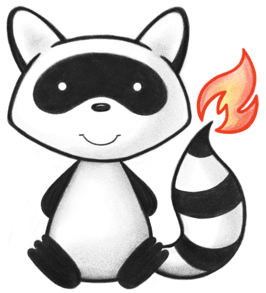
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Significant health conditions for a person related to the patient relevant in 049 * the context of care for the patient. 050 */ 051@ResourceDef(name = "FamilyMemberHistory", profile = "http://hl7.org/fhir/StructureDefinition/FamilyMemberHistory") 052public class FamilyMemberHistory extends DomainResource { 053 054 public enum FamilyHistoryStatus { 055 /** 056 * Some health information is known and captured, but not complete - see notes 057 * for details. 058 */ 059 PARTIAL, 060 /** 061 * All available related health information is captured as of the date (and 062 * possibly time) when the family member history was taken. 063 */ 064 COMPLETED, 065 /** 066 * This instance should not have been part of this patient's medical record. 067 */ 068 ENTEREDINERROR, 069 /** 070 * Health information for this family member is unavailable/unknown. 071 */ 072 HEALTHUNKNOWN, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 078 public static FamilyHistoryStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("partial".equals(codeString)) 082 return PARTIAL; 083 if ("completed".equals(codeString)) 084 return COMPLETED; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if ("health-unknown".equals(codeString)) 088 return HEALTHUNKNOWN; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 093 } 094 095 public String toCode() { 096 switch (this) { 097 case PARTIAL: 098 return "partial"; 099 case COMPLETED: 100 return "completed"; 101 case ENTEREDINERROR: 102 return "entered-in-error"; 103 case HEALTHUNKNOWN: 104 return "health-unknown"; 105 case NULL: 106 return null; 107 default: 108 return "?"; 109 } 110 } 111 112 public String getSystem() { 113 switch (this) { 114 case PARTIAL: 115 return "http://hl7.org/fhir/history-status"; 116 case COMPLETED: 117 return "http://hl7.org/fhir/history-status"; 118 case ENTEREDINERROR: 119 return "http://hl7.org/fhir/history-status"; 120 case HEALTHUNKNOWN: 121 return "http://hl7.org/fhir/history-status"; 122 case NULL: 123 return null; 124 default: 125 return "?"; 126 } 127 } 128 129 public String getDefinition() { 130 switch (this) { 131 case PARTIAL: 132 return "Some health information is known and captured, but not complete - see notes for details."; 133 case COMPLETED: 134 return "All available related health information is captured as of the date (and possibly time) when the family member history was taken."; 135 case ENTEREDINERROR: 136 return "This instance should not have been part of this patient's medical record."; 137 case HEALTHUNKNOWN: 138 return "Health information for this family member is unavailable/unknown."; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getDisplay() { 147 switch (this) { 148 case PARTIAL: 149 return "Partial"; 150 case COMPLETED: 151 return "Completed"; 152 case ENTEREDINERROR: 153 return "Entered in Error"; 154 case HEALTHUNKNOWN: 155 return "Health Unknown"; 156 case NULL: 157 return null; 158 default: 159 return "?"; 160 } 161 } 162 } 163 164 public static class FamilyHistoryStatusEnumFactory implements EnumFactory<FamilyHistoryStatus> { 165 public FamilyHistoryStatus fromCode(String codeString) throws IllegalArgumentException { 166 if (codeString == null || "".equals(codeString)) 167 if (codeString == null || "".equals(codeString)) 168 return null; 169 if ("partial".equals(codeString)) 170 return FamilyHistoryStatus.PARTIAL; 171 if ("completed".equals(codeString)) 172 return FamilyHistoryStatus.COMPLETED; 173 if ("entered-in-error".equals(codeString)) 174 return FamilyHistoryStatus.ENTEREDINERROR; 175 if ("health-unknown".equals(codeString)) 176 return FamilyHistoryStatus.HEALTHUNKNOWN; 177 throw new IllegalArgumentException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 178 } 179 180 public Enumeration<FamilyHistoryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 181 if (code == null) 182 return null; 183 if (code.isEmpty()) 184 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.NULL, code); 185 String codeString = code.asStringValue(); 186 if (codeString == null || "".equals(codeString)) 187 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.NULL, code); 188 if ("partial".equals(codeString)) 189 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.PARTIAL, code); 190 if ("completed".equals(codeString)) 191 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.COMPLETED, code); 192 if ("entered-in-error".equals(codeString)) 193 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.ENTEREDINERROR, code); 194 if ("health-unknown".equals(codeString)) 195 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.HEALTHUNKNOWN, code); 196 throw new FHIRException("Unknown FamilyHistoryStatus code '" + codeString + "'"); 197 } 198 199 public String toCode(FamilyHistoryStatus code) { 200 if (code == FamilyHistoryStatus.NULL) 201 return null; 202 if (code == FamilyHistoryStatus.PARTIAL) 203 return "partial"; 204 if (code == FamilyHistoryStatus.COMPLETED) 205 return "completed"; 206 if (code == FamilyHistoryStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 if (code == FamilyHistoryStatus.HEALTHUNKNOWN) 209 return "health-unknown"; 210 return "?"; 211 } 212 213 public String toSystem(FamilyHistoryStatus code) { 214 return code.getSystem(); 215 } 216 } 217 218 @Block() 219 public static class FamilyMemberHistoryConditionComponent extends BackboneElement implements IBaseBackboneElement { 220 /** 221 * The actual condition specified. Could be a coded condition (like MI or 222 * Diabetes) or a less specific string like 'cancer' depending on how much is 223 * known about the condition and the capabilities of the creating system. 224 */ 225 @Child(name = "code", type = { 226 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 227 @Description(shortDefinition = "Condition suffered by relation", formalDefinition = "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.") 228 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 229 protected CodeableConcept code; 230 231 /** 232 * Indicates what happened following the condition. If the condition resulted in 233 * death, deceased date is captured on the relation. 234 */ 235 @Child(name = "outcome", type = { 236 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 237 @Description(shortDefinition = "deceased | permanent disability | etc.", formalDefinition = "Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.") 238 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-outcome") 239 protected CodeableConcept outcome; 240 241 /** 242 * This condition contributed to the cause of death of the related person. If 243 * contributedToDeath is not populated, then it is unknown. 244 */ 245 @Child(name = "contributedToDeath", type = { 246 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 247 @Description(shortDefinition = "Whether the condition contributed to the cause of death", formalDefinition = "This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.") 248 protected BooleanType contributedToDeath; 249 250 /** 251 * Either the age of onset, range of approximate age or descriptive string can 252 * be recorded. For conditions with multiple occurrences, this describes the 253 * first known occurrence. 254 */ 255 @Child(name = "onset", type = { Age.class, Range.class, Period.class, 256 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 257 @Description(shortDefinition = "When condition first manifested", formalDefinition = "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.") 258 protected Type onset; 259 260 /** 261 * An area where general notes can be placed about this specific condition. 262 */ 263 @Child(name = "note", type = { 264 Annotation.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 265 @Description(shortDefinition = "Extra information about condition", formalDefinition = "An area where general notes can be placed about this specific condition.") 266 protected List<Annotation> note; 267 268 private static final long serialVersionUID = 1230182301L; 269 270 /** 271 * Constructor 272 */ 273 public FamilyMemberHistoryConditionComponent() { 274 super(); 275 } 276 277 /** 278 * Constructor 279 */ 280 public FamilyMemberHistoryConditionComponent(CodeableConcept code) { 281 super(); 282 this.code = code; 283 } 284 285 /** 286 * @return {@link #code} (The actual condition specified. Could be a coded 287 * condition (like MI or Diabetes) or a less specific string like 288 * 'cancer' depending on how much is known about the condition and the 289 * capabilities of the creating system.) 290 */ 291 public CodeableConcept getCode() { 292 if (this.code == null) 293 if (Configuration.errorOnAutoCreate()) 294 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.code"); 295 else if (Configuration.doAutoCreate()) 296 this.code = new CodeableConcept(); // cc 297 return this.code; 298 } 299 300 public boolean hasCode() { 301 return this.code != null && !this.code.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #code} (The actual condition specified. Could be a coded 306 * condition (like MI or Diabetes) or a less specific string like 307 * 'cancer' depending on how much is known about the condition and 308 * the capabilities of the creating system.) 309 */ 310 public FamilyMemberHistoryConditionComponent setCode(CodeableConcept value) { 311 this.code = value; 312 return this; 313 } 314 315 /** 316 * @return {@link #outcome} (Indicates what happened following the condition. If 317 * the condition resulted in death, deceased date is captured on the 318 * relation.) 319 */ 320 public CodeableConcept getOutcome() { 321 if (this.outcome == null) 322 if (Configuration.errorOnAutoCreate()) 323 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.outcome"); 324 else if (Configuration.doAutoCreate()) 325 this.outcome = new CodeableConcept(); // cc 326 return this.outcome; 327 } 328 329 public boolean hasOutcome() { 330 return this.outcome != null && !this.outcome.isEmpty(); 331 } 332 333 /** 334 * @param value {@link #outcome} (Indicates what happened following the 335 * condition. If the condition resulted in death, deceased date is 336 * captured on the relation.) 337 */ 338 public FamilyMemberHistoryConditionComponent setOutcome(CodeableConcept value) { 339 this.outcome = value; 340 return this; 341 } 342 343 /** 344 * @return {@link #contributedToDeath} (This condition contributed to the cause 345 * of death of the related person. If contributedToDeath is not 346 * populated, then it is unknown.). This is the underlying object with 347 * id, value and extensions. The accessor "getContributedToDeath" gives 348 * direct access to the value 349 */ 350 public BooleanType getContributedToDeathElement() { 351 if (this.contributedToDeath == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.contributedToDeath"); 354 else if (Configuration.doAutoCreate()) 355 this.contributedToDeath = new BooleanType(); // bb 356 return this.contributedToDeath; 357 } 358 359 public boolean hasContributedToDeathElement() { 360 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 361 } 362 363 public boolean hasContributedToDeath() { 364 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #contributedToDeath} (This condition contributed to the 369 * cause of death of the related person. If contributedToDeath is 370 * not populated, then it is unknown.). This is the underlying 371 * object with id, value and extensions. The accessor 372 * "getContributedToDeath" gives direct access to the value 373 */ 374 public FamilyMemberHistoryConditionComponent setContributedToDeathElement(BooleanType value) { 375 this.contributedToDeath = value; 376 return this; 377 } 378 379 /** 380 * @return This condition contributed to the cause of death of the related 381 * person. If contributedToDeath is not populated, then it is unknown. 382 */ 383 public boolean getContributedToDeath() { 384 return this.contributedToDeath == null || this.contributedToDeath.isEmpty() ? false 385 : this.contributedToDeath.getValue(); 386 } 387 388 /** 389 * @param value This condition contributed to the cause of death of the related 390 * person. If contributedToDeath is not populated, then it is 391 * unknown. 392 */ 393 public FamilyMemberHistoryConditionComponent setContributedToDeath(boolean value) { 394 if (this.contributedToDeath == null) 395 this.contributedToDeath = new BooleanType(); 396 this.contributedToDeath.setValue(value); 397 return this; 398 } 399 400 /** 401 * @return {@link #onset} (Either the age of onset, range of approximate age or 402 * descriptive string can be recorded. For conditions with multiple 403 * occurrences, this describes the first known occurrence.) 404 */ 405 public Type getOnset() { 406 return this.onset; 407 } 408 409 /** 410 * @return {@link #onset} (Either the age of onset, range of approximate age or 411 * descriptive string can be recorded. For conditions with multiple 412 * occurrences, this describes the first known occurrence.) 413 */ 414 public Age getOnsetAge() throws FHIRException { 415 if (this.onset == null) 416 this.onset = new Age(); 417 if (!