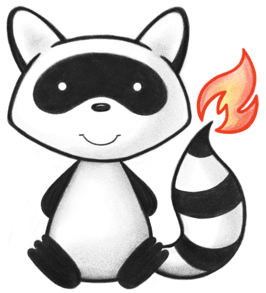
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Details of a Health Insurance product/plan provided by an organization. 050 */ 051@ResourceDef(name = "InsurancePlan", profile = "http://hl7.org/fhir/StructureDefinition/InsurancePlan") 052public class InsurancePlan extends DomainResource { 053 054 @Block() 055 public static class InsurancePlanContactComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * Indicates a purpose for which the contact can be reached. 058 */ 059 @Child(name = "purpose", type = { 060 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 061 @Description(shortDefinition = "The type of contact", formalDefinition = "Indicates a purpose for which the contact can be reached.") 062 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/contactentity-type") 063 protected CodeableConcept purpose; 064 065 /** 066 * A name associated with the contact. 067 */ 068 @Child(name = "name", type = { HumanName.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 069 @Description(shortDefinition = "A name associated with the contact", formalDefinition = "A name associated with the contact.") 070 protected HumanName name; 071 072 /** 073 * A contact detail (e.g. a telephone number or an email address) by which the 074 * party may be contacted. 075 */ 076 @Child(name = "telecom", type = { 077 ContactPoint.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 078 @Description(shortDefinition = "Contact details (telephone, email, etc.) for a contact", formalDefinition = "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.") 079 protected List<ContactPoint> telecom; 080 081 /** 082 * Visiting or postal addresses for the contact. 083 */ 084 @Child(name = "address", type = { Address.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 085 @Description(shortDefinition = "Visiting or postal addresses for the contact", formalDefinition = "Visiting or postal addresses for the contact.") 086 protected Address address; 087 088 private static final long serialVersionUID = 1831121305L; 089 090 /** 091 * Constructor 092 */ 093 public InsurancePlanContactComponent() { 094 super(); 095 } 096 097 /** 098 * @return {@link #purpose} (Indicates a purpose for which the contact can be 099 * reached.) 100 */ 101 public CodeableConcept getPurpose() { 102 if (this.purpose == null) 103 if (Configuration.errorOnAutoCreate()) 104 throw new Error("Attempt to auto-create InsurancePlanContactComponent.purpose"); 105 else if (Configuration.doAutoCreate()) 106 this.purpose = new CodeableConcept(); // cc 107 return this.purpose; 108 } 109 110 public boolean hasPurpose() { 111 return this.purpose != null && !this.purpose.isEmpty(); 112 } 113 114 /** 115 * @param value {@link #purpose} (Indicates a purpose for which the contact can 116 * be reached.) 117 */ 118 public InsurancePlanContactComponent setPurpose(CodeableConcept value) { 119 this.purpose = value; 120 return this; 121 } 122 123 /** 124 * @return {@link #name} (A name associated with the contact.) 125 */ 126 public HumanName getName() { 127 if (this.name == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create InsurancePlanContactComponent.name"); 130 else if (Configuration.doAutoCreate()) 131 this.name = new HumanName(); // cc 132 return this.name; 133 } 134 135 public boolean hasName() { 136 return this.name != null && !this.name.isEmpty(); 137 } 138 139 /** 140 * @param value {@link #name} (A name associated with the contact.) 141 */ 142 public InsurancePlanContactComponent setName(HumanName value) { 143 this.name = value; 144 return this; 145 } 146 147 /** 148 * @return {@link #telecom} (A contact detail (e.g. a telephone number or an 149 * email address) by which the party may be contacted.) 150 */ 151 public List<ContactPoint> getTelecom() { 152 if (this.telecom == null) 153 this.telecom = new ArrayList<ContactPoint>(); 154 return this.telecom; 155 } 156 157 /** 158 * @return Returns a reference to <code>this</code> for easy method chaining 159 */ 160 public InsurancePlanContactComponent setTelecom(List<ContactPoint> theTelecom) { 161 this.telecom = theTelecom; 162 return this; 163 } 164 165 public boolean hasTelecom() { 166 if (this.telecom == null) 167 return false; 168 for (ContactPoint item : this.telecom) 169 if (!item.isEmpty()) 170 return true; 171 return false; 172 } 173 174 public ContactPoint addTelecom() { // 3 175 ContactPoint t = new ContactPoint(); 176 if (this.telecom == null) 177 this.telecom = new ArrayList<ContactPoint>(); 178 this.telecom.add(t); 179 return t; 180 } 181 182 public InsurancePlanContactComponent addTelecom(ContactPoint t) { // 3 183 if (t == null) 184 return this; 185 if (this.telecom == null) 186 this.telecom = new ArrayList<ContactPoint>(); 187 this.telecom.add(t); 188 return this; 189 } 190 191 /** 192 * @return The first repetition of repeating field {@link #telecom}, creating it 193 * if it does not already exist 194 */ 195 public ContactPoint getTelecomFirstRep() { 196 if (getTelecom().isEmpty()) { 197 addTelecom(); 198 } 199 return getTelecom().get(0); 200 } 201 202 /** 203 * @return {@link #address} (Visiting or postal addresses for the contact.) 204 */ 205 public Address getAddress() { 206 if (this.address == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create InsurancePlanContactComponent.address"); 209 else if (Configuration.doAutoCreate()) 210 this.address = new Address(); // cc 211 return this.address; 212 } 213 214 public boolean hasAddress() { 215 return this.address != null && !this.address.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #address} (Visiting or postal addresses for the contact.) 220 */ 221 public InsurancePlanContactComponent setAddress(Address value) { 222 this.address = value; 223 return this; 224 } 225 226 protected void listChildren(List<Property> children) { 227 super.listChildren(children); 228 children.add(new Property("purpose", "CodeableConcept", 229 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose)); 230 children.add(new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name)); 231 children.add(new Property("telecom", "ContactPoint", 232 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 233 java.lang.Integer.MAX_VALUE, telecom)); 234 children.add(new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, address)); 235 } 236 237 @Override 238 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 239 switch (_hash) { 240 case -220463842: 241 /* purpose */ return new Property("purpose", "CodeableConcept", 242 "Indicates a purpose for which the contact can be reached.", 0, 1, purpose); 243 case 3373707: 244 /* name */ return new Property("name", "HumanName", "A name associated with the contact.", 0, 1, name); 245 case -1429363305: 246 /* telecom */ return new Property("telecom", "ContactPoint", 247 "A contact detail (e.g. a telephone number or an email address) by which the party may be contacted.", 0, 248 java.lang.Integer.MAX_VALUE, telecom); 249 case -1147692044: 250 /* address */ return new Property("address", "Address", "Visiting or postal addresses for the contact.", 0, 1, 251 address); 252 default: 253 return super.getNamedProperty(_hash, _name, _checkValid); 254 } 255 256 } 257 258 @Override 259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 260 switch (hash) { 261 case -220463842: 262 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // CodeableConcept 263 case 3373707: 264 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // HumanName 265 case -1429363305: 266 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 267 case -1147692044: 268 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 269 default: 270 return super.getProperty(hash, name, checkValid); 271 } 272 273 } 274 275 @Override 276 public Base setProperty(int hash, String name, Base value) throws FHIRException { 277 switch (hash) { 278 case -220463842: // purpose 279 this.purpose = castToCodeableConcept(value); // CodeableConcept 280 return value; 281 case 3373707: // name 282 this.name = castToHumanName(value); // HumanName 283 return value; 284 case -1429363305: // telecom 285 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 286 return value; 287 case -1147692044: // address 288 this.address = castToAddress(value); // Address 289 return value; 290 default: 291 return super.setProperty(hash, name, value); 292 } 293 294 } 295 296 @Override 297 public Base setProperty(String name, Base value) throws FHIRException { 298 if (name.equals("purpose")) { 299 this.purpose = castToCodeableConcept(value); // CodeableConcept 300 } else if (name.equals("name")) { 301 this.name = castToHumanName(value); // HumanName 302 } else if (name.equals("telecom")) { 303 this.getTelecom().add(castToContactPoint(value)); 304 } else if (name.equals("address")) { 305 this.address = castToAddress(value); // Address 306 } else 307 return super.setProperty(name, value); 308 return value; 309 } 310 311 @Override 312 public void removeChild(String name, Base value) throws FHIRException { 313 if (name.equals("purpose")) { 314 this.purpose = null; 315 } else if (name.equals("name")) { 316 this.name = null; 317 } else if (name.equals("telecom")) { 318 this.getTelecom().remove(castToContactPoint(value)); 319 } else if (name.equals("address")) { 320 this.address = null; 321 } else 322 super.removeChild(name, value); 323 324 } 325 326 @Override 327 public Base makeProperty(int hash, String name) throws FHIRException { 328 switch (hash) { 329 case -220463842: 330 return getPurpose(); 331 case 3373707: 332 return getName(); 333 case -1429363305: 334 return addTelecom(); 335 case -1147692044: 336 return getAddress(); 337 default: 338 return super.makeProperty(hash, name); 339 } 340 341 } 342 343 @Override 344 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 345 switch (hash) { 346 case -220463842: 347 /* purpose */ return new String[] { "CodeableConcept" }; 348 case 3373707: 349 /* name */ return new String[] { "HumanName" }; 350 case -1429363305: 351 /* telecom */ return new String[] { "ContactPoint" }; 352 case -1147692044: 353 /* address */ return new String[] { "Address" }; 354 default: 355 return super.getTypesForProperty(hash, name); 356 } 357 358 } 359 360 @Override 361 public Base addChild(String name) throws FHIRException { 362 if (name.equals("purpose")) { 363 this.purpose = new CodeableConcept(); 364 return this.purpose; 365 } else if (name.equals("name")) { 366 this.name = new HumanName(); 367 return this.name; 368 } else if (name.equals("telecom")) { 369 return addTelecom(); 370 } else if (name.equals("address")) { 371 this.address = new Address(); 372 return this.address; 373 } else 374 return super.addChild(name); 375 } 376 377 public InsurancePlanContactComponent copy() { 378 InsurancePlanContactComponent dst = new InsurancePlanContactComponent(); 379 copyValues(dst); 380 return dst; 381 } 382 383 public void copyValues(InsurancePlanContactComponent dst) { 384 super.copyValues(dst); 385 dst.purpose = purpose == null ? null : purpose.copy(); 386 dst.name = name == null ? null : name.copy(); 387 if (telecom != null) { 388 dst.telecom = new ArrayList<ContactPoint>(); 389 for (ContactPoint i : telecom) 390 dst.telecom.add(i.copy()); 391 } 392 ; 393 dst.address = address == null ? null : address.copy(); 394 } 395 396 @Override 397 public boolean equalsDeep(Base other_) { 398 if (!super.equalsDeep(other_)) 399 return false; 400 if (!(other_ instanceof InsurancePlanContactComponent)) 401 return false; 402 InsurancePlanContactComponent o = (InsurancePlanContactComponent) other_; 403 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) 404 && compareDeep(telecom, o.telecom, true) && compareDeep(address, o.address, true); 405 } 406 407 @Override 408 public boolean equalsShallow(Base other_) { 409 if (!super.equalsShallow(other_)) 410 return false; 411 if (!(other_ instanceof InsurancePlanContactComponent)) 412 return false; 413 InsurancePlanContactComponent o = (InsurancePlanContactComponent) other_; 414 return true; 415 } 416 417 public boolean isEmpty() { 418 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, name, telecom, address); 419 } 420 421 public String fhirType() { 422 return "InsurancePlan.contact"; 423 424 } 425 426 } 427 428 @Block() 429 public static class InsurancePlanCoverageComponent extends BackboneElement implements IBaseBackboneElement { 430 /** 431 * Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; 432 * Drug; Short Term; Long Term Care; Hospice; Home Health). 433 */ 434 @Child(name = "type", type = { 435 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 436 @Description(shortDefinition = "Type of coverage", formalDefinition = "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).") 437 protected CodeableConcept type; 438 439 /** 440 * Reference to the network that providing the type of coverage. 441 */ 442 @Child(name = "network", type = { 443 Organization.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 444 @Description(shortDefinition = "What networks provide coverage", formalDefinition = "Reference to the network that providing the type of coverage.") 445 protected List<Reference> network; 446 /** 447 * The actual objects that are the target of the reference (Reference to the 448 * network that providing the type of coverage.) 449 */ 450 protected List<Organization> networkTarget; 451 452 /** 453 * Specific benefits under this type of coverage. 454 */ 455 @Child(name = "benefit", type = {}, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 456 @Description(shortDefinition = "List of benefits", formalDefinition = "Specific benefits under this type of coverage.") 457 protected List<CoverageBenefitComponent> benefit; 458 459 private static final long serialVersionUID = -1186191877L; 460 461 /** 462 * Constructor 463 */ 464 public InsurancePlanCoverageComponent() { 465 super(); 466 } 467 468 /** 469 * Constructor 470 */ 471 public InsurancePlanCoverageComponent(CodeableConcept type) { 472 super(); 473 this.type = type; 474 } 475 476 /** 477 * @return {@link #type} (Type of coverage (Medical; Dental; Mental Health; 478 * Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; 479 * Home Health).) 480 */ 481 public CodeableConcept getType() { 482 if (this.type == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create InsurancePlanCoverageComponent.type"); 485 else if (Configuration.doAutoCreate()) 486 this.type = new CodeableConcept(); // cc 487 return this.type; 488 } 489 490 public boolean hasType() { 491 return this.type != null && !this.type.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #type} (Type of coverage (Medical; Dental; Mental Health; 496 * Substance Abuse; Vision; Drug; Short Term; Long Term Care; 497 * Hospice; Home Health).) 498 */ 499 public InsurancePlanCoverageComponent setType(CodeableConcept value) { 500 this.type = value; 501 return this; 502 } 503 504 /** 505 * @return {@link #network} (Reference to the network that providing the type of 506 * coverage.) 507 */ 508 public List<Reference> getNetwork() { 509 if (this.network == null) 510 this.network = new ArrayList<Reference>(); 511 return this.network; 512 } 513 514 /** 515 * @return Returns a reference to <code>this</code> for easy method chaining 516 */ 517 public InsurancePlanCoverageComponent setNetwork(List<Reference> theNetwork) { 518 this.network = theNetwork; 519 return this; 520 } 521 522 public boolean hasNetwork() { 523 if (this.network == null) 524 return false; 525 for (Reference item : this.network) 526 if (!item.isEmpty()) 527 return true; 528 return false; 529 } 530 531 public Reference addNetwork() { // 3 532 Reference t = new Reference(); 533 if (this.network == null) 534 this.network = new ArrayList<Reference>(); 535 this.network.add(t); 536 return t; 537 } 538 539 public InsurancePlanCoverageComponent addNetwork(Reference t) { // 3 540 if (t == null) 541 return this; 542 if (this.network == null) 543 this.network = new ArrayList<Reference>(); 544 this.network.add(t); 545 return this; 546 } 547 548 /** 549 * @return The first repetition of repeating field {@link #network}, creating it 550 * if it does not already exist 551 */ 552 public Reference getNetworkFirstRep() { 553 if (getNetwork().isEmpty()) { 554 addNetwork(); 555 } 556 return getNetwork().get(0); 557 } 558 559 /** 560 * @return {@link #benefit} (Specific benefits under this type of coverage.) 561 */ 562 public List<CoverageBenefitComponent> getBenefit() { 563 if (this.benefit == null) 564 this.benefit = new ArrayList<CoverageBenefitComponent>(); 565 return this.benefit; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public InsurancePlanCoverageComponent setBenefit(List<CoverageBenefitComponent> theBenefit) { 572 this.benefit = theBenefit; 573 return this; 574 } 575 576 public boolean hasBenefit() { 577 if (this.benefit == null) 578 return false; 579 for (CoverageBenefitComponent item : this.benefit) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 public CoverageBenefitComponent addBenefit() { // 3 586 CoverageBenefitComponent t = new CoverageBenefitComponent(); 587 if (this.benefit == null) 588 this.benefit = new ArrayList<CoverageBenefitComponent>(); 589 this.benefit.add(t); 590 return t; 591 } 592 593 public InsurancePlanCoverageComponent addBenefit(CoverageBenefitComponent t) { // 3 594 if (t == null) 595 return this; 596 if (this.benefit == null) 597 this.benefit = new ArrayList<CoverageBenefitComponent>(); 598 this.benefit.add(t); 599 return this; 600 } 601 602 /** 603 * @return The first repetition of repeating field {@link #benefit}, creating it 604 * if it does not already exist 605 */ 606 public CoverageBenefitComponent getBenefitFirstRep() { 607 if (getBenefit().isEmpty()) { 608 addBenefit(); 609 } 610 return getBenefit().get(0); 611 } 612 613 protected void listChildren(List<Property> children) { 614 super.listChildren(children); 615 children.add(new Property("type", "CodeableConcept", 616 "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 617 0, 1, type)); 618 children.add(new Property("network", "Reference(Organization)", 619 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 620 children.add(new Property("benefit", "", "Specific benefits under this type of coverage.", 0, 621 java.lang.Integer.MAX_VALUE, benefit)); 622 } 623 624 @Override 625 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 626 switch (_hash) { 627 case 3575610: 628 /* type */ return new Property("type", "CodeableConcept", 629 "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 630 0, 1, type); 631 case 1843485230: 632 /* network */ return new Property("network", "Reference(Organization)", 633 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 634 case -222710633: 635 /* benefit */ return new Property("benefit", "", "Specific benefits under this type of coverage.", 0, 636 java.lang.Integer.MAX_VALUE, benefit); 637 default: 638 return super.getNamedProperty(_hash, _name, _checkValid); 639 } 640 641 } 642 643 @Override 644 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 645 switch (hash) { 646 case 3575610: 647 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 648 case 1843485230: 649 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 650 case -222710633: 651 /* benefit */ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // CoverageBenefitComponent 652 default: 653 return super.getProperty(hash, name, checkValid); 654 } 655 656 } 657 658 @Override 659 public Base setProperty(int hash, String name, Base value) throws FHIRException { 660 switch (hash) { 661 case 3575610: // type 662 this.type = castToCodeableConcept(value); // CodeableConcept 663 return value; 664 case 1843485230: // network 665 this.getNetwork().add(castToReference(value)); // Reference 666 return value; 667 case -222710633: // benefit 668 this.getBenefit().add((CoverageBenefitComponent) value); // CoverageBenefitComponent 669 return value; 670 default: 671 return super.setProperty(hash, name, value); 672 } 673 674 } 675 676 @Override 677 public Base setProperty(String name, Base value) throws FHIRException { 678 if (name.equals("type")) { 679 this.type = castToCodeableConcept(value); // CodeableConcept 680 } else if (name.equals("network")) { 681 this.getNetwork().add(castToReference(value)); 682 } else if (name.equals("benefit")) { 683 this.getBenefit().add((CoverageBenefitComponent) value); 684 } else 685 return super.setProperty(name, value); 686 return value; 687 } 688 689 @Override 690 public void removeChild(String name, Base value) throws FHIRException { 691 if (name.equals("type")) { 692 this.type = null; 693 } else if (name.equals("network")) { 694 this.getNetwork().remove(castToReference(value)); 695 } else if (name.equals("benefit")) { 696 this.getBenefit().remove((CoverageBenefitComponent) value); 697 } else 698 super.removeChild(name, value); 699 700 } 701 702 @Override 703 public Base makeProperty(int hash, String name) throws FHIRException { 704 switch (hash) { 705 case 3575610: 706 return getType(); 707 case 1843485230: 708 return addNetwork(); 709 case -222710633: 710 return addBenefit(); 711 default: 712 return super.makeProperty(hash, name); 713 } 714 715 } 716 717 @Override 718 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 719 switch (hash) { 720 case 3575610: 721 /* type */ return new String[] { "CodeableConcept" }; 722 case 1843485230: 723 /* network */ return new String[] { "Reference" }; 724 case -222710633: 725 /* benefit */ return new String[] {}; 726 default: 727 return super.getTypesForProperty(hash, name); 728 } 729 730 } 731 732 @Override 733 public Base addChild(String name) throws FHIRException { 734 if (name.equals("type")) { 735 this.type = new CodeableConcept(); 736 return this.type; 737 } else if (name.equals("network")) { 738 return addNetwork(); 739 } else if (name.equals("benefit")) { 740 return addBenefit(); 741 } else 742 return super.addChild(name); 743 } 744 745 public InsurancePlanCoverageComponent copy() { 746 InsurancePlanCoverageComponent dst = new InsurancePlanCoverageComponent(); 747 copyValues(dst); 748 return dst; 749 } 750 751 public void copyValues(InsurancePlanCoverageComponent dst) { 752 super.copyValues(dst); 753 dst.type = type == null ? null : type.copy(); 754 if (network != null) { 755 dst.network = new ArrayList<Reference>(); 756 for (Reference i : network) 757 dst.network.add(i.copy()); 758 } 759 ; 760 if (benefit != null) { 761 dst.benefit = new ArrayList<CoverageBenefitComponent>(); 762 for (CoverageBenefitComponent i : benefit) 763 dst.benefit.add(i.copy()); 764 } 765 ; 766 } 767 768 @Override 769 public boolean equalsDeep(Base other_) { 770 if (!super.equalsDeep(other_)) 771 return false; 772 if (!