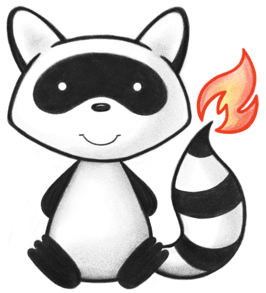
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Describes the event of a patient being administered a vaccine or a record of 049 * an immunization as reported by a patient, a clinician or another party. 050 */ 051@ResourceDef(name = "Immunization", profile = "http://hl7.org/fhir/StructureDefinition/Immunization") 052public class Immunization extends DomainResource { 053 054 public enum ImmunizationStatus { 055 /** 056 * null 057 */ 058 COMPLETED, 059 /** 060 * null 061 */ 062 ENTEREDINERROR, 063 /** 064 * null 065 */ 066 NOTDONE, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static ImmunizationStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("completed".equals(codeString)) 076 return COMPLETED; 077 if ("entered-in-error".equals(codeString)) 078 return ENTEREDINERROR; 079 if ("not-done".equals(codeString)) 080 return NOTDONE; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown ImmunizationStatus code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case COMPLETED: 090 return "completed"; 091 case ENTEREDINERROR: 092 return "entered-in-error"; 093 case NOTDONE: 094 return "not-done"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case COMPLETED: 105 return "http://hl7.org/fhir/event-status"; 106 case ENTEREDINERROR: 107 return "http://hl7.org/fhir/event-status"; 108 case NOTDONE: 109 return "http://hl7.org/fhir/event-status"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case COMPLETED: 120 return ""; 121 case ENTEREDINERROR: 122 return ""; 123 case NOTDONE: 124 return ""; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case COMPLETED: 135 return "completed"; 136 case ENTEREDINERROR: 137 return "entered-in-error"; 138 case NOTDONE: 139 return "not-done"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class ImmunizationStatusEnumFactory implements EnumFactory<ImmunizationStatus> { 149 public ImmunizationStatus fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("completed".equals(codeString)) 154 return ImmunizationStatus.COMPLETED; 155 if ("entered-in-error".equals(codeString)) 156 return ImmunizationStatus.ENTEREDINERROR; 157 if ("not-done".equals(codeString)) 158 return ImmunizationStatus.NOTDONE; 159 throw new IllegalArgumentException("Unknown ImmunizationStatus code '" + codeString + "'"); 160 } 161 162 public Enumeration<ImmunizationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NULL, code); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NULL, code); 170 if ("completed".equals(codeString)) 171 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.COMPLETED, code); 172 if ("entered-in-error".equals(codeString)) 173 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.ENTEREDINERROR, code); 174 if ("not-done".equals(codeString)) 175 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.NOTDONE, code); 176 throw new FHIRException("Unknown ImmunizationStatus code '" + codeString + "'"); 177 } 178 179 public String toCode(ImmunizationStatus code) { 180 if (code == ImmunizationStatus.COMPLETED) 181 return "completed"; 182 if (code == ImmunizationStatus.ENTEREDINERROR) 183 return "entered-in-error"; 184 if (code == ImmunizationStatus.NOTDONE) 185 return "not-done"; 186 return "?"; 187 } 188 189 public String toSystem(ImmunizationStatus code) { 190 return code.getSystem(); 191 } 192 } 193 194 @Block() 195 public static class ImmunizationPerformerComponent extends BackboneElement implements IBaseBackboneElement { 196 /** 197 * Describes the type of performance (e.g. ordering provider, administering 198 * provider, etc.). 199 */ 200 @Child(name = "function", type = { 201 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 202 @Description(shortDefinition = "What type of performance was done", formalDefinition = "Describes the type of performance (e.g. ordering provider, administering provider, etc.).") 203 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-function") 204 protected CodeableConcept function; 205 206 /** 207 * The practitioner or organization who performed the action. 208 */ 209 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, 210 Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 211 @Description(shortDefinition = "Individual or organization who was performing", formalDefinition = "The practitioner or organization who performed the action.") 212 protected Reference actor; 213 214 /** 215 * The actual object that is the target of the reference (The practitioner or 216 * organization who performed the action.) 217 */ 218 protected Resource actorTarget; 219 220 private static final long serialVersionUID = 1424001049L; 221 222 /** 223 * Constructor 224 */ 225 public ImmunizationPerformerComponent() { 226 super(); 227 } 228 229 /** 230 * Constructor 231 */ 232 public ImmunizationPerformerComponent(Reference actor) { 233 super(); 234 this.actor = actor; 235 } 236 237 /** 238 * @return {@link #function} (Describes the type of performance (e.g. ordering 239 * provider, administering provider, etc.).) 240 */ 241 public CodeableConcept getFunction() { 242 if (this.function == null) 243 if (Configuration.errorOnAutoCreate()) 244 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.function"); 245 else if (Configuration.doAutoCreate()) 246 this.function = new CodeableConcept(); // cc 247 return this.function; 248 } 249 250 public boolean hasFunction() { 251 return this.function != null && !this.function.isEmpty(); 252 } 253 254 /** 255 * @param value {@link #function} (Describes the type of performance (e.g. 256 * ordering provider, administering provider, etc.).) 257 */ 258 public ImmunizationPerformerComponent setFunction(CodeableConcept value) { 259 this.function = value; 260 return this; 261 } 262 263 /** 264 * @return {@link #actor} (The practitioner or organization who performed the 265 * action.) 266 */ 267 public Reference getActor() { 268 if (this.actor == null) 269 if (Configuration.errorOnAutoCreate()) 270 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.actor"); 271 else if (Configuration.doAutoCreate()) 272 this.actor = new Reference(); // cc 273 return this.actor; 274 } 275 276 public boolean hasActor() { 277 return this.actor != null && !this.actor.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #actor} (The practitioner or organization who performed 282 * the action.) 283 */ 284 public ImmunizationPerformerComponent setActor(Reference value) { 285 this.actor = value; 286 return this; 287 } 288 289 /** 290 * @return {@link #actor} The actual object that is the target of the reference. 291 * The reference library doesn't populate this, but you can use it to 292 * hold the resource if you resolve it. (The practitioner or 293 * organization who performed the action.) 294 */ 295 public Resource getActorTarget() { 296 return this.actorTarget; 297 } 298 299 /** 300 * @param value {@link #actor} The actual object that is the target of the 301 * reference. The reference library doesn't use these, but you can 302 * use it to hold the resource if you resolve it. (The practitioner 303 * or organization who performed the action.) 304 */ 305 public ImmunizationPerformerComponent setActorTarget(Resource value) { 306 this.actorTarget = value; 307 return this; 308 } 309 310 protected void listChildren(List<Property> children) { 311 super.listChildren(children); 312 children.add(new Property("function", "CodeableConcept", 313 "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, function)); 314 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization)", 315 "The practitioner or organization who performed the action.", 0, 1, actor)); 316 } 317 318 @Override 319 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 320 switch (_hash) { 321 case 1380938712: 322 /* function */ return new Property("function", "CodeableConcept", 323 "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, 324 function); 325 case 92645877: 326 /* actor */ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization)", 327 "The practitioner or organization who performed the action.", 0, 1, actor); 328 default: 329 return super.getNamedProperty(_hash, _name, _checkValid); 330 } 331 332 } 333 334 @Override 335 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 336 switch (hash) { 337 case 1380938712: 338 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 339 case 92645877: 340 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 341 default: 342 return super.getProperty(hash, name, checkValid); 343 } 344 345 } 346 347 @Override 348 public Base setProperty(int hash, String name, Base value) throws FHIRException { 349 switch (hash) { 350 case 1380938712: // function 351 this.function = castToCodeableConcept(value); // CodeableConcept 352 return value; 353 case 92645877: // actor 354 this.actor = castToReference(value); // Reference 355 return value; 356 default: 357 return super.setProperty(hash, name, value); 358 } 359 360 } 361 362 @Override 363 public Base setProperty(String name, Base value) throws FHIRException { 364 if (name.equals("function")) { 365 this.function = castToCodeableConcept(value); // CodeableConcept 366 } else if (name.equals("actor")) { 367 this.actor = castToReference(value); // Reference 368 } else 369 return super.setProperty(name, value); 370 return value; 371 } 372 373 @Override 374 public Base makeProperty(int hash, String name) throws FHIRException { 375 switch (hash) { 376 case 1380938712: 377 return getFunction(); 378 case 92645877: 379 return getActor(); 380 default: 381 return super.makeProperty(hash, name); 382 } 383 384 } 385 386 @Override 387 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 388 switch (hash) { 389 case 1380938712: 390 /* function */ return new String[] { "CodeableConcept" }; 391 case 92645877: 392 /* actor */ return new String[] { "Reference" }; 393 default: 394 return super.getTypesForProperty(hash, name); 395 } 396 397 } 398 399 @Override 400 public Base addChild(String name) throws FHIRException { 401 if (name.equals("function")) { 402 this.function = new CodeableConcept(); 403 return this.function; 404 } else if (name.equals("actor")) { 405 this.actor = new Reference(); 406 return this.actor; 407 } else 408 return super.addChild(name); 409 } 410 411 public ImmunizationPerformerComponent copy() { 412 ImmunizationPerformerComponent dst = new ImmunizationPerformerComponent(); 413 copyValues(dst); 414 return dst; 415 } 416 417 public void copyValues(ImmunizationPerformerComponent dst) { 418 super.copyValues(dst); 419 dst.function = function == null ? null : function.copy(); 420 dst.actor = actor == null ? null : actor.copy(); 421 } 422 423 @Override 424 public boolean equalsDeep(Base other_) { 425 if (!super.equalsDeep(other_)) 426 return false; 427 if (!(other_ instanceof ImmunizationPerformerComponent)) 428 return false; 429 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 430 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 431 } 432 433 @Override 434 public boolean equalsShallow(Base other_) { 435 if (!super.equalsShallow(other_)) 436 return false; 437 if (!(other_ instanceof ImmunizationPerformerComponent)) 438 return false; 439 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 440 return true; 441 } 442 443 public boolean isEmpty() { 444 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 445 } 446 447 public String fhirType() { 448 return "Immunization.performer"; 449 450 } 451 452 } 453 454 @Block() 455 public static class ImmunizationEducationComponent extends BackboneElement implements IBaseBackboneElement { 456 /** 457 * Identifier of the material presented to the patient. 458 */ 459 @Child(name = "documentType", type = { 460 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 461 @Description(shortDefinition = "Educational material document identifier", formalDefinition = "Identifier of the material presented to the patient.") 462 protected StringType documentType; 463 464 /** 465 * Reference pointer to the educational material given to the patient if the 466 * information was on line. 467 */ 468 @Child(name = "reference", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 469 @Description(shortDefinition = "Educational material reference pointer", formalDefinition = "Reference pointer to the educational material given to the patient if the information was on line.") 470 protected UriType reference; 471 472 /** 473 * Date the educational material was published. 474 */ 475 @Child(name = "publicationDate", type = { 476 DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 477 @Description(shortDefinition = "Educational material publication date", formalDefinition = "Date the educational material was published.") 478 protected DateTimeType publicationDate; 479 480 /** 481 * Date the educational material was given to the patient. 482 */ 483 @Child(name = "presentationDate", type = { 484 DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 485 @Description(shortDefinition = "Educational material presentation date", formalDefinition = "Date the educational material was given to the patient.") 486 protected DateTimeType presentationDate; 487 488 private static final long serialVersionUID = -1277654827L; 489 490 /** 491 * Constructor 492 */ 493 public ImmunizationEducationComponent() { 494 super(); 495 } 496 497 /** 498 * @return {@link #documentType} (Identifier of the material presented to the 499 * patient.). This is the underlying object with id, value and 500 * extensions. The accessor "getDocumentType" gives direct access to the 501 * value 502 */ 503 public StringType getDocumentTypeElement() { 504 if (this.documentType == null) 505 if (Configuration.errorOnAutoCreate()) 506 throw new Error("Attempt to auto-create ImmunizationEducationComponent.documentType"); 507 else if (Configuration.doAutoCreate()) 508 this.documentType = new StringType(); // bb 509 return this.documentType; 510 } 511 512 public boolean hasDocumentTypeElement() { 513 return this.documentType != null && !this.documentType.isEmpty(); 514 } 515 516 public boolean hasDocumentType() { 517 return this.documentType != null && !this.documentType.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #documentType} (Identifier of the material presented to 522 * the patient.). This is the underlying object with id, value and 523 * extensions. The accessor "getDocumentType" gives direct access 524 * to the value 525 */ 526 public ImmunizationEducationComponent setDocumentTypeElement(StringType value) { 527 this.documentType = value; 528 return this; 529 } 530 531 /** 532 * @return Identifier of the material presented to the patient. 533 */ 534 public String getDocumentType() { 535 return this.documentType == null ? null : this.documentType.getValue(); 536 } 537 538 /** 539 * @param value Identifier of the material presented to the patient. 540 */ 541 public ImmunizationEducationComponent setDocumentType(String value) { 542 if (Utilities.noString(value)) 543 this.documentType = null; 544 else { 545 if (this.documentType == null) 546 this.documentType = new StringType(); 547 this.documentType.setValue(value); 548 } 549 return this; 550 } 551 552 /** 553 * @return {@link #reference} (Reference pointer to the educational material 554 * given to the patient if the information was on line.). This is the 555 * underlying object with id, value and extensions. The accessor 556 * "getReference" gives direct access to the value 557 */ 558 public UriType getReferenceElement() { 559 if (this.reference == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create ImmunizationEducationComponent.reference"); 562 else if (Configuration.doAutoCreate()) 563 this.reference = new UriType(); // bb 564 return this.reference; 565 } 566 567 public boolean hasReferenceElement() { 568 return this.reference != null && !this.reference.isEmpty(); 569 } 570 571 public boolean hasReference() { 572 return this.reference != null && !this.reference.isEmpty(); 573 } 574 575 /** 576 * @param value {@link #reference} (Reference pointer to the educational 577 * material given to the patient if the information was on line.). 578 * This is the underlying object with id, value and extensions. The 579 * accessor "getReference" gives direct access to the value 580 */ 581 public ImmunizationEducationComponent setReferenceElement(UriType value) { 582 this.reference = value; 583 return this; 584 } 585 586 /** 587 * @return Reference pointer to the educational material given to the patient if 588 * the information was on line. 589 */ 590 public String getReference() { 591 return this.reference == null ? null : this.reference.getValue(); 592 } 593 594 /** 595 * @param value Reference pointer to the educational material given to the 596 * patient if the information was on line. 597 */ 598 public ImmunizationEducationComponent setReference(String value) { 599 if (Utilities.noString(value)) 600 this.reference = null; 601 else { 602 if (this.reference == null) 603 this.reference = new UriType(); 604 this.reference.setValue(value); 605 } 606 return this; 607 } 608 609 /** 610 * @return {@link #publicationDate} (Date the educational material was 611 * published.). This is the underlying object with id, value and 612 * extensions. The accessor "getPublicationDate" gives direct access to 613 * the value 614 */ 615 public DateTimeType getPublicationDateElement() { 616 if (this.publicationDate == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create ImmunizationEducationComponent.publicationDate"); 619 else if (Configuration.doAutoCreate()) 620 this.publicationDate = new DateTimeType(); // bb 621 return this.publicationDate; 622 } 623 624 public boolean hasPublicationDateElement() { 625 return this.publicationDate != null && !this.publicationDate.isEmpty(); 626 } 627 628 public boolean hasPublicationDate() { 629 return this.publicationDate != null && !this.publicationDate.isEmpty(); 630 } 631 632 /** 633 * @param value {@link #publicationDate} (Date the educational material was 634 * published.). This is the underlying object with id, value and 635 * extensions. The accessor "getPublicationDate" gives direct 636 * access to the value 637 */ 638 public ImmunizationEducationComponent setPublicationDateElement(DateTimeType value) { 639 this.publicationDate = value; 640 return this; 641 } 642 643 /** 644 * @return Date the educational material was published. 645 */ 646 public Date getPublicationDate() { 647 return this.publicationDate == null ? null : this.publicationDate.getValue(); 648 } 649 650 /** 651 * @param value Date the educational material was published. 652 */ 653 public ImmunizationEducationComponent setPublicationDate(Date value) { 654 if (value == null) 655 this.publicationDate = null; 656 else { 657 if (this.publicationDate == null) 658 this.publicationDate = new DateTimeType(); 659 this.publicationDate.setValue(value); 660 } 661 return this; 662 } 663 664 /** 665 * @return {@link #presentationDate} (Date the educational material was given to 666 * the patient.). This is the underlying object with id, value and 667 * extensions. The accessor "getPresentationDate" gives direct access to 668 * the value 669 */ 670 public DateTimeType getPresentationDateElement() { 671 if (this.presentationDate == null) 672 if (Configuration.errorOnAutoCreate()) 673 throw new Error("Attempt to auto-create ImmunizationEducationComponent.presentationDate"); 674 else if (Configuration.doAutoCreate()) 675 this.presentationDate = new DateTimeType(); // bb 676 return this.presentationDate; 677 } 678 679 public boolean hasPresentationDateElement() { 680 return this.presentationDate != null && !this.presentationDate.isEmpty(); 681 } 682 683 public boolean hasPresentationDate() { 684 return this.presentationDate != null && !this.presentationDate.isEmpty(); 685 } 686 687 /** 688 * @param value {@link #presentationDate} (Date the educational material was 689 * given to the patient.). This is the underlying object with id, 690 * value and extensions. The accessor "getPresentationDate" gives 691 * direct access to the value 692 */ 693 public ImmunizationEducationComponent setPresentationDateElement(DateTimeType value) { 694 this.presentationDate = value; 695 return this; 696 } 697 698 /** 699 * @return Date the educational material was given to the patient. 700 */ 701 public Date getPresentationDate() { 702 return this.presentationDate == null ? null : this.presentationDate.getValue(); 703 } 704 705 /** 706 * @param value Date the educational material was given to the patient. 