(this.onset instanceof Age)) 418 throw new FHIRException( 419 "Type mismatch: the type Age was expected, but " + this.onset.getClass().getName() + " was encountered"); 420 return (Age) this.onset; 421 } 422 423 public boolean hasOnsetAge() { 424 return this != null && this.onset instanceof Age; 425 } 426 427 /** 428 * @return {@link #onset} (Either the age of onset, range of approximate age or 429 * descriptive string can be recorded. For conditions with multiple 430 * occurrences, this describes the first known occurrence.) 431 */ 432 public Range getOnsetRange() throws FHIRException { 433 if (this.onset == null) 434 this.onset = new Range(); 435 if (!(this.onset instanceof Range)) 436 throw new FHIRException( 437 "Type mismatch: the type Range was expected, but " + this.onset.getClass().getName() + " was encountered"); 438 return (Range) this.onset; 439 } 440 441 public boolean hasOnsetRange() { 442 return this != null && this.onset instanceof Range; 443 } 444 445 /** 446 * @return {@link #onset} (Either the age of onset, range of approximate age or 447 * descriptive string can be recorded. For conditions with multiple 448 * occurrences, this describes the first known occurrence.) 449 */ 450 public Period getOnsetPeriod() throws FHIRException { 451 if (this.onset == null) 452 this.onset = new Period(); 453 if (!(this.onset instanceof Period)) 454 throw new FHIRException( 455 "Type mismatch: the type Period was expected, but " + this.onset.getClass().getName() + " was encountered"); 456 return (Period) this.onset; 457 } 458 459 public boolean hasOnsetPeriod() { 460 return this != null && this.onset instanceof Period; 461 } 462 463 /** 464 * @return {@link #onset} (Either the age of onset, range of approximate age or 465 * descriptive string can be recorded. For conditions with multiple 466 * occurrences, this describes the first known occurrence.) 467 */ 468 public StringType getOnsetStringType() throws FHIRException { 469 if (this.onset == null) 470 this.onset = new StringType(); 471 if (!(this.onset instanceof StringType)) 472 throw new FHIRException("Type mismatch: the type StringType was expected, but " 473 + this.onset.getClass().getName() + " was encountered"); 474 return (StringType) this.onset; 475 } 476 477 public boolean hasOnsetStringType() { 478 return this != null && this.onset instanceof StringType; 479 } 480 481 public boolean hasOnset() { 482 return this.onset != null && !this.onset.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #onset} (Either the age of onset, range of approximate 487 * age or descriptive string can be recorded. For conditions with 488 * multiple occurrences, this describes the first known 489 * occurrence.) 490 */ 491 public FamilyMemberHistoryConditionComponent setOnset(Type value) { 492 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof Period 493 || value instanceof StringType)) 494 throw new Error("Not the right type for FamilyMemberHistory.condition.onset[x]: " + value.fhirType()); 495 this.onset = value; 496 return this; 497 } 498 499 /** 500 * @return {@link #note} (An area where general notes can be placed about this 501 * specific condition.) 502 */ 503 public List<Annotation> getNote() { 504 if (this.note == null) 505 this.note = new ArrayList<Annotation>(); 506 return this.note; 507 } 508 509 /** 510 * @return Returns a reference to <code>this</code> for easy method chaining 511 */ 512 public FamilyMemberHistoryConditionComponent setNote(List<Annotation> theNote) { 513 this.note = theNote; 514 return this; 515 } 516 517 public boolean hasNote() { 518 if (this.note == null) 519 return false; 520 for (Annotation item : this.note) 521 if (!item.isEmpty()) 522 return true; 523 return false; 524 } 525 526 public Annotation addNote() { // 3 527 Annotation t = new Annotation(); 528 if (this.note == null) 529 this.note = new ArrayList<Annotation>(); 530 this.note.add(t); 531 return t; 532 } 533 534 public FamilyMemberHistoryConditionComponent addNote(Annotation t) { // 3 535 if (t == null) 536 return this; 537 if (this.note == null) 538 this.note = new ArrayList<Annotation>(); 539 this.note.add(t); 540 return this; 541 } 542 543 /** 544 * @return The first repetition of repeating field {@link #note}, creating it if 545 * it does not already exist 546 */ 547 public Annotation getNoteFirstRep() { 548 if (getNote().isEmpty()) { 549 addNote(); 550 } 551 return getNote().get(0); 552 } 553 554 protected void listChildren(List<Property> children) { 555 super.listChildren(children); 556 children.add(new Property("code", "CodeableConcept", 557 "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 558 0, 1, code)); 559 children.add(new Property("outcome", "CodeableConcept", 560 "Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.", 561 0, 1, outcome)); 562 children.add(new Property("contributedToDeath", "boolean", 563 "This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 564 0, 1, contributedToDeath)); 565 children.add(new Property("onset[x]", "Age|Range|Period|string", 566 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 567 0, 1, onset)); 568 children.add( 569 new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 570 0, java.lang.Integer.MAX_VALUE, note)); 571 } 572 573 @Override 574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 575 switch (_hash) { 576 case 3059181: 577 /* code */ return new Property("code", "CodeableConcept", 578 "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 579 0, 1, code); 580 case -1106507950: 581 /* outcome */ return new Property("outcome", "CodeableConcept", 582 "Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.", 583 0, 1, outcome); 584 case -363644638: 585 /* contributedToDeath */ return new Property("contributedToDeath", "boolean", 586 "This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 587 0, 1, contributedToDeath); 588 case -1886216323: 589 /* onset[x] */ return new Property("onset[x]", "Age|Range|Period|string", 590 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 591 0, 1, onset); 592 case 105901603: 593 /* onset */ return new Property("onset[x]", "Age|Range|Period|string", 594 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 595 0, 1, onset); 596 case -1886241828: 597 /* onsetAge */ return new Property("onset[x]", "Age|Range|Period|string", 598 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 599 0, 1, onset); 600 case -186664742: 601 /* onsetRange */ return new Property("onset[x]", "Age|Range|Period|string", 602 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 603 0, 1, onset); 604 case -1545082428: 605 /* onsetPeriod */ return new Property("onset[x]", "Age|Range|Period|string", 606 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 607 0, 1, onset); 608 case -1445342188: 609 /* onsetString */ return new Property("onset[x]", "Age|Range|Period|string", 610 "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 611 0, 1, onset); 612 case 3387378: 613 /* note */ return new Property("note", "Annotation", 614 "An area where general notes can be placed about this specific condition.", 0, java.lang.Integer.MAX_VALUE, 615 note); 616 default: 617 return super.getNamedProperty(_hash, _name, _checkValid); 618 } 619 620 } 621 622 @Override 623 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 624 switch (hash) { 625 case 3059181: 626 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 627 case -1106507950: 628 /* outcome */ return this.outcome == null ? new Base[0] : new Base[] { this.outcome }; // CodeableConcept 629 case -363644638: 630 /* contributedToDeath */ return this.contributedToDeath == null ? new Base[0] 631 : new Base[] { this.contributedToDeath }; // BooleanType 632 case 105901603: 633 /* onset */ return this.onset == null ? new Base[0] : new Base[] { this.onset }; // Type 634 case 3387378: 635 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 636 default: 637 return super.getProperty(hash, name, checkValid); 638 } 639 640 } 641 642 @Override 643 public Base setProperty(int hash, String name, Base value) throws FHIRException { 644 switch (hash) { 645 case 3059181: // code 646 this.code = castToCodeableConcept(value); // CodeableConcept 647 return value; 648 case -1106507950: // outcome 649 this.outcome = castToCodeableConcept(value); // CodeableConcept 650 return value; 651 case -363644638: // contributedToDeath 652 this.contributedToDeath = castToBoolean(value); // BooleanType 653 return value; 654 case 105901603: // onset 655 this.onset = castToType(value); // Type 656 return value; 657 case 3387378: // note 658 this.getNote().add(castToAnnotation(value)); // Annotation 659 return value; 660 default: 661 return super.setProperty(hash, name, value); 662 } 663 664 } 665 666 @Override 667 public Base setProperty(String name, Base value) throws FHIRException { 668 if (name.equals("code")) { 669 this.code = castToCodeableConcept(value); // CodeableConcept 670 } else if (name.equals("outcome")) { 671 this.outcome = castToCodeableConcept(value); // CodeableConcept 672 } else if (name.equals("contributedToDeath")) { 673 this.contributedToDeath = castToBoolean(value); // BooleanType 674 } else if (name.equals("onset[x]")) { 675 this.onset = castToType(value); // Type 676 } else if (name.equals("note")) { 677 this.getNote().add(castToAnnotation(value)); 678 } else 679 return super.setProperty(name, value); 680 return value; 681 } 682 683 @Override 684 public void removeChild(String name, Base value) throws FHIRException { 685 if (name.equals("code")) { 686 this.code = null; 687 } else if (name.equals("outcome")) { 688 this.outcome = null; 689 } else if (name.equals("contributedToDeath")) { 690 this.contributedToDeath = null; 691 } else if (name.equals("onset[x]")) { 692 this.onset = null; 693 } else if (name.equals("note")) { 694 this.getNote().remove(castToAnnotation(value)); 695 } else 696 super.removeChild(name, value); 697 698 } 699 700 @Override 701 public Base makeProperty(int hash, String name) throws FHIRException { 702 switch (hash) { 703 case 3059181: 704 return getCode(); 705 case -1106507950: 706 return getOutcome(); 707 case -363644638: 708 return getContributedToDeathElement(); 709 case -1886216323: 710 return getOnset(); 711 case 105901603: 712 return getOnset(); 713 case 3387378: 714 return addNote(); 715 default: 716 return super.makeProperty(hash, name); 717 } 718 719 } 720 721 @Override 722 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 723 switch (hash) { 724 case 3059181: 725 /* code */ return new String[] { "CodeableConcept" }; 726 case -1106507950: 727 /* outcome */ return new String[] { "CodeableConcept" }; 728 case -363644638: 729 /* contributedToDeath */ return new String[] { "boolean" }; 730 case 105901603: 731 /* onset */ return new String[] { "Age", "Range", "Period", "string" }; 732 case 3387378: 733 /* note */ return new String[] { "Annotation" }; 734 default: 735 return super.getTypesForProperty(hash, name); 736 } 737 738 } 739 740 @Override 741 public Base addChild(String name) throws FHIRException { 742 if (name.equals("code")) { 743 this.code = new CodeableConcept(); 744 return this.code; 745 } else if (name.equals("outcome")) { 746 this.outcome = new CodeableConcept(); 747 return this.outcome; 748 } else if (name.equals("contributedToDeath")) { 749 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.contributedToDeath"); 750 } else if (name.equals("onsetAge")) { 751 this.onset = new Age(); 752 return this.onset; 753 } else if (name.equals("onsetRange")) { 754 this.onset = new Range(); 755 return this.onset; 756 } else if (name.equals("onsetPeriod")) { 757 this.onset = new Period(); 758 return this.onset; 759 } else if (name.equals("onsetString")) { 760 this.onset = new StringType(); 761 return this.onset; 762 } else if (name.equals("note")) { 763 return addNote(); 764 } else 765 return super.addChild(name); 766 } 767 768 public FamilyMemberHistoryConditionComponent copy() { 769 FamilyMemberHistoryConditionComponent dst = new FamilyMemberHistoryConditionComponent(); 770 copyValues(dst); 771 return dst; 772 } 773 774 public void copyValues(FamilyMemberHistoryConditionComponent dst) { 775 super.copyValues(dst); 776 dst.code = code == null ? null : code.copy(); 777 dst.outcome = outcome == null ? null : outcome.copy(); 778 dst.contributedToDeath = contributedToDeath == null ? null : contributedToDeath.copy(); 779 dst.onset = onset == null ? null : onset.copy(); 780 if (note != null) { 781 dst.note = new ArrayList<Annotation>(); 782 for (Annotation i : note) 783 dst.note.add(i.copy()); 784 } 785 ; 786 } 787 788 @Override 789 public boolean equalsDeep(Base other_) { 790 if (!super.equalsDeep(other_)) 791 return false; 792 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 793 return false; 794 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 795 return compareDeep(code, o.code, true) && compareDeep(outcome, o.outcome, true) 796 && compareDeep(contributedToDeath, o.contributedToDeath, true) && compareDeep(onset, o.onset, true) 797 && compareDeep(note, o.note, true); 798 } 799 800 @Override 801 public boolean equalsShallow(Base other_) { 802 if (!super.equalsShallow(other_)) 803 return false; 804 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 805 return false; 806 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 807 return compareValues(contributedToDeath, o.contributedToDeath, true); 808 } 809 810 public boolean isEmpty() { 811 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, outcome, contributedToDeath, onset, note); 812 } 813 814 public String fhirType() { 815 return "FamilyMemberHistory.condition"; 816 817 } 818 819 } 820 821 /** 822 * Business identifiers assigned to this family member history by the performer 823 * or other systems which remain constant as the resource is updated and 824 * propagates from server to server. 