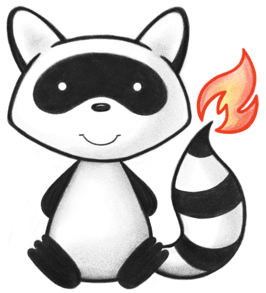
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042 043/** 044 * Prospective warnings of potential issues when providing care to the patient. 045 */ 046@ResourceDef(name = "Flag", profile = "http://hl7.org/fhir/StructureDefinition/Flag") 047public class Flag extends DomainResource { 048 049 public enum FlagStatus { 050 /** 051 * A current flag that should be displayed to a user. A system may use the 052 * category to determine which user roles should view the flag. 053 */ 054 ACTIVE, 055 /** 056 * The flag no longer needs to be displayed. 057 */ 058 INACTIVE, 059 /** 060 * The flag was added in error and should no longer be displayed. 061 */ 062 ENTEREDINERROR, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 068 public static FlagStatus fromCode(String codeString) throws FHIRException { 069 if (codeString == null || "".equals(codeString)) 070 return null; 071 if ("active".equals(codeString)) 072 return ACTIVE; 073 if ("inactive".equals(codeString)) 074 return INACTIVE; 075 if ("entered-in-error".equals(codeString)) 076 return ENTEREDINERROR; 077 if (Configuration.isAcceptInvalidEnums()) 078 return null; 079 else 080 throw new FHIRException("Unknown FlagStatus code '" + codeString + "'"); 081 } 082 083 public String toCode() { 084 switch (this) { 085 case ACTIVE: 086 return "active"; 087 case INACTIVE: 088 return "inactive"; 089 case ENTEREDINERROR: 090 return "entered-in-error"; 091 case NULL: 092 return null; 093 default: 094 return "?"; 095 } 096 } 097 098 public String getSystem() { 099 switch (this) { 100 case ACTIVE: 101 return "http://hl7.org/fhir/flag-status"; 102 case INACTIVE: 103 return "http://hl7.org/fhir/flag-status"; 104 case ENTEREDINERROR: 105 return "http://hl7.org/fhir/flag-status"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getDefinition() { 114 switch (this) { 115 case ACTIVE: 116 return "A current flag that should be displayed to a user. A system may use the category to determine which user roles should view the flag."; 117 case INACTIVE: 118 return "The flag no longer needs to be displayed."; 119 case ENTEREDINERROR: 120 return "The flag was added in error and should no longer be displayed."; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDisplay() { 129 switch (this) { 130 case ACTIVE: 131 return "Active"; 132 case INACTIVE: 133 return "Inactive"; 134 case ENTEREDINERROR: 135 return "Entered in Error"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 } 143 144 public static class FlagStatusEnumFactory implements EnumFactory<FlagStatus> { 145 public FlagStatus fromCode(String codeString) throws IllegalArgumentException { 146 if (codeString == null || "".equals(codeString)) 147 if (codeString == null || "".equals(codeString)) 148 return null; 149 if ("active".equals(codeString)) 150 return FlagStatus.ACTIVE; 151 if ("inactive".equals(codeString)) 152 return FlagStatus.INACTIVE; 153 if ("entered-in-error".equals(codeString)) 154 return FlagStatus.ENTEREDINERROR; 155 throw new IllegalArgumentException("Unknown FlagStatus code '" + codeString + "'"); 156 } 157 158 public Enumeration<FlagStatus> fromType(PrimitiveType<?> code) throws FHIRException { 159 if (code == null) 160 return null; 161 if (code.isEmpty()) 162 return new Enumeration<FlagStatus>(this, FlagStatus.NULL, code); 163 String codeString = code.asStringValue(); 164 if (codeString == null || "".equals(codeString)) 165 return new Enumeration<FlagStatus>(this, FlagStatus.NULL, code); 166 if ("active".equals(codeString)) 167 return new Enumeration<FlagStatus>(this, FlagStatus.ACTIVE, code); 168 if ("inactive".equals(codeString)) 169 return new Enumeration<FlagStatus>(this, FlagStatus.INACTIVE, code); 170 if ("entered-in-error".equals(codeString)) 171 return new Enumeration<FlagStatus>(this, FlagStatus.ENTEREDINERROR, code); 172 throw new FHIRException("Unknown FlagStatus code '" + codeString + "'"); 173 } 174 175 public String toCode(FlagStatus code) { 176 if (code == FlagStatus.NULL) 177 return null; 178 if (code == FlagStatus.ACTIVE) 179 return "active"; 180 if (code == FlagStatus.INACTIVE) 181 return "inactive"; 182 if (code == FlagStatus.ENTEREDINERROR) 183 return "entered-in-error"; 184 return "?"; 185 } 186 187 public String toSystem(FlagStatus code) { 188 return code.getSystem(); 189 } 190 } 191 192 /** 193 * Business identifiers assigned to this flag by the performer or other systems 194 * which remain constant as the resource is updated and propagates from server 195 * to server. 