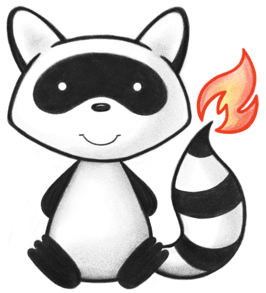
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048 049/** 050 * Invoice containing collected ChargeItems from an Account with calculated 051 * individual and total price for Billing purpose. 052 */ 053@ResourceDef(name = "Invoice", profile = "http://hl7.org/fhir/StructureDefinition/Invoice") 054public class Invoice extends DomainResource { 055 056 public enum InvoiceStatus { 057 /** 058 * the invoice has been prepared but not yet finalized. 059 */ 060 DRAFT, 061 /** 062 * the invoice has been finalized and sent to the recipient. 063 */ 064 ISSUED, 065 /** 066 * the invoice has been balaced / completely paid. 067 */ 068 BALANCED, 069 /** 070 * the invoice was cancelled. 071 */ 072 CANCELLED, 073 /** 074 * the invoice was determined as entered in error before it was issued. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 082 public static InvoiceStatus fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("issued".equals(codeString)) 088 return ISSUED; 089 if ("balanced".equals(codeString)) 090 return BALANCED; 091 if ("cancelled".equals(codeString)) 092 return CANCELLED; 093 if ("entered-in-error".equals(codeString)) 094 return ENTEREDINERROR; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown InvoiceStatus code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case DRAFT: 104 return "draft"; 105 case ISSUED: 106 return "issued"; 107 case BALANCED: 108 return "balanced"; 109 case CANCELLED: 110 return "cancelled"; 111 case ENTEREDINERROR: 112 return "entered-in-error"; 113 case NULL: 114 return null; 115 default: 116 return "?"; 117 } 118 } 119 120 public String getSystem() { 121 switch (this) { 122 case DRAFT: 123 return "http://hl7.org/fhir/invoice-status"; 124 case ISSUED: 125 return "http://hl7.org/fhir/invoice-status"; 126 case BALANCED: 127 return "http://hl7.org/fhir/invoice-status"; 128 case CANCELLED: 129 return "http://hl7.org/fhir/invoice-status"; 130 case ENTEREDINERROR: 131 return "http://hl7.org/fhir/invoice-status"; 132 case NULL: 133 return null; 134 default: 135 return "?"; 136 } 137 } 138 139 public String getDefinition() { 140 switch (this) { 141 case DRAFT: 142 return "the invoice has been prepared but not yet finalized."; 143 case ISSUED: 144 return "the invoice has been finalized and sent to the recipient."; 145 case BALANCED: 146 return "the invoice has been balaced / completely paid."; 147 case CANCELLED: 148 return "the invoice was cancelled."; 149 case ENTEREDINERROR: 150 return "the invoice was determined as entered in error before it was issued."; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDisplay() { 159 switch (this) { 160 case DRAFT: 161 return "draft"; 162 case ISSUED: 163 return "issued"; 164 case BALANCED: 165 return "balanced"; 166 case CANCELLED: 167 return "cancelled"; 168 case ENTEREDINERROR: 169 return "entered in error"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 } 177 178 public static class InvoiceStatusEnumFactory implements EnumFactory<InvoiceStatus> { 179 public InvoiceStatus fromCode(String codeString) throws IllegalArgumentException { 180 if (codeString == null || "".equals(codeString)) 181 if (codeString == null || "".equals(codeString)) 182 return null; 183 if ("draft".equals(codeString)) 184 return InvoiceStatus.DRAFT; 185 if ("issued".equals(codeString)) 186 return InvoiceStatus.ISSUED; 187 if ("balanced".equals(codeString)) 188 return InvoiceStatus.BALANCED; 189 if ("cancelled".equals(codeString)) 190 return InvoiceStatus.CANCELLED; 191 if ("entered-in-error".equals(codeString)) 192 return InvoiceStatus.ENTEREDINERROR; 193 throw new IllegalArgumentException("Unknown InvoiceStatus code '" + codeString + "'"); 194 } 195 196 public Enumeration<InvoiceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 197 if (code == null) 198 return null; 199 if (code.isEmpty()) 200 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 201 String codeString = code.asStringValue(); 202 if (codeString == null || "".equals(codeString)) 203 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 204 if ("draft".equals(codeString)) 205 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.DRAFT, code); 206 if ("issued".equals(codeString)) 207 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ISSUED, code); 208 if ("balanced".equals(codeString)) 209 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.BALANCED, code); 210 if ("cancelled".equals(codeString)) 211 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.CANCELLED, code); 212 if ("entered-in-error".equals(codeString)) 213 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ENTEREDINERROR, code); 214 throw new FHIRException("Unknown InvoiceStatus code '" + codeString + "'"); 215 } 216 217 public String toCode(InvoiceStatus code) { 218 if (code == InvoiceStatus.NULL) 219 return null; 220 if (code == InvoiceStatus.DRAFT) 221 return "draft"; 222 if (code == InvoiceStatus.ISSUED) 223 return "issued"; 224 if (code == InvoiceStatus.BALANCED) 225 return "balanced"; 226 if (code == InvoiceStatus.CANCELLED) 227 return "cancelled"; 228 if (code == InvoiceStatus.ENTEREDINERROR) 229 return "entered-in-error"; 230 return "?"; 231 } 232 233 public String toSystem(InvoiceStatus code) { 234 return code.getSystem(); 235 } 236 } 237 238 public enum InvoicePriceComponentType { 239 /** 240 * the amount is the base price used for calculating the total price before 241 * applying surcharges, discount or taxes. 242 */ 243 BASE, 244 /** 245 * the amount is a surcharge applied on the base price. 246 */ 247 SURCHARGE, 248 /** 249 * the amount is a deduction applied on the base price. 250 */ 251 DEDUCTION, 252 /** 253 * the amount is a discount applied on the base price. 254 */ 255 DISCOUNT, 256 /** 257 * the amount is the tax component of the total price. 258 */ 259 TAX, 260 /** 261 * the amount is of informational character, it has not been applied in the 262 * calculation of the total price. 263 */ 264 INFORMATIONAL, 265 /** 266 * added to help the parsers with the generic types 267 */ 268 NULL; 269 270 public static InvoicePriceComponentType fromCode(String codeString) throws FHIRException { 271 if (codeString == null || "".equals(codeString)) 272 return null; 273 if ("base".equals(codeString)) 274 return BASE; 275 if ("surcharge".equals(codeString)) 276 return SURCHARGE; 277 if ("deduction".equals(codeString)) 278 return DEDUCTION; 279 if ("discount".equals(codeString)) 280 return DISCOUNT; 281 if ("tax".equals(codeString)) 282 return TAX; 283 if ("informational".equals(codeString)) 284 return INFORMATIONAL; 285 if (Configuration.isAcceptInvalidEnums()) 286 return null; 287 else 288 throw new FHIRException("Unknown InvoicePriceComponentType code '" + codeString + "'"); 289 } 290 291 public String toCode() { 292 switch (this) { 293 case BASE: 294 return "base"; 295 case SURCHARGE: 296 return "surcharge"; 297 case DEDUCTION: 298 return "deduction"; 299 case DISCOUNT: 300 return "discount"; 301 case TAX: 302 return "tax"; 303 case INFORMATIONAL: 304 return "informational"; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 312 public String getSystem() { 313 switch (this) { 314 case BASE: 315 return "http://hl7.org/fhir/invoice-priceComponentType"; 316 case SURCHARGE: 317 return "http://hl7.org/fhir/invoice-priceComponentType"; 318 case DEDUCTION: 319 return "http://hl7.org/fhir/invoice-priceComponentType"; 320 case DISCOUNT: 321 return "http://hl7.org/fhir/invoice-priceComponentType"; 322 case TAX: 323 return "http://hl7.org/fhir/invoice-priceComponentType"; 324 case INFORMATIONAL: 325 return "http://hl7.org/fhir/invoice-priceComponentType"; 326 case NULL: 327 return null; 328 default: 329 return "?"; 330 } 331 } 332 333 public String getDefinition() { 334 switch (this) { 335 case BASE: 336 return "the amount is the base price used for calculating the total price before applying surcharges, discount or taxes."; 337 case SURCHARGE: 338 return "the amount is a surcharge applied on the base price."; 339 case DEDUCTION: 340 return "the amount is a deduction applied on the base price."; 341 case DISCOUNT: 342 return "the amount is a discount applied on the base price."; 343 case TAX: 344 return "the amount is the tax component of the total price."; 345 case INFORMATIONAL: 346 return "the amount is of informational character, it has not been applied in the calculation of the total price."; 347 case NULL: 348 return null; 349 default: 350 return "?"; 351 } 352 } 353 354 public String getDisplay() { 355 switch (this) { 356 case BASE: 357 return "base price"; 358 case SURCHARGE: 359 return "surcharge"; 360 case DEDUCTION: 361 return "deduction"; 362 case DISCOUNT: 363 return "discount"; 364 case TAX: 365 return "tax"; 366 case INFORMATIONAL: 367 return "informational"; 368 case NULL: 369 return null; 370 default: 371 return "?"; 372 } 373 } 374 } 375 376 public static class InvoicePriceComponentTypeEnumFactory implements EnumFactory<InvoicePriceComponentType> { 377 public InvoicePriceComponentType fromCode(String codeString) throws IllegalArgumentException { 378 if (codeString == null || "".equals(codeString)) 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("base".equals(codeString)) 382 return InvoicePriceComponentType.BASE; 383 if ("surcharge".equals(codeString)) 384 return InvoicePriceComponentType.SURCHARGE; 385 if ("deduction".equals(codeString)) 386 return InvoicePriceComponentType.DEDUCTION; 387 if ("discount".equals(codeString)) 388 return InvoicePriceComponentType.DISCOUNT; 389 if ("tax".equals(codeString)) 390 return InvoicePriceComponentType.TAX; 391 if ("informational".equals(codeString)) 392 return InvoicePriceComponentType.INFORMATIONAL; 393 throw new IllegalArgumentException("Unknown InvoicePriceComponentType code '" + codeString + "'"); 394 } 395 396 public Enumeration<InvoicePriceComponentType> fromType(PrimitiveType<?> code) throws FHIRException { 397 if (code == null) 398 return null; 399 if (code.isEmpty()) 400 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.NULL, code); 401 String codeString = code.asStringValue(); 402 if (codeString == null || "".equals(codeString)) 403 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.NULL, code); 404 if ("base".equals(codeString)) 405 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.BASE, code); 406 if ("surcharge".equals(codeString)) 407 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.SURCHARGE, code); 408 if ("deduction".equals(codeString)) 409 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.DEDUCTION, code); 410 if ("discount".equals(codeString)) 411 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.DISCOUNT, code); 412 if ("tax".equals(codeString)) 413 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.TAX, code); 414 if ("informational".equals(codeString)) 415 return new Enumeration<InvoicePriceComponentType>(this, InvoicePriceComponentType.INFORMATIONAL, code); 416 throw new FHIRException("Unknown InvoicePriceComponentType code '" + codeString + "'"); 417 } 418 419 public String toCode(InvoicePriceComponentType code) { 420 if (code == InvoicePriceComponentType.NULL) 421 return null; 422 if (code == InvoicePriceComponentType.BASE) 423 return "base"; 424 if (code == InvoicePriceComponentType.SURCHARGE) 425 return "surcharge"; 426 if (code == InvoicePriceComponentType.DEDUCTION) 427 return "deduction"; 428 if (code == InvoicePriceComponentType.DISCOUNT) 429 return "discount"; 430 if (code == InvoicePriceComponentType.TAX) 431 return "tax"; 432 if (code == InvoicePriceComponentType.INFORMATIONAL) 433 return "informational"; 434 return "?"; 435 } 436 437 public String toSystem(InvoicePriceComponentType code) { 438 return code.getSystem(); 439 } 440 } 441 442 @Block() 443 public static class InvoiceParticipantComponent extends BackboneElement implements IBaseBackboneElement { 444 /** 445 * Describes the type of involvement (e.g. transcriptionist, creator etc.). If 446 * the invoice has been created automatically, the Participant may be a billing 447 * engine or another kind of device. 448 */ 449 @Child(name = "role", type = { 450 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 451 @Description(shortDefinition = "Type of involvement in creation of this Invoice", formalDefinition = "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.") 452 protected CodeableConcept role; 453 454 /** 455 * The device, practitioner, etc. who performed or participated in the service. 456 */ 457 @Child(name = "actor", type = { Practitioner.class, Organization.class, Patient.class, PractitionerRole.class, 458 Device.class, RelatedPerson.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 459 @Description(shortDefinition = "Individual who was involved", formalDefinition = "The device, practitioner, etc. who performed or participated in the service.") 460 protected Reference actor; 461 462 /** 463 * The actual object that is the target of the reference (The device, 464 * practitioner, etc. who performed or participated in the service.) 