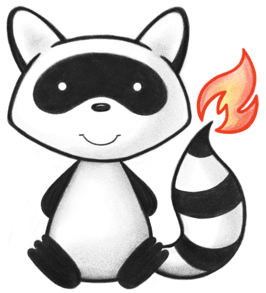
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Represents a defined collection of entities that may be discussed or acted 048 * upon collectively but which are not expected to act collectively, and are not 049 * formally or legally recognized; i.e. a collection of entities that isn't an 050 * Organization. 051 */ 052@ResourceDef(name = "Group", profile = "http://hl7.org/fhir/StructureDefinition/Group") 053public class Group extends DomainResource { 054 055 public enum GroupType { 056 /** 057 * Group contains "person" Patient resources. 058 */ 059 PERSON, 060 /** 061 * Group contains "animal" Patient resources. 062 */ 063 ANIMAL, 064 /** 065 * Group contains healthcare practitioner resources (Practitioner or 066 * PractitionerRole). 067 */ 068 PRACTITIONER, 069 /** 070 * Group contains Device resources. 071 */ 072 DEVICE, 073 /** 074 * Group contains Medication resources. 075 */ 076 MEDICATION, 077 /** 078 * Group contains Substance resources. 079 */ 080 SUBSTANCE, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static GroupType fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("person".equals(codeString)) 090 return PERSON; 091 if ("animal".equals(codeString)) 092 return ANIMAL; 093 if ("practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("device".equals(codeString)) 096 return DEVICE; 097 if ("medication".equals(codeString)) 098 return MEDICATION; 099 if ("substance".equals(codeString)) 100 return SUBSTANCE; 101 if (Configuration.isAcceptInvalidEnums()) 102 return null; 103 else 104 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 105 } 106 107 public String toCode() { 108 switch (this) { 109 case PERSON: 110 return "person"; 111 case ANIMAL: 112 return "animal"; 113 case PRACTITIONER: 114 return "practitioner"; 115 case DEVICE: 116 return "device"; 117 case MEDICATION: 118 return "medication"; 119 case SUBSTANCE: 120 return "substance"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getSystem() { 129 switch (this) { 130 case PERSON: 131 return "http://hl7.org/fhir/group-type"; 132 case ANIMAL: 133 return "http://hl7.org/fhir/group-type"; 134 case PRACTITIONER: 135 return "http://hl7.org/fhir/group-type"; 136 case DEVICE: 137 return "http://hl7.org/fhir/group-type"; 138 case MEDICATION: 139 return "http://hl7.org/fhir/group-type"; 140 case SUBSTANCE: 141 return "http://hl7.org/fhir/group-type"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149 public String getDefinition() { 150 switch (this) { 151 case PERSON: 152 return "Group contains \"person\" Patient resources."; 153 case ANIMAL: 154 return "Group contains \"animal\" Patient resources."; 155 case PRACTITIONER: 156 return "Group contains healthcare practitioner resources (Practitioner or PractitionerRole)."; 157 case DEVICE: 158 return "Group contains Device resources."; 159 case MEDICATION: 160 return "Group contains Medication resources."; 161 case SUBSTANCE: 162 return "Group contains Substance resources."; 163 case NULL: 164 return null; 165 default: 166 return "?"; 167 } 168 } 169 170 public String getDisplay() { 171 switch (this) { 172 case PERSON: 173 return "Person"; 174 case ANIMAL: 175 return "Animal"; 176 case PRACTITIONER: 177 return "Practitioner"; 178 case DEVICE: 179 return "Device"; 180 case MEDICATION: 181 return "Medication"; 182 case SUBSTANCE: 183 return "Substance"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 } 191 192 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 193 public GroupType fromCode(String codeString) throws IllegalArgumentException { 194 if (codeString == null || "".equals(codeString)) 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("person".equals(codeString)) 198 return GroupType.PERSON; 199 if ("animal".equals(codeString)) 200 return GroupType.ANIMAL; 201 if ("practitioner".equals(codeString)) 202 return GroupType.PRACTITIONER; 203 if ("device".equals(codeString)) 204 return GroupType.DEVICE; 205 if ("medication".equals(codeString)) 206 return GroupType.MEDICATION; 207 if ("substance".equals(codeString)) 208 return GroupType.SUBSTANCE; 209 throw new IllegalArgumentException("Unknown GroupType code '" + codeString + "'"); 210 } 211 212 public Enumeration<GroupType> fromType(PrimitiveType<?> code) throws FHIRException { 213 if (code == null) 214 return null; 215 if (code.isEmpty()) 216 return new Enumeration<GroupType>(this, GroupType.NULL, code); 217 String codeString = code.asStringValue(); 218 if (codeString == null || "".equals(codeString)) 219 return new Enumeration<GroupType>(this, GroupType.NULL, code); 220 if ("person".equals(codeString)) 221 return new Enumeration<GroupType>(this, GroupType.PERSON, code); 222 if ("animal".equals(codeString)) 223 return new Enumeration<GroupType>(this, GroupType.ANIMAL, code); 224 if ("practitioner".equals(codeString)) 225 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER, code); 226 if ("device".equals(codeString)) 227 return new Enumeration<GroupType>(this, GroupType.DEVICE, code); 228 if ("medication".equals(codeString)) 229 return new Enumeration<GroupType>(this, GroupType.MEDICATION, code); 230 if ("substance".equals(codeString)) 231 return new Enumeration<GroupType>(this, GroupType.SUBSTANCE, code); 232 throw new FHIRException("Unknown GroupType code '" + codeString + "'"); 233 } 234 235 public String toCode(GroupType code) { 236 if (code == GroupType.NULL) 237 return null; 238 if (code == GroupType.PERSON) 239 return "person"; 240 if (code == GroupType.ANIMAL) 241 return "animal"; 242 if (code == GroupType.PRACTITIONER) 243 return "practitioner"; 244 if (code == GroupType.DEVICE) 245 return "device"; 246 if (code == GroupType.MEDICATION) 247 return "medication"; 248 if (code == GroupType.SUBSTANCE) 249 return "substance"; 250 return "?"; 251 } 252 253 public String toSystem(GroupType code) { 254 return code.getSystem(); 255 } 256 } 257 258 @Block() 259 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 260 /** 261 * A code that identifies the kind of trait being asserted. 262 */ 263 @Child(name = "code", type = { 264 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 265 @Description(shortDefinition = "Kind of characteristic", formalDefinition = "A code that identifies the kind of trait being asserted.") 266 protected CodeableConcept code; 267 268 /** 269 * The value of the trait that holds (or does not hold - see 'exclude') for 270 * members of the group. 271 */ 272 @Child(name = "value", type = { CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, 273 Reference.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 274 @Description(shortDefinition = "Value held by characteristic", formalDefinition = "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.") 275 protected Type value; 276 277 /** 278 * If true, indicates the characteristic is one that is NOT held by members of 279 * the group. 280 */ 281 @Child(name = "exclude", type = { 282 BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = false) 283 @Description(shortDefinition = "Group includes or excludes", formalDefinition = "If true, indicates the characteristic is one that is NOT held by members of the group.") 284 protected BooleanType exclude; 285 286 /** 287 * The period over which the characteristic is tested; e.g. the patient had an 288 * operation during the month of June. 289 */ 290 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 291 @Description(shortDefinition = "Period over which characteristic is tested", formalDefinition = "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.") 292 protected Period period; 293 294 private static final long serialVersionUID = -1000688967L; 295 296 /** 297 * Constructor 298 */ 299 public GroupCharacteristicComponent() { 300 super(); 301 } 302 303 /** 304 * Constructor 305 */ 306 public GroupCharacteristicComponent(CodeableConcept code, Type value, BooleanType exclude) { 307 super(); 308 this.code = code; 309 this.value = value; 310 this.exclude = exclude; 311 } 312 313 /** 314 * @return {@link #code} (A code that identifies the kind of trait being 315 * asserted.) 316 */ 317 public CodeableConcept getCode() { 318 if (this.code == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 321 else if (Configuration.doAutoCreate()) 322 this.code = new CodeableConcept(); // cc 323 return this.code; 324 } 325 326 public boolean hasCode() { 327 return this.code != null && !this.code.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #code} (A code that identifies the kind of trait being 332 * asserted.) 333 */ 334 public GroupCharacteristicComponent setCode(CodeableConcept value) { 335 this.code = value; 336 return this; 337 } 338 339 /** 340 * @return {@link #value} (The value of the trait that holds (or does not hold - 341 * see 'exclude') for members of the group.) 342 */ 343 public Type getValue() { 344 return this.value; 345 } 346 347 /** 348 * @return {@link #value} (The value of the trait that holds (or does not hold - 349 * see 'exclude') for members of the group.) 350 */ 351 public CodeableConcept getValueCodeableConcept() throws FHIRException { 352 if (this.value == null) 353 this.value = new CodeableConcept(); 354 if (!(this.value instanceof CodeableConcept)) 355 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 356 + this.value.getClass().getName() + " was encountered"); 357 return (CodeableConcept) this.value; 358 } 359 360 public boolean hasValueCodeableConcept() { 361 return this != null && this.value instanceof CodeableConcept; 362 } 363 364 /** 365 * @return {@link #value} (The value of the trait that holds (or does not hold - 366 * see 'exclude') for members of the group.) 367 */ 368 public BooleanType getValueBooleanType() throws FHIRException { 369 if (this.value == null) 370 this.value = new BooleanType(); 371 if (!