(other_ instanceof InsurancePlanCoverageComponent)) 773 return false; 774 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 775 return compareDeep(type, o.type, true) && compareDeep(network, o.network, true) 776 && compareDeep(benefit, o.benefit, true); 777 } 778 779 @Override 780 public boolean equalsShallow(Base other_) { 781 if (!super.equalsShallow(other_)) 782 return false; 783 if (!(other_ instanceof InsurancePlanCoverageComponent)) 784 return false; 785 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 786 return true; 787 } 788 789 public boolean isEmpty() { 790 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, network, benefit); 791 } 792 793 public String fhirType() { 794 return "InsurancePlan.coverage"; 795 796 } 797 798 } 799 800 @Block() 801 public static class CoverageBenefitComponent extends BackboneElement implements IBaseBackboneElement { 802 /** 803 * Type of benefit (primary care; speciality care; inpatient; outpatient). 804 */ 805 @Child(name = "type", type = { 806 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 807 @Description(shortDefinition = "Type of benefit", formalDefinition = "Type of benefit (primary care; speciality care; inpatient; outpatient).") 808 protected CodeableConcept type; 809 810 /** 811 * The referral requirements to have access/coverage for this benefit. 812 */ 813 @Child(name = "requirement", type = { 814 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 815 @Description(shortDefinition = "Referral requirements", formalDefinition = "The referral requirements to have access/coverage for this benefit.") 816 protected StringType requirement; 817 818 /** 819 * The specific limits on the benefit. 820 */ 821 @Child(name = "limit", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 822 @Description(shortDefinition = "Benefit limits", formalDefinition = "The specific limits on the benefit.") 823 protected List<CoverageBenefitLimitComponent> limit; 824 825 private static final long serialVersionUID = -113658449L; 826 827 /** 828 * Constructor 829 */ 830 public CoverageBenefitComponent() { 831 super(); 832 } 833 834 /** 835 * Constructor 836 */ 837 public CoverageBenefitComponent(CodeableConcept type) { 838 super(); 839 this.type = type; 840 } 841 842 /** 843 * @return {@link #type} (Type of benefit (primary care; speciality care; 844 * inpatient; outpatient).) 845 */ 846 public CodeableConcept getType() { 847 if (this.type == null) 848 if (Configuration.errorOnAutoCreate()) 849 throw new Error("Attempt to auto-create CoverageBenefitComponent.type"); 850 else if (Configuration.doAutoCreate()) 851 this.type = new CodeableConcept(); // cc 852 return this.type; 853 } 854 855 public boolean hasType() { 856 return this.type != null && !this.type.isEmpty(); 857 } 858 859 /** 860 * @param value {@link #type} (Type of benefit (primary care; speciality care; 861 * inpatient; outpatient).) 862 */ 863 public CoverageBenefitComponent setType(CodeableConcept value) { 864 this.type = value; 865 return this; 866 } 867 868 /** 869 * @return {@link #requirement} (The referral requirements to have 870 * access/coverage for this benefit.). This is the underlying object 871 * with id, value and extensions. The accessor "getRequirement" gives 872 * direct access to the value 873 */ 874 public StringType getRequirementElement() { 875 if (this.requirement == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create CoverageBenefitComponent.requirement"); 878 else if (Configuration.doAutoCreate()) 879 this.requirement = new StringType(); // bb 880 return this.requirement; 881 } 882 883 public boolean hasRequirementElement() { 884 return this.requirement != null && !this.requirement.isEmpty(); 885 } 886 887 public boolean hasRequirement() { 888 return this.requirement != null && !this.requirement.isEmpty(); 889 } 890 891 /** 892 * @param value {@link #requirement} (The referral requirements to have 893 * access/coverage for this benefit.). This is the underlying 894 * object with id, value and extensions. The accessor 895 * "getRequirement" gives direct access to the value 896 */ 897 public CoverageBenefitComponent setRequirementElement(StringType value) { 898 this.requirement = value; 899 return this; 900 } 901 902 /** 903 * @return The referral requirements to have access/coverage for this benefit. 904 */ 905 public String getRequirement() { 906 return this.requirement == null ? null : this.requirement.getValue(); 907 } 908 909 /** 910 * @param value The referral requirements to have access/coverage for this 911 * benefit. 912 */ 913 public CoverageBenefitComponent setRequirement(String value) { 914 if (Utilities.noString(value)) 915 this.requirement = null; 916 else { 917 if (this.requirement == null) 918 this.requirement = new StringType(); 919 this.requirement.setValue(value); 920 } 921 return this; 922 } 923 924 /** 925 * @return {@link #limit} (The specific limits on the benefit.) 926 */ 927 public List<CoverageBenefitLimitComponent> getLimit() { 928 if (this.limit == null) 929 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 930 return this.limit; 931 } 932 933 /** 934 * @return Returns a reference to <code>this</code> for easy method chaining 935 */ 936 public CoverageBenefitComponent setLimit(List<CoverageBenefitLimitComponent> theLimit) { 937 this.limit = theLimit; 938 return this; 939 } 940 941 public boolean hasLimit() { 942 if (this.limit == null) 943 return false; 944 for (CoverageBenefitLimitComponent item : this.limit) 945 if (!item.isEmpty()) 946 return true; 947 return false; 948 } 949 950 public CoverageBenefitLimitComponent addLimit() { // 3 951 CoverageBenefitLimitComponent t = new CoverageBenefitLimitComponent(); 952 if (this.limit == null) 953 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 954 this.limit.add(t); 955 return t; 956 } 957 958 public CoverageBenefitComponent addLimit(CoverageBenefitLimitComponent t) { // 3 959 if (t == null) 960 return this; 961 if (this.limit == null) 962 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 963 this.limit.add(t); 964 return this; 965 } 966 967 /** 968 * @return The first repetition of repeating field {@link #limit}, creating it 969 * if it does not already exist 970 */ 971 public CoverageBenefitLimitComponent getLimitFirstRep() { 972 if (getLimit().isEmpty()) { 973 addLimit(); 974 } 975 return getLimit().get(0); 976 } 977 978 protected void listChildren(List<Property> children) { 979 super.listChildren(children); 980 children.add(new Property("type", "CodeableConcept", 981 "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type)); 982 children.add(new Property("requirement", "string", 983 "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement)); 984 children 985 .add(new Property("limit", "", "The specific limits on the benefit.", 0, java.lang.Integer.MAX_VALUE, limit)); 986 } 987 988 @Override 989 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 990 switch (_hash) { 991 case 3575610: 992 /* type */ return new Property("type", "CodeableConcept", 993 "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type); 994 case 363387971: 995 /* requirement */ return new Property("requirement", "string", 996 "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement); 997 case 102976443: 998 /* limit */ return new Property("limit", "", "The specific limits on the benefit.", 0, 999 java.lang.Integer.MAX_VALUE, limit); 1000 default: 1001 return super.getNamedProperty(_hash, _name, _checkValid); 1002 } 1003 1004 } 1005 1006 @Override 1007 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1008 switch (hash) { 1009 case 3575610: 1010 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1011 case 363387971: 1012 /* requirement */ return this.requirement == null ? new Base[0] : new Base[] { this.requirement }; // StringType 1013 case 102976443: 1014 /* limit */ return this.limit == null ? new Base[0] : this.limit.toArray(new Base[this.limit.size()]); // CoverageBenefitLimitComponent 1015 default: 1016 return super.getProperty(hash, name, checkValid); 1017 } 1018 1019 } 1020 1021 @Override 1022 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1023 switch (hash) { 1024 case 3575610: // type 1025 this.type = castToCodeableConcept(value); // CodeableConcept 1026 return value; 1027 case 363387971: // requirement 1028 this.requirement = castToString(value); // StringType 1029 return value; 1030 case 102976443: // limit 1031 this.getLimit().add((CoverageBenefitLimitComponent) value); // CoverageBenefitLimitComponent 1032 return value; 1033 default: 1034 return super.setProperty(hash, name, value); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base setProperty(String name, Base value) throws FHIRException { 1041 if (name.equals("type")) { 1042 this.type = castToCodeableConcept(value); // CodeableConcept 1043 } else if (name.equals("requirement")) { 1044 this.requirement = castToString(value); // StringType 1045 } else if (name.equals("limit")) { 1046 this.getLimit().add((CoverageBenefitLimitComponent) value); 1047 } else 1048 return super.setProperty(name, value); 1049 return value; 1050 } 1051 1052 @Override 1053 public void removeChild(String name, Base value) throws FHIRException { 1054 if (name.equals("type")) { 1055 this.type = null; 1056 } else if (name.equals("requirement")) { 1057 this.requirement = null; 1058 } else if (name.equals("limit")) { 1059 this.getLimit().remove((CoverageBenefitLimitComponent) value); 1060 } else 1061 super.removeChild(name, value); 1062 1063 } 1064 1065 @Override 1066 public Base makeProperty(int hash, String name) throws FHIRException { 1067 switch (hash) { 1068 case 3575610: 1069 return getType(); 1070 case 363387971: 1071 return getRequirementElement(); 1072 case 102976443: 1073 return addLimit(); 1074 default: 1075 return super.makeProperty(hash, name); 1076 } 1077 1078 } 1079 1080 @Override 1081 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1082 switch (hash) { 1083 case 3575610: 1084 /* type */ return new String[] { "CodeableConcept" }; 1085 case 363387971: 1086 /* requirement */ return new String[] { "string" }; 1087 case 102976443: 1088 /* limit */ return new String[] {}; 1089 default: 1090 return super.getTypesForProperty(hash, name); 1091 } 1092 1093 } 1094 1095 @Override 1096 public Base addChild(String name) throws FHIRException { 1097 if (name.equals("type")) { 1098 this.type = new CodeableConcept(); 1099 return this.type; 1100 } else if (name.equals("requirement")) { 1101 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.requirement"); 1102 } else if (name.equals("limit")) { 1103 return addLimit(); 1104 } else 1105 return super.addChild(name); 1106 } 1107 1108 public CoverageBenefitComponent copy() { 1109 CoverageBenefitComponent dst = new CoverageBenefitComponent(); 1110 copyValues(dst); 1111 return dst; 1112 } 1113 1114 public void copyValues(CoverageBenefitComponent dst) { 1115 super.copyValues(dst); 1116 dst.type = type == null ? null : type.copy(); 1117 dst.requirement = requirement == null ? null : requirement.copy(); 1118 if (limit != null) { 1119 dst.limit = new ArrayList<CoverageBenefitLimitComponent>(); 1120 for (CoverageBenefitLimitComponent i : limit) 1121 dst.limit.add(i.copy()); 1122 } 1123 ; 1124 } 1125 1126 @Override 1127 public boolean equalsDeep(Base other_) { 1128 if (!super.equalsDeep(other_)) 1129 return false; 1130 if (!(other_ instanceof CoverageBenefitComponent)) 1131 return false; 1132 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 1133 return compareDeep(type, o.type, true) && compareDeep(requirement, o.requirement, true) 1134 && compareDeep(limit, o.limit, true); 1135 } 1136 1137 @Override 1138 public boolean equalsShallow(Base other_) { 1139 if (!super.equalsShallow(other_)) 1140 return false; 1141 if (!(other_ instanceof CoverageBenefitComponent)) 1142 return false; 1143 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 1144 return compareValues(requirement, o.requirement, true); 1145 } 1146 1147 public boolean isEmpty() { 1148 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, requirement, limit); 1149 } 1150 1151 public String fhirType() { 1152 return "InsurancePlan.coverage.benefit"; 1153 1154 } 1155 1156 } 1157 1158 @Block() 1159 public static class CoverageBenefitLimitComponent extends BackboneElement implements IBaseBackboneElement { 1160 /** 1161 * The maximum amount of a service item a plan will pay for a covered benefit. 1162 * For examples. wellness visits, or eyeglasses. 1163 */ 1164 @Child(name = "value", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1165 @Description(shortDefinition = "Maximum value allowed", formalDefinition = "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.") 1166 protected Quantity value; 1167 1168 /** 1169 * The specific limit on the benefit. 1170 */ 1171 @Child(name = "code", type = { 1172 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1173 @Description(shortDefinition = "Benefit limit details", formalDefinition = "The specific limit on the benefit.") 1174 protected CodeableConcept code; 1175 1176 private static final long serialVersionUID = -304318128L; 1177 1178 /** 1179 * Constructor 1180 */ 1181 public CoverageBenefitLimitComponent() { 1182 super(); 1183 } 1184 1185 /** 1186 * @return {@link #value} (The maximum amount of a service item a plan will pay 1187 * for a covered benefit. For examples. wellness visits, or eyeglasses.) 1188 */ 1189 public Quantity getValue() { 1190 if (this.value == null) 1191 if (Configuration.errorOnAutoCreate()) 1192 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.value"); 1193 else if (Configuration.doAutoCreate()) 1194 this.value = new Quantity(); // cc 1195 return this.value; 1196 } 1197 1198 public boolean hasValue() { 1199 return this.value != null && !this.value.isEmpty(); 1200 } 1201 1202 /** 1203 * @param value {@link #value} (The maximum amount of a service item a plan will 1204 * pay for a covered benefit. For examples. wellness visits, or 1205 * eyeglasses.) 1206 */ 1207 public CoverageBenefitLimitComponent setValue(Quantity value) { 1208 this.value = value; 1209 return this; 1210 } 1211 1212 /** 1213 * @return {@link #code} (The specific limit on the benefit.) 1214 */ 1215 public CodeableConcept getCode() { 1216 if (this.code == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.code"); 1219 else if (Configuration.doAutoCreate()) 1220 this.code = new CodeableConcept(); // cc 1221 return this.code; 1222 } 1223 1224 public boolean hasCode() { 1225 return this.code != null && !this.code.isEmpty(); 1226 } 1227 1228 /** 1229 * @param value {@link #code} (The specific limit on the benefit.) 1230 */ 1231 public CoverageBenefitLimitComponent setCode(CodeableConcept value) { 1232 this.code = value; 1233 return this; 1234 } 1235 1236 protected void listChildren(List<Property> children) { 1237 super.listChildren(children); 1238 children.add(new Property("value", "Quantity", 1239 "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 1240 0, 1, value)); 1241 children.add(new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code)); 1242 } 1243 1244 @Override 1245 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1246 switch (_hash) { 1247 case 111972721: 1248 /* value */ return new Property("value", "Quantity", 1249 "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 1250 0, 1, value); 1251 case 3059181: 1252 /* code */ return new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code); 1253 default: 1254 return super.getNamedProperty(_hash, _name, _checkValid); 1255 } 1256 1257 } 1258 1259 @Override 1260 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1261 switch (hash) { 1262 case 111972721: 1263 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 1264 case 3059181: 1265 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1266 default: 1267 return super.getProperty(hash, name, checkValid); 1268 } 1269 1270 } 1271 1272 @Override 1273 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1274 switch (hash) { 1275 case 111972721: // value 1276 this.value = castToQuantity(value); // Quantity 1277 return value; 1278 case 3059181: // code 1279 this.code = castToCodeableConcept(value); // CodeableConcept 1280 return value; 1281 default: 1282 return super.setProperty(hash, name, value); 1283 } 1284 1285 } 1286 1287 @Override 1288 public Base setProperty(String name, Base value) throws FHIRException { 1289 if (name.equals("value")) { 1290 this.value = castToQuantity(value); // Quantity 1291 } else if (name.equals("code")) { 1292 this.code = castToCodeableConcept(value); // CodeableConcept 1293 } else 1294 return super.setProperty(name, value); 1295 return value; 1296 } 1297 1298 @Override 1299 public void removeChild(String name, Base value) throws FHIRException { 1300 if (name.equals("value")) { 1301 this.value = null; 1302 } else if (name.equals("code")) { 1303 this.code = null; 1304 } else 1305 super.removeChild(name, value); 1306 1307 } 1308 1309 @Override 1310 public Base makeProperty(int hash, String name) throws FHIRException { 1311 switch (hash) { 1312 case 111972721: 1313 return getValue(); 1314 case 3059181: 1315 return getCode(); 1316 default: 1317 return super.makeProperty(hash, name); 1318 } 1319 1320 } 1321 1322 @Override 1323 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1324 switch (hash) { 1325 case 111972721: 1326 /* value */ return new String[] { "Quantity" }; 1327 case 3059181: 1328 /* code */ return new String[] { "CodeableConcept" }; 1329 default: 1330 return super.getTypesForProperty(hash, name); 1331 } 1332 1333 } 1334 1335 @Override 1336 public Base addChild(String name) throws FHIRException { 1337 if (name.equals("value")) { 1338 this.value = new Quantity(); 1339 return this.value; 1340 } else if (name.equals("code")) { 1341 this.code = new CodeableConcept(); 1342 return this.code; 1343 } else 1344 return super.addChild(name); 1345 } 1346 1347 public CoverageBenefitLimitComponent copy() { 1348 CoverageBenefitLimitComponent dst = new CoverageBenefitLimitComponent(); 1349 copyValues(dst); 1350 return dst; 1351 } 1352 1353 public void copyValues(CoverageBenefitLimitComponent dst) { 1354 super.copyValues(dst); 1355 dst.value = value == null ? null : value.copy(); 1356 dst.code = code == null ? null : code.copy(); 1357 } 1358 1359 @Override 1360 public boolean equalsDeep(Base other_) { 1361 if (!super.equalsDeep(other_)) 1362 return false; 1363 if (!(other_ instanceof CoverageBenefitLimitComponent)) 1364 return false; 1365 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 1366 return compareDeep(value, o.value, true) && compareDeep(code, o.code, true); 1367 } 1368 1369 @Override 1370 public boolean equalsShallow(Base other_) { 1371 if (!super.equalsShallow(other_)) 1372 return false; 1373 if (!(other_ instanceof CoverageBenefitLimitComponent)) 1374 return false; 1375 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 1376 return true; 1377 } 1378 1379 public boolean isEmpty() { 1380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, code); 1381 } 1382 1383 public String fhirType() { 1384 return "InsurancePlan.coverage.benefit.limit"; 1385 1386 } 1387 1388 } 1389 1390 @Block() 1391 public static class InsurancePlanPlanComponent extends BackboneElement implements IBaseBackboneElement { 1392 /** 1393 * Business identifiers assigned to this health insurance plan which remain 1394 * constant as the resource is updated and propagates from server to server. 1395 */ 1396 @Child(name = "identifier", type = { 1397 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1398 @Description(shortDefinition = "Business Identifier for Product", formalDefinition = "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.") 