707 */ 708 public ImmunizationEducationComponent setPresentationDate(Date value) { 709 if (value == null) 710 this.presentationDate = null; 711 else { 712 if (this.presentationDate == null) 713 this.presentationDate = new DateTimeType(); 714 this.presentationDate.setValue(value); 715 } 716 return this; 717 } 718 719 protected void listChildren(List<Property> children) { 720 super.listChildren(children); 721 children.add(new Property("documentType", "string", "Identifier of the material presented to the patient.", 0, 1, 722 documentType)); 723 children.add(new Property("reference", "uri", 724 "Reference pointer to the educational material given to the patient if the information was on line.", 0, 1, 725 reference)); 726 children.add(new Property("publicationDate", "dateTime", "Date the educational material was published.", 0, 1, 727 publicationDate)); 728 children.add(new Property("presentationDate", "dateTime", 729 "Date the educational material was given to the patient.", 0, 1, presentationDate)); 730 } 731 732 @Override 733 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 734 switch (_hash) { 735 case -1473196299: 736 /* documentType */ return new Property("documentType", "string", 737 "Identifier of the material presented to the patient.", 0, 1, documentType); 738 case -925155509: 739 /* reference */ return new Property("reference", "uri", 740 "Reference pointer to the educational material given to the patient if the information was on line.", 0, 1, 741 reference); 742 case 1470566394: 743 /* publicationDate */ return new Property("publicationDate", "dateTime", 744 "Date the educational material was published.", 0, 1, publicationDate); 745 case 1602373096: 746 /* presentationDate */ return new Property("presentationDate", "dateTime", 747 "Date the educational material was given to the patient.", 0, 1, presentationDate); 748 default: 749 return super.getNamedProperty(_hash, _name, _checkValid); 750 } 751 752 } 753 754 @Override 755 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 756 switch (hash) { 757 case -1473196299: 758 /* documentType */ return this.documentType == null ? new Base[0] : new Base[] { this.documentType }; // StringType 759 case -925155509: 760 /* reference */ return this.reference == null ? new Base[0] : new Base[] { this.reference }; // UriType 761 case 1470566394: 762 /* publicationDate */ return this.publicationDate == null ? new Base[0] : new Base[] { this.publicationDate }; // DateTimeType 763 case 1602373096: 764 /* presentationDate */ return this.presentationDate == null ? new Base[0] 765 : new Base[] { this.presentationDate }; // DateTimeType 766 default: 767 return super.getProperty(hash, name, checkValid); 768 } 769 770 } 771 772 @Override 773 public Base setProperty(int hash, String name, Base value) throws FHIRException { 774 switch (hash) { 775 case -1473196299: // documentType 776 this.documentType = castToString(value); // StringType 777 return value; 778 case -925155509: // reference 779 this.reference = castToUri(value); // UriType 780 return value; 781 case 1470566394: // publicationDate 782 this.publicationDate = castToDateTime(value); // DateTimeType 783 return value; 784 case 1602373096: // presentationDate 785 this.presentationDate = castToDateTime(value); // DateTimeType 786 return value; 787 default: 788 return super.setProperty(hash, name, value); 789 } 790 791 } 792 793 @Override 794 public Base setProperty(String name, Base value) throws FHIRException { 795 if (name.equals("documentType")) { 796 this.documentType = castToString(value); // StringType 797 } else if (name.equals("reference")) { 798 this.reference = castToUri(value); // UriType 799 } else if (name.equals("publicationDate")) { 800 this.publicationDate = castToDateTime(value); // DateTimeType 801 } else if (name.equals("presentationDate")) { 802 this.presentationDate = castToDateTime(value); // DateTimeType 803 } else 804 return super.setProperty(name, value); 805 return value; 806 } 807 808 @Override 809 public Base makeProperty(int hash, String name) throws FHIRException { 810 switch (hash) { 811 case -1473196299: 812 return getDocumentTypeElement(); 813 case -925155509: 814 return getReferenceElement(); 815 case 1470566394: 816 return getPublicationDateElement(); 817 case 1602373096: 818 return getPresentationDateElement(); 819 default: 820 return super.makeProperty(hash, name); 821 } 822 823 } 824 825 @Override 826 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case -1473196299: 829 /* documentType */ return new String[] { "string" }; 830 case -925155509: 831 /* reference */ return new String[] { "uri" }; 832 case 1470566394: 833 /* publicationDate */ return new String[] { "dateTime" }; 834 case 1602373096: 835 /* presentationDate */ return new String[] { "dateTime" }; 836 default: 837 return super.getTypesForProperty(hash, name); 838 } 839 840 } 841 842 @Override 843 public Base addChild(String name) throws FHIRException { 844 if (name.equals("documentType")) { 845 throw new FHIRException("Cannot call addChild on a singleton property Immunization.documentType"); 846 } else if (name.equals("reference")) { 847 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reference"); 848 } else if (name.equals("publicationDate")) { 849 throw new FHIRException("Cannot call addChild on a singleton property Immunization.publicationDate"); 850 } else if (name.equals("presentationDate")) { 851 throw new FHIRException("Cannot call addChild on a singleton property Immunization.presentationDate"); 852 } else 853 return super.addChild(name); 854 } 855 856 public ImmunizationEducationComponent copy() { 857 ImmunizationEducationComponent dst = new ImmunizationEducationComponent(); 858 copyValues(dst); 859 return dst; 860 } 861 862 public void copyValues(ImmunizationEducationComponent dst) { 863 super.copyValues(dst); 864 dst.documentType = documentType == null ? null : documentType.copy(); 865 dst.reference = reference == null ? null : reference.copy(); 866 dst.publicationDate = publicationDate == null ? null : publicationDate.copy(); 867 dst.presentationDate = presentationDate == null ? null : presentationDate.copy(); 868 } 869 870 @Override 871 public boolean equalsDeep(Base other_) { 872 if (!super.equalsDeep(other_)) 873 return false; 874 if (!(other_ instanceof ImmunizationEducationComponent)) 875 return false; 876 ImmunizationEducationComponent o = (ImmunizationEducationComponent) other_; 877 return compareDeep(documentType, o.documentType, true) && compareDeep(reference, o.reference, true) 878 && compareDeep(publicationDate, o.publicationDate, true) 879 && compareDeep(presentationDate, o.presentationDate, true); 880 } 881 882 @Override 883 public boolean equalsShallow(Base other_) { 884 if (!super.equalsShallow(other_)) 885 return false; 886 if (!(other_ instanceof ImmunizationEducationComponent)) 887 return false; 888 ImmunizationEducationComponent o = (ImmunizationEducationComponent) other_; 889 return compareValues(documentType, o.documentType, true) && compareValues(reference, o.reference, true) 890 && compareValues(publicationDate, o.publicationDate, true) 891 && compareValues(presentationDate, o.presentationDate, true); 892 } 893 894 public boolean isEmpty() { 895 return super.isEmpty() 896 && ca.uhn.fhir.util.ElementUtil.isEmpty(documentType, reference, publicationDate, presentationDate); 897 } 898 899 public String fhirType() { 900 return "Immunization.education"; 901 902 } 903 904 } 905 906 @Block() 907 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 908 /** 909 * Date of reaction to the immunization. 910 */ 911 @Child(name = "date", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 912 @Description(shortDefinition = "When reaction started", formalDefinition = "Date of reaction to the immunization.") 913 protected DateTimeType date; 914 915 /** 916 * Details of the reaction. 917 */ 918 @Child(name = "detail", type = { 919 Observation.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 920 @Description(shortDefinition = "Additional information on reaction", formalDefinition = "Details of the reaction.") 921 protected Reference detail; 922 923 /** 924 * The actual object that is the target of the reference (Details of the 925 * reaction.) 926 */ 927 protected Observation detailTarget; 928 929 /** 930 * Self-reported indicator. 931 */ 932 @Child(name = "reported", type = { 933 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 934 @Description(shortDefinition = "Indicates self-reported reaction", formalDefinition = "Self-reported indicator.") 935 protected BooleanType reported; 936 937 private static final long serialVersionUID = -1297668556L; 938 939 /** 940 * Constructor 941 */ 942 public ImmunizationReactionComponent() { 943 super(); 944 } 945 946 /** 947 * @return {@link #date} (Date of reaction to the immunization.). This is the 948 * underlying object with id, value and extensions. The accessor 949 * "getDate" gives direct access to the value 950 */ 951 public DateTimeType getDateElement() { 952 if (this.date == null) 953 if (Configuration.errorOnAutoCreate()) 954 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 955 else if (Configuration.doAutoCreate()) 956 this.date = new DateTimeType(); // bb 957 return this.date; 958 } 959 960 public boolean hasDateElement() { 961 return this.date != null && !this.date.isEmpty(); 962 } 963 964 public boolean hasDate() { 965 return this.date != null && !this.date.isEmpty(); 966 } 967 968 /** 969 * @param value {@link #date} (Date of reaction to the immunization.). This is 970 * the underlying object with id, value and extensions. The 971 * accessor "getDate" gives direct access to the value 972 */ 973 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 974 this.date = value; 975 return this; 976 } 977 978 /** 979 * @return Date of reaction to the immunization. 980 */ 981 public Date getDate() { 982 return this.date == null ? null : this.date.getValue(); 983 } 984 985 /** 986 * @param value Date of reaction to the immunization. 987 */ 988 public ImmunizationReactionComponent setDate(Date value) { 989 if (value == null) 990 this.date = null; 991 else { 992 if (this.date == null) 993 this.date = new DateTimeType(); 994 this.date.setValue(value); 995 } 996 return this; 997 } 998 999 /** 1000 * @return {@link #detail} (Details of the reaction.) 1001 */ 1002 public Reference getDetail() { 1003 if (this.detail == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 1006 else if (Configuration.doAutoCreate()) 1007 this.detail = new Reference(); // cc 1008 return this.detail; 1009 } 1010 1011 public boolean hasDetail() { 1012 return this.detail != null && !this.detail.isEmpty(); 1013 } 1014 1015 /** 1016 * @param value {@link #detail} (Details of the reaction.) 1017 */ 1018 public ImmunizationReactionComponent setDetail(Reference value) { 1019 this.detail = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #detail} The actual object that is the target of the 1025 * reference. The reference library doesn't populate this, but you can 1026 * use it to hold the resource if you resolve it. (Details of the 1027 * reaction.) 1028 */ 1029 public Observation getDetailTarget() { 1030 if (this.detailTarget == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 1033 else if (Configuration.doAutoCreate()) 1034 this.detailTarget = new Observation(); // aa 1035 return this.detailTarget; 1036 } 1037 1038 /** 1039 * @param value {@link #detail} The actual object that is the target of the 1040 * reference. The reference library doesn't use these, but you can 1041 * use it to hold the resource if you resolve it. (Details of the 1042 * reaction.) 1043 */ 1044 public ImmunizationReactionComponent setDetailTarget(Observation value) { 1045 this.detailTarget = value; 1046 return this; 1047 } 1048 1049 /** 1050 * @return {@link #reported} (Self-reported indicator.). This is the underlying 1051 * object with id, value and extensions. The accessor "getReported" 1052 * gives direct access to the value 1053 */ 1054 public BooleanType getReportedElement() { 1055 if (this.reported == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 1058 else if (Configuration.doAutoCreate()) 1059 this.reported = new BooleanType(); // bb 1060 return this.reported; 1061 } 1062 1063 public boolean hasReportedElement() { 1064 return this.reported != null && !this.reported.isEmpty(); 1065 } 1066 1067 public boolean hasReported() { 1068 return this.reported != null && !this.reported.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #reported} (Self-reported indicator.). This is the 1073 * underlying object with id, value and extensions. The accessor 1074 * "getReported" gives direct access to the value 1075 */ 1076 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 1077 this.reported = value; 1078 return this; 1079 } 1080 1081 /** 1082 * @return Self-reported indicator. 1083 */ 1084 public boolean getReported() { 1085 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 1086 } 1087 1088 /** 1089 * @param value Self-reported indicator. 1090 */ 1091 public ImmunizationReactionComponent setReported(boolean value) { 1092 if (this.reported == null) 1093 this.reported = new BooleanType(); 1094 this.reported.setValue(value); 1095 return this; 1096 } 1097 1098 protected void listChildren(List<Property> children) { 1099 super.listChildren(children); 1100 children.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date)); 1101 children.add(new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail)); 1102 children.add(new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported)); 1103 } 1104 1105 @Override 1106 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1107 switch (_hash) { 1108 case 3076014: 1109 /* date */ return new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date); 1110 case -1335224239: 1111 /* detail */ return new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail); 1112 case -427039533: 1113 /* reported */ return new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported); 1114 default: 1115 return super.getNamedProperty(_hash, _name, _checkValid); 1116 } 1117 1118 } 1119 1120 @Override 1121 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1122 switch (hash) { 1123 case 3076014: 1124 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1125 case -1335224239: 1126 /* detail */ return this.detail == null ? new Base[0] : new Base[] { this.detail }; // Reference 1127 case -427039533: 1128 /* reported */ return this.reported == null ? new Base[0] : new Base[] { this.reported }; // BooleanType 1129 default: 1130 return super.getProperty(hash, name, checkValid); 1131 } 1132 1133 } 1134 1135 @Override 1136 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1137 switch (hash) { 1138 case 3076014: // date 1139 this.date = castToDateTime(value); // DateTimeType 1140 return value; 1141 case -1335224239: // detail 1142 this.detail = castToReference(value); // Reference 1143 return value; 1144 case -427039533: // reported 1145 this.reported = castToBoolean(value); // BooleanType 1146 return value; 1147 default: 1148 return super.setProperty(hash, name, value); 1149 } 1150 1151 } 1152 1153 @Override 1154 public Base setProperty(String name, Base value) throws FHIRException { 1155 if (name.equals("date")) { 1156 this.date = castToDateTime(value); // DateTimeType 1157 } else if (name.equals("detail")) { 1158 this.detail = castToReference(value); // Reference 1159 } else if (name.equals("reported")) { 1160 this.reported = castToBoolean(value); // BooleanType 1161 } else 1162 return super.setProperty(name, value); 1163 return value; 1164 } 1165 1166 @Override 1167 public Base makeProperty(int hash, String name) throws FHIRException { 1168 switch (hash) { 1169 case 3076014: 1170 return getDateElement(); 1171 case -1335224239: 1172 return getDetail(); 1173 case -427039533: 1174 return getReportedElement(); 1175 default: 1176 return super.makeProperty(hash, name); 1177 } 1178 1179 } 1180 1181 @Override 1182 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1183 switch (hash) { 1184 case 3076014: 1185 /* date */ return new String[] { "dateTime" }; 1186 case -1335224239: 1187 /* detail */ return new String[] { "Reference" }; 1188 case -427039533: 1189 /* reported */ return new String[] { "boolean" }; 1190 default: 1191 return super.getTypesForProperty(hash, name); 1192 } 1193 1194 } 1195 1196 @Override 1197 public Base addChild(String name) throws FHIRException { 1198 if (name.equals("date")) { 1199 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 1200 } else if (name.equals("detail")) { 1201 this.detail = new Reference(); 1202 return this.detail; 1203 } else if (name.equals("reported")) { 1204 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 1205 } else 1206 return super.addChild(name); 1207 } 1208 1209 public ImmunizationReactionComponent copy() { 1210 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 1211 copyValues(dst); 1212 return dst; 1213 } 1214 1215 public void copyValues(ImmunizationReactionComponent dst) { 1216 super.copyValues(dst); 1217 dst.date = date == null ? null : date.copy(); 1218 dst.detail = detail == null ? null : detail.copy(); 1219 dst.reported = reported == null ? null : reported.copy(); 1220 } 1221 1222 @Override 1223 public boolean equalsDeep(Base other_) { 1224 if (!super.equalsDeep(other_)) 1225 return false; 1226 if (!(other_ instanceof ImmunizationReactionComponent)) 1227 return false; 1228 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 1229 return compareDeep(date, o.date, true) && compareDeep(detail, o.detail, true) 1230 && compareDeep(reported, o.reported, true); 1231 } 1232 1233 @Override 1234 public boolean equalsShallow(Base other_) { 1235 if (!super.equalsShallow(other_)) 1236 return false; 1237 if (!(other_ instanceof ImmunizationReactionComponent)) 1238 return false; 1239 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 1240 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 1241 } 1242 1243 public boolean isEmpty() { 1244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, detail, reported); 1245 } 1246 1247 public String fhirType() { 1248 return "Immunization.reaction"; 1249 1250 } 1251 1252 } 1253 1254 @Block() 1255 public static class ImmunizationProtocolAppliedComponent extends BackboneElement implements IBaseBackboneElement { 1256 /** 1257 * One possible path to achieve presumed immunity against a disease - within the 1258 * context of an authority. 1259 */ 1260 @Child(name = "series", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1261 @Description(shortDefinition = "Name of vaccine series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 1262 protected StringType series; 1263 1264 /** 1265 * Indicates the authority who published the protocol (e.g. ACIP) that is being 1266 * followed. 1267 */ 1268 @Child(name = "authority", type = { 1269 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1270 @Description(shortDefinition = "Who is responsible for publishing the recommendations", formalDefinition = "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.") 1271 protected Reference authority; 1272 1273 /** 1274 * The actual object that is the target of the reference (Indicates the 1275 * authority who published the protocol (e.g. ACIP) that is being followed.) 1276 */ 1277 protected Organization authorityTarget; 1278 1279 /** 1280 * The vaccine preventable disease the dose is being administered against. 1281 */ 1282 @Child(name = "targetDisease", type = { 1283 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1284 @Description(shortDefinition = "Vaccine preventatable disease being targetted", formalDefinition = "The vaccine preventable disease the dose is being administered against.") 1285 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-target-disease") 1286 protected List<CodeableConcept> targetDisease; 1287 1288 /** 1289 * Nominal position in a series. 1290 */ 1291 @Child(name = "doseNumber", type = { PositiveIntType.class, 1292 StringType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 1293 @Description(shortDefinition = "Dose number within series", formalDefinition = "Nominal position in a series.") 1294 protected Type doseNumber; 1295 1296 /** 1297 * The recommended number of doses to achieve immunity. 1298 */ 1299 @Child(name = "seriesDoses", type = { PositiveIntType.class, 1300 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1301 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 1302 protected Type seriesDoses; 1303 1304 private static final long serialVersionUID = -1022717242L; 1305 1306 /** 1307 * Constructor 1308 */ 1309 public ImmunizationProtocolAppliedComponent() { 1310 super(); 1311 } 1312 1313 /** 1314 * Constructor 1315 */ 1316 public ImmunizationProtocolAppliedComponent(Type doseNumber) { 1317 super(); 1318 this.doseNumber = doseNumber; 1319 } 1320 1321 /** 1322 * @return {@link #series} (One possible path to achieve presumed immunity 1323 * against a disease - within the context of an authority.). This is the 1324 * underlying object with id, value and extensions. The accessor 1325 * "getSeries" gives direct access to the value 1326 */ 1327 public StringType getSeriesElement() { 1328 if (this.series == null) 1329 if (Configuration.errorOnAutoCreate()) 1330 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.series"); 1331 else if (Configuration.doAutoCreate()) 1332 this.series = new StringType(); // bb 1333 return this.series; 1334 } 1335 1336 public boolean hasSeriesElement() { 1337 return this.series != null && !this.series.isEmpty(); 1338 } 1339 1340 public boolean hasSeries() { 1341 return this.series != null && !this.series.