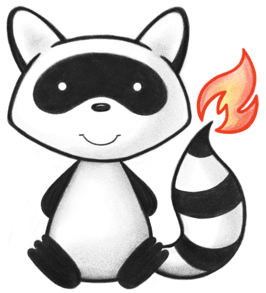
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A guidance response is the formal response to a guidance request, including 046 * any output parameters returned by the evaluation, as well as the description 047 * of any proposed actions to be taken. 048 */ 049@ResourceDef(name = "GuidanceResponse", profile = "http://hl7.org/fhir/StructureDefinition/GuidanceResponse") 050public class GuidanceResponse extends DomainResource { 051 052 public enum GuidanceResponseStatus { 053 /** 054 * The request was processed successfully. 055 */ 056 SUCCESS, 057 /** 058 * The request was processed successfully, but more data may result in a more 059 * complete evaluation. 060 */ 061 DATAREQUESTED, 062 /** 063 * The request was processed, but more data is required to complete the 064 * evaluation. 065 */ 066 DATAREQUIRED, 067 /** 068 * The request is currently being processed. 069 */ 070 INPROGRESS, 071 /** 072 * The request was not processed successfully. 073 */ 074 FAILURE, 075 /** 076 * The response was entered in error. 077 */ 078 ENTEREDINERROR, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 084 public static GuidanceResponseStatus fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("success".equals(codeString)) 088 return SUCCESS; 089 if ("data-requested".equals(codeString)) 090 return DATAREQUESTED; 091 if ("data-required".equals(codeString)) 092 return DATAREQUIRED; 093 if ("in-progress".equals(codeString)) 094 return INPROGRESS; 095 if ("failure".equals(codeString)) 096 return FAILURE; 097 if ("entered-in-error".equals(codeString)) 098 return ENTEREDINERROR; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown GuidanceResponseStatus code '" + codeString + "'"); 103 } 104 105 public String toCode() { 106 switch (this) { 107 case SUCCESS: 108 return "success"; 109 case DATAREQUESTED: 110 return "data-requested"; 111 case DATAREQUIRED: 112 return "data-required"; 113 case INPROGRESS: 114 return "in-progress"; 115 case FAILURE: 116 return "failure"; 117 case ENTEREDINERROR: 118 return "entered-in-error"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getSystem() { 127 switch (this) { 128 case SUCCESS: 129 return "http://hl7.org/fhir/guidance-response-status"; 130 case DATAREQUESTED: 131 return "http://hl7.org/fhir/guidance-response-status"; 132 case DATAREQUIRED: 133 return "http://hl7.org/fhir/guidance-response-status"; 134 case INPROGRESS: 135 return "http://hl7.org/fhir/guidance-response-status"; 136 case FAILURE: 137 return "http://hl7.org/fhir/guidance-response-status"; 138 case ENTEREDINERROR: 139 return "http://hl7.org/fhir/guidance-response-status"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDefinition() { 148 switch (this) { 149 case SUCCESS: 150 return "The request was processed successfully."; 151 case DATAREQUESTED: 152 return "The request was processed successfully, but more data may result in a more complete evaluation."; 153 case DATAREQUIRED: 154 return "The request was processed, but more data is required to complete the evaluation."; 155 case INPROGRESS: 156 return "The request is currently being processed."; 157 case FAILURE: 158 return "The request was not processed successfully."; 159 case ENTEREDINERROR: 160 return "The response was entered in error."; 161 case NULL: 162 return null; 163 default: 164 return "?"; 165 } 166 } 167 168 public String getDisplay() { 169 switch (this) { 170 case SUCCESS: 171 return "Success"; 172 case DATAREQUESTED: 173 return "Data Requested"; 174 case DATAREQUIRED: 175 return "Data Required"; 176 case INPROGRESS: 177 return "In Progress"; 178 case FAILURE: 179 return "Failure"; 180 case ENTEREDINERROR: 181 return "Entered In Error"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 } 189 190 public static class GuidanceResponseStatusEnumFactory implements EnumFactory<GuidanceResponseStatus> { 191 public GuidanceResponseStatus fromCode(String codeString) throws IllegalArgumentException { 192 if (codeString == null || "".equals(codeString)) 193 if (codeString == null || "".equals(codeString)) 194 return null; 195 if ("success".equals(codeString)) 196 return GuidanceResponseStatus.SUCCESS; 197 if ("data-requested".equals(codeString)) 198 return GuidanceResponseStatus.DATAREQUESTED; 199 if ("data-required".equals(codeString)) 200 return GuidanceResponseStatus.DATAREQUIRED; 201 if ("in-progress".equals(codeString)) 202 return GuidanceResponseStatus.INPROGRESS; 203 if ("failure".equals(codeString)) 204 return GuidanceResponseStatus.FAILURE; 205 if ("entered-in-error".equals(codeString)) 206 return GuidanceResponseStatus.ENTEREDINERROR; 207 throw new IllegalArgumentException("Unknown GuidanceResponseStatus code '" + codeString + "'"); 208 } 209 210 public Enumeration<GuidanceResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.NULL, code); 215 String codeString = code.asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.NULL, code); 218 if ("success".equals(codeString)) 219 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.SUCCESS, code); 220 if ("data-requested".equals(codeString)) 221 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUESTED, code); 222 if ("data-required".equals(codeString)) 223 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUIRED, code); 224 if ("in-progress".equals(codeString)) 225 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.INPROGRESS, code); 226 if ("failure".equals(codeString)) 227 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.FAILURE, code); 228 if ("entered-in-error".equals(codeString)) 229 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.ENTEREDINERROR, code); 230 throw new FHIRException("Unknown GuidanceResponseStatus code '" + codeString + "'"); 231 } 232 233 public String toCode(GuidanceResponseStatus code) { 234 if (code == GuidanceResponseStatus.NULL) 235 return null; 236 if (code == GuidanceResponseStatus.SUCCESS) 237 return "success"; 238 if (code == GuidanceResponseStatus.DATAREQUESTED) 239 return "data-requested"; 240 if (code == GuidanceResponseStatus.DATAREQUIRED) 241 return "data-required"; 242 if (code == GuidanceResponseStatus.INPROGRESS) 243 return "in-progress"; 244 if (code == GuidanceResponseStatus.FAILURE) 245 return "failure"; 246 if (code == GuidanceResponseStatus.ENTEREDINERROR) 247 return "entered-in-error"; 248 return "?"; 249 } 250 251 public String toSystem(GuidanceResponseStatus code) { 252 return code.getSystem(); 253 } 254 } 255 256 /** 257 * The identifier of the request associated with this response. If an identifier 258 * was given as part of the request, it will be reproduced here to enable the 259 * requester to more easily identify the response in a multi-request scenario. 260 */ 261 @Child(name = "requestIdentifier", type = { 262 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 263 @Description(shortDefinition = "The identifier of the request associated with this response, if any", formalDefinition = "The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.") 264 protected Identifier requestIdentifier; 265 266 /** 267 * Allows a service to provide unique, business identifiers for the response. 268 */ 269 @Child(name = "identifier", type = { 270 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 271 @Description(shortDefinition = "Business identifier", formalDefinition = "Allows a service to provide unique, business identifiers for the response.") 272 protected List<Identifier> identifier; 273 274 /** 275 * An identifier, CodeableConcept or canonical reference to the guidance that 276 * was requested. 277 */ 278 @Child(name = "module", type = { UriType.class, CanonicalType.class, 279 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 280 @Description(shortDefinition = "What guidance was requested", formalDefinition = "An identifier, CodeableConcept or canonical reference to the guidance that was requested.") 281 protected Type module; 282 283 /** 284 * The status of the response. If the evaluation is completed successfully, the 285 * status will indicate success. However, in order to complete the evaluation, 286 * the engine may require more information. In this case, the status will be 287 * data-required, and the response will contain a description of the additional 288 * required information. If the evaluation completed successfully, but the 289 * engine determines that a potentially more accurate response could be provided 290 * if more data was available, the status will be data-requested, and the 291 * response will contain a description of the additional requested information. 292 */ 293 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 294 @Description(shortDefinition = "success | data-requested | data-required | in-progress | failure | entered-in-error", formalDefinition = "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.") 295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/guidance-response-status") 296 protected Enumeration<GuidanceResponseStatus> status; 297 298 /** 299 * The patient for which the request was processed. 