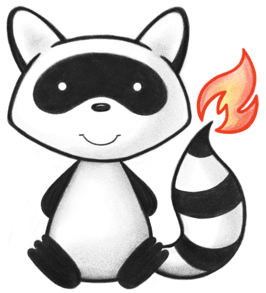
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A patient's point-in-time set of recommendations (i.e. forecasting) according 049 * to a published schedule with optional supporting justification. 050 */ 051@ResourceDef(name = "ImmunizationRecommendation", profile = "http://hl7.org/fhir/StructureDefinition/ImmunizationRecommendation") 052public class ImmunizationRecommendation extends DomainResource { 053 054 @Block() 055 public static class ImmunizationRecommendationRecommendationComponent extends BackboneElement 056 implements IBaseBackboneElement { 057 /** 058 * Vaccine(s) or vaccine group that pertain to the recommendation. 059 */ 060 @Child(name = "vaccineCode", type = { 061 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 062 @Description(shortDefinition = "Vaccine or vaccine group recommendation applies to", formalDefinition = "Vaccine(s) or vaccine group that pertain to the recommendation.") 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 064 protected List<CodeableConcept> vaccineCode; 065 066 /** 067 * The targeted disease for the recommendation. 068 */ 069 @Child(name = "targetDisease", type = { 070 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 071 @Description(shortDefinition = "Disease to be immunized against", formalDefinition = "The targeted disease for the recommendation.") 072 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-target-disease") 073 protected CodeableConcept targetDisease; 074 075 /** 076 * Vaccine(s) which should not be used to fulfill the recommendation. 077 */ 078 @Child(name = "contraindicatedVaccineCode", type = { 079 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 080 @Description(shortDefinition = "Vaccine which is contraindicated to fulfill the recommendation", formalDefinition = "Vaccine(s) which should not be used to fulfill the recommendation.") 081 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/vaccine-code") 082 protected List<CodeableConcept> contraindicatedVaccineCode; 083 084 /** 085 * Indicates the patient status with respect to the path to immunity for the 086 * target disease. 087 */ 088 @Child(name = "forecastStatus", type = { 089 CodeableConcept.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 090 @Description(shortDefinition = "Vaccine recommendation status", formalDefinition = "Indicates the patient status with respect to the path to immunity for the target disease.") 091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-status") 092 protected CodeableConcept forecastStatus; 093 094 /** 095 * The reason for the assigned forecast status. 096 */ 097 @Child(name = "forecastReason", type = { 098 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 099 @Description(shortDefinition = "Vaccine administration status reason", formalDefinition = "The reason for the assigned forecast status.") 100 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-reason") 101 protected List<CodeableConcept> forecastReason; 102 103 /** 104 * Vaccine date recommendations. For example, earliest date to administer, 105 * latest date to administer, etc. 106 */ 107 @Child(name = "dateCriterion", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 108 @Description(shortDefinition = "Dates governing proposed immunization", formalDefinition = "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.") 109 protected List<ImmunizationRecommendationRecommendationDateCriterionComponent> dateCriterion; 110 111 /** 112 * Contains the description about the protocol under which the vaccine was 113 * administered. 114 */ 115 @Child(name = "description", type = { 116 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 117 @Description(shortDefinition = "Protocol details", formalDefinition = "Contains the description about the protocol under which the vaccine was administered.") 118 protected StringType description; 119 120 /** 121 * One possible path to achieve presumed immunity against a disease - within the 122 * context of an authority. 123 */ 124 @Child(name = "series", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 125 @Description(shortDefinition = "Name of vaccination series", formalDefinition = "One possible path to achieve presumed immunity against a disease - within the context of an authority.") 126 protected StringType series; 127 128 /** 129 * Nominal position of the recommended dose in a series (e.g. dose 2 is the next 130 * recommended dose). 131 */ 132 @Child(name = "doseNumber", type = { PositiveIntType.class, 133 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 134 @Description(shortDefinition = "Recommended dose number within series", formalDefinition = "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).") 135 protected Type doseNumber; 136 137 /** 138 * The recommended number of doses to achieve immunity. 139 */ 140 @Child(name = "seriesDoses", type = { PositiveIntType.class, 141 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 142 @Description(shortDefinition = "Recommended number of doses for immunity", formalDefinition = "The recommended number of doses to achieve immunity.") 143 protected Type seriesDoses; 144 145 /** 146 * Immunization event history and/or evaluation that supports the status and 147 * recommendation. 148 */ 149 @Child(name = "supportingImmunization", type = { Immunization.class, 150 ImmunizationEvaluation.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 151 @Description(shortDefinition = "Past immunizations supporting recommendation", formalDefinition = "Immunization event history and/or evaluation that supports the status and recommendation.") 152 protected List<Reference> supportingImmunization; 153 /** 154 * The actual objects that are the target of the reference (Immunization event 155 * history and/or evaluation that supports the status and recommendation.) 156 */ 157 protected List<Resource> supportingImmunizationTarget; 158 159 /** 160 * Patient Information that supports the status and recommendation. This 161 * includes patient observations, adverse reactions and allergy/intolerance 162 * information. 163 */ 164 @Child(name = "supportingPatientInformation", type = { 165 Reference.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 166 @Description(shortDefinition = "Patient observations supporting recommendation", formalDefinition = "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.") 167 protected List<Reference> supportingPatientInformation; 168 /** 169 * The actual objects that are the target of the reference (Patient Information 170 * that supports the status and recommendation. This includes patient 171 * observations, adverse reactions and allergy/intolerance information.) 172 */ 173 protected List<Resource> supportingPatientInformationTarget; 174 175 private static final long serialVersionUID = -667399405L; 176 177 /** 178 * Constructor 179 */ 180 public ImmunizationRecommendationRecommendationComponent() { 181 super(); 182 } 183 184 /** 185 * Constructor 186 */ 187 public ImmunizationRecommendationRecommendationComponent(CodeableConcept forecastStatus) { 188 super(); 189 this.forecastStatus = forecastStatus; 190 } 191 192 /** 193 * @return {@link #vaccineCode} (Vaccine(s) or vaccine group that pertain to the 194 * recommendation.) 195 */ 196 public List<CodeableConcept> getVaccineCode() { 197 if (this.vaccineCode == null) 198 this.vaccineCode = new ArrayList<CodeableConcept>(); 199 return this.vaccineCode; 200 } 201 202 /** 203 * @return Returns a reference to <code>this</code> for easy method chaining 204 */ 205 public ImmunizationRecommendationRecommendationComponent setVaccineCode(List<CodeableConcept> theVaccineCode) { 206 this.vaccineCode = theVaccineCode; 207 return this; 208 } 209 210 public boolean hasVaccineCode() { 211 if (this.vaccineCode == null) 212 return false; 213 for (CodeableConcept item : this.vaccineCode) 214 if (!item.isEmpty()) 215 return true; 216 return false; 217 } 218 219 public CodeableConcept addVaccineCode() { // 3 220 CodeableConcept t = new CodeableConcept(); 221 if (this.vaccineCode == null) 222 this.vaccineCode = new ArrayList<CodeableConcept>(); 223 this.vaccineCode.add(t); 224 return t; 225 } 226 227 public ImmunizationRecommendationRecommendationComponent addVaccineCode(CodeableConcept t) { // 3 228 if (t == null) 229 return this; 230 if (this.vaccineCode == null) 231 this.vaccineCode = new ArrayList<CodeableConcept>(); 232 this.vaccineCode.add(t); 233 return this; 234 } 235 236 /** 237 * @return The first repetition of repeating field {@link #vaccineCode}, 238 * creating it if it does not already exist 239 */ 240 public CodeableConcept getVaccineCodeFirstRep() { 241 if (getVaccineCode().isEmpty()) { 242 addVaccineCode(); 243 } 244 return getVaccineCode().get(0); 245 } 246 247 /** 248 * @return {@link #targetDisease} (The targeted disease for the recommendation.) 249 */ 250 public CodeableConcept getTargetDisease() { 251 if (this.targetDisease == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.targetDisease"); 254 else if (Configuration.doAutoCreate()) 255 this.targetDisease = new CodeableConcept(); // cc 256 return this.targetDisease; 257 } 258 259 public boolean hasTargetDisease() { 260 return this.targetDisease != null && !this.targetDisease.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #targetDisease} (The targeted disease for the 265 * recommendation.) 266 */ 267 public ImmunizationRecommendationRecommendationComponent setTargetDisease(CodeableConcept value) { 268 this.targetDisease = value; 269 return this; 270 } 271 272 /** 273 * @return {@link #contraindicatedVaccineCode} (Vaccine(s) which should not be 274 * used to fulfill the recommendation.) 