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #series} (One possible path to achieve presumed immunity 1346 * against a disease - within the context of an authority.). This 1347 * is the underlying object with id, value and extensions. The 1348 * accessor "getSeries" gives direct access to the value 1349 */ 1350 public ImmunizationProtocolAppliedComponent setSeriesElement(StringType value) { 1351 this.series = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return One possible path to achieve presumed immunity against a disease - 1357 * within the context of an authority. 1358 */ 1359 public String getSeries() { 1360 return this.series == null ? null : this.series.getValue(); 1361 } 1362 1363 /** 1364 * @param value One possible path to achieve presumed immunity against a disease 1365 * - within the context of an authority. 1366 */ 1367 public ImmunizationProtocolAppliedComponent setSeries(String value) { 1368 if (Utilities.noString(value)) 1369 this.series = null; 1370 else { 1371 if (this.series == null) 1372 this.series = new StringType(); 1373 this.series.setValue(value); 1374 } 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #authority} (Indicates the authority who published the 1380 * protocol (e.g. ACIP) that is being followed.) 1381 */ 1382 public Reference getAuthority() { 1383 if (this.authority == null) 1384 if (Configuration.errorOnAutoCreate()) 1385 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.authority"); 1386 else if (Configuration.doAutoCreate()) 1387 this.authority = new Reference(); // cc 1388 return this.authority; 1389 } 1390 1391 public boolean hasAuthority() { 1392 return this.authority != null && !this.authority.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #authority} (Indicates the authority who published the 1397 * protocol (e.g. ACIP) that is being followed.) 1398 */ 1399 public ImmunizationProtocolAppliedComponent setAuthority(Reference value) { 1400 this.authority = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #authority} The actual object that is the target of the 1406 * reference. The reference library doesn't populate this, but you can 1407 * use it to hold the resource if you resolve it. (Indicates the 1408 * authority who published the protocol (e.g. ACIP) that is being 1409 * followed.) 1410 */ 1411 public Organization getAuthorityTarget() { 1412 if (this.authorityTarget == null) 1413 if (Configuration.errorOnAutoCreate()) 1414 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.authority"); 1415 else if (Configuration.doAutoCreate()) 1416 this.authorityTarget = new Organization(); // aa 1417 return this.authorityTarget; 1418 } 1419 1420 /** 1421 * @param value {@link #authority} The actual object that is the target of the 1422 * reference. The reference library doesn't use these, but you can 1423 * use it to hold the resource if you resolve it. (Indicates the 1424 * authority who published the protocol (e.g. ACIP) that is being 1425 * followed.) 1426 */ 1427 public ImmunizationProtocolAppliedComponent setAuthorityTarget(Organization value) { 1428 this.authorityTarget = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return {@link #targetDisease} (The vaccine preventable disease the dose is 1434 * being administered against.) 1435 */ 1436 public List<CodeableConcept> getTargetDisease() { 1437 if (this.targetDisease == null) 1438 this.targetDisease = new ArrayList<CodeableConcept>(); 1439 return this.targetDisease; 1440 } 1441 1442 /** 1443 * @return Returns a reference to <code>this</code> for easy method chaining 1444 */ 1445 public ImmunizationProtocolAppliedComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 1446 this.targetDisease = theTargetDisease; 1447 return this; 1448 } 1449 1450 public boolean hasTargetDisease() { 1451 if (this.targetDisease == null) 1452 return false; 1453 for (CodeableConcept item : this.targetDisease) 1454 if (!item.isEmpty()) 1455 return true; 1456 return false; 1457 } 1458 1459 public CodeableConcept addTargetDisease() { // 3 1460 CodeableConcept t = new CodeableConcept(); 1461 if (this.targetDisease == null) 1462 this.targetDisease = new ArrayList<CodeableConcept>(); 1463 this.targetDisease.add(t); 1464 return t; 1465 } 1466 1467 public ImmunizationProtocolAppliedComponent addTargetDisease(CodeableConcept t) { // 3 1468 if (t == null) 1469 return this; 1470 if (this.targetDisease == null) 1471 this.targetDisease = new ArrayList<CodeableConcept>(); 1472 this.targetDisease.add(t); 1473 return this; 1474 } 1475 1476 /** 1477 * @return The first repetition of repeating field {@link #targetDisease}, 1478 * creating it if it does not already exist 1479 */ 1480 public CodeableConcept getTargetDiseaseFirstRep() { 1481 if (getTargetDisease().isEmpty()) { 1482 addTargetDisease(); 1483 } 1484 return getTargetDisease().get(0); 1485 } 1486 1487 /** 1488 * @return {@link #doseNumber} (Nominal position in a series.) 1489 */ 1490 public Type getDoseNumber() { 1491 return this.doseNumber; 1492 } 1493 1494 /** 1495 * @return {@link #doseNumber} (Nominal position in a series.) 1496 */ 1497 public PositiveIntType getDoseNumberPositiveIntType() throws FHIRException { 1498 if (this.doseNumber == null) 1499 this.doseNumber = new PositiveIntType(); 1500 if (!(this.doseNumber instanceof PositiveIntType)) 1501 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 1502 + this.doseNumber.getClass().getName() + " was encountered"); 1503 return (PositiveIntType) this.doseNumber; 1504 } 1505 1506 public boolean hasDoseNumberPositiveIntType() { 1507 return this != null && this.doseNumber instanceof PositiveIntType; 1508 } 1509 1510 /** 1511 * @return {@link #doseNumber} (Nominal position in a series.) 1512 */ 1513 public StringType getDoseNumberStringType() throws FHIRException { 1514 if (this.doseNumber == null) 1515 this.doseNumber = new StringType(); 1516 if (!(this.doseNumber instanceof StringType)) 1517 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1518 + this.doseNumber.getClass().getName() + " was encountered"); 1519 return (StringType) this.doseNumber; 1520 } 1521 1522 public boolean hasDoseNumberStringType() { 1523 return this != null && this.doseNumber instanceof StringType; 1524 } 1525 1526 public boolean hasDoseNumber() { 1527 return this.doseNumber != null && !this.doseNumber.isEmpty(); 1528 } 1529 1530 /** 1531 * @param value {@link #doseNumber} (Nominal position in a series.) 1532 */ 1533 public ImmunizationProtocolAppliedComponent setDoseNumber(Type value) { 1534 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 1535 throw new Error("Not the right type for Immunization.protocolApplied.doseNumber[x]: " + value.fhirType()); 1536 this.doseNumber = value; 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1542 * immunity.) 1543 */ 1544 public Type getSeriesDoses() { 1545 return this.seriesDoses; 1546 } 1547 1548 /** 1549 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1550 * immunity.) 1551 */ 1552 public PositiveIntType getSeriesDosesPositiveIntType() throws FHIRException { 1553 if (this.seriesDoses == null) 1554 this.seriesDoses = new PositiveIntType(); 1555 if (!(this.seriesDoses instanceof PositiveIntType)) 1556 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 1557 + this.seriesDoses.getClass().getName() + " was encountered"); 1558 return (PositiveIntType) this.seriesDoses; 1559 } 1560 1561 public boolean hasSeriesDosesPositiveIntType() { 1562 return this != null && this.seriesDoses instanceof PositiveIntType; 1563 } 1564 1565 /** 1566 * @return {@link #seriesDoses} (The recommended number of doses to achieve 1567 * immunity.) 1568 */ 1569 public StringType getSeriesDosesStringType() throws FHIRException { 1570 if (this.seriesDoses == null) 1571 this.seriesDoses = new StringType(); 1572 if (!(this.seriesDoses instanceof StringType)) 1573 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1574 + this.seriesDoses.getClass().getName() + " was encountered"); 1575 return (StringType) this.seriesDoses; 1576 } 1577 1578 public boolean hasSeriesDosesStringType() { 1579 return this != null && this.seriesDoses instanceof StringType; 1580 } 1581 1582 public boolean hasSeriesDoses() { 1583 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1584 } 1585 1586 /** 1587 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 1588 * immunity.) 1589 */ 1590 public ImmunizationProtocolAppliedComponent setSeriesDoses(Type value) { 1591 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 1592 throw new Error("Not the right type for Immunization.protocolApplied.seriesDoses[x]: " + value.fhirType()); 1593 this.seriesDoses = value; 1594 return this; 1595 } 1596 1597 protected void listChildren(List<Property> children) { 1598 super.listChildren(children); 1599 children.add(new Property("series", "string", 1600 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1601 1, series)); 1602 children.add(new Property("authority", "Reference(Organization)", 1603 "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority)); 1604 children.add(new Property("targetDisease", "CodeableConcept", 1605 "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, 1606 targetDisease)); 1607 children 1608 .add(new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1, doseNumber)); 1609 children.add(new Property("seriesDoses[x]", "positiveInt|string", 1610 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 1611 } 1612 1613 @Override 1614 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1615 switch (_hash) { 1616 case -905838985: 1617 /* series */ return new Property("series", "string", 1618 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1619 1, series); 1620 case 1475610435: 1621 /* authority */ return new Property("authority", "Reference(Organization)", 1622 "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority); 1623 case -319593813: 1624 /* targetDisease */ return new Property("targetDisease", "CodeableConcept", 1625 "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, 1626 targetDisease); 1627 case -1632295686: 1628 /* doseNumber[x] */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 1629 0, 1, doseNumber); 1630 case -887709242: 1631 /* doseNumber */ return new Property("doseNumber[x]", "positiveInt|string", "Nominal position in a series.", 0, 1632 1, doseNumber); 1633 case -1826134640: 1634 /* doseNumberPositiveInt */ return new Property("doseNumber[x]", "positiveInt|string", 1635 "Nominal position in a series.", 0, 1, doseNumber); 1636 case -333053577: 1637 /* doseNumberString */ return new Property("doseNumber[x]", "positiveInt|string", 1638 "Nominal position in a series.", 0, 1, doseNumber); 1639 case 1553560673: 1640 /* seriesDoses[x] */ return new Property("seriesDoses[x]", "positiveInt|string", 1641 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1642 case -1936727105: 1643 /* seriesDoses */ return new Property("seriesDoses[x]", "positiveInt|string", 1644 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1645 case -220897801: 1646 /* seriesDosesPositiveInt */ return new Property("seriesDoses[x]", "positiveInt|string", 1647 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1648 case -673569616: 1649 /* seriesDosesString */ return new Property("seriesDoses[x]", "positiveInt|string", 1650 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1651 default: 1652 return super.getNamedProperty(_hash, _name, _checkValid); 1653 } 1654 1655 } 1656 1657 @Override 1658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1659 switch (hash) { 1660 case -905838985: 1661 /* series */ return this.series == null ? new Base[0] : new Base[] { this.series }; // StringType 1662 case 1475610435: 1663 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // Reference 1664 case -319593813: 1665 /* targetDisease */ return this.targetDisease == null ? new Base[0] 1666 : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 1667 case -887709242: 1668 /* doseNumber */ return this.doseNumber == null ? new Base[0] : new Base[] { this.doseNumber }; // Type 1669 case -1936727105: 1670 /* seriesDoses */ return this.seriesDoses == null ? new Base[0] : new Base[] { this.seriesDoses }; // Type 1671 default: 1672 return super.getProperty(hash, name, checkValid); 1673 } 1674 1675 } 1676 1677 @Override 1678 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1679 switch (hash) { 1680 case -905838985: // series 1681 this.series = castToString(value); // StringType 1682 return value; 1683 case 1475610435: // authority 1684 this.authority = castToReference(value); // Reference 1685 return value; 1686 case -319593813: // targetDisease 1687 this.getTargetDisease().add(castToCodeableConcept(value)); // CodeableConcept 1688 return value; 1689 case -887709242: // doseNumber 1690 this.doseNumber = castToType(value); // Type 1691 return value; 1692 case -1936727105: // seriesDoses 1693 this.seriesDoses = castToType(value); // Type 1694 return value; 1695 default: 1696 return super.setProperty(hash, name, value); 1697 } 1698 1699 } 1700 1701 @Override 1702 public Base setProperty(String name, Base value) throws FHIRException { 1703 if (name.equals("series")) { 1704 this.series = castToString(value); // StringType 1705 } else if (name.equals("authority")) { 1706 this.authority = castToReference(value); // Reference 1707 } else if (name.equals("targetDisease")) { 1708 this.getTargetDisease().add(castToCodeableConcept(value)); 1709 } else if (name.equals("doseNumber[x]")) { 1710 this.doseNumber = castToType(value); // Type 1711 } else if (name.equals("seriesDoses[x]")) { 1712 this.seriesDoses = castToType(value); // Type 1713 } else 1714 return super.setProperty(name, value); 1715 return value; 1716 } 1717 1718 @Override 1719 public Base makeProperty(int hash, String name) throws FHIRException { 1720 switch (hash) { 1721 case -905838985: 1722 return getSeriesElement(); 1723 case 1475610435: 1724 return getAuthority(); 1725 case -319593813: 1726 return addTargetDisease(); 1727 case -1632295686: 1728 return getDoseNumber(); 1729 case -887709242: 1730 return getDoseNumber(); 1731 case 1553560673: 1732 return getSeriesDoses(); 1733 case -1936727105: 1734 return getSeriesDoses(); 1735 default: 1736 return super.makeProperty(hash, name); 1737 } 1738 1739 } 1740 1741 @Override 1742 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1743 switch (hash) { 1744 case -905838985: 1745 /* series */ return new String[] { "string" }; 1746 case 1475610435: 1747 /* authority */ return new String[] { "Reference" }; 1748 case -319593813: 1749 /* targetDisease */ return new String[] { "CodeableConcept" }; 1750 case -887709242: 1751 /* doseNumber */ return new String[] { "positiveInt", "string" }; 1752 case -1936727105: 1753 /* seriesDoses */ return new String[] { "positiveInt", "string" }; 1754 default: 1755 return super.getTypesForProperty(hash, name); 1756 } 1757 1758 } 1759 1760 @Override 1761 public Base addChild(String name) throws FHIRException { 1762 if (name.equals("series")) { 1763 throw new FHIRException("Cannot call addChild on a singleton property Immunization.series"); 1764 } else if (name.equals("authority")) { 1765 this.authority = new Reference(); 1766 return this.authority; 1767 } else if (name.equals("targetDisease")) { 1768 return addTargetDisease(); 1769 } else if (name.equals("doseNumberPositiveInt")) { 1770 this.doseNumber = new PositiveIntType(); 1771 return this.doseNumber; 1772 } else if (name.equals("doseNumberString")) { 1773 this.doseNumber = new StringType(); 1774 return this.doseNumber; 1775 } else if (name.equals("seriesDosesPositiveInt")) { 1776 this.seriesDoses = new PositiveIntType(); 1777 return this.seriesDoses; 1778 } else if (name.equals("seriesDosesString")) { 1779 this.seriesDoses = new StringType(); 1780 return this.seriesDoses; 1781 } else 1782 return super.addChild(name); 1783 } 1784 1785 public ImmunizationProtocolAppliedComponent copy() { 1786 ImmunizationProtocolAppliedComponent dst = new ImmunizationProtocolAppliedComponent(); 1787 copyValues(dst); 1788 return dst; 1789 } 1790 1791 public void copyValues(ImmunizationProtocolAppliedComponent dst) { 1792 super.copyValues(dst); 1793 dst.series = series == null ? null : series.copy(); 1794 dst.authority = authority == null ? null : authority.copy(); 1795 if (targetDisease != null) { 1796 dst.targetDisease = new ArrayList<CodeableConcept>(); 1797 for (CodeableConcept i : targetDisease) 1798 dst.targetDisease.add(i.copy()); 1799 } 1800 ; 1801 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1802 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1803 } 1804 1805 @Override 1806 public boolean equalsDeep(Base other_) { 1807 if (!super.equalsDeep(other_)) 1808 return false; 1809 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1810 return false; 1811 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1812 return compareDeep(series, o.series, true) && compareDeep(authority, o.authority, true) 1813 && compareDeep(targetDisease, o.targetDisease, true) && compareDeep(doseNumber, o.doseNumber, true) 1814 && compareDeep(seriesDoses, o.seriesDoses, true); 1815 } 1816 1817 @Override 1818 public boolean equalsShallow(Base other_) { 1819 if (!super.equalsShallow(other_)) 1820 return false; 1821 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1822 return false; 1823 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1824 return compareValues(series, o.series, true); 1825 } 1826 1827 public boolean isEmpty() { 1828 return super.isEmpty() 1829 && ca.uhn.fhir.util.ElementUtil.isEmpty(series, authority, targetDisease, doseNumber, seriesDoses); 1830 } 1831 1832 public String fhirType() { 1833 return "Immunization.protocolApplied"; 1834 1835 } 1836 1837 } 1838 1839 /** 1840 * A unique identifier assigned to this immunization record. 1841 */ 1842 @Child(name = "identifier", type = { 1843 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1844 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this immunization record.") 1845 protected List<Identifier> identifier; 1846 1847 /** 1848 * Indicates the current status of the immunization event. 1849 */ 1850 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1851 @Description(shortDefinition = "completed | entered-in-error | not-done", formalDefinition = "Indicates the current status of the immunization event.") 1852 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-status") 1853 protected Enumeration<ImmunizationStatus> status; 1854 1855 /** 1856 * Indicates the reason the immunization event was not performed. 1857 */ 1858 @Child(name = "statusReason", type = { 1859 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1860 @Description(shortDefinition = "Reason not done", formalDefinition = "Indicates the reason the immunization event was not performed.") 1861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-status-reason") 1862 protected CodeableConcept statusReason; 1863 1864 /** 1865 * Vaccine that was administered or was to be administered. 1866 */ 1867 @Child(name = "vaccineCode", type = { 1868 CodeableConcept.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 1869 @Description(shortDefinition = "Vaccine product administered", formalDefinition = "Vaccine that was administered or was to be administered.") 1870 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 1871 protected CodeableConcept vaccineCode; 1872 1873 /** 1874 * The patient who either received or did not receive the immunization. 1875 */ 1876 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1877 @Description(shortDefinition = "Who was immunized", formalDefinition = "The patient who either received or did not receive the immunization.") 1878 protected Reference patient; 1879 1880 /** 1881 * The actual object that is the target of the reference (The patient who either 1882 * received or did not receive the immunization.) 1883 */ 1884 protected Patient patientTarget; 1885 1886 /** 1887 * The visit or admission or other contact between patient and health care 1888 * provider the immunization was performed as part of. 1889 */ 1890 @Child(name = "encounter", type = { Encounter.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1891 @Description(shortDefinition = "Encounter immunization was part of", formalDefinition = "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.") 1892 protected Reference encounter; 1893 1894 /** 1895 * The actual object that is the target of the reference (The visit or admission 1896 * or other contact between patient and health care provider the immunization 1897 * was performed as part of.) 1898 */ 1899 protected Encounter encounterTarget; 1900 1901 /** 1902 * Date vaccine administered or was to be administered. 