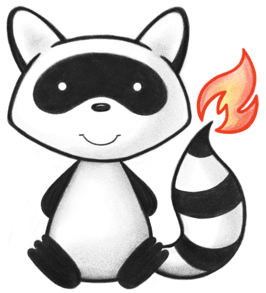
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * Example of workflow instance. 052 */ 053@ResourceDef(name = "ExampleScenario", profile = "http://hl7.org/fhir/StructureDefinition/ExampleScenario") 054@ChildOrder(names = { "url", "identifier", "version", "name", "status", "experimental", "date", "publisher", "contact", 055 "useContext", "jurisdiction", "copyright", "purpose", "actor", "instance", "process", "workflow" }) 056public class ExampleScenario extends MetadataResource { 057 058 public enum ExampleScenarioActorType { 059 /** 060 * A person. 061 */ 062 PERSON, 063 /** 064 * A system. 065 */ 066 ENTITY, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static ExampleScenarioActorType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("person".equals(codeString)) 076 return PERSON; 077 if ("entity".equals(codeString)) 078 return ENTITY; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 083 } 084 085 public String toCode() { 086 switch (this) { 087 case PERSON: 088 return "person"; 089 case ENTITY: 090 return "entity"; 091 case NULL: 092 return null; 093 default: 094 return "?"; 095 } 096 } 097 098 public String getSystem() { 099 switch (this) { 100 case PERSON: 101 return "http://hl7.org/fhir/examplescenario-actor-type"; 102 case ENTITY: 103 return "http://hl7.org/fhir/examplescenario-actor-type"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getDefinition() { 112 switch (this) { 113 case PERSON: 114 return "A person."; 115 case ENTITY: 116 return "A system."; 117 case NULL: 118 return null; 119 default: 120 return "?"; 121 } 122 } 123 124 public String getDisplay() { 125 switch (this) { 126 case PERSON: 127 return "Person"; 128 case ENTITY: 129 return "System"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 } 137 138 public static class ExampleScenarioActorTypeEnumFactory implements EnumFactory<ExampleScenarioActorType> { 139 public ExampleScenarioActorType fromCode(String codeString) throws IllegalArgumentException { 140 if (codeString == null || "".equals(codeString)) 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("person".equals(codeString)) 144 return ExampleScenarioActorType.PERSON; 145 if ("entity".equals(codeString)) 146 return ExampleScenarioActorType.ENTITY; 147 throw new IllegalArgumentException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 148 } 149 150 public Enumeration<ExampleScenarioActorType> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.NULL, code); 155 String codeString = code.asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.NULL, code); 158 if ("person".equals(codeString)) 159 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.PERSON, code); 160 if ("entity".equals(codeString)) 161 return new Enumeration<ExampleScenarioActorType>(this, ExampleScenarioActorType.ENTITY, code); 162 throw new FHIRException("Unknown ExampleScenarioActorType code '" + codeString + "'"); 163 } 164 165 public String toCode(ExampleScenarioActorType code) { 166 if (code == ExampleScenarioActorType.NULL) 167 return null; 168 if (code == ExampleScenarioActorType.PERSON) 169 return "person"; 170 if (code == ExampleScenarioActorType.ENTITY) 171 return "entity"; 172 return "?"; 173 } 174 175 public String toSystem(ExampleScenarioActorType code) { 176 return code.getSystem(); 177 } 178 } 179 180 public enum FHIRResourceType { 181 /** 182 * A financial tool for tracking value accrued for a particular purpose. In the 183 * healthcare field, used to track charges for a patient, cost centers, etc. 184 */ 185 ACCOUNT, 186 /** 187 * This resource allows for the definition of some activity to be performed, 188 * independent of a particular patient, practitioner, or other performance 189 * context. 190 */ 191 ACTIVITYDEFINITION, 192 /** 193 * Actual or potential/avoided event causing unintended physical injury 194 * resulting from or contributed to by medical care, a research study or other 195 * healthcare setting factors that requires additional monitoring, treatment, or 196 * hospitalization, or that results in death. 197 */ 198 ADVERSEEVENT, 199 /** 200 * Risk of harmful or undesirable, physiological response which is unique to an 201 * individual and associated with exposure to a substance. 202 */ 203 ALLERGYINTOLERANCE, 204 /** 205 * A booking of a healthcare event among patient(s), practitioner(s), related 206 * person(s) and/or device(s) for a specific date/time. This may result in one 207 * or more Encounter(s). 208 */ 209 APPOINTMENT, 210 /** 211 * A reply to an appointment request for a patient and/or practitioner(s), such 212 * as a confirmation or rejection. 213 */ 214 APPOINTMENTRESPONSE, 215 /** 216 * A record of an event made for purposes of maintaining a security log. Typical 217 * uses include detection of intrusion attempts and monitoring for inappropriate 218 * usage. 219 */ 220 AUDITEVENT, 221 /** 222 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 223 * resources that don't map to an existing resource, and custom resources not 224 * appropriate for inclusion in the FHIR specification. 225 */ 226 BASIC, 227 /** 228 * A resource that represents the data of a single raw artifact as digital 229 * content accessible in its native format. A Binary resource can contain any 230 * content, whether text, image, pdf, zip archive, etc. 231 */ 232 BINARY, 233 /** 234 * A material substance originating from a biological entity intended to be 235 * transplanted or infused into another (possibly the same) biological entity. 236 */ 237 BIOLOGICALLYDERIVEDPRODUCT, 238 /** 239 * Record details about an anatomical structure. This resource may be used when 240 * a coded concept does not provide the necessary detail needed for the use 241 * case. 242 */ 243 BODYSTRUCTURE, 244 /** 245 * A container for a collection of resources. 246 */ 247 BUNDLE, 248 /** 249 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR 250 * Server for a particular version of FHIR that may be used as a statement of 251 * actual server functionality or a statement of required or desired server 252 * implementation. 253 */ 254 CAPABILITYSTATEMENT, 255 /** 256 * Describes the intention of how one or more practitioners intend to deliver 257 * care for a particular patient, group or community for a period of time, 258 * possibly limited to care for a specific condition or set of conditions. 259 */ 260 CAREPLAN, 261 /** 262 * The Care Team includes all the people and organizations who plan to 263 * participate in the coordination and delivery of care for a patient. 264 */ 265 CARETEAM, 266 /** 267 * Catalog entries are wrappers that contextualize items included in a catalog. 268 */ 269 CATALOGENTRY, 270 /** 271 * The resource ChargeItem describes the provision of healthcare provider 272 * products for a certain patient, therefore referring not only to the product, 273 * but containing in addition details of the provision, like date, time, amounts 274 * and participating organizations and persons. Main Usage of the ChargeItem is 275 * to enable the billing process and internal cost allocation. 276 */ 277 CHARGEITEM, 278 /** 279 * The ChargeItemDefinition resource provides the properties that apply to the 280 * (billing) codes necessary to calculate costs and prices. The properties may 281 * differ largely depending on type and realm, therefore this resource gives 282 * only a rough structure and requires profiling for each type of billing code 283 * system. 284 */ 285 CHARGEITEMDEFINITION, 286 /** 287 * A provider issued list of professional services and products which have been 288 * provided, or are to be provided, to a patient which is sent to an insurer for 289 * reimbursement. 290 */ 291 CLAIM, 292 /** 293 * This resource provides the adjudication details from the processing of a 294 * Claim resource. 295 */ 296 CLAIMRESPONSE, 297 /** 298 * A record of a clinical assessment performed to determine what problem(s) may 299 * affect the patient and before planning the treatments or management 300 * strategies that are best to manage a patient's condition. Assessments are 301 * often 1:1 with a clinical consultation / encounter, but this varies greatly 302 * depending on the clinical workflow. This resource is called 303 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 304 * the recording of assessment tools such as Apgar score. 305 */ 306 CLINICALIMPRESSION, 307 /** 308 * The CodeSystem resource is used to declare the existence of and describe a 309 * code system or code system supplement and its key properties, and optionally 310 * define a part or all of its content. 311 */ 312 CODESYSTEM, 313 /** 314 * An occurrence of information being transmitted; e.g. an alert that was sent 315 * to a responsible provider, a public health agency that was notified about a 316 * reportable condition. 317 */ 318 COMMUNICATION, 319 /** 320 * A request to convey information; e.g. the CDS system proposes that an alert 321 * be sent to a responsible provider, the CDS system proposes that the public 322 * health agency be notified about a reportable condition. 323 */ 324 COMMUNICATIONREQUEST, 325 /** 326 * A compartment definition that defines how resources are accessed on a server. 327 */ 328 COMPARTMENTDEFINITION, 329 /** 330 * A set of healthcare-related information that is assembled together into a 331 * single logical package that provides a single coherent statement of meaning, 332 * establishes its own context and that has clinical attestation with regard to 333 * who is making the statement. A Composition defines the structure and 334 * narrative content necessary for a document. However, a Composition alone does 335 * not constitute a document. Rather, the Composition must be the first entry in 336 * a Bundle where Bundle.type=document, and any other resources referenced from 337 * Composition must be included as subsequent entries in the Bundle (for example 338 * Patient, Practitioner, Encounter, etc.). 339 */ 340 COMPOSITION, 341 /** 342 * A statement of relationships from one set of concepts to one or more other 343 * concepts - either concepts in code systems, or data element/data element 344 * concepts, or classes in class models. 345 */ 346 CONCEPTMAP, 347 /** 348 * A clinical condition, problem, diagnosis, or other event, situation, issue, 349 * or clinical concept that has risen to a level of concern. 350 */ 351 CONDITION, 352 /** 353 * A record of a healthcare consumer?s choices, which permits or denies 354 * identified recipient(s) or recipient role(s) to perform one or more actions 355 * within a given policy context, for specific purposes and periods of time. 356 */ 357 CONSENT, 358 /** 359 * Legally enforceable, formally recorded unilateral or bilateral directive 360 * i.e., a policy or agreement. 361 */ 362 CONTRACT, 363 /** 364 * Financial instrument which may be used to reimburse or pay for health care 365 * products and services. Includes both insurance and self-payment. 366 */ 367 COVERAGE, 368 /** 369 * The CoverageEligibilityRequest provides patient and insurance coverage 370 * information to an insurer for them to respond, in the form of an 371 * CoverageEligibilityResponse, with information regarding whether the stated 372 * coverage is valid and in-force and optionally to provide the insurance 373 * details of the policy. 374 */ 375 COVERAGEELIGIBILITYREQUEST, 376 /** 377 * This resource provides eligibility and plan details from the processing of an 378 * CoverageEligibilityRequest resource. 379 */ 380 COVERAGEELIGIBILITYRESPONSE, 381 /** 382 * Indicates an actual or potential clinical issue with or between one or more 383 * active or proposed clinical actions for a patient; e.g. Drug-drug 384 * interaction, Ineffective treatment frequency, Procedure-condition conflict, 385 * etc. 386 */ 387 DETECTEDISSUE, 388 /** 389 * A type of a manufactured item that is used in the provision of healthcare 390 * without being substantially changed through that activity. The device may be 391 * a medical or non-medical device. 392 */ 393 DEVICE, 394 /** 395 * The characteristics, operational status and capabilities of a medical-related 396 * component of a medical device. 397 */ 398 DEVICEDEFINITION, 399 /** 400 * Describes a measurement, calculation or setting capability of a medical 401 * device. 402 */ 403 DEVICEMETRIC, 404 /** 405 * Represents a request for a patient to employ a medical device. The device may 406 * be an implantable device, or an external assistive device, such as a walker. 407 */ 408 DEVICEREQUEST, 409 /** 410 * A record of a device being used by a patient where the record is the result 411 * of a report from the patient or another clinician. 412 */ 413 DEVICEUSESTATEMENT, 414 /** 415 * The findings and interpretation of diagnostic tests performed on patients, 416 * groups of patients, devices, and locations, and/or specimens derived from 417 * these. The report includes clinical context such as requesting and provider 418 * information, and some mix of atomic results, images, textual and coded 419 * interpretations, and formatted representation of diagnostic reports. 420 */ 421 DIAGNOSTICREPORT, 422 /** 423 * A collection of documents compiled for a purpose together with metadata that 424 * applies to the collection. 425 */ 426 DOCUMENTMANIFEST, 427 /** 428 * A reference to a document of any kind for any purpose. Provides metadata 429 * about the document so that the document can be discovered and managed. The 430 * scope of a document is any seralized object with a mime-type, so includes 431 * formal patient centric documents (CDA), cliical notes, scanned paper, and 432 * non-patient specific documents like policy text. 433 */ 434 DOCUMENTREFERENCE, 435 /** 436 * A resource that includes narrative, extensions, and contained resources. 437 */ 438 DOMAINRESOURCE, 439 /** 440 * The EffectEvidenceSynthesis resource describes the difference in an outcome 441 * between exposures states in a population where the effect estimate is derived 442 * from a combination of research studies. 443 */ 444 EFFECTEVIDENCESYNTHESIS, 445 /** 446 * An interaction between a patient and healthcare provider(s) for the purpose 447 * of providing healthcare service(s) or assessing the health status of a 448 * patient. 449 */ 450 ENCOUNTER, 451 /** 452 * The technical details of an endpoint that can be used for electronic 453 * services, such as for web services providing XDS.b or a REST endpoint for 454 * another FHIR server. This may include any security context information. 455 */ 456 ENDPOINT, 457 /** 458 * This resource provides the insurance enrollment details to the insurer 459 * regarding a specified coverage. 460 */ 461 ENROLLMENTREQUEST, 462 /** 463 * This resource provides enrollment and plan details from the processing of an 464 * EnrollmentRequest resource. 465 */ 466 ENROLLMENTRESPONSE, 467 /** 468 * An association between a patient and an organization / healthcare provider(s) 469 * during which time encounters may occur. The managing organization assumes a 470 * level of responsibility for the patient during this time. 471 */ 472 EPISODEOFCARE, 473 /** 474 * The EventDefinition resource provides a reusable description of when a 475 * particular event can occur. 476 */ 477 EVENTDEFINITION, 478 /** 479 * The Evidence resource describes the conditional state (population and any 480 * exposures being compared within the population) and outcome (if specified) 481 * that the knowledge (evidence, assertion, recommendation) is about. 482 */ 483 EVIDENCE, 484 /** 485 * The EvidenceVariable resource describes a "PICO" element that knowledge 486 * (evidence, assertion, recommendation) is about. 487 */ 488 EVIDENCEVARIABLE, 489 /** 490 * Example of workflow instance. 491 */ 492 EXAMPLESCENARIO, 493 /** 494 * This resource provides: the claim details; adjudication details from the 495 * processing of a Claim; and optionally account balance information, for 496 * informing the subscriber of the benefits provided. 497 */ 498 EXPLANATIONOFBENEFIT, 499 /** 500 * Significant health conditions for a person related to the patient relevant in 501 * the context of care for the patient. 502 */ 503 FAMILYMEMBERHISTORY, 504 /** 505 * Prospective warnings of potential issues when providing care to the patient. 506 */ 507 FLAG, 508 /** 509 * Describes the intended objective(s) for a patient, group or organization 510 * care, for example, weight loss, restoring an activity of daily living, 511 * obtaining herd immunity via immunization, meeting a process improvement 512 * objective, etc. 513 */ 514 GOAL, 515 /** 516 * A formal computable definition of a graph of resources - that is, a coherent 517 * set of resources that form a graph by following references. The Graph 518 * Definition resource defines a set and makes rules about the set. 519 */ 520 GRAPHDEFINITION, 521 /** 522 * Represents a defined collection of entities that may be discussed or acted 523 * upon collectively but which are not expected to act collectively, and are not 524 * formally or legally recognized; i.e. a collection of entities that isn't an 525 * Organization. 526 */ 527 GROUP, 528 /** 529 * A guidance response is the formal response to a guidance request, including 530 * any output parameters returned by the evaluation, as well as the description 531 * of any proposed actions to be taken. 532 */ 533 GUIDANCERESPONSE, 534 /** 535 * The details of a healthcare service available at a location. 536 */ 537 HEALTHCARESERVICE, 538 /** 539 * Representation of the content produced in a DICOM imaging study. A study 540 * comprises a set of series, each of which includes a set of Service-Object 541 * Pair Instances (SOP Instances - images or other data) acquired or produced in 542 * a common context. A series is of only one modality (e.g. X-ray, CT, MR, 543 * ultrasound), but a study may have multiple series of different modalities. 544 */ 545 IMAGINGSTUDY, 546 /** 547 * Describes the event of a patient being administered a vaccine or a record of 548 * an immunization as reported by a patient, a clinician or another party. 549 */ 550 IMMUNIZATION, 551 /** 552 * Describes a comparison of an immunization event against published 553 * recommendations to determine if the administration is "valid" in relation to 554 * those recommendations. 555 */ 556 IMMUNIZATIONEVALUATION, 557 /** 558 * A patient's point-in-time set of recommendations (i.e. forecasting) according 559 * to a published schedule with optional supporting justification. 560 */ 561 IMMUNIZATIONRECOMMENDATION, 562 /** 563 * A set of rules of how a particular interoperability or standards problem is 564 * solved - typically through the use of FHIR resources. This resource is used 565 * to gather all the parts of an implementation guide into a logical whole and 566 * to publish a computable definition of all the parts. 567 */ 568 IMPLEMENTATIONGUIDE, 569 /** 570 * Details of a Health Insurance product/plan provided by an organization. 571 */ 572 INSURANCEPLAN, 573 /** 574 * Invoice containing collected ChargeItems from an Account with calculated 575 * individual and total price for Billing purpose. 576 */ 577 INVOICE, 578 /** 579 * The Library resource is a general-purpose container for knowledge asset 580 * definitions. It can be used to describe and expose existing knowledge assets 581 * such as logic libraries and information model descriptions, as well as to 582 * describe a collection of knowledge assets. 583 */ 584 LIBRARY, 585 /** 586 * Identifies two or more records (resource instances) that refer to the same 587 * real-world "occurrence". 588 */ 589 LINKAGE, 590 /** 591 * A list is a curated collection of resources. 592 */ 593 LIST, 594 /** 595 * Details and position information for a physical place where services are 596 * provided and resources and participants may be stored, found, contained, or 597 * accommodated. 598 */ 599 LOCATION, 600 /** 601 * The Measure resource provides the definition of a quality measure. 602 */ 603 MEASURE, 604 /** 605 * The MeasureReport resource contains the results of the calculation of a 606 * measure; and optionally a reference to the resources involved in that 607 * calculation. 608 */ 609 MEASUREREPORT, 610 /** 611 * A photo, video, or audio recording acquired or used in healthcare. The actual 612 * content may be inline or provided by direct reference. 613 */ 614 MEDIA, 615 /** 616 * This resource is primarily used for the identification and definition of a 617 * medication for the purposes of prescribing, dispensing, and administering a 618 * medication as well as for making statements about medication use. 619 */ 620 MEDICATION, 621 /** 622 * Describes the event of a patient consuming or otherwise being administered a 623 * medication. This may be as simple as swallowing a tablet or it may be a long 624 * running infusion. Related resources tie this event to the authorizing 625 * prescription, and the specific encounter between patient and health care 626 * practitioner. 627 */ 628 MEDICATIONADMINISTRATION, 629 /** 630 * Indicates that a medication product is to be or has been dispensed for a 631 * named person/patient. This includes a description of the medication product 632 * (supply) provided and the instructions for administering the medication. The 633 * medication dispense is the result of a pharmacy system responding to a 634 * medication order. 635 */ 636 MEDICATIONDISPENSE, 637 /** 638 * Information about a medication that is used to support knowledge. 639 */ 640 MEDICATIONKNOWLEDGE, 641 /** 642 * An order or request for both supply of the medication and the instructions 643 * for administration of the medication to a patient. The resource is called 644 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 645 * to generalize the use across inpatient and outpatient settings, including 646 * care plans, etc., and to harmonize with workflow patterns. 647 */ 648 MEDICATIONREQUEST, 649 /** 650 * A record of a medication that is being consumed by a patient. A 651 * MedicationStatement may indicate that the patient may be taking the 652 * medication now or has taken the medication in the past or will be taking the 653 * medication in the future. The source of this information can be the patient, 654 * significant other (such as a family member or spouse), or a clinician. A 655 * common scenario where this information is captured is during the history 656 * taking process during a patient visit or stay. The medication information may 657 * come from sources such as the patient's memory, from a prescription bottle, 658 * or from a list of medications the patient, clinician or other party 659 * maintains. 660 * 661 * The primary difference between a medication statement and a medication 662 * administration is that the medication administration has complete 663 * administration information and is based on actual administration information 664 * from the person who administered the medication. A medication statement is 665 * often, if not always, less specific. There is no required date/time when the 666 * medication was administered, in fact we only know that a source has reported 667 * the patient is taking this medication, where details such as time, quantity, 668 * or rate or even medication product may be incomplete or missing or less 669 * precise. As stated earlier, the medication statement information may come 670 * from the patient's memory, from a prescription bottle or from a list of 671 * medications the patient, clinician or other party maintains. Medication 672 * administration is more formal and is not missing detailed information. 673 */ 674 MEDICATIONSTATEMENT, 675 /** 676 * Detailed definition of a medicinal product, typically for uses other than 677 * direct patient care (e.g. regulatory use). 678 */ 679 MEDICINALPRODUCT, 680 /** 681 * The regulatory authorization of a medicinal product. 682 */ 683 MEDICINALPRODUCTAUTHORIZATION, 684 /** 685 * The clinical particulars - indications, contraindications etc. of a medicinal 686 * product, including for regulatory purposes. 687 */ 688 MEDICINALPRODUCTCONTRAINDICATION, 689 /** 690 * Indication for the Medicinal Product. 691 */ 692 MEDICINALPRODUCTINDICATION, 693 /** 694 * An ingredient of a manufactured item or pharmaceutical product. 695 */ 696 MEDICINALPRODUCTINGREDIENT, 697 /** 698 * The interactions of the medicinal product with other medicinal products, or 699 * other forms of interactions. 700 */ 701 MEDICINALPRODUCTINTERACTION, 702 /** 703 * The manufactured item as contained in the packaged medicinal product. 704 */ 705 MEDICINALPRODUCTMANUFACTURED, 706 /** 707 * A medicinal product in a container or package. 708 */ 709 MEDICINALPRODUCTPACKAGED, 710 /** 711 * A pharmaceutical product described in terms of its composition and dose form. 712 */ 713 MEDICINALPRODUCTPHARMACEUTICAL, 714 /** 715 * Describe the undesirable effects of the medicinal product. 716 */ 717 MEDICINALPRODUCTUNDESIRABLEEFFECT, 718 /** 719 * Defines the characteristics of a message that can be shared between systems, 720 * including the type of event that initiates the message, the content to be 721 * transmitted and what response(s), if any, are permitted. 722 */ 723 MESSAGEDEFINITION, 724 /** 725 * The header for a message exchange that is either requesting or responding to 726 * an action. The reference(s) that are the subject of the action as well as 727 * other information related to the action are typically transmitted in a bundle 728 * in which the MessageHeader resource instance is the first resource in the 729 * bundle. 730 */ 731 MESSAGEHEADER, 732 /** 733 * Raw data describing a biological sequence. 734 */ 735 MOLECULARSEQUENCE, 736 /** 737 * A curated namespace that issues unique symbols within that namespace for the 738 * identification of concepts, people, devices, etc. Represents a "System" used 739 * within the Identifier and Coding data types. 740 */ 741 NAMINGSYSTEM, 742 /** 743 * A request to supply a diet, formula feeding (enteral) or oral nutritional 744 * supplement to a patient/resident. 745 */ 746 NUTRITIONORDER, 747 /** 748 * Measurements and simple assertions made about a patient, device or other 749 * subject. 750 */ 751 OBSERVATION, 752 /** 753 * Set of definitional characteristics for a kind of observation or measurement 754 * produced or consumed by an orderable health care service. 755 */ 756 OBSERVATIONDEFINITION, 757 /** 758 * A formal computable definition of an operation (on the RESTful interface) or 759 * a named query (using the search interaction). 760 */ 761 OPERATIONDEFINITION, 762 /** 763 * A collection of error, warning, or information messages that result from a 764 * system action. 765 */ 766 OPERATIONOUTCOME, 767 /** 768 * A formally or informally recognized grouping of people or organizations 769 * formed for the purpose of achieving some form of collective action. Includes 770 * companies, institutions, corporations, departments, community groups, 771 * healthcare practice groups, payer/insurer, etc. 772 */ 773 ORGANIZATION, 774 /** 775 * Defines an affiliation/assotiation/relationship between 2 distinct 776 * oganizations, that is not a part-of relationship/sub-division relationship. 777 */ 778 ORGANIZATIONAFFILIATION, 779 /** 780 * This resource is a non-persisted resource used to pass information into and 781 * back from an [operation](operations.html). It has no other use, and there is 782 * no RESTful endpoint associated with it. 783 */ 784 PARAMETERS, 785 /** 786 * Demographics and other administrative information about an individual or 787 * animal receiving care or other health-related services. 788 */ 789 PATIENT, 790 /** 791 * This resource provides the status of the payment for goods and services 792 * rendered, and the request and response resource references. 793 */ 794 PAYMENTNOTICE, 795 /** 796 * This resource provides the details including amount of a payment and 797 * allocates the payment items being paid. 798 */ 799 PAYMENTRECONCILIATION, 800 /** 801 * Demographics and administrative information about a person independent of a 802 * specific health-related context. 803 */ 804 PERSON, 805 /** 806 * This resource allows for the definition of various types of plans as a 807 * sharable, consumable, and executable artifact. The resource is general enough 808 * to support the description of a broad range of clinical artifacts such as 809 * clinical decision support rules, order sets and protocols. 810 */ 811 PLANDEFINITION, 812 /** 813 * A person who is directly or indirectly involved in the provisioning of 814 * healthcare. 815 */ 816 PRACTITIONER, 817 /** 818 * A specific set of Roles/Locations/specialties/services that a practitioner 819 * may perform at an organization for a period of time. 820 */ 821 PRACTITIONERROLE, 822 /** 823 * An action that is or was performed on or for a patient. This can be a 824 * physical intervention like an operation, or less invasive like long term 825 * services, counseling, or hypnotherapy. 826 */ 827 PROCEDURE, 828 /** 829 * Provenance of a resource is a record that describes entities and processes 830 * involved in producing and delivering or otherwise influencing that resource. 831 * Provenance provides a critical foundation for assessing authenticity, 832 * enabling trust, and allowing reproducibility. Provenance assertions are a 833 * form of contextual metadata and can themselves become important records with 834 * their own provenance. Provenance statement indicates clinical significance in 835 * terms of confidence in authenticity, reliability, and trustworthiness, 836 * integrity, and stage in lifecycle (e.g. Document Completion - has the 837 * artifact been legally authenticated), all of which may impact security, 838 * privacy, and trust policies. 839 */ 840 PROVENANCE, 841 /** 842 * A structured set of questions intended to guide the collection of answers 843 * from end-users. Questionnaires provide detailed control over order, 844 * presentation, phraseology and grouping to allow coherent, consistent data 845 * collection. 846 */ 847 QUESTIONNAIRE, 848 /** 849 * A structured set of questions and their answers. The questions are ordered 850 * and grouped into coherent subsets, corresponding to the structure of the 851 * grouping of the questionnaire being responded to. 852 */ 853 QUESTIONNAIRERESPONSE, 854 /** 855 * Information about a person that is involved in the care for a patient, but 856 * who is not the target of healthcare, nor has a formal responsibility in the 857 * care process. 858 */ 859 RELATEDPERSON, 860 /** 861 * A group of related requests that can be used to capture intended activities 862 * that have inter-dependencies such as "give this medication after that one". 863 */ 864 REQUESTGROUP, 865 /** 866 * The ResearchDefinition resource describes the conditional state (population 867 * and any exposures being compared within the population) and outcome (if 868 * specified) that the knowledge (evidence, assertion, recommendation) is about. 869 */ 870 RESEARCHDEFINITION, 871 /** 872 * The ResearchElementDefinition resource describes a "PICO" element that 873 * knowledge (evidence, assertion, recommendation) is about. 874 */ 875 RESEARCHELEMENTDEFINITION, 876 /** 877 * A process where a researcher or organization plans and then executes a series 878 * of steps intended to increase the field of healthcare-related knowledge. This 879 * includes studies of safety, efficacy, comparative effectiveness and other 880 * information about medications, devices, therapies and other interventional 881 * and investigative techniques. A ResearchStudy involves the gathering of 882 * information about human or animal subjects. 883 */ 884 RESEARCHSTUDY, 885 /** 886 * A physical entity which is the primary unit of operational and/or 887 * administrative interest in a study. 888 */ 889 RESEARCHSUBJECT, 890 /** 891 * This is the base resource type for everything. 892 */ 893 RESOURCE, 894 /** 895 * An assessment of the likely outcome(s) for a patient or other subject as well 896 * as the likelihood of each outcome. 897 */ 898 RISKASSESSMENT, 899 /** 900 * The RiskEvidenceSynthesis resource describes the likelihood of an outcome in 901 * a population plus exposure state where the risk estimate is derived from a 902 * combination of research studies. 903 */ 904 RISKEVIDENCESYNTHESIS, 905 /** 906 * A container for slots of time that may be available for booking appointments. 907 */ 908 SCHEDULE, 909 /** 910 * A search parameter that defines a named search item that can be used to 911 * search/filter on a resource. 912 */ 913 SEARCHPARAMETER, 914 /** 915 * A record of a request for service such as diagnostic investigations, 916 * treatments, or operations to be performed. 917 */ 918 SERVICEREQUEST, 919 /** 920 * A slot of time on a schedule that may be available for booking appointments. 921 */ 922 SLOT, 923 /** 924 * A sample to be used for analysis. 925 */ 926 SPECIMEN, 927 /** 928 * A kind of specimen with associated set of requirements. 929 */ 930 SPECIMENDEFINITION, 931 /** 932 * A definition of a FHIR structure. This resource is used to describe the 933 * underlying resources, data types defined in FHIR, and also for describing 934 * extensions and constraints on resources and data types. 935 */ 936 STRUCTUREDEFINITION, 937 /** 938 * A Map of relationships between 2 structures that can be used to transform 939 * data. 940 */ 941 STRUCTUREMAP, 942 /** 943 * The subscription resource is used to define a push-based subscription from a 944 * server to another system. Once a subscription is registered with the server, 945 * the server checks every resource that is created or updated, and if the 946 * resource matches the given criteria, it sends a message on the defined 947 * "channel" so that another system can take an appropriate action. 948 */ 949 SUBSCRIPTION, 950 /** 951 * A homogeneous material with a definite composition. 952 */ 953 SUBSTANCE, 954 /** 955 * Nucleic acids are defined by three distinct elements: the base, sugar and 956 * linkage. Individual substance/moiety IDs will be created for each of these 957 * elements. The nucleotide sequence will be always entered in the 5?-3? 958 * direction. 959 */ 960 SUBSTANCENUCLEICACID, 961 /** 962 * Todo. 963 */ 964 SUBSTANCEPOLYMER, 965 /** 966 * A SubstanceProtein is defined as a single unit of a linear amino acid 967 * sequence, or a combination of subunits that are either covalently linked or 968 * have a defined invariant stoichiometric relationship. This includes all 969 * synthetic, recombinant and purified SubstanceProteins of defined sequence, 970 * whether the use is therapeutic or prophylactic. This set of elements will be 971 * used to describe albumins, coagulation factors, cytokines, growth factors, 972 * peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant 973 * vaccines, and immunomodulators. 974 */ 975 SUBSTANCEPROTEIN, 976 /** 977 * Todo. 978 */ 979 SUBSTANCEREFERENCEINFORMATION, 980 /** 981 * Source material shall capture information on the taxonomic and anatomical 982 * origins as well as the fraction of a material that can result in or can be 983 * modified to form a substance. This set of data elements shall be used to 984 * define polymer substances isolated from biological matrices. Taxonomic and 985 * anatomical origins shall be described using a controlled vocabulary as 986 * required. This information is captured for naturally derived polymers ( . 987 * starch) and structurally diverse substances. For Organisms belonging to the 988 * Kingdom Plantae the Substance level defines the fresh material of a single 989 * species or infraspecies, the Herbal Drug and the Herbal preparation. For 990 * Herbal preparations, the fraction information will be captured at the 991 * Substance information level and additional information for herbal extracts 992 * will be captured at the Specified Substance Group 1 information level. See 993 * for further explanation the Substance Class: Structurally Diverse and the 994 * herbal annex. 995 */ 996 SUBSTANCESOURCEMATERIAL, 997 /** 998 * The detailed description of a substance, typically at a level beyond what is 999 * used for prescribing. 1000 */ 1001 SUBSTANCESPECIFICATION, 1002 /** 1003 * Record of delivery of what is supplied. 1004 */ 1005 SUPPLYDELIVERY, 1006 /** 1007 * A record of a request for a medication, substance or device used in the 1008 * healthcare setting. 1009 */ 1010 SUPPLYREQUEST, 1011 /** 1012 * A task to be performed. 1013 */ 1014 TASK, 1015 /** 1016 * A TerminologyCapabilities resource documents a set of capabilities 1017 * (behaviors) of a FHIR Terminology Server that may be used as a statement of 1018 * actual server functionality or a statement of required or desired server 1019 * implementation. 1020 */ 1021 TERMINOLOGYCAPABILITIES, 1022 /** 1023 * A summary of information based on the results of executing a TestScript. 1024 */ 1025 TESTREPORT, 1026 /** 1027 * A structured set of tests against a FHIR server or client implementation to 1028 * determine compliance against the FHIR specification. 1029 */ 1030 TESTSCRIPT, 1031 /** 1032 * A ValueSet resource instance specifies a set of codes drawn from one or more 1033 * code systems, intended for use in a particular context. Value sets link 1034 * between [[[CodeSystem]]] definitions and their use in [coded 1035 * elements](terminologies.html). 1036 */ 1037 VALUESET, 1038 /** 1039 * Describes validation requirements, source(s), status and dates for one or 1040 * more elements. 1041 */ 1042 VERIFICATIONRESULT, 1043 /** 1044 * An authorization for the provision of glasses and/or contact lenses to a 1045 * patient. 1046 */ 1047 VISIONPRESCRIPTION, 1048 /** 1049 * added to help the parsers with the generic types 1050 */ 1051 NULL; 1052 1053 public static FHIRResourceType fromCode(String codeString) throws FHIRException { 1054 if (codeString == null || "".equals(codeString)) 1055 return null; 1056 if ("Account".equals(codeString)) 1057 return ACCOUNT; 1058 if ("ActivityDefinition".equals(codeString)) 1059 return ACTIVITYDEFINITION; 1060 if ("AdverseEvent".equals(codeString)) 1061 return ADVERSEEVENT; 1062 if ("AllergyIntolerance".equals(codeString)) 1063 return ALLERGYINTOLERANCE; 1064 if ("Appointment".equals(codeString)) 1065 return APPOINTMENT; 1066 if ("AppointmentResponse".equals(codeString)) 1067 return APPOINTMENTRESPONSE; 1068 if ("AuditEvent".equals(codeString)) 1069 return AUDITEVENT; 1070 if ("Basic".equals(codeString)) 1071 return BASIC; 1072 if ("Binary".equals(codeString)) 1073 return BINARY; 1074 if ("BiologicallyDerivedProduct".equals(codeString)) 1075 return BIOLOGICALLYDERIVEDPRODUCT; 1076 if ("BodyStructure".equals(codeString)) 1077 return BODYSTRUCTURE; 1078 if ("Bundle".equals(codeString)) 1079 return BUNDLE; 1080 if ("CapabilityStatement".equals(codeString)) 1081 return CAPABILITYSTATEMENT; 1082 if ("CarePlan".equals(codeString)) 1083 return CAREPLAN; 1084 if ("CareTeam".equals(codeString)) 1085 return CARETEAM; 1086 if ("CatalogEntry".equals(codeString)) 1087 return CATALOGENTRY; 1088 if ("ChargeItem".equals(codeString)) 1089 return CHARGEITEM; 1090 if ("ChargeItemDefinition".equals(codeString)) 1091 return CHARGEITEMDEFINITION; 1092 if ("Claim".equals(codeString)) 1093 return CLAIM; 1094 if ("ClaimResponse".equals(codeString)) 1095 return CLAIMRESPONSE; 1096 if ("ClinicalImpression".equals(codeString)) 1097 return CLINICALIMPRESSION; 1098 if ("CodeSystem".equals(codeString)) 1099 return CODESYSTEM; 1100 if ("Communication".equals(codeString)) 1101 return COMMUNICATION; 1102 if ("CommunicationRequest".equals(codeString)) 1103 return COMMUNICATIONREQUEST; 1104 if ("CompartmentDefinition".equals(codeString)) 1105 return COMPARTMENTDEFINITION; 1106 if ("Composition".equals(codeString)) 1107 return COMPOSITION; 1108 if ("ConceptMap".equals(codeString)) 1109 return CONCEPTMAP; 1110 if ("Condition".equals(codeString)) 1111 return CONDITION; 1112 if ("Consent".equals(codeString)) 1113 return CONSENT; 1114 if ("Contract".equals(codeString)) 1115 return CONTRACT; 1116 if ("Coverage".equals(codeString)) 1117 return COVERAGE; 1118 if ("CoverageEligibilityRequest".equals(codeString)) 1119 return COVERAGEELIGIBILITYREQUEST; 1120 if ("CoverageEligibilityResponse".equals(codeString)) 1121 return COVERAGEELIGIBILITYRESPONSE; 1122 if ("DetectedIssue".equals(codeString)) 1123 return DETECTEDISSUE; 1124 if ("Device".equals(codeString)) 1125 return DEVICE; 1126 if ("DeviceDefinition".equals(codeString)) 1127 return DEVICEDEFINITION; 1128 if ("DeviceMetric".equals(codeString)) 1129 return DEVICEMETRIC; 1130 if ("DeviceRequest".equals(codeString)) 1131 return DEVICEREQUEST; 1132 if ("DeviceUseStatement".equals(codeString)) 1133 return DEVICEUSESTATEMENT; 1134 if ("DiagnosticReport".equals(codeString)) 1135 return DIAGNOSTICREPORT; 1136 if ("DocumentManifest".equals(codeString)) 1137 return DOCUMENTMANIFEST; 1138 if ("DocumentReference".equals(codeString)) 1139 return DOCUMENTREFERENCE; 1140 if ("DomainResource".equals(codeString)) 1141 return DOMAINRESOURCE; 1142 if ("EffectEvidenceSynthesis".equals(codeString)) 1143 return EFFECTEVIDENCESYNTHESIS; 1144 if ("Encounter".equals(codeString)) 1145 return ENCOUNTER; 1146 if ("Endpoint".equals(codeString)) 1147 return ENDPOINT; 1148 if ("EnrollmentRequest".equals(codeString)) 1149 return ENROLLMENTREQUEST; 1150 if ("EnrollmentResponse".equals(codeString)) 1151 return ENROLLMENTRESPONSE; 1152 if ("EpisodeOfCare".equals(codeString)) 1153 return EPISODEOFCARE; 1154 if ("EventDefinition".equals(codeString)) 1155 return EVENTDEFINITION; 1156 if ("Evidence".equals(codeString)) 1157 return EVIDENCE; 1158 if ("EvidenceVariable".equals(codeString)) 1159 return EVIDENCEVARIABLE; 1160 if ("ExampleScenario".equals(codeString)) 1161 return EXAMPLESCENARIO; 1162 if ("ExplanationOfBenefit".equals(codeString)) 1163 return EXPLANATIONOFBENEFIT; 1164 if ("FamilyMemberHistory".equals(codeString)) 1165 return FAMILYMEMBERHISTORY; 1166 if ("Flag".equals(codeString)) 1167 return FLAG; 1168 if ("Goal".equals(codeString)) 1169 return GOAL; 1170 if ("GraphDefinition".equals(codeString)) 1171 return GRAPHDEFINITION; 1172 if ("Group".equals(codeString)) 1173 return GROUP; 1174 if ("GuidanceResponse".equals(codeString)) 1175 return GUIDANCERESPONSE; 1176 if ("HealthcareService".equals(codeString)) 1177 return HEALTHCARESERVICE; 1178 if ("ImagingStudy".equals(codeString)) 1179 return IMAGINGSTUDY; 1180 if ("Immunization".equals(codeString)) 1181 return IMMUNIZATION; 1182 if ("ImmunizationEvaluation".equals(codeString)) 1183 return IMMUNIZATIONEVALUATION; 1184 if ("ImmunizationRecommendation".equals(codeString)) 1185 return IMMUNIZATIONRECOMMENDATION; 1186 if ("ImplementationGuide".equals(codeString)) 1187 return IMPLEMENTATIONGUIDE; 1188 if ("InsurancePlan".equals(codeString)) 1189 return INSURANCEPLAN; 1190 if ("Invoice".equals(codeString)) 1191 return INVOICE; 1192 if ("Library".equals(codeString)) 1193 return LIBRARY; 1194 if ("Linkage".equals(codeString)) 1195 return LINKAGE; 1196 if ("List".equals(codeString)) 1197 return LIST; 1198 if ("Location".equals(codeString)) 1199 return LOCATION; 1200 if ("Measure".equals(codeString)) 1201 return MEASURE; 1202 if ("MeasureReport".equals(codeString)) 1203 return MEASUREREPORT; 1204 if ("Media".equals(codeString)) 1205 return MEDIA; 1206 if ("Medication".equals(codeString)) 1207 return MEDICATION; 1208 if ("MedicationAdministration".equals(codeString)) 1209 return MEDICATIONADMINISTRATION; 1210 if ("MedicationDispense".equals(codeString)) 1211 return MEDICATIONDISPENSE; 1212 if ("MedicationKnowledge".equals(codeString)) 1213 return MEDICATIONKNOWLEDGE; 1214 if ("MedicationRequest".equals(codeString)) 1215 return MEDICATIONREQUEST; 1216 if ("MedicationStatement".equals(codeString)) 1217 return MEDICATIONSTATEMENT; 1218 if ("MedicinalProduct".equals(codeString)) 1219 return MEDICINALPRODUCT; 1220 if ("MedicinalProductAuthorization".equals(codeString)) 1221 return MEDICINALPRODUCTAUTHORIZATION; 1222 if ("MedicinalProductContraindication".equals(codeString)) 1223 return MEDICINALPRODUCTCONTRAINDICATION; 1224 if ("MedicinalProductIndication".equals(codeString)) 1225 return MEDICINALPRODUCTINDICATION; 1226 if ("MedicinalProductIngredient".equals(codeString)) 1227 return MEDICINALPRODUCTINGREDIENT; 1228 if ("MedicinalProductInteraction".equals(codeString)) 1229 return MEDICINALPRODUCTINTERACTION; 1230 if ("MedicinalProductManufactured".equals(codeString)) 1231 return MEDICINALPRODUCTMANUFACTURED; 1232 if ("MedicinalProductPackaged".equals(codeString)) 1233 return MEDICINALPRODUCTPACKAGED; 1234 if ("MedicinalProductPharmaceutical".equals(codeString)) 1235 return MEDICINALPRODUCTPHARMACEUTICAL; 1236 if ("MedicinalProductUndesirableEffect".equals(codeString)) 1237 return MEDICINALPRODUCTUNDESIRABLEEFFECT; 1238 if ("MessageDefinition".equals(codeString)) 1239 return MESSAGEDEFINITION; 1240 if ("MessageHeader".equals(codeString)) 1241 return MESSAGEHEADER; 1242 if ("MolecularSequence".equals(codeString)) 1243 return MOLECULARSEQUENCE; 1244 if ("NamingSystem".equals(codeString)) 1245 return NAMINGSYSTEM; 1246 if ("NutritionOrder".equals(codeString)) 1247 return NUTRITIONORDER; 1248 if ("Observation".equals(codeString)) 1249 return OBSERVATION; 1250 if ("ObservationDefinition".equals(codeString)) 1251 return OBSERVATIONDEFINITION; 1252 if ("OperationDefinition".equals(codeString)) 1253 return OPERATIONDEFINITION; 1254 if ("OperationOutcome".equals(codeString)) 1255 return OPERATIONOUTCOME; 1256 if ("Organization".equals(codeString)) 1257 return ORGANIZATION; 1258 if ("OrganizationAffiliation".equals(codeString)) 1259 return ORGANIZATIONAFFILIATION; 1260 if ("Parameters".equals(codeString)) 1261 return PARAMETERS; 1262 if ("Patient".equals(codeString)) 1263 return PATIENT; 1264 if ("PaymentNotice".equals(codeString)) 1265 return PAYMENTNOTICE; 1266 if ("PaymentReconciliation".equals(codeString)) 1267 return PAYMENTRECONCILIATION; 1268 if ("Person".equals(codeString)) 1269 return PERSON; 1270 if ("PlanDefinition".equals(codeString)) 1271 return PLANDEFINITION; 1272 if ("Practitioner".equals(codeString)) 1273 return PRACTITIONER; 1274 if ("PractitionerRole".equals(codeString)) 1275 return PRACTITIONERROLE; 1276 if ("Procedure".equals(codeString)) 1277 return PROCEDURE; 1278 if ("Provenance".equals(codeString)) 1279 return PROVENANCE; 1280 if ("Questionnaire".equals(codeString)) 1281 return QUESTIONNAIRE; 1282 if ("QuestionnaireResponse".equals(codeString)) 1283 return QUESTIONNAIRERESPONSE; 1284 if ("RelatedPerson".equals(codeString)) 1285 return RELATEDPERSON; 1286 if ("RequestGroup".equals(codeString)) 1287 return REQUESTGROUP; 1288 if ("ResearchDefinition".equals(codeString)) 1289 return RESEARCHDEFINITION; 1290 if ("ResearchElementDefinition".equals(codeString)) 1291 return RESEARCHELEMENTDEFINITION; 1292 if ("ResearchStudy".equals(codeString)) 1293 return RESEARCHSTUDY; 1294 if ("ResearchSubject".equals(codeString)) 1295 return RESEARCHSUBJECT; 1296 if ("Resource".equals(codeString)) 1297 return RESOURCE; 1298 if ("RiskAssessment".equals(codeString)) 1299 return RISKASSESSMENT; 1300 if ("RiskEvidenceSynthesis".equals(codeString)) 1301 return RISKEVIDENCESYNTHESIS; 1302 if ("Schedule".equals(codeString)) 1303 return SCHEDULE; 1304 if ("SearchParameter".equals(codeString)) 1305 return SEARCHPARAMETER; 1306 if ("ServiceRequest".equals(codeString)) 1307 return SERVICEREQUEST; 1308 if ("Slot".equals(codeString)) 1309 return SLOT; 1310 if ("Specimen".equals(codeString)) 1311 return SPECIMEN; 1312 if ("SpecimenDefinition".equals(codeString)) 1313 return SPECIMENDEFINITION; 1314 if ("StructureDefinition".equals(codeString)) 1315 return STRUCTUREDEFINITION; 1316 if ("StructureMap".equals(codeString)) 1317 return STRUCTUREMAP; 1318 if ("Subscription".equals(codeString)) 1319 return SUBSCRIPTION; 1320 if ("Substance".equals(codeString)) 1321 return SUBSTANCE; 1322 if ("SubstanceNucleicAcid".equals(codeString)) 1323 return SUBSTANCENUCLEICACID; 1324 if ("SubstancePolymer".equals(codeString)) 1325 return SUBSTANCEPOLYMER; 1326 if ("SubstanceProtein".equals(codeString)) 1327 return SUBSTANCEPROTEIN; 1328 if ("SubstanceReferenceInformation".equals(codeString)) 1329 return SUBSTANCEREFERENCEINFORMATION; 1330 if ("SubstanceSourceMaterial".equals(codeString)) 1331 return SUBSTANCESOURCEMATERIAL; 1332 if ("SubstanceSpecification".equals(codeString)) 1333 return SUBSTANCESPECIFICATION; 1334 if ("SupplyDelivery".equals(codeString)) 1335 return SUPPLYDELIVERY; 1336 if ("SupplyRequest".equals(codeString)) 1337 return SUPPLYREQUEST; 1338 if ("Task".equals(codeString)) 1339 return TASK; 1340 if ("TerminologyCapabilities".equals(codeString)) 1341 return TERMINOLOGYCAPABILITIES; 1342 if ("TestReport".equals(codeString)) 1343 return TESTREPORT; 1344 if ("TestScript".equals(codeString)) 1345 return TESTSCRIPT; 1346 if ("ValueSet".equals(codeString)) 1347 return VALUESET; 1348 if ("VerificationResult".equals(codeString)) 1349 return VERIFICATIONRESULT; 1350 if ("VisionPrescription".equals(codeString)) 1351 return VISIONPRESCRIPTION; 1352 if (Configuration.isAcceptInvalidEnums()) 1353 return null; 1354 else 1355 throw new FHIRException("Unknown FHIRResourceType code '" + codeString + "'"); 1356 } 1357 1358 public String toCode() { 1359 switch (this) { 1360 case ACCOUNT: 1361 return "Account"; 1362 case ACTIVITYDEFINITION: 1363 return "ActivityDefinition"; 1364 case ADVERSEEVENT: 1365 return "AdverseEvent"; 1366 case ALLERGYINTOLERANCE: 1367 return "AllergyIntolerance"; 1368 case APPOINTMENT: 1369 return "Appointment"; 1370 case APPOINTMENTRESPONSE: 1371 return "AppointmentResponse"; 1372 case AUDITEVENT: 1373 return "AuditEvent"; 1374 case BASIC: 1375 return "Basic"; 1376 case BINARY: 1377 return "Binary"; 1378 case BIOLOGICALLYDERIVEDPRODUCT: 1379 return "BiologicallyDerivedProduct"; 1380 case BODYSTRUCTURE: 1381 return "BodyStructure"; 1382 case BUNDLE: 1383 return "Bundle"; 1384 case CAPABILITYSTATEMENT: 1385 return "CapabilityStatement"; 1386 case CAREPLAN: 1387 return "CarePlan"; 1388 case CARETEAM: 1389 return "CareTeam"; 1390 case CATALOGENTRY: 1391 return "CatalogEntry"; 1392 case CHARGEITEM: 1393 return "ChargeItem"; 1394 case CHARGEITEMDEFINITION: 1395 return "ChargeItemDefinition"; 1396 case CLAIM: 1397 return "Claim"; 1398 case CLAIMRESPONSE: 1399 return "ClaimResponse"; 1400 case CLINICALIMPRESSION: 1401 return "ClinicalImpression"; 1402 case CODESYSTEM: 1403 return "CodeSystem"; 1404 case COMMUNICATION: 1405 return "Communication"; 1406 case COMMUNICATIONREQUEST: 1407 return "CommunicationRequest"; 1408 case COMPARTMENTDEFINITION: 1409 return "CompartmentDefinition"; 1410 case COMPOSITION: 1411 return "Composition"; 1412 case CONCEPTMAP: 1413 return "ConceptMap"; 1414 case CONDITION: 1415 return "Condition"; 1416 case CONSENT: 1417 return "Consent"; 1418 case CONTRACT: 1419 return "Contract"; 1420 case COVERAGE: 1421 return "Coverage"; 1422 case COVERAGEELIGIBILITYREQUEST: 1423 return "CoverageEligibilityRequest"; 1424 case COVERAGEELIGIBILITYRESPONSE: 1425 return "CoverageEligibilityResponse"; 1426 case DETECTEDISSUE: 1427 return "DetectedIssue"; 1428 case DEVICE: 1429 return "Device"; 1430 case DEVICEDEFINITION: 1431 return "DeviceDefinition"; 1432 case DEVICEMETRIC: 1433 return "DeviceMetric"; 1434 case DEVICEREQUEST: 1435 return "DeviceRequest"; 1436 case DEVICEUSESTATEMENT: 1437 return "DeviceUseStatement"; 1438 case DIAGNOSTICREPORT: 1439 return "DiagnosticReport"; 1440 case DOCUMENTMANIFEST: 1441 return "DocumentManifest"; 1442 case DOCUMENTREFERENCE: 1443 return "DocumentReference"; 1444 case DOMAINRESOURCE: 1445 return "DomainResource"; 1446 case EFFECTEVIDENCESYNTHESIS: 1447 return "EffectEvidenceSynthesis"; 1448 case ENCOUNTER: 1449 return "Encounter"; 1450 case ENDPOINT: 1451 return "Endpoint"; 1452 case ENROLLMENTREQUEST: 1453 return "EnrollmentRequest"; 1454 case ENROLLMENTRESPONSE: 1455 return "EnrollmentResponse"; 1456 case EPISODEOFCARE: 1457 return "EpisodeOfCare"; 1458 case EVENTDEFINITION: 1459 return "EventDefinition"; 1460 case EVIDENCE: 1461 return "Evidence"; 1462 case EVIDENCEVARIABLE: 1463 return "EvidenceVariable"; 1464 case EXAMPLESCENARIO: 1465 return "ExampleScenario"; 1466 case EXPLANATIONOFBENEFIT: 1467 return "ExplanationOfBenefit"; 1468 case FAMILYMEMBERHISTORY: 1469 return "FamilyMemberHistory"; 1470 case FLAG: 1471 return "Flag"; 1472 case GOAL: 1473 return "Goal"; 1474 case GRAPHDEFINITION: 1475 return "GraphDefinition"; 1476 case GROUP: 1477 return "Group"; 1478 case GUIDANCERESPONSE: 1479 return "GuidanceResponse"; 1480 case HEALTHCARESERVICE: 1481 return "HealthcareService"; 1482 case IMAGINGSTUDY: 1483 return "ImagingStudy"; 1484 case IMMUNIZATION: 1485 return "Immunization"; 1486 case IMMUNIZATIONEVALUATION: 1487 return "ImmunizationEvaluation"; 1488 case IMMUNIZATIONRECOMMENDATION: 1489 return "ImmunizationRecommendation"; 1490 case IMPLEMENTATIONGUIDE: 1491 return "ImplementationGuide"; 1492 case INSURANCEPLAN: 1493 return "InsurancePlan"; 1494 case INVOICE: 1495 return "Invoice"; 1496 case LIBRARY: 1497 return "Library"; 1498 case LINKAGE: 1499 return "Linkage"; 1500 case LIST: 1501 return "List"; 1502 case LOCATION: 1503 return "Location"; 1504 case MEASURE: 1505 return "Measure"; 1506 case MEASUREREPORT: 1507 return "MeasureReport"; 1508 case MEDIA: 1509 return "Media"; 1510 case MEDICATION: 1511 return "Medication"; 1512 case MEDICATIONADMINISTRATION: 1513 return "MedicationAdministration"; 1514 case MEDICATIONDISPENSE: 1515 return "MedicationDispense"; 1516 case MEDICATIONKNOWLEDGE: 1517 return "MedicationKnowledge"; 1518 case MEDICATIONREQUEST: 1519 return "MedicationRequest"; 1520 case MEDICATIONSTATEMENT: 1521 return "MedicationStatement"; 1522 case MEDICINALPRODUCT: 1523 return "MedicinalProduct"; 1524 case MEDICINALPRODUCTAUTHORIZATION: 1525 return "MedicinalProductAuthorization"; 1526 case MEDICINALPRODUCTCONTRAINDICATION: 1527 return "MedicinalProductContraindication"; 1528 case MEDICINALPRODUCTINDICATION: 1529 return "MedicinalProductIndication"; 1530 case MEDICINALPRODUCTINGREDIENT: 1531 return "MedicinalProductIngredient"; 1532 case MEDICINALPRODUCTINTERACTION: 1533 return "MedicinalProductInteraction"; 1534 case MEDICINALPRODUCTMANUFACTURED: 1535 return "MedicinalProductManufactured"; 1536 case MEDICINALPRODUCTPACKAGED: 1537 return "MedicinalProductPackaged"; 1538 case MEDICINALPRODUCTPHARMACEUTICAL: 1539 return "MedicinalProductPharmaceutical"; 1540 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 1541 return "MedicinalProductUndesirableEffect"; 1542 case MESSAGEDEFINITION: 1543 return "MessageDefinition"; 1544 case MESSAGEHEADER: 1545 return "MessageHeader"; 1546 case MOLECULARSEQUENCE: 1547 return "MolecularSequence"; 1548 case NAMINGSYSTEM: 1549 return "NamingSystem"; 1550 case NUTRITIONORDER: 1551 return "NutritionOrder"; 1552 case OBSERVATION: 1553 return "Observation"; 1554 case OBSERVATIONDEFINITION: 1555 return "ObservationDefinition"; 1556 case OPERATIONDEFINITION: 1557 return "OperationDefinition"; 1558 case OPERATIONOUTCOME: 1559 return "OperationOutcome"; 1560 case ORGANIZATION: 1561 return "Organization"; 1562 case ORGANIZATIONAFFILIATION: 1563 return "OrganizationAffiliation"; 1564 case PARAMETERS: 1565 return "Parameters"; 1566 case PATIENT: 1567 return "Patient"; 1568 case PAYMENTNOTICE: 1569 return "PaymentNotice"; 1570 case PAYMENTRECONCILIATION: 1571 return "PaymentReconciliation"; 1572 case PERSON: 1573 return "Person"; 1574 case PLANDEFINITION: 1575 return "PlanDefinition"; 1576 case PRACTITIONER: 1577 return "Practitioner"; 1578 case PRACTITIONERROLE: 1579 return "PractitionerRole"; 1580 case PROCEDURE: 1581 return "Procedure"; 1582 case PROVENANCE: 1583 return "Provenance"; 1584 case QUESTIONNAIRE: 1585 return "Questionnaire"; 1586 case QUESTIONNAIRERESPONSE: 1587 return "QuestionnaireResponse"; 1588 case RELATEDPERSON: 1589 return "RelatedPerson"; 1590 case REQUESTGROUP: 1591 return "RequestGroup"; 1592 case RESEARCHDEFINITION: 1593 return "ResearchDefinition"; 1594 case RESEARCHELEMENTDEFINITION: 1595 return "ResearchElementDefinition"; 1596 case RESEARCHSTUDY: 1597 return "ResearchStudy"; 1598 case RESEARCHSUBJECT: 1599 return "ResearchSubject"; 1600 case RESOURCE: 1601 return "Resource"; 1602 case RISKASSESSMENT: 1603 return "RiskAssessment"; 1604 case RISKEVIDENCESYNTHESIS: 1605 return "RiskEvidenceSynthesis"; 1606 case SCHEDULE: 1607 return "Schedule"; 1608 case SEARCHPARAMETER: 1609 return "SearchParameter"; 1610 case SERVICEREQUEST: 1611 return "ServiceRequest"; 1612 case SLOT: 1613 return "Slot"; 1614 case SPECIMEN: 1615 return "Specimen"; 1616 case SPECIMENDEFINITION: 1617 return "SpecimenDefinition"; 1618 case STRUCTUREDEFINITION: 1619 return "StructureDefinition"; 1620 case STRUCTUREMAP: 1621 return "StructureMap"; 1622 case SUBSCRIPTION: 1623 return "Subscription"; 1624 case SUBSTANCE: 1625 return "Substance"; 1626 case SUBSTANCENUCLEICACID: 1627 return "SubstanceNucleicAcid"; 1628 case SUBSTANCEPOLYMER: 1629 return "SubstancePolymer"; 1630 case SUBSTANCEPROTEIN: 1631 return "SubstanceProtein"; 1632 case SUBSTANCEREFERENCEINFORMATION: 1633 return "SubstanceReferenceInformation"; 1634 case SUBSTANCESOURCEMATERIAL: 1635 return "SubstanceSourceMaterial"; 1636 case SUBSTANCESPECIFICATION: 1637 return "SubstanceSpecification"; 1638 case SUPPLYDELIVERY: 1639 return "SupplyDelivery"; 1640 case SUPPLYREQUEST: 1641 return "SupplyRequest"; 1642 case TASK: 1643 return "Task"; 1644 case TERMINOLOGYCAPABILITIES: 1645 return "TerminologyCapabilities"; 1646 case TESTREPORT: 1647 return "TestReport"; 1648 case TESTSCRIPT: 1649 return "TestScript"; 1650 case VALUESET: 1651 return "ValueSet"; 1652 case VERIFICATIONRESULT: 1653 return "VerificationResult"; 1654 case VISIONPRESCRIPTION: 1655 return "VisionPrescription"; 1656 case NULL: 1657 return null; 1658 default: 1659 return "?"; 1660 } 1661 } 1662 1663 public String getSystem() { 1664 switch (this) { 1665 case ACCOUNT: 1666 return "http://hl7.org/fhir/resource-types"; 1667 case ACTIVITYDEFINITION: 1668 return "http://hl7.org/fhir/resource-types"; 1669 case ADVERSEEVENT: 1670 return "http://hl7.org/fhir/resource-types"; 1671 case ALLERGYINTOLERANCE: 1672 return "http://hl7.org/fhir/resource-types"; 1673 case APPOINTMENT: 1674 return "http://hl7.org/fhir/resource-types"; 1675 case APPOINTMENTRESPONSE: 1676 return "http://hl7.org/fhir/resource-types"; 1677 case AUDITEVENT: 1678 return "http://hl7.org/fhir/resource-types"; 1679 case BASIC: 1680 return "http://hl7.org/fhir/resource-types"; 1681 case BINARY: 1682 return "http://hl7.org/fhir/resource-types"; 1683 case BIOLOGICALLYDERIVEDPRODUCT: 1684 return "http://hl7.org/fhir/resource-types"; 1685 case BODYSTRUCTURE: 1686 return "http://hl7.org/fhir/resource-types"; 1687 case BUNDLE: 1688 return "http://hl7.org/fhir/resource-types"; 1689 case CAPABILITYSTATEMENT: 1690 return "http://hl7.org/fhir/resource-types"; 1691 case CAREPLAN: 1692 return "http://hl7.org/fhir/resource-types"; 1693 case CARETEAM: 1694 return "http://hl7.org/fhir/resource-types"; 1695 case CATALOGENTRY: 1696 return "http://hl7.org/fhir/resource-types"; 1697 case CHARGEITEM: 1698 return "http://hl7.org/fhir/resource-types"; 1699 case CHARGEITEMDEFINITION: 1700 return "http://hl7.org/fhir/resource-types"; 1701 case CLAIM: 1702 return "http://hl7.org/fhir/resource-types"; 1703 case CLAIMRESPONSE: 1704 return "http://hl7.org/fhir/resource-types"; 1705 case CLINICALIMPRESSION: 1706 return "http://hl7.org/fhir/resource-types"; 1707 case CODESYSTEM: 1708 return "http://hl7.org/fhir/resource-types"; 1709 case COMMUNICATION: 1710 return "http://hl7.org/fhir/resource-types"; 1711 case COMMUNICATIONREQUEST: 1712 return "http://hl7.org/fhir/resource-types"; 1713 case COMPARTMENTDEFINITION: 1714 return "http://hl7.org/fhir/resource-types"; 1715 case COMPOSITION: 1716 return "http://hl7.org/fhir/resource-types"; 1717 case CONCEPTMAP: 1718 return "http://hl7.org/fhir/resource-types"; 1719 case CONDITION: 1720 return "http://hl7.org/fhir/resource-types"; 1721 case CONSENT: 1722 return "http://hl7.org/fhir/resource-types"; 1723 case CONTRACT: 1724 return "http://hl7.org/fhir/resource-types"; 1725 case COVERAGE: 1726 return "http://hl7.org/fhir/resource-types"; 1727 case COVERAGEELIGIBILITYREQUEST: 1728 return "http://hl7.org/fhir/resource-types"; 1729 case COVERAGEELIGIBILITYRESPONSE: 1730 return "http://hl7.org/fhir/resource-types"; 1731 case DETECTEDISSUE: 1732 return "http://hl7.org/fhir/resource-types"; 1733 case DEVICE: 1734 return "http://hl7.org/fhir/resource-types"; 1735 case DEVICEDEFINITION: 1736 return "http://hl7.org/fhir/resource-types"; 1737 case DEVICEMETRIC: 1738 return "http://hl7.org/fhir/resource-types"; 1739 case DEVICEREQUEST: 1740 return "http://hl7.org/fhir/resource-types"; 1741 case DEVICEUSESTATEMENT: 1742 return "http://hl7.org/fhir/resource-types"; 1743 case DIAGNOSTICREPORT: 1744 return "http://hl7.org/fhir/resource-types"; 1745 case DOCUMENTMANIFEST: 1746 return "http://hl7.org/fhir/resource-types"; 1747 case DOCUMENTREFERENCE: 1748 return "http://hl7.org/fhir/resource-types"; 1749 case DOMAINRESOURCE: 1750 return "http://hl7.org/fhir/resource-types"; 1751 case EFFECTEVIDENCESYNTHESIS: 1752 return "http://hl7.org/fhir/resource-types"; 1753 case ENCOUNTER: 1754 return "http://hl7.org/fhir/resource-types"; 1755 case ENDPOINT: 1756 return "http://hl7.org/fhir/resource-types"; 1757 case ENROLLMENTREQUEST: 1758 return "http://hl7.org/fhir/resource-types"; 1759 case ENROLLMENTRESPONSE: 1760 return "http://hl7.org/fhir/resource-types"; 1761 case EPISODEOFCARE: 1762 return "http://hl7.org/fhir/resource-types"; 1763 case EVENTDEFINITION: 1764 return "http://hl7.org/fhir/resource-types"; 1765 case EVIDENCE: 1766 return "http://hl7.org/fhir/resource-types"; 1767 case EVIDENCEVARIABLE: 1768 return "http://hl7.org/fhir/resource-types"; 1769 case EXAMPLESCENARIO: 1770 return "http://hl7.org/fhir/resource-types"; 1771 case EXPLANATIONOFBENEFIT: 1772 return "http://hl7.org/fhir/resource-types"; 1773 case FAMILYMEMBERHISTORY: 1774 return "http://hl7.org/fhir/resource-types"; 1775 case FLAG: 1776 return "http://hl7.org/fhir/resource-types"; 1777 case GOAL: 1778 return "http://hl7.org/fhir/resource-types"; 1779 case GRAPHDEFINITION: 1780 return "http://hl7.org/fhir/resource-types"; 1781 case GROUP: 1782 return "http://hl7.org/fhir/resource-types"; 1783 case GUIDANCERESPONSE: 1784 return "http://hl7.org/fhir/resource-types"; 1785 case HEALTHCARESERVICE: 1786 return "http://hl7.org/fhir/resource-types"; 1787 case IMAGINGSTUDY: 1788 return "http://hl7.org/fhir/resource-types"; 1789 case IMMUNIZATION: 1790 return "http://hl7.org/fhir/resource-types"; 1791 case IMMUNIZATIONEVALUATION: 1792 return "http://hl7.org/fhir/resource-types"; 1793 case IMMUNIZATIONRECOMMENDATION: 1794 return "http://hl7.org/fhir/resource-types"; 1795 case IMPLEMENTATIONGUIDE: 1796 return "http://hl7.org/fhir/resource-types"; 1797 case INSURANCEPLAN: 1798 return "http://hl7.org/fhir/resource-types"; 1799 case INVOICE: 1800 return "http://hl7.org/fhir/resource-types"; 1801 case LIBRARY: 1802 return "http://hl7.org/fhir/resource-types"; 1803 case LINKAGE: 1804 return "http://hl7.org/fhir/resource-types"; 1805 case LIST: 1806 return "http://hl7.org/fhir/resource-types"; 1807 case LOCATION: 1808 return "http://hl7.org/fhir/resource-types"; 1809 case MEASURE: 1810 return "http://hl7.org/fhir/resource-types"; 1811 case MEASUREREPORT: 1812 return "http://hl7.org/fhir/resource-types"; 1813 case MEDIA: 1814 return "http://hl7.org/fhir/resource-types"; 1815 case MEDICATION: 1816 return "http://hl7.org/fhir/resource-types"; 1817 case MEDICATIONADMINISTRATION: 1818 return "http://hl7.org/fhir/resource-types"; 1819 case MEDICATIONDISPENSE: 1820 return "http://hl7.org/fhir/resource-types"; 1821 case MEDICATIONKNOWLEDGE: 1822 return "http://hl7.org/fhir/resource-types"; 1823 case MEDICATIONREQUEST: 1824 return "http://hl7.org/fhir/resource-types"; 1825 case MEDICATIONSTATEMENT: 1826 return "http://hl7.org/fhir/resource-types"; 1827 case MEDICINALPRODUCT: 1828 return "http://hl7.org/fhir/resource-types"; 1829 case MEDICINALPRODUCTAUTHORIZATION: 1830 return "http://hl7.org/fhir/resource-types"; 1831 case MEDICINALPRODUCTCONTRAINDICATION: 1832 return "http://hl7.org/fhir/resource-types"; 1833 case MEDICINALPRODUCTINDICATION: 1834 return "http://hl7.org/fhir/resource-types"; 1835 case MEDICINALPRODUCTINGREDIENT: 1836 return "http://hl7.org/fhir/resource-types"; 1837 case MEDICINALPRODUCTINTERACTION: 1838 return "http://hl7.org/fhir/resource-types"; 1839 case MEDICINALPRODUCTMANUFACTURED: 1840 return "http://hl7.org/fhir/resource-types"; 1841 case MEDICINALPRODUCTPACKAGED: 1842 return "http://hl7.org/fhir/resource-types"; 1843 case MEDICINALPRODUCTPHARMACEUTICAL: 1844 return "http://hl7.org/fhir/resource-types"; 1845 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 1846 return "http://hl7.org/fhir/resource-types"; 1847 case MESSAGEDEFINITION: 1848 return "http://hl7.org/fhir/resource-types"; 1849 case MESSAGEHEADER: 1850 return "http://hl7.org/fhir/resource-types"; 1851 case MOLECULARSEQUENCE: 1852 return "http://hl7.org/fhir/resource-types"; 1853 case NAMINGSYSTEM: 1854 return "http://hl7.org/fhir/resource-types"; 1855 case NUTRITIONORDER: 1856 return "http://hl7.org/fhir/resource-types"; 1857 case OBSERVATION: 1858 return "http://hl7.org/fhir/resource-types"; 1859 case OBSERVATIONDEFINITION: 1860 return "http://hl7.org/fhir/resource-types"; 1861 case OPERATIONDEFINITION: 1862 return "http://hl7.org/fhir/resource-types"; 1863 case OPERATIONOUTCOME: 1864 return "http://hl7.org/fhir/resource-types"; 1865 case ORGANIZATION: 1866 return "http://hl7.org/fhir/resource-types"; 1867 case ORGANIZATIONAFFILIATION: 1868 return "http://hl7.org/fhir/resource-types"; 1869 case PARAMETERS: 1870 return "http://hl7.org/fhir/resource-types"; 1871 case PATIENT: 1872 return "http://hl7.org/fhir/resource-types"; 1873 case PAYMENTNOTICE: 1874 return "http://hl7.org/fhir/resource-types"; 1875 case PAYMENTRECONCILIATION: 1876 return "http://hl7.org/fhir/resource-types"; 1877 case PERSON: 1878 return "http://hl7.org/fhir/resource-types"; 1879 case PLANDEFINITION: 1880 return "http://hl7.org/fhir/resource-types"; 1881 case PRACTITIONER: 1882 return "http://hl7.org/fhir/resource-types"; 1883 case PRACTITIONERROLE: 1884 return "http://hl7.org/fhir/resource-types"; 1885 case PROCEDURE: 1886 return "http://hl7.org/fhir/resource-types"; 1887 case PROVENANCE: 1888 return "http://hl7.org/fhir/resource-types"; 1889 case QUESTIONNAIRE: 1890 return "http://hl7.org/fhir/resource-types"; 1891 case QUESTIONNAIRERESPONSE: 1892 return "http://hl7.org/fhir/resource-types"; 1893 case RELATEDPERSON: 1894 return "http://hl7.org/fhir/resource-types"; 1895 case REQUESTGROUP: 1896 return "http://hl7.org/fhir/resource-types"; 1897 case RESEARCHDEFINITION: 1898 return "http://hl7.org/fhir/resource-types"; 1899 case RESEARCHELEMENTDEFINITION: 1900 return "http://hl7.org/fhir/resource-types"; 1901 case RESEARCHSTUDY: 1902 return "http://hl7.org/fhir/resource-types"; 1903 case RESEARCHSUBJECT: 1904 return "http://hl7.org/fhir/resource-types"; 1905 case RESOURCE: 1906 return "http://hl7.org/fhir/resource-types"; 1907 case RISKASSESSMENT: 1908 return "http://hl7.org/fhir/resource-types"; 1909 case RISKEVIDENCESYNTHESIS: 1910 return "http://hl7.org/fhir/resource-types"; 1911 case SCHEDULE: 1912 return "http://hl7.org/fhir/resource-types"; 1913 case SEARCHPARAMETER: 1914 return "http://hl7.org/fhir/resource-types"; 1915 case SERVICEREQUEST: 1916 return "http://hl7.org/fhir/resource-types"; 1917 case SLOT: 1918 return "http://hl7.org/fhir/resource-types"; 1919 case SPECIMEN: 1920 return "http://hl7.org/fhir/resource-types"; 1921 case SPECIMENDEFINITION: 1922 return "http://hl7.org/fhir/resource-types"; 1923 case STRUCTUREDEFINITION: 1924 return "http://hl7.org/fhir/resource-types"; 1925 case STRUCTUREMAP: 1926 return "http://hl7.org/fhir/resource-types"; 1927 case SUBSCRIPTION: 1928 return "http://hl7.org/fhir/resource-types"; 1929 case SUBSTANCE: 1930 return "http://hl7.org/fhir/resource-types"; 1931 case SUBSTANCENUCLEICACID: 1932 return "http://hl7.org/fhir/resource-types"; 1933 case SUBSTANCEPOLYMER: 1934 return "http://hl7.org/fhir/resource-types"; 1935 case SUBSTANCEPROTEIN: 1936 return "http://hl7.org/fhir/resource-types"; 1937 case SUBSTANCEREFERENCEINFORMATION: 1938 return "http://hl7.org/fhir/resource-types"; 1939 case SUBSTANCESOURCEMATERIAL: 1940 return "http://hl7.org/fhir/resource-types"; 1941 case SUBSTANCESPECIFICATION: 1942 return "http://hl7.org/fhir/resource-types"; 1943 case SUPPLYDELIVERY: 1944 return "http://hl7.org/fhir/resource-types"; 1945 case SUPPLYREQUEST: 1946 return "http://hl7.org/fhir/resource-types"; 1947 case TASK: 1948 return "http://hl7.org/fhir/resource-types"; 1949 case TERMINOLOGYCAPABILITIES: 1950 return "http://hl7.org/fhir/resource-types"; 1951 case TESTREPORT: 1952 return "http://hl7.org/fhir/resource-types"; 1953 case TESTSCRIPT: 1954 return "http://hl7.org/fhir/resource-types"; 1955 case VALUESET: 1956 return "http://hl7.org/fhir/resource-types"; 1957 case VERIFICATIONRESULT: 1958 return "http://hl7.org/fhir/resource-types"; 1959 case VISIONPRESCRIPTION: 1960 return "http://hl7.org/fhir/resource-types"; 1961 case NULL: 1962 return null; 1963 default: 1964 return "?"; 1965 } 1966 } 1967 1968 public String getDefinition() { 1969 switch (this) { 1970 case ACCOUNT: 1971 return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 1972 case ACTIVITYDEFINITION: 1973 return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 1974 case ADVERSEEVENT: 1975 return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 1976 case ALLERGYINTOLERANCE: 1977 return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 1978 case APPOINTMENT: 1979 return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 1980 case APPOINTMENTRESPONSE: 1981 return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 1982 case AUDITEVENT: 1983 return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 1984 case BASIC: 1985 return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 1986 case BINARY: 1987 return "A resource that represents the data of a single raw artifact as digital content accessible in its native format. A Binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 1988 case BIOLOGICALLYDERIVEDPRODUCT: 1989 return "A material substance originating from a biological entity intended to be transplanted or infused\ninto another (possibly the same) biological entity."; 1990 case BODYSTRUCTURE: 1991 return "Record details about an anatomical structure. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 1992 case BUNDLE: 1993 return "A container for a collection of resources."; 1994 case CAPABILITYSTATEMENT: 1995 return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server for a particular version of FHIR that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 1996 case CAREPLAN: 1997 return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 1998 case CARETEAM: 1999 return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 2000 case CATALOGENTRY: 2001 return "Catalog entries are wrappers that contextualize items included in a catalog."; 2002 case CHARGEITEM: 2003 return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 2004 case CHARGEITEMDEFINITION: 2005 return "The ChargeItemDefinition resource provides the properties that apply to the (billing) codes necessary to calculate costs and prices. The properties may differ largely depending on type and realm, therefore this resource gives only a rough structure and requires profiling for each type of billing code system."; 2006 case CLAIM: 2007 return "A provider issued list of professional services and products which have been provided, or are to be provided, to a patient which is sent to an insurer for reimbursement."; 2008 case CLAIMRESPONSE: 2009 return "This resource provides the adjudication details from the processing of a Claim resource."; 2010 case CLINICALIMPRESSION: 2011 return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 2012 case CODESYSTEM: 2013 return "The CodeSystem resource is used to declare the existence of and describe a code system or code system supplement and its key properties, and optionally define a part or all of its content."; 2014 case COMMUNICATION: 2015 return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency that was notified about a reportable condition."; 2016 case COMMUNICATIONREQUEST: 2017 return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 2018 case COMPARTMENTDEFINITION: 2019 return "A compartment definition that defines how resources are accessed on a server."; 2020 case COMPOSITION: 2021 return "A set of healthcare-related information that is assembled together into a single logical package that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. A Composition defines the structure and narrative content necessary for a document. However, a Composition alone does not constitute a document. Rather, the Composition must be the first entry in a Bundle where Bundle.type=document, and any other resources referenced from Composition must be included as subsequent entries in the Bundle (for example Patient, Practitioner, Encounter, etc.)."; 2022 case CONCEPTMAP: 2023 return "A statement of relationships from one set of concepts to one or more other concepts - either concepts in code systems, or data element/data element concepts, or classes in class models."; 2024 case CONDITION: 2025 return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 2026 case CONSENT: 2027 return "A record of a healthcare consumer?s choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 2028 case CONTRACT: 2029 return "Legally enforceable, formally recorded unilateral or bilateral directive i.e., a policy or agreement."; 2030 case COVERAGE: 2031 return "Financial instrument which may be used to reimburse or pay for health care products and services. Includes both insurance and self-payment."; 2032 case COVERAGEELIGIBILITYREQUEST: 2033 return "The CoverageEligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an CoverageEligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 2034 case COVERAGEELIGIBILITYRESPONSE: 2035 return "This resource provides eligibility and plan details from the processing of an CoverageEligibilityRequest resource."; 2036 case DETECTEDISSUE: 2037 return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 2038 case DEVICE: 2039 return "A type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device."; 2040 case DEVICEDEFINITION: 2041 return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 2042 case DEVICEMETRIC: 2043 return "Describes a measurement, calculation or setting capability of a medical device."; 2044 case DEVICEREQUEST: 2045 return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 2046 case DEVICEUSESTATEMENT: 2047 return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 2048 case DIAGNOSTICREPORT: 2049 return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 2050 case DOCUMENTMANIFEST: 2051 return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 2052 case DOCUMENTREFERENCE: 2053 return "A reference to a document of any kind for any purpose. Provides metadata about the document so that the document can be discovered and managed. The scope of a document is any seralized object with a mime-type, so includes formal patient centric documents (CDA), cliical notes, scanned paper, and non-patient specific documents like policy text."; 2054 case DOMAINRESOURCE: 2055 return "A resource that includes narrative, extensions, and contained resources."; 2056 case EFFECTEVIDENCESYNTHESIS: 2057 return "The EffectEvidenceSynthesis resource describes the difference in an outcome between exposures states in a population where the effect estimate is derived from a combination of research studies."; 2058 case ENCOUNTER: 2059 return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 2060 case ENDPOINT: 2061 return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 2062 case ENROLLMENTREQUEST: 2063 return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 2064 case ENROLLMENTRESPONSE: 2065 return "This resource provides enrollment and plan details from the processing of an EnrollmentRequest resource."; 2066 case EPISODEOFCARE: 2067 return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 2068 case EVENTDEFINITION: 2069 return "The EventDefinition resource provides a reusable description of when a particular event can occur."; 2070 case EVIDENCE: 2071 return "The Evidence resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 2072 case EVIDENCEVARIABLE: 2073 return "The EvidenceVariable resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 2074 case EXAMPLESCENARIO: 2075 return "Example of workflow instance."; 2076 case EXPLANATIONOFBENEFIT: 2077 return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 2078 case FAMILYMEMBERHISTORY: 2079 return "Significant health conditions for a person related to the patient relevant in the context of care for the patient."; 2080 case FLAG: 2081 return "Prospective warnings of potential issues when providing care to the patient."; 2082 case GOAL: 2083 return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 2084 case GRAPHDEFINITION: 2085 return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 2086 case GROUP: 2087 return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 2088 case GUIDANCERESPONSE: 2089 return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 2090 case HEALTHCARESERVICE: 2091 return "The details of a healthcare service available at a location."; 2092 case IMAGINGSTUDY: 2093 return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 2094 case IMMUNIZATION: 2095 return "Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party."; 2096 case IMMUNIZATIONEVALUATION: 2097 return "Describes a comparison of an immunization event against published recommendations to determine if the administration is \"valid\" in relation to those recommendations."; 2098 case IMMUNIZATIONRECOMMENDATION: 2099 return "A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification."; 2100 case IMPLEMENTATIONGUIDE: 2101 return "A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 2102 case INSURANCEPLAN: 2103 return "Details of a Health Insurance product/plan provided by an organization."; 2104 case INVOICE: 2105 return "Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose."; 2106 case LIBRARY: 2107 return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 2108 case LINKAGE: 2109 return "Identifies two or more records (resource instances) that refer to the same real-world \"occurrence\"."; 2110 case LIST: 2111 return "A list is a curated collection of resources."; 2112 case LOCATION: 2113 return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained, or accommodated."; 2114 case MEASURE: 2115 return "The Measure resource provides the definition of a quality measure."; 2116 case MEASUREREPORT: 2117 return "The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation."; 2118 case MEDIA: 2119 return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 2120 case MEDICATION: 2121 return "This resource is primarily used for the identification and definition of a medication for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use."; 2122 case MEDICATIONADMINISTRATION: 2123 return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 2124 case MEDICATIONDISPENSE: 2125 return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 2126 case MEDICATIONKNOWLEDGE: 2127 return "Information about a medication that is used to support knowledge."; 2128 case MEDICATIONREQUEST: 2129 return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 2130 case MEDICATIONSTATEMENT: 2131 return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains. \n\nThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 2132 case MEDICINALPRODUCT: 2133 return "Detailed definition of a medicinal product, typically for uses other than direct patient care (e.g. regulatory use)."; 2134 case MEDICINALPRODUCTAUTHORIZATION: 2135 return "The regulatory authorization of a medicinal product."; 2136 case MEDICINALPRODUCTCONTRAINDICATION: 2137 return "The clinical particulars - indications, contraindications etc. of a medicinal product, including for regulatory purposes."; 2138 case MEDICINALPRODUCTINDICATION: 2139 return "Indication for the Medicinal Product."; 2140 case MEDICINALPRODUCTINGREDIENT: 2141 return "An ingredient of a manufactured item or pharmaceutical product."; 2142 case MEDICINALPRODUCTINTERACTION: 2143 return "The interactions of the medicinal product with other medicinal products, or other forms of interactions."; 2144 case MEDICINALPRODUCTMANUFACTURED: 2145 return "The manufactured item as contained in the packaged medicinal product."; 2146 case MEDICINALPRODUCTPACKAGED: 2147 return "A medicinal product in a container or package."; 2148 case MEDICINALPRODUCTPHARMACEUTICAL: 2149 return "A pharmaceutical product described in terms of its composition and dose form."; 2150 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 2151 return "Describe the undesirable effects of the medicinal product."; 2152 case MESSAGEDEFINITION: 2153 return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 2154 case MESSAGEHEADER: 2155 return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 2156 case MOLECULARSEQUENCE: 2157 return "Raw data describing a biological sequence."; 2158 case NAMINGSYSTEM: 2159 return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 2160 case NUTRITIONORDER: 2161 return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 2162 case OBSERVATION: 2163 return "Measurements and simple assertions made about a patient, device or other subject."; 2164 case OBSERVATIONDEFINITION: 2165 return "Set of definitional characteristics for a kind of observation or measurement produced or consumed by an orderable health care service."; 2166 case OPERATIONDEFINITION: 2167 return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 2168 case OPERATIONOUTCOME: 2169 return "A collection of error, warning, or information messages that result from a system action."; 2170 case ORGANIZATION: 2171 return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, payer/insurer, etc."; 2172 case ORGANIZATIONAFFILIATION: 2173 return "Defines an affiliation/assotiation/relationship between 2 distinct oganizations, that is not a part-of relationship/sub-division relationship."; 2174 case PARAMETERS: 2175 return "This resource is a non-persisted resource used to pass information into and back from an [operation](operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 2176 case PATIENT: 2177 return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 2178 case PAYMENTNOTICE: 2179 return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 2180 case PAYMENTRECONCILIATION: 2181 return "This resource provides the details including amount of a payment and allocates the payment items being paid."; 2182 case PERSON: 2183 return "Demographics and administrative information about a person independent of a specific health-related context."; 2184 case PLANDEFINITION: 2185 return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 2186 case PRACTITIONER: 2187 return "A person who is directly or indirectly involved in the provisioning of healthcare."; 2188 case PRACTITIONERROLE: 2189 return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 2190 case PROCEDURE: 2191 return "An action that is or was performed on or for a patient. This can be a physical intervention like an operation, or less invasive like long term services, counseling, or hypnotherapy."; 2192 case PROVENANCE: 2193 return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 2194 case QUESTIONNAIRE: 2195 return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 2196 case QUESTIONNAIRERESPONSE: 2197 return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 2198 case RELATEDPERSON: 2199 return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 2200 case REQUESTGROUP: 2201 return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 2202 case RESEARCHDEFINITION: 2203 return "The ResearchDefinition resource describes the conditional state (population and any exposures being compared within the population) and outcome (if specified) that the knowledge (evidence, assertion, recommendation) is about."; 2204 case RESEARCHELEMENTDEFINITION: 2205 return "The ResearchElementDefinition resource describes a \"PICO\" element that knowledge (evidence, assertion, recommendation) is about."; 2206 case RESEARCHSTUDY: 2207 return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 2208 case RESEARCHSUBJECT: 2209 return "A physical entity which is the primary unit of operational and/or administrative interest in a study."; 2210 case RESOURCE: 2211 return "This is the base resource type for everything."; 2212 case RISKASSESSMENT: 2213 return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 2214 case RISKEVIDENCESYNTHESIS: 2215 return "The RiskEvidenceSynthesis resource describes the likelihood of an outcome in a population plus exposure state where the risk estimate is derived from a combination of research studies."; 2216 case SCHEDULE: 2217 return "A container for slots of time that may be available for booking appointments."; 2218 case SEARCHPARAMETER: 2219 return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 2220 case SERVICEREQUEST: 2221 return "A record of a request for service such as diagnostic investigations, treatments, or operations to be performed."; 2222 case SLOT: 2223 return "A slot of time on a schedule that may be available for booking appointments."; 2224 case SPECIMEN: 2225 return "A sample to be used for analysis."; 2226 case SPECIMENDEFINITION: 2227 return "A kind of specimen with associated set of requirements."; 2228 case STRUCTUREDEFINITION: 2229 return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 2230 case STRUCTUREMAP: 2231 return "A Map of relationships between 2 structures that can be used to transform data."; 2232 case SUBSCRIPTION: 2233 return "The subscription resource is used to define a push-based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system can take an appropriate action."; 2234 case SUBSTANCE: 2235 return "A homogeneous material with a definite composition."; 2236 case SUBSTANCENUCLEICACID: 2237 return "Nucleic acids are defined by three distinct elements: the base, sugar and linkage. Individual substance/moiety IDs will be created for each of these elements. The nucleotide sequence will be always entered in the 5?