465 */ 466 protected Resource actorTarget; 467 468 private static final long serialVersionUID = 805521719L; 469 470 /** 471 * Constructor 472 */ 473 public InvoiceParticipantComponent() { 474 super(); 475 } 476 477 /** 478 * Constructor 479 */ 480 public InvoiceParticipantComponent(Reference actor) { 481 super(); 482 this.actor = actor; 483 } 484 485 /** 486 * @return {@link #role} (Describes the type of involvement (e.g. 487 * transcriptionist, creator etc.). If the invoice has been created 488 * automatically, the Participant may be a billing engine or another 489 * kind of device.) 490 */ 491 public CodeableConcept getRole() { 492 if (this.role == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create InvoiceParticipantComponent.role"); 495 else if (Configuration.doAutoCreate()) 496 this.role = new CodeableConcept(); // cc 497 return this.role; 498 } 499 500 public boolean hasRole() { 501 return this.role != null && !this.role.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #role} (Describes the type of involvement (e.g. 506 * transcriptionist, creator etc.). If the invoice has been created 507 * automatically, the Participant may be a billing engine or 508 * another kind of device.) 509 */ 510 public InvoiceParticipantComponent setRole(CodeableConcept value) { 511 this.role = value; 512 return this; 513 } 514 515 /** 516 * @return {@link #actor} (The device, practitioner, etc. who performed or 517 * participated in the service.) 518 */ 519 public Reference getActor() { 520 if (this.actor == null) 521 if (Configuration.errorOnAutoCreate()) 522 throw new Error("Attempt to auto-create InvoiceParticipantComponent.actor"); 523 else if (Configuration.doAutoCreate()) 524 this.actor = new Reference(); // cc 525 return this.actor; 526 } 527 528 public boolean hasActor() { 529 return this.actor != null && !this.actor.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #actor} (The device, practitioner, etc. who performed or 534 * participated in the service.) 535 */ 536 public InvoiceParticipantComponent setActor(Reference value) { 537 this.actor = value; 538 return this; 539 } 540 541 /** 542 * @return {@link #actor} The actual object that is the target of the reference. 543 * The reference library doesn't populate this, but you can use it to 544 * hold the resource if you resolve it. (The device, practitioner, etc. 545 * who performed or participated in the service.) 546 */ 547 public Resource getActorTarget() { 548 return this.actorTarget; 549 } 550 551 /** 552 * @param value {@link #actor} The actual object that is the target of the 553 * reference. The reference library doesn't use these, but you can 554 * use it to hold the resource if you resolve it. (The device, 555 * practitioner, etc. who performed or participated in the 556 * service.) 557 */ 558 public InvoiceParticipantComponent setActorTarget(Resource value) { 559 this.actorTarget = value; 560 return this; 561 } 562 563 protected void listChildren(List<Property> children) { 564 super.listChildren(children); 565 children.add(new Property("role", "CodeableConcept", 566 "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 567 0, 1, role)); 568 children.add( 569 new Property("actor", "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", 570 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 571 } 572 573 @Override 574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 575 switch (_hash) { 576 case 3506294: 577 /* role */ return new Property("role", "CodeableConcept", 578 "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 579 0, 1, role); 580 case 92645877: 581 /* actor */ return new Property("actor", 582 "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", 583 "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 584 default: 585 return super.getNamedProperty(_hash, _name, _checkValid); 586 } 587 588 } 589 590 @Override 591 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 592 switch (hash) { 593 case 3506294: 594 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 595 case 92645877: 596 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 597 default: 598 return super.getProperty(hash, name, checkValid); 599 } 600 601 } 602 603 @Override 604 public Base setProperty(int hash, String name, Base value) throws FHIRException { 605 switch (hash) { 606 case 3506294: // role 607 this.role = castToCodeableConcept(value); // CodeableConcept 608 return value; 609 case 92645877: // actor 610 this.actor = castToReference(value); // Reference 611 return value; 612 default: 613 return super.setProperty(hash, name, value); 614 } 615 616 } 617 618 @Override 619 public Base setProperty(String name, Base value) throws FHIRException { 620 if (name.equals("role")) { 621 this.role = castToCodeableConcept(value); // CodeableConcept 622 } else if (name.equals("actor")) { 623 this.actor = castToReference(value); // Reference 624 } else 625 return super.setProperty(name, value); 626 return value; 627 } 628 629 @Override 630 public void removeChild(String name, Base value) throws FHIRException { 631 if (name.equals("role")) { 632 this.role = null; 633 } else if (name.equals("actor")) { 634 this.actor = null; 635 } else 636 super.removeChild(name, value); 637 638 } 639 640 @Override 641 public Base makeProperty(int hash, String name) throws FHIRException { 642 switch (hash) { 643 case 3506294: 644 return getRole(); 645 case 92645877: 646 return getActor(); 647 default: 648 return super.makeProperty(hash, name); 649 } 650 651 } 652 653 @Override 654 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 655 switch (hash) { 656 case 3506294: 657 /* role */ return new String[] { "CodeableConcept" }; 658 case 92645877: 659 /* actor */ return new String[] { "Reference" }; 660 default: 661 return super.getTypesForProperty(hash, name); 662 } 663 664 } 665 666 @Override 667 public Base addChild(String name) throws FHIRException { 668 if (name.equals("role")) { 669 this.role = new CodeableConcept(); 670 return this.role; 671 } else if (name.equals("actor")) { 672 this.actor = new Reference(); 673 return this.actor; 674 } else 675 return super.addChild(name); 676 } 677 678 public InvoiceParticipantComponent copy() { 679 InvoiceParticipantComponent dst = new InvoiceParticipantComponent(); 680 copyValues(dst); 681 return dst; 682 } 683 684 public void copyValues(InvoiceParticipantComponent dst) { 685 super.copyValues(dst); 686 dst.role = role == null ? null : role.copy(); 687 dst.actor = actor == null ? null : actor.copy(); 688 } 689 690 @Override 691 public boolean equalsDeep(Base other_) { 692 if (!super.equalsDeep(other_)) 693 return false; 694 if (!(other_ instanceof InvoiceParticipantComponent)) 695 return false; 696 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 697 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 698 } 699 700 @Override 701 public boolean equalsShallow(Base other_) { 702 if (!super.equalsShallow(other_)) 703 return false; 704 if (!(other_ instanceof InvoiceParticipantComponent)) 705 return false; 706 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 707 return true; 708 } 709 710 public boolean isEmpty() { 711 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 712 } 713 714 public String fhirType() { 715 return "Invoice.participant"; 716 717 } 718 719 } 720 721 @Block() 722 public static class InvoiceLineItemComponent extends BackboneElement implements IBaseBackboneElement { 723 /** 724 * Sequence in which the items appear on the invoice. 725 */ 726 @Child(name = "sequence", type = { 727 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 728 @Description(shortDefinition = "Sequence number of line item", formalDefinition = "Sequence in which the items appear on the invoice.") 729 protected PositiveIntType sequence; 730 731 /** 732 * The ChargeItem contains information such as the billing code, date, amount 733 * etc. If no further details are required for the lineItem, inline billing 734 * codes can be added using the CodeableConcept data type instead of the 735 * Reference. 736 */ 737 @Child(name = "chargeItem", type = { ChargeItem.class, 738 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 739 @Description(shortDefinition = "Reference to ChargeItem containing details of this line item or an inline billing code", formalDefinition = "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.") 740 protected Type chargeItem; 741 742 /** 743 * The price for a ChargeItem may be calculated as a base price with 744 * surcharges/deductions that apply in certain conditions. A 745 * ChargeItemDefinition resource that defines the prices, factors and conditions 746 * that apply to a billing code is currently under development. The 747 * priceComponent element can be used to offer transparency to the recipient of 748 * the Invoice as to how the prices have been calculated. 749 */ 750 @Child(name = "priceComponent", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 751 @Description(shortDefinition = "Components of total line item price", formalDefinition = "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.") 752 protected List<InvoiceLineItemPriceComponentComponent> priceComponent; 753 754 private static final long serialVersionUID = -1013610189L; 755 756 /** 757 * Constructor 758 */ 759 public InvoiceLineItemComponent() { 760 super(); 761 } 762 763 /** 764 * Constructor 765 */ 766 public InvoiceLineItemComponent(Type chargeItem) { 767 super(); 768 this.chargeItem = chargeItem; 769 } 770 771 /** 772 * @return {@link #sequence} (Sequence in which the items appear on the 773 * invoice.). This is the underlying object with id, value and 774 * extensions. The accessor "getSequence" gives direct access to the 775 * value 776 */ 777 public PositiveIntType getSequenceElement() { 778 if (this.sequence == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create InvoiceLineItemComponent.sequence"); 781 else if (Configuration.doAutoCreate()) 782 this.sequence = new PositiveIntType(); // bb 783 return this.sequence; 784 } 785 786 public boolean hasSequenceElement() { 787 return this.sequence != null && !this.sequence.isEmpty(); 788 } 789 790 public boolean hasSequence() { 791 return this.sequence != null && !this.sequence.isEmpty(); 792 } 793 794 /** 795 * @param value {@link #sequence} (Sequence in which the items appear on the 796 * invoice.). This is the underlying object with id, value and 797 * extensions. The accessor "getSequence" gives direct access to 798 * the value 799 */ 800 public InvoiceLineItemComponent setSequenceElement(PositiveIntType value) { 801 this.sequence = value; 802 return this; 803 } 804 805 /** 806 * @return Sequence in which the items appear on the invoice. 807 */ 808 public int getSequence() { 809 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 810 } 811 812 /** 813 * @param value Sequence in which the items appear on the invoice. 814 */ 815 public InvoiceLineItemComponent setSequence(int value) { 816 if (this.sequence == null) 817 this.sequence = new PositiveIntType(); 818 this.sequence.setValue(value); 819 return this; 820 } 821 822 /** 823 * @return {@link #chargeItem} (The ChargeItem contains information such as the 824 * billing code, date, amount etc. If no further details are required 825 * for the lineItem, inline billing codes can be added using the 826 * CodeableConcept data type instead of the Reference.) 827 */ 828 public Type getChargeItem() { 829 return this.chargeItem; 830 } 831 832 /** 833 * @return {@link #chargeItem} (The ChargeItem contains information such as the 834 * billing code, date, amount etc. If no further details are required 835 * for the lineItem, inline billing codes can be added using the 836 * CodeableConcept data type instead of the Reference.) 837 */ 838 public Reference getChargeItemReference() throws FHIRException { 839 if (this.chargeItem == null) 840 this.chargeItem = new Reference(); 841 if (!(this.chargeItem instanceof Reference)) 842 throw new FHIRException("Type mismatch: the type Reference was expected, but " 843 + this.chargeItem.getClass().getName() + " was encountered"); 844 return (Reference) this.chargeItem; 845 } 846 847 public boolean hasChargeItemReference() { 848 return this != null && this.chargeItem instanceof Reference; 849 } 850 851 /** 852 * @return {@link #chargeItem} (The ChargeItem contains information such as the 853 * billing code, date, amount etc. If no further details are required 854 * for the lineItem, inline billing codes can be added using the 855 * CodeableConcept data type instead of the Reference.) 856 */ 857 public CodeableConcept getChargeItemCodeableConcept() throws FHIRException { 858 if (this.chargeItem == null) 859 this.chargeItem = new CodeableConcept(); 860 if (!