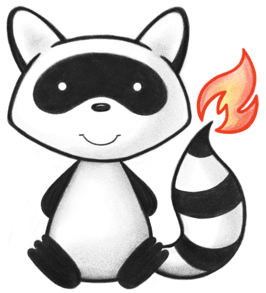
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The details of a healthcare service available at a location. 048 */ 049@ResourceDef(name = "HealthcareService", profile = "http://hl7.org/fhir/StructureDefinition/HealthcareService") 050public class HealthcareService extends DomainResource { 051 052 public enum DaysOfWeek { 053 /** 054 * Monday. 055 */ 056 MON, 057 /** 058 * Tuesday. 059 */ 060 TUE, 061 /** 062 * Wednesday. 063 */ 064 WED, 065 /** 066 * Thursday. 067 */ 068 THU, 069 /** 070 * Friday. 071 */ 072 FRI, 073 /** 074 * Saturday. 075 */ 076 SAT, 077 /** 078 * Sunday. 079 */ 080 SUN, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 086 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("mon".equals(codeString)) 090 return MON; 091 if ("tue".equals(codeString)) 092 return TUE; 093 if ("wed".equals(codeString)) 094 return WED; 095 if ("thu".equals(codeString)) 096 return THU; 097 if ("fri".equals(codeString)) 098 return FRI; 099 if ("sat".equals(codeString)) 100 return SAT; 101 if ("sun".equals(codeString)) 102 return SUN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 107 } 108 109 public String toCode() { 110 switch (this) { 111 case MON: 112 return "mon"; 113 case TUE: 114 return "tue"; 115 case WED: 116 return "wed"; 117 case THU: 118 return "thu"; 119 case FRI: 120 return "fri"; 121 case SAT: 122 return "sat"; 123 case SUN: 124 return "sun"; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getSystem() { 133 switch (this) { 134 case MON: 135 return "http://hl7.org/fhir/days-of-week"; 136 case TUE: 137 return "http://hl7.org/fhir/days-of-week"; 138 case WED: 139 return "http://hl7.org/fhir/days-of-week"; 140 case THU: 141 return "http://hl7.org/fhir/days-of-week"; 142 case FRI: 143 return "http://hl7.org/fhir/days-of-week"; 144 case SAT: 145 return "http://hl7.org/fhir/days-of-week"; 146 case SUN: 147 return "http://hl7.org/fhir/days-of-week"; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDefinition() { 156 switch (this) { 157 case MON: 158 return "Monday."; 159 case TUE: 160 return "Tuesday."; 161 case WED: 162 return "Wednesday."; 163 case THU: 164 return "Thursday."; 165 case FRI: 166 return "Friday."; 167 case SAT: 168 return "Saturday."; 169 case SUN: 170 return "Sunday."; 171 case NULL: 172 return null; 173 default: 174 return "?"; 175 } 176 } 177 178 public String getDisplay() { 179 switch (this) { 180 case MON: 181 return "Monday"; 182 case TUE: 183 return "Tuesday"; 184 case WED: 185 return "Wednesday"; 186 case THU: 187 return "Thursday"; 188 case FRI: 189 return "Friday"; 190 case SAT: 191 return "Saturday"; 192 case SUN: 193 return "Sunday"; 194 case NULL: 195 return null; 196 default: 197 return "?"; 198 } 199 } 200 } 201 202 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 203 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 204 if (codeString == null || "".equals(codeString)) 205 if (codeString == null || "".equals(codeString)) 206 return null; 207 if ("mon".equals(codeString)) 208 return DaysOfWeek.MON; 209 if ("tue".equals(codeString)) 210 return DaysOfWeek.TUE; 211 if ("wed".equals(codeString)) 212 return DaysOfWeek.WED; 213 if ("thu".equals(codeString)) 214 return DaysOfWeek.THU; 215 if ("fri".equals(codeString)) 216 return DaysOfWeek.FRI; 217 if ("sat".equals(codeString)) 218 return DaysOfWeek.SAT; 219 if ("sun".equals(codeString)) 220 return DaysOfWeek.SUN; 221 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 222 } 223 224 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 225 if (code == null) 226 return null; 227 if (code.isEmpty()) 228 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 229 String codeString = code.asStringValue(); 230 if (codeString == null || "".equals(codeString)) 231 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 232 if ("mon".equals(codeString)) 233 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 234 if ("tue".equals(codeString)) 235 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 236 if ("wed".equals(codeString)) 237 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 238 if ("thu".equals(codeString)) 239 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 240 if ("fri".equals(codeString)) 241 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 242 if ("sat".equals(codeString)) 243 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 244 if ("sun".equals(codeString)) 245 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 246 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 247 } 248 249 public String toCode(DaysOfWeek code) { 250 if (code == DaysOfWeek.NULL) 251 return null; 252 if (code == DaysOfWeek.MON) 253 return "mon"; 254 if (code == DaysOfWeek.TUE) 255 return "tue"; 256 if (code == DaysOfWeek.WED) 257 return "wed"; 258 if (code == DaysOfWeek.THU) 259 return "thu"; 260 if (code == DaysOfWeek.FRI) 261 return "fri"; 262 if (code == DaysOfWeek.SAT) 263 return "sat"; 264 if (code == DaysOfWeek.SUN) 265 return "sun"; 266 return "?"; 267 } 268 269 public String toSystem(DaysOfWeek code) { 270 return code.getSystem(); 271 } 272 } 273 274 @Block() 275 public static class HealthcareServiceEligibilityComponent extends BackboneElement implements IBaseBackboneElement { 276 /** 277 * Coded value for the eligibility. 278 */ 279 @Child(name = "code", type = { 280 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 281 @Description(shortDefinition = "Coded value for the eligibility", formalDefinition = "Coded value for the eligibility.") 282 protected CodeableConcept code; 283 284 /** 285 * Describes the eligibility conditions for the service. 286 */ 287 @Child(name = "comment", type = { 288 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 289 @Description(shortDefinition = "Describes the eligibility conditions for the service", formalDefinition = "Describes the eligibility conditions for the service.") 290 protected MarkdownType comment; 291 292 private static final long serialVersionUID = 1078065348L; 293 294 /** 295 * Constructor 296 */ 297 public HealthcareServiceEligibilityComponent() { 298 super(); 299 } 300 301 /** 302 * @return {@link #code} (Coded value for the eligibility.) 303 */ 304 public CodeableConcept getCode() { 305 if (this.code == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.code"); 308 else if (Configuration.doAutoCreate()) 309 this.code = new CodeableConcept(); // cc 310 return this.code; 311 } 312 313 public boolean hasCode() { 314 return this.code != null && !this.code.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #code} (Coded value for the eligibility.) 319 */ 320 public HealthcareServiceEligibilityComponent setCode(CodeableConcept value) { 321 this.code = value; 322 return this; 323 } 324 325 /** 326 * @return {@link #comment} (Describes the eligibility conditions for the 327 * service.). This is the underlying object with id, value and 328 * extensions. The accessor "getComment" gives direct access to the 329 * value 330 */ 331 public MarkdownType getCommentElement() { 332 if (this.comment == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.comment"); 335 else if (Configuration.doAutoCreate()) 336 this.comment = new MarkdownType(); // bb 337 return this.comment; 338 } 339 340 public boolean hasCommentElement() { 341 return this.comment != null && !this.comment.isEmpty(); 342 } 343 344 public boolean hasComment() { 345 return this.comment != null && !this.comment.isEmpty(); 346 } 347 348 /** 349 * @param value {@link #comment} (Describes the eligibility conditions for the 350 * service.). This is the underlying object with id, value and 351 * extensions. The accessor "getComment" gives direct access to the 352 * value 353 */ 354 public HealthcareServiceEligibilityComponent setCommentElement(MarkdownType value) { 355 this.comment = value; 356 return this; 357 } 358 359 /** 360 * @return Describes the eligibility conditions for the service. 361 */ 362 public String getComment() { 363 return this.comment == null ? null : this.comment.getValue(); 364 } 365 366 /** 367 * @param value Describes the eligibility conditions for the service. 368 */ 369 public HealthcareServiceEligibilityComponent setComment(String value) { 370 if (value == null) 371 this.comment = null; 372 else { 373 if (this.comment == null) 374 this.comment = new MarkdownType(); 375 this.comment.setValue(value); 376 } 377 return this; 378 } 379 380 protected void listChildren(List<Property> children) { 381 super.listChildren(children); 382 children.add(new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code)); 383 children.add( 384 new Property("comment", "markdown", "Describes the eligibility conditions for the service.", 0, 1, comment)); 385 } 386 387 @Override 388 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 389 switch (_hash) { 390 case 3059181: 391 /* code */ return new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code); 392 case 950398559: 393 /* comment */ return new Property("comment", "markdown", 394 "Describes the eligibility conditions for the service.", 0, 1, comment); 395 default: 396 return super.getNamedProperty(_hash, _name, _checkValid); 397 } 398 399 } 400 401 @Override 402 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 403 switch (hash) { 404 case 3059181: 405 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 406 case 950398559: 407 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // MarkdownType 408 default: 409 return super.getProperty(hash, name, checkValid); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(int hash, String name, Base value) throws FHIRException { 416 switch (hash) { 417 case 3059181: // code 418 this.code = castToCodeableConcept(value); // CodeableConcept 419 return value; 420 case 950398559: // comment 421 this.comment = castToMarkdown(value); // MarkdownType 422 return value; 423 default: 424 return super.setProperty(hash, name, value); 425 } 426 427 } 428 429 @Override 430 public Base setProperty(String name, Base value) throws FHIRException { 431 if (name.equals("code")) { 432 this.code = castToCodeableConcept(value); // CodeableConcept 433 } else if (name.equals("comment")) { 434 this.comment = castToMarkdown(value); // MarkdownType 435 } else 436 return super.setProperty(name, value); 437 return value; 438 } 439 440 @Override 441 public void removeChild(String name, Base value) throws FHIRException { 442 if (name.equals("code")) { 443 this.code = null; 444 } else if (name.equals("comment")) { 445 this.comment = null; 446 } else 447 super.removeChild(name, value); 448 449 } 450 451 @Override 452 public Base makeProperty(int hash, String name) throws FHIRException { 453 switch (hash) { 454 case 3059181: 455 return getCode(); 456 case 950398559: 457 return getCommentElement(); 458 default: 459 return super.makeProperty(hash, name); 460 } 461 462 } 463 464 @Override 465 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 466 switch (hash) { 467 case 3059181: 468 /* code */ return new String[] { "CodeableConcept" }; 469 case 950398559: 470 /* comment */ return new String[] { "markdown" }; 471 default: 472 return super.getTypesForProperty(hash, name); 473 } 474 475 } 476 477 @Override 478 public Base addChild(String name) throws FHIRException { 479 if (name.equals("code")) { 480 this.code = new CodeableConcept(); 481 return this.code; 482 } else if (name.equals("comment")) { 483 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 484 } else 485 return super.addChild(name); 486 } 487 488 public HealthcareServiceEligibilityComponent copy() { 489 HealthcareServiceEligibilityComponent dst = new HealthcareServiceEligibilityComponent(); 490 copyValues(dst); 491 return dst; 492 } 493 494 public void copyValues(HealthcareServiceEligibilityComponent dst) { 495 super.copyValues(dst); 496 dst.code = code == null ? null : code.copy(); 497 dst.comment = comment == null ? null : comment.copy(); 498 } 499 500 @Override 501 public boolean equalsDeep(Base other_) { 502 if (!super.equalsDeep(other_)) 503 return false; 504 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 505 return false; 506 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 507 return compareDeep(code, o.code, true) && compareDeep(comment, o.comment, true); 508 } 509 510 @Override 511 public boolean equalsShallow(Base other_) { 512 if (!super.equalsShallow(other_)) 513 return false; 514 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 515 return false; 516 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 517 return compareValues(comment, o.comment, true); 518 } 519 520 public boolean isEmpty() { 521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, comment); 522 } 523 524 public String fhirType() { 525 return "HealthcareService.eligibility"; 526 527 } 528 529 } 530 531 @Block() 532 public static class HealthcareServiceAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 533 /** 534 * Indicates which days of the week are available between the start and end 535 * Times. 536 */ 537 @Child(name = "daysOfWeek", type = { 538 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 539 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 540 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 541 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 542 543 /** 544 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 545 */ 546 @Child(name = "allDay", type = { 547 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 548 @Description(shortDefinition = "Always available? e.g. 24 hour service", formalDefinition = "Is this always available? (hence times are irrelevant) e.g. 24 hour service.") 549 protected BooleanType allDay; 550 551 /** 552 * The opening time of day. Note: If the AllDay flag is set, then this time is 553 * ignored. 554 */ 555 @Child(name = "availableStartTime", type = { 556 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 557 @Description(shortDefinition = "Opening time of day (ignored if allDay = true)", formalDefinition = "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.") 558 protected TimeType availableStartTime; 559 560 /** 561 * The closing time of day. Note: If the AllDay flag is set, then this time is 562 * ignored. 563 */ 564 @Child(name = "availableEndTime", type = { 565 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 566 @Description(shortDefinition = "Closing time of day (ignored if allDay = true)", formalDefinition = "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.") 567 protected TimeType availableEndTime; 568 569 private static final long serialVersionUID = -2139510127L; 570 571 /** 572 * Constructor 573 */ 574 public HealthcareServiceAvailableTimeComponent() { 575 super(); 576 } 577 578 /** 579 * @return {@link #daysOfWeek} (Indicates which days of the week are available 580 * between the start and end Times.) 581 */ 582 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 583 if (this.daysOfWeek == null) 584 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 585 return this.daysOfWeek; 586 } 587 588 /** 589 * @return Returns a reference to <code>this</code> for easy method chaining 590 */ 591 public HealthcareServiceAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 592 this.daysOfWeek = theDaysOfWeek; 593 return this; 594 } 595 596 public boolean hasDaysOfWeek() { 597 if (this.daysOfWeek == null) 598 return false; 599 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 600 if (!item.isEmpty()) 601 return true; 602 return false; 603 } 604 605 /** 606 * @return {@link #daysOfWeek} (Indicates which days of the week are available 607 * between the start and end Times.) 608 */ 609 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 610 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 611 if (this.daysOfWeek == null) 612 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 613 this.daysOfWeek.add(t); 614 return t; 615 } 616 617 /** 618 * @param value {@link #daysOfWeek} (Indicates which days of the week are 619 * available between the start and end Times.) 620 */ 621 public HealthcareServiceAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { // 1 622 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 623 t.setValue(value); 624 if (this.daysOfWeek == null) 625 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 626 this.daysOfWeek.add(t); 627 return this; 628 } 629 630 /** 631 * @param value {@link #daysOfWeek} (Indicates which days of the week are 632 * available between the start and end Times.) 633 */ 634 public boolean hasDaysOfWeek(DaysOfWeek value) { 635 if (this.daysOfWeek == null) 636 return false; 637 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 638 if (v.getValue().equals(value)) // code 639 return true; 640 return false; 641 } 642 643 /** 644 * @return {@link #allDay} (Is this always available? (hence times are 645 * irrelevant) e.g. 24 hour service.). This is the underlying object 646 * with id, value and extensions. The accessor "getAllDay" gives direct 647 * access to the value 648 */ 649 public BooleanType getAllDayElement() { 650 if (this.allDay == null) 651 if (Configuration.errorOnAutoCreate()) 652 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.allDay"); 653 else if (Configuration.doAutoCreate()) 654 this.allDay = new BooleanType(); // bb 655 return this.allDay; 656 } 657 658 public boolean hasAllDayElement() { 659 return this.allDay != null && !this.allDay.isEmpty(); 660 } 661 662 public boolean hasAllDay() { 663 return this.allDay != null && !this.allDay.