-3? direction."; 2238 case SUBSTANCEPOLYMER: 2239 return "Todo."; 2240 case SUBSTANCEPROTEIN: 2241 return "A SubstanceProtein is defined as a single unit of a linear amino acid sequence, or a combination of subunits that are either covalently linked or have a defined invariant stoichiometric relationship. This includes all synthetic, recombinant and purified SubstanceProteins of defined sequence, whether the use is therapeutic or prophylactic. This set of elements will be used to describe albumins, coagulation factors, cytokines, growth factors, peptide/SubstanceProtein hormones, enzymes, toxins, toxoids, recombinant vaccines, and immunomodulators."; 2242 case SUBSTANCEREFERENCEINFORMATION: 2243 return "Todo."; 2244 case SUBSTANCESOURCEMATERIAL: 2245 return "Source material shall capture information on the taxonomic and anatomical origins as well as the fraction of a material that can result in or can be modified to form a substance. This set of data elements shall be used to define polymer substances isolated from biological matrices. Taxonomic and anatomical origins shall be described using a controlled vocabulary as required. This information is captured for naturally derived polymers ( . starch) and structurally diverse substances. For Organisms belonging to the Kingdom Plantae the Substance level defines the fresh material of a single species or infraspecies, the Herbal Drug and the Herbal preparation. For Herbal preparations, the fraction information will be captured at the Substance information level and additional information for herbal extracts will be captured at the Specified Substance Group 1 information level. See for further explanation the Substance Class: Structurally Diverse and the herbal annex."; 2246 case SUBSTANCESPECIFICATION: 2247 return "The detailed description of a substance, typically at a level beyond what is used for prescribing."; 2248 case SUPPLYDELIVERY: 2249 return "Record of delivery of what is supplied."; 2250 case SUPPLYREQUEST: 2251 return "A record of a request for a medication, substance or device used in the healthcare setting."; 2252 case TASK: 2253 return "A task to be performed."; 2254 case TERMINOLOGYCAPABILITIES: 2255 return "A TerminologyCapabilities resource documents a set of capabilities (behaviors) of a FHIR Terminology Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 2256 case TESTREPORT: 2257 return "A summary of information based on the results of executing a TestScript."; 2258 case TESTSCRIPT: 2259 return "A structured set of tests against a FHIR server or client implementation to determine compliance against the FHIR specification."; 2260 case VALUESET: 2261 return "A ValueSet resource instance specifies a set of codes drawn from one or more code systems, intended for use in a particular context. Value sets link between [[[CodeSystem]]] definitions and their use in [coded elements](terminologies.html)."; 2262 case VERIFICATIONRESULT: 2263 return "Describes validation requirements, source(s), status and dates for one or more elements."; 2264 case VISIONPRESCRIPTION: 2265 return "An authorization for the provision of glasses and/or contact lenses to a patient."; 2266 case NULL: 2267 return null; 2268 default: 2269 return "?"; 2270 } 2271 } 2272 2273 public String getDisplay() { 2274 switch (this) { 2275 case ACCOUNT: 2276 return "Account"; 2277 case ACTIVITYDEFINITION: 2278 return "ActivityDefinition"; 2279 case ADVERSEEVENT: 2280 return "AdverseEvent"; 2281 case ALLERGYINTOLERANCE: 2282 return "AllergyIntolerance"; 2283 case APPOINTMENT: 2284 return "Appointment"; 2285 case APPOINTMENTRESPONSE: 2286 return "AppointmentResponse"; 2287 case AUDITEVENT: 2288 return "AuditEvent"; 2289 case BASIC: 2290 return "Basic"; 2291 case BINARY: 2292 return "Binary"; 2293 case BIOLOGICALLYDERIVEDPRODUCT: 2294 return "BiologicallyDerivedProduct"; 2295 case BODYSTRUCTURE: 2296 return "BodyStructure"; 2297 case BUNDLE: 2298 return "Bundle"; 2299 case CAPABILITYSTATEMENT: 2300 return "CapabilityStatement"; 2301 case CAREPLAN: 2302 return "CarePlan"; 2303 case CARETEAM: 2304 return "CareTeam"; 2305 case CATALOGENTRY: 2306 return "CatalogEntry"; 2307 case CHARGEITEM: 2308 return "ChargeItem"; 2309 case CHARGEITEMDEFINITION: 2310 return "ChargeItemDefinition"; 2311 case CLAIM: 2312 return "Claim"; 2313 case CLAIMRESPONSE: 2314 return "ClaimResponse"; 2315 case CLINICALIMPRESSION: 2316 return "ClinicalImpression"; 2317 case CODESYSTEM: 2318 return "CodeSystem"; 2319 case COMMUNICATION: 2320 return "Communication"; 2321 case COMMUNICATIONREQUEST: 2322 return "CommunicationRequest"; 2323 case COMPARTMENTDEFINITION: 2324 return "CompartmentDefinition"; 2325 case COMPOSITION: 2326 return "Composition"; 2327 case CONCEPTMAP: 2328 return "ConceptMap"; 2329 case CONDITION: 2330 return "Condition"; 2331 case CONSENT: 2332 return "Consent"; 2333 case CONTRACT: 2334 return "Contract"; 2335 case COVERAGE: 2336 return "Coverage"; 2337 case COVERAGEELIGIBILITYREQUEST: 2338 return "CoverageEligibilityRequest"; 2339 case COVERAGEELIGIBILITYRESPONSE: 2340 return "CoverageEligibilityResponse"; 2341 case DETECTEDISSUE: 2342 return "DetectedIssue"; 2343 case DEVICE: 2344 return "Device"; 2345 case DEVICEDEFINITION: 2346 return "DeviceDefinition"; 2347 case DEVICEMETRIC: 2348 return "DeviceMetric"; 2349 case DEVICEREQUEST: 2350 return "DeviceRequest"; 2351 case DEVICEUSESTATEMENT: 2352 return "DeviceUseStatement"; 2353 case DIAGNOSTICREPORT: 2354 return "DiagnosticReport"; 2355 case DOCUMENTMANIFEST: 2356 return "DocumentManifest"; 2357 case DOCUMENTREFERENCE: 2358 return "DocumentReference"; 2359 case DOMAINRESOURCE: 2360 return "DomainResource"; 2361 case EFFECTEVIDENCESYNTHESIS: 2362 return "EffectEvidenceSynthesis"; 2363 case ENCOUNTER: 2364 return "Encounter"; 2365 case ENDPOINT: 2366 return "Endpoint"; 2367 case ENROLLMENTREQUEST: 2368 return "EnrollmentRequest"; 2369 case ENROLLMENTRESPONSE: 2370 return "EnrollmentResponse"; 2371 case EPISODEOFCARE: 2372 return "EpisodeOfCare"; 2373 case EVENTDEFINITION: 2374 return "EventDefinition"; 2375 case EVIDENCE: 2376 return "Evidence"; 2377 case EVIDENCEVARIABLE: 2378 return "EvidenceVariable"; 2379 case EXAMPLESCENARIO: 2380 return "ExampleScenario"; 2381 case EXPLANATIONOFBENEFIT: 2382 return "ExplanationOfBenefit"; 2383 case FAMILYMEMBERHISTORY: 2384 return "FamilyMemberHistory"; 2385 case FLAG: 2386 return "Flag"; 2387 case GOAL: 2388 return "Goal"; 2389 case GRAPHDEFINITION: 2390 return "GraphDefinition"; 2391 case GROUP: 2392 return "Group"; 2393 case GUIDANCERESPONSE: 2394 return "GuidanceResponse"; 2395 case HEALTHCARESERVICE: 2396 return "HealthcareService"; 2397 case IMAGINGSTUDY: 2398 return "ImagingStudy"; 2399 case IMMUNIZATION: 2400 return "Immunization"; 2401 case IMMUNIZATIONEVALUATION: 2402 return "ImmunizationEvaluation"; 2403 case IMMUNIZATIONRECOMMENDATION: 2404 return "ImmunizationRecommendation"; 2405 case IMPLEMENTATIONGUIDE: 2406 return "ImplementationGuide"; 2407 case INSURANCEPLAN: 2408 return "InsurancePlan"; 2409 case INVOICE: 2410 return "Invoice"; 2411 case LIBRARY: 2412 return "Library"; 2413 case LINKAGE: 2414 return "Linkage"; 2415 case LIST: 2416 return "List"; 2417 case LOCATION: 2418 return "Location"; 2419 case MEASURE: 2420 return "Measure"; 2421 case MEASUREREPORT: 2422 return "MeasureReport"; 2423 case MEDIA: 2424 return "Media"; 2425 case MEDICATION: 2426 return "Medication"; 2427 case MEDICATIONADMINISTRATION: 2428 return "MedicationAdministration"; 2429 case MEDICATIONDISPENSE: 2430 return "MedicationDispense"; 2431 case MEDICATIONKNOWLEDGE: 2432 return "MedicationKnowledge"; 2433 case MEDICATIONREQUEST: 2434 return "MedicationRequest"; 2435 case MEDICATIONSTATEMENT: 2436 return "MedicationStatement"; 2437 case MEDICINALPRODUCT: 2438 return "MedicinalProduct"; 2439 case MEDICINALPRODUCTAUTHORIZATION: 2440 return "MedicinalProductAuthorization"; 2441 case MEDICINALPRODUCTCONTRAINDICATION: 2442 return "MedicinalProductContraindication"; 2443 case MEDICINALPRODUCTINDICATION: 2444 return "MedicinalProductIndication"; 2445 case MEDICINALPRODUCTINGREDIENT: 2446 return "MedicinalProductIngredient"; 2447 case MEDICINALPRODUCTINTERACTION: 2448 return "MedicinalProductInteraction"; 2449 case MEDICINALPRODUCTMANUFACTURED: 2450 return "MedicinalProductManufactured"; 2451 case MEDICINALPRODUCTPACKAGED: 2452 return "MedicinalProductPackaged"; 2453 case MEDICINALPRODUCTPHARMACEUTICAL: 2454 return "MedicinalProductPharmaceutical"; 2455 case MEDICINALPRODUCTUNDESIRABLEEFFECT: 2456 return "MedicinalProductUndesirableEffect"; 2457 case MESSAGEDEFINITION: 2458 return "MessageDefinition"; 2459 case MESSAGEHEADER: 2460 return "MessageHeader"; 2461 case MOLECULARSEQUENCE: 2462 return "MolecularSequence"; 2463 case NAMINGSYSTEM: 2464 return "NamingSystem"; 2465 case NUTRITIONORDER: 2466 return "NutritionOrder"; 2467 case OBSERVATION: 2468 return "Observation"; 2469 case OBSERVATIONDEFINITION: 2470 return "ObservationDefinition"; 2471 case OPERATIONDEFINITION: 2472 return "OperationDefinition"; 2473 case OPERATIONOUTCOME: 2474 return "OperationOutcome"; 2475 case ORGANIZATION: 2476 return "Organization"; 2477 case ORGANIZATIONAFFILIATION: 2478 return "OrganizationAffiliation"; 2479 case PARAMETERS: 2480 return "Parameters"; 2481 case PATIENT: 2482 return "Patient"; 2483 case PAYMENTNOTICE: 2484 return "PaymentNotice"; 2485 case PAYMENTRECONCILIATION: 2486 return "PaymentReconciliation"; 2487 case PERSON: 2488 return "Person"; 2489 case PLANDEFINITION: 2490 return "PlanDefinition"; 2491 case PRACTITIONER: 2492 return "Practitioner"; 2493 case PRACTITIONERROLE: 2494 return "PractitionerRole"; 2495 case PROCEDURE: 2496 return "Procedure"; 2497 case PROVENANCE: 2498 return "Provenance"; 2499 case QUESTIONNAIRE: 2500 return "Questionnaire"; 2501 case QUESTIONNAIRERESPONSE: 2502 return "QuestionnaireResponse"; 2503 case RELATEDPERSON: 2504 return "RelatedPerson"; 2505 case REQUESTGROUP: 2506 return "RequestGroup"; 2507 case RESEARCHDEFINITION: 2508 return "ResearchDefinition"; 2509 case RESEARCHELEMENTDEFINITION: 2510 return "ResearchElementDefinition"; 2511 case RESEARCHSTUDY: 2512 return "ResearchStudy"; 2513 case RESEARCHSUBJECT: 2514 return "ResearchSubject"; 2515 case RESOURCE: 2516 return "Resource"; 2517 case RISKASSESSMENT: 2518 return "RiskAssessment"; 2519 case RISKEVIDENCESYNTHESIS: 2520 return "RiskEvidenceSynthesis"; 2521 case SCHEDULE: 2522 return "Schedule"; 2523 case SEARCHPARAMETER: 2524 return "SearchParameter"; 2525 case SERVICEREQUEST: 2526 return "ServiceRequest"; 2527 case SLOT: 2528 return "Slot"; 2529 case SPECIMEN: 2530 return "Specimen"; 2531 case SPECIMENDEFINITION: 2532 return "SpecimenDefinition"; 2533 case STRUCTUREDEFINITION: 2534 return "StructureDefinition"; 2535 case STRUCTUREMAP: 2536 return "StructureMap"; 2537 case SUBSCRIPTION: 2538 return "Subscription"; 2539 case SUBSTANCE: 2540 return "Substance"; 2541 case SUBSTANCENUCLEICACID: 2542 return "SubstanceNucleicAcid"; 2543 case SUBSTANCEPOLYMER: 2544 return "SubstancePolymer"; 2545 case SUBSTANCEPROTEIN: 2546 return "SubstanceProtein"; 2547 case SUBSTANCEREFERENCEINFORMATION: 2548 return "SubstanceReferenceInformation"; 2549 case SUBSTANCESOURCEMATERIAL: 2550 return "SubstanceSourceMaterial"; 2551 case SUBSTANCESPECIFICATION: 2552 return "SubstanceSpecification"; 2553 case SUPPLYDELIVERY: 2554 return "SupplyDelivery"; 2555 case SUPPLYREQUEST: 2556 return "SupplyRequest"; 2557 case TASK: 2558 return "Task"; 2559 case TERMINOLOGYCAPABILITIES: 2560 return "TerminologyCapabilities"; 2561 case TESTREPORT: 2562 return "TestReport"; 2563 case TESTSCRIPT: 2564 return "TestScript"; 2565 case VALUESET: 2566 return "ValueSet"; 2567 case VERIFICATIONRESULT: 2568 return "VerificationResult"; 2569 case VISIONPRESCRIPTION: 2570 return "VisionPrescription"; 2571 case NULL: 2572 return null; 2573 default: 2574 return "?"; 2575 } 2576 } 2577 } 2578 2579 public static class FHIRResourceTypeEnumFactory implements EnumFactory<FHIRResourceType> { 2580 public FHIRResourceType fromCode(String codeString) throws IllegalArgumentException { 2581 if (codeString == null || "".equals(codeString)) 2582 if (codeString == null || "".equals(codeString)) 2583 return null; 2584 if ("Account".equals(codeString)) 2585 return FHIRResourceType.ACCOUNT; 2586 if ("ActivityDefinition".equals(codeString)) 2587 return FHIRResourceType.ACTIVITYDEFINITION; 2588 if ("AdverseEvent".equals(codeString)) 2589 return FHIRResourceType.ADVERSEEVENT; 2590 if ("AllergyIntolerance".equals(codeString)) 2591 return FHIRResourceType.ALLERGYINTOLERANCE; 2592 if ("Appointment".equals(codeString)) 2593 return FHIRResourceType.APPOINTMENT; 2594 if ("AppointmentResponse".equals(codeString)) 2595 return FHIRResourceType.APPOINTMENTRESPONSE; 2596 if ("AuditEvent".equals(codeString)) 2597 return FHIRResourceType.AUDITEVENT; 2598 if ("Basic".equals(codeString)) 2599 return FHIRResourceType.BASIC; 2600 if ("Binary".equals(codeString)) 2601 return FHIRResourceType.BINARY; 2602 if ("BiologicallyDerivedProduct".equals(codeString)) 2603 return FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT; 2604 if ("BodyStructure".equals(codeString)) 2605 return FHIRResourceType.BODYSTRUCTURE; 2606 if ("Bundle".equals(codeString)) 2607 return FHIRResourceType.BUNDLE; 2608 if ("CapabilityStatement".equals(codeString)) 2609 return FHIRResourceType.CAPABILITYSTATEMENT; 2610 if ("CarePlan".equals(codeString)) 2611 return FHIRResourceType.CAREPLAN; 2612 if ("CareTeam".equals(codeString)) 2613 return FHIRResourceType.CARETEAM; 2614 if ("CatalogEntry".equals(codeString)) 2615 return FHIRResourceType.CATALOGENTRY; 2616 if ("ChargeItem".equals(codeString)) 2617 return FHIRResourceType.CHARGEITEM; 2618 if ("ChargeItemDefinition".equals(codeString)) 2619 return FHIRResourceType.CHARGEITEMDEFINITION; 2620 if ("Claim".equals(codeString)) 2621 return FHIRResourceType.CLAIM; 2622 if ("ClaimResponse".equals(codeString)) 2623 return FHIRResourceType.CLAIMRESPONSE; 2624 if ("ClinicalImpression".equals(codeString)) 2625 return FHIRResourceType.CLINICALIMPRESSION; 2626 if ("CodeSystem".equals(codeString)) 2627 return FHIRResourceType.CODESYSTEM; 2628 if ("Communication".equals(codeString)) 2629 return FHIRResourceType.COMMUNICATION; 2630 if ("CommunicationRequest".equals(codeString)) 2631 return FHIRResourceType.COMMUNICATIONREQUEST; 2632 if ("CompartmentDefinition".equals(codeString)) 2633 return FHIRResourceType.COMPARTMENTDEFINITION; 2634 if ("Composition".equals(codeString)) 2635 return FHIRResourceType.COMPOSITION; 2636 if ("ConceptMap".equals(codeString)) 2637 return FHIRResourceType.CONCEPTMAP; 2638 if ("Condition".equals(codeString)) 2639 return FHIRResourceType.CONDITION; 2640 if ("Consent".equals(codeString)) 2641 return FHIRResourceType.CONSENT; 2642 if ("Contract".equals(codeString)) 2643 return FHIRResourceType.CONTRACT; 2644 if ("Coverage".equals(codeString)) 2645 return FHIRResourceType.COVERAGE; 2646 if ("CoverageEligibilityRequest".equals(codeString)) 2647 return FHIRResourceType.COVERAGEELIGIBILITYREQUEST; 2648 if ("CoverageEligibilityResponse".equals(codeString)) 2649 return FHIRResourceType.COVERAGEELIGIBILITYRESPONSE; 2650 if ("DetectedIssue".equals(codeString)) 2651 return FHIRResourceType.DETECTEDISSUE; 2652 if ("Device".equals(codeString)) 2653 return FHIRResourceType.DEVICE; 2654 if ("DeviceDefinition".equals(codeString)) 2655 return FHIRResourceType.DEVICEDEFINITION; 2656 if ("DeviceMetric".equals(codeString)) 2657 return FHIRResourceType.DEVICEMETRIC; 2658 if ("DeviceRequest".equals(codeString)) 2659 return FHIRResourceType.DEVICEREQUEST; 2660 if ("DeviceUseStatement".equals(codeString)) 2661 return FHIRResourceType.DEVICEUSESTATEMENT; 2662 if ("DiagnosticReport".equals(codeString)) 2663 return FHIRResourceType.DIAGNOSTICREPORT; 2664 if ("DocumentManifest".equals(codeString)) 2665 return FHIRResourceType.DOCUMENTMANIFEST; 2666 if ("DocumentReference".equals(codeString)) 2667 return FHIRResourceType.DOCUMENTREFERENCE; 2668 if ("DomainResource".equals(codeString)) 2669 return FHIRResourceType.DOMAINRESOURCE; 2670 if ("EffectEvidenceSynthesis".equals(codeString)) 2671 return FHIRResourceType.EFFECTEVIDENCESYNTHESIS; 2672 if ("Encounter".equals(codeString)) 2673 return FHIRResourceType.ENCOUNTER; 2674 if ("Endpoint".equals(codeString)) 2675 return FHIRResourceType.ENDPOINT; 2676 if ("EnrollmentRequest".equals(codeString)) 2677 return FHIRResourceType.ENROLLMENTREQUEST; 2678 if ("EnrollmentResponse".equals(codeString)) 2679 return FHIRResourceType.ENROLLMENTRESPONSE; 2680 if ("EpisodeOfCare".equals(codeString)) 2681 return FHIRResourceType.EPISODEOFCARE; 2682 if ("EventDefinition".equals(codeString)) 2683 return FHIRResourceType.EVENTDEFINITION; 2684 if ("Evidence".equals(codeString)) 2685 return FHIRResourceType.EVIDENCE; 2686 if ("EvidenceVariable".equals(codeString)) 2687 return FHIRResourceType.EVIDENCEVARIABLE; 2688 if ("ExampleScenario".equals(codeString)) 2689 return FHIRResourceType.EXAMPLESCENARIO; 2690 if ("ExplanationOfBenefit".equals(codeString)) 2691 return FHIRResourceType.EXPLANATIONOFBENEFIT; 2692 if ("FamilyMemberHistory".equals(codeString)) 2693 return FHIRResourceType.FAMILYMEMBERHISTORY; 2694 if ("Flag".equals(codeString)) 2695 return FHIRResourceType.FLAG; 2696 if ("Goal".equals(codeString)) 2697 return FHIRResourceType.GOAL; 2698 if ("GraphDefinition".equals(codeString)) 2699 return FHIRResourceType.GRAPHDEFINITION; 2700 if ("Group".equals(codeString)) 2701 return FHIRResourceType.GROUP; 2702 if ("GuidanceResponse".equals(codeString)) 2703 return FHIRResourceType.GUIDANCERESPONSE; 2704 if ("HealthcareService".equals(codeString)) 2705 return FHIRResourceType.HEALTHCARESERVICE; 2706 if ("ImagingStudy".equals(codeString)) 2707 return FHIRResourceType.IMAGINGSTUDY; 2708 if ("Immunization".equals(codeString)) 2709 return FHIRResourceType.IMMUNIZATION; 2710 if ("ImmunizationEvaluation".equals(codeString)) 2711 return FHIRResourceType.IMMUNIZATIONEVALUATION; 2712 if ("ImmunizationRecommendation".equals(codeString)) 2713 return FHIRResourceType.IMMUNIZATIONRECOMMENDATION; 2714 if ("ImplementationGuide".equals(codeString)) 2715 return FHIRResourceType.IMPLEMENTATIONGUIDE; 2716 if ("InsurancePlan".equals(codeString)) 2717 return FHIRResourceType.INSURANCEPLAN; 2718 if ("Invoice".equals(codeString)) 2719 return FHIRResourceType.INVOICE; 2720 if ("Library".equals(codeString)) 2721 return FHIRResourceType.LIBRARY; 2722 if ("Linkage".equals(codeString)) 2723 return FHIRResourceType.LINKAGE; 2724 if ("List".equals(codeString)) 2725 return FHIRResourceType.LIST; 2726 if ("Location".equals(codeString)) 2727 return FHIRResourceType.LOCATION; 2728 if ("Measure".equals(codeString)) 2729 return FHIRResourceType.MEASURE; 2730 if ("MeasureReport".equals(codeString)) 2731 return FHIRResourceType.MEASUREREPORT; 2732 if ("Media".equals(codeString)) 2733 return FHIRResourceType.MEDIA; 2734 if ("Medication".equals(codeString)) 2735 return FHIRResourceType.MEDICATION; 2736 if ("MedicationAdministration".equals(codeString)) 2737 return FHIRResourceType.MEDICATIONADMINISTRATION; 2738 if ("MedicationDispense".equals(codeString)) 2739 return FHIRResourceType.MEDICATIONDISPENSE; 2740 if ("MedicationKnowledge".equals(codeString)) 2741 return FHIRResourceType.MEDICATIONKNOWLEDGE; 2742 if ("MedicationRequest".equals(codeString)) 2743 return FHIRResourceType.MEDICATIONREQUEST; 2744 if ("MedicationStatement".equals(codeString)) 2745 return FHIRResourceType.MEDICATIONSTATEMENT; 2746 if ("MedicinalProduct".equals(codeString)) 2747 return FHIRResourceType.MEDICINALPRODUCT; 2748 if ("MedicinalProductAuthorization".equals(codeString)) 2749 return FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION; 2750 if ("MedicinalProductContraindication".equals(codeString)) 2751 return FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION; 2752 if ("MedicinalProductIndication".equals(codeString)) 2753 return FHIRResourceType.MEDICINALPRODUCTINDICATION; 2754 if ("MedicinalProductIngredient".equals(codeString)) 2755 return FHIRResourceType.MEDICINALPRODUCTINGREDIENT; 2756 if ("MedicinalProductInteraction".equals(codeString)) 2757 return FHIRResourceType.MEDICINALPRODUCTINTERACTION; 2758 if ("MedicinalProductManufactured".equals(codeString)) 2759 return FHIRResourceType.MEDICINALPRODUCTMANUFACTURED; 2760 if ("MedicinalProductPackaged".equals(codeString)) 2761 return FHIRResourceType.MEDICINALPRODUCTPACKAGED; 2762 if ("MedicinalProductPharmaceutical".equals(codeString)) 2763 return FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL; 2764 if ("MedicinalProductUndesirableEffect".equals(codeString)) 2765 return FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT; 2766 if ("MessageDefinition".equals(codeString)) 2767 return FHIRResourceType.MESSAGEDEFINITION; 2768 if ("MessageHeader".equals(codeString)) 2769 return FHIRResourceType.MESSAGEHEADER; 2770 if ("MolecularSequence".equals(codeString)) 2771 return FHIRResourceType.MOLECULARSEQUENCE; 2772 if ("NamingSystem".equals(codeString)) 2773 return FHIRResourceType.NAMINGSYSTEM; 2774 if ("NutritionOrder".equals(codeString)) 2775 return FHIRResourceType.NUTRITIONORDER; 2776 if ("Observation".equals(codeString)) 2777 return FHIRResourceType.OBSERVATION; 2778 if ("ObservationDefinition".equals(codeString)) 2779 return FHIRResourceType.OBSERVATIONDEFINITION; 2780 if ("OperationDefinition".equals(codeString)) 2781 return FHIRResourceType.OPERATIONDEFINITION; 2782 if ("OperationOutcome".equals(codeString)) 2783 return FHIRResourceType.OPERATIONOUTCOME; 2784 if ("Organization".equals(codeString)) 2785 return FHIRResourceType.ORGANIZATION; 2786 if ("OrganizationAffiliation".equals(codeString)) 2787 return FHIRResourceType.ORGANIZATIONAFFILIATION; 2788 if ("Parameters".equals(codeString)) 2789 return FHIRResourceType.PARAMETERS; 2790 if ("Patient".equals(codeString)) 2791 return FHIRResourceType.PATIENT; 2792 if ("PaymentNotice".equals(codeString)) 2793 return FHIRResourceType.PAYMENTNOTICE; 2794 if ("PaymentReconciliation".equals(codeString)) 2795 return FHIRResourceType.PAYMENTRECONCILIATION; 2796 if ("Person".equals(codeString)) 2797 return FHIRResourceType.PERSON; 2798 if ("PlanDefinition".equals(codeString)) 2799 return FHIRResourceType.PLANDEFINITION; 2800 if ("Practitioner".equals(codeString)) 2801 return FHIRResourceType.PRACTITIONER; 2802 if ("PractitionerRole".equals(codeString)) 2803 return FHIRResourceType.PRACTITIONERROLE; 2804 if ("Procedure".equals(codeString)) 2805 return FHIRResourceType.PROCEDURE; 2806 if ("Provenance".equals(codeString)) 2807 return FHIRResourceType.PROVENANCE; 2808 if ("Questionnaire".equals(codeString)) 2809 return FHIRResourceType.QUESTIONNAIRE; 2810 if ("QuestionnaireResponse".equals(codeString)) 2811 return FHIRResourceType.QUESTIONNAIRERESPONSE; 2812 if ("RelatedPerson".equals(codeString)) 2813 return FHIRResourceType.RELATEDPERSON; 2814 if ("RequestGroup".equals(codeString)) 2815 return FHIRResourceType.REQUESTGROUP; 2816 if ("ResearchDefinition".equals(codeString)) 2817 return FHIRResourceType.RESEARCHDEFINITION; 2818 if ("ResearchElementDefinition".equals(codeString)) 2819 return FHIRResourceType.RESEARCHELEMENTDEFINITION; 2820 if ("ResearchStudy".equals(codeString)) 2821 return FHIRResourceType.RESEARCHSTUDY; 2822 if ("ResearchSubject".equals(codeString)) 2823 return FHIRResourceType.RESEARCHSUBJECT; 2824 if ("Resource".equals(codeString)) 2825 return FHIRResourceType.RESOURCE; 2826 if ("RiskAssessment".equals(codeString)) 2827 return FHIRResourceType.RISKASSESSMENT; 2828 if ("RiskEvidenceSynthesis".equals(codeString)) 2829 return FHIRResourceType.RISKEVIDENCESYNTHESIS; 2830 if ("Schedule".equals(codeString)) 2831 return FHIRResourceType.SCHEDULE; 2832 if ("SearchParameter".equals(codeString)) 2833 return FHIRResourceType.SEARCHPARAMETER; 2834 if ("ServiceRequest".equals(codeString)) 2835 return FHIRResourceType.SERVICEREQUEST; 2836 if ("Slot".equals(codeString)) 2837 return FHIRResourceType.SLOT; 2838 if ("Specimen".equals(codeString)) 2839 return FHIRResourceType.SPECIMEN; 2840 if ("SpecimenDefinition".equals(codeString)) 2841 return FHIRResourceType.SPECIMENDEFINITION; 2842 if ("StructureDefinition".equals(codeString)) 2843 return FHIRResourceType.STRUCTUREDEFINITION; 2844 if ("StructureMap".equals(codeString)) 2845 return FHIRResourceType.STRUCTUREMAP; 2846 if ("Subscription".equals(codeString)) 2847 return FHIRResourceType.SUBSCRIPTION; 2848 if ("Substance".equals(codeString)) 2849 return FHIRResourceType.SUBSTANCE; 2850 if ("SubstanceNucleicAcid".equals(codeString)) 2851 return FHIRResourceType.SUBSTANCENUCLEICACID; 2852 if ("SubstancePolymer".equals(codeString)) 2853 return FHIRResourceType.SUBSTANCEPOLYMER; 2854 if ("SubstanceProtein".equals(codeString)) 2855 return FHIRResourceType.SUBSTANCEPROTEIN; 2856 if ("SubstanceReferenceInformation".equals(codeString)) 2857 return FHIRResourceType.SUBSTANCEREFERENCEINFORMATION; 2858 if ("SubstanceSourceMaterial".equals(codeString)) 2859 return FHIRResourceType.SUBSTANCESOURCEMATERIAL; 2860 if ("SubstanceSpecification".equals(codeString)) 2861 return FHIRResourceType.SUBSTANCESPECIFICATION; 2862 if ("SupplyDelivery".equals(codeString)) 2863 return FHIRResourceType.SUPPLYDELIVERY; 2864 if ("SupplyRequest".equals(codeString)) 2865 return FHIRResourceType.SUPPLYREQUEST; 2866 if ("Task".equals(codeString)) 2867 return FHIRResourceType.TASK; 2868 if ("TerminologyCapabilities".equals(codeString)) 2869 return FHIRResourceType.TERMINOLOGYCAPABILITIES; 2870 if ("TestReport".equals(codeString)) 2871 return FHIRResourceType.TESTREPORT; 2872 if ("TestScript".equals(codeString)) 2873 return FHIRResourceType.TESTSCRIPT; 2874 if ("ValueSet".equals(codeString)) 2875 return FHIRResourceType.VALUESET; 2876 if ("VerificationResult".equals(codeString)) 2877 return FHIRResourceType.VERIFICATIONRESULT; 2878 if ("VisionPrescription".equals(codeString)) 2879 return FHIRResourceType.VISIONPRESCRIPTION; 2880 throw new IllegalArgumentException("Unknown FHIRResourceType code '" + codeString + "'"); 2881 } 2882 2883 public Enumeration<FHIRResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 2884 if (code == null) 2885 return null; 2886 if (code.isEmpty()) 2887 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NULL, code); 2888 String codeString = code.asStringValue(); 2889 if (codeString == null || "".equals(codeString)) 2890 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NULL, code); 2891 if ("Account".equals(codeString)) 2892 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ACCOUNT, code); 2893 if ("ActivityDefinition".equals(codeString)) 2894 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ACTIVITYDEFINITION, code); 2895 if ("AdverseEvent".equals(codeString)) 2896 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ADVERSEEVENT, code); 2897 if ("AllergyIntolerance".equals(codeString)) 2898 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ALLERGYINTOLERANCE, code); 2899 if ("Appointment".equals(codeString)) 2900 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.APPOINTMENT, code); 2901 if ("AppointmentResponse".equals(codeString)) 2902 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.APPOINTMENTRESPONSE, code); 2903 if ("AuditEvent".equals(codeString)) 2904 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.AUDITEVENT, code); 2905 if ("Basic".equals(codeString)) 2906 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BASIC, code); 2907 if ("Binary".equals(codeString)) 2908 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BINARY, code); 2909 if ("BiologicallyDerivedProduct".equals(codeString)) 2910 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT, code); 2911 if ("BodyStructure".equals(codeString)) 2912 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BODYSTRUCTURE, code); 2913 if ("Bundle".equals(codeString)) 2914 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.BUNDLE, code); 2915 if ("CapabilityStatement".equals(codeString)) 2916 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CAPABILITYSTATEMENT, code); 2917 if ("CarePlan".equals(codeString)) 2918 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CAREPLAN, code); 2919 if ("CareTeam".equals(codeString)) 2920 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CARETEAM, code); 2921 if ("CatalogEntry".equals(codeString)) 2922 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CATALOGENTRY, code); 2923 if ("ChargeItem".equals(codeString)) 2924 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CHARGEITEM, code); 2925 if ("ChargeItemDefinition".equals(codeString)) 2926 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CHARGEITEMDEFINITION, code); 2927 if ("Claim".equals(codeString)) 2928 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLAIM, code); 2929 if ("ClaimResponse".equals(codeString)) 2930 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLAIMRESPONSE, code); 2931 if ("ClinicalImpression".equals(codeString)) 2932 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CLINICALIMPRESSION, code); 2933 if ("CodeSystem".equals(codeString)) 2934 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CODESYSTEM, code); 2935 if ("Communication".equals(codeString)) 2936 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMMUNICATION, code); 2937 if ("CommunicationRequest".equals(codeString)) 2938 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMMUNICATIONREQUEST, code); 2939 if ("CompartmentDefinition".equals(codeString)) 2940 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMPARTMENTDEFINITION, code); 2941 if ("Composition".equals(codeString)) 2942 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COMPOSITION, code); 2943 if ("ConceptMap".equals(codeString)) 2944 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONCEPTMAP, code); 2945 if ("Condition".equals(codeString)) 2946 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONDITION, code); 2947 if ("Consent".equals(codeString)) 2948 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONSENT, code); 2949 if ("Contract".equals(codeString)) 2950 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.CONTRACT, code); 2951 if ("Coverage".equals(codeString)) 2952 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGE, code); 2953 if ("CoverageEligibilityRequest".equals(codeString)) 2954 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGEELIGIBILITYREQUEST, code); 2955 if ("CoverageEligibilityResponse".equals(codeString)) 2956 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.COVERAGEELIGIBILITYRESPONSE, code); 2957 if ("DetectedIssue".equals(codeString)) 2958 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DETECTEDISSUE, code); 2959 if ("Device".equals(codeString)) 2960 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICE, code); 2961 if ("DeviceDefinition".equals(codeString)) 2962 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEDEFINITION, code); 2963 if ("DeviceMetric".equals(codeString)) 2964 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEMETRIC, code); 2965 if ("DeviceRequest".equals(codeString)) 2966 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEREQUEST, code); 2967 if ("DeviceUseStatement".equals(codeString)) 2968 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DEVICEUSESTATEMENT, code); 2969 if ("DiagnosticReport".equals(codeString)) 2970 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DIAGNOSTICREPORT, code); 2971 if ("DocumentManifest".equals(codeString)) 2972 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOCUMENTMANIFEST, code); 2973 if ("DocumentReference".equals(codeString)) 2974 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOCUMENTREFERENCE, code); 2975 if ("DomainResource".equals(codeString)) 2976 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.DOMAINRESOURCE, code); 2977 if ("EffectEvidenceSynthesis".equals(codeString)) 2978 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EFFECTEVIDENCESYNTHESIS, code); 2979 if ("Encounter".equals(codeString)) 2980 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENCOUNTER, code); 2981 if ("Endpoint".equals(codeString)) 2982 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENDPOINT, code); 2983 if ("EnrollmentRequest".equals(codeString)) 2984 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENROLLMENTREQUEST, code); 2985 if ("EnrollmentResponse".equals(codeString)) 2986 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ENROLLMENTRESPONSE, code); 2987 if ("EpisodeOfCare".equals(codeString)) 2988 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EPISODEOFCARE, code); 2989 if ("EventDefinition".equals(codeString)) 2990 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVENTDEFINITION, code); 2991 if ("Evidence".equals(codeString)) 2992 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVIDENCE, code); 2993 if ("EvidenceVariable".equals(codeString)) 2994 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EVIDENCEVARIABLE, code); 2995 if ("ExampleScenario".equals(codeString)) 2996 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EXAMPLESCENARIO, code); 2997 if ("ExplanationOfBenefit".equals(codeString)) 2998 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.EXPLANATIONOFBENEFIT, code); 2999 if ("FamilyMemberHistory".equals(codeString)) 3000 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.FAMILYMEMBERHISTORY, code); 3001 if ("Flag".equals(codeString)) 3002 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.FLAG, code); 3003 if ("Goal".equals(codeString)) 3004 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GOAL, code); 3005 if ("GraphDefinition".equals(codeString)) 3006 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GRAPHDEFINITION, code); 3007 if ("Group".equals(codeString)) 3008 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GROUP, code); 3009 if ("GuidanceResponse".equals(codeString)) 3010 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.GUIDANCERESPONSE, code); 3011 if ("HealthcareService".equals(codeString)) 3012 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.HEALTHCARESERVICE, code); 3013 if ("ImagingStudy".equals(codeString)) 3014 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMAGINGSTUDY, code); 3015 if ("Immunization".equals(codeString)) 3016 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATION, code); 3017 if ("ImmunizationEvaluation".equals(codeString)) 3018 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATIONEVALUATION, code); 3019 if ("ImmunizationRecommendation".equals(codeString)) 3020 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMMUNIZATIONRECOMMENDATION, code); 3021 if ("ImplementationGuide".equals(codeString)) 3022 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.IMPLEMENTATIONGUIDE, code); 3023 if ("InsurancePlan".equals(codeString)) 3024 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.INSURANCEPLAN, code); 3025 if ("Invoice".equals(codeString)) 3026 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.INVOICE, code); 3027 if ("Library".equals(codeString)) 3028 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LIBRARY, code); 3029 if ("Linkage".equals(codeString)) 3030 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LINKAGE, code); 3031 if ("List".equals(codeString)) 3032 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LIST, code); 3033 if ("Location".equals(codeString)) 3034 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.LOCATION, code); 3035 if ("Measure".equals(codeString)) 3036 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEASURE, code); 3037 if ("MeasureReport".equals(codeString)) 3038 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEASUREREPORT, code); 3039 if ("Media".equals(codeString)) 3040 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDIA, code); 3041 if ("Medication".equals(codeString)) 3042 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATION, code); 3043 if ("MedicationAdministration".equals(codeString)) 3044 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONADMINISTRATION, code); 3045 if ("MedicationDispense".equals(codeString)) 3046 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONDISPENSE, code); 3047 if ("MedicationKnowledge".equals(codeString)) 3048 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONKNOWLEDGE, code); 3049 if ("MedicationRequest".equals(codeString)) 3050 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONREQUEST, code); 3051 if ("MedicationStatement".equals(codeString)) 3052 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICATIONSTATEMENT, code); 3053 if ("MedicinalProduct".equals(codeString)) 3054 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCT, code); 3055 if ("MedicinalProductAuthorization".equals(codeString)) 3056 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION, code); 3057 if ("MedicinalProductContraindication".equals(codeString)) 3058 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION, code); 3059 if ("MedicinalProductIndication".equals(codeString)) 3060 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINDICATION, code); 3061 if ("MedicinalProductIngredient".equals(codeString)) 3062 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINGREDIENT, code); 3063 if ("MedicinalProductInteraction".equals(codeString)) 3064 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTINTERACTION, code); 3065 if ("MedicinalProductManufactured".equals(codeString)) 3066 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTMANUFACTURED, code); 3067 if ("MedicinalProductPackaged".equals(codeString)) 3068 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTPACKAGED, code); 3069 if ("MedicinalProductPharmaceutical".equals(codeString)) 3070 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL, code); 3071 if ("MedicinalProductUndesirableEffect".equals(codeString)) 3072 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT, code); 3073 if ("MessageDefinition".equals(codeString)) 3074 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MESSAGEDEFINITION, code); 3075 if ("MessageHeader".equals(codeString)) 3076 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MESSAGEHEADER, code); 3077 if ("MolecularSequence".equals(codeString)) 3078 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.MOLECULARSEQUENCE, code); 3079 if ("NamingSystem".equals(codeString)) 3080 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NAMINGSYSTEM, code); 3081 if ("NutritionOrder".equals(codeString)) 3082 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.NUTRITIONORDER, code); 3083 if ("Observation".equals(codeString)) 3084 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OBSERVATION, code); 3085 if ("ObservationDefinition".equals(codeString)) 3086 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OBSERVATIONDEFINITION, code); 3087 if ("OperationDefinition".equals(codeString)) 3088 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OPERATIONDEFINITION, code); 3089 if ("OperationOutcome".equals(codeString)) 3090 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.OPERATIONOUTCOME, code); 3091 if ("Organization".equals(codeString)) 3092 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ORGANIZATION, code); 3093 if ("OrganizationAffiliation".equals(codeString)) 3094 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.ORGANIZATIONAFFILIATION, code); 3095 if ("Parameters".equals(codeString)) 3096 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PARAMETERS, code); 3097 if ("Patient".equals(codeString)) 3098 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PATIENT, code); 3099 if ("PaymentNotice".equals(codeString)) 3100 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PAYMENTNOTICE, code); 3101 if ("PaymentReconciliation".equals(codeString)) 3102 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PAYMENTRECONCILIATION, code); 3103 if ("Person".equals(codeString)) 3104 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PERSON, code); 3105 if ("PlanDefinition".equals(codeString)) 3106 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PLANDEFINITION, code); 3107 if ("Practitioner".equals(codeString)) 3108 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PRACTITIONER, code); 3109 if ("PractitionerRole".equals(codeString)) 3110 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PRACTITIONERROLE, code); 3111 if ("Procedure".equals(codeString)) 3112 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PROCEDURE, code); 3113 if ("Provenance".equals(codeString)) 3114 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.PROVENANCE, code); 3115 if ("Questionnaire".equals(codeString)) 3116 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.QUESTIONNAIRE, code); 3117 if ("QuestionnaireResponse".equals(codeString)) 3118 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.QUESTIONNAIRERESPONSE, code); 3119 if ("RelatedPerson".equals(codeString)) 3120 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RELATEDPERSON, code); 3121 if ("RequestGroup".equals(codeString)) 3122 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.REQUESTGROUP, code); 3123 if ("ResearchDefinition".equals(codeString)) 3124 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHDEFINITION, code); 3125 if ("ResearchElementDefinition".equals(codeString)) 3126 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHELEMENTDEFINITION, code); 3127 if ("ResearchStudy".equals(codeString)) 3128 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHSTUDY, code); 3129 if ("ResearchSubject".equals(codeString)) 3130 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESEARCHSUBJECT, code); 3131 if ("Resource".equals(codeString)) 3132 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RESOURCE, code); 3133 if ("RiskAssessment".equals(codeString)) 3134 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RISKASSESSMENT, code); 3135 if ("RiskEvidenceSynthesis".equals(codeString)) 3136 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.RISKEVIDENCESYNTHESIS, code); 3137 if ("Schedule".equals(codeString)) 3138 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SCHEDULE, code); 3139 if ("SearchParameter".equals(codeString)) 3140 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SEARCHPARAMETER, code); 3141 if ("ServiceRequest".equals(codeString)) 3142 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SERVICEREQUEST, code); 3143 if ("Slot".equals(codeString)) 3144 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SLOT, code); 3145 if ("Specimen".equals(codeString)) 3146 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SPECIMEN, code); 3147 if ("SpecimenDefinition".equals(codeString)) 3148 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SPECIMENDEFINITION, code); 3149 if ("StructureDefinition".equals(codeString)) 3150 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.STRUCTUREDEFINITION, code); 3151 if ("StructureMap".equals(codeString)) 3152 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.STRUCTUREMAP, code); 3153 if ("Subscription".equals(codeString)) 3154 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSCRIPTION, code); 3155 if ("Substance".equals(codeString)) 3156 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCE, code); 3157 if ("SubstanceNucleicAcid".equals(codeString)) 3158 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCENUCLEICACID, code); 3159 if ("SubstancePolymer".equals(codeString)) 3160 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEPOLYMER, code); 3161 if ("SubstanceProtein".equals(codeString)) 3162 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEPROTEIN, code); 3163 if ("SubstanceReferenceInformation".equals(codeString)) 3164 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCEREFERENCEINFORMATION, code); 3165 if ("SubstanceSourceMaterial".equals(codeString)) 3166 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCESOURCEMATERIAL, code); 3167 if ("SubstanceSpecification".equals(codeString)) 3168 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUBSTANCESPECIFICATION, code); 3169 if ("SupplyDelivery".equals(codeString)) 3170 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUPPLYDELIVERY, code); 3171 if ("SupplyRequest".equals(codeString)) 3172 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.SUPPLYREQUEST, code); 3173 if ("Task".equals(codeString)) 3174 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TASK, code); 3175 if ("TerminologyCapabilities".equals(codeString)) 3176 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TERMINOLOGYCAPABILITIES, code); 3177 if ("TestReport".equals(codeString)) 3178 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TESTREPORT, code); 3179 if ("TestScript".equals(codeString)) 3180 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.TESTSCRIPT, code); 3181 if ("ValueSet".equals(codeString)) 3182 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VALUESET, code); 3183 if ("VerificationResult".equals(codeString)) 3184 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VERIFICATIONRESULT, code); 3185 if ("VisionPrescription".equals(codeString)) 3186 return new Enumeration<FHIRResourceType>(this, FHIRResourceType.VISIONPRESCRIPTION, code); 3187 throw new FHIRException("Unknown FHIRResourceType code '" + codeString + "'"); 3188 } 3189 3190 public String toCode(FHIRResourceType code) { 3191 if (code == FHIRResourceType.NULL) 3192 return null; 3193 if (code == FHIRResourceType.ACCOUNT) 3194 return "Account"; 3195 if (code == FHIRResourceType.ACTIVITYDEFINITION) 3196 return "ActivityDefinition"; 3197 if (code == FHIRResourceType.ADVERSEEVENT) 3198 return "AdverseEvent"; 3199 if (code == FHIRResourceType.ALLERGYINTOLERANCE) 3200 return "AllergyIntolerance"; 3201 if (code == FHIRResourceType.APPOINTMENT) 3202 return "Appointment"; 3203 if (code == FHIRResourceType.APPOINTMENTRESPONSE) 3204 return "AppointmentResponse"; 3205 if (code == FHIRResourceType.AUDITEVENT) 3206 return "AuditEvent"; 3207 if (code == FHIRResourceType.BASIC) 3208 return "Basic"; 3209 if (code == FHIRResourceType.BINARY) 3210 return "Binary"; 3211 if (code == FHIRResourceType.BIOLOGICALLYDERIVEDPRODUCT) 3212 return "BiologicallyDerivedProduct"; 3213 if (code == FHIRResourceType.BODYSTRUCTURE) 3214 return "BodyStructure"; 3215 if (code == FHIRResourceType.BUNDLE) 3216 return "Bundle"; 3217 if (code == FHIRResourceType.CAPABILITYSTATEMENT) 3218 return "CapabilityStatement"; 3219 if (code == FHIRResourceType.CAREPLAN) 3220 return "CarePlan"; 3221 if (code == FHIRResourceType.CARETEAM) 3222 return "CareTeam"; 3223 if (code == FHIRResourceType.CATALOGENTRY) 3224 return "CatalogEntry"; 3225 if (code == FHIRResourceType.CHARGEITEM) 3226 return "ChargeItem"; 3227 if (code == FHIRResourceType.CHARGEITEMDEFINITION) 3228 return "ChargeItemDefinition"; 3229 if (code == FHIRResourceType.CLAIM) 3230 return "Claim"; 3231 if (code == FHIRResourceType.CLAIMRESPONSE) 3232 return "ClaimResponse"; 3233 if (code == FHIRResourceType.CLINICALIMPRESSION) 3234 return "ClinicalImpression"; 3235 if (code == FHIRResourceType.CODESYSTEM) 3236 return "CodeSystem"; 3237 if (code == FHIRResourceType.COMMUNICATION) 3238 return "Communication"; 3239 if (code == FHIRResourceType.COMMUNICATIONREQUEST) 3240 return "CommunicationRequest"; 3241 if (code == FHIRResourceType.COMPARTMENTDEFINITION) 3242 return "CompartmentDefinition"; 3243 if (code == FHIRResourceType.COMPOSITION) 3244 return "Composition"; 3245 if (code == FHIRResourceType.CONCEPTMAP) 3246 return "ConceptMap"; 3247 if (code == FHIRResourceType.CONDITION) 3248 return "Condition"; 3249 if (code == FHIRResourceType.CONSENT) 3250 return "Consent"; 3251 if (code == FHIRResourceType.CONTRACT) 3252 return "Contract"; 3253 if (code == FHIRResourceType.COVERAGE) 3254 return "Coverage"; 3255 if (code == FHIRResourceType.COVERAGEELIGIBILITYREQUEST) 3256 return "CoverageEligibilityRequest"; 3257 if (code == FHIRResourceType.COVERAGEELIGIBILITYRESPONSE) 3258 return "CoverageEligibilityResponse"; 3259 if (code == FHIRResourceType.DETECTEDISSUE) 3260 return "DetectedIssue"; 3261 if (code == FHIRResourceType.DEVICE) 3262 return "Device"; 3263 if (code == FHIRResourceType.DEVICEDEFINITION) 3264 return "DeviceDefinition"; 3265 if (code == FHIRResourceType.DEVICEMETRIC) 3266 return "DeviceMetric"; 3267 if (code == FHIRResourceType.DEVICEREQUEST) 3268 return "DeviceRequest"; 3269 if (code == FHIRResourceType.DEVICEUSESTATEMENT) 3270 return "DeviceUseStatement"; 3271 if (code == FHIRResourceType.DIAGNOSTICREPORT) 3272 return "DiagnosticReport"; 3273 if (code == FHIRResourceType.DOCUMENTMANIFEST) 3274 return "DocumentManifest"; 3275 if (code == FHIRResourceType.DOCUMENTREFERENCE) 3276 return "DocumentReference"; 3277 if (code == FHIRResourceType.DOMAINRESOURCE) 3278 return "DomainResource"; 3279 if (code == FHIRResourceType.EFFECTEVIDENCESYNTHESIS) 3280 return "EffectEvidenceSynthesis"; 3281 if (code == FHIRResourceType.ENCOUNTER) 3282 return "Encounter"; 3283 if (code == FHIRResourceType.ENDPOINT) 3284 return "Endpoint"; 3285 if (code == FHIRResourceType.ENROLLMENTREQUEST) 3286 return "EnrollmentRequest"; 3287 if (code == FHIRResourceType.ENROLLMENTRESPONSE) 3288 return "EnrollmentResponse"; 3289 if (code == FHIRResourceType.EPISODEOFCARE) 3290 return "EpisodeOfCare"; 3291 if (code == FHIRResourceType.EVENTDEFINITION) 3292 return "EventDefinition"; 3293 if (code == FHIRResourceType.EVIDENCE) 3294 return "Evidence"; 3295 if (code == FHIRResourceType.EVIDENCEVARIABLE) 3296 return "EvidenceVariable"; 3297 if (code == FHIRResourceType.EXAMPLESCENARIO) 3298 return "ExampleScenario"; 3299 if (code == FHIRResourceType.EXPLANATIONOFBENEFIT) 3300 return "ExplanationOfBenefit"; 3301 if (code == FHIRResourceType.FAMILYMEMBERHISTORY) 3302 return "FamilyMemberHistory"; 3303 if (code == FHIRResourceType.FLAG) 3304 return "Flag"; 3305 if (code == FHIRResourceType.GOAL) 3306 return "Goal"; 3307 if (code == FHIRResourceType.GRAPHDEFINITION) 3308 return "GraphDefinition"; 3309 if (code == FHIRResourceType.GROUP) 3310 return "Group"; 3311 if (code == FHIRResourceType.GUIDANCERESPONSE) 3312 return "GuidanceResponse"; 3313 if (code == FHIRResourceType.HEALTHCARESERVICE) 3314 return "HealthcareService"; 3315 if (code == FHIRResourceType.IMAGINGSTUDY) 3316 return "ImagingStudy"; 3317 if (code == FHIRResourceType.IMMUNIZATION) 3318 return "Immunization"; 3319 if (code == FHIRResourceType.IMMUNIZATIONEVALUATION) 3320 return "ImmunizationEvaluation"; 3321 if (code == FHIRResourceType.IMMUNIZATIONRECOMMENDATION) 3322 return "ImmunizationRecommendation"; 3323 if (code == FHIRResourceType.IMPLEMENTATIONGUIDE) 3324 return "ImplementationGuide"; 3325 if (code == FHIRResourceType.INSURANCEPLAN) 3326 return "InsurancePlan"; 3327 if (code == FHIRResourceType.INVOICE) 3328 return "Invoice"; 3329 if (code == FHIRResourceType.LIBRARY) 3330 return "Library"; 3331 if (code == FHIRResourceType.LINKAGE) 3332 return "Linkage"; 3333 if (code == FHIRResourceType.LIST) 3334 return "List"; 3335 if (code == FHIRResourceType.LOCATION) 3336 return "Location"; 3337 if (code == FHIRResourceType.MEASURE) 3338 return "Measure"; 3339 if (code == FHIRResourceType.MEASUREREPORT) 3340 return "MeasureReport"; 3341 if (code == FHIRResourceType.MEDIA) 3342 return "Media"; 3343 if (code == FHIRResourceType.MEDICATION) 3344 return "Medication"; 3345 if (code == FHIRResourceType.MEDICATIONADMINISTRATION) 3346 return "MedicationAdministration"; 3347 if (code == FHIRResourceType.MEDICATIONDISPENSE) 3348 return "MedicationDispense"; 3349 if (code == FHIRResourceType.MEDICATIONKNOWLEDGE) 3350 return "MedicationKnowledge"; 3351 if (code == FHIRResourceType.MEDICATIONREQUEST) 3352 return "MedicationRequest"; 3353 if (code == FHIRResourceType.MEDICATIONSTATEMENT) 3354 return "MedicationStatement"; 3355 if (code == FHIRResourceType.MEDICINALPRODUCT) 3356 return "MedicinalProduct"; 3357 if (code == FHIRResourceType.MEDICINALPRODUCTAUTHORIZATION) 3358 return "MedicinalProductAuthorization"; 3359 if (code == FHIRResourceType.MEDICINALPRODUCTCONTRAINDICATION) 3360 return "MedicinalProductContraindication"; 3361 if (code == FHIRResourceType.MEDICINALPRODUCTINDICATION) 3362 return "MedicinalProductIndication"; 3363 if (code == FHIRResourceType.MEDICINALPRODUCTINGREDIENT) 3364 return "MedicinalProductIngredient"; 3365 if (code == FHIRResourceType.MEDICINALPRODUCTINTERACTION) 3366 return "MedicinalProductInteraction"; 3367 if (code == FHIRResourceType.MEDICINALPRODUCTMANUFACTURED) 3368 return "MedicinalProductManufactured"; 3369 if (code == FHIRResourceType.MEDICINALPRODUCTPACKAGED) 3370 return "MedicinalProductPackaged"; 3371 if (code == FHIRResourceType.MEDICINALPRODUCTPHARMACEUTICAL) 3372 return "MedicinalProductPharmaceutical"; 3373 if (code == FHIRResourceType.MEDICINALPRODUCTUNDESIRABLEEFFECT) 3374 return "MedicinalProductUndesirableEffect"; 3375 if (code == FHIRResourceType.MESSAGEDEFINITION) 3376 return "MessageDefinition"; 3377 if (code == FHIRResourceType.MESSAGEHEADER) 3378 return "MessageHeader"; 3379 if (code == FHIRResourceType.MOLECULARSEQUENCE) 3380 return "MolecularSequence"; 3381 if (code == FHIRResourceType.NAMINGSYSTEM) 3382 return "NamingSystem"; 3383 if (code == FHIRResourceType.NUTRITIONORDER) 3384 return "NutritionOrder"; 3385 if (code == FHIRResourceType.OBSERVATION) 3386 return "Observation"; 3387 if (code == FHIRResourceType.OBSERVATIONDEFINITION) 3388 return "ObservationDefinition"; 3389 if (code == FHIRResourceType.OPERATIONDEFINITION) 3390 return "OperationDefinition"; 3391 if (code == FHIRResourceType.OPERATIONOUTCOME) 3392 return "OperationOutcome"; 3393 if (code == FHIRResourceType.ORGANIZATION) 3394 return "Organization"; 3395 if (code == FHIRResourceType.ORGANIZATIONAFFILIATION) 3396 return "OrganizationAffiliation"; 3397 if (code == FHIRResourceType.PARAMETERS) 3398 return "Parameters"; 3399 if (code == FHIRResourceType.PATIENT) 3400 return "Patient"; 3401 if (code == FHIRResourceType.PAYMENTNOTICE) 3402 return "PaymentNotice"; 3403 if (code == FHIRResourceType.PAYMENTRECONCILIATION) 3404 return "PaymentReconciliation"; 3405 if (code == FHIRResourceType.PERSON) 3406 return "Person"; 3407 if (code == FHIRResourceType.PLANDEFINITION) 3408 return "PlanDefinition"; 3409 if (code == FHIRResourceType.PRACTITIONER) 3410 return "Practitioner"; 3411 if (code == FHIRResourceType.PRACTITIONERROLE) 3412 return "PractitionerRole"; 3413 if (code == FHIRResourceType.PROCEDURE) 3414 return "Procedure"; 3415 if (code == FHIRResourceType.PROVENANCE) 3416 return "Provenance"; 3417 if (code == FHIRResourceType.QUESTIONNAIRE) 3418 return "Questionnaire"; 3419 if (code == FHIRResourceType.QUESTIONNAIRERESPONSE) 3420 return "QuestionnaireResponse"; 3421 if (code == FHIRResourceType.RELATEDPERSON) 3422 return "RelatedPerson"; 3423 if (code == FHIRResourceType.REQUESTGROUP) 3424 return "RequestGroup"; 3425 if (code == FHIRResourceType.RESEARCHDEFINITION) 3426 return "ResearchDefinition"; 3427 if (code == FHIRResourceType.RESEARCHELEMENTDEFINITION) 3428 return "ResearchElementDefinition"; 3429 if (code == FHIRResourceType.RESEARCHSTUDY) 3430 return "ResearchStudy"; 3431 if (code == FHIRResourceType.RESEARCHSUBJECT) 3432 return "ResearchSubject"; 3433 if (code == FHIRResourceType.RESOURCE) 3434 return "Resource"; 3435 if (code == FHIRResourceType.RISKASSESSMENT) 3436 return "RiskAssessment"; 3437 if (code == FHIRResourceType.RISKEVIDENCESYNTHESIS) 3438 return "RiskEvidenceSynthesis"; 3439 if (code == FHIRResourceType.SCHEDULE) 3440 return "Schedule"; 3441 if (code == FHIRResourceType.SEARCHPARAMETER) 3442 return "SearchParameter"; 3443 if (code == FHIRResourceType.SERVICEREQUEST) 3444 return "ServiceRequest"; 3445 if (code == FHIRResourceType.SLOT) 3446 return "Slot"; 3447 if (code == FHIRResourceType.SPECIMEN) 3448 return "Specimen"; 3449 if (code == FHIRResourceType.SPECIMENDEFINITION) 3450 return "SpecimenDefinition"; 3451 if (code == FHIRResourceType.STRUCTUREDEFINITION) 3452 return "StructureDefinition"; 3453 if (code == FHIRResourceType.STRUCTUREMAP) 3454 return "StructureMap"; 3455 if (code == FHIRResourceType.SUBSCRIPTION) 3456 return "Subscription"; 3457 if (code == FHIRResourceType.SUBSTANCE) 3458 return "Substance"; 3459 if (code == FHIRResourceType.SUBSTANCENUCLEICACID) 3460 return "SubstanceNucleicAcid"; 3461 if (code == FHIRResourceType.SUBSTANCEPOLYMER) 3462 return "SubstancePolymer"; 3463 if (code == FHIRResourceType.SUBSTANCEPROTEIN) 3464 return "SubstanceProtein"; 3465 if (code == FHIRResourceType.SUBSTANCEREFERENCEINFORMATION) 3466 return "SubstanceReferenceInformation"; 3467 if (code == FHIRResourceType.SUBSTANCESOURCEMATERIAL) 3468 return "SubstanceSourceMaterial"; 3469 if (code == FHIRResourceType.SUBSTANCESPECIFICATION) 3470 return "SubstanceSpecification"; 3471 if (code == FHIRResourceType.SUPPLYDELIVERY) 3472 return "SupplyDelivery"; 3473 if (code == FHIRResourceType.SUPPLYREQUEST) 3474 return "SupplyRequest"; 3475 if (code == FHIRResourceType.TASK) 3476 return "Task"; 3477 if (code == FHIRResourceType.TERMINOLOGYCAPABILITIES) 3478 return "TerminologyCapabilities"; 3479 if (code == FHIRResourceType.TESTREPORT) 3480 return "TestReport"; 3481 if (code == FHIRResourceType.TESTSCRIPT) 3482 return "TestScript"; 3483 if (code == FHIRResourceType.VALUESET) 3484 return "ValueSet"; 3485 if (code == FHIRResourceType.VERIFICATIONRESULT) 3486 return "VerificationResult"; 3487 if (code == FHIRResourceType.VISIONPRESCRIPTION) 3488 return "VisionPrescription"; 3489 return "?"; 3490 } 3491 3492 public String toSystem(FHIRResourceType code) { 3493 return code.getSystem(); 3494 } 3495 } 3496 3497 @Block() 3498 public static class ExampleScenarioActorComponent extends BackboneElement implements IBaseBackboneElement { 3499 /** 3500 * ID or acronym of actor. 3501 */ 3502 @Child(name = "actorId", type = { 3503 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3504 @Description(shortDefinition = "ID or acronym of the actor", formalDefinition = "ID or acronym of actor.") 3505 protected StringType actorId; 3506 3507 /** 3508 * The type of actor - person or system. 3509 */ 3510 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3511 @Description(shortDefinition = "person | entity", formalDefinition = "The type of actor - person or system.") 3512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/examplescenario-actor-type") 3513 protected Enumeration<ExampleScenarioActorType> type; 3514 3515 /** 3516 * The name of the actor as shown in the page. 3517 */ 3518 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3519 @Description(shortDefinition = "The name of the actor as shown in the page", formalDefinition = "The name of the actor as shown in the page.") 3520 protected StringType name; 3521 3522 /** 3523 * The description of the actor. 3524 */ 3525 @Child(name = "description", type = { 3526 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3527 @Description(shortDefinition = "The description of the actor", formalDefinition = "The description of the actor.") 3528 protected MarkdownType description; 3529 3530 private static final long serialVersionUID = 1348364162L; 3531 3532 /** 3533 * Constructor 3534 */ 3535 public ExampleScenarioActorComponent() { 3536 super(); 3537 } 3538 3539 /** 3540 * Constructor 3541 */ 3542 public ExampleScenarioActorComponent(StringType actorId, Enumeration<ExampleScenarioActorType> type) { 3543 super(); 3544 this.actorId = actorId; 3545 this.type = type; 3546 } 3547 3548 /** 3549 * @return {@link #actorId} (ID or acronym of actor.). This is the underlying 3550 * object with id, value and extensions. The accessor "getActorId" gives 3551 * direct access to the value 3552 */ 3553 public StringType getActorIdElement() { 3554 if (this.actorId == null) 3555 if (Configuration.errorOnAutoCreate()) 3556 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.actorId"); 3557 else if (Configuration.doAutoCreate()) 3558 this.actorId = new StringType(); // bb 3559 return this.actorId; 3560 } 3561 3562 public boolean hasActorIdElement() { 3563 return this.actorId != null && !this.actorId.isEmpty(); 3564 } 3565 3566 public boolean hasActorId() { 3567 return this.actorId != null && !this.actorId.isEmpty(); 3568 } 3569 3570 /** 3571 * @param value {@link #actorId} (ID or acronym of actor.). This is the 3572 * underlying object with id, value and extensions. The accessor 3573 * "getActorId" gives direct access to the value 3574 */ 3575 public ExampleScenarioActorComponent setActorIdElement(StringType value) { 3576 this.actorId = value; 3577 return this; 3578 } 3579 3580 /** 3581 * @return ID or acronym of actor. 3582 */ 3583 public String getActorId() { 3584 return this.actorId == null ? null : this.actorId.getValue(); 3585 } 3586 3587 /** 3588 * @param value ID or acronym of actor. 3589 */ 3590 public ExampleScenarioActorComponent setActorId(String value) { 3591 if (this.actorId == null) 3592 this.actorId = new StringType(); 3593 this.actorId.setValue(value); 3594 return this; 3595 } 3596 3597 /** 3598 * @return {@link #type} (The type of actor - person or system.). This is the 3599 * underlying object with id, value and extensions. The accessor 3600 * "getType" gives direct access to the value 3601 */ 3602 public Enumeration<ExampleScenarioActorType> getTypeElement() { 3603 if (this.type == null) 3604 if (Configuration.errorOnAutoCreate()) 3605 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.type"); 3606 else if (Configuration.doAutoCreate()) 3607 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); // bb 3608 return this.type; 3609 } 3610 3611 public boolean hasTypeElement() { 3612 return this.type != null && !this.type.isEmpty(); 3613 } 3614 3615 public boolean hasType() { 3616 return this.type != null && !this.type.isEmpty(); 3617 } 3618 3619 /** 3620 * @param value {@link #type} (The type of actor - person or system.). This is 3621 * the underlying object with id, value and extensions. The 3622 * accessor "getType" gives direct access to the value 3623 */ 3624 public ExampleScenarioActorComponent setTypeElement(Enumeration<ExampleScenarioActorType> value) { 3625 this.type = value; 3626 return this; 3627 } 3628 3629 /** 3630 * @return The type of actor - person or system. 3631 */ 3632 public ExampleScenarioActorType getType() { 3633 return this.type == null ? null : this.type.getValue(); 3634 } 3635 3636 /** 3637 * @param value The type of actor - person or system. 3638 */ 3639 public ExampleScenarioActorComponent setType(ExampleScenarioActorType value) { 3640 if (this.type == null) 3641 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); 3642 this.type.setValue(value); 3643 return this; 3644 } 3645 3646 /** 3647 * @return {@link #name} (The name of the actor as shown in the page.). This is 3648 * the underlying object with id, value and extensions. The accessor 3649 * "getName" gives direct access to the value 3650 */ 3651 public StringType getNameElement() { 3652 if (this.name == null) 3653 if (Configuration.errorOnAutoCreate()) 3654 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.name"); 3655 else if (Configuration.doAutoCreate()) 3656 this.name = new StringType(); // bb 3657 return this.name; 3658 } 3659 3660 public boolean hasNameElement() { 3661 return this.name != null && !this.name.isEmpty(); 3662 } 3663 3664 public boolean hasName() { 3665 return this.name != null && !this.name.isEmpty(); 3666 } 3667 3668 /** 3669 * @param value {@link #name} (The name of the actor as shown in the page.). 3670 * This is the underlying object with id, value and extensions. The 3671 * accessor "getName" gives direct access to the value 3672 */ 3673 public ExampleScenarioActorComponent setNameElement(StringType value) { 3674 this.name = value; 3675 return this; 3676 } 3677 3678 /** 3679 * @return The name of the actor as shown in the page. 3680 */ 3681 public String getName() { 3682 return this.name == null ? null : this.name.getValue(); 3683 } 3684 3685 /** 3686 * @param value The name of the actor as shown in the page. 3687 */ 3688 public ExampleScenarioActorComponent setName(String value) { 3689 if (Utilities.noString(value)) 3690 this.name = null; 3691 else { 3692 if (this.name == null) 3693 this.name = new StringType(); 3694 this.name.setValue(value); 3695 } 3696 return this; 3697 } 3698 3699 /** 3700 * @return {@link #description} (The description of the actor.). This is the 3701 * underlying object with id, value and extensions. The accessor 3702 * "getDescription" gives direct access to the value 3703 */ 3704 public MarkdownType getDescriptionElement() { 3705 if (this.description == null) 3706 if (Configuration.errorOnAutoCreate()) 3707 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.description"); 3708 else if (Configuration.doAutoCreate()) 3709 this.description = new MarkdownType(); // bb 3710 return this.description; 3711 } 3712 3713 public boolean hasDescriptionElement() { 3714 return this.description != null && !this.description.isEmpty(); 3715 } 3716 3717 public boolean hasDescription() { 3718 return this.description != null && !this.description.isEmpty(); 3719 } 3720 3721 /** 3722 * @param value {@link #description} (The description of the actor.). This is 3723 * the underlying object with id, value and extensions. The 3724 * accessor "getDescription" gives direct access to the value 3725 */ 3726 public ExampleScenarioActorComponent setDescriptionElement(MarkdownType value) { 3727 this.description = value; 3728 return this; 3729 } 3730 3731 /** 3732 * @return The description of the actor. 3733 */ 3734 public String getDescription() { 3735 return this.description == null ? null : this.description.getValue(); 3736 } 3737 3738 /** 3739 * @param value The description of the actor. 3740 */ 3741 public ExampleScenarioActorComponent setDescription(String value) { 3742 if (value == null) 3743 this.description = null; 3744 else { 3745 if (this.description == null) 3746 this.description = new MarkdownType(); 3747 this.description.setValue(value); 3748 } 3749 return this; 3750 } 3751 3752 protected void listChildren(List<Property> children) { 3753 super.listChildren(children); 3754 children.add(new Property("actorId", "string", "ID or acronym of actor.", 0, 1, actorId)); 3755 children.add(new Property("type", "code", "The type of actor - person or system.", 0, 1, type)); 3756 children.add(new Property("name", "string", "The name of the actor as shown in the page.", 0, 1, name)); 3757 children.add(new Property("description", "markdown", "The description of the actor.", 0, 1, description)); 3758 } 3759 3760 @Override 3761 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3762 switch (_hash) { 3763 case -1161623056: 3764 /* actorId */ return new Property("actorId", "string", "ID or acronym of actor.", 0, 1, actorId); 3765 case 3575610: 3766 /* type */ return new Property("type", "code", "The type of actor - person or system.", 0, 1, type); 3767 case 3373707: 3768 /* name */ return new Property("name", "string", "The name of the actor as shown in the page.", 0, 1, name); 3769 case -1724546052: 3770 /* description */ return new Property("description", "markdown", "The description of the actor.", 0, 1, 3771 description); 3772 default: 3773 return super.getNamedProperty(_hash, _name, _checkValid); 3774 } 3775 3776 } 3777 3778 @Override 3779 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3780 switch (hash) { 3781 case -1161623056: 3782 /* actorId */ return this.actorId == null ? new Base[0] : new Base[] { this.actorId }; // StringType 3783 case 3575610: 3784 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<ExampleScenarioActorType> 3785 case 3373707: 3786 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3787 case -1724546052: 3788 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 3789 default: 3790 return super.getProperty(hash, name, checkValid); 3791 } 3792 3793 } 3794 3795 @Override 3796 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3797 switch (hash) { 3798 case -1161623056: // actorId 3799 this.actorId = castToString(value); // StringType 3800 return value; 3801 case 3575610: // type 3802 value = new ExampleScenarioActorTypeEnumFactory().fromType(castToCode(value)); 3803 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 3804 return value; 3805 case 3373707: // name 3806 this.name = castToString(value); // StringType 3807 return value; 3808 case -1724546052: // description 3809 this.description = castToMarkdown(value); // MarkdownType 3810 return value; 3811 default: 3812 return super.setProperty(hash, name, value); 3813 } 3814 3815 } 3816 3817 @Override 3818 public Base setProperty(String name, Base value) throws FHIRException { 3819 if (name.equals("actorId")) { 3820 this.actorId = castToString(value); // StringType 3821 } else if (name.equals("type")) { 3822 value = new ExampleScenarioActorTypeEnumFactory().fromType(castToCode(value)); 3823 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 3824 } else if (name.equals("name")) { 3825 this.name = castToString(value); // StringType 3826 } else if (name.equals("description")) { 3827 this.description = castToMarkdown(value); // MarkdownType 3828 } else 3829 return super.setProperty(name, value); 3830 return value; 3831 } 3832 3833 @Override 3834 public void removeChild(String name, Base value) throws FHIRException { 3835 if (name.equals("actorId")) { 3836 this.actorId = null; 3837 } else if (name.equals("type")) { 3838 this.type = null; 3839 } else if (name.equals("name")) { 3840 this.name = null; 3841 } else if (name.equals("description")) { 3842 this.description = null; 3843 } else 3844 super.removeChild(name, value); 3845 3846 } 3847 3848 @Override 3849 public Base makeProperty(int hash, String name) throws FHIRException { 3850 switch (hash) { 3851 case -1161623056: 3852 return getActorIdElement(); 3853 case 3575610: 3854 return getTypeElement(); 3855 case 3373707: 3856 return getNameElement(); 3857 case -1724546052: 3858 return getDescriptionElement(); 3859 default: 3860 return super.makeProperty(hash, name); 3861 } 3862 3863 } 3864 3865 @Override 3866 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3867 switch (hash) { 3868 case -1161623056: 3869 /* actorId */ return new String[] { "string" }; 3870 case 3575610: 3871 /* type */ return new String[] { "code" }; 3872 case 3373707: 3873 /* name */ return new String[] { "string" }; 3874 case -1724546052: 3875 /* description */ return new String[] { "markdown" }; 3876 default: 3877 return super.getTypesForProperty(hash, name); 3878 } 3879 3880 } 3881 3882 @Override 3883 public Base addChild(String name) throws FHIRException { 3884 if (name.equals("actorId")) { 3885 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actorId"); 3886 } else if (name.equals("type")) { 3887 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.type"); 3888 } else if (name.equals("name")) { 3889 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 3890 } else if (name.equals("description")) { 3891 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 3892 } else 3893 return super.addChild(name); 3894 } 3895 3896 public ExampleScenarioActorComponent copy() { 3897 ExampleScenarioActorComponent dst = new ExampleScenarioActorComponent(); 3898 copyValues(dst); 3899 return dst; 3900 } 3901 3902 public void copyValues(ExampleScenarioActorComponent dst) { 3903 super.copyValues(dst); 3904 dst.actorId = actorId == null ? null : actorId.copy(); 3905 dst.type = type == null ? null : type.copy(); 3906 dst.name = name == null ? null : name.copy(); 3907 dst.description = description == null ? null : description.copy(); 3908 } 3909 3910 @Override 3911 public boolean equalsDeep(Base other_) { 3912 if (!super.equalsDeep(other_)) 3913 return false; 3914 if (!(other_ instanceof ExampleScenarioActorComponent)) 3915 return false; 3916 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 3917 return compareDeep(actorId, o.actorId, true) && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) 3918 && compareDeep(description, o.description, true); 3919 } 3920 3921 @Override 3922 public boolean equalsShallow(Base other_) { 3923 if (!super.equalsShallow(other_)) 3924 return false; 3925 if (!(other_ instanceof ExampleScenarioActorComponent)) 3926 return false; 3927 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 3928 return compareValues(actorId, o.actorId, true) && compareValues(type, o.type, true) 3929 && compareValues(name, o.name, true) && compareValues(description, o.description, true); 3930 } 3931 3932 public boolean isEmpty() { 3933 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actorId, type, name, description); 3934 } 3935 3936 public String fhirType() { 3937 return "ExampleScenario.actor"; 3938 3939 } 3940 3941 } 3942 3943 @Block() 3944 public static class ExampleScenarioInstanceComponent extends BackboneElement implements IBaseBackboneElement { 3945 /** 3946 * The id of the resource for referencing. 3947 */ 3948 @Child(name = "resourceId", type = { 3949 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3950 @Description(shortDefinition = "The id of the resource for referencing", formalDefinition = "The id of the resource for referencing.") 3951 protected StringType resourceId; 3952 3953 /** 3954 * The type of the resource. 3955 */ 3956 @Child(name = "resourceType", type = { 3957 CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3958 @Description(shortDefinition = "The type of the resource", formalDefinition = "The type of the resource.") 3959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 3960 protected Enumeration<FHIRResourceType> resourceType; 3961 3962 /** 3963 * A short name for the resource instance. 3964 */ 3965 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 3966 @Description(shortDefinition = "A short name for the resource instance", formalDefinition = "A short name for the resource instance.") 3967 protected StringType name; 3968 3969 /** 3970 * Human-friendly description of the resource instance. 3971 */ 3972 @Child(name = "description", type = { 3973 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3974 @Description(shortDefinition = "Human-friendly description of the resource instance", formalDefinition = "Human-friendly description of the resource instance.") 3975 protected MarkdownType description; 3976 3977 /** 3978 * A specific version of the resource. 3979 */ 3980 @Child(name = "version", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3981 @Description(shortDefinition = "A specific version of the resource", formalDefinition = "A specific version of the resource.") 3982 protected List<ExampleScenarioInstanceVersionComponent> version; 3983 3984 /** 3985 * Resources contained in the instance (e.g. the observations contained in a 3986 * bundle). 3987 */ 3988 @Child(name = "containedInstance", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3989 @Description(shortDefinition = "Resources contained in the instance", formalDefinition = "Resources contained in the instance (e.g. the observations contained in a bundle).") 3990 protected List<ExampleScenarioInstanceContainedInstanceComponent> containedInstance; 3991 3992 private static final long serialVersionUID = -1131860669L; 3993 3994 /** 3995 * Constructor 3996 */ 3997 public ExampleScenarioInstanceComponent() { 3998 super(); 3999 } 4000 4001 /** 4002 * Constructor 4003 */ 4004 public ExampleScenarioInstanceComponent(StringType resourceId, Enumeration<FHIRResourceType> resourceType) { 4005 super(); 4006 this.resourceId = resourceId; 4007 this.resourceType = resourceType; 4008 } 4009 4010 /** 4011 * @return {@link #resourceId} (The id of the resource for referencing.). This 4012 * is the underlying object with id, value and extensions. The accessor 4013 * "getResourceId" gives direct access to the value 4014 */ 4015 public StringType getResourceIdElement() { 4016 if (this.resourceId == null) 4017 if (Configuration.errorOnAutoCreate()) 4018 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.resourceId"); 4019 else if (Configuration.doAutoCreate()) 4020 this.resourceId = new StringType(); // bb 4021 return this.resourceId; 4022 } 4023 4024 public boolean hasResourceIdElement() { 4025 return this.resourceId != null && !this.resourceId.isEmpty(); 4026 } 4027 4028 public boolean hasResourceId() { 4029 return this.resourceId != null && !this.resourceId.isEmpty(); 4030 } 4031 4032 /** 4033 * @param value {@link #resourceId} (The id of the resource for referencing.). 4034 * This is the underlying object with id, value and extensions. The 4035 * accessor "getResourceId" gives direct access to the value 4036 */ 4037 public ExampleScenarioInstanceComponent setResourceIdElement(StringType value) { 4038 this.resourceId = value; 4039 return this; 4040 } 4041 4042 /** 4043 * @return The id of the resource for referencing. 4044 */ 4045 public String getResourceId() { 4046 return this.resourceId == null ? null : this.resourceId.getValue(); 4047 } 4048 4049 /** 4050 * @param value The id of the resource for referencing. 4051 */ 4052 public ExampleScenarioInstanceComponent setResourceId(String value) { 4053 if (this.resourceId == null) 4054 this.resourceId = new StringType(); 4055 this.resourceId.setValue(value); 4056 return this; 4057 } 4058 4059 /** 4060 * @return {@link #resourceType} (The type of the resource.). This is the 4061 * underlying object with id, value and extensions. The accessor 4062 * "getResourceType" gives direct access to the value 4063 */ 4064 public Enumeration<FHIRResourceType> getResourceTypeElement() { 4065 if (this.resourceType == null) 4066 if (Configuration.errorOnAutoCreate()) 4067 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.resourceType"); 4068 else if (Configuration.doAutoCreate()) 4069 this.resourceType = new Enumeration<FHIRResourceType>(new FHIRResourceTypeEnumFactory()); // bb 4070 return this.resourceType; 4071 } 4072 4073 public boolean hasResourceTypeElement() { 4074 return this.resourceType != null && !this.resourceType.isEmpty(); 4075 } 4076 4077 public boolean hasResourceType() { 4078 return this.resourceType != null && !this.resourceType.isEmpty(); 4079 } 4080 4081 /** 4082 * @param value {@link #resourceType} (The type of the resource.). This is the 4083 * underlying object with id, value and extensions. The accessor 4084 * "getResourceType" gives direct access to the value 4085 */ 4086 public ExampleScenarioInstanceComponent setResourceTypeElement(Enumeration<FHIRResourceType> value) { 4087 this.resourceType = value; 4088 return this; 4089 } 4090 4091 /** 4092 * @return The type of the resource. 4093 */ 4094 public FHIRResourceType getResourceType() { 4095 return this.resourceType == null ? null : this.resourceType.getValue(); 4096 } 4097 4098 /** 4099 * @param value The type of the resource. 4100 */ 4101 public ExampleScenarioInstanceComponent setResourceType(FHIRResourceType value) { 4102 if (this.resourceType == null) 4103 this.resourceType = new Enumeration<FHIRResourceType>(new FHIRResourceTypeEnumFactory()); 4104 this.resourceType.setValue(value); 4105 return this; 4106 } 4107 4108 /** 4109 * @return {@link #name} (A short name for the resource instance.). This is the 4110 * underlying object with id, value and extensions. The accessor 4111 * "getName" gives direct access to the value 4112 */ 4113 public StringType getNameElement() { 4114 if (this.name == null) 4115 if (Configuration.errorOnAutoCreate()) 4116 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.name"); 4117 else if (Configuration.doAutoCreate()) 4118 this.name = new StringType(); // bb 4119 return this.name; 4120 } 4121 4122 public boolean hasNameElement() { 4123 return this.name != null && !this.name.isEmpty(); 4124 } 4125 4126 public boolean hasName() { 4127 return this.name != null && !this.name.isEmpty(); 4128 } 4129 4130 /** 4131 * @param value {@link #name} (A short name for the resource instance.). This is 4132 * the underlying object with id, value and extensions. The 4133 * accessor "getName" gives direct access to the value 4134 */ 4135 public ExampleScenarioInstanceComponent setNameElement(StringType value) { 4136 this.name = value; 4137 return this; 4138 } 4139 4140 /** 4141 * @return A short name for the resource instance. 4142 */ 4143 public String getName() { 4144 return this.name == null ? null : this.name.getValue(); 4145 } 4146 4147 /** 4148 * @param value A short name for the resource instance. 4149 */ 4150 public ExampleScenarioInstanceComponent setName(String value) { 4151 if (Utilities.noString(value)) 4152 this.name = null; 4153 else { 4154 if (this.name == null) 4155 this.name = new StringType(); 4156 this.name.setValue(value); 4157 } 4158 return this; 4159 } 4160 4161 /** 4162 * @return {@link #description} (Human-friendly description of the resource 4163 * instance.). This is the underlying object with id, value and 4164 * extensions. The accessor "getDescription" gives direct access to the 4165 * value 4166 */ 4167 public MarkdownType getDescriptionElement() { 4168 if (this.description == null) 4169 if (Configuration.errorOnAutoCreate()) 4170 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.description"); 4171 else if (Configuration.doAutoCreate()) 4172 this.description = new MarkdownType(); // bb 4173 return this.description; 4174 } 4175 4176 public boolean hasDescriptionElement() { 4177 return this.description != null && !this.description.isEmpty(); 4178 } 4179 4180 public boolean hasDescription() { 4181 return this.description != null && !this.description.isEmpty(); 4182 } 4183 4184 /** 4185 * @param value {@link #description} (Human-friendly description of the resource 4186 * instance.). This is the underlying object with id, value and 4187 * extensions. The accessor "getDescription" gives direct access to 4188 * the value 4189 */ 4190 public ExampleScenarioInstanceComponent setDescriptionElement(MarkdownType value) { 4191 this.description = value; 4192 return this; 4193 } 4194 4195 /** 4196 * @return Human-friendly description of the resource instance. 4197 */ 4198 public String getDescription() { 4199 return this.description == null ? null : this.description.getValue(); 4200 } 4201 4202 /** 4203 * @param value Human-friendly description of the resource instance. 4204 */ 4205 public ExampleScenarioInstanceComponent setDescription(String value) { 4206 if (value == null) 4207 this.description = null; 4208 else { 4209 if (this.description == null) 4210 this.description = new MarkdownType(); 4211 this.description.setValue(value); 4212 } 4213 return this; 4214 } 4215 4216 /** 4217 * @return {@link #version} (A specific version of the resource.) 4218 */ 4219 public List<ExampleScenarioInstanceVersionComponent> getVersion() { 4220 if (this.version == null) 4221 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4222 return this.version; 4223 } 4224 4225 /** 4226 * @return Returns a reference to <code>this</code> for easy method chaining 4227 */ 4228 public ExampleScenarioInstanceComponent setVersion(List<ExampleScenarioInstanceVersionComponent> theVersion) { 4229 this.version = theVersion; 4230 return this; 4231 } 4232 4233 public boolean hasVersion() { 4234 if (this.version == null) 4235 return false; 4236 for (ExampleScenarioInstanceVersionComponent item : this.version) 4237 if (!item.isEmpty()) 4238 return true; 4239 return false; 4240 } 4241 4242 public ExampleScenarioInstanceVersionComponent addVersion() { // 3 4243 ExampleScenarioInstanceVersionComponent t = new ExampleScenarioInstanceVersionComponent(); 4244 if (this.version == null) 4245 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4246 this.version.add(t); 4247 return t; 4248 } 4249 4250 public ExampleScenarioInstanceComponent addVersion(ExampleScenarioInstanceVersionComponent t) { // 3 4251 if (t == null) 4252 return this; 4253 if (this.version == null) 4254 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4255 this.version.add(t); 4256 return this; 4257 } 4258 4259 /** 4260 * @return The first repetition of repeating field {@link #version}, creating it 4261 * if it does not already exist 4262 */ 4263 public ExampleScenarioInstanceVersionComponent getVersionFirstRep() { 4264 if (getVersion().isEmpty()) { 4265 addVersion(); 4266 } 4267 return getVersion().get(0); 4268 } 4269 4270 /** 4271 * @return {@link #containedInstance} (Resources contained in the instance (e.g. 4272 * the observations contained in a bundle).) 4273 */ 4274 public List<ExampleScenarioInstanceContainedInstanceComponent> getContainedInstance() { 4275 if (this.containedInstance == null) 4276 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4277 return this.containedInstance; 4278 } 4279 4280 /** 4281 * @return Returns a reference to <code>this</code> for easy method chaining 4282 */ 4283 public ExampleScenarioInstanceComponent setContainedInstance( 4284 List<ExampleScenarioInstanceContainedInstanceComponent> theContainedInstance) { 4285 this.containedInstance = theContainedInstance; 4286 return this; 4287 } 4288 4289 public boolean hasContainedInstance() { 4290 if (this.containedInstance == null) 4291 return false; 4292 for (ExampleScenarioInstanceContainedInstanceComponent item : this.containedInstance) 4293 if (!item.isEmpty()) 4294 return true; 4295 return false; 4296 } 4297 4298 public ExampleScenarioInstanceContainedInstanceComponent addContainedInstance() { // 3 4299 ExampleScenarioInstanceContainedInstanceComponent t = new ExampleScenarioInstanceContainedInstanceComponent(); 4300 if (this.containedInstance == null) 4301 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4302 this.containedInstance.add(t); 4303 return t; 4304 } 4305 4306 public ExampleScenarioInstanceComponent addContainedInstance(ExampleScenarioInstanceContainedInstanceComponent t) { // 3 4307 if (t == null) 4308 return this; 4309 if (this.containedInstance == null) 4310 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4311 this.containedInstance.add(t); 4312 return this; 4313 } 4314 4315 /** 4316 * @return The first repetition of repeating field {@link #containedInstance}, 4317 * creating it if it does not already exist 4318 */ 4319 public ExampleScenarioInstanceContainedInstanceComponent getContainedInstanceFirstRep() { 4320 if (getContainedInstance().isEmpty()) { 4321 addContainedInstance(); 4322 } 4323 return getContainedInstance().get(0); 4324 } 4325 4326 protected void listChildren(List<Property> children) { 4327 super.listChildren(children); 4328 children.add(new Property("resourceId", "string", "The id of the resource for referencing.", 0, 1, resourceId)); 4329 children.add(new Property("resourceType", "code", "The type of the resource.", 0, 1, resourceType)); 4330 children.add(new Property("name", "string", "A short name for the resource instance.", 0, 1, name)); 4331 children.add(new Property("description", "markdown", "Human-friendly description of the resource instance.", 0, 1, 4332 description)); 4333 children.add( 4334 new Property("version", "", "A specific version of the resource.", 0, java.lang.Integer.MAX_VALUE, version)); 4335 children.add(new Property("containedInstance", "", 4336 "Resources contained in the instance (e.g. the observations contained in a bundle).", 0, 4337 java.lang.Integer.MAX_VALUE, containedInstance)); 4338 } 4339 4340 @Override 4341 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4342 switch (_hash) { 4343 case -1345650231: 4344 /* resourceId */ return new Property("resourceId", "string", "The id of the resource for referencing.", 0, 1, 4345 resourceId); 4346 case -384364440: 4347 /* resourceType */ return new Property("resourceType", "code", "The type of the resource.", 0, 1, resourceType); 4348 case 3373707: 4349 /* name */ return new Property("name", "string", "A short name for the resource instance.", 0, 1, name); 4350 case -1724546052: 4351 /* description */ return new Property("description", "markdown", 4352 "Human-friendly description of the resource instance.", 0, 1, description); 4353 case 351608024: 4354 /* version */ return new Property("version", "", "A specific version of the resource.", 0, 4355 java.lang.Integer.MAX_VALUE, version); 4356 case -417062360: 4357 /* containedInstance */ return new Property("containedInstance", "", 4358 "Resources contained in the instance (e.g. the observations contained in a bundle).", 0, 4359 java.lang.Integer.MAX_VALUE, containedInstance); 4360 default: 4361 return super.getNamedProperty(_hash, _name, _checkValid); 4362 } 4363 4364 } 4365 4366 @Override 4367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4368 switch (hash) { 4369 case -1345650231: 4370 /* resourceId */ return this.resourceId == null ? new Base[0] : new Base[] { this.resourceId }; // StringType 4371 case -384364440: 4372 /* resourceType */ return this.resourceType == null ? new Base[0] : new Base[] { this.resourceType }; // Enumeration<FHIRResourceType> 4373 case 3373707: 4374 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4375 case -1724546052: 4376 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4377 case 351608024: 4378 /* version */ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // ExampleScenarioInstanceVersionComponent 4379 case -417062360: 4380 /* containedInstance */ return this.containedInstance == null ? new Base[0] 4381 : this.containedInstance.toArray(new Base[this.containedInstance.size()]); // ExampleScenarioInstanceContainedInstanceComponent 4382 default: 4383 return super.getProperty(hash, name, checkValid); 4384 } 4385 4386 } 4387 4388 @Override 4389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4390 switch (hash) { 4391 case -1345650231: // resourceId 4392 this.resourceId = castToString(value); // StringType 4393 return value; 4394 case -384364440: // resourceType 4395 value = new FHIRResourceTypeEnumFactory().fromType(castToCode(value)); 4396 this.resourceType = (Enumeration) value; // Enumeration<FHIRResourceType> 4397 return value; 4398 case 3373707: // name 4399 this.name = castToString(value); // StringType 4400 return value; 4401 case -1724546052: // description 4402 this.description = castToMarkdown(value); // MarkdownType 4403 return value; 4404 case 351608024: // version 4405 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); // ExampleScenarioInstanceVersionComponent 4406 return value; 4407 case -417062360: // containedInstance 4408 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); // ExampleScenarioInstanceContainedInstanceComponent 4409 return value; 4410 default: 4411 return super.setProperty(hash, name, value); 4412 } 4413 4414 } 4415 4416 @Override 4417 public Base setProperty(String name, Base value) throws FHIRException { 4418 if (name.equals("resourceId")) { 4419 this.resourceId = castToString(value); // StringType 4420 } else if (name.equals("resourceType")) { 4421 value = new FHIRResourceTypeEnumFactory().fromType(castToCode(value)); 4422 this.resourceType = (Enumeration) value; // Enumeration<FHIRResourceType> 4423 } else if (name.equals("name")) { 4424 this.name = castToString(value); // StringType 4425 } else if (name.equals("description")) { 4426 this.description = castToMarkdown(value); // MarkdownType 4427 } else if (name.equals("version")) { 4428 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); 4429 } else if (name.equals("containedInstance")) { 4430 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); 4431 } else 4432 return super.setProperty(name, value); 4433 return value; 4434 } 4435 4436 @Override 4437 public void removeChild(String name, Base value) throws FHIRException { 4438 if (name.equals("resourceId")) { 4439 this.resourceId = null; 4440 } else if (name.equals("resourceType")) { 4441 this.resourceType = null; 4442 } else if (name.equals("name")) { 4443 this.name = null; 4444 } else if (name.equals("description")) { 4445 this.description = null; 4446 } else if (name.equals("version")) { 4447 this.getVersion().remove((ExampleScenarioInstanceVersionComponent) value); 4448 } else if (name.equals("containedInstance")) { 4449 this.getContainedInstance().remove((ExampleScenarioInstanceContainedInstanceComponent) value); 4450 } else 4451 super.removeChild(name, value); 4452 4453 } 4454 4455 @Override 4456 public Base makeProperty(int hash, String name) throws FHIRException { 4457 switch (hash) { 4458 case -1345650231: 4459 return getResourceIdElement(); 4460 case -384364440: 4461 return getResourceTypeElement(); 4462 case 3373707: 4463 return getNameElement(); 4464 case -1724546052: 4465 return getDescriptionElement(); 4466 case 351608024: 4467 return addVersion(); 4468 case -417062360: 4469 return addContainedInstance(); 4470 default: 4471 return super.makeProperty(hash, name); 4472 } 4473 4474 } 4475 4476 @Override 4477 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4478 switch (hash) { 4479 case -1345650231: 4480 /* resourceId */ return new String[] { "string" }; 4481 case -384364440: 4482 /* resourceType */ return new String[] { "code" }; 4483 case 3373707: 4484 /* name */ return new String[] { "string" }; 4485 case -1724546052: 4486 /* description */ return new String[] { "markdown" }; 4487 case 351608024: 4488 /* version */ return new String[] {}; 4489 case -417062360: 4490 /* containedInstance */ return new String[] {}; 4491 default: 4492 return super.getTypesForProperty(hash, name); 4493 } 4494 4495 } 4496 4497 @Override 4498 public Base addChild(String name) throws FHIRException { 4499 if (name.equals("resourceId")) { 4500 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceId"); 4501 } else if (name.equals("resourceType")) { 4502 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceType"); 4503 } else if (name.equals("name")) { 4504 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 4505 } else if (name.equals("description")) { 4506 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 4507 } else if (name.equals("version")) { 4508 return addVersion(); 4509 } else if (name.equals("containedInstance")) { 4510 return addContainedInstance(); 4511 } else 4512 return super.addChild(name); 4513 } 4514 4515 public ExampleScenarioInstanceComponent copy() { 4516 ExampleScenarioInstanceComponent dst = new ExampleScenarioInstanceComponent(); 4517 copyValues(dst); 4518 return dst; 4519 } 4520 4521 public void copyValues(ExampleScenarioInstanceComponent dst) { 4522 super.copyValues(dst); 4523 dst.resourceId = resourceId == null ? null : resourceId.copy(); 4524 dst.resourceType = resourceType == null ? null : resourceType.copy(); 4525 dst.name = name == null ? null : name.copy(); 4526 dst.description = description == null ? null : description.copy(); 4527 if (version != null) { 4528 dst.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 4529 for (ExampleScenarioInstanceVersionComponent i : version) 4530 dst.version.add(i.copy()); 4531 } 4532 ; 4533 if (containedInstance != null) { 4534 dst.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 4535 for (ExampleScenarioInstanceContainedInstanceComponent i : containedInstance) 4536 dst.containedInstance.add(i.copy()); 4537 } 4538 ; 4539 } 4540 4541 @Override 4542 public boolean equalsDeep(Base other_) { 4543 if (!super.equalsDeep(other_)) 4544 return false; 4545 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 4546 return false; 4547 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 4548 return compareDeep(resourceId, o.resourceId, true) && compareDeep(resourceType, o.resourceType, true) 4549 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 4550 && compareDeep(version, o.version, true) && compareDeep(containedInstance, o.containedInstance, true); 4551 } 4552 4553 @Override 4554 public boolean equalsShallow(Base other_) { 4555 if (!super.equalsShallow(other_)) 4556 return false; 4557 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 4558 return false; 4559 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 4560 return compareValues(resourceId, o.resourceId, true) && compareValues(resourceType, o.resourceType, true) 4561 && compareValues(name, o.name, true) && compareValues(description, o.description, true); 4562 } 4563 4564 public boolean isEmpty() { 4565 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resourceId, resourceType, name, description, 4566 version, containedInstance); 4567 } 4568 4569 public String fhirType() { 4570 return "ExampleScenario.instance"; 4571 4572 } 4573 4574 } 4575 4576 @Block() 4577 public static class ExampleScenarioInstanceVersionComponent extends BackboneElement implements IBaseBackboneElement { 4578 /** 4579 * The identifier of a specific version of a resource. 4580 */ 4581 @Child(name = "versionId", type = { 4582 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4583 @Description(shortDefinition = "The identifier of a specific version of a resource", formalDefinition = "The identifier of a specific version of a resource.") 4584 protected StringType versionId; 4585 4586 /** 4587 * The description of the resource version. 4588 */ 4589 @Child(name = "description", type = { 4590 MarkdownType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 4591 @Description(shortDefinition = "The description of the resource version", formalDefinition = "The description of the resource version.") 4592 protected MarkdownType description; 4593 4594 private static final long serialVersionUID = 960821913L; 4595 4596 /** 4597 * Constructor 4598 */ 4599 public ExampleScenarioInstanceVersionComponent() { 4600 super(); 4601 } 4602 4603 /** 4604 * Constructor 4605 */ 4606 public ExampleScenarioInstanceVersionComponent(StringType versionId, MarkdownType description) { 4607 super(); 4608 this.versionId = versionId; 4609 this.description = description; 4610 } 4611 4612 /** 4613 * @return {@link #versionId} (The identifier of a specific version of a 4614 * resource.). This is the underlying object with id, value and 4615 * extensions. The accessor "getVersionId" gives direct access to the 4616 * value 4617 */ 4618 public StringType getVersionIdElement() { 4619 if (this.versionId == null) 4620 if (Configuration.errorOnAutoCreate()) 4621 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.versionId"); 4622 else if (Configuration.doAutoCreate()) 4623 this.versionId = new StringType(); // bb 4624 return this.versionId; 4625 } 4626 4627 public boolean hasVersionIdElement() { 4628 return this.versionId != null && !this.versionId.isEmpty(); 4629 } 4630 4631 public boolean hasVersionId() { 4632 return this.versionId != null && !this.versionId.isEmpty(); 4633 } 4634 4635 /** 4636 * @param value {@link #versionId} (The identifier of a specific version of a 4637 * resource.). This is the underlying object with id, value and 4638 * extensions. The accessor "getVersionId" gives direct access to 4639 * the value 4640 */ 4641 public ExampleScenarioInstanceVersionComponent setVersionIdElement(StringType value) { 4642 this.versionId = value; 4643 return this; 4644 } 4645 4646 /** 4647 * @return The identifier of a specific version of a resource. 4648 */ 4649 public String getVersionId() { 4650 return this.versionId == null ? null : this.versionId.getValue(); 4651 } 4652 4653 /** 4654 * @param value The identifier of a specific version of a resource. 4655 */ 4656 public ExampleScenarioInstanceVersionComponent setVersionId(String value) { 4657 if (this.versionId == null) 4658 this.versionId = new StringType(); 4659 this.versionId.setValue(value); 4660 return this; 4661 } 4662 4663 /** 4664 * @return {@link #description} (The description of the resource version.). This 4665 * is the underlying object with id, value and extensions. The accessor 4666 * "getDescription" gives direct access to the value 4667 */ 4668 public MarkdownType getDescriptionElement() { 4669 if (this.description == null) 4670 if (Configuration.errorOnAutoCreate()) 4671 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.description"); 4672 else if (Configuration.doAutoCreate()) 4673 this.description = new MarkdownType(); // bb 4674 return this.description; 4675 } 4676 4677 public boolean hasDescriptionElement() { 4678 return this.description != null && !this.description.isEmpty(); 4679 } 4680 4681 public boolean hasDescription() { 4682 return this.description != null && !this.description.isEmpty(); 4683 } 4684 4685 /** 4686 * @param value {@link #description} (The description of the resource version.). 4687 * This is the underlying object with id, value and extensions. The 4688 * accessor "getDescription" gives direct access to the value 4689 */ 4690 public ExampleScenarioInstanceVersionComponent setDescriptionElement(MarkdownType value) { 4691 this.description = value; 4692 return this; 4693 } 4694 4695 /** 4696 * @return The description of the resource version. 4697 */ 4698 public String getDescription() { 4699 return this.description == null ? null : this.description.getValue(); 4700 } 4701 4702 /** 4703 * @param value The description of the resource version. 4704 */ 4705 public ExampleScenarioInstanceVersionComponent setDescription(String value) { 4706 if (this.description == null) 4707 this.description = new MarkdownType(); 4708 this.description.setValue(value); 4709 return this; 4710 } 4711 4712 protected void listChildren(List<Property> children) { 4713 super.listChildren(children); 4714 children.add( 4715 new Property("versionId", "string", "The identifier of a specific version of a resource.", 0, 1, versionId)); 4716 children 4717 .add(new Property("description", "markdown", "The description of the resource version.", 0, 1, description)); 4718 } 4719 4720 @Override 4721 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4722 switch (_hash) { 4723 case -1407102957: 4724 /* versionId */ return new Property("versionId", "string", 4725 "The identifier of a specific version of a resource.", 0, 1, versionId); 4726 case -1724546052: 4727 /* description */ return new Property("description", "markdown", "The description of the resource version.", 0, 4728 1, description); 4729 default: 4730 return super.getNamedProperty(_hash, _name, _checkValid); 4731 } 4732 4733 } 4734 4735 @Override 4736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4737 switch (hash) { 4738 case -1407102957: 4739 /* versionId */ return this.versionId == null ? new Base[0] : new Base[] { this.versionId }; // StringType 4740 case -1724546052: 4741 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 4742 default: 4743 return super.getProperty(hash, name, checkValid); 4744 } 4745 4746 } 4747 4748 @Override 4749 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4750 switch (hash) { 4751 case -1407102957: // versionId 4752 this.versionId = castToString(value); // StringType 4753 return value; 4754 case -1724546052: // description 4755 this.description = castToMarkdown(value); // MarkdownType 4756 return value; 4757 default: 4758 return super.setProperty(hash, name, value); 4759 } 4760 4761 } 4762 4763 @Override 4764 public Base setProperty(String name, Base value) throws FHIRException { 4765 if (name.equals("versionId")) { 4766 this.versionId = castToString(value); // StringType 4767 } else if (name.equals("description")) { 4768 this.description = castToMarkdown(value); // MarkdownType 4769 } else 4770 return super.setProperty(name, value); 4771 return value; 4772 } 4773 4774 @Override 4775 public void removeChild(String name, Base value) throws FHIRException { 4776 if (name.equals("versionId")) { 4777 this.versionId = null; 4778 } else if (name.equals("description")) { 4779 this.description = null; 4780 } else 4781 super.removeChild(name, value); 4782 4783 } 4784 4785 @Override 4786 public Base makeProperty(int hash, String name) throws FHIRException { 4787 switch (hash) { 4788 case -1407102957: 4789 return getVersionIdElement(); 4790 case -1724546052: 4791 return getDescriptionElement(); 4792 default: 4793 return super.makeProperty(hash, name); 4794 } 4795 4796 } 4797 4798 @Override 4799 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4800 switch (hash) { 4801 case -1407102957: 4802 /* versionId */ return new String[] { "string" }; 4803 case -1724546052: 4804 /* description */ return new String[] { "markdown" }; 4805 default: 4806 return super.getTypesForProperty(hash, name); 4807 } 4808 4809 } 4810 4811 @Override 4812 public Base addChild(String name) throws FHIRException { 4813 if (name.equals("versionId")) { 4814 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.versionId"); 4815 } else if (name.equals("description")) { 4816 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 4817 } else 4818 return super.addChild(name); 4819 } 4820 4821 public ExampleScenarioInstanceVersionComponent copy() { 4822 ExampleScenarioInstanceVersionComponent dst = new ExampleScenarioInstanceVersionComponent(); 4823 copyValues(dst); 4824 return dst; 4825 } 4826 4827 public void copyValues(ExampleScenarioInstanceVersionComponent dst) { 4828 super.copyValues(dst); 4829 dst.versionId = versionId == null ? null : versionId.copy(); 4830 dst.description = description == null ? null : description.copy(); 4831 } 4832 4833 @Override 4834 public boolean equalsDeep(Base other_) { 4835 if (!super.equalsDeep(other_)) 4836 return false; 4837 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 4838 return false; 4839 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 4840 return compareDeep(versionId, o.versionId, true) && compareDeep(description, o.description, true); 4841 } 4842 4843 @Override 4844 public boolean equalsShallow(Base other_) { 4845 if (!super.equalsShallow(other_)) 4846 return false; 4847 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 4848 return false; 4849 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 4850 return compareValues(versionId, o.versionId, true) && compareValues(description, o.description, true); 4851 } 4852 4853 public boolean isEmpty() { 4854 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(versionId, description); 4855 } 4856 4857 public String fhirType() { 4858 return "ExampleScenario.instance.version"; 4859 4860 } 4861 4862 } 4863 4864 @Block() 4865 public static class ExampleScenarioInstanceContainedInstanceComponent extends BackboneElement 4866 implements IBaseBackboneElement { 4867 /** 4868 * Each resource contained in the instance. 4869 */ 4870 @Child(name = "resourceId", type = { 4871 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 4872 @Description(shortDefinition = "Each resource contained in the instance", formalDefinition = "Each resource contained in the instance.") 4873 protected StringType resourceId; 4874 4875 /** 4876 * A specific version of a resource contained in the instance. 4877 */ 4878 @Child(name = "versionId", type = { 4879 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 4880 @Description(shortDefinition = "A specific version of a resource contained in the instance", formalDefinition = "A specific version of a resource contained in the instance.") 4881 protected StringType versionId; 4882 4883 private static final long serialVersionUID = 908084124L; 4884 4885 /** 4886 * Constructor 4887 */ 4888 public ExampleScenarioInstanceContainedInstanceComponent() { 4889 super(); 4890 } 4891 4892 /** 4893 * Constructor 4894 */ 4895 public ExampleScenarioInstanceContainedInstanceComponent(StringType resourceId) { 4896 super(); 4897 this.resourceId = resourceId; 4898 } 4899 4900 /** 4901 * @return {@link #resourceId} (Each resource contained in the instance.). This 4902 * is the underlying object with id, value and extensions. The accessor 4903 * "getResourceId" gives direct access to the value 4904 */ 4905 public StringType getResourceIdElement() { 4906 if (this.resourceId == null) 4907 if (Configuration.errorOnAutoCreate()) 4908 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.resourceId"); 4909 else if (Configuration.doAutoCreate()) 4910 this.resourceId = new StringType(); // bb 4911 return this.resourceId; 4912 } 4913 4914 public boolean hasResourceIdElement() { 4915 return this.resourceId != null && !this.resourceId.isEmpty(); 4916 } 4917 4918 public boolean hasResourceId() { 4919 return this.resourceId != null && !this.resourceId.isEmpty(); 4920 } 4921 4922 /** 4923 * @param value {@link #resourceId} (Each resource contained in the instance.). 4924 * This is the underlying object with id, value and extensions. The 4925 * accessor "getResourceId" gives direct access to the value 4926 */ 4927 public ExampleScenarioInstanceContainedInstanceComponent setResourceIdElement(StringType value) { 4928 this.resourceId = value; 4929 return this; 4930 } 4931 4932 /** 4933 * @return Each resource contained in the instance. 4934 */ 4935 public String getResourceId() { 4936 return this.resourceId == null ? null : this.resourceId.getValue(); 4937 } 4938 4939 /** 4940 * @param value Each resource contained in the instance. 4941 */ 4942 public ExampleScenarioInstanceContainedInstanceComponent setResourceId(String value) { 4943 if (this.resourceId == null) 4944 this.resourceId = new StringType(); 4945 this.resourceId.setValue(value); 4946 return this; 4947 } 4948 4949 /** 4950 * @return {@link #versionId} (A specific version of a resource contained in the 4951 * instance.). This is the underlying object with id, value and 4952 * extensions. The accessor "getVersionId" gives direct access to the 4953 * value 4954 */ 4955 public StringType getVersionIdElement() { 4956 if (this.versionId == null) 4957 if (Configuration.errorOnAutoCreate()) 4958 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.versionId"); 4959 else if (Configuration.doAutoCreate()) 4960 this.versionId = new StringType(); // bb 4961 return this.versionId; 4962 } 4963 4964 public boolean hasVersionIdElement() { 4965 return this.versionId != null && !this.versionId.isEmpty(); 4966 } 4967 4968 public boolean hasVersionId() { 4969 return this.versionId != null && !this.versionId.isEmpty(); 4970 } 4971 4972 /** 4973 * @param value {@link #versionId} (A specific version of a resource contained 4974 * in the instance.). This is the underlying object with id, value 4975 * and extensions. The accessor "getVersionId" gives direct access 4976 * to the value 4977 */ 4978 public ExampleScenarioInstanceContainedInstanceComponent setVersionIdElement(StringType value) { 4979 this.versionId = value; 4980 return this; 4981 } 4982 4983 /** 4984 * @return A specific version of a resource contained in the instance. 4985 */ 4986 public String getVersionId() { 4987 return this.versionId == null ? null : this.versionId.getValue(); 4988 } 4989 4990 /** 4991 * @param value A specific version of a resource contained in the instance. 4992 */ 4993 public ExampleScenarioInstanceContainedInstanceComponent setVersionId(String value) { 4994 if (Utilities.noString(value)) 4995 this.versionId = null; 4996 else { 4997 if (this.versionId == null) 4998 this.versionId = new StringType(); 4999 this.versionId.setValue(value); 5000 } 5001 return this; 5002 } 5003 5004 protected void listChildren(List<Property> children) { 5005 super.listChildren(children); 5006 children.add(new Property("resourceId", "string", "Each resource contained in the instance.", 0, 1, resourceId)); 5007 children.add(new Property("versionId", "string", "A specific version of a resource contained in the instance.", 0, 5008 1, versionId)); 5009 } 5010 5011 @Override 5012 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5013 switch (_hash) { 5014 case -1345650231: 5015 /* resourceId */ return new Property("resourceId", "string", "Each resource contained in the instance.", 0, 1, 5016 resourceId); 5017 case -1407102957: 5018 /* versionId */ return new Property("versionId", "string", 5019 "A specific version of a resource contained in the instance.", 0, 1, versionId); 5020 default: 5021 return super.getNamedProperty(_hash, _name, _checkValid); 5022 } 5023 5024 } 5025 5026 @Override 5027 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5028 switch (hash) { 5029 case -1345650231: 5030 /* resourceId */ return this.resourceId == null ? new Base[0] : new Base[] { this.resourceId }; // StringType 5031 case -1407102957: 5032 /* versionId */ return this.versionId == null ? new Base[0] : new Base[] { this.versionId }; // StringType 5033 default: 5034 return super.getProperty(hash, name, checkValid); 5035 } 5036 5037 } 5038 5039 @Override 5040 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5041 switch (hash) { 5042 case -1345650231: // resourceId 5043 this.resourceId = castToString(value); // StringType 5044 return value; 5045 case -1407102957: // versionId 5046 this.versionId = castToString(value); // StringType 5047 return value; 5048 default: 5049 return super.setProperty(hash, name, value); 5050 } 5051 5052 } 5053 5054 @Override 5055 public Base setProperty(String name, Base value) throws FHIRException { 5056 if (name.equals("resourceId")) { 5057 this.resourceId = castToString(value); // StringType 5058 } else if (name.equals("versionId")) { 5059 this.versionId = castToString(value); // StringType 5060 } else 5061 return super.setProperty(name, value); 5062 return value; 5063 } 5064 5065 @Override 5066 public void removeChild(String name, Base value) throws FHIRException { 5067 if (name.equals("resourceId")) { 5068 this.resourceId = null; 5069 } else if (name.equals("versionId")) { 5070 this.versionId = null; 5071 } else 5072 super.removeChild(name, value); 5073 5074 } 5075 5076 @Override 5077 public Base makeProperty(int hash, String name) throws FHIRException { 5078 switch (hash) { 5079 case -1345650231: 5080 return getResourceIdElement(); 5081 case -1407102957: 5082 return getVersionIdElement(); 5083 default: 5084 return super.makeProperty(hash, name); 5085 } 5086 5087 } 5088 5089 @Override 5090 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5091 switch (hash) { 5092 case -1345650231: 5093 /* resourceId */ return new String[] { "string" }; 5094 case -1407102957: 5095 /* versionId */ return new String[] { "string" }; 5096 default: 5097 return super.getTypesForProperty(hash, name); 5098 } 5099 5100 } 5101 5102 @Override 5103 public Base addChild(String name) throws FHIRException { 5104 if (name.equals("resourceId")) { 5105 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.resourceId"); 5106 } else if (name.equals("versionId")) { 5107 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.versionId"); 5108 } else 5109 return super.addChild(name); 5110 } 5111 5112 public ExampleScenarioInstanceContainedInstanceComponent copy() { 5113 ExampleScenarioInstanceContainedInstanceComponent dst = new ExampleScenarioInstanceContainedInstanceComponent(); 5114 copyValues(dst); 5115 return dst; 5116 } 5117 5118 public void copyValues(ExampleScenarioInstanceContainedInstanceComponent dst) { 5119 super.copyValues(dst); 5120 dst.resourceId = resourceId == null ? null : resourceId.copy(); 5121 dst.versionId = versionId == null ? null : versionId.copy(); 5122 } 5123 5124 @Override 5125 public boolean equalsDeep(Base other_) { 5126 if (!super.equalsDeep(other_)) 5127 return false; 5128 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 5129 return false; 5130 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 5131 return compareDeep(resourceId, o.resourceId, true) && compareDeep(versionId, o.versionId, true); 5132 } 5133 5134 @Override 5135 public boolean equalsShallow(Base other_) { 5136 if (!super.equalsShallow(other_)) 5137 return false; 5138 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 5139 return false; 5140 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 5141 return compareValues(resourceId, o.resourceId, true) && compareValues(versionId, o.versionId, true); 5142 } 5143 5144 public boolean isEmpty() { 5145 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(resourceId, versionId); 5146 } 5147 5148 public String fhirType() { 5149 return "ExampleScenario.instance.containedInstance"; 5150 5151 } 5152 5153 } 5154 5155 @Block() 5156 public static class ExampleScenarioProcessComponent extends BackboneElement implements IBaseBackboneElement { 5157 /** 5158 * The diagram title of the group of operations. 5159 */ 5160 @Child(name = "title", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 5161 @Description(shortDefinition = "The diagram title of the group of operations", formalDefinition = "The diagram title of the group of operations.") 5162 protected StringType title; 5163 5164 /** 5165 * A longer description of the group of operations. 5166 */ 5167 @Child(name = "description", type = { 5168 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5169 @Description(shortDefinition = "A longer description of the group of operations", formalDefinition = "A longer description of the group of operations.") 5170 protected MarkdownType description; 5171 5172 /** 5173 * Description of initial status before the process starts. 5174 */ 5175 @Child(name = "preConditions", type = { 5176 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 5177 @Description(shortDefinition = "Description of initial status before the process starts", formalDefinition = "Description of initial status before the process starts.") 5178 protected MarkdownType preConditions; 5179 5180 /** 5181 * Description of final status after the process ends. 5182 */ 5183 @Child(name = "postConditions", type = { 5184 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 5185 @Description(shortDefinition = "Description of final status after the process ends", formalDefinition = "Description of final status after the process ends.") 5186 protected MarkdownType postConditions; 5187 5188 /** 5189 * Each step of the process. 5190 */ 5191 @Child(name = "step", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5192 @Description(shortDefinition = "Each step of the process", formalDefinition = "Each step of the process.") 5193 protected List<ExampleScenarioProcessStepComponent> step; 5194 5195 private static final long serialVersionUID = 325578043L; 5196 5197 /** 5198 * Constructor 5199 */ 5200 public ExampleScenarioProcessComponent() { 5201 super(); 5202 } 5203 5204 /** 5205 * Constructor 5206 */ 5207 public ExampleScenarioProcessComponent(StringType title) { 5208 super(); 5209 this.title = title; 5210 } 5211 5212 /** 5213 * @return {@link #title} (The diagram title of the group of operations.). This 5214 * is the underlying object with id, value and extensions. The accessor 5215 * "getTitle" gives direct access to the value 5216 */ 5217 public StringType getTitleElement() { 5218 if (this.title == null) 5219 if (Configuration.errorOnAutoCreate()) 5220 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.title"); 5221 else if (Configuration.doAutoCreate()) 5222 this.title = new StringType(); // bb 5223 return this.title; 5224 } 5225 5226 public boolean hasTitleElement() { 5227 return this.title != null && !this.title.isEmpty(); 5228 } 5229 5230 public boolean hasTitle() { 5231 return this.title != null && !this.title.isEmpty(); 5232 } 5233 5234 /** 5235 * @param value {@link #title} (The diagram title of the group of operations.). 5236 * This is the underlying object with id, value and extensions. The 5237 * accessor "getTitle" gives direct access to the value 5238 */ 5239 public ExampleScenarioProcessComponent setTitleElement(StringType value) { 5240 this.title = value; 5241 return this; 5242 } 5243 5244 /** 5245 * @return The diagram title of the group of operations. 5246 */ 5247 public String getTitle() { 5248 return this.title == null ? null : this.title.getValue(); 5249 } 5250 5251 /** 5252 * @param value The diagram title of the group of operations. 5253 */ 5254 public ExampleScenarioProcessComponent setTitle(String value) { 5255 if (this.title == null) 5256 this.title = new StringType(); 5257 this.title.setValue(value); 5258 return this; 5259 } 5260 5261 /** 5262 * @return {@link #description} (A longer description of the group of 5263 * operations.). This is the underlying object with id, value and 5264 * extensions. The accessor "getDescription" gives direct access to the 5265 * value 5266 */ 5267 public MarkdownType getDescriptionElement() { 5268 if (this.description == null) 5269 if (Configuration.errorOnAutoCreate()) 5270 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.description"); 5271 else if (Configuration.doAutoCreate()) 5272 this.description = new MarkdownType(); // bb 5273 return this.description; 5274 } 5275 5276 public boolean hasDescriptionElement() { 5277 return this.description != null && !this.description.isEmpty(); 5278 } 5279 5280 public boolean hasDescription() { 5281 return this.description != null && !this.description.isEmpty(); 5282 } 5283 5284 /** 5285 * @param value {@link #description} (A longer description of the group of 5286 * operations.). This is the underlying object with id, value and 5287 * extensions. The accessor "getDescription" gives direct access to 5288 * the value 5289 */ 5290 public ExampleScenarioProcessComponent setDescriptionElement(MarkdownType value) { 5291 this.description = value; 5292 return this; 5293 } 5294 5295 /** 5296 * @return A longer description of the group of operations. 5297 */ 5298 public String getDescription() { 5299 return this.description == null ? null : this.description.getValue(); 5300 } 5301 5302 /** 5303 * @param value A longer description of the group of operations. 5304 */ 5305 public ExampleScenarioProcessComponent setDescription(String value) { 5306 if (value == null) 5307 this.description = null; 5308 else { 5309 if (this.description == null) 5310 this.description = new MarkdownType(); 5311 this.description.setValue(value); 5312 } 5313 return this; 5314 } 5315 5316 /** 5317 * @return {@link #preConditions} (Description of initial status before the 5318 * process starts.). This is the underlying object with id, value and 5319 * extensions. The accessor "getPreConditions" gives direct access to 5320 * the value 5321 */ 5322 public MarkdownType getPreConditionsElement() { 5323 if (this.preConditions == null) 5324 if (Configuration.errorOnAutoCreate()) 5325 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.preConditions"); 5326 else if (Configuration.doAutoCreate()) 5327 this.preConditions = new MarkdownType(); // bb 5328 return this.preConditions; 5329 } 5330 5331 public boolean hasPreConditionsElement() { 5332 return this.preConditions != null && !this.preConditions.isEmpty(); 5333 } 5334 5335 public boolean hasPreConditions() { 5336 return this.preConditions != null && !this.preConditions.isEmpty(); 5337 } 5338 5339 /** 5340 * @param value {@link #preConditions} (Description of initial status before the 5341 * process starts.). This is the underlying object with id, value 5342 * and extensions. The accessor "getPreConditions" gives direct 5343 * access to the value 5344 */ 5345 public ExampleScenarioProcessComponent setPreConditionsElement(MarkdownType value) { 5346 this.preConditions = value; 5347 return this; 5348 } 5349 5350 /** 5351 * @return Description of initial status before the process starts. 5352 */ 5353 public String getPreConditions() { 5354 return this.preConditions == null ? null : this.preConditions.getValue(); 5355 } 5356 5357 /** 5358 * @param value Description of initial status before the process starts. 5359 */ 5360 public ExampleScenarioProcessComponent setPreConditions(String value) { 5361 if (value == null) 5362 this.preConditions = null; 5363 else { 5364 if (this.preConditions == null) 5365 this.preConditions = new MarkdownType(); 5366 this.preConditions.setValue(value); 5367 } 5368 return this; 5369 } 5370 5371 /** 5372 * @return {@link #postConditions} (Description of final status after the 5373 * process ends.). This is the underlying object with id, value and 5374 * extensions. The accessor "getPostConditions" gives direct access to 5375 * the value 5376 */ 5377 public MarkdownType getPostConditionsElement() { 5378 if (this.postConditions == null) 5379 if (Configuration.errorOnAutoCreate()) 5380 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.postConditions"); 5381 else if (Configuration.doAutoCreate()) 5382 this.postConditions = new MarkdownType(); // bb 5383 return this.postConditions; 5384 } 5385 5386 public boolean hasPostConditionsElement() { 5387 return this.postConditions != null && !this.postConditions.isEmpty(); 5388 } 5389 5390 public boolean hasPostConditions() { 5391 return this.postConditions != null && !this.postConditions.isEmpty(); 5392 } 5393 5394 /** 5395 * @param value {@link #postConditions} (Description of final status after the 5396 * process ends.). This is the underlying object with id, value and 5397 * extensions. The accessor "getPostConditions" gives direct access 5398 * to the value 5399 */ 5400 public ExampleScenarioProcessComponent setPostConditionsElement(MarkdownType value) { 5401 this.postConditions = value; 5402 return this; 5403 } 5404 5405 /** 5406 * @return Description of final status after the process ends. 5407 */ 5408 public String getPostConditions() { 5409 return this.postConditions == null ? null : this.postConditions.getValue(); 5410 } 5411 5412 /** 5413 * @param value Description of final status after the process ends. 5414 */ 5415 public ExampleScenarioProcessComponent setPostConditions(String value) { 5416 if (value == null) 5417 this.postConditions = null; 5418 else { 5419 if (this.postConditions == null) 5420 this.postConditions = new MarkdownType(); 5421 this.postConditions.setValue(value); 5422 } 5423 return this; 5424 } 5425 5426 /** 5427 * @return {@link #step} (Each step of the process.) 5428 */ 5429 public List<ExampleScenarioProcessStepComponent> getStep() { 5430 if (this.step == null) 5431 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5432 return this.step; 5433 } 5434 5435 /** 5436 * @return Returns a reference to <code>this</code> for easy method chaining 5437 */ 5438 public ExampleScenarioProcessComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 5439 this.step = theStep; 5440 return this; 5441 } 5442 5443 public boolean hasStep() { 5444 if (this.step == null) 5445 return false; 5446 for (ExampleScenarioProcessStepComponent item : this.step) 5447 if (!item.isEmpty()) 5448 return true; 5449 return false; 5450 } 5451 5452 public ExampleScenarioProcessStepComponent addStep() { // 3 5453 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 5454 if (this.step == null) 5455 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5456 this.step.add(t); 5457 return t; 5458 } 5459 5460 public ExampleScenarioProcessComponent addStep(ExampleScenarioProcessStepComponent t) { // 3 5461 if (t == null) 5462 return this; 5463 if (this.step == null) 5464 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5465 this.step.add(t); 5466 return this; 5467 } 5468 5469 /** 5470 * @return The first repetition of repeating field {@link #step}, creating it if 5471 * it does not already exist 5472 */ 5473 public ExampleScenarioProcessStepComponent getStepFirstRep() { 5474 if (getStep().isEmpty()) { 5475 addStep(); 5476 } 5477 return getStep().get(0); 5478 } 5479 5480 protected void listChildren(List<Property> children) { 5481 super.listChildren(children); 5482 children.add(new Property("title", "string", "The diagram title of the group of operations.", 0, 1, title)); 5483 children.add(new Property("description", "markdown", "A longer description of the group of operations.", 0, 1, 5484 description)); 5485 children.add(new Property("preConditions", "markdown", "Description of initial status before the process starts.", 5486 0, 1, preConditions)); 5487 children.add(new Property("postConditions", "markdown", "Description of final status after the process ends.", 0, 5488 1, postConditions)); 5489 children.add(new Property("step", "", "Each step of the process.", 0, java.lang.Integer.MAX_VALUE, step)); 5490 } 5491 5492 @Override 5493 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5494 switch (_hash) { 5495 case 110371416: 5496 /* title */ return new Property("title", "string", "The diagram title of the group of operations.", 0, 1, 5497 title); 5498 case -1724546052: 5499 /* description */ return new Property("description", "markdown", 5500 "A longer description of the group of operations.", 0, 1, description); 5501 case -1006692933: 5502 /* preConditions */ return new Property("preConditions", "markdown", 5503 "Description of initial status before the process starts.", 0, 1, preConditions); 5504 case 1738302328: 5505 /* postConditions */ return new Property("postConditions", "markdown", 5506 "Description of final status after the process ends.", 0, 1, postConditions); 5507 case 3540684: 5508 /* step */ return new Property("step", "", "Each step of the process.", 0, java.lang.Integer.MAX_VALUE, step); 5509 default: 5510 return super.getNamedProperty(_hash, _name, _checkValid); 5511 } 5512 5513 } 5514 5515 @Override 5516 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5517 switch (hash) { 5518 case 110371416: 5519 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 5520 case -1724546052: 5521 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 5522 case -1006692933: 5523 /* preConditions */ return this.preConditions == null ? new Base[0] : new Base[] { this.preConditions }; // MarkdownType 5524 case 1738302328: 5525 /* postConditions */ return this.postConditions == null ? new Base[0] : new Base[] { this.postConditions }; // MarkdownType 5526 case 3540684: 5527 /* step */ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 5528 default: 5529 return super.getProperty(hash, name, checkValid); 5530 } 5531 5532 } 5533 5534 @Override 5535 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5536 switch (hash) { 5537 case 110371416: // title 5538 this.title = castToString(value); // StringType 5539 return value; 5540 case -1724546052: // description 5541 this.description = castToMarkdown(value); // MarkdownType 5542 return value; 5543 case -1006692933: // preConditions 5544 this.preConditions = castToMarkdown(value); // MarkdownType 5545 return value; 5546 case 1738302328: // postConditions 5547 this.postConditions = castToMarkdown(value); // MarkdownType 5548 return value; 5549 case 3540684: // step 5550 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 5551 return value; 5552 default: 5553 return super.setProperty(hash, name, value); 5554 } 5555 5556 } 5557 5558 @Override 5559 public Base setProperty(String name, Base value) throws FHIRException { 5560 if (name.equals("title")) { 5561 this.title = castToString(value); // StringType 5562 } else if (name.equals("description")) { 5563 this.description = castToMarkdown(value); // MarkdownType 5564 } else if (name.equals("preConditions")) { 5565 this.preConditions = castToMarkdown(value); // MarkdownType 5566 } else if (name.equals("postConditions")) { 5567 this.postConditions = castToMarkdown(value); // MarkdownType 5568 } else if (name.equals("step")) { 5569 this.getStep().add((ExampleScenarioProcessStepComponent) value); 5570 } else 5571 return super.setProperty(name, value); 5572 return value; 5573 } 5574 5575 @Override 5576 public void removeChild(String name, Base value) throws FHIRException { 5577 if (name.equals("title")) { 5578 this.title = null; 5579 } else if (name.equals("description")) { 5580 this.description = null; 5581 } else if (name.equals("preConditions")) { 5582 this.preConditions = null; 5583 } else if (name.equals("postConditions")) { 5584 this.postConditions = null; 5585 } else if (name.equals("step")) { 5586 this.getStep().remove((ExampleScenarioProcessStepComponent) value); 5587 } else 5588 super.removeChild(name, value); 5589 5590 } 5591 5592 @Override 5593 public Base makeProperty(int hash, String name) throws FHIRException { 5594 switch (hash) { 5595 case 110371416: 5596 return getTitleElement(); 5597 case -1724546052: 5598 return getDescriptionElement(); 5599 case -1006692933: 5600 return getPreConditionsElement(); 5601 case 1738302328: 5602 return getPostConditionsElement(); 5603 case 3540684: 5604 return addStep(); 5605 default: 5606 return super.makeProperty(hash, name); 5607 } 5608 5609 } 5610 5611 @Override 5612 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5613 switch (hash) { 5614 case 110371416: 5615 /* title */ return new String[] { "string" }; 5616 case -1724546052: 5617 /* description */ return new String[] { "markdown" }; 5618 case -1006692933: 5619 /* preConditions */ return new String[] { "markdown" }; 5620 case 1738302328: 5621 /* postConditions */ return new String[] { "markdown" }; 5622 case 3540684: 5623 /* step */ return new String[] {}; 5624 default: 5625 return super.getTypesForProperty(hash, name); 5626 } 5627 5628 } 5629 5630 @Override 5631 public Base addChild(String name) throws FHIRException { 5632 if (name.equals("title")) { 5633 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.title"); 5634 } else if (name.equals("description")) { 5635 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 5636 } else if (name.equals("preConditions")) { 5637 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.preConditions"); 5638 } else if (name.equals("postConditions")) { 5639 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.postConditions"); 5640 } else if (name.equals("step")) { 5641 return addStep(); 5642 } else 5643 return super.addChild(name); 5644 } 5645 5646 public ExampleScenarioProcessComponent copy() { 5647 ExampleScenarioProcessComponent dst = new ExampleScenarioProcessComponent(); 5648 copyValues(dst); 5649 return dst; 5650 } 5651 5652 public void copyValues(ExampleScenarioProcessComponent dst) { 5653 super.copyValues(dst); 5654 dst.title = title == null ? null : title.copy(); 5655 dst.description = description == null ? null : description.copy(); 5656 dst.preConditions = preConditions == null ? null : preConditions.copy(); 5657 dst.postConditions = postConditions == null ? null : postConditions.copy(); 5658 if (step != null) { 5659 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 5660 for (ExampleScenarioProcessStepComponent i : step) 5661 dst.step.add(i.copy()); 5662 } 5663 ; 5664 } 5665 5666 @Override 5667 public boolean equalsDeep(Base other_) { 5668 if (!super.equalsDeep(other_)) 5669 return false; 5670 if (!(other_ instanceof ExampleScenarioProcessComponent)) 5671 return false; 5672 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 5673 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 5674 && compareDeep(preConditions, o.preConditions, true) && compareDeep(postConditions, o.postConditions, true) 5675 && compareDeep(step, o.step, true); 5676 } 5677 5678 @Override 5679 public boolean equalsShallow(Base other_) { 5680 if (!super.equalsShallow(other_)) 5681 return false; 5682 if (!(other_ instanceof ExampleScenarioProcessComponent)) 5683 return false; 5684 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 5685 return compareValues(title, o.title, true) && compareValues(description, o.description, true) 5686 && compareValues(preConditions, o.preConditions, true) 5687 && compareValues(postConditions, o.postConditions, true); 5688 } 5689 5690 public boolean isEmpty() { 5691 return super.isEmpty() 5692 && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, preConditions, postConditions, step); 5693 } 5694 5695 public String fhirType() { 5696 return "ExampleScenario.process"; 5697 5698 } 5699 5700 } 5701 5702 @Block() 5703 public static class ExampleScenarioProcessStepComponent extends BackboneElement implements IBaseBackboneElement { 5704 /** 5705 * Nested process. 5706 */ 5707 @Child(name = "process", type = { 5708 ExampleScenarioProcessComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5709 @Description(shortDefinition = "Nested process", formalDefinition = "Nested process.") 5710 protected List<ExampleScenarioProcessComponent> process; 5711 5712 /** 5713 * If there is a pause in the flow. 5714 */ 5715 @Child(name = "pause", type = { BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 5716 @Description(shortDefinition = "If there is a pause in the flow", formalDefinition = "If there is a pause in the flow.") 5717 protected BooleanType pause; 5718 5719 /** 5720 * Each interaction or action. 