300 */ 301 @Child(name = "subject", type = { Patient.class, 302 Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 303 @Description(shortDefinition = "Patient the request was performed for", formalDefinition = "The patient for which the request was processed.") 304 protected Reference subject; 305 306 /** 307 * The actual object that is the target of the reference (The patient for which 308 * the request was processed.) 309 */ 310 protected Resource subjectTarget; 311 312 /** 313 * The encounter during which this response was created or to which the creation 314 * of this record is tightly associated. 315 */ 316 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 317 @Description(shortDefinition = "Encounter during which the response was returned", formalDefinition = "The encounter during which this response was created or to which the creation of this record is tightly associated.") 318 protected Reference encounter; 319 320 /** 321 * The actual object that is the target of the reference (The encounter during 322 * which this response was created or to which the creation of this record is 323 * tightly associated.) 324 */ 325 protected Encounter encounterTarget; 326 327 /** 328 * Indicates when the guidance response was processed. 329 */ 330 @Child(name = "occurrenceDateTime", type = { 331 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 332 @Description(shortDefinition = "When the guidance response was processed", formalDefinition = "Indicates when the guidance response was processed.") 333 protected DateTimeType occurrenceDateTime; 334 335 /** 336 * Provides a reference to the device that performed the guidance. 337 */ 338 @Child(name = "performer", type = { Device.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 339 @Description(shortDefinition = "Device returning the guidance", formalDefinition = "Provides a reference to the device that performed the guidance.") 340 protected Reference performer; 341 342 /** 343 * The actual object that is the target of the reference (Provides a reference 344 * to the device that performed the guidance.) 345 */ 346 protected Device performerTarget; 347 348 /** 349 * Describes the reason for the guidance response in coded or textual form. 350 */ 351 @Child(name = "reasonCode", type = { 352 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 353 @Description(shortDefinition = "Why guidance is needed", formalDefinition = "Describes the reason for the guidance response in coded or textual form.") 354 protected List<CodeableConcept> reasonCode; 355 356 /** 357 * Indicates the reason the request was initiated. This is typically provided as 358 * a parameter to the evaluation and echoed by the service, although for some 359 * use cases, such as subscription- or event-based scenarios, it may provide an 360 * indication of the cause for the response. 361 */ 362 @Child(name = "reasonReference", type = { Condition.class, Observation.class, DiagnosticReport.class, 363 DocumentReference.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 364 @Description(shortDefinition = "Why guidance is needed", formalDefinition = "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.") 365 protected List<Reference> reasonReference; 366 /** 367 * The actual objects that are the target of the reference (Indicates the reason 368 * the request was initiated. This is typically provided as a parameter to the 369 * evaluation and echoed by the service, although for some use cases, such as 370 * subscription- or event-based scenarios, it may provide an indication of the 371 * cause for the response.) 372 */ 373 protected List<Resource> reasonReferenceTarget; 374 375 /** 376 * Provides a mechanism to communicate additional information about the 377 * response. 378 */ 379 @Child(name = "note", type = { 380 Annotation.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 381 @Description(shortDefinition = "Additional notes about the response", formalDefinition = "Provides a mechanism to communicate additional information about the response.") 382 protected List<Annotation> note; 383 384 /** 385 * Messages resulting from the evaluation of the artifact or artifacts. As part 386 * of evaluating the request, the engine may produce informational or warning 387 * messages. These messages will be provided by this element. 388 */ 389 @Child(name = "evaluationMessage", type = { 390 OperationOutcome.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 391 @Description(shortDefinition = "Messages resulting from the evaluation of the artifact or artifacts", formalDefinition = "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.") 392 protected List<Reference> evaluationMessage; 393 /** 394 * The actual objects that are the target of the reference (Messages resulting 395 * from the evaluation of the artifact or artifacts. As part of evaluating the 396 * request, the engine may produce informational or warning messages. These 397 * messages will be provided by this element.) 398 */ 399 protected List<OperationOutcome> evaluationMessageTarget; 400 401 /** 402 * The output parameters of the evaluation, if any. Many modules will result in 403 * the return of specific resources such as procedure or communication requests 404 * that are returned as part of the operation result. However, modules may 405 * define specific outputs that would be returned as the result of the 406 * evaluation, and these would be returned in this element. 407 */ 408 @Child(name = "outputParameters", type = { 409 Parameters.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 410 @Description(shortDefinition = "The output parameters of the evaluation, if any", formalDefinition = "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.") 411 protected Reference outputParameters; 412 413 /** 414 * The actual object that is the target of the reference (The output parameters 415 * of the evaluation, if any. Many modules will result in the return of specific 416 * resources such as procedure or communication requests that are returned as 417 * part of the operation result. However, modules may define specific outputs 418 * that would be returned as the result of the evaluation, and these would be 419 * returned in this element.) 420 */ 421 protected Parameters outputParametersTarget; 422 423 /** 424 * The actions, if any, produced by the evaluation of the artifact. 425 */ 426 @Child(name = "result", type = { CarePlan.class, 427 RequestGroup.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 428 @Description(shortDefinition = "Proposed actions, if any", formalDefinition = "The actions, if any, produced by the evaluation of the artifact.") 429 protected Reference result; 430 431 /** 432 * The actual object that is the target of the reference (The actions, if any, 433 * produced by the evaluation of the artifact.) 434 */ 435 protected Resource resultTarget; 436 437 /** 438 * If the evaluation could not be completed due to lack of information, or 439 * additional information would potentially result in a more accurate response, 440 * this element will a description of the data required in order to proceed with 441 * the evaluation. A subsequent request to the service should include this data. 442 */ 443 @Child(name = "dataRequirement", type = { 444 DataRequirement.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 445 @Description(shortDefinition = "Additional required data", formalDefinition = "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.") 446 protected List<DataRequirement> dataRequirement; 447 448 private static final long serialVersionUID = -760182193L; 449 450 /** 451 * Constructor 452 */ 453 public GuidanceResponse() { 454 super(); 455 } 456 457 /** 458 * Constructor 459 */ 460 public GuidanceResponse(Type module, Enumeration<GuidanceResponseStatus> status) { 461 super(); 462 this.module = module; 463 this.status = status; 464 } 465 466 /** 467 * @return {@link #requestIdentifier} (The identifier of the request associated 468 * with this response. If an identifier was given as part of the 469 * request, it will be reproduced here to enable the requester to more 470 * easily identify the response in a multi-request scenario.) 471 */ 472 public Identifier getRequestIdentifier() { 473 if (this.requestIdentifier == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create GuidanceResponse.requestIdentifier"); 476 else if (Configuration.doAutoCreate()) 477 this.requestIdentifier = new Identifier(); // cc 478 return this.requestIdentifier; 479 } 480 481 public boolean hasRequestIdentifier() { 482 return this.requestIdentifier != null && !this.requestIdentifier.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #requestIdentifier} (The identifier of the request 487 * associated with this response. If an identifier was given as 488 * part of the request, it will be reproduced here to enable the 489 * requester to more easily identify the response in a 490 * multi-request scenario.) 491 */ 492 public GuidanceResponse setRequestIdentifier(Identifier value) { 493 this.requestIdentifier = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #identifier} (Allows a service to provide unique, business 499 * identifiers for the response.) 