3518 } else if (name.equals("shortTitle")) { 3519 this.shortTitle = null; 3520 } else if (name.equals("subtitle")) { 3521 this.subtitle = null; 3522 } else if (name.equals("status")) { 3523 this.status = null; 3524 } else if (name.equals("date")) { 3525 this.date = null; 3526 } else if (name.equals("publisher")) { 3527 this.publisher = null; 3528 } else if (name.equals("contact")) { 3529 this.getContact().remove(castToContactDetail(value)); 3530 } else if (name.equals("description")) { 3531 this.description = null; 3532 } else if (name.equals("note")) { 3533 this.getNote().remove(castToAnnotation(value)); 3534 } else if (name.equals("useContext")) { 3535 this.getUseContext().remove(castToUsageContext(value)); 3536 } else if (name.equals("jurisdiction")) { 3537 this.getJurisdiction().remove(castToCodeableConcept(value)); 3538 } else if (name.equals("copyright")) { 3539 this.copyright = null; 3540 } else if (name.equals("approvalDate")) { 3541 this.approvalDate = null; 3542 } else if (name.equals("lastReviewDate")) { 3543 this.lastReviewDate = null; 3544 } else if (name.equals("effectivePeriod")) { 3545 this.effectivePeriod = null; 3546 } else if (name.equals("topic")) { 3547 this.getTopic().remove(castToCodeableConcept(value)); 3548 } else if (name.equals("author")) { 3549 this.getAuthor().remove(castToContactDetail(value)); 3550 } else if (name.equals("editor")) { 3551 this.getEditor().remove(castToContactDetail(value)); 3552 } else if (name.equals("reviewer")) { 3553 this.getReviewer().remove(castToContactDetail(value)); 3554 } else if (name.equals("endorser")) { 3555 this.getEndorser().remove(castToContactDetail(value)); 3556 } else if (name.equals("relatedArtifact")) { 3557 this.getRelatedArtifact().remove(castToRelatedArtifact(value)); 3558 } else if (name.equals("type")) { 3559 this.type = null; 3560 } else if (name.equals("characteristic")) { 3561 this.getCharacteristic().remove((EvidenceVariableCharacteristicComponent) value); 3562 } else 3563 super.removeChild(name, value); 3564 3565 } 3566 3567 @Override 3568 public Base makeProperty(int hash, String name) throws FHIRException { 3569 switch (hash) { 3570 case 116079: 3571 return getUrlElement(); 3572 case -1618432855: 3573 return addIdentifier(); 3574 case 351608024: 3575 return getVersionElement(); 3576 case 3373707: 3577 return getNameElement(); 3578 case 110371416: 3579 return getTitleElement(); 3580 case 1555503932: 3581 return getShortTitleElement(); 3582 case -2060497896: 3583 return getSubtitleElement(); 3584 case -892481550: 3585 return getStatusElement(); 3586 case 3076014: 3587 return getDateElement(); 3588 case 1447404028: 3589 return getPublisherElement(); 3590 case 951526432: 3591 return addContact(); 3592 case -1724546052: 3593 return getDescriptionElement(); 3594 case 3387378: 3595 return addNote(); 3596 case -669707736: 3597 return addUseContext(); 3598 case -507075711: 3599 return addJurisdiction(); 3600 case 1522889671: 3601 return getCopyrightElement(); 3602 case 223539345: 3603 return getApprovalDateElement(); 3604 case -1687512484: 3605 return getLastReviewDateElement(); 3606 case -403934648: 3607 return getEffectivePeriod(); 3608 case 110546223: 3609 return addTopic(); 3610 case -1406328437: 3611 return addAuthor(); 3612 case -1307827859: 3613 return addEditor(); 3614 case -261190139: 3615 return addReviewer(); 3616 case 1740277666: 3617 return addEndorser(); 3618 case 666807069: 3619 return addRelatedArtifact(); 3620 case 3575610: 3621 return getTypeElement(); 3622 case 366313883: 3623 return addCharacteristic(); 3624 default: 3625 return super.makeProperty(hash, name); 3626 } 3627 3628 } 3629 3630 @Override 3631 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3632 switch (hash) { 3633 case 116079: 3634 /* url */ return new String[] { "uri" }; 3635 case -1618432855: 3636 /* identifier */ return new String[] { "Identifier" }; 3637 case 351608024: 3638 /* version */ return new String[] { "string" }; 3639 case 3373707: 3640 /* name */ return new String[] { "string" }; 3641 case 110371416: 3642 /* title */ return new String[] { "string" }; 3643 case 1555503932: 3644 /* shortTitle */ return new