196 */ 197 @Child(name = "identifier", type = { 198 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 199 @Description(shortDefinition = "Business identifier", formalDefinition = "Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.") 200 protected List<Identifier> identifier; 201 202 /** 203 * Supports basic workflow. 204 */ 205 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 206 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "Supports basic workflow.") 207 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/flag-status") 208 protected Enumeration<FlagStatus> status; 209 210 /** 211 * Allows a flag to be divided into different categories like clinical, 212 * administrative etc. Intended to be used as a means of filtering which flags 213 * are displayed to particular user or in a given context. 214 */ 215 @Child(name = "category", type = { 216 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 217 @Description(shortDefinition = "Clinical, administrative, etc.", formalDefinition = "Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.") 218 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/flag-category") 219 protected List<CodeableConcept> category; 220 221 /** 222 * The coded value or textual component of the flag to display to the user. 223 */ 224 @Child(name = "code", type = { CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 225 @Description(shortDefinition = "Coded or textual message to display to user", formalDefinition = "The coded value or textual component of the flag to display to the user.") 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/flag-code") 227 protected CodeableConcept code; 228 229 /** 230 * The patient, location, group, organization, or practitioner etc. this is 231 * about record this flag is associated with. 232 */ 233 @Child(name = "subject", type = { Patient.class, Location.class, Group.class, Organization.class, Practitioner.class, 234 PlanDefinition.class, Medication.class, 235 Procedure.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 236 @Description(shortDefinition = "Who/What is flag about?", formalDefinition = "The patient, location, group, organization, or practitioner etc. this is about record this flag is associated with.") 237 protected Reference subject; 238 239 /** 240 * The actual object that is the target of the reference (The patient, location, 241 * group, organization, or practitioner etc. this is about record this flag is 242 * associated with.) 243 */ 244 protected Resource subjectTarget; 245 246 /** 247 * The period of time from the activation of the flag to inactivation of the 248 * flag. If the flag is active, the end of the period should be unspecified. 249 */ 250 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 251 @Description(shortDefinition = "Time period when flag is active", formalDefinition = "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.") 252 protected Period period; 253 254 /** 255 * This alert is only relevant during the encounter. 256 */ 257 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 258 @Description(shortDefinition = "Alert relevant during encounter", formalDefinition = "This alert is only relevant during the encounter.") 259 protected Reference encounter; 260 261 /** 262 * The actual object that is the target of the reference (This alert is only 263 * relevant during the encounter.) 264 */ 265 protected Encounter encounterTarget; 266 267 /** 268 * The person, organization or device that created the flag. 269 */ 270 @Child(name = "author", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 271 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 272 @Description(shortDefinition = "Flag creator", formalDefinition = "The person, organization or device that created the flag.") 273 protected Reference author; 274 275 /** 276 * The actual object that is the target of the reference (The person, 277 * organization or device that created the flag.) 278 */ 279 protected Resource authorTarget; 280 281 private static final long serialVersionUID = 163791439L; 282 283 /** 284 * Constructor 285 */ 286 public Flag() { 287 super(); 288 } 289 290 /** 291 * Constructor 292 */ 293 public Flag(Enumeration<FlagStatus> status, CodeableConcept code, Reference subject) { 294 super(); 295 this.status = status; 296 this.code = code; 297 this.subject = subject; 298 } 299 300 /** 301 * @return {@link #identifier} (Business identifiers assigned to this flag by 302 * the performer or other systems which remain constant as the resource 303 * is updated and propagates from server to server.) 