(this.value instanceof BooleanType)) 372 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 373 + this.value.getClass().getName() + " was encountered"); 374 return (BooleanType) this.value; 375 } 376 377 public boolean hasValueBooleanType() { 378 return this != null && this.value instanceof BooleanType; 379 } 380 381 /** 382 * @return {@link #value} (The value of the trait that holds (or does not hold - 383 * see 'exclude') for members of the group.) 384 */ 385 public Quantity getValueQuantity() throws FHIRException { 386 if (this.value == null) 387 this.value = new Quantity(); 388 if (!(this.value instanceof Quantity)) 389 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 390 + " was encountered"); 391 return (Quantity) this.value; 392 } 393 394 public boolean hasValueQuantity() { 395 return this != null && this.value instanceof Quantity; 396 } 397 398 /** 399 * @return {@link #value} (The value of the trait that holds (or does not hold - 400 * see 'exclude') for members of the group.) 401 */ 402 public Range getValueRange() throws FHIRException { 403 if (this.value == null) 404 this.value = new Range(); 405 if (!(this.value instanceof Range)) 406 throw new FHIRException( 407 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 408 return (Range) this.value; 409 } 410 411 public boolean hasValueRange() { 412 return this != null && this.value instanceof Range; 413 } 414 415 /** 416 * @return {@link #value} (The value of the trait that holds (or does not hold - 417 * see 'exclude') for members of the group.) 418 */ 419 public Reference getValueReference() throws FHIRException { 420 if (this.value == null) 421 this.value = new Reference(); 422 if (!(this.value instanceof Reference)) 423 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.value.getClass().getName() 424 + " was encountered"); 425 return (Reference) this.value; 426 } 427 428 public boolean hasValueReference() { 429 return this != null && this.value instanceof Reference; 430 } 431 432 public boolean hasValue() { 433 return this.value != null && !this.value.isEmpty(); 434 } 435 436 /** 437 * @param value {@link #value} (The value of the trait that holds (or does not 438 * hold - see 'exclude') for members of the group.) 439 */ 440 public GroupCharacteristicComponent setValue(Type value) { 441 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType 442 || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 443 throw new Error("Not the right type for Group.characteristic.value[x]: " + value.fhirType()); 444 this.value = value; 445 return this; 446 } 447 448 /** 449 * @return {@link #exclude} (If true, indicates the characteristic is one that 450 * is NOT held by members of the group.). This is the underlying object 451 * with id, value and extensions. The accessor "getExclude" gives direct 452 * access to the value 453 */ 454 public BooleanType getExcludeElement() { 455 if (this.exclude == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 458 else if (Configuration.doAutoCreate()) 459 this.exclude = new BooleanType(); // bb 460 return this.exclude; 461 } 462 463 public boolean hasExcludeElement() { 464 return this.exclude != null && !this.exclude.isEmpty(); 465 } 466 467 public boolean hasExclude() { 468 return this.exclude != null && !this.exclude.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #exclude} (If true, indicates the characteristic is one 473 * that is NOT held by members of the group.). This is the 474 * underlying object with id, value and extensions. The accessor 475 * "getExclude" gives direct access to the value 476 */ 477 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 478 this.exclude = value; 479 return this; 480 } 481 482 /** 483 * @return If true, indicates the characteristic is one that is NOT held by 484 * members of the group. 485 */ 486 public boolean getExclude() { 487 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 488 } 489 490 /** 491 * @param value If true, indicates the characteristic is one that is NOT held by 492 * members of the group. 493 */ 494 public GroupCharacteristicComponent setExclude(boolean value) { 495 if (this.exclude == null) 496 this.exclude = new BooleanType(); 497 this.exclude.setValue(value); 498 return this; 499 } 500 501 /** 502 * @return {@link #period} (The period over which the characteristic is tested; 503 * e.g. the patient had an operation during the month of June.) 504 */ 505 public Period getPeriod() { 506 if (this.period == null) 507 if (Configuration.errorOnAutoCreate()) 508 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 509 else if (Configuration.doAutoCreate()) 510 this.period = new Period(); // cc 511 return this.period; 512 } 513 514 public boolean hasPeriod() { 515 return this.period != null && !this.period.isEmpty(); 516 } 517 518 /** 519 * @param value {@link #period} (The period over which the characteristic is 520 * tested; e.g. the patient had an operation during the month of 521 * June.) 522 */ 523 public GroupCharacteristicComponent setPeriod(Period value) { 524 this.period = value; 525 return this; 526 } 527 528 protected void listChildren(List<Property> children) { 529 super.listChildren(children); 530 children.add(new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 531 0, 1, code)); 532 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 533 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 534 value)); 535 children.add(new Property("exclude", "boolean", 536 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude)); 537 children.add(new Property("period", "Period", 538 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 539 0, 1, period)); 540 } 541 542 @Override 543 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 544 switch (_hash) { 545 case 3059181: 546 /* code */ return new Property("code", "CodeableConcept", 547 "A code that identifies the kind of trait being asserted.", 0, 1, code); 548 case -1410166417: 549 /* value[x] */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 550 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 551 value); 552 case 111972721: 553 /* value */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 554 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 555 value); 556 case 924902896: 557 /* valueCodeableConcept */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 558 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 559 value); 560 case 733421943: 561 /* valueBoolean */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 562 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 563 value); 564 case -2029823716: 565 /* valueQuantity */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 566 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 567 value); 568 case 2030761548: 569 /* valueRange */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 570 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 571 value); 572 case 1755241690: 573 /* valueReference */ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", 574 "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, 575 value); 576 case -1321148966: 577 /* exclude */ return new Property("exclude", "boolean", 578 "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude); 579 case -991726143: 580 /* period */ return new Property("period", "Period", 581 "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 582 0, 1, period); 583 default: 584 return super.getNamedProperty(_hash, _name, _checkValid); 585 } 586 587 } 588 589 @Override 590 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 591 switch (hash) { 592 case 3059181: 593 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 594 case 111972721: 595 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 596 case -1321148966: 597 /* exclude */ return this.exclude == null ? new Base[0] : new Base[] { this.exclude }; // BooleanType 598 case -991726143: 599 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 600 default: 601 return super.getProperty(hash, name, checkValid); 602 } 603 604 } 605 606 @Override 607 public Base setProperty(int hash, String name, Base value) throws FHIRException { 608 switch (hash) { 609 case 3059181: // code 610 this.code = castToCodeableConcept(value); // CodeableConcept 611 return value; 612 case 111972721: // value 613 this.value = castToType(value); // Type 614 return value; 615 case -1321148966: // exclude 616 this.exclude = castToBoolean(value); // BooleanType 617 return value; 618 case -991726143: // period 619 this.period = castToPeriod(value); // Period 620 return value; 621 default: 622 return super.setProperty(hash, name, value); 623 } 624 625 } 626 627 @Override 628 public Base setProperty(String name, Base value) throws FHIRException { 629 if (name.equals("code")) { 630 this.code = castToCodeableConcept(value); // CodeableConcept 631 } else if (name.equals("value[x]")) { 632 this.value = castToType(value); // Type 633 } else if (name.equals("exclude")) { 634 this.exclude = castToBoolean(value); // BooleanType 635 } else if (name.equals("period")) { 636 this.period = castToPeriod(value); // Period 637 } else 638 return super.setProperty(name, value); 639 return value; 640 } 641 642 @Override 643 public void removeChild(String name, Base value) throws FHIRException { 644 if (name.equals("code")) { 645 this.code = null; 646 } else if (name.equals("value[x]")) { 647 this.value = null; 648 } else if (name.equals("exclude")) { 649 this.exclude = null; 650 } else if (name.equals("period")) { 651 this.period = null; 652 } else 653 super.removeChild(name, value); 654 655 } 656 657 @Override 658 public Base makeProperty(int hash, String name) throws FHIRException { 659 switch (hash) { 660 case 3059181: 661 return getCode(); 662 case -1410166417: 663 return getValue(); 664 case 111972721: 665 return getValue(); 666 case -1321148966: 667 return getExcludeElement(); 