275 */ 276 public List<CodeableConcept> getContraindicatedVaccineCode() { 277 if (this.contraindicatedVaccineCode == null) 278 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 279 return this.contraindicatedVaccineCode; 280 } 281 282 /** 283 * @return Returns a reference to <code>this</code> for easy method chaining 284 */ 285 public ImmunizationRecommendationRecommendationComponent setContraindicatedVaccineCode( 286 List<CodeableConcept> theContraindicatedVaccineCode) { 287 this.contraindicatedVaccineCode = theContraindicatedVaccineCode; 288 return this; 289 } 290 291 public boolean hasContraindicatedVaccineCode() { 292 if (this.contraindicatedVaccineCode == null) 293 return false; 294 for (CodeableConcept item : this.contraindicatedVaccineCode) 295 if (!item.isEmpty()) 296 return true; 297 return false; 298 } 299 300 public CodeableConcept addContraindicatedVaccineCode() { // 3 301 CodeableConcept t = new CodeableConcept(); 302 if (this.contraindicatedVaccineCode == null) 303 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 304 this.contraindicatedVaccineCode.add(t); 305 return t; 306 } 307 308 public ImmunizationRecommendationRecommendationComponent addContraindicatedVaccineCode(CodeableConcept t) { // 3 309 if (t == null) 310 return this; 311 if (this.contraindicatedVaccineCode == null) 312 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 313 this.contraindicatedVaccineCode.add(t); 314 return this; 315 } 316 317 /** 318 * @return The first repetition of repeating field 319 * {@link #contraindicatedVaccineCode}, creating it if it does not 320 * already exist 321 */ 322 public CodeableConcept getContraindicatedVaccineCodeFirstRep() { 323 if (getContraindicatedVaccineCode().isEmpty()) { 324 addContraindicatedVaccineCode(); 325 } 326 return getContraindicatedVaccineCode().get(0); 327 } 328 329 /** 330 * @return {@link #forecastStatus} (Indicates the patient status with respect to 331 * the path to immunity for the target disease.) 332 */ 333 public CodeableConcept getForecastStatus() { 334 if (this.forecastStatus == null) 335 if (Configuration.errorOnAutoCreate()) 336 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.forecastStatus"); 337 else if (Configuration.doAutoCreate()) 338 this.forecastStatus = new CodeableConcept(); // cc 339 return this.forecastStatus; 340 } 341 342 public boolean hasForecastStatus() { 343 return this.forecastStatus != null && !this.forecastStatus.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #forecastStatus} (Indicates the patient status with 348 * respect to the path to immunity for the target disease.) 349 */ 350 public ImmunizationRecommendationRecommendationComponent setForecastStatus(CodeableConcept value) { 351 this.forecastStatus = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #forecastReason} (The reason for the assigned forecast 357 * status.) 358 */ 359 public List<CodeableConcept> getForecastReason() { 360 if (this.forecastReason == null) 361 this.forecastReason = new ArrayList<CodeableConcept>(); 362 return this.forecastReason; 363 } 364 365 /** 366 * @return Returns a reference to <code>this</code> for easy method chaining 367 */ 368 public ImmunizationRecommendationRecommendationComponent setForecastReason( 369 List<CodeableConcept> theForecastReason) { 370 this.forecastReason = theForecastReason; 371 return this; 372 } 373 374 public boolean hasForecastReason() { 375 if (this.forecastReason == null) 376 return false; 377 for (CodeableConcept item : this.forecastReason) 378 if (!item.isEmpty()) 379 return true; 380 return false; 381 } 382 383 public CodeableConcept addForecastReason() { // 3 384 CodeableConcept t = new CodeableConcept(); 385 if (this.forecastReason == null) 386 this.forecastReason = new ArrayList<CodeableConcept>(); 387 this.forecastReason.add(t); 388 return t; 389 } 390 391 public ImmunizationRecommendationRecommendationComponent addForecastReason(CodeableConcept t) { // 3 392 if (t == null) 393 return this; 394 if (this.forecastReason == null) 395 this.forecastReason = new ArrayList<CodeableConcept>(); 396 this.forecastReason.add(t); 397 return this; 398 } 399 400 /** 401 * @return The first repetition of repeating field {@link #forecastReason}, 402 * creating it if it does not already exist 403 */ 404 public CodeableConcept getForecastReasonFirstRep() { 405 if (getForecastReason().isEmpty()) { 406 addForecastReason(); 407 } 408 return getForecastReason().get(0); 409 } 410 411 /** 412 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, 413 * earliest date to administer, latest date to administer, etc.) 414 */ 415 public List<ImmunizationRecommendationRecommendationDateCriterionComponent> getDateCriterion() { 416 if (this.dateCriterion == null) 417 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 418 return this.dateCriterion; 419 } 420 421 /** 422 * @return Returns a reference to <code>this</code> for easy method chaining 423 */ 424 public ImmunizationRecommendationRecommendationComponent setDateCriterion( 425 List<ImmunizationRecommendationRecommendationDateCriterionComponent> theDateCriterion) { 426 this.dateCriterion = theDateCriterion; 427 return this; 428 } 429 430 public boolean hasDateCriterion() { 431 if (this.dateCriterion == null) 432 return false; 433 for (ImmunizationRecommendationRecommendationDateCriterionComponent item : this.dateCriterion) 434 if (!item.isEmpty()) 435 return true; 436 return false; 437 } 438 439 public ImmunizationRecommendationRecommendationDateCriterionComponent addDateCriterion() { // 3 440 ImmunizationRecommendationRecommendationDateCriterionComponent t = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 441 if (this.dateCriterion == null) 442 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 443 this.dateCriterion.add(t); 444 return t; 445 } 446 447 public ImmunizationRecommendationRecommendationComponent addDateCriterion( 448 ImmunizationRecommendationRecommendationDateCriterionComponent t) { // 3 449 if (t == null) 450 return this; 451 if (this.dateCriterion == null) 452 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 453 this.dateCriterion.add(t); 454 return this; 455 } 456 457 /** 458 * @return The first repetition of repeating field {@link #dateCriterion}, 459 * creating it if it does not already exist 460 */ 461 public ImmunizationRecommendationRecommendationDateCriterionComponent getDateCriterionFirstRep() { 462 if (getDateCriterion().isEmpty()) { 463 addDateCriterion(); 464 } 465 return getDateCriterion().get(0); 466 } 467 468 /** 469 * @return {@link #description} (Contains the description about the protocol 470 * under which the vaccine was administered.). This is the underlying 471 * object with id, value and extensions. The accessor "getDescription" 472 * gives direct access to the value 473 */ 474 public StringType getDescriptionElement() { 475 if (this.description == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.description"); 478 else if (Configuration.doAutoCreate()) 479 this.description = new StringType(); // bb 480 return this.description; 481 } 482 483 public boolean hasDescriptionElement() { 484 return this.description != null && !this.description.isEmpty(); 485 } 486 487 public boolean hasDescription() { 488 return this.description != null && !this.description.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #description} (Contains the description about the 493 * protocol under which the vaccine was administered.). This is the 494 * underlying object with id, value and extensions. The accessor 495 * "getDescription" gives direct access to the value 496 */ 497 public ImmunizationRecommendationRecommendationComponent setDescriptionElement(StringType value) { 498 this.description = value; 499 return this; 500 } 501 502 /** 503 * @return Contains the description about the protocol under which the vaccine 504 * was administered. 505 */ 506 public String getDescription() { 507 return this.description == null ? null : this.description.getValue(); 508 } 509 510 /** 511 * @param value Contains the description about the protocol under which the 512 * vaccine was administered. 513 */ 514 public ImmunizationRecommendationRecommendationComponent setDescription(String value) { 515 if (Utilities.noString(value)) 516 this.description = null; 517 else { 518 if (this.description == null) 519 this.description = new StringType(); 520 this.description.setValue(value); 521 } 522 return this; 523 } 524 525 /** 526 * @return {@link #series} (One possible path to achieve presumed immunity 527 * against a disease - within the context of an authority.). This is the 528 * underlying object with id, value and extensions. The accessor 529 * "getSeries" gives direct access to the value 530 */ 531 public StringType getSeriesElement() { 532 if (this.series == null) 533 if (Configuration.errorOnAutoCreate()) 534 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.series"); 535 else if (Configuration.doAutoCreate()) 536 this.series = new StringType(); // bb 537 return this.series; 538 } 539 540 public boolean hasSeriesElement() { 541 return this.series != null && !this.series.isEmpty(); 542 } 543 544 public boolean hasSeries() { 545 return this.series != null && !this.series.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #series} (One possible path to achieve presumed immunity 550 * against a disease - within the context of an authority.). This 551 * is the underlying object with id, value and extensions. The 552 * accessor "getSeries" gives direct access to the value 553 */ 554 public ImmunizationRecommendationRecommendationComponent setSeriesElement(StringType value) { 555 this.series = value; 556 return this; 557 } 558 559 /** 560 * @return One possible path to achieve presumed immunity against a disease - 561 * within the context of an authority. 562 */ 563 public String getSeries() { 564 return this.series == null ? null : this.series.getValue(); 565 } 566 567 /** 568 * @param value One possible path to achieve presumed immunity against a disease 569 * - within the context of an authority. 570 */ 571 public ImmunizationRecommendationRecommendationComponent setSeries(String value) { 572 if (Utilities.noString(value)) 573 this.series = null; 574 else { 575 if (this.series == null) 576 this.series = new StringType(); 577 this.series.setValue(value); 578 } 579 return this; 580 } 581 582 /** 583 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 584 * series (e.g. dose 2 is the next recommended dose).) 585 */ 586 public Type getDoseNumber() { 587 return this.doseNumber; 588 } 589 590 /** 591 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 592 * series (e.g. dose 2 is the next recommended dose).) 593 */ 594 public PositiveIntType getDoseNumberPositiveIntType() throws FHIRException { 595 if (this.doseNumber == null) 596 this.doseNumber = new PositiveIntType(); 597 if (!(this.doseNumber instanceof PositiveIntType)) 598 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 599 + this.doseNumber.getClass().getName() + " was encountered"); 600 return (PositiveIntType) this.doseNumber; 601 } 602 603 public boolean hasDoseNumberPositiveIntType() { 604 return this.doseNumber instanceof PositiveIntType; 605 } 606 607 /** 608 * @return {@link #doseNumber} (Nominal position of the recommended dose in a 609 * series (e.g. dose 2 is the next recommended dose).) 