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #allDay} (Is this always available? (hence times are 668 * irrelevant) e.g. 24 hour service.). This is the underlying 669 * object with id, value and extensions. The accessor "getAllDay" 670 * gives direct access to the value 671 */ 672 public HealthcareServiceAvailableTimeComponent setAllDayElement(BooleanType value) { 673 this.allDay = value; 674 return this; 675 } 676 677 /** 678 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour 679 * service. 680 */ 681 public boolean getAllDay() { 682 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 683 } 684 685 /** 686 * @param value Is this always available? (hence times are irrelevant) e.g. 24 687 * hour service. 688 */ 689 public HealthcareServiceAvailableTimeComponent setAllDay(boolean value) { 690 if (this.allDay == null) 691 this.allDay = new BooleanType(); 692 this.allDay.setValue(value); 693 return this; 694 } 695 696 /** 697 * @return {@link #availableStartTime} (The opening time of day. Note: If the 698 * AllDay flag is set, then this time is ignored.). This is the 699 * underlying object with id, value and extensions. The accessor 700 * "getAvailableStartTime" gives direct access to the value 701 */ 702 public TimeType getAvailableStartTimeElement() { 703 if (this.availableStartTime == null) 704 if (Configuration.errorOnAutoCreate()) 705 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableStartTime"); 706 else if (Configuration.doAutoCreate()) 707 this.availableStartTime = new TimeType(); // bb 708 return this.availableStartTime; 709 } 710 711 public boolean hasAvailableStartTimeElement() { 712 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 713 } 714 715 public boolean hasAvailableStartTime() { 716 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 717 } 718 719 /** 720 * @param value {@link #availableStartTime} (The opening time of day. Note: If 721 * the AllDay flag is set, then this time is ignored.). This is the 722 * underlying object with id, value and extensions. The accessor 723 * "getAvailableStartTime" gives direct access to the value 724 */ 725 public HealthcareServiceAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 726 this.availableStartTime = value; 727 return this; 728 } 729 730 /** 731 * @return The opening time of day. Note: If the AllDay flag is set, then this 732 * time is ignored. 733 */ 734 public String getAvailableStartTime() { 735 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 736 } 737 738 /** 739 * @param value The opening time of day. Note: If the AllDay flag is set, then 740 * this time is ignored. 741 */ 742 public HealthcareServiceAvailableTimeComponent setAvailableStartTime(String value) { 743 if (value == null) 744 this.availableStartTime = null; 745 else { 746 if (this.availableStartTime == null) 747 this.availableStartTime = new TimeType(); 748 this.availableStartTime.setValue(value); 749 } 750 return this; 751 } 752 753 /** 754 * @return {@link #availableEndTime} (The closing time of day. Note: If the 755 * AllDay flag is set, then this time is ignored.). This is the 756 * underlying object with id, value and extensions. The accessor 757 * "getAvailableEndTime" gives direct access to the value 758 */ 759 public TimeType getAvailableEndTimeElement() { 760 if (this.availableEndTime == null) 761 if (Configuration.errorOnAutoCreate()) 762 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableEndTime"); 763 else if (Configuration.doAutoCreate()) 764 this.availableEndTime = new TimeType(); // bb 765 return this.availableEndTime; 766 } 767 768 public boolean hasAvailableEndTimeElement() { 769 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 770 } 771 772 public boolean hasAvailableEndTime() { 773 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 774 } 775 776 /** 777 * @param value {@link #availableEndTime} (The closing time of day. Note: If the 778 * AllDay flag is set, then this time is ignored.). This is the 779 * underlying object with id, value and extensions. The accessor 780 * "getAvailableEndTime" gives direct access to the value 781 */ 782 public HealthcareServiceAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 783 this.availableEndTime = value; 784 return this; 785 } 786 787 /** 788 * @return The closing time of day. Note: If the AllDay flag is set, then this 789 * time is ignored. 790 */ 791 public String getAvailableEndTime() { 792 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 793 } 794 795 /** 796 * @param value The closing time of day. Note: If the AllDay flag is set, then 797 * this time is ignored. 798 */ 799 public HealthcareServiceAvailableTimeComponent setAvailableEndTime(String value) { 800 if (value == null) 801 this.availableEndTime = null; 802 else { 803 if (this.availableEndTime == null) 804 this.availableEndTime = new TimeType(); 805 this.availableEndTime.setValue(value); 806 } 807 return this; 808 } 809 810 protected void listChildren(List<Property> children) { 811 super.listChildren(children); 812 children.add(new Property("daysOfWeek", "code", 813 "Indicates which days of the week are available between the start and end Times.", 0, 814 java.lang.Integer.MAX_VALUE, daysOfWeek)); 815 children.add(new Property("allDay", "boolean", 816 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 817 children.add(new Property("availableStartTime", "time", 818 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 819 availableStartTime)); 820 children.add(new Property("availableEndTime", "time", 821 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 822 availableEndTime)); 823 } 824 825 @Override 826 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 827 switch (_hash) { 828 case 68050338: 829 /* daysOfWeek */ return new Property("daysOfWeek", "code", 830 "Indicates which days of the week are available between the start and end Times.", 0, 831 java.lang.Integer.MAX_VALUE, daysOfWeek); 832 case -1414913477: 833 /* allDay */ return new Property("allDay", "boolean", 834 "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 835 case -1039453818: 836 /* availableStartTime */ return new Property("availableStartTime", "time", 837 "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 838 availableStartTime); 839 case 101151551: 840 /* availableEndTime */ return new Property("availableEndTime", "time", 841 "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, 842 availableEndTime); 843 default: 844 return super.getNamedProperty(_hash, _name, _checkValid); 845 } 846 847 } 848 849 @Override 850 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 851 switch (hash) { 852 case 68050338: 853 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 854 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 855 case -1414913477: 856 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 857 case -1039453818: 858 /* availableStartTime */ return this.availableStartTime == null ? new Base[0] 859 : new Base[] { this.availableStartTime }; // TimeType 860 case 101151551: 861 /* availableEndTime */ return this.availableEndTime == null ? new Base[0] 862 : new Base[] { this.availableEndTime }; // TimeType 863 default: 864 return super.getProperty(hash, name, checkValid); 865 } 866 867 } 868 869 @Override 870 public Base setProperty(int hash, String name, Base value) throws FHIRException { 871 switch (hash) { 872 case 68050338: // daysOfWeek 873 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 874 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 875 return value; 876 case -1414913477: // allDay 877 this.allDay = castToBoolean(value); // BooleanType 878 return value; 879 case -1039453818: // availableStartTime 880 this.availableStartTime = castToTime(value); // TimeType 881 return value; 882 case 101151551: // availableEndTime 883 this.availableEndTime = castToTime(value); // TimeType 884 return value; 885 default: 886 return super.setProperty(hash, name, value); 887 } 888 889 } 890 891 @Override 892 public Base setProperty(String name, Base value) throws FHIRException { 893 if (name.equals("daysOfWeek")) { 894 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 895 this.getDaysOfWeek().add((Enumeration) value); 896 } else if (name.equals("allDay")) { 897 this.allDay = castToBoolean(value); // BooleanType 898 } else if (name.equals("availableStartTime")) { 899 this.availableStartTime = castToTime(value); // TimeType 900 } else if (name.equals("availableEndTime")) { 901 this.availableEndTime = castToTime(value); // TimeType 902 } else 903 return super.setProperty(name, value); 904 return value; 905 } 906 907 @Override 908 public void removeChild(String name, Base value) throws FHIRException { 909 if (name.equals("daysOfWeek")) { 910 this.getDaysOfWeek().remove((Enumeration) value); 911 } else if (name.equals("allDay")) { 912 this.allDay = null; 913 } else if (name.equals("availableStartTime")) { 914 this.availableStartTime = null; 915 } else if (name.equals("availableEndTime")) { 916 this.availableEndTime = null; 917 } else 918 super.removeChild(name, value); 919 920 } 921 922 @Override 923 public Base makeProperty(int hash, String name) throws FHIRException { 924 switch (hash) { 925 case 68050338: 926 return addDaysOfWeekElement(); 927 case -1414913477: 928 return getAllDayElement(); 929 case -1039453818: 930 return getAvailableStartTimeElement(); 931 case 101151551: 932 return getAvailableEndTimeElement(); 933 default: 934 return super.makeProperty(hash, name); 935 } 936 937 } 938 939 @Override 940 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 941 switch (hash) { 942 case 68050338: 943 /* daysOfWeek */ return new String[] { "code" }; 944 case -1414913477: 945 /* allDay */ return new String[] { "boolean" }; 946 case -1039453818: 947 /* availableStartTime */ return new String[] { "time" }; 948 case 101151551: 949 /* availableEndTime */ return new String[] { "time" }; 950 default: 951 return super.getTypesForProperty(hash, name); 952 } 953 954 } 955 956 @Override 957 public Base addChild(String name) throws FHIRException { 958 if (name.equals("daysOfWeek")) { 959 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.daysOfWeek"); 960 } else if (name.equals("allDay")) { 961 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.allDay"); 962 } else if (name.equals("availableStartTime")) { 963 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableStartTime"); 964 } else if (name.equals("availableEndTime")) { 965 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableEndTime"); 966 } else 967 return super.addChild(name); 968 } 969 970 public HealthcareServiceAvailableTimeComponent copy() { 971 HealthcareServiceAvailableTimeComponent dst = new HealthcareServiceAvailableTimeComponent(); 972 copyValues(dst); 973 return dst; 974 } 975 976 public void copyValues(HealthcareServiceAvailableTimeComponent dst) { 977 super.copyValues(dst); 978 if (daysOfWeek != null) { 979 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 980 for (Enumeration<DaysOfWeek> i : daysOfWeek) 981 dst.daysOfWeek.add(i.copy()); 982 } 983 ; 984 dst.allDay = allDay == null ? null : allDay.copy(); 985 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 986 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 987 } 988 989 @Override 990 public boolean equalsDeep(Base other_) { 991 if (!super.equalsDeep(other_)) 992 return false; 993 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 994 return false; 995 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 996 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 997 && compareDeep(availableStartTime, o.availableStartTime, true) 998 && compareDeep(availableEndTime, o.availableEndTime, true); 999 } 1000 1001 @Override 1002 public boolean equalsShallow(Base other_) { 1003 if (!super.equalsShallow(other_)) 1004 return false; 1005 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 1006 return false; 1007 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 1008 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 1009 && compareValues(availableStartTime, o.availableStartTime, true) 1010 && compareValues(availableEndTime, o.availableEndTime, true); 1011 } 1012 1013 public boolean isEmpty() { 1014 return super.isEmpty() 1015 && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime, availableEndTime); 1016 } 1017 1018 public String fhirType() { 1019 return "HealthcareService.availableTime"; 1020 1021 } 1022 1023 } 1024 1025 @Block() 1026 public static class HealthcareServiceNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 1027 /** 1028 * The reason that can be presented to the user as to why this time is not 1029 * available. 1030 */ 1031 @Child(name = "description", type = { 1032 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1033 @Description(shortDefinition = "Reason presented to the user explaining why time not available", formalDefinition = "The reason that can be presented to the user as to why this time is not available.") 1034 protected StringType description; 1035 1036 /** 1037 * Service is not available (seasonally or for a public holiday) from this date. 1038 */ 1039 @Child(name = "during", type = { Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1040 @Description(shortDefinition = "Service not available from this date", formalDefinition = "Service is not available (seasonally or for a public holiday) from this date.") 1041 protected Period during; 1042 1043 private static final long serialVersionUID = 310849929L; 1044 1045 /** 1046 * Constructor 1047 */ 1048 public HealthcareServiceNotAvailableComponent() { 1049 super(); 1050 } 1051 1052 /** 1053 * Constructor 1054 */ 1055 public HealthcareServiceNotAvailableComponent(StringType description) { 1056 super(); 1057 this.description = description; 1058 } 1059 1060 /** 1061 * @return {@link #description} (The reason that can be presented to the user as 1062 * to why this time is not available.). This is the underlying object 1063 * with id, value and extensions. The accessor "getDescription" gives 1064 * direct access to the value 1065 */ 1066 public StringType getDescriptionElement() { 1067 if (this.description == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.description"); 1070 else if (Configuration.doAutoCreate()) 1071 this.description = new StringType(); // bb 1072 return this.description; 1073 } 1074 1075 public boolean hasDescriptionElement() { 1076 return this.description != null && !this.description.isEmpty(); 1077 } 1078 1079 public boolean hasDescription() { 1080 return this.description != null && !this.description.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #description} (The reason that can be presented to the 1085 * user as to why this time is not available.). This is the 1086 * underlying object with id, value and extensions. The accessor 1087 * "getDescription" gives direct access to the value 1088 */ 1089 public HealthcareServiceNotAvailableComponent setDescriptionElement(StringType value) { 1090 this.description = value; 1091 return this; 1092 } 1093 1094 /** 1095 * @return The reason that can be presented to the user as to why this time is 1096 * not available. 1097 */ 1098 public String getDescription() { 1099 return this.description == null ? null : this.description.getValue(); 1100 } 1101 1102 /** 1103 * @param value The reason that can be presented to the user as to why this time 1104 * is not available. 1105 */ 1106 public HealthcareServiceNotAvailableComponent setDescription(String value) { 1107 if (this.description == null) 1108 this.description = new StringType(); 1109 this.description.setValue(value); 1110 return this; 1111 } 1112 1113 /** 1114 * @return {@link #during} (Service is not available (seasonally or for a public 1115 * holiday) from this date.) 1116 */ 1117 public Period getDuring() { 1118 if (this.during == null) 1119 if (Configuration.errorOnAutoCreate()) 1120 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.during"); 1121 else if (Configuration.doAutoCreate()) 1122 this.during = new Period(); // cc 1123 return this.during; 1124 } 1125 1126 public boolean hasDuring() { 1127 return this.during != null && !this.during.