500 */ 501 public List<Identifier> getIdentifier() { 502 if (this.identifier == null) 503 this.identifier = new ArrayList<Identifier>(); 504 return this.identifier; 505 } 506 507 /** 508 * @return Returns a reference to <code>this</code> for easy method chaining 509 */ 510 public GuidanceResponse setIdentifier(List<Identifier> theIdentifier) { 511 this.identifier = theIdentifier; 512 return this; 513 } 514 515 public boolean hasIdentifier() { 516 if (this.identifier == null) 517 return false; 518 for (Identifier item : this.identifier) 519 if (!item.isEmpty()) 520 return true; 521 return false; 522 } 523 524 public Identifier addIdentifier() { // 3 525 Identifier t = new Identifier(); 526 if (this.identifier == null) 527 this.identifier = new ArrayList<Identifier>(); 528 this.identifier.add(t); 529 return t; 530 } 531 532 public GuidanceResponse addIdentifier(Identifier t) { // 3 533 if (t == null) 534 return this; 535 if (this.identifier == null) 536 this.identifier = new ArrayList<Identifier>(); 537 this.identifier.add(t); 538 return this; 539 } 540 541 /** 542 * @return The first repetition of repeating field {@link #identifier}, creating 543 * it if it does not already exist 544 */ 545 public Identifier getIdentifierFirstRep() { 546 if (getIdentifier().isEmpty()) { 547 addIdentifier(); 548 } 549 return getIdentifier().get(0); 550 } 551 552 /** 553 * @return {@link #module} (An identifier, CodeableConcept or canonical 554 * reference to the guidance that was requested.) 555 */ 556 public Type getModule() { 557 return this.module; 558 } 559 560 /** 561 * @return {@link #module} (An identifier, CodeableConcept or canonical 562 * reference to the guidance that was requested.) 563 */ 564 public UriType getModuleUriType() throws FHIRException { 565 if (this.module == null) 566 this.module = new UriType(); 567 if (!(this.module instanceof UriType)) 568 throw new FHIRException( 569 "Type mismatch: the type UriType was expected, but " + this.module.getClass().getName() + " was encountered"); 570 return (UriType) this.module; 571 } 572 573 public boolean hasModuleUriType() { 574 return this.module instanceof UriType; 575 } 576 577 /** 578 * @return {@link #module} (An identifier, CodeableConcept or canonical 579 * reference to the guidance that was requested.) 580 */ 581 public CanonicalType getModuleCanonicalType() throws FHIRException { 582 if (this.module == null) 583 this.module = new CanonicalType(); 584 if (!(this.module instanceof CanonicalType)) 585 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but " 586 + this.module.getClass().getName() + " was encountered"); 587 return (CanonicalType) this.module; 588 } 589 590 public boolean hasModuleCanonicalType() { 591 return this.module instanceof CanonicalType; 592 } 593 594 /** 595 * @return {@link #module} (An identifier, CodeableConcept or canonical 596 * reference to the guidance that was requested.) 597 */ 598 public CodeableConcept getModuleCodeableConcept() throws FHIRException { 599 if (this.module == null) 600 this.module = new CodeableConcept(); 601 if (!(this.module instanceof CodeableConcept)) 602 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 603 + this.module.getClass().getName() + " was encountered"); 604 return (CodeableConcept) this.module; 605 } 606 607 public boolean hasModuleCodeableConcept() { 608 return this.module instanceof CodeableConcept; 609 } 610 611 public boolean hasModule() { 612 return this.module != null && !this.module.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #module} (An identifier, CodeableConcept or canonical 617 * reference to the guidance that was requested.) 618 */ 619 public GuidanceResponse setModule(Type value) { 620 if (value != null 621 && !(value instanceof UriType || value instanceof CanonicalType || value instanceof CodeableConcept)) 622 throw new Error("Not the right type for GuidanceResponse.module[x]: " + value.fhirType()); 623 this.module = value; 624 return this; 625 } 626 627 /** 628 * @return {@link #status} (The status of the response. If the evaluation is 629 * completed successfully, the status will indicate success. However, in 630 * order to complete the evaluation, the engine may require more 631 * information. In this case, the status will be data-required, and the 632 * response will contain a description of the additional required 633 * information. If the evaluation completed successfully, but the engine 634 * determines that a potentially more accurate response could be 635 * provided if more data was available, the status will be 636 * data-requested, and the response will contain a description of the 637 * additional requested information.). This is the underlying object 638 * with id, value and extensions. The accessor "getStatus" gives direct 639 * access to the value 640 */ 641 public Enumeration<GuidanceResponseStatus> getStatusElement() { 642 if (this.status == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create GuidanceResponse.status"); 645 else if (Configuration.doAutoCreate()) 646 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); // bb 647 return this.status; 648 } 649 650 public boolean hasStatusElement() { 651 return this.status != null && !this.status.isEmpty(); 652 } 653 654 public boolean hasStatus() { 655 return this.status != null && !this.status.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #status} (The status of the response. If the evaluation 660 * is completed successfully, the status will indicate success. 661 * However, in order to complete the evaluation, the engine may 662 * require more information. In this case, the status will be 663 * data-required, and the response will contain a description of 664 * the additional required information. If the evaluation completed 665 * successfully, but the engine determines that a potentially more 666 * accurate response could be provided if more data was available, 667 * the status will be data-requested, and the response will contain 668 * a description of the additional requested information.). This is 669 * the underlying object with id, value and extensions. The 670 * accessor "getStatus" gives direct access to the value 671 */ 672 public GuidanceResponse setStatusElement(Enumeration<GuidanceResponseStatus> value) { 673 this.status = value; 674 return this; 675 } 676 677 /** 678 * @return The status of the response. If the evaluation is completed 679 * successfully, the status will indicate success. However, in order to 680 * complete the evaluation, the engine may require more information. In 681 * this case, the status will be data-required, and the response will 682 * contain a description of the additional required information. If the 683 * evaluation completed successfully, but the engine determines that a 684 * potentially more accurate response could be provided if more data was 685 * available, the status will be data-requested, and the response will 686 * contain a description of the additional requested information. 687 */ 688 public GuidanceResponseStatus getStatus() { 689 return this.status == null ? null : this.status.getValue(); 690 } 691 692 /** 693 * @param value The status of the response. If the evaluation is completed 694 * successfully, the status will indicate success. However, in 695 * order to complete the evaluation, the engine may require more 696 * information. In this case, the status will be data-required, and 697 * the response will contain a description of the additional 698 * required information. If the evaluation completed successfully, 699 * but the engine determines that a potentially more accurate 700 * response could be provided if more data was available, the 701 * status will be data-requested, and the response will contain a 702 * description of the additional requested information. 703 */ 704 public GuidanceResponse setStatus(GuidanceResponseStatus value) { 705 if (this.status == null) 706 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); 707 this.status.setValue(value); 708 return this; 709 } 710 711 /** 712 * @return {@link #subject} (The patient for which the request was processed.) 713 */ 714 public Reference getSubject() { 715 if (this.subject == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create GuidanceResponse.subject"); 718 else if (Configuration.doAutoCreate()) 719 this.subject = new Reference(); // cc 720 return this.subject; 721 } 722 723 public boolean hasSubject() { 724 return this.subject != null && !this.subject.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #subject} (The patient for which the request was 729 * processed.) 730 */ 731 public GuidanceResponse setSubject(Reference value) { 732 this.subject = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #subject} The actual object that is the target of the 738 * reference. The reference library doesn't populate this, but you can 739 * use it to hold the resource if you resolve it. (The patient for which 740 * the request was processed.) 741 */ 742 public Resource getSubjectTarget() { 743 return this.subjectTarget; 744 } 745 746 /** 747 * @param value {@link #subject} The actual object that is the target of the 748 * reference. The reference library doesn't use these, but you can 749 * use it to hold the resource if you resolve it. (The patient for 750 * which the request was processed.) 