1399 protected List<Identifier> identifier; 1400 1401 /** 1402 * Type of plan. For example, "Platinum" or "High Deductable". 1403 */ 1404 @Child(name = "type", type = { 1405 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1406 @Description(shortDefinition = "Type of plan", formalDefinition = "Type of plan. For example, \"Platinum\" or \"High Deductable\".") 1407 protected CodeableConcept type; 1408 1409 /** 1410 * The geographic region in which a health insurance plan's benefits apply. 1411 */ 1412 @Child(name = "coverageArea", type = { 1413 Location.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1414 @Description(shortDefinition = "Where product applies", formalDefinition = "The geographic region in which a health insurance plan's benefits apply.") 1415 protected List<Reference> coverageArea; 1416 /** 1417 * The actual objects that are the target of the reference (The geographic 1418 * region in which a health insurance plan's benefits apply.) 1419 */ 1420 protected List<Location> coverageAreaTarget; 1421 1422 /** 1423 * Reference to the network that providing the type of coverage. 1424 */ 1425 @Child(name = "network", type = { 1426 Organization.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1427 @Description(shortDefinition = "What networks provide coverage", formalDefinition = "Reference to the network that providing the type of coverage.") 1428 protected List<Reference> network; 1429 /** 1430 * The actual objects that are the target of the reference (Reference to the 1431 * network that providing the type of coverage.) 1432 */ 1433 protected List<Organization> networkTarget; 1434 1435 /** 1436 * Overall costs associated with the plan. 1437 */ 1438 @Child(name = "generalCost", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1439 @Description(shortDefinition = "Overall costs", formalDefinition = "Overall costs associated with the plan.") 1440 protected List<InsurancePlanPlanGeneralCostComponent> generalCost; 1441 1442 /** 1443 * Costs associated with the coverage provided by the product. 1444 */ 1445 @Child(name = "specificCost", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1446 @Description(shortDefinition = "Specific costs", formalDefinition = "Costs associated with the coverage provided by the product.") 1447 protected List<InsurancePlanPlanSpecificCostComponent> specificCost; 1448 1449 private static final long serialVersionUID = -2063324071L; 1450 1451 /** 1452 * Constructor 1453 */ 1454 public InsurancePlanPlanComponent() { 1455 super(); 1456 } 1457 1458 /** 1459 * @return {@link #identifier} (Business identifiers assigned to this health 1460 * insurance plan which remain constant as the resource is updated and 1461 * propagates from server to server.) 1462 */ 1463 public List<Identifier> getIdentifier() { 1464 if (this.identifier == null) 1465 this.identifier = new ArrayList<Identifier>(); 1466 return this.identifier; 1467 } 1468 1469 /** 1470 * @return Returns a reference to <code>this</code> for easy method chaining 1471 */ 1472 public InsurancePlanPlanComponent setIdentifier(List<Identifier> theIdentifier) { 1473 this.identifier = theIdentifier; 1474 return this; 1475 } 1476 1477 public boolean hasIdentifier() { 1478 if (this.identifier == null) 1479 return false; 1480 for (Identifier item : this.identifier) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 public Identifier addIdentifier() { // 3 1487 Identifier t = new Identifier(); 1488 if (this.identifier == null) 1489 this.identifier = new ArrayList<Identifier>(); 1490 this.identifier.add(t); 1491 return t; 1492 } 1493 1494 public InsurancePlanPlanComponent addIdentifier(Identifier t) { // 3 1495 if (t == null) 1496 return this; 1497 if (this.identifier == null) 1498 this.identifier = new ArrayList<Identifier>(); 1499 this.identifier.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return The first repetition of repeating field {@link #identifier}, creating 1505 * it if it does not already exist 1506 */ 1507 public Identifier getIdentifierFirstRep() { 1508 if (getIdentifier().isEmpty()) { 1509 addIdentifier(); 1510 } 1511 return getIdentifier().get(0); 1512 } 1513 1514 /** 1515 * @return {@link #type} (Type of plan. For example, "Platinum" or "High 1516 * Deductable".) 1517 */ 1518 public CodeableConcept getType() { 1519 if (this.type == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create InsurancePlanPlanComponent.type"); 1522 else if (Configuration.doAutoCreate()) 1523 this.type = new CodeableConcept(); // cc 1524 return this.type; 1525 } 1526 1527 public boolean hasType() { 1528 return this.type != null && !this.type.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #type} (Type of plan. For example, "Platinum" or "High 1533 * Deductable".) 1534 */ 1535 public InsurancePlanPlanComponent setType(CodeableConcept value) { 1536 this.type = value; 1537 return this; 1538 } 1539 1540 /** 1541 * @return {@link #coverageArea} (The geographic region in which a health 1542 * insurance plan's benefits apply.) 1543 */ 1544 public List<Reference> getCoverageArea() { 1545 if (this.coverageArea == null) 1546 this.coverageArea = new ArrayList<Reference>(); 1547 return this.coverageArea; 1548 } 1549 1550 /** 1551 * @return Returns a reference to <code>this</code> for easy method chaining 1552 */ 1553 public InsurancePlanPlanComponent setCoverageArea(List<Reference> theCoverageArea) { 1554 this.coverageArea = theCoverageArea; 1555 return this; 1556 } 1557 1558 public boolean hasCoverageArea() { 1559 if (this.coverageArea == null) 1560 return false; 1561 for (Reference item : this.coverageArea) 1562 if (!item.isEmpty()) 1563 return true; 1564 return false; 1565 } 1566 1567 public Reference addCoverageArea() { // 3 1568 Reference t = new Reference(); 1569 if (this.coverageArea == null) 1570 this.coverageArea = new ArrayList<Reference>(); 1571 this.coverageArea.add(t); 1572 return t; 1573 } 1574 1575 public InsurancePlanPlanComponent addCoverageArea(Reference t) { // 3 1576 if (t == null) 1577 return this; 1578 if (this.coverageArea == null) 1579 this.coverageArea = new ArrayList<Reference>(); 1580 this.coverageArea.add(t); 1581 return this; 1582 } 1583 1584 /** 1585 * @return The first repetition of repeating field {@link #coverageArea}, 1586 * creating it if it does not already exist 1587 */ 1588 public Reference getCoverageAreaFirstRep() { 1589 if (getCoverageArea().isEmpty()) { 1590 addCoverageArea(); 1591 } 1592 return getCoverageArea().get(0); 1593 } 1594 1595 /** 1596 * @return {@link #network} (Reference to the network that providing the type of 1597 * coverage.) 1598 */ 1599 public List<Reference> getNetwork() { 1600 if (this.network == null) 1601 this.network = new ArrayList<Reference>(); 1602 return this.network; 1603 } 1604 1605 /** 1606 * @return Returns a reference to <code>this</code> for easy method chaining 1607 */ 1608 public InsurancePlanPlanComponent setNetwork(List<Reference> theNetwork) { 1609 this.network = theNetwork; 1610 return this; 1611 } 1612 1613 public boolean hasNetwork() { 1614 if (this.network == null) 1615 return false; 1616 for (Reference item : this.network) 1617 if (!item.isEmpty()) 1618 return true; 1619 return false; 1620 } 1621 1622 public Reference addNetwork() { // 3 1623 Reference t = new Reference(); 1624 if (this.network == null) 1625 this.network = new ArrayList<Reference>(); 1626 this.network.add(t); 1627 return t; 1628 } 1629 1630 public InsurancePlanPlanComponent addNetwork(Reference t) { // 3 1631 if (t == null) 1632 return this; 1633 if (this.network == null) 1634 this.network = new ArrayList<Reference>(); 1635 this.network.add(t); 1636 return this; 1637 } 1638 1639 /** 1640 * @return The first repetition of repeating field {@link #network}, creating it 1641 * if it does not already exist 1642 */ 1643 public Reference getNetworkFirstRep() { 1644 if (getNetwork().isEmpty()) { 1645 addNetwork(); 1646 } 1647 return getNetwork().get(0); 1648 } 1649 1650 /** 1651 * @return {@link #generalCost} (Overall costs associated with the plan.) 1652 */ 1653 public List<InsurancePlanPlanGeneralCostComponent> getGeneralCost() { 1654 if (this.generalCost == null) 1655 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1656 return this.generalCost; 1657 } 1658 1659 /** 1660 * @return Returns a reference to <code>this</code> for easy method chaining 1661 */ 1662 public InsurancePlanPlanComponent setGeneralCost(List<InsurancePlanPlanGeneralCostComponent> theGeneralCost) { 1663 this.generalCost = theGeneralCost; 1664 return this; 1665 } 1666 1667 public boolean hasGeneralCost() { 1668 if (this.generalCost == null) 1669 return false; 1670 for (InsurancePlanPlanGeneralCostComponent item : this.generalCost) 1671 if (!item.isEmpty()) 1672 return true; 1673 return false; 1674 } 1675 1676 public InsurancePlanPlanGeneralCostComponent addGeneralCost() { // 3 1677 InsurancePlanPlanGeneralCostComponent t = new InsurancePlanPlanGeneralCostComponent(); 1678 if (this.generalCost == null) 1679 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1680 this.generalCost.add(t); 1681 return t; 1682 } 1683 1684 public InsurancePlanPlanComponent addGeneralCost(InsurancePlanPlanGeneralCostComponent t) { // 3 1685 if (t == null) 1686 return this; 1687 if (this.generalCost == null) 1688 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1689 this.generalCost.add(t); 1690 return this; 1691 } 1692 1693 /** 1694 * @return The first repetition of repeating field {@link #generalCost}, 1695 * creating it if it does not already exist 1696 */ 1697 public InsurancePlanPlanGeneralCostComponent getGeneralCostFirstRep() { 1698 if (getGeneralCost().isEmpty()) { 1699 addGeneralCost(); 1700 } 1701 return getGeneralCost().get(0); 1702 } 1703 1704 /** 1705 * @return {@link #specificCost} (Costs associated with the coverage provided by 1706 * the product.) 1707 */ 1708 public List<InsurancePlanPlanSpecificCostComponent> getSpecificCost() { 1709 if (this.specificCost == null) 1710 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1711 return this.specificCost; 1712 } 1713 1714 /** 1715 * @return Returns a reference to <code>this</code> for easy method chaining 1716 */ 1717 public InsurancePlanPlanComponent setSpecificCost(List<InsurancePlanPlanSpecificCostComponent> theSpecificCost) { 1718 this.specificCost = theSpecificCost; 1719 return this; 1720 } 1721 1722 public boolean hasSpecificCost() { 1723 if (this.specificCost == null) 1724 return false; 1725 for (InsurancePlanPlanSpecificCostComponent item : this.specificCost) 1726 if (!item.isEmpty()) 1727 return true; 1728 return false; 1729 } 1730 1731 public InsurancePlanPlanSpecificCostComponent addSpecificCost() { // 3 1732 InsurancePlanPlanSpecificCostComponent t = new InsurancePlanPlanSpecificCostComponent(); 1733 if (this.specificCost == null) 1734 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1735 this.specificCost.add(t); 1736 return t; 1737 } 1738 1739 public InsurancePlanPlanComponent addSpecificCost(InsurancePlanPlanSpecificCostComponent t) { // 3 1740 if (t == null) 1741 return this; 1742 if (this.specificCost == null) 1743 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1744 this.specificCost.add(t); 1745 return this; 1746 } 1747 1748 /** 1749 * @return The first repetition of repeating field {@link #specificCost}, 1750 * creating it if it does not already exist 1751 */ 1752 public InsurancePlanPlanSpecificCostComponent getSpecificCostFirstRep() { 1753 if (getSpecificCost().isEmpty()) { 1754 addSpecificCost(); 1755 } 1756 return getSpecificCost().get(0); 1757 } 1758 1759 protected void listChildren(List<Property> children) { 1760 super.listChildren(children); 1761 children.add(new Property("identifier", "Identifier", 1762 "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 1763 0, java.lang.Integer.MAX_VALUE, identifier)); 1764 children.add(new Property("type", "CodeableConcept", 1765 "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type)); 1766 children.add(new Property("coverageArea", "Reference(Location)", 1767 "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 1768 coverageArea)); 1769 children.add(new Property("network", "Reference(Organization)", 1770 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 1771 children.add(new Property("generalCost", "", "Overall costs associated with the plan.", 0, 1772 java.lang.Integer.MAX_VALUE, generalCost)); 1773 children.add(new Property("specificCost", "", "Costs associated with the coverage provided by the product.", 0, 1774 java.lang.Integer.MAX_VALUE, specificCost)); 1775 } 1776 1777 @Override 1778 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1779 switch (_hash) { 1780 case -1618432855: 1781 /* identifier */ return new Property("identifier", "Identifier", 1782 "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 1783 0, java.lang.Integer.MAX_VALUE, identifier); 1784 case 3575610: 1785 /* type */ return new Property("type", "CodeableConcept", 1786 "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type); 1787 case -1532328299: 1788 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 1789 "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 1790 coverageArea); 1791 case 1843485230: 1792 /* network */ return new Property("network", "Reference(Organization)", 1793 "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 1794 case 878344405: 1795 /* generalCost */ return new Property("generalCost", "", "Overall costs associated with the plan.", 0, 1796 java.lang.Integer.MAX_VALUE, generalCost); 1797 case -1205656545: 1798 /* specificCost */ return new Property("specificCost", "", 1799 "Costs associated with the coverage provided by the product.", 0, java.lang.Integer.MAX_VALUE, 1800 specificCost); 1801 default: 1802 return super.getNamedProperty(_hash, _name, _checkValid); 1803 } 1804 1805 } 1806 1807 @Override 1808 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1809 switch (hash) { 1810 case -1618432855: 1811 /* identifier */ return this.identifier == null ? new Base[0] 1812 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1813 case 3575610: 1814 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1815 case -1532328299: 1816 /* coverageArea */ return this.coverageArea == null ? new Base[0] 1817 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 1818 case 1843485230: 1819 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 1820 case 878344405: 1821 /* generalCost */ return this.generalCost == null ? new Base[0] 1822 : this.generalCost.toArray(new Base[this.generalCost.size()]); // InsurancePlanPlanGeneralCostComponent 1823 case -1205656545: 1824 /* specificCost */ return this.specificCost == null ? new Base[0] 1825 : this.specificCost.toArray(new Base[this.specificCost.size()]); // InsurancePlanPlanSpecificCostComponent 1826 default: 1827 return super.getProperty(hash, name, checkValid); 1828 } 1829 1830 } 1831 1832 @Override 1833 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1834 switch (hash) { 1835 case -1618432855: // identifier 1836 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1837 return value; 1838 case 3575610: // type 1839 this.type = castToCodeableConcept(value); // CodeableConcept 1840 return value; 1841 case -1532328299: // coverageArea 1842 this.getCoverageArea().add(castToReference(value)); // Reference 1843 return value; 1844 case 1843485230: // network 1845 this.getNetwork().add(castToReference(value)); // Reference 1846 return value; 1847 case 878344405: // generalCost 1848 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); // InsurancePlanPlanGeneralCostComponent 1849 return value; 1850 case -1205656545: // specificCost 1851 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); // InsurancePlanPlanSpecificCostComponent 1852 return value; 1853 default: 1854 return super.setProperty(hash, name, value); 1855 } 1856 1857 } 1858 1859 @Override 1860 public Base setProperty(String name, Base value) throws FHIRException { 1861 if (name.equals("identifier")) { 1862 this.getIdentifier().add(castToIdentifier(value)); 1863 } else if (name.equals("type")) { 1864 this.type = castToCodeableConcept(value); // CodeableConcept 1865 } else if (name.equals("coverageArea")) { 1866 this.getCoverageArea().add(castToReference(value)); 1867 } else if (name.equals("network")) { 1868 this.getNetwork().add(castToReference(value)); 1869 } else if (name.equals("generalCost")) { 1870 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); 1871 } else if (name.equals("specificCost")) { 1872 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); 1873 } else 1874 return super.setProperty(name, value); 1875 return value; 1876 } 1877 1878 @Override 1879 public void removeChild(String name, Base value) throws FHIRException { 1880 if (name.equals("identifier")) { 1881 this.getIdentifier().remove(castToIdentifier(value)); 1882 } else if (name.equals("type")) { 1883 this.type = null; 1884 } else if (name.equals("coverageArea")) { 1885 this.getCoverageArea().remove(castToReference(value)); 1886 } else if (name.equals("network")) { 1887 this.getNetwork().remove(castToReference(value)); 1888 } else if (name.equals("generalCost")) { 1889 this.getGeneralCost().remove((InsurancePlanPlanGeneralCostComponent) value); 1890 } else if (name.equals("specificCost")) { 1891 this.getSpecificCost().remove((InsurancePlanPlanSpecificCostComponent) value); 1892 } else 1893 super.removeChild(name, value); 1894 1895 } 1896 1897 @Override 1898 public Base makeProperty(int hash, String name) throws FHIRException { 1899 switch (hash) { 1900 case -1618432855: 1901 return addIdentifier(); 1902 case 3575610: 1903 return getType(); 1904 case -1532328299: 1905 return addCoverageArea(); 1906 case 1843485230: 1907 return addNetwork(); 1908 case 878344405: 1909 return addGeneralCost(); 1910 case -1205656545: 1911 return addSpecificCost(); 1912 default: 1913 return super.makeProperty(hash, name); 1914 } 1915 1916 } 1917 1918 @Override 1919 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1920 switch (hash) { 1921 case -1618432855: 1922 /* identifier */ return new String[] { "Identifier" }; 1923 case 3575610: 1924 /* type */ return new String[] { "CodeableConcept" }; 1925 case -1532328299: 1926 /* coverageArea */ return new String[] { "Reference" }; 1927 case 1843485230: 1928 /* network */ return new String[] { "Reference" }; 1929 case 878344405: 1930 /* generalCost */ return new String[] {}; 1931 case -1205656545: 1932 /* specificCost */ return new String[] {}; 1933 default: 1934 return super.getTypesForProperty(hash, name); 1935 } 1936 1937 } 1938 1939 @Override 1940 public Base addChild(String name) throws FHIRException { 1941 if (name.equals("identifier")) { 1942 return addIdentifier(); 1943 } else if (name.equals("type")) { 1944 this.type = new CodeableConcept(); 1945 return this.type; 1946 } else if (name.equals("coverageArea")) { 1947 return addCoverageArea(); 1948 } else if (name.equals("network")) { 1949 return addNetwork(); 1950 } else if (name.equals("generalCost")) { 1951 return addGeneralCost(); 1952 } else if (name.equals("specificCost")) { 1953 return addSpecificCost(); 1954 } else 1955 return super.addChild(name); 1956 } 1957 1958 public InsurancePlanPlanComponent copy() { 1959 InsurancePlanPlanComponent dst = new InsurancePlanPlanComponent(); 1960 copyValues(dst); 1961 return dst; 1962 } 1963 1964 public void copyValues(InsurancePlanPlanComponent dst) { 1965 super.copyValues(dst); 1966 if (identifier != null) { 1967 dst.identifier = new ArrayList<Identifier>(); 1968 for (Identifier i : identifier) 1969 dst.identifier.add(i.copy()); 1970 } 1971 ; 1972 dst.type = type == null ? null : type.copy(); 1973 if (coverageArea != null) { 1974 dst.coverageArea = new ArrayList<Reference>(); 1975 for (Reference i : coverageArea) 1976 dst.coverageArea.add(i.copy()); 1977 } 1978 ; 1979 if (network != null) { 1980 dst.network = new ArrayList<Reference>(); 1981 for (Reference i : network) 1982 dst.network.add(i.copy()); 1983 } 1984 ; 1985 if (generalCost != null) { 1986 dst.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1987 for (InsurancePlanPlanGeneralCostComponent i : generalCost) 1988 dst.generalCost.add(i.copy()); 1989 } 1990 ; 1991 if (specificCost != null) { 1992 dst.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1993 for (InsurancePlanPlanSpecificCostComponent i : specificCost) 1994 dst.specificCost.add(i.copy()); 1995 } 1996 ; 1997 } 1998 1999 @Override 2000 public boolean equalsDeep(Base other_) { 2001 if (!super.equalsDeep(other_)) 2002 return false; 2003 if (!(other_ instanceof InsurancePlanPlanComponent)) 2004 return false; 2005 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 2006 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 2007 && compareDeep(coverageArea, o.coverageArea, true) && compareDeep(network, o.network, true) 2008 && compareDeep(generalCost, o.generalCost, true) && compareDeep(specificCost, o.specificCost, true); 2009 } 2010 2011 @Override 2012 public boolean equalsShallow(Base other_) { 2013 if (!super.equalsShallow(other_)) 2014 return false; 2015 if (!