(this.chargeItem instanceof CodeableConcept)) 861 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 862 + this.chargeItem.getClass().getName() + " was encountered"); 863 return (CodeableConcept) this.chargeItem; 864 } 865 866 public boolean hasChargeItemCodeableConcept() { 867 return this != null && this.chargeItem instanceof CodeableConcept; 868 } 869 870 public boolean hasChargeItem() { 871 return this.chargeItem != null && !this.chargeItem.isEmpty(); 872 } 873 874 /** 875 * @param value {@link #chargeItem} (The ChargeItem contains information such as 876 * the billing code, date, amount etc. If no further details are 877 * required for the lineItem, inline billing codes can be added 878 * using the CodeableConcept data type instead of the Reference.) 879 */ 880 public InvoiceLineItemComponent setChargeItem(Type value) { 881 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 882 throw new Error("Not the right type for Invoice.lineItem.chargeItem[x]: " + value.fhirType()); 883 this.chargeItem = value; 884 return this; 885 } 886 887 /** 888 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated 889 * as a base price with surcharges/deductions that apply in certain 890 * conditions. A ChargeItemDefinition resource that defines the prices, 891 * factors and conditions that apply to a billing code is currently 892 * under development. The priceComponent element can be used to offer 893 * transparency to the recipient of the Invoice as to how the prices 894 * have been calculated.) 895 */ 896 public List<InvoiceLineItemPriceComponentComponent> getPriceComponent() { 897 if (this.priceComponent == null) 898 this.priceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 899 return this.priceComponent; 900 } 901 902 /** 903 * @return Returns a reference to <code>this</code> for easy method chaining 904 */ 905 public InvoiceLineItemComponent setPriceComponent(List<InvoiceLineItemPriceComponentComponent> thePriceComponent) { 906 this.priceComponent = thePriceComponent; 907 return this; 908 } 909 910 public boolean hasPriceComponent() { 911 if (this.priceComponent == null) 912 return false; 913 for (InvoiceLineItemPriceComponentComponent item : this.priceComponent) 914 if (!item.isEmpty()) 915 return true; 916 return false; 917 } 918 919 public InvoiceLineItemPriceComponentComponent addPriceComponent() { // 3 920 InvoiceLineItemPriceComponentComponent t = new InvoiceLineItemPriceComponentComponent(); 921 if (this.priceComponent == null) 922 this.priceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 923 this.priceComponent.add(t); 924 return t; 925 } 926 927 public InvoiceLineItemComponent addPriceComponent(InvoiceLineItemPriceComponentComponent t) { // 3 928 if (t == null) 929 return this; 930 if (this.priceComponent == null) 931 this.priceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 932 this.priceComponent.add(t); 933 return this; 934 } 935 936 /** 937 * @return The first repetition of repeating field {@link #priceComponent}, 938 * creating it if it does not already exist 939 */ 940 public InvoiceLineItemPriceComponentComponent getPriceComponentFirstRep() { 941 if (getPriceComponent().isEmpty()) { 942 addPriceComponent(); 943 } 944 return getPriceComponent().get(0); 945 } 946 947 protected void listChildren(List<Property> children) { 948 super.listChildren(children); 949 children.add(new Property("sequence", "positiveInt", "Sequence in which the items appear on the invoice.", 0, 1, 950 sequence)); 951 children.add(new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", 952 "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 953 0, 1, chargeItem)); 954 children.add(new Property("priceComponent", "", 955 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 956 0, java.lang.Integer.MAX_VALUE, priceComponent)); 957 } 958 959 @Override 960 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 961 switch (_hash) { 962 case 1349547969: 963 /* sequence */ return new Property("sequence", "positiveInt", 964 "Sequence in which the items appear on the invoice.", 0, 1, sequence); 965 case 351104825: 966 /* chargeItem[x] */ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", 967 "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 968 0, 1, chargeItem); 969 case 1417779175: 970 /* chargeItem */ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", 971 "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 972 0, 1, chargeItem); 973 case 753580836: 974 /* chargeItemReference */ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", 975 "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 976 0, 1, chargeItem); 977 case 1226532026: 978 /* chargeItemCodeableConcept */ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", 979 "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 980 0, 1, chargeItem); 981 case 1219095988: 982 /* priceComponent */ return new Property("priceComponent", "", 983 "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 984 0, java.lang.Integer.MAX_VALUE, priceComponent); 985 default: 986 return super.getNamedProperty(_hash, _name, _checkValid); 987 } 988 989 } 990 991 @Override 992 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 993 switch (hash) { 994 case 1349547969: 995 /* sequence */ return this.sequence == null ? new Base[0] : new Base[] { this.sequence }; // PositiveIntType 996 case 1417779175: 997 /* chargeItem */ return this.chargeItem == null ? new Base[0] : new Base[] { this.chargeItem }; // Type 998 case 1219095988: 999 /* priceComponent */ return this.priceComponent == null ? new Base[0] 1000 : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // InvoiceLineItemPriceComponentComponent 1001 default: 1002 return super.getProperty(hash, name, checkValid); 1003 } 1004 1005 } 1006 1007 @Override 1008 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1009 switch (hash) { 1010 case 1349547969: // sequence 1011 this.sequence = castToPositiveInt(value); // PositiveIntType 1012 return value; 1013 case 1417779175: // chargeItem 1014 this.chargeItem = castToType(value); // Type 1015 return value; 1016 case 1219095988: // priceComponent 1017 this.getPriceComponent().add((InvoiceLineItemPriceComponentComponent) value); // InvoiceLineItemPriceComponentComponent 1018 return value; 1019 default: 1020 return super.setProperty(hash, name, value); 1021 } 1022 1023 } 1024 1025 @Override 1026 public Base setProperty(String name, Base value) throws FHIRException { 1027 if (name.equals("sequence")) { 1028 this.sequence = castToPositiveInt(value); // PositiveIntType 1029 } else if (name.equals("chargeItem[x]")) { 1030 this.chargeItem = castToType(value); // Type 1031 } else if (name.equals("priceComponent")) { 1032 this.getPriceComponent().add((InvoiceLineItemPriceComponentComponent) value); 1033 } else 1034 return super.setProperty(name, value); 1035 return value; 1036 } 1037 1038 @Override 1039 public void removeChild(String name, Base value) throws FHIRException { 1040 if (name.equals("sequence")) { 1041 this.sequence = null; 1042 } else if (name.equals("chargeItem[x]")) { 1043 this.chargeItem = null; 1044 } else if (name.equals("priceComponent")) { 1045 this.getPriceComponent().remove((InvoiceLineItemPriceComponentComponent) value); 1046 } else 1047 super.removeChild(name, value); 1048 1049 } 1050 1051 @Override 1052 public Base makeProperty(int hash, String name) throws FHIRException { 1053 switch (hash) { 1054 case 1349547969: 1055 return getSequenceElement(); 1056 case 351104825: 1057 return getChargeItem(); 1058 case 1417779175: 1059 return getChargeItem(); 1060 case 1219095988: 1061 return addPriceComponent(); 1062 default: 1063 return super.makeProperty(hash, name); 1064 } 1065 1066 } 1067 1068 @Override 1069 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1070 switch (hash) { 1071 case 1349547969: 1072 /* sequence */ return new String[] { "positiveInt" }; 1073 case 1417779175: 1074 /* chargeItem */ return new String[] { "Reference", "CodeableConcept" }; 1075 case 1219095988: 1076 /* priceComponent */ return new String[] {}; 1077 default: 1078 return super.getTypesForProperty(hash, name); 1079 } 1080 1081 } 1082 1083 @Override 1084 public Base addChild(String name) throws FHIRException { 1085 if (name.equals("sequence")) { 1086 throw new FHIRException("Cannot call addChild on a singleton property Invoice.sequence"); 1087 } else if (name.equals("chargeItemReference")) { 1088 this.chargeItem = new Reference(); 1089 return this.chargeItem; 1090 } else if (name.equals("chargeItemCodeableConcept")) { 1091 this.chargeItem = new CodeableConcept(); 1092 return this.chargeItem; 1093 } else if (name.equals("priceComponent")) { 1094 return addPriceComponent(); 1095 } else 1096 return super.addChild(name); 1097 } 1098 1099 public InvoiceLineItemComponent copy() { 1100 InvoiceLineItemComponent dst = new InvoiceLineItemComponent(); 1101 copyValues(dst); 1102 return dst; 1103 } 1104 1105 public void copyValues(InvoiceLineItemComponent dst) { 1106 super.copyValues(dst); 1107 dst.sequence = sequence == null ? null : sequence.copy(); 1108 dst.chargeItem = chargeItem == null ? null : chargeItem.copy(); 1109 if (priceComponent != null) { 1110 dst.priceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 1111 for (InvoiceLineItemPriceComponentComponent i : priceComponent) 1112 dst.priceComponent.add(i.copy()); 1113 } 1114 ; 1115 } 1116 1117 @Override 1118 public boolean equalsDeep(Base other_) { 1119 if (!super.equalsDeep(other_)) 1120 return false; 1121 if (!(other_ instanceof InvoiceLineItemComponent)) 1122 return false; 1123 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 1124 return compareDeep(sequence, o.sequence, true) && compareDeep(chargeItem, o.chargeItem, true) 1125 && compareDeep(priceComponent, o.priceComponent, true); 1126 } 1127 1128 @Override 1129 public boolean equalsShallow(Base other_) { 1130 if (!super.equalsShallow(other_)) 1131 return false; 1132 if (!(other_ instanceof InvoiceLineItemComponent)) 1133 return false; 1134 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 1135 return compareValues(sequence, o.sequence, true); 1136 } 1137 1138 public boolean isEmpty() { 1139 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, chargeItem, priceComponent); 1140 } 1141 1142 public String fhirType() { 1143 return "Invoice.lineItem"; 1144 1145 } 1146 1147 } 1148 1149 @Block() 1150 public static class InvoiceLineItemPriceComponentComponent extends BackboneElement implements IBaseBackboneElement { 1151 /** 1152 * This code identifies the type of the component. 1153 */ 1154 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1155 @Description(shortDefinition = "base | surcharge | deduction | discount | tax | informational", formalDefinition = "This code identifies the type of the component.") 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/invoice-priceComponentType") 1157 protected Enumeration<InvoicePriceComponentType> type; 1158 1159 /** 1160 * A code that identifies the component. Codes may be used to differentiate 1161 * between kinds of taxes, surcharges, discounts etc. 1162 */ 1163 @Child(name = "code", type = { 1164 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1165 @Description(shortDefinition = "Code identifying the specific component", formalDefinition = "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.") 1166 protected CodeableConcept code; 1167 1168 /** 1169 * The factor that has been applied on the base price for calculating this 1170 * component. 1171 */ 1172 @Child(name = "factor", type = { 1173 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1174 @Description(shortDefinition = "Factor used for calculating this component", formalDefinition = "The factor that has been applied on the base price for calculating this component.") 1175 protected DecimalType factor; 1176 1177 /** 1178 * The amount calculated for this component. 1179 */ 1180 @Child(name = "amount", type = { Money.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1181 @Description(shortDefinition = "Monetary amount associated with this component", formalDefinition = "The amount calculated for this component.") 1182 protected Money amount; 1183 1184 private static final long serialVersionUID = 1223988958L; 1185 1186 /** 1187 * Constructor 1188 */ 1189 public InvoiceLineItemPriceComponentComponent() { 1190 super(); 1191 } 1192 1193 /** 1194 * Constructor 1195 */ 1196 public InvoiceLineItemPriceComponentComponent(Enumeration<InvoicePriceComponentType> type) { 1197 super(); 1198 this.type = type; 1199 } 1200 1201 /** 1202 * @return {@link #type} (This code identifies the type of the component.). This 1203 * is the underlying object with id, value and extensions. The accessor 1204 * "getType" gives direct access to the value 1205 */ 1206 public Enumeration<InvoicePriceComponentType> getTypeElement() { 1207 if (this.type == null) 1208 if (Configuration.errorOnAutoCreate()) 1209 throw new Error("Attempt to auto-create InvoiceLineItemPriceComponentComponent.type"); 1210 else if (Configuration.doAutoCreate()) 1211 this.type = new Enumeration<InvoicePriceComponentType>(new InvoicePriceComponentTypeEnumFactory()); // bb 1212 return this.type; 1213 } 1214 1215 public boolean hasTypeElement() { 1216 return this.type != null && !this.type.isEmpty(); 1217 } 1218 1219 public boolean hasType() { 1220 return this.type != null && !this.type.isEmpty(); 1221 } 1222 1223 /** 1224 * @param value {@link #type} (This code identifies the type of the component.). 