String[] { "string" }; 3645 case -2060497896: 3646 /* subtitle */ return new String[] { "string" }; 3647 case -892481550: 3648 /* status */ return new String[] { "code" }; 3649 case 3076014: 3650 /* date */ return new String[] { "dateTime" }; 3651 case 1447404028: 3652 /* publisher */ return new String[] { "string" }; 3653 case 951526432: 3654 /* contact */ return new String[] { "ContactDetail" }; 3655 case -1724546052: 3656 /* description */ return new String[] { "markdown" }; 3657 case 3387378: 3658 /* note */ return new String[] { "Annotation" }; 3659 case -669707736: 3660 /* useContext */ return new String[] { "UsageContext" }; 3661 case -507075711: 3662 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3663 case 1522889671: 3664 /* copyright */ return new String[] { "markdown" }; 3665 case 223539345: 3666 /* approvalDate */ return new String[] { "date" }; 3667 case -1687512484: 3668 /* lastReviewDate */ return new String[] { "date" }; 3669 case -403934648: 3670 /* effectivePeriod */ return new String[] { "Period" }; 3671 case 110546223: 3672 /* topic */ return new String[] { "CodeableConcept" }; 3673 case -1406328437: 3674 /* author */ return new String[] { "ContactDetail" }; 3675 case -1307827859: 3676 /* editor */ return new String[] { "ContactDetail" }; 3677 case -261190139: 3678 /* reviewer */ return new String[] { "ContactDetail" }; 3679 case 1740277666: 3680 /* endorser */ return new String[] { "ContactDetail" }; 3681 case 666807069: 3682 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 3683 case 3575610: 3684 /* type */ return new String[] { "code" }; 3685 case 366313883: 3686 /* characteristic */ return new String[] {}; 3687 default: 3688 return super.getTypesForProperty(hash, name); 3689 } 3690 3691 } 3692 3693 @Override 3694 public Base addChild(String name) throws FHIRException { 3695 if (name.equals("url")) { 3696 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.url"); 3697 } else if (name.equals("identifier")) { 3698 return addIdentifier(); 3699 } else if (name.equals("version")) { 3700 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.version"); 3701 } else if (name.equals("name")) { 3702 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.name"); 3703 } else if (name.equals("title")) { 3704 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.title"); 3705 } else if (name.equals("shortTitle")) { 3706 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.shortTitle"); 3707 } else if (name.equals("subtitle")) { 3708 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.subtitle"); 3709 } else if (name.equals("status")) { 3710 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.status"); 3711 } else if (name.equals("date")) { 3712 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.date"); 3713 } else if (name.equals("publisher")) { 3714 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.publisher"); 3715 } else if (name.equals("contact")) { 3716 return addContact(); 3717 } else if (name.equals("description")) { 3718 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.description"); 3719 } else if (name.equals("note")) { 3720 return addNote(); 3721 } else if (name.equals("useContext")) { 3722 return addUseContext(); 3723 } else if (name.equals("jurisdiction")) { 3724 return addJurisdiction(); 3725 } else if (name.equals("copyright")) { 3726 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.copyright"); 3727 } else if (name.equals("approvalDate")) { 3728 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.approvalDate"); 3729 } else if (name.equals("lastReviewDate")) { 3730 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.lastReviewDate"); 3731 } else if (name.equals("effectivePeriod")) { 3732 this.effectivePeriod = new Period(); 3733 return this.effectivePeriod; 3734 } else if (name.equals("topic")) { 3735 return addTopic(); 3736 } else if (name.equals("author")) { 3737 return addAuthor(); 3738 } else if (name.equals("editor")) { 3739 return addEditor(); 3740 } else if (name.equals("reviewer")) { 3741 return addReviewer(); 3742 } else if (name.equals("endorser")) { 3743 return addEndorser(); 3744 } else if (name.equals("relatedArtifact")) { 3745 return addRelatedArtifact(); 3746 } else if (name.equals("type")) { 3747 throw new FHIRException("Cannot call addChild on a singleton property EvidenceVariable.type"); 3748 } else if (name.equals("characteristic")) { 3749 return addCharacteristic(); 3750 } else 3751 return super.addChild(name); 3752 } 3753 3754 public String fhirType() { 3755 return "EvidenceVariable"; 3756 3757 } 3758 3759 public EvidenceVariable copy() { 3760 EvidenceVariable dst = new EvidenceVariable(); 3761 copyValues(dst); 3762 return dst; 3763 } 3764 3765 public void copyValues(EvidenceVariable dst) { 3766 super.copyValues(dst); 3767 dst.url = url == null ? null : url.copy(); 3768 if (identifier != null) { 3769 dst.identifier = new ArrayList<Identifier>(); 3770 for (Identifier i : identifier) 3771 dst.identifier.add(i.copy()); 3772 } 3773 ; 3774 dst.version = version == null ? null : version.copy(); 3775 dst.name = name == null ? null : name.copy(); 3776 dst.title = title == null ? null : title.copy(); 3777 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 3778 dst.subtitle = subtitle == null ? null : subtitle.copy(); 3779 dst.status = status == null ? null : status.copy(); 3780 dst.date = date == null ? null : date.copy(); 3781 dst.publisher = publisher == null ? null : publisher.copy(); 3782 if (contact != null) { 3783 dst.contact = new ArrayList<ContactDetail>(); 3784 for (ContactDetail i : contact) 3785 dst.contact.add(i.copy()); 3786 } 3787 ; 3788 dst.description = description == null ? null : description.copy(); 3789 if (note != null) { 3790 dst.note = new ArrayList<Annotation>(); 3791 for (Annotation i : note) 3792 dst.note.add(i.copy()); 3793 } 3794 ; 3795 if (useContext != null) { 3796 dst.useContext = new ArrayList<UsageContext>(); 3797 for (UsageContext i : useContext) 3798 dst.useContext.add(i.copy()); 3799 } 3800 ; 3801 if (jurisdiction != null) { 3802 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3803 for (CodeableConcept i : jurisdiction) 3804 dst.jurisdiction.add(i.copy()); 3805 } 3806 ; 3807 dst.copyright = copyright == null ? null : copyright.copy(); 3808 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 3809 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 3810 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 3811 if (topic != null) { 3812 dst.topic = new ArrayList<CodeableConcept>(); 3813 for (CodeableConcept i : topic) 3814 dst.topic.add(i.copy()); 3815 } 3816 ; 3817 if (author != null) { 3818 dst.author = new ArrayList<ContactDetail>(); 3819 for (ContactDetail i : author) 3820 dst.author.add(i.copy()); 3821 } 3822 ; 3823 if (editor != null) { 3824 dst.editor = new ArrayList<ContactDetail>(); 3825 for (ContactDetail i : editor) 3826 dst.editor.add(i.copy()); 3827 } 3828 ; 3829 if (reviewer != null) { 3830 dst.reviewer = new ArrayList<ContactDetail>(); 3831 for (ContactDetail i : reviewer) 3832 dst.reviewer.add(i.copy()); 3833 } 3834 ; 3835 if (endorser != null) { 3836 dst.endorser = new ArrayList<ContactDetail>(); 3837 for (ContactDetail i : endorser) 3838 dst.endorser.add(i.copy()); 3839 } 3840 ; 3841 if (relatedArtifact != null) { 3842 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 3843 for (RelatedArtifact i : relatedArtifact) 3844 dst.relatedArtifact.add(i.copy()); 3845 } 3846 ; 3847 dst.type = type == null ? null : type.copy(); 3848 if (characteristic != null) { 3849 dst.characteristic = new ArrayList<EvidenceVariableCharacteristicComponent>(); 3850 for (EvidenceVariableCharacteristicComponent i : characteristic) 3851 dst.characteristic.add(i.copy()); 3852 } 3853 ; 3854 } 3855 3856 protected EvidenceVariable typedCopy() { 3857 return copy(); 3858 } 3859 3860 @Override 3861 public boolean equalsDeep(Base other_) { 3862 if (!super.equalsDeep(other_)) 3863 return false; 3864 if (!