(other_ instanceof InsurancePlanPlanComponent)) 2016 return false; 2017 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 2018 return true; 2019 } 2020 2021 public boolean isEmpty() { 2022 return super.isEmpty() 2023 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coverageArea, network, generalCost, specificCost); 2024 } 2025 2026 public String fhirType() { 2027 return "InsurancePlan.plan"; 2028 2029 } 2030 2031 } 2032 2033 @Block() 2034 public static class InsurancePlanPlanGeneralCostComponent extends BackboneElement implements IBaseBackboneElement { 2035 /** 2036 * Type of cost. 2037 */ 2038 @Child(name = "type", type = { 2039 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2040 @Description(shortDefinition = "Type of cost", formalDefinition = "Type of cost.") 2041 protected CodeableConcept type; 2042 2043 /** 2044 * Number of participants enrolled in the plan. 2045 */ 2046 @Child(name = "groupSize", type = { 2047 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2048 @Description(shortDefinition = "Number of enrollees", formalDefinition = "Number of participants enrolled in the plan.") 2049 protected PositiveIntType groupSize; 2050 2051 /** 2052 * Value of the cost. 2053 */ 2054 @Child(name = "cost", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2055 @Description(shortDefinition = "Cost value", formalDefinition = "Value of the cost.") 2056 protected Money cost; 2057 2058 /** 2059 * Additional information about the general costs associated with this plan. 2060 */ 2061 @Child(name = "comment", type = { 2062 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2063 @Description(shortDefinition = "Additional cost information", formalDefinition = "Additional information about the general costs associated with this plan.") 2064 protected StringType comment; 2065 2066 private static final long serialVersionUID = 1563949866L; 2067 2068 /** 2069 * Constructor 2070 */ 2071 public InsurancePlanPlanGeneralCostComponent() { 2072 super(); 2073 } 2074 2075 /** 2076 * @return {@link #type} (Type of cost.) 2077 */ 2078 public CodeableConcept getType() { 2079 if (this.type == null) 2080 if (Configuration.errorOnAutoCreate()) 2081 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.type"); 2082 else if (Configuration.doAutoCreate()) 2083 this.type = new CodeableConcept(); // cc 2084 return this.type; 2085 } 2086 2087 public boolean hasType() { 2088 return this.type != null && !this.type.isEmpty(); 2089 } 2090 2091 /** 2092 * @param value {@link #type} (Type of cost.) 2093 */ 2094 public InsurancePlanPlanGeneralCostComponent setType(CodeableConcept value) { 2095 this.type = value; 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #groupSize} (Number of participants enrolled in the plan.). 2101 * This is the underlying object with id, value and extensions. The 2102 * accessor "getGroupSize" gives direct access to the value 2103 */ 2104 public PositiveIntType getGroupSizeElement() { 2105 if (this.groupSize == null) 2106 if (Configuration.errorOnAutoCreate()) 2107 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.groupSize"); 2108 else if (Configuration.doAutoCreate()) 2109 this.groupSize = new PositiveIntType(); // bb 2110 return this.groupSize; 2111 } 2112 2113 public boolean hasGroupSizeElement() { 2114 return this.groupSize != null && !this.groupSize.isEmpty(); 2115 } 2116 2117 public boolean hasGroupSize() { 2118 return this.groupSize != null && !this.groupSize.isEmpty(); 2119 } 2120 2121 /** 2122 * @param value {@link #groupSize} (Number of participants enrolled in the 2123 * plan.). This is the underlying object with id, value and 2124 * extensions. The accessor "getGroupSize" gives direct access to 2125 * the value 2126 */ 2127 public InsurancePlanPlanGeneralCostComponent setGroupSizeElement(PositiveIntType value) { 2128 this.groupSize = value; 2129 return this; 2130 } 2131 2132 /** 2133 * @return Number of participants enrolled in the plan. 2134 */ 2135 public int getGroupSize() { 2136 return this.groupSize == null || this.groupSize.isEmpty() ? 0 : this.groupSize.getValue(); 2137 } 2138 2139 /** 2140 * @param value Number of participants enrolled in the plan. 2141 */ 2142 public InsurancePlanPlanGeneralCostComponent setGroupSize(int value) { 2143 if (this.groupSize == null) 2144 this.groupSize = new PositiveIntType(); 2145 this.groupSize.setValue(value); 2146 return this; 2147 } 2148 2149 /** 2150 * @return {@link #cost} (Value of the cost.) 2151 */ 2152 public Money getCost() { 2153 if (this.cost == null) 2154 if (Configuration.errorOnAutoCreate()) 2155 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.cost"); 2156 else if (Configuration.doAutoCreate()) 2157 this.cost = new Money(); // cc 2158 return this.cost; 2159 } 2160 2161 public boolean hasCost() { 2162 return this.cost != null && !this.cost.isEmpty(); 2163 } 2164 2165 /** 2166 * @param value {@link #cost} (Value of the cost.) 2167 */ 2168 public InsurancePlanPlanGeneralCostComponent setCost(Money value) { 2169 this.cost = value; 2170 return this; 2171 } 2172 2173 /** 2174 * @return {@link #comment} (Additional information about the general costs 2175 * associated with this plan.). This is the underlying object with id, 2176 * value and extensions. The accessor "getComment" gives direct access 2177 * to the value 2178 */ 2179 public StringType getCommentElement() { 2180 if (this.comment == null) 2181 if (Configuration.errorOnAutoCreate()) 2182 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.comment"); 2183 else if (Configuration.doAutoCreate()) 2184 this.comment = new StringType(); // bb 2185 return this.comment; 2186 } 2187 2188 public boolean hasCommentElement() { 2189 return this.comment != null && !this.comment.isEmpty(); 2190 } 2191 2192 public boolean hasComment() { 2193 return this.comment != null && !this.comment.isEmpty(); 2194 } 2195 2196 /** 2197 * @param value {@link #comment} (Additional information about the general costs 2198 * associated with this plan.). This is the underlying object with 2199 * id, value and extensions. The accessor "getComment" gives direct 2200 * access to the value 2201 */ 2202 public InsurancePlanPlanGeneralCostComponent setCommentElement(StringType value) { 2203 this.comment = value; 2204 return this; 2205 } 2206 2207 /** 2208 * @return Additional information about the general costs associated with this 2209 * plan. 2210 */ 2211 public String getComment() { 2212 return this.comment == null ? null : this.comment.getValue(); 2213 } 2214 2215 /** 2216 * @param value Additional information about the general costs associated with 2217 * this plan. 2218 */ 2219 public InsurancePlanPlanGeneralCostComponent setComment(String value) { 2220 if (Utilities.noString(value)) 2221 this.comment = null; 2222 else { 2223 if (this.comment == null) 2224 this.comment = new StringType(); 2225 this.comment.setValue(value); 2226 } 2227 return this; 2228 } 2229 2230 protected void listChildren(List<Property> children) { 2231 super.listChildren(children); 2232 children.add(new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type)); 2233 children.add( 2234 new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 0, 1, groupSize)); 2235 children.add(new Property("cost", "Money", "Value of the cost.", 0, 1, cost)); 2236 children.add(new Property("comment", "string", 2237 "Additional information about the general costs associated with this plan.", 0, 1, comment)); 2238 } 2239 2240 @Override 2241 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2242 switch (_hash) { 2243 case 3575610: 2244 /* type */ return new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type); 2245 case -1483017440: 2246 /* groupSize */ return new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 2247 0, 1, groupSize); 2248 case 3059661: 2249 /* cost */ return new Property("cost", "Money", "Value of the cost.", 0, 1, cost); 2250 case 950398559: 2251 /* comment */ return new Property("comment", "string", 2252 "Additional information about the general costs associated with this plan.", 0, 1, comment); 2253 default: 2254 return super.getNamedProperty(_hash, _name, _checkValid); 2255 } 2256 2257 } 2258 2259 @Override 2260 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2261 switch (hash) { 2262 case 3575610: 2263 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2264 case -1483017440: 2265 /* groupSize */ return this.groupSize == null ? new Base[0] : new Base[] { this.groupSize }; // PositiveIntType 2266 case 3059661: 2267 /* cost */ return this.cost == null ? new Base[0] : new Base[] { this.cost }; // Money 2268 case 950398559: 2269 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 2270 default: 2271 return super.getProperty(hash, name, checkValid); 2272 } 2273 2274 } 2275 2276 @Override 2277 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2278 switch (hash) { 2279 case 3575610: // type 2280 this.type = castToCodeableConcept(value); // CodeableConcept 2281 return value; 2282 case -1483017440: // groupSize 2283 this.groupSize = castToPositiveInt(value); // PositiveIntType 2284 return value; 2285 case 3059661: // cost 2286 this.cost = castToMoney(value); // Money 2287 return value; 2288 case 950398559: // comment 2289 this.comment = castToString(value); // StringType 2290 return value; 2291 default: 2292 return super.setProperty(hash, name, value); 2293 } 2294 2295 } 2296 2297 @Override 2298 public Base setProperty(String name, Base value) throws FHIRException { 2299 if (name.equals("type")) { 2300 this.type = castToCodeableConcept(value); // CodeableConcept 2301 } else if (name.equals("groupSize")) { 2302 this.groupSize = castToPositiveInt(value); // PositiveIntType 2303 } else if (name.equals("cost")) { 2304 this.cost = castToMoney(value); // Money 2305 } else if (name.equals("comment")) { 2306 this.comment = castToString(value); // StringType 2307 } else 2308 return super.setProperty(name, value); 2309 return value; 2310 } 2311 2312 @Override 2313 public void removeChild(String name, Base value) throws FHIRException { 2314 if (name.equals("type")) { 2315 this.type = null; 2316 } else if (name.equals("groupSize")) { 2317 this.groupSize = null; 2318 } else if (name.equals("cost")) { 2319 this.cost = null; 2320 } else if (name.equals("comment")) { 2321 this.comment = null; 2322 } else 2323 super.removeChild(name, value); 2324 2325 } 2326 2327 @Override 2328 public Base makeProperty(int hash, String name) throws FHIRException { 2329 switch (hash) { 2330 case 3575610: 2331 return getType(); 2332 case -1483017440: 2333 return getGroupSizeElement(); 2334 case 3059661: 2335 return getCost(); 2336 case 950398559: 2337 return getCommentElement(); 2338 default: 2339 return super.makeProperty(hash, name); 2340 } 2341 2342 } 2343 2344 @Override 2345 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2346 switch (hash) { 2347 case 3575610: 2348 /* type */ return new String[] { "CodeableConcept" }; 2349 case -1483017440: 2350 /* groupSize */ return new String[] { "positiveInt" }; 2351 case 3059661: 2352 /* cost */ return new String[] { "Money" }; 2353 case 950398559: 2354 /* comment */ return new String[] { "string" }; 2355 default: 2356 return super.getTypesForProperty(hash, name); 2357 } 2358 2359 } 2360 2361 @Override 2362 public Base addChild(String name) throws FHIRException { 2363 if (name.equals("type")) { 2364 this.type = new CodeableConcept(); 2365 return this.type; 2366 } else if (name.equals("groupSize")) { 2367 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.groupSize"); 2368 } else if (name.equals("cost")) { 2369 this.cost = new Money(); 2370 return this.cost; 2371 } else if (name.equals("comment")) { 2372 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.comment"); 2373 } else 2374 return super.addChild(name); 2375 } 2376 2377 public InsurancePlanPlanGeneralCostComponent copy() { 2378 InsurancePlanPlanGeneralCostComponent dst = new InsurancePlanPlanGeneralCostComponent(); 2379 copyValues(dst); 2380 return dst; 2381 } 2382 2383 public void copyValues(InsurancePlanPlanGeneralCostComponent dst) { 2384 super.copyValues(dst); 2385 dst.type = type == null ? null : type.copy(); 2386 dst.groupSize = groupSize == null ? null : groupSize.copy(); 2387 dst.cost = cost == null ? null : cost.copy(); 2388 dst.comment = comment == null ? null : comment.copy(); 2389 } 2390 2391 @Override 2392 public boolean equalsDeep(Base other_) { 2393 if (!super.equalsDeep(other_)) 2394 return false; 2395 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 2396 return false; 2397 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 2398 return compareDeep(type, o.type, true) && compareDeep(groupSize, o.groupSize, true) 2399 && compareDeep(cost, o.cost, true) && compareDeep(comment, o.comment, true); 2400 } 2401 2402 @Override 2403 public boolean equalsShallow(Base other_) { 2404 if (!super.equalsShallow(other_)) 2405 return false; 2406 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 2407 return false; 2408 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 2409 return compareValues(groupSize, o.groupSize, true) && compareValues(comment, o.comment, true); 2410 } 2411 2412 public boolean isEmpty() { 2413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, groupSize, cost, comment); 2414 } 2415 2416 public String fhirType() { 2417 return "InsurancePlan.plan.generalCost"; 2418 2419 } 2420 2421 } 2422 2423 @Block() 2424 public static class InsurancePlanPlanSpecificCostComponent extends BackboneElement implements IBaseBackboneElement { 2425 /** 2426 * General category of benefit (Medical; Dental; Vision; Drug; Mental Health; 2427 * Substance Abuse; Hospice, Home Health). 2428 */ 2429 @Child(name = "category", type = { 2430 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2431 @Description(shortDefinition = "General category of benefit", formalDefinition = "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).") 2432 protected CodeableConcept category; 2433 2434 /** 2435 * List of the specific benefits under this category of benefit. 2436 */ 2437 @Child(name = "benefit", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2438 @Description(shortDefinition = "Benefits list", formalDefinition = "List of the specific benefits under this category of benefit.") 2439 protected List<PlanBenefitComponent> benefit; 2440 2441 private static final long serialVersionUID = 922585525L; 2442 2443 /** 2444 * Constructor 2445 */ 2446 public InsurancePlanPlanSpecificCostComponent() { 2447 super(); 2448 } 2449 2450 /** 2451 * Constructor 2452 */ 2453 public InsurancePlanPlanSpecificCostComponent(CodeableConcept category) { 2454 super(); 2455 this.category = category; 2456 } 2457 2458 /** 2459 * @return {@link #category} (General category of benefit (Medical; Dental; 2460 * Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).) 2461 */ 2462 public CodeableConcept getCategory() { 2463 if (this.category == null) 2464 if (Configuration.errorOnAutoCreate()) 2465 throw new Error("Attempt to auto-create InsurancePlanPlanSpecificCostComponent.category"); 2466 else if (Configuration.doAutoCreate()) 2467 this.category = new CodeableConcept(); // cc 2468 return this.category; 2469 } 2470 2471 public boolean hasCategory() { 2472 return this.category != null && !this.category.isEmpty(); 2473 } 2474 2475 /** 2476 * @param value {@link #category} (General category of benefit (Medical; Dental; 2477 * Vision; Drug; Mental Health; Substance Abuse; Hospice, Home 2478 * Health).) 2479 */ 2480 public InsurancePlanPlanSpecificCostComponent setCategory(CodeableConcept value) { 2481 this.category = value; 2482 return this; 2483 } 2484 2485 /** 2486 * @return {@link #benefit} (List of the specific benefits under this category 2487 * of benefit.) 2488 */ 2489 public List<PlanBenefitComponent> getBenefit() { 2490 if (this.benefit == null) 2491 this.benefit = new ArrayList<PlanBenefitComponent>(); 2492 return this.benefit; 2493 } 2494 2495 /** 2496 * @return Returns a reference to <code>this</code> for easy method chaining 2497 */ 2498 public InsurancePlanPlanSpecificCostComponent setBenefit(List<PlanBenefitComponent> theBenefit) { 2499 this.benefit = theBenefit; 2500 return this; 2501 } 2502 2503 public boolean hasBenefit() { 2504 if (this.benefit == null) 2505 return false; 2506 for (PlanBenefitComponent item : this.benefit) 2507 if (!item.isEmpty()) 2508 return true; 2509 return false; 2510 } 2511 2512 public PlanBenefitComponent addBenefit() { // 3 2513 PlanBenefitComponent t = new PlanBenefitComponent(); 2514 if (this.benefit == null) 2515 this.benefit = new ArrayList<PlanBenefitComponent>(); 2516 this.benefit.add(t); 2517 return t; 2518 } 2519 2520 public InsurancePlanPlanSpecificCostComponent addBenefit(PlanBenefitComponent t) { // 3 2521 if (t == null) 2522 return this; 2523 if (this.benefit == null) 2524 this.benefit = new ArrayList<PlanBenefitComponent>(); 2525 this.benefit.add(t); 2526 return this; 2527 } 2528 2529 /** 2530 * @return The first repetition of repeating field {@link #benefit}, creating it 2531 * if it does not already exist 2532 */ 2533 public PlanBenefitComponent getBenefitFirstRep() { 2534 if (getBenefit().isEmpty()) { 2535 addBenefit(); 2536 } 2537 return getBenefit().get(0); 2538 } 2539 2540 protected void listChildren(List<Property> children) { 2541 super.listChildren(children); 2542 children.add(new Property("category", "CodeableConcept", 2543 "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 2544 0, 1, category)); 2545 children.add(new Property("benefit", "", "List of the specific benefits under this category of benefit.", 0, 2546 java.lang.Integer.MAX_VALUE, benefit)); 2547 } 2548 2549 @Override 2550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2551 switch (_hash) { 2552 case 50511102: 2553 /* category */ return new Property("category", "CodeableConcept", 2554 "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 2555 0, 1, category); 2556 case -222710633: 2557 /* benefit */ return new Property("benefit", "", 2558 "List of the specific benefits under this category of benefit.", 0, java.lang.Integer.MAX_VALUE, benefit); 2559 default: 2560 return super.getNamedProperty(_hash, _name, _checkValid); 2561 } 2562 2563 } 2564 2565 @Override 2566 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2567 switch (hash) { 2568 case 50511102: 2569 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2570 case -222710633: 2571 /* benefit */ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // PlanBenefitComponent 2572 default: 2573 return super.getProperty(hash, name, checkValid); 2574 } 2575 2576 } 2577 2578 @Override 2579 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2580 switch (hash) { 2581 case 50511102: // category 2582 this.category = castToCodeableConcept(value); // CodeableConcept 2583 return value; 2584 case -222710633: // benefit 2585 this.getBenefit().add((PlanBenefitComponent) value); // PlanBenefitComponent 2586 return value; 2587 default: 2588 return super.setProperty(hash, name, value); 2589 } 2590 2591 } 2592 2593 @Override 2594 public Base setProperty(String name, Base value) throws FHIRException { 2595 if (name.equals("category")) { 2596 this.category = castToCodeableConcept(value); // CodeableConcept 2597 } else if (name.equals("benefit")) { 2598 this.getBenefit().add((PlanBenefitComponent) value); 2599 } else 2600 return super.setProperty(name, value); 2601 return value; 2602 } 2603 2604 @Override 2605 public void removeChild(String name, Base value) throws FHIRException { 2606 if (name.equals("category")) { 2607 this.category = null; 2608 } else if (name.equals("benefit")) { 2609 this.getBenefit().remove((PlanBenefitComponent) value); 2610 } else 2611 super.removeChild(name, value); 2612 2613 } 2614 2615 @Override 2616 public Base makeProperty(int hash, String name) throws FHIRException { 2617 switch (hash) { 2618 case 50511102: 2619 return getCategory(); 2620 case -222710633: 2621 return addBenefit(); 2622 default: 2623 return super.makeProperty(hash, name); 2624 } 2625 2626 } 2627 2628 @Override 2629 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2630 switch (hash) { 2631 case 50511102: 2632 /* category */ return new String[] { "CodeableConcept" }; 2633 case -222710633: 2634 /* benefit */ return new String[] {}; 2635 default: 2636 return super.getTypesForProperty(hash, name); 2637 } 2638 2639 } 2640 2641 @Override 2642 public Base addChild(String name) throws FHIRException { 2643 if (name.equals("category")) { 2644 this.category = new CodeableConcept(); 2645 return this.category; 2646 } else if (name.equals("benefit")) { 2647 return addBenefit(); 2648 } else 2649 return super.addChild(name); 2650 } 2651 2652 public InsurancePlanPlanSpecificCostComponent copy() { 2653 InsurancePlanPlanSpecificCostComponent dst = new InsurancePlanPlanSpecificCostComponent(); 2654 copyValues(dst); 2655 return dst; 2656 } 2657 2658 public void copyValues(InsurancePlanPlanSpecificCostComponent dst) { 2659 super.copyValues(dst); 2660 dst.category = category == null ? null : category.copy(); 2661 if (benefit != null) { 2662 dst.benefit = new ArrayList<PlanBenefitComponent>(); 2663 for (PlanBenefitComponent i : benefit) 2664 dst.benefit.add(i.copy()); 2665 } 2666 ; 2667 } 2668 2669 @Override 2670 public boolean equalsDeep(Base other_) { 2671 if (!super.equalsDeep(other_)) 2672 return false; 2673 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2674 return false; 2675 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2676 return compareDeep(category, o.category, true) && compareDeep(benefit, o.benefit, true); 2677 } 2678 2679 @Override 2680 public boolean equalsShallow(Base other_) { 2681 if (!super.equalsShallow(other_)) 2682 return false; 2683 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2684 return false; 2685 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2686 return true; 2687 } 2688 2689 public boolean isEmpty() { 2690 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, benefit); 2691 } 2692 2693 public String fhirType() { 2694 return "InsurancePlan.plan.specificCost"; 2695 2696 } 2697 2698 } 2699 2700 @Block() 2701 public static class PlanBenefitComponent extends BackboneElement implements IBaseBackboneElement { 2702 /** 2703 * Type of specific benefit (preventative; primary care office visit; speciality 2704 * office visit; hospitalization; emergency room; urgent care). 