825 */ 826 @Child(name = "identifier", type = { 827 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 828 @Description(shortDefinition = "External Id(s) for this record", formalDefinition = "Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 829 protected List<Identifier> identifier; 830 831 /** 832 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other 833 * definition that is adhered to in whole or in part by this 834 * FamilyMemberHistory. 835 */ 836 @Child(name = "instantiatesCanonical", type = { 837 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 838 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.") 839 protected List<CanonicalType> instantiatesCanonical; 840 841 /** 842 * The URL pointing to an externally maintained protocol, guideline, orderset or 843 * other definition that is adhered to in whole or in part by this 844 * FamilyMemberHistory. 845 */ 846 @Child(name = "instantiatesUri", type = { 847 UriType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 848 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.") 849 protected List<UriType> instantiatesUri; 850 851 /** 852 * A code specifying the status of the record of the family history of a 853 * specific family member. 854 */ 855 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 856 @Description(shortDefinition = "partial | completed | entered-in-error | health-unknown", formalDefinition = "A code specifying the status of the record of the family history of a specific family member.") 857 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/history-status") 858 protected Enumeration<FamilyHistoryStatus> status; 859 860 /** 861 * Describes why the family member's history is not available. 862 */ 863 @Child(name = "dataAbsentReason", type = { 864 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 865 @Description(shortDefinition = "subject-unknown | withheld | unable-to-obtain | deferred", formalDefinition = "Describes why the family member's history is not available.") 866 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/history-absent-reason") 867 protected CodeableConcept dataAbsentReason; 868 869 /** 870 * The person who this history concerns. 871 */ 872 @Child(name = "patient", type = { Patient.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 873 @Description(shortDefinition = "Patient history is about", formalDefinition = "The person who this history concerns.") 874 protected Reference patient; 875 876 /** 877 * The actual object that is the target of the reference (The person who this 878 * history concerns.) 879 */ 880 protected Patient patientTarget; 881 882 /** 883 * The date (and possibly time) when the family member history was recorded or 884 * last updated. 885 */ 886 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 887 @Description(shortDefinition = "When history was recorded or last updated", formalDefinition = "The date (and possibly time) when the family member history was recorded or last updated.") 888 protected DateTimeType date; 889 890 /** 891 * This will either be a name or a description; e.g. "Aunt Susan", "my cousin 892 * with the red hair". 893 */ 894 @Child(name = "name", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 895 @Description(shortDefinition = "The family member described", formalDefinition = "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".") 896 protected StringType name; 897 898 /** 899 * The type of relationship this person has to the patient (father, mother, 900 * brother etc.). 901 */ 902 @Child(name = "relationship", type = { 903 CodeableConcept.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 904 @Description(shortDefinition = "Relationship to the subject", formalDefinition = "The type of relationship this person has to the patient (father, mother, brother etc.).") 905 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-FamilyMember") 906 protected CodeableConcept relationship; 907 908 /** 909 * The birth sex of the family member. 910 */ 911 @Child(name = "sex", type = { CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 912 @Description(shortDefinition = "male | female | other | unknown", formalDefinition = "The birth sex of the family member.") 913 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administrative-gender") 914 protected CodeableConcept sex; 915 916 /** 917 * The actual or approximate date of birth of the relative. 918 */ 919 @Child(name = "born", type = { Period.class, DateType.class, 920 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 921 @Description(shortDefinition = "(approximate) date of birth", formalDefinition = "The actual or approximate date of birth of the relative.") 922 protected Type born; 923 924 /** 925 * The age of the relative at the time the family member history is recorded. 926 */ 927 @Child(name = "age", type = { Age.class, Range.class, 928 StringType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 929 @Description(shortDefinition = "(approximate) age", formalDefinition = "The age of the relative at the time the family member history is recorded.") 930 protected Type age; 931 932 /** 933 * If true, indicates that the age value specified is an estimated value. 934 */ 935 @Child(name = "estimatedAge", type = { 936 BooleanType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 937 @Description(shortDefinition = "Age is estimated?", formalDefinition = "If true, indicates that the age value specified is an estimated value.") 938 protected BooleanType estimatedAge; 939 940 /** 941 * Deceased flag or the actual or approximate age of the relative at the time of 942 * death for the family member history record. 943 */ 944 @Child(name = "deceased", type = { BooleanType.class, Age.class, Range.class, DateType.class, 945 StringType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 946 @Description(shortDefinition = "Dead? How old/when?", formalDefinition = "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.") 947 protected Type deceased; 948 949 /** 950 * Describes why the family member history occurred in coded or textual form. 951 */ 952 @Child(name = "reasonCode", type = { 953 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 954 @Description(shortDefinition = "Why was family member history performed?", formalDefinition = "Describes why the family member history occurred in coded or textual form.") 955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/clinical-findings") 956 protected List<CodeableConcept> reasonCode; 957 958 /** 959 * Indicates a Condition, Observation, AllergyIntolerance, or 960 * QuestionnaireResponse that justifies this family member history event. 961 */ 962 @Child(name = "reasonReference", type = { Condition.class, Observation.class, AllergyIntolerance.class, 963 QuestionnaireResponse.class, DiagnosticReport.class, 964 DocumentReference.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 965 @Description(shortDefinition = "Why was family member history performed?", formalDefinition = "Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.") 966 protected List<Reference> reasonReference; 967 /** 968 * The actual objects that are the target of the reference (Indicates a 969 * Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that 970 * justifies this family member history event.) 971 */ 972 protected List<Resource> reasonReferenceTarget; 973 974 /** 975 * This property allows a non condition-specific note to the made about the 976 * related person. Ideally, the note would be in the condition property, but 977 * this is not always possible. 978 */ 979 @Child(name = "note", type = { 980 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 981 @Description(shortDefinition = "General note about related person", formalDefinition = "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.") 982 protected List<Annotation> note; 983 984 /** 985 * The significant Conditions (or condition) that the family member had. This is 986 * a repeating section to allow a system to represent more than one condition 987 * per resource, though there is nothing stopping multiple resources - one per 988 * condition. 989 */ 990 @Child(name = "condition", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 991 @Description(shortDefinition = "Condition that the related person had", formalDefinition = "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.") 992 protected List<FamilyMemberHistoryConditionComponent> condition; 993 994 private static final long serialVersionUID = -455261406L; 995 996 /** 997 * Constructor 998 */ 999 public FamilyMemberHistory() { 1000 super(); 1001 } 1002 1003 /** 1004 * Constructor 1005 */ 1006 public FamilyMemberHistory(Enumeration<FamilyHistoryStatus> status, Reference patient, CodeableConcept relationship) { 1007 super(); 1008 this.status = status; 1009 this.patient = patient; 1010 this.relationship = relationship; 1011 } 1012 1013 /** 1014 * @return {@link #identifier} (Business identifiers assigned to this family 1015 * member history by the performer or other systems which remain 1016 * constant as the resource is updated and propagates from server to 1017 * server.) 1018 */ 1019 public List<Identifier> getIdentifier() { 1020 if (this.identifier == null) 1021 this.identifier = new ArrayList<Identifier>(); 1022 return this.identifier; 1023 } 1024 1025 /** 1026 * @return Returns a reference to <code>this</code> for easy method chaining 1027 */ 1028 public FamilyMemberHistory setIdentifier(List<Identifier> theIdentifier) { 1029 this.identifier = theIdentifier; 1030 return this; 1031 } 1032 1033 public boolean hasIdentifier() { 1034 if (this.identifier == null) 1035 return false; 1036 for (Identifier item : this.identifier) 1037 if (!item.isEmpty()) 1038 return true; 1039 return false; 1040 } 1041 1042 public Identifier addIdentifier() { // 3 1043 Identifier t = new Identifier(); 1044 if (this.identifier == null) 1045 this.identifier = new ArrayList<Identifier>(); 1046 this.identifier.add(t); 1047 return t; 1048 } 1049 1050 public FamilyMemberHistory addIdentifier(Identifier t) { // 3 1051 if (t == null) 1052 return this; 1053 if (this.identifier == null) 1054 this.identifier = new ArrayList<Identifier>(); 1055 this.identifier.add(t); 1056 return this; 1057 } 1058 1059 /** 1060 * @return The first repetition of repeating field {@link #identifier}, creating 1061 * it if it does not already exist 1062 */ 1063 public Identifier getIdentifierFirstRep() { 1064 if (getIdentifier().isEmpty()) { 1065 addIdentifier(); 1066 } 1067 return getIdentifier().get(0); 1068 } 1069 1070 /** 1071 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1072 * protocol, guideline, orderset or other definition that is adhered to 1073 * in whole or in part by this FamilyMemberHistory.) 1074 */ 1075 public List<CanonicalType> getInstantiatesCanonical() { 1076 if (this.instantiatesCanonical == null) 1077 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1078 return this.instantiatesCanonical; 1079 } 1080 1081 /** 1082 * @return Returns a reference to <code>this</code> for easy method chaining 1083 */ 1084 public FamilyMemberHistory setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1085 this.instantiatesCanonical = theInstantiatesCanonical; 1086 return this; 1087 } 1088 1089 public boolean hasInstantiatesCanonical() { 1090 if (this.instantiatesCanonical == null) 1091 return false; 1092 for (CanonicalType item : this.instantiatesCanonical) 1093 if (!item.isEmpty()) 1094 return true; 1095 return false; 1096 } 1097 1098 /** 1099 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined 1100 * protocol, guideline, orderset or other definition that is adhered to 1101 * in whole or in part by this FamilyMemberHistory.) 1102 */ 1103 public CanonicalType addInstantiatesCanonicalElement() {// 2 1104 CanonicalType t = new CanonicalType(); 1105 if (this.instantiatesCanonical == null) 1106 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1107 this.instantiatesCanonical.add(t); 1108 return t; 1109 } 1110 1111 /** 1112 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1113 * FHIR-defined protocol, guideline, orderset or other definition 1114 * that is adhered to in whole or in part by this 1115 * FamilyMemberHistory.) 1116 */ 1117 public FamilyMemberHistory addInstantiatesCanonical(String value) { // 1 1118 CanonicalType t = new CanonicalType(); 1119 t.setValue(value); 1120 if (this.instantiatesCanonical == null) 1121 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1122 this.instantiatesCanonical.add(t); 1123 return this; 1124 } 1125 1126 /** 1127 * @param value {@link #instantiatesCanonical} (The URL pointing to a 1128 * FHIR-defined protocol, guideline, orderset or other definition 1129 * that is adhered to in whole or in part by this 1130 * FamilyMemberHistory.) 