1225 * This is the underlying object with id, value and extensions. The 1226 * accessor "getType" gives direct access to the value 1227 */ 1228 public InvoiceLineItemPriceComponentComponent setTypeElement(Enumeration<InvoicePriceComponentType> value) { 1229 this.type = value; 1230 return this; 1231 } 1232 1233 /** 1234 * @return This code identifies the type of the component. 1235 */ 1236 public InvoicePriceComponentType getType() { 1237 return this.type == null ? null : this.type.getValue(); 1238 } 1239 1240 /** 1241 * @param value This code identifies the type of the component. 1242 */ 1243 public InvoiceLineItemPriceComponentComponent setType(InvoicePriceComponentType value) { 1244 if (this.type == null) 1245 this.type = new Enumeration<InvoicePriceComponentType>(new InvoicePriceComponentTypeEnumFactory()); 1246 this.type.setValue(value); 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #code} (A code that identifies the component. Codes may be 1252 * used to differentiate between kinds of taxes, surcharges, discounts 1253 * etc.) 1254 */ 1255 public CodeableConcept getCode() { 1256 if (this.code == null) 1257 if (Configuration.errorOnAutoCreate()) 1258 throw new Error("Attempt to auto-create InvoiceLineItemPriceComponentComponent.code"); 1259 else if (Configuration.doAutoCreate()) 1260 this.code = new CodeableConcept(); // cc 1261 return this.code; 1262 } 1263 1264 public boolean hasCode() { 1265 return this.code != null && !this.code.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #code} (A code that identifies the component. Codes may 1270 * be used to differentiate between kinds of taxes, surcharges, 1271 * discounts etc.) 1272 */ 1273 public InvoiceLineItemPriceComponentComponent setCode(CodeableConcept value) { 1274 this.code = value; 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #factor} (The factor that has been applied on the base price 1280 * for calculating this component.). This is the underlying object with 1281 * id, value and extensions. The accessor "getFactor" gives direct 1282 * access to the value 1283 */ 1284 public DecimalType getFactorElement() { 1285 if (this.factor == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create InvoiceLineItemPriceComponentComponent.factor"); 1288 else if (Configuration.doAutoCreate()) 1289 this.factor = new DecimalType(); // bb 1290 return this.factor; 1291 } 1292 1293 public boolean hasFactorElement() { 1294 return this.factor != null && !this.factor.isEmpty(); 1295 } 1296 1297 public boolean hasFactor() { 1298 return this.factor != null && !this.factor.isEmpty(); 1299 } 1300 1301 /** 1302 * @param value {@link #factor} (The factor that has been applied on the base 1303 * price for calculating this component.). This is the underlying 1304 * object with id, value and extensions. The accessor "getFactor" 1305 * gives direct access to the value 1306 */ 1307 public InvoiceLineItemPriceComponentComponent setFactorElement(DecimalType value) { 1308 this.factor = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return The factor that has been applied on the base price for calculating 1314 * this component. 1315 */ 1316 public BigDecimal getFactor() { 1317 return this.factor == null ? null : this.factor.getValue(); 1318 } 1319 1320 /** 1321 * @param value The factor that has been applied on the base price for 1322 * calculating this component. 1323 */ 1324 public InvoiceLineItemPriceComponentComponent setFactor(BigDecimal value) { 1325 if (value == null) 1326 this.factor = null; 1327 else { 1328 if (this.factor == null) 1329 this.factor = new DecimalType(); 1330 this.factor.setValue(value); 1331 } 1332 return this; 1333 } 1334 1335 /** 1336 * @param value The factor that has been applied on the base price for 1337 * calculating this component. 1338 */ 1339 public InvoiceLineItemPriceComponentComponent setFactor(long value) { 1340 this.factor = new DecimalType(); 1341 this.factor.setValue(value); 1342 return this; 1343 } 1344 1345 /** 1346 * @param value The factor that has been applied on the base price for 1347 * calculating this component. 1348 */ 1349 public InvoiceLineItemPriceComponentComponent setFactor(double value) { 1350 this.factor = new DecimalType(); 1351 this.factor.setValue(value); 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #amount} (The amount calculated for this component.) 1357 */ 1358 public Money getAmount() { 1359 if (this.amount == null) 1360 if (Configuration.errorOnAutoCreate()) 1361 throw new Error("Attempt to auto-create InvoiceLineItemPriceComponentComponent.amount"); 1362 else if (Configuration.doAutoCreate()) 1363 this.amount = new Money(); // cc 1364 return this.amount; 1365 } 1366 1367 public boolean hasAmount() { 1368 return this.amount != null && !this.amount.isEmpty(); 1369 } 1370 1371 /** 1372 * @param value {@link #amount} (The amount calculated for this component.) 1373 */ 1374 public InvoiceLineItemPriceComponentComponent setAmount(Money value) { 1375 this.amount = value; 1376 return this; 1377 } 1378 1379 protected void listChildren(List<Property> children) { 1380 super.listChildren(children); 1381 children.add(new Property("type", "code", "This code identifies the type of the component.", 0, 1, type)); 1382 children.add(new Property("code", "CodeableConcept", 1383 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1384 0, 1, code)); 1385 children.add(new Property("factor", "decimal", 1386 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor)); 1387 children.add(new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount)); 1388 } 1389 1390 @Override 1391 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1392 switch (_hash) { 1393 case 3575610: 1394 /* type */ return new Property("type", "code", "This code identifies the type of the component.", 0, 1, type); 1395 case 3059181: 1396 /* code */ return new Property("code", "CodeableConcept", 1397 "A code that identifies the component. Codes may be used to differentiate between kinds of taxes, surcharges, discounts etc.", 1398 0, 1, code); 1399 case -1282148017: 1400 /* factor */ return new Property("factor", "decimal", 1401 "The factor that has been applied on the base price for calculating this component.", 0, 1, factor); 1402 case -1413853096: 1403 /* amount */ return new Property("amount", "Money", "The amount calculated for this component.", 0, 1, amount); 1404 default: 1405 return super.getNamedProperty(_hash, _name, _checkValid); 1406 } 1407 1408 } 1409 1410 @Override 1411 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1412 switch (hash) { 1413 case 3575610: 1414 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<InvoicePriceComponentType> 1415 case 3059181: 1416 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1417 case -1282148017: 1418 /* factor */ return this.factor == null ? new Base[0] : new Base[] { this.factor }; // DecimalType 1419 case -1413853096: 1420 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Money 1421 default: 1422 return super.getProperty(hash, name, checkValid); 1423 } 1424 1425 } 1426 1427 @Override 1428 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1429 switch (hash) { 1430 case 3575610: // type 1431 value = new InvoicePriceComponentTypeEnumFactory().fromType(castToCode(value)); 1432 this.type = (Enumeration) value; // Enumeration<InvoicePriceComponentType> 1433 return value; 1434 case 3059181: // code 1435 this.code = castToCodeableConcept(value); // CodeableConcept 1436 return value; 1437 case -1282148017: // factor 1438 this.factor = castToDecimal(value); // DecimalType 1439 return value; 1440 case -1413853096: // amount 1441 this.amount = castToMoney(value); // Money 1442 return value; 1443 default: 1444 return super.setProperty(hash, name, value); 1445 } 1446 1447 } 1448 1449 @Override 1450 public Base setProperty(String name, Base value) throws FHIRException { 1451 if (name.equals("type")) { 1452 value = new InvoicePriceComponentTypeEnumFactory().fromType(castToCode(value)); 1453 this.type = (Enumeration) value; // Enumeration<InvoicePriceComponentType> 1454 } else if (name.equals("code")) { 1455 this.code = castToCodeableConcept(value); // CodeableConcept 1456 } else if (name.equals("factor")) { 1457 this.factor = castToDecimal(value); // DecimalType 1458 } else if (name.equals("amount")) { 1459 this.amount = castToMoney(value); // Money 1460 } else 1461 return super.setProperty(name, value); 1462 return value; 1463 } 1464 1465 @Override 1466 public void removeChild(String name, Base value) throws FHIRException { 1467 if (name.equals("type")) { 1468 this.type = null; 1469 } else if (name.equals("code")) { 1470 this.code = null; 1471 } else if (name.equals("factor")) { 1472 this.factor = null; 1473 } else if (name.equals("amount")) { 1474 this.amount = null; 1475 } else 1476 super.removeChild(name, value); 1477 1478 } 1479 1480 @Override 1481 public Base makeProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case 3575610: 1484 return getTypeElement(); 1485 case 3059181: 1486 return getCode(); 1487 case -1282148017: 1488 return getFactorElement(); 1489 case -1413853096: 1490 return getAmount(); 1491 default: 1492 return super.makeProperty(hash, name); 1493 } 1494 1495 } 1496 1497 @Override 1498 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1499 switch (hash) { 1500 case 3575610: 1501 /* type */ return new String[] { "code" }; 1502 case 3059181: 1503 /* code */ return new String[] { "CodeableConcept" }; 1504 case -1282148017: 1505 /* factor */ return new String[] { "decimal" }; 1506 case -1413853096: 1507 /* amount */ return new String[] { "Money" }; 1508 default: 1509 return super.getTypesForProperty(hash, name); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base addChild(String name) throws FHIRException { 1516 if (name.equals("type")) { 1517 throw new FHIRException("Cannot call addChild on a singleton property Invoice.type"); 1518 } else if (name.equals("code")) { 1519 this.code = new CodeableConcept(); 1520 return this.code; 1521 } else if (name.equals("factor")) { 1522 throw new FHIRException("Cannot call addChild on a singleton property Invoice.factor"); 1523 } else if (name.equals("amount")) { 1524 this.amount = new Money(); 1525 return this.amount; 1526 } else 1527 return super.addChild(name); 1528 } 1529 1530 public InvoiceLineItemPriceComponentComponent copy() { 1531 InvoiceLineItemPriceComponentComponent dst = new InvoiceLineItemPriceComponentComponent(); 1532 copyValues(dst); 1533 return dst; 1534 } 1535 1536 public void copyValues(InvoiceLineItemPriceComponentComponent dst) { 1537 super.copyValues(dst); 1538 dst.type = type == null ? null : type.copy(); 1539 dst.code = code == null ? null : code.copy(); 1540 dst.factor = factor == null ? null : factor.copy(); 1541 dst.amount = amount == null ? null : amount.copy(); 1542 } 1543 1544 @Override 1545 public boolean equalsDeep(Base other_) { 1546 if (!super.equalsDeep(other_)) 1547 return false; 1548 if (!(other_ instanceof InvoiceLineItemPriceComponentComponent)) 1549 return false; 1550 InvoiceLineItemPriceComponentComponent o = (InvoiceLineItemPriceComponentComponent) other_; 1551 return compareDeep(type, o.type, true) && compareDeep(code, o.code, true) && compareDeep(factor, o.factor, true) 1552 && compareDeep(amount, o.amount, true); 1553 } 1554 1555 @Override 1556 public boolean equalsShallow(Base other_) { 1557 if (!super.equalsShallow(other_)) 1558 return false; 1559 if (!(other_ instanceof InvoiceLineItemPriceComponentComponent)) 1560 return false; 1561 InvoiceLineItemPriceComponentComponent o = (InvoiceLineItemPriceComponentComponent) other_; 1562 return compareValues(type, o.type, true) && compareValues(factor, o.factor, true); 1563 } 1564 1565 public boolean isEmpty() { 1566 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, code, factor, amount); 1567 } 1568 1569 public String fhirType() { 1570 return "Invoice.lineItem.priceComponent"; 1571 1572 } 1573 1574 } 1575 1576 /** 1577 * Identifier of this Invoice, often used for reference in correspondence about 1578 * this invoice or for tracking of payments. 1579 */ 1580 @Child(name = "identifier", type = { 1581 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1582 @Description(shortDefinition = "Business Identifier for item", formalDefinition = "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.") 1583 protected List<Identifier> identifier; 1584 1585 /** 1586 * The current state of the Invoice. 1587 */ 1588 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1589 @Description(shortDefinition = "draft | issued | balanced | cancelled | entered-in-error", formalDefinition = "The current state of the Invoice.") 1590 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/invoice-status") 1591 protected Enumeration<InvoiceStatus> status; 1592 1593 /** 1594 * In case of Invoice cancellation a reason must be given (entered in error, 1595 * superseded by corrected invoice etc.). 