751 */ 752 public GuidanceResponse setSubjectTarget(Resource value) { 753 this.subjectTarget = value; 754 return this; 755 } 756 757 /** 758 * @return {@link #encounter} (The encounter during which this response was 759 * created or to which the creation of this record is tightly 760 * associated.) 761 */ 762 public Reference getEncounter() { 763 if (this.encounter == null) 764 if (Configuration.errorOnAutoCreate()) 765 throw new Error("Attempt to auto-create GuidanceResponse.encounter"); 766 else if (Configuration.doAutoCreate()) 767 this.encounter = new Reference(); // cc 768 return this.encounter; 769 } 770 771 public boolean hasEncounter() { 772 return this.encounter != null && !this.encounter.isEmpty(); 773 } 774 775 /** 776 * @param value {@link #encounter} (The encounter during which this response was 777 * created or to which the creation of this record is tightly 778 * associated.) 779 */ 780 public GuidanceResponse setEncounter(Reference value) { 781 this.encounter = value; 782 return this; 783 } 784 785 /** 786 * @return {@link #encounter} The actual object that is the target of the 787 * reference. The reference library doesn't populate this, but you can 788 * use it to hold the resource if you resolve it. (The encounter during 789 * which this response was created or to which the creation of this 790 * record is tightly associated.) 791 */ 792 public Encounter getEncounterTarget() { 793 if (this.encounterTarget == null) 794 if (Configuration.errorOnAutoCreate()) 795 throw new Error("Attempt to auto-create GuidanceResponse.encounter"); 796 else if (Configuration.doAutoCreate()) 797 this.encounterTarget = new Encounter(); // aa 798 return this.encounterTarget; 799 } 800 801 /** 802 * @param value {@link #encounter} The actual object that is the target of the 803 * reference. The reference library doesn't use these, but you can 804 * use it to hold the resource if you resolve it. (The encounter 805 * during which this response was created or to which the creation 806 * of this record is tightly associated.) 807 */ 808 public GuidanceResponse setEncounterTarget(Encounter value) { 809 this.encounterTarget = value; 810 return this; 811 } 812 813 /** 814 * @return {@link #occurrenceDateTime} (Indicates when the guidance response was 815 * processed.). This is the underlying object with id, value and 816 * extensions. The accessor "getOccurrenceDateTime" gives direct access 817 * to the value 818 */ 819 public DateTimeType getOccurrenceDateTimeElement() { 820 if (this.occurrenceDateTime == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create GuidanceResponse.occurrenceDateTime"); 823 else if (Configuration.doAutoCreate()) 824 this.occurrenceDateTime = new DateTimeType(); // bb 825 return this.occurrenceDateTime; 826 } 827 828 public boolean hasOccurrenceDateTimeElement() { 829 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 830 } 831 832 public boolean hasOccurrenceDateTime() { 833 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 834 } 835 836 /** 837 * @param value {@link #occurrenceDateTime} (Indicates when the guidance 838 * response was processed.). This is the underlying object with id, 839 * value and extensions. The accessor "getOccurrenceDateTime" gives 840 * direct access to the value 841 */ 842 public GuidanceResponse setOccurrenceDateTimeElement(DateTimeType value) { 843 this.occurrenceDateTime = value; 844 return this; 845 } 846 847 /** 848 * @return Indicates when the guidance response was processed. 849 */ 850 public Date getOccurrenceDateTime() { 851 return this.occurrenceDateTime == null ? null : this.occurrenceDateTime.getValue(); 852 } 853 854 /** 855 * @param value Indicates when the guidance response was processed. 856 */ 857 public GuidanceResponse setOccurrenceDateTime(Date value) { 858 if (value == null) 859 this.occurrenceDateTime = null; 860 else { 861 if (this.occurrenceDateTime == null) 862 this.occurrenceDateTime = new DateTimeType(); 863 this.occurrenceDateTime.setValue(value); 864 } 865 return this; 866 } 867 868 /** 869 * @return {@link #performer} (Provides a reference to the device that performed 870 * the guidance.) 871 */ 872 public Reference getPerformer() { 873 if (this.performer == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create GuidanceResponse.performer"); 876 else if (Configuration.doAutoCreate()) 877 this.performer = new Reference(); // cc 878 return this.performer; 879 } 880 881 public boolean hasPerformer() { 882 return this.performer != null && !this.performer.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #performer} (Provides a reference to the device that 887 * performed the guidance.) 888 */ 889 public GuidanceResponse setPerformer(Reference value) { 890 this.performer = value; 891 return this; 892 } 893 894 /** 895 * @return {@link #performer} The actual object that is the target of the 896 * reference. The reference library doesn't populate this, but you can 897 * use it to hold the resource if you resolve it. (Provides a reference 898 * to the device that performed the guidance.) 899 */ 900 public Device getPerformerTarget() { 901 if (this.performerTarget == null) 902 if (Configuration.errorOnAutoCreate()) 903 throw new Error("Attempt to auto-create GuidanceResponse.performer"); 904 else if (Configuration.doAutoCreate()) 905 this.performerTarget = new Device(); // aa 906 return this.performerTarget; 907 } 908 909 /** 910 * @param value {@link #performer} The actual object that is the target of the 911 * reference. The reference library doesn't use these, but you can 912 * use it to hold the resource if you resolve it. (Provides a 913 * reference to the device that performed the guidance.) 914 */ 915 public GuidanceResponse setPerformerTarget(Device value) { 916 this.performerTarget = value; 917 return this; 918 } 919 920 /** 921 * @return {@link #reasonCode} (Describes the reason for the guidance response 922 * in coded or textual form.) 923 */ 924 public List<CodeableConcept> getReasonCode() { 925 if (this.reasonCode == null) 926 this.reasonCode = new ArrayList<CodeableConcept>(); 927 return this.reasonCode; 928 } 929 930 /** 931 * @return Returns a reference to <code>this</code> for easy method chaining 932 */ 933 public GuidanceResponse setReasonCode(List<CodeableConcept> theReasonCode) { 934 this.reasonCode = theReasonCode; 935 return this; 936 } 937 938 public boolean hasReasonCode() { 939 if (this.reasonCode == null) 940 return false; 941 for (CodeableConcept item : this.reasonCode) 942 if (!item.isEmpty()) 943 return true; 944 return false; 945 } 946 947 public CodeableConcept addReasonCode() { // 3 948 CodeableConcept t = new CodeableConcept(); 949 if (this.reasonCode == null) 950 this.reasonCode = new ArrayList<CodeableConcept>(); 951 this.reasonCode.add(t); 952 return t; 953 } 954 955 public GuidanceResponse addReasonCode(CodeableConcept t) { // 3 956 if (t == null) 957 return this; 958 if (this.reasonCode == null) 959 this.reasonCode = new ArrayList<CodeableConcept>(); 960 this.reasonCode.add(t); 961 return this; 962 } 963 964 /** 965 * @return The first repetition of repeating field {@link #reasonCode}, creating 966 * it if it does not already exist 967 */ 968 public CodeableConcept getReasonCodeFirstRep() { 969 if (getReasonCode().isEmpty()) { 970 addReasonCode(); 971 } 972 return getReasonCode().get(0); 973 } 974 975 /** 976 * @return {@link #reasonReference} (Indicates the reason the request was 977 * initiated. This is typically provided as a parameter to the 978 * evaluation and echoed by the service, although for some use cases, 979 * such as subscription- or event-based scenarios, it may provide an 980 * indication of the cause for the response.) 981 */ 982 public List<Reference> getReasonReference() { 983 if (this.reasonReference == null) 984 this.reasonReference = new ArrayList<Reference>(); 985 return this.reasonReference; 986 } 987 988 /** 989 * @return Returns a reference to <code>this</code> for easy method chaining 990 */ 991 public GuidanceResponse setReasonReference(List<Reference> theReasonReference) { 992 this.reasonReference = theReasonReference; 993 return this; 994 } 995 996 public boolean hasReasonReference() { 997 if (this.reasonReference == null) 998 return false; 999 for (Reference item : this.reasonReference) 1000 if (!item.isEmpty()) 1001 return true; 1002 return false; 1003 } 1004 1005 public Reference addReasonReference() { // 3 1006 Reference t = new Reference(); 1007 if (this.reasonReference == null) 1008 this.reasonReference = new ArrayList<Reference>(); 1009 this.reasonReference.add(t); 1010 return t; 1011 } 1012 1013 public GuidanceResponse addReasonReference(Reference t) { // 3 1014 if (t == null) 1015 return this; 1016 if (this.reasonReference == null) 1017 this.reasonReference = new ArrayList<Reference>(); 1018 this.reasonReference.add(t); 1019 return this; 1020 } 1021 1022 /** 1023 * @return The first repetition of repeating field {@link #reasonReference}, 1024 * creating it if it does not already exist 1025 */ 1026 public Reference getReasonReferenceFirstRep() { 1027 if (getReasonReference().isEmpty()) { 1028 addReasonReference(); 1029 } 1030 return getReasonReference().get(0); 1031 } 1032 1033 /** 1034 * @return {@link #note} (Provides a mechanism to communicate additional 1035 * information about the response.) 