668 case -991726143: 669 return getPeriod(); 670 default: 671 return super.makeProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 678 switch (hash) { 679 case 3059181: 680 /* code */ return new String[] { "CodeableConcept" }; 681 case 111972721: 682 /* value */ return new String[] { "CodeableConcept", "boolean", "Quantity", "Range", "Reference" }; 683 case -1321148966: 684 /* exclude */ return new String[] { "boolean" }; 685 case -991726143: 686 /* period */ return new String[] { "Period" }; 687 default: 688 return super.getTypesForProperty(hash, name); 689 } 690 691 } 692 693 @Override 694 public Base addChild(String name) throws FHIRException { 695 if (name.equals("code")) { 696 this.code = new CodeableConcept(); 697 return this.code; 698 } else if (name.equals("valueCodeableConcept")) { 699 this.value = new CodeableConcept(); 700 return this.value; 701 } else if (name.equals("valueBoolean")) { 702 this.value = new BooleanType(); 703 return this.value; 704 } else if (name.equals("valueQuantity")) { 705 this.value = new Quantity(); 706 return this.value; 707 } else if (name.equals("valueRange")) { 708 this.value = new Range(); 709 return this.value; 710 } else if (name.equals("valueReference")) { 711 this.value = new Reference(); 712 return this.value; 713 } else if (name.equals("exclude")) { 714 throw new FHIRException("Cannot call addChild on a singleton property Group.exclude"); 715 } else if (name.equals("period")) { 716 this.period = new Period(); 717 return this.period; 718 } else 719 return super.addChild(name); 720 } 721 722 public GroupCharacteristicComponent copy() { 723 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 724 copyValues(dst); 725 return dst; 726 } 727 728 public void copyValues(GroupCharacteristicComponent dst) { 729 super.copyValues(dst); 730 dst.code = code == null ? null : code.copy(); 731 dst.value = value == null ? null : value.copy(); 732 dst.exclude = exclude == null ? null : exclude.copy(); 733 dst.period = period == null ? null : period.copy(); 734 } 735 736 @Override 737 public boolean equalsDeep(Base other_) { 738 if (!super.equalsDeep(other_)) 739 return false; 740 if (!(other_ instanceof GroupCharacteristicComponent)) 741 return false; 742 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 743 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 744 && compareDeep(exclude, o.exclude, true) && compareDeep(period, o.period, true); 745 } 746 747 @Override 748 public boolean equalsShallow(Base other_) { 749 if (!super.equalsShallow(other_)) 750 return false; 751 if (!(other_ instanceof GroupCharacteristicComponent)) 752 return false; 753 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 754 return compareValues(exclude, o.exclude, true); 755 } 756 757 public boolean isEmpty() { 758 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period); 759 } 760 761 public String fhirType() { 762 return "Group.characteristic"; 763 764 } 765 766 } 767 768 @Block() 769 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 770 /** 771 * A reference to the entity that is a member of the group. Must be consistent 772 * with Group.type. If the entity is another group, then the type must be the 773 * same. 774 */ 775 @Child(name = "entity", type = { Patient.class, Practitioner.class, PractitionerRole.class, Device.class, 776 Medication.class, Substance.class, 777 Group.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 778 @Description(shortDefinition = "Reference to the group member", formalDefinition = "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.") 779 protected Reference entity; 780 781 /** 782 * The actual object that is the target of the reference (A reference to the 783 * entity that is a member of the group. Must be consistent with Group.type. If 784 * the entity is another group, then the type must be the same.) 785 */ 786 protected Resource entityTarget; 787 788 /** 789 * The period that the member was in the group, if known. 790 */ 791 @Child(name = "period", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 792 @Description(shortDefinition = "Period member belonged to the group", formalDefinition = "The period that the member was in the group, if known.") 793 protected Period period; 794 795 /** 796 * A flag to indicate that the member is no longer in the group, but previously 797 * may have been a member. 798 */ 799 @Child(name = "inactive", type = { 800 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 801 @Description(shortDefinition = "If member is no longer in group", formalDefinition = "A flag to indicate that the member is no longer in the group, but previously may have been a member.") 802 protected BooleanType inactive; 803 804 private static final long serialVersionUID = -333869055L; 805 806 /** 807 * Constructor 808 */ 809 public GroupMemberComponent() { 810 super(); 811 } 812 813 /** 814 * Constructor 815 */ 816 public GroupMemberComponent(Reference entity) { 817 super(); 818 this.entity = entity; 819 } 820 821 /** 822 * @return {@link #entity} (A reference to the entity that is a member of the 823 * group. Must be consistent with Group.type. If the entity is another 824 * group, then the type must be the same.) 825 */ 826 public Reference getEntity() { 827 if (this.entity == null) 828 if (Configuration.errorOnAutoCreate()) 829 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 830 else if (Configuration.doAutoCreate()) 831 this.entity = new Reference(); // cc 832 return this.entity; 833 } 834 835 public boolean hasEntity() { 836 return this.entity != null && !this.entity.isEmpty(); 837 } 838 839 /** 840 * @param value {@link #entity} (A reference to the entity that is a member of 841 * the group. Must be consistent with Group.type. If the entity is 842 * another group, then the type must be the same.) 843 */ 844 public GroupMemberComponent setEntity(Reference value) { 845 this.entity = value; 846 return this; 847 } 848 849 /** 850 * @return {@link #entity} The actual object that is the target of the 851 * reference. The reference library doesn't populate this, but you can 852 * use it to hold the resource if you resolve it. (A reference to the 853 * entity that is a member of the group. Must be consistent with 854 * Group.type. If the entity is another group, then the type must be the 855 * same.) 856 */ 857 public Resource getEntityTarget() { 858 return this.entityTarget; 859 } 860 861 /** 862 * @param value {@link #entity} The actual object that is the target of the 863 * reference. The reference library doesn't use these, but you can 864 * use it to hold the resource if you resolve it. (A reference to 865 * the entity that is a member of the group. Must be consistent 866 * with Group.type. If the entity is another group, then the type 867 * must be the same.) 868 */ 869 public GroupMemberComponent setEntityTarget(Resource value) { 870 this.entityTarget = value; 871 return this; 872 } 873 874 /** 875 * @return {@link #period} (The period that the member was in the group, if 876 * known.) 877 */ 878 public Period getPeriod() { 879 if (this.period == null) 880 if (Configuration.errorOnAutoCreate()) 881 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 882 else if (Configuration.doAutoCreate()) 883 this.period = new Period(); // cc 884 return this.period; 885 } 886 887 public boolean hasPeriod() { 888 return this.period != null && !this.period.isEmpty(); 889 } 890 891 /** 892 * @param value {@link #period} (The period that the member was in the group, if 893 * known.) 894 */ 895 public GroupMemberComponent setPeriod(Period value) { 896 this.period = value; 897 return this; 898 } 899 900 /** 901 * @return {@link #inactive} (A flag to indicate that the member is no longer in 902 * the group, but previously may have been a member.). This is the 903 * underlying object with id, value and extensions. The accessor 904 * "getInactive" gives direct access to the value 905 */ 906 public BooleanType getInactiveElement() { 907 if (this.inactive == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 910 else if (Configuration.doAutoCreate()) 911 this.inactive = new BooleanType(); // bb 912 return this.inactive; 913 } 914 915 public boolean hasInactiveElement() { 916 return this.inactive != null && !this.inactive.isEmpty(); 917 } 918 919 public boolean hasInactive() { 920 return this.inactive != null && !this.inactive.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #inactive} (A flag to indicate that the member is no 925 * longer in the group, but previously may have been a member.). 926 * This is the underlying object with id, value and extensions. The 927 * accessor "getInactive" gives direct access to the value 928 */ 929 public GroupMemberComponent setInactiveElement(BooleanType value) { 930 this.inactive = value; 931 return this; 932 } 933 934 /** 935 * @return A flag to indicate that the member is no longer in the group, but 936 * previously may have been a member. 937 */ 938 public boolean getInactive() { 939 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 940 } 941 942 /** 943 * @param value A flag to indicate that the member is no longer in the group, 944 * but previously may have been a member. 