1596 */ 1597 @Child(name = "cancelledReason", type = { 1598 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1599 @Description(shortDefinition = "Reason for cancellation of this Invoice", formalDefinition = "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).") 1600 protected StringType cancelledReason; 1601 1602 /** 1603 * Type of Invoice depending on domain, realm an usage (e.g. internal/external, 1604 * dental, preliminary). 1605 */ 1606 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1607 @Description(shortDefinition = "Type of Invoice", formalDefinition = "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).") 1608 protected CodeableConcept type; 1609 1610 /** 1611 * The individual or set of individuals receiving the goods and services billed 1612 * in this invoice. 1613 */ 1614 @Child(name = "subject", type = { Patient.class, 1615 Group.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1616 @Description(shortDefinition = "Recipient(s) of goods and services", formalDefinition = "The individual or set of individuals receiving the goods and services billed in this invoice.") 1617 protected Reference subject; 1618 1619 /** 1620 * The actual object that is the target of the reference (The individual or set 1621 * of individuals receiving the goods and services billed in this invoice.) 1622 */ 1623 protected Resource subjectTarget; 1624 1625 /** 1626 * The individual or Organization responsible for balancing of this invoice. 1627 */ 1628 @Child(name = "recipient", type = { Organization.class, Patient.class, 1629 RelatedPerson.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1630 @Description(shortDefinition = "Recipient of this invoice", formalDefinition = "The individual or Organization responsible for balancing of this invoice.") 1631 protected Reference recipient; 1632 1633 /** 1634 * The actual object that is the target of the reference (The individual or 1635 * Organization responsible for balancing of this invoice.) 1636 */ 1637 protected Resource recipientTarget; 1638 1639 /** 1640 * Date/time(s) of when this Invoice was posted. 1641 */ 1642 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1643 @Description(shortDefinition = "Invoice date / posting date", formalDefinition = "Date/time(s) of when this Invoice was posted.") 1644 protected DateTimeType date; 1645 1646 /** 1647 * Indicates who or what performed or participated in the charged service. 1648 */ 1649 @Child(name = "participant", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1650 @Description(shortDefinition = "Participant in creation of this Invoice", formalDefinition = "Indicates who or what performed or participated in the charged service.") 1651 protected List<InvoiceParticipantComponent> participant; 1652 1653 /** 1654 * The organizationissuing the Invoice. 1655 */ 1656 @Child(name = "issuer", type = { Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1657 @Description(shortDefinition = "Issuing Organization of Invoice", formalDefinition = "The organizationissuing the Invoice.") 1658 protected Reference issuer; 1659 1660 /** 1661 * The actual object that is the target of the reference (The 1662 * organizationissuing the Invoice.) 1663 */ 1664 protected Organization issuerTarget; 1665 1666 /** 1667 * Account which is supposed to be balanced with this Invoice. 1668 */ 1669 @Child(name = "account", type = { Account.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1670 @Description(shortDefinition = "Account that is being balanced", formalDefinition = "Account which is supposed to be balanced with this Invoice.") 1671 protected Reference account; 1672 1673 /** 1674 * The actual object that is the target of the reference (Account which is 1675 * supposed to be balanced with this Invoice.) 1676 */ 1677 protected Account accountTarget; 1678 1679 /** 1680 * Each line item represents one charge for goods and services rendered. Details 1681 * such as date, code and amount are found in the referenced ChargeItem 1682 * resource. 1683 */ 1684 @Child(name = "lineItem", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1685 @Description(shortDefinition = "Line items of this Invoice", formalDefinition = "Each line item represents one charge for goods and services rendered. Details such as date, code and amount are found in the referenced ChargeItem resource.") 1686 protected List<InvoiceLineItemComponent> lineItem; 1687 1688 /** 1689 * The total amount for the Invoice may be calculated as the sum of the line 1690 * items with surcharges/deductions that apply in certain conditions. The 1691 * priceComponent element can be used to offer transparency to the recipient of 1692 * the Invoice of how the total price was calculated. 1693 */ 1694 @Child(name = "totalPriceComponent", type = { 1695 InvoiceLineItemPriceComponentComponent.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1696 @Description(shortDefinition = "Components of Invoice total", formalDefinition = "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.") 1697 protected List<InvoiceLineItemPriceComponentComponent> totalPriceComponent; 1698 1699 /** 1700 * Invoice total , taxes excluded. 1701 */ 1702 @Child(name = "totalNet", type = { Money.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1703 @Description(shortDefinition = "Net total of this Invoice", formalDefinition = "Invoice total , taxes excluded.") 1704 protected Money totalNet; 1705 1706 /** 1707 * Invoice total, tax included. 1708 */ 1709 @Child(name = "totalGross", type = { Money.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1710 @Description(shortDefinition = "Gross total of this Invoice", formalDefinition = "Invoice total, tax included.") 1711 protected Money totalGross; 1712 1713 /** 1714 * Payment details such as banking details, period of payment, deductibles, 1715 * methods of payment. 1716 */ 1717 @Child(name = "paymentTerms", type = { 1718 MarkdownType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1719 @Description(shortDefinition = "Payment details", formalDefinition = "Payment details such as banking details, period of payment, deductibles, methods of payment.") 1720 protected MarkdownType paymentTerms; 1721 1722 /** 1723 * Comments made about the invoice by the issuer, subject, or other 1724 * participants. 1725 */ 1726 @Child(name = "note", type = { 1727 Annotation.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1728 @Description(shortDefinition = "Comments made about the invoice", formalDefinition = "Comments made about the invoice by the issuer, subject, or other participants.") 1729 protected List<Annotation> note; 1730 1731 private static final long serialVersionUID = -62357265L; 1732 1733 /** 1734 * Constructor 1735 */ 1736 public Invoice() { 1737 super(); 1738 } 1739 1740 /** 1741 * Constructor 1742 */ 1743 public Invoice(Enumeration<InvoiceStatus> status) { 1744 super(); 1745 this.status = status; 1746 } 1747 1748 /** 1749 * @return {@link #identifier} (Identifier of this Invoice, often used for 1750 * reference in correspondence about this invoice or for tracking of 1751 * payments.) 1752 */ 1753 public List<Identifier> getIdentifier() { 1754 if (this.identifier == null) 1755 this.identifier = new ArrayList<Identifier>(); 1756 return this.identifier; 1757 } 1758 1759 /** 1760 * @return Returns a reference to <code>this</code> for easy method chaining 1761 */ 1762 public Invoice setIdentifier(List<Identifier> theIdentifier) { 1763 this.identifier = theIdentifier; 1764 return this; 1765 } 1766 1767 public boolean hasIdentifier() { 1768 if (this.identifier == null) 1769 return false; 1770 for (Identifier item : this.identifier) 1771 if (!item.isEmpty()) 1772 return true; 1773 return false; 1774 } 1775 1776 public Identifier addIdentifier() { // 3 1777 Identifier t = new Identifier(); 1778 if (this.identifier == null) 1779 this.identifier = new ArrayList<Identifier>(); 1780 this.identifier.add(t); 1781 return t; 1782 } 1783 1784 public Invoice addIdentifier(Identifier t) { // 3 1785 if (t == null) 1786 return this; 1787 if (this.identifier == null) 1788 this.identifier = new ArrayList<Identifier>(); 1789 this.identifier.add(t); 1790 return this; 1791 } 1792 1793 /** 1794 * @return The first repetition of repeating field {@link #identifier}, creating 1795 * it if it does not already exist 1796 */ 1797 public Identifier getIdentifierFirstRep() { 1798 if (getIdentifier().isEmpty()) { 1799 addIdentifier(); 1800 } 1801 return getIdentifier().get(0); 1802 } 1803 1804 /** 1805 * @return {@link #status} (The current state of the Invoice.). This is the 1806 * underlying object with id, value and extensions. The accessor 1807 * "getStatus" gives direct access to the value 1808 */ 1809 public Enumeration<InvoiceStatus> getStatusElement() { 1810 if (this.status == null) 1811 if (Configuration.errorOnAutoCreate()) 1812 throw new Error("Attempt to auto-create Invoice.status"); 1813 else if (Configuration.doAutoCreate()) 1814 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); // bb 1815 return this.status; 1816 } 1817 1818 public boolean hasStatusElement() { 1819 return this.status != null && !this.status.isEmpty(); 1820 } 1821 1822 public boolean hasStatus() { 1823 return this.status != null && !this.status.isEmpty(); 1824 } 1825 1826 /** 1827 * @param value {@link #status} (The current state of the Invoice.). This is the 1828 * underlying object with id, value and extensions. The accessor 1829 * "getStatus" gives direct access to the value 1830 */ 1831 public Invoice setStatusElement(Enumeration<InvoiceStatus> value) { 1832 this.status = value; 1833 return this; 1834 } 1835 1836 /** 1837 * @return The current state of the Invoice. 1838 */ 1839 public InvoiceStatus getStatus() { 1840 return this.status == null ? null : this.status.getValue(); 1841 } 1842 1843 /** 1844 * @param value The current state of the Invoice. 1845 */ 1846 public Invoice setStatus(InvoiceStatus value) { 1847 if (this.status == null) 1848 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); 1849 this.status.setValue(value); 1850 return this; 1851 } 1852 1853 /** 1854 * @return {@link #cancelledReason} (In case of Invoice cancellation a reason 1855 * must be given (entered in error, superseded by corrected invoice 1856 * etc.).). This is the underlying object with id, value and extensions. 1857 * The accessor "getCancelledReason" gives direct access to the value 1858 */ 1859 public StringType getCancelledReasonElement() { 1860 if (this.cancelledReason == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error("Attempt to auto-create Invoice.cancelledReason"); 1863 else if (Configuration.doAutoCreate()) 1864 this.cancelledReason = new StringType(); // bb 1865 return this.cancelledReason; 1866 } 1867 1868 public boolean hasCancelledReasonElement() { 1869 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1870 } 1871 1872 public boolean hasCancelledReason() { 1873 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1874 } 1875 1876 /** 1877 * @param value {@link #cancelledReason} (In case of Invoice cancellation a 1878 * reason must be given (entered in error, superseded by corrected 1879 * invoice etc.).). This is the underlying object with id, value 1880 * and extensions. The accessor "getCancelledReason" gives direct 1881 * access to the value 1882 */ 1883 public Invoice setCancelledReasonElement(StringType value) { 1884 this.cancelledReason = value; 1885 return this; 1886 } 1887 1888 /** 1889 * @return In case of Invoice cancellation a reason must be given (entered in 1890 * error, superseded by corrected invoice etc.). 1891 */ 1892 public String getCancelledReason() { 1893 return this.cancelledReason == null ? null : this.cancelledReason.getValue(); 1894 } 1895 1896 /** 1897 * @param value In case of Invoice cancellation a reason must be given (entered 1898 * in error, superseded by corrected invoice etc.). 1899 */ 1900 public Invoice setCancelledReason(String value) { 1901 if (Utilities.noString(value)) 1902 this.cancelledReason = null; 1903 else { 1904 if (this.cancelledReason == null) 1905 this.cancelledReason = new StringType(); 1906 this.cancelledReason.setValue(value); 1907 } 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #type} (Type of Invoice depending on domain, realm an usage 1913 * (e.g. internal/external, dental, preliminary).) 1914 */ 1915 public CodeableConcept getType() { 1916 if (this.type == null) 1917 if (Configuration.errorOnAutoCreate()) 1918 throw new Error("Attempt to auto-create Invoice.type"); 1919 else if (Configuration.doAutoCreate()) 1920 this.type = new CodeableConcept(); // cc 1921 return this.type; 1922 } 1923 1924 public boolean hasType() { 1925 return this.type != null && !this.type.isEmpty(); 1926 } 1927 1928 /** 1929 * @param value {@link #type} (Type of Invoice depending on domain, realm an 1930 * usage (e.g. internal/external, dental, preliminary).) 