1036 */ 1037 public List<Annotation> getNote() { 1038 if (this.note == null) 1039 this.note = new ArrayList<Annotation>(); 1040 return this.note; 1041 } 1042 1043 /** 1044 * @return Returns a reference to <code>this</code> for easy method chaining 1045 */ 1046 public GuidanceResponse setNote(List<Annotation> theNote) { 1047 this.note = theNote; 1048 return this; 1049 } 1050 1051 public boolean hasNote() { 1052 if (this.note == null) 1053 return false; 1054 for (Annotation item : this.note) 1055 if (!item.isEmpty()) 1056 return true; 1057 return false; 1058 } 1059 1060 public Annotation addNote() { // 3 1061 Annotation t = new Annotation(); 1062 if (this.note == null) 1063 this.note = new ArrayList<Annotation>(); 1064 this.note.add(t); 1065 return t; 1066 } 1067 1068 public GuidanceResponse addNote(Annotation t) { // 3 1069 if (t == null) 1070 return this; 1071 if (this.note == null) 1072 this.note = new ArrayList<Annotation>(); 1073 this.note.add(t); 1074 return this; 1075 } 1076 1077 /** 1078 * @return The first repetition of repeating field {@link #note}, creating it if 1079 * it does not already exist 1080 */ 1081 public Annotation getNoteFirstRep() { 1082 if (getNote().isEmpty()) { 1083 addNote(); 1084 } 1085 return getNote().get(0); 1086 } 1087 1088 /** 1089 * @return {@link #evaluationMessage} (Messages resulting from the evaluation of 1090 * the artifact or artifacts. As part of evaluating the request, the 1091 * engine may produce informational or warning messages. These messages 1092 * will be provided by this element.) 1093 */ 1094 public List<Reference> getEvaluationMessage() { 1095 if (this.evaluationMessage == null) 1096 this.evaluationMessage = new ArrayList<Reference>(); 1097 return this.evaluationMessage; 1098 } 1099 1100 /** 1101 * @return Returns a reference to <code>this</code> for easy method chaining 1102 */ 1103 public GuidanceResponse setEvaluationMessage(List<Reference> theEvaluationMessage) { 1104 this.evaluationMessage = theEvaluationMessage; 1105 return this; 1106 } 1107 1108 public boolean hasEvaluationMessage() { 1109 if (this.evaluationMessage == null) 1110 return false; 1111 for (Reference item : this.evaluationMessage) 1112 if (!item.isEmpty()) 1113 return true; 1114 return false; 1115 } 1116 1117 public Reference addEvaluationMessage() { // 3 1118 Reference t = new Reference(); 1119 if (this.evaluationMessage == null) 1120 this.evaluationMessage = new ArrayList<Reference>(); 1121 this.evaluationMessage.add(t); 1122 return t; 1123 } 1124 1125 public GuidanceResponse addEvaluationMessage(Reference t) { // 3 1126 if (t == null) 1127 return this; 1128 if (this.evaluationMessage == null) 1129 this.evaluationMessage = new ArrayList<Reference>(); 1130 this.evaluationMessage.add(t); 1131 return this; 1132 } 1133 1134 /** 1135 * @return The first repetition of repeating field {@link #evaluationMessage}, 1136 * creating it if it does not already exist 1137 */ 1138 public Reference getEvaluationMessageFirstRep() { 1139 if (getEvaluationMessage().isEmpty()) { 1140 addEvaluationMessage(); 1141 } 1142 return getEvaluationMessage().get(0); 1143 } 1144 1145 /** 1146 * @return {@link #outputParameters} (The output parameters of the evaluation, 1147 * if any. Many modules will result in the return of specific resources 1148 * such as procedure or communication requests that are returned as part 1149 * of the operation result. However, modules may define specific outputs 1150 * that would be returned as the result of the evaluation, and these 1151 * would be returned in this element.) 1152 */ 1153 public Reference getOutputParameters() { 1154 if (this.outputParameters == null) 1155 if (Configuration.errorOnAutoCreate()) 1156 throw new Error("Attempt to auto-create GuidanceResponse.outputParameters"); 1157 else if (Configuration.doAutoCreate()) 1158 this.outputParameters = new Reference(); // cc 1159 return this.outputParameters; 1160 } 1161 1162 public boolean hasOutputParameters() { 1163 return this.outputParameters != null && !this.outputParameters.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #outputParameters} (The output parameters of the 1168 * evaluation, if any. Many modules will result in the return of 1169 * specific resources such as procedure or communication requests 1170 * that are returned as part of the operation result. However, 1171 * modules may define specific outputs that would be returned as 1172 * the result of the evaluation, and these would be returned in 1173 * this element.) 1174 */ 1175 public GuidanceResponse setOutputParameters(Reference value) { 1176 this.outputParameters = value; 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #outputParameters} The actual object that is the target of the 1182 * reference. The reference library doesn't populate this, but you can 1183 * use it to hold the resource if you resolve it. (The output parameters 1184 * of the evaluation, if any. Many modules will result in the return of 1185 * specific resources such as procedure or communication requests that 1186 * are returned as part of the operation result. However, modules may 1187 * define specific outputs that would be returned as the result of the 1188 * evaluation, and these would be returned in this element.) 1189 */ 1190 public Parameters getOutputParametersTarget() { 1191 if (this.outputParametersTarget == null) 1192 if (Configuration.errorOnAutoCreate()) 1193 throw new Error("Attempt to auto-create GuidanceResponse.outputParameters"); 1194 else if (Configuration.doAutoCreate()) 1195 this.outputParametersTarget = new Parameters(); // aa 1196 return this.outputParametersTarget; 1197 } 1198 1199 /** 1200 * @param value {@link #outputParameters} The actual object that is the target 1201 * of the reference. The reference library doesn't use these, but 1202 * you can use it to hold the resource if you resolve it. (The 1203 * output parameters of the evaluation, if any. Many modules will 1204 * result in the return of specific resources such as procedure or 1205 * communication requests that are returned as part of the 1206 * operation result. However, modules may define specific outputs 1207 * that would be returned as the result of the evaluation, and 1208 * these would be returned in this element.) 1209 */ 1210 public GuidanceResponse setOutputParametersTarget(Parameters value) { 1211 this.outputParametersTarget = value; 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #result} (The actions, if any, produced by the evaluation of 1217 * the artifact.) 1218 */ 1219 public Reference getResult() { 1220 if (this.result == null) 1221 if (Configuration.errorOnAutoCreate()) 1222 throw new Error("Attempt to auto-create GuidanceResponse.result"); 1223 else if (Configuration.doAutoCreate()) 1224 this.result = new Reference(); // cc 1225 return this.result; 1226 } 1227 1228 public boolean hasResult() { 1229 return this.result != null && !this.result.isEmpty(); 1230 } 1231 1232 /** 1233 * @param value {@link #result} (The actions, if any, produced by the evaluation 1234 * of the artifact.) 1235 */ 1236 public GuidanceResponse setResult(Reference value) { 1237 this.result = value; 1238 return this; 1239 } 1240 1241 /** 1242 * @return {@link #result} The actual object that is the target of the 1243 * reference. The reference library doesn't populate this, but you can 1244 * use it to hold the resource if you resolve it. (The actions, if any, 1245 * produced by the evaluation of the artifact.) 1246 */ 1247 public Resource getResultTarget() { 1248 return this.resultTarget; 1249 } 1250 1251 /** 1252 * @param value {@link #result} The actual object that is the target of the 1253 * reference. The reference library doesn't use these, but you can 1254 * use it to hold the resource if you resolve it. (The actions, if 1255 * any, produced by the evaluation of the artifact.) 