2705 */ 2706 @Child(name = "type", type = { 2707 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2708 @Description(shortDefinition = "Type of specific benefit", formalDefinition = "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).") 2709 protected CodeableConcept type; 2710 2711 /** 2712 * List of the costs associated with a specific benefit. 2713 */ 2714 @Child(name = "cost", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2715 @Description(shortDefinition = "List of the costs", formalDefinition = "List of the costs associated with a specific benefit.") 2716 protected List<PlanBenefitCostComponent> cost; 2717 2718 private static final long serialVersionUID = 792296200L; 2719 2720 /** 2721 * Constructor 2722 */ 2723 public PlanBenefitComponent() { 2724 super(); 2725 } 2726 2727 /** 2728 * Constructor 2729 */ 2730 public PlanBenefitComponent(CodeableConcept type) { 2731 super(); 2732 this.type = type; 2733 } 2734 2735 /** 2736 * @return {@link #type} (Type of specific benefit (preventative; primary care 2737 * office visit; speciality office visit; hospitalization; emergency 2738 * room; urgent care).) 2739 */ 2740 public CodeableConcept getType() { 2741 if (this.type == null) 2742 if (Configuration.errorOnAutoCreate()) 2743 throw new Error("Attempt to auto-create PlanBenefitComponent.type"); 2744 else if (Configuration.doAutoCreate()) 2745 this.type = new CodeableConcept(); // cc 2746 return this.type; 2747 } 2748 2749 public boolean hasType() { 2750 return this.type != null && !this.type.isEmpty(); 2751 } 2752 2753 /** 2754 * @param value {@link #type} (Type of specific benefit (preventative; primary 2755 * care office visit; speciality office visit; hospitalization; 2756 * emergency room; urgent care).) 2757 */ 2758 public PlanBenefitComponent setType(CodeableConcept value) { 2759 this.type = value; 2760 return this; 2761 } 2762 2763 /** 2764 * @return {@link #cost} (List of the costs associated with a specific benefit.) 2765 */ 2766 public List<PlanBenefitCostComponent> getCost() { 2767 if (this.cost == null) 2768 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2769 return this.cost; 2770 } 2771 2772 /** 2773 * @return Returns a reference to <code>this</code> for easy method chaining 2774 */ 2775 public PlanBenefitComponent setCost(List<PlanBenefitCostComponent> theCost) { 2776 this.cost = theCost; 2777 return this; 2778 } 2779 2780 public boolean hasCost() { 2781 if (this.cost == null) 2782 return false; 2783 for (PlanBenefitCostComponent item : this.cost) 2784 if (!item.isEmpty()) 2785 return true; 2786 return false; 2787 } 2788 2789 public PlanBenefitCostComponent addCost() { // 3 2790 PlanBenefitCostComponent t = new PlanBenefitCostComponent(); 2791 if (this.cost == null) 2792 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2793 this.cost.add(t); 2794 return t; 2795 } 2796 2797 public PlanBenefitComponent addCost(PlanBenefitCostComponent t) { // 3 2798 if (t == null) 2799 return this; 2800 if (this.cost == null) 2801 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2802 this.cost.add(t); 2803 return this; 2804 } 2805 2806 /** 2807 * @return The first repetition of repeating field {@link #cost}, creating it if 2808 * it does not already exist 2809 */ 2810 public PlanBenefitCostComponent getCostFirstRep() { 2811 if (getCost().isEmpty()) { 2812 addCost(); 2813 } 2814 return getCost().get(0); 2815 } 2816 2817 protected void listChildren(List<Property> children) { 2818 super.listChildren(children); 2819 children.add(new Property("type", "CodeableConcept", 2820 "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 2821 0, 1, type)); 2822 children.add(new Property("cost", "", "List of the costs associated with a specific benefit.", 0, 2823 java.lang.Integer.MAX_VALUE, cost)); 2824 } 2825 2826 @Override 2827 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2828 switch (_hash) { 2829 case 3575610: 2830 /* type */ return new Property("type", "CodeableConcept", 2831 "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 2832 0, 1, type); 2833 case 3059661: 2834 /* cost */ return new Property("cost", "", "List of the costs associated with a specific benefit.", 0, 2835 java.lang.Integer.MAX_VALUE, cost); 2836 default: 2837 return super.getNamedProperty(_hash, _name, _checkValid); 2838 } 2839 2840 } 2841 2842 @Override 2843 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2844 switch (hash) { 2845 case 3575610: 2846 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2847 case 3059661: 2848 /* cost */ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // PlanBenefitCostComponent 2849 default: 2850 return super.getProperty(hash, name, checkValid); 2851 } 2852 2853 } 2854 2855 @Override 2856 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2857 switch (hash) { 2858 case 3575610: // type 2859 this.type = castToCodeableConcept(value); // CodeableConcept 2860 return value; 2861 case 3059661: // cost 2862 this.getCost().add((PlanBenefitCostComponent) value); // PlanBenefitCostComponent 2863 return value; 2864 default: 2865 return super.setProperty(hash, name, value); 2866 } 2867 2868 } 2869 2870 @Override 2871 public Base setProperty(String name, Base value) throws FHIRException { 2872 if (name.equals("type")) { 2873 this.type = castToCodeableConcept(value); // CodeableConcept 2874 } else if (name.equals("cost")) { 2875 this.getCost().add((PlanBenefitCostComponent) value); 2876 } else 2877 return super.setProperty(name, value); 2878 return value; 2879 } 2880 2881 @Override 2882 public void removeChild(String name, Base value) throws FHIRException { 2883 if (name.equals("type")) { 2884 this.type = null; 2885 } else if (name.equals("cost")) { 2886 this.getCost().remove((PlanBenefitCostComponent) value); 2887 } else 2888 super.removeChild(name, value); 2889 2890 } 2891 2892 @Override 2893 public Base makeProperty(int hash, String name) throws FHIRException { 2894 switch (hash) { 2895 case 3575610: 2896 return getType(); 2897 case 3059661: 2898 return addCost(); 2899 default: 2900 return super.makeProperty(hash, name); 2901 } 2902 2903 } 2904 2905 @Override 2906 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2907 switch (hash) { 2908 case 3575610: 2909 /* type */ return new String[] { "CodeableConcept" }; 2910 case 3059661: 2911 /* cost */ return new String[] {}; 2912 default: 2913 return super.getTypesForProperty(hash, name); 2914 } 2915 2916 } 2917 2918 @Override 2919 public Base addChild(String name) throws FHIRException { 2920 if (name.equals("type")) { 2921 this.type = new CodeableConcept(); 2922 return this.type; 2923 } else if (name.equals("cost")) { 2924 return addCost(); 2925 } else 2926 return super.addChild(name); 2927 } 2928 2929 public PlanBenefitComponent copy() { 2930 PlanBenefitComponent dst = new PlanBenefitComponent(); 2931 copyValues(dst); 2932 return dst; 2933 } 2934 2935 public void copyValues(PlanBenefitComponent dst) { 2936 super.copyValues(dst); 2937 dst.type = type == null ? null : type.copy(); 2938 if (cost != null) { 2939 dst.cost = new ArrayList<PlanBenefitCostComponent>(); 2940 for (PlanBenefitCostComponent i : cost) 2941 dst.cost.add(i.copy()); 2942 } 2943 ; 2944 } 2945 2946 @Override 2947 public boolean equalsDeep(Base other_) { 2948 if (!super.equalsDeep(other_)) 2949 return false; 2950 if (!(other_ instanceof PlanBenefitComponent)) 2951 return false; 2952 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2953 return compareDeep(type, o.type, true) && compareDeep(cost, o.cost, true); 2954 } 2955 2956 @Override 2957 public boolean equalsShallow(Base other_) { 2958 if (!super.equalsShallow(other_)) 2959 return false; 2960 if (!(other_ instanceof PlanBenefitComponent)) 2961 return false; 2962 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2963 return true; 2964 } 2965 2966 public boolean isEmpty() { 2967 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, cost); 2968 } 2969 2970 public String fhirType() { 2971 return "InsurancePlan.plan.specificCost.benefit"; 2972 2973 } 2974 2975 } 2976 2977 @Block() 2978 public static class PlanBenefitCostComponent extends BackboneElement implements IBaseBackboneElement { 2979 /** 2980 * Type of cost (copay; individual cap; family cap; coinsurance; deductible). 2981 */ 2982 @Child(name = "type", type = { 2983 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 2984 @Description(shortDefinition = "Type of cost", formalDefinition = "Type of cost (copay; individual cap; family cap; coinsurance; deductible).") 2985 protected CodeableConcept type; 2986 2987 /** 2988 * Whether the cost applies to in-network or out-of-network providers 2989 * (in-network; out-of-network; other). 2990 */ 2991 @Child(name = "applicability", type = { 2992 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 2993 @Description(shortDefinition = "in-network | out-of-network | other", formalDefinition = "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).") 2994 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/insuranceplan-applicability") 2995 protected CodeableConcept applicability; 2996 2997 /** 2998 * Additional information about the cost, such as information about funding 2999 * sources (e.g. HSA, HRA, FSA, RRA). 3000 */ 3001 @Child(name = "qualifiers", type = { 3002 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3003 @Description(shortDefinition = "Additional information about the cost", formalDefinition = "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).") 3004 protected List<CodeableConcept> qualifiers; 3005 3006 /** 3007 * The actual cost value. (some of the costs may be represented as percentages 3008 * rather than currency, e.g. 10% coinsurance). 3009 */ 3010 @Child(name = "value", type = { Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 3011 @Description(shortDefinition = "The actual cost value", formalDefinition = "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).") 3012 protected Quantity value; 3013 3014 private static final long serialVersionUID = -340688733L; 3015 3016 /** 3017 * Constructor 3018 */ 3019 public PlanBenefitCostComponent() { 3020 super(); 3021 } 3022 3023 /** 3024 * Constructor 3025 */ 3026 public PlanBenefitCostComponent(CodeableConcept type) { 3027 super(); 3028 this.type = type; 3029 } 3030 3031 /** 3032 * @return {@link #type} (Type of cost (copay; individual cap; family cap; 3033 * coinsurance; deductible).) 3034 */ 3035 public CodeableConcept getType() { 3036 if (this.type == null) 3037 if (Configuration.errorOnAutoCreate()) 3038 throw new Error("Attempt to auto-create PlanBenefitCostComponent.type"); 3039 else if (Configuration.doAutoCreate()) 3040 this.type = new CodeableConcept(); // cc 3041 return this.type; 3042 } 3043 3044 public boolean hasType() { 3045 return this.type != null && !this.type.isEmpty(); 3046 } 3047 3048 /** 3049 * @param value {@link #type} (Type of cost (copay; individual cap; family cap; 3050 * coinsurance; deductible).) 3051 */ 3052 public PlanBenefitCostComponent setType(CodeableConcept value) { 3053 this.type = value; 3054 return this; 3055 } 3056 3057 /** 3058 * @return {@link #applicability} (Whether the cost applies to in-network or 3059 * out-of-network providers (in-network; out-of-network; other).) 3060 */ 3061 public CodeableConcept getApplicability() { 3062 if (this.applicability == null) 3063 if (Configuration.errorOnAutoCreate()) 3064 throw new Error("Attempt to auto-create PlanBenefitCostComponent.applicability"); 3065 else if (Configuration.doAutoCreate()) 3066 this.applicability = new CodeableConcept(); // cc 3067 return this.applicability; 3068 } 3069 3070 public boolean hasApplicability() { 3071 return this.applicability != null && !this.applicability.isEmpty(); 3072 } 3073 3074 /** 3075 * @param value {@link #applicability} (Whether the cost applies to in-network 3076 * or out-of-network providers (in-network; out-of-network; 3077 * other).) 3078 */ 3079 public PlanBenefitCostComponent setApplicability(CodeableConcept value) { 3080 this.applicability = value; 3081 return this; 3082 } 3083 3084 /** 3085 * @return {@link #qualifiers} (Additional information about the cost, such as 3086 * information about funding sources (e.g. HSA, HRA, FSA, RRA).) 3087 */ 3088 public List<CodeableConcept> getQualifiers() { 3089 if (this.qualifiers == null) 3090 this.qualifiers = new ArrayList<CodeableConcept>(); 3091 return this.qualifiers; 3092 } 3093 3094 /** 3095 * @return Returns a reference to <code>this</code> for easy method chaining 3096 */ 3097 public PlanBenefitCostComponent setQualifiers(List<CodeableConcept> theQualifiers) { 3098 this.qualifiers = theQualifiers; 3099 return this; 3100 } 3101 3102 public boolean hasQualifiers() { 3103 if (this.qualifiers == null) 3104 return false; 3105 for (CodeableConcept item : this.qualifiers) 3106 if (!item.isEmpty()) 3107 return true; 3108 return false; 3109 } 3110 3111 public CodeableConcept addQualifiers() { // 3 3112 CodeableConcept t = new CodeableConcept(); 3113 if (this.qualifiers == null) 3114 this.qualifiers = new ArrayList<CodeableConcept>(); 3115 this.qualifiers.add(t); 3116 return t; 3117 } 3118 3119 public PlanBenefitCostComponent addQualifiers(CodeableConcept t) { // 3 3120 if (t == null) 3121 return this; 3122 if (this.qualifiers == null) 3123 this.qualifiers = new ArrayList<CodeableConcept>(); 3124 this.qualifiers.add(t); 3125 return this; 3126 } 3127 3128 /** 3129 * @return The first repetition of repeating field {@link #qualifiers}, creating 3130 * it if it does not already exist 3131 */ 3132 public CodeableConcept getQualifiersFirstRep() { 3133 if (getQualifiers().isEmpty()) { 3134 addQualifiers(); 3135 } 3136 return getQualifiers().get(0); 3137 } 3138 3139 /** 3140 * @return {@link #value} (The actual cost value. (some of the costs may be 3141 * represented as percentages rather than currency, e.g. 10% 3142 * coinsurance).) 3143 */ 3144 public Quantity getValue() { 3145 if (this.value == null) 3146 if (Configuration.errorOnAutoCreate()) 3147 throw new Error("Attempt to auto-create PlanBenefitCostComponent.value"); 3148 else if (Configuration.doAutoCreate()) 3149 this.value = new Quantity(); // cc 3150 return this.value; 3151 } 3152 3153 public boolean hasValue() { 3154 return this.value != null && !this.value.isEmpty(); 3155 } 3156 3157 /** 3158 * @param value {@link #value} (The actual cost value. (some of the costs may be 3159 * represented as percentages rather than currency, e.g. 10% 3160 * coinsurance).) 3161 */ 3162 public PlanBenefitCostComponent setValue(Quantity value) { 3163 this.value = value; 3164 return this; 3165 } 3166 3167 protected void listChildren(List<Property> children) { 3168 super.listChildren(children); 3169 children.add(new Property("type", "CodeableConcept", 3170 "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type)); 3171 children.add(new Property("applicability", "CodeableConcept", 3172 "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 0, 3173 1, applicability)); 3174 children.add(new Property("qualifiers", "CodeableConcept", 3175 "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 3176 0, java.lang.Integer.MAX_VALUE, qualifiers)); 3177 children.add(new Property("value", "Quantity", 3178 "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 3179 0, 1, value)); 3180 } 3181 3182 @Override 3183 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3184 switch (_hash) { 3185 case 3575610: 3186 /* type */ return new Property("type", "CodeableConcept", 3187 "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type); 3188 case -1526770491: 3189 /* applicability */ return new Property("applicability", "CodeableConcept", 3190 "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 3191 0, 1, applicability); 3192 case -31447799: 3193 /* qualifiers */ return new Property("qualifiers", "CodeableConcept", 3194 "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 3195 0, java.lang.Integer.MAX_VALUE, qualifiers); 3196 case 111972721: 3197 /* value */ return new Property("value", "Quantity", 3198 "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 3199 0, 1, value); 3200 default: 3201 return super.getNamedProperty(_hash, _name, _checkValid); 3202 } 3203 3204 } 3205 3206 @Override 3207 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3208 switch (hash) { 3209 case 3575610: 3210 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 3211 case -1526770491: 3212 /* applicability */ return this.applicability == null ? new Base[0] : new Base[] { this.applicability }; // CodeableConcept 3213 case -31447799: 3214 /* qualifiers */ return this.qualifiers == null ? new Base[0] 3215 : this.qualifiers.toArray(new Base[this.qualifiers.size()]); // CodeableConcept 3216 case 111972721: 3217 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 3218 default: 3219 return super.getProperty(hash, name, checkValid); 3220 } 3221 3222 } 3223 3224 @Override 3225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3226 switch (hash) { 3227 case 3575610: // type 3228 this.type = castToCodeableConcept(value); // CodeableConcept 3229 return value; 3230 case -1526770491: // applicability 3231 this.applicability = castToCodeableConcept(value); // CodeableConcept 3232 return value; 3233 case -31447799: // qualifiers 3234 this.getQualifiers().add(castToCodeableConcept(value)); // CodeableConcept 3235 return value; 3236 case 111972721: // value 3237 this.value = castToQuantity(value); // Quantity 3238 return value; 3239 default: 3240 return super.setProperty(hash, name, value); 3241 } 3242 3243 } 3244 3245 @Override 3246 public Base setProperty(String name, Base value) throws FHIRException { 3247 if (name.equals("type")) { 3248 this.type = castToCodeableConcept(value); // CodeableConcept 3249 } else if (name.equals("applicability")) { 3250 this.applicability = castToCodeableConcept(value); // CodeableConcept 3251 } else if (name.equals("qualifiers")) { 3252 this.getQualifiers().add(castToCodeableConcept(value)); 3253 } else if (name.equals("value")) { 3254 this.value = castToQuantity(value); // Quantity 3255 } else 3256 return super.setProperty(name, value); 3257 return value; 3258 } 3259 3260 @Override 3261 public void removeChild(String name, Base value) throws FHIRException { 3262 if (name.equals("type")) { 3263 this.type = null; 3264 } else if (name.equals("applicability")) { 3265 this.applicability = null; 3266 } else if (name.equals("qualifiers")) { 3267 this.getQualifiers().remove(castToCodeableConcept(value)); 3268 } else if (name.equals("value")) { 3269 this.value = null; 3270 } else 3271 super.removeChild(name, value); 3272 3273 } 3274 3275 @Override 3276 public Base makeProperty(int hash, String name) throws FHIRException { 3277 switch (hash) { 3278 case 3575610: 3279 return getType(); 3280 case -1526770491: 3281 return getApplicability(); 3282 case -31447799: 3283 return addQualifiers(); 3284 case 111972721: 3285 return getValue(); 3286 default: 3287 return super.makeProperty(hash, name); 3288 } 3289 3290 } 3291 3292 @Override 3293 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3294 switch (hash) { 3295 case 3575610: 3296 /* type */ return new String[] { "CodeableConcept" }; 3297 case -1526770491: 3298 /* applicability */ return new String[] { "CodeableConcept" }; 3299 case -31447799: 3300 /* qualifiers */ return new String[] { "CodeableConcept" }; 3301 case 111972721: 3302 /* value */ return new String[] { "Quantity" }; 3303 default: 3304 return super.getTypesForProperty(hash, name); 3305 } 3306 3307 } 3308 3309 @Override 3310 public Base addChild(String name) throws FHIRException { 3311 if (name.equals("type")) { 3312 this.type = new CodeableConcept(); 3313 return this.type; 3314 } else if (name.equals("applicability")) { 3315 this.applicability = new CodeableConcept(); 3316 return this.applicability; 3317 } else if (name.equals("qualifiers")) { 3318 return addQualifiers(); 3319 } else if (name.equals("value")) { 3320 this.value = new Quantity(); 3321 return this.value; 3322 } else 3323 return super.addChild(name); 3324 } 3325 3326 public PlanBenefitCostComponent copy() { 3327 PlanBenefitCostComponent dst = new PlanBenefitCostComponent(); 3328 copyValues(dst); 3329 return dst; 3330 } 3331 3332 public void copyValues(PlanBenefitCostComponent dst) { 3333 super.copyValues(dst); 3334 dst.type = type == null ? null : type.copy(); 3335 dst.applicability = applicability == null ? null : applicability.copy(); 3336 if (qualifiers != null) { 3337 dst.qualifiers = new ArrayList<CodeableConcept>(); 3338 for (CodeableConcept i : qualifiers) 3339 dst.qualifiers.add(i.copy()); 3340 } 3341 ; 3342 dst.value = value == null ? null : value.copy(); 3343 } 3344 3345 @Override 3346 public boolean equalsDeep(Base other_) { 3347 if (!super.equalsDeep(other_)) 3348 return false; 3349 if (!