1903 */ 1904 @Child(name = "occurrence", type = { DateTimeType.class, 1905 StringType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1906 @Description(shortDefinition = "Vaccine administration date", formalDefinition = "Date vaccine administered or was to be administered.") 1907 protected Type occurrence; 1908 1909 /** 1910 * The date the occurrence of the immunization was first captured in the record 1911 * - potentially significantly after the occurrence of the event. 1912 */ 1913 @Child(name = "recorded", type = { 1914 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1915 @Description(shortDefinition = "When the immunization was first captured in the subject's record", formalDefinition = "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.") 1916 protected DateTimeType recorded; 1917 1918 /** 1919 * An indication that the content of the record is based on information from the 1920 * person who administered the vaccine. This reflects the context under which 1921 * the data was originally recorded. 1922 */ 1923 @Child(name = "primarySource", type = { 1924 BooleanType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1925 @Description(shortDefinition = "Indicates context the data was recorded in", formalDefinition = "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.") 1926 protected BooleanType primarySource; 1927 1928 /** 1929 * The source of the data when the report of the immunization event is not based 1930 * on information from the person who administered the vaccine. 1931 */ 1932 @Child(name = "reportOrigin", type = { 1933 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1934 @Description(shortDefinition = "Indicates the source of a secondarily reported record", formalDefinition = "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.") 1935 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-origin") 1936 protected CodeableConcept reportOrigin; 1937 1938 /** 1939 * The service delivery location where the vaccine administration occurred. 1940 */ 1941 @Child(name = "location", type = { Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1942 @Description(shortDefinition = "Where immunization occurred", formalDefinition = "The service delivery location where the vaccine administration occurred.") 1943 protected Reference location; 1944 1945 /** 1946 * The actual object that is the target of the reference (The service delivery 1947 * location where the vaccine administration occurred.) 1948 */ 1949 protected Location locationTarget; 1950 1951 /** 1952 * Name of vaccine manufacturer. 1953 */ 1954 @Child(name = "manufacturer", type = { 1955 Organization.class }, order = 11, min = 0, max = 1, modifier = false, summary = false) 1956 @Description(shortDefinition = "Vaccine manufacturer", formalDefinition = "Name of vaccine manufacturer.") 1957 protected Reference manufacturer; 1958 1959 /** 1960 * The actual object that is the target of the reference (Name of vaccine 1961 * manufacturer.) 1962 */ 1963 protected Organization manufacturerTarget; 1964 1965 /** 1966 * Lot number of the vaccine product. 1967 */ 1968 @Child(name = "lotNumber", type = { 1969 StringType.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1970 @Description(shortDefinition = "Vaccine lot number", formalDefinition = "Lot number of the vaccine product.") 1971 protected StringType lotNumber; 1972 1973 /** 1974 * Date vaccine batch expires. 1975 */ 1976 @Child(name = "expirationDate", type = { 1977 DateType.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1978 @Description(shortDefinition = "Vaccine expiration date", formalDefinition = "Date vaccine batch expires.") 1979 protected DateType expirationDate; 1980 1981 /** 1982 * Body site where vaccine was administered. 1983 */ 1984 @Child(name = "site", type = { 1985 CodeableConcept.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1986 @Description(shortDefinition = "Body site vaccine was administered", formalDefinition = "Body site where vaccine was administered.") 1987 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-site") 1988 protected CodeableConcept site; 1989 1990 /** 1991 * The path by which the vaccine product is taken into the body. 1992 */ 1993 @Child(name = "route", type = { 1994 CodeableConcept.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1995 @Description(shortDefinition = "How vaccine entered body", formalDefinition = "The path by which the vaccine product is taken into the body.") 1996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-route") 1997 protected CodeableConcept route; 1998 1999 /** 2000 * The quantity of vaccine product that was administered. 2001 */ 2002 @Child(name = "doseQuantity", type = { 2003 Quantity.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2004 @Description(shortDefinition = "Amount of vaccine administered", formalDefinition = "The quantity of vaccine product that was administered.") 2005 protected Quantity doseQuantity; 2006 2007 /** 2008 * Indicates who performed the immunization event. 2009 */ 2010 @Child(name = "performer", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2011 @Description(shortDefinition = "Who performed event", formalDefinition = "Indicates who performed the immunization event.") 2012 protected List<ImmunizationPerformerComponent> performer; 2013 2014 /** 2015 * Extra information about the immunization that is not conveyed by the other 2016 * attributes. 2017 */ 2018 @Child(name = "note", type = { 2019 Annotation.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2020 @Description(shortDefinition = "Additional immunization notes", formalDefinition = "Extra information about the immunization that is not conveyed by the other attributes.") 2021 protected List<Annotation> note; 2022 2023 /** 2024 * Reasons why the vaccine was administered. 2025 */ 2026 @Child(name = "reasonCode", type = { 2027 CodeableConcept.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2028 @Description(shortDefinition = "Why immunization occurred", formalDefinition = "Reasons why the vaccine was administered.") 2029 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-reason") 2030 protected List<CodeableConcept> reasonCode; 2031 2032 /** 2033 * Condition, Observation or DiagnosticReport that supports why the immunization 2034 * was administered. 2035 */ 2036 @Child(name = "reasonReference", type = { Condition.class, Observation.class, 2037 DiagnosticReport.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2038 @Description(shortDefinition = "Why immunization occurred", formalDefinition = "Condition, Observation or DiagnosticReport that supports why the immunization was administered.") 2039 protected List<Reference> reasonReference; 2040 /** 2041 * The actual objects that are the target of the reference (Condition, 2042 * Observation or DiagnosticReport that supports why the immunization was 2043 * administered.) 2044 */ 2045 protected List<Resource> reasonReferenceTarget; 2046 2047 /** 2048 * Indication if a dose is considered to be subpotent. By default, a dose should 2049 * be considered to be potent. 2050 */ 2051 @Child(name = "isSubpotent", type = { 2052 BooleanType.class }, order = 21, min = 0, max = 1, modifier = true, summary = true) 2053 @Description(shortDefinition = "Dose potency", formalDefinition = "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.") 2054 protected BooleanType isSubpotent; 2055 2056 /** 2057 * Reason why a dose is considered to be subpotent. 2058 */ 2059 @Child(name = "subpotentReason", type = { 2060 CodeableConcept.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2061 @Description(shortDefinition = "Reason for being subpotent", formalDefinition = "Reason why a dose is considered to be subpotent.") 2062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-subpotent-reason") 2063 protected List<CodeableConcept> subpotentReason; 2064 2065 /** 2066 * Educational material presented to the patient (or guardian) at the time of 2067 * vaccine administration. 2068 */ 2069 @Child(name = "education", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2070 @Description(shortDefinition = "Educational material presented to patient", formalDefinition = "Educational material presented to the patient (or guardian) at the time of vaccine administration.") 2071 protected List<ImmunizationEducationComponent> education; 2072 2073 /** 2074 * Indicates a patient's eligibility for a funding program. 2075 */ 2076 @Child(name = "programEligibility", type = { 2077 CodeableConcept.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2078 @Description(shortDefinition = "Patient eligibility for a vaccination program", formalDefinition = "Indicates a patient's eligibility for a funding program.") 2079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-program-eligibility") 2080 protected List<CodeableConcept> programEligibility; 2081 2082 /** 2083 * Indicates the source of the vaccine actually administered. This may be 2084 * different than the patient eligibility (e.g. the patient may be eligible for 2085 * a publically purchased vaccine but due to inventory issues, vaccine purchased 2086 * with private funds was actually administered). 2087 */ 2088 @Child(name = "fundingSource", type = { 2089 CodeableConcept.class }, order = 25, min = 0, max = 1, modifier = false, summary = false) 2090 @Description(shortDefinition = "Funding source for the vaccine", formalDefinition = "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).") 2091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-funding-source") 2092 protected CodeableConcept fundingSource; 2093 2094 /** 2095 * Categorical data indicating that an adverse event is associated in time to an 2096 * immunization. 2097 */ 2098 @Child(name = "reaction", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2099 @Description(shortDefinition = "Details of a reaction that follows immunization", formalDefinition = "Categorical data indicating that an adverse event is associated in time to an immunization.") 2100 protected List<ImmunizationReactionComponent> reaction; 2101 2102 /** 2103 * The protocol (set of recommendations) being followed by the provider who 2104 * administered the dose. 2105 */ 2106 @Child(name = "protocolApplied", type = {}, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2107 @Description(shortDefinition = "Protocol followed by the provider", formalDefinition = "The protocol (set of recommendations) being followed by the provider who administered the dose.") 2108 protected List<ImmunizationProtocolAppliedComponent> protocolApplied; 2109 2110 private static final long serialVersionUID = 1946730839L; 2111 2112 /** 2113 * Constructor 2114 */ 2115 public Immunization() { 2116 super(); 2117 } 2118 2119 /** 2120 * Constructor 2121 */ 2122 public Immunization(Enumeration<ImmunizationStatus> status, CodeableConcept vaccineCode, Reference patient, 2123 Type occurrence) { 2124 super(); 2125 this.status = status; 2126 this.vaccineCode = vaccineCode; 2127 this.patient = patient; 2128 this.occurrence = occurrence; 2129 } 2130 2131 /** 2132 * @return {@link #identifier} (A unique identifier assigned to this 2133 * immunization record.) 2134 */ 2135 public List<Identifier> getIdentifier() { 2136 if (this.identifier == null) 2137 this.identifier = new ArrayList<Identifier>(); 2138 return this.identifier; 2139 } 2140 2141 /** 2142 * @return Returns a reference to <code>this</code> for easy method chaining 2143 */ 2144 public Immunization setIdentifier(List<Identifier> theIdentifier) { 2145 this.identifier = theIdentifier; 2146 return this; 2147 } 2148 2149 public boolean hasIdentifier() { 2150 if (this.identifier == null) 2151 return false; 2152 for (Identifier item : this.identifier) 2153 if (!item.isEmpty()) 2154 return true; 2155 return false; 2156 } 2157 2158 public Identifier addIdentifier() { // 3 2159 Identifier t = new Identifier(); 2160 if (this.identifier == null) 2161 this.identifier = new ArrayList<Identifier>(); 2162 this.identifier.add(t); 2163 return t; 2164 } 2165 2166 public Immunization addIdentifier(Identifier t) { // 3 2167 if (t == null) 2168 return this; 2169 if (this.identifier == null) 2170 this.identifier = new ArrayList<Identifier>(); 2171 this.identifier.add(t); 2172 return this; 2173 } 2174 2175 /** 2176 * @return The first repetition of repeating field {@link #identifier}, creating 2177 * it if it does not already exist 2178 */ 2179 public Identifier getIdentifierFirstRep() { 2180 if (getIdentifier().isEmpty()) { 2181 addIdentifier(); 2182 } 2183 return getIdentifier().get(0); 2184 } 2185 2186 /** 2187 * @return {@link #status} (Indicates the current status of the immunization 2188 * event.). This is the underlying object with id, value and extensions. 2189 * The accessor "getStatus" gives direct access to the value 2190 */ 2191 public Enumeration<ImmunizationStatus> getStatusElement() { 2192 if (this.status == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create Immunization.status"); 2195 else if (Configuration.doAutoCreate()) 2196 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); // bb 2197 return this.status; 2198 } 2199 2200 public boolean hasStatusElement() { 2201 return this.status != null && !this.status.isEmpty(); 2202 } 2203 2204 public boolean hasStatus() { 2205 return this.status != null && !this.status.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #status} (Indicates the current status of the 2210 * immunization event.). This is the underlying object with id, 2211 * value and extensions. The accessor "getStatus" gives direct 2212 * access to the value 2213 */ 2214 public Immunization setStatusElement(Enumeration<ImmunizationStatus> value) { 2215 this.status = value; 2216 return this; 2217 } 2218 2219 /** 2220 * @return Indicates the current status of the immunization event. 2221 */ 2222 public ImmunizationStatus getStatus() { 2223 return this.status == null ? null : this.status.getValue(); 2224 } 2225 2226 /** 2227 * @param value Indicates the current status of the immunization event. 2228 */ 2229 public Immunization setStatus(ImmunizationStatus value) { 2230 if (this.status == null) 2231 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); 2232 this.status.setValue(value); 2233 return this; 2234 } 2235 2236 /** 2237 * @return {@link #statusReason} (Indicates the reason the immunization event 2238 * was not performed.) 2239 */ 2240 public CodeableConcept getStatusReason() { 2241 if (this.statusReason == null) 2242 if (Configuration.errorOnAutoCreate()) 2243 throw new Error("Attempt to auto-create Immunization.statusReason"); 2244 else if (Configuration.doAutoCreate()) 2245 this.statusReason = new CodeableConcept(); // cc 2246 return this.statusReason; 2247 } 2248 2249 public boolean hasStatusReason() { 2250 return this.statusReason != null && !this.statusReason.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #statusReason} (Indicates the reason the immunization 2255 * event was not performed.) 2256 */ 2257 public Immunization setStatusReason(CodeableConcept value) { 2258 this.statusReason = value; 2259 return this; 2260 } 2261 2262 /** 2263 * @return {@link #vaccineCode} (Vaccine that was administered or was to be 2264 * administered.) 2265 */ 2266 public CodeableConcept getVaccineCode() { 2267 if (this.vaccineCode == null) 2268 if (Configuration.errorOnAutoCreate()) 2269 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 2270 else if (Configuration.doAutoCreate()) 2271 this.vaccineCode = new CodeableConcept(); // cc 2272 return this.vaccineCode; 2273 } 2274 2275 public boolean hasVaccineCode() { 2276 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 2277 } 2278 2279 /** 2280 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be 2281 * administered.) 2282 */ 2283 public Immunization setVaccineCode(CodeableConcept value) { 2284 this.vaccineCode = value; 2285 return this; 2286 } 2287 2288 /** 2289 * @return {@link #patient} (The patient who either received or did not receive 2290 * the immunization.) 2291 */ 2292 public Reference getPatient() { 2293 if (this.patient == null) 2294 if (Configuration.errorOnAutoCreate()) 2295 throw new Error("Attempt to auto-create Immunization.patient"); 2296 else if (Configuration.doAutoCreate()) 2297 this.patient = new Reference(); // cc 2298 return this.patient; 2299 } 2300 2301 public boolean hasPatient() { 2302 return this.patient != null && !this.patient.isEmpty(); 2303 } 2304 2305 /** 2306 * @param value {@link #patient} (The patient who either received or did not 2307 * receive the immunization.) 2308 */ 2309 public Immunization setPatient(Reference value) { 2310 this.patient = value; 2311 return this; 2312 } 2313 2314 /** 2315 * @return {@link #patient} The actual object that is the target of the 2316 * reference. The reference library doesn't populate this, but you can 2317 * use it to hold the resource if you resolve it. (The patient who 2318 * either received or did not receive the immunization.) 2319 */ 2320 public Patient getPatientTarget() { 2321 if (this.patientTarget == null) 2322 if (Configuration.errorOnAutoCreate()) 2323 throw new Error("Attempt to auto-create Immunization.patient"); 2324 else if (Configuration.doAutoCreate()) 2325 this.patientTarget = new Patient(); // aa 2326 return this.patientTarget; 2327 } 2328 2329 /** 2330 * @param value {@link #patient} The actual object that is the target of the 2331 * reference. The reference library doesn't use these, but you can 2332 * use it to hold the resource if you resolve it. (The patient who 2333 * either received or did not receive the immunization.) 2334 */ 2335 public Immunization setPatientTarget(Patient value) { 2336 this.patientTarget = value; 2337 return this; 2338 } 2339 2340 /** 2341 * @return {@link #encounter} (The visit or admission or other contact between 2342 * patient and health care provider the immunization was performed as 2343 * part of.) 2344 */ 2345 public Reference getEncounter() { 2346 if (this.encounter == null) 2347 if (Configuration.errorOnAutoCreate()) 2348 throw new Error("Attempt to auto-create Immunization.encounter"); 2349 else if (Configuration.doAutoCreate()) 2350 this.encounter = new Reference(); // cc 2351 return this.encounter; 2352 } 2353 2354 public boolean hasEncounter() { 2355 return this.encounter != null && !this.encounter.isEmpty(); 2356 } 2357 2358 /** 2359 * @param value {@link #encounter} (The visit or admission or other contact 2360 * between patient and health care provider the immunization was 2361 * performed as part of.) 2362 */ 2363 public Immunization setEncounter(Reference value) { 2364 this.encounter = value; 2365 return this; 2366 } 2367 2368 /** 2369 * @return {@link #encounter} The actual object that is the target of the 2370 * reference. The reference library doesn't populate this, but you can 2371 * use it to hold the resource if you resolve it. (The visit or 2372 * admission or other contact between patient and health care provider 2373 * the immunization was performed as part of.) 2374 */ 2375 public Encounter getEncounterTarget() { 2376 if (this.encounterTarget == null) 2377 if (Configuration.errorOnAutoCreate()) 2378 throw new Error("Attempt to auto-create Immunization.encounter"); 2379 else if (Configuration.doAutoCreate()) 2380 this.encounterTarget = new Encounter(); // aa 2381 return this.encounterTarget; 2382 } 2383 2384 /** 2385 * @param value {@link #encounter} The actual object that is the target of the 2386 * reference. The reference library doesn't use these, but you can 2387 * use it to hold the resource if you resolve it. (The visit or 2388 * admission or other contact between patient and health care 2389 * provider the immunization was performed as part of.) 2390 */ 2391 public Immunization setEncounterTarget(Encounter value) { 2392 this.encounterTarget = value; 2393 return this; 2394 } 2395 2396 /** 2397 * @return {@link #occurrence} (Date vaccine administered or was to be 2398 * administered.) 2399 */ 2400 public Type getOccurrence() { 2401 return this.occurrence; 2402 } 2403 2404 /** 2405 * @return {@link #occurrence} (Date vaccine administered or was to be 2406 * administered.) 2407 */ 2408 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2409 if (this.occurrence == null) 2410 this.occurrence = new DateTimeType(); 2411 if (!(this.occurrence instanceof DateTimeType)) 2412 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2413 + this.occurrence.getClass().getName() + " was encountered"); 2414 return (DateTimeType) this.occurrence; 2415 } 2416 2417 public boolean hasOccurrenceDateTimeType() { 2418 return this != null && this.occurrence instanceof DateTimeType; 2419 } 2420 2421 /** 2422 * @return {@link #occurrence} (Date vaccine administered or was to be 2423 * administered.) 