1256 */ 1257 public GuidanceResponse setResultTarget(Resource value) { 1258 this.resultTarget = value; 1259 return this; 1260 } 1261 1262 /** 1263 * @return {@link #dataRequirement} (If the evaluation could not be completed 1264 * due to lack of information, or additional information would 1265 * potentially result in a more accurate response, this element will a 1266 * description of the data required in order to proceed with the 1267 * evaluation. A subsequent request to the service should include this 1268 * data.) 1269 */ 1270 public List<DataRequirement> getDataRequirement() { 1271 if (this.dataRequirement == null) 1272 this.dataRequirement = new ArrayList<DataRequirement>(); 1273 return this.dataRequirement; 1274 } 1275 1276 /** 1277 * @return Returns a reference to <code>this</code> for easy method chaining 1278 */ 1279 public GuidanceResponse setDataRequirement(List<DataRequirement> theDataRequirement) { 1280 this.dataRequirement = theDataRequirement; 1281 return this; 1282 } 1283 1284 public boolean hasDataRequirement() { 1285 if (this.dataRequirement == null) 1286 return false; 1287 for (DataRequirement item : this.dataRequirement) 1288 if (!item.isEmpty()) 1289 return true; 1290 return false; 1291 } 1292 1293 public DataRequirement addDataRequirement() { // 3 1294 DataRequirement t = new DataRequirement(); 1295 if (this.dataRequirement == null) 1296 this.dataRequirement = new ArrayList<DataRequirement>(); 1297 this.dataRequirement.add(t); 1298 return t; 1299 } 1300 1301 public GuidanceResponse addDataRequirement(DataRequirement t) { // 3 1302 if (t == null) 1303 return this; 1304 if (this.dataRequirement == null) 1305 this.dataRequirement = new ArrayList<DataRequirement>(); 1306 this.dataRequirement.add(t); 1307 return this; 1308 } 1309 1310 /** 1311 * @return The first repetition of repeating field {@link #dataRequirement}, 1312 * creating it if it does not already exist 1313 */ 1314 public DataRequirement getDataRequirementFirstRep() { 1315 if (getDataRequirement().isEmpty()) { 1316 addDataRequirement(); 1317 } 1318 return getDataRequirement().get(0); 1319 } 1320 1321 protected void listChildren(List<Property> children) { 1322 super.listChildren(children); 1323 children.add(new Property("requestIdentifier", "Identifier", 1324 "The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 1325 0, 1, requestIdentifier)); 1326 children.add(new Property("identifier", "Identifier", 1327 "Allows a service to provide unique, business identifiers for the response.", 0, java.lang.Integer.MAX_VALUE, 1328 identifier)); 1329 children.add(new Property("module[x]", "uri|canonical|CodeableConcept", 1330 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module)); 1331 children.add(new Property("status", "code", 1332 "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 1333 0, 1, status)); 1334 children.add(new Property("subject", "Reference(Patient|Group)", "The patient for which the request was processed.", 1335 0, 1, subject)); 1336 children.add(new Property("encounter", "Reference(Encounter)", 1337 "The encounter during which this response was created or to which the creation of this record is tightly associated.", 1338 0, 1, encounter)); 1339 children.add(new Property("occurrenceDateTime", "dateTime", "Indicates when the guidance response was processed.", 1340 0, 1, occurrenceDateTime)); 1341 children.add(new Property("performer", "Reference(Device)", 1342 "Provides a reference to the device that performed the guidance.", 0, 1, performer)); 1343 children.add(new Property("reasonCode", "CodeableConcept", 1344 "Describes the reason for the guidance response in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 1345 reasonCode)); 1346 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1347 "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 1348 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1349 children.add(new Property("note", "Annotation", 1350 "Provides a mechanism to communicate additional information about the response.", 0, 1351 java.lang.Integer.MAX_VALUE, note)); 1352 children.add(new Property("evaluationMessage", "Reference(OperationOutcome)", 1353 "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 1354 0, java.lang.Integer.MAX_VALUE, evaluationMessage)); 1355 children.add(new Property("outputParameters", "Reference(Parameters)", 1356 "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 1357 0, 1, outputParameters)); 1358 children.add(new Property("result", "Reference(CarePlan|RequestGroup)", 1359 "The actions, if any, produced by the evaluation of the artifact.", 0, 1, result)); 1360 children.add(new Property("dataRequirement", "DataRequirement", 1361 "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 1362 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 1363 } 1364 1365 @Override 1366 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1367 switch (_hash) { 1368 case -354233192: 1369 /* requestIdentifier */ return new Property("requestIdentifier", "Identifier", 1370 "The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 1371 0, 1, requestIdentifier); 1372 case -1618432855: 1373 /* identifier */ return new Property("identifier", "Identifier", 1374 "Allows a service to provide unique, business identifiers for the response.", 0, java.lang.Integer.MAX_VALUE, 1375 identifier); 1376 case -1552083308: 1377 /* module[x] */ return new Property("module[x]", "uri|canonical|CodeableConcept", 1378 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 1379 case -1068784020: 1380 /* module */ return new Property("module[x]", "uri|canonical|CodeableConcept", 1381 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 1382 case -1552089248: 1383 /* moduleUri */ return new Property("module[x]", "uri|canonical|CodeableConcept", 1384 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 1385 case -1153656856: 1386 /* moduleCanonical */ return new Property("module[x]", "uri|canonical|CodeableConcept", 1387 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 1388 case -1157899371: 1389 /* moduleCodeableConcept */ return new Property("module[x]", "uri|canonical|CodeableConcept", 1390 "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 1391 case -892481550: 1392 /* status */ return new Property("status", "code", 1393 "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 1394 0, 1, status); 1395 case -1867885268: 1396 /* subject */ return new Property("subject", "Reference(Patient|Group)", 1397 "The patient for which the request was processed.", 0, 1, subject); 1398 case 1524132147: 1399 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1400 "The encounter during which this response was created or to which the creation of this record is tightly associated.", 1401 0, 1, encounter); 1402 case -298443636: 1403 /* occurrenceDateTime */ return new Property("occurrenceDateTime", "dateTime", 1404 "Indicates when the guidance response was processed.", 0, 1, occurrenceDateTime); 1405 case 481140686: 1406 /* performer */ return new Property("performer", "Reference(Device)", 1407 "Provides a reference to the device that performed the guidance.", 0, 1, performer); 1408 case 722137681: 1409 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1410 "Describes the reason for the guidance response in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, 1411 reasonCode); 1412 case -1146218137: 1413 /* reasonReference */ return new Property("reasonReference", 1414 "Reference(Condition|Observation|DiagnosticReport|DocumentReference)", 1415 "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 1416 0, java.lang.Integer.MAX_VALUE, reasonReference); 1417 case 3387378: 1418 /* note */ return new Property("note", "Annotation", 1419 "Provides a mechanism to communicate additional information about the response.", 0, 1420 java.lang.Integer.MAX_VALUE, note); 1421 case 1081619755: 1422 /* evaluationMessage */ return new Property("evaluationMessage", "Reference(OperationOutcome)", 1423 "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 1424 0, java.lang.Integer.MAX_VALUE, evaluationMessage); 1425 case 525609419: 1426 /* outputParameters */ return new Property("outputParameters", "Reference(Parameters)", 1427 "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 1428 0, 1, outputParameters); 1429 case -934426595: 1430 /* result */ return new Property("result", "Reference(CarePlan|RequestGroup)", 1431 "The actions, if any, produced by the evaluation of the artifact.", 0, 1, result); 1432 case 629147193: 1433 /* dataRequirement */ return new Property("dataRequirement", "DataRequirement", 1434 "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 1435 0, java.lang.Integer.MAX_VALUE, dataRequirement); 1436 default: 1437 return super.getNamedProperty(_hash, _name, _checkValid); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1444 switch (hash) { 1445 case -354233192: 1446 /* requestIdentifier */ return this.requestIdentifier == null ? new Base[0] 1447 : new Base[] { this.requestIdentifier }; // Identifier 1448 case -1618432855: 1449 /* identifier */ return this.identifier == null ? new Base[0] 1450 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1451 case -1068784020: 1452 /* module */ return this.module == null ? new Base[0] : new Base[] { this.module }; // Type 1453 case -892481550: 1454 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<GuidanceResponseStatus> 1455 case -1867885268: 1456 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1457 case 1524132147: 1458 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1459 case -298443636: 1460 /* occurrenceDateTime */ return this.occurrenceDateTime == null ? new Base[0] 1461 : new Base[] { this.occurrenceDateTime }; // DateTimeType 1462 case 481140686: 1463 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 1464 case 722137681: 1465 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1466 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1467 case -1146218137: 1468 /* reasonReference */ return this.reasonReference == null ? new Base[0] 1469 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1470 case 3387378: 1471 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1472 case 1081619755: 1473 /* evaluationMessage */ return this.evaluationMessage == null ? new Base[0] 1474 : this.evaluationMessage.toArray(new Base[this.evaluationMessage.size()]); // Reference 1475 case 525609419: 1476 /* outputParameters */ return this.outputParameters == null ? new Base[0] : new Base[] { this.outputParameters }; // Reference 1477 case -934426595: 1478 /* result */ return this.result == null ? new Base[0] : new Base[] { this.result }; // Reference 1479 case 629147193: 1480 /* dataRequirement */ return this.dataRequirement == null ? new Base[0] 1481 : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 1482 default: 1483 return super.getProperty(hash, name, checkValid); 1484 } 1485 1486 } 1487 1488 @Override 1489 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1490 switch (hash) { 1491 case -354233192: // requestIdentifier 1492 this.requestIdentifier = castToIdentifier(value); // Identifier 1493 return value; 1494 case -1618432855: // identifier 1495 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1496 return value; 1497 case -1068784020: // module 1498 this.module = castToType(value); // Type 1499 return value; 1500 case -892481550: // status 1501 value = new GuidanceResponseStatusEnumFactory().fromType(castToCode(value)); 1502 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1503 return value; 1504 case -1867885268: // subject 1505 this.subject = castToReference(value); // Reference 1506 return value; 1507 case 1524132147: // encounter 1508 this.encounter = castToReference(value); // Reference 1509 return value; 1510 case -298443636: // occurrenceDateTime 1511 this.occurrenceDateTime = castToDateTime(value); // DateTimeType 1512 return value; 1513 case 481140686: // performer 1514 this.performer = castToReference(value); // Reference 1515 return value; 1516 case 722137681: // reasonCode 1517 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1518 return value; 1519 case -1146218137: // reasonReference 1520 this.getReasonReference().add(castToReference(value)); // Reference 1521 return value; 1522 case 3387378: // note 1523 this.getNote().add(castToAnnotation(value)); // Annotation 1524 return value; 1525 case 1081619755: // evaluationMessage 1526 this.getEvaluationMessage().add(castToReference(value)); // Reference 1527 return value; 1528 case 525609419: // outputParameters 1529 this.outputParameters = castToReference(value); // Reference 1530 return value; 1531 case -934426595: // result 1532 this.result = castToReference(value); // Reference 1533 return value; 1534 case 629147193: // dataRequirement 1535 this.getDataRequirement().add(castToDataRequirement(value)); // DataRequirement 1536 return value; 1537 default: 1538 return super.setProperty(hash, name, value); 1539 } 1540 1541 } 1542 1543 @Override 1544 public Base setProperty(String name, Base value) throws FHIRException { 1545 if (name.equals("requestIdentifier")) { 1546 this.requestIdentifier = castToIdentifier(value); // Identifier 1547 } else if (name.equals("identifier")) { 1548 this.getIdentifier().add(castToIdentifier(value)); 1549 } else if (name.equals("module[x]")) { 1550 this.module = castToType(value); // Type 1551 } else if (name.equals("status")) { 1552 value = new GuidanceResponseStatusEnumFactory().fromType(castToCode(value)); 1553 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1554 } else if (name.equals("subject")) { 1555 this.subject = castToReference(value); // Reference 1556 } else if (name.equals("encounter")) { 1557 this.encounter = castToReference(value); // Reference 1558 } else if (name.equals("occurrenceDateTime")) { 1559 this.occurrenceDateTime = castToDateTime(value); // DateTimeType 1560 } else if (name.equals("performer")) { 1561 this.performer = castToReference(value); // Reference 1562 } else if (name.equals("reasonCode")) { 1563 this.getReasonCode().add(castToCodeableConcept(value)); 1564 } else if (name.equals("reasonReference")) { 1565 this.getReasonReference().add(castToReference(value)); 1566 } else if (name.equals("note")) { 1567 this.getNote().add(castToAnnotation(value)); 1568 } else if (name.equals("evaluationMessage")) { 1569 this.getEvaluationMessage().add(castToReference(value)); 1570 } else if (name.equals("outputParameters")) { 1571 this.outputParameters = castToReference(value); // Reference 1572 } else if (name.equals("result")) { 1573 this.result = castToReference(value); // Reference 1574 } else if (name.equals("dataRequirement")) { 1575 this.getDataRequirement().add(castToDataRequirement(value)); 1576 } else 1577 return super.setProperty(name, value); 1578 return value; 1579 } 1580 1581 @Override 1582 public void removeChild(String name, Base value) throws FHIRException { 1583 if (name.equals("requestIdentifier")) { 1584 this.requestIdentifier = null; 1585 } else if (name.equals("identifier")) { 1586 this.getIdentifier().remove(castToIdentifier(value)); 1587 } else if (name.equals("module[x]")) { 1588 this.module = null; 1589 } else if (name.equals("status")) { 1590 this.status = null; 1591 } else if (name.equals("subject")) { 1592 this.subject = null; 1593 } else if (name.equals("encounter")) { 1594 this.encounter = null; 1595 } else if (name.equals("occurrenceDateTime")) { 1596 this.occurrenceDateTime = null; 1597 } else if (name.equals("performer")) { 1598 this.performer = null; 1599 } else if (name.equals("reasonCode")) { 1600 this.getReasonCode().remove(castToCodeableConcept(value)); 1601 } else if (name.equals("reasonReference")) { 1602 this.getReasonReference().remove(castToReference(value)); 1603 } else if (name.equals("note")) { 1604 this.getNote().remove(castToAnnotation(value)); 1605 } else if (name.equals("evaluationMessage")) { 1606 this.getEvaluationMessage().remove(castToReference(value)); 1607 } else if (name.equals("outputParameters")) { 1608 this.outputParameters = null; 1609 } else if (name.equals("result")) { 1610 this.result = null; 1611 } else if (name.equals("dataRequirement")) { 1612 this.getDataRequirement().remove(castToDataRequirement(value)); 1613 } else 1614 super.removeChild(name, value); 1615 1616 } 1617 1618 @Override 1619 public Base makeProperty(int hash, String name) throws FHIRException { 1620 switch (hash) { 1621 case -354233192: 1622 return getRequestIdentifier(); 1623 case -1618432855: 1624 return addIdentifier(); 1625 case -1552083308: 1626 return getModule(); 1627 case -1068784020: 1628 return getModule(); 1629 case -892481550: 1630 return getStatusElement(); 1631 case -1867885268: 1632 return getSubject(); 1633 case 1524132147: 1634 return getEncounter(); 1635 case -298443636: 1636 return getOccurrenceDateTimeElement(); 1637 case 481140686: 1638 return getPerformer(); 1639 case 722137681: 1640 return addReasonCode(); 1641 case -1146218137: 1642 return addReasonReference(); 1643 case 3387378: 1644 return addNote(); 1645 case 1081619755: 1646 return addEvaluationMessage(); 1647 case 525609419: 1648 return getOutputParameters(); 1649 case -934426595: 1650 return getResult(); 1651 case 629147193: 1652 return addDataRequirement(); 1653 default: 1654 return super.makeProperty(hash, name); 1655 } 1656 1657 } 1658 1659 @Override 1660 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1661 switch (hash) { 1662 case -354233192: 1663 /* requestIdentifier */ return new String[] { "Identifier" }; 1664 case -1618432855: 1665 /* identifier */ return new String[] { "Identifier" }; 1666 case -1068784020: 1667 /* module */ return new String[] { "uri", "canonical", "CodeableConcept" }; 1668 case -892481550: 1669 /* status */ return new String[] { "code" }; 1670 case -1867885268: 1671 /* subject */ return new String[] { "Reference" }; 1672 case 1524132147: 1673 /* encounter */ return new String[] { "Reference" }; 1674 case -298443636: 1675 /* occurrenceDateTime */ return new String[] { "dateTime" }; 1676 case 481140686: 1677 /* performer */ return new String[] { "Reference" }; 1678 case 722137681: 1679 /* reasonCode */ return new String[] { "CodeableConcept" }; 1680 case -1146218137: 1681 /* reasonReference */ return new String[] { "Reference" }; 1682 case 3387378: 1683 /* note */ return new String[] { "Annotation" }; 1684 case 1081619755: 1685 /* evaluationMessage */ return new String[] { "Reference" }; 1686 case 525609419: 1687 /* outputParameters */ return new String[] { "Reference" }; 1688 case -934426595: 1689 /* result */ return new String[] { "Reference" }; 1690 case 629147193: 1691 /* dataRequirement */ return new String[] { "DataRequirement" }; 1692 default: 1693 return super.getTypesForProperty(hash, name); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base addChild(String name) throws FHIRException { 1700 if (name.equals("requestIdentifier")) { 1701 this.requestIdentifier = new Identifier(); 1702 return this.requestIdentifier; 1703 } else if (name.equals("identifier")) { 1704 return addIdentifier(); 1705 } else if (name.equals("moduleUri")) { 1706 this.module = new UriType(); 1707 return this.module; 1708 } else if (name.equals("moduleCanonical")) { 1709 this.module = new CanonicalType(); 1710 return this.module; 1711 } else if (name.equals("moduleCodeableConcept")) { 1712 this.module = new CodeableConcept(); 1713 return