610 */ 611 public StringType getDoseNumberStringType() throws FHIRException { 612 if (this.doseNumber == null) 613 this.doseNumber = new StringType(); 614 if (!(this.doseNumber instanceof StringType)) 615 throw new FHIRException("Type mismatch: the type StringType was expected, but " 616 + this.doseNumber.getClass().getName() + " was encountered"); 617 return (StringType) this.doseNumber; 618 } 619 620 public boolean hasDoseNumberStringType() { 621 return this.doseNumber instanceof StringType; 622 } 623 624 public boolean hasDoseNumber() { 625 return this.doseNumber != null && !this.doseNumber.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #doseNumber} (Nominal position of the recommended dose in 630 * a series (e.g. dose 2 is the next recommended dose).) 631 */ 632 public ImmunizationRecommendationRecommendationComponent setDoseNumber(Type value) { 633 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 634 throw new Error( 635 "Not the right type for ImmunizationRecommendation.recommendation.doseNumber[x]: " + value.fhirType()); 636 this.doseNumber = value; 637 return this; 638 } 639 640 /** 641 * @return {@link #seriesDoses} (The recommended number of doses to achieve 642 * immunity.) 643 */ 644 public Type getSeriesDoses() { 645 return this.seriesDoses; 646 } 647 648 /** 649 * @return {@link #seriesDoses} (The recommended number of doses to achieve 650 * immunity.) 651 */ 652 public PositiveIntType getSeriesDosesPositiveIntType() throws FHIRException { 653 if (this.seriesDoses == null) 654 this.seriesDoses = new PositiveIntType(); 655 if (!(this.seriesDoses instanceof PositiveIntType)) 656 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but " 657 + this.seriesDoses.getClass().getName() + " was encountered"); 658 return (PositiveIntType) this.seriesDoses; 659 } 660 661 public boolean hasSeriesDosesPositiveIntType() { 662 return this.seriesDoses instanceof PositiveIntType; 663 } 664 665 /** 666 * @return {@link #seriesDoses} (The recommended number of doses to achieve 667 * immunity.) 668 */ 669 public StringType getSeriesDosesStringType() throws FHIRException { 670 if (this.seriesDoses == null) 671 this.seriesDoses = new StringType(); 672 if (!(this.seriesDoses instanceof StringType)) 673 throw new FHIRException("Type mismatch: the type StringType was expected, but " 674 + this.seriesDoses.getClass().getName() + " was encountered"); 675 return (StringType) this.seriesDoses; 676 } 677 678 public boolean hasSeriesDosesStringType() { 679 return this.seriesDoses instanceof StringType; 680 } 681 682 public boolean hasSeriesDoses() { 683 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #seriesDoses} (The recommended number of doses to achieve 688 * immunity.) 689 */ 690 public ImmunizationRecommendationRecommendationComponent setSeriesDoses(Type value) { 691 if (value != null && !(value instanceof PositiveIntType || value instanceof StringType)) 692 throw new Error( 693 "Not the right type for ImmunizationRecommendation.recommendation.seriesDoses[x]: " + value.fhirType()); 694 this.seriesDoses = value; 695 return this; 696 } 697 698 /** 699 * @return {@link #supportingImmunization} (Immunization event history and/or 700 * evaluation that supports the status and recommendation.) 701 */ 702 public List<Reference> getSupportingImmunization() { 703 if (this.supportingImmunization == null) 704 this.supportingImmunization = new ArrayList<Reference>(); 705 return this.supportingImmunization; 706 } 707 708 /** 709 * @return Returns a reference to <code>this</code> for easy method chaining 710 */ 711 public ImmunizationRecommendationRecommendationComponent setSupportingImmunization( 712 List<Reference> theSupportingImmunization) { 713 this.supportingImmunization = theSupportingImmunization; 714 return this; 715 } 716 717 public boolean hasSupportingImmunization() { 718 if (this.supportingImmunization == null) 719 return false; 720 for (Reference item : this.supportingImmunization) 721 if (!item.isEmpty()) 722 return true; 723 return false; 724 } 725 726 public Reference addSupportingImmunization() { // 3 727 Reference t = new Reference(); 728 if (this.supportingImmunization == null) 729 this.supportingImmunization = new ArrayList<Reference>(); 730 this.supportingImmunization.add(t); 731 return t; 732 } 733 734 public ImmunizationRecommendationRecommendationComponent addSupportingImmunization(Reference t) { // 3 735 if (t == null) 736 return this; 737 if (this.supportingImmunization == null) 738 this.supportingImmunization = new ArrayList<Reference>(); 739 this.supportingImmunization.add(t); 740 return this; 741 } 742 743 /** 744 * @return The first repetition of repeating field 745 * {@link #supportingImmunization}, creating it if it does not already 746 * exist 747 */ 748 public Reference getSupportingImmunizationFirstRep() { 749 if (getSupportingImmunization().isEmpty()) { 750 addSupportingImmunization(); 751 } 752 return getSupportingImmunization().get(0); 753 } 754 755 /** 756 * @return {@link #supportingPatientInformation} (Patient Information that 757 * supports the status and recommendation. This includes patient 758 * observations, adverse reactions and allergy/intolerance information.) 759 */ 760 public List<Reference> getSupportingPatientInformation() { 761 if (this.supportingPatientInformation == null) 762 this.supportingPatientInformation = new ArrayList<Reference>(); 763 return this.supportingPatientInformation; 764 } 765 766 /** 767 * @return Returns a reference to <code>this</code> for easy method chaining 768 */ 769 public ImmunizationRecommendationRecommendationComponent setSupportingPatientInformation( 770 List<Reference> theSupportingPatientInformation) { 771 this.supportingPatientInformation = theSupportingPatientInformation; 772 return this; 773 } 774 775 public boolean hasSupportingPatientInformation() { 776 if (this.supportingPatientInformation == null) 777 return false; 778 for (Reference item : this.supportingPatientInformation) 779 if (!item.isEmpty()) 780 return true; 781 return false; 782 } 783 784 public Reference addSupportingPatientInformation() { // 3 785 Reference t = new Reference(); 786 if (this.supportingPatientInformation == null) 787 this.supportingPatientInformation = new ArrayList<Reference>(); 788 this.supportingPatientInformation.add(t); 789 return t; 790 } 791 792 public ImmunizationRecommendationRecommendationComponent addSupportingPatientInformation(Reference t) { // 3 793 if (t == null) 794 return this; 795 if (this.supportingPatientInformation == null) 796 this.supportingPatientInformation = new ArrayList<Reference>(); 797 this.supportingPatientInformation.add(t); 798 return this; 799 } 800 801 /** 802 * @return The first repetition of repeating field 803 * {@link #supportingPatientInformation}, creating it if it does not 804 * already exist 805 */ 806 public Reference getSupportingPatientInformationFirstRep() { 807 if (getSupportingPatientInformation().isEmpty()) { 808 addSupportingPatientInformation(); 809 } 810 return getSupportingPatientInformation().get(0); 811 } 812 813 protected void listChildren(List<Property> children) { 814 super.listChildren(children); 815 children.add(new Property("vaccineCode", "CodeableConcept", 816 "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, 817 vaccineCode)); 818 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, 819 1, targetDisease)); 820 children.add(new Property("contraindicatedVaccineCode", "CodeableConcept", 821 "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, 822 contraindicatedVaccineCode)); 823 children.add(new Property("forecastStatus", "CodeableConcept", 824 "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, 825 forecastStatus)); 826 children.add(new Property("forecastReason", "CodeableConcept", "The reason for the assigned forecast status.", 0, 827 java.lang.Integer.MAX_VALUE, forecastReason)); 828 children.add(new Property("dateCriterion", "", 829 "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, 830 java.lang.Integer.MAX_VALUE, dateCriterion)); 831 children.add(new Property("description", "string", 832 "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 833 children.add(new Property("series", "string", 834 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 835 1, series)); 836 children.add(new Property("doseNumber[x]", "positiveInt|string", 837 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 838 doseNumber)); 839 children.add(new Property("seriesDoses[x]", "positiveInt|string", 840 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 841 children.add(new Property("supportingImmunization", "Reference(Immunization|ImmunizationEvaluation)", 842 "Immunization event history and/or evaluation that supports the status and recommendation.", 0, 843 java.lang.Integer.MAX_VALUE, supportingImmunization)); 844 children.add(new Property("supportingPatientInformation", "Reference(Any)", 845 "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 846 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation)); 847 } 848 849 @Override 850 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 851 switch (_hash) { 852 case 664556354: 853 /* vaccineCode */ return new Property("vaccineCode", "CodeableConcept", 854 "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, 855 vaccineCode); 856 case -319593813: 857 /* targetDisease */ return new Property("targetDisease", "CodeableConcept", 858 "The targeted disease for the recommendation.", 0, 1, targetDisease); 859 case 571105240: 860 /* contraindicatedVaccineCode */ return new Property("contraindicatedVaccineCode", "CodeableConcept", 861 "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, 862 contraindicatedVaccineCode); 863 case 1904598477: 864 /* forecastStatus */ return new Property("forecastStatus", "CodeableConcept", 865 "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, 866 forecastStatus); 867 case 1862115359: 868 /* forecastReason */ return new Property("forecastReason", "CodeableConcept", 869 "The reason for the assigned forecast status.", 0, java.lang.Integer.MAX_VALUE, forecastReason); 870 case 2087518867: 871 /* dateCriterion */ return new Property("dateCriterion", "", 872 "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 873 0, java.lang.Integer.MAX_VALUE, dateCriterion); 874 case -1724546052: 875 /* description */ return new Property("description", "string", 876 "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 877 case -905838985: 878 /* series */ return new Property("series", "string", 879 "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 880 1, series); 881 case -1632295686: 