304 */ 305 public List<Identifier> getIdentifier() { 306 if (this.identifier == null) 307 this.identifier = new ArrayList<Identifier>(); 308 return this.identifier; 309 } 310 311 /** 312 * @return Returns a reference to <code>this</code> for easy method chaining 313 */ 314 public Flag setIdentifier(List<Identifier> theIdentifier) { 315 this.identifier = theIdentifier; 316 return this; 317 } 318 319 public boolean hasIdentifier() { 320 if (this.identifier == null) 321 return false; 322 for (Identifier item : this.identifier) 323 if (!item.isEmpty()) 324 return true; 325 return false; 326 } 327 328 public Identifier addIdentifier() { // 3 329 Identifier t = new Identifier(); 330 if (this.identifier == null) 331 this.identifier = new ArrayList<Identifier>(); 332 this.identifier.add(t); 333 return t; 334 } 335 336 public Flag addIdentifier(Identifier t) { // 3 337 if (t == null) 338 return this; 339 if (this.identifier == null) 340 this.identifier = new ArrayList<Identifier>(); 341 this.identifier.add(t); 342 return this; 343 } 344 345 /** 346 * @return The first repetition of repeating field {@link #identifier}, creating 347 * it if it does not already exist 348 */ 349 public Identifier getIdentifierFirstRep() { 350 if (getIdentifier().isEmpty()) { 351 addIdentifier(); 352 } 353 return getIdentifier().get(0); 354 } 355 356 /** 357 * @return {@link #status} (Supports basic workflow.). This is the underlying 358 * object with id, value and extensions. The accessor "getStatus" gives 359 * direct access to the value 360 */ 361 public Enumeration<FlagStatus> getStatusElement() { 362 if (this.status == null) 363 if (Configuration.errorOnAutoCreate()) 364 throw new Error("Attempt to auto-create Flag.status"); 365 else if (Configuration.doAutoCreate()) 366 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); // bb 367 return this.status; 368 } 369 370 public boolean hasStatusElement() { 371 return this.status != null && !this.status.isEmpty(); 372 } 373 374 public boolean hasStatus() { 375 return this.status != null && !this.status.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #status} (Supports basic workflow.). This is the 380 * underlying object with id, value and extensions. The accessor 381 * "getStatus" gives direct access to the value 382 */ 383 public Flag setStatusElement(Enumeration<FlagStatus> value) { 384 this.status = value; 385 return this; 386 } 387 388 /** 389 * @return Supports basic workflow. 390 */ 391 public FlagStatus getStatus() { 392 return this.status == null ? null : this.status.getValue(); 393 } 394 395 /** 396 * @param value Supports basic workflow. 397 */ 398 public Flag setStatus(FlagStatus value) { 399 if (this.status == null) 400 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); 401 this.status.setValue(value); 402 return this; 403 } 404 405 /** 406 * @return {@link #category} (Allows a flag to be divided into different 407 * categories like clinical, administrative etc. Intended to be used as 408 * a means of filtering which flags are displayed to particular user or 409 * in a given context.) 410 */ 411 public List<CodeableConcept> getCategory() { 412 if (this.category == null) 413 this.category = new ArrayList<CodeableConcept>(); 414 return this.category; 415 } 416 417 /** 418 * @return Returns a reference to <code>this</code> for easy method chaining 419 */ 420 public Flag setCategory(List<CodeableConcept> theCategory) { 421 this.category = theCategory; 422 return this; 423 } 424 425 public boolean hasCategory() { 426 if (this.category == null) 427 return false; 428 for (CodeableConcept item : this.category) 429 if (!item.isEmpty()) 430 return true; 431 return false; 432 } 433 434 public CodeableConcept addCategory() { // 3 435 CodeableConcept t = new CodeableConcept(); 436 if (this.category == null) 437 this.category = new ArrayList<CodeableConcept>(); 438 this.category.add(t); 439 return t; 440 } 441 442 public Flag addCategory(CodeableConcept t) { // 3 443 if (t == null) 444 return this; 445 if (this.category == null) 446 this.category = new ArrayList<CodeableConcept>(); 447 this.category.add(t); 448 return this; 449 } 450 451 /** 452 * @return The first repetition of repeating field {@link #category}, creating 453 * it if it does not already exist 454 */ 455 public CodeableConcept getCategoryFirstRep() { 456 if (getCategory().isEmpty()) { 457 addCategory(); 458 } 459 return getCategory().get(0); 460 } 461 462 /** 463 * @return {@link #code} (The coded value or textual component of the flag to 464 * display to the user.) 465 */ 466 public CodeableConcept getCode() { 467 if (this.code == null) 468 if (Configuration.errorOnAutoCreate()) 469 throw new Error("Attempt to auto-create Flag.code"); 470 else if (Configuration.doAutoCreate()) 471 this.code = new CodeableConcept(); // cc 472 return this.code; 473 } 474 475 public boolean hasCode() { 476 return this.code != null && !this.code.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #code} (The coded value or textual component of the flag 481 * to display to the user.) 482 */ 483 public Flag setCode(CodeableConcept value) { 484 this.code = value; 485 return this; 486 } 487 488 /** 489 * @return {@link #subject} (The patient, location, group, organization, or 490 * practitioner etc. this is about record this flag is associated with.) 491 */ 492 public Reference getSubject() { 493 if (this.subject == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create Flag.subject"); 496 else if (Configuration.doAutoCreate()) 497 this.subject = new Reference(); // cc 498 return this.subject; 499 } 500 501 public boolean hasSubject() { 502 return this.subject != null && !this.subject.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #subject} (The patient, location, group, organization, or 507 * practitioner etc. this is about record this flag is associated 508 * with.) 509 */ 510 public Flag setSubject(Reference value) { 511 this.subject = value; 512 return this; 513 } 514 515 /** 516 * @return {@link #subject} The actual object that is the target of the 517 * reference. The reference library doesn't populate this, but you can 518 * use it to hold the resource if you resolve it. (The patient, 519 * location, group, organization, or practitioner etc. this is about 520 * record this flag is associated with.) 521 */ 522 public Resource getSubjectTarget() { 523 return this.subjectTarget; 524 } 525 526 /** 527 * @param value {@link #subject} The actual object that is the target of the 528 * reference. The reference library doesn't use these, but you can 529 * use it to hold the resource if you resolve it. (The patient, 530 * location, group, organization, or practitioner etc. this is 531 * about record this flag is associated with.) 532 */ 533 public Flag setSubjectTarget(Resource value) { 534 this.subjectTarget = value; 535 return this; 536 } 537 538 /** 539 * @return {@link #period} (The period of time from the activation of the flag 540 * to inactivation of the flag. If the flag is active, the end of the 541 * period should be unspecified.) 542 */ 543 public Period getPeriod() { 544 if (this.period == null) 545 if (Configuration.errorOnAutoCreate()) 546 throw new Error("Attempt to auto-create Flag.period"); 547 else if (Configuration.doAutoCreate()) 548 this.period = new Period(); // cc 549 return this.period; 550 } 551 552 public boolean hasPeriod() { 553 return this.period != null && !this.period.isEmpty(); 554 } 555 556 /** 557 * @param value {@link #period} (The period of time from the activation of the 558 * flag to inactivation of the flag. If the flag is active, the end 559 * of the period should be unspecified.) 560 */ 561 public Flag setPeriod(Period value) { 562 this.period = value; 563 return this; 564 } 565 566 /** 567 * @return {@link #encounter} (This alert is only relevant during the 568 * encounter.) 569 */ 570 public Reference getEncounter() { 571 if (this.encounter == null) 572 if (Configuration.errorOnAutoCreate()) 573 throw new Error("Attempt to auto-create Flag.encounter"); 574 else if (Configuration.doAutoCreate()) 575 this.encounter = new Reference(); // cc 576 return this.encounter; 577 } 578 579 public boolean hasEncounter() { 580 return this.encounter != null && !this.encounter.isEmpty(); 581 } 582 583 /** 584 * @param value {@link #encounter} (This alert is only relevant during the 585 * encounter.) 586 */ 587 public Flag setEncounter(Reference value) { 588 this.encounter = value; 589 return this; 590 } 591 592 /** 593 * @return {@link #encounter} The actual object that is the target of the 594 * reference. The reference library doesn't populate this, but you can 595 * use it to hold the resource if you resolve it. (This alert is only 596 * relevant during the encounter.) 597 */ 598 public Encounter getEncounterTarget() { 599 if (this.encounterTarget == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create Flag.encounter"); 602 else if (Configuration.doAutoCreate()) 603 this.encounterTarget = new Encounter(); // aa 604 return this.encounterTarget; 605 } 606 607 /** 608 * @param value {@link #encounter} The actual object that is the target of the 609 * reference. The reference library doesn't use these, but you can 610 * use it to hold the resource if you resolve it. (This alert is 611 * only relevant during the encounter.) 612 */ 613 public Flag setEncounterTarget(Encounter value) { 614 this.encounterTarget = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #author} (The person, organization or device that created the 620 * flag.) 621 */ 622 public Reference getAuthor() { 623 if (this.author == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create Flag.author"); 626 else if (Configuration.doAutoCreate()) 627 this.author = new Reference(); // cc 628 return this.author; 629 } 630 631 public boolean hasAuthor() { 632 return this.author != null && !this.author.isEmpty(); 633 } 634 635 /** 636 * @param value {@link #author} (The person, organization or device that created 637 * the flag.) 638 */ 639 public Flag setAuthor(Reference value) { 640 this.author = value; 641 return this; 642 } 643 644 /** 645 * @return {@link #author} The actual object that is the target of the 646 * reference. The reference library doesn't populate this, but you can 647 * use it to hold the resource if you resolve it. (The person, 648 * organization or device that created the flag.) 649 */ 650 public Resource getAuthorTarget() { 651 return this.authorTarget; 652 } 653 654 /** 655 * @param value {@link #author} The actual object that is the target of the 656 * reference. The reference library doesn't use these, but you can 657 * use it to hold the resource if you resolve it. (The person, 658 * organization or device that created the flag.) 659 */ 660 public Flag setAuthorTarget(Resource value) { 661 this.authorTarget = value; 662 return this; 663 } 664 665 protected void listChildren(List<Property> children) { 666 super.listChildren(children); 667 children.add(new Property("identifier", "Identifier", 668 "Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 669 0, java.lang.Integer.MAX_VALUE, identifier)); 670 children.add(new Property("status", "code", "Supports basic workflow.", 0, 1, status)); 671 children.add(new Property("category", "CodeableConcept", 672 "Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 673 0, java.lang.Integer.MAX_VALUE, category)); 674 children.add(new Property("code", "CodeableConcept", 675 "The coded value or textual component of the flag to display to the user.", 0, 1, code)); 676 children.add(new Property("subject", 677 "Reference(Patient|Location|Group|Organization|Practitioner|PlanDefinition|Medication|Procedure)", 678 "The patient, location, group, organization, or practitioner etc. this is about record this flag is associated with.", 679 0, 1, subject)); 680 children.add(new Property("period", "Period", 681 "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 682 0, 1, period)); 683 children.add(new Property("encounter", "Reference(Encounter)", "This alert is only relevant during the encounter.", 684 0, 1, encounter)); 685 children.add(new Property("author", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole)", 686 "The person, organization or device that created the flag.", 0, 1, author)); 687 } 688 689 @Override 690 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 691 switch (_hash) { 692 case -1618432855: 693 /* identifier */ return new Property("identifier", "Identifier", 694 "Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 695 0, java.lang.Integer.MAX_VALUE, identifier); 696 case -892481550: 697 /* status */ return new Property("status", "code", "Supports basic workflow.", 0, 1, status); 698 case 50511102: 699 /* category */ return new Property("category", "CodeableConcept", 700 "Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 701 0, java.lang.Integer.MAX_VALUE, category); 702 case 3059181: 703 /* code */ return new Property("code", "CodeableConcept", 704 "The coded value or textual component of the flag to display to the user.", 0, 1, code); 705 case -1867885268: 706 /* subject */ return new Property("subject", 707 "Reference(Patient|Location|Group|Organization|Practitioner|PlanDefinition|Medication|Procedure)", 708 "The patient, location, group, organization, or practitioner etc. this is about record this flag is associated with.", 709 0, 1, subject); 710 case -991726143: 711 /* period */ return new Property("period", "Period", 712 "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 713 0, 1, period); 714 case 1524132147: 715 /* encounter */ return new Property("encounter", "Reference(Encounter)", 716 "This alert is only relevant during the encounter.", 0, 1, encounter); 717 case -1406328437: 718 /* author */ return new Property("author", "Reference(Device|Organization|Patient|Practitioner|PractitionerRole)", 719 "The person, organization or device that created the flag.", 0, 1, author); 720 default: 721 return super.getNamedProperty(_hash, _name, _checkValid); 722 } 723 724 } 725 726 @Override 727 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 728 switch (hash) { 729 case -1618432855: 730 /* identifier */ return this.identifier == null ? new Base[0] 731 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 732 case -892481550: 733 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<FlagStatus> 734 case 50511102: 735 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 736 case 3059181: 737 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 738 case -1867885268: 739 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 740 case -991726143: 741 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 742 case 1524132147: 743 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 744 case -1406328437: 745 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 746 default: 747 return super.getProperty(hash, name, checkValid); 748 } 749 750 } 751 752 @Override 753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 754 switch (hash) { 755 case -1618432855: // identifier 756 this.getIdentifier().add(castToIdentifier(value)); // Identifier 757 return value; 758 case -892481550: // status 759 value = new FlagStatusEnumFactory().fromType(castToCode(value)); 760 this.status = (Enumeration) value; // Enumeration<FlagStatus> 761 return value; 762 case 50511102: // category 763 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 764 return value; 765 case 3059181: // code 766 this.code = castToCodeableConcept(value); // CodeableConcept 767 return value; 768 case -1867885268: // subject 769 this.subject = castToReference(value); // Reference 770 return value; 771 case -991726143: // period 772 this.period = castToPeriod(value); // Period 773 return value; 774 case 1524132147: // encounter 775 this.encounter = castToReference(value); // Reference 776 return value; 777 case -1406328437: // author 778 this.author = castToReference(value); // Reference 779 return value; 780 default: 781 return super.setProperty(hash, name, value); 782 } 783 784 } 785 786 @Override 787 public Base setProperty(String name, Base value) throws FHIRException { 788 if (name.equals("identifier")) { 789 this.getIdentifier().add(castToIdentifier(value)); 790 } else if (name.equals("status")) { 791 value = new FlagStatusEnumFactory().fromType(castToCode(value)); 792 this.status = (Enumeration) value; // Enumeration<FlagStatus> 793 } else if (name.equals("category")) { 794 this.getCategory().add(castToCodeableConcept(value)); 795 } else if (name.equals("code")) { 796 this.code = castToCodeableConcept(value); // CodeableConcept 797 } else if (name.equals("subject")) { 798 this.subject = castToReference(value); // Reference 799 } else if (name.equals("period")) { 800 this.period = castToPeriod(value); // Period 801 } else if (name.equals("encounter")) { 802 this.encounter = castToReference(value); // Reference 803 } else if (name.equals("author")) { 804 this.author = castToReference(value); // Reference 805 } else 806 return super.setProperty(name, value); 807 return value; 808 } 809 810 @Override 811 public void removeChild(String name, Base value) throws FHIRException { 812 if (name.equals("identifier")) { 813 this.getIdentifier().remove(castToIdentifier(value)); 814 } else if (name.equals("status")) { 815 this.status = null; 816 } else if (name.equals("category")) { 817 this.getCategory().remove(castToCodeableConcept(value)); 818 } else if (name.equals("code")) { 819 this.code = null; 820 } else if (name.equals("subject")) { 821 this.subject = null; 822 } else if (name.equals("period")) { 823 this.period = null; 824 } else if (name.equals("encounter")) { 825 this.encounter = null; 826 } else if (name.equals("author")) { 827 this.author = null; 828 } else 829 super.removeChild(name, value); 830 831 } 832 833 @Override 834 public Base makeProperty(int hash, String name) throws FHIRException { 835 switch (hash) { 836 case -1618432855: 837 return addIdentifier(); 838 case -892481550: 839 return getStatusElement(); 840 case 50511102: 841 return addCategory(); 842 case 3059181: 843 return getCode(); 844 case -1867885268: 845 return getSubject(); 846 case -991726143: 847 return getPeriod(); 848 case 1524132147: 849 return getEncounter(); 850 case -1406328437: 851 return getAuthor(); 852 default: 853 return super.makeProperty(hash, name); 854 } 855 856 } 857 858 @Override 859 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 860 switch (hash) { 861 case -1618432855: 862 /* identifier */ return new String[] { "Identifier" }; 863 case -892481550: 864 /* status */ return new String[] { "code" }; 865 case 50511102: 866 /* category */ return new String[] { "CodeableConcept" }; 867 case 3059181: 868 /* code */ return new String[] { "CodeableConcept" }; 869 case -1867885268: 870 /* subject */ return new String[] { "Reference" }; 871 case -991726143: 872 /* period */ return new String[] { "Period" }; 873 case 1524132147: 874 /* encounter */ return new String[] { "Reference" }; 875 case -1406328437: 876 /* author */ return new String[] { "Reference" }; 877 default: 878 return super.getTypesForProperty(hash, name); 879 } 880 881 } 882 883 @Override 884 public Base addChild(String name) throws FHIRException { 885 if (name.equals("identifier")) { 886 return addIdentifier(); 887 } else if (name.equals("status")) { 888 throw new FHIRException("Cannot call addChild on a singleton property Flag.status"); 889 } else if (name.equals("category")) { 890 return addCategory(); 891 } else if (name.equals("code")) { 892 this.code = new CodeableConcept(); 893 return this.code; 894 } else if (name.equals("subject")) { 895 this.subject = new Reference(); 896 return this.subject; 897 } else if (name.equals("period")) { 898 this.period = new Period(); 899 return this.period; 900 } else if (name.equals("encounter")) { 901 this.encounter = new Reference(); 902 return this.encounter; 903 } else if (name.equals("author")) { 904 this.author = new Reference(); 905 return this.author; 906 } else 907 return super.addChild(name); 908 } 909 910 public String fhirType() { 911 return "Flag"; 912 913 } 914 915 public Flag copy() { 916 Flag dst = new Flag(); 917 copyValues(dst); 918 return dst; 919 } 920 921 public void copyValues(Flag dst) { 922 super.copyValues(dst); 923 if (identifier != null) { 924 dst.identifier = new ArrayList<Identifier>(); 925 for (Identifier i : identifier) 926 dst.identifier.add(i.copy()); 927 } 928 ; 929 dst.status = status == null ? null : status.copy(); 930 if (category != null) { 931 dst.category = new ArrayList<CodeableConcept>(); 932 for (CodeableConcept i : category) 933 dst.category.add(i.copy()); 934 } 935 ; 936 dst.code = code == null ? null : code.copy(); 937 dst.subject = subject == null ? null : subject.copy(); 938 dst.period = period == null ? null : period.copy(); 939 dst.encounter = encounter == null ? null : encounter.copy(); 940 dst.author = author == null ? null : author.copy(); 941 } 942 943 protected Flag typedCopy() { 944 return copy(); 945 } 946 947 @Override 948 public boolean equalsDeep(Base other_) { 949 if (!super.equalsDeep(other_)) 950 return false; 951 if (!(other_ instanceof Flag)) 952 return false; 953 Flag o = (Flag) other_; 954 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 955 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 956 && compareDeep(subject, o.subject, true) && compareDeep(period, o.period, true) 957 && compareDeep(encounter, o.encounter, true) && compareDeep(author, o.author, true); 958 } 959 960 @Override 961 public boolean equalsShallow(Base other_) { 962 if (!super.equalsShallow(other_)) 963 return false; 964 if (!(other_ instanceof Flag)) 965 return false; 966 Flag o = (Flag) other_; 967 return compareValues(status, o.status, true); 968 } 969 970 public boolean isEmpty() { 971 return super.isEmpty() 972 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category, code, subject, period, encounter, author); 973 } 974 975 @Override 976 public ResourceType getResourceType() { 977 return ResourceType.Flag; 978 } 979 980 /** 981 * Search parameter: <b>date</b> 982 * <p> 983 * Description: <b>Time period when flag is active</b><br> 984 * Type: <b>date</b><br> 985 * Path: <b>Flag.period</b><br> 986 * </p> 987 */ 988 @SearchParamDefinition(name = "date", path = "Flag.period", description = "Time period when flag is active", type = "date") 989 public static final String SP_DATE = "date"; 990 /** 991 * <b>Fluent Client</b> search parameter constant for <b>date</b> 992 * <p> 993 * Description: <b>Time period when flag is active</b><br> 994 * Type: <b>date</b><br> 995 * Path: <b>Flag.period</b><br> 996 * </p> 997 */ 998 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 999 SP_DATE); 1000 1001 /** 1002 * Search parameter: <b>identifier</b> 1003 * <p> 1004 * Description: <b>Business identifier</b><br> 1005 * Type: <b>token</b><br> 1006 * Path: <b>Flag.identifier</b><br> 1007 * </p> 1008 */ 1009 @SearchParamDefinition(name = "identifier", path = "Flag.identifier", description = "Business identifier", type = "token") 1010 public static final String SP_IDENTIFIER = "identifier"; 1011 /** 1012 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1013 * <p> 1014 * Description: <b>Business identifier</b><br> 1015 * Type: <b>token</b><br> 1016 * Path: <b>Flag.identifier</b><br> 1017 * </p> 1018 */ 1019 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1020 SP_IDENTIFIER); 1021 1022 /** 1023 * Search parameter: <b>subject</b> 1024 * <p> 1025 * Description: <b>The identity of a subject to list flags for</b><br> 1026 * Type: <b>reference</b><br> 1027 * Path: <b>Flag.subject</b><br> 1028 * </p> 1029 */ 1030 @SearchParamDefinition(name = "subject", path = "Flag.subject", description = "The identity of a subject to list flags for", type = "reference", target = { 1031 Group.class, Location.class, Medication.class, Organization.class, Patient.class, PlanDefinition.class, 1032 Practitioner.class, Procedure.class }) 1033 public static final String SP_SUBJECT = "subject"; 1034 /** 1035 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1036 * <p> 1037 * Description: <b>The identity of a subject to list flags for</b><br> 1038 * Type: <b>reference</b><br> 1039 * Path: <b>Flag.subject</b><br> 1040 * </p> 1041 */ 1042 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1043 SP_SUBJECT); 1044 1045 /** 1046 * Constant for fluent queries to be used to add include statements. Specifies 1047 * the path value of "<b>Flag:subject</b>". 1048 */ 1049 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Flag:subject") 1050 .toLocked(); 1051 1052 /** 1053 * Search parameter: <b>patient</b> 1054 * <p> 1055 * Description: <b>The identity of a subject to list flags for</b><br> 1056 * Type: <b>reference</b><br> 1057 * Path: <b>Flag.subject</b><br> 1058 * </p> 1059 */ 1060 @SearchParamDefinition(name = "patient", path = "Flag.subject.where(resolve() is Patient)", description = "The identity of a subject to list flags for", type = "reference", providesMembershipIn = { 1061 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 1062 public static final String SP_PATIENT = "patient"; 1063 /** 1064 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1065 * <p> 1066 * Description: <b>The identity of a subject to list flags for</b><br> 1067 * Type: <b>reference</b><br> 1068 * Path: <b>Flag.subject</b><br> 1069 * </p> 1070 */ 1071 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1072 SP_PATIENT); 1073 1074 /** 1075 * Constant for fluent queries to be used to add include statements. Specifies 1076 * the path value of "<b>Flag:patient</b>". 1077 */ 1078 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Flag:patient") 1079 .toLocked(); 1080 1081 /** 1082 * Search parameter: <b>author</b> 1083 * <p> 1084 * Description: <b>Flag creator</b><br> 1085 * Type: <b>reference</b><br> 1086 * Path: <b>Flag.author</b><br> 1087 * </p> 1088 */ 1089 @SearchParamDefinition(name = "author", path = "Flag.author", description = "Flag creator", type = "reference", providesMembershipIn = { 1090 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 1091 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 1092 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class }) 1093 public static final String SP_AUTHOR = "author"; 1094 /** 1095 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1096 * <p> 1097 * Description: <b>Flag creator</b><br> 1098 * Type: <b>reference</b><br> 1099 * Path: <b>Flag.author</b><br> 1100 * </p> 1101 */ 1102 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1103 SP_AUTHOR); 1104 1105 /** 1106 * Constant for fluent queries to be used to add include statements. Specifies 1107 * the path value of "<b>Flag:author</b>". 1108 */ 1109 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Flag:author") 1110 .toLocked(); 1111 1112 /** 1113 * Search parameter: <b>encounter</b> 1114 * <p> 1115 * Description: <b>Alert relevant during encounter</b><br> 1116 * Type: <b>reference</b><br> 1117 * Path: <b>Flag.encounter</b><br> 1118 * </p> 1119 */ 1120 @SearchParamDefinition(name = "encounter", path = "Flag.encounter", description = "Alert relevant during encounter", type = "reference", target = { 1121 Encounter.class }) 1122 public static final String SP_ENCOUNTER = "encounter"; 1123 /** 1124 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1125 * <p> 1126 * Description: <b>Alert relevant during encounter</b><br> 1127 * Type: <b>reference</b><br> 1128 * Path: <b>Flag.encounter</b><br> 1129 * </p> 1130 */ 1131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1132 SP_ENCOUNTER); 1133 1134 /** 1135 * Constant for fluent queries to be used to add include statements. Specifies 1136 * the path value of "<b>Flag:encounter</b>". 1137 */ 1138 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 1139 "Flag:encounter").toLocked(); 1140 1141}