(other_ instanceof EvidenceVariable)) 3865 return false; 3866 EvidenceVariable o = (EvidenceVariable) other_; 3867 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 3868 && compareDeep(subtitle, o.subtitle, true) && compareDeep(note, o.note, true) 3869 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 3870 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 3871 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 3872 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 3873 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 3874 && compareDeep(type, o.type, true) && compareDeep(characteristic, o.characteristic, true); 3875 } 3876 3877 @Override 3878 public boolean equalsShallow(Base other_) { 3879 if (!super.equalsShallow(other_)) 3880 return false; 3881 if (!(other_ instanceof EvidenceVariable)) 3882 return false; 3883 EvidenceVariable o = (EvidenceVariable) other_; 3884 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 3885 && compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 3886 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(type, o.type, true); 3887 } 3888 3889 public boolean isEmpty() { 3890 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, note, copyright, 3891 approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, type, 3892 characteristic); 3893 } 3894 3895 @Override 3896 public ResourceType getResourceType() { 3897 return ResourceType.EvidenceVariable; 3898 } 3899 3900 /** 3901 * Search parameter: <b>date</b> 3902 * <p> 3903 * Description: <b>The evidence variable publication date</b><br> 3904 * Type: <b>date</b><br> 3905 * Path: <b>EvidenceVariable.date</b><br> 3906 * </p> 3907 */ 3908 @SearchParamDefinition(name = "date", path = "EvidenceVariable.date", description = "The evidence variable publication date", type = "date") 3909 public static final String SP_DATE = "date"; 3910 /** 3911 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3912 * <p> 3913 * Description: <b>The evidence variable publication date</b><br> 3914 * Type: <b>date</b><br> 3915 * Path: <b>EvidenceVariable.date</b><br> 3916 * </p> 3917 */ 3918 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3919 SP_DATE); 3920 3921 /** 3922 * Search parameter: <b>identifier</b> 3923 * <p> 3924 * Description: <b>External identifier for the evidence variable</b><br> 3925 * Type: <b>token</b><br> 3926 * Path: <b>EvidenceVariable.identifier</b><br> 3927 * </p> 3928 */ 3929 @SearchParamDefinition(name = "identifier", path = "EvidenceVariable.identifier", description = "External identifier for the evidence variable", type = "token") 3930 public static final String SP_IDENTIFIER = "identifier"; 3931 /** 3932 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3933 * <p> 3934 * Description: <b>External identifier for the evidence variable</b><br> 3935 * Type: <b>token</b><br> 3936 * Path: <b>EvidenceVariable.identifier</b><br> 3937 * </p> 3938 */ 3939 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3940 SP_IDENTIFIER); 3941 3942 /** 3943 * Search parameter: <b>successor</b> 3944 * <p> 3945 * Description: <b>What resource is being referenced</b><br> 3946 * Type: <b>reference</b><br> 3947 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 3948 * </p> 3949 */ 3950 @SearchParamDefinition(name = "successor", path = "EvidenceVariable.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 3951 public static final String SP_SUCCESSOR = "successor"; 3952 /** 3953 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 3954 * <p> 3955 * Description: <b>What resource is being referenced</b><br> 3956 * Type: <b>reference</b><br> 3957 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 3958 * </p> 3959 */ 3960 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3961 SP_SUCCESSOR); 3962 3963 /** 3964 * Constant for fluent queries to be used to add include statements. Specifies 3965 * the path value of "<b>EvidenceVariable:successor</b>". 