882 /* doseNumber[x] */ return new Property("doseNumber[x]", "positiveInt|string", 883 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 884 doseNumber); 885 case -887709242: 886 /* doseNumber */ return new Property("doseNumber[x]", "positiveInt|string", 887 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 888 doseNumber); 889 case -1826134640: 890 /* doseNumberPositiveInt */ return new Property("doseNumber[x]", "positiveInt|string", 891 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 892 doseNumber); 893 case -333053577: 894 /* doseNumberString */ return new Property("doseNumber[x]", "positiveInt|string", 895 "Nominal position of the recommended dose in a series (e.g. dose 2 is the next recommended dose).", 0, 1, 896 doseNumber); 897 case 1553560673: 898 /* seriesDoses[x] */ return new Property("seriesDoses[x]", "positiveInt|string", 899 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 900 case -1936727105: 901 /* seriesDoses */ return new Property("seriesDoses[x]", "positiveInt|string", 902 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 903 case -220897801: 904 /* seriesDosesPositiveInt */ return new Property("seriesDoses[x]", "positiveInt|string", 905 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 906 case -673569616: 907 /* seriesDosesString */ return new Property("seriesDoses[x]", "positiveInt|string", 908 "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 909 case 1171592021: 910 /* supportingImmunization */ return new Property("supportingImmunization", 911 "Reference(Immunization|ImmunizationEvaluation)", 912 "Immunization event history and/or evaluation that supports the status and recommendation.", 0, 913 java.lang.Integer.MAX_VALUE, supportingImmunization); 914 case -1234160646: 915 /* supportingPatientInformation */ return new Property("supportingPatientInformation", "Reference(Any)", 916 "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 917 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation); 918 default: 919 return super.getNamedProperty(_hash, _name, _checkValid); 920 } 921 922 } 923 924 @Override 925 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 926 switch (hash) { 927 case 664556354: 928 /* vaccineCode */ return this.vaccineCode == null ? new Base[0] 929 : this.vaccineCode.toArray(new Base[this.vaccineCode.size()]); // CodeableConcept 930 case -319593813: 931 /* targetDisease */ return this.targetDisease == null ? new Base[0] : new Base[] { this.targetDisease }; // CodeableConcept 932 case 571105240: 933 /* contraindicatedVaccineCode */ return this.contraindicatedVaccineCode == null ? new Base[0] 934 : this.contraindicatedVaccineCode.toArray(new Base[this.contraindicatedVaccineCode.size()]); // CodeableConcept 935 case 1904598477: 936 /* forecastStatus */ return this.forecastStatus == null ? new Base[0] : new Base[] { this.forecastStatus }; // CodeableConcept 937 case 1862115359: 938 /* forecastReason */ return this.forecastReason == null ? new Base[0] 939 : this.forecastReason.toArray(new Base[this.forecastReason.size()]); // CodeableConcept 940 case 2087518867: 941 /* dateCriterion */ return this.dateCriterion == null ? new Base[0] 942 : this.dateCriterion.toArray(new Base[this.dateCriterion.size()]); // ImmunizationRecommendationRecommendationDateCriterionComponent 943 case -1724546052: 944 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 945 case -905838985: 946 /* series */ return this.series == null ? new Base[0] : new Base[] { this.series }; // StringType 947 case -887709242: 948 /* doseNumber */ return this.doseNumber == null ? new Base[0] : new Base[] { this.doseNumber }; // Type 949 case -1936727105: 950 /* seriesDoses */ return this.seriesDoses == null ? new Base[0] : new Base[] { this.seriesDoses }; // Type 951 case 1171592021: 952 /* supportingImmunization */ return this.supportingImmunization == null ? new Base[0] 953 : this.supportingImmunization.toArray(new Base[this.supportingImmunization.size()]); // Reference 954 case -1234160646: 955 /* supportingPatientInformation */ return this.supportingPatientInformation == null ? new Base[0] 956 : this.supportingPatientInformation.toArray(new Base[this.supportingPatientInformation.size()]); // Reference 957 default: 958 return super.getProperty(hash, name, checkValid); 959 } 960 961 } 962 963 @Override 964 public Base setProperty(int hash, String name, Base value) throws FHIRException { 965 switch (hash) { 966 case 664556354: // vaccineCode 967 this.getVaccineCode().add(castToCodeableConcept(value)); // CodeableConcept 968 return value; 969 case -319593813: // targetDisease 970 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 971 return value; 972 case 571105240: // contraindicatedVaccineCode 973 this.getContraindicatedVaccineCode().add(castToCodeableConcept(value)); // CodeableConcept 974 return value; 975 case 1904598477: // forecastStatus 976 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 977 return value; 978 case 1862115359: // forecastReason 979 this.getForecastReason().add(castToCodeableConcept(value)); // CodeableConcept 980 return value; 981 case 2087518867: // dateCriterion 982 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); // ImmunizationRecommendationRecommendationDateCriterionComponent 983 return value; 984 case -1724546052: // description 985 this.description = castToString(value); // StringType 986 return value; 987 case -905838985: // series 988 this.series = castToString(value); // StringType 989 return value; 990 case -887709242: // doseNumber 991 this.doseNumber = castToType(value); // Type 992 return value; 993 case -1936727105: // seriesDoses 994 this.seriesDoses = castToType(value); // Type 995 return value; 996 case 1171592021: // supportingImmunization 997 this.getSupportingImmunization().add(castToReference(value)); // Reference 998 return value; 999 case -1234160646: // supportingPatientInformation 1000 this.getSupportingPatientInformation().add(castToReference(value)); // Reference 1001 return value; 1002 default: 1003 return super.setProperty(hash, name, value); 1004 } 1005 1006 } 1007 1008 @Override 1009 public Base setProperty(String name, Base value) throws FHIRException { 1010 if (name.equals("vaccineCode")) { 1011 this.getVaccineCode().add(castToCodeableConcept(value)); 1012 } else if (name.equals("targetDisease")) { 1013 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 1014 } else if (name.equals("contraindicatedVaccineCode")) { 1015 this.getContraindicatedVaccineCode().add(castToCodeableConcept(value)); 1016 } else if (name.equals("forecastStatus")) { 1017 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 1018 } else if (name.equals("forecastReason")) { 1019 this.getForecastReason().add(castToCodeableConcept(value)); 1020 } else if (name.equals("dateCriterion")) { 1021 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 1022 } else if (name.equals("description")) { 1023 this.description = castToString(value); // StringType 1024 } else if (name.equals("series")) { 1025 this.series = castToString(value); // StringType 1026 } else if (name.equals("doseNumber[x]")) { 1027 this.doseNumber = castToType(value); // Type 1028 } else if (name.equals("seriesDoses[x]")) { 1029 this.seriesDoses = castToType(value); // Type 1030 } else if (name.equals("supportingImmunization")) { 1031 this.getSupportingImmunization().add(castToReference(value)); 1032 } else if (name.equals("supportingPatientInformation")) { 1033 this.getSupportingPatientInformation().add(castToReference(value)); 1034 } else 1035 return super.setProperty(name, value); 1036 return value; 1037 } 1038 1039 @Override 1040 public void removeChild(String name, Base value) throws FHIRException { 1041 if (name.equals("vaccineCode")) { 1042 this.getVaccineCode().remove(castToCodeableConcept(value)); 1043 } else if (name.equals("targetDisease")) { 1044 this.targetDisease = null; 1045 } else if (name.equals("contraindicatedVaccineCode")) { 1046 this.getContraindicatedVaccineCode().remove(castToCodeableConcept(value)); 1047 } else if (name.equals("forecastStatus")) { 1048 this.forecastStatus = null; 1049 } else if (name.equals("forecastReason")) { 1050 this.getForecastReason().remove(castToCodeableConcept(value)); 1051 } else if (name.equals("dateCriterion")) { 1052 this.getDateCriterion().remove((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 1053 } else if (name.equals("description")) { 1054 this.description = null; 1055 } else if (name.equals("series")) { 1056 this.series = null; 1057 } else if (name.equals("doseNumber[x]")) { 1058 this.doseNumber = null; 1059 } else if (name.equals("seriesDoses[x]")) { 1060 this.seriesDoses = null; 1061 } else if (name.equals("supportingImmunization")) { 1062 this.getSupportingImmunization().remove(castToReference(value)); 1063 } else if (name.equals("supportingPatientInformation")) { 1064 this.getSupportingPatientInformation().remove(castToReference(value)); 1065 } else 1066 super.removeChild(name, value); 1067 1068 } 1069 1070 @Override 1071 public Base makeProperty(int hash, String name) throws FHIRException { 1072 switch (hash) { 1073 case 664556354: 1074 return addVaccineCode(); 1075 case -319593813: 1076 return getTargetDisease(); 1077 case 571105240: 1078 return addContraindicatedVaccineCode(); 1079 case 1904598477: 1080 return getForecastStatus(); 1081 case 1862115359: 1082 return addForecastReason(); 1083 case 2087518867: 1084 return addDateCriterion(); 1085 case -1724546052: 1086 return getDescriptionElement(); 1087 case -905838985: 1088 return getSeriesElement(); 1089 case -1632295686: 1090 return getDoseNumber(); 1091 case -887709242: 1092 return getDoseNumber(); 1093 case 1553560673: 1094 return getSeriesDoses(); 1095 case -1936727105: 1096 return getSeriesDoses(); 1097 case 1171592021: 1098 return addSupportingImmunization(); 1099 case -1234160646: 1100 return addSupportingPatientInformation(); 1101 default: 1102 return super.makeProperty(hash, name); 1103 } 1104 1105 } 1106 1107 @Override 1108 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1109 switch (hash) { 1110 case 664556354: 1111 /* vaccineCode */ return new String[] { "CodeableConcept" }; 1112 case -319593813: 1113 /* targetDisease */ return new String[] { "CodeableConcept" }; 1114 case 571105240: 1115 /* contraindicatedVaccineCode */ return new String[] { "CodeableConcept" }; 1116 case 1904598477: 1117 /* forecastStatus */ return new String[] { "CodeableConcept" }; 1118 case 1862115359: 1119 /* forecastReason */ return new String[] { "CodeableConcept" }; 1120 case 