1131 */ 1132 public boolean hasInstantiatesCanonical(String value) { 1133 if (this.instantiatesCanonical == null) 1134 return false; 1135 for (CanonicalType v : this.instantiatesCanonical) 1136 if (v.getValue().equals(value)) // canonical(PlanDefinition|Questionnaire|ActivityDefinition|Measure|OperationDefinition) 1137 return true; 1138 return false; 1139 } 1140 1141 /** 1142 * @return {@link #instantiatesUri} (The URL pointing to an externally 1143 * maintained protocol, guideline, orderset or other definition that is 1144 * adhered to in whole or in part by this FamilyMemberHistory.) 1145 */ 1146 public List<UriType> getInstantiatesUri() { 1147 if (this.instantiatesUri == null) 1148 this.instantiatesUri = new ArrayList<UriType>(); 1149 return this.instantiatesUri; 1150 } 1151 1152 /** 1153 * @return Returns a reference to <code>this</code> for easy method chaining 1154 */ 1155 public FamilyMemberHistory setInstantiatesUri(List<UriType> theInstantiatesUri) { 1156 this.instantiatesUri = theInstantiatesUri; 1157 return this; 1158 } 1159 1160 public boolean hasInstantiatesUri() { 1161 if (this.instantiatesUri == null) 1162 return false; 1163 for (UriType item : this.instantiatesUri) 1164 if (!item.isEmpty()) 1165 return true; 1166 return false; 1167 } 1168 1169 /** 1170 * @return {@link #instantiatesUri} (The URL pointing to an externally 1171 * maintained protocol, guideline, orderset or other definition that is 1172 * adhered to in whole or in part by this FamilyMemberHistory.) 1173 */ 1174 public UriType addInstantiatesUriElement() {// 2 1175 UriType t = new UriType(); 1176 if (this.instantiatesUri == null) 1177 this.instantiatesUri = new ArrayList<UriType>(); 1178 this.instantiatesUri.add(t); 1179 return t; 1180 } 1181 1182 /** 1183 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1184 * maintained protocol, guideline, orderset or other definition 1185 * that is adhered to in whole or in part by this 1186 * FamilyMemberHistory.) 1187 */ 1188 public FamilyMemberHistory addInstantiatesUri(String value) { // 1 1189 UriType t = new UriType(); 1190 t.setValue(value); 1191 if (this.instantiatesUri == null) 1192 this.instantiatesUri = new ArrayList<UriType>(); 1193 this.instantiatesUri.add(t); 1194 return this; 1195 } 1196 1197 /** 1198 * @param value {@link #instantiatesUri} (The URL pointing to an externally 1199 * maintained protocol, guideline, orderset or other definition 1200 * that is adhered to in whole or in part by this 1201 * FamilyMemberHistory.) 1202 */ 1203 public boolean hasInstantiatesUri(String value) { 1204 if (this.instantiatesUri == null) 1205 return false; 1206 for (UriType v : this.instantiatesUri) 1207 if (v.getValue().equals(value)) // uri 1208 return true; 1209 return false; 1210 } 1211 1212 /** 1213 * @return {@link #status} (A code specifying the status of the record of the 1214 * family history of a specific family member.). This is the underlying 1215 * object with id, value and extensions. The accessor "getStatus" gives 1216 * direct access to the value 1217 */ 1218 public Enumeration<FamilyHistoryStatus> getStatusElement() { 1219 if (this.status == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create FamilyMemberHistory.status"); 1222 else if (Configuration.doAutoCreate()) 1223 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); // bb 1224 return this.status; 1225 } 1226 1227 public boolean hasStatusElement() { 1228 return this.status != null && !this.status.isEmpty(); 1229 } 1230 1231 public boolean hasStatus() { 1232 return this.status != null && !this.status.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #status} (A code specifying the status of the record of 1237 * the family history of a specific family member.). This is the 1238 * underlying object with id, value and extensions. The accessor 1239 * "getStatus" gives direct access to the value 1240 */ 1241 public FamilyMemberHistory setStatusElement(Enumeration<FamilyHistoryStatus> value) { 1242 this.status = value; 1243 return this; 1244 } 1245 1246 /** 1247 * @return A code specifying the status of the record of the family history of a 1248 * specific family member. 1249 */ 1250 public FamilyHistoryStatus getStatus() { 1251 return this.status == null ? null : this.status.getValue(); 1252 } 1253 1254 /** 1255 * @param value A code specifying the status of the record of the family history 1256 * of a specific family member. 1257 */ 1258 public FamilyMemberHistory setStatus(FamilyHistoryStatus value) { 1259 if (this.status == null) 1260 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); 1261 this.status.setValue(value); 1262 return this; 1263 } 1264 1265 /** 1266 * @return {@link #dataAbsentReason} (Describes why the family member's history 1267 * is not available.) 1268 */ 1269 public CodeableConcept getDataAbsentReason() { 1270 if (this.dataAbsentReason == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create FamilyMemberHistory.dataAbsentReason"); 1273 else if (Configuration.doAutoCreate()) 1274 this.dataAbsentReason = new CodeableConcept(); // cc 1275 return this.dataAbsentReason; 1276 } 1277 1278 public boolean hasDataAbsentReason() { 1279 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1280 } 1281 1282 /** 1283 * @param value {@link #dataAbsentReason} (Describes why the family member's 1284 * history is not available.) 1285 */ 1286 public FamilyMemberHistory setDataAbsentReason(CodeableConcept value) { 1287 this.dataAbsentReason = value; 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #patient} (The person who this history concerns.) 1293 */ 1294 public Reference getPatient() { 1295 if (this.patient == null) 1296 if (Configuration.errorOnAutoCreate()) 1297 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 1298 else if (Configuration.doAutoCreate()) 1299 this.patient = new Reference(); // cc 1300 return this.patient; 1301 } 1302 1303 public boolean hasPatient() { 1304 return this.patient != null && !this.patient.isEmpty(); 1305 } 1306 1307 /** 1308 * @param value {@link #patient} (The person who this history concerns.) 1309 */ 1310 public FamilyMemberHistory setPatient(Reference value) { 1311 this.patient = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return {@link #patient} The actual object that is the target of the 1317 * reference. The reference library doesn't populate this, but you can 1318 * use it to hold the resource if you resolve it. (The person who this 1319 * history concerns.) 1320 */ 1321 public Patient getPatientTarget() { 1322 if (this.patientTarget == null) 1323 if (Configuration.errorOnAutoCreate()) 1324 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 1325 else if (Configuration.doAutoCreate()) 1326 this.patientTarget = new Patient(); // aa 1327 return this.patientTarget; 1328 } 1329 1330 /** 1331 * @param value {@link #patient} The actual object that is the target of the 1332 * reference. The reference library doesn't use these, but you can 1333 * use it to hold the resource if you resolve it. (The person who 1334 * this history concerns.) 1335 */ 1336 public FamilyMemberHistory setPatientTarget(Patient value) { 1337 this.patientTarget = value; 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #date} (The date (and possibly time) when the family member 1343 * history was recorded or last updated.). This is the underlying object 1344 * with id, value and extensions. The accessor "getDate" gives direct 1345 * access to the value 1346 */ 1347 public DateTimeType getDateElement() { 1348 if (this.date == null) 1349 if (Configuration.errorOnAutoCreate()) 1350 throw new Error("Attempt to auto-create FamilyMemberHistory.date"); 1351 else if (Configuration.doAutoCreate()) 1352 this.date = new DateTimeType(); // bb 1353 return this.date; 1354 } 1355 1356 public boolean hasDateElement() { 1357 return this.date != null && !this.date.isEmpty(); 1358 } 1359 1360 public boolean hasDate() { 1361 return this.date != null && !this.date.isEmpty(); 1362 } 1363 1364 /** 1365 * @param value {@link #date} (The date (and possibly time) when the family 1366 * member history was recorded or last updated.). This is the 1367 * underlying object with id, value and extensions. The accessor 1368 * "getDate" gives direct access to the value 1369 */ 1370 public FamilyMemberHistory setDateElement(DateTimeType value) { 1371 this.date = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return The date (and possibly time) when the family member history was 1377 * recorded or last updated. 1378 */ 1379 public Date getDate() { 1380 return this.date == null ? null : this.date.getValue(); 1381 } 1382 1383 /** 1384 * @param value The date (and possibly time) when the family member history was 1385 * recorded or last updated. 1386 */ 1387 public FamilyMemberHistory setDate(Date value) { 1388 if (value == null) 1389 this.date = null; 1390 else { 1391 if (this.date == null) 1392 this.date = new DateTimeType(); 1393 this.date.setValue(value); 1394 } 1395 return this; 1396 } 1397 1398 /** 1399 * @return {@link #name} (This will either be a name or a description; e.g. 1400 * "Aunt Susan", "my cousin with the red hair".). This is the underlying 1401 * object with id, value and extensions. The accessor "getName" gives 1402 * direct access to the value 1403 */ 1404 public StringType getNameElement() { 1405 if (this.name == null) 1406 if (Configuration.errorOnAutoCreate()) 1407 throw new Error("Attempt to auto-create FamilyMemberHistory.name"); 1408 else if (Configuration.doAutoCreate()) 1409 this.name = new StringType(); // bb 1410 return this.name; 1411 } 1412 1413 public boolean hasNameElement() { 1414 return this.name != null && !this.name.isEmpty(); 1415 } 1416 1417 public boolean hasName() { 1418 return this.name != null && !this.name.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #name} (This will either be a name or a description; e.g. 1423 * "Aunt Susan", "my cousin with the red hair".). This is the 1424 * underlying object with id, value and extensions. The accessor 1425 * "getName" gives direct access to the value 1426 */ 1427 public FamilyMemberHistory setNameElement(StringType value) { 1428 this.name = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return This will either be a name or a description; e.g. "Aunt Susan", "my 1434 * cousin with the red hair". 1435 */ 1436 public String getName() { 1437 return this.name == null ? null : this.name.getValue(); 1438 } 1439 1440 /** 1441 * @param value This will either be a name or a description; e.g. "Aunt Susan", 1442 * "my cousin with the red hair". 1443 */ 1444 public FamilyMemberHistory setName(String value) { 1445 if (Utilities.noString(value)) 1446 this.name = null; 1447 else { 1448 if (this.name == null) 1449 this.name = new StringType(); 1450 this.name.setValue(value); 1451 } 1452 return this; 1453 } 1454 1455 /** 1456 * @return {@link #relationship} (The type of relationship this person has to 1457 * the patient (father, mother, brother etc.).) 1458 */ 1459 public CodeableConcept getRelationship() { 1460 if (this.relationship == null) 1461 if (Configuration.errorOnAutoCreate()) 1462 throw new Error("Attempt to auto-create FamilyMemberHistory.relationship"); 1463 else if (Configuration.doAutoCreate()) 1464 this.relationship = new CodeableConcept(); // cc 1465 return this.relationship; 1466 } 1467 1468 public boolean hasRelationship() { 1469 return this.relationship != null && !this.relationship.isEmpty(); 1470 } 1471 1472 /** 1473 * @param value {@link #relationship} (The type of relationship this person has 1474 * to the patient (father, mother, brother etc.).) 1475 */ 1476 public FamilyMemberHistory setRelationship(CodeableConcept value) { 1477 this.relationship = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return {@link #sex} (The birth sex of the family member.) 1483 */ 1484 public CodeableConcept getSex() { 1485 if (this.sex == null) 1486 if (Configuration.errorOnAutoCreate()) 1487 throw new Error("Attempt to auto-create FamilyMemberHistory.sex"); 1488 else if (Configuration.doAutoCreate()) 1489 this.sex = new CodeableConcept(); // cc 1490 return this.sex; 1491 } 1492 1493 public boolean hasSex() { 1494 return this.sex != null && !this.sex.isEmpty(); 1495 } 1496 1497 /** 1498 * @param value {@link #sex} (The birth sex of the family member.) 1499 */ 1500 public FamilyMemberHistory setSex(CodeableConcept value) { 1501 this.sex = value; 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #born} (The actual or approximate date of birth of the 1507 * relative.) 1508 */ 1509 public Type getBorn() { 1510 return this.born; 1511 } 1512 1513 /** 1514 * @return {@link #born} (The actual or approximate date of birth of the 1515 * relative.) 1516 */ 1517 public Period getBornPeriod() throws FHIRException { 1518 if (this.born == null) 1519 this.born = new Period(); 1520 if (!(this.born instanceof Period)) 1521 throw new FHIRException( 1522 "Type mismatch: the type Period was expected, but " + this.born.getClass().getName() + " was encountered"); 1523 return (Period) this.born; 1524 } 1525 1526 public boolean hasBornPeriod() { 1527 return this != null && this.born instanceof Period; 1528 } 1529 1530 /** 1531 * @return {@link #born} (The actual or approximate date of birth of the 1532 * relative.) 