(other_ instanceof PlanBenefitCostComponent)) 3350 return false; 3351 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 3352 return compareDeep(type, o.type, true) && compareDeep(applicability, o.applicability, true) 3353 && compareDeep(qualifiers, o.qualifiers, true) && compareDeep(value, o.value, true); 3354 } 3355 3356 @Override 3357 public boolean equalsShallow(Base other_) { 3358 if (!super.equalsShallow(other_)) 3359 return false; 3360 if (!(other_ instanceof PlanBenefitCostComponent)) 3361 return false; 3362 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 3363 return true; 3364 } 3365 3366 public boolean isEmpty() { 3367 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, applicability, qualifiers, value); 3368 } 3369 3370 public String fhirType() { 3371 return "InsurancePlan.plan.specificCost.benefit.cost"; 3372 3373 } 3374 3375 } 3376 3377 /** 3378 * Business identifiers assigned to this health insurance product which remain 3379 * constant as the resource is updated and propagates from server to server. 3380 */ 3381 @Child(name = "identifier", type = { 3382 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3383 @Description(shortDefinition = "Business Identifier for Product", formalDefinition = "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.") 3384 protected List<Identifier> identifier; 3385 3386 /** 3387 * The current state of the health insurance product. 3388 */ 3389 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 3390 @Description(shortDefinition = "draft | active | retired | unknown", formalDefinition = "The current state of the health insurance product.") 3391 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/publication-status") 3392 protected Enumeration<PublicationStatus> status; 3393 3394 /** 3395 * The kind of health insurance product. 3396 */ 3397 @Child(name = "type", type = { 3398 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3399 @Description(shortDefinition = "Kind of product", formalDefinition = "The kind of health insurance product.") 3400 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/insuranceplan-type") 3401 protected List<CodeableConcept> type; 3402 3403 /** 3404 * Official name of the health insurance product as designated by the owner. 3405 */ 3406 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3407 @Description(shortDefinition = "Official name", formalDefinition = "Official name of the health insurance product as designated by the owner.") 3408 protected StringType name; 3409 3410 /** 3411 * A list of alternate names that the product is known as, or was known as in 3412 * the past. 3413 */ 3414 @Child(name = "alias", type = { 3415 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3416 @Description(shortDefinition = "Alternate names", formalDefinition = "A list of alternate names that the product is known as, or was known as in the past.") 3417 protected List<StringType> alias; 3418 3419 /** 3420 * The period of time that the health insurance product is available. 3421 */ 3422 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 3423 @Description(shortDefinition = "When the product is available", formalDefinition = "The period of time that the health insurance product is available.") 3424 protected Period period; 3425 3426 /** 3427 * The entity that is providing the health insurance product and underwriting 3428 * the risk. This is typically an insurance carriers, other third-party payers, 3429 * or health plan sponsors comonly referred to as 'payers'. 3430 */ 3431 @Child(name = "ownedBy", type = { Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3432 @Description(shortDefinition = "Plan issuer", formalDefinition = "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.") 3433 protected Reference ownedBy; 3434 3435 /** 3436 * The actual object that is the target of the reference (The entity that is 3437 * providing the health insurance product and underwriting the risk. This is 3438 * typically an insurance carriers, other third-party payers, or health plan 3439 * sponsors comonly referred to as 'payers'.) 3440 */ 3441 protected Organization ownedByTarget; 3442 3443 /** 3444 * An organization which administer other services such as underwriting, 3445 * customer service and/or claims processing on behalf of the health insurance 3446 * product owner. 3447 */ 3448 @Child(name = "administeredBy", type = { 3449 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3450 @Description(shortDefinition = "Product administrator", formalDefinition = "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.") 3451 protected Reference administeredBy; 3452 3453 /** 3454 * The actual object that is the target of the reference (An organization which 3455 * administer other services such as underwriting, customer service and/or 3456 * claims processing on behalf of the health insurance product owner.) 3457 */ 3458 protected Organization administeredByTarget; 3459 3460 /** 3461 * The geographic region in which a health insurance product's benefits apply. 3462 */ 3463 @Child(name = "coverageArea", type = { 3464 Location.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3465 @Description(shortDefinition = "Where product applies", formalDefinition = "The geographic region in which a health insurance product's benefits apply.") 3466 protected List<Reference> coverageArea; 3467 /** 3468 * The actual objects that are the target of the reference (The geographic 3469 * region in which a health insurance product's benefits apply.) 3470 */ 3471 protected List<Location> coverageAreaTarget; 3472 3473 /** 3474 * The contact for the health insurance product for a certain purpose. 3475 */ 3476 @Child(name = "contact", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3477 @Description(shortDefinition = "Contact for the product", formalDefinition = "The contact for the health insurance product for a certain purpose.") 3478 protected List<InsurancePlanContactComponent> contact; 3479 3480 /** 3481 * The technical endpoints providing access to services operated for the health 3482 * insurance product. 3483 */ 3484 @Child(name = "endpoint", type = { 3485 Endpoint.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3486 @Description(shortDefinition = "Technical endpoint", formalDefinition = "The technical endpoints providing access to services operated for the health insurance product.") 3487 protected List<Reference> endpoint; 3488 /** 3489 * The actual objects that are the target of the reference (The technical 3490 * endpoints providing access to services operated for the health insurance 3491 * product.) 3492 */ 3493 protected List<Endpoint> endpointTarget; 3494 3495 /** 3496 * Reference to the network included in the health insurance product. 3497 */ 3498 @Child(name = "network", type = { 3499 Organization.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3500 @Description(shortDefinition = "What networks are Included", formalDefinition = "Reference to the network included in the health insurance product.") 3501 protected List<Reference> network; 3502 /** 3503 * The actual objects that are the target of the reference (Reference to the 3504 * network included in the health insurance product.) 3505 */ 3506 protected List<Organization> networkTarget; 3507 3508 /** 3509 * Details about the coverage offered by the insurance product. 3510 */ 3511 @Child(name = "coverage", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3512 @Description(shortDefinition = "Coverage details", formalDefinition = "Details about the coverage offered by the insurance product.") 3513 protected List<InsurancePlanCoverageComponent> coverage; 3514 3515 /** 3516 * Details about an insurance plan. 3517 */ 3518 @Child(name = "plan", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3519 @Description(shortDefinition = "Plan details", formalDefinition = "Details about an insurance plan.") 3520 protected List<InsurancePlanPlanComponent> plan; 3521 3522 private static final long serialVersionUID = -1910594688L; 3523 3524 /** 3525 * Constructor 3526 */ 3527 public InsurancePlan() { 3528 super(); 3529 } 3530 3531 /** 3532 * @return {@link #identifier} (Business identifiers assigned to this health 3533 * insurance product which remain constant as the resource is updated 3534 * and propagates from server to server.) 3535 */ 3536 public List<Identifier> getIdentifier() { 3537 if (this.identifier == null) 3538 this.identifier = new ArrayList<Identifier>(); 3539 return this.identifier; 3540 } 3541 3542 /** 3543 * @return Returns a reference to <code>this</code> for easy method chaining 3544 */ 3545 public InsurancePlan setIdentifier(List<Identifier> theIdentifier) { 3546 this.identifier = theIdentifier; 3547 return this; 3548 } 3549 3550 public boolean hasIdentifier() { 3551 if (this.identifier == null) 3552 return false; 3553 for (Identifier item : this.identifier) 3554 if (!item.isEmpty()) 3555 return true; 3556 return false; 3557 } 3558 3559 public Identifier addIdentifier() { // 3 3560 Identifier t = new Identifier(); 3561 if (this.identifier == null) 3562 this.identifier = new ArrayList<Identifier>(); 3563 this.identifier.add(t); 3564 return t; 3565 } 3566 3567 public InsurancePlan addIdentifier(Identifier t) { // 3 3568 if (t == null) 3569 return this; 3570 if (this.identifier == null) 3571 this.identifier = new ArrayList<Identifier>(); 3572 this.identifier.add(t); 3573 return this; 3574 } 3575 3576 /** 3577 * @return The first repetition of repeating field {@link #identifier}, creating 3578 * it if it does not already exist 3579 */ 3580 public Identifier getIdentifierFirstRep() { 3581 if (getIdentifier().isEmpty()) { 3582 addIdentifier(); 3583 } 3584 return getIdentifier().get(0); 3585 } 3586 3587 /** 3588 * @return {@link #status} (The current state of the health insurance product.). 3589 * This is the underlying object with id, value and extensions. The 3590 * accessor "getStatus" gives direct access to the value 3591 */ 3592 public Enumeration<PublicationStatus> getStatusElement() { 3593 if (this.status == null) 3594 if (Configuration.errorOnAutoCreate()) 3595 throw new Error("Attempt to auto-create InsurancePlan.status"); 3596 else if (Configuration.doAutoCreate()) 3597 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3598 return this.status; 3599 } 3600 3601 public boolean hasStatusElement() { 3602 return this.status != null && !this.status.isEmpty(); 3603 } 3604 3605 public boolean hasStatus() { 3606 return this.status != null && !this.status.isEmpty(); 3607 } 3608 3609 /** 3610 * @param value {@link #status} (The current state of the health insurance 3611 * product.). This is the underlying object with id, value and 3612 * extensions. The accessor "getStatus" gives direct access to the 3613 * value 3614 */ 3615 public InsurancePlan setStatusElement(Enumeration<PublicationStatus> value) { 3616 this.status = value; 3617 return this; 3618 } 3619 3620 /** 3621 * @return The current state of the health insurance product. 3622 */ 3623 public PublicationStatus getStatus() { 3624 return this.status == null ? null : this.status.getValue(); 3625 } 3626 3627 /** 3628 * @param value The current state of the health insurance product. 3629 */ 3630 public InsurancePlan setStatus(PublicationStatus value) { 3631 if (value == null) 3632 this.status = null; 3633 else { 3634 if (this.status == null) 3635 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3636 this.status.setValue(value); 3637 } 3638 return this; 3639 } 3640 3641 /** 3642 * @return {@link #type} (The kind of health insurance product.) 3643 */ 3644 public List<CodeableConcept> getType() { 3645 if (this.type == null) 3646 this.type = new ArrayList<CodeableConcept>(); 3647 return this.type; 3648 } 3649 3650 /** 3651 * @return Returns a reference to <code>this</code> for easy method chaining 3652 */ 3653 public InsurancePlan setType(List<CodeableConcept> theType) { 3654 this.type = theType; 3655 return this; 3656 } 3657 3658 public boolean hasType() { 3659 if (this.type == null) 3660 return false; 3661 for (CodeableConcept item : this.type) 3662 if (!item.isEmpty()) 3663 return true; 3664 return false; 3665 } 3666 3667 public CodeableConcept addType() { // 3 3668 CodeableConcept t = new CodeableConcept(); 3669 if (this.type == null) 3670 this.type = new ArrayList<CodeableConcept>(); 3671 this.type.add(t); 3672 return t; 3673 } 3674 3675 public InsurancePlan addType(CodeableConcept t) { // 3 3676 if (t == null) 3677 return this; 3678 if (this.type == null) 3679 this.type = new ArrayList<CodeableConcept>(); 3680 this.type.add(t); 3681 return this; 3682 } 3683 3684 /** 3685 * @return The first repetition of repeating field {@link #type}, creating it if 3686 * it does not already exist 3687 */ 3688 public CodeableConcept getTypeFirstRep() { 3689 if (getType().isEmpty()) { 3690 addType(); 3691 } 3692 return getType().get(0); 3693 } 3694 3695 /** 3696 * @return {@link #name} (Official name of the health insurance product as 3697 * designated by the owner.). This is the underlying object with id, 3698 * value and extensions. The accessor "getName" gives direct access to 3699 * the value 3700 */ 3701 public StringType getNameElement() { 3702 if (this.name == null) 3703 if (Configuration.errorOnAutoCreate()) 3704 throw new Error("Attempt to auto-create InsurancePlan.name"); 3705 else if (Configuration.doAutoCreate()) 3706 this.name = new StringType(); // bb 3707 return this.name; 3708 } 3709 3710 public boolean hasNameElement() { 3711 return this.name != null && !this.name.isEmpty(); 3712 } 3713 3714 public boolean hasName() { 3715 return this.name != null && !this.name.isEmpty(); 3716 } 3717 3718 /** 3719 * @param value {@link #name} (Official name of the health insurance product as 3720 * designated by the owner.). This is the underlying object with 3721 * id, value and extensions. The accessor "getName" gives direct 3722 * access to the value 3723 */ 3724 public InsurancePlan setNameElement(StringType value) { 3725 this.name = value; 3726 return this; 3727 } 3728 3729 /** 3730 * @return Official name of the health insurance product as designated by the 3731 * owner. 3732 */ 3733 public String getName() { 3734 return this.name == null ? null : this.name.getValue(); 3735 } 3736 3737 /** 3738 * @param value Official name of the health insurance product as designated by 3739 * the owner. 3740 */ 3741 public InsurancePlan setName(String value) { 3742 if (Utilities.noString(value)) 3743 this.name = null; 3744 else { 3745 if (this.name == null) 3746 this.name = new StringType(); 3747 this.name.setValue(value); 3748 } 3749 return this; 3750 } 3751 3752 /** 3753 * @return {@link #alias} (A list of alternate names that the product is known 3754 * as, or was known as in the past.) 3755 */ 3756 public List<StringType> getAlias() { 3757 if (this.alias == null) 3758 this.alias = new ArrayList<StringType>(); 3759 return this.alias; 3760 } 3761 3762 /** 3763 * @return Returns a reference to <code>this</code> for easy method chaining 3764 */ 3765 public InsurancePlan setAlias(List<StringType> theAlias) { 3766 this.alias = theAlias; 3767 return this; 3768 } 3769 3770 public boolean hasAlias() { 3771 if (this.alias == null) 3772 return false; 3773 for (StringType item : this.alias) 3774 if (!item.isEmpty()) 3775 return true; 3776 return false; 3777 } 3778 3779 /** 3780 * @return {@link #alias} (A list of alternate names that the product is known 3781 * as, or was known as in the past.) 3782 */ 3783 public StringType addAliasElement() {// 2 3784 StringType t = new StringType(); 3785 if (this.alias == null) 3786 this.alias = new ArrayList<StringType>(); 3787 this.alias.add(t); 3788 return t; 3789 } 3790 3791 /** 3792 * @param value {@link #alias} (A list of alternate names that the product is 3793 * known as, or was known as in the past.) 3794 */ 3795 public InsurancePlan addAlias(String value) { // 1 3796 StringType t = new StringType(); 3797 t.setValue(value); 3798 if (this.alias == null) 3799 this.alias = new ArrayList<StringType>(); 3800 this.alias.add(t); 3801 return this; 3802 } 3803 3804 /** 3805 * @param value {@link #alias} (A list of alternate names that the product is 3806 * known as, or was known as in the past.) 3807 */ 3808 public boolean hasAlias(String value) { 3809 if (this.alias == null) 3810 return false; 3811 for (StringType v : this.alias) 3812 if (v.getValue().equals(value)) // string 3813 return true; 3814 return false; 3815 } 3816 3817 /** 3818 * @return {@link #period} (The period of time that the health insurance product 3819 * is available.) 3820 */ 3821 public Period getPeriod() { 3822 if (this.period == null) 3823 if (Configuration.errorOnAutoCreate()) 3824 throw new Error("Attempt to auto-create InsurancePlan.period"); 3825 else if (Configuration.doAutoCreate()) 3826 this.period = new Period(); // cc 3827 return this.period; 3828 } 3829 3830 public boolean hasPeriod() { 3831 return this.period != null && !this.period.isEmpty(); 3832 } 3833 3834 /** 3835 * @param value {@link #period} (The period of time that the health insurance 3836 * product is available.) 3837 */ 3838 public InsurancePlan setPeriod(Period value) { 3839 this.period = value; 3840 return this; 3841 } 3842 3843 /** 3844 * @return {@link #ownedBy} (The entity that is providing the health insurance 3845 * product and underwriting the risk. This is typically an insurance 3846 * carriers, other third-party payers, or health plan sponsors comonly 3847 * referred to as 'payers'.) 3848 */ 3849 public Reference getOwnedBy() { 3850 if (this.ownedBy == null) 3851 if (Configuration.errorOnAutoCreate()) 3852 throw new Error("Attempt to auto-create InsurancePlan.ownedBy"); 3853 else if (Configuration.doAutoCreate()) 3854 this.ownedBy = new Reference(); // cc 3855 return this.ownedBy; 3856 } 3857 3858 public boolean hasOwnedBy() { 3859 return this.ownedBy != null && !this.ownedBy.isEmpty(); 3860 } 3861 3862 /** 3863 * @param value {@link #ownedBy} (The entity that is providing the health 3864 * insurance product and underwriting the risk. This is typically 3865 * an insurance carriers, other third-party payers, or health plan 3866 * sponsors comonly referred to as 'payers'.) 3867 */ 3868 public InsurancePlan setOwnedBy(Reference value) { 3869 this.ownedBy = value; 3870 return this; 3871 } 3872 3873 /** 3874 * @return {@link #ownedBy} The actual object that is the target of the 3875 * reference. The reference library doesn't populate this, but you can 3876 * use it to hold the resource if you resolve it. (The entity that is 3877 * providing the health insurance product and underwriting the risk. 3878 * This is typically an insurance carriers, other third-party payers, or 3879 * health plan sponsors comonly referred to as 'payers'.) 3880 */ 3881 public Organization getOwnedByTarget() { 3882 if (this.ownedByTarget == null) 3883 if (Configuration.errorOnAutoCreate()) 3884 throw new Error("Attempt to auto-create InsurancePlan.ownedBy"); 3885 else if (Configuration.doAutoCreate()) 3886 this.ownedByTarget = new Organization(); // aa 3887 return this.ownedByTarget; 3888 } 3889 3890 /** 3891 * @param value {@link #ownedBy} The actual object that is the target of the 3892 * reference. The reference library doesn't use these, but you can 3893 * use it to hold the resource if you resolve it. (The entity that 3894 * is providing the health insurance product and underwriting the 3895 * risk. This is typically an insurance carriers, other third-party 3896 * payers, or health plan sponsors comonly referred to as 3897 * 'payers'.) 3898 */ 3899 public InsurancePlan setOwnedByTarget(Organization value) { 3900 this.ownedByTarget = value; 3901 return this; 3902 } 3903 3904 /** 3905 * @return {@link #administeredBy} (An organization which administer other 3906 * services such as underwriting, customer service and/or claims 3907 * processing on behalf of the health insurance product owner.) 3908 */ 3909 public Reference getAdministeredBy() { 3910 if (this.administeredBy == null) 3911 if (Configuration.errorOnAutoCreate()) 3912 throw new Error("Attempt to auto-create InsurancePlan.administeredBy"); 3913 else if (Configuration.doAutoCreate()) 3914 this.administeredBy = new Reference(); // cc 3915 return this.administeredBy; 3916 } 3917 3918 public boolean hasAdministeredBy() { 3919 return this.administeredBy != null && !this.administeredBy.isEmpty(); 3920 } 3921 3922 /** 3923 * @param value {@link #administeredBy} (An organization which administer other 3924 * services such as underwriting, customer service and/or claims 3925 * processing on behalf of the health insurance product owner.) 