2087518867: 1121 /* dateCriterion */ return new String[] {}; 1122 case -1724546052: 1123 /* description */ return new String[] { "string" }; 1124 case -905838985: 1125 /* series */ return new String[] { "string" }; 1126 case -887709242: 1127 /* doseNumber */ return new String[] { "positiveInt", "string" }; 1128 case -1936727105: 1129 /* seriesDoses */ return new String[] { "positiveInt", "string" }; 1130 case 1171592021: 1131 /* supportingImmunization */ return new String[] { "Reference" }; 1132 case -1234160646: 1133 /* supportingPatientInformation */ return new String[] { "Reference" }; 1134 default: 1135 return super.getTypesForProperty(hash, name); 1136 } 1137 1138 } 1139 1140 @Override 1141 public Base addChild(String name) throws FHIRException { 1142 if (name.equals("vaccineCode")) { 1143 return addVaccineCode(); 1144 } else if (name.equals("targetDisease")) { 1145 this.targetDisease = new CodeableConcept(); 1146 return this.targetDisease; 1147 } else if (name.equals("contraindicatedVaccineCode")) { 1148 return addContraindicatedVaccineCode(); 1149 } else if (name.equals("forecastStatus")) { 1150 this.forecastStatus = new CodeableConcept(); 1151 return this.forecastStatus; 1152 } else if (name.equals("forecastReason")) { 1153 return addForecastReason(); 1154 } else if (name.equals("dateCriterion")) { 1155 return addDateCriterion(); 1156 } else if (name.equals("description")) { 1157 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.description"); 1158 } else if (name.equals("series")) { 1159 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.series"); 1160 } else if (name.equals("doseNumberPositiveInt")) { 1161 this.doseNumber = new PositiveIntType(); 1162 return this.doseNumber; 1163 } else if (name.equals("doseNumberString")) { 1164 this.doseNumber = new StringType(); 1165 return this.doseNumber; 1166 } else if (name.equals("seriesDosesPositiveInt")) { 1167 this.seriesDoses = new PositiveIntType(); 1168 return this.seriesDoses; 1169 } else if (name.equals("seriesDosesString")) { 1170 this.seriesDoses = new StringType(); 1171 return this.seriesDoses; 1172 } else if (name.equals("supportingImmunization")) { 1173 return addSupportingImmunization(); 1174 } else if (name.equals("supportingPatientInformation")) { 1175 return addSupportingPatientInformation(); 1176 } else 1177 return super.addChild(name); 1178 } 1179 1180 public ImmunizationRecommendationRecommendationComponent copy() { 1181 ImmunizationRecommendationRecommendationComponent dst = new ImmunizationRecommendationRecommendationComponent(); 1182 copyValues(dst); 1183 return dst; 1184 } 1185 1186 public void copyValues(ImmunizationRecommendationRecommendationComponent dst) { 1187 super.copyValues(dst); 1188 if (vaccineCode != null) { 1189 dst.vaccineCode = new ArrayList<CodeableConcept>(); 1190 for (CodeableConcept i : vaccineCode) 1191 dst.vaccineCode.add(i.copy()); 1192 } 1193 ; 1194 dst.targetDisease = targetDisease == null ? null : targetDisease.copy(); 1195 if (contraindicatedVaccineCode != null) { 1196 dst.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 1197 for (CodeableConcept i : contraindicatedVaccineCode) 1198 dst.contraindicatedVaccineCode.add(i.copy()); 1199 } 1200 ; 1201 dst.forecastStatus = forecastStatus == null ? null : forecastStatus.copy(); 1202 if (forecastReason != null) { 1203 dst.forecastReason = new ArrayList<CodeableConcept>(); 1204 for (CodeableConcept i : forecastReason) 1205 dst.forecastReason.add(i.copy()); 1206 } 1207 ; 1208 if (dateCriterion != null) { 1209 dst.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 1210 for (ImmunizationRecommendationRecommendationDateCriterionComponent i : dateCriterion) 1211 dst.dateCriterion.add(i.copy()); 1212 } 1213 ; 1214 dst.description = description == null ? null : description.copy(); 1215 dst.series = series == null ? null : series.copy(); 1216 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1217 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1218 if (supportingImmunization != null) { 1219 dst.supportingImmunization = new ArrayList<Reference>(); 1220 for (Reference i : supportingImmunization) 1221 dst.supportingImmunization.add(i.copy()); 1222 } 1223 ; 1224 if (supportingPatientInformation != null) { 1225 dst.supportingPatientInformation = new ArrayList<Reference>(); 1226 for (Reference i : supportingPatientInformation) 1227 dst.supportingPatientInformation.add(i.copy()); 1228 } 1229 ; 1230 } 1231 1232 @Override 1233 public boolean equalsDeep(Base other_) { 1234 if (!super.equalsDeep(other_)) 1235 return false; 1236 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1237 return false; 1238 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1239 return compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(targetDisease, o.targetDisease, true) 1240 && compareDeep(contraindicatedVaccineCode, o.contraindicatedVaccineCode, true) 1241 && compareDeep(forecastStatus, o.forecastStatus, true) && compareDeep(forecastReason, o.forecastReason, true) 1242 && compareDeep(dateCriterion, o.dateCriterion, true) && compareDeep(description, o.description, true) 1243 && compareDeep(series, o.series, true) && compareDeep(doseNumber, o.doseNumber, true) 1244 && compareDeep(seriesDoses, o.seriesDoses, true) 1245 && compareDeep(supportingImmunization, o.supportingImmunization, true) 1246 && compareDeep(supportingPatientInformation, o.supportingPatientInformation, true); 1247 } 1248 1249 @Override 1250 public boolean equalsShallow(Base other_) { 1251 if (!super.equalsShallow(other_)) 1252 return false; 1253 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1254 return false; 1255 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1256 return compareValues(description, o.description, true) && compareValues(series, o.series, true); 1257 } 1258 1259 public boolean isEmpty() { 1260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(vaccineCode, targetDisease, 1261 contraindicatedVaccineCode, forecastStatus, forecastReason, dateCriterion, description, series, doseNumber, 1262 seriesDoses, supportingImmunization, supportingPatientInformation); 1263 } 1264 1265 public String fhirType() { 1266 return "ImmunizationRecommendation.recommendation"; 1267 1268 } 1269 1270 } 1271 1272 @Block() 1273 public static class ImmunizationRecommendationRecommendationDateCriterionComponent extends BackboneElement 1274 implements IBaseBackboneElement { 1275 /** 1276 * Date classification of recommendation. For example, earliest date to give, 1277 * latest date to give, etc. 1278 */ 1279 @Child(name = "code", type = { 1280 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1281 @Description(shortDefinition = "Type of date", formalDefinition = "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.") 1282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/immunization-recommendation-date-criterion") 1283 protected CodeableConcept code; 1284 1285 /** 1286 * The date whose meaning is specified by dateCriterion.code. 1287 */ 1288 @Child(name = "value", type = { 1289 DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 1290 @Description(shortDefinition = "Recommended date", formalDefinition = "The date whose meaning is specified by dateCriterion.code.") 1291 protected DateTimeType value; 1292 1293 private static final long serialVersionUID = 1036994566L; 1294 1295 /** 1296 * Constructor 1297 */ 1298 public ImmunizationRecommendationRecommendationDateCriterionComponent() { 1299 super(); 1300 } 1301 1302 /** 1303 * Constructor 1304 */ 1305 public ImmunizationRecommendationRecommendationDateCriterionComponent(CodeableConcept code, DateTimeType value) { 1306 super(); 1307 this.code = code; 1308 this.value = value; 1309 } 1310 1311 /** 1312 * @return {@link #code} (Date classification of recommendation. For example, 1313 * earliest date to give, latest date to give, etc.) 1314 */ 1315 public CodeableConcept getCode() { 1316 if (this.code == null) 1317 if (Configuration.errorOnAutoCreate()) 1318 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.code"); 1319 else if (Configuration.doAutoCreate()) 1320 this.code = new CodeableConcept(); // cc 1321 return this.code; 1322 } 1323 1324 public boolean hasCode() { 1325 return this.code != null && !this.code.isEmpty(); 1326 } 1327 1328 /** 1329 * @param value {@link #code} (Date classification of recommendation. For 1330 * example, earliest date to give, latest date to give, etc.) 1331 */ 1332 public ImmunizationRecommendationRecommendationDateCriterionComponent setCode(CodeableConcept value) { 1333 this.code = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #value} (The date whose meaning is specified by 1339 * dateCriterion.code.). This is the underlying object with id, value 1340 * and extensions. The accessor "getValue" gives direct access to the 1341 * value 1342 */ 1343 public DateTimeType getValueElement() { 1344 if (this.value == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error( 1347 "Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.value"); 1348 else if (Configuration.doAutoCreate()) 1349 this.value = new DateTimeType(); // bb 1350 return this.value; 1351 } 1352 1353 public boolean hasValueElement() { 1354 return this.value != null && !this.value.isEmpty(); 1355 } 1356 1357 public boolean hasValue() { 1358 return this.value != null && !this.value.isEmpty(); 1359 } 1360 1361 /** 1362 * @param value {@link #value} (The date whose meaning is specified by 1363 * dateCriterion.code.). This is the underlying object with id, 1364 * value and extensions. The accessor "getValue" gives direct 1365 * access to the value 1366 */ 1367 public ImmunizationRecommendationRecommendationDateCriterionComponent setValueElement(DateTimeType value) { 1368 this.value = value; 1369 return this; 1370 } 1371 1372 /** 1373 * @return The date whose meaning is specified by dateCriterion.code. 1374 */ 1375 public Date getValue() { 1376 return this.value == null ? null : this.value.getValue(); 1377 } 1378 1379 /** 1380 * @param value The date whose meaning is specified by dateCriterion.code. 1381 */ 1382 public ImmunizationRecommendationRecommendationDateCriterionComponent setValue(Date value) { 1383 if (this.value == null) 1384 this.value = new DateTimeType(); 1385 this.value.setValue(value); 1386 return this; 1387 } 1388 1389 protected void listChildren(List<Property> children) { 1390 super.listChildren(children); 1391 children.add(new Property("code", "CodeableConcept", 1392 "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, 1393 code)); 1394 children.add( 1395 