this.module; 1714 } else if (name.equals("status")) { 1715 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.status"); 1716 } else if (name.equals("subject")) { 1717 this.subject = new Reference(); 1718 return this.subject; 1719 } else if (name.equals("encounter")) { 1720 this.encounter = new Reference(); 1721 return this.encounter; 1722 } else if (name.equals("occurrenceDateTime")) { 1723 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.occurrenceDateTime"); 1724 } else if (name.equals("performer")) { 1725 this.performer = new Reference(); 1726 return this.performer; 1727 } else if (name.equals("reasonCode")) { 1728 return addReasonCode(); 1729 } else if (name.equals("reasonReference")) { 1730 return addReasonReference(); 1731 } else if (name.equals("note")) { 1732 return addNote(); 1733 } else if (name.equals("evaluationMessage")) { 1734 return addEvaluationMessage(); 1735 } else if (name.equals("outputParameters")) { 1736 this.outputParameters = new Reference(); 1737 return this.outputParameters; 1738 } else if (name.equals("result")) { 1739 this.result = new Reference(); 1740 return this.result; 1741 } else if (name.equals("dataRequirement")) { 1742 return addDataRequirement(); 1743 } else 1744 return super.addChild(name); 1745 } 1746 1747 public String fhirType() { 1748 return "GuidanceResponse"; 1749 1750 } 1751 1752 public GuidanceResponse copy() { 1753 GuidanceResponse dst = new GuidanceResponse(); 1754 copyValues(dst); 1755 return dst; 1756 } 1757 1758 public void copyValues(GuidanceResponse dst) { 1759 super.copyValues(dst); 1760 dst.requestIdentifier = requestIdentifier == null ? null : requestIdentifier.copy(); 1761 if (identifier != null) { 1762 dst.identifier = new ArrayList<Identifier>(); 1763 for (Identifier i : identifier) 1764 dst.identifier.add(i.copy()); 1765 } 1766 ; 1767 dst.module = module == null ? null : module.copy(); 1768 dst.status = status == null ? null : status.copy(); 1769 dst.subject = subject == null ? null : subject.copy(); 1770 dst.encounter = encounter == null ? null : encounter.copy(); 1771 dst.occurrenceDateTime = occurrenceDateTime == null ? null : occurrenceDateTime.copy(); 1772 dst.performer = performer == null ? null : performer.copy(); 1773 if (reasonCode != null) { 1774 dst.reasonCode = new ArrayList<CodeableConcept>(); 1775 for (CodeableConcept i : reasonCode) 1776 dst.reasonCode.add(i.copy()); 1777 } 1778 ; 1779 if (reasonReference != null) { 1780 dst.reasonReference = new ArrayList<Reference>(); 1781 for (Reference i : reasonReference) 1782 dst.reasonReference.add(i.copy()); 1783 } 1784 ; 1785 if (note != null) { 1786 dst.note = new ArrayList<Annotation>(); 1787 for (Annotation i : note) 1788 dst.note.add(i.copy()); 1789 } 1790 ; 1791 if (evaluationMessage != null) { 1792 dst.evaluationMessage = new ArrayList<Reference>(); 1793 for (Reference i : evaluationMessage) 1794 dst.evaluationMessage.add(i.copy()); 1795 } 1796 ; 1797 dst.outputParameters = outputParameters == null ? null : outputParameters.copy(); 1798 dst.result = result == null ? null : result.copy(); 1799 if (dataRequirement != null) { 1800 dst.dataRequirement = new ArrayList<DataRequirement>(); 1801 for (DataRequirement i : dataRequirement) 1802 dst.dataRequirement.add(i.copy()); 1803 } 1804 ; 1805 } 1806 1807 protected GuidanceResponse typedCopy() { 1808 return copy(); 1809 } 1810 1811 @Override 1812 public boolean equalsDeep(Base other_) { 1813 if (!super.equalsDeep(other_)) 1814 return false; 1815 if (!(other_ instanceof GuidanceResponse)) 1816 return false; 1817 GuidanceResponse o = (GuidanceResponse) other_; 1818 return compareDeep(requestIdentifier, o.requestIdentifier, true) && compareDeep(identifier, o.identifier, true) 1819 && compareDeep(module, o.module, true) && compareDeep(status, o.status, true) 1820 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1821 && compareDeep(occurrenceDateTime, o.occurrenceDateTime, true) && compareDeep(performer, o.performer, true) 1822 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1823 && compareDeep(note, o.note, true) && compareDeep(evaluationMessage, o.evaluationMessage, true) 1824 && compareDeep(outputParameters, o.outputParameters, true) && compareDeep(result, o.result, true) 1825 && compareDeep(dataRequirement, o.dataRequirement, true); 1826 } 1827 1828 @Override 1829 public boolean equalsShallow(Base other_) { 1830 if (!super.equalsShallow(other_)) 1831 return false; 1832 if (!(other_ instanceof GuidanceResponse)) 1833 return false; 1834 GuidanceResponse o = (GuidanceResponse) other_; 1835 return compareValues(status, o.status, true) && compareValues(occurrenceDateTime, o.occurrenceDateTime, true); 1836 } 1837 1838 public boolean isEmpty() { 1839 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(requestIdentifier, identifier, module, status, 1840 subject, encounter, occurrenceDateTime, performer, reasonCode, reasonReference, note, evaluationMessage, 1841 outputParameters, result, dataRequirement); 1842 } 1843 1844 @Override 1845 public ResourceType getResourceType() { 1846 return ResourceType.GuidanceResponse; 1847 } 1848 1849 /** 1850 * Search parameter: <b>request</b> 1851 * <p> 1852 * Description: <b>The identifier of the request associated with the 1853 * response</b><br> 1854 * Type: <b>token</b><br> 1855 * Path: <b>GuidanceResponse.requestIdentifier</b><br> 1856 * </p> 1857 */ 1858 @SearchParamDefinition(name = "request", path = "GuidanceResponse.requestIdentifier", description = "The identifier of the request associated with the response", type = "token") 1859 public static final String SP_REQUEST = "request"; 1860 /** 1861 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1862 * <p> 1863 * Description: <b>The identifier of the request associated with the 1864 * response</b><br> 1865 * Type: <b>token</b><br> 1866 * Path: <b>GuidanceResponse.requestIdentifier</b><br> 1867 * </p> 1868 */ 1869 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUEST = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1870 SP_REQUEST); 1871 1872 /** 1873 * Search parameter: <b>identifier</b> 1874 * <p> 1875 * Description: <b>The identifier of the guidance response</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>GuidanceResponse.identifier</b><br> 1878 * </p> 1879 */ 1880 @SearchParamDefinition(name = "identifier", path = "GuidanceResponse.identifier", description = "The identifier of the guidance response", type = "token") 1881 public static final String SP_IDENTIFIER = "identifier"; 1882 /** 1883 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1884 * <p> 1885 * Description: <b>The identifier of the guidance response</b><br> 1886 * Type: <b>token</b><br> 1887 * Path: <b>GuidanceResponse.identifier</b><br> 1888 * </p> 1889 */ 1890 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1891 SP_IDENTIFIER); 1892 1893 /** 1894 * Search parameter: <b>patient</b> 1895 * <p> 1896 * Description: <b>The identity of a patient to search for guidance response 1897 * results</b><br> 1898 * Type: <b>reference</b><br> 1899 * Path: <b>GuidanceResponse.subject</b><br> 1900 * </p> 1901 */ 1902 @SearchParamDefinition(name = "patient", path = "GuidanceResponse.subject.where(resolve() is Patient)", description = "The identity of a patient to search for guidance response results", type = "reference", target = { 1903 Patient.class }) 1904 public static final String SP_PATIENT = "patient"; 1905 /** 1906 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1907 * <p> 1908 * Description: <b>The identity of a patient to search for guidance response 1909 * results</b><br> 1910 * Type: <b>reference</b><br> 1911 * Path: <b>GuidanceResponse.subject</b><br> 1912 * </p> 1913 */ 1914 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1915 SP_PATIENT); 1916 1917 /** 1918 * Constant for fluent queries to be used to add include statements. Specifies 1919 * the path value of "<b>GuidanceResponse:patient</b>". 1920 */ 1921 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 1922 "GuidanceResponse:patient").toLocked(); 1923 1924 /** 1925 * Search parameter: <b>subject</b> 1926 * <p> 1927 * Description: <b>The subject that the guidance response is about</b><br> 1928 * Type: <b>reference</b><br> 1929 * Path: <b>GuidanceResponse.subject</b><br> 1930 * </p> 1931 */ 1932 @SearchParamDefinition(name = "subject", path = "GuidanceResponse.subject", description = "The subject that the guidance response is about", type = "reference", target = { 1933 Group.class, Patient.class }) 1934 public static final String SP_SUBJECT = "subject"; 1935 /** 1936 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1937 * <p> 1938 * Description: <b>The subject that the guidance response is about</b><br> 1939 * Type: <b>reference</b><br> 1940 * Path: <b>GuidanceResponse.subject</b><br> 1941 * </p> 1942 */ 1943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1944 SP_SUBJECT); 1945 1946 /** 1947 * Constant for fluent queries to be used to add include statements. Specifies 1948 * the path value of "<b>GuidanceResponse:subject</b>". 1949 */ 1950 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1951 "GuidanceResponse:subject").toLocked(); 1952 1953}