2424 */ 2425 public StringType getOccurrenceStringType() throws FHIRException { 2426 if (this.occurrence == null) 2427 this.occurrence = new StringType(); 2428 if (!(this.occurrence instanceof StringType)) 2429 throw new FHIRException("Type mismatch: the type StringType was expected, but " 2430 + this.occurrence.getClass().getName() + " was encountered"); 2431 return (StringType) this.occurrence; 2432 } 2433 2434 public boolean hasOccurrenceStringType() { 2435 return this != null && this.occurrence instanceof StringType; 2436 } 2437 2438 public boolean hasOccurrence() { 2439 return this.occurrence != null && !this.occurrence.isEmpty(); 2440 } 2441 2442 /** 2443 * @param value {@link #occurrence} (Date vaccine administered or was to be 2444 * administered.) 2445 */ 2446 public Immunization setOccurrence(Type value) { 2447 if (value != null && !(value instanceof DateTimeType || value instanceof StringType)) 2448 throw new Error("Not the right type for Immunization.occurrence[x]: " + value.fhirType()); 2449 this.occurrence = value; 2450 return this; 2451 } 2452 2453 /** 2454 * @return {@link #recorded} (The date the occurrence of the immunization was 2455 * first captured in the record - potentially significantly after the 2456 * occurrence of the event.). This is the underlying object with id, 2457 * value and extensions. The accessor "getRecorded" gives direct access 2458 * to the value 2459 */ 2460 public DateTimeType getRecordedElement() { 2461 if (this.recorded == null) 2462 if (Configuration.errorOnAutoCreate()) 2463 throw new Error("Attempt to auto-create Immunization.recorded"); 2464 else if (Configuration.doAutoCreate()) 2465 this.recorded = new DateTimeType(); // bb 2466 return this.recorded; 2467 } 2468 2469 public boolean hasRecordedElement() { 2470 return this.recorded != null && !this.recorded.isEmpty(); 2471 } 2472 2473 public boolean hasRecorded() { 2474 return this.recorded != null && !this.recorded.isEmpty(); 2475 } 2476 2477 /** 2478 * @param value {@link #recorded} (The date the occurrence of the immunization 2479 * was first captured in the record - potentially significantly 2480 * after the occurrence of the event.). This is the underlying 2481 * object with id, value and extensions. The accessor "getRecorded" 2482 * gives direct access to the value 2483 */ 2484 public Immunization setRecordedElement(DateTimeType value) { 2485 this.recorded = value; 2486 return this; 2487 } 2488 2489 /** 2490 * @return The date the occurrence of the immunization was first captured in the 2491 * record - potentially significantly after the occurrence of the event. 2492 */ 2493 public Date getRecorded() { 2494 return this.recorded == null ? null : this.recorded.getValue(); 2495 } 2496 2497 /** 2498 * @param value The date the occurrence of the immunization was first captured 2499 * in the record - potentially significantly after the occurrence 2500 * of the event. 2501 */ 2502 public Immunization setRecorded(Date value) { 2503 if (value == null) 2504 this.recorded = null; 2505 else { 2506 if (this.recorded == null) 2507 this.recorded = new DateTimeType(); 2508 this.recorded.setValue(value); 2509 } 2510 return this; 2511 } 2512 2513 /** 2514 * @return {@link #primarySource} (An indication that the content of the record 2515 * is based on information from the person who administered the vaccine. 2516 * This reflects the context under which the data was originally 2517 * recorded.). This is the underlying object with id, value and 2518 * extensions. The accessor "getPrimarySource" gives direct access to 2519 * the value 2520 */ 2521 public BooleanType getPrimarySourceElement() { 2522 if (this.primarySource == null) 2523 if (Configuration.errorOnAutoCreate()) 2524 throw new Error("Attempt to auto-create Immunization.primarySource"); 2525 else if (Configuration.doAutoCreate()) 2526 this.primarySource = new BooleanType(); // bb 2527 return this.primarySource; 2528 } 2529 2530 public boolean hasPrimarySourceElement() { 2531 return this.primarySource != null && !this.primarySource.isEmpty(); 2532 } 2533 2534 public boolean hasPrimarySource() { 2535 return this.primarySource != null && !this.primarySource.isEmpty(); 2536 } 2537 2538 /** 2539 * @param value {@link #primarySource} (An indication that the content of the 2540 * record is based on information from the person who administered 2541 * the vaccine. This reflects the context under which the data was 2542 * originally recorded.). This is the underlying object with id, 2543 * value and extensions. The accessor "getPrimarySource" gives 2544 * direct access to the value 2545 */ 2546 public Immunization setPrimarySourceElement(BooleanType value) { 2547 this.primarySource = value; 2548 return this; 2549 } 2550 2551 /** 2552 * @return An indication that the content of the record is based on information 2553 * from the person who administered the vaccine. This reflects the 2554 * context under which the data was originally recorded. 2555 */ 2556 public boolean getPrimarySource() { 2557 return this.primarySource == null || this.primarySource.isEmpty() ? false : this.primarySource.getValue(); 2558 } 2559 2560 /** 2561 * @param value An indication that the content of the record is based on 2562 * information from the person who administered the vaccine. This 2563 * reflects the context under which the data was originally 2564 * recorded. 2565 */ 2566 public Immunization setPrimarySource(boolean value) { 2567 if (this.primarySource == null) 2568 this.primarySource = new BooleanType(); 2569 this.primarySource.setValue(value); 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #reportOrigin} (The source of the data when the report of the 2575 * immunization event is not based on information from the person who 2576 * administered the vaccine.) 2577 */ 2578 public CodeableConcept getReportOrigin() { 2579 if (this.reportOrigin == null) 2580 if (Configuration.errorOnAutoCreate()) 2581 throw new Error("Attempt to auto-create Immunization.reportOrigin"); 2582 else if (Configuration.doAutoCreate()) 2583 this.reportOrigin = new CodeableConcept(); // cc 2584 return this.reportOrigin; 2585 } 2586 2587 public boolean hasReportOrigin() { 2588 return this.reportOrigin != null && !this.reportOrigin.isEmpty(); 2589 } 2590 2591 /** 2592 * @param value {@link #reportOrigin} (The source of the data when the report of 2593 * the immunization event is not based on information from the 2594 * person who administered the vaccine.) 2595 */ 2596 public Immunization setReportOrigin(CodeableConcept value) { 2597 this.reportOrigin = value; 2598 return this; 2599 } 2600 2601 /** 2602 * @return {@link #location} (The service delivery location where the vaccine 2603 * administration occurred.) 2604 */ 2605 public Reference getLocation() { 2606 if (this.location == null) 2607 if (Configuration.errorOnAutoCreate()) 2608 throw new Error("Attempt to auto-create Immunization.location"); 2609 else if (Configuration.doAutoCreate()) 2610 this.location = new Reference(); // cc 2611 return this.location; 2612 } 2613 2614 public boolean hasLocation() { 2615 return this.location != null && !this.location.isEmpty(); 2616 } 2617 2618 /** 2619 * @param value {@link #location} (The service delivery location where the 2620 * vaccine administration occurred.) 2621 */ 2622 public Immunization setLocation(Reference value) { 2623 this.location = value; 2624 return this; 2625 } 2626 2627 /** 2628 * @return {@link #location} The actual object that is the target of the 2629 * reference. The reference library doesn't populate this, but you can 2630 * use it to hold the resource if you resolve it. (The service delivery 2631 * location where the vaccine administration occurred.) 2632 */ 2633 public Location getLocationTarget() { 2634 if (this.locationTarget == null) 2635 if (Configuration.errorOnAutoCreate()) 2636 throw new Error("Attempt to auto-create Immunization.location"); 2637 else if (Configuration.doAutoCreate()) 2638 this.locationTarget = new Location(); // aa 2639 return this.locationTarget; 2640 } 2641 2642 /** 2643 * @param value {@link #location} The actual object that is the target of the 2644 * reference. The reference library doesn't use these, but you can 2645 * use it to hold the resource if you resolve it. (The service 2646 * delivery location where the vaccine administration occurred.) 2647 */ 2648 public Immunization setLocationTarget(Location value) { 2649 this.locationTarget = value; 2650 return this; 2651 } 2652 2653 /** 2654 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 2655 */ 2656 public Reference getManufacturer() { 2657 if (this.manufacturer == null) 2658 if (Configuration.errorOnAutoCreate()) 2659 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2660 else if (Configuration.doAutoCreate()) 2661 this.manufacturer = new Reference(); // cc 2662 return this.manufacturer; 2663 } 2664 2665 public boolean hasManufacturer() { 2666 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2667 } 2668 2669 /** 2670 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 2671 */ 2672 public Immunization setManufacturer(Reference value) { 2673 this.manufacturer = value; 2674 return this; 2675 } 2676 2677 /** 2678 * @return {@link #manufacturer} The actual object that is the target of the 2679 * reference. The reference library doesn't populate this, but you can 2680 * use it to hold the resource if you resolve it. (Name of vaccine 2681 * manufacturer.) 2682 */ 2683 public Organization getManufacturerTarget() { 2684 if (this.manufacturerTarget == null) 2685 if (Configuration.errorOnAutoCreate()) 2686 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2687 else if (Configuration.doAutoCreate()) 2688 this.manufacturerTarget = new Organization(); // aa 2689 return this.manufacturerTarget; 2690 } 2691 2692 /** 2693 * @param value {@link #manufacturer} The actual object that is the target of 2694 * the reference. The reference library doesn't use these, but you 2695 * can use it to hold the resource if you resolve it. (Name of 2696 * vaccine manufacturer.) 2697 */ 2698 public Immunization setManufacturerTarget(Organization value) { 2699 this.manufacturerTarget = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the 2705 * underlying object with id, value and extensions. The accessor 2706 * "getLotNumber" gives direct access to the value 2707 */ 2708 public StringType getLotNumberElement() { 2709 if (this.lotNumber == null) 2710 if (Configuration.errorOnAutoCreate()) 2711 throw new Error("Attempt to auto-create Immunization.lotNumber"); 2712 else if (Configuration.doAutoCreate()) 2713 this.lotNumber = new StringType(); // bb 2714 return this.lotNumber; 2715 } 2716 2717 public boolean hasLotNumberElement() { 2718 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2719 } 2720 2721 public boolean hasLotNumber() { 2722 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2723 } 2724 2725 /** 2726 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is 2727 * the underlying object with id, value and extensions. The 2728 * accessor "getLotNumber" gives direct access to the value 2729 */ 2730 public Immunization setLotNumberElement(StringType value) { 2731 this.lotNumber = value; 2732 return this; 2733 } 2734 2735 /** 2736 * @return Lot number of the vaccine product. 2737 */ 2738 public String getLotNumber() { 2739 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2740 } 2741 2742 /** 2743 * @param value Lot number of the vaccine product. 2744 */ 2745 public Immunization setLotNumber(String value) { 2746 if (Utilities.noString(value)) 2747 this.lotNumber = null; 2748 else { 2749 if (this.lotNumber == null) 2750 this.lotNumber = new StringType(); 2751 this.lotNumber.setValue(value); 2752 } 2753 return this; 2754 } 2755 2756 /** 2757 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the 2758 * underlying object with id, value and extensions. The accessor 2759 * "getExpirationDate" gives direct access to the value 2760 */ 2761 public DateType getExpirationDateElement() { 2762 if (this.expirationDate == null) 2763 if (Configuration.errorOnAutoCreate()) 2764 throw new Error("Attempt to auto-create Immunization.expirationDate"); 2765 else if (Configuration.doAutoCreate()) 2766 this.expirationDate = new DateType(); // bb 2767 return this.expirationDate; 2768 } 2769 2770 public boolean hasExpirationDateElement() { 2771 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2772 } 2773 2774 public boolean hasExpirationDate() { 2775 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2776 } 2777 2778 /** 2779 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is 2780 * the underlying object with id, value and extensions. The 2781 * accessor "getExpirationDate" gives direct access to the value 2782 */ 2783 public Immunization setExpirationDateElement(DateType value) { 2784 this.expirationDate = value; 2785 return this; 2786 } 2787 2788 /** 2789 * @return Date vaccine batch expires. 2790 */ 2791 public Date getExpirationDate() { 2792 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2793 } 2794 2795 /** 2796 * @param value Date vaccine batch expires. 2797 */ 2798 public Immunization setExpirationDate(Date value) { 2799 if (value == null) 2800 this.expirationDate = null; 2801 else { 2802 if (this.expirationDate == null) 2803 this.expirationDate = new DateType(); 2804 this.expirationDate.setValue(value); 2805 } 2806 return this; 2807 } 2808 2809 /** 2810 * @return {@link #site} (Body site where vaccine was administered.) 2811 */ 2812 public CodeableConcept getSite() { 2813 if (this.site == null) 2814 if (Configuration.errorOnAutoCreate()) 2815 throw new Error("Attempt to auto-create Immunization.site"); 2816 else if (Configuration.doAutoCreate()) 2817 this.site = new CodeableConcept(); // cc 2818 return this.site; 2819 } 2820 2821 public boolean hasSite() { 2822 return this.site != null && !this.site.isEmpty(); 2823 } 2824 2825 /** 2826 * @param value {@link #site} (Body site where vaccine was administered.) 2827 */ 2828 public Immunization setSite(CodeableConcept value) { 2829 this.site = value; 2830 return this; 2831 } 2832 2833 /** 2834 * @return {@link #route} (The path by which the vaccine product is taken into 2835 * the body.) 2836 */ 2837 public CodeableConcept getRoute() { 2838 if (this.route == null) 2839 if (Configuration.errorOnAutoCreate()) 2840 throw new Error("Attempt to auto-create Immunization.route"); 2841 else if (Configuration.doAutoCreate()) 2842 this.route = new CodeableConcept(); // cc 2843 return this.route; 2844 } 2845 2846 public boolean hasRoute() { 2847 return this.route != null && !this.route.isEmpty(); 2848 } 2849 2850 /** 2851 * @param value {@link #route} (The path by which the vaccine product is taken 2852 * into the body.) 2853 */ 2854 public Immunization setRoute(CodeableConcept value) { 2855 this.route = value; 2856 return this; 2857 } 2858 2859 /** 2860 * @return {@link #doseQuantity} (The quantity of vaccine product that was 2861 * administered.) 2862 */ 2863 public Quantity getDoseQuantity() { 2864 if (this.doseQuantity == null) 2865 if (Configuration.errorOnAutoCreate()) 2866 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2867 else if (Configuration.doAutoCreate()) 2868 this.doseQuantity = new Quantity(); // cc 2869 return this.doseQuantity; 2870 } 2871 2872 public boolean hasDoseQuantity() { 2873 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2874 } 2875 2876 /** 2877 * @param value {@link #doseQuantity} (The quantity of vaccine product that was 2878 * administered.) 2879 */ 2880 public Immunization setDoseQuantity(Quantity value) { 2881 this.doseQuantity = value; 2882 return this; 2883 } 2884 2885 /** 2886 * @return {@link #performer} (Indicates who performed the immunization event.) 2887 */ 2888 public List<ImmunizationPerformerComponent> getPerformer() { 2889 if (this.performer == null) 2890 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2891 return this.performer; 2892 } 2893 2894 /** 2895 * @return Returns a reference to <code>this</code> for easy method chaining 2896 */ 2897 public Immunization setPerformer(List<ImmunizationPerformerComponent> thePerformer) { 2898 this.performer = thePerformer; 2899 return this; 2900 } 2901 2902 public boolean hasPerformer() { 2903 if (this.performer == null) 2904 return false; 2905 for (ImmunizationPerformerComponent item : this.performer) 2906 if (!item.isEmpty()) 2907 return true; 2908 return false; 2909 } 2910 2911 public ImmunizationPerformerComponent addPerformer() { // 3 2912 ImmunizationPerformerComponent t = new ImmunizationPerformerComponent(); 2913 if (this.performer == null) 2914 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2915 this.performer.add(t); 2916 return t; 2917 } 2918 2919 public Immunization addPerformer(ImmunizationPerformerComponent t) { // 3 2920 if (t == null) 2921 return this; 2922 if (this.performer == null) 2923 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2924 this.performer.add(t); 2925 return this; 2926 } 2927 2928 /** 2929 * @return The first repetition of repeating field {@link #performer}, creating 2930 * it if it does not already exist 2931 */ 2932 public ImmunizationPerformerComponent getPerformerFirstRep() { 2933 if (getPerformer().isEmpty()) { 2934 addPerformer(); 2935 } 2936 return getPerformer().get(0); 2937 } 2938 2939 /** 2940 * @return {@link #note} (Extra information about the immunization that is not 2941 * conveyed by the other attributes.) 2942 */ 2943 public List<Annotation> getNote() { 2944 if (this.note == null) 2945 this.note = new ArrayList<Annotation>(); 2946 return this.note; 2947 } 2948 2949 /** 2950 * @return Returns a reference to <code>this</code> for easy method chaining 2951 */ 2952 public Immunization setNote(List<Annotation> theNote) { 2953 this.note = theNote; 2954 return this; 2955 } 2956 2957 public boolean hasNote() { 2958 if (this.note == null) 2959 return false; 2960 for (Annotation item : this.note) 2961 if (!item.isEmpty()) 2962 return true; 2963 return false; 2964 } 2965 2966 public Annotation addNote() { // 3 2967 Annotation t = new Annotation(); 2968 if (this.note == null) 2969 this.note = new ArrayList<Annotation>(); 2970 this.note.add(t); 2971 return t; 2972 } 2973 2974 public Immunization addNote(Annotation t) { // 3 2975 if (t == null) 2976 return this; 2977 if (this.note == null) 2978 this.note = new ArrayList<Annotation>(); 2979 this.note.add(t); 2980 return this; 2981 } 2982 2983 /** 2984 * @return The first repetition of repeating field {@link #note}, creating it if 2985 * it does not already exist 2986 */ 2987 public Annotation getNoteFirstRep() { 2988 if (getNote().isEmpty()) { 2989 addNote(); 2990 } 2991 return getNote().get(0); 2992 } 2993 2994 /** 2995 * @return {@link #reasonCode} (Reasons why the vaccine was administered.) 2996 */ 2997 public List<CodeableConcept> getReasonCode() { 2998 if (this.reasonCode == null) 2999 this.reasonCode = new ArrayList<CodeableConcept>(); 3000 return this.reasonCode; 3001 } 3002 3003 /** 3004 * @return Returns a reference to <code>this</code> for easy method chaining 3005 */ 3006 public Immunization setReasonCode(List<CodeableConcept> theReasonCode) { 3007 this.reasonCode = theReasonCode; 3008 return this; 3009 } 3010 3011 public boolean hasReasonCode() { 3012 if (this.reasonCode == null) 3013 return false; 3014 for (CodeableConcept item : this.reasonCode) 3015 if (!item.isEmpty()) 3016 return true; 3017 return false; 3018 } 3019 3020 public CodeableConcept addReasonCode() { // 3 3021 CodeableConcept t = new CodeableConcept(); 3022 if (this.reasonCode == null) 3023 this.reasonCode = new ArrayList<CodeableConcept>(); 3024 this.reasonCode.add(t); 3025 return t; 3026 } 3027 3028 public Immunization addReasonCode(CodeableConcept t) { // 3 3029 if (t == null) 3030 return this; 3031 if (this.reasonCode == null) 3032 this.reasonCode = new ArrayList<CodeableConcept>(); 3033 this.reasonCode.add(t); 3034 return this; 3035 } 3036 3037 /** 3038 * @return The first repetition of repeating field {@link #reasonCode}, creating 3039 * it if it does not already exist 3040 */ 3041 public CodeableConcept getReasonCodeFirstRep() { 3042 if (getReasonCode().isEmpty()) { 3043 addReasonCode(); 3044 } 3045 return getReasonCode().get(0); 3046 } 3047 3048 /** 3049 * @return {@link #reasonReference} (Condition, Observation or DiagnosticReport 3050 * that supports why the immunization was administered.) 