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #during} (Service is not available (seasonally or for a 1132 * public holiday) from this date.) 1133 */ 1134 public HealthcareServiceNotAvailableComponent setDuring(Period value) { 1135 this.during = value; 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> children) { 1140 super.listChildren(children); 1141 children.add(new Property("description", "string", 1142 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 1143 children.add(new Property("during", "Period", 1144 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 1145 } 1146 1147 @Override 1148 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1149 switch (_hash) { 1150 case -1724546052: 1151 /* description */ return new Property("description", "string", 1152 "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 1153 case -1320499647: 1154 /* during */ return new Property("during", "Period", 1155 "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 1156 default: 1157 return super.getNamedProperty(_hash, _name, _checkValid); 1158 } 1159 1160 } 1161 1162 @Override 1163 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1164 switch (hash) { 1165 case -1724546052: 1166 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 1167 case -1320499647: 1168 /* during */ return this.during == null ? new Base[0] : new Base[] { this.during }; // Period 1169 default: 1170 return super.getProperty(hash, name, checkValid); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1177 switch (hash) { 1178 case -1724546052: // description 1179 this.description = castToString(value); // StringType 1180 return value; 1181 case -1320499647: // during 1182 this.during = castToPeriod(value); // Period 1183 return value; 1184 default: 1185 return super.setProperty(hash, name, value); 1186 } 1187 1188 } 1189 1190 @Override 1191 public Base setProperty(String name, Base value) throws FHIRException { 1192 if (name.equals("description")) { 1193 this.description = castToString(value); // StringType 1194 } else if (name.equals("during")) { 1195 this.during = castToPeriod(value); // Period 1196 } else 1197 return super.setProperty(name, value); 1198 return value; 1199 } 1200 1201 @Override 1202 public void removeChild(String name, Base value) throws FHIRException { 1203 if (name.equals("description")) { 1204 this.description = null; 1205 } else if (name.equals("during")) { 1206 this.during = null; 1207 } else 1208 super.removeChild(name, value); 1209 1210 } 1211 1212 @Override 1213 public Base makeProperty(int hash, String name) throws FHIRException { 1214 switch (hash) { 1215 case -1724546052: 1216 return getDescriptionElement(); 1217 case -1320499647: 1218 return getDuring(); 1219 default: 1220 return super.makeProperty(hash, name); 1221 } 1222 1223 } 1224 1225 @Override 1226 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1227 switch (hash) { 1228 case -1724546052: 1229 /* description */ return new String[] { "string" }; 1230 case -1320499647: 1231 /* during */ return new String[] { "Period" }; 1232 default: 1233 return super.getTypesForProperty(hash, name); 1234 } 1235 1236 } 1237 1238 @Override 1239 public Base addChild(String name) throws FHIRException { 1240 if (name.equals("description")) { 1241 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.description"); 1242 } else if (name.equals("during")) { 1243 this.during = new Period(); 1244 return this.during; 1245 } else 1246 return super.addChild(name); 1247 } 1248 1249 public HealthcareServiceNotAvailableComponent copy() { 1250 HealthcareServiceNotAvailableComponent dst = new HealthcareServiceNotAvailableComponent(); 1251 copyValues(dst); 1252 return dst; 1253 } 1254 1255 public void copyValues(HealthcareServiceNotAvailableComponent dst) { 1256 super.copyValues(dst); 1257 dst.description = description == null ? null : description.copy(); 1258 dst.during = during == null ? null : during.copy(); 1259 } 1260 1261 @Override 1262 public boolean equalsDeep(Base other_) { 1263 if (!super.equalsDeep(other_)) 1264 return false; 1265 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 1266 return false; 1267 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 1268 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 1269 } 1270 1271 @Override 1272 public boolean equalsShallow(Base other_) { 1273 if (!super.equalsShallow(other_)) 1274 return false; 1275 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 1276 return false; 1277 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 1278 return compareValues(description, o.description, true); 1279 } 1280 1281 public boolean isEmpty() { 1282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 1283 } 1284 1285 public String fhirType() { 1286 return "HealthcareService.notAvailable"; 1287 1288 } 1289 1290 } 1291 1292 /** 1293 * External identifiers for this item. 1294 */ 1295 @Child(name = "identifier", type = { 1296 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1297 @Description(shortDefinition = "External identifiers for this item", formalDefinition = "External identifiers for this item.") 1298 protected List<Identifier> identifier; 1299 1300 /** 1301 * This flag is used to mark the record to not be used. This is not used when a 1302 * center is closed for maintenance, or for holidays, the notAvailable period is 1303 * to be used for this. 1304 */ 1305 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1306 @Description(shortDefinition = "Whether this HealthcareService record is in active use", formalDefinition = "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.") 1307 protected BooleanType active; 1308 1309 /** 1310 * The organization that provides this healthcare service. 1311 */ 1312 @Child(name = "providedBy", type = { 1313 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1314 @Description(shortDefinition = "Organization that provides this service", formalDefinition = "The organization that provides this healthcare service.") 1315 protected Reference providedBy; 1316 1317 /** 1318 * The actual object that is the target of the reference (The organization that 1319 * provides this healthcare service.) 1320 */ 1321 protected Organization providedByTarget; 1322 1323 /** 1324 * Identifies the broad category of service being performed or delivered. 1325 */ 1326 @Child(name = "category", type = { 1327 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1328 @Description(shortDefinition = "Broad category of service being performed or delivered", formalDefinition = "Identifies the broad category of service being performed or delivered.") 1329 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 1330 protected List<CodeableConcept> category; 1331 1332 /** 1333 * The specific type of service that may be delivered or performed. 1334 */ 1335 @Child(name = "type", type = { 1336 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1337 @Description(shortDefinition = "Type of service that may be delivered or performed", formalDefinition = "The specific type of service that may be delivered or performed.") 1338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 1339 protected List<CodeableConcept> type; 1340 1341 /** 1342 * Collection of specialties handled by the service site. This is more of a 1343 * medical term. 1344 */ 1345 @Child(name = "specialty", type = { 1346 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1347 @Description(shortDefinition = "Specialties handled by the HealthcareService", formalDefinition = "Collection of specialties handled by the service site. This is more of a medical term.") 1348 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 1349 protected List<CodeableConcept> specialty; 1350 1351 /** 1352 * The location(s) where this healthcare service may be provided. 1353 */ 1354 @Child(name = "location", type = { 1355 Location.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1356 @Description(shortDefinition = "Location(s) where service may be provided", formalDefinition = "The location(s) where this healthcare service may be provided.") 1357 protected List<Reference> location; 1358 /** 1359 * The actual objects that are the target of the reference (The location(s) 1360 * where this healthcare service may be provided.) 1361 */ 1362 protected List<Location> locationTarget; 1363 1364 /** 1365 * Further description of the service as it would be presented to a consumer 1366 * while searching. 1367 */ 1368 @Child(name = "name", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1369 @Description(shortDefinition = "Description of service as presented to a consumer while searching", formalDefinition = "Further description of the service as it would be presented to a consumer while searching.") 1370 protected StringType name; 1371 1372 /** 1373 * Any additional description of the service and/or any specific issues not 1374 * covered by the other attributes, which can be displayed as further detail 1375 * under the serviceName. 1376 */ 1377 @Child(name = "comment", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1378 @Description(shortDefinition = "Additional description and/or any specific issues not covered elsewhere", formalDefinition = "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.") 1379 protected StringType comment; 1380 1381 /** 1382 * Extra details about the service that can't be placed in the other fields. 1383 */ 1384 @Child(name = "extraDetails", type = { 1385 MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1386 @Description(shortDefinition = "Extra details about the service that can't be placed in the other fields", formalDefinition = "Extra details about the service that can't be placed in the other fields.") 1387 protected MarkdownType extraDetails; 1388 1389 /** 1390 * If there is a photo/symbol associated with this HealthcareService, it may be 1391 * included here to facilitate quick identification of the service in a list. 1392 */ 1393 @Child(name = "photo", type = { Attachment.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1394 @Description(shortDefinition = "Facilitates quick identification of the service", formalDefinition = "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.") 1395 protected Attachment photo; 1396 1397 /** 1398 * List of contacts related to this specific healthcare service. 1399 */ 1400 @Child(name = "telecom", type = { 1401 ContactPoint.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1402 @Description(shortDefinition = "Contacts related to the healthcare service", formalDefinition = "List of contacts related to this specific healthcare service.") 1403 protected List<ContactPoint> telecom; 1404 1405 /** 1406 * The location(s) that this service is available to (not where the service is 1407 * provided). 1408 */ 1409 @Child(name = "coverageArea", type = { 1410 Location.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1411 @Description(shortDefinition = "Location(s) service is intended for/available to", formalDefinition = "The location(s) that this service is available to (not where the service is provided).") 1412 protected List<Reference> coverageArea; 1413 /** 1414 * The actual objects that are the target of the reference (The location(s) that 1415 * this service is available to (not where the service is provided).) 1416 */ 1417 protected List<Location> coverageAreaTarget; 1418 1419 /** 1420 * The code(s) that detail the conditions under which the healthcare service is 1421 * available/offered. 1422 */ 1423 @Child(name = "serviceProvisionCode", type = { 1424 CodeableConcept.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1425 @Description(shortDefinition = "Conditions under which service is available/offered", formalDefinition = "The code(s) that detail the conditions under which the healthcare service is available/offered.") 1426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-provision-conditions") 1427 protected List<CodeableConcept> serviceProvisionCode; 1428 1429 /** 1430 * Does this service have specific eligibility requirements that need to be met 1431 * in order to use the service? 1432 */ 1433 @Child(name = "eligibility", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1434 @Description(shortDefinition = "Specific eligibility requirements required to use the service", formalDefinition = "Does this service have specific eligibility requirements that need to be met in order to use the service?") 1435 protected List<HealthcareServiceEligibilityComponent> eligibility; 1436 1437 /** 1438 * Programs that this service is applicable to. 1439 */ 1440 @Child(name = "program", type = { 1441 CodeableConcept.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1442 @Description(shortDefinition = "Programs that this service is applicable to", formalDefinition = "Programs that this service is applicable to.") 1443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/program") 1444 protected List<CodeableConcept> program; 1445 1446 /** 1447 * Collection of characteristics (attributes). 1448 */ 1449 @Child(name = "characteristic", type = { 1450 CodeableConcept.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1451 @Description(shortDefinition = "Collection of characteristics (attributes)", formalDefinition = "Collection of characteristics (attributes).") 1452 protected List<CodeableConcept> characteristic; 1453 1454 /** 1455 * Some services are specifically made available in multiple languages, this 1456 * property permits a directory to declare the languages this is offered in. 1457 * Typically this is only provided where a service operates in communities with 1458 * mixed languages used. 1459 */ 1460 @Child(name = "communication", type = { 1461 CodeableConcept.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1462 @Description(shortDefinition = "The language that this service is offered in", formalDefinition = "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.") 1463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/languages") 1464 protected List<CodeableConcept> communication; 1465 1466 /** 1467 * Ways that the service accepts referrals, if this is not provided then it is 1468 * implied that no referral is required. 1469 */ 1470 @Child(name = "referralMethod", type = { 1471 CodeableConcept.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1472 @Description(shortDefinition = "Ways that the service accepts referrals", formalDefinition = "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.") 1473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-referral-method") 1474 protected List<CodeableConcept> referralMethod; 1475 1476 /** 1477 * Indicates whether or not a prospective consumer will require an appointment 1478 * for a particular service at a site to be provided by the Organization. 1479 * Indicates if an appointment is required for access to this service. 1480 */ 1481 @Child(name = "appointmentRequired", type = { 1482 BooleanType.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1483 @Description(shortDefinition = "If an appointment is required for access to this service", formalDefinition = "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.") 1484 protected BooleanType appointmentRequired; 1485 1486 /** 1487 * A collection of times that the Service Site is available. 1488 */ 1489 @Child(name = "availableTime", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1490 @Description(shortDefinition = "Times the Service Site is available", formalDefinition = "A collection of times that the Service Site is available.") 1491 protected List<HealthcareServiceAvailableTimeComponent> availableTime; 1492 1493 /** 1494 * The HealthcareService is not available during this period of time due to the 1495 * provided reason. 1496 */ 1497 @Child(name = "notAvailable", type = {}, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1498 @Description(shortDefinition = "Not available during this time due to provided reason", formalDefinition = "The HealthcareService is not available during this period of time due to the provided reason.") 1499 protected List<HealthcareServiceNotAvailableComponent> notAvailable; 1500 1501 /** 1502 * A description of site availability exceptions, e.g. public holiday 1503 * availability. Succinctly describing all possible exceptions to normal site 1504 * availability as details in the available Times and not available Times. 1505 */ 1506 @Child(name = "availabilityExceptions", type = { 1507 StringType.class }, order = 22, min = 0, max = 1, modifier = false, summary = false) 1508 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.") 1509 protected StringType availabilityExceptions; 1510 1511 /** 1512 * Technical endpoints providing access to services operated for the specific 1513 * healthcare services defined at this resource. 1514 */ 1515 @Child(name = "endpoint", type = { 1516 Endpoint.class }, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1517 @Description(shortDefinition = "Technical endpoints providing access to electronic services operated for the healthcare service", formalDefinition = "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.") 1518 protected List<Reference> endpoint; 1519 /** 1520 * The actual objects that are the target of the reference (Technical endpoints 1521 * providing access to services operated for the specific healthcare services 1522 * defined at this resource.) 