3966 */ 3967 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 3968 "EvidenceVariable:successor").toLocked(); 3969 3970 /** 3971 * Search parameter: <b>context-type-value</b> 3972 * <p> 3973 * Description: <b>A use context type and value assigned to the evidence 3974 * variable</b><br> 3975 * Type: <b>composite</b><br> 3976 * Path: <b></b><br> 3977 * </p> 3978 */ 3979 @SearchParamDefinition(name = "context-type-value", path = "EvidenceVariable.useContext", description = "A use context type and value assigned to the evidence variable", type = "composite", compositeOf = { 3980 "context-type", "context" }) 3981 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3982 /** 3983 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3984 * <p> 3985 * Description: <b>A use context type and value assigned to the evidence 3986 * variable</b><br> 3987 * Type: <b>composite</b><br> 3988 * Path: <b></b><br> 3989 * </p> 3990 */ 3991 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3992 SP_CONTEXT_TYPE_VALUE); 3993 3994 /** 3995 * Search parameter: <b>jurisdiction</b> 3996 * <p> 3997 * Description: <b>Intended jurisdiction for the evidence variable</b><br> 3998 * Type: <b>token</b><br> 3999 * Path: <b>EvidenceVariable.jurisdiction</b><br> 4000 * </p> 4001 */ 4002 @SearchParamDefinition(name = "jurisdiction", path = "EvidenceVariable.jurisdiction", description = "Intended jurisdiction for the evidence variable", type = "token") 4003 public static final String SP_JURISDICTION = "jurisdiction"; 4004 /** 4005 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4006 * <p> 4007 * Description: <b>Intended jurisdiction for the evidence variable</b><br> 4008 * Type: <b>token</b><br> 4009 * Path: <b>EvidenceVariable.jurisdiction</b><br> 4010 * </p> 4011 */ 4012 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4013 SP_JURISDICTION); 4014 4015 /** 4016 * Search parameter: <b>description</b> 4017 * <p> 4018 * Description: <b>The description of the evidence variable</b><br> 4019 * Type: <b>string</b><br> 4020 * Path: <b>EvidenceVariable.description</b><br> 4021 * </p> 4022 */ 4023 @SearchParamDefinition(name = "description", path = "EvidenceVariable.description", description = "The description of the evidence variable", type = "string") 4024 public static final String SP_DESCRIPTION = "description"; 4025 /** 4026 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4027 * <p> 4028 * Description: <b>The description of the evidence variable</b><br> 4029 * Type: <b>string</b><br> 4030 * Path: <b>EvidenceVariable.description</b><br> 4031 * </p> 4032 */ 4033 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 4034 SP_DESCRIPTION); 4035 4036 /** 4037 * Search parameter: <b>derived-from</b> 4038 * <p> 4039 * Description: <b>What resource is being referenced</b><br> 4040 * Type: <b>reference</b><br> 4041 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4042 * </p> 4043 */ 4044 @SearchParamDefinition(name = "derived-from", path = "EvidenceVariable.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 4045 public static final String SP_DERIVED_FROM = "derived-from"; 4046 /** 4047 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4048 * <p> 4049 * Description: <b>What resource is being referenced</b><br> 4050 * Type: <b>reference</b><br> 4051 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4052 * </p> 4053 */ 4054 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4055 SP_DERIVED_FROM); 4056 4057 /** 4058 * Constant for fluent queries to be used to add include statements. Specifies 4059 * the path value of "<b>EvidenceVariable:derived-from</b>". 4060 */ 4061 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 4062 "EvidenceVariable:derived-from").toLocked(); 4063 4064 /** 4065 * Search parameter: <b>context-type</b> 4066 * <p> 4067 * Description: <b>A type of use context assigned to the evidence 4068 * variable</b><br> 4069 * Type: <b>token</b><br> 4070 * Path: <b>EvidenceVariable.useContext.code</b><br> 4071 * </p> 4072 */ 4073 @SearchParamDefinition(name = "context-type", path = "EvidenceVariable.useContext.code", description = "A type of use context assigned to the evidence variable", type = "token") 4074 public static final String SP_CONTEXT_TYPE = "context-type"; 4075 /** 4076 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 4077 * <p> 4078 * Description: <b>A type of use context assigned to the evidence 4079 * variable</b><br> 4080 * Type: <b>token</b><br> 4081 * Path: <b>EvidenceVariable.useContext.code</b><br> 4082 * </p> 4083 */ 4084 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4085 SP_CONTEXT_TYPE); 4086 4087 /** 4088 * Search parameter: <b>predecessor</b> 4089 * <p> 4090 * Description: <b>What resource is being referenced</b><br> 4091 * Type: <b>reference</b><br> 4092 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4093 * </p> 4094 */ 4095 @SearchParamDefinition(name = "predecessor", path = "EvidenceVariable.