1931 */ 1932 public Invoice setType(CodeableConcept value) { 1933 this.type = value; 1934 return this; 1935 } 1936 1937 /** 1938 * @return {@link #subject} (The individual or set of individuals receiving the 1939 * goods and services billed in this invoice.) 1940 */ 1941 public Reference getSubject() { 1942 if (this.subject == null) 1943 if (Configuration.errorOnAutoCreate()) 1944 throw new Error("Attempt to auto-create Invoice.subject"); 1945 else if (Configuration.doAutoCreate()) 1946 this.subject = new Reference(); // cc 1947 return this.subject; 1948 } 1949 1950 public boolean hasSubject() { 1951 return this.subject != null && !this.subject.isEmpty(); 1952 } 1953 1954 /** 1955 * @param value {@link #subject} (The individual or set of individuals receiving 1956 * the goods and services billed in this invoice.) 1957 */ 1958 public Invoice setSubject(Reference value) { 1959 this.subject = value; 1960 return this; 1961 } 1962 1963 /** 1964 * @return {@link #subject} The actual object that is the target of the 1965 * reference. The reference library doesn't populate this, but you can 1966 * use it to hold the resource if you resolve it. (The individual or set 1967 * of individuals receiving the goods and services billed in this 1968 * invoice.) 1969 */ 1970 public Resource getSubjectTarget() { 1971 return this.subjectTarget; 1972 } 1973 1974 /** 1975 * @param value {@link #subject} The actual object that is the target of the 1976 * reference. The reference library doesn't use these, but you can 1977 * use it to hold the resource if you resolve it. (The individual 1978 * or set of individuals receiving the goods and services billed in 1979 * this invoice.) 1980 */ 1981 public Invoice setSubjectTarget(Resource value) { 1982 this.subjectTarget = value; 1983 return this; 1984 } 1985 1986 /** 1987 * @return {@link #recipient} (The individual or Organization responsible for 1988 * balancing of this invoice.) 1989 */ 1990 public Reference getRecipient() { 1991 if (this.recipient == null) 1992 if (Configuration.errorOnAutoCreate()) 1993 throw new Error("Attempt to auto-create Invoice.recipient"); 1994 else if (Configuration.doAutoCreate()) 1995 this.recipient = new Reference(); // cc 1996 return this.recipient; 1997 } 1998 1999 public boolean hasRecipient() { 2000 return this.recipient != null && !this.recipient.isEmpty(); 2001 } 2002 2003 /** 2004 * @param value {@link #recipient} (The individual or Organization responsible 2005 * for balancing of this invoice.) 2006 */ 2007 public Invoice setRecipient(Reference value) { 2008 this.recipient = value; 2009 return this; 2010 } 2011 2012 /** 2013 * @return {@link #recipient} The actual object that is the target of the 2014 * reference. The reference library doesn't populate this, but you can 2015 * use it to hold the resource if you resolve it. (The individual or 2016 * Organization responsible for balancing of this invoice.) 2017 */ 2018 public Resource getRecipientTarget() { 2019 return this.recipientTarget; 2020 } 2021 2022 /** 2023 * @param value {@link #recipient} The actual object that is the target of the 2024 * reference. The reference library doesn't use these, but you can 2025 * use it to hold the resource if you resolve it. (The individual 2026 * or Organization responsible for balancing of this invoice.) 2027 */ 2028 public Invoice setRecipientTarget(Resource value) { 2029 this.recipientTarget = value; 2030 return this; 2031 } 2032 2033 /** 2034 * @return {@link #date} (Date/time(s) of when this Invoice was posted.). This 2035 * is the underlying object with id, value and extensions. The accessor 2036 * "getDate" gives direct access to the value 2037 */ 2038 public DateTimeType getDateElement() { 2039 if (this.date == null) 2040 if (Configuration.errorOnAutoCreate()) 2041 throw new Error("Attempt to auto-create Invoice.date"); 2042 else if (Configuration.doAutoCreate()) 2043 this.date = new DateTimeType(); // bb 2044 return this.date; 2045 } 2046 2047 public boolean hasDateElement() { 2048 return this.date != null && !this.date.isEmpty(); 2049 } 2050 2051 public boolean hasDate() { 2052 return this.date != null && !this.date.isEmpty(); 2053 } 2054 2055 /** 2056 * @param value {@link #date} (Date/time(s) of when this Invoice was posted.). 2057 * This is the underlying object with id, value and extensions. The 2058 * accessor "getDate" gives direct access to the value 2059 */ 2060 public Invoice setDateElement(DateTimeType value) { 2061 this.date = value; 2062 return this; 2063 } 2064 2065 /** 2066 * @return Date/time(s) of when this Invoice was posted. 2067 */ 2068 public Date getDate() { 2069 return this.date == null ? null : this.date.getValue(); 2070 } 2071 2072 /** 2073 * @param value Date/time(s) of when this Invoice was posted. 2074 */ 2075 public Invoice setDate(Date value) { 2076 if (value == null) 2077 this.date = null; 2078 else { 2079 if (this.date == null) 2080 this.date = new DateTimeType(); 2081 this.date.setValue(value); 2082 } 2083 return this; 2084 } 2085 2086 /** 2087 * @return {@link #participant} (Indicates who or what performed or participated 2088 * in the charged service.) 2089 */ 2090 public List<InvoiceParticipantComponent> getParticipant() { 2091 if (this.participant == null) 2092 this.participant = new ArrayList<InvoiceParticipantComponent>(); 2093 return this.participant; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public Invoice setParticipant(List<InvoiceParticipantComponent> theParticipant) { 2100 this.participant = theParticipant; 2101 return this; 2102 } 2103 2104 public boolean hasParticipant() { 2105 if (this.participant == null) 2106 return false; 2107 for (InvoiceParticipantComponent item : this.participant) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 public InvoiceParticipantComponent addParticipant() { // 3 2114 InvoiceParticipantComponent t = new InvoiceParticipantComponent(); 2115 if (this.participant == null) 2116 this.participant = new ArrayList<InvoiceParticipantComponent>(); 2117 this.participant.add(t); 2118 return t; 2119 } 2120 2121 public Invoice addParticipant(InvoiceParticipantComponent t) { // 3 2122 if (t == null) 2123 return this; 2124 if (this.participant == null) 2125 this.participant = new ArrayList<InvoiceParticipantComponent>(); 2126 this.participant.add(t); 2127 return this; 2128 } 2129 2130 /** 2131 * @return The first repetition of repeating field {@link #participant}, 2132 * creating it if it does not already exist 2133 */ 2134 public InvoiceParticipantComponent getParticipantFirstRep() { 2135 if (getParticipant().isEmpty()) { 2136 addParticipant(); 2137 } 2138 return getParticipant().get(0); 2139 } 2140 2141 /** 2142 * @return {@link #issuer} (The organizationissuing the Invoice.) 2143 */ 2144 public Reference getIssuer() { 2145 if (this.issuer == null) 2146 if (Configuration.errorOnAutoCreate()) 2147 throw new Error("Attempt to auto-create Invoice.issuer"); 2148 else if (Configuration.doAutoCreate()) 2149 this.issuer = new Reference(); // cc 2150 return this.issuer; 2151 } 2152 2153 public boolean hasIssuer() { 2154 return this.issuer != null && !this.issuer.isEmpty(); 2155 } 2156 2157 /** 2158 * @param value {@link #issuer} (The organizationissuing the Invoice.) 2159 */ 2160 public Invoice setIssuer(Reference value) { 2161 this.issuer = value; 2162 return this; 2163 } 2164 2165 /** 2166 * @return {@link #issuer} The actual object that is the target of the 2167 * reference. The reference library doesn't populate this, but you can 2168 * use it to hold the resource if you resolve it. (The 2169 * organizationissuing the Invoice.) 2170 */ 2171 public Organization getIssuerTarget() { 2172 if (this.issuerTarget == null) 2173 if (Configuration.errorOnAutoCreate()) 2174 throw new Error("Attempt to auto-create Invoice.issuer"); 2175 else if (Configuration.doAutoCreate()) 2176 this.issuerTarget = new Organization(); // aa 2177 return this.issuerTarget; 2178 } 2179 2180 /** 2181 * @param value {@link #issuer} The actual object that is the target of the 2182 * reference. The reference library doesn't use these, but you can 2183 * use it to hold the resource if you resolve it. (The 2184 * organizationissuing the Invoice.) 2185 */ 2186 public Invoice setIssuerTarget(Organization value) { 2187 this.issuerTarget = value; 2188 return this; 2189 } 2190 2191 /** 2192 * @return {@link #account} (Account which is supposed to be balanced with this 2193 * Invoice.) 2194 */ 2195 public Reference getAccount() { 2196 if (this.account == null) 2197 if (Configuration.errorOnAutoCreate()) 2198 throw new Error("Attempt to auto-create Invoice.account"); 2199 else if (Configuration.doAutoCreate()) 2200 this.account = new Reference(); // cc 2201 return this.account; 2202 } 2203 2204 public boolean hasAccount() { 2205 return this.account != null && !this.account.isEmpty(); 2206 } 2207 2208 /** 2209 * @param value {@link #account} (Account which is supposed to be balanced with 2210 * this Invoice.) 2211 */ 2212 public Invoice setAccount(Reference value) { 2213 this.account = value; 2214 return this; 2215 } 2216 2217 /** 2218 * @return {@link #account} The actual object that is the target of the 2219 * reference. The reference library doesn't populate this, but you can 2220 * use it to hold the resource if you resolve it. (Account which is 2221 * supposed to be balanced with this Invoice.) 2222 */ 2223 public Account getAccountTarget() { 2224 if (this.accountTarget == null) 2225 if (Configuration.errorOnAutoCreate()) 2226 throw new Error("Attempt to auto-create Invoice.account"); 2227 else if (Configuration.doAutoCreate()) 2228 this.accountTarget = new Account(); // aa 2229 return this.accountTarget; 2230 } 2231 2232 /** 2233 * @param value {@link #account} The actual object that is the target of the 2234 * reference. The reference library doesn't use these, but you can 2235 * use it to hold the resource if you resolve it. (Account which is 2236 * supposed to be balanced with this Invoice.) 2237 */ 2238 public Invoice setAccountTarget(Account value) { 2239 this.accountTarget = value; 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #lineItem} (Each line item represents one charge for goods and 2245 * services rendered. Details such as date, code and amount are found in 2246 * the referenced ChargeItem resource.) 2247 */ 2248 public List<InvoiceLineItemComponent> getLineItem() { 2249 if (this.lineItem == null) 2250 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2251 return this.lineItem; 2252 } 2253 2254 /** 2255 * @return Returns a reference to <code>this</code> for easy method chaining 2256 */ 2257 public Invoice setLineItem(List<InvoiceLineItemComponent> theLineItem) { 2258 this.lineItem = theLineItem; 2259 return this; 2260 } 2261 2262 public boolean hasLineItem() { 2263 if (this.lineItem == null) 2264 return false; 2265 for (InvoiceLineItemComponent item : this.lineItem) 2266 if (!item.isEmpty()) 2267 return true; 2268 return false; 2269 } 2270 2271 public InvoiceLineItemComponent addLineItem() { // 3 2272 InvoiceLineItemComponent t = new InvoiceLineItemComponent(); 2273 if (this.lineItem == null) 2274 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2275 this.lineItem.add(t); 2276 return t; 2277 } 2278 2279 public Invoice addLineItem(InvoiceLineItemComponent t) { // 3 2280 if (t == null) 2281 return this; 2282 if (this.lineItem == null) 2283 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2284 this.lineItem.add(t); 2285 return this; 2286 } 2287 2288 /** 2289 * @return The first repetition of repeating field {@link #lineItem}, creating 2290 * it if it does not already exist 2291 */ 2292 public InvoiceLineItemComponent getLineItemFirstRep() { 2293 if (getLineItem().isEmpty()) { 2294 addLineItem(); 2295 } 2296 return getLineItem().get(0); 2297 } 2298 2299 /** 2300 * @return {@link #totalPriceComponent} (The total amount for the Invoice may be 2301 * calculated as the sum of the line items with surcharges/deductions 2302 * that apply in certain conditions. The priceComponent element can be 2303 * used to offer transparency to the recipient of the Invoice of how the 2304 * total price was calculated.) 