945 */ 946 public GroupMemberComponent setInactive(boolean value) { 947 if (this.inactive == null) 948 this.inactive = new BooleanType(); 949 this.inactive.setValue(value); 950 return this; 951 } 952 953 protected void listChildren(List<Property> children) { 954 super.listChildren(children); 955 children.add(new Property("entity", 956 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 957 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 958 0, 1, entity)); 959 children.add( 960 new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period)); 961 children.add(new Property("inactive", "boolean", 962 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, 963 inactive)); 964 } 965 966 @Override 967 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 968 switch (_hash) { 969 case -1298275357: 970 /* entity */ return new Property("entity", 971 "Reference(Patient|Practitioner|PractitionerRole|Device|Medication|Substance|Group)", 972 "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 973 0, 1, entity); 974 case -991726143: 975 /* period */ return new Property("period", "Period", "The period that the member was in the group, if known.", 976 0, 1, period); 977 case 24665195: 978 /* inactive */ return new Property("inactive", "boolean", 979 "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 980 1, inactive); 981 default: 982 return super.getNamedProperty(_hash, _name, _checkValid); 983 } 984 985 } 986 987 @Override 988 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 989 switch (hash) { 990 case -1298275357: 991 /* entity */ return this.entity == null ? new Base[0] : new Base[] { this.entity }; // Reference 992 case -991726143: 993 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 994 case 24665195: 995 /* inactive */ return this.inactive == null ? new Base[0] : new Base[] { this.inactive }; // BooleanType 996 default: 997 return super.getProperty(hash, name, checkValid); 998 } 999 1000 } 1001 1002 @Override 1003 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1004 switch (hash) { 1005 case -1298275357: // entity 1006 this.entity = castToReference(value); // Reference 1007 return value; 1008 case -991726143: // period 1009 this.period = castToPeriod(value); // Period 1010 return value; 1011 case 24665195: // inactive 1012 this.inactive = castToBoolean(value); // BooleanType 1013 return value; 1014 default: 1015 return super.setProperty(hash, name, value); 1016 } 1017 1018 } 1019 1020 @Override 1021 public Base setProperty(String name, Base value) throws FHIRException { 1022 if (name.equals("entity")) { 1023 this.entity = castToReference(value); // Reference 1024 } else if (name.equals("period")) { 1025 this.period = castToPeriod(value); // Period 1026 } else if (name.equals("inactive")) { 1027 this.inactive = castToBoolean(value); // BooleanType 1028 } else 1029 return super.setProperty(name, value); 1030 return value; 1031 } 1032 1033 @Override 1034 public void removeChild(String name, Base value) throws FHIRException { 1035 if (name.equals("entity")) { 1036 this.entity = null; 1037 } else if (name.equals("period")) { 1038 this.period = null; 1039 } else if (name.equals("inactive")) { 1040 this.inactive = null; 1041 } else 1042 super.removeChild(name, value); 1043 1044 } 1045 1046 @Override 1047 public Base makeProperty(int hash, String name) throws FHIRException { 1048 switch (hash) { 1049 case -1298275357: 1050 return getEntity(); 1051 case -991726143: 1052 return getPeriod(); 1053 case 24665195: 1054 return getInactiveElement(); 1055 default: 1056 return super.makeProperty(hash, name); 1057 } 1058 1059 } 1060 1061 @Override 1062 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1063 switch (hash) { 1064 case -1298275357: 1065 /* entity */ return new String[] { "Reference" }; 1066 case -991726143: 1067 /* period */ return new String[] { "Period" }; 1068 case 24665195: 1069 /* inactive */ return new String[] { "boolean" }; 1070 default: 1071 return super.getTypesForProperty(hash, name); 1072 } 1073 1074 } 1075 1076 @Override 1077 public Base addChild(String name) throws FHIRException { 1078 if (name.equals("entity")) { 1079 this.entity = new Reference(); 1080 return this.entity; 1081 } else if (name.equals("period")) { 1082 this.period = new Period(); 1083 return this.period; 1084 } else if (name.equals("inactive")) { 1085 throw new FHIRException("Cannot call addChild on a singleton property Group.inactive"); 1086 } else 1087 return super.addChild(name); 1088 } 1089 1090 public GroupMemberComponent copy() { 1091 GroupMemberComponent dst = new GroupMemberComponent(); 1092 copyValues(dst); 1093 return dst; 1094 } 1095 1096 public void copyValues(GroupMemberComponent dst) { 1097 super.copyValues(dst); 1098 dst.entity = entity == null ? null : entity.copy(); 1099 dst.period = period == null ? null : period.copy(); 1100 dst.inactive = inactive == null ? null : inactive.copy(); 1101 } 1102 1103 @Override 1104 public boolean equalsDeep(Base other_) { 1105 if (!super.equalsDeep(other_)) 1106 return false; 1107 if (!(other_ instanceof GroupMemberComponent)) 1108 return false; 1109 GroupMemberComponent o = (GroupMemberComponent) other_; 1110 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) 1111 && compareDeep(inactive, o.inactive, true); 1112 } 1113 1114 @Override 1115 public boolean equalsShallow(Base other_) { 1116 if (!super.equalsShallow(other_)) 1117 return false; 1118 if (!(other_ instanceof GroupMemberComponent)) 1119 return false; 1120 GroupMemberComponent o = (GroupMemberComponent) other_; 1121 return compareValues(inactive, o.inactive, true); 1122 } 1123 1124 public boolean isEmpty() { 1125 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, period, inactive); 1126 } 1127 1128 public String fhirType() { 1129 return "Group.member"; 1130 1131 } 1132 1133 } 1134 1135 /** 1136 * A unique business identifier for this group. 1137 */ 1138 @Child(name = "identifier", type = { 1139 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1140 @Description(shortDefinition = "Unique id", formalDefinition = "A unique business identifier for this group.") 1141 protected List<Identifier> identifier; 1142 1143 /** 1144 * Indicates whether the record for the group is available for use or is merely 1145 * being retained for historical purposes. 1146 */ 1147 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1148 @Description(shortDefinition = "Whether this group's record is in active use", formalDefinition = "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.") 1149 protected BooleanType active; 1150 1151 /** 1152 * Identifies the broad classification of the kind of resources the group 1153 * includes. 1154 */ 1155 @Child(name = "type", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1156 @Description(shortDefinition = "person | animal | practitioner | device | medication | substance", formalDefinition = "Identifies the broad classification of the kind of resources the group includes.") 1157 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/group-type") 1158 protected Enumeration<GroupType> type; 1159 1160 /** 1161 * If true, indicates that the resource refers to a specific group of real 1162 * individuals. If false, the group defines a set of intended individuals. 1163 */ 1164 @Child(name = "actual", type = { BooleanType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1165 @Description(shortDefinition = "Descriptive or actual", formalDefinition = "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.") 1166 protected BooleanType actual; 1167 1168 /** 1169 * Provides a specific type of resource the group includes; e.g. "cow", 1170 * "syringe", etc. 1171 */ 1172 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1173 @Description(shortDefinition = "Kind of Group members", formalDefinition = "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.") 1174 protected CodeableConcept code; 1175 1176 /** 1177 * A label assigned to the group for human identification and communication. 1178 */ 1179 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1180 @Description(shortDefinition = "Label for Group", formalDefinition = "A label assigned to the group for human identification and communication.") 1181 protected StringType name; 1182 1183 /** 1184 * A count of the number of resource instances that are part of the group. 1185 */ 1186 @Child(name = "quantity", type = { 1187 UnsignedIntType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1188 @Description(shortDefinition = "Number of members", formalDefinition = "A count of the number of resource instances that are part of the group.") 1189 protected UnsignedIntType quantity; 1190 1191 /** 1192 * Entity responsible for defining and maintaining Group characteristics and/or 1193 * registered members. 1194 */ 1195 @Child(name = "managingEntity", type = { Organization.class, RelatedPerson.class, Practitioner.class, 1196 PractitionerRole.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1197 @Description(shortDefinition = "Entity that is the custodian of the Group's definition", formalDefinition = "Entity responsible for defining and maintaining Group characteristics and/or registered members.") 1198 protected Reference managingEntity; 1199 1200 /** 1201 * The actual object that is the target of the reference (Entity responsible for 1202 * defining and maintaining Group characteristics and/or registered members.) 1203 */ 1204 protected Resource managingEntityTarget; 1205 1206 /** 1207 * Identifies traits whose presence r absence is shared by members of the group. 1208 */ 1209 @Child(name = "characteristic", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1210 @Description(shortDefinition = "Include / Exclude group members by Trait", formalDefinition = "Identifies traits whose presence r absence is shared by members of the group.") 1211 protected List<GroupCharacteristicComponent> characteristic; 1212 1213 /** 1214 * Identifies the resource instances that are members of the group. 1215 */ 1216 @Child(name = "member", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1217 @Description(shortDefinition = "Who or what is in group", formalDefinition = "Identifies the resource instances that are members of the group.") 1218 protected List<GroupMemberComponent> member; 1219 1220 private static final long serialVersionUID = -550945963L; 1221 1222 /** 1223 * Constructor 1224 */ 1225 public Group() { 1226 super(); 1227 } 1228 1229 /** 1230 * Constructor 1231 */ 1232 public Group(Enumeration<GroupType> type, BooleanType actual) { 1233 super(); 1234 this.type = type; 1235 this.actual = actual; 1236 } 1237 1238 /** 1239 * @return {@link #identifier} (A unique business identifier for this group.) 