3926 */ 3927 public InsurancePlan setAdministeredBy(Reference value) { 3928 this.administeredBy = value; 3929 return this; 3930 } 3931 3932 /** 3933 * @return {@link #administeredBy} The actual object that is the target of the 3934 * reference. The reference library doesn't populate this, but you can 3935 * use it to hold the resource if you resolve it. (An organization which 3936 * administer other services such as underwriting, customer service 3937 * and/or claims processing on behalf of the health insurance product 3938 * owner.) 3939 */ 3940 public Organization getAdministeredByTarget() { 3941 if (this.administeredByTarget == null) 3942 if (Configuration.errorOnAutoCreate()) 3943 throw new Error("Attempt to auto-create InsurancePlan.administeredBy"); 3944 else if (Configuration.doAutoCreate()) 3945 this.administeredByTarget = new Organization(); // aa 3946 return this.administeredByTarget; 3947 } 3948 3949 /** 3950 * @param value {@link #administeredBy} The actual object that is the target of 3951 * the reference. The reference library doesn't use these, but you 3952 * can use it to hold the resource if you resolve it. (An 3953 * organization which administer other services such as 3954 * underwriting, customer service and/or claims processing on 3955 * behalf of the health insurance product owner.) 3956 */ 3957 public InsurancePlan setAdministeredByTarget(Organization value) { 3958 this.administeredByTarget = value; 3959 return this; 3960 } 3961 3962 /** 3963 * @return {@link #coverageArea} (The geographic region in which a health 3964 * insurance product's benefits apply.) 3965 */ 3966 public List<Reference> getCoverageArea() { 3967 if (this.coverageArea == null) 3968 this.coverageArea = new ArrayList<Reference>(); 3969 return this.coverageArea; 3970 } 3971 3972 /** 3973 * @return Returns a reference to <code>this</code> for easy method chaining 3974 */ 3975 public InsurancePlan setCoverageArea(List<Reference> theCoverageArea) { 3976 this.coverageArea = theCoverageArea; 3977 return this; 3978 } 3979 3980 public boolean hasCoverageArea() { 3981 if (this.coverageArea == null) 3982 return false; 3983 for (Reference item : this.coverageArea) 3984 if (!item.isEmpty()) 3985 return true; 3986 return false; 3987 } 3988 3989 public Reference addCoverageArea() { // 3 3990 Reference t = new Reference(); 3991 if (this.coverageArea == null) 3992 this.coverageArea = new ArrayList<Reference>(); 3993 this.coverageArea.add(t); 3994 return t; 3995 } 3996 3997 public InsurancePlan addCoverageArea(Reference t) { // 3 3998 if (t == null) 3999 return this; 4000 if (this.coverageArea == null) 4001 this.coverageArea = new ArrayList<Reference>(); 4002 this.coverageArea.add(t); 4003 return this; 4004 } 4005 4006 /** 4007 * @return The first repetition of repeating field {@link #coverageArea}, 4008 * creating it if it does not already exist 4009 */ 4010 public Reference getCoverageAreaFirstRep() { 4011 if (getCoverageArea().isEmpty()) { 4012 addCoverageArea(); 4013 } 4014 return getCoverageArea().get(0); 4015 } 4016 4017 /** 4018 * @return {@link #contact} (The contact for the health insurance product for a 4019 * certain purpose.) 4020 */ 4021 public List<InsurancePlanContactComponent> getContact() { 4022 if (this.contact == null) 4023 this.contact = new ArrayList<InsurancePlanContactComponent>(); 4024 return this.contact; 4025 } 4026 4027 /** 4028 * @return Returns a reference to <code>this</code> for easy method chaining 4029 */ 4030 public InsurancePlan setContact(List<InsurancePlanContactComponent> theContact) { 4031 this.contact = theContact; 4032 return this; 4033 } 4034 4035 public boolean hasContact() { 4036 if (this.contact == null) 4037 return false; 4038 for (InsurancePlanContactComponent item : this.contact) 4039 if (!item.isEmpty()) 4040 return true; 4041 return false; 4042 } 4043 4044 public InsurancePlanContactComponent addContact() { // 3 4045 InsurancePlanContactComponent t = new InsurancePlanContactComponent(); 4046 if (this.contact == null) 4047 this.contact = new ArrayList<InsurancePlanContactComponent>(); 4048 this.contact.add(t); 4049 return t; 4050 } 4051 4052 public InsurancePlan addContact(InsurancePlanContactComponent t) { // 3 4053 if (t == null) 4054 return this; 4055 if (this.contact == null) 4056 this.contact = new ArrayList<InsurancePlanContactComponent>(); 4057 this.contact.add(t); 4058 return this; 4059 } 4060 4061 /** 4062 * @return The first repetition of repeating field {@link #contact}, creating it 4063 * if it does not already exist 4064 */ 4065 public InsurancePlanContactComponent getContactFirstRep() { 4066 if (getContact().isEmpty()) { 4067 addContact(); 4068 } 4069 return getContact().get(0); 4070 } 4071 4072 /** 4073 * @return {@link #endpoint} (The technical endpoints providing access to 4074 * services operated for the health insurance product.) 4075 */ 4076 public List<Reference> getEndpoint() { 4077 if (this.endpoint == null) 4078 this.endpoint = new ArrayList<Reference>(); 4079 return this.endpoint; 4080 } 4081 4082 /** 4083 * @return Returns a reference to <code>this</code> for easy method chaining 4084 */ 4085 public InsurancePlan setEndpoint(List<Reference> theEndpoint) { 4086 this.endpoint = theEndpoint; 4087 return this; 4088 } 4089 4090 public boolean hasEndpoint() { 4091 if (this.endpoint == null) 4092 return false; 4093 for (Reference item : this.endpoint) 4094 if (!item.isEmpty()) 4095 return true; 4096 return false; 4097 } 4098 4099 public Reference addEndpoint() { // 3 4100 Reference t = new Reference(); 4101 if (this.endpoint == null) 4102 this.endpoint = new ArrayList<Reference>(); 4103 this.endpoint.add(t); 4104 return t; 4105 } 4106 4107 public InsurancePlan addEndpoint(Reference t) { // 3 4108 if (t == null) 4109 return this; 4110 if (this.endpoint == null) 4111 this.endpoint = new ArrayList<Reference>(); 4112 this.endpoint.add(t); 4113 return this; 4114 } 4115 4116 /** 4117 * @return The first repetition of repeating field {@link #endpoint}, creating 4118 * it if it does not already exist 4119 */ 4120 public Reference getEndpointFirstRep() { 4121 if (getEndpoint().isEmpty()) { 4122 addEndpoint(); 4123 } 4124 return getEndpoint().get(0); 4125 } 4126 4127 /** 4128 * @return {@link #network} (Reference to the network included in the health 4129 * insurance product.) 4130 */ 4131 public List<Reference> getNetwork() { 4132 if (this.network == null) 4133 this.network = new ArrayList<Reference>(); 4134 return this.network; 4135 } 4136 4137 /** 4138 * @return Returns a reference to <code>this</code> for easy method chaining 4139 */ 4140 public InsurancePlan setNetwork(List<Reference> theNetwork) { 4141 this.network = theNetwork; 4142 return this; 4143 } 4144 4145 public boolean hasNetwork() { 4146 if (this.network == null) 4147 return false; 4148 for (Reference item : this.network) 4149 if (!item.isEmpty()) 4150 return true; 4151 return false; 4152 } 4153 4154 public Reference addNetwork() { // 3 4155 Reference t = new Reference(); 4156 if (this.network == null) 4157 this.network = new ArrayList<Reference>(); 4158 this.network.add(t); 4159 return t; 4160 } 4161 4162 public InsurancePlan addNetwork(Reference t) { // 3 4163 if (t == null) 4164 return this; 4165 if (this.network == null) 4166 this.network = new ArrayList<Reference>(); 4167 this.network.add(t); 4168 return this; 4169 } 4170 4171 /** 4172 * @return The first repetition of repeating field {@link #network}, creating it 4173 * if it does not already exist 4174 */ 4175 public Reference getNetworkFirstRep() { 4176 if (getNetwork().isEmpty()) { 4177 addNetwork(); 4178 } 4179 return getNetwork().get(0); 4180 } 4181 4182 /** 4183 * @return {@link #coverage} (Details about the coverage offered by the 4184 * insurance product.) 4185 */ 4186 public List<InsurancePlanCoverageComponent> getCoverage() { 4187 if (this.coverage == null) 4188 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4189 return this.coverage; 4190 } 4191 4192 /** 4193 * @return Returns a reference to <code>this</code> for easy method chaining 4194 */ 4195 public InsurancePlan setCoverage(List<InsurancePlanCoverageComponent> theCoverage) { 4196 this.coverage = theCoverage; 4197 return this; 4198 } 4199 4200 public boolean hasCoverage() { 4201 if (this.coverage == null) 4202 return false; 4203 for (InsurancePlanCoverageComponent item : this.coverage) 4204 if (!item.isEmpty()) 4205 return true; 4206 return false; 4207 } 4208 4209 public InsurancePlanCoverageComponent addCoverage() { // 3 4210 InsurancePlanCoverageComponent t = new InsurancePlanCoverageComponent(); 4211 if (this.coverage == null) 4212 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4213 this.coverage.add(t); 4214 return t; 4215 } 4216 4217 public InsurancePlan addCoverage(InsurancePlanCoverageComponent t) { // 3 4218 if (t == null) 4219 return this; 4220 if (this.coverage == null) 4221 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4222 this.coverage.add(t); 4223 return this; 4224 } 4225 4226 /** 4227 * @return The first repetition of repeating field {@link #coverage}, creating 4228 * it if it does not already exist 4229 */ 4230 public InsurancePlanCoverageComponent getCoverageFirstRep() { 4231 if (getCoverage().isEmpty()) { 4232 addCoverage(); 4233 } 4234 return getCoverage().get(0); 4235 } 4236 4237 /** 4238 * @return {@link #plan} (Details about an insurance plan.) 4239 */ 4240 public List<InsurancePlanPlanComponent> getPlan() { 4241 if (this.plan == null) 4242 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4243 return this.plan; 4244 } 4245 4246 /** 4247 * @return Returns a reference to <code>this</code> for easy method chaining 4248 */ 4249 public InsurancePlan setPlan(List<InsurancePlanPlanComponent> thePlan) { 4250 this.plan = thePlan; 4251 return this; 4252 } 4253 4254 public boolean hasPlan() { 4255 if (this.plan == null) 4256 return false; 4257 for (InsurancePlanPlanComponent item : this.plan) 4258 if (!item.isEmpty()) 4259 return true; 4260 return false; 4261 } 4262 4263 public InsurancePlanPlanComponent addPlan() { // 3 4264 InsurancePlanPlanComponent t = new InsurancePlanPlanComponent(); 4265 if (this.plan == null) 4266 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4267 this.plan.add(t); 4268 return t; 4269 } 4270 4271 public InsurancePlan addPlan(InsurancePlanPlanComponent t) { // 3 4272 if (t == null) 4273 return this; 4274 if (this.plan == null) 4275 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 4276 this.plan.add(t); 4277 return this; 4278 } 4279 4280 /** 4281 * @return The first repetition of repeating field {@link #plan}, creating it if 4282 * it does not already exist 4283 */ 4284 public InsurancePlanPlanComponent getPlanFirstRep() { 4285 if (getPlan().isEmpty()) { 4286 addPlan(); 4287 } 4288 return getPlan().get(0); 4289 } 4290 4291 protected void listChildren(List<Property> children) { 4292 super.listChildren(children); 4293 children.add(new Property("identifier", "Identifier", 4294 "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 4295 0, java.lang.Integer.MAX_VALUE, identifier)); 4296 children.add(new Property("status", "code", "The current state of the health insurance product.", 0, 1, status)); 4297 children.add(new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, 4298 java.lang.Integer.MAX_VALUE, type)); 4299 children.add(new Property("name", "string", 4300 "Official name of the health insurance product as designated by the owner.", 0, 1, name)); 4301 children.add(new Property("alias", "string", 4302 "A list of alternate names that the product is known as, or was known as in the past.", 0, 4303 java.lang.Integer.MAX_VALUE, alias)); 4304 children.add(new Property("period", "Period", "The period of time that the health insurance product is available.", 4305 0, 1, period)); 4306 children.add(new Property("ownedBy", "Reference(Organization)", 4307 "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 4308 0, 1, ownedBy)); 4309 children.add(new Property("administeredBy", "Reference(Organization)", 4310 "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 4311 0, 1, administeredBy)); 4312 children.add(new Property("coverageArea", "Reference(Location)", 4313 "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 4314 coverageArea)); 4315 children.add(new Property("contact", "", "The contact for the health insurance product for a certain purpose.", 0, 4316 java.lang.Integer.MAX_VALUE, contact)); 4317 children.add(new Property("endpoint", "Reference(Endpoint)", 4318 "The technical endpoints providing access to services operated for the health insurance product.", 0, 4319 java.lang.Integer.MAX_VALUE, endpoint)); 4320 children.add(new Property("network", "Reference(Organization)", 4321 "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, network)); 4322 children.add(new Property("coverage", "", "Details about the coverage offered by the insurance product.", 0, 4323 java.lang.Integer.MAX_VALUE, coverage)); 4324 children.add(new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, plan)); 4325 } 4326 4327 @Override 4328 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4329 switch (_hash) { 4330 case -1618432855: 4331 /* identifier */ return new Property("identifier", "Identifier", 4332 "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 4333 0, java.lang.Integer.MAX_VALUE, identifier); 4334 case -892481550: 4335 /* status */ return new Property("status", "code", "The current state of the health insurance product.", 0, 1, 4336 status); 4337 case 3575610: 4338 /* type */ return new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, 4339 java.lang.Integer.MAX_VALUE, type); 4340 case 3373707: 4341 /* name */ return new Property("name", "string", 4342 "Official name of the health insurance product as designated by the owner.", 0, 1, name); 4343 case 92902992: 4344 /* alias */ return new Property("alias", "string", 4345 "A list of alternate names that the product is known as, or was known as in the past.", 0, 4346 java.lang.Integer.MAX_VALUE, alias); 4347 case -991726143: 4348 /* period */ return new Property("period", "Period", 4349 "The period of time that the health insurance product is available.", 0, 1, period); 4350 case -1054743076: 4351 /* ownedBy */ return new Property("ownedBy", "Reference(Organization)", 4352 "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 4353 0, 1, ownedBy); 4354 case 898770462: 4355 /* administeredBy */ return new Property("administeredBy", "Reference(Organization)", 4356 "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 4357 0, 1, administeredBy); 4358 case -1532328299: 4359 /* coverageArea */ return new Property("coverageArea", "Reference(Location)", 4360 "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, 4361 coverageArea); 4362 case 951526432: 4363 /* contact */ return new Property("contact", "", 4364 "The contact for the health insurance product for a certain purpose.", 0, java.lang.Integer.MAX_VALUE, 4365 contact); 4366 case 1741102485: 4367 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 4368 "The technical endpoints providing access to services operated for the health insurance product.", 0, 4369 java.lang.Integer.MAX_VALUE, endpoint); 4370 case 1843485230: 4371 /* network */ return new Property("network", "Reference(Organization)", 4372 "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, 4373 network); 4374 case -351767064: 4375 /* coverage */ return new Property("coverage", "", "Details about the coverage offered by the insurance product.", 4376 0, java.lang.Integer.MAX_VALUE, coverage); 4377 case 3443497: 4378 /* plan */ return new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, 4379 plan); 4380 default: 4381 return super.getNamedProperty(_hash, _name, _checkValid); 4382 } 4383 4384 } 4385 4386 @Override 4387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4388 switch (hash) { 4389 case -1618432855: 4390 /* identifier */ return this.identifier == null ? new Base[0] 4391 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4392 case -892481550: 4393 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 4394 case 3575610: 4395 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 4396 case 3373707: 4397 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 4398 case 92902992: 4399 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 4400 case -991726143: 4401 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 4402 case -1054743076: 4403 /* ownedBy */ return this.ownedBy == null ? new Base[0] : new Base[] { this.ownedBy }; // Reference 4404 case 898770462: 4405 /* administeredBy */ return this.administeredBy == null ? new Base[0] : new Base[] { this.administeredBy }; // Reference 4406 case -1532328299: 4407 /* coverageArea */ return this.coverageArea == null ? new Base[0] 4408 : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 4409 case 951526432: 4410 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // InsurancePlanContactComponent 4411 case 1741102485: 4412 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 4413 case 1843485230: 4414 /* network */ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 4415 case -351767064: 4416 /* coverage */ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // InsurancePlanCoverageComponent 4417 case 3443497: 4418 /* plan */ return this.plan == null ? new Base[0] : this.plan.toArray(new Base[this.plan.size()]); // InsurancePlanPlanComponent 4419 default: 4420 return super.getProperty(hash, name, checkValid); 4421 } 4422 4423 } 4424 4425 @Override 4426 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4427 switch (hash) { 4428 case -1618432855: // identifier 4429 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4430 return value; 4431 case -892481550: // status 4432 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4433 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4434 return value; 4435 case 3575610: // type 4436 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 4437 return value; 4438 case 3373707: // name 4439 this.name = castToString(value); // StringType 4440 return value; 4441 case 92902992: // alias 4442 this.getAlias().add(castToString(value)); // StringType 4443 return value; 4444 case -991726143: // period 4445 this.period = castToPeriod(value); // Period 4446 return value; 4447 case -1054743076: // ownedBy 4448 this.ownedBy = castToReference(value); // Reference 4449 return value; 4450 case 898770462: // administeredBy 4451 this.administeredBy = castToReference(value); // Reference 4452 return value; 4453 case -1532328299: // coverageArea 4454 this.getCoverageArea().add(castToReference(value)); // Reference 4455 return value; 4456 case 951526432: // contact 4457 this.getContact().add((InsurancePlanContactComponent) value); // InsurancePlanContactComponent 4458 return value; 4459 case 1741102485: // endpoint 4460 this.getEndpoint().add(castToReference(value)); // Reference 4461 return value; 4462 case 1843485230: // network 4463 this.getNetwork().add(castToReference(value)); // Reference 4464 return value; 4465 case -351767064: // coverage 4466 this.getCoverage().add((InsurancePlanCoverageComponent) value); // InsurancePlanCoverageComponent 4467 return value; 4468 case 3443497: // plan 4469 this.getPlan().add((InsurancePlanPlanComponent) value); // InsurancePlanPlanComponent 4470 return value; 4471 default: 4472 return super.setProperty(hash, name, value); 4473 } 4474 4475 } 4476 4477 @Override 4478 public Base setProperty(String name, Base value) throws FHIRException { 4479 if (name.equals("identifier")) { 4480 this.getIdentifier().add(castToIdentifier(value)); 4481 } else if (name.equals("status")) { 4482 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 4483 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 4484 } else if (name.equals("type")) { 4485 this.getType().add(castToCodeableConcept(value)); 4486 } else if (name.equals("name")) { 4487 this.name = castToString(value); // StringType 4488 } else if (name.equals("alias")) { 4489 this.getAlias().add(castToString(value)); 4490 } else if (name.equals("period")) { 4491 this.period = castToPeriod(value); // Period 4492 } else if (name.equals("ownedBy")) { 4493 this.ownedBy = castToReference(value); // Reference 4494 } else if (name.equals("administeredBy")) { 4495 this.administeredBy = castToReference(value); // Reference 4496 } else if (name.equals("coverageArea")) { 4497 this.getCoverageArea().add(castToReference(value)); 4498 } else if (name.equals("contact")) { 4499 this.getContact().add((InsurancePlanContactComponent) value); 4500 } else if (name.equals("endpoint")) { 4501 this.getEndpoint().add(castToReference(value)); 4502 } else if (name.equals("network")) { 4503 this.getNetwork().add(castToReference(value)); 4504 } else if (name.equals("coverage")) { 4505 this.getCoverage().add((InsurancePlanCoverageComponent) value); 4506 } else if (name.equals("plan")) { 4507 this.getPlan().add((InsurancePlanPlanComponent) value); 4508 } else 4509 return super.setProperty(name, value); 4510 return value; 4511 } 4512 4513 @Override 4514 public void removeChild(String name, Base value) throws FHIRException { 4515 if (name.equals("identifier")) { 4516 this.getIdentifier().remove(castToIdentifier(value)); 4517 } else if (name.equals("status")) { 4518 this.status = null; 4519 } else if (name.equals("type")) { 4520 this.getType().remove(castToCodeableConcept(value)); 4521 } else if (name.equals("name")) { 4522 this.name = null; 4523 } else if (name.equals("alias")) { 4524 this.getAlias().remove(castToString(value)); 4525 } else if (name.equals("period")) { 4526 this.period = null; 4527 } else if (name.equals("ownedBy")) { 4528 this.ownedBy = null; 4529 } else if (name.equals("administeredBy")) { 4530 this.administeredBy = null; 4531 } else if (name.equals("coverageArea")) { 4532 this.getCoverageArea().remove(castToReference(value)); 4533 } else if (name.equals("contact")) { 4534 this.getContact().remove((InsurancePlanContactComponent) value); 4535 } else if (name.equals("endpoint")) { 4536 this.getEndpoint().remove(castToReference(value)); 4537 } else if (name.equals("network")) { 4538 this.getNetwork().remove(castToReference(value)); 4539 } else if (name.equals("coverage")) { 4540 this.getCoverage().remove((InsurancePlanCoverageComponent) value); 4541 } else if (name.equals("plan")) { 4542 this.getPlan().remove((InsurancePlanPlanComponent) value); 4543 } else 4544 super.removeChild(name, value); 4545 4546 } 4547 4548 @Override 4549 public Base makeProperty(int hash, String name) throws FHIRException { 4550 switch (hash) { 4551 case -1618432855: 4552 return addIdentifier(); 4553 case -892481550: 4554 return getStatusElement(); 4555 case 3575610: 4556 return addType(); 4557 case 3373707: 4558 return getNameElement(); 4559 case 92902992: 4560 return addAliasElement(); 4561 case -991726143: 4562 return getPeriod(); 4563 case -1054743076: 4564 return getOwnedBy(); 4565 case 898770462: 4566 return getAdministeredBy(); 4567 case -1532328299: 4568 return addCoverageArea(); 4569 case 951526432: 4570 return addContact(); 4571 case 1741102485: 4572 return addEndpoint(); 4573 case 1843485230: 4574 return addNetwork(); 4575 case -351767064: 4576 return addCoverage(); 4577 case 3443497: 4578 return addPlan(); 4579 default: 4580 return super.makeProperty(hash, name); 4581 } 4582 4583 } 4584 4585 @Override 4586 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4587 switch (hash) { 4588 case -1618432855: 4589 /* identifier */ return new String[] { "Identifier" }; 4590 case -892481550: 4591 /* status */ return new String[] { "code" }; 4592 case 3575610: 4593 /* type */ return new String[] { "CodeableConcept" }; 4594 case 3373707: 4595 /* name */ return new String[] { "string" }; 4596 case 92902992: 4597 /* alias */ return new String[] { "string" }; 4598 case -991726143: 4599 /* period */ return new String[] { "Period" }; 4600 case -1054743076: 4601 /* ownedBy */ return new String[] { "Reference" }; 4602 case 898770462: 4603 /* administeredBy */ return new String[] { "Reference" }; 4604 case -1532328299: 4605 /* coverageArea */ return new String[] { "Reference" }; 4606 case 951526432: 4607 /* contact */ return new String[] {}; 4608 case 1741102485: 4609 /* endpoint */ return new String[] { "Reference" }; 4610 case 1843485230: 4611 /* network */ return new String[] { "Reference" }; 4612 case -351767064: 4613 /* coverage */ return new String[] {}; 4614 case 3443497: 4615 /* plan */ return new String[] {}; 4616 default: 4617 return super.getTypesForProperty(hash, name); 4618 } 4619 4620 } 4621 4622 @Override 4623 public Base addChild(String name) throws FHIRException { 4624 if (name.equals("identifier")) { 4625 return addIdentifier(); 4626 } else if (name.equals("status")) { 4627 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.status"); 4628 } else if (name.equals("type")) { 4629 return addType(); 4630 } else if (name.equals("name")) { 4631 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.name"); 4632 } else if (name.equals("alias")) { 4633 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.alias"); 4634 } else if (name.equals("period")) { 4635 this.period = new Period(); 4636 return this.period; 4637 } else if (name.equals("ownedBy")) { 4638 this.ownedBy = new Reference(); 4639 return this.ownedBy; 4640 } else if (name.equals("administeredBy")) { 4641 this.administeredBy = new Reference(); 4642 return this.administeredBy; 4643 } else if (name.equals("coverageArea")) { 4644 return addCoverageArea(); 4645 } else if (name.equals("contact")) { 4646 return addContact(); 4647 } else if (name.equals("endpoint")) { 4648 return addEndpoint(); 4649 } else if (name.equals("network")) { 4650 return addNetwork(); 4651 } else if (name.equals("coverage")) { 4652 return addCoverage(); 4653 } else if (name.equals("plan")) { 4654 return addPlan(); 4655 } else 4656 return super.addChild(name); 4657 } 4658 4659 public String fhirType() { 4660 return "InsurancePlan"; 4661 4662 } 4663 4664 public InsurancePlan copy() { 4665 InsurancePlan dst = new InsurancePlan(); 4666 copyValues(dst); 4667 return dst; 4668 } 4669 4670 public void copyValues(InsurancePlan dst) { 4671 super.copyValues(dst); 4672 if (identifier != null) { 4673 dst.identifier = new ArrayList<Identifier>(); 4674 for (Identifier i : identifier) 4675 dst.identifier.add(i.copy()); 4676 } 4677 ; 4678 dst.status = status == null ? null : status.copy(); 4679 if (type != null) { 4680 dst.type = new ArrayList<CodeableConcept>(); 4681 for (CodeableConcept i : type) 4682 dst.type.add(i.copy()); 4683 } 4684 ; 4685 dst.name = name == null ? null : name.copy(); 4686 if (alias != null) { 4687 dst.alias = new ArrayList<StringType>(); 4688 for (StringType i : alias) 4689 dst.alias.add(i.copy()); 4690 } 4691 ; 4692 dst.period = period == null ? null : period.copy(); 4693 dst.ownedBy = ownedBy == null ? null : ownedBy.copy(); 4694 dst.administeredBy = administeredBy == null ? null : administeredBy.copy(); 4695 if (coverageArea != null) { 4696 dst.coverageArea = new ArrayList<Reference>(); 4697 for (Reference i : coverageArea) 4698 dst.coverageArea.add(i.copy()); 4699 } 4700 ; 4701 if (contact != null) { 4702 dst.contact = new ArrayList<InsurancePlanContactComponent>(); 4703 for (InsurancePlanContactComponent i : contact) 4704 dst.contact.add(i.copy()); 4705 } 4706 ; 4707 if (endpoint != null) { 4708 dst.endpoint = new ArrayList<Reference>(); 4709 for (Reference i : endpoint) 4710 dst.endpoint.add(i.copy()); 4711 } 4712 ; 4713 if (network != null) { 4714 dst.network = new ArrayList<Reference>(); 4715 for (Reference i : network) 4716 dst.network.add(i.copy()); 4717 } 4718 ; 4719 if (coverage != null) { 4720 dst.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 4721 for (InsurancePlanCoverageComponent i : coverage) 4722 dst.coverage.add(i.copy()); 4723 } 4724 ; 4725 if (plan != null) { 4726 dst.plan = new ArrayList<InsurancePlanPlanComponent>(); 4727 for (InsurancePlanPlanComponent i : plan) 4728 dst.plan.add(i.copy()); 4729 } 4730 ; 4731 } 4732 4733 protected InsurancePlan typedCopy() { 4734 return copy(); 4735 } 4736 4737 @Override 4738 public boolean equalsDeep(Base other_) { 4739 if (!super.equalsDeep(other_)) 4740 return false; 4741 if (!(other_ instanceof InsurancePlan)) 4742 return false; 4743 InsurancePlan o = (InsurancePlan) other_; 4744 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4745 && compareDeep(type, o.type, true) && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) 4746 && compareDeep(period, o.period, true) && compareDeep(ownedBy, o.ownedBy, true) 4747 && compareDeep(administeredBy, o.administeredBy, true) && compareDeep(coverageArea, o.coverageArea, true) 4748 && compareDeep(contact, o.contact, true) && compareDeep(endpoint, o.endpoint, true) 4749 && compareDeep(network, o.network, true) && compareDeep(coverage, o.coverage, true) 4750 && compareDeep(plan, o.plan, true); 4751 } 4752 4753 @Override 4754 public boolean equalsShallow(Base other_) { 4755 if (!super.equalsShallow(other_)) 4756 return false; 4757 if (!(other_ instanceof InsurancePlan)) 4758 return false; 4759 InsurancePlan o = (InsurancePlan) other_; 4760 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 4761 && compareValues(alias, o.alias, true); 4762 } 4763 4764 public boolean isEmpty() { 4765 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type, name, alias, period, 4766 ownedBy, administeredBy, coverageArea, contact, endpoint, network, coverage, plan); 4767 } 4768 4769 @Override 4770 public ResourceType getResourceType() { 4771 return ResourceType.InsurancePlan; 4772 } 4773 4774 /** 4775 * Search parameter: <b>identifier</b> 4776 * <p> 4777 * Description: <b>Any identifier for the organization (not the accreditation 4778 * issuer's identifier)</b><br> 4779 * Type: <b>token</b><br> 4780 * Path: <b>InsurancePlan.identifier</b><br> 4781 * </p> 4782 */ 4783 @SearchParamDefinition(name = "identifier", path = "InsurancePlan.identifier", description = "Any identifier for the organization (not the accreditation issuer's identifier)", type = "token") 4784 public static final String SP_IDENTIFIER = "identifier"; 4785 /** 4786 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4787 * <p> 4788 * Description: <b>Any identifier for the organization (not the accreditation 4789 * issuer's identifier)</b><br> 4790 * Type: <b>token</b><br> 4791 * Path: <b>InsurancePlan.identifier</b><br> 4792 * </p> 4793 */ 4794 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4795 SP_IDENTIFIER); 4796 4797 /** 4798 * Search parameter: <b>address</b> 4799 * <p> 4800 * Description: <b>A server defined search that may match any of the string 4801 * fields in the Address, including line, city, district, state, country, 4802 * postalCode, and/or text</b><br> 4803 * Type: <b>string</b><br> 4804 * Path: <b>InsurancePlan.contact.address</b><br> 4805 * </p> 4806 */ 4807 @SearchParamDefinition(name = "address", path = "InsurancePlan.contact.address", description = "A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type = "string") 4808 public static final String SP_ADDRESS = "address"; 4809 /** 4810 * <b>Fluent Client</b> search parameter constant for <b>address</b> 4811 * <p> 4812 * Description: <b>A server defined search that may match any of the string 4813 * fields in the Address, including line, city, district, state, country, 4814 * postalCode, and/or text</b><br> 4815 * Type: <b>string</b><br> 4816 * Path: <b>InsurancePlan.contact.address</b><br> 4817 * </p> 4818 */ 4819 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 4820 SP_ADDRESS); 4821 4822 /** 4823 * Search parameter: <b>address-state</b> 4824 * <p> 4825 * Description: <b>A state specified in an address</b><br> 4826 * Type: <b>string</b><br> 4827 * Path: <b>InsurancePlan.contact.address.state</b><br> 4828 * </p> 4829 */ 4830 @SearchParamDefinition(name = "address-state", path = "InsurancePlan.contact.address.state", description = "A state specified in an address", type = "string") 4831 public static final String SP_ADDRESS_STATE = "address-state"; 4832 /** 4833 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 4834 * <p> 4835 * Description: <b>A state specified in an address</b><br> 4836 * Type: <b>string</b><br> 4837 * Path: <b>InsurancePlan.contact.address.state</b><br> 4838 * </p> 4839 */ 4840 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4841 SP_ADDRESS_STATE); 4842 4843 /** 4844 * Search parameter: <b>owned-by</b> 4845 * <p> 4846 * Description: <b>An organization of which this organization forms a 4847 * part</b><br> 4848 * Type: <b>reference</b><br> 4849 * Path: <b>InsurancePlan.ownedBy</b><br> 4850 * </p> 4851 */ 4852 @SearchParamDefinition(name = "owned-by", path = "InsurancePlan.ownedBy", description = "An organization of which this organization forms a part", type = "reference", target = { 4853 Organization.class }) 4854 public static final String SP_OWNED_BY = "owned-by"; 4855 /** 4856 * <b>Fluent Client</b> search parameter constant for <b>owned-by</b> 4857 * <p> 4858 * Description: <b>An organization of which this organization forms a 4859 * part</b><br> 4860 * Type: <b>reference</b><br> 4861 * Path: <b>InsurancePlan.ownedBy</b><br> 4862 * </p> 4863 */ 4864 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4865 SP_OWNED_BY); 4866 4867 /** 4868 * Constant for fluent queries to be used to add include statements. Specifies 4869 * the path value of "<b>InsurancePlan:owned-by</b>". 4870 */ 4871 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNED_BY = new ca.uhn.fhir.model.api.Include( 4872 "InsurancePlan:owned-by").toLocked(); 4873 4874 /** 4875 * Search parameter: <b>type</b> 4876 * <p> 4877 * Description: <b>A code for the type of organization</b><br> 4878 * Type: <b>token</b><br> 4879 * Path: <b>InsurancePlan.type</b><br> 4880 * </p> 4881 */ 4882 @SearchParamDefinition(name = "type", path = "InsurancePlan.type", description = "A code for the type of organization", type = "token") 4883 public static final String SP_TYPE = "type"; 4884 /** 4885 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4886 * <p> 4887 * Description: <b>A code for the type of organization</b><br> 4888 * Type: <b>token</b><br> 4889 * Path: <b>InsurancePlan.type</b><br> 4890 * </p> 4891 */ 4892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4893 SP_TYPE); 4894 4895 /** 4896 * Search parameter: <b>address-postalcode</b> 4897 * <p> 4898 * Description: <b>A postal code specified in an address</b><br> 4899 * Type: <b>string</b><br> 4900 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 4901 * </p> 4902 */ 4903 @SearchParamDefinition(name = "address-postalcode", path = "InsurancePlan.contact.address.postalCode", description = "A postal code specified in an address", type = "string") 4904 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 4905 /** 4906 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 4907 * <p> 4908 * Description: <b>A postal code specified in an address</b><br> 4909 * Type: <b>string</b><br> 4910 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 4911 * </p> 4912 */ 4913 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 4914 SP_ADDRESS_POSTALCODE); 4915 4916 /** 4917 * Search parameter: <b>administered-by</b> 4918 * <p> 4919 * Description: <b>Product administrator</b><br> 4920 * Type: <b>reference</b><br> 4921 * Path: <b>InsurancePlan.administeredBy</b><br> 4922 * </p> 4923 */ 4924 @SearchParamDefinition(name = "administered-by", path = "InsurancePlan.administeredBy", description = "Product administrator", type = "reference", target = { 4925 Organization.class }) 4926 public static final String SP_ADMINISTERED_BY = "administered-by"; 4927 /** 4928 * <b>Fluent Client</b> search parameter constant for <b>administered-by</b> 4929 * <p> 4930 * Description: <b>Product administrator</b><br> 4931 * Type: <b>reference</b><br> 4932 * Path: <b>InsurancePlan.administeredBy</b><br> 4933 * </p> 4934 */ 4935 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ADMINISTERED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4936 SP_ADMINISTERED_BY); 4937 4938 /** 4939 * Constant for fluent queries to be used to add include statements. Specifies 4940 * the path value of "<b>InsurancePlan:administered-by</b>". 4941 */ 4942 public static final ca.uhn.fhir.model.api.Include INCLUDE_ADMINISTERED_BY = new ca.uhn.fhir.model.api.Include( 4943 "InsurancePlan:administered-by").toLocked(); 4944 4945 /** 4946 * Search parameter: <b>address-country</b> 4947 * <p> 4948 * Description: <b>A country specified in an address</b><br> 4949 * Type: <b>string</b><br> 4950 * Path: <b>InsurancePlan.contact.address.country</b><br> 4951 * </p> 4952 */ 4953 @SearchParamDefinition(name = "address-country", path = "InsurancePlan.contact.address.country", description = "A country specified in an address", type = "string") 4954 public static final String SP_ADDRESS_COUNTRY = "address-country"; 4955 /** 4956 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 4957 * <p> 4958 * Description: <b>A country specified in an address</b><br> 4959 * Type: <b>string</b><br> 4960 * Path: <b>InsurancePlan.contact.address.country</b><br> 4961 * </p> 4962 */ 4963 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 4964 SP_ADDRESS_COUNTRY); 4965 4966 /** 4967 * Search parameter: <b>endpoint</b> 4968 * <p> 4969 * Description: <b>Technical endpoint</b><br> 4970 * Type: <b>reference</b><br> 4971 * Path: <b>InsurancePlan.endpoint</b><br> 4972 * </p> 4973 */ 4974 @SearchParamDefinition(name = "endpoint", path = "InsurancePlan.endpoint", description = "Technical endpoint", type = "reference", target = { 4975 Endpoint.class }) 4976 public static final String SP_ENDPOINT = "endpoint"; 4977 /** 4978 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 4979 * <p> 4980 * Description: <b>Technical endpoint</b><br> 4981 * Type: <b>reference</b><br> 4982 * Path: <b>InsurancePlan.endpoint</b><br> 4983 * </p> 4984 */ 4985 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4986 SP_ENDPOINT); 4987 4988 /** 4989 * Constant for fluent queries to be used to add include statements. Specifies 4990 * the path value of "<b>InsurancePlan:endpoint</b>". 4991 */ 4992 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 4993 "InsurancePlan:endpoint").toLocked(); 4994 4995 /** 4996 * Search parameter: <b>phonetic</b> 4997 * <p> 4998 * Description: <b>A portion of the organization's name using some kind of 4999 * phonetic matching algorithm</b><br> 5000 * Type: <b>string</b><br> 5001 * Path: <b>InsurancePlan.name</b><br> 5002 * </p> 5003 */ 5004 @SearchParamDefinition(name = "phonetic", path = "InsurancePlan.name", description = "A portion of the organization's name using some kind of phonetic matching algorithm", type = "string") 5005 public static final String SP_PHONETIC = "phonetic"; 5006 /** 5007 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 5008 * <p> 5009 * Description: <b>A portion of the organization's name using some kind of 5010 * phonetic matching algorithm</b><br> 5011 * Type: <b>string</b><br> 5012 * Path: <b>InsurancePlan.name</b><br> 5013 * </p> 5014 */ 5015 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam( 5016 SP_PHONETIC); 5017 5018 /** 5019 * Search parameter: <b>name</b> 5020 * <p> 5021 * Description: <b>A portion of the organization's name or alias</b><br> 5022 * Type: <b>string</b><br> 5023 * Path: <b>InsurancePlan.name, InsurancePlan.alias</b><br> 5024 * </p> 5025 */ 5026 @SearchParamDefinition(name = "name", path = "name | alias", description = "A portion of the organization's name or alias", type = "string") 5027 public static final String SP_NAME = "name"; 5028 /** 5029 * <b>Fluent Client</b> search parameter constant for <b>name</b> 5030 * <p> 5031 * Description: <b>A portion of the organization's name or alias</b><br> 5032 * Type: <b>string</b><br> 5033 * Path: <b>InsurancePlan.name, InsurancePlan.alias</b><br> 5034 * </p> 5035 */ 5036 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 5037 SP_NAME); 5038 5039 /** 5040 * Search parameter: <b>address-use</b> 5041 * <p> 5042 * Description: <b>A use code specified in an address</b><br> 5043 * Type: <b>token</b><br> 5044 * Path: <b>InsurancePlan.contact.address.use</b><br> 5045 * </p> 5046 */ 5047 @SearchParamDefinition(name = "address-use", path = "InsurancePlan.contact.address.use", description = "A use code specified in an address", type = "token") 5048 public static final String SP_ADDRESS_USE = "address-use"; 5049 /** 5050 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 5051 * <p> 5052 * Description: <b>A use code specified in an address</b><br> 5053 * Type: <b>token</b><br> 5054 * Path: <b>InsurancePlan.contact.address.use</b><br> 5055 * </p> 5056 */ 5057 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5058 SP_ADDRESS_USE); 5059 5060 /** 5061 * Search parameter: <b>address-city</b> 5062 * <p> 5063 * Description: <b>A city specified in an address</b><br> 5064 * Type: <b>string</b><br> 5065 * Path: <b>InsurancePlan.contact.address.city</b><br> 5066 * </p> 5067 */ 5068 @SearchParamDefinition(name = "address-city", path = "InsurancePlan.contact.address.city", description = "A city specified in an address", type = "string") 5069 public static final String SP_ADDRESS_CITY = "address-city"; 5070 /** 5071 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 5072 * <p> 5073 * Description: <b>A city specified in an address</b><br> 5074 * Type: <b>string</b><br> 5075 * Path: <b>InsurancePlan.contact.address.city</b><br> 5076 * </p> 5077 */ 5078 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 5079 SP_ADDRESS_CITY); 5080 5081 /** 5082 * Search parameter: <b>status</b> 5083 * <p> 5084 * Description: <b>Is the Organization record active</b><br> 5085 * Type: <b>token</b><br> 5086 * Path: <b>InsurancePlan.status</b><br> 5087 * </p> 5088 */ 5089 @SearchParamDefinition(name = "status", path = "InsurancePlan.status", description = "Is the Organization record active", type = "token") 5090 public static final String SP_STATUS = "status"; 5091 /** 5092 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5093 * <p> 5094 * Description: <b>Is the Organization record active</b><br> 5095 * Type: <b>token</b><br> 5096 * Path: <b>InsurancePlan.status</b><br> 5097 * </p> 5098 */ 5099 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5100 SP_STATUS); 5101 5102}