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 4096 public static final String SP_PREDECESSOR = "predecessor"; 4097 /** 4098 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4099 * <p> 4100 * Description: <b>What resource is being referenced</b><br> 4101 * Type: <b>reference</b><br> 4102 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4103 * </p> 4104 */ 4105 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4106 SP_PREDECESSOR); 4107 4108 /** 4109 * Constant for fluent queries to be used to add include statements. Specifies 4110 * the path value of "<b>EvidenceVariable:predecessor</b>". 4111 */ 4112 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 4113 "EvidenceVariable:predecessor").toLocked(); 4114 4115 /** 4116 * Search parameter: <b>title</b> 4117 * <p> 4118 * Description: <b>The human-friendly name of the evidence variable</b><br> 4119 * Type: <b>string</b><br> 4120 * Path: <b>EvidenceVariable.title</b><br> 4121 * </p> 4122 */ 4123 @SearchParamDefinition(name = "title", path = "EvidenceVariable.title", description = "The human-friendly name of the evidence variable", type = "string") 4124 public static final String SP_TITLE = "title"; 4125 /** 4126 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4127 * <p> 4128 * Description: <b>The human-friendly name of the evidence variable</b><br> 4129 * Type: <b>string</b><br> 4130 * Path: <b>EvidenceVariable.title</b><br> 4131 * </p> 4132 */ 4133 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4134 SP_TITLE); 4135 4136 /** 4137 * Search parameter: <b>composed-of</b> 4138 * <p> 4139 * Description: <b>What resource is being referenced</b><br> 4140 * Type: <b>reference</b><br> 4141 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4142 * </p> 4143 */ 4144 @SearchParamDefinition(name = "composed-of", path = "EvidenceVariable.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 4145 public static final String SP_COMPOSED_OF = "composed-of"; 4146 /** 4147 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 4148 * <p> 4149 * Description: <b>What resource is being referenced</b><br> 4150 * Type: <b>reference</b><br> 4151 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4152 * </p> 4153 */ 4154 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4155 SP_COMPOSED_OF); 4156 4157 /** 4158 * Constant for fluent queries to be used to add include statements. Specifies 4159 * the path value of "<b>EvidenceVariable:composed-of</b>". 4160 */ 4161 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 4162 "EvidenceVariable:composed-of").toLocked(); 4163 4164 /** 4165 * Search parameter: <b>version</b> 4166 * <p> 4167 * Description: <b>The business version of the evidence variable</b><br> 4168 * Type: <b>token</b><br> 4169 * Path: <b>EvidenceVariable.version</b><br> 4170 * </p> 4171 */ 4172 @SearchParamDefinition(name = "version", path = "EvidenceVariable.version", description = "The business version of the evidence variable", type = "token") 4173 public static final String SP_VERSION = "version"; 4174 /** 4175 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4176 * <p> 4177 * Description: <b>The business version of the evidence variable</b><br> 4178 * Type: <b>token</b><br> 4179 * Path: <b>EvidenceVariable.version</b><br> 4180 * </p> 4181 */ 4182 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4183 SP_VERSION); 4184 4185 /** 4186 * Search parameter: <b>url</b> 4187 * <p> 4188 * Description: <b>The uri that identifies the evidence variable</b><br> 4189 * Type: <b>uri</b><br> 4190 * Path: <b>EvidenceVariable.url</b><br> 4191 * </p> 4192 */ 4193 @SearchParamDefinition(name = "url", path = "EvidenceVariable.url", description = "The uri that identifies the evidence variable", type = "uri") 4194 public static final String SP_URL = "url"; 4195 /** 4196 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4197 * <p> 4198 * Description: <b>The uri that identifies the evidence variable</b><br> 4199 * Type: <b>uri</b><br> 4200 * Path: <b>EvidenceVariable.url</b><br> 4201 * </p> 4202 */ 4203 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4204 4205 /** 4206 * Search parameter: <b>context-quantity</b> 4207 * <p> 4208 * Description: <b>A quantity- or range-valued use context assigned to the 4209 * evidence variable</b><br> 4210 * Type: <b>quantity</b><br> 4211 * Path: <b>EvidenceVariable.useContext.valueQuantity, 4212 * EvidenceVariable.useContext.valueRange</b><br> 4213 * </p> 4214 */ 4215 @SearchParamDefinition(name = "context-quantity", path = "(EvidenceVariable.useContext.value as Quantity) | (EvidenceVariable.