3051 */ 3052 public List<Reference> getReasonReference() { 3053 if (this.reasonReference == null) 3054 this.reasonReference = new ArrayList<Reference>(); 3055 return this.reasonReference; 3056 } 3057 3058 /** 3059 * @return Returns a reference to <code>this</code> for easy method chaining 3060 */ 3061 public Immunization setReasonReference(List<Reference> theReasonReference) { 3062 this.reasonReference = theReasonReference; 3063 return this; 3064 } 3065 3066 public boolean hasReasonReference() { 3067 if (this.reasonReference == null) 3068 return false; 3069 for (Reference item : this.reasonReference) 3070 if (!item.isEmpty()) 3071 return true; 3072 return false; 3073 } 3074 3075 public Reference addReasonReference() { // 3 3076 Reference t = new Reference(); 3077 if (this.reasonReference == null) 3078 this.reasonReference = new ArrayList<Reference>(); 3079 this.reasonReference.add(t); 3080 return t; 3081 } 3082 3083 public Immunization addReasonReference(Reference t) { // 3 3084 if (t == null) 3085 return this; 3086 if (this.reasonReference == null) 3087 this.reasonReference = new ArrayList<Reference>(); 3088 this.reasonReference.add(t); 3089 return this; 3090 } 3091 3092 /** 3093 * @return The first repetition of repeating field {@link #reasonReference}, 3094 * creating it if it does not already exist 3095 */ 3096 public Reference getReasonReferenceFirstRep() { 3097 if (getReasonReference().isEmpty()) { 3098 addReasonReference(); 3099 } 3100 return getReasonReference().get(0); 3101 } 3102 3103 /** 3104 * @deprecated Use Reference#setResource(IBaseResource) instead 3105 */ 3106 @Deprecated 3107 public List<Resource> getReasonReferenceTarget() { 3108 if (this.reasonReferenceTarget == null) 3109 this.reasonReferenceTarget = new ArrayList<Resource>(); 3110 return this.reasonReferenceTarget; 3111 } 3112 3113 /** 3114 * @return {@link #isSubpotent} (Indication if a dose is considered to be 3115 * subpotent. By default, a dose should be considered to be potent.). 3116 * This is the underlying object with id, value and extensions. The 3117 * accessor "getIsSubpotent" gives direct access to the value 3118 */ 3119 public BooleanType getIsSubpotentElement() { 3120 if (this.isSubpotent == null) 3121 if (Configuration.errorOnAutoCreate()) 3122 throw new Error("Attempt to auto-create Immunization.isSubpotent"); 3123 else if (Configuration.doAutoCreate()) 3124 this.isSubpotent = new BooleanType(); // bb 3125 return this.isSubpotent; 3126 } 3127 3128 public boolean hasIsSubpotentElement() { 3129 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 3130 } 3131 3132 public boolean hasIsSubpotent() { 3133 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 3134 } 3135 3136 /** 3137 * @param value {@link #isSubpotent} (Indication if a dose is considered to be 3138 * subpotent. By default, a dose should be considered to be 3139 * potent.). This is the underlying object with id, value and 3140 * extensions. The accessor "getIsSubpotent" gives direct access to 3141 * the value 3142 */ 3143 public Immunization setIsSubpotentElement(BooleanType value) { 3144 this.isSubpotent = value; 3145 return this; 3146 } 3147 3148 /** 3149 * @return Indication if a dose is considered to be subpotent. By default, a 3150 * dose should be considered to be potent. 3151 */ 3152 public boolean getIsSubpotent() { 3153 return this.isSubpotent == null || this.isSubpotent.isEmpty() ? false : this.isSubpotent.getValue(); 3154 } 3155 3156 /** 3157 * @param value Indication if a dose is considered to be subpotent. By default, 3158 * a dose should be considered to be potent. 3159 */ 3160 public Immunization setIsSubpotent(boolean value) { 3161 if (this.isSubpotent == null) 3162 this.isSubpotent = new BooleanType(); 3163 this.isSubpotent.setValue(value); 3164 return this; 3165 } 3166 3167 /** 3168 * @return {@link #subpotentReason} (Reason why a dose is considered to be 3169 * subpotent.) 3170 */ 3171 public List<CodeableConcept> getSubpotentReason() { 3172 if (this.subpotentReason == null) 3173 this.subpotentReason = new ArrayList<CodeableConcept>(); 3174 return this.subpotentReason; 3175 } 3176 3177 /** 3178 * @return Returns a reference to <code>this</code> for easy method chaining 3179 */ 3180 public Immunization setSubpotentReason(List<CodeableConcept> theSubpotentReason) { 3181 this.subpotentReason = theSubpotentReason; 3182 return this; 3183 } 3184 3185 public boolean hasSubpotentReason() { 3186 if (this.subpotentReason == null) 3187 return false; 3188 for (CodeableConcept item : this.subpotentReason) 3189 if (!item.isEmpty()) 3190 return true; 3191 return false; 3192 } 3193 3194 public CodeableConcept addSubpotentReason() { // 3 3195 CodeableConcept t = new CodeableConcept(); 3196 if (this.subpotentReason == null) 3197 this.subpotentReason = new ArrayList<CodeableConcept>(); 3198 this.subpotentReason.add(t); 3199 return t; 3200 } 3201 3202 public Immunization addSubpotentReason(CodeableConcept t) { // 3 3203 if (t == null) 3204 return this; 3205 if (this.subpotentReason == null) 3206 this.subpotentReason = new ArrayList<CodeableConcept>(); 3207 this.subpotentReason.add(t); 3208 return this; 3209 } 3210 3211 /** 3212 * @return The first repetition of repeating field {@link #subpotentReason}, 3213 * creating it if it does not already exist 3214 */ 3215 public CodeableConcept getSubpotentReasonFirstRep() { 3216 if (getSubpotentReason().isEmpty()) { 3217 addSubpotentReason(); 3218 } 3219 return getSubpotentReason().get(0); 3220 } 3221 3222 /** 3223 * @return {@link #education} (Educational material presented to the patient (or 3224 * guardian) at the time of vaccine administration.) 3225 */ 3226 public List<ImmunizationEducationComponent> getEducation() { 3227 if (this.education == null) 3228 this.education = new ArrayList<ImmunizationEducationComponent>(); 3229 return this.education; 3230 } 3231 3232 /** 3233 * @return Returns a reference to <code>this</code> for easy method chaining 3234 */ 3235 public Immunization setEducation(List<ImmunizationEducationComponent> theEducation) { 3236 this.education = theEducation; 3237 return this; 3238 } 3239 3240 public boolean hasEducation() { 3241 if (this.education == null) 3242 return false; 3243 for (ImmunizationEducationComponent item : this.education) 3244 if (!item.isEmpty()) 3245 return true; 3246 return false; 3247 } 3248 3249 public ImmunizationEducationComponent addEducation() { // 3 3250 ImmunizationEducationComponent t = new ImmunizationEducationComponent(); 3251 if (this.education == null) 3252 this.education = new ArrayList<ImmunizationEducationComponent>(); 3253 this.education.add(t); 3254 return t; 3255 } 3256 3257 public Immunization addEducation(ImmunizationEducationComponent t) { // 3 3258 if (t == null) 3259 return this; 3260 if (this.education == null) 3261 this.education = new ArrayList<ImmunizationEducationComponent>(); 3262 this.education.add(t); 3263 return this; 3264 } 3265 3266 /** 3267 * @return The first repetition of repeating field {@link #education}, creating 3268 * it if it does not already exist 3269 */ 3270 public ImmunizationEducationComponent getEducationFirstRep() { 3271 if (getEducation().isEmpty()) { 3272 addEducation(); 3273 } 3274 return getEducation().get(0); 3275 } 3276 3277 /** 3278 * @return {@link #programEligibility} (Indicates a patient's eligibility for a 3279 * funding program.) 3280 */ 3281 public List<CodeableConcept> getProgramEligibility() { 3282 if (this.programEligibility == null) 3283 this.programEligibility = new ArrayList<CodeableConcept>(); 3284 return this.programEligibility; 3285 } 3286 3287 /** 3288 * @return Returns a reference to <code>this</code> for easy method chaining 3289 */ 3290 public Immunization setProgramEligibility(List<CodeableConcept> theProgramEligibility) { 3291 this.programEligibility = theProgramEligibility; 3292 return this; 3293 } 3294 3295 public boolean hasProgramEligibility() { 3296 if (this.programEligibility == null) 3297 return false; 3298 for (CodeableConcept item : this.programEligibility) 3299 if (!item.isEmpty()) 3300 return true; 3301 return false; 3302 } 3303 3304 public CodeableConcept addProgramEligibility() { // 3 3305 CodeableConcept t = new CodeableConcept(); 3306 if (this.programEligibility == null) 3307 this.programEligibility = new ArrayList<CodeableConcept>(); 3308 this.programEligibility.add(t); 3309 return t; 3310 } 3311 3312 public Immunization addProgramEligibility(CodeableConcept t) { // 3 3313 if (t == null) 3314 return this; 3315 if (this.programEligibility == null) 3316 this.programEligibility = new ArrayList<CodeableConcept>(); 3317 this.programEligibility.add(t); 3318 return this; 3319 } 3320 3321 /** 3322 * @return The first repetition of repeating field {@link #programEligibility}, 3323 * creating it if it does not already exist 3324 */ 3325 public CodeableConcept getProgramEligibilityFirstRep() { 3326 if (getProgramEligibility().isEmpty()) { 3327 addProgramEligibility(); 3328 } 3329 return getProgramEligibility().get(0); 3330 } 3331 3332 /** 3333 * @return {@link #fundingSource} (Indicates the source of the vaccine actually 3334 * administered. This may be different than the patient eligibility 3335 * (e.g. the patient may be eligible for a publically purchased vaccine 3336 * but due to inventory issues, vaccine purchased with private funds was 3337 * actually administered).) 3338 */ 3339 public CodeableConcept getFundingSource() { 3340 if (this.fundingSource == null) 3341 if (Configuration.errorOnAutoCreate()) 3342 throw new Error("Attempt to auto-create Immunization.fundingSource"); 3343 else if (Configuration.doAutoCreate()) 3344 this.fundingSource = new CodeableConcept(); // cc 3345 return this.fundingSource; 3346 } 3347 3348 public boolean hasFundingSource() { 3349 return this.fundingSource != null && !this.fundingSource.isEmpty(); 3350 } 3351 3352 /** 3353 * @param value {@link #fundingSource} (Indicates the source of the vaccine 3354 * actually administered. This may be different than the patient 3355 * eligibility (e.g. the patient may be eligible for a publically 3356 * purchased vaccine but due to inventory issues, vaccine purchased 3357 * with private funds was actually administered).) 3358 */ 3359 public Immunization setFundingSource(CodeableConcept value) { 3360 this.fundingSource = value; 3361 return this; 3362 } 3363 3364 /** 3365 * @return {@link #reaction} (Categorical data indicating that an adverse event 3366 * is associated in time to an immunization.) 3367 */ 3368 public List<ImmunizationReactionComponent> getReaction() { 3369 if (this.reaction == null) 3370 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3371 return this.reaction; 3372 } 3373 3374 /** 3375 * @return Returns a reference to <code>this</code> for easy method chaining 3376 */ 3377 public Immunization setReaction(List<ImmunizationReactionComponent> theReaction) { 3378 this.reaction = theReaction; 3379 return this; 3380 } 3381 3382 public boolean hasReaction() { 3383 if (this.reaction == null) 3384 return false; 3385 for (ImmunizationReactionComponent item : this.reaction) 3386 if (!item.isEmpty()) 3387 return true; 3388 return false; 3389 } 3390 3391 public ImmunizationReactionComponent addReaction() { // 3 3392 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 3393 if (this.reaction == null) 3394 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3395 this.reaction.add(t); 3396 return t; 3397 } 3398 3399 public Immunization addReaction(ImmunizationReactionComponent t) { // 3 3400 if (t == null) 3401 return this; 3402 if (this.reaction == null) 3403 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 3404 this.reaction.add(t); 3405 return this; 3406 } 3407 3408 /** 3409 * @return The first repetition of repeating field {@link #reaction}, creating 3410 * it if it does not already exist 3411 */ 3412 public ImmunizationReactionComponent getReactionFirstRep() { 3413 if (getReaction().isEmpty()) { 3414 addReaction(); 3415 } 3416 return getReaction().get(0); 3417 } 3418 3419 /** 3420 * @return {@link #protocolApplied} (The protocol (set of recommendations) being 3421 * followed by the provider who administered the dose.) 3422 */ 3423 public List<ImmunizationProtocolAppliedComponent> getProtocolApplied() { 3424 if (this.protocolApplied == null) 3425 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3426 return this.protocolApplied; 3427 } 3428 3429 /** 3430 * @return Returns a reference to <code>this</code> for easy method chaining 3431 */ 3432 public Immunization setProtocolApplied(List<ImmunizationProtocolAppliedComponent> theProtocolApplied) { 3433 this.protocolApplied = theProtocolApplied; 3434 return this; 3435 } 3436 3437 public boolean hasProtocolApplied() { 3438 if (this.protocolApplied == null) 3439 return false; 3440 for (ImmunizationProtocolAppliedComponent item : this.protocolApplied) 3441 if (!item.isEmpty()) 3442 return true; 3443 return false; 3444 } 3445 3446 public ImmunizationProtocolAppliedComponent addProtocolApplied() { // 3 3447 ImmunizationProtocolAppliedComponent t = new ImmunizationProtocolAppliedComponent(); 3448 if (this.protocolApplied == null) 3449 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3450 this.protocolApplied.add(t); 3451 return t; 3452 } 3453 3454 public Immunization addProtocolApplied(ImmunizationProtocolAppliedComponent t) { // 3 3455 if (t == null) 3456 return this; 3457 if (this.protocolApplied == null) 3458 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3459 this.protocolApplied.add(t); 3460 return this; 3461 } 3462 3463 /** 3464 * @return The first repetition of repeating field {@link #protocolApplied}, 3465 * creating it if it does not already exist 3466 */ 3467 public ImmunizationProtocolAppliedComponent getProtocolAppliedFirstRep() { 3468 if (getProtocolApplied().isEmpty()) { 3469 addProtocolApplied(); 3470 } 3471 return getProtocolApplied().get(0); 3472 } 3473 3474 protected void listChildren(List<Property> children) { 3475 super.listChildren(children); 3476 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 3477 0, java.lang.Integer.MAX_VALUE, identifier)); 3478 children 3479 .add(new Property("status", "code", "Indicates the current status of the immunization event.", 0, 1, status)); 3480 children.add(new Property("statusReason", "CodeableConcept", 3481 "Indicates the reason the immunization event was not performed.", 0, 1, statusReason)); 3482 children.add(new Property("vaccineCode", "CodeableConcept", 3483 "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode)); 3484 children.add(new Property("patient", "Reference(Patient)", 3485 "The patient who either received or did not receive the immunization.", 0, 1, patient)); 3486 children.add(new Property("encounter", "Reference(Encounter)", 3487 "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 3488 0, 1, encounter)); 3489 children.add(new Property("occurrence[x]", "dateTime|string", 3490 "Date vaccine administered or was to be administered.", 0, 1, occurrence)); 3491 children.add(new Property("recorded", "dateTime", 3492 "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.", 3493 0, 1, recorded)); 3494 children.add(new Property("primarySource", "boolean", 3495 "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 3496 0, 1, primarySource)); 3497 children.add(new Property("reportOrigin", "CodeableConcept", 3498 "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 3499 0, 1, reportOrigin)); 3500 children.add(new Property("location", "Reference(Location)", 3501 "The service delivery location where the vaccine administration occurred.", 0, 1, location)); 3502 children.add( 3503 new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer)); 3504 children.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber)); 3505 children.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate)); 3506 children.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site)); 3507 children.add(new Property("route", "CodeableConcept", 3508 "The path by which the vaccine product is taken into the body.", 0, 1, route)); 3509 children.add(new Property("doseQuantity", "SimpleQuantity", 3510 "The quantity of vaccine product that was administered.", 0, 1, doseQuantity)); 3511 children.add(new Property("performer", "", "Indicates who performed the immunization event.", 0, 3512 java.lang.Integer.MAX_VALUE, performer)); 3513 children.add(new Property("note", "Annotation", 3514 "Extra information about the immunization that is not conveyed by the other attributes.", 0, 3515 java.lang.Integer.MAX_VALUE, note)); 3516 children.add(new Property("reasonCode", "CodeableConcept", "Reasons why the vaccine was administered.", 0, 3517 java.lang.Integer.MAX_VALUE, reasonCode)); 3518 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 3519 "Condition, Observation or DiagnosticReport that supports why the immunization was administered.", 0, 3520 java.lang.Integer.MAX_VALUE, reasonReference)); 3521 children.add(new Property("isSubpotent", "boolean", 3522 "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 0, 3523 1, isSubpotent)); 3524 children.add(new Property("subpotentReason", "CodeableConcept", "Reason why a dose is considered to be subpotent.", 3525 0, java.lang.Integer.MAX_VALUE, subpotentReason)); 3526 children.add(new Property("education", "", 3527 "Educational material presented to the patient (or guardian) at the time of vaccine administration.", 0, 3528 java.lang.Integer.MAX_VALUE, education)); 3529 children.add(new Property("programEligibility", "CodeableConcept", 3530 "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, 3531 programEligibility)); 3532 children.add(new Property("fundingSource", "CodeableConcept", 3533 "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 3534 0, 1, fundingSource)); 3535 children.add(new Property("reaction", "", 3536 "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, 3537 java.lang.Integer.MAX_VALUE, reaction)); 3538 children.add(new Property("protocolApplied", "", 3539 "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, 3540 java.lang.Integer.MAX_VALUE, protocolApplied)); 3541 } 3542 3543 @Override 3544 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3545 switch (_hash) { 3546 case -1618432855: 3547 /* identifier */ return new Property("identifier", "Identifier", 3548 "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier); 3549 case -892481550: 3550 /* status */ return new Property("status", "code", "Indicates the current status of the immunization event.", 0, 3551 1, status); 3552 case 2051346646: 3553 /* statusReason */ return new Property("statusReason", "CodeableConcept", 3554 "Indicates the reason the immunization event was not performed.", 0, 1, statusReason); 3555 case 664556354: 3556 /* vaccineCode */ return new Property("vaccineCode", "CodeableConcept", 3557 "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode); 3558 case -791418107: 3559 /* patient */ return new Property("patient", "Reference(Patient)", 3560 "The patient who either received or did not receive the immunization.", 0, 1, patient); 3561 case 1524132147: 3562 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3563 "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 3564 0, 1, encounter); 3565 case -2022646513: 3566 /* occurrence[x] */ return new Property("occurrence[x]", "dateTime|string", 3567 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3568 case 1687874001: 3569 /* occurrence */ return new Property("occurrence[x]", "dateTime|string", 3570 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3571 case -298443636: 3572 /* occurrenceDateTime */ return new Property("occurrence[x]", "dateTime|string", 3573 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3574 case 1496896834: 3575 /* occurrenceString */ return new Property("occurrence[x]", "dateTime|string", 3576 "Date vaccine administered or was to be administered.", 0, 1, occurrence); 3577 case -799233872: 3578 /* recorded */ return new Property("recorded", "dateTime", 3579 "The date the occurrence of the immunization was first captured in the record - potentially significantly after the occurrence of the event.", 3580 0, 1, recorded); 3581 case -528721731: 3582 /* primarySource */ return new Property("primarySource", "boolean", 3583 "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 3584 0, 1, primarySource); 3585 case 486750586: 3586 /* reportOrigin */ return new Property("reportOrigin", "CodeableConcept", 3587 "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 3588 0, 1, reportOrigin); 3589 case 1901043637: 3590 /* location */ return new Property("location", "Reference(Location)", 3591 "The service delivery location where the vaccine administration occurred.", 0, 1, location); 3592 case -1969347631: 3593 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 3594 0, 1, manufacturer); 3595 case 462547450: 3596 /* lotNumber */ return new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, 3597 lotNumber); 3598 case -668811523: 3599 /* expirationDate */ return new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, 3600 expirationDate); 3601 case 3530567: 3602 /* site */ return new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, 3603 site); 3604 case 108704329: 3605 /* route */ return new Property("route", "CodeableConcept", 3606 "The path by which the vaccine product is taken into the body.", 0, 1, route); 3607 case -2083618872: 3608 /* doseQuantity */ return new Property("doseQuantity", "SimpleQuantity", 3609 "The quantity of vaccine product that was administered.", 0, 1, doseQuantity); 3610 case 481140686: 3611 /* performer */ return new Property("performer", "", "Indicates who performed the immunization event.", 0, 3612 java.lang.Integer.MAX_VALUE, performer); 3613 case 3387378: 3614 /* note */ return new Property("note", "Annotation", 3615 "Extra information about the immunization that is not conveyed by the other attributes.", 0, 3616 java.lang.Integer.MAX_VALUE, note); 3617 case 722137681: 3618 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", "Reasons why the vaccine was administered.", 3619 0, java.lang.Integer.MAX_VALUE, reasonCode); 3620 case -1146218137: 3621 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 3622 "Condition, Observation or DiagnosticReport that supports why the immunization was administered.", 0, 3623 java.lang.Integer.MAX_VALUE, reasonReference); 3624 case 1618512556: 3625 /* isSubpotent */ return new Property("isSubpotent", "boolean", 3626 "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 3627 0, 1, isSubpotent); 3628 case 805168794: 3629 /* subpotentReason */ return new Property("subpotentReason", "CodeableConcept", 3630 "Reason why a dose is considered to be subpotent.", 0, java.lang.Integer.MAX_VALUE, subpotentReason); 3631 case -290756696: 3632 /* education */ return new Property("education", "", 3633 "Educational material presented to the patient (or guardian) at the time of vaccine administration.", 0, 3634 java.lang.Integer.MAX_VALUE, education); 3635 case 1207530089: 3636 /* programEligibility */ return new Property("programEligibility", "CodeableConcept", 3637 "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, 3638 programEligibility); 3639 case 1120150904: 3640 /* fundingSource */ return new Property("fundingSource", "CodeableConcept", 3641 "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 3642 0, 1, fundingSource); 3643 case -867509719: 3644 /* reaction */ return new Property("reaction", "", 3645 "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, 3646 java.lang.Integer.MAX_VALUE, reaction); 3647 case 607985349: 3648 /* protocolApplied */ return new Property("protocolApplied", "", 3649 "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, 3650 java.lang.Integer.MAX_VALUE, protocolApplied); 3651 default: 3652 return super.getNamedProperty(_hash, _name, _checkValid); 3653 } 3654 3655 } 3656 3657 @Override 3658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3659 switch (hash) { 3660 case -1618432855: 3661 /* identifier */ return this.identifier == null ? new Base[0] 3662 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3663 case -892481550: 3664 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ImmunizationStatus> 3665 case 2051346646: 3666 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 3667 case 664556354: 3668 /* vaccineCode */ return this.vaccineCode == null ? new Base[0] : new Base[] { this.vaccineCode }; // CodeableConcept 3669 case -791418107: 3670 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 3671 case 1524132147: 3672 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3673 case 1687874001: 3674 /* occurrence */ return this.occurrence == null ? new Base[0] : new Base[] { this.occurrence }; // Type 3675 case -799233872: 3676 /* recorded */ return this.recorded == null ? new Base[0] : new Base[] { this.recorded }; // DateTimeType 3677 case -528721731: 3678 /* primarySource */ return this.primarySource == null ? new Base[0] : new Base[] { this.primarySource }; // BooleanType 3679 case 486750586: 3680 /* reportOrigin */ return this.reportOrigin == null ? new Base[0] : new Base[] { this.reportOrigin }; // CodeableConcept 3681 case 1901043637: 3682 /* location */ return this.location == null ? new Base[0] : new Base[] { this.location }; // Reference 3683 case -1969347631: 3684 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Reference 3685 case 462547450: 3686 /* lotNumber */ return this.lotNumber == null ? new Base[0] : new Base[] { this.lotNumber }; // StringType 3687 case -668811523: 3688 /* expirationDate */ return this.expirationDate == null ? new Base[0] : new Base[] { this.expirationDate }; // DateType 3689 case 3530567: 3690 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // CodeableConcept 3691 case 108704329: 3692 /* route */ return this.route == null ? new Base[0] : new Base[] { this.route }; // CodeableConcept 3693 case -2083618872: 3694 /* doseQuantity */ return this.doseQuantity == null ? new Base[0] : new Base[] { this.doseQuantity }; // Quantity 3695 case 481140686: 3696 /* performer */ return this.performer == null ? new Base[0] 3697 : this.performer.toArray(new Base[this.performer.size()]); // ImmunizationPerformerComponent 3698 case 3387378: 3699 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3700 case 722137681: 3701 /* reasonCode */ return this.reasonCode == null ? new Base[0] 3702 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 3703 case -1146218137: 3704 /* reasonReference */ return this.reasonReference == null ? new Base[0] 3705 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 3706 case 1618512556: 3707 /* isSubpotent */ return this.isSubpotent == null ? new Base[0] : new Base[] { this.isSubpotent }; // BooleanType 3708 case 805168794: 3709 /* subpotentReason */ return this.subpotentReason == null ? new Base[0] 3710 : this.subpotentReason.toArray(new Base[this.subpotentReason.size()]); // CodeableConcept 3711 case -290756696: 3712 /* education */ return this.education == null ? new Base[0] 3713 : this.education.toArray(new Base[this.education.size()]); // ImmunizationEducationComponent 3714 case 1207530089: 3715 /* programEligibility */ return this.programEligibility == null ? new Base[0] 3716 : this.programEligibility.toArray(new Base[this.programEligibility.size()]); // CodeableConcept 3717 case 1120150904: 3718 /* fundingSource */ return this.fundingSource == null ? new Base[0] : new Base[] { this.fundingSource }; // CodeableConcept 3719 case -867509719: 3720 /* reaction */ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // ImmunizationReactionComponent 3721 case 607985349: 3722 /* protocolApplied */ return this.protocolApplied == null ? new Base[0] 3723 : this.protocolApplied.toArray(new Base[this.protocolApplied.size()]); // ImmunizationProtocolAppliedComponent 3724 default: 3725 return super.getProperty(hash, name, checkValid); 3726 } 3727 3728 } 3729 3730 @Override 3731 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3732 switch (hash) { 3733 case -1618432855: // identifier 3734 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3735 return value; 3736 case -892481550: // status 3737 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 3738 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 3739 return value; 3740 case 2051346646: // statusReason 3741 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3742 return value; 3743 case 664556354: // vaccineCode 3744 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 3745 return value; 3746 case -791418107: // patient 3747 this.patient = castToReference(value); // Reference 3748 return value; 3749 case 1524132147: // encounter 3750 this.encounter = castToReference(value); // Reference 3751 return value; 3752 case 1687874001: // occurrence 3753 this.occurrence = castToType(value); // Type 3754 return value; 3755 case -799233872: // recorded 3756 this.recorded = castToDateTime(value); // DateTimeType 3757 return value; 3758 case -528721731: // primarySource 3759 this.primarySource = castToBoolean(value); // BooleanType 3760 return value; 3761 case 486750586: // reportOrigin 3762 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 3763 return value; 3764 case 1901043637: // location 3765 this.location = castToReference(value); // Reference 3766 return value; 3767 case -1969347631: // manufacturer 3768 this.manufacturer = castToReference(value); // Reference 3769 return value; 3770 case 462547450: // lotNumber 3771 this.lotNumber = castToString(value); // StringType 3772 return value; 3773 case -668811523: // expirationDate 3774 this.expirationDate = castToDate(value); // DateType 3775 return value; 3776 case 3530567: // site 3777 this.site = castToCodeableConcept(value); // CodeableConcept 3778 return value; 3779 case 108704329: // route 3780 this.route = castToCodeableConcept(value); // CodeableConcept 3781 return value; 3782 case -2083618872: // doseQuantity 3783 this.doseQuantity = castToQuantity(value); // Quantity 3784 return value; 3785 case 481140686: // performer 3786 this.getPerformer().add((ImmunizationPerformerComponent) value); // ImmunizationPerformerComponent 3787 return value; 3788 case 3387378: // note 3789 this.getNote().add(castToAnnotation(value)); // Annotation 3790 return value; 3791 case 722137681: // reasonCode 3792 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 3793 return value; 3794 case -1146218137: // reasonReference 3795 this.getReasonReference().add(castToReference(value)); // Reference 3796 return value; 3797 case 1618512556: // isSubpotent 3798 this.isSubpotent = castToBoolean(value); // BooleanType 3799 return value; 3800 case 805168794: // subpotentReason 3801 this.getSubpotentReason().add(castToCodeableConcept(value)); // CodeableConcept 3802 return value; 3803 case -290756696: // education 3804 this.getEducation().add((ImmunizationEducationComponent) value); // ImmunizationEducationComponent 3805 return value; 3806 case 1207530089: // programEligibility 3807 this.getProgramEligibility().add(castToCodeableConcept(value)); // CodeableConcept 3808 return value; 3809 case 1120150904: // fundingSource 3810 this.fundingSource = castToCodeableConcept(value); // CodeableConcept 3811 return value; 3812 case -867509719: // reaction 3813 this.getReaction().add((ImmunizationReactionComponent) value); // ImmunizationReactionComponent 3814 return value; 3815 case 607985349: // protocolApplied 3816 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); // ImmunizationProtocolAppliedComponent 3817 return value; 3818 default: 3819 return super.setProperty(hash, name, value); 3820 } 3821 3822 } 3823 3824 @Override 3825 public Base setProperty(String name, Base value) throws FHIRException { 3826 if (name.equals("identifier")) { 3827 this.getIdentifier().add(castToIdentifier(value)); 3828 } else if (name.equals("status")) { 3829 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 3830 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 3831 } else if (name.equals("statusReason")) { 3832 this.statusReason = castToCodeableConcept(value); // CodeableConcept 3833 } else if (name.equals("vaccineCode")) { 3834 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 3835 } else if (name.equals("patient")) { 3836 this.patient = castToReference(value); // Reference 3837 } else if (name.equals("encounter")) { 3838 this.encounter = castToReference(value); // Reference 3839 } else if (name.equals("occurrence[x]")) { 3840 this.occurrence = castToType(value); // Type 3841 } else if (name.equals("recorded")) { 3842 this.recorded = castToDateTime(value); // DateTimeType 3843 } else if (name.equals("primarySource")) { 3844 this.primarySource = castToBoolean(value); // BooleanType 3845 } else if (name.equals("reportOrigin")) { 3846 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 3847 } else if (name.equals("location")) { 3848 this.location = castToReference(value); // Reference 3849 } else if (name.equals("manufacturer")) { 3850 this.manufacturer = castToReference(value); // Reference 3851 } else if (name.equals("lotNumber")) { 3852 this.lotNumber = castToString(value); // StringType 3853 } else if (name.equals("expirationDate")) { 3854 this.expirationDate = castToDate(value); // DateType 3855 } else if (name.equals("site")) { 3856 this.site = castToCodeableConcept(value); // CodeableConcept 3857 } else if (name.equals("route")) { 3858 this.route = castToCodeableConcept(value); // CodeableConcept 3859 } else if (name.equals("doseQuantity")) { 3860 this.doseQuantity = castToQuantity(value); // Quantity 3861 } else if (name.equals("performer")) { 3862 this.getPerformer().add((ImmunizationPerformerComponent) value); 3863 } else if (name.equals("note")) { 3864 this.getNote().add(castToAnnotation(value)); 3865 } else if (name.equals("reasonCode")) { 3866 this.getReasonCode().add(castToCodeableConcept(value)); 3867 } else if (name.equals("reasonReference")) { 3868 this.getReasonReference().add(castToReference(value)); 3869 } else if (name.equals("isSubpotent")) { 3870 this.isSubpotent = castToBoolean(value); // BooleanType 3871 } else if (name.equals("subpotentReason")) { 3872 this.getSubpotentReason().add(castToCodeableConcept(value)); 3873 } else if (name.equals("education")) { 3874 this.getEducation().add((ImmunizationEducationComponent) value); 3875 } else if (name.equals("programEligibility")) { 3876 this.getProgramEligibility().add(castToCodeableConcept(value)); 3877 } else if (name.equals("fundingSource")) { 3878 this.fundingSource = castToCodeableConcept(value); // CodeableConcept 3879 } else if (name.equals("reaction")) { 3880 this.getReaction().add((ImmunizationReactionComponent) value); 3881 } else if (name.equals("protocolApplied")) { 3882 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); 3883 } else 3884 return super.setProperty(name, value); 3885 return value; 3886 } 3887 3888 @Override 3889 public Base makeProperty(int hash, String name) throws FHIRException { 3890 switch (hash) { 3891 case -1618432855: 3892 return addIdentifier(); 3893 case -892481550: 3894 return getStatusElement(); 3895 case 2051346646: 3896 return getStatusReason(); 3897 case 664556354: 3898 return getVaccineCode(); 3899 case -791418107: 3900 return getPatient(); 3901 case 1524132147: 3902 return getEncounter(); 3903 case -2022646513: 3904 return getOccurrence(); 3905 case 1687874001: 3906 return getOccurrence(); 3907 case -799233872: 3908 return getRecordedElement(); 3909 case -528721731: 3910 return getPrimarySourceElement(); 3911 case 486750586: 3912 return getReportOrigin(); 3913 case 1901043637: 3914 return getLocation(); 3915 case -1969347631: 3916 return getManufacturer(); 3917 case 462547450: 3918 return getLotNumberElement(); 3919 case -668811523: 3920 return getExpirationDateElement(); 3921 case 3530567: 3922 return getSite(); 3923 case 108704329: 3924 return getRoute(); 3925 case -2083618872: 3926 return getDoseQuantity(); 3927 case 481140686: 3928 return addPerformer(); 3929 case 3387378: 3930 return addNote(); 3931 case 722137681: 3932 return addReasonCode(); 3933 case -1146218137: 3934 return addReasonReference(); 3935 case 1618512556: 3936 return getIsSubpotentElement(); 3937 case 805168794: 3938 return addSubpotentReason(); 3939 case -290756696: 3940 return addEducation(); 3941 case 1207530089: 3942 return addProgramEligibility(); 3943 case 1120150904: 3944 return getFundingSource(); 3945 case -867509719: 3946 return addReaction(); 3947 case 607985349: 3948 return addProtocolApplied(); 3949 default: 3950 return super.makeProperty(hash, name); 3951 } 3952 3953 } 3954 3955 @Override 3956 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3957 switch (hash) { 3958 case -1618432855: 3959 /* identifier */ return new String[] { "Identifier" }; 3960 case -892481550: 3961 /* status */ return new String[] { "code" }; 3962 case 2051346646: 3963 /* statusReason */ return new String[] { "CodeableConcept" }; 3964 case 664556354: 3965 /* vaccineCode */ return new String[] { "CodeableConcept" }; 3966 case -791418107: 3967 /* patient */ return new String[] { "Reference" }; 3968 case 1524132147: 3969 /* encounter */ return new String[] { "Reference" }; 3970 case 1687874001: 3971 /* occurrence */ return new String[] { "dateTime", "string" }; 3972 case -799233872: 3973 /* recorded */ return new String[] { "dateTime" }; 3974 case -528721731: 3975 /* primarySource */ return new String[] { "boolean" }; 3976 case 486750586: 3977 /* reportOrigin */ return new String[] { "CodeableConcept" }; 3978 case 1901043637: 3979 /* location */ return new String[] { "Reference" }; 3980 case -1969347631: 3981 /* manufacturer */ return new String[] { "Reference" }; 3982 case 462547450: 3983 /* lotNumber */ return new String[] { "string" }; 3984 case -668811523: 3985 /* expirationDate */ return new String[] { "date" }; 3986 case 3530567: 3987 /* site */ return new String[] { "CodeableConcept" }; 3988 case 108704329: 3989 /* route */ return new String[] { "CodeableConcept" }; 3990 case -2083618872: 3991 /* doseQuantity */ return new String[] { "SimpleQuantity" }; 3992 case 481140686: 3993 /* performer */ return new String[] {}; 3994 case 3387378: 3995 /* note */ return new String[] { "Annotation" }; 3996 case 722137681: 3997 /* reasonCode */ return new String[] { "CodeableConcept" }; 3998 case -1146218137: 3999 /* reasonReference */ return new String[] { "Reference" }; 4000 case 1618512556: 4001 /* isSubpotent */ return new String[] { "boolean" }; 4002 case 805168794: 4003 /* subpotentReason */ return new String[] { "CodeableConcept" }; 4004 case -290756696: 4005 /* education */ return new String[] {}; 4006 case 1207530089: 4007 /* programEligibility */ return new String[] { "CodeableConcept" }; 4008 case 1120150904: 4009 /* fundingSource */ return new String[] { "CodeableConcept" }; 4010 case -867509719: 4011 /* reaction */ return new String[] {}; 4012 case 607985349: 4013 /* protocolApplied */ return new String[] {}; 4014 default: 4015 return super.getTypesForProperty(hash, name); 4016 } 4017 4018 } 4019 4020 @Override 4021 public Base addChild(String name) throws FHIRException { 4022 if (name.equals("identifier")) { 4023 return addIdentifier(); 4024 } else if (name.equals("status")) { 4025 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 4026 } else if (name.equals("statusReason")) { 4027 this.statusReason = new CodeableConcept(); 4028 return this.statusReason; 4029 } else if (name.equals("vaccineCode")) { 4030 this.vaccineCode = new CodeableConcept(); 4031 return this.vaccineCode; 4032 } else if (name.equals("patient")) { 4033 this.patient = new Reference(); 4034 return this.patient; 4035 } else if (name.equals("encounter")) { 4036 this.encounter = new Reference(); 4037 return this.encounter; 4038 } else if (name.equals("occurrenceDateTime")) { 4039 this.occurrence = new DateTimeType(); 4040 return this.occurrence; 4041 } else if (name.equals("occurrenceString")) { 4042 this.occurrence = new StringType(); 4043 return this.occurrence; 4044 } else if (name.equals("recorded")) { 4045 throw new FHIRException("Cannot call addChild on a singleton property Immunization.recorded"); 4046 } else if (name.equals("primarySource")) { 4047 throw new FHIRException("Cannot call addChild on a singleton property Immunization.primarySource"); 4048 } else if (name.equals("reportOrigin")) { 4049 this.reportOrigin = new CodeableConcept(); 4050 return this.reportOrigin; 4051 } else if (name.equals("location")) { 4052 this.location = new Reference(); 4053 return this.location; 4054 } else if (name.equals("manufacturer")) { 4055 this.manufacturer = new Reference(); 4056 return this.manufacturer; 4057 } else if (name.equals("lotNumber")) { 4058 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 4059 } else if (name.equals("expirationDate")) { 4060 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 4061 } else if (name.equals("site")) { 4062 this.site = new CodeableConcept(); 4063 return this.site; 4064 } else if (name.equals("route")) { 4065 this.route = new CodeableConcept(); 4066 return this.route; 4067 } else if (name.equals("doseQuantity")) { 4068 this.doseQuantity = new Quantity(); 4069 return this.doseQuantity; 4070 } else if (name.equals("performer")) { 4071 return addPerformer(); 4072 } else if (name.equals("note")) { 4073 return addNote(); 4074 } else if (name.equals("reasonCode")) { 4075 return addReasonCode(); 4076 } else if (name.equals("reasonReference")) { 4077 return addReasonReference(); 4078 } else if (name.equals("isSubpotent")) { 4079 throw new FHIRException("Cannot call addChild on a singleton property Immunization.isSubpotent"); 4080 } else if (name.equals("subpotentReason")) { 4081 return addSubpotentReason(); 4082 } else if (name.equals("education")) { 4083 return addEducation(); 4084 } else if (name.equals("programEligibility")) { 4085 return addProgramEligibility(); 4086 } else if (name.equals("fundingSource")) { 4087 this.fundingSource = new CodeableConcept(); 4088 return this.fundingSource; 4089 } else if (name.equals("reaction")) { 4090 return addReaction(); 4091 } else if (name.equals("protocolApplied")) { 4092 return addProtocolApplied(); 4093 } else 4094 return super.addChild(name); 4095 } 4096 4097 public String fhirType() { 4098 return "Immunization"; 4099 4100 } 4101 4102 public Immunization copy() { 4103 Immunization dst = new Immunization(); 4104 copyValues(dst); 4105 return dst; 4106 } 4107 4108 public void copyValues(Immunization dst) { 4109 super.copyValues(dst); 4110 if (identifier != null) { 4111 dst.identifier = new ArrayList<Identifier>(); 4112 for (Identifier i : identifier) 4113 dst.identifier.add(i.copy()); 4114 } 4115 ; 4116 dst.status = status == null ? null : status.copy(); 4117 dst.statusReason = statusReason == null ? null : statusReason.copy(); 4118 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 4119 dst.patient = patient == null ? null : patient.copy(); 4120 dst.encounter = encounter == null ? null : encounter.copy(); 4121 dst.occurrence = occurrence == null ? null : occurrence.copy(); 4122 dst.recorded = recorded == null ? null : recorded.copy(); 4123 dst.primarySource = primarySource == null ? null : primarySource.copy(); 4124 dst.reportOrigin = reportOrigin == null ? null : reportOrigin.copy(); 4125 dst.location = location == null ? null : location.copy(); 4126 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 4127 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 4128 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 4129 dst.site = site == null ? null : site.copy(); 4130 dst.route = route == null ? null : route.copy(); 4131 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 4132 if (performer != null) { 4133 dst.performer = new ArrayList<ImmunizationPerformerComponent>(); 4134 for (ImmunizationPerformerComponent i : performer) 4135 dst.performer.add(i.copy()); 4136 } 4137 ; 4138 if (note != null) { 4139 dst.note = new ArrayList<Annotation>(); 4140 for (Annotation i : note) 4141 dst.note.add(i.copy()); 4142 } 4143 ; 4144 if (reasonCode != null) { 4145 dst.reasonCode = new ArrayList<CodeableConcept>(); 4146 for (CodeableConcept i : reasonCode) 4147 dst.reasonCode.add(i.copy()); 4148 } 4149 ; 4150 if (reasonReference != null) { 4151 dst.reasonReference = new ArrayList<Reference>(); 4152 for (Reference i : reasonReference) 4153 dst.reasonReference.add(i.copy()); 4154 } 4155 ; 4156 dst.isSubpotent = isSubpotent == null ? null : isSubpotent.copy(); 4157 if (subpotentReason != null) { 4158 dst.subpotentReason = new ArrayList<CodeableConcept>(); 4159 for (CodeableConcept i : subpotentReason) 4160 dst.subpotentReason.add(i.copy()); 4161 } 4162 ; 4163 if (education != null) { 4164 dst.education = new ArrayList<ImmunizationEducationComponent>(); 4165 for (ImmunizationEducationComponent i : education) 4166 dst.education.add(i.copy()); 4167 } 4168 ; 4169 if (programEligibility != null) { 4170 dst.programEligibility = new ArrayList<CodeableConcept>(); 4171 for (CodeableConcept i : programEligibility) 4172 dst.programEligibility.add(i.copy()); 4173 } 4174 ; 4175 dst.fundingSource = fundingSource == null ? null : fundingSource.copy(); 4176 if (reaction != null) { 4177 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 4178 for (ImmunizationReactionComponent i : reaction) 4179 dst.reaction.add(i.copy()); 4180 } 4181 ; 4182 if (protocolApplied != null) { 4183 dst.