new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value)); 1396 } 1397 1398 @Override 1399 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1400 switch (_hash) { 1401 case 3059181: 1402 /* code */ return new Property("code", "CodeableConcept", 1403 "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1404 1, code); 1405 case 111972721: 1406 /* value */ return new Property("value", "dateTime", 1407 "The date whose meaning is specified by dateCriterion.code.", 0, 1, value); 1408 default: 1409 return super.getNamedProperty(_hash, _name, _checkValid); 1410 } 1411 1412 } 1413 1414 @Override 1415 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1416 switch (hash) { 1417 case 3059181: 1418 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1419 case 111972721: 1420 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // DateTimeType 1421 default: 1422 return super.getProperty(hash, name, checkValid); 1423 } 1424 1425 } 1426 1427 @Override 1428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1429 switch (hash) { 1430 case 3059181: // code 1431 this.code = castToCodeableConcept(value); // CodeableConcept 1432 return value; 1433 case 111972721: // value 1434 this.value = castToDateTime(value); // DateTimeType 1435 return value; 1436 default: 1437 return super.setProperty(hash, name, value); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base setProperty(String name, Base value) throws FHIRException { 1444 if (name.equals("code")) { 1445 this.code = castToCodeableConcept(value); // CodeableConcept 1446 } else if (name.equals("value")) { 1447 this.value = castToDateTime(value); // DateTimeType 1448 } else 1449 return super.setProperty(name, value); 1450 return value; 1451 } 1452 1453 @Override 1454 public void removeChild(String name, Base value) throws FHIRException { 1455 if (name.equals("code")) { 1456 this.code = null; 1457 } else if (name.equals("value")) { 1458 this.value = null; 1459 } else 1460 super.removeChild(name, value); 1461 1462 } 1463 1464 @Override 1465 public Base makeProperty(int hash, String name) throws FHIRException { 1466 switch (hash) { 1467 case 3059181: 1468 return getCode(); 1469 case 111972721: 1470 return getValueElement(); 1471 default: 1472 return super.makeProperty(hash, name); 1473 } 1474 1475 } 1476 1477 @Override 1478 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1479 switch (hash) { 1480 case 3059181: 1481 /* code */ return new String[] { "CodeableConcept" }; 1482 case 111972721: 1483 /* value */ return new String[] { "dateTime" }; 1484 default: 1485 return super.getTypesForProperty(hash, name); 1486 } 1487 1488 } 1489 1490 @Override 1491 public Base addChild(String name) throws FHIRException { 1492 if (name.equals("code")) { 1493 this.code = new CodeableConcept(); 1494 return this.code; 1495 } else if (name.equals("value")) { 1496 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.value"); 1497 } else 1498 return super.addChild(name); 1499 } 1500 1501 public ImmunizationRecommendationRecommendationDateCriterionComponent copy() { 1502 ImmunizationRecommendationRecommendationDateCriterionComponent dst = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 1503 copyValues(dst); 1504 return dst; 1505 } 1506 1507 public void copyValues(ImmunizationRecommendationRecommendationDateCriterionComponent dst) { 1508 super.copyValues(dst); 1509 dst.code = code == null ? null : code.copy(); 1510 dst.value = value == null ? null : value.copy(); 1511 } 1512 1513 @Override 1514 public boolean equalsDeep(Base other_) { 1515 if (!super.equalsDeep(other_)) 1516 return false; 1517 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1518 return false; 1519 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1520 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 1521 } 1522 1523 @Override 1524 public boolean equalsShallow(Base other_) { 1525 if (!super.equalsShallow(other_)) 1526 return false; 1527 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1528 return false; 1529 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1530 return compareValues(value, o.value, true); 1531 } 1532 1533 public boolean isEmpty() { 1534 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 1535 } 1536 1537 public String fhirType() { 1538 return "ImmunizationRecommendation.recommendation.dateCriterion"; 1539 1540 } 1541 1542 } 1543 1544 /** 1545 * A unique identifier assigned to this particular recommendation record. 1546 */ 1547 @Child(name = "identifier", type = { 1548 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1549 @Description(shortDefinition = "Business identifier", formalDefinition = "A unique identifier assigned to this particular recommendation record.") 1550 protected List<Identifier> identifier; 1551 1552 /** 1553 * The patient the recommendation(s) are for. 1554 */ 1555 @Child(name = "patient", type = { Patient.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1556 @Description(shortDefinition = "Who this profile is for", formalDefinition = "The patient the recommendation(s) are for.") 1557 protected Reference patient; 1558 1559 /** 1560 * The actual object that is the target of the reference (The patient the 1561 * recommendation(s) are for.) 1562 */ 1563 protected Patient patientTarget; 1564 1565 /** 1566 * The date the immunization recommendation(s) were created. 1567 */ 1568 @Child(name = "date", type = { DateTimeType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1569 @Description(shortDefinition = "Date recommendation(s) created", formalDefinition = "The date the immunization recommendation(s) were created.") 1570 protected DateTimeType date; 1571 1572 /** 1573 * Indicates the authority who published the protocol (e.g. ACIP). 1574 */ 1575 @Child(name = "authority", type = { 1576 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1577 @Description(shortDefinition = "Who is responsible for protocol", formalDefinition = "Indicates the authority who published the protocol (e.g. ACIP).") 1578 protected Reference authority; 1579 1580 /** 1581 * The actual object that is the target of the reference (Indicates the 1582 * authority who published the protocol (e.g. ACIP).) 1583 */ 1584 protected Organization authorityTarget; 1585 1586 /** 1587 * Vaccine administration recommendations. 1588 */ 1589 @Child(name = "recommendation", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1590 @Description(shortDefinition = "Vaccine administration recommendations", formalDefinition = "Vaccine administration recommendations.") 1591 protected List<ImmunizationRecommendationRecommendationComponent> recommendation; 1592 1593 private static final long serialVersionUID = -2031711761L; 1594 1595 /** 1596 * Constructor 1597 */ 1598 public ImmunizationRecommendation() { 1599 super(); 1600 } 1601 1602 /** 1603 * Constructor 1604 */ 1605 public ImmunizationRecommendation(Reference patient, DateTimeType date) { 1606 super(); 1607 this.patient = patient; 1608 this.date = date; 1609 } 1610 1611 /** 1612 * @return {@link #identifier} (A unique identifier assigned to this particular 1613 * recommendation record.) 1614 */ 1615 public List<Identifier> getIdentifier() { 1616 if (this.identifier == null) 1617 this.identifier = new ArrayList<Identifier>(); 1618 return this.identifier; 1619 } 1620 1621 /** 1622 * @return Returns a reference to <code>this</code> for easy method chaining 1623 */ 1624 public ImmunizationRecommendation setIdentifier(List<Identifier> theIdentifier) { 1625 this.identifier = theIdentifier; 1626 return this; 1627 } 1628 1629 public boolean hasIdentifier() { 1630 if (this.identifier == null) 1631 return false; 1632 for (Identifier item : this.identifier) 1633 if (!item.isEmpty()) 1634 return true; 1635 return false; 1636 } 1637 1638 public Identifier addIdentifier() { // 3 1639 Identifier t = new Identifier(); 1640 if (this.identifier == null) 1641 this.identifier = new ArrayList<Identifier>(); 1642 this.identifier.add(t); 1643 return t; 1644 } 1645 1646 public ImmunizationRecommendation addIdentifier(Identifier t) { // 3 1647 if (t == null) 1648 return this; 1649 if (this.identifier == null) 1650 this.identifier = new ArrayList<Identifier>(); 1651 this.identifier.add(t); 1652 return this; 1653 } 1654 1655 /** 1656 * @return The first repetition of repeating field {@link #identifier}, creating 1657 * it if it does not already exist 1658 */ 1659 public Identifier getIdentifierFirstRep() { 1660 if (getIdentifier().isEmpty()) { 1661 addIdentifier(); 1662 } 1663 return getIdentifier().get(0); 1664 } 1665 1666 /** 1667 * @return {@link #patient} (The patient the recommendation(s) are for.) 1668 */ 1669 public Reference getPatient() { 1670 if (this.patient == null) 1671 if (Configuration.errorOnAutoCreate()) 1672 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1673 else if (Configuration.doAutoCreate()) 1674 this.patient = new Reference(); // cc 1675 return this.patient; 1676 } 1677 1678 public boolean hasPatient() { 1679 return this.patient != null && !this.patient.isEmpty(); 1680 } 1681 1682 /** 1683 * @param value {@link #patient} (The patient the recommendation(s) are for.) 1684 */ 1685 public ImmunizationRecommendation setPatient(Reference value) { 1686 this.patient = value; 1687 return this; 1688 } 1689 1690 /** 1691 * @return {@link #patient} The actual object that is the target of the 1692 * reference. The reference library doesn't populate this, but you can 1693 * use it to hold the resource if you resolve it. (The patient the 1694 * recommendation(s) are for.) 1695 */ 1696 public Patient getPatientTarget() { 1697 if (this.patientTarget == null) 1698 if (Configuration.errorOnAutoCreate()) 1699 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1700 else if (Configuration.doAutoCreate()) 1701 this.patientTarget = new Patient(); // aa 1702 return this.patientTarget; 1703 } 1704 1705 /** 1706 * @param value {@link #patient} The actual object that is the target of the 1707 * reference. The reference library doesn't use these, but you can 1708 * use it to hold the resource if you resolve it. (The patient the 1709 * recommendation(s) are for.) 1710 */ 1711 public ImmunizationRecommendation setPatientTarget(Patient value) { 1712 this.patientTarget = value; 1713 return this; 1714 } 1715 1716 /** 1717 * @return {@link #date} (The date the immunization recommendation(s) were 1718 * created.). This is the underlying object with id, value and 1719 * extensions. The accessor "getDate" gives direct access to the value 1720 */ 1721 public DateTimeType getDateElement() { 1722 if (this.date == null) 1723 if (Configuration.errorOnAutoCreate()) 1724 throw new Error("Attempt to auto-create ImmunizationRecommendation.date"); 1725 else if (Configuration.doAutoCreate()) 1726 this.date = new DateTimeType(); // bb 1727 return this.date; 1728 } 1729 1730 public boolean hasDateElement() { 1731 return this.date != null && !this.date.isEmpty(); 1732 } 1733 1734 public boolean hasDate() { 1735 return this.date != null && !this.date.isEmpty(); 1736 } 1737 1738 /** 1739 * @param value {@link #date} (The date the immunization recommendation(s) were 1740 * created.). This is the underlying object with id, value and 1741 * extensions. The accessor "getDate" gives direct access to the 1742 * value 1743 */ 1744 public ImmunizationRecommendation setDateElement(DateTimeType value) { 1745 this.date = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return The date the immunization recommendation(s) were created. 1751 */ 1752 public Date getDate() { 1753 return this.date == null ? null : this.date.getValue(); 1754 } 1755 1756 /** 1757 * @param value The date the immunization recommendation(s) were created. 1758 */ 1759 public ImmunizationRecommendation setDate(Date value) { 1760 if (this.date == null) 1761 this.date = new DateTimeType(); 1762 this.date.setValue(value); 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #authority} (Indicates the authority who published the 1768 * protocol (e.g. ACIP).) 1769 */ 1770 public Reference getAuthority() { 1771 if (this.authority == null) 1772 if (Configuration.errorOnAutoCreate()) 1773 throw new Error("Attempt to auto-create ImmunizationRecommendation.authority"); 1774 else if (Configuration.doAutoCreate()) 1775 this.authority = new Reference(); // cc 1776 return this.authority; 1777 } 1778 1779 public boolean hasAuthority() { 1780 return this.authority != null && !this.authority.isEmpty(); 1781 } 1782 1783 /** 1784 * @param value {@link #authority} (Indicates the authority who published the 1785 * protocol (e.g. ACIP).) 1786 */ 1787 public ImmunizationRecommendation setAuthority(Reference value) { 1788 this.authority = value; 1789 return this; 1790 } 1791 1792 /** 1793 * @return {@link #authority} The actual object that is the target of the 1794 * reference. The reference library doesn't populate this, but you can 1795 * use it to hold the resource if you resolve it. (Indicates the 1796 * authority who published the protocol (e.g. ACIP).) 1797 */ 1798 public Organization getAuthorityTarget() { 1799 if (this.authorityTarget == null) 1800 if (Configuration.errorOnAutoCreate()) 1801 throw new Error("Attempt to auto-create ImmunizationRecommendation.authority"); 1802 else if (Configuration.doAutoCreate()) 1803 this.authorityTarget = new Organization(); // aa 1804 return this.authorityTarget; 1805 } 1806 1807 /** 1808 * @param value {@link #authority} The actual object that is the target of the 1809 * reference. The reference library doesn't use these, but you can 1810 * use it to hold the resource if you resolve it. (Indicates the 1811 * authority who published the protocol (e.g. ACIP).) 1812 */ 1813 public ImmunizationRecommendation setAuthorityTarget(Organization value) { 1814 this.authorityTarget = value; 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #recommendation} (Vaccine administration recommendations.) 1820 */ 1821 public List<ImmunizationRecommendationRecommendationComponent> getRecommendation() { 1822 if (this.recommendation == null) 1823 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1824 return this.recommendation; 1825 } 1826 1827 /** 1828 * @return Returns a reference to <code>this</code> for easy method chaining 1829 */ 1830 public ImmunizationRecommendation setRecommendation( 1831 List<ImmunizationRecommendationRecommendationComponent> theRecommendation) { 1832 this.recommendation = theRecommendation; 1833 return this; 1834 } 1835 1836 public boolean hasRecommendation() { 1837 if (this.recommendation == null) 1838 return false; 1839 for (ImmunizationRecommendationRecommendationComponent item : this.recommendation) 1840 if (!item.isEmpty()) 1841 return true; 1842 return false; 1843 } 1844 1845 public ImmunizationRecommendationRecommendationComponent addRecommendation() { // 3 1846 ImmunizationRecommendationRecommendationComponent t = new ImmunizationRecommendationRecommendationComponent(); 1847 if (this.recommendation == null) 1848 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1849 this.recommendation.add(t); 1850 return t; 1851 } 1852 1853 public ImmunizationRecommendation addRecommendation(ImmunizationRecommendationRecommendationComponent t) { // 3 1854 if (t == null) 1855 return this; 1856 if (this.recommendation == null) 1857 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1858 this.recommendation.add(t); 1859 return this; 1860 } 1861 1862 /** 1863 * @return The first repetition of repeating field {@link #recommendation}, 1864 * creating it if it does not already exist 1865 */ 1866 public ImmunizationRecommendationRecommendationComponent getRecommendationFirstRep() { 1867 if (getRecommendation().isEmpty()) { 1868 addRecommendation(); 1869 } 1870 return getRecommendation().get(0); 1871 } 1872 1873 protected void listChildren(List<Property> children) { 1874 super.listChildren(children); 1875 children.add(new Property("identifier", "Identifier", 1876 "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, 1877 identifier)); 1878 children.add( 1879 new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 0, 1, patient)); 1880 children 1881 .add(new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1, date)); 1882 children.add(new Property("authority", "Reference(Organization)", 1883 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority)); 1884 children.add(new Property("recommendation", "", "Vaccine administration recommendations.", 0, 1885 java.lang.Integer.MAX_VALUE, recommendation)); 1886 } 1887 1888 @Override 1889 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1890 switch (_hash) { 1891 case -1618432855: 1892 /* identifier */ return new Property("identifier", "Identifier", 1893 "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, 1894 identifier); 1895 case -791418107: 1896 /* patient */ return new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 1897 0, 1, patient); 1898 case 3076014: 1899 /* date */ return new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1900 1, date); 1901 case 1475610435: 1902 /* authority */ return new Property("authority", "Reference(Organization)", 1903 "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority); 1904 case -1028636743: 1905 /* recommendation */ return new Property("recommendation", "", "Vaccine administration recommendations.", 0, 1906 java.lang.Integer.MAX_VALUE, recommendation); 1907 default: 1908 return super.getNamedProperty(_hash, _name, _checkValid); 1909 } 1910 1911 } 1912 1913 @Override 1914 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1915 switch (hash) { 1916 case -1618432855: 1917 /* identifier */ return this.identifier == null ? new Base[0] 1918 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1919 case -791418107: 1920 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 1921 case 3076014: 1922 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1923 case 1475610435: 1924 /* authority */ return this.authority == null ? new Base[0] : new Base[] { this.authority }; // Reference 1925 case -1028636743: 1926 /* recommendation */ return this.recommendation == null ? new Base[0] 1927 : this.recommendation.toArray(new Base[this.recommendation.size()]); // ImmunizationRecommendationRecommendationComponent 1928 default: 1929 return super.getProperty(hash, name, checkValid); 1930 } 1931 1932 } 1933 1934 @Override 1935 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1936 switch (hash) { 1937 case -1618432855: // identifier 1938 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1939 return value; 1940 case -791418107: // patient 1941 this.patient = castToReference(value); // Reference 1942 return value; 1943 case 3076014: // date 1944 this.date = castToDateTime(value); // DateTimeType 1945 return value; 1946 case 1475610435: // authority 1947 this.authority = castToReference(value); // Reference 1948 return value; 1949 case -1028636743: // recommendation 1950 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); // ImmunizationRecommendationRecommendationComponent 1951 return value; 1952 default: 1953 return super.setProperty(hash, name, value); 1954 } 1955 1956 } 1957 1958 @Override 1959 public Base setProperty(String name, Base value) throws FHIRException { 1960 if (name.equals("identifier")) { 1961 this.getIdentifier().add(castToIdentifier(value)); 1962 } else if (name.equals("patient")) { 1963 this.patient = castToReference(value); // Reference 1964 } else if (name.equals("date")) { 1965 this.date = castToDateTime(value); // DateTimeType 1966 } else if (name.equals("authority")) { 1967 this.authority = castToReference(value); // Reference 1968 } else if (name.equals("recommendation")) { 1969 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); 1970 } else 1971 return super.setProperty(name, value); 1972 return value; 1973 } 1974 1975 @Override 1976 public void removeChild(String name, Base value) throws FHIRException { 1977 if (name.equals("identifier")) { 1978 this.getIdentifier().remove(castToIdentifier(value)); 1979 } else if (name.equals("patient")) { 1980 this.patient = null; 1981 } else if (name.equals("date")) { 1982 this.date = null; 1983 } else if (name.equals("authority")) { 1984 this.authority = null; 1985 } else if (name.equals("recommendation")) { 1986 this.getRecommendation().remove((ImmunizationRecommendationRecommendationComponent) value); 1987 } else 1988 super.removeChild(name, value); 1989 1990 } 1991 1992 @Override 1993 public Base makeProperty(int hash, String name) throws FHIRException { 1994 switch (hash) { 1995 case -1618432855: 1996 return addIdentifier(); 1997 case -791418107: 1998 return getPatient(); 1999 case 3076014: 2000 return getDateElement(); 2001 case 1475610435: 2002 return getAuthority(); 2003 case -1028636743: 2004 return addRecommendation(); 2005 default: 2006 return super.makeProperty(hash, name); 2007 } 2008 2009 } 2010 2011 @Override 2012 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2013 switch (hash) { 2014 case -1618432855: 2015 /* identifier */ return new String[] { "Identifier" }; 2016 case -791418107: 2017 /* patient */ return new String[] { "Reference" }; 2018 case 3076014: 2019 /* date */ return new String[] { "dateTime" }; 2020 case 1475610435: 2021 /* authority */ return new String[] { "Reference" }; 2022 case -1028636743: 2023 /* recommendation */ return new String[] {}; 2024 default: 2025 return super.getTypesForProperty(hash, name); 2026 } 2027 2028 } 2029 2030 @Override 2031 public Base addChild(String name) throws FHIRException { 2032 if (name.equals("identifier")) { 2033 return addIdentifier(); 2034 } else if (name.equals("patient")) { 2035 this.patient = new Reference(); 2036 return this.patient; 2037 } else if (name.equals("date")) { 2038 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.date"); 2039 } else if (name.equals("authority")) { 2040 this.authority = new Reference(); 2041 return this.authority; 2042 } else if (name.equals("recommendation")) { 2043 return addRecommendation(); 2044 } else 2045 return super.addChild(name); 2046 } 2047 2048 public String fhirType() { 2049 return "ImmunizationRecommendation"; 2050 2051 } 2052 2053 public ImmunizationRecommendation copy() { 2054 ImmunizationRecommendation dst = new ImmunizationRecommendation(); 2055 copyValues(dst); 2056 return dst; 2057 } 2058 2059 public void copyValues(ImmunizationRecommendation dst) { 2060 super.copyValues(dst); 2061 if (identifier != null) { 2062 dst.identifier = new ArrayList<Identifier>(); 2063 for (Identifier i : identifier) 2064 dst.identifier.add(i.copy()); 2065 } 2066 ; 2067 dst.patient = patient == null ? null : patient.copy(); 2068 dst.date = date == null ? null : date.copy(); 2069 dst.authority = authority == null ? null : authority.copy(); 2070 if (recommendation != null) { 2071 dst.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 2072 for (ImmunizationRecommendationRecommendationComponent i : recommendation) 2073 dst.recommendation.add(i.copy()); 2074 } 2075 ; 2076 } 2077 2078 protected ImmunizationRecommendation typedCopy() { 2079 return copy(); 2080 } 2081 2082 @Override 2083 public boolean equalsDeep(Base other_) { 2084 if (!super.equalsDeep(other_)) 2085 return false; 2086 if (!(other_ instanceof ImmunizationRecommendation)) 2087 return false; 2088 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 2089 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) 2090 && compareDeep(date, o.date, true) && compareDeep(authority, o.authority, true) 2091 && compareDeep(recommendation, o.recommendation, true); 2092 } 2093 2094 @Override 2095 public boolean equalsShallow(Base other_) { 2096 if (!super.equalsShallow(other_)) 2097 return false; 2098 if (!(other_ instanceof ImmunizationRecommendation)) 2099 return false; 2100 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 2101 return compareValues(date, o.date, true); 2102 } 2103 2104 public boolean isEmpty() { 2105 return super.isEmpty() 2106 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, patient, date, authority, recommendation); 2107 } 2108 2109 @Override 2110 public ResourceType getResourceType() { 2111 return ResourceType.ImmunizationRecommendation; 2112 } 2113 2114 /** 2115 * Search parameter: <b>date</b> 2116 * <p> 2117 * Description: <b>Date recommendation(s) created</b><br> 2118 * Type: <b>date</b><br> 2119 * Path: <b>ImmunizationRecommendation.date</b><br> 2120 * </p> 2121 */ 2122 @SearchParamDefinition(name = "date", path = "ImmunizationRecommendation.date", description = "Date recommendation(s) created", type = "date") 2123 public static final String SP_DATE = "date"; 2124 /** 2125 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2126 * <p> 2127 * Description: <b>Date recommendation(s) created</b><br> 2128 * Type: <b>date</b><br> 2129 * Path: <b>ImmunizationRecommendation.date</b><br> 2130 * </p> 2131 */ 2132 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2133 SP_DATE); 2134 2135 /** 2136 * Search parameter: <b>identifier</b> 2137 * <p> 2138 * Description: <b>Business identifier</b><br> 2139 * Type: <b>token</b><br> 2140 * Path: <b>ImmunizationRecommendation.identifier</b><br> 2141 * </p> 2142 */ 2143 @SearchParamDefinition(name = "identifier", path = "ImmunizationRecommendation.identifier", description = "Business identifier", type = "token") 2144 public static final String SP_IDENTIFIER = "identifier"; 2145 /** 2146 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2147 * <p> 2148 * Description: <b>Business identifier</b><br> 2149 * Type: <b>token</b><br> 2150 * Path: <b>ImmunizationRecommendation.identifier</b><br> 2151 * </p> 2152 */ 2153 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2154 SP_IDENTIFIER); 2155 2156 /** 2157 * Search parameter: <b>target-disease</b> 2158 * <p> 2159 * Description: <b>Disease to be immunized against</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 2162 * </p> 2163 */ 2164 @SearchParamDefinition(name = "target-disease", path = "ImmunizationRecommendation.recommendation.targetDisease", description = "Disease to be immunized against", type = "token") 2165 public static final String SP_TARGET_DISEASE = "target-disease"; 2166 /** 2167 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 2168 * <p> 2169 * Description: <b>Disease to be immunized against</b><br> 2170 * Type: <b>token</b><br> 2171 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 2172 * </p> 2173 */ 2174 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2175 SP_TARGET_DISEASE); 2176 2177 /** 2178 * Search parameter: <b>patient</b> 2179 * <p> 2180 * Description: <b>Who this profile is for</b><br> 2181 * Type: <b>reference</b><br> 2182 * Path: <b>ImmunizationRecommendation.patient</b><br> 2183 * </p> 2184 */ 2185 @SearchParamDefinition(name = "patient", path = "ImmunizationRecommendation.patient", description = "Who this profile is for", type = "reference", providesMembershipIn = { 2186 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 2187 public static final String SP_PATIENT = "patient"; 2188 /** 2189 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2190 * <p> 2191 * Description: <b>Who this profile is for</b><br> 2192 * Type: <b>reference</b><br> 2193 * Path: <b>ImmunizationRecommendation.patient</b><br> 2194 * </p> 2195 */ 2196 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2197 SP_PATIENT); 2198 2199 /** 2200 * Constant for fluent queries to be used to add include statements. Specifies 2201 * the path value of "<b>ImmunizationRecommendation:patient</b>". 2202 */ 2203 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 2204 "ImmunizationRecommendation:patient").toLocked(); 2205 2206 /** 2207 * Search parameter: <b>vaccine-type</b> 2208 * <p> 2209 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 2212 * </p> 2213 */ 2214 @SearchParamDefinition(name = "vaccine-type", path = "ImmunizationRecommendation.recommendation.vaccineCode", description = "Vaccine or vaccine group recommendation applies to", type = "token") 2215 public static final String SP_VACCINE_TYPE = "vaccine-type"; 2216 /** 2217 * <b>Fluent Client</b> search parameter constant for <b>vaccine-type</b> 2218 * <p> 2219 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 2220 * Type: <b>token</b><br> 2221 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 2222 * </p> 2223 */ 2224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2225 SP_VACCINE_TYPE); 2226 2227 /** 2228 * Search parameter: <b>information</b> 2229 * <p> 2230 * Description: <b>Patient observations supporting recommendation</b><br> 2231 * Type: <b>reference</b><br> 2232 * Path: 2233 * <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name = "information", path = "ImmunizationRecommendation.recommendation.supportingPatientInformation", description = "Patient observations supporting recommendation", type = "reference") 2237 public static final String SP_INFORMATION = "information"; 2238 /** 2239 * <b>Fluent Client</b> search parameter constant for <b>information</b> 2240 * <p> 2241 * Description: <b>Patient observations supporting recommendation</b><br> 2242 * Type: <b>reference</b><br> 2243 * Path: 2244 * <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 2245 * </p> 2246 */ 2247 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INFORMATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2248 SP_INFORMATION); 2249 2250 /** 2251 * Constant for fluent queries to be used to add include statements. Specifies 2252 * the path value of "<b>ImmunizationRecommendation:information</b>". 2253 */ 2254 public static final ca.uhn.fhir.model.api.Include INCLUDE_INFORMATION = new ca.uhn.fhir.model.api.Include( 2255 "ImmunizationRecommendation:information").toLocked(); 2256 2257 /** 2258 * Search parameter: <b>support</b> 2259 * <p> 2260 * Description: <b>Past immunizations supporting recommendation</b><br> 2261 * Type: <b>reference</b><br> 2262 * Path: 2263 * <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name = "support", path = "ImmunizationRecommendation.recommendation.supportingImmunization", description = "Past immunizations supporting recommendation", type = "reference", target = { 2267 Immunization.class, ImmunizationEvaluation.class }) 2268 public static final String SP_SUPPORT = "support"; 2269 /** 2270 * <b>Fluent Client</b> search parameter constant for <b>support</b> 2271 * <p> 2272 * Description: <b>Past immunizations supporting recommendation</b><br> 2273 * Type: <b>reference</b><br> 2274 * Path: 2275 * <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 2276 * </p> 2277 */ 2278 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2279 SP_SUPPORT); 2280 2281 /** 2282 * Constant for fluent queries to be used to add include statements. Specifies 2283 * the path value of "<b>ImmunizationRecommendation:support</b>". 2284 */ 2285 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORT = new ca.uhn.fhir.model.api.Include( 2286 "ImmunizationRecommendation:support").toLocked(); 2287 2288 /** 2289 * Search parameter: <b>status</b> 2290 * <p> 2291 * Description: <b>Vaccine recommendation status</b><br> 2292 * Type: <b>token</b><br> 2293 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 2294 * </p> 2295 */ 2296 @SearchParamDefinition(name = "status", path = "ImmunizationRecommendation.recommendation.forecastStatus", description = "Vaccine recommendation status", type = "token") 2297 public static final String SP_STATUS = "status"; 2298 /** 2299 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2300 * <p> 2301 * Description: <b>Vaccine recommendation status</b><br> 2302 * Type: <b>token</b><br> 2303 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 2304 * </p> 2305 */ 2306 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2307 SP_STATUS); 2308 2309}