1523 */ 1524 protected List<Endpoint> endpointTarget; 1525 1526 private static final long serialVersionUID = -2002412666L; 1527 1528 /** 1529 * Constructor 1530 */ 1531 public HealthcareService() { 1532 super(); 1533 } 1534 1535 /** 1536 * @return {@link #identifier} (External identifiers for this item.) 1537 */ 1538 public List<Identifier> getIdentifier() { 1539 if (this.identifier == null) 1540 this.identifier = new ArrayList<Identifier>(); 1541 return this.identifier; 1542 } 1543 1544 /** 1545 * @return Returns a reference to <code>this</code> for easy method chaining 1546 */ 1547 public HealthcareService setIdentifier(List<Identifier> theIdentifier) { 1548 this.identifier = theIdentifier; 1549 return this; 1550 } 1551 1552 public boolean hasIdentifier() { 1553 if (this.identifier == null) 1554 return false; 1555 for (Identifier item : this.identifier) 1556 if (!item.isEmpty()) 1557 return true; 1558 return false; 1559 } 1560 1561 public Identifier addIdentifier() { // 3 1562 Identifier t = new Identifier(); 1563 if (this.identifier == null) 1564 this.identifier = new ArrayList<Identifier>(); 1565 this.identifier.add(t); 1566 return t; 1567 } 1568 1569 public HealthcareService addIdentifier(Identifier t) { // 3 1570 if (t == null) 1571 return this; 1572 if (this.identifier == null) 1573 this.identifier = new ArrayList<Identifier>(); 1574 this.identifier.add(t); 1575 return this; 1576 } 1577 1578 /** 1579 * @return The first repetition of repeating field {@link #identifier}, creating 1580 * it if it does not already exist 1581 */ 1582 public Identifier getIdentifierFirstRep() { 1583 if (getIdentifier().isEmpty()) { 1584 addIdentifier(); 1585 } 1586 return getIdentifier().get(0); 1587 } 1588 1589 /** 1590 * @return {@link #active} (This flag is used to mark the record to not be used. 1591 * This is not used when a center is closed for maintenance, or for 1592 * holidays, the notAvailable period is to be used for this.). This is 1593 * the underlying object with id, value and extensions. The accessor 1594 * "getActive" gives direct access to the value 1595 */ 1596 public BooleanType getActiveElement() { 1597 if (this.active == null) 1598 if (Configuration.errorOnAutoCreate()) 1599 throw new Error("Attempt to auto-create HealthcareService.active"); 1600 else if (Configuration.doAutoCreate()) 1601 this.active = new BooleanType(); // bb 1602 return this.active; 1603 } 1604 1605 public boolean hasActiveElement() { 1606 return this.active != null && !this.active.isEmpty(); 1607 } 1608 1609 public boolean hasActive() { 1610 return this.active != null && !this.active.isEmpty(); 1611 } 1612 1613 /** 1614 * @param value {@link #active} (This flag is used to mark the record to not be 1615 * used. This is not used when a center is closed for maintenance, 1616 * or for holidays, the notAvailable period is to be used for 1617 * this.). This is the underlying object with id, value and 1618 * extensions. The accessor "getActive" gives direct access to the 1619 * value 1620 */ 1621 public HealthcareService setActiveElement(BooleanType value) { 1622 this.active = value; 1623 return this; 1624 } 1625 1626 /** 1627 * @return This flag is used to mark the record to not be used. This is not used 1628 * when a center is closed for maintenance, or for holidays, the 1629 * notAvailable period is to be used for this. 1630 */ 1631 public boolean getActive() { 1632 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1633 } 1634 1635 /** 1636 * @param value This flag is used to mark the record to not be used. This is not 1637 * used when a center is closed for maintenance, or for holidays, 1638 * the notAvailable period is to be used for this. 1639 */ 1640 public HealthcareService setActive(boolean value) { 1641 if (this.active == null) 1642 this.active = new BooleanType(); 1643 this.active.setValue(value); 1644 return this; 1645 } 1646 1647 /** 1648 * @return {@link #providedBy} (The organization that provides this healthcare 1649 * service.) 1650 */ 1651 public Reference getProvidedBy() { 1652 if (this.providedBy == null) 1653 if (Configuration.errorOnAutoCreate()) 1654 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1655 else if (Configuration.doAutoCreate()) 1656 this.providedBy = new Reference(); // cc 1657 return this.providedBy; 1658 } 1659 1660 public boolean hasProvidedBy() { 1661 return this.providedBy != null && !this.providedBy.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #providedBy} (The organization that provides this 1666 * healthcare service.) 1667 */ 1668 public HealthcareService setProvidedBy(Reference value) { 1669 this.providedBy = value; 1670 return this; 1671 } 1672 1673 /** 1674 * @return {@link #providedBy} The actual object that is the target of the 1675 * reference. The reference library doesn't populate this, but you can 1676 * use it to hold the resource if you resolve it. (The organization that 1677 * provides this healthcare service.) 1678 */ 1679 public Organization getProvidedByTarget() { 1680 if (this.providedByTarget == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1683 else if (Configuration.doAutoCreate()) 1684 this.providedByTarget = new Organization(); // aa 1685 return this.providedByTarget; 1686 } 1687 1688 /** 1689 * @param value {@link #providedBy} The actual object that is the target of the 1690 * reference. The reference library doesn't use these, but you can 1691 * use it to hold the resource if you resolve it. (The organization 1692 * that provides this healthcare service.) 1693 */ 1694 public HealthcareService setProvidedByTarget(Organization value) { 1695 this.providedByTarget = value; 1696 return this; 1697 } 1698 1699 /** 1700 * @return {@link #category} (Identifies the broad category of service being 1701 * performed or delivered.) 1702 */ 1703 public List<CodeableConcept> getCategory() { 1704 if (this.category == null) 1705 this.category = new ArrayList<CodeableConcept>(); 1706 return this.category; 1707 } 1708 1709 /** 1710 * @return Returns a reference to <code>this</code> for easy method chaining 1711 */ 1712 public HealthcareService setCategory(List<CodeableConcept> theCategory) { 1713 this.category = theCategory; 1714 return this; 1715 } 1716 1717 public boolean hasCategory() { 1718 if (this.category == null) 1719 return false; 1720 for (CodeableConcept item : this.category) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 public CodeableConcept addCategory() { // 3 1727 CodeableConcept t = new CodeableConcept(); 1728 if (this.category == null) 1729 this.category = new ArrayList<CodeableConcept>(); 1730 this.category.add(t); 1731 return t; 1732 } 1733 1734 public HealthcareService addCategory(CodeableConcept t) { // 3 1735 if (t == null) 1736 return this; 1737 if (this.category == null) 1738 this.category = new ArrayList<CodeableConcept>(); 1739 this.category.add(t); 1740 return this; 1741 } 1742 1743 /** 1744 * @return The first repetition of repeating field {@link #category}, creating 1745 * it if it does not already exist 1746 */ 1747 public CodeableConcept getCategoryFirstRep() { 1748 if (getCategory().isEmpty()) { 1749 addCategory(); 1750 } 1751 return getCategory().get(0); 1752 } 1753 1754 /** 1755 * @return {@link #type} (The specific type of service that may be delivered or 1756 * performed.) 1757 */ 1758 public List<CodeableConcept> getType() { 1759 if (this.type == null) 1760 this.type = new ArrayList<CodeableConcept>(); 1761 return this.type; 1762 } 1763 1764 /** 1765 * @return Returns a reference to <code>this</code> for easy method chaining 1766 */ 1767 public HealthcareService setType(List<CodeableConcept> theType) { 1768 this.type = theType; 1769 return this; 1770 } 1771 1772 public boolean hasType() { 1773 if (this.type == null) 1774 return false; 1775 for (CodeableConcept item : this.type) 1776 if (!item.isEmpty()) 1777 return true; 1778 return false; 1779 } 1780 1781 public CodeableConcept addType() { // 3 1782 CodeableConcept t = new CodeableConcept(); 1783 if (this.type == null) 1784 this.type = new ArrayList<CodeableConcept>(); 1785 this.type.add(t); 1786 return t; 1787 } 1788 1789 public HealthcareService addType(CodeableConcept t) { // 3 1790 if (t == null) 1791 return this; 1792 if (this.type == null) 1793 this.type = new ArrayList<CodeableConcept>(); 1794 this.type.add(t); 1795 return this; 1796 } 1797 1798 /** 1799 * @return The first repetition of repeating field {@link #type}, creating it if 1800 * it does not already exist 1801 */ 1802 public CodeableConcept getTypeFirstRep() { 1803 if (getType().isEmpty()) { 1804 addType(); 1805 } 1806 return getType().get(0); 1807 } 1808 1809 /** 1810 * @return {@link #specialty} (Collection of specialties handled by the service 1811 * site. This is more of a medical term.) 1812 */ 1813 public List<CodeableConcept> getSpecialty() { 1814 if (this.specialty == null) 1815 this.specialty = new ArrayList<CodeableConcept>(); 1816 return this.specialty; 1817 } 1818 1819 /** 1820 * @return Returns a reference to <code>this</code> for easy method chaining 1821 */ 1822 public HealthcareService setSpecialty(List<CodeableConcept> theSpecialty) { 1823 this.specialty = theSpecialty; 1824 return this; 1825 } 1826 1827 public boolean hasSpecialty() { 1828 if (this.specialty == null) 1829 return false; 1830 for (CodeableConcept item : this.specialty) 1831 if (!item.isEmpty()) 1832 return true; 1833 return false; 1834 } 1835 1836 public CodeableConcept addSpecialty() { // 3 1837 CodeableConcept t = new CodeableConcept(); 1838 if (this.specialty == null) 1839 this.specialty = new ArrayList<CodeableConcept>(); 1840 this.specialty.add(t); 1841 return t; 1842 } 1843 1844 public HealthcareService addSpecialty(CodeableConcept t) { // 3 1845 if (t == null) 1846 return this; 1847 if (this.specialty == null) 1848 this.specialty = new ArrayList<CodeableConcept>(); 1849 this.specialty.add(t); 1850 return this; 1851 } 1852 1853 /** 1854 * @return The first repetition of repeating field {@link #specialty}, creating 1855 * it if it does not already exist 1856 */ 1857 public CodeableConcept getSpecialtyFirstRep() { 1858 if (getSpecialty().isEmpty()) { 1859 addSpecialty(); 1860 } 1861 return getSpecialty().get(0); 1862 } 1863 1864 /** 1865 * @return {@link #location} (The location(s) where this healthcare service may 1866 * be provided.) 1867 */ 1868 public List<Reference> getLocation() { 1869 if (this.location == null) 1870 this.location = new ArrayList<Reference>(); 1871 return this.location; 1872 } 1873 1874 /** 1875 * @return Returns a reference to <code>this</code> for easy method chaining 1876 */ 1877 public HealthcareService setLocation(List<Reference> theLocation) { 1878 this.location = theLocation; 1879 return this; 1880 } 1881 1882 public boolean hasLocation() { 1883 if (this.location == null) 1884 return false; 1885 for (Reference item : this.location) 1886 if (!item.isEmpty()) 1887 return true; 1888 return false; 1889 } 1890 1891 public Reference addLocation() { // 3 1892 Reference t = new Reference(); 1893 if (this.location == null) 1894 this.location = new ArrayList<Reference>(); 1895 this.location.add(t); 1896 return t; 1897 } 1898 1899 public HealthcareService addLocation(Reference t) { // 3 1900 if (t == null) 1901 return this; 1902 if (this.location == null) 1903 this.location = new ArrayList<Reference>(); 1904 this.location.add(t); 1905 return this; 1906 } 1907 1908 /** 1909 * @return The first repetition of repeating field {@link #location}, creating 1910 * it if it does not already exist 1911 */ 1912 public Reference getLocationFirstRep() { 1913 if (getLocation().isEmpty()) { 1914 addLocation(); 1915 } 1916 return getLocation().get(0); 1917 } 1918 1919 /** 1920 * @return {@link #name} (Further description of the service as it would be 1921 * presented to a consumer while searching.). This is the underlying 1922 * object with id, value and extensions. The accessor "getName" gives 1923 * direct access to the value 1924 */ 1925 public StringType getNameElement() { 1926 if (this.name == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create HealthcareService.name"); 1929 else if (Configuration.doAutoCreate()) 1930 this.name = new StringType(); // bb 1931 return this.name; 1932 } 1933 1934 public boolean hasNameElement() { 1935 return this.name != null && !this.name.isEmpty(); 1936 } 1937 1938 public boolean hasName() { 1939 return this.name != null && !this.name.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #name} (Further description of the service as it would be 1944 * presented to a consumer while searching.). This is the 1945 * underlying object with id, value and extensions. The accessor 1946 * "getName" gives direct access to the value 1947 */ 1948 public HealthcareService setNameElement(StringType value) { 1949 this.name = value; 1950 return this; 1951 } 1952 1953 /** 1954 * @return Further description of the service as it would be presented to a 1955 * consumer while searching. 1956 */ 1957 public String getName() { 1958 return this.name == null ? null : this.name.getValue(); 1959 } 1960 1961 /** 1962 * @param value Further description of the service as it would be presented to a 1963 * consumer while searching. 1964 */ 1965 public HealthcareService setName(String value) { 1966 if (Utilities.noString(value)) 1967 this.name = null; 1968 else { 1969 if (this.name == null) 1970 this.name = new StringType(); 1971 this.name.setValue(value); 1972 } 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #comment} (Any additional description of the service and/or 1978 * any specific issues not covered by the other attributes, which can be 1979 * displayed as further detail under the serviceName.). This is the 1980 * underlying object with id, value and extensions. The accessor 1981 * "getComment" gives direct access to the value 1982 */ 1983 public StringType getCommentElement() { 1984 if (this.comment == null) 1985 if (Configuration.errorOnAutoCreate()) 1986 throw new Error("Attempt to auto-create HealthcareService.comment"); 1987 else if (Configuration.doAutoCreate()) 1988 this.comment = new StringType(); // bb 1989 return this.comment; 1990 } 1991 1992 public boolean hasCommentElement() { 1993 return this.comment != null && !this.comment.isEmpty(); 1994 } 1995 1996 public boolean hasComment() { 1997 return this.comment != null && !this.comment.isEmpty(); 1998 } 1999 2000 /** 2001 * @param value {@link #comment} (Any additional description of the service 2002 * and/or any specific issues not covered by the other attributes, 2003 * which can be displayed as further detail under the 2004 * serviceName.). This is the underlying object with id, value and 2005 * extensions. The accessor "getComment" gives direct access to the 2006 * value 2007 */ 2008 public HealthcareService setCommentElement(StringType value) { 2009 this.comment = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return Any additional description of the service and/or any specific issues 2015 * not covered by the other attributes, which can be displayed as 2016 * further detail under the serviceName. 2017 */ 2018 public String getComment() { 2019 return this.comment == null ? null : this.comment.getValue(); 2020 } 2021 2022 /** 2023 * @param value Any additional description of the service and/or any specific 2024 * issues not covered by the other attributes, which can be 2025 * displayed as further detail under the serviceName. 2026 */ 2027 public HealthcareService setComment(String value) { 2028 if (Utilities.noString(value)) 2029 this.comment = null; 2030 else { 2031 if (this.comment == null) 2032 this.comment = new StringType(); 2033 this.comment.setValue(value); 2034 } 2035 return this; 2036 } 2037 2038 /** 2039 * @return {@link #extraDetails} (Extra details about the service that can't be 2040 * placed in the other fields.). This is the underlying object with id, 2041 * value and extensions. The accessor "getExtraDetails" gives direct 2042 * access to the value 2043 */ 2044 public MarkdownType getExtraDetailsElement() { 2045 if (this.extraDetails == null) 2046 if (Configuration.errorOnAutoCreate()) 2047 throw new Error("Attempt to auto-create HealthcareService.extraDetails"); 2048 else if (Configuration.doAutoCreate()) 2049 this.extraDetails = new MarkdownType(); // bb 2050 return this.extraDetails; 2051 } 2052 2053 public boolean hasExtraDetailsElement() { 2054 return this.extraDetails != null && !this.extraDetails.isEmpty(); 2055 } 2056 2057 public boolean hasExtraDetails() { 2058 return this.extraDetails != null && !this.extraDetails.isEmpty(); 2059 } 2060 2061 /** 2062 * @param value {@link #extraDetails} (Extra details about the service that 2063 * can't be placed in the other fields.). This is the underlying 2064 * object with id, value and extensions. The accessor 2065 * "getExtraDetails" gives direct access to the value 2066 */ 2067 public HealthcareService setExtraDetailsElement(MarkdownType value) { 2068 this.extraDetails = value; 2069 return this; 2070 } 2071 2072 /** 2073 * @return Extra details about the service that can't be placed in the other 2074 * fields. 2075 */ 2076 public String getExtraDetails() { 2077 return this.extraDetails == null ? null : this.extraDetails.getValue(); 2078 } 2079 2080 /** 2081 * @param value Extra details about the service that can't be placed in the 2082 * other fields. 