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 4184 for (ImmunizationProtocolAppliedComponent i : protocolApplied) 4185 dst.protocolApplied.add(i.copy()); 4186 } 4187 ; 4188 } 4189 4190 protected Immunization typedCopy() { 4191 return copy(); 4192 } 4193 4194 @Override 4195 public boolean equalsDeep(Base other_) { 4196 if (!super.equalsDeep(other_)) 4197 return false; 4198 if (!(other_ instanceof Immunization)) 4199 return false; 4200 Immunization o = (Immunization) other_; 4201 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4202 && compareDeep(statusReason, o.statusReason, true) && compareDeep(vaccineCode, o.vaccineCode, true) 4203 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) 4204 && compareDeep(occurrence, o.occurrence, true) && compareDeep(recorded, o.recorded, true) 4205 && compareDeep(primarySource, o.primarySource, true) && compareDeep(reportOrigin, o.reportOrigin, true) 4206 && compareDeep(location, o.location, true) && compareDeep(manufacturer, o.manufacturer, true) 4207 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 4208 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 4209 && compareDeep(doseQuantity, o.doseQuantity, true) && compareDeep(performer, o.performer, true) 4210 && compareDeep(note, o.note, true) && compareDeep(reasonCode, o.reasonCode, true) 4211 && compareDeep(reasonReference, o.reasonReference, true) && compareDeep(isSubpotent, o.isSubpotent, true) 4212 && compareDeep(subpotentReason, o.subpotentReason, true) && compareDeep(education, o.education, true) 4213 && compareDeep(programEligibility, o.programEligibility, true) 4214 && compareDeep(fundingSource, o.fundingSource, true) && compareDeep(reaction, o.reaction, true) 4215 && compareDeep(protocolApplied, o.protocolApplied, true); 4216 } 4217 4218 @Override 4219 public boolean equalsShallow(Base other_) { 4220 if (!super.equalsShallow(other_)) 4221 return false; 4222 if (!(other_ instanceof Immunization)) 4223 return false; 4224 Immunization o = (Immunization) other_; 4225 return compareValues(status, o.status, true) && compareValues(recorded, o.recorded, true) 4226 && compareValues(primarySource, o.primarySource, true) && compareValues(lotNumber, o.lotNumber, true) 4227 && compareValues(expirationDate, o.expirationDate, true) && compareValues(isSubpotent, o.isSubpotent, true); 4228 } 4229 4230 public boolean isEmpty() { 4231 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason, vaccineCode, 4232 patient, encounter, occurrence, recorded, primarySource, reportOrigin, location, manufacturer, lotNumber, 4233 expirationDate, site, route, doseQuantity, performer, note, reasonCode, reasonReference, isSubpotent, 4234 subpotentReason, education, programEligibility, fundingSource, reaction, protocolApplied); 4235 } 4236 4237 @Override 4238 public ResourceType getResourceType() { 4239 return ResourceType.Immunization; 4240 } 4241 4242 /** 4243 * Search parameter: <b>date</b> 4244 * <p> 4245 * Description: <b>Vaccination (non)-Administration Date</b><br> 4246 * Type: <b>date</b><br> 4247 * Path: <b>Immunization.occurrence[x]</b><br> 4248 * </p> 4249 */ 4250 @SearchParamDefinition(name = "date", path = "Immunization.occurrence", description = "Vaccination (non)-Administration Date", type = "date") 4251 public static final String SP_DATE = "date"; 4252 /** 4253 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4254 * <p> 4255 * Description: <b>Vaccination (non)-Administration Date</b><br> 4256 * Type: <b>date</b><br> 4257 * Path: <b>Immunization.occurrence[x]</b><br> 4258 * </p> 4259 */ 4260 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4261 SP_DATE); 4262 4263 /** 4264 * Search parameter: <b>identifier</b> 4265 * <p> 4266 * Description: <b>Business identifier</b><br> 4267 * Type: <b>token</b><br> 4268 * Path: <b>Immunization.identifier</b><br> 4269 * </p> 4270 */ 4271 @SearchParamDefinition(name = "identifier", path = "Immunization.identifier", description = "Business identifier", type = "token") 4272 public static final String SP_IDENTIFIER = "identifier"; 4273 /** 4274 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4275 * <p> 4276 * Description: <b>Business identifier</b><br> 4277 * Type: <b>token</b><br> 4278 * Path: <b>Immunization.identifier</b><br> 4279 * </p> 4280 */ 4281 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4282 SP_IDENTIFIER); 4283 4284 /** 4285 * Search parameter: <b>performer</b> 4286 * <p> 4287 * Description: <b>The practitioner or organization who played a role in the 4288 * vaccination</b><br> 4289 * Type: <b>reference</b><br> 4290 * Path: <b>Immunization.performer.actor</b><br> 4291 * </p> 4292 */ 4293 @SearchParamDefinition(name = "performer", path = "Immunization.performer.actor", description = "The practitioner or organization who played a role in the vaccination", type = "reference", providesMembershipIn = { 4294 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Organization.class, 4295 Practitioner.class, PractitionerRole.class }) 4296 public static final String SP_PERFORMER = "performer"; 4297 /** 4298 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4299 * <p> 4300 * Description: <b>The practitioner or organization who played a role in the 4301 * vaccination</b><br> 4302 * Type: <b>reference</b><br> 4303 * Path: <b>Immunization.performer.actor</b><br> 4304 * </p> 4305 */ 4306 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4307 SP_PERFORMER); 4308 4309 /** 4310 * Constant for fluent queries to be used to add include statements. Specifies 4311 * the path value of "<b>Immunization:performer</b>". 4312 */ 4313 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4314 "Immunization:performer").toLocked(); 4315 4316 /** 4317 * Search parameter: <b>reaction</b> 4318 * <p> 4319 * Description: <b>Additional information on reaction</b><br> 4320 * Type: <b>reference</b><br> 4321 * Path: <b>Immunization.reaction.detail</b><br> 4322 * </p> 4323 */ 4324 @SearchParamDefinition(name = "reaction", path = "Immunization.reaction.detail", description = "Additional information on reaction", type = "reference", target = { 4325 Observation.class }) 4326 public static final String SP_REACTION = "reaction"; 4327 /** 4328 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 4329 * <p> 4330 * Description: <b>Additional information on reaction</b><br> 4331 * Type: <b>reference</b><br> 4332 * Path: <b>Immunization.reaction.detail</b><br> 4333 * </p> 4334 */ 4335 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4336 SP_REACTION); 4337 4338 /** 4339 * Constant for fluent queries to be used to add include statements. Specifies 4340 * the path value of "<b>Immunization:reaction</b>". 4341 */ 4342 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include( 4343 "Immunization:reaction").toLocked(); 4344 4345 /** 4346 * Search parameter: <b>lot-number</b> 4347 * <p> 4348 * Description: <b>Vaccine Lot Number</b><br> 4349 * Type: <b>string</b><br> 4350 * Path: <b>Immunization.lotNumber</b><br> 4351 * </p> 4352 */ 4353 @SearchParamDefinition(name = "lot-number", path = "Immunization.lotNumber", description = "Vaccine Lot Number", type = "string") 4354 public static final String SP_LOT_NUMBER = "lot-number"; 4355 /** 4356 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 4357 * <p> 4358 * Description: <b>Vaccine Lot Number</b><br> 4359 * Type: <b>string</b><br> 4360 * Path: <b>Immunization.lotNumber</b><br> 4361 * </p> 4362 */ 4363 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam( 4364 SP_LOT_NUMBER); 4365 4366 /** 4367 * Search parameter: <b>status-reason</b> 4368 * <p> 4369 * Description: <b>Reason why the vaccine was not administered</b><br> 4370 * Type: <b>token</b><br> 4371 * Path: <b>Immunization.statusReason</b><br> 4372 * </p> 4373 */ 4374 @SearchParamDefinition(name = "status-reason", path = "Immunization.statusReason", description = "Reason why the vaccine was not administered", type = "token") 4375 public static final String SP_STATUS_REASON = "status-reason"; 4376 /** 4377 * <b>Fluent Client</b> search parameter constant for <b>status-reason</b> 4378 * <p> 4379 * Description: <b>Reason why the vaccine was not administered</b><br> 4380 * Type: <b>token</b><br> 4381 * Path: <b>Immunization.statusReason</b><br> 4382 * </p> 4383 */ 4384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4385 SP_STATUS_REASON); 4386 4387 /** 4388 * Search parameter: <b>reason-code</b> 4389 * <p> 4390 * Description: <b>Reason why the vaccine was administered</b><br> 4391 * Type: <b>token</b><br> 4392 * Path: <b>Immunization.reasonCode</b><br> 4393 * </p> 4394 */ 4395 @SearchParamDefinition(name = "reason-code", path = "Immunization.reasonCode", description = "Reason why the vaccine was administered", type = "token") 4396 public static final String SP_REASON_CODE = "reason-code"; 4397 /** 4398 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 4399 * <p> 4400 * Description: <b>Reason why the vaccine was administered</b><br> 4401 * Type: <b>token</b><br> 4402 * Path: <b>Immunization.reasonCode</b><br> 4403 * </p> 4404 */ 4405 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4406 SP_REASON_CODE); 4407 4408 /** 4409 * Search parameter: <b>manufacturer</b> 4410 * <p> 4411 * Description: <b>Vaccine Manufacturer</b><br> 4412 * Type: <b>reference</b><br> 4413 * Path: <b>Immunization.manufacturer</b><br> 4414 * </p> 4415 */ 4416 @SearchParamDefinition(name = "manufacturer", path = "Immunization.manufacturer", description = "Vaccine Manufacturer", type = "reference", target = { 4417 Organization.class }) 4418 public static final String SP_MANUFACTURER = "manufacturer"; 4419 /** 4420 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 4421 * <p> 4422 * Description: <b>Vaccine Manufacturer</b><br> 4423 * Type: <b>reference</b><br> 4424 * Path: <b>Immunization.manufacturer</b><br> 4425 * </p> 4426 */ 4427 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4428 SP_MANUFACTURER); 4429 4430 /** 4431 * Constant for fluent queries to be used to add include statements. Specifies 4432 * the path value of "<b>Immunization:manufacturer</b>". 4433 */ 4434 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include( 4435 "Immunization:manufacturer").toLocked(); 4436 4437 /** 4438 * Search parameter: <b>target-disease</b> 4439 * <p> 4440 * Description: <b>The target disease the dose is being administered 4441 * against</b><br> 4442 * Type: <b>token</b><br> 4443 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 4444 * </p> 4445 */ 4446 @SearchParamDefinition(name = "target-disease", path = "Immunization.protocolApplied.targetDisease", description = "The target disease the dose is being administered against", type = "token") 4447 public static final String SP_TARGET_DISEASE = "target-disease"; 4448 /** 4449 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 4450 * <p> 4451 * Description: <b>The target disease the dose is being administered 4452 * against</b><br> 4453 * Type: <b>token</b><br> 4454 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 4455 * </p> 4456 */ 4457 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4458 SP_TARGET_DISEASE); 4459 4460 /** 4461 * Search parameter: <b>patient</b> 4462 * <p> 4463 * Description: <b>The patient for the vaccination record</b><br> 4464 * Type: <b>reference</b><br> 4465 * Path: <b>Immunization.patient</b><br> 4466 * </p> 4467 */ 4468 @SearchParamDefinition(name = "patient", path = "Immunization.patient", description = "The patient for the vaccination record", type = "reference", providesMembershipIn = { 4469 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 4470 public static final String SP_PATIENT = "patient"; 4471 /** 4472 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4473 * <p> 4474 * Description: <b>The patient for the vaccination record</b><br> 4475 * Type: <b>reference</b><br> 4476 * Path: <b>Immunization.patient</b><br> 4477 * </p> 4478 */ 4479 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4480 SP_PATIENT); 4481 4482 /** 4483 * Constant for fluent queries to be used to add include statements. Specifies 4484 * the path value of "<b>Immunization:patient</b>". 4485 */ 4486 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4487 "Immunization:patient").toLocked(); 4488 4489 /** 4490 * Search parameter: <b>series</b> 4491 * <p> 4492 * Description: <b>The series being followed by the provider</b><br> 4493 * Type: <b>string</b><br> 4494 * Path: <b>Immunization.protocolApplied.series</b><br> 4495 * </p> 4496 */ 4497 @SearchParamDefinition(name = "series", path = "Immunization.protocolApplied.series", description = "The series being followed by the provider", type = "string") 4498 public static final String SP_SERIES = "series"; 4499 /** 4500 * <b>Fluent Client</b> search parameter constant for <b>series</b> 4501 * <p> 4502 * Description: <b>The series being followed by the provider</b><br> 4503 * Type: <b>string</b><br> 4504 * Path: <b>Immunization.protocolApplied.series</b><br> 4505 * </p> 4506 */ 4507 public static final ca.uhn.fhir.rest.gclient.StringClientParam SERIES = new ca.uhn.fhir.rest.gclient.StringClientParam( 4508 SP_SERIES); 4509 4510 /** 4511 * Search parameter: <b>vaccine-code</b> 4512 * <p> 4513 * Description: <b>Vaccine Product Administered</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>Immunization.vaccineCode</b><br> 4516 * </p> 4517 */ 4518 @SearchParamDefinition(name = "vaccine-code", path = "Immunization.vaccineCode", description = "Vaccine Product Administered", type = "token") 4519 public static final String SP_VACCINE_CODE = "vaccine-code"; 4520 /** 4521 * <b>Fluent Client</b> search parameter constant for <b>vaccine-code</b> 4522 * <p> 4523 * Description: <b>Vaccine Product Administered</b><br> 4524 * Type: <b>token</b><br> 4525 * Path: <b>Immunization.vaccineCode</b><br> 4526 * </p> 4527 */ 4528 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4529 SP_VACCINE_CODE); 4530 4531 /** 4532 * Search parameter: <b>reason-reference</b> 4533 * <p> 4534 * Description: <b>Why immunization occurred</b><br> 4535 * Type: <b>reference</b><br> 4536 * Path: <b>Immunization.reasonReference</b><br> 4537 * </p> 4538 */ 4539 @SearchParamDefinition(name = "reason-reference", path = "Immunization.reasonReference", description = "Why immunization occurred", type = "reference", target = { 4540 Condition.class, DiagnosticReport.class, Observation.class }) 4541 public static final String SP_REASON_REFERENCE = "reason-reference"; 4542 /** 4543 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 4544 * <p> 4545 * Description: <b>Why immunization occurred</b><br> 4546 * Type: <b>reference</b><br> 4547 * Path: <b>Immunization.reasonReference</b><br> 4548 * </p> 4549 */ 4550 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4551 SP_REASON_REFERENCE); 4552 4553 /** 4554 * Constant for fluent queries to be used to add include statements. Specifies 4555 * the path value of "<b>Immunization:reason-reference</b>". 4556 */ 4557 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include( 4558 "Immunization:reason-reference").toLocked(); 4559 4560 /** 4561 * Search parameter: <b>location</b> 4562 * <p> 4563 * Description: <b>The service delivery location or facility in which the 4564 * vaccine was / was to be administered</b><br> 4565 * Type: <b>reference</b><br> 4566 * Path: <b>Immunization.location</b><br> 4567 * </p> 4568 */ 4569 @SearchParamDefinition(name = "location", path = "Immunization.location", description = "The service delivery location or facility in which the vaccine was / was to be administered", type = "reference", target = { 4570 Location.class }) 4571 public static final String SP_LOCATION = "location"; 4572 /** 4573 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4574 * <p> 4575 * Description: <b>The service delivery location or facility in which the 4576 * vaccine was / was to be administered</b><br> 4577 * Type: <b>reference</b><br> 4578 * Path: <b>Immunization.location</b><br> 4579 * </p> 4580 */ 4581 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4582 SP_LOCATION); 4583 4584 /** 4585 * Constant for fluent queries to be used to add include statements. Specifies 4586 * the path value of "<b>Immunization:location</b>". 4587 */ 4588 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include( 4589 "Immunization:location").toLocked(); 4590 4591 /** 4592 * Search parameter: <b>status</b> 4593 * <p> 4594 * Description: <b>Immunization event status</b><br> 4595 * Type: <b>token</b><br> 4596 * Path: <b>Immunization.status</b><br> 4597 * </p> 4598 */ 4599 @SearchParamDefinition(name = "status", path = "Immunization.status", description = "Immunization event status", type = "token") 4600 public static final String SP_STATUS = "status"; 4601 /** 4602 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4603 * <p> 4604 * Description: <b>Immunization event status</b><br> 4605 * Type: <b>token</b><br> 4606 * Path: <b>Immunization.status</b><br> 4607 * </p> 4608 */ 4609 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4610 SP_STATUS); 4611 4612 /** 4613 * Search parameter: <b>reaction-date</b> 4614 * <p> 4615 * Description: <b>When reaction started</b><br> 4616 * Type: <b>date</b><br> 4617 * Path: <b>Immunization.reaction.date</b><br> 4618 * </p> 4619 */ 4620 @SearchParamDefinition(name = "reaction-date", path = "Immunization.reaction.date", description = "When reaction started", type = "date") 4621 public static final String SP_REACTION_DATE = "reaction-date"; 4622 /** 4623 * <b>Fluent Client</b> search parameter constant for <b>reaction-date</b> 4624 * <p> 4625 * Description: <b>When reaction started</b><br> 4626 * Type: <b>date</b><br> 4627 * Path: <b>Immunization.reaction.date</b><br> 4628 * </p> 4629 */ 4630 public static final ca.uhn.fhir.rest.gclient.DateClientParam REACTION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4631 SP_REACTION_DATE); 4632 4633}