1240 */ 1241 public List<Identifier> getIdentifier() { 1242 if (this.identifier == null) 1243 this.identifier = new ArrayList<Identifier>(); 1244 return this.identifier; 1245 } 1246 1247 /** 1248 * @return Returns a reference to <code>this</code> for easy method chaining 1249 */ 1250 public Group setIdentifier(List<Identifier> theIdentifier) { 1251 this.identifier = theIdentifier; 1252 return this; 1253 } 1254 1255 public boolean hasIdentifier() { 1256 if (this.identifier == null) 1257 return false; 1258 for (Identifier item : this.identifier) 1259 if (!item.isEmpty()) 1260 return true; 1261 return false; 1262 } 1263 1264 public Identifier addIdentifier() { // 3 1265 Identifier t = new Identifier(); 1266 if (this.identifier == null) 1267 this.identifier = new ArrayList<Identifier>(); 1268 this.identifier.add(t); 1269 return t; 1270 } 1271 1272 public Group addIdentifier(Identifier t) { // 3 1273 if (t == null) 1274 return this; 1275 if (this.identifier == null) 1276 this.identifier = new ArrayList<Identifier>(); 1277 this.identifier.add(t); 1278 return this; 1279 } 1280 1281 /** 1282 * @return The first repetition of repeating field {@link #identifier}, creating 1283 * it if it does not already exist 1284 */ 1285 public Identifier getIdentifierFirstRep() { 1286 if (getIdentifier().isEmpty()) { 1287 addIdentifier(); 1288 } 1289 return getIdentifier().get(0); 1290 } 1291 1292 /** 1293 * @return {@link #active} (Indicates whether the record for the group is 1294 * available for use or is merely being retained for historical 1295 * purposes.). This is the underlying object with id, value and 1296 * extensions. The accessor "getActive" gives direct access to the value 1297 */ 1298 public BooleanType getActiveElement() { 1299 if (this.active == null) 1300 if (Configuration.errorOnAutoCreate()) 1301 throw new Error("Attempt to auto-create Group.active"); 1302 else if (Configuration.doAutoCreate()) 1303 this.active = new BooleanType(); // bb 1304 return this.active; 1305 } 1306 1307 public boolean hasActiveElement() { 1308 return this.active != null && !this.active.isEmpty(); 1309 } 1310 1311 public boolean hasActive() { 1312 return this.active != null && !this.active.isEmpty(); 1313 } 1314 1315 /** 1316 * @param value {@link #active} (Indicates whether the record for the group is 1317 * available for use or is merely being retained for historical 1318 * purposes.). This is the underlying object with id, value and 1319 * extensions. The accessor "getActive" gives direct access to the 1320 * value 1321 */ 1322 public Group setActiveElement(BooleanType value) { 1323 this.active = value; 1324 return this; 1325 } 1326 1327 /** 1328 * @return Indicates whether the record for the group is available for use or is 1329 * merely being retained for historical purposes. 1330 */ 1331 public boolean getActive() { 1332 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1333 } 1334 1335 /** 1336 * @param value Indicates whether the record for the group is available for use 1337 * or is merely being retained for historical purposes. 1338 */ 1339 public Group setActive(boolean value) { 1340 if (this.active == null) 1341 this.active = new BooleanType(); 1342 this.active.setValue(value); 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #type} (Identifies the broad classification of the kind of 1348 * resources the group includes.). This is the underlying object with 1349 * id, value and extensions. The accessor "getType" gives direct access 1350 * to the value 1351 */ 1352 public Enumeration<GroupType> getTypeElement() { 1353 if (this.type == null) 1354 if (Configuration.errorOnAutoCreate()) 1355 throw new Error("Attempt to auto-create Group.type"); 1356 else if (Configuration.doAutoCreate()) 1357 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 1358 return this.type; 1359 } 1360 1361 public boolean hasTypeElement() { 1362 return this.type != null && !this.type.isEmpty(); 1363 } 1364 1365 public boolean hasType() { 1366 return this.type != null && !this.type.isEmpty(); 1367 } 1368 1369 /** 1370 * @param value {@link #type} (Identifies the broad classification of the kind 1371 * of resources the group includes.). This is the underlying object 1372 * with id, value and extensions. The accessor "getType" gives 1373 * direct access to the value 1374 */ 1375 public Group setTypeElement(Enumeration<GroupType> value) { 1376 this.type = value; 1377 return this; 1378 } 1379 1380 /** 1381 * @return Identifies the broad classification of the kind of resources the 1382 * group includes. 1383 */ 1384 public GroupType getType() { 1385 return this.type == null ? null : this.type.getValue(); 1386 } 1387 1388 /** 1389 * @param value Identifies the broad classification of the kind of resources the 1390 * group includes. 1391 */ 1392 public Group setType(GroupType value) { 1393 if (this.type == null) 1394 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1395 this.type.setValue(value); 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #actual} (If true, indicates that the resource refers to a 1401 * specific group of real individuals. If false, the group defines a set 1402 * of intended individuals.). This is the underlying object with id, 1403 * value and extensions. The accessor "getActual" gives direct access to 1404 * the value 1405 */ 1406 public BooleanType getActualElement() { 1407 if (this.actual == null) 1408 if (Configuration.errorOnAutoCreate()) 1409 throw new Error("Attempt to auto-create Group.actual"); 1410 else if (Configuration.doAutoCreate()) 1411 this.actual = new BooleanType(); // bb 1412 return this.actual; 1413 } 1414 1415 public boolean hasActualElement() { 1416 return this.actual != null && !this.actual.isEmpty(); 1417 } 1418 1419 public boolean hasActual() { 1420 return this.actual != null && !this.actual.isEmpty(); 1421 } 1422 1423 /** 1424 * @param value {@link #actual} (If true, indicates that the resource refers to 1425 * a specific group of real individuals. If false, the group 1426 * defines a set of intended individuals.). This is the underlying 1427 * object with id, value and extensions. The accessor "getActual" 1428 * gives direct access to the value 1429 */ 1430 public Group setActualElement(BooleanType value) { 1431 this.actual = value; 1432 return this; 1433 } 1434 1435 /** 1436 * @return If true, indicates that the resource refers to a specific group of 1437 * real individuals. If false, the group defines a set of intended 1438 * individuals. 1439 */ 1440 public boolean getActual() { 1441 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1442 } 1443 1444 /** 1445 * @param value If true, indicates that the resource refers to a specific group 1446 * of real individuals. If false, the group defines a set of 1447 * intended individuals. 1448 */ 1449 public Group setActual(boolean value) { 1450 if (this.actual == null) 1451 this.actual = new BooleanType(); 1452 this.actual.setValue(value); 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #code} (Provides a specific type of resource the group 1458 * includes; e.g. "cow", "syringe", etc.) 1459 */ 1460 public CodeableConcept getCode() { 1461 if (this.code == null) 1462 if (Configuration.errorOnAutoCreate()) 1463 throw new Error("Attempt to auto-create Group.code"); 1464 else if (Configuration.doAutoCreate()) 1465 this.code = new CodeableConcept(); // cc 1466 return this.code; 1467 } 1468 1469 public boolean hasCode() { 1470 return this.code != null && !this.code.isEmpty(); 1471 } 1472 1473 /** 1474 * @param value {@link #code} (Provides a specific type of resource the group 1475 * includes; e.g. "cow", "syringe", etc.) 1476 */ 1477 public Group setCode(CodeableConcept value) { 1478 this.code = value; 1479 return this; 1480 } 1481 1482 /** 1483 * @return {@link #name} (A label assigned to the group for human identification 1484 * and communication.). This is the underlying object with id, value and 1485 * extensions. The accessor "getName" gives direct access to the value 1486 */ 1487 public StringType getNameElement() { 1488 if (this.name == null) 1489 if (Configuration.errorOnAutoCreate()) 1490 throw new Error("Attempt to auto-create Group.name"); 1491 else if (Configuration.doAutoCreate()) 1492 this.name = new StringType(); // bb 1493 return this.name; 1494 } 1495 1496 public boolean hasNameElement() { 1497 return this.name != null && !this.name.isEmpty(); 1498 } 1499 1500 public boolean hasName() { 1501 return this.name != null && !this.name.isEmpty(); 1502 } 1503 1504 /** 1505 * @param value {@link #name} (A label assigned to the group for human 1506 * identification and communication.). This is the underlying 1507 * object with id, value and extensions. The accessor "getName" 1508 * gives direct access to the value 1509 */ 1510 public Group setNameElement(StringType value) { 1511 this.name = value; 1512 return this; 1513 } 1514 1515 /** 1516 * @return A label assigned to the group for human identification and 1517 * communication. 1518 */ 1519 public String getName() { 1520 return this.name == null ? null : this.name.getValue(); 1521 } 1522 1523 /** 1524 * @param value A label assigned to the group for human identification and 1525 * communication. 1526 */ 1527 public Group setName(String value) { 1528 if (Utilities.noString(value)) 1529 this.name = null; 1530 else { 1531 if (this.name == null) 1532 this.name = new StringType(); 1533 this.name.setValue(value); 1534 } 1535 return this; 1536 } 1537 1538 /** 1539 * @return {@link #quantity} (A count of the number of resource instances that 1540 * are part of the group.). This is the underlying object with id, value 1541 * and extensions. The accessor "getQuantity" gives direct access to the 1542 * value 1543 */ 1544 public UnsignedIntType getQuantityElement() { 1545 if (this.quantity == null) 1546 if (Configuration.errorOnAutoCreate()) 1547 throw new Error("Attempt to auto-create Group.quantity"); 1548 else if (Configuration.doAutoCreate()) 1549 this.quantity = new UnsignedIntType(); // bb 1550 return this.quantity; 1551 } 1552 1553 public boolean hasQuantityElement() { 1554 return this.quantity != null && !this.quantity.isEmpty(); 1555 } 1556 1557 public boolean hasQuantity() { 1558 return this.quantity != null && !this.quantity.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #quantity} (A count of the number of resource instances 1563 * that are part of the group.). This is the underlying object with 1564 * id, value and extensions. The accessor "getQuantity" gives 1565 * direct access to the value 1566 */ 1567 public Group setQuantityElement(UnsignedIntType value) { 1568 this.quantity = value; 1569 return this; 1570 } 1571 1572 /** 1573 * @return A count of the number of resource instances that are part of the 1574 * group. 1575 */ 1576 public int getQuantity() { 1577 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1578 } 1579 1580 /** 1581 * @param value A count of the number of resource instances that are part of the 1582 * group. 1583 */ 1584 public Group setQuantity(int value) { 1585 if (this.quantity == null) 1586 this.quantity = new UnsignedIntType(); 1587 this.quantity.setValue(value); 1588 return this; 1589 } 1590 1591 /** 1592 * @return {@link #managingEntity} (Entity responsible for defining and 1593 * maintaining Group characteristics and/or registered members.) 1594 */ 1595 public Reference getManagingEntity() { 1596 if (this.managingEntity == null) 1597 if (Configuration.errorOnAutoCreate()) 1598 throw new Error("Attempt to auto-create Group.managingEntity"); 1599 else if (Configuration.doAutoCreate()) 1600 this.managingEntity = new Reference(); // cc 1601 return this.managingEntity; 1602 } 1603 1604 public boolean hasManagingEntity() { 1605 return this.managingEntity != null && !this.managingEntity.isEmpty(); 1606 } 1607 1608 /** 1609 * @param value {@link #managingEntity} (Entity responsible for defining and 1610 * maintaining Group characteristics and/or registered members.) 1611 */ 1612 public Group setManagingEntity(Reference value) { 1613 this.managingEntity = value; 1614 return this; 1615 } 1616 1617 /** 1618 * @return {@link #managingEntity} The actual object that is the target of the 1619 * reference. The reference library doesn't populate this, but you can 1620 * use it to hold the resource if you resolve it. (Entity responsible 1621 * for defining and maintaining Group characteristics and/or registered 1622 * members.) 1623 */ 1624 public Resource getManagingEntityTarget() { 1625 return this.managingEntityTarget; 1626 } 1627 1628 /** 1629 * @param value {@link #managingEntity} The actual object that is the target of 1630 * the reference. The reference library doesn't use these, but you 1631 * can use it to hold the resource if you resolve it. (Entity 1632 * responsible for defining and maintaining Group characteristics 1633 * and/or registered members.) 1634 */ 1635 public Group setManagingEntityTarget(Resource value) { 1636 this.managingEntityTarget = value; 1637 return this; 1638 } 1639 1640 /** 1641 * @return {@link #characteristic} (Identifies traits whose presence r absence 1642 * is shared by members of the group.) 1643 */ 1644 public List<GroupCharacteristicComponent> getCharacteristic() { 1645 if (this.characteristic == null) 1646 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1647 return this.characteristic; 1648 } 1649 1650 /** 1651 * @return Returns a reference to <code>this</code> for easy method chaining 1652 */ 1653 public Group setCharacteristic(List<GroupCharacteristicComponent> theCharacteristic) { 1654 this.characteristic = theCharacteristic; 1655 return this; 1656 } 1657 1658 public boolean hasCharacteristic() { 1659 if (this.characteristic == null) 1660 return false; 1661 for (GroupCharacteristicComponent item : this.characteristic) 1662 if (!item.isEmpty()) 1663 return true; 1664 return false; 1665 } 1666 1667 public GroupCharacteristicComponent addCharacteristic() { // 3 1668 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1669 if (this.characteristic == null) 1670 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1671 this.characteristic.add(t); 1672 return t; 1673 } 1674 1675 public Group addCharacteristic(GroupCharacteristicComponent t) { // 3 1676 if (t == null) 1677 return this; 1678 if (this.characteristic == null) 1679 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1680 this.characteristic.add(t); 1681 return this; 1682 } 1683 1684 /** 1685 * @return The first repetition of repeating field {@link #characteristic}, 1686 * creating it if it does not already exist 1687 */ 1688 public GroupCharacteristicComponent getCharacteristicFirstRep() { 1689 if (getCharacteristic().isEmpty()) { 1690 addCharacteristic(); 1691 } 1692 return getCharacteristic().get(0); 1693 } 1694 1695 /** 1696 * @return {@link #member} (Identifies the resource instances that are members 1697 * of the group.) 1698 */ 1699 public List<GroupMemberComponent> getMember() { 1700 if (this.member == null) 1701 this.member = new ArrayList<GroupMemberComponent>(); 1702 return this.member; 1703 } 1704 1705 /** 1706 * @return Returns a reference to <code>this</code> for easy method chaining 1707 */ 1708 public Group setMember(List<GroupMemberComponent> theMember) { 1709 this.member = theMember; 1710 return this; 1711 } 1712 1713 public boolean hasMember() { 1714 if (this.member == null) 1715 return false; 1716 for (GroupMemberComponent item : this.member) 1717 if (!item.isEmpty()) 1718 return true; 1719 return false; 1720 } 1721 1722 public GroupMemberComponent addMember() { // 3 1723 GroupMemberComponent t = new GroupMemberComponent(); 1724 if (this.member == null) 1725 this.member = new ArrayList<GroupMemberComponent>(); 1726 this.member.add(t); 1727 return t; 1728 } 1729 1730 public Group addMember(GroupMemberComponent t) { // 3 1731 if (t == null) 1732 return this; 1733 if (this.member == null) 1734 this.member = new ArrayList<GroupMemberComponent>(); 1735 this.member.add(t); 1736 return this; 1737 } 1738 1739 /** 1740 * @return The first repetition of repeating field {@link #member}, creating it 1741 * if it does not already exist 1742 */ 1743 public GroupMemberComponent getMemberFirstRep() { 1744 if (getMember().isEmpty()) { 1745 addMember(); 1746 } 1747 return getMember().get(0); 1748 } 1749 1750 protected void listChildren(List<Property> children) { 1751 super.listChildren(children); 1752 children.add(new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, 1753 java.lang.Integer.MAX_VALUE, identifier)); 1754 children.add(new Property("active", "boolean", 1755 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1756 0, 1, active)); 1757 children.add(new Property("type", "code", 1758 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type)); 1759 children.add(new Property("actual", "boolean", 1760 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1761 0, 1, actual)); 1762 children.add(new Property("code", "CodeableConcept", 1763 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code)); 1764 children.add(new Property("name", "string", 1765 "A label assigned to the group for human identification and communication.", 0, 1, name)); 1766 children.add(new Property("quantity", "unsignedInt", 1767 "A count of the number of resource instances that are part of the group.", 0, 1, quantity)); 1768 children.add(new Property("managingEntity", "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1769 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1770 managingEntity)); 1771 children.add(new Property("characteristic", "", 1772 "Identifies traits whose presence r absence is shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, 1773 characteristic)); 1774 children.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, 1775 java.lang.Integer.MAX_VALUE, member)); 1776 } 1777 1778 @Override 1779 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1780 switch (_hash) { 1781 case -1618432855: 1782 /* identifier */ return new Property("identifier", "Identifier", "A unique business identifier for this group.", 1783 0, java.lang.Integer.MAX_VALUE, identifier); 1784 case -1422950650: 1785 /* active */ return new Property("active", "boolean", 1786 "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 1787 0, 1, active); 1788 case 3575610: 1789 /* type */ return new Property("type", "code", 1790 "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type); 1791 case -1422939762: 1792 /* actual */ return new Property("actual", "boolean", 1793 "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 1794 0, 1, actual); 1795 case 3059181: 1796 /* code */ return new Property("code", "CodeableConcept", 1797 "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code); 1798 case 3373707: 1799 /* name */ return new Property("name", "string", 1800 "A label assigned to the group for human identification and communication.", 0, 1, name); 1801 case -1285004149: 1802 /* quantity */ return new Property("quantity", "unsignedInt", 1803 "A count of the number of resource instances that are part of the group.", 0, 1, quantity); 1804 case -988474523: 1805 /* managingEntity */ return new Property("managingEntity", 1806 "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", 1807 "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, 1808 managingEntity); 1809 case 366313883: 1810 /* characteristic */ return new Property("characteristic", "", 1811 "Identifies traits whose presence