2305 */ 2306 public List<InvoiceLineItemPriceComponentComponent> getTotalPriceComponent() { 2307 if (this.totalPriceComponent == null) 2308 this.totalPriceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 2309 return this.totalPriceComponent; 2310 } 2311 2312 /** 2313 * @return Returns a reference to <code>this</code> for easy method chaining 2314 */ 2315 public Invoice setTotalPriceComponent(List<InvoiceLineItemPriceComponentComponent> theTotalPriceComponent) { 2316 this.totalPriceComponent = theTotalPriceComponent; 2317 return this; 2318 } 2319 2320 public boolean hasTotalPriceComponent() { 2321 if (this.totalPriceComponent == null) 2322 return false; 2323 for (InvoiceLineItemPriceComponentComponent item : this.totalPriceComponent) 2324 if (!item.isEmpty()) 2325 return true; 2326 return false; 2327 } 2328 2329 public InvoiceLineItemPriceComponentComponent addTotalPriceComponent() { // 3 2330 InvoiceLineItemPriceComponentComponent t = new InvoiceLineItemPriceComponentComponent(); 2331 if (this.totalPriceComponent == null) 2332 this.totalPriceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 2333 this.totalPriceComponent.add(t); 2334 return t; 2335 } 2336 2337 public Invoice addTotalPriceComponent(InvoiceLineItemPriceComponentComponent t) { // 3 2338 if (t == null) 2339 return this; 2340 if (this.totalPriceComponent == null) 2341 this.totalPriceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 2342 this.totalPriceComponent.add(t); 2343 return this; 2344 } 2345 2346 /** 2347 * @return The first repetition of repeating field {@link #totalPriceComponent}, 2348 * creating it if it does not already exist 2349 */ 2350 public InvoiceLineItemPriceComponentComponent getTotalPriceComponentFirstRep() { 2351 if (getTotalPriceComponent().isEmpty()) { 2352 addTotalPriceComponent(); 2353 } 2354 return getTotalPriceComponent().get(0); 2355 } 2356 2357 /** 2358 * @return {@link #totalNet} (Invoice total , taxes excluded.) 2359 */ 2360 public Money getTotalNet() { 2361 if (this.totalNet == null) 2362 if (Configuration.errorOnAutoCreate()) 2363 throw new Error("Attempt to auto-create Invoice.totalNet"); 2364 else if (Configuration.doAutoCreate()) 2365 this.totalNet = new Money(); // cc 2366 return this.totalNet; 2367 } 2368 2369 public boolean hasTotalNet() { 2370 return this.totalNet != null && !this.totalNet.isEmpty(); 2371 } 2372 2373 /** 2374 * @param value {@link #totalNet} (Invoice total , taxes excluded.) 2375 */ 2376 public Invoice setTotalNet(Money value) { 2377 this.totalNet = value; 2378 return this; 2379 } 2380 2381 /** 2382 * @return {@link #totalGross} (Invoice total, tax included.) 2383 */ 2384 public Money getTotalGross() { 2385 if (this.totalGross == null) 2386 if (Configuration.errorOnAutoCreate()) 2387 throw new Error("Attempt to auto-create Invoice.totalGross"); 2388 else if (Configuration.doAutoCreate()) 2389 this.totalGross = new Money(); // cc 2390 return this.totalGross; 2391 } 2392 2393 public boolean hasTotalGross() { 2394 return this.totalGross != null && !this.totalGross.isEmpty(); 2395 } 2396 2397 /** 2398 * @param value {@link #totalGross} (Invoice total, tax included.) 2399 */ 2400 public Invoice setTotalGross(Money value) { 2401 this.totalGross = value; 2402 return this; 2403 } 2404 2405 /** 2406 * @return {@link #paymentTerms} (Payment details such as banking details, 2407 * period of payment, deductibles, methods of payment.). This is the 2408 * underlying object with id, value and extensions. The accessor 2409 * "getPaymentTerms" gives direct access to the value 2410 */ 2411 public MarkdownType getPaymentTermsElement() { 2412 if (this.paymentTerms == null) 2413 if (Configuration.errorOnAutoCreate()) 2414 throw new Error("Attempt to auto-create Invoice.paymentTerms"); 2415 else if (Configuration.doAutoCreate()) 2416 this.paymentTerms = new MarkdownType(); // bb 2417 return this.paymentTerms; 2418 } 2419 2420 public boolean hasPaymentTermsElement() { 2421 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 2422 } 2423 2424 public boolean hasPaymentTerms() { 2425 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 2426 } 2427 2428 /** 2429 * @param value {@link #paymentTerms} (Payment details such as banking details, 2430 * period of payment, deductibles, methods of payment.). This is 2431 * the underlying object with id, value and extensions. The 2432 * accessor "getPaymentTerms" gives direct access to the value 2433 */ 2434 public Invoice setPaymentTermsElement(MarkdownType value) { 2435 this.paymentTerms = value; 2436 return this; 2437 } 2438 2439 /** 2440 * @return Payment details such as banking details, period of payment, 2441 * deductibles, methods of payment. 2442 */ 2443 public String getPaymentTerms() { 2444 return this.paymentTerms == null ? null : this.paymentTerms.getValue(); 2445 } 2446 2447 /** 2448 * @param value Payment details such as banking details, period of payment, 2449 * deductibles, methods of payment. 2450 */ 2451 public Invoice setPaymentTerms(String value) { 2452 if (value == null) 2453 this.paymentTerms = null; 2454 else { 2455 if (this.paymentTerms == null) 2456 this.paymentTerms = new MarkdownType(); 2457 this.paymentTerms.setValue(value); 2458 } 2459 return this; 2460 } 2461 2462 /** 2463 * @return {@link #note} (Comments made about the invoice by the issuer, 2464 * subject, or other participants.) 2465 */ 2466 public List<Annotation> getNote() { 2467 if (this.note == null) 2468 this.note = new ArrayList<Annotation>(); 2469 return this.note; 2470 } 2471 2472 /** 2473 * @return Returns a reference to <code>this</code> for easy method chaining 2474 */ 2475 public Invoice setNote(List<Annotation> theNote) { 2476 this.note = theNote; 2477 return this; 2478 } 2479 2480 public boolean hasNote() { 2481 if (this.note == null) 2482 return false; 2483 for (Annotation item : this.note) 2484 if (!item.isEmpty()) 2485 return true; 2486 return false; 2487 } 2488 2489 public Annotation addNote() { // 3 2490 Annotation t = new Annotation(); 2491 if (this.note == null) 2492 this.note = new ArrayList<Annotation>(); 2493 this.note.add(t); 2494 return t; 2495 } 2496 2497 public Invoice addNote(Annotation t) { // 3 2498 if (t == null) 2499 return this; 2500 if (this.note == null) 2501 this.note = new ArrayList<Annotation>(); 2502 this.note.add(t); 2503 return this; 2504 } 2505 2506 /** 2507 * @return The first repetition of repeating field {@link #note}, creating it if 2508 * it does not already exist 2509 */ 2510 public Annotation getNoteFirstRep() { 2511 if (getNote().isEmpty()) { 2512 addNote(); 2513 } 2514 return getNote().get(0); 2515 } 2516 2517 protected void listChildren(List<Property> children) { 2518 super.listChildren(children); 2519 children.add(new Property("identifier", "Identifier", 2520 "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 2521 0, java.lang.Integer.MAX_VALUE, identifier)); 2522 children.add(new Property("status", "code", "The current state of the Invoice.", 0, 1, status)); 2523 children.add(new Property("cancelledReason", "string", 2524 "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 2525 0, 1, cancelledReason)); 2526 children.add(new Property("type", "CodeableConcept", 2527 "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, 2528 type)); 2529 children.add(new Property("subject", "Reference(Patient|Group)", 2530 "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, 2531 subject)); 2532 children.add(new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", 2533 "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient)); 2534 children.add(new Property("date", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, date)); 2535 children 2536 .add(new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 2537 0, java.lang.Integer.MAX_VALUE, participant)); 2538 children 2539 .add(new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 1, issuer)); 2540 children.add(new Property("account", "Reference(Account)", 2541 "Account which is supposed to be balanced with this Invoice.", 0, 1, account)); 2542 children.add(new Property("lineItem", "", 2543 "Each line item represents one charge for goods and services rendered. Details such as date, code and amount are found in the referenced ChargeItem resource.", 2544 0, java.lang.Integer.MAX_VALUE, lineItem)); 2545 children.add(new Property("totalPriceComponent", "@Invoice.lineItem.priceComponent", 2546 "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 2547 0, java.lang.Integer.MAX_VALUE, totalPriceComponent)); 2548 children.add(new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet)); 2549 children.add(new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross)); 2550 children.add(new Property("paymentTerms", "markdown", 2551 "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, 2552 paymentTerms)); 2553 children.add(new Property("note", "Annotation", 2554 "Comments made about the invoice by the issuer, subject, or other participants.", 0, 2555 java.lang.Integer.MAX_VALUE, note)); 2556 } 2557 2558 @Override 2559 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2560 switch (_hash) { 2561 case -1618432855: 2562 /* identifier */ return new Property("identifier", "Identifier", 2563 "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 2564 0, java.lang.Integer.MAX_VALUE, identifier); 2565 case -892481550: 2566 /* status */ return new Property("status", "code", "The current state of the Invoice.", 0, 1, status); 2567 case 1550362357: 2568 /* cancelledReason */ return new Property("cancelledReason", "string", 2569 "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 2570 0, 1, cancelledReason); 2571 case 3575610: 2572 /* type */ return new Property("type", "CodeableConcept", 2573 "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, 2574 type); 2575 case -1867885268: 2576 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2577 "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, 2578 subject); 2579 case 820081177: 2580 /* recipient */ return new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", 2581 "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient); 2582 case 3076014: 2583 /* date */ return new Property("date", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, date); 2584 case 767422259: 2585 /* participant */ return new Property("participant", "", 2586 "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, 2587 participant); 2588 case -1179159879: 2589 /* issuer */ return new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 2590 1, issuer); 2591 case -1177318867: 2592 /* account */ return new Property("account", "Reference(Account)", 2593 "Account which is supposed to be balanced with this Invoice.", 0, 1, account); 2594 case 1188332839: 2595 /* lineItem */ return new Property("lineItem", "", 2596 "Each line item represents one charge for goods and services rendered. Details such as date, code and amount are found in the referenced ChargeItem resource.", 2597 0, java.lang.Integer.MAX_VALUE, lineItem); 2598 case 1731497496: 2599 /* totalPriceComponent */ return new Property("totalPriceComponent", "@Invoice.lineItem.priceComponent", 2600 "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 2601 0, java.lang.Integer.MAX_VALUE, totalPriceComponent); 2602 case -849911879: 2603 /* totalNet */ return new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet); 2604 case -727607968: 2605 /* totalGross */ return new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross); 2606 case -507544799: 2607 /* paymentTerms */ return new Property("paymentTerms", "markdown", 2608 "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, 2609 paymentTerms); 2610 case 3387378: 2611 /* note */ return new Property("note", "Annotation", 2612 "Comments made about the invoice by the issuer, subject, or other participants.", 0, 2613 java.lang.Integer.MAX_VALUE, note); 2614 default: 2615 return super.getNamedProperty(_hash, _name, _checkValid); 2616 } 2617 2618 } 2619 2620 @Override 2621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2622 switch (hash) { 2623 case -1618432855: 2624 /* identifier */ return this.identifier == null ? new Base[0] 2625 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2626 case -892481550: 2627 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<InvoiceStatus> 2628 case 1550362357: 2629 /* cancelledReason */ return this.cancelledReason == null ? new Base[0] : new Base[] { this.cancelledReason }; // StringType 2630 case 3575610: 2631 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 2632 case -1867885268: 2633 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2634 case 820081177: 2635 /* recipient */ return this.recipient == null ? new Base[0] : new Base[] { this.recipient }; // Reference 2636 case 3076014: 2637 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2638 case 767422259: 2639 /* participant */ return this.participant == null ? new Base[0] 2640 : this.participant.toArray(new Base[this.participant.size()]); // InvoiceParticipantComponent 2641 case -1179159879: 2642 /* issuer */ return this.issuer == null ? new Base[0] : new Base[] { this.issuer }; // Reference 2643 case -1177318867: 2644 /* account */ return this.account == null ? new Base[0] : new Base[] { this.account }; // Reference 2645 case 1188332839: 2646 /* lineItem */ return this.lineItem == null ? new Base[0] : this.lineItem.toArray(new Base[this.lineItem.size()]); // InvoiceLineItemComponent 2647 case 1731497496: 2648 /* totalPriceComponent */ return this.totalPriceComponent == null ? new Base[0] 2649 : this.totalPriceComponent.toArray(new Base[this.totalPriceComponent.size()]); // InvoiceLineItemPriceComponentComponent 2650 case -849911879: 2651 /* totalNet */ return this.totalNet == null ? new Base[0] : new Base[] { this.totalNet }; // Money 2652 case -727607968: 2653 /* totalGross */ return this.totalGross == null ? new Base[0] : new Base[] { this.totalGross }; // Money 2654 case -507544799: 2655 /* paymentTerms */ return this.paymentTerms == null ? new Base[0] : new Base[] { this.paymentTerms }; // MarkdownType 2656 case 3387378: 2657 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2658 default: 2659 return super.getProperty(hash, name, checkValid); 2660 } 2661 2662 } 2663 2664 @Override 2665 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2666 switch (hash) { 2667 case -1618432855: // identifier 2668 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2669 return value; 2670 case -892481550: // status 2671 value = new InvoiceStatusEnumFactory().fromType(castToCode(value)); 2672 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 2673 return value; 2674 case 1550362357: // cancelledReason 2675 this.cancelledReason = castToString(value); // StringType 2676 return value; 2677 case 3575610: // type 2678 this.type = castToCodeableConcept(value); // CodeableConcept 2679 return value; 2680 case -1867885268: // subject 2681 this.subject = castToReference(value); // Reference 2682 return value; 2683 case 820081177: // recipient 2684 this.recipient = castToReference(value); // Reference 2685 return value; 2686 case 3076014: // date 2687 this.date = castToDateTime(value); // DateTimeType 2688 return value; 2689 case 767422259: // participant 2690 this.getParticipant().add((InvoiceParticipantComponent) value); // InvoiceParticipantComponent 2691 return value; 2692 case -1179159879: // issuer 2693 this.issuer = castToReference(value); // Reference 2694 return value; 2695 case -1177318867: // account 2696 this.account = castToReference(value); // Reference 2697 return value; 2698 case 1188332839: // lineItem 2699 this.getLineItem().add((InvoiceLineItemComponent) value); // InvoiceLineItemComponent 2700 return value; 2701 case 1731497496: // totalPriceComponent 2702 this.getTotalPriceComponent().add((InvoiceLineItemPriceComponentComponent) value); // InvoiceLineItemPriceComponentComponent 2703 return value; 2704 case -849911879: // totalNet 2705 this.totalNet = castToMoney(value); // Money 2706 return value; 2707 case -727607968: // totalGross 2708 this.totalGross = castToMoney(value); // Money 2709 return value; 2710 case -507544799: // paymentTerms 2711 this.paymentTerms = castToMarkdown(value); // MarkdownType 2712 return value; 2713 case 3387378: // note 2714 this.getNote().add(castToAnnotation(value)); // Annotation 2715 return value; 2716 default: 2717 return super.setProperty(hash, name, value); 2718 } 2719 2720 } 2721 2722 @Override 2723 public Base setProperty(String name, Base value) throws FHIRException { 2724 if (name.equals("identifier")) { 2725 this.getIdentifier().add(castToIdentifier(value)); 2726 } else if (name.equals("status")) { 2727 value = new InvoiceStatusEnumFactory().fromType(castToCode(value)); 2728 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 2729 } else if (name.equals("cancelledReason")) { 2730 this.cancelledReason = castToString(value); // StringType 2731 } else if (name.equals("type")) { 2732 this.type = castToCodeableConcept(value); // CodeableConcept 2733 } else if (name.equals("subject")) { 2734 this.subject = castToReference(value); // Reference 2735 } else if (name.equals("recipient")) { 2736 this.recipient = castToReference(value); // Reference 2737 } else if (name.equals("date")) { 2738 this.date = castToDateTime(value); // DateTimeType 2739 } else if (name.equals("participant")) { 2740 this.getParticipant().add((InvoiceParticipantComponent) value); 2741 } else if (name.equals("issuer")) { 2742 this.issuer = castToReference(value); // Reference 2743 } else if (name.equals("account")) { 2744 this.account = castToReference(value); // Reference 2745 } else if (name.equals("lineItem")) { 2746 this.getLineItem().add((InvoiceLineItemComponent) value); 2747 } else if (name.equals("totalPriceComponent")) { 2748 this.getTotalPriceComponent().add((InvoiceLineItemPriceComponentComponent) value); 2749 } else if (name.equals("totalNet")) { 2750 this.totalNet = castToMoney(value); // Money 2751 } else if (name.equals("totalGross")) { 2752 this.totalGross = castToMoney(value); // Money 2753 } else if (name.equals("paymentTerms")) { 2754 this.paymentTerms = castToMarkdown(value); // MarkdownType 2755 } else if (name.equals("note")) { 2756 this.getNote().add(castToAnnotation(value)); 2757 } else 2758 return super.setProperty(name, value); 2759 return value; 2760 } 2761 2762 @Override 2763 public void removeChild(String name, Base value) throws FHIRException { 2764 if (name.equals("identifier")) { 2765 this.getIdentifier().remove(castToIdentifier(value)); 2766 } else if (name.equals("status")) { 2767 this.status = null; 2768 } else if (name.equals("cancelledReason")) { 2769 this.cancelledReason = null; 2770 } else if (name.equals("type")) { 2771 this.type = null; 2772 } else if (name.equals("subject")) { 2773 this.subject = null; 2774 } else if (name.equals("recipient")) { 2775 this.recipient = null; 2776 } else if (name.equals("date")) { 2777 this.date = null; 2778 } else if (name.equals("participant")) { 2779 this.getParticipant().remove((InvoiceParticipantComponent) value); 2780 } else if (name.equals("issuer")) { 2781 this.issuer = null; 2782 } else if (name.equals("account")) { 2783 this.account = null; 2784 } else if (name.equals("lineItem")) { 2785 this.getLineItem().remove((InvoiceLineItemComponent) value); 2786 } else if (name.equals("totalPriceComponent")) { 2787 this.getTotalPriceComponent().remove((InvoiceLineItemPriceComponentComponent) value); 2788 } else if (name.equals("totalNet")) { 2789 this.totalNet = null; 2790 } else if (name.equals("totalGross")) { 2791 this.totalGross = null; 2792 } else if (name.equals("paymentTerms")) { 2793 this.paymentTerms = null; 2794 } else if (name.equals("note")) { 2795 this.getNote().remove(castToAnnotation(value)); 2796 } else 2797 super.removeChild(name, value); 2798 2799 } 2800 2801 @Override 2802 public Base makeProperty(int hash, String name) throws FHIRException { 2803 switch (hash) { 2804 case -1618432855: 2805 return addIdentifier(); 2806 case -892481550: 2807 return getStatusElement(); 2808 case 1550362357: 2809 return getCancelledReasonElement(); 2810 case 3575610: 2811 return getType(); 2812 case -1867885268: 2813 return getSubject(); 2814 case 820081177: 2815 return getRecipient(); 2816 case 3076014: 2817 return getDateElement(); 2818 case 767422259: 2819 return addParticipant(); 2820 case -1179159879: 2821 return getIssuer(); 2822 case -1177318867: 2823 return getAccount(); 2824 case 1188332839: 2825 return addLineItem(); 2826 case 1731497496: 2827 return addTotalPriceComponent(); 2828 case -849911879: 2829 return getTotalNet(); 2830 case -727607968: 2831 return getTotalGross(); 2832 case -507544799: 2833 return getPaymentTermsElement(); 2834 case 3387378: 2835 return addNote(); 2836 default: 2837 return super.makeProperty(hash, name); 2838 } 2839 2840 } 2841 2842 @Override 2843 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2844 switch (hash) { 2845 case -1618432855: 2846 /* identifier */ return new String[] { "Identifier" }; 2847 case -892481550: 2848 /* status */ return new String[] { "code" }; 2849 case 1550362357: 2850 /* cancelledReason */ return new String[] { "string" }; 2851 case 3575610: 2852 /* type */ return new String[] { "CodeableConcept" }; 2853 case -1867885268: 2854 /* subject */ return new String[] { "Reference" }; 2855 case 820081177: 2856 /* recipient */ return new String[] { "Reference" }; 2857 case 3076014: 2858 /* date */ return new String[] { "dateTime" }; 2859 case 767422259: 2860 /* participant */ return new String[] {}; 2861 case -1179159879: 2862 /* issuer */ return new String[] { "Reference" }; 2863 case -1177318867: 2864 /* account */ return new String[] { "Reference" }; 2865 case 1188332839: 2866 /* lineItem */ return new String[] {}; 2867 case 1731497496: 2868 /* totalPriceComponent */ return new String[] { "@Invoice.lineItem.priceComponent" }; 2869 case -849911879: 2870 /* totalNet */ return new String[] { "Money" }; 2871 case -727607968: 2872 /* totalGross */ return new String[] { "Money" }; 2873 case -507544799: 2874 /* paymentTerms */ return new String[] { "markdown" }; 2875 case 3387378: 2876 /* note */ return new String[] { "Annotation" }; 2877 default: 2878 return super.getTypesForProperty(hash, name); 2879 } 2880 2881 } 2882 2883 @Override 2884 public Base addChild(String name) throws FHIRException { 2885 if (name.equals("identifier")) { 2886 return addIdentifier(); 2887 } else if (name.equals("status")) { 2888 throw new FHIRException("Cannot call addChild on a singleton property Invoice.status"); 2889 } else if (name.equals("cancelledReason")) { 2890 throw new FHIRException("Cannot call addChild on a singleton property Invoice.cancelledReason"); 2891 } else if (name.equals("type")) { 2892 this.type = new CodeableConcept(); 2893 return this.type; 2894 } else if (name.equals("subject")) { 2895 this.subject = new Reference(); 2896 return this.subject; 2897 } else if (name.equals("recipient")) { 2898 this.recipient = new Reference(); 2899 return this.recipient; 2900 } else if (name.equals("date")) { 2901 throw new FHIRException("Cannot call addChild on a singleton property Invoice.date"); 2902 } else if (name.equals("participant")) { 2903 return addParticipant(); 2904 } else if (name.equals("issuer")) { 2905 this.issuer = new Reference(); 2906 return this.issuer; 2907 } else if (name.equals("account")) { 2908 this.account = new Reference(); 2909 return this.account; 2910 } else if (name.equals("lineItem")) { 2911 return addLineItem(); 2912 } else if (name.equals("totalPriceComponent")) { 2913 return addTotalPriceComponent(); 2914 } else if (name.equals("totalNet")) { 2915 this.totalNet = new Money(); 2916 return this.totalNet; 2917 } else if (name.equals("totalGross")) { 2918 this.totalGross = new Money(); 2919 return this.totalGross; 2920 } else if (name.equals("paymentTerms")) { 2921 throw new FHIRException("Cannot call addChild on a singleton property Invoice.paymentTerms"); 2922 } else if (name.equals("note")) { 2923 return addNote(); 2924 } else 2925 return super.addChild(name); 2926 } 2927 2928 public String fhirType() { 2929 return "Invoice"; 2930 2931 } 2932 2933 public Invoice copy() { 2934 Invoice dst = new Invoice(); 2935 copyValues(dst); 2936 return dst; 2937 } 2938 2939 public void copyValues(Invoice dst) { 2940 super.copyValues(dst); 2941 if (identifier != null) { 2942 dst.identifier = new ArrayList<Identifier>(); 2943 for (Identifier i : identifier) 2944 dst.identifier.add(i.copy()); 2945 } 2946 ; 2947 dst.status = status == null ? null : status.copy(); 2948 dst.cancelledReason = cancelledReason == null ? null : cancelledReason.copy(); 2949 dst.type = type == null ? null : type.copy(); 2950 dst.subject = subject == null ? null : subject.copy(); 2951 dst.recipient = recipient == null ? null : recipient.copy(); 2952 dst.date = date == null ? null : date.copy(); 2953 if (participant != null) { 2954 dst.participant = new ArrayList<InvoiceParticipantComponent>(); 2955 for (InvoiceParticipantComponent i : participant) 2956 dst.participant.add(i.copy()); 2957 } 2958 ; 2959 dst.issuer = issuer == null ? null : issuer.copy(); 2960 dst.account = account == null ? null : account.copy(); 2961 if (lineItem != null) { 2962 dst.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2963 for (InvoiceLineItemComponent i : lineItem) 2964 dst.lineItem.add(i.copy()); 2965 } 2966 ; 2967 if (totalPriceComponent != null) { 2968 dst.totalPriceComponent = new ArrayList<InvoiceLineItemPriceComponentComponent>(); 2969 for (InvoiceLineItemPriceComponentComponent i : totalPriceComponent) 2970 dst.totalPriceComponent.add(i.copy()); 2971 } 2972 ; 2973 dst.totalNet = totalNet == null ? null : totalNet.copy(); 2974 dst.totalGross = totalGross == null ? null : totalGross.copy(); 2975 dst.paymentTerms = paymentTerms == null ? null : paymentTerms.copy(); 2976 if (note != null) { 2977 dst.note = new ArrayList<Annotation>(); 2978 for (Annotation i : note) 2979 dst.note.add(i.copy()); 2980 } 2981 ; 2982 } 2983 2984 protected Invoice typedCopy() { 2985 return copy(); 2986 } 2987 2988 @Override 2989 public boolean equalsDeep(Base other_) { 2990 if (!super.equalsDeep(other_)) 2991 return false; 2992 if (!(other_ instanceof Invoice)) 2993 return false; 2994 Invoice o = (Invoice) other_; 2995 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2996 && compareDeep(cancelledReason, o.cancelledReason, true) && compareDeep(type, o.type, true) 2997 && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 2998 && compareDeep(date, o.date, true) && compareDeep(participant, o.participant, true) 2999 && compareDeep(issuer, o.issuer, true) && compareDeep(account, o.account, true) 3000 && compareDeep(lineItem, o.lineItem, true) && compareDeep(totalPriceComponent, o.totalPriceComponent, true) 3001 && compareDeