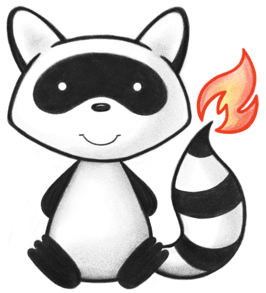
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032import org.hl7.fhir.exceptions.FHIRException; 033 034/** 035 * in a language with helper classes, this would be a helper class (at least, 036 * the base exgtension helpers would be) 037 * 038 * @author Grahame 039 * 040 */ 041public class ExtensionHelper { 042 043 /** 044 * @param name the identity of the extension of interest 045 * @return true if the named extension is on this element. Will check modifier 046 * extensions too if appropriate 047 */ 048 public static boolean hasExtension(Element element, String name) { 049 if (element != null && element instanceof BackboneElement) 050 return hasExtension((BackboneElement) element, name); 051 052 if (name == null || element == null || !element.hasExtension()) 053 return false; 054 for (Extension e : element.getExtension()) { 055 if (name.equals(e.getUrl())) 056 return true; 057 } 058 return false; 059 } 060 061 /** 062 * @param name the identity of the extension of interest 063 * @return true if the named extension is on this element. Will check modifier 064 * extensions 065 */ 066 public static boolean hasExtension(BackboneElement element, String name) { 067 if (name == null || element == null || !(element.hasExtension() || element.hasModifierExtension())) 068 return false; 069 for (Extension e : element.getModifierExtension()) { 070 if (name.equals(e.getUrl())) 071 return true; 072 } 073 for (Extension e : element.getExtension()) { 074 if (name.equals(e.getUrl())) 075 return true; 076 } 077 return false; 078 } 079 080 /** 081 * @param name the identity of the extension of interest 082 * @return The extension, if on this element, else null. will check modifier 083 * extensions too, if appropriate 084 */ 085 public static Extension getExtension(Element element, String name) { 086 if (element != null && element instanceof BackboneElement) 087 return getExtension((BackboneElement) element, name); 088 089 if (name == null || element == null || !element.hasExtension()) 090 return null; 091 for (Extension e : element.getExtension()) { 092 if (name.equals(e.getUrl())) 093 return e; 094 } 095 return null; 096 } 097 098 /** 099 * @param name the identity of the extension of interest 100 * @return The extension, if on this element, else null. will check modifier 101 * extensions too, if appropriate 102 */ 103 public static Extension getExtension(DomainResource resource, String name) { 104 105 if (name == null || resource == null || !resource.hasExtension()) 106 return null; 107 for (Extension e : resource.getExtension()) { 108 if (name.equals(e.getUrl())) 109 return e; 110 } 111 return null; 112 } 113 114 /** 115 * @param name the identity of the extension of interest 116 * @return The extension, if on this element, else null. will check modifier 117 * extensions too 118 */ 119 public static Extension getExtension(BackboneElement element, String name) { 120 if (name == null || element == null || !element.hasExtension()) 121 return null; 122 for (Extension e : element.getModifierExtension()) { 123 if (name.equals(e.getUrl())) 124 return e; 125 } 126 for (Extension e : element.getExtension()) { 127 if (name.equals(e.getUrl())) 128 return e; 129 } 130 return null; 131 } 132 133 /** 134 * set the value of an extension on the element. if value == null, make sure it 135 * doesn't exist 136 * 137 * @param element - the element to act on. Can also be a backbone element 138 * @param modifier - whether this is a modifier. Note that this is a 139 * definitional property of the extension; don't alternate 140 * @param uri - the identifier for the extension 141 * @param value - the value of the extension. Delete if this is null 142 * @throws Exception - if the modifier logic is incorrect 143 */ 144 public static void setExtension(Element element, boolean modifier, String uri, Type value) throws FHIRException { 145 if (value == null) { 146 // deleting the extension 147 if (element instanceof BackboneElement) 148 for (Extension e : ((BackboneElement) element).getModifierExtension()) { 149 if (uri.equals(e.getUrl())) 150 ((BackboneElement) element).getModifierExtension().remove(e); 151 } 152 for (Extension e : element.getExtension()) { 153 if (uri.equals(e.getUrl())) 154 element.getExtension().remove(e); 155 } 156 } else { 157 // it would probably be easier to delete and then create, but this would 158 // re-order the extensions 159 // not that order matters, but we'll preserve it anyway 160 boolean found = false; 161 if (element instanceof BackboneElement) 162 for (Extension e : ((BackboneElement) element).getModifierExtension()) { 163 if (uri.equals(e.getUrl())) { 164 if (!modifier) 165 throw new FHIRException("Error adding extension \"" + uri 166 + "\": found an existing modifier extension, and the extension is not marked as a modifier"); 167 e.setValue(value); 168 found = true; 169 } 170 } 171 for (Extension e : element.getExtension()) { 172 if (uri.equals(e.getUrl())) { 173 if (modifier) 174 throw new FHIRException("Error adding extension \"" + uri 175 + "\": found an existing extension, and the extension is marked as a modifier"); 176 e.setValue(value); 177 found = true; 178 } 179 } 180 if (!found) { 181 Extension ex = new Extension().setUrl(uri).setValue(value); 182 if (modifier) { 183 if (!(element instanceof BackboneElement)) 184 throw new FHIRException("Error adding extension \"" + uri 185 + "\": extension is marked as a modifier, but element is not a backbone element"); 186 ((BackboneElement) element).getModifierExtension().add(ex); 187 188 } else { 189 element.getExtension().add(ex); 190 } 191 } 192 } 193 } 194 195 public static boolean hasExtensions(Element element) { 196 if (element instanceof BackboneElement) 197 return element.hasExtension() || ((BackboneElement) element).hasModifierExtension(); 198 else 199 return element.hasExtension(); 200 } 201 202}