2083 */ 2084 public HealthcareService setExtraDetails(String value) { 2085 if (value == null) 2086 this.extraDetails = null; 2087 else { 2088 if (this.extraDetails == null) 2089 this.extraDetails = new MarkdownType(); 2090 this.extraDetails.setValue(value); 2091 } 2092 return this; 2093 } 2094 2095 /** 2096 * @return {@link #photo} (If there is a photo/symbol associated with this 2097 * HealthcareService, it may be included here to facilitate quick 2098 * identification of the service in a list.) 2099 */ 2100 public Attachment getPhoto() { 2101 if (this.photo == null) 2102 if (Configuration.errorOnAutoCreate()) 2103 throw new Error("Attempt to auto-create HealthcareService.photo"); 2104 else if (Configuration.doAutoCreate()) 2105 this.photo = new Attachment(); // cc 2106 return this.photo; 2107 } 2108 2109 public boolean hasPhoto() { 2110 return this.photo != null && !this.photo.isEmpty(); 2111 } 2112 2113 /** 2114 * @param value {@link #photo} (If there is a photo/symbol associated with this 2115 * HealthcareService, it may be included here to facilitate quick 2116 * identification of the service in a list.) 2117 */ 2118 public HealthcareService setPhoto(Attachment value) { 2119 this.photo = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return {@link #telecom} (List of contacts related to this specific 2125 * healthcare service.) 2126 */ 2127 public List<ContactPoint> getTelecom() { 2128 if (this.telecom == null) 2129 this.telecom = new ArrayList<ContactPoint>(); 2130 return this.telecom; 2131 } 2132 2133 /** 2134 * @return Returns a reference to <code>this</code> for easy method chaining 2135 */ 2136 public HealthcareService setTelecom(List<ContactPoint> theTelecom) { 2137 this.telecom = theTelecom; 2138 return this; 2139 } 2140 2141 public boolean hasTelecom() { 2142 if (this.telecom == null) 2143 return false; 2144 for (ContactPoint item : this.telecom) 2145 if (!item.isEmpty()) 2146 return true; 2147 return false; 2148 } 2149 2150 public ContactPoint addTelecom() { // 3 2151 ContactPoint t = new ContactPoint(); 2152 if (this.telecom == null) 2153 this.telecom = new ArrayList<ContactPoint>(); 2154 this.telecom.add(t); 2155 return t; 2156 } 2157 2158 public HealthcareService addTelecom(ContactPoint t) { // 3 2159 if (t == null) 2160 return this; 2161 if (this.telecom == null) 2162 this.telecom = new ArrayList<ContactPoint>(); 2163 this.telecom.add(t); 2164 return this; 2165 } 2166 2167 /** 2168 * @return The first repetition of repeating field {@link #telecom}, creating it 2169 * if it does not already exist 2170 */ 2171 public ContactPoint getTelecomFirstRep() { 2172 if (getTelecom().isEmpty()) { 2173 addTelecom(); 2174 } 2175 return getTelecom().get(0); 2176 } 2177 2178 /** 2179 * @return {@link #coverageArea} (The location(s) that this service is available 2180 * to (not where the service is provided).) 2181 */ 2182 public List<Reference> getCoverageArea() { 2183 if (this.coverageArea == null) 2184 this.coverageArea = new ArrayList<Reference>(); 2185 return this.coverageArea; 2186 } 2187 2188 /** 2189 * @return Returns a reference to <code>this</code> for easy method chaining 2190 */ 2191 public HealthcareService setCoverageArea(List<Reference> theCoverageArea) { 2192 this.coverageArea = theCoverageArea; 2193 return this; 2194 } 2195 2196 public boolean hasCoverageArea() { 2197 if (this.coverageArea == null) 2198 return false; 2199 for (Reference item : this.coverageArea) 2200 if (!item.isEmpty()) 2201 return true; 2202 return false; 2203 } 2204 2205 public Reference addCoverageArea() { // 3 2206 Reference t = new Reference(); 2207 if (this.coverageArea == null) 2208 this.coverageArea = new ArrayList<Reference>(); 2209 this.coverageArea.add(t); 2210 return t; 2211 } 2212 2213 public HealthcareService addCoverageArea(Reference t) { // 3 2214 if (t == null) 2215 return this; 2216 if (this.coverageArea == null) 2217 this.coverageArea = new ArrayList<Reference>(); 2218 this.coverageArea.add(t); 2219 return this; 2220 } 2221 2222 /** 2223 * @return The first repetition of repeating field {@link #coverageArea}, 2224 * creating it if it does not already exist 2225 */ 2226 public Reference getCoverageAreaFirstRep() { 2227 if (getCoverageArea().isEmpty()) { 2228 addCoverageArea(); 2229 } 2230 return getCoverageArea().get(0); 2231 } 2232 2233 /** 2234 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions 2235 * under which the healthcare service is available/offered.) 2236 */ 2237 public List<CodeableConcept> getServiceProvisionCode() { 2238 if (this.serviceProvisionCode == null) 2239 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2240 return this.serviceProvisionCode; 2241 } 2242 2243 /** 2244 * @return Returns a reference to <code>this</code> for easy method chaining 2245 */ 2246 public HealthcareService setServiceProvisionCode(List<CodeableConcept> theServiceProvisionCode) { 2247 this.serviceProvisionCode = theServiceProvisionCode; 2248 return this; 2249 } 2250 2251 public boolean hasServiceProvisionCode() { 2252 if (this.serviceProvisionCode == null) 2253 return false; 2254 for (CodeableConcept item : this.serviceProvisionCode) 2255 if (!item.isEmpty()) 2256 return true; 2257 return false; 2258 } 2259 2260 public CodeableConcept addServiceProvisionCode() { // 3 2261 CodeableConcept t = new CodeableConcept(); 2262 if (this.serviceProvisionCode == null) 2263 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2264 this.serviceProvisionCode.add(t); 2265 return t; 2266 } 2267 2268 public HealthcareService addServiceProvisionCode(CodeableConcept t) { // 3 2269 if (t == null) 2270 return this; 2271 if (this.serviceProvisionCode == null) 2272 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2273 this.serviceProvisionCode.add(t); 2274 return this; 2275 } 2276 2277 /** 2278 * @return The first repetition of repeating field 2279 * {@link #serviceProvisionCode}, creating it if it does not already 2280 * exist 2281 */ 2282 public CodeableConcept getServiceProvisionCodeFirstRep() { 2283 if (getServiceProvisionCode().isEmpty()) { 2284 addServiceProvisionCode(); 2285 } 2286 return getServiceProvisionCode().get(0); 2287 } 2288 2289 /** 2290 * @return {@link #eligibility} (Does this service have specific eligibility 2291 * requirements that need to be met in order to use the service?) 2292 */ 2293 public List<HealthcareServiceEligibilityComponent> getEligibility() { 2294 if (this.eligibility == null) 2295 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2296 return this.eligibility; 2297 } 2298 2299 /** 2300 * @return Returns a reference to <code>this</code> for easy method chaining 2301 */ 2302 public HealthcareService setEligibility(List<HealthcareServiceEligibilityComponent> theEligibility) { 2303 this.eligibility = theEligibility; 2304 return this; 2305 } 2306 2307 public boolean hasEligibility() { 2308 if (this.eligibility == null) 2309 return false; 2310 for (HealthcareServiceEligibilityComponent item : this.eligibility) 2311 if (!item.isEmpty()) 2312 return true; 2313 return false; 2314 } 2315 2316 public HealthcareServiceEligibilityComponent addEligibility() { // 3 2317 HealthcareServiceEligibilityComponent t = new HealthcareServiceEligibilityComponent(); 2318 if (this.eligibility == null) 2319 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2320 this.eligibility.add(t); 2321 return t; 2322 } 2323 2324 public HealthcareService addEligibility(HealthcareServiceEligibilityComponent t) { // 3 2325 if (t == null) 2326 return this; 2327 if (this.eligibility == null) 2328 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2329 this.eligibility.add(t); 2330 return this; 2331 } 2332 2333 /** 2334 * @return The first repetition of repeating field {@link #eligibility}, 2335 * creating it if it does not already exist 2336 */ 2337 public HealthcareServiceEligibilityComponent getEligibilityFirstRep() { 2338 if (getEligibility().isEmpty()) { 2339 addEligibility(); 2340 } 2341 return getEligibility().get(0); 2342 } 2343 2344 /** 2345 * @return {@link #program} (Programs that this service is applicable to.) 2346 */ 2347 public List<CodeableConcept> getProgram() { 2348 if (this.program == null) 2349 this.program = new ArrayList<CodeableConcept>(); 2350 return this.program; 2351 } 2352 2353 /** 2354 * @return Returns a reference to <code>this</code> for easy method chaining 2355 */ 2356 public HealthcareService setProgram(List<CodeableConcept> theProgram) { 2357 this.program = theProgram; 2358 return this; 2359 } 2360 2361 public boolean hasProgram() { 2362 if (this.program == null) 2363 return false; 2364 for (CodeableConcept item : this.program) 2365 if (!item.isEmpty()) 2366 return true; 2367 return false; 2368 } 2369 2370 public CodeableConcept addProgram() { // 3 2371 CodeableConcept t = new CodeableConcept(); 2372 if (this.program == null) 2373 this.program = new ArrayList<CodeableConcept>(); 2374 this.program.add(t); 2375 return t; 2376 } 2377 2378 public HealthcareService addProgram(CodeableConcept t) { // 3 2379 if (t == null) 2380 return this; 2381 if (this.program == null) 2382 this.program = new ArrayList<CodeableConcept>(); 2383 this.program.add(t); 2384 return this; 2385 } 2386 2387 /** 2388 * @return The first repetition of repeating field {@link #program}, creating it 2389 * if it does not already exist 2390 */ 2391 public CodeableConcept getProgramFirstRep() { 2392 if (getProgram().isEmpty()) { 2393 addProgram(); 2394 } 2395 return getProgram().get(0); 2396 } 2397 2398 /** 2399 * @return {@link #characteristic} (Collection of characteristics (attributes).) 2400 */ 2401 public List<CodeableConcept> getCharacteristic() { 2402 if (this.characteristic == null) 2403 this.characteristic = new ArrayList<CodeableConcept>(); 2404 return this.characteristic; 2405 } 2406 2407 /** 2408 * @return Returns a reference to <code>this</code> for easy method chaining 2409 */ 2410 public HealthcareService setCharacteristic(List<CodeableConcept> theCharacteristic) { 2411 this.characteristic = theCharacteristic; 2412 return this; 2413 } 2414 2415 public boolean hasCharacteristic() { 2416 if (this.characteristic == null) 2417 return false; 2418 for (CodeableConcept item : this.characteristic) 2419 if (!item.isEmpty()) 2420 return true; 2421 return false; 2422 } 2423 2424 public CodeableConcept addCharacteristic() { // 3 2425 CodeableConcept t = new CodeableConcept(); 2426 if (this.characteristic == null) 2427 this.characteristic = new ArrayList<CodeableConcept>(); 2428 this.characteristic.add(t); 2429 return t; 2430 } 2431 2432 public HealthcareService addCharacteristic(CodeableConcept t) { // 3 2433 if (t == null) 2434 return this; 2435 if (this.characteristic == null) 2436 this.characteristic = new ArrayList<CodeableConcept>(); 2437 this.characteristic.add(t); 2438 return this; 2439 } 2440 2441 /** 2442 * @return The first repetition of repeating field {@link #characteristic}, 2443 * creating it if it does not already exist 2444 */ 2445 public CodeableConcept getCharacteristicFirstRep() { 2446 if (getCharacteristic().isEmpty()) { 2447 addCharacteristic(); 2448 } 2449 return getCharacteristic().get(0); 2450 } 2451 2452 /** 2453 * @return {@link #communication} (Some services are specifically made available 2454 * in multiple languages, this property permits a directory to declare 2455 * the languages this is offered in. Typically this is only provided 2456 * where a service operates in communities with mixed languages used.) 2457 */ 2458 public List<CodeableConcept> getCommunication() { 2459 if (this.communication == null) 2460 this.communication = new ArrayList<CodeableConcept>(); 2461 return this.communication; 2462 } 2463 2464 /** 2465 * @return Returns a reference to <code>this</code> for easy method chaining 2466 */ 2467 public HealthcareService setCommunication(List<CodeableConcept> theCommunication) { 2468 this.communication = theCommunication; 2469 return this; 2470 } 2471 2472 public boolean hasCommunication() { 2473 if (this.communication == null) 2474 return false; 2475 for (CodeableConcept item : this.communication) 2476 if (!item.isEmpty()) 2477 return true; 2478 return false; 2479 } 2480 2481 public CodeableConcept addCommunication() { // 3 2482 CodeableConcept t = new CodeableConcept(); 2483 if (this.communication == null) 2484 this.communication = new ArrayList<CodeableConcept>(); 2485 this.communication.add(t); 2486 return t; 2487 } 2488 2489 public HealthcareService addCommunication(CodeableConcept t) { // 3 2490 if (t == null) 2491 return this; 2492 if (this.communication == null) 2493 this.communication = new ArrayList<CodeableConcept>(); 2494 this.communication.add(t); 2495 return this; 2496 } 2497 2498 /** 2499 * @return The first repetition of repeating field {@link #communication}, 2500 * creating it if it does not already exist 2501 */ 2502 public CodeableConcept getCommunicationFirstRep() { 2503 if (getCommunication().isEmpty()) { 2504 addCommunication(); 2505 } 2506 return getCommunication().get(0); 2507 } 2508 2509 /** 2510 * @return {@link #referralMethod} (Ways that the service accepts referrals, if 2511 * this is not provided then it is implied that no referral is 2512 * required.) 2513 */ 2514 public List<CodeableConcept> getReferralMethod() { 2515 if (this.referralMethod == null) 2516 this.referralMethod = new ArrayList<CodeableConcept>(); 2517 return this.referralMethod; 2518 } 2519 2520 /** 2521 * @return Returns a reference to <code>this</code> for easy method chaining 2522 */ 2523 public HealthcareService setReferralMethod(List<CodeableConcept> theReferralMethod) { 2524 this.referralMethod = theReferralMethod; 2525 return this; 2526 } 2527 2528 public boolean hasReferralMethod() { 2529 if (this.referralMethod == null) 2530 return false; 2531 for (CodeableConcept item : this.referralMethod) 2532 if (!item.isEmpty()) 2533 return true; 2534 return false; 2535 } 2536 2537 public CodeableConcept addReferralMethod() { // 3 2538 CodeableConcept t = new CodeableConcept(); 2539 if (this.referralMethod == null) 2540 this.referralMethod = new ArrayList<CodeableConcept>(); 2541 this.referralMethod.add(t); 2542 return t; 2543 } 2544 2545 public HealthcareService addReferralMethod(CodeableConcept t) { // 3 2546 if (t == null) 2547 return this; 2548 if (this.referralMethod == null) 2549 this.referralMethod = new ArrayList<CodeableConcept>(); 2550 this.referralMethod.add(t); 2551 return this; 2552 } 2553 2554 /** 2555 * @return The first repetition of repeating field {@link #referralMethod}, 2556 * creating it if it does not already exist 2557 */ 2558 public CodeableConcept getReferralMethodFirstRep() { 2559 if (getReferralMethod().isEmpty()) { 2560 addReferralMethod(); 2561 } 2562 return getReferralMethod().get(0); 2563 } 2564 2565 /** 2566 * @return {@link #appointmentRequired} (Indicates whether or not a prospective 2567 * consumer will require an appointment for a particular service at a 2568 * site to be provided by the Organization. Indicates if an appointment 2569 * is required for access to this service.). This is the underlying 2570 * object with id, value and extensions. The accessor 2571 * "getAppointmentRequired" gives direct access to the value 2572 */ 2573 public BooleanType getAppointmentRequiredElement() { 2574 if (this.appointmentRequired == null) 2575 if (Configuration.errorOnAutoCreate()) 2576 throw new Error("Attempt to auto-create HealthcareService.appointmentRequired"); 2577 else if (Configuration.doAutoCreate()) 2578 this.appointmentRequired = new BooleanType(); // bb 2579 return this.appointmentRequired; 2580 } 2581 2582 public boolean hasAppointmentRequiredElement() { 2583 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2584 } 2585 2586 public boolean hasAppointmentRequired() { 2587 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2588 } 2589 2590 /** 2591 * @param value {@link #appointmentRequired} (Indicates whether or not a 2592 * prospective consumer will require an appointment for a 2593 * particular service at a site to be provided by the Organization. 2594 * Indicates if an appointment is required for access to this 2595 * service.). This is the underlying object with id, value and 2596 * extensions. The accessor "getAppointmentRequired" gives direct 2597 * access to the value 2598 */ 2599 public HealthcareService setAppointmentRequiredElement(BooleanType value) { 2600 this.appointmentRequired = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return Indicates whether or not a prospective consumer will require an 2606 * appointment for a particular service at a site to be provided by the 2607 * Organization. Indicates if an appointment is required for access to 2608 * this service. 2609 */ 2610 public boolean getAppointmentRequired() { 2611 return this.appointmentRequired == null || this.appointmentRequired.isEmpty() ? false 2612 : this.appointmentRequired.getValue(); 2613 } 2614 2615 /** 2616 * @param value Indicates whether or not a prospective consumer will require an 2617 * appointment for a particular service at a site to be provided by 2618 * the Organization. Indicates if an appointment is required for 2619 * access to this service. 2620 */ 2621 public HealthcareService setAppointmentRequired(boolean value) { 2622 if (this.appointmentRequired == null) 2623 this.appointmentRequired = new BooleanType(); 2624 this.appointmentRequired.setValue(value); 2625 return this; 2626 } 2627 2628 /** 2629 * @return {@link #availableTime} (A collection of times that the Service Site 2630 * is available.) 2631 */ 2632 public List<HealthcareServiceAvailableTimeComponent> getAvailableTime() { 2633 if (this.availableTime == null) 2634 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2635 return this.availableTime; 2636 } 2637 2638 /** 2639 * @return Returns a reference to <code>this</code> for easy method chaining 2640 */ 2641 public HealthcareService setAvailableTime(List<HealthcareServiceAvailableTimeComponent> theAvailableTime) { 2642 this.availableTime = theAvailableTime; 2643 return this; 2644 } 2645 2646 public boolean hasAvailableTime() { 2647 if (this.availableTime == null) 2648 return false; 2649 for (HealthcareServiceAvailableTimeComponent item : this.availableTime) 2650 if (!item.isEmpty()) 2651 return true; 2652 return false; 2653 } 2654 2655 public HealthcareServiceAvailableTimeComponent addAvailableTime() { // 3 2656 HealthcareServiceAvailableTimeComponent t = new HealthcareServiceAvailableTimeComponent(); 2657 if (this.availableTime == null) 2658 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2659 this.availableTime.add(t); 2660 return t; 2661 } 2662 2663 public HealthcareService addAvailableTime(HealthcareServiceAvailableTimeComponent t) { // 3 2664 if (t == null) 2665 return this; 2666 if (this.availableTime == null) 2667 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2668 this.availableTime.add(t); 2669 return this; 2670 } 2671 2672 /** 2673 * @return The first repetition of repeating field {@link #availableTime}, 2674 * creating it if it does not already exist 2675 */ 2676 public HealthcareServiceAvailableTimeComponent getAvailableTimeFirstRep() { 2677 if (getAvailableTime().isEmpty()) { 2678 addAvailableTime(); 2679 } 2680 return getAvailableTime().get(0); 2681 } 2682 2683 /** 2684 * @return {@link #notAvailable} (The HealthcareService is not available during 2685 * this period of time due to the provided reason.) 2686 */ 2687 public List<HealthcareServiceNotAvailableComponent> getNotAvailable() { 2688 if (this.notAvailable == null) 2689 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2690 return this.notAvailable; 2691 } 2692 2693 /** 2694 * @return Returns a reference to <code>this</code> for easy method chaining 2695 */ 2696 public HealthcareService setNotAvailable(List<HealthcareServiceNotAvailableComponent> theNotAvailable) { 2697 this.notAvailable = theNotAvailable; 2698 return this; 2699 } 2700 2701 public boolean hasNotAvailable() { 2702 if (this.notAvailable == null) 2703 return false; 2704 for (HealthcareServiceNotAvailableComponent item : this.notAvailable) 2705 if (!item.isEmpty()) 2706 return true; 2707 return false; 2708 } 2709 2710 public HealthcareServiceNotAvailableComponent addNotAvailable() { // 3 2711 HealthcareServiceNotAvailableComponent t = new HealthcareServiceNotAvailableComponent(); 2712 if (this.notAvailable == null) 2713 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2714 this.notAvailable.add(t); 2715 return t; 2716 } 2717 2718 public HealthcareService addNotAvailable(HealthcareServiceNotAvailableComponent t) { // 3 2719 if (t == null) 2720 return this; 2721 if (this.notAvailable == null) 2722 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2723 this.notAvailable.add(t); 2724 return this; 2725 } 2726 2727 /** 2728 * @return The first repetition of repeating field {@link #notAvailable}, 2729 * creating it if it does not already exist 2730 */ 2731 public HealthcareServiceNotAvailableComponent getNotAvailableFirstRep() { 2732 if (getNotAvailable().isEmpty()) { 2733 addNotAvailable(); 2734 } 2735 return getNotAvailable().get(0); 2736 } 2737 2738 /** 2739 * @return {@link #availabilityExceptions} (A description of site availability 2740 * exceptions, e.g. public holiday availability. Succinctly describing 2741 * all possible exceptions to normal site availability as details in the 2742 * available Times and not available Times.). This is the underlying 2743 * object with id, value and extensions. The accessor 2744 * "getAvailabilityExceptions" gives direct access to the value 2745 */ 2746 public StringType getAvailabilityExceptionsElement() { 2747 if (this.availabilityExceptions == null) 2748 if (Configuration.errorOnAutoCreate()) 2749 throw new Error("Attempt to auto-create HealthcareService.availabilityExceptions"); 2750 else if (Configuration.doAutoCreate()) 2751 this.availabilityExceptions = new StringType(); // bb 2752 return this.availabilityExceptions; 2753 } 2754 2755 public boolean hasAvailabilityExceptionsElement() { 2756 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2757 } 2758 2759 public boolean hasAvailabilityExceptions() { 2760 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2761 } 2762 2763 /** 2764 * @param value {@link #availabilityExceptions} (A description of site 2765 * availability exceptions, e.g. public holiday availability. 2766 * Succinctly describing all possible exceptions to normal site 2767 * availability as details in the available Times and not available 2768 * Times.). This is the underlying object with id, value and 2769 * extensions. The accessor "getAvailabilityExceptions" gives 2770 * direct access to the value 2771 */ 2772 public HealthcareService setAvailabilityExceptionsElement(StringType value) { 2773 this.availabilityExceptions = value; 2774 return this; 2775 } 2776 2777 /** 2778 * @return A description of site availability exceptions, e.g. public holiday 2779 * availability. Succinctly describing all possible exceptions to normal 2780 * site availability as details in the available Times and not available 2781 * Times. 2782 */ 2783 public String getAvailabilityExceptions() { 2784 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2785 } 2786 2787 /** 2788 * @param value A description of site availability exceptions, e.g. public 2789 * holiday availability. Succinctly describing all possible 2790 * exceptions to normal site availability as details in the 2791 * available Times and not available Times. 2792 */ 2793 public HealthcareService setAvailabilityExceptions(String value) { 2794 if (Utilities.noString(value)) 2795 this.availabilityExceptions = null; 2796 else { 2797 if (this.availabilityExceptions == null) 2798 this.availabilityExceptions = new StringType(); 2799 this.availabilityExceptions.setValue(value); 2800 } 2801 return this; 2802 } 2803 2804 /** 2805 * @return {@link #endpoint} (Technical endpoints providing access to services 2806 * operated for the specific healthcare services defined at this 2807 * resource.) 2808 */ 2809 public List<Reference> getEndpoint() { 2810 if (this.endpoint == null) 2811 this.endpoint = new ArrayList<Reference>(); 2812 return this.endpoint; 2813 } 2814 2815 /** 2816 * @return Returns a reference to <code>this</code> for easy method chaining 2817 */ 2818 public HealthcareService setEndpoint(List<Reference> theEndpoint) { 2819 this.endpoint = theEndpoint; 2820 return this; 2821 } 2822 2823 public boolean hasEndpoint() { 2824 if (this.endpoint == null) 2825 return false; 2826 for (Reference item : this.endpoint) 2827 if (!item.isEmpty()) 2828 return true; 2829 return false; 2830 } 2831 2832 public Reference addEndpoint() { // 3 2833 Reference t = new Reference(); 2834 if (this.endpoint == null) 2835 this.endpoint = new ArrayList<Reference>(); 2836 this.endpoint.add(t); 2837 return t; 2838 } 2839 2840 public HealthcareService addEndpoint(Reference t) { // 3 2841 if (t == null) 2842 return this; 2843 if (this.endpoint == null) 2844 this.endpoint = new ArrayList<Reference>(); 2845 this.endpoint.add(t); 2846 return this; 2847 } 2848 2849 /** 2850 * @return The first repetition of repeating field {@link #endpoint}, creating 2851 * it if it does not already exist 2852 */ 2853 public Reference getEndpointFirstRep() { 2854 if (getEndpoint().isEmpty()) { 2855 addEndpoint(); 2856 } 2857 return getEndpoint().get(0); 2858 } 2859 2860 protected void listChildren(List<Property> children) { 2861 super.listChildren(children); 2862 children.add(new Property("identifier", "Identifier", "External identifiers for this item.", 0, 2863 java.lang.Integer.MAX_VALUE, identifier)); 2864 children.add(new Property("active", "boolean", 2865 "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 2866 0, 1, active)); 2867 children.add(new Property("providedBy", "Reference(Organization)", 2868 "The organization that provides this healthcare service.", 0, 1, providedBy)); 2869 children.add(new Property("category", "CodeableConcept", 2870 "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, 2871 category)); 2872 children.add(new Property("type", "CodeableConcept", 2873 "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type)); 2874 children.add(new Property("specialty", "CodeableConcept", 2875 "Collection of specialties handled by the service site. This is more of a medical term.", 0, 2876 java.lang.Integer.MAX_VALUE, specialty)); 2877 children.add(new Property("location", "Reference(Location)", 2878 "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location)); 2879 children.add(new Property("name", "string", 2880 "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name)); 2881 children.add(new Property("comment", "string", 2882 "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 2883 0, 1, comment)); 2884 children.add(new Property("extraDetails", "markdown", 2885 "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails)); 2886 children.add(new Property("photo", "Attachment", 2887 "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 2888 0, 1, photo)); 2889 children.add(new Property("telecom", "ContactPoint", 2890 "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2891 children.add(new Property("coverageArea", "Reference(Location)", 2892 "The location(s) that this service is available to (not where the service is provided).", 0, 2893 java.lang.Integer.MAX_VALUE, coverageArea)); 2894 children.add(new Property("serviceProvisionCode", "CodeableConcept", 2895 "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, 2896 java.lang.Integer.MAX_VALUE, serviceProvisionCode)); 2897 children.add(new Property("eligibility", "", 2898 "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, 2899 java.lang.Integer.MAX_VALUE, eligibility)); 2900 children.add(new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, 2901 java.lang.Integer.MAX_VALUE, program)); 2902 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, 2903 java.lang.Integer.MAX_VALUE, characteristic)); 2904 children.add(new Property("communication", "CodeableConcept", 2905 "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 2906 0, java.lang.Integer.MAX_VALUE, communication)); 2907 children.add(new Property("referralMethod", "CodeableConcept", 2908 "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 2909 0, java.lang.Integer.MAX_VALUE, referralMethod)); 2910 children.add(new Property("appointmentRequired", "boolean", 2911 "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 2912 0, 1, appointmentRequired)); 2913 children.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, 2914 java.lang.Integer.MAX_VALUE, availableTime)); 2915 children.add(new Property("notAvailable", "", 2916 "The HealthcareService is not available during this period of time due to the provided reason.", 0, 2917 java.lang.Integer.MAX_VALUE, notAvailable)); 2918 children.add(new Property("availabilityExceptions", "string", 2919 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 2920 0, 1, availabilityExceptions)); 2921 children.add(new Property("endpoint", "Reference(Endpoint)", 2922 "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 2923 0, java.lang.Integer.MAX_VALUE, endpoint)); 2924 } 2925 2926 @Override 2927 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2928 switch (_hash) { 2929 case -1618432855: 2930 /* identifier */ return new Property("identifier", "Identifier", "External identifiers for this item.", 0, 2931 java.lang.Integer.MAX_VALUE, identifier); 2932 case -1422950650: 2933 /* active */ return new Property("active", "boolean", 2934 "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 2935 0, 1, active); 2936 case 205136282: 2937 /* providedBy */ return new Property("providedBy", "Reference(Organization)", 2938 "The organization that provides this healthcare service.", 0, 1, providedBy); 2939 case 50511102: 2940 /* category */ return new Property("category", "CodeableConcept", 2941 "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, 2942 category); 2943 case 3575610: 2944 /* type */ return new Property("type", "CodeableConcept", 2945 "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type); 2946 case -1694759682: 2947 /* specialty */ return new Property("specialty", "CodeableConcept", 2948 "Collection of specialties handled by the service site. This is more of a medical term.", 0, 2949 java.lang.Integer.MAX_VALUE, specialty); 2950 case 1901043637: 2951 /* location */ return new Property("location", "Reference(Location)", 2952 "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location); 2953 case 3373707: 2954 /* name */ return new Property("name", "string", 2955 "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name); 2956 case 950398559: 2957 /* comment */ return new Property("comment", "string", 2958 "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 2959 0, 1, comment); 2960 case -1469168622: 2961 /* extraDetails */ return new Property("extraDetails", "markdown", 2962 "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails); 2963 case 106642994: 2964 /* photo */ return new Property("photo", "Attachment", 2965 "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 2966 0, 1, photo); 2967 case -1429363305: 2968 /* telecom */ return new Property("telecom", "ContactPoint", 2969 "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom); 2970 case -1532328299: 2971 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 2972 "The location(s) that this service is available to (not where the service is provided).", 0, 2973 java.lang.Integer.MAX_VALUE, coverageArea); 2974 case 1504575405: 2975 /* serviceProvisionCode */ return new Property("serviceProvisionCode", "CodeableConcept", 2976 "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, 2977 java.lang.Integer.MAX_VALUE, serviceProvisionCode); 2978 case -930847859: 2979 /* eligibility */ return new Property("eligibility", "", 2980 "Does this service have specific eligibility requirements that need to be met in order to use the service?", 2981 0, java.lang.Integer.MAX_VALUE, eligibility); 2982 case -309387644: 2983 /* program */ return new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, 2984 java.lang.Integer.MAX_VALUE, program); 2985 case 366313883: 2986 /* characteristic */ return new Property("characteristic", "CodeableConcept", 2987 "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 2988 case -1035284522: 2989 /* communication */ return new Property("communication", "CodeableConcept", 2990 "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 2991 0, java.lang.Integer.MAX_VALUE, communication); 2992 case -2092740898: 2993 /* referralMethod */ return new Property("referralMethod", "CodeableConcept", 2994 "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 2995 0, java.lang.Integer.MAX_VALUE, referralMethod); 2996 case 427220062: 2997 /* appointmentRequired */ return new Property("appointmentRequired", "boolean", 2998 "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 2999 0, 1, appointmentRequired); 3000 case 1873069366: 3001 /* availableTime */ return new Property("availableTime", "", 3002 "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 3003 case -629572298: 3004 /* notAvailable */ return new Property("notAvailable", "", 3005 "The HealthcareService is not available during this period of time due to the provided reason.", 0, 3006 java.lang.Integer.MAX_VALUE, notAvailable); 3007 case -1149143617: 3008 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 3009 "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 3010 0, 1, availabilityExceptions); 3011 case 1741102485: 3012 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 3013 "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 3014 0, java.lang.Integer.MAX_VALUE, endpoint); 3015 default: 3016 return super.getNamedProperty(_hash, _name, _checkValid); 3017 } 3018 3019 } 3020 3021 @Override 3022 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3023 switch (hash) { 3024 case -1618432855: 3025 /* identifier */ return this.identifier == null ? new Base[0] 3026 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3027 case -1422950650: 3028 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 3029 case 205136282: 3030 /* providedBy */ return this.providedBy == null ? new Base[0] : new Base[] { this.providedBy }; // Reference 3031 case 50511102: 3032 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3033 case 3575610: 3034 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3035 case -1694759682: 3036 /* specialty */ return this.specialty == null ? new Base[0] 3037 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 3038 case 1901043637: 3039 /* location */ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 3040 case 3373707: 3041 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 3042 case 950398559: 3043 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 3044 case -1469168622: 3045 /* extraDetails */ return this.extraDetails == null ? new Base[0] : new Base[] { this.extraDetails }; // MarkdownType 3046 case 106642994: 3047 /* photo */ return this.photo == null ? new Base[0] : new Base[] { this.photo }; // Attachment 3048 case -1429363305: 3049 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 3050 case -1532328299: 3051 /* coverageArea */ return this.coverageArea == null ? new Base[0] 3052 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 3053 case 1504575405: 3054 /* serviceProvisionCode */ return this.serviceProvisionCode == null ? new Base[0] 3055 : this.serviceProvisionCode.toArray(new Base[this.serviceProvisionCode.size()]); // CodeableConcept 3056 case -930847859: 3057 /* eligibility */ return this.eligibility == null ? new Base[0] 3058 : this.eligibility.toArray(new Base[this.eligibility.size()]); // HealthcareServiceEligibilityComponent 3059 case -309387644: 3060 /* program */ return this.program == null ? new Base[0] : this.program.toArray(new Base[this.program.size()]); // CodeableConcept 3061 case 366313883: 3062 /* characteristic */ return this.characteristic == null ? new Base[0] 3063 : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 3064 case -1035284522: 3065 /* communication */ return this.communication == null ? new Base[0] 3066 : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 3067 case -2092740898: 3068 /* referralMethod */ return this.referralMethod == null ? new Base[0] 3069 : this.referralMethod.toArray(new Base[this.referralMethod.size()]); // CodeableConcept 3070 case 427220062: 3071 /* appointmentRequired */ return this.appointmentRequired == null ? new Base[0] 3072 : new Base[] { this.appointmentRequired }; // BooleanType 3073 case 1873069366: 3074 /* availableTime */ return this.availableTime == null ? new Base[0] 3075 : this.availableTime.toArray(new Base[this.availableTime.size()]); // HealthcareServiceAvailableTimeComponent 3076 case -629572298: 3077 /* notAvailable */ return this.notAvailable == null ? new Base[0] 3078 : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // HealthcareServiceNotAvailableComponent 3079 case -1149143617: 3080 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 3081 : new Base[] { this.availabilityExceptions }; // StringType 3082 case 1741102485: 3083 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 3084 default: 3085 return super.getProperty(hash, name, checkValid); 3086 } 3087 3088 } 3089 3090 @Override 3091 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3092 switch (hash) { 3093 case -1618432855: // identifier 3094 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3095 return value; 3096 case -1422950650: // active 3097 this.active = castToBoolean(value); // BooleanType 3098 return value; 3099 case 205136282: // providedBy 3100 this.providedBy = castToReference(value); // Reference 3101 return value; 3102 case 50511102: // category 3103 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3104 return value; 3105 case 3575610: // type 3106 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3107 return value; 3108 case -1694759682: // specialty 3109 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 3110 return value; 3111 case 1901043637: // location 3112 this.getLocation().add(castToReference(value)); // Reference 3113 return value; 3114 case 3373707: // name 3115 this.name = castToString(value); // StringType 3116 return value; 3117 case 950398559: // comment 3118 this.comment = castToString(value); // StringType 3119 return value; 3120 case -1469168622: // extraDetails 3121 this.extraDetails = castToMarkdown(value); // MarkdownType 3122 return value; 3123 case 106642994: // photo 3124 this.photo = castToAttachment(value); // Attachment 3125 return value; 3126 case -1429363305: // telecom 3127 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 3128 return value; 3129 case -1532328299: // coverageArea 3130 this.getCoverageArea().add(castToReference(value)); // Reference 3131 return value; 3132 case 1504575405: // serviceProvisionCode 3133 this.getServiceProvisionCode().add(castToCodeableConcept(value)); // CodeableConcept 3134 return value; 3135 case -930847859: // eligibility 3136 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); // HealthcareServiceEligibilityComponent 3137 return value; 3138 case -309387644: // program 3139 this.getProgram().add(castToCodeableConcept(value)); // CodeableConcept 3140 return value; 3141 case 366313883: // characteristic 3142 this.getCharacteristic().add(castToCodeableConcept(value)); // CodeableConcept 3143 return value; 3144 case -1035284522: // communication 3145 this.getCommunication().add(castToCodeableConcept(value)); // CodeableConcept 3146 return value; 3147 case -2092740898: // referralMethod 3148 this.getReferralMethod().add(castToCodeableConcept(value)); // CodeableConcept 3149 return value; 3150 case 427220062: // appointmentRequired 3151 this.appointmentRequired = castToBoolean(value); // BooleanType 3152 return value; 3153 case 1873069366: // availableTime 3154 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); // HealthcareServiceAvailableTimeComponent 3155 return value; 3156 case -629572298: // notAvailable 3157 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); // HealthcareServiceNotAvailableComponent 3158 return value; 3159 case -1149143617: // availabilityExceptions 3160 this.availabilityExceptions = castToString(value); // StringType 3161 return value; 3162 case 1741102485: // endpoint 3163 this.getEndpoint().add(castToReference(value)); // Reference 3164 return value; 3165 default: 3166 return super.setProperty(hash, name, value); 3167 } 3168 3169 } 3170 3171 @Override 3172 public Base setProperty(String name, Base value) throws FHIRException { 3173 if (name.equals("identifier")) { 3174 this.getIdentifier().add(castToIdentifier(value)); 3175 } else if (name.equals("active")) { 3176 this.active = castToBoolean(value); // BooleanType 3177 } else if (name.equals("providedBy")) { 3178 this.providedBy = castToReference(value); // Reference 3179 } else if (name.equals("category")) { 3180 this.getCategory().add(castToCodeableConcept(value)); 3181 } else if (name.equals("type")) { 3182 this.getType().add(castToCodeableConcept(value)); 3183 } else if (name.equals("specialty")) { 3184 this.getSpecialty().add(castToCodeableConcept(value)); 3185 } else if (name.equals("location")) { 3186 this.getLocation().add(castToReference(value)); 3187 } else if (name.equals("name")) { 3188 this.name = castToString(value); // StringType 3189 } else if (name.equals("comment")) { 3190 this.comment = castToString(value); // StringType 3191 } else if (name.equals("extraDetails")) { 3192 this.extraDetails = castToMarkdown(value); // MarkdownType 3193 } else if (name.equals("photo")) { 3194 this.photo = castToAttachment(value); // Attachment 3195 } else if (name.equals("telecom")) { 3196 this.getTelecom().add(castToContactPoint(value)); 3197 } else if (name.equals("coverageArea")) { 3198 this.getCoverageArea().add(castToReference(value)); 3199 } else if (name.equals("serviceProvisionCode")) { 3200 this.getServiceProvisionCode().add(castToCodeableConcept(value)); 3201 } else if (name.equals("eligibility")) { 3202 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); 3203 } else if (name.equals("program")) { 3204 this.getProgram().add(castToCodeableConcept(value)); 3205 } else if (name.equals("characteristic")) { 3206 this.getCharacteristic().add(castToCodeableConcept(value)); 3207 } else if (name.equals("communication")) { 3208 this.getCommunication().add(castToCodeableConcept(value)); 3209 } else if (name.equals("referralMethod")) { 3210 this.getReferralMethod().add(castToCodeableConcept(value)); 3211 } else if (name.equals("appointmentRequired")) { 3212 this.appointmentRequired = castToBoolean(value); // BooleanType 3213 } else if (name.equals("availableTime")) { 3214 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); 3215 } else if (name.equals("notAvailable")) { 3216 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); 3217 } else if (name.equals("availabilityExceptions")) { 3218 this.availabilityExceptions = castToString(value); // StringType 3219 } else if (name.equals("endpoint")) { 3220 this.getEndpoint().add(castToReference(value)); 3221 } else 3222 return super.setProperty(name, value); 3223 return value; 3224 } 3225 3226 @Override 3227 public void removeChild(String name, Base value) throws FHIRException { 3228 if (name.equals("identifier")) { 3229 this.getIdentifier().remove(castToIdentifier(value)); 3230 } else if (name.equals("active")) { 3231 this.active = null; 3232 } else if (name.equals("providedBy")) { 3233 this.providedBy = null; 3234 } else if (name.equals("category")) { 3235 this.getCategory().remove(castToCodeableConcept(value)); 3236 } else if (name.equals("type")) { 3237 this.getType().remove(castToCodeableConcept(value)); 3238 } else if (name.equals("specialty")) { 3239 this.getSpecialty().remove(castToCodeableConcept(value)); 3240 } else if (name.equals("location")) { 3241 this.getLocation().remove(castToReference(value)); 3242 } else if (name.equals("name")) { 3243 this.name = null; 3244 } else if (name.equals("comment")) { 3245 this.comment = null; 3246 } else if (name.equals("extraDetails")) { 3247 this.extraDetails = null; 3248 } else if (name.equals("photo")) { 3249 this.photo = null; 3250 } else if (name.equals("telecom")) { 3251 this.getTelecom().remove(castToContactPoint(value)); 3252 } else if (name.equals("coverageArea")) { 3253 this.getCoverageArea().remove(castToReference(value)); 3254 } else if (name.equals("serviceProvisionCode")) { 3255 this.getServiceProvisionCode().remove(castToCodeableConcept(value)); 3256 } else if (name.equals("eligibility")) { 3257 this.getEligibility().remove((HealthcareServiceEligibilityComponent) value); 3258 } else if (name.equals("program")) { 3259 this.getProgram().remove(castToCodeableConcept(value)); 3260 } else if (name.equals("characteristic")) { 3261 this.getCharacteristic().remove(castToCodeableConcept(value)); 3262 } else if (name.equals("communication")) { 3263 this.getCommunication().remove(castToCodeableConcept(value)); 3264 } else if (name.equals("referralMethod")) { 3265 this.getReferralMethod().remove(castToCodeableConcept(value)); 3266 } else if (name.equals("appointmentRequired")) { 3267 this.appointmentRequired = null; 3268 } else if (name.equals("availableTime")) { 3269 this.getAvailableTime().remove((HealthcareServiceAvailableTimeComponent) value); 3270 } else if (name.equals("notAvailable")) { 3271 this.getNotAvailable().remove((HealthcareServiceNotAvailableComponent) value); 3272 } else if (name.equals("availabilityExceptions")) { 3273 this.availabilityExceptions = null; 3274 } else if (name.equals("endpoint")) { 3275 this.getEndpoint().remove(castToReference(value)); 3276 } else 3277 super.removeChild(name, value); 3278 3279 } 3280 3281 @Override 3282 public Base makeProperty(int hash, String name) throws FHIRException { 3283 switch (hash) { 3284 case -1618432855: 3285 return addIdentifier(); 3286 case -1422950650: 3287 return getActiveElement(); 3288 case 205136282: 3289 return getProvidedBy(); 3290 case 50511102: 3291 return addCategory(); 3292 case 3575610: 3293 return addType(); 3294 case -1694759682: 3295 return addSpecialty(); 3296 case 1901043637: 3297 return addLocation(); 3298 case 3373707: 3299 return getNameElement(); 3300 case 950398559: 3301 return getCommentElement(); 3302 case -1469168622: 3303 return getExtraDetailsElement(); 3304 case 106642994: 3305 return getPhoto(); 3306 case -1429363305: 3307 return addTelecom(); 3308 case -1532328299: 3309 return addCoverageArea(); 3310 case 1504575405: 3311 return addServiceProvisionCode(); 3312 case -930847859: 3313 return addEligibility(); 3314 case -309387644: 3315 return addProgram(); 3316 case 366313883: 3317 return addCharacteristic(); 3318 case -1035284522: 3319 return addCommunication(); 3320 case -2092740898: 3321 return addReferralMethod(); 3322 case 427220062: 3323 return getAppointmentRequiredElement(); 3324 case 1873069366: 3325 return addAvailableTime(); 3326 case -629572298: 3327 return addNotAvailable(); 3328 case -1149143617: 3329 return getAvailabilityExceptionsElement(); 3330 case 1741102485: 3331 return addEndpoint(); 3332 default: 3333 return super.makeProperty(hash, name); 3334 } 3335 3336 } 3337 3338 @Override 3339 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3340 switch (hash) { 3341 case -1618432855: 3342 /* identifier */ return new String[] { "Identifier" }; 3343 case -1422950650: 3344 /* active */ return new String[] { "boolean" }; 3345 case 205136282: 3346 /* providedBy */ return new String[] { "Reference" }; 3347 case 50511102: 3348 /* category */ return new String[] { "CodeableConcept" }; 3349 case 3575610: 3350 /* type */ return new String[] { "CodeableConcept" }; 3351 case -1694759682: 3352 /* specialty */ return new String[] { "CodeableConcept" }; 3353 case 1901043637: 3354 /* location */ return new String[] { "Reference" }; 3355 case 3373707: 3356 /* name */ return new String[] { "string" }; 3357 case 950398559: 3358 /* comment */ return new String[] { "string" }; 3359 case -1469168622: 3360 /* extraDetails */ return new String[] { "markdown" }; 3361 case 106642994: 3362 /* photo */ return new String[] { "Attachment" }; 3363 case -1429363305: 3364 /* telecom */ return new String[] { "ContactPoint" }; 3365 case -1532328299: 3366 /* coverageArea */ return new String[] { "Reference" }; 3367 case 1504575405: 3368 /* serviceProvisionCode */ return new String[] { "CodeableConcept" }; 3369 case -930847859: 3370 /* eligibility */ return new String[] {}; 3371 case -309387644: 3372 /* program */ return new String[] { "CodeableConcept" }; 3373 case 366313883: 3374 /* characteristic */ return new String[] { "CodeableConcept" }; 3375 case -1035284522: 3376 /* communication */ return new String[] { "CodeableConcept" }; 3377 case -2092740898: 3378 /* referralMethod */ return new String[] { "CodeableConcept" }; 3379 case 427220062: 3380 /* appointmentRequired */ return new String[] { "boolean" }; 3381 case 1873069366: 3382 /* availableTime */ return new String[] {}; 3383 case -629572298: 3384 /* notAvailable */ return new String[] {}; 3385 case -1149143617: 3386 /* availabilityExceptions */ return new String[] { "string" }; 3387 case 1741102485: 3388 /* endpoint */ return new String[] { "Reference" }; 3389 default: 3390 return super.getTypesForProperty(hash, name); 3391 } 3392 3393 } 3394 3395 @Override 3396 public Base addChild(String name) throws FHIRException { 3397 if (name.equals("identifier")) { 3398 return addIdentifier(); 3399 } else if (name.equals("active")) { 3400 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.active"); 3401 } else if (name.equals("providedBy")) { 3402 this.providedBy = new Reference(); 3403 return this.providedBy; 3404 } else if (name.equals("category")) { 3405 return addCategory(); 3406 } else if (name.equals("type")) { 3407 return addType(); 3408 } else if (name.equals("specialty")) { 3409 return addSpecialty(); 3410 } else if (name.equals("location")) { 3411 return addLocation(); 3412 } else if (name.equals("name")) { 3413 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.name"); 3414 } else if (name.equals("comment")) { 3415 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 3416 } else if (name.equals("extraDetails")) { 3417 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.extraDetails"); 3418 } else if (name.equals("photo")) { 3419 this.photo = new Attachment(); 3420 return this.photo; 3421 } else if (name.equals("telecom")) { 3422 return addTelecom(); 3423 } else if (name.equals("coverageArea")) { 3424 return addCoverageArea(); 3425 } else if (name.equals("serviceProvisionCode")) { 3426 return addServiceProvisionCode(); 3427 } else if (name.equals("eligibility")) { 3428 return addEligibility(); 3429 } else if (name.equals("program")) { 3430 return addProgram(); 3431 } else if (name.equals("characteristic")) { 3432 return addCharacteristic(); 3433 } else if (name.equals("communication")) { 3434 return addCommunication(); 3435 } else if (name.equals("referralMethod")) { 3436 return addReferralMethod(); 3437 } else if (name.equals("appointmentRequired")) { 3438 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.appointmentRequired"); 3439 } else if (name.equals("availableTime")) { 3440 return addAvailableTime(); 3441 } else if (name.equals("notAvailable")) { 3442 return addNotAvailable(); 3443 } else if (name.equals("availabilityExceptions")) { 3444 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availabilityExceptions"); 3445 } else if (name.equals("endpoint")) { 3446 return addEndpoint(); 3447 } else 3448 return super.addChild(name); 3449 } 3450 3451 public String fhirType() { 3452 return "HealthcareService"; 3453 3454 } 3455 3456 public HealthcareService copy() { 3457 HealthcareService dst = new HealthcareService(); 3458 copyValues(dst); 3459 return dst; 3460 } 3461 3462 public void copyValues(HealthcareService dst) { 3463 super.copyValues(dst); 3464 if (identifier != null) { 3465 dst.identifier = new ArrayList<Identifier>(); 3466 for (Identifier i : identifier) 3467 dst.identifier.add(i.copy()); 3468 } 3469 ; 3470 dst.active = active == null ? null : active.copy(); 3471 dst.providedBy = providedBy == null ? null : providedBy.copy(); 3472 if (category != null) { 3473 dst.category = new ArrayList<CodeableConcept>(); 3474 for (CodeableConcept i : category) 3475 dst.category.add(i.copy()); 3476 } 3477 ; 3478 if (type != null) { 3479 dst.type = new ArrayList<CodeableConcept>(); 3480 for (CodeableConcept i : type) 3481 dst.type.add(i.copy()); 3482 } 3483 ; 3484 if (specialty != null) { 3485 dst.specialty = new ArrayList<CodeableConcept>(); 3486 for (CodeableConcept i : specialty) 3487 dst.specialty.add(i.copy()); 3488 } 3489 ; 3490 if (location != null) { 3491 dst.location = new ArrayList<Reference>(); 3492 for (Reference i : location) 3493 dst.location.add(i.copy()); 3494 } 3495 ; 3496 dst.name = name == null ? null : name.copy(); 3497 dst.comment = comment == null ? null : comment.copy(); 3498 dst.extraDetails = extraDetails == null ? null : extraDetails.copy(); 3499 dst.photo = photo == null ? null : photo.copy(); 3500 if (telecom != null) { 3501 dst.telecom = new ArrayList<ContactPoint>(); 3502 for (ContactPoint i : telecom) 3503 dst.telecom.add(i.copy()); 3504 } 3505 ; 3506 if (coverageArea != null) { 3507 dst.coverageArea = new ArrayList<Reference>(); 3508 for (Reference i : coverageArea) 3509 dst.coverageArea.add(i.copy()); 3510 } 3511 ; 3512 if (serviceProvisionCode != null) { 3513 dst.serviceProvisionCode = new ArrayList<CodeableConcept>(); 3514 for (CodeableConcept i : serviceProvisionCode) 3515 dst.serviceProvisionCode.add(i.copy()); 3516 } 3517 ; 3518 if (eligibility != null) { 3519 dst.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 3520 for (HealthcareServiceEligibilityComponent i : eligibility) 3521 dst.eligibility.add(i.copy()); 3522 } 3523 ; 3524 if (program != null) { 3525 dst.program = new ArrayList<CodeableConcept>(); 3526 for (CodeableConcept i : program) 3527 dst.program.add(i.copy()); 3528 } 3529 ; 3530 if (characteristic != null) { 3531 dst.characteristic = new ArrayList<CodeableConcept>(); 3532 for (CodeableConcept i : characteristic) 3533 dst.characteristic.add(i.copy()); 3534 } 3535 ; 3536 if (communication != null) { 3537 dst.communication = new ArrayList<CodeableConcept>(); 3538 for (CodeableConcept i : communication) 3539 dst.communication.add(i.copy()); 3540 } 3541 ; 3542 if (referralMethod != null) { 3543 dst.referralMethod = new ArrayList<CodeableConcept>(); 3544 for (CodeableConcept i : referralMethod) 3545 dst.referralMethod.add(i.copy()); 3546 } 3547 ; 3548 dst.appointmentRequired = appointmentRequired == null ? null : appointmentRequired.copy(); 3549 if (availableTime != null) { 3550 dst.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 3551 for (HealthcareServiceAvailableTimeComponent i : availableTime) 3552 dst.availableTime.add(i.copy()); 3553 } 3554 ; 3555 if (notAvailable != null) { 3556 dst.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 3557 for (HealthcareServiceNotAvailableComponent i : notAvailable) 3558 dst.notAvailable.add(i.copy()); 3559 } 3560 ; 3561 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 3562 if (endpoint != null) { 3563 dst.endpoint = new ArrayList<Reference>(); 3564 for (Reference i : endpoint) 3565 dst.endpoint.add(i.copy()); 3566 } 3567 ; 3568 } 3569 3570 protected HealthcareService typedCopy() { 3571 return copy(); 3572 } 3573 3574 @Override 3575 public boolean equalsDeep(Base other_) { 3576 if (!super.equalsDeep(other_)) 3577 return false; 3578 if (!(other_ instanceof HealthcareService)) 3579 return false; 3580 HealthcareService o = (HealthcareService) other_; 3581 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 3582 && compareDeep(providedBy, o.providedBy, true) && compareDeep(category, o.category, true) 3583 && compareDeep(type, o.type, true) && compareDeep(specialty, o.specialty, true) 3584 && compareDeep(location, o.location, true) && compareDeep(name, o.name, true) 3585 && compareDeep(comment, o.comment, true) && compareDeep(extraDetails, o.extraDetails, true) 3586 && compareDeep(photo, o.photo, true) && compareDeep(telecom, o.telecom, true) 3587 && compareDeep(coverageArea, o.coverageArea, true) 3588 && compareDeep(serviceProvisionCode, o.serviceProvisionCode, true) 3589 && compareDeep(eligibility, o.eligibility, true) && compareDeep(program, o.program, true) 3590 && compareDeep(characteristic, o.characteristic, true) && compareDeep(communication, o.communication, true) 3591 && compareDeep(referralMethod, o.referralMethod, true) 3592 && compareDeep(appointmentRequired, o.appointmentRequired, true) 3593 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 3594 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 3595 && compareDeep(endpoint, o.endpoint, true); 3596 } 3597 3598 @Override 3599 public boolean equalsShallow(Base other_) { 3600 if (!super.equalsShallow(other_)) 3601 return false; 3602 if (!(other_ instanceof HealthcareService)) 3603 return false; 3604 HealthcareService o = (HealthcareService) other_; 3605 return compareValues(active, o.active, true) && compareValues(name, o.name, true) 3606 && compareValues(comment, o.comment, true) && compareValues(extraDetails, o.extraDetails, true) 3607 && compareValues(appointmentRequired, o.appointmentRequired, true) 3608 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 3609 } 3610 3611 public boolean isEmpty() { 3612 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, providedBy, category, type, 3613 specialty, location, name, comment, extraDetails, photo, telecom, coverageArea, serviceProvisionCode, 3614 eligibility, program, characteristic, communication, referralMethod, appointmentRequired, availableTime, 3615 notAvailable, availabilityExceptions, endpoint); 3616 } 3617 3618 @Override 3619 public ResourceType getResourceType() { 3620 return ResourceType.HealthcareService; 3621 } 3622 3623 /** 3624 * Search parameter: <b>identifier</b> 3625 * <p> 3626 * Description: <b>External identifiers for this item</b><br> 3627 * Type: <b>token</b><br> 3628 * Path: <b>HealthcareService.identifier</b><br> 3629 * </p> 3630 */ 3631 @SearchParamDefinition(name = "identifier", path = "HealthcareService.identifier", description = "External identifiers for this item", type = "token") 3632 public static final String SP_IDENTIFIER = "identifier"; 3633 /** 3634 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3635 * <p> 3636 * Description: <b>External identifiers for this item</b><br> 3637 * Type: <b>token</b><br> 3638 * Path: <b>HealthcareService.identifier</b><br> 3639 * </p> 3640 */ 3641 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3642 SP_IDENTIFIER); 3643 3644 /** 3645 * Search parameter: <b>specialty</b> 3646 * <p> 3647 * Description: <b>The specialty of the service provided by this healthcare 3648 * service</b><br> 3649 * Type: <b>token</b><br> 3650 * Path: <b>HealthcareService.specialty</b><br> 3651 * </p> 3652 */ 3653 @SearchParamDefinition(name = "specialty", path = "HealthcareService.specialty", description = "The specialty of the service provided by this healthcare service", type = "token") 3654 public static final String SP_SPECIALTY = "specialty"; 3655 /** 3656 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 3657 * <p> 3658 * Description: <b>The specialty of the service provided by this healthcare 3659 * service</b><br> 3660 * Type: <b>token</b><br> 3661 * Path: <b>HealthcareService.specialty</b><br> 3662 * </p> 3663 */ 3664 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3665 SP_SPECIALTY); 3666 3667 /** 3668 * Search parameter: <b>endpoint</b> 3669 * <p> 3670 * Description: <b>Technical endpoints providing access to electronic services 3671 * operated for the healthcare service</b><br> 3672 * Type: <b>reference</b><br> 3673 * Path: <b>HealthcareService.endpoint</b><br> 3674 * </p> 3675 */ 3676 @SearchParamDefinition(name = "endpoint", path = "HealthcareService.endpoint", description = "Technical endpoints providing access to electronic services operated for the healthcare service", type = "reference", target = { 3677 Endpoint.class }) 3678 public static final String SP_ENDPOINT = "endpoint"; 3679 /** 3680 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3681 * <p> 3682 * Description: <b>Technical endpoints providing access to electronic services 3683 * operated for the healthcare service</b><br> 3684 * Type: <b>reference</b><br> 3685 * Path: <b>HealthcareService.endpoint</b><br> 3686 * </p> 3687 */ 3688 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3689 SP_ENDPOINT); 3690 3691 /** 3692 * Constant for fluent queries to be used to add include statements. Specifies 3693 * the path value of "<b>HealthcareService:endpoint</b>". 3694 */ 3695 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 3696 "HealthcareService:endpoint").toLocked(); 3697 3698 /** 3699 * Search parameter: <b>service-category</b> 3700 * <p> 3701 * Description: <b>Service Category of the Healthcare Service</b><br> 3702 * Type: <b>token</b><br> 3703 * Path: <b>HealthcareService.category</b><br> 3704 * </p> 3705 */ 3706 @SearchParamDefinition(name = "service-category", path = "HealthcareService.category", description = "Service Category of the Healthcare Service", type = "token") 3707 public static final String SP_SERVICE_CATEGORY = "service-category"; 3708 /** 3709 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 3710 * <p> 3711 * Description: <b>Service Category of the Healthcare Service</b><br> 3712 * Type: <b>token</b><br> 3713 * Path: <b>HealthcareService.category</b><br> 3714 * </p> 3715 */ 3716 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3717 SP_SERVICE_CATEGORY); 3718 3719 /** 3720 * Search parameter: <b>coverage-area</b> 3721 * <p> 3722 * Description: <b>Location(s) service is intended for/available to</b><br> 3723 * Type: <b>reference</b><br> 3724 * Path: <b>HealthcareService.coverageArea</b><br> 3725 * </p> 3726 */ 3727 @SearchParamDefinition(name = "coverage-area", path = "HealthcareService.coverageArea", description = "Location(s) service is intended for/available to", type = "reference", target = { 3728 Location.class }) 3729 public static final String SP_COVERAGE_AREA = "coverage-area"; 3730 /** 3731 * <b>Fluent Client</b> search parameter constant for <b>coverage-area</b> 3732 * <p> 3733 * Description: <b>Location(s) service is intended for/available to</b><br> 3734 * Type: <b>reference</b><br> 3735 * Path: <b>HealthcareService.coverageArea</b><br> 3736 * </p> 3737 */ 3738 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE_AREA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3739 SP_COVERAGE_AREA); 3740 3741 /** 3742 * Constant for fluent queries to be used to add include statements. Specifies 3743 * the path value of "<b>HealthcareService:coverage-area</b>". 3744 */ 3745 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE_AREA = new ca.uhn.fhir.model.api.Include( 3746 "HealthcareService:coverage-area").toLocked(); 3747 3748 /** 3749 * Search parameter: <b>service-type</b> 3750 * <p> 3751 * Description: <b>The type of service provided by this healthcare 3752 * service</b><br> 3753 * Type: <b>token</b><br> 3754 * Path: <b>HealthcareService.type</b><br> 3755 * </p> 3756 */ 3757 @SearchParamDefinition(name = "service-type", path = "HealthcareService.type", description = "The type of service provided by this healthcare service", type = "token") 3758 public static final String SP_SERVICE_TYPE = "service-type"; 3759 /** 3760 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 3761 * <p> 3762 * Description: <b>The type of service provided by this healthcare 3763 * service</b><br> 3764 * Type: <b>token</b><br> 3765 * Path: <b>HealthcareService.type</b><br> 3766 * </p> 3767 */ 3768 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3769 SP_SERVICE_TYPE); 3770 3771 /** 3772 * Search parameter: <b>organization</b> 3773 * <p> 3774 * Description: <b>The organization that provides this Healthcare 3775 * Service</b><br> 3776 * Type: <b>reference</b><br> 3777 * Path: <b>HealthcareService.providedBy</b><br> 3778 * </p> 3779 */ 3780 @SearchParamDefinition(name = "organization", path = "HealthcareService.providedBy", description = "The organization that provides this Healthcare Service", type = "reference", target = { 3781 Organization.class }) 3782 public static final String SP_ORGANIZATION = "organization"; 3783 /** 3784 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3785 * <p> 3786 * Description: <b>The organization that provides this Healthcare 3787 * Service</b><br> 3788 * Type: <b>reference</b><br> 3789 * Path: <b>HealthcareService.providedBy</b><br> 3790 * </p> 3791 */ 3792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3793 SP_ORGANIZATION); 3794 3795 /** 3796 * Constant for fluent queries to be used to add include statements. Specifies 3797 * the path value of "<b>HealthcareService:organization</b>". 3798 */ 3799 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3800 "HealthcareService:organization").toLocked(); 3801 3802 /** 3803 * Search parameter: <b>name</b> 3804 * <p> 3805 * Description: <b>A portion of the Healthcare service name</b><br> 3806 * Type: <b>string</b><br> 3807 * Path: <b>HealthcareService.name</b><br> 3808 * </p> 3809 */ 3810 @SearchParamDefinition(name = "name", path = "HealthcareService.name", description = "A portion of the Healthcare service name", type = "string") 3811 public static final String SP_NAME = "name"; 3812 /** 3813 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3814 * <p> 3815 * Description: <b>A portion of the Healthcare service name</b><br> 3816 * Type: <b>string</b><br> 3817 * Path: <b>HealthcareService.name</b><br> 3818 * </p> 3819 */ 3820 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3821 SP_NAME); 3822 3823 /** 3824 * Search parameter: <b>active</b> 3825 * <p> 3826 * Description: <b>The Healthcare Service is currently marked as active</b><br> 3827 * Type: <b>token</b><br> 3828 * Path: <b>HealthcareService.active</b><br> 3829 * </p> 3830 */ 3831 @SearchParamDefinition(name = "active", path = "HealthcareService.active", description = "The Healthcare Service is currently marked as active", type = "token") 3832 public static final String SP_ACTIVE = "active"; 3833 /** 3834 * <b>Fluent Client</b> search parameter constant for <b>active</b> 3835 * <p> 3836 * Description: <b>The Healthcare Service is currently marked as active</b><br> 3837 * Type: <b>token</b><br> 3838 * Path: <b>HealthcareService.active</b><br> 3839 * </p> 3840 */ 3841 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3842 SP_ACTIVE); 3843 3844 /** 3845 * Search parameter: <b>location</b> 3846 * <p> 3847 * Description: <b>The location of the Healthcare Service</b><br> 3848 * Type: <b>reference</b><br> 3849 * Path: <b>HealthcareService.location</b><br> 3850 * </p> 3851 */ 3852 @SearchParamDefinition(name = "location", path = "HealthcareService.location", description = "The location of the Healthcare Service", type = "reference", target = { 3853 Location.class }) 3854 public static final String SP_LOCATION = "location"; 3855 /** 3856 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3857 * <p> 3858 * Description: <b>The location of the Healthcare Service</b><br> 3859 * Type: <b>reference</b><br> 3860 * Path: <b>HealthcareService.location</b><br> 3861 * </p> 3862 */ 3863 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3864 SP_LOCATION); 3865 3866 /** 3867 * Constant for fluent queries to be used to add include statements. Specifies 3868 * the path value of "<b>HealthcareService:location</b>". 3869 */ 3870 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 3871 "HealthcareService:location").toLocked(); 3872 3873 /** 3874 * Search parameter: <b>program</b> 3875 * <p> 3876 * Description: <b>One of the Programs supported by this 3877 * HealthcareService</b><br> 3878 * Type: <b>token</b><br> 3879 * Path: <b>HealthcareService.program</b><br> 3880 * </p> 3881 */ 3882 @SearchParamDefinition(name = "program", path = "HealthcareService.program", description = "One of the Programs supported by this HealthcareService", type = "token") 3883 public static final String SP_PROGRAM = "program"; 3884 /** 3885 * <b>Fluent Client</b> search parameter constant for <b>program</b> 3886 * <p> 3887 * Description: <b>One of the Programs supported by this 3888 * HealthcareService</b><br> 3889 * Type: <b>token</b><br> 3890 * Path: <b>HealthcareService.program</b><br> 3891 * </p> 3892 */ 3893 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PROGRAM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3894 SP_PROGRAM); 3895 3896 /** 3897 * Search parameter: <b>characteristic</b> 3898 * <p> 3899 * Description: <b>One of the HealthcareService's characteristics</b><br> 3900 * Type: <b>token</b><br> 3901 * Path: <b>HealthcareService.characteristic</b><br> 3902 * </p> 3903 */ 3904 @SearchParamDefinition(name = "characteristic", path = "HealthcareService.characteristic", description = "One of the HealthcareService's characteristics", type = "token") 3905 public static final String SP_CHARACTERISTIC = "characteristic"; 3906 /** 3907 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 3908 * <p> 3909 * Description: <b>One of the HealthcareService's characteristics</b><br> 3910 * Type: <b>token</b><br> 3911 * Path: <b>HealthcareService.characteristic</b><br> 3912 * </p> 3913 */ 3914 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3915 SP_CHARACTERISTIC); 3916 3917}