r absence is shared by members of the group.", 0, 1812 java.lang.Integer.MAX_VALUE, characteristic); 1813 case -1077769574: 1814 /* member */ return new Property("member", "", "Identifies the resource instances that are members of the group.", 1815 0, java.lang.Integer.MAX_VALUE, member); 1816 default: 1817 return super.getNamedProperty(_hash, _name, _checkValid); 1818 } 1819 1820 } 1821 1822 @Override 1823 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1824 switch (hash) { 1825 case -1618432855: 1826 /* identifier */ return this.identifier == null ? new Base[0] 1827 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1828 case -1422950650: 1829 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 1830 case 3575610: 1831 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<GroupType> 1832 case -1422939762: 1833 /* actual */ return this.actual == null ? new Base[0] : new Base[] { this.actual }; // BooleanType 1834 case 3059181: 1835 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1836 case 3373707: 1837 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1838 case -1285004149: 1839 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // UnsignedIntType 1840 case -988474523: 1841 /* managingEntity */ return this.managingEntity == null ? new Base[0] : new Base[] { this.managingEntity }; // Reference 1842 case 366313883: 1843 /* characteristic */ return this.characteristic == null ? new Base[0] 1844 : this.characteristic.toArray(new Base[this.characteristic.size()]); // GroupCharacteristicComponent 1845 case -1077769574: 1846 /* member */ return this.member == null ? new Base[0] : this.member.toArray(new Base[this.member.size()]); // GroupMemberComponent 1847 default: 1848 return super.getProperty(hash, name, checkValid); 1849 } 1850 1851 } 1852 1853 @Override 1854 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1855 switch (hash) { 1856 case -1618432855: // identifier 1857 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1858 return value; 1859 case -1422950650: // active 1860 this.active = castToBoolean(value); // BooleanType 1861 return value; 1862 case 3575610: // type 1863 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1864 this.type = (Enumeration) value; // Enumeration<GroupType> 1865 return value; 1866 case -1422939762: // actual 1867 this.actual = castToBoolean(value); // BooleanType 1868 return value; 1869 case 3059181: // code 1870 this.code = castToCodeableConcept(value); // CodeableConcept 1871 return value; 1872 case 3373707: // name 1873 this.name = castToString(value); // StringType 1874 return value; 1875 case -1285004149: // quantity 1876 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1877 return value; 1878 case -988474523: // managingEntity 1879 this.managingEntity = castToReference(value); // Reference 1880 return value; 1881 case 366313883: // characteristic 1882 this.getCharacteristic().add((GroupCharacteristicComponent) value); // GroupCharacteristicComponent 1883 return value; 1884 case -1077769574: // member 1885 this.getMember().add((GroupMemberComponent) value); // GroupMemberComponent 1886 return value; 1887 default: 1888 return super.setProperty(hash, name, value); 1889 } 1890 1891 } 1892 1893 @Override 1894 public Base setProperty(String name, Base value) throws FHIRException { 1895 if (name.equals("identifier")) { 1896 this.getIdentifier().add(castToIdentifier(value)); 1897 } else if (name.equals("active")) { 1898 this.active = castToBoolean(value); // BooleanType 1899 } else if (name.equals("type")) { 1900 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1901 this.type = (Enumeration) value; // Enumeration<GroupType> 1902 } else if (name.equals("actual")) { 1903 this.actual = castToBoolean(value); // BooleanType 1904 } else if (name.equals("code")) { 1905 this.code = castToCodeableConcept(value); // CodeableConcept 1906 } else if (name.equals("name")) { 1907 this.name = castToString(value); // StringType 1908 } else if (name.equals("quantity")) { 1909 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1910 } else if (name.equals("managingEntity")) { 1911 this.managingEntity = castToReference(value); // Reference 1912 } else if (name.equals("characteristic")) { 1913 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1914 } else if (name.equals("member")) { 1915 this.getMember().add((GroupMemberComponent) value); 1916 } else 1917 return super.setProperty(name, value); 1918 return value; 1919 } 1920 1921 @Override 1922 public void removeChild(String name, Base value) throws FHIRException { 1923 if (name.equals("identifier")) { 1924 this.getIdentifier().remove(castToIdentifier(value)); 1925 } else if (name.equals("active")) { 1926 this.active = null; 1927 } else if (name.equals("type")) { 1928 this.type = null; 1929 } else if (name.equals("actual")) { 1930 this.actual = null; 1931 } else if (name.equals("code")) { 1932 this.code = null; 1933 } else if (name.equals("name")) { 1934 this.name = null; 1935 } else if (name.equals("quantity")) { 1936 this.quantity = null; 1937 } else if (name.equals("managingEntity")) { 1938 this.managingEntity = null; 1939 } else if (name.equals("characteristic")) { 1940 this.getCharacteristic().remove((GroupCharacteristicComponent) value); 1941 } else if (name.equals("member")) { 1942 this.getMember().remove((GroupMemberComponent) value); 1943 } else 1944 super.removeChild(name, value); 1945 1946 } 1947 1948 @Override 1949 public Base makeProperty(int hash, String name) throws FHIRException { 1950 switch (hash) { 1951 case -1618432855: 1952 return addIdentifier(); 1953 case -1422950650: 1954 return getActiveElement(); 1955 case 3575610: 1956 return getTypeElement(); 1957 case -1422939762: 1958 return getActualElement(); 1959 case 3059181: 1960 return getCode(); 1961 case 3373707: 1962 return getNameElement(); 1963 case -1285004149: 1964 return getQuantityElement(); 1965 case -988474523: 1966 return getManagingEntity(); 1967 case 366313883: 1968 return addCharacteristic(); 1969 case -1077769574: 1970 return addMember(); 1971 default: 1972 return super.makeProperty(hash, name); 1973 } 1974 1975 } 1976 1977 @Override 1978 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1979 switch (hash) { 1980 case -1618432855: 1981 /* identifier */ return new String[] { "Identifier" }; 1982 case -1422950650: 1983 /* active */ return new String[] { "boolean" }; 1984 case 3575610: 1985 /* type */ return new String[] { "code" }; 1986 case -1422939762: 1987 /* actual */ return new String[] { "boolean" }; 1988 case 3059181: 1989 /* code */ return new String[] { "CodeableConcept" }; 1990 case 3373707: 1991 /* name */ return new String[] { "string" }; 1992 case -1285004149: 1993 /* quantity */ return new String[] { "unsignedInt" }; 1994 case -988474523: 1995 /* managingEntity */ return new String[] { "Reference" }; 1996 case 366313883: 1997 /* characteristic */ return new String[] {}; 1998 case -1077769574: 1999 /* member */ return new String[] {}; 2000 default: 2001 return super.getTypesForProperty(hash, name); 2002 } 2003 2004 } 2005 2006 @Override 2007 public Base addChild(String name) throws FHIRException { 2008 if (name.equals("identifier")) { 2009 return addIdentifier(); 2010 } else if (name.equals("active")) { 2011 throw new FHIRException("Cannot call addChild on a singleton property Group.active"); 2012 } else if (name.equals("type")) { 2013 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 2014 } else if (name.equals("actual")) { 2015 throw new FHIRException("Cannot call addChild on a singleton property Group.actual"); 2016 } else if (name.equals("code")) { 2017 this.code = new CodeableConcept(); 2018 return this.code; 2019 } else if (name.equals("name")) { 2020 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 2021 } else if (name.equals("quantity")) { 2022 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 2023 } else if (name.equals("managingEntity")) { 2024 this.managingEntity = new Reference(); 2025 return this.managingEntity; 2026 } else if (name.equals("characteristic")) { 2027 return addCharacteristic(); 2028 } else if (name.equals("member")) { 2029 return addMember(); 2030 } else 2031 return super.addChild(name); 2032 } 2033 2034 public String fhirType() { 2035 return "Group"; 2036 2037 } 2038 2039 public Group copy() { 2040 Group dst = new Group(); 2041 copyValues(dst); 2042 return dst; 2043 } 2044 2045 public void copyValues(Group dst) { 2046 super.copyValues(dst); 2047 if (identifier != null) { 2048 dst.identifier = new ArrayList<Identifier>(); 2049 for (Identifier i : identifier) 2050 dst.identifier.add(i.copy()); 2051 } 2052 ; 2053 dst.active = active == null ? null : active.copy(); 2054 dst.type = type == null ? null : type.copy(); 2055 dst.actual = actual == null ? null : actual.copy(); 2056 dst.code = code == null ? null : code.copy(); 2057 dst.name = name == null ? null : name.copy(); 2058 dst.quantity = quantity == null ? null : quantity.copy(); 2059 dst.managingEntity = managingEntity == null ? null : managingEntity.copy(); 2060 if (characteristic != null) { 2061 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 2062 for (GroupCharacteristicComponent i : characteristic) 2063 dst.characteristic.add(i.copy()); 2064 } 2065 ; 2066 if (member != null) { 2067 dst.member = new ArrayList<GroupMemberComponent>(); 2068 for (GroupMemberComponent i : member) 2069 dst.member.add(i.copy()); 2070 } 2071 ; 2072 } 2073 2074 protected Group typedCopy() { 2075 return copy(); 2076 } 2077 2078 @Override 2079 public boolean equalsDeep(Base other_) { 2080 if (!super.equalsDeep(other_)) 2081 return false; 2082 if (!