1533 */ 1534 public DateType getBornDateType() throws FHIRException { 1535 if (this.born == null) 1536 this.born = new DateType(); 1537 if (!(this.born instanceof DateType)) 1538 throw new FHIRException( 1539 "Type mismatch: the type DateType was expected, but " + this.born.getClass().getName() + " was encountered"); 1540 return (DateType) this.born; 1541 } 1542 1543 public boolean hasBornDateType() { 1544 return this != null && this.born instanceof DateType; 1545 } 1546 1547 /** 1548 * @return {@link #born} (The actual or approximate date of birth of the 1549 * relative.) 1550 */ 1551 public StringType getBornStringType() throws FHIRException { 1552 if (this.born == null) 1553 this.born = new StringType(); 1554 if (!(this.born instanceof StringType)) 1555 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.born.getClass().getName() 1556 + " was encountered"); 1557 return (StringType) this.born; 1558 } 1559 1560 public boolean hasBornStringType() { 1561 return this != null && this.born instanceof StringType; 1562 } 1563 1564 public boolean hasBorn() { 1565 return this.born != null && !this.born.isEmpty(); 1566 } 1567 1568 /** 1569 * @param value {@link #born} (The actual or approximate date of birth of the 1570 * relative.) 1571 */ 1572 public FamilyMemberHistory setBorn(Type value) { 1573 if (value != null && !(value instanceof Period || value instanceof DateType || value instanceof StringType)) 1574 throw new Error("Not the right type for FamilyMemberHistory.born[x]: " + value.fhirType()); 1575 this.born = value; 1576 return this; 1577 } 1578 1579 /** 1580 * @return {@link #age} (The age of the relative at the time the family member 1581 * history is recorded.) 1582 */ 1583 public Type getAge() { 1584 return this.age; 1585 } 1586 1587 /** 1588 * @return {@link #age} (The age of the relative at the time the family member 1589 * history is recorded.) 1590 */ 1591 public Age getAgeAge() throws FHIRException { 1592 if (this.age == null) 1593 this.age = new Age(); 1594 if (!(this.age instanceof Age)) 1595 throw new FHIRException( 1596 "Type mismatch: the type Age was expected, but " + this.age.getClass().getName() + " was encountered"); 1597 return (Age) this.age; 1598 } 1599 1600 public boolean hasAgeAge() { 1601 return this != null && this.age instanceof Age; 1602 } 1603 1604 /** 1605 * @return {@link #age} (The age of the relative at the time the family member 1606 * history is recorded.) 1607 */ 1608 public Range getAgeRange() throws FHIRException { 1609 if (this.age == null) 1610 this.age = new Range(); 1611 if (!(this.age instanceof Range)) 1612 throw new FHIRException( 1613 "Type mismatch: the type Range was expected, but " + this.age.getClass().getName() + " was encountered"); 1614 return (Range) this.age; 1615 } 1616 1617 public boolean hasAgeRange() { 1618 return this != null && this.age instanceof Range; 1619 } 1620 1621 /** 1622 * @return {@link #age} (The age of the relative at the time the family member 1623 * history is recorded.) 1624 */ 1625 public StringType getAgeStringType() throws FHIRException { 1626 if (this.age == null) 1627 this.age = new StringType(); 1628 if (!(this.age instanceof StringType)) 1629 throw new FHIRException( 1630 "Type mismatch: the type StringType was expected, but " + this.age.getClass().getName() + " was encountered"); 1631 return (StringType) this.age; 1632 } 1633 1634 public boolean hasAgeStringType() { 1635 return this != null && this.age instanceof StringType; 1636 } 1637 1638 public boolean hasAge() { 1639 return this.age != null && !this.age.isEmpty(); 1640 } 1641 1642 /** 1643 * @param value {@link #age} (The age of the relative at the time the family 1644 * member history is recorded.) 1645 */ 1646 public FamilyMemberHistory setAge(Type value) { 1647 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof StringType)) 1648 throw new Error("Not the right type for FamilyMemberHistory.age[x]: " + value.fhirType()); 1649 this.age = value; 1650 return this; 1651 } 1652 1653 /** 1654 * @return {@link #estimatedAge} (If true, indicates that the age value 1655 * specified is an estimated value.). This is the underlying object with 1656 * id, value and extensions. The accessor "getEstimatedAge" gives direct 1657 * access to the value 1658 */ 1659 public BooleanType getEstimatedAgeElement() { 1660 if (this.estimatedAge == null) 1661 if (Configuration.errorOnAutoCreate()) 1662 throw new Error("Attempt to auto-create FamilyMemberHistory.estimatedAge"); 1663 else if (Configuration.doAutoCreate()) 1664 this.estimatedAge = new BooleanType(); // bb 1665 return this.estimatedAge; 1666 } 1667 1668 public boolean hasEstimatedAgeElement() { 1669 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 1670 } 1671 1672 public boolean hasEstimatedAge() { 1673 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 1674 } 1675 1676 /** 1677 * @param value {@link #estimatedAge} (If true, indicates that the age value 1678 * specified is an estimated value.). This is the underlying object 1679 * with id, value and extensions. The accessor "getEstimatedAge" 1680 * gives direct access to the value 1681 */ 1682 public FamilyMemberHistory setEstimatedAgeElement(BooleanType value) { 1683 this.estimatedAge = value; 1684 return this; 1685 } 1686 1687 /** 1688 * @return If true, indicates that the age value specified is an estimated 1689 * value. 1690 */ 1691 public boolean getEstimatedAge() { 1692 return this.estimatedAge == null || this.estimatedAge.isEmpty() ? false : this.estimatedAge.getValue(); 1693 } 1694 1695 /** 1696 * @param value If true, indicates that the age value specified is an estimated 1697 * value. 1698 */ 1699 public FamilyMemberHistory setEstimatedAge(boolean value) { 1700 if (this.estimatedAge == null) 1701 this.estimatedAge = new BooleanType(); 1702 this.estimatedAge.setValue(value); 1703 return this; 1704 } 1705 1706 /** 1707 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1708 * the relative at the time of death for the family member history 1709 * record.) 1710 */ 1711 public Type getDeceased() { 1712 return this.deceased; 1713 } 1714 1715 /** 1716 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1717 * the relative at the time of death for the family member history 1718 * record.) 1719 */ 1720 public BooleanType getDeceasedBooleanType() throws FHIRException { 1721 if (this.deceased == null) 1722 this.deceased = new BooleanType(); 1723 if (!(this.deceased instanceof BooleanType)) 1724 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1725 + this.deceased.getClass().getName() + " was encountered"); 1726 return (BooleanType) this.deceased; 1727 } 1728 1729 public boolean hasDeceasedBooleanType() { 1730 return this != null && this.deceased instanceof BooleanType; 1731 } 1732 1733 /** 1734 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1735 * the relative at the time of death for the family member history 1736 * record.) 1737 */ 1738 public Age getDeceasedAge() throws FHIRException { 1739 if (this.deceased == null) 1740 this.deceased = new Age(); 1741 if (!(this.deceased instanceof Age)) 1742 throw new FHIRException( 1743 "Type mismatch: the type Age was expected, but " + this.deceased.getClass().getName() + " was encountered"); 1744 return (Age) this.deceased; 1745 } 1746 1747 public boolean hasDeceasedAge() { 1748 return this != null && this.deceased instanceof Age; 1749 } 1750 1751 /** 1752 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1753 * the relative at the time of death for the family member history 1754 * record.) 1755 */ 1756 public Range getDeceasedRange() throws FHIRException { 1757 if (this.deceased == null) 1758 this.deceased = new Range(); 1759 if (!(this.deceased instanceof Range)) 1760 throw new FHIRException( 1761 "Type mismatch: the type Range was expected, but " + this.deceased.getClass().getName() + " was encountered"); 1762 return (Range) this.deceased; 1763 } 1764 1765 public boolean hasDeceasedRange() { 1766 return this != null && this.deceased instanceof Range; 1767 } 1768 1769 /** 1770 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1771 * the relative at the time of death for the family member history 1772 * record.) 1773 */ 1774 public DateType getDeceasedDateType() throws FHIRException { 1775 if (this.deceased == null) 1776 this.deceased = new DateType(); 1777 if (!(this.deceased instanceof DateType)) 1778 throw new FHIRException("Type mismatch: the type DateType was expected, but " + this.deceased.getClass().getName() 1779 + " was encountered"); 1780 return (DateType) this.deceased; 1781 } 1782 1783 public boolean hasDeceasedDateType() { 1784 return this != null && this.deceased instanceof DateType; 1785 } 1786 1787 /** 1788 * @return {@link #deceased} (Deceased flag or the actual or approximate age of 1789 * the relative at the time of death for the family member history 1790 * record.) 1791 */ 1792 public StringType getDeceasedStringType() throws FHIRException { 1793 if (this.deceased == null) 1794 this.deceased = new StringType(); 1795 if (!(this.deceased instanceof StringType)) 1796 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1797 + this.deceased.getClass().getName() + " was encountered"); 1798 return (StringType) this.deceased; 1799 } 1800 1801 public boolean hasDeceasedStringType() { 1802 return this != null && this.deceased instanceof StringType; 1803 } 1804 1805 public boolean hasDeceased() { 1806 return this.deceased != null && !this.deceased.isEmpty(); 1807 } 1808 1809 /** 1810 * @param value {@link #deceased} (Deceased flag or the actual or approximate 1811 * age of the relative at the time of death for the family member 1812 * history record.) 1813 */ 1814 public FamilyMemberHistory setDeceased(Type value) { 1815 if (value != null && !(value instanceof BooleanType || value instanceof Age || value instanceof Range 1816 || value instanceof DateType || value instanceof StringType)) 1817 throw new Error("Not the right type for FamilyMemberHistory.deceased[x]: " + value.fhirType()); 1818 this.deceased = value; 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #reasonCode} (Describes why the family member history occurred 1824 * in coded or textual form.) 1825 */ 1826 public List<CodeableConcept> getReasonCode() { 1827 if (this.reasonCode == null) 1828 this.reasonCode = new ArrayList<CodeableConcept>(); 1829 return this.reasonCode; 1830 } 1831 1832 /** 1833 * @return Returns a reference to <code>this</code> for easy method chaining 1834 */ 1835 public FamilyMemberHistory setReasonCode(List<CodeableConcept> theReasonCode) { 1836 this.reasonCode = theReasonCode; 1837 return this; 1838 } 1839 1840 public boolean hasReasonCode() { 1841 if (this.reasonCode == null) 1842 return false; 1843 for (CodeableConcept item : this.reasonCode) 1844 if (!item.isEmpty()) 1845 return true; 1846 return false; 1847 } 1848 1849 public CodeableConcept addReasonCode() { // 3 1850 CodeableConcept t = new CodeableConcept(); 1851 if (this.reasonCode == null) 1852 this.reasonCode = new ArrayList<CodeableConcept>(); 1853 this.reasonCode.add(t); 1854 return t; 1855 } 1856 1857 public FamilyMemberHistory addReasonCode(CodeableConcept t) { // 3 1858 if (t == null) 1859 return this; 1860 if (this.reasonCode == null) 1861 this.reasonCode = new ArrayList<CodeableConcept>(); 1862 this.reasonCode.add(t); 1863 return this; 1864 } 1865 1866 /** 1867 * @return The first repetition of repeating field {@link #reasonCode}, creating 1868 * it if it does not already exist 1869 */ 1870 public CodeableConcept getReasonCodeFirstRep() { 1871 if (getReasonCode().isEmpty()) { 1872 addReasonCode(); 1873 } 1874 return getReasonCode().get(0); 1875 } 1876 1877 /** 1878 * @return {@link #reasonReference} (Indicates a Condition, Observation, 1879 * AllergyIntolerance, or QuestionnaireResponse that justifies this 1880 * family member history event.) 1881 */ 1882 public List<Reference> getReasonReference() { 1883 if (this.reasonReference == null) 1884 this.reasonReference = new ArrayList<Reference>(); 1885 return this.reasonReference; 1886 } 1887 1888 /** 1889 * @return Returns a reference to <code>this</code> for easy method chaining 1890 */ 1891 public FamilyMemberHistory setReasonReference(List<Reference> theReasonReference) { 1892 this.reasonReference = theReasonReference; 1893 return this; 1894 } 1895 1896 public boolean hasReasonReference() { 1897 if (this.reasonReference == null) 1898 return false; 1899 for (Reference item : this.reasonReference) 1900 if (!item.isEmpty()) 1901 return true; 1902 return false; 1903 } 1904 1905 public Reference addReasonReference() { // 3 1906 Reference t = new Reference(); 1907 if (this.reasonReference == null) 1908 this.reasonReference = new ArrayList<Reference>(); 1909 this.reasonReference.add(t); 1910 return t; 1911 } 1912 1913 public FamilyMemberHistory addReasonReference(Reference t) { // 3 1914 if (t == null) 1915 return this; 1916 if (this.reasonReference == null) 1917 this.reasonReference = new ArrayList<Reference>(); 1918 this.reasonReference.add(t); 1919 return this; 1920 } 1921 1922 /** 1923 * @return The first repetition of repeating field {@link #reasonReference}, 1924 * creating it if it does not already exist 1925 */ 1926 public Reference getReasonReferenceFirstRep() { 1927 if (getReasonReference().isEmpty()) { 1928 addReasonReference(); 1929 } 1930 return getReasonReference().get(0); 1931 } 1932 1933 /** 1934 * @deprecated Use Reference#setResource(IBaseResource) instead 1935 */ 1936 @Deprecated 1937 public List<Resource> getReasonReferenceTarget() { 1938 if (this.reasonReferenceTarget == null) 1939 this.reasonReferenceTarget = new ArrayList<Resource>(); 1940 return this.reasonReferenceTarget; 1941 } 1942 1943 /** 1944 * @return {@link #note} (This property allows a non condition-specific note to 1945 * the made about the related person. Ideally, the note would be in the 1946 * condition property, but this is not always possible.) 1947 */ 1948 public List<Annotation> getNote() { 1949 if (this.note == null) 1950 this.note = new ArrayList<Annotation>(); 1951 return this.note; 1952 } 1953 1954 /** 1955 * @return Returns a reference to <code>this</code> for easy method chaining 1956 */ 1957 public FamilyMemberHistory setNote(List<Annotation> theNote) { 1958 this.note = theNote; 1959 return this; 1960 } 1961 1962 public boolean hasNote() { 1963 if (this.note == null) 1964 return false; 1965 for (Annotation item : this.note) 1966 if (!item.isEmpty()) 1967 return true; 1968 return false; 1969 } 1970 1971 public Annotation addNote() { // 3 1972 Annotation t = new Annotation(); 1973 if (this.note == null) 1974 this.note = new ArrayList<Annotation>(); 1975 this.note.add(t); 1976 return t; 1977 } 1978 1979 public FamilyMemberHistory addNote(Annotation t) { // 3 1980 if (t == null) 1981 return this; 1982 if (this.note == null) 1983 this.note = new ArrayList<Annotation>(); 1984 this.note.add(t); 1985 return this; 1986 } 1987 1988 /** 1989 * @return The first repetition of repeating field {@link #note}, creating it if 1990 * it does not already exist 1991 */ 1992 public Annotation getNoteFirstRep() { 1993 if (getNote().isEmpty()) { 1994 addNote(); 1995 } 1996 return getNote().get(0); 1997 } 1998 1999 /** 2000 * @return {@link #condition} (The significant Conditions (or condition) that 2001 * the family member had. This is a repeating section to allow a system 2002 * to represent more than one condition per resource, though there is 2003 * nothing stopping multiple resources - one per condition.) 2004 */ 2005 public List<FamilyMemberHistoryConditionComponent> getCondition() { 2006 if (this.condition == null) 2007 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2008 return this.condition; 2009 } 2010 2011 /** 2012 * @return Returns a reference to <code>this</code> for easy method chaining 2013 */ 2014 public FamilyMemberHistory setCondition(List<FamilyMemberHistoryConditionComponent> theCondition) { 2015 this.condition = theCondition; 2016 return this; 2017 } 2018 2019 public boolean hasCondition() { 2020 if (this.condition == null) 2021 return false; 2022 for (FamilyMemberHistoryConditionComponent item : this.condition) 2023 if (!item.isEmpty()) 2024 return true; 2025 return false; 2026 } 2027 2028 public FamilyMemberHistoryConditionComponent addCondition() { // 3 2029 FamilyMemberHistoryConditionComponent t = new FamilyMemberHistoryConditionComponent(); 2030 if (this.condition == null) 2031 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2032 this.condition.add(t); 2033 return t; 2034 } 2035 2036 public FamilyMemberHistory addCondition(FamilyMemberHistoryConditionComponent t) { // 3 2037 if (t == null) 2038 return this; 2039 if (this.condition == null) 2040 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2041 this.condition.add(t); 2042 return this; 2043 } 2044 2045 /** 2046 * @return The first repetition of repeating field {@link #condition}, creating 2047 * it if it does not already exist 2048 */ 2049 public FamilyMemberHistoryConditionComponent getConditionFirstRep() { 2050 if (getCondition().isEmpty()) { 2051 addCondition(); 2052 } 2053 return getCondition().get(0); 2054 } 2055 2056 protected void listChildren(List<Property> children) { 2057 super.listChildren(children); 2058 children.add(new Property("identifier", "Identifier", 2059 "Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2060 0, java.lang.Integer.MAX_VALUE, identifier)); 2061 children.add(new Property("instantiatesCanonical", 2062 "canonical(PlanDefinition|Questionnaire|ActivityDefinition|Measure|OperationDefinition)", 2063 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 2064 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2065 children.add(new Property("instantiatesUri", "uri", 2066 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 2067 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2068 children.add(new Property("status", "code", 2069 "A code specifying the status of the record of the family history of a specific family member.", 0, 1, status)); 2070 children.add(new Property("dataAbsentReason", "CodeableConcept", 2071 "Describes why the family member's history is not available.", 0, 1, dataAbsentReason)); 2072 children.add(new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, patient)); 2073 children.add(new Property("date", "dateTime", 2074 "The date (and possibly time) when the family member history was recorded or last updated.", 0, 1, date)); 2075 children.add(new Property("name", "string", 2076 "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, 2077 name)); 2078 children.add(new Property("relationship", "CodeableConcept", 2079 "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, relationship)); 2080 children.add(new Property("sex", "CodeableConcept", "The birth sex of the family member.", 0, 1, sex)); 2081 children.add(new Property("born[x]", "Period|date|string", 2082 "The actual or approximate date of birth of the relative.", 0, 1, born)); 2083 children.add(new Property("age[x]", "Age|Range|string", 2084 "The age of the relative at the time the family member history is recorded.", 0, 1, age)); 2085 children.add(new Property("estimatedAge", "boolean", 2086 "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge)); 2087 children.add(new Property("deceased[x]", "boolean|Age|Range|date|string", 2088 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2089 0, 1, deceased)); 2090 children.add(new Property("reasonCode", "CodeableConcept", 2091 "Describes why the family member history occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2092 reasonCode)); 2093 children.add(new Property("reasonReference", 2094 "Reference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse|DiagnosticReport|DocumentReference)", 2095 "Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 2096 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2097 children.add(new Property("note", "Annotation", 2098 "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 2099 0, java.lang.Integer.MAX_VALUE, note)); 2100 children.add(new Property("condition", "", 2101 "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 2102 0, java.lang.Integer.MAX_VALUE, condition)); 2103 } 2104 2105 @Override 2106 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2107 switch (_hash) { 2108 case -1618432855: 2109 /* identifier */ return new Property("identifier", "Identifier", 2110 "Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 2111 0, java.lang.Integer.MAX_VALUE, identifier); 2112 case 8911915: 2113 /* instantiatesCanonical */ return new Property("instantiatesCanonical", 2114 "canonical(PlanDefinition|Questionnaire|ActivityDefinition|Measure|OperationDefinition)", 2115 "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 2116 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2117 case -1926393373: 2118 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 2119 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 2120 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2121 case -892481550: 2122 /* status */ return new Property("status", "code", 2123 "A code specifying the status of the record of the family history of a specific family member.", 0, 1, 2124 status); 2125 case 1034315687: 2126 /* dataAbsentReason */ return new Property("dataAbsentReason", "CodeableConcept", 2127 "Describes why the family member's history is not available.", 0, 1, dataAbsentReason); 2128 case -791418107: 2129 /* patient */ return new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, 2130 patient); 2131 case 3076014: 2132 /* date */ return new Property("date", "dateTime", 2133 "The date (and possibly time) when the family member history was recorded or last updated.", 0, 1, date); 2134 case 3373707: 2135 /* name */ return new Property("name", "string", 2136 "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, 2137 name); 2138 case -261851592: 2139 /* relationship */ return new Property("relationship", "CodeableConcept", 2140 "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, 2141 relationship); 2142 case 113766: 2143 /* sex */ return new Property("sex", "CodeableConcept", "The birth sex of the family member.", 0, 1, sex); 2144 case 67532951: 2145 /* born[x] */ return new Property("born[x]", "Period|date|string", 2146 "The actual or approximate date of birth of the relative.", 0, 1, born); 2147 case 3029833: 2148 /* born */ return new Property("born[x]", "Period|date|string", 2149 "The actual or approximate date of birth of the relative.", 0, 1, born); 2150 case 1497711210: 2151 /* bornPeriod */ return new Property("born[x]", "Period|date|string", 2152 "The actual or approximate date of birth of the relative.", 0, 1, born); 2153 case 2092814999: 2154 /* bornDate */ return new Property("born[x]", "Period|date|string", 2155 "The actual or approximate date of birth of the relative.", 0, 1, born); 2156 case 1597451450: 2157 /* bornString */ return new Property("born[x]", "Period|date|string", 2158 "The actual or approximate date of birth of the relative.", 0, 1, born); 2159 case -1419716831: 2160 /* age[x] */ return new Property("age[x]", "Age|Range|string", 2161 "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2162 case 96511: 2163 /* age */ return new Property("age[x]", "Age|Range|string", 2164 "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2165 case -1419742336: 2166 /* ageAge */ return new Property("age[x]", "Age|Range|string", 2167 "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2168 case 1442748286: 2169 /* ageRange */ return new Property("age[x]", "Age|Range|string", 2170 "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2171 case 1821821424: 2172 /* ageString */ return new Property("age[x]", "Age|Range|string", 2173 "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2174 case 2130167587: 2175 /* estimatedAge */ return new Property("estimatedAge", "boolean", 2176 "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge); 2177 case -1311442804: 2178 /* deceased[x] */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2179 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2180 0, 1, deceased); 2181 case 561497972: 2182 /* deceased */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2183 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2184 0, 1, deceased); 2185 case 497463828: 2186 /* deceasedBoolean */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2187 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2188 0, 1, deceased); 2189 case -1311468309: 2190 /* deceasedAge */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2191 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2192 0, 1, deceased); 2193 case -1880094167: 2194 /* deceasedRange */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2195 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2196 0, 1, deceased); 2197 case -2000727742: 2198 /* deceasedDate */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2199 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2200 0, 1, deceased); 2201 case 1892920485: 2202 /* deceasedString */ return new Property("deceased[x]", "boolean|Age|Range|date|string", 2203 "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 2204 0, 1, deceased); 2205 case 722137681: 2206 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2207 "Describes why the family member history occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 2208 reasonCode); 2209 case -1146218137: 2210 /* reasonReference */ return new Property("reasonReference", 2211 "Reference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse|DiagnosticReport|DocumentReference)", 2212 "Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 2213 0, java.lang.Integer.MAX_VALUE, reasonReference); 2214 case 3387378: 2215 /* note */ return new Property("note", "Annotation", 2216 "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 2217 0, java.lang.Integer.MAX_VALUE, note); 2218 case -861311717: 2219 /* condition */ return new Property("condition", "", 2220 "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 2221 0, java.lang.Integer.MAX_VALUE, condition); 2222 default: 2223 return super.getNamedProperty(_hash, _name, _checkValid); 2224 } 2225 2226 } 2227 2228 @Override 2229 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2230 switch (hash) { 2231 case -1618432855: 2232 /* identifier */ return this.identifier == null ? new Base[0] 2233 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2234 case 8911915: 2235 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 2236 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2237 case -1926393373: 2238 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 2239 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2240 case -892481550: 2241 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<FamilyHistoryStatus> 2242 case 1034315687: 2243 /* dataAbsentReason */ return this.dataAbsentReason == null ? new Base[0] : new Base[] { this.dataAbsentReason }; // CodeableConcept 2244 case -791418107: 2245 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 2246 case 3076014: 2247 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2248 case 3373707: 2249 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2250 case -261851592: 2251 /* relationship */ return this.relationship == null ? new Base[0] : new Base[] { this.relationship }; // CodeableConcept 2252 case 113766: 2253 /* sex */ return this.sex == null ? new Base[0] : new Base[] { this.sex }; // CodeableConcept 2254 case 3029833: 2255 /* born */ return this.born == null ? new Base[0] : new Base[] { this.born }; // Type 2256 case 96511: 2257 /* age */ return this.age == null ? new Base[0] : new Base[] { this.age }; // Type 2258 case 2130167587: 2259 /* estimatedAge */ return this.estimatedAge == null ? new Base[0] : new Base[] { this.estimatedAge }; // BooleanType 2260 case 561497972: 2261 /* deceased */ return this.deceased == null ? new Base[0] : new Base[] { this.deceased }; // Type 2262 case 722137681: 2263 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2264 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2265 case -1146218137: 2266 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2267 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2268 case 3387378: 2269 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2270 case -861311717: 2271 /* condition */ return this.condition == null ? new Base[0] 2272 : this.condition.toArray(new Base[this.condition.size()]); // FamilyMemberHistoryConditionComponent 2273 default: 2274 return super.getProperty(hash, name, checkValid); 2275 } 2276 2277 } 2278 2279 @Override 2280 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2281 switch (hash) { 2282 case -1618432855: // identifier 2283 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2284 return value; 2285 case 8911915: // instantiatesCanonical 2286 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 2287 return value; 2288 case -1926393373: // instantiatesUri 2289 this.getInstantiatesUri().add(castToUri(value)); // UriType 2290 return value; 2291 case -892481550: // status 2292 value = new FamilyHistoryStatusEnumFactory().fromType(castToCode(value)); 2293 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 2294 return value; 2295 case 1034315687: // dataAbsentReason 2296 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 2297 return value; 2298 case -791418107: // patient 2299 this.patient = castToReference(value); // Reference 2300 return value; 2301 case 3076014: // date 2302 this.date = castToDateTime(value); // DateTimeType 2303 return value; 2304 case 3373707: // name 2305 this.name = castToString(value); // StringType 2306 return value; 2307 case -261851592: // relationship 2308 this.relationship = castToCodeableConcept(value); // CodeableConcept 2309 return value; 2310 case 113766: // sex 2311 this.sex = castToCodeableConcept(value); // CodeableConcept 2312 return value; 2313 case 3029833: // born 2314 this.born = castToType(value); // Type 2315 return value; 2316 case 96511: // age 2317 this.age = castToType(value); // Type 2318 return value; 2319 case 2130167587: // estimatedAge 2320 this.estimatedAge = castToBoolean(value); // BooleanType 2321 return value; 2322 case 561497972: // deceased 2323 this.deceased = castToType(value); // Type 2324 return value; 2325 case 722137681: // reasonCode 2326 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2327 return value; 2328 case -1146218137: // reasonReference 2329 this.getReasonReference().add(castToReference(value)); // Reference 2330 return value; 2331 case 3387378: // note 2332 this.getNote().add(castToAnnotation(value)); // Annotation 2333 return value; 2334 case -861311717: // condition 2335 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); // FamilyMemberHistoryConditionComponent 2336 return value; 2337 default: 2338 return super.setProperty(hash, name, value); 2339 } 2340 2341 } 2342 2343 @Override 2344 public Base setProperty(String name, Base value) throws FHIRException { 2345 if (name.equals("identifier")) { 2346 this.getIdentifier().add(castToIdentifier(value)); 2347 } else if (name.equals("instantiatesCanonical")) { 2348 this.getInstantiatesCanonical().add(castToCanonical(value)); 2349 } else if (name.equals("instantiatesUri")) { 2350 this.getInstantiatesUri().add(castToUri(value)); 2351 } else if (name.equals("status")) { 2352 value = new FamilyHistoryStatusEnumFactory().fromType(castToCode(value)); 2353 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 2354 } else if (name.equals("dataAbsentReason")) { 2355 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 2356 } else if (name.equals("patient")) { 2357 this.patient = castToReference(value); // Reference 2358 } else if (name.equals("date")) { 2359 this.date = castToDateTime(value); // DateTimeType 2360 } else if (name.equals("name")) { 2361 this.name = castToString(value); // StringType 2362 } else if (name.equals("relationship")) { 2363 this.relationship = castToCodeableConcept(value); // CodeableConcept 2364 } else if (name.equals("sex")) { 2365 this.sex = castToCodeableConcept(value); // CodeableConcept 2366 } else if (name.equals("born[x]")) { 2367 this.born = castToType(value); // Type 2368 } else if (name.equals("age[x]")) { 2369 this.age = castToType(value); // Type 2370 } else if (name.equals("estimatedAge")) { 2371 this.estimatedAge = castToBoolean(value); // BooleanType 2372 } else if (name.equals("deceased[x]")) { 2373 this.deceased = castToType(value); // Type 2374 } else if (name.equals("reasonCode")) { 2375 this.getReasonCode().add(castToCodeableConcept(value)); 2376 } else if (name.equals("reasonReference")) { 2377 this.getReasonReference().add(castToReference(value)); 2378 } else if (name.equals("note")) { 2379 this.getNote().add(castToAnnotation(value)); 2380 } else if (name.equals("condition")) { 2381 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); 2382 } else 2383 return super.setProperty(name, value); 2384 return value; 2385 } 2386 2387 @Override 2388 public void removeChild(String name, Base value) throws FHIRException { 2389 if (name.equals("identifier")) { 2390 this.getIdentifier().remove(castToIdentifier(value)); 2391 } else if (name.equals("instantiatesCanonical")) { 2392 this.getInstantiatesCanonical().remove(castToCanonical(value)); 2393 } else if (name.equals("instantiatesUri")) { 2394 this.getInstantiatesUri().remove(castToUri(value)); 2395 } else if (name.equals("status")) { 2396 this.status = null; 2397 } else if (name.equals("dataAbsentReason")) { 2398 this.dataAbsentReason = null; 2399 } else if (name.equals("patient")) { 2400 this.patient = null; 2401 } else if (name.equals("date")) { 2402 this.date = null; 2403 } else if (name.equals("name")) { 2404 this.name = null; 2405 } else if (name.equals("relationship")) { 2406 this.relationship = null; 2407 } else if (name.equals("sex")) { 2408 this.sex = null; 2409 } else if (name.equals("born[x]")) { 2410 this.born = null; 2411 } else if (name.equals("age[x]")) { 2412 this.age = null; 2413 } else if (name.equals("estimatedAge")) { 2414 this.estimatedAge = null; 2415 } else if (name.equals("deceased[x]")) { 2416 this.deceased = null; 2417 } else if (name.equals("reasonCode")) { 2418 this.getReasonCode().remove(castToCodeableConcept(value)); 2419 } else if (name.equals("reasonReference")) { 2420 this.getReasonReference().remove(castToReference(value)); 2421 } else if (name.equals("note")) { 2422 this.getNote().remove(castToAnnotation(value)); 2423 } else if (name.equals("condition")) { 2424 this.getCondition().remove((FamilyMemberHistoryConditionComponent) value); 2425 } else 2426 super.removeChild(name, value); 2427 2428 } 2429 2430 @Override 2431 public Base makeProperty(int hash, String name) throws FHIRException { 2432 switch (hash) { 2433 case -1618432855: 2434 return addIdentifier(); 2435 case 8911915: 2436 return addInstantiatesCanonicalElement(); 2437 case -1926393373: 2438 return addInstantiatesUriElement(); 2439 case -892481550: 2440 return getStatusElement(); 2441 case 1034315687: 2442 return getDataAbsentReason(); 2443 case -791418107: 2444 return getPatient(); 2445 case 3076014: 2446 return getDateElement(); 2447 case 3373707: 2448 return getNameElement(); 2449 case -261851592: 2450 return getRelationship(); 2451 case 113766: 2452 return getSex(); 2453 case 67532951: 2454 return getBorn(); 2455 case 3029833: 2456 return getBorn(); 2457 case -1419716831: 2458 return getAge(); 2459 case 96511: 2460 return getAge(); 2461 case 2130167587: 2462 return getEstimatedAgeElement(); 2463 case -1311442804: 2464 return getDeceased(); 2465 case 561497972: 2466 return getDeceased(); 2467 case 722137681: 2468 return addReasonCode(); 2469 case -1146218137: 2470 return addReasonReference(); 2471 case 3387378: 2472 return addNote(); 2473 case -861311717: 2474 return addCondition(); 2475 default: 2476 return super.makeProperty(hash, name); 2477 } 2478 2479 } 2480 2481 @Override 2482 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2483 switch (hash) { 2484 case -1618432855: 2485 /* identifier */ return new String[] { "Identifier" }; 2486 case 8911915: 2487 /* instantiatesCanonical */ return new String[] { "canonical" }; 2488 case -1926393373: 2489 /* instantiatesUri */ return new String[] { "uri" }; 2490 case -892481550: 2491 /* status */ return new String[] { "code" }; 2492 case 1034315687: 2493 /* dataAbsentReason */ return new String[] { "CodeableConcept" }; 2494 case -791418107: 2495 /* patient */ return new String[] { "Reference" }; 2496 case 3076014: 2497 /* date */ return new String[] { "dateTime" }; 2498 case 3373707: 2499 /* name */ return new String[] { "string" }; 2500 case -261851592: 2501 /* relationship */ return new String[] { "CodeableConcept" }; 2502 case 113766: 2503 /* sex */ return new String[] { "CodeableConcept" }; 2504 case 3029833: 2505 /* born */ return new String[] { "Period", "date", "string" }; 2506 case 96511: 2507 /* age */ return new String[] { "Age", "Range", "string" }; 2508 case 2130167587: 2509 /* estimatedAge */ return new String[] { "boolean" }; 2510 case 561497972: 2511 /* deceased */ return new String[] { "boolean", "Age", "Range", "date", "string" }; 2512 case 722137681: 2513 /* reasonCode */ return new String[] { "CodeableConcept" }; 2514 case -1146218137: 2515 /* reasonReference */ return new String[] { "Reference" }; 2516 case 3387378: 2517 /* note */ return new String[] { "Annotation" }; 2518 case -861311717: 2519 /* condition */ return new String[] {}; 2520 default: 2521 return super.getTypesForProperty(hash, name); 2522 } 2523 2524 } 2525 2526 @Override 2527 public Base addChild(String name) throws FHIRException { 2528 if (name.equals("identifier")) { 2529 return addIdentifier(); 2530 } else if (name.equals("instantiatesCanonical")) { 2531 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.instantiatesCanonical"); 2532 } else if (name.equals("instantiatesUri")) { 2533 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.instantiatesUri"); 2534 } else if (name.equals("status")) { 2535 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.status"); 2536 } else if (name.equals("dataAbsentReason")) { 2537 this.dataAbsentReason = new CodeableConcept(); 2538 return this.dataAbsentReason; 2539 } else if (name.equals("patient")) { 2540 this.patient = new Reference(); 2541 return this.patient; 2542 } else if (name.equals("date")) { 2543 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.date"); 2544 } else if (name.equals("name")) { 2545 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.name"); 2546 } else if (name.equals("relationship")) { 2547 this.relationship = new CodeableConcept(); 2548 return this.relationship; 2549 } else if (name.equals("sex")) { 2550 this.sex = new CodeableConcept(); 2551 return this.sex; 2552 } else if (name.equals("bornPeriod")) { 2553 this.born = new Period(); 2554 return this.born; 2555 } else if (name.equals("bornDate")) { 2556 this.born = new DateType(); 2557 return this.born; 2558 } else if (name.equals("bornString")) { 2559 this.born = new StringType(); 2560 return this.born; 2561 } else if (name.equals("ageAge")) { 2562 this.age = new Age(); 2563 return this.age; 2564 } else if (name.equals("ageRange")) { 2565 this.age = new Range(); 2566 return this.age; 2567 } else if (name.equals("ageString")) { 2568 this.age = new StringType(); 2569 return this.age; 2570 } else if (name.equals("estimatedAge")) { 2571 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.estimatedAge"); 2572 } else if (name.equals("deceasedBoolean")) { 2573 this.deceased = new BooleanType(); 2574 return this.deceased; 2575 } else