5721 */ 5722 @Child(name = "operation", type = {}, order = 3, min = 0, max = 1, modifier = false, summary = false) 5723 @Description(shortDefinition = "Each interaction or action", formalDefinition = "Each interaction or action.") 5724 protected ExampleScenarioProcessStepOperationComponent operation; 5725 5726 /** 5727 * Indicates an alternative step that can be taken instead of the operations on 5728 * the base step in exceptional/atypical circumstances. 5729 */ 5730 @Child(name = "alternative", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 5731 @Description(shortDefinition = "Alternate non-typical step action", formalDefinition = "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.") 5732 protected List<ExampleScenarioProcessStepAlternativeComponent> alternative; 5733 5734 private static final long serialVersionUID = -894029605L; 5735 5736 /** 5737 * Constructor 5738 */ 5739 public ExampleScenarioProcessStepComponent() { 5740 super(); 5741 } 5742 5743 /** 5744 * @return {@link #process} (Nested process.) 5745 */ 5746 public List<ExampleScenarioProcessComponent> getProcess() { 5747 if (this.process == null) 5748 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5749 return this.process; 5750 } 5751 5752 /** 5753 * @return Returns a reference to <code>this</code> for easy method chaining 5754 */ 5755 public ExampleScenarioProcessStepComponent setProcess(List<ExampleScenarioProcessComponent> theProcess) { 5756 this.process = theProcess; 5757 return this; 5758 } 5759 5760 public boolean hasProcess() { 5761 if (this.process == null) 5762 return false; 5763 for (ExampleScenarioProcessComponent item : this.process) 5764 if (!item.isEmpty()) 5765 return true; 5766 return false; 5767 } 5768 5769 public ExampleScenarioProcessComponent addProcess() { // 3 5770 ExampleScenarioProcessComponent t = new ExampleScenarioProcessComponent(); 5771 if (this.process == null) 5772 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5773 this.process.add(t); 5774 return t; 5775 } 5776 5777 public ExampleScenarioProcessStepComponent addProcess(ExampleScenarioProcessComponent t) { // 3 5778 if (t == null) 5779 return this; 5780 if (this.process == null) 5781 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5782 this.process.add(t); 5783 return this; 5784 } 5785 5786 /** 5787 * @return The first repetition of repeating field {@link #process}, creating it 5788 * if it does not already exist 5789 */ 5790 public ExampleScenarioProcessComponent getProcessFirstRep() { 5791 if (getProcess().isEmpty()) { 5792 addProcess(); 5793 } 5794 return getProcess().get(0); 5795 } 5796 5797 /** 5798 * @return {@link #pause} (If there is a pause in the flow.). This is the 5799 * underlying object with id, value and extensions. The accessor 5800 * "getPause" gives direct access to the value 5801 */ 5802 public BooleanType getPauseElement() { 5803 if (this.pause == null) 5804 if (Configuration.errorOnAutoCreate()) 5805 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.pause"); 5806 else if (Configuration.doAutoCreate()) 5807 this.pause = new BooleanType(); // bb 5808 return this.pause; 5809 } 5810 5811 public boolean hasPauseElement() { 5812 return this.pause != null && !this.pause.isEmpty(); 5813 } 5814 5815 public boolean hasPause() { 5816 return this.pause != null && !this.pause.isEmpty(); 5817 } 5818 5819 /** 5820 * @param value {@link #pause} (If there is a pause in the flow.). This is the 5821 * underlying object with id, value and extensions. The accessor 5822 * "getPause" gives direct access to the value 5823 */ 5824 public ExampleScenarioProcessStepComponent setPauseElement(BooleanType value) { 5825 this.pause = value; 5826 return this; 5827 } 5828 5829 /** 5830 * @return If there is a pause in the flow. 5831 */ 5832 public boolean getPause() { 5833 return this.pause == null || this.pause.isEmpty() ? false : this.pause.getValue(); 5834 } 5835 5836 /** 5837 * @param value If there is a pause in the flow. 5838 */ 5839 public ExampleScenarioProcessStepComponent setPause(boolean value) { 5840 if (this.pause == null) 5841 this.pause = new BooleanType(); 5842 this.pause.setValue(value); 5843 return this; 5844 } 5845 5846 /** 5847 * @return {@link #operation} (Each interaction or action.) 5848 */ 5849 public ExampleScenarioProcessStepOperationComponent getOperation() { 5850 if (this.operation == null) 5851 if (Configuration.errorOnAutoCreate()) 5852 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.operation"); 5853 else if (Configuration.doAutoCreate()) 5854 this.operation = new ExampleScenarioProcessStepOperationComponent(); // cc 5855 return this.operation; 5856 } 5857 5858 public boolean hasOperation() { 5859 return this.operation != null && !this.operation.isEmpty(); 5860 } 5861 5862 /** 5863 * @param value {@link #operation} (Each interaction or action.) 5864 */ 5865 public ExampleScenarioProcessStepComponent setOperation(ExampleScenarioProcessStepOperationComponent value) { 5866 this.operation = value; 5867 return this; 5868 } 5869 5870 /** 5871 * @return {@link #alternative} (Indicates an alternative step that can be taken 5872 * instead of the operations on the base step in exceptional/atypical 5873 * circumstances.) 5874 */ 5875 public List<ExampleScenarioProcessStepAlternativeComponent> getAlternative() { 5876 if (this.alternative == null) 5877 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5878 return this.alternative; 5879 } 5880 5881 /** 5882 * @return Returns a reference to <code>this</code> for easy method chaining 5883 */ 5884 public ExampleScenarioProcessStepComponent setAlternative( 5885 List<ExampleScenarioProcessStepAlternativeComponent> theAlternative) { 5886 this.alternative = theAlternative; 5887 return this; 5888 } 5889 5890 public boolean hasAlternative() { 5891 if (this.alternative == null) 5892 return false; 5893 for (ExampleScenarioProcessStepAlternativeComponent item : this.alternative) 5894 if (!item.isEmpty()) 5895 return true; 5896 return false; 5897 } 5898 5899 public ExampleScenarioProcessStepAlternativeComponent addAlternative() { // 3 5900 ExampleScenarioProcessStepAlternativeComponent t = new ExampleScenarioProcessStepAlternativeComponent(); 5901 if (this.alternative == null) 5902 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5903 this.alternative.add(t); 5904 return t; 5905 } 5906 5907 public ExampleScenarioProcessStepComponent addAlternative(ExampleScenarioProcessStepAlternativeComponent t) { // 3 5908 if (t == null) 5909 return this; 5910 if (this.alternative == null) 5911 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 5912 this.alternative.add(t); 5913 return this; 5914 } 5915 5916 /** 5917 * @return The first repetition of repeating field {@link #alternative}, 5918 * creating it if it does not already exist 5919 */ 5920 public ExampleScenarioProcessStepAlternativeComponent getAlternativeFirstRep() { 5921 if (getAlternative().isEmpty()) { 5922 addAlternative(); 5923 } 5924 return getAlternative().get(0); 5925 } 5926 5927 protected void listChildren(List<Property> children) { 5928 super.listChildren(children); 5929 children.add(new Property("process", "@ExampleScenario.process", "Nested process.", 0, 5930 java.lang.Integer.MAX_VALUE, process)); 5931 children.add(new Property("pause", "boolean", "If there is a pause in the flow.", 0, 1, pause)); 5932 children.add(new Property("operation", "", "Each interaction or action.", 0, 1, operation)); 5933 children.add(new Property("alternative", "", 5934 "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.", 5935 0, java.lang.Integer.MAX_VALUE, alternative)); 5936 } 5937 5938 @Override 5939 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5940 switch (_hash) { 5941 case -309518737: 5942 /* process */ return new Property("process", "@ExampleScenario.process", "Nested process.", 0, 5943 java.lang.Integer.MAX_VALUE, process); 5944 case 106440182: 5945 /* pause */ return new Property("pause", "boolean", "If there is a pause in the flow.", 0, 1, pause); 5946 case 1662702951: 5947 /* operation */ return new Property("operation", "", "Each interaction or action.", 0, 1, operation); 5948 case -196794451: 5949 /* alternative */ return new Property("alternative", "", 5950 "Indicates an alternative step that can be taken instead of the operations on the base step in exceptional/atypical circumstances.", 5951 0, java.lang.Integer.MAX_VALUE, alternative); 5952 default: 5953 return super.getNamedProperty(_hash, _name, _checkValid); 5954 } 5955 5956 } 5957 5958 @Override 5959 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5960 switch (hash) { 5961 case -309518737: 5962 /* process */ return this.process == null ? new Base[0] : this.process.toArray(new Base[this.process.size()]); // ExampleScenarioProcessComponent 5963 case 106440182: 5964 /* pause */ return this.pause == null ? new Base[0] : new Base[] { this.pause }; // BooleanType 5965 case 1662702951: 5966 /* operation */ return this.operation == null ? new Base[0] : new Base[] { this.operation }; // ExampleScenarioProcessStepOperationComponent 5967 case -196794451: 5968 /* alternative */ return this.alternative == null ? new Base[0] 5969 : this.alternative.toArray(new Base[this.alternative.size()]); // ExampleScenarioProcessStepAlternativeComponent 5970 default: 5971 return super.getProperty(hash, name, checkValid); 5972 } 5973 5974 } 5975 5976 @Override 5977 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5978 switch (hash) { 5979 case -309518737: // process 5980 this.getProcess().add((ExampleScenarioProcessComponent) value); // ExampleScenarioProcessComponent 5981 return value; 5982 case 106440182: // pause 5983 this.pause = castToBoolean(value); // BooleanType 5984 return value; 5985 case 1662702951: // operation 5986 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 5987 return value; 5988 case -196794451: // alternative 5989 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); // ExampleScenarioProcessStepAlternativeComponent 5990 return value; 5991 default: 5992 return super.setProperty(hash, name, value); 5993 } 5994 5995 } 5996 5997 @Override 5998 public Base setProperty(String name, Base value) throws FHIRException { 5999 if (name.equals("process")) { 6000 this.getProcess().add((ExampleScenarioProcessComponent) value); 6001 } else if (name.equals("pause")) { 6002 this.pause = castToBoolean(value); // BooleanType 6003 } else if (name.equals("operation")) { 6004 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 6005 } else if (name.equals("alternative")) { 6006 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); 6007 } else 6008 return super.setProperty(name, value); 6009 return value; 6010 } 6011 6012 @Override 6013 public void removeChild(String name, Base value) throws FHIRException { 6014 if (name.equals("process")) { 6015 this.getProcess().remove((ExampleScenarioProcessComponent) value); 6016 } else if (name.equals("pause")) { 6017 this.pause = null; 6018 } else if (name.equals("operation")) { 6019 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 6020 } else if (name.equals("alternative")) { 6021 this.getAlternative().remove((ExampleScenarioProcessStepAlternativeComponent) value); 6022 } else 6023 super.removeChild(name, value); 6024 6025 } 6026 6027 @Override 6028 public Base makeProperty(int hash, String name) throws FHIRException { 6029 switch (hash) { 6030 case -309518737: 6031 return addProcess(); 6032 case 106440182: 6033 return getPauseElement(); 6034 case 1662702951: 6035 return getOperation(); 6036 case -196794451: 6037 return addAlternative(); 6038 default: 6039 return super.makeProperty(hash, name); 6040 } 6041 6042 } 6043 6044 @Override 6045 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6046 switch (hash) { 6047 case -309518737: 6048 /* process */ return new String[] { "@ExampleScenario.process" }; 6049 case 106440182: 6050 /* pause */ return new String[] { "boolean" }; 6051 case 1662702951: 6052 /* operation */ return new String[] {}; 6053 case -196794451: 6054 /* alternative */ return new String[] {}; 6055 default: 6056 return super.getTypesForProperty(hash, name); 6057 } 6058 6059 } 6060 6061 @Override 6062 public Base addChild(String name) throws FHIRException { 6063 if (name.equals("process")) { 6064 return addProcess(); 6065 } else if (name.equals("pause")) { 6066 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.pause"); 6067 } else if (name.equals("operation")) { 6068 this.operation = new ExampleScenarioProcessStepOperationComponent(); 6069 return this.operation; 6070 } else if (name.equals("alternative")) { 6071 return addAlternative(); 6072 } else 6073 return super.addChild(name); 6074 } 6075 6076 public ExampleScenarioProcessStepComponent copy() { 6077 ExampleScenarioProcessStepComponent dst = new ExampleScenarioProcessStepComponent(); 6078 copyValues(dst); 6079 return dst; 6080 } 6081 6082 public void copyValues(ExampleScenarioProcessStepComponent dst) { 6083 super.copyValues(dst); 6084 if (process != null) { 6085 dst.process = new ArrayList<ExampleScenarioProcessComponent>(); 6086 for (ExampleScenarioProcessComponent i : process) 6087 dst.process.add(i.copy()); 6088 } 6089 ; 6090 dst.pause = pause == null ? null : pause.copy(); 6091 dst.operation = operation == null ? null : operation.copy(); 6092 if (alternative != null) { 6093 dst.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 6094 for (ExampleScenarioProcessStepAlternativeComponent i : alternative) 6095 dst.alternative.add(i.copy()); 6096 } 6097 ; 6098 } 6099 6100 @Override 6101 public boolean equalsDeep(Base other_) { 6102 if (!super.equalsDeep(other_)) 6103 return false; 6104 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 6105 return false; 6106 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 6107 return compareDeep(process, o.process, true) && compareDeep(pause, o.pause, true) 6108 && compareDeep(operation, o.operation, true) && compareDeep(alternative, o.alternative, true); 6109 } 6110 6111 @Override 6112 public boolean equalsShallow(Base other_) { 6113 if (!super.equalsShallow(other_)) 6114 return false; 6115 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 6116 return false; 6117 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 6118 return compareValues(pause, o.pause, true); 6119 } 6120 6121 public boolean isEmpty() { 6122 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(process, pause, operation, alternative); 6123 } 6124 6125 public String fhirType() { 6126 return "ExampleScenario.process.step"; 6127 6128 } 6129 6130 } 6131 6132 @Block() 6133 public static class ExampleScenarioProcessStepOperationComponent extends BackboneElement 6134 implements IBaseBackboneElement { 6135 /** 6136 * The sequential number of the interaction, e.g. 1.2.5. 6137 */ 6138 @Child(name = "number", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 6139 @Description(shortDefinition = "The sequential number of the interaction", formalDefinition = "The sequential number of the interaction, e.g. 1.2.5.") 6140 protected StringType number; 6141 6142 /** 6143 * The type of operation - CRUD. 6144 */ 6145 @Child(name = "type", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 6146 @Description(shortDefinition = "The type of operation - CRUD", formalDefinition = "The type of operation - CRUD.") 6147 protected StringType type; 6148 6149 /** 6150 * The human-friendly name of the interaction. 6151 */ 6152 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 6153 @Description(shortDefinition = "The human-friendly name of the interaction", formalDefinition = "The human-friendly name of the interaction.") 6154 protected StringType name; 6155 6156 /** 6157 * Who starts the transaction. 6158 */ 6159 @Child(name = "initiator", type = { 6160 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 6161 @Description(shortDefinition = "Who starts the transaction", formalDefinition = "Who starts the transaction.") 6162 protected StringType initiator; 6163 6164 /** 6165 * Who receives the transaction. 6166 */ 6167 @Child(name = "receiver", type = { 6168 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 6169 @Description(shortDefinition = "Who receives the transaction", formalDefinition = "Who receives the transaction.") 6170 protected StringType receiver; 6171 6172 /** 6173 * A comment to be inserted in the diagram. 6174 */ 6175 @Child(name = "description", type = { 6176 MarkdownType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 6177 @Description(shortDefinition = "A comment to be inserted in the diagram", formalDefinition = "A comment to be inserted in the diagram.") 6178 protected MarkdownType description; 6179 6180 /** 6181 * Whether the initiator is deactivated right after the transaction. 6182 */ 6183 @Child(name = "initiatorActive", type = { 6184 BooleanType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 6185 @Description(shortDefinition = "Whether the initiator is deactivated right after the transaction", formalDefinition = "Whether the initiator is deactivated right after the transaction.") 6186 protected BooleanType initiatorActive; 6187 6188 /** 6189 * Whether the receiver is deactivated right after the transaction. 6190 */ 6191 @Child(name = "receiverActive", type = { 6192 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 6193 @Description(shortDefinition = "Whether the receiver is deactivated right after the transaction", formalDefinition = "Whether the receiver is deactivated right after the transaction.") 6194 protected BooleanType receiverActive; 6195 6196 /** 6197 * Each resource instance used by the initiator. 6198 */ 6199 @Child(name = "request", type = { 6200 ExampleScenarioInstanceContainedInstanceComponent.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 6201 @Description(shortDefinition = "Each resource instance used by the initiator", formalDefinition = "Each resource instance used by the initiator.") 6202 protected ExampleScenarioInstanceContainedInstanceComponent request; 6203 6204 /** 6205 * Each resource instance used by the responder. 6206 */ 6207 @Child(name = "response", type = { 6208 ExampleScenarioInstanceContainedInstanceComponent.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 6209 @Description(shortDefinition = "Each resource instance used by the responder", formalDefinition = "Each resource instance used by the responder.") 6210 protected ExampleScenarioInstanceContainedInstanceComponent response; 6211 6212 private static final long serialVersionUID = 911241906L; 6213 6214 /** 6215 * Constructor 6216 */ 6217 public ExampleScenarioProcessStepOperationComponent() { 6218 super(); 6219 } 6220 6221 /** 6222 * Constructor 6223 */ 6224 public ExampleScenarioProcessStepOperationComponent(StringType number) { 6225 super(); 6226 this.number = number; 6227 } 6228 6229 /** 6230 * @return {@link #number} (The sequential number of the interaction, e.g. 6231 * 1.2.5.). This is the underlying object with id, value and extensions. 6232 * The accessor "getNumber" gives direct access to the value 6233 */ 6234 public StringType getNumberElement() { 6235 if (this.number == null) 6236 if (Configuration.errorOnAutoCreate()) 6237 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.number"); 6238 else if (Configuration.doAutoCreate()) 6239 this.number = new StringType(); // bb 6240 return this.number; 6241 } 6242 6243 public boolean hasNumberElement() { 6244 return this.number != null && !this.number.isEmpty(); 6245 } 6246 6247 public boolean hasNumber() { 6248 return this.number != null && !this.number.isEmpty(); 6249 } 6250 6251 /** 6252 * @param value {@link #number} (The sequential number of the interaction, e.g. 6253 * 1.2.5.). This is the underlying object with id, value and 6254 * extensions. The accessor "getNumber" gives direct access to the 6255 * value 6256 */ 6257 public ExampleScenarioProcessStepOperationComponent setNumberElement(StringType value) { 6258 this.number = value; 6259 return this; 6260 } 6261 6262 /** 6263 * @return The sequential number of the interaction, e.g. 1.2.5. 6264 */ 6265 public String getNumber() { 6266 return this.number == null ? null : this.number.getValue(); 6267 } 6268 6269 /** 6270 * @param value The sequential number of the interaction, e.g. 1.2.5. 6271 */ 6272 public ExampleScenarioProcessStepOperationComponent setNumber(String value) { 6273 if (this.number == null) 6274 this.number = new StringType(); 6275 this.number.setValue(value); 6276 return this; 6277 } 6278 6279 /** 6280 * @return {@link #type} (The type of operation - CRUD.). This is the underlying 6281 * object with id, value and extensions. The accessor "getType" gives 6282 * direct access to the value 6283 */ 6284 public StringType getTypeElement() { 6285 if (this.type == null) 6286 if (Configuration.errorOnAutoCreate()) 6287 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.type"); 6288 else if (Configuration.doAutoCreate()) 6289 this.type = new StringType(); // bb 6290 return this.type; 6291 } 6292 6293 public boolean hasTypeElement() { 6294 return this.type != null && !this.type.isEmpty(); 6295 } 6296 6297 public boolean hasType() { 6298 return this.type != null && !this.type.isEmpty(); 6299 } 6300 6301 /** 6302 * @param value {@link #type} (The type of operation - CRUD.). This is the 6303 * underlying object with id, value and extensions. The accessor 6304 * "getType" gives direct access to the value 6305 */ 6306 public ExampleScenarioProcessStepOperationComponent setTypeElement(StringType value) { 6307 this.type = value; 6308 return this; 6309 } 6310 6311 /** 6312 * @return The type of operation - CRUD. 6313 */ 6314 public String getType() { 6315 return this.type == null ? null : this.type.getValue(); 6316 } 6317 6318 /** 6319 * @param value The type of operation - CRUD. 6320 */ 6321 public ExampleScenarioProcessStepOperationComponent setType(String value) { 6322 if (Utilities.noString(value)) 6323 this.type = null; 6324 else { 6325 if (this.type == null) 6326 this.type = new StringType(); 6327 this.type.setValue(value); 6328 } 6329 return this; 6330 } 6331 6332 /** 6333 * @return {@link #name} (The human-friendly name of the interaction.). This is 6334 * the underlying object with id, value and extensions. The accessor 6335 * "getName" gives direct access to the value 6336 */ 6337 public StringType getNameElement() { 6338 if (this.name == null) 6339 if (Configuration.errorOnAutoCreate()) 6340 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.name"); 6341 else if (Configuration.doAutoCreate()) 6342 this.name = new StringType(); // bb 6343 return this.name; 6344 } 6345 6346 public boolean hasNameElement() { 6347 return this.name != null && !this.name.isEmpty(); 6348 } 6349 6350 public boolean hasName() { 6351 return this.name != null && !this.name.isEmpty(); 6352 } 6353 6354 /** 6355 * @param value {@link #name} (The human-friendly name of the interaction.). 6356 * This is the underlying object with id, value and extensions. The 6357 * accessor "getName" gives direct access to the value 6358 */ 6359 public ExampleScenarioProcessStepOperationComponent setNameElement(StringType value) { 6360 this.name = value; 6361 return this; 6362 } 6363 6364 /** 6365 * @return The human-friendly name of the interaction. 6366 */ 6367 public String getName() { 6368 return this.name == null ? null : this.name.getValue(); 6369 } 6370 6371 /** 6372 * @param value The human-friendly name of the interaction. 6373 */ 6374 public ExampleScenarioProcessStepOperationComponent setName(String value) { 6375 if (Utilities.noString(value)) 6376 this.name = null; 6377 else { 6378 if (this.name == null) 6379 this.name = new StringType(); 6380 this.name.setValue(value); 6381 } 6382 return this; 6383 } 6384 6385 /** 6386 * @return {@link #initiator} (Who starts the transaction.). This is the 6387 * underlying object with id, value and extensions. The accessor 6388 * "getInitiator" gives direct access to the value 6389 */ 6390 public StringType getInitiatorElement() { 6391 if (this.initiator == null) 6392 if (Configuration.errorOnAutoCreate()) 6393 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiator"); 6394 else if (Configuration.doAutoCreate()) 6395 this.initiator = new StringType(); // bb 6396 return this.initiator; 6397 } 6398 6399 public boolean hasInitiatorElement() { 6400 return this.initiator != null && !this.initiator.isEmpty(); 6401 } 6402 6403 public boolean hasInitiator() { 6404 return this.initiator != null && !this.initiator.isEmpty(); 6405 } 6406 6407 /** 6408 * @param value {@link #initiator} (Who starts the transaction.). This is the 6409 * underlying object with id, value and extensions. The accessor 6410 * "getInitiator" gives direct access to the value 6411 */ 6412 public ExampleScenarioProcessStepOperationComponent setInitiatorElement(StringType value) { 6413 this.initiator = value; 6414 return this; 6415 } 6416 6417 /** 6418 * @return Who starts the transaction. 6419 */ 6420 public String getInitiator() { 6421 return this.initiator == null ? null : this.initiator.getValue(); 6422 } 6423 6424 /** 6425 * @param value Who starts the transaction. 6426 */ 6427 public ExampleScenarioProcessStepOperationComponent setInitiator(String value) { 6428 if (Utilities.noString(value)) 6429 this.initiator = null; 6430 else { 6431 if (this.initiator == null) 6432 this.initiator = new StringType(); 6433 this.initiator.setValue(value); 6434 } 6435 return this; 6436 } 6437 6438 /** 6439 * @return {@link #receiver} (Who receives the transaction.). This is the 6440 * underlying object with id, value and extensions. The accessor 6441 * "getReceiver" gives direct access to the value 6442 */ 6443 public StringType getReceiverElement() { 6444 if (this.receiver == null) 6445 if (Configuration.errorOnAutoCreate()) 6446 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiver"); 6447 else if (Configuration.doAutoCreate()) 6448 this.receiver = new StringType(); // bb 6449 return this.receiver; 6450 } 6451 6452 public boolean hasReceiverElement() { 6453 return this.receiver != null && !this.receiver.isEmpty(); 6454 } 6455 6456 public boolean hasReceiver() { 6457 return this.receiver != null && !this.receiver.isEmpty(); 6458 } 6459 6460 /** 6461 * @param value {@link #receiver} (Who receives the transaction.). This is the 6462 * underlying object with id, value and extensions. The accessor 6463 * "getReceiver" gives direct access to the value 6464 */ 6465 public ExampleScenarioProcessStepOperationComponent setReceiverElement(StringType value) { 6466 this.receiver = value; 6467 return this; 6468 } 6469 6470 /** 6471 * @return Who receives the transaction. 6472 */ 6473 public String getReceiver() { 6474 return this.receiver == null ? null : this.receiver.getValue(); 6475 } 6476 6477 /** 6478 * @param value Who receives the transaction. 6479 */ 6480 public ExampleScenarioProcessStepOperationComponent setReceiver(String value) { 6481 if (Utilities.noString(value)) 6482 this.receiver = null; 6483 else { 6484 if (this.receiver == null) 6485 this.receiver = new StringType(); 6486 this.receiver.setValue(value); 6487 } 6488 return this; 6489 } 6490 6491 /** 6492 * @return {@link #description} (A comment to be inserted in the diagram.). This 6493 * is the underlying object with id, value and extensions. The accessor 6494 * "getDescription" gives direct access to the value 6495 */ 6496 public MarkdownType getDescriptionElement() { 6497 if (this.description == null) 6498 if (Configuration.errorOnAutoCreate()) 6499 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.description"); 6500 else if (Configuration.doAutoCreate()) 6501 this.description = new MarkdownType(); // bb 6502 return this.description; 6503 } 6504 6505 public boolean hasDescriptionElement() { 6506 return this.description != null && !this.description.isEmpty(); 6507 } 6508 6509 public boolean hasDescription() { 6510 return this.description != null && !this.description.isEmpty(); 6511 } 6512 6513 /** 6514 * @param value {@link #description} (A comment to be inserted in the diagram.). 6515 * This is the underlying object with id, value and extensions. The 6516 * accessor "getDescription" gives direct access to the value 6517 */ 6518 public ExampleScenarioProcessStepOperationComponent setDescriptionElement(MarkdownType value) { 6519 this.description = value; 6520 return this; 6521 } 6522 6523 /** 6524 * @return A comment to be inserted in the diagram. 6525 */ 6526 public String getDescription() { 6527 return this.description == null ? null : this.description.getValue(); 6528 } 6529 6530 /** 6531 * @param value A comment to be inserted in the diagram. 6532 */ 6533 public ExampleScenarioProcessStepOperationComponent setDescription(String value) { 6534 if (value == null) 6535 this.description = null; 6536 else { 6537 if (this.description == null) 6538 this.description = new MarkdownType(); 6539 this.description.setValue(value); 6540 } 6541 return this; 6542 } 6543 6544 /** 6545 * @return {@link #initiatorActive} (Whether the initiator is deactivated right 6546 * after the transaction.). This is the underlying object with id, value 6547 * and extensions. The accessor "getInitiatorActive" gives direct access 6548 * to the value 6549 */ 6550 public BooleanType getInitiatorActiveElement() { 6551 if (this.initiatorActive == null) 6552 if (Configuration.errorOnAutoCreate()) 6553 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiatorActive"); 6554 else if (Configuration.doAutoCreate()) 6555 this.initiatorActive = new BooleanType(); // bb 6556 return this.initiatorActive; 6557 } 6558 6559 public boolean hasInitiatorActiveElement() { 6560 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 6561 } 6562 6563 public boolean hasInitiatorActive() { 6564 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 6565 } 6566 6567 /** 6568 * @param value {@link #initiatorActive} (Whether the initiator is deactivated 6569 * right after the transaction.). This is the underlying object 6570 * with id, value and extensions. The accessor "getInitiatorActive" 6571 * gives direct access to the value 6572 */ 6573 public ExampleScenarioProcessStepOperationComponent setInitiatorActiveElement(BooleanType value) { 6574 this.initiatorActive = value; 6575 return this; 6576 } 6577 6578 /** 6579 * @return Whether the initiator is deactivated right after the transaction. 6580 */ 6581 public boolean getInitiatorActive() { 6582 return this.initiatorActive == null || this.initiatorActive.isEmpty() ? false : this.initiatorActive.getValue(); 6583 } 6584 6585 /** 6586 * @param value Whether the initiator is deactivated right after the 6587 * transaction. 6588 */ 6589 public ExampleScenarioProcessStepOperationComponent setInitiatorActive(boolean value) { 6590 if (this.initiatorActive == null) 6591 this.initiatorActive = new BooleanType(); 6592 this.initiatorActive.setValue(value); 6593 return this; 6594 } 6595 6596 /** 6597 * @return {@link #receiverActive} (Whether the receiver is deactivated right 6598 * after the transaction.). This is the underlying object with id, value 6599 * and extensions. The accessor "getReceiverActive" gives direct access 6600 * to the value 6601 */ 6602 public BooleanType getReceiverActiveElement() { 6603 if (this.receiverActive == null) 6604 if (Configuration.errorOnAutoCreate()) 6605 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiverActive"); 6606 else if (Configuration.doAutoCreate()) 6607 this.receiverActive = new BooleanType(); // bb 6608 return this.receiverActive; 6609 } 6610 6611 public boolean hasReceiverActiveElement() { 6612 return this.receiverActive != null && !this.receiverActive.isEmpty(); 6613 } 6614 6615 public boolean hasReceiverActive() { 6616 return this.receiverActive != null && !this.receiverActive.isEmpty(); 6617 } 6618 6619 /** 6620 * @param value {@link #receiverActive} (Whether the receiver is deactivated 6621 * right after the transaction.). This is the underlying object 6622 * with id, value and extensions. The accessor "getReceiverActive" 6623 * gives direct access to the value 6624 */ 6625 public ExampleScenarioProcessStepOperationComponent setReceiverActiveElement(BooleanType value) { 6626 this.receiverActive = value; 6627 return this; 6628 } 6629 6630 /** 6631 * @return Whether the receiver is deactivated right after the transaction. 6632 */ 6633 public boolean getReceiverActive() { 6634 return this.receiverActive == null || this.receiverActive.isEmpty() ? false : this.receiverActive.getValue(); 6635 } 6636 6637 /** 6638 * @param value Whether the receiver is deactivated right after the transaction. 6639 */ 6640 public ExampleScenarioProcessStepOperationComponent setReceiverActive(boolean value) { 6641 if (this.receiverActive == null) 6642 this.receiverActive = new BooleanType(); 6643 this.receiverActive.setValue(value); 6644 return this; 6645 } 6646 6647 /** 6648 * @return {@link #request} (Each resource instance used by the initiator.) 6649 */ 6650 public ExampleScenarioInstanceContainedInstanceComponent getRequest() { 6651 if (this.request == null) 6652 if (Configuration.errorOnAutoCreate()) 6653 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.request"); 6654 else if (Configuration.doAutoCreate()) 6655 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 6656 return this.request; 6657 } 6658 6659 public boolean hasRequest() { 6660 return this.request != null && !this.request.isEmpty(); 6661 } 6662 6663 /** 6664 * @param value {@link #request} (Each resource instance used by the initiator.) 6665 */ 6666 public ExampleScenarioProcessStepOperationComponent setRequest( 6667 ExampleScenarioInstanceContainedInstanceComponent value) { 6668 this.request = value; 6669 return this; 6670 } 6671 6672 /** 6673 * @return {@link #response} (Each resource instance used by the responder.) 6674 */ 6675 public ExampleScenarioInstanceContainedInstanceComponent getResponse() { 6676 if (this.response == null) 6677 if (Configuration.errorOnAutoCreate()) 6678 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.response"); 6679 else if (Configuration.doAutoCreate()) 6680 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 6681 return this.response; 6682 } 6683 6684 public boolean hasResponse() { 6685 return this.response != null && !this.response.isEmpty(); 6686 } 6687 6688 /** 6689 * @param value {@link #response} (Each resource instance used by the 6690 * responder.) 6691 */ 6692 public ExampleScenarioProcessStepOperationComponent setResponse( 6693 ExampleScenarioInstanceContainedInstanceComponent value) { 6694 this.response = value; 6695 return this; 6696 } 6697 6698 protected void listChildren(List<Property> children) { 6699 super.listChildren(children); 6700 children 6701 .add(new Property("number", "string", "The sequential number of the interaction, e.g. 1.2.5.", 0, 1, number)); 6702 children.add(new Property("type", "string", "The type of operation - CRUD.", 0, 1, type)); 6703 children.add(new Property("name", "string", "The human-friendly name of the interaction.", 0, 1, name)); 6704 children.add(new Property("initiator", "string", "Who starts the transaction.", 0, 1, initiator)); 6705 children.add(new Property("receiver", "string", "Who receives the transaction.", 0, 1, receiver)); 6706 children 6707 .add(new Property("description", "markdown", "A comment to be inserted in the diagram.", 0, 1, description)); 6708 children.add(new Property("initiatorActive", "boolean", 6709 "Whether the initiator is deactivated right after the transaction.", 0, 1, initiatorActive)); 6710 children.add(new Property("receiverActive", "boolean", 6711 "Whether the receiver is deactivated right after the transaction.", 0, 1, receiverActive)); 6712 children.add(new Property("request", "@ExampleScenario.instance.containedInstance", 6713 "Each resource instance used by the initiator.", 0, 1, request)); 6714 children.add(new Property("response", "@ExampleScenario.instance.containedInstance", 6715 "Each resource instance used by the responder.", 0, 1, response)); 6716 } 6717 6718 @Override 6719 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6720 switch (_hash) { 6721 case -1034364087: 6722 /* number */ return new Property("number", "string", "The sequential number of the interaction, e.g. 1.2.5.", 0, 6723 1, number); 6724 case 3575610: 6725 /* type */ return new Property("type", "string", "The type of operation - CRUD.", 0, 1, type); 6726 case 3373707: 6727 /* name */ return new Property("name", "string", "The human-friendly name of the interaction.", 0, 1, name); 6728 case -248987089: 6729 /* initiator */ return new Property("initiator", "string", "Who starts the transaction.", 0, 1, initiator); 6730 case -808719889: 6731 /* receiver */ return new Property("receiver", "string", "Who receives the transaction.", 0, 1, receiver); 6732 case -1724546052: 6733 /* description */ return new Property("description", "markdown", "A comment to be inserted in the diagram.", 0, 6734 1, description); 6735 case 384339477: 6736 /* initiatorActive */ return new Property("initiatorActive", "boolean", 6737 "Whether the initiator is deactivated right after the transaction.", 0, 1, initiatorActive); 6738 case -285284907: 6739 /* receiverActive */ return new Property("receiverActive", "boolean", 6740 "Whether the receiver is deactivated right after the transaction.", 0, 1, receiverActive); 6741 case 1095692943: 6742 /* request */ return new Property("request", "@ExampleScenario.instance.containedInstance", 6743 "Each resource instance used by the initiator.", 0, 1, request); 6744 case -340323263: 6745 /* response */ return new Property("response", "@ExampleScenario.instance.containedInstance", 6746 "Each resource instance used by the responder.", 0, 1, response); 6747 default: 6748 return super.getNamedProperty(_hash, _name, _checkValid); 6749 } 6750 6751 } 6752 6753 @Override 6754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6755 switch (hash) { 6756 case -1034364087: 6757 /* number */ return this.number == null ? new Base[0] : new Base[] { this.number }; // StringType 6758 case 3575610: 6759 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // StringType 6760 case 3373707: 6761 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 6762 case -248987089: 6763 /* initiator */ return this.initiator == null ? new Base[0] : new Base[] { this.initiator }; // StringType 6764 case -808719889: 6765 /* receiver */ return this.receiver == null ? new Base[0] : new Base[] { this.receiver }; // StringType 6766 case -1724546052: 6767 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 6768 case 384339477: 6769 /* initiatorActive */ return this.initiatorActive == null ? new Base[0] : new Base[] { this.initiatorActive }; // BooleanType 6770 case -285284907: 6771 /* receiverActive */ return this.receiverActive == null ? new Base[0] : new Base[] { this.receiverActive }; // BooleanType 6772 case 1095692943: 6773 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // ExampleScenarioInstanceContainedInstanceComponent 6774 case -340323263: 6775 /* response */ return this.response == null ? new Base[0] : new Base[] { this.response }; // ExampleScenarioInstanceContainedInstanceComponent 6776 default: 6777 return super.getProperty(hash, name, checkValid); 6778 } 6779 6780 } 6781 6782 @Override 6783 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6784 switch (hash) { 6785 case -1034364087: // number 6786 this.number = castToString(value); // StringType 6787 return value; 6788 case 3575610: // type 6789 this.type = castToString(value); // StringType 6790 return value; 6791 case 3373707: // name 6792 this.name = castToString(value); // StringType 6793 return value; 6794 case -248987089: // initiator 6795 this.initiator = castToString(value); // StringType 6796 return value; 6797 case -808719889: // receiver 6798 this.receiver = castToString(value); // StringType 6799 return value; 6800 case -1724546052: // description 6801 this.description = castToMarkdown(value); // MarkdownType 6802 return value; 6803 case 384339477: // initiatorActive 6804 this.initiatorActive = castToBoolean(value); // BooleanType 6805 return value; 6806 case -285284907: // receiverActive 6807 this.receiverActive = castToBoolean(value); // BooleanType 6808 return value; 6809 case 1095692943: // request 6810 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6811 return value; 6812 case -340323263: // response 6813 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6814 return value; 6815 default: 6816 return super.setProperty(hash, name, value); 6817 } 6818 6819 } 6820 6821 @Override 6822 public Base setProperty(String name, Base value) throws FHIRException { 6823 if (name.equals("number")) { 6824 this.number = castToString(value); // StringType 6825 } else if (name.equals("type")) { 6826 this.type = castToString(value); // StringType 6827 } else if (name.equals("name")) { 6828 this.name = castToString(value); // StringType 6829 } else if (name.equals("initiator")) { 6830 this.initiator = castToString(value); // StringType 6831 } else if (name.equals("receiver")) { 6832 this.receiver = castToString(value); // StringType 6833 } else if (name.equals("description")) { 6834 this.description = castToMarkdown(value); // MarkdownType 6835 } else if (name.equals("initiatorActive")) { 6836 this.initiatorActive = castToBoolean(value); // BooleanType 6837 } else if (name.equals("receiverActive")) { 6838 this.receiverActive = castToBoolean(value); // BooleanType 6839 } else if (name.equals("request")) { 6840 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6841 } else if (name.equals("response")) { 6842 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6843 } else 6844 return super.setProperty(name, value); 6845 return value; 6846 } 6847 6848 @Override 6849 public void removeChild(String name, Base value) throws FHIRException { 6850 if (name.equals("number")) { 6851 this.number = null; 6852 } else if (name.equals("type")) { 6853 this.type = null; 6854 } else if (name.equals("name")) { 6855 this.name = null; 6856 } else if (name.equals("initiator")) { 6857 this.initiator = null; 6858 } else if (name.equals("receiver")) { 6859 this.receiver = null; 6860 } else if (name.equals("description")) { 6861 this.description = null; 6862 } else if (name.equals("initiatorActive")) { 6863 this.initiatorActive = null; 6864 } else if (name.equals("receiverActive")) { 6865 this.receiverActive = null; 6866 } else if (name.equals("request")) { 6867 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6868 } else if (name.equals("response")) { 6869 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 6870 } else 6871 super.removeChild(name, value); 6872 6873 } 6874 6875 @Override 6876 public Base makeProperty(int hash, String name) throws FHIRException { 6877 switch (hash) { 6878 case -1034364087: 6879 return getNumberElement(); 6880 case 3575610: 6881 return getTypeElement(); 6882 case 3373707: 6883 return getNameElement(); 6884 case -248987089: 6885 return getInitiatorElement(); 6886 case -808719889: 6887 return getReceiverElement(); 6888 case -1724546052: 6889 return getDescriptionElement(); 6890 case 384339477: 6891 return getInitiatorActiveElement(); 6892 case -285284907: 6893 return getReceiverActiveElement(); 6894 case 1095692943: 6895 return getRequest(); 6896 case -340323263: 6897 return getResponse(); 6898 default: 6899 return super.makeProperty(hash, name); 6900 } 6901 6902 } 6903 6904 @Override 6905 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6906 switch (hash) { 6907 case -1034364087: 6908 /* number */ return new String[] { "string" }; 6909 case 3575610: 6910 /* type */ return new String[] { "string" }; 6911 case 3373707: 6912 /* name */ return new String[] { "string" }; 6913 case -248987089: 6914 /* initiator */ return new String[] { "string" }; 6915 case -808719889: 6916 /* receiver */ return new String[] { "string" }; 6917 case -1724546052: 6918 /* description */ return new String[] { "markdown" }; 6919 case 384339477: 6920 /* initiatorActive */ return new String[] { "boolean" }; 6921 case -285284907: 6922 /* receiverActive */ return new String[] { "boolean" }; 6923 case 1095692943: 6924 /* request */ return new String[] { "@ExampleScenario.instance.containedInstance" }; 6925 case -340323263: 6926 /* response */ return new String[] { "@ExampleScenario.instance.containedInstance" }; 6927 default: 6928 return super.getTypesForProperty(hash, name); 6929 } 6930 6931 } 6932 6933 @Override 6934 public Base addChild(String name) throws FHIRException { 6935 if (name.equals("number")) { 6936 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.number"); 6937 } else if (name.equals("type")) { 6938 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.type"); 6939 } else if (name.equals("name")) { 6940 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 6941 } else if (name.equals("initiator")) { 6942 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.initiator"); 6943 } else if (name.equals("receiver")) { 6944 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.receiver"); 6945 } else if (name.equals("description")) { 6946 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 6947 } else if (name.equals("initiatorActive")) { 6948 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.initiatorActive"); 6949 } else if (name.equals("receiverActive")) { 6950 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.receiverActive"); 6951 } else if (name.equals("request")) { 6952 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); 6953 return this.request; 6954 } else if (name.equals("response")) { 6955 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); 6956 return this.response; 6957 } else 6958 return super.addChild(name); 6959 } 6960 6961 public ExampleScenarioProcessStepOperationComponent copy() { 6962 ExampleScenarioProcessStepOperationComponent dst = new ExampleScenarioProcessStepOperationComponent(); 6963 copyValues(dst); 6964 return dst; 6965 } 6966 6967 public void copyValues(ExampleScenarioProcessStepOperationComponent dst) { 6968 super.copyValues(dst); 6969 dst.number = number == null ? null : number.copy(); 6970 dst.type = type == null ? null : type.copy(); 6971 dst.name = name == null ? null : name.copy(); 6972 dst.initiator = initiator == null ? null : initiator.copy(); 6973 dst.receiver = receiver == null ? null : receiver.copy(); 6974 dst.description = description == null ? null : description.copy(); 6975 dst.initiatorActive = initiatorActive == null ? null : initiatorActive.copy(); 6976 dst.receiverActive = receiverActive == null ? null : receiverActive.copy(); 6977 dst.request = request == null ? null : request.copy(); 6978 dst.response = response == null ? null : response.copy(); 6979 } 6980 6981 @Override 6982 public boolean equalsDeep(Base other_) { 6983 if (!super.equalsDeep(other_)) 6984 return false; 6985 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 6986 return false; 6987 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 6988 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) 6989 && compareDeep(initiator, o.initiator, true) && compareDeep(receiver, o.receiver, true) 6990 && compareDeep(description, o.description, true) && compareDeep(initiatorActive, o.initiatorActive, true) 6991 && compareDeep(receiverActive, o.receiverActive, true) && compareDeep(request, o.request, true) 6992 && compareDeep(response, o.response, true); 6993 } 6994 6995 @Override 6996 public boolean equalsShallow(Base other_) { 6997 if (!super.equalsShallow(other_)) 6998 return false; 6999 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 7000 return false; 7001 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 7002 return compareValues(number, o.number, true) && compareValues(type, o.type, true) 7003 && compareValues(name, o.name, true) && compareValues(initiator, o.initiator, true) 7004 && compareValues(receiver, o.receiver, true) && compareValues(description, o.description, true) 7005 && compareValues(initiatorActive, o.initiatorActive, true) 7006 && compareValues(receiverActive, o.receiverActive, true); 7007 } 7008 7009 public boolean isEmpty() { 7010 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, name, initiator, receiver, 7011 description, initiatorActive, receiverActive, request, response); 7012 } 7013 7014 public String fhirType() { 7015 return "ExampleScenario.process.step.operation"; 7016 7017 } 7018 7019 } 7020 7021 @Block() 7022 public static class ExampleScenarioProcessStepAlternativeComponent extends BackboneElement 7023 implements IBaseBackboneElement { 7024 /** 7025 * The label to display for the alternative that gives a sense of the 7026 * circumstance in which the alternative should be invoked. 7027 */ 7028 @Child(name = "title", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 7029 @Description(shortDefinition = "Label for alternative", formalDefinition = "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.") 7030 protected StringType title; 7031 7032 /** 7033 * A human-readable description of the alternative explaining when the 7034 * alternative should occur rather than the base step. 7035 */ 7036 @Child(name = "description", type = { 7037 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7038 @Description(shortDefinition = "A human-readable description of each option", formalDefinition = "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.") 7039 protected MarkdownType description; 7040 7041 /** 7042 * What happens in each alternative option. 7043 */ 7044 @Child(name = "step", type = { 7045 ExampleScenarioProcessStepComponent.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7046 @Description(shortDefinition = "What happens in each alternative option", formalDefinition = "What happens in each alternative option.") 7047 protected List<ExampleScenarioProcessStepComponent> step; 7048 7049 private static final long serialVersionUID = -254687460L; 7050 7051 /** 7052 * Constructor 7053 */ 7054 public ExampleScenarioProcessStepAlternativeComponent() { 7055 super(); 7056 } 7057 7058 /** 7059 * Constructor 7060 */ 7061 public ExampleScenarioProcessStepAlternativeComponent(StringType title) { 7062 super(); 7063 this.title = title; 7064 } 7065 7066 /** 7067 * @return {@link #title} (The label to display for the alternative that gives a 7068 * sense of the circumstance in which the alternative should be 7069 * invoked.). This is the underlying object with id, value and 7070 * extensions. The accessor "getTitle" gives direct access to the value 7071 */ 7072 public StringType getTitleElement() { 7073 if (this.title == null) 7074 if (Configuration.errorOnAutoCreate()) 7075 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.title"); 7076 else if (Configuration.doAutoCreate()) 7077 this.title = new StringType(); // bb 7078 return this.title; 7079 } 7080 7081 public boolean hasTitleElement() { 7082 return this.title != null && !this.title.isEmpty(); 7083 } 7084 7085 public boolean hasTitle() { 7086 return this.title != null && !this.title.isEmpty(); 7087 } 7088 7089 /** 7090 * @param value {@link #title} (The label to display for the alternative that 7091 * gives a sense of the circumstance in which the alternative 7092 * should be invoked.). This is the underlying object with id, 7093 * value and extensions. The accessor "getTitle" gives direct 7094 * access to the value 7095 */ 7096 public ExampleScenarioProcessStepAlternativeComponent setTitleElement(StringType value) { 7097 this.title = value; 7098 return this; 7099 } 7100 7101 /** 7102 * @return The label to display for the alternative that gives a sense of the 7103 * circumstance in which the alternative should be invoked. 7104 */ 7105 public String getTitle() { 7106 return this.title == null ? null : this.title.getValue(); 7107 } 7108 7109 /** 7110 * @param value The label to display for the alternative that gives a sense of 7111 * the circumstance in which the alternative should be invoked. 7112 */ 7113 public ExampleScenarioProcessStepAlternativeComponent setTitle(String value) { 7114 if (this.title == null) 7115 this.title = new StringType(); 7116 this.title.setValue(value); 7117 return this; 7118 } 7119 7120 /** 7121 * @return {@link #description} (A human-readable description of the alternative 7122 * explaining when the alternative should occur rather than the base 7123 * step.). This is the underlying object with id, value and extensions. 7124 * The accessor "getDescription" gives direct access to the value 7125 */ 7126 public MarkdownType getDescriptionElement() { 7127 if (this.description == null) 7128 if (Configuration.errorOnAutoCreate()) 7129 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.description"); 7130 else if (Configuration.doAutoCreate()) 7131 this.description = new MarkdownType(); // bb 7132 return this.description; 7133 } 7134 7135 public boolean hasDescriptionElement() { 7136 return this.description != null && !this.description.isEmpty(); 7137 } 7138 7139 public boolean hasDescription() { 7140 return this.description != null && !this.description.isEmpty(); 7141 } 7142 7143 /** 7144 * @param value {@link #description} (A human-readable description of the 7145 * alternative explaining when the alternative should occur rather 7146 * than the base step.). This is the underlying object with id, 7147 * value and extensions. The accessor "getDescription" gives direct 7148 * access to the value 7149 */ 7150 public ExampleScenarioProcessStepAlternativeComponent setDescriptionElement(MarkdownType value) { 7151 this.description = value; 7152 return this; 7153 } 7154 7155 /** 7156 * @return A human-readable description of the alternative explaining when the 7157 * alternative should occur rather than the base step. 7158 */ 7159 public String getDescription() { 7160 return this.description == null ? null : this.description.getValue(); 7161 } 7162 7163 /** 7164 * @param value A human-readable description of the alternative explaining when 7165 * the alternative should occur rather than the base step. 7166 */ 7167 public ExampleScenarioProcessStepAlternativeComponent setDescription(String value) { 7168 if (value == null) 7169 this.description = null; 7170 else { 7171 if (this.description == null) 7172 this.description = new MarkdownType(); 7173 this.description.setValue(value); 7174 } 7175 return this; 7176 } 7177 7178 /** 7179 * @return {@link #step} (What happens in each alternative option.) 7180 */ 7181 public List<ExampleScenarioProcessStepComponent> getStep() { 7182 if (this.step == null) 7183 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7184 return this.step; 7185 } 7186 7187 /** 7188 * @return Returns a reference to <code>this</code> for easy method chaining 7189 */ 7190 public ExampleScenarioProcessStepAlternativeComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 7191 this.step = theStep; 7192 return this; 7193 } 7194 7195 public boolean hasStep() { 7196 if (this.step == null) 7197 return false; 7198 for (ExampleScenarioProcessStepComponent item : this.step) 7199 if (!item.isEmpty()) 7200 return true; 7201 return false; 7202 } 7203 7204 public ExampleScenarioProcessStepComponent addStep() { // 3 7205 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 7206 if (this.step == null) 7207 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7208 this.step.add(t); 7209 return t; 7210 } 7211 7212 public ExampleScenarioProcessStepAlternativeComponent addStep(ExampleScenarioProcessStepComponent t) { // 3 7213 if (t == null) 7214 return this; 7215 if (this.step == null) 7216 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7217 this.step.add(t); 7218 return this; 7219 } 7220 7221 /** 7222 * @return The first repetition of repeating field {@link #step}, creating it if 7223 * it does not already exist 7224 */ 7225 public ExampleScenarioProcessStepComponent getStepFirstRep() { 7226 if (getStep().isEmpty()) { 7227 addStep(); 7228 } 7229 return getStep().get(0); 7230 } 7231 7232 protected void listChildren(List<Property> children) { 7233 super.listChildren(children); 7234 children.add(new Property("title", "string", 7235 "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 7236 0, 1, title)); 7237 children.add(new Property("description", "markdown", 7238 "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 7239 0, 1, description)); 7240 children.add(new Property("step", "@ExampleScenario.process.step", "What happens in each alternative option.", 0, 7241 java.lang.Integer.MAX_VALUE, step)); 7242 } 7243 7244 @Override 7245 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7246 switch (_hash) { 7247 case 110371416: 7248 /* title */ return new Property("title", "string", 7249 "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 7250 0, 1, title); 7251 case -1724546052: 7252 /* description */ return new Property("description", "markdown", 7253 "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 7254 0, 1, description); 7255 case 3540684: 7256 /* step */ return new Property("step", "@ExampleScenario.process.step", 7257 "What happens in each alternative option.", 0, java.lang.Integer.MAX_VALUE, step); 7258 default: 7259 return super.getNamedProperty(_hash, _name, _checkValid); 7260 } 7261 7262 } 7263 7264 @Override 7265 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7266 switch (hash) { 7267 case 110371416: 7268 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 7269 case -1724546052: 7270 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 7271 case 3540684: 7272 /* step */ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 7273 default: 7274 return super.getProperty(hash, name, checkValid); 7275 } 7276 7277 } 7278 7279 @Override 7280 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7281 switch (hash) { 7282 case 110371416: // title 7283 this.title = castToString(value); // StringType 7284 return value; 7285 case -1724546052: // description 7286 this.description = castToMarkdown(value); // MarkdownType 7287 return value; 7288 case 3540684: // step 7289 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 7290 return value; 7291 default: 7292 return super.setProperty(hash, name, value); 7293 } 7294 7295 } 7296 7297 @Override 7298 public Base setProperty(String name, Base value) throws FHIRException { 7299 if (name.equals("title")) { 7300 this.title = castToString(value); // StringType 7301 } else if (name.equals("description")) { 7302 this.description = castToMarkdown(value); // MarkdownType 7303 } else if (name.equals("step")) { 7304 this.getStep().add((ExampleScenarioProcessStepComponent) value); 7305 } else 7306 return super.setProperty(name, value); 7307 return value; 7308 } 7309 7310 @Override 7311 public void removeChild(String name, Base value) throws FHIRException { 7312 if (name.equals("title")) { 7313 this.title = null; 7314 } else if (name.equals("description")) { 7315 this.description = null; 7316 } else if (name.equals("step")) { 7317 this.getStep().remove((ExampleScenarioProcessStepComponent) value); 7318 } else 7319 super.removeChild(name, value); 7320 7321 } 7322 7323 @Override 7324 public Base makeProperty(int hash, String name) throws FHIRException { 7325 switch (hash) { 7326 case 110371416: 7327 return getTitleElement(); 7328 case -1724546052: 7329 return getDescriptionElement(); 7330 case 3540684: 7331 return addStep(); 7332 default: 7333 return super.makeProperty(hash, name); 7334 } 7335 7336 } 7337 7338 @Override 7339 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7340 switch (hash) { 7341 case 110371416: 7342 /* title */ return new String[] { "string" }; 7343 case -1724546052: 7344 /* description */ return new String[] { "markdown" }; 7345 case 3540684: 7346 /* step */ return new String[] { "@ExampleScenario.process.step" }; 7347 default: 7348 return super.getTypesForProperty(hash, name); 7349 } 7350 7351 } 7352 7353 @Override 7354 public Base addChild(String name) throws FHIRException { 7355 if (name.equals("title")) { 7356 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.title"); 7357 } else if (name.equals("description")) { 7358 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 7359 } else if (name.equals("step")) { 7360 return addStep(); 7361 } else 7362 return super.addChild(name); 7363 } 7364 7365 public ExampleScenarioProcessStepAlternativeComponent copy() { 7366 ExampleScenarioProcessStepAlternativeComponent dst = new ExampleScenarioProcessStepAlternativeComponent(); 7367 copyValues(dst); 7368 return dst; 7369 } 7370 7371 public void copyValues(ExampleScenarioProcessStepAlternativeComponent dst) { 7372 super.copyValues(dst); 7373 dst.title = title == null ? null : title.copy(); 7374 dst.description = description == null ? null : description.copy(); 7375 if (step != null) { 7376 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 7377 for (ExampleScenarioProcessStepComponent i : step) 7378 dst.step.add(i.copy()); 7379 } 7380 ; 7381 } 7382 7383 @Override 7384 public boolean equalsDeep(Base other_) { 7385 if (!super.equalsDeep(other_)) 7386 return false; 7387 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 7388 return false; 7389 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 7390 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 7391 && compareDeep(step, o.step, true); 7392 } 7393 7394 @Override 7395 public boolean equalsShallow(Base other_) { 7396 if (!super.equalsShallow(other_)) 7397 return false; 7398 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 7399 return false; 7400 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 7401 return compareValues(title, o.title, true) && compareValues(description, o.description, true); 7402 } 7403 7404 public boolean isEmpty() { 7405 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, step); 7406 } 7407 7408 public String fhirType() { 7409 return "ExampleScenario.process.step.alternative"; 7410 7411 } 7412 7413 } 7414 7415 /** 7416 * A formal identifier that is used to identify this example scenario when it is 7417 * represented in other formats, or referenced in a specification, model, design 7418 * or an instance. 7419 */ 7420 @Child(name = "identifier", type = { 7421 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 7422 @Description(shortDefinition = "Additional identifier for the example scenario", formalDefinition = "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.") 7423 protected List<Identifier> identifier; 7424 7425 /** 7426 * A copyright statement relating to the example scenario and/or its contents. 7427 * Copyright statements are generally legal restrictions on the use and 7428 * publishing of the example scenario. 7429 */ 7430 @Child(name = "copyright", type = { 7431 MarkdownType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 7432 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.") 7433 protected MarkdownType copyright; 7434 7435 /** 7436 * What the example scenario resource is created for. This should not be used to 7437 * show the business purpose of the scenario itself, but the purpose of 7438 * documenting a scenario. 7439 */ 7440 @Child(name = "purpose", type = { 7441 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 7442 @Description(shortDefinition = "The purpose of the example, e.g. to illustrate a scenario", formalDefinition = "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.") 7443 protected MarkdownType purpose; 7444 7445 /** 7446 * Actor participating in the resource. 7447 */ 7448 @Child(name = "actor", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7449 @Description(shortDefinition = "Actor participating in the resource", formalDefinition = "Actor participating in the resource.") 7450 protected List<ExampleScenarioActorComponent> actor; 7451 7452 /** 7453 * Each resource and each version that is present in the workflow. 7454 */ 7455 @Child(name = "instance", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7456 @Description(shortDefinition = "Each resource and each version that is present in the workflow", formalDefinition = "Each resource and each version that is present in the workflow.") 7457 protected List<ExampleScenarioInstanceComponent> instance; 7458 7459 /** 7460 * Each major process - a group of operations. 7461 */ 7462 @Child(name = "process", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7463 @Description(shortDefinition = "Each major process - a group of operations", formalDefinition = "Each major process - a group of operations.") 7464 protected List<ExampleScenarioProcessComponent> process; 7465 7466 /** 7467 * Another nested workflow. 7468 */ 7469 @Child(name = "workflow", type = { 7470 CanonicalType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 7471 @Description(shortDefinition = "Another nested workflow", formalDefinition = "Another nested workflow.") 7472 protected List<CanonicalType> workflow; 7473 7474 private static final long serialVersionUID = 758248907L; 7475 7476 /** 7477 * Constructor 7478 */ 7479 public ExampleScenario() { 7480 super(); 7481 } 7482 7483 /** 7484 * Constructor 7485 */ 7486 public ExampleScenario(Enumeration<PublicationStatus> status) { 7487 super(); 7488 this.status = status; 7489 } 7490 7491 /** 7492 * @return {@link #url} (An absolute URI that is used to identify this example 7493 * scenario when it is referenced in a specification, model, design or 7494 * an instance; also called its canonical identifier. This SHOULD be 7495 * globally unique and SHOULD be a literal address at which at which an 7496 * authoritative instance of this example scenario is (or will be) 7497 * published. This URL can be the target of a canonical reference. It 7498 * SHALL remain the same when the example scenario is stored on 7499 * different servers.). This is the underlying object with id, value and 7500 * extensions. The accessor "getUrl" gives direct access to the value 7501 */ 7502 public UriType getUrlElement() { 7503 if (this.url == null) 7504 if (Configuration.errorOnAutoCreate()) 7505 throw new Error("Attempt to auto-create ExampleScenario.url"); 7506 else if (Configuration.doAutoCreate()) 7507 this.url = new UriType(); // bb 7508 return this.url; 7509 } 7510 7511 public boolean hasUrlElement() { 7512 return this.url != null && !this.url.isEmpty(); 7513 } 7514 7515 public boolean hasUrl() { 7516 return this.url != null && !this.url.isEmpty(); 7517 } 7518 7519 /** 7520 * @param value {@link #url} (An absolute URI that is used to identify this 7521 * example scenario when it is referenced in a specification, 7522 * model, design or an instance; also called its canonical 7523 * identifier. This SHOULD be globally unique and SHOULD be a 7524 * literal address at which at which an authoritative instance of 7525 * this example scenario is (or will be) published. This URL can be 7526 * the target of a canonical reference. It SHALL remain the same 7527 * when the example scenario is stored on different servers.). This 7528 * is the underlying object with id, value and extensions. The 7529 * accessor "getUrl" gives direct access to the value 7530 */ 7531 public ExampleScenario setUrlElement(UriType value) { 7532 this.url = value; 7533 return this; 7534 } 7535 7536 /** 7537 * @return An absolute URI that is used to identify this example scenario when 7538 * it is referenced in a specification, model, design or an instance; 7539 * also called its canonical identifier. This SHOULD be globally unique 7540 * and SHOULD be a literal address at which at which an authoritative 7541 * instance of this example scenario is (or will be) published. This URL 7542 * can be the target of a canonical reference. It SHALL remain the same 7543 * when the example scenario is stored on different servers. 7544 */ 7545 public String getUrl() { 7546 return this.url == null ? null : this.url.getValue(); 7547 } 7548 7549 /** 7550 * @param value An absolute URI that is used to identify this example scenario 7551 * when it is referenced in a specification, model, design or an 7552 * instance; also called its canonical identifier. This SHOULD be 7553 * globally unique and SHOULD be a literal address at which at 7554 * which an authoritative instance of this example scenario is (or 7555 * will be) published. This URL can be the target of a canonical 7556 * reference. It SHALL remain the same when the example scenario is 7557 * stored on different servers. 7558 */ 7559 public ExampleScenario setUrl(String value) { 7560 if (Utilities.noString(value)) 7561 this.url = null; 7562 else { 7563 if (this.url == null) 7564 this.url = new UriType(); 7565 this.url.setValue(value); 7566 } 7567 return this; 7568 } 7569 7570 /** 7571 * @return {@link #identifier} (A formal identifier that is used to identify 7572 * this example scenario when it is represented in other formats, or 7573 * referenced in a specification, model, design or an instance.) 7574 */ 7575 public List<Identifier> getIdentifier() { 7576 if (this.identifier == null) 7577 this.identifier = new ArrayList<Identifier>(); 7578 return this.identifier; 7579 } 7580 7581 /** 7582 * @return Returns a reference to <code>this</code> for easy method chaining 7583 */ 7584 public ExampleScenario setIdentifier(List<Identifier> theIdentifier) { 7585 this.identifier = theIdentifier; 7586 return this; 7587 } 7588 7589 public boolean hasIdentifier() { 7590 if (this.identifier == null) 7591 return false; 7592 for (Identifier item : this.identifier) 7593 if (!item.isEmpty()) 7594 return true; 7595 return false; 7596 } 7597 7598 public Identifier addIdentifier() { // 3 7599 Identifier t = new Identifier(); 7600 if (this.identifier == null) 7601 this.identifier = new ArrayList<Identifier>(); 7602 this.identifier.add(t); 7603 return t; 7604 } 7605 7606 public ExampleScenario addIdentifier(Identifier t) { // 3 7607 if (t == null) 7608 return this; 7609 if (this.identifier == null) 7610 this.identifier = new ArrayList<Identifier>(); 7611 this.identifier.add(t); 7612 return this; 7613 } 7614 7615 /** 7616 * @return The first repetition of repeating field {@link #identifier}, creating 7617 * it if it does not already exist 7618 */ 7619 public Identifier getIdentifierFirstRep() { 7620 if (getIdentifier().isEmpty()) { 7621 addIdentifier(); 7622 } 7623 return getIdentifier().get(0); 7624 } 7625 7626 /** 7627 * @return {@link #version} (The identifier that is used to identify this 7628 * version of the example scenario when it is referenced in a 7629 * specification, model, design or instance. This is an arbitrary value 7630 * managed by the example scenario author and is not expected to be 7631 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 7632 * if a managed version is not available. There is also no expectation 7633 * that versions can be placed in a lexicographical sequence.). This is 7634 * the underlying object with id, value and extensions. The accessor 7635 * "getVersion" gives direct access to the value 7636 */ 7637 public StringType getVersionElement() { 7638 if (this.version == null) 7639 if (Configuration.errorOnAutoCreate()) 7640 throw new Error("Attempt to auto-create ExampleScenario.version"); 7641 else if (Configuration.doAutoCreate()) 7642 this.version = new StringType(); // bb 7643 return this.version; 7644 } 7645 7646 public boolean hasVersionElement() { 7647 return this.version != null && !this.version.isEmpty(); 7648 } 7649 7650 public boolean hasVersion() { 7651 return this.version != null && !this.version.isEmpty(); 7652 } 7653 7654 /** 7655 * @param value {@link #version} (The identifier that is used to identify this 7656 * version of the example scenario when it is referenced in a 7657 * specification, model, design or instance. This is an arbitrary 7658 * value managed by the example scenario author and is not expected 7659 * to be globally unique. For example, it might be a timestamp 7660 * (e.g. yyyymmdd) if a managed version is not available. There is 7661 * also no expectation that versions can be placed in a 7662 * lexicographical sequence.). This is the underlying object with 7663 * id, value and extensions. The accessor "getVersion" gives direct 7664 * access to the value 7665 */ 7666 public ExampleScenario setVersionElement(StringType value) { 7667 this.version = value; 7668 return this; 7669 } 7670 7671 /** 7672 * @return The identifier that is used to identify this version of the example 7673 * scenario when it is referenced in a specification, model, design or 7674 * instance. This is an arbitrary value managed by the example scenario 7675 * author and is not expected to be globally unique. For example, it 7676 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 7677 * available. There is also no expectation that versions can be placed 7678 * in a lexicographical sequence. 7679 */ 7680 public String getVersion() { 7681 return this.version == null ? null : this.version.getValue(); 7682 } 7683 7684 /** 7685 * @param value The identifier that is used to identify this version of the 7686 * example scenario when it is referenced in a specification, 7687 * model, design or instance. This is an arbitrary value managed by 7688 * the example scenario author and is not expected to be globally 7689 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 7690 * a managed version is not available. There is also no expectation 7691 * that versions can be placed in a lexicographical sequence. 7692 */ 7693 public ExampleScenario setVersion(String value) { 7694 if (Utilities.noString(value)) 7695 this.version = null; 7696 else { 7697 if (this.version == null) 7698 this.version = new StringType(); 7699 this.version.setValue(value); 7700 } 7701 return this; 7702 } 7703 7704 /** 7705 * @return {@link #name} (A natural language name identifying the example 7706 * scenario. This name should be usable as an identifier for the module 7707 * by machine processing applications such as code generation.). This is 7708 * the underlying object with id, value and extensions. The accessor 7709 * "getName" gives direct access to the value 7710 */ 7711 public StringType getNameElement() { 7712 if (this.name == null) 7713 if (Configuration.errorOnAutoCreate()) 7714 throw new Error("Attempt to auto-create ExampleScenario.name"); 7715 else if (Configuration.doAutoCreate()) 7716 this.name = new StringType(); // bb 7717 return this.name; 7718 } 7719 7720 public boolean hasNameElement() { 7721 return this.name != null && !this.name.isEmpty(); 7722 } 7723 7724 public boolean hasName() { 7725 return this.name != null && !this.name.isEmpty(); 7726 } 7727 7728 /** 7729 * @param value {@link #name} (A natural language name identifying the example 7730 * scenario. This name should be usable as an identifier for the 7731 * module by machine processing applications such as code 7732 * generation.). This is the underlying object with id, value and 7733 * extensions. The accessor "getName" gives direct access to the 7734 * value 7735 */ 7736 public ExampleScenario setNameElement(StringType value) { 7737 this.name = value; 7738 return this; 7739 } 7740 7741 /** 7742 * @return A natural language name identifying the example scenario. This name 7743 * should be usable as an identifier for the module by machine 7744 * processing applications such as code generation. 7745 */ 7746 public String getName() { 7747 return this.name == null ? null : this.name.getValue(); 7748 } 7749 7750 /** 7751 * @param value A natural language name identifying the example scenario. This 7752 * name should be usable as an identifier for the module by machine 7753 * processing applications such as code generation. 7754 */ 7755 public ExampleScenario setName(String value) { 7756 if (Utilities.noString(value)) 7757 this.name = null; 7758 else { 7759 if (this.name == null) 7760 this.name = new StringType(); 7761 this.name.setValue(value); 7762 } 7763 return this; 7764 } 7765 7766 /** 7767 * @return {@link #status} (The status of this example scenario. Enables 7768 * tracking the life-cycle of the content.). This is the underlying 7769 * object with id, value and extensions. The accessor "getStatus" gives 7770 * direct access to the value 7771 */ 7772 public Enumeration<PublicationStatus> getStatusElement() { 7773 if (this.status == null) 7774 if (Configuration.errorOnAutoCreate()) 7775 throw new Error("Attempt to auto-create ExampleScenario.status"); 7776 else if (Configuration.doAutoCreate()) 7777 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 7778 return this.status; 7779 } 7780 7781 public boolean hasStatusElement() { 7782 return this.status != null && !this.status.isEmpty(); 7783 } 7784 7785 public boolean hasStatus() { 7786 return this.status != null && !this.status.isEmpty(); 7787 } 7788 7789 /** 7790 * @param value {@link #status} (The status of this example scenario. Enables 7791 * tracking the life-cycle of the content.). This is the underlying 7792 * object with id, value and extensions. The accessor "getStatus" 7793 * gives direct access to the value 7794 */ 7795 public ExampleScenario setStatusElement(Enumeration<PublicationStatus> value) { 7796 this.status = value; 7797 return this; 7798 } 7799 7800 /** 7801 * @return The status of this example scenario. Enables tracking the life-cycle 7802 * of the content. 7803 */ 7804 public PublicationStatus getStatus() { 7805 return this.status == null ? null : this.status.getValue(); 7806 } 7807 7808 /** 7809 * @param value The status of this example scenario. Enables tracking the 7810 * life-cycle of the content. 7811 */ 7812 public ExampleScenario setStatus(PublicationStatus value) { 7813 if (this.status == null) 7814 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 7815 this.status.setValue(value); 7816 return this; 7817 } 7818 7819 /** 7820 * @return {@link #experimental} (A Boolean value to indicate that this example 7821 * scenario is authored for testing purposes (or 7822 * education/evaluation/marketing) and is not intended to be used for 7823 * genuine usage.). This is the underlying object with id, value and 7824 * extensions. The accessor "getExperimental" gives direct access to the 7825 * value 7826 */ 7827 public BooleanType getExperimentalElement() { 7828 if (this.experimental == null) 7829 if (Configuration.errorOnAutoCreate()) 7830 throw new Error("Attempt to auto-create ExampleScenario.experimental"); 7831 else if (Configuration.doAutoCreate()) 7832 this.experimental = new BooleanType(); // bb 7833 return this.experimental; 7834 } 7835 7836 public boolean hasExperimentalElement() { 7837 return this.experimental != null && !this.experimental.isEmpty(); 7838 } 7839 7840 public boolean hasExperimental() { 7841 return this.experimental != null && !this.experimental.isEmpty(); 7842 } 7843 7844 /** 7845 * @param value {@link #experimental} (A Boolean value to indicate that this 7846 * example scenario is authored for testing purposes (or 7847 * education/evaluation/marketing) and is not intended to be used 7848 * for genuine usage.). This is the underlying object with id, 7849 * value and extensions. The accessor "getExperimental" gives 7850 * direct access to the value 7851 */ 7852 public ExampleScenario setExperimentalElement(BooleanType value) { 7853 this.experimental = value; 7854 return this; 7855 } 7856 7857 /** 7858 * @return A Boolean value to indicate that this example scenario is authored 7859 * for testing purposes (or education/evaluation/marketing) and is not 7860 * intended to be used for genuine usage. 7861 */ 7862 public boolean getExperimental() { 7863 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 7864 } 7865 7866 /** 7867 * @param value A Boolean value to indicate that this example scenario is 7868 * authored for testing purposes (or 7869 * education/evaluation/marketing) and is not intended to be used 7870 * for genuine usage. 7871 */ 7872 public ExampleScenario setExperimental(boolean value) { 7873 if (this.experimental == null) 7874 this.experimental = new BooleanType(); 7875 this.experimental.setValue(value); 7876 return this; 7877 } 7878 7879 /** 7880 * @return {@link #date} (The date (and optionally time) when the example 7881 * scenario was published. The date must change when the business 7882 * version changes and it must change if the status code changes. In 7883 * addition, it should change when the substantive content of the 7884 * example scenario changes. (e.g. the 'content logical definition').). 7885 * This is the underlying object with id, value and extensions. The 7886 * accessor "getDate" gives direct access to the value 7887 */ 7888 public DateTimeType getDateElement() { 7889 if (this.date == null) 7890 if (Configuration.errorOnAutoCreate()) 7891 throw new Error("Attempt to auto-create ExampleScenario.date"); 7892 else if (Configuration.doAutoCreate()) 7893 this.date = new DateTimeType(); // bb 7894 return this.date; 7895 } 7896 7897 public boolean hasDateElement() { 7898 return this.date != null && !this.date.isEmpty(); 7899 } 7900 7901 public boolean hasDate() { 7902 return this.date != null && !this.date.isEmpty(); 7903 } 7904 7905 /** 7906 * @param value {@link #date} (The date (and optionally time) when the example 7907 * scenario was published. The date must change when the business 7908 * version changes and it must change if the status code changes. 7909 * In addition, it should change when the substantive content of 7910 * the example scenario changes. (e.g. the 'content logical 7911 * definition').). This is the underlying object with id, value and 7912 * extensions. The accessor "getDate" gives direct access to the 7913 * value 7914 */ 7915 public ExampleScenario setDateElement(DateTimeType value) { 7916 this.date = value; 7917 return this; 7918 } 7919 7920 /** 7921 * @return The date (and optionally time) when the example scenario was 7922 * published. The date must change when the business version changes and 7923 * it must change if the status code changes. In addition, it should 7924 * change when the substantive content of the example scenario changes. 7925 * (e.g. the 'content logical definition'). 7926 */ 7927 public Date getDate() { 7928 return this.date == null ? null : this.date.getValue(); 7929 } 7930 7931 /** 7932 * @param value The date (and optionally time) when the example scenario was 7933 * published. The date must change when the business version 7934 * changes and it must change if the status code changes. In 7935 * addition, it should change when the substantive content of the 7936 * example scenario changes. (e.g. the 'content logical 7937 * definition'). 7938 */ 7939 public ExampleScenario setDate(Date value) { 7940 if (value == null) 7941 this.date = null; 7942 else { 7943 if (this.date == null) 7944 this.date = new DateTimeType(); 7945 this.date.setValue(value); 7946 } 7947 return this; 7948 } 7949 7950 /** 7951 * @return {@link #publisher} (The name of the organization or individual that 7952 * published the example scenario.). This is the underlying object with 7953 * id, value and extensions. The accessor "getPublisher" gives direct 7954 * access to the value 7955 */ 7956 public StringType getPublisherElement() { 7957 if (this.publisher == null) 7958 if (Configuration.errorOnAutoCreate()) 7959 throw new Error("Attempt to auto-create ExampleScenario.publisher"); 7960 else if (Configuration.doAutoCreate()) 7961 this.publisher = new StringType(); // bb 7962 return this.publisher; 7963 } 7964 7965 public boolean hasPublisherElement() { 7966 return this.publisher != null && !this.publisher.isEmpty(); 7967 } 7968 7969 public boolean hasPublisher() { 7970 return this.publisher != null && !this.publisher.isEmpty(); 7971 } 7972 7973 /** 7974 * @param value {@link #publisher} (The name of the organization or individual 7975 * that published the example scenario.). This is the underlying 7976 * object with id, value and extensions. The accessor 7977 * "getPublisher" gives direct access to the value 7978 */ 7979 public ExampleScenario setPublisherElement(StringType value) { 7980 this.publisher = value; 7981 return this; 7982 } 7983 7984 /** 7985 * @return The name of the organization or individual that published the example 7986 * scenario. 7987 */ 7988 public String getPublisher() { 7989 return this.publisher == null ? null : this.publisher.getValue(); 7990 } 7991 7992 /** 7993 * @param value The name of the organization or individual that published the 7994 * example scenario. 7995 */ 7996 public ExampleScenario setPublisher(String value) { 7997 if (Utilities.noString(value)) 7998 this.publisher = null; 7999 else { 8000 if (this.publisher == null) 8001 this.publisher = new StringType(); 8002 this.publisher.setValue(value); 8003 } 8004 return this; 8005 } 8006 8007 /** 8008 * @return {@link #contact} (Contact details to assist a user in finding and 8009 * communicating with the publisher.) 8010 */ 8011 public List<ContactDetail> getContact() { 8012 if (this.contact == null) 8013 this.contact = new ArrayList<ContactDetail>(); 8014 return this.contact; 8015 } 8016 8017 /** 8018 * @return Returns a reference to <code>this</code> for easy method chaining 8019 */ 8020 public ExampleScenario setContact(List<ContactDetail> theContact) { 8021 this.contact = theContact; 8022 return this; 8023 } 8024 8025 public boolean hasContact() { 8026 if (this.contact == null) 8027 return false; 8028 for (ContactDetail item : this.contact) 8029 if (!item.isEmpty()) 8030 return true; 8031 return false; 8032 } 8033 8034 public ContactDetail addContact() { // 3 8035 ContactDetail t = new ContactDetail(); 8036 if (this.contact == null) 8037 this.contact = new ArrayList<ContactDetail>(); 8038 this.contact.add(t); 8039 return t; 8040 } 8041 8042 public ExampleScenario addContact(ContactDetail t) { // 3 8043 if (t == null) 8044 return this; 8045 if (this.contact == null) 8046 this.contact = new ArrayList<ContactDetail>(); 8047 this.contact.add(t); 8048 return this; 8049 } 8050 8051 /** 8052 * @return The first repetition of repeating field {@link #contact}, creating it 8053 * if it does not already exist 8054 */ 8055 public ContactDetail getContactFirstRep() { 8056 if (getContact().isEmpty()) { 8057 addContact(); 8058 } 8059 return getContact().get(0); 8060 } 8061 8062 /** 8063 * @return {@link #useContext} (The content was developed with a focus and 8064 * intent of supporting the contexts that are listed. These contexts may 8065 * be general categories (gender, age, ...) or may be references to 8066 * specific programs (insurance plans, studies, ...) and may be used to 8067 * assist with indexing and searching for appropriate example scenario 8068 * instances.) 8069 */ 8070 public List<UsageContext> getUseContext() { 8071 if (this.useContext == null) 8072 this.useContext = new ArrayList<UsageContext>(); 8073 return this.useContext; 8074 } 8075 8076 /** 8077 * @return Returns a reference to <code>this</code> for easy method chaining 8078 */ 8079 public ExampleScenario setUseContext(List<UsageContext> theUseContext) { 8080 this.useContext = theUseContext; 8081 return this; 8082 } 8083 8084 public boolean hasUseContext() { 8085 if (this.useContext == null) 8086 return false; 8087 for (UsageContext item : this.useContext) 8088 if (!item.isEmpty()) 8089 return true; 8090 return false; 8091 } 8092 8093 public UsageContext addUseContext() { // 3 8094 UsageContext t = new UsageContext(); 8095 if (this.useContext == null) 8096 this.useContext = new ArrayList<UsageContext>(); 8097 this.useContext.add(t); 8098 return t; 8099 } 8100 8101 public ExampleScenario addUseContext(UsageContext t) { // 3 8102 if (t == null) 8103 return this; 8104 if (this.useContext == null) 8105 this.useContext = new ArrayList<UsageContext>(); 8106 this.useContext.add(t); 8107 return this; 8108 } 8109 8110 /** 8111 * @return The first repetition of repeating field {@link #useContext}, creating 8112 * it if it does not already exist 8113 */ 8114 public UsageContext getUseContextFirstRep() { 8115 if (getUseContext().isEmpty()) { 8116 addUseContext(); 8117 } 8118 return getUseContext().get(0); 8119 } 8120 8121 /** 8122 * @return {@link #jurisdiction} (A legal or geographic region in which the 8123 * example scenario is intended to be used.) 8124 */ 8125 public List<CodeableConcept> getJurisdiction() { 8126 if (this.jurisdiction == null) 8127 this.jurisdiction = new ArrayList<CodeableConcept>(); 8128 return this.jurisdiction; 8129 } 8130 8131 /** 8132 * @return Returns a reference to <code>this</code> for easy method chaining 8133 */ 8134 public ExampleScenario setJurisdiction(List<CodeableConcept> theJurisdiction) { 8135 this.jurisdiction = theJurisdiction; 8136 return this; 8137 } 8138 8139 public boolean hasJurisdiction() { 8140 if (this.jurisdiction == null) 8141 return false; 8142 for (CodeableConcept item : this.jurisdiction) 8143 if (!item.isEmpty()) 8144 return true; 8145 return false; 8146 } 8147 8148 public CodeableConcept addJurisdiction() { // 3 8149 CodeableConcept t = new CodeableConcept(); 8150 if (this.jurisdiction == null) 8151 this.jurisdiction = new ArrayList<CodeableConcept>(); 8152 this.jurisdiction.add(t); 8153 return t; 8154 } 8155 8156 public ExampleScenario addJurisdiction(CodeableConcept t) { // 3 8157 if (t == null) 8158 return this; 8159 if (this.jurisdiction == null) 8160 this.jurisdiction = new ArrayList<CodeableConcept>(); 8161 this.jurisdiction.add(t); 8162 return this; 8163 } 8164 8165 /** 8166 * @return The first repetition of repeating field {@link #jurisdiction}, 8167 * creating it if it does not already exist 8168 */ 8169 public CodeableConcept getJurisdictionFirstRep() { 8170 if (getJurisdiction().isEmpty()) { 8171 addJurisdiction(); 8172 } 8173 return getJurisdiction().get(0); 8174 } 8175 8176 /** 8177 * @return {@link #copyright} (A copyright statement relating to the example 8178 * scenario and/or its contents. Copyright statements are generally 8179 * legal restrictions on the use and publishing of the example 8180 * scenario.). This is the underlying object with id, value and 8181 * extensions. The accessor "getCopyright" gives direct access to the 8182 * value 8183 */ 8184 public MarkdownType getCopyrightElement() { 8185 if (this.copyright == null) 8186 if (Configuration.errorOnAutoCreate()) 8187 throw new Error("Attempt to auto-create ExampleScenario.copyright"); 8188 else if (Configuration.doAutoCreate()) 8189 this.copyright = new MarkdownType(); // bb 8190 return this.copyright; 8191 } 8192 8193 public boolean hasCopyrightElement() { 8194 return this.copyright != null && !this.copyright.isEmpty(); 8195 } 8196 8197 public boolean hasCopyright() { 8198 return this.copyright != null && !this.copyright.isEmpty(); 8199 } 8200 8201 /** 8202 * @param value {@link #copyright} (A copyright statement relating to the 8203 * example scenario and/or its contents. Copyright statements are 8204 * generally legal restrictions on the use and publishing of the 8205 * example scenario.). This is the underlying object with id, value 8206 * and extensions. The accessor "getCopyright" gives direct access 8207 * to the value 8208 */ 8209 public ExampleScenario setCopyrightElement(MarkdownType value) { 8210 this.copyright = value; 8211 return this; 8212 } 8213 8214 /** 8215 * @return A copyright statement relating to the example scenario and/or its 8216 * contents. Copyright statements are generally legal restrictions on 8217 * the use and publishing of the example scenario. 8218 */ 8219 public String getCopyright() { 8220 return this.copyright == null ? null : this.copyright.getValue(); 8221 } 8222 8223 /** 8224 * @param value A copyright statement relating to the example scenario and/or 8225 * its contents. Copyright statements are generally legal 8226 * restrictions on the use and publishing of the example scenario. 8227 */ 8228 public ExampleScenario setCopyright(String value) { 8229 if (value == null) 8230 this.copyright = null; 8231 else { 8232 if (this.copyright == null) 8233 this.copyright = new MarkdownType(); 8234 this.copyright.setValue(value); 8235 } 8236 return this; 8237 } 8238 8239 /** 8240 * @return {@link #purpose} (What the example scenario resource is created for. 8241 * This should not be used to show the business purpose of the scenario 8242 * itself, but the purpose of documenting a scenario.). This is the 8243 * underlying object with id, value and extensions. The accessor 8244 * "getPurpose" gives direct access to the value 8245 */ 8246 public MarkdownType getPurposeElement() { 8247 if (this.purpose == null) 8248 if (Configuration.errorOnAutoCreate()) 8249 throw new Error("Attempt to auto-create ExampleScenario.purpose"); 8250 else if (Configuration.doAutoCreate()) 8251 this.purpose = new MarkdownType(); // bb 8252 return this.purpose; 8253 } 8254 8255 public boolean hasPurposeElement() { 8256 return this.purpose != null && !this.purpose.isEmpty(); 8257 } 8258 8259 public boolean hasPurpose() { 8260 return this.purpose != null && !this.purpose.isEmpty(); 8261 } 8262 8263 /** 8264 * @param value {@link #purpose} (What the example scenario resource is created 8265 * for. This should not be used to show the business purpose of the 8266 * scenario itself, but the purpose of documenting a scenario.). 8267 * This is the underlying object with id, value and extensions. The 8268 * accessor "getPurpose" gives direct access to the value 8269 */ 8270 public ExampleScenario setPurposeElement(MarkdownType value) { 8271 this.purpose = value; 8272 return this; 8273 } 8274 8275 /** 8276 * @return What the example scenario resource is created for. This should not be 8277 * used to show the business purpose of the scenario itself, but the 8278 * purpose of documenting a scenario. 8279 */ 8280 public String getPurpose() { 8281 return this.purpose == null ? null : this.purpose.getValue(); 8282 } 8283 8284 /** 8285 * @param value What the example scenario resource is created for. This should 8286 * not be used to show the business purpose of the scenario itself, 8287 * but the purpose of documenting a scenario. 8288 */ 8289 public ExampleScenario setPurpose(String value) { 8290 if (value == null) 8291 this.purpose = null; 8292 else { 8293 if (this.purpose == null) 8294 this.purpose = new MarkdownType(); 8295 this.purpose.setValue(value); 8296 } 8297 return this; 8298 } 8299 8300 /** 8301 * @return {@link #actor} (Actor participating in the resource.) 8302 */ 8303 public List<ExampleScenarioActorComponent> getActor() { 8304 if (this.actor == null) 8305 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8306 return this.actor; 8307 } 8308 8309 /** 8310 * @return Returns a reference to <code>this</code> for easy method chaining 8311 */ 8312 public ExampleScenario setActor(List<ExampleScenarioActorComponent> theActor) { 8313 this.actor = theActor; 8314 return this; 8315 } 8316 8317 public boolean hasActor() { 8318 if (this.actor == null) 8319 return false; 8320 for (ExampleScenarioActorComponent item : this.actor) 8321 if (!item.isEmpty()) 8322 return true; 8323 return false; 8324 } 8325 8326 public ExampleScenarioActorComponent addActor() { // 3 8327 ExampleScenarioActorComponent t = new ExampleScenarioActorComponent(); 8328 if (this.actor == null) 8329 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8330 this.actor.add(t); 8331 return t; 8332 } 8333 8334 public ExampleScenario addActor(ExampleScenarioActorComponent t) { // 3 8335 if (t == null) 8336 return this; 8337 if (this.actor == null) 8338 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 8339 this.actor.add(t); 8340 return this; 8341 } 8342 8343 /** 8344 * @return The first repetition of repeating field {@link #actor}, creating it 8345 * if it does not already exist 8346 */ 8347 public ExampleScenarioActorComponent getActorFirstRep() { 8348 if (getActor().isEmpty()) { 8349 addActor(); 8350 } 8351 return getActor().get(0); 8352 } 8353 8354 /** 8355 * @return {@link #instance} (Each resource and each version that is present in 8356 * the workflow.) 8357 */ 8358 public List<ExampleScenarioInstanceComponent> getInstance() { 8359 if (this.instance == null) 8360 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8361 return this.instance; 8362 } 8363 8364 /** 8365 * @return Returns a reference to <code>this</code> for easy method chaining 8366 */ 8367 public ExampleScenario setInstance(List<ExampleScenarioInstanceComponent> theInstance) { 8368 this.instance = theInstance; 8369 return this; 8370 } 8371 8372 public boolean hasInstance() { 8373 if (this.instance == null) 8374 return false; 8375 for (ExampleScenarioInstanceComponent item : this.instance) 8376 if (!item.isEmpty()) 8377 return true; 8378 return false; 8379 } 8380 8381 public ExampleScenarioInstanceComponent addInstance() { // 3 8382 ExampleScenarioInstanceComponent t = new ExampleScenarioInstanceComponent(); 8383 if (this.instance == null) 8384 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8385 this.instance.add(t); 8386 return t; 8387 } 8388 8389 public ExampleScenario addInstance(ExampleScenarioInstanceComponent t) { // 3 8390 if (t == null) 8391 return this; 8392 if (this.instance == null) 8393 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 8394 this.instance.add(t); 8395 return this; 8396 } 8397 8398 /** 8399 * @return The first repetition of repeating field {@link #instance}, creating 8400 * it if it does not already exist 8401 */ 8402 public ExampleScenarioInstanceComponent getInstanceFirstRep() { 8403 if (getInstance().isEmpty()) { 8404 addInstance(); 8405 } 8406 return getInstance().get(0); 8407 } 8408 8409 /** 8410 * @return {@link #process} (Each major process - a group of operations.) 8411 */ 8412 public List<ExampleScenarioProcessComponent> getProcess() { 8413 if (this.process == null) 8414 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8415 return this.process; 8416 } 8417 8418 /** 8419 * @return Returns a reference to <code>this</code> for easy method chaining 8420 */ 8421 public ExampleScenario setProcess(List<ExampleScenarioProcessComponent> theProcess) { 8422 this.process = theProcess; 8423 return this; 8424 } 8425 8426 public boolean hasProcess() { 8427 if (this.process == null) 8428 return false; 8429 for (ExampleScenarioProcessComponent item : this.process) 8430 if (!item.isEmpty()) 8431 return true; 8432 return false; 8433 } 8434 8435 public ExampleScenarioProcessComponent addProcess() { // 3 8436 ExampleScenarioProcessComponent t = new ExampleScenarioProcessComponent(); 8437 if (this.process == null) 8438 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8439 this.process.add(t); 8440 return t; 8441 } 8442 8443 public ExampleScenario addProcess(ExampleScenarioProcessComponent t) { // 3 8444 if (t == null) 8445 return this; 8446 if (this.process == null) 8447 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 8448 this.process.add(t); 8449 return this; 8450 } 8451 8452 /** 8453 * @return The first repetition of repeating field {@link #process}, creating it 8454 * if it does not already exist 8455 */ 8456 public ExampleScenarioProcessComponent getProcessFirstRep() { 8457 if (getProcess().isEmpty()) { 8458 addProcess(); 8459 } 8460 return getProcess().get(0); 8461 } 8462 8463 /** 8464 * @return {@link #workflow} (Another nested workflow.) 8465 */ 8466 public List<CanonicalType> getWorkflow() { 8467 if (this.workflow == null) 8468 this.workflow = new ArrayList<CanonicalType>(); 8469 return this.workflow; 8470 } 8471 8472 /** 8473 * @return Returns a reference to <code>this</code> for easy method chaining 8474 */ 8475 public ExampleScenario setWorkflow(List<CanonicalType> theWorkflow) { 8476 this.workflow = theWorkflow; 8477 return this; 8478 } 8479 8480 public boolean hasWorkflow() { 8481 if (this.workflow == null) 8482 return false; 8483 for (CanonicalType item : this.workflow) 8484 if (!item.isEmpty()) 8485 return true; 8486 return false; 8487 } 8488 8489 /** 8490 * @return {@link #workflow} (Another nested workflow.) 8491 */ 8492 public CanonicalType addWorkflowElement() {// 2 8493 CanonicalType t = new CanonicalType(); 8494 if (this.workflow == null) 8495 this.workflow = new ArrayList<CanonicalType>(); 8496 this.workflow.add(t); 8497 return t; 8498 } 8499 8500 /** 8501 * @param value {@link #workflow} (Another nested workflow.) 8502 */ 8503 public ExampleScenario addWorkflow(String value) { // 1 8504 CanonicalType t = new CanonicalType(); 8505 t.setValue(value); 8506 if (this.workflow == null) 8507 this.workflow = new ArrayList<CanonicalType>(); 8508 this.workflow.add(t); 8509 return this; 8510 } 8511 8512 /** 8513 * @param value {@link #workflow} (Another nested workflow.) 8514 */ 8515 public boolean hasWorkflow(String value) { 8516 if (this.workflow == null) 8517 return false; 8518 for (CanonicalType v : this.workflow) 8519 if (v.getValue().equals(value)) // canonical(ExampleScenario) 8520 return true; 8521 return false; 8522 } 8523 8524 protected void listChildren(List<Property> children) { 8525 super.listChildren(children); 8526 children.add(new Property("url", "uri", 8527 "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 8528 0, 1, url)); 8529 children.add(new Property("identifier", "Identifier", 8530 "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8531 0, java.lang.Integer.MAX_VALUE, identifier)); 8532 children.add(new Property("version", "string", 8533 "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 8534 0, 1, version)); 8535 children.add(new Property("name", "string", 8536 "A natural language name identifying the example scenario. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8537 0, 1, name)); 8538 children.add(new Property("status", "code", 8539 "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status)); 8540 children.add(new Property("experimental", "boolean", 8541 "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8542 0, 1, experimental)); 8543 children.add(new Property("date", "dateTime", 8544 "The date (and optionally time) when the example scenario was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 8545 0, 1, date)); 8546 children.add(new Property("publisher", "string", 8547 "The name of the organization or individual that published the example scenario.", 0, 1, publisher)); 8548 children.add(new Property("contact", "ContactDetail", 8549 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8550 java.lang.Integer.MAX_VALUE, contact)); 8551 children.add(new Property("useContext", "UsageContext", 8552 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 8553 0, java.lang.Integer.MAX_VALUE, useContext)); 8554 children.add(new Property("jurisdiction", "CodeableConcept", 8555 "A legal or geographic region in which the example scenario is intended to be used.", 0, 8556 java.lang.Integer.MAX_VALUE, jurisdiction)); 8557 children.add(new Property("copyright", "markdown", 8558 "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 8559 0, 1, copyright)); 8560 children.add(new Property("purpose", "markdown", 8561 "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 8562 0, 1, purpose)); 8563 children 8564 .add(new Property("actor", "", "Actor participating in the resource.", 0, java.lang.Integer.MAX_VALUE, actor)); 8565 children.add(new Property("instance", "", "Each resource and each version that is present in the workflow.", 0, 8566 java.lang.Integer.MAX_VALUE, instance)); 8567 children.add(new Property("process", "", "Each major process - a group of operations.", 0, 8568 java.lang.Integer.MAX_VALUE, process)); 8569 children.add(new Property("workflow", "canonical(ExampleScenario)", "Another nested workflow.", 0, 8570 java.lang.Integer.MAX_VALUE, workflow)); 8571 } 8572 8573 @Override 8574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8575 switch (_hash) { 8576 case 116079: 8577 /* url */ return new Property("url", "uri", 8578 "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 8579 0, 1, url); 8580 case -1618432855: 8581 /* identifier */ return new Property("identifier", "Identifier", 8582 "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 8583 0, java.lang.Integer.MAX_VALUE, identifier); 8584 case 351608024: 8585 /* version */ return new Property("version", "string", 8586 "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 8587 0, 1, version); 8588 case 3373707: 8589 /* name */ return new Property("name", "string", 8590 "A natural language name identifying the example scenario. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 8591 0, 1, name); 8592 case -892481550: 8593 /* status */ return new Property("status", "code", 8594 "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status); 8595 case -404562712: 8596 /* experimental */ return new Property("experimental", "boolean", 8597 "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 8598 0, 1, experimental); 8599 case 3076014: 8600 /* date */ return new Property("date", "dateTime", 8601 "The date (and optionally time) when the example scenario was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 8602 0, 1, date); 8603 case 1447404028: 8604 /* publisher */ return new Property("publisher", "string", 8605 "The name of the organization or individual that published the example scenario.", 0, 1, publisher); 8606 case 951526432: 8607 /* contact */ return new Property("contact", "ContactDetail", 8608 "Contact details to assist a user in finding and communicating with the publisher.", 0, 8609 java.lang.Integer.MAX_VALUE, contact); 8610 case -669707736: 8611 /* useContext */ return new Property("useContext", "UsageContext", 8612 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 8613 0, java.lang.Integer.MAX_VALUE, useContext); 8614 case -507075711: 8615 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 8616 "A legal or geographic region in which the example scenario is intended to be used.", 0, 8617 java.lang.Integer.MAX_VALUE, jurisdiction); 8618 case 1522889671: 8619 /* copyright */ return new Property("copyright", "markdown", 8620 "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 8621 0, 1, copyright); 8622 case -220463842: 8623 /* purpose */ return new Property("purpose", "markdown", 8624 "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 8625 0, 1, purpose); 8626 case 92645877: 8627 /* actor */ return new Property("actor", "", "Actor participating in the resource.", 0, 8628 java.lang.Integer.MAX_VALUE, actor); 8629 case 555127957: 8630 /* instance */ return new Property("instance", "", 8631 "Each resource and each version that is present in the workflow.", 0, java.lang.Integer.MAX_VALUE, instance); 8632 case -309518737: 8633 /* process */ return new Property("process", "", "Each major process - a group of operations.", 0, 8634 java.lang.Integer.MAX_VALUE, process); 8635 case 35379135: 8636 /* workflow */ return new Property("workflow", "canonical(ExampleScenario)", "Another nested workflow.", 0, 8637 java.lang.Integer.MAX_VALUE, workflow); 8638 default: 8639 return super.getNamedProperty(_hash, _name, _checkValid); 8640 } 8641 8642 } 8643 8644 @Override 8645 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8646 switch (hash) { 8647 case 116079: 8648 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 8649 case -1618432855: 8650 /* identifier */ return this.identifier == null ? new Base[0] 8651 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 8652 case 351608024: 8653 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 8654 case 3373707: 8655 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 8656 case -892481550: 8657 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 8658 case -404562712: 8659 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 8660 case 3076014: 8661 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 8662 case 1447404028: 8663 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 8664 case 951526432: 8665 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 8666 case -669707736: 8667 /* useContext */ return this.useContext == null ? new Base[0] 8668 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 8669 case -507075711: 8670 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 8671 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 8672 case 1522889671: 8673 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 8674 case -220463842: 8675 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 8676 case 92645877: 8677 /* actor */ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ExampleScenarioActorComponent 8678 case 555127957: 8679 /* instance */ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ExampleScenarioInstanceComponent 8680 case -309518737: 8681 /* process */ return this.process == null ? new Base[0] : this.process.toArray(new Base[this.process.size()]); // ExampleScenarioProcessComponent 8682 case 35379135: 8683 /* workflow */ return this.workflow == null ? new Base[0] : this.workflow.toArray(new Base[this.workflow.size()]); // CanonicalType 8684 default: 8685 return super.getProperty(hash, name, checkValid); 8686 } 8687 8688 } 8689 8690 @Override 8691 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8692 switch (hash) { 8693 case 116079: // url 8694 this.url = castToUri(value); // UriType 8695 return value; 8696 case -1618432855: // identifier 8697 this.getIdentifier().add(castToIdentifier(value)); // Identifier 8698 return value; 8699 case 351608024: // version 8700 this.version = castToString(value); // StringType 8701 return value; 8702 case 3373707: // name 8703 this.name = castToString(value); // StringType 8704 return value; 8705 case -892481550: // status 8706 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8707 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8708 return value; 8709 case -404562712: // experimental 8710 this.experimental = castToBoolean(value); // BooleanType 8711 return value; 8712 case 3076014: // date 8713 this.date = castToDateTime(value); // DateTimeType 8714 return value; 8715 case 1447404028: // publisher 8716 this.publisher = castToString(value); // StringType 8717 return value; 8718 case 951526432: // contact 8719 this.getContact().add(castToContactDetail(value)); // ContactDetail 8720 return value; 8721 case -669707736: // useContext 8722 this.getUseContext().add(castToUsageContext(value)); // UsageContext 8723 return value; 8724 case -507075711: // jurisdiction 8725 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 8726 return value; 8727 case 1522889671: // copyright 8728 this.copyright = castToMarkdown(value); // MarkdownType 8729 return value; 8730 case -220463842: // purpose 8731 this.purpose = castToMarkdown(value); // MarkdownType 8732 return value; 8733 case 92645877: // actor 8734 this.getActor().add((ExampleScenarioActorComponent) value); // ExampleScenarioActorComponent 8735 return value; 8736 case 555127957: // instance 8737 this.getInstance().add((ExampleScenarioInstanceComponent) value); // ExampleScenarioInstanceComponent 8738 return value; 8739 case -309518737: // process 8740 this.getProcess().add((ExampleScenarioProcessComponent) value); // ExampleScenarioProcessComponent 8741 return value; 8742 case 35379135: // workflow 8743 this.getWorkflow().add(castToCanonical(value)); // CanonicalType 8744 return value; 8745 default: 8746 return super.setProperty(hash, name, value); 8747 } 8748 8749 } 8750 8751 @Override 8752 public Base setProperty(String name, Base value) throws FHIRException { 8753 if (name.equals("url")) { 8754 this.url = castToUri(value); // UriType 8755 } else if (name.equals("identifier")) { 8756 this.getIdentifier().add(castToIdentifier(value)); 8757 } else if (name.equals("version")) { 8758 this.version = castToString(value); // StringType 8759 } else if (name.equals("name")) { 8760 this.name = castToString(value); // StringType 8761 } else if (name.equals("status")) { 8762 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 8763 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 8764 } else if (name.equals("experimental")) { 8765 this.experimental = castToBoolean(value); // BooleanType 8766 } else if (name.equals("date")) { 8767 this.date = castToDateTime(value); // DateTimeType 8768 } else if (name.equals("publisher")) { 8769 this.publisher = castToString(value); // StringType 8770 } else if (name.equals("contact")) { 8771 this.getContact().add(castToContactDetail(value)); 8772 } else if (name.equals("useContext")) { 8773 this.getUseContext().add(castToUsageContext(value)); 8774 } else if (name.equals("jurisdiction")) { 8775 this.getJurisdiction().add(castToCodeableConcept(value)); 8776 } else if (name.equals("copyright")) { 8777 this.copyright = castToMarkdown(value); // MarkdownType 8778 } else if (name.equals("purpose")) { 8779 this.purpose = castToMarkdown(value); // MarkdownType 8780 } else if (name.equals("actor")) { 8781 this.getActor().add((ExampleScenarioActorComponent) value); 8782 } else if (name.equals("instance")) { 8783 this.getInstance().add((ExampleScenarioInstanceComponent) value); 8784 } else if (name.equals("process")) { 8785 this.getProcess().add((ExampleScenarioProcessComponent) value); 8786 } else if (name.equals("workflow")) { 8787 this.getWorkflow().add(castToCanonical(value)); 8788 } else 8789 return super.setProperty(name, value); 8790 return value; 8791 } 8792 8793 @Override 8794 public void removeChild(String name, Base value) throws FHIRException { 8795 if (name.equals("url")) { 8796 this.url = null; 8797 } else if (name.equals("identifier")) { 8798 this.getIdentifier().remove(castToIdentifier(value)); 8799 } else if (name.equals("version")) { 8800 this.version = null; 8801 } else if (name.equals("name")) { 8802 this.name = null; 8803 } else if (name.equals("status")) { 8804 this.status = null; 8805 } else if (name.equals("experimental")) { 8806 this.experimental = null; 8807 } else if (name.equals("date")) { 8808 this.date = null; 8809 } else if (name.equals("publisher")) { 8810 this.publisher = null; 8811 } else if (name.equals("contact")) { 8812 this.getContact().remove(castToContactDetail(value)); 8813 } else if (name.equals("useContext")) { 8814 this.getUseContext().remove(castToUsageContext(value)); 8815 } else if (name.equals("jurisdiction")) { 8816 this.getJurisdiction().remove(castToCodeableConcept(value)); 8817 } else if (name.equals("copyright")) { 8818 this.copyright = null; 8819 } else if (name.equals("purpose")) { 8820 this.purpose = null; 8821 } else if (name.equals("actor")) { 8822 this.getActor().remove((ExampleScenarioActorComponent) value); 8823 } else if (name.equals("instance")) { 8824 this.getInstance().remove((ExampleScenarioInstanceComponent) value); 8825 } else if (name.equals("process")) { 8826 this.getProcess().remove((ExampleScenarioProcessComponent) value); 8827 } else if (name.equals("workflow")) { 8828 this.getWorkflow().remove(castToCanonical(value)); 8829 } else 8830 super.removeChild(name, value); 8831 8832 } 8833 8834 @Override 8835 public Base makeProperty(int hash, String name) throws FHIRException { 8836 switch (hash) { 8837 case 116079: 8838 return getUrlElement(); 8839 case -1618432855: 8840 return addIdentifier(); 8841 case 351608024: 8842 return getVersionElement(); 8843 case 3373707: 8844 return getNameElement(); 8845 case -892481550: 8846 return getStatusElement(); 8847 case -404562712: 8848 return getExperimentalElement(); 8849 case 3076014: 8850 return getDateElement(); 8851 case 1447404028: 8852 return getPublisherElement(); 8853 case 951526432: 8854 return addContact(); 8855 case -669707736: 8856 return addUseContext(); 8857 case -507075711: 8858 return addJurisdiction(); 8859 case 1522889671: 8860 return getCopyrightElement(); 8861 case -220463842: 8862 return getPurposeElement(); 8863 case 92645877: 8864 return addActor(); 8865 case 555127957: 8866 return addInstance(); 8867 case -309518737: 8868 return addProcess(); 8869 case 35379135: 8870 return addWorkflowElement(); 8871 default: 8872 return super.makeProperty(hash, name); 8873 } 8874 8875 } 8876 8877 @Override 8878 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8879 switch (hash) { 8880 case 116079: 8881 /* url */ return new String[] { "uri" }; 8882 case -1618432855: 8883 /* identifier */ return new String[] { "Identifier" }; 8884 case 351608024: 8885 /* version */ return new String[] { "string" }; 8886 case 3373707: 8887 /* name */ return new String[] { "string" }; 8888 case -892481550: 8889 /* status */ return new String[] { "code" }; 8890 case -404562712: 8891 /* experimental */ return new String[] { "boolean" }; 8892 case 3076014: 8893 /* date */ return new String[] { "dateTime" }; 8894 case 1447404028: 8895 /* publisher */ return new String[] { "string" }; 8896 case 951526432: 8897 /* contact */ return new String[] { "ContactDetail" }; 8898 case -669707736: 8899 /* useContext */ return new String[] { "UsageContext" }; 8900 case -507075711: 8901 /* jurisdiction */ return new String[] { "CodeableConcept" }; 8902 case 1522889671: 8903 /* copyright */ return new String[] { "markdown" }; 8904 case -220463842: 8905 /* purpose */ return new String[] { "markdown" }; 8906 case 92645877: 8907 /* actor */ return new String[] {}; 8908 case 555127957: 8909 /* instance */ return new String[] {}; 8910 case -309518737: 8911 /* process */ return new String[] {}; 8912 case 35379135: 8913 /* workflow */ return new String[] { "canonical" }; 8914 default: 8915 return super.getTypesForProperty(hash, name); 8916 } 8917 8918 } 8919 8920 @Override 8921 public Base addChild(String name) throws FHIRException { 8922 if (name.equals("url")) { 8923 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.url"); 8924 } else if (name.equals("identifier")) { 8925 return addIdentifier(); 8926 } else if (name.equals("version")) { 8927 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.version"); 8928 } else if (name.equals("name")) { 8929 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 8930 } else if (name.equals("status")) { 8931 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.status"); 8932 } else if (name.equals("experimental")) { 8933 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.experimental"); 8934 } else if (name.equals("date")) { 8935 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.date"); 8936 } else if (name.equals("publisher")) { 8937 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.publisher"); 8938 } else if (name.equals("contact")) { 8939 return addContact(); 8940 } else if (name.equals("useContext")) { 8941 return addUseContext(); 8942 } else if (name.equals("jurisdiction")) { 8943 return addJurisdiction(); 8944 } else if (name.equals("copyright")) { 8945 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.copyright"); 8946 } else if (name.equals("purpose")) { 8947 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.purpose"); 8948 } else if (name.equals("actor")) { 8949 return addActor(); 8950 } else if (name.equals("instance")) { 8951 return addInstance(); 8952 } else if (name.equals("process")) { 8953 return addProcess(); 8954 } else if (name.equals("workflow")) { 8955 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.workflow"); 8956 } else 8957 return super.addChild(name); 8958 } 8959 8960 public String fhirType() { 8961 return "ExampleScenario"; 8962 8963 } 8964 8965 public ExampleScenario copy() { 8966 ExampleScenario dst = new ExampleScenario(); 8967 copyValues(dst); 8968 return dst; 8969 } 8970 8971 public void copyValues(ExampleScenario dst) { 8972 super.copyValues(dst); 8973 dst.url = url == null ? null : url.copy(); 8974 if (identifier != null) { 8975 dst.identifier = new ArrayList<Identifier>(); 8976 for (Identifier i : identifier) 8977 dst.identifier.add(i.copy()); 8978 } 8979 ; 8980 dst.version = version == null ? null : version.copy(); 8981 dst.name = name == null ? null : name.copy(); 8982 dst.status = status == null ? null : status.copy(); 8983 dst.experimental = experimental == null ? null : experimental.copy(); 8984 dst.date = date == null ? null : date.copy(); 8985 dst.publisher = publisher == null ? null : publisher.copy(); 8986 if (contact != null) { 8987 dst.contact = new ArrayList<ContactDetail>(); 8988 for (ContactDetail i : contact) 8989 dst.contact.add(i.copy()); 8990 } 8991 ; 8992 if (useContext != null) { 8993 dst.useContext = new ArrayList<UsageContext>(); 8994 for (UsageContext i : useContext) 8995 dst.useContext.add(i.copy()); 8996 } 8997 ; 8998 if (jurisdiction != null) { 8999 dst.jurisdiction = new ArrayList<CodeableConcept>(); 9000 for (CodeableConcept i : jurisdiction) 9001 dst.jurisdiction.add(i.copy()); 9002 } 9003 ; 9004 dst.copyright = copyright == null ? null : copyright.copy(); 9005 dst.purpose = purpose == null ? null : purpose.copy(); 9006 if (actor != null) { 9007 dst.actor = new ArrayList<ExampleScenarioActorComponent>(); 9008 for (ExampleScenarioActorComponent i : actor) 9009 dst.actor.add(i.copy()); 9010 } 9011 ; 9012 if (instance != null) { 9013 dst.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 9014 for (ExampleScenarioInstanceComponent i : instance) 9015 dst.instance.add(i.copy()); 9016 } 9017 ; 9018 if (process != null) { 9019 dst.process = new ArrayList<ExampleScenarioProcessComponent>(); 9020 for (ExampleScenarioProcessComponent i : process) 9021 dst.process.add(i.copy()); 9022 } 9023 ; 9024 if (workflow != null) { 9025 dst.workflow = new ArrayList<CanonicalType>(); 9026 for (CanonicalType i : workflow) 9027 dst.workflow.add(i.copy()); 9028 } 9029 ; 9030 } 9031 9032 protected ExampleScenario typedCopy() { 9033 return copy(); 9034 } 9035 9036 @Override 9037 public boolean equalsDeep(Base other_) { 9038 if (!super.equalsDeep(other_)) 9039 return false; 9040 if (!(other_ instanceof ExampleScenario)) 9041 return false; 9042 ExampleScenario o = (ExampleScenario) other_; 9043 return compareDeep(identifier, o.identifier, true) && compareDeep(copyright, o.copyright, true) 9044 && compareDeep(purpose, o.purpose, true) && compareDeep(actor, o.actor, true) 9045 && compareDeep(instance, o.instance, true) && compareDeep(process, o.process, true) 9046 && compareDeep(workflow, o.workflow, true); 9047 } 9048 9049 @Override 9050 public boolean equalsShallow(Base other_) { 9051 if (!super.equalsShallow(other_)) 9052 return false; 9053 if (!(other_ instanceof ExampleScenario)) 9054 return false; 9055 ExampleScenario o = (ExampleScenario) other_; 9056 return compareValues(copyright, o.copyright, true) && compareValues(purpose, o.purpose, true); 9057 } 9058 9059 public boolean isEmpty() { 9060 return super.isEmpty() 9061 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, copyright, purpose, actor, instance, process, workflow); 9062 } 9063 9064 @Override 9065 public ResourceType getResourceType() { 9066 return ResourceType.ExampleScenario; 9067 } 9068 9069 /** 9070 * Search parameter: <b>date</b> 9071 * <p> 9072 * Description: <b>The example scenario publication date</b><br> 9073 * Type: <b>date</b><br> 9074 * Path: <b>ExampleScenario.date</b><br> 9075 * </p> 9076 */ 9077 @SearchParamDefinition(name = "date", path = "ExampleScenario.date", description = "The example scenario publication date", type = "date") 9078 public static final String SP_DATE = "date"; 9079 /** 9080 * <b>Fluent Client</b> search parameter constant for <b>date</b> 9081 * <p> 9082 * Description: <b>The example scenario publication date</b><br> 9083 * Type: <b>date</b><br> 9084 * Path: <b>ExampleScenario.date</b><br> 9085 * </p> 9086 */ 9087 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 9088 SP_DATE); 9089 9090 /** 9091 * Search parameter: <b>identifier</b> 9092 * <p> 9093 * Description: <b>External identifier for the example scenario</b><br> 9094 * Type: <b>token</b><br> 9095 * Path: <b>ExampleScenario.identifier</b><br> 9096 * </p> 9097 */ 9098 @SearchParamDefinition(name = "identifier", path = "ExampleScenario.identifier", description = "External identifier for the example scenario", type = "token") 9099 public static final String SP_IDENTIFIER = "identifier"; 9100 /** 9101 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 9102 * <p> 9103 * Description: <b>External identifier for the example scenario</b><br> 9104 * Type: <b>token</b><br> 9105 * Path: <b>ExampleScenario.identifier</b><br> 9106 * </p> 9107 */ 9108 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9109 SP_IDENTIFIER); 9110 9111 /** 9112 * Search parameter: <b>context-type-value</b> 9113 * <p> 9114 * Description: <b>A use context type and value assigned to the example 9115 * scenario</b><br> 9116 * Type: <b>composite</b><br> 9117 * Path: <b></b><br> 9118 * </p> 9119 */ 9120 @SearchParamDefinition(name = "context-type-value", path = "ExampleScenario.useContext", description = "A use context type and value assigned to the example scenario", type = "composite", compositeOf = { 9121 "context-type", "context" }) 9122 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 9123 /** 9124 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 9125 * <p> 9126 * Description: <b>A use context type and value assigned to the example 9127 * scenario</b><br> 9128 * Type: <b>composite</b><br> 9129 * Path: <b></b><br> 9130 * </p> 9131 */ 9132 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 9133 SP_CONTEXT_TYPE_VALUE); 9134 9135 /** 9136 * Search parameter: <b>jurisdiction</b> 9137 * <p> 9138 * Description: <b>Intended jurisdiction for the example scenario</b><br> 9139 * Type: <b>token</b><br> 9140 * Path: <b>ExampleScenario.jurisdiction</b><br> 9141 * </p> 9142 */ 9143 @SearchParamDefinition(name = "jurisdiction", path = "ExampleScenario.jurisdiction", description = "Intended jurisdiction for the example scenario", type = "token") 9144 public static final String SP_JURISDICTION = "jurisdiction"; 9145 /** 9146 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 9147 * <p> 9148 * Description: <b>Intended jurisdiction for the example scenario</b><br> 9149 * Type: <b>token</b><br> 9150 * Path: <b>ExampleScenario.jurisdiction</b><br> 9151 * </p> 9152 */ 9153 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9154 SP_JURISDICTION); 9155 9156 /** 9157 * Search parameter: <b>context-type</b> 9158 * <p> 9159 * Description: <b>A type of use context assigned to the example 9160 * scenario</b><br> 9161 * Type: <b>token</b><br> 9162 * Path: <b>ExampleScenario.useContext.code</b><br> 9163 * </p> 9164 */ 9165 @SearchParamDefinition(name = "context-type", path = "ExampleScenario.useContext.code", description = "A type of use context assigned to the example scenario", type = "token") 9166 public static final String SP_CONTEXT_TYPE = "context-type"; 9167 /** 9168 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 9169 * <p> 9170 * Description: <b>A type of use context assigned to the example 9171 * scenario</b><br> 9172 * Type: <b>token</b><br> 9173 * Path: <b>ExampleScenario.useContext.code</b><br> 9174 * </p> 9175 */ 9176 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9177 SP_CONTEXT_TYPE); 9178 9179 /** 9180 * Search parameter: <b>version</b> 9181 * <p> 9182 * Description: <b>The business version of the example scenario</b><br> 9183 * Type: <b>token</b><br> 9184 * Path: <b>ExampleScenario.version</b><br> 9185 * </p> 9186 */ 9187 @SearchParamDefinition(name = "version", path = "ExampleScenario.version", description = "The business version of the example scenario", type = "token") 9188 public static final String SP_VERSION = "version"; 9189 /** 9190 * <b>Fluent Client</b> search parameter constant for <b>version</b> 9191 * <p> 9192 * Description: <b>The business version of the example scenario</b><br> 9193 * Type: <b>token</b><br> 9194 * Path: <b>ExampleScenario.version</b><br> 9195 * </p> 9196 */ 9197 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9198 SP_VERSION); 9199 9200 /** 9201 * Search parameter: <b>url</b> 9202 * <p> 9203 * Description: <b>The uri that identifies the example scenario</b><br> 9204 * Type: <b>uri</b><br> 9205 * Path: <b>ExampleScenario.url</b><br> 9206 * </p> 9207 */ 9208 @SearchParamDefinition(name = "url", path = "ExampleScenario.url", description = "The uri that identifies the example scenario", type = "uri") 9209 public static final String SP_URL = "url"; 9210 /** 9211 * <b>Fluent Client</b> search parameter constant for <b>url</b> 9212 * <p> 9213 * Description: <b>The uri that identifies the example scenario</b><br> 9214 * Type: <b>uri</b><br> 9215 * Path: <b>ExampleScenario.url</b><br> 9216 * </p> 9217 */ 9218 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 9219 9220 /** 9221 * Search parameter: <b>context-quantity</b> 9222 * <p> 9223 * Description: <b>A quantity- or range-valued use context assigned to the 9224 * example scenario</b><br> 9225 * Type: <b>quantity</b><br> 9226 * Path: <b>ExampleScenario.useContext.valueQuantity, 9227 * ExampleScenario.useContext.valueRange</b><br> 9228 * </p> 9229 */ 9230 @SearchParamDefinition(name = "context-quantity", path = "(ExampleScenario.useContext.value as Quantity) | (ExampleScenario.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the example scenario", type = "quantity") 9231 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 9232 /** 9233 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 9234 * <p> 9235 * Description: <b>A quantity- or range-valued use context assigned to the 9236 * example scenario</b><br> 9237 * Type: <b>quantity</b><br> 9238 * Path: <b>ExampleScenario.useContext.valueQuantity, 9239 * ExampleScenario.useContext.valueRange</b><br> 9240 * </p> 9241 */ 9242 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 9243 SP_CONTEXT_QUANTITY); 9244 9245 /** 9246 * Search parameter: <b>name</b> 9247 * <p> 9248 * Description: <b>Computationally friendly name of the example scenario</b><br> 9249 * Type: <b>string</b><br> 9250 * Path: <b>ExampleScenario.name</b><br> 9251 * </p> 9252 */ 9253 @SearchParamDefinition(name = "name", path = "ExampleScenario.name", description = "Computationally friendly name of the example scenario", type = "string") 9254 public static final String SP_NAME = "name"; 9255 /** 9256 * <b>Fluent Client</b> search parameter constant for <b>name</b> 9257 * <p> 9258 * Description: <b>Computationally friendly name of the example scenario</b><br> 9259 * Type: <b>string</b><br> 9260 * Path: <b>ExampleScenario.name</b><br> 9261 * </p> 9262 */ 9263 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 9264 SP_NAME); 9265 9266 /** 9267 * Search parameter: <b>context</b> 9268 * <p> 9269 * Description: <b>A use context assigned to the example scenario</b><br> 9270 * Type: <b>token</b><br> 9271 * Path: <b>ExampleScenario.useContext.valueCodeableConcept</b><br> 9272 * </p> 9273 */ 9274 @SearchParamDefinition(name = "context", path = "(ExampleScenario.useContext.value as CodeableConcept)", description = "A use context assigned to the example scenario", type = "token") 9275 public static final String SP_CONTEXT = "context"; 9276 /** 9277 * <b>Fluent Client</b> search parameter constant for <b>context</b> 9278 * <p> 9279 * Description: <b>A use context assigned to the example scenario</b><br> 9280 * Type: <b>token</b><br> 9281 * Path: <b>ExampleScenario.useContext.valueCodeableConcept</b><br> 9282 * </p> 9283 */ 9284 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9285 SP_CONTEXT); 9286 9287 /** 9288 * Search parameter: <b>publisher</b> 9289 * <p> 9290 * Description: <b>Name of the publisher of the example scenario</b><br> 9291 * Type: <b>string</b><br> 9292 * Path: <b>ExampleScenario.publisher</b><br> 9293 * </p> 9294 */ 9295 @SearchParamDefinition(name = "publisher", path = "ExampleScenario.publisher", description = "Name of the publisher of the example scenario", type = "string") 9296 public static final String SP_PUBLISHER = "publisher"; 9297 /** 9298 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 9299 * <p> 9300 * Description: <b>Name of the publisher of the example scenario</b><br> 9301 * Type: <b>string</b><br> 9302 * Path: <b>ExampleScenario.publisher</b><br> 9303 * </p> 9304 */ 9305 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 9306 SP_PUBLISHER); 9307 9308 /** 9309 * Search parameter: <b>context-type-quantity</b> 9310 * <p> 9311 * Description: <b>A use context type and quantity- or range-based value 9312 * assigned to the example scenario</b><br> 9313 * Type: <b>composite</b><br> 9314 * Path: <b></b><br> 9315 * </p> 9316 */ 9317 @SearchParamDefinition(name = "context-type-quantity", path = "ExampleScenario.useContext", description = "A use context type and quantity- or range-based value assigned to the example scenario", type = "composite", compositeOf = { 9318 "context-type", "context-quantity" }) 9319 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 9320 /** 9321 * <b>Fluent Client</b> search parameter constant for 9322 * <b>context-type-quantity</b> 9323 * <p> 9324 * Description: <b>A use context type and quantity- or range-based value 9325 * assigned to the example scenario</b><br> 9326 * Type: <b>composite</b><br> 9327 * Path: <b></b><br> 9328 * </p> 9329 */ 9330 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 9331 SP_CONTEXT_TYPE_QUANTITY); 9332 9333 /** 9334 * Search parameter: <b>status</b> 9335 * <p> 9336 * Description: <b>The current status of the example scenario</b><br> 9337 * Type: <b>token</b><br> 9338 * Path: <b>ExampleScenario.status</b><br> 9339 * </p> 9340 */ 9341 @SearchParamDefinition(name = "status", path = "ExampleScenario.status", description = "The current status of the example scenario", type = "token") 9342 public static final String SP_STATUS = "status"; 9343 /** 9344 * <b>Fluent Client</b> search parameter constant for <b>status</b> 9345 * <p> 9346 * Description: <b>The current status of the example scenario</b><br> 9347 * Type: <b>token</b><br> 9348 * Path: <b>ExampleScenario.status</b><br> 9349 * </p> 9350 */ 9351 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 9352 SP_STATUS); 9353 9354}