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the evidence variable", type = "quantity") 4216 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 4217 /** 4218 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 4219 * <p> 4220 * Description: <b>A quantity- or range-valued use context assigned to the 4221 * evidence variable</b><br> 4222 * Type: <b>quantity</b><br> 4223 * Path: <b>EvidenceVariable.useContext.valueQuantity, 4224 * EvidenceVariable.useContext.valueRange</b><br> 4225 * </p> 4226 */ 4227 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 4228 SP_CONTEXT_QUANTITY); 4229 4230 /** 4231 * Search parameter: <b>effective</b> 4232 * <p> 4233 * Description: <b>The time during which the evidence variable is intended to be 4234 * in use</b><br> 4235 * Type: <b>date</b><br> 4236 * Path: <b>EvidenceVariable.effectivePeriod</b><br> 4237 * </p> 4238 */ 4239 @SearchParamDefinition(name = "effective", path = "EvidenceVariable.effectivePeriod", description = "The time during which the evidence variable is intended to be in use", type = "date") 4240 public static final String SP_EFFECTIVE = "effective"; 4241 /** 4242 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4243 * <p> 4244 * Description: <b>The time during which the evidence variable is intended to be 4245 * in use</b><br> 4246 * Type: <b>date</b><br> 4247 * Path: <b>EvidenceVariable.effectivePeriod</b><br> 4248 * </p> 4249 */ 4250 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4251 SP_EFFECTIVE); 4252 4253 /** 4254 * Search parameter: <b>depends-on</b> 4255 * <p> 4256 * Description: <b>What resource is being referenced</b><br> 4257 * Type: <b>reference</b><br> 4258 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4259 * </p> 4260 */ 4261 @SearchParamDefinition(name = "depends-on", path = "EvidenceVariable.relatedArtifact.where(type='depends-on').resource", description = "What resource is being referenced", type = "reference") 4262 public static final String SP_DEPENDS_ON = "depends-on"; 4263 /** 4264 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 4265 * <p> 4266 * Description: <b>What resource is being referenced</b><br> 4267 * Type: <b>reference</b><br> 4268 * Path: <b>EvidenceVariable.relatedArtifact.resource</b><br> 4269 * </p> 4270 */ 4271 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4272 SP_DEPENDS_ON); 4273 4274 /** 4275 * Constant for fluent queries to be used to add include statements. Specifies 4276 * the path value of "<b>EvidenceVariable:depends-on</b>". 4277 */ 4278 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 4279 "EvidenceVariable:depends-on").toLocked(); 4280 4281 /** 4282 * Search parameter: <b>name</b> 4283 * <p> 4284 * Description: <b>Computationally friendly name of the evidence 4285 * variable</b><br> 4286 * Type: <b>string</b><br> 4287 * Path: <b>EvidenceVariable.name</b><br> 4288 * </p> 4289 */ 4290 @SearchParamDefinition(name = "name", path = "EvidenceVariable.name", description = "Computationally friendly name of the evidence variable", type = "string") 4291 public static final String SP_NAME = "name"; 4292 /** 4293 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4294 * <p> 4295 * Description: <b>Computationally friendly name of the evidence 4296 * variable</b><br> 4297 * Type: <b>string</b><br> 4298 * Path: <b>EvidenceVariable.name</b><br> 4299 * </p> 4300 */ 4301 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 4302 SP_NAME); 4303 4304 /** 4305 * Search parameter: <b>context</b> 4306 * <p> 4307 * Description: <b>A use context assigned to the evidence