if (name.equals("deceasedAge")) { 2576 this.deceased = new Age(); 2577 return this.deceased; 2578 } else if (name.equals("deceasedRange")) { 2579 this.deceased = new Range(); 2580 return this.deceased; 2581 } else if (name.equals("deceasedDate")) { 2582 this.deceased = new DateType(); 2583 return this.deceased; 2584 } else if (name.equals("deceasedString")) { 2585 this.deceased = new StringType(); 2586 return this.deceased; 2587 } else if (name.equals("reasonCode")) { 2588 return addReasonCode(); 2589 } else if (name.equals("reasonReference")) { 2590 return addReasonReference(); 2591 } else if (name.equals("note")) { 2592 return addNote(); 2593 } else if (name.equals("condition")) { 2594 return addCondition(); 2595 } else 2596 return super.addChild(name); 2597 } 2598 2599 public String fhirType() { 2600 return "FamilyMemberHistory"; 2601 2602 } 2603 2604 public FamilyMemberHistory copy() { 2605 FamilyMemberHistory dst = new FamilyMemberHistory(); 2606 copyValues(dst); 2607 return dst; 2608 } 2609 2610 public void copyValues(FamilyMemberHistory dst) { 2611 super.copyValues(dst); 2612 if (identifier != null) { 2613 dst.identifier = new ArrayList<Identifier>(); 2614 for (Identifier i : identifier) 2615 dst.identifier.add(i.copy()); 2616 } 2617 ; 2618 if (instantiatesCanonical != null) { 2619 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2620 for (CanonicalType i : instantiatesCanonical) 2621 dst.instantiatesCanonical.add(i.copy()); 2622 } 2623 ; 2624 if (instantiatesUri != null) { 2625 dst.instantiatesUri = new ArrayList<UriType>(); 2626 for (UriType i : instantiatesUri) 2627 dst.instantiatesUri.add(i.copy()); 2628 } 2629 ; 2630 dst.status = status == null ? null : status.copy(); 2631 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 2632 dst.patient = patient == null ? null : patient.copy(); 2633 dst.date = date == null ? null : date.copy(); 2634 dst.name = name == null ? null : name.copy(); 2635 dst.relationship = relationship == null ? null : relationship.copy(); 2636 dst.sex = sex == null ? null : sex.copy(); 2637 dst.born = born == null ? null : born.copy(); 2638 dst.age = age == null ? null : age.copy(); 2639 dst.estimatedAge = estimatedAge == null ? null : estimatedAge.copy(); 2640 dst.deceased = deceased == null ? null : deceased.copy(); 2641 if (reasonCode != null) { 2642 dst.reasonCode = new ArrayList<CodeableConcept>(); 2643 for (CodeableConcept i : reasonCode) 2644 dst.reasonCode.add(i.copy()); 2645 } 2646 ; 2647 if (reasonReference != null) { 2648 dst.reasonReference = new ArrayList<Reference>(); 2649 for (Reference i : reasonReference) 2650 dst.reasonReference.add(i.copy()); 2651 } 2652 ; 2653 if (note != null) { 2654 dst.note = new ArrayList<Annotation>(); 2655 for (Annotation i : note) 2656 dst.note.add(i.copy()); 2657 } 2658 ; 2659 if (condition != null) { 2660 dst.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2661 for (FamilyMemberHistoryConditionComponent i : condition) 2662 dst.condition.add(i.copy()); 2663 } 2664 ; 2665 } 2666 2667 protected FamilyMemberHistory typedCopy() { 2668 return copy(); 2669 } 2670 2671 @Override 2672 public boolean equalsDeep(Base other_) { 2673 if (!super.equalsDeep(other_)) 2674 return false; 2675 if (!(other_ instanceof FamilyMemberHistory)) 2676 return false; 2677 FamilyMemberHistory o = (FamilyMemberHistory) other_; 2678 return compareDeep(identifier, o.identifier, true) 2679 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 2680 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(status, o.status, true) 2681 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) && compareDeep(patient, o.patient, true) 2682 && compareDeep(date, o.date, true) && compareDeep(name, o.name, true) 2683 && compareDeep(relationship, o.relationship, true) && compareDeep(sex, o.sex, true) 2684 && compareDeep(born, o.born, true) && compareDeep(age, o.age, true) 2685 && compareDeep(estimatedAge, o.estimatedAge, true) && compareDeep(deceased, o.deceased, true) 2686 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2687 && compareDeep(note, o.note, true) && compareDeep(condition, o.condition, true); 2688 } 2689 2690 @Override 2691 public boolean equalsShallow(Base other_) { 2692 if (!super.equalsShallow(other_)) 2693 return false; 2694 if (!(other_ instanceof FamilyMemberHistory)) 2695 return false; 2696 FamilyMemberHistory o = (FamilyMemberHistory) other_; 2697 return compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(status, o.status, true) 2698 && compareValues(date, o.date, true) && compareValues(name, o.name, true) 2699 && compareValues(estimatedAge, o.estimatedAge, true); 2700 } 2701 2702 public boolean isEmpty() { 2703 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical, instantiatesUri, 2704 status, dataAbsentReason, patient, date, name, relationship, sex, born, age, estimatedAge, deceased, reasonCode, 2705 reasonReference, note, condition); 2706 } 2707 2708 @Override 2709 public ResourceType getResourceType() { 2710 return ResourceType.FamilyMemberHistory; 2711 } 2712 2713 /** 2714 * Search parameter: <b>date</b> 2715 * <p> 2716 * Description: <b>When history was recorded or last updated</b><br> 2717 * Type: <b>date</b><br> 2718 * Path: <b>FamilyMemberHistory.date</b><br> 2719 * </p> 2720 */ 2721 @SearchParamDefinition(name = "date", path = "FamilyMemberHistory.date", description = "When history was recorded or last updated", type = "date") 2722 public static final String SP_DATE = "date"; 2723 /** 2724 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2725 * <p> 2726 * Description: <b>When history was recorded or last updated</b><br> 2727 * Type: <b>date</b><br> 2728 * Path: <b>FamilyMemberHistory.date</b><br> 2729 * </p> 2730 */ 2731 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2732 SP_DATE); 2733 2734 /** 2735 * Search parameter: <b>identifier</b> 2736 * <p> 2737 * Description: <b>A search by a record identifier</b><br> 2738 * Type: <b>token</b><br> 2739 * Path: <b>FamilyMemberHistory.identifier</b><br> 2740 * </p> 2741 */ 2742 @SearchParamDefinition(name = "identifier", path = "FamilyMemberHistory.identifier", description = "A search by a record identifier", type = "token") 2743 public static final String SP_IDENTIFIER = "identifier"; 2744 /** 2745 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2746 * <p> 2747 * Description: <b>A search by a record identifier</b><br> 2748 * Type: <b>token</b><br> 2749 * Path: <b>FamilyMemberHistory.identifier</b><br> 2750 * </p> 2751 */ 2752 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2753 SP_IDENTIFIER); 2754 2755 /** 2756 * Search parameter: <b>code</b> 2757 * <p> 2758 * Description: <b>A search by a condition code</b><br> 2759 * Type: <b>token</b><br> 2760 * Path: <b>FamilyMemberHistory.condition.code</b><br> 2761 * </p> 2762 */ 2763 @SearchParamDefinition(name = "code", path = "FamilyMemberHistory.condition.code", description = "A search by a condition code", type = "token") 2764 public static final String SP_CODE = "code"; 2765 /** 2766 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2767 * <p> 2768 * Description: <b>A search by a condition code</b><br> 2769 * Type: <b>token</b><br> 2770 * Path: <b>FamilyMemberHistory.condition.code</b><br> 2771 * </p> 2772 */ 2773 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2774 SP_CODE); 2775 2776 /** 2777 * Search parameter: <b>patient</b> 2778 * <p> 2779 * Description: <b>The identity of a subject to list family member history items 2780 * for</b><br> 2781 * Type: <b>reference</b><br> 2782 * Path: <b>FamilyMemberHistory.patient</b><br> 2783 * </p> 2784 */ 2785 @SearchParamDefinition(name = "patient", path = "FamilyMemberHistory.patient", description = "The identity of a subject to list family member history items for", type = "reference", providesMembershipIn = { 2786 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2787 public static final String SP_PATIENT = "patient"; 2788 /** 2789 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2790 * <p> 2791 * Description: <b>The identity of a subject to list family member history items 2792 * for</b><br> 2793 * Type: <b>reference</b><br> 2794 * Path: <b>FamilyMemberHistory.patient</b><br> 2795 * </p> 2796 */ 2797 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2798 SP_PATIENT); 2799 2800 /** 2801 * Constant for fluent queries to be used to add include statements. Specifies 2802 * the path value of "<b>FamilyMemberHistory:patient</b>". 2803 */ 2804 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2805 "FamilyMemberHistory:patient").toLocked(); 2806 2807 /** 2808 * Search parameter: <b>sex</b> 2809 * <p> 2810 * Description: <b>A search by a sex code of a family member</b><br> 2811 * Type: <b>token</b><br> 2812 * Path: <b>FamilyMemberHistory.sex</b><br> 2813 * </p> 2814 */ 2815 @SearchParamDefinition(name = "sex", path = "FamilyMemberHistory.sex", description = "A search by a sex code of a family member", type = "token") 2816 public static final String SP_SEX = "sex"; 2817 /** 2818 * <b>Fluent Client</b> search parameter constant for <b>sex</b> 2819 * <p> 2820 * Description: <b>A search by a sex code of a family member</b><br> 2821 * Type: <b>token</b><br> 2822 * Path: <b>FamilyMemberHistory.sex</b><br> 2823 * </p> 2824 */ 2825 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEX = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2826 SP_SEX); 2827 2828 /** 2829 * Search parameter: <b>instantiates-canonical</b> 2830 * <p> 2831 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2832 * Type: <b>reference</b><br> 2833 * Path: <b>FamilyMemberHistory.instantiatesCanonical</b><br> 2834 * </p> 2835 */ 2836 @SearchParamDefinition(name = "instantiates-canonical", path = "FamilyMemberHistory.instantiatesCanonical", description = "Instantiates FHIR protocol or definition", type = "reference", target = { 2837 ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class }) 2838 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 2839 /** 2840 * <b>Fluent Client</b> search parameter constant for 2841 * <b>instantiates-canonical</b> 2842 * <p> 2843 * Description: <b>Instantiates FHIR protocol or definition</b><br> 2844 * Type: <b>reference</b><br> 2845 * Path: <b>FamilyMemberHistory.instantiatesCanonical</b><br> 2846 * </p> 2847 */ 2848 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2849 SP_INSTANTIATES_CANONICAL); 2850 2851 /** 2852 * Constant for fluent queries to be used to add include statements. Specifies 2853 * the path value of "<b>FamilyMemberHistory:instantiates-canonical</b>". 2854 */ 2855 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include( 2856 "FamilyMemberHistory:instantiates-canonical").toLocked(); 2857 2858 /** 2859 * Search parameter: <b>instantiates-uri</b> 2860 * <p> 2861 * Description: <b>Instantiates external protocol or definition</b><br> 2862 * Type: <b>uri</b><br> 2863 * Path: <b>FamilyMemberHistory.instantiatesUri</b><br> 2864 * </p> 2865 */ 2866 @SearchParamDefinition(name = "instantiates-uri", path = "FamilyMemberHistory.instantiatesUri", description = "Instantiates external protocol or definition", type = "uri") 2867 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 2868 /** 2869 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 2870 * <p> 2871 * Description: <b>Instantiates external protocol or definition</b><br> 2872 * Type: <b>uri</b><br> 2873 * Path: <b>FamilyMemberHistory.instantiatesUri</b><br> 2874 * </p> 2875 */ 2876 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam( 2877 SP_INSTANTIATES_URI); 2878 2879 /** 2880 * Search parameter: <b>relationship</b> 2881 * <p> 2882 * Description: <b>A search by a relationship type</b><br> 2883 * Type: <b>token</b><br> 2884 * Path: <b>FamilyMemberHistory.relationship</b><br> 2885 * </p> 2886 */ 2887 @SearchParamDefinition(name = "relationship", path = "FamilyMemberHistory.relationship", description = "A search by a relationship type", type = "token") 2888 public static final String SP_RELATIONSHIP = "relationship"; 2889 /** 2890 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 2891 * <p> 2892 * Description: <b>A search by a relationship type</b><br> 2893 * Type: <b>token</b><br> 2894 * Path: <b>FamilyMemberHistory.relationship</b><br> 2895 * </p> 2896 */ 2897 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATIONSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2898 SP_RELATIONSHIP); 2899 2900 /** 2901 * Search parameter: <b>status</b> 2902 * <p> 2903 * Description: <b>partial | completed | entered-in-error | 2904 * health-unknown</b><br> 2905 * Type: <b>token</b><br> 2906 * Path: <b>FamilyMemberHistory.status</b><br> 2907 * </p> 2908 */ 2909 @SearchParamDefinition(name = "status", path = "FamilyMemberHistory.status", description = "partial | completed | entered-in-error | health-unknown", type = "token") 2910 public static final String SP_STATUS = "status"; 2911 /** 2912 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2913 * <p> 2914 * Description: <b>partial | completed | entered-in-error | 2915 * health-unknown</b><br> 2916 * Type: <b>token</b><br> 2917 * Path: <b>FamilyMemberHistory.status</b><br> 2918 * </p> 2919 */ 2920 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2921 SP_STATUS); 2922 2923}