(other_ instanceof Group)) 2083 return false; 2084 Group o = (Group) other_; 2085 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 2086 && compareDeep(type, o.type, true) && compareDeep(actual, o.actual, true) && compareDeep(code, o.code, true) 2087 && compareDeep(name, o.name, true) && compareDeep(quantity, o.quantity, true) 2088 && compareDeep(managingEntity, o.managingEntity, true) && compareDeep(characteristic, o.characteristic, true) 2089 && compareDeep(member, o.member, true); 2090 } 2091 2092 @Override 2093 public boolean equalsShallow(Base other_) { 2094 if (!super.equalsShallow(other_)) 2095 return false; 2096 if (!(other_ instanceof Group)) 2097 return false; 2098 Group o = (Group) other_; 2099 return compareValues(active, o.active, true) && compareValues(type, o.type, true) 2100 && compareValues(actual, o.actual, true) && compareValues(name, o.name, true) 2101 && compareValues(quantity, o.quantity, true); 2102 } 2103 2104 public boolean isEmpty() { 2105 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type, actual, code, name, 2106 quantity, managingEntity, characteristic, member); 2107 } 2108 2109 @Override 2110 public ResourceType getResourceType() { 2111 return ResourceType.Group; 2112 } 2113 2114 /** 2115 * Search parameter: <b>actual</b> 2116 * <p> 2117 * Description: <b>Descriptive or actual</b><br> 2118 * Type: <b>token</b><br> 2119 * Path: <b>Group.actual</b><br> 2120 * </p> 2121 */ 2122 @SearchParamDefinition(name = "actual", path = "Group.actual", description = "Descriptive or actual", type = "token") 2123 public static final String SP_ACTUAL = "actual"; 2124 /** 2125 * <b>Fluent Client</b> search parameter constant for <b>actual</b> 2126 * <p> 2127 * Description: <b>Descriptive or actual</b><br> 2128 * Type: <b>token</b><br> 2129 * Path: <b>Group.actual</b><br> 2130 * </p> 2131 */ 2132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUAL = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2133 SP_ACTUAL); 2134 2135 /** 2136 * Search parameter: <b>identifier</b> 2137 * <p> 2138 * Description: <b>Unique id</b><br> 2139 * Type: <b>token</b><br> 2140 * Path: <b>Group.identifier</b><br> 2141 * </p> 2142 */ 2143 @SearchParamDefinition(name = "identifier", path = "Group.identifier", description = "Unique id", type = "token") 2144 public static final String SP_IDENTIFIER = "identifier"; 2145 /** 2146 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2147 * <p> 2148 * Description: <b>Unique id</b><br> 2149 * Type: <b>token</b><br> 2150 * Path: <b>Group.identifier</b><br> 2151 * </p> 2152 */ 2153 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2154 SP_IDENTIFIER); 2155 2156 /** 2157 * Search parameter: <b>characteristic-value</b> 2158 * <p> 2159 * Description: <b>A composite of both characteristic and value</b><br> 2160 * Type: <b>composite</b><br> 2161 * Path: <b></b><br> 2162 * </p> 2163 */ 2164 @SearchParamDefinition(name = "characteristic-value", path = "Group.characteristic", description = "A composite of both characteristic and value", type = "composite", compositeOf = { 2165 "characteristic", "value" }) 2166 public static final String SP_CHARACTERISTIC_VALUE = "characteristic-value"; 2167 /** 2168 * <b>Fluent Client</b> search parameter constant for 2169 * <b>characteristic-value</b> 2170 * <p> 2171 * Description: <b>A composite of both characteristic and value</b><br> 2172 * Type: <b>composite</b><br> 2173 * Path: <b></b><br> 2174 * </p> 2175 */ 2176 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CHARACTERISTIC_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2177 SP_CHARACTERISTIC_VALUE); 2178 2179 /** 2180 * Search parameter: <b>managing-entity</b> 2181 * <p> 2182 * Description: <b>Entity that is the custodian of the Group's 2183 * definition</b><br> 2184 * Type: <b>reference</b><br> 2185 * Path: <b>Group.managingEntity</b><br> 2186 * </p> 2187 */ 2188 @SearchParamDefinition(name = "managing-entity", path = "Group.managingEntity", description = "Entity that is the custodian of the Group's definition", type = "reference", target = { 2189 Organization.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2190 public static final String SP_MANAGING_ENTITY = "managing-entity"; 2191 /** 2192 * <b>Fluent Client</b> search parameter constant for <b>managing-entity</b> 2193 * <p> 2194 * Description: <b>Entity that is the custodian of the Group's 2195 * definition</b><br> 2196 * Type: <b>reference</b><br> 2197 * Path: <b>Group.managingEntity</b><br> 2198 * </p> 2199 */ 2200 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGING_ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2201 SP_MANAGING_ENTITY); 2202 2203 /** 2204 * Constant for fluent queries to be used to add include statements. Specifies 2205 * the path value of "<b>Group:managing-entity</b>". 2206 */ 2207 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGING_ENTITY = new ca.uhn.fhir.model.api.Include( 2208 "Group:managing-entity").toLocked(); 2209 2210 /** 2211 * Search parameter: <b>code</b> 2212 * <p> 2213 * Description: <b>The kind of resources contained</b><br> 2214 * Type: <b>token</b><br> 2215 * Path: <b>Group.code</b><br> 2216 * </p> 2217 */ 2218 @SearchParamDefinition(name = "code", path = "Group.code", description = "The kind of resources contained", type = "token") 2219 public static final String SP_CODE = "code"; 2220 /** 2221 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2222 * <p> 2223 * Description: <b>The kind of resources contained</b><br> 2224 * Type: <b>token</b><br> 2225 * Path: <b>Group.code</b><br> 2226 * </p> 2227 */ 2228 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2229 SP_CODE); 2230 2231 /** 2232 * Search parameter: <b>member</b> 2233 * <p> 2234 * Description: <b>Reference to the group member</b><br> 2235 * Type: <b>reference</b><br> 2236 * Path: <b>Group.member.entity</b><br> 2237 * </p> 2238 */ 2239 @SearchParamDefinition(name = "member", path = "Group.member.entity", description = "Reference to the group member", type = "reference", providesMembershipIn = { 2240 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2241 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2242 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 2243 Medication.class, Patient.class, Practitioner.class, PractitionerRole.class, Substance.class }) 2244 public static final String SP_MEMBER = "member"; 2245 /** 2246 * <b>Fluent Client</b> search parameter constant for <b>member</b> 2247 * <p> 2248 * Description: <b>Reference to the group member</b><br> 2249 * Type: <b>reference</b><br> 2250 * Path: <b>Group.member.entity</b><br> 2251 * </p> 2252 */ 2253 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2254 SP_MEMBER); 2255 2256 /** 2257 * Constant for fluent queries to be used to add include statements. Specifies 2258 * the path value of "<b>Group:member</b>". 2259 */ 2260 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEMBER = new ca.uhn.fhir.model.api.Include("Group:member") 2261 .toLocked(); 2262 2263 /** 2264 * Search parameter: <b>exclude</b> 2265 * <p> 2266 * Description: <b>Group includes or excludes</b><br> 2267 * Type: <b>token</b><br> 2268 * Path: <b>Group.characteristic.exclude</b><br> 2269 * </p> 2270 */ 2271 @SearchParamDefinition(name = "exclude", path = "Group.characteristic.exclude", description = "Group includes or excludes", type = "token") 2272 public static final String SP_EXCLUDE = "exclude"; 2273 /** 2274 * <b>Fluent Client</b> search parameter constant for <b>exclude</b> 2275 * <p> 2276 * Description: <b>Group includes or excludes</b><br> 2277 * Type: <b>token</b><br> 2278 * Path: <b>Group.characteristic.exclude</b><br> 2279 * </p> 2280 */ 2281 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2282 SP_EXCLUDE); 2283 2284 /** 2285 * Search parameter: <b>type</b> 2286 * <p> 2287 * Description: <b>The type of resources the group contains</b><br> 2288 * Type: <b>token</b><br> 2289 * Path: <b>Group.type</b><br> 2290 * </p> 2291 */ 2292 @SearchParamDefinition(name = "type", path = "Group.type", description = "The type of resources the group contains", type = "token") 2293 public static final String SP_TYPE = "type"; 2294 /** 2295 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2296 * <p> 2297 * Description: <b>The type of resources the group contains</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>Group.type</b><br> 2300 * </p> 2301 */ 2302 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2303 SP_TYPE); 2304 2305 /** 2306 * Search parameter: <b>value</b> 2307 * <p> 2308 * Description: <b>Value held by characteristic</b><br> 2309 * Type: <b>token</b><br> 2310 * Path: <b>Group.characteristic.value[x]</b><br> 2311 * </p> 2312 */ 2313 @SearchParamDefinition(name = "value", path = "(Group.characteristic.value as CodeableConcept) | (Group.characteristic.value as boolean)", description = "Value held by characteristic", type = "token") 2314 public static final String SP_VALUE = "value"; 2315 /** 2316 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2317 * <p> 2318 * Description: <b>Value held by characteristic</b><br> 2319 * Type: <b>token</b><br> 2320 * Path: <b>Group.characteristic.value[x]</b><br> 2321 * </p> 2322 */ 2323 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2324 SP_VALUE); 2325 2326 /** 2327 * Search parameter: <b>characteristic</b> 2328 * <p> 2329 * Description: <b>Kind of characteristic</b><br> 2330 * Type: <b>token</b><br> 2331 * Path: <b>Group.characteristic.code</b><br> 2332 * </p> 2333 */ 2334 @SearchParamDefinition(name = "characteristic", path = "Group.characteristic.code", description = "Kind of characteristic", type = "token") 2335 public static final String SP_CHARACTERISTIC = "characteristic"; 2336 /** 2337 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2338 * <p> 2339 * Description: <b>Kind of characteristic</b><br> 2340 * Type: <b>token</b><br> 2341 * Path: <b>Group.characteristic.code</b><br> 2342 * </p> 2343 */ 2344 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2345 SP_CHARACTERISTIC); 2346 2347}