variable</b><br> 4308 * Type: <b>token</b><br> 4309 * Path: <b>EvidenceVariable.useContext.valueCodeableConcept</b><br> 4310 * </p> 4311 */ 4312 @SearchParamDefinition(name = "context", path = "(EvidenceVariable.useContext.value as CodeableConcept)", description = "A use context assigned to the evidence variable", type = "token") 4313 public static final String SP_CONTEXT = "context"; 4314 /** 4315 * <b>Fluent Client</b> search parameter constant for <b>context</b> 4316 * <p> 4317 * Description: <b>A use context assigned to the evidence variable</b><br> 4318 * Type: <b>token</b><br> 4319 * Path: <b>EvidenceVariable.useContext.valueCodeableConcept</b><br> 4320 * </p> 4321 */ 4322 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4323 SP_CONTEXT); 4324 4325 /** 4326 * Search parameter: <b>publisher</b> 4327 * <p> 4328 * Description: <b>Name of the publisher of the evidence variable</b><br> 4329 * Type: <b>string</b><br> 4330 * Path: <b>EvidenceVariable.publisher</b><br> 4331 * </p> 4332 */ 4333 @SearchParamDefinition(name = "publisher", path = "EvidenceVariable.publisher", description = "Name of the publisher of the evidence variable", type = "string") 4334 public static final String SP_PUBLISHER = "publisher"; 4335 /** 4336 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4337 * <p> 4338 * Description: <b>Name of the publisher of the evidence variable</b><br> 4339 * Type: <b>string</b><br> 4340 * Path: <b>EvidenceVariable.publisher</b><br> 4341 * </p> 4342 */ 4343 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4344 SP_PUBLISHER); 4345 4346 /** 4347 * Search parameter: <b>topic</b> 4348 * <p> 4349 * Description: <b>Topics associated with the EvidenceVariable</b><br> 4350 * Type: <b>token</b><br> 4351 * Path: <b>EvidenceVariable.topic</b><br> 4352 * </p> 4353 */ 4354 @SearchParamDefinition(name = "topic", path = "EvidenceVariable.topic", description = "Topics associated with the EvidenceVariable", type = "token") 4355 public static final String SP_TOPIC = "topic"; 4356 /** 4357 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4358 * <p> 4359 * Description: <b>Topics associated with the EvidenceVariable</b><br> 4360 * Type: <b>token</b><br> 4361 * Path: <b>EvidenceVariable.topic</b><br> 4362 * </p> 4363 */ 4364 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4365 SP_TOPIC); 4366 4367 /** 4368 * Search parameter: <b>context-type-quantity</b> 4369 * <p> 4370 * Description: <b>A use context type and quantity- or range-based value 4371 * assigned to the evidence variable</b><br> 4372 * Type: <b>composite</b><br> 4373 * Path: <b></b><br> 4374 * </p> 4375 */ 4376 @SearchParamDefinition(name = "context-type-quantity", path = "EvidenceVariable.useContext", description = "A use context type and quantity- or range-based value assigned to the evidence variable", type = "composite", compositeOf = { 4377 "context-type", "context-quantity" }) 4378 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 4379 /** 4380 * <b>Fluent Client</b> search parameter constant for 4381 * <b>context-type-quantity</b> 4382 * <p> 4383 * Description: <b>A use context type and quantity- or range-based value 4384 * assigned to the evidence variable</b><br> 4385 * Type: <b>composite</b><br> 4386 * Path: <b></b><br> 4387 * </p> 4388 */ 4389 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4390 SP_CONTEXT_TYPE_QUANTITY); 4391 4392 /** 4393 * Search parameter: <b>status</b> 4394 * <p> 4395 * Description: <b>The current status of the evidence variable</b><br> 4396 * Type: <b>token</b><br> 4397 * Path: <b>EvidenceVariable.status</b><br> 4398 * </p> 4399 */ 4400 @SearchParamDefinition(name = "status", path = "EvidenceVariable.status", description = "The current status of the evidence variable", type = "token") 4401 public static final String SP_STATUS = "status"; 4402 /** 4403 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4404 * <p> 4405 * Description: <b>The current status of the evidence variable</b><br> 4406 * Type: <b>token</b><br> 4407 * Path: <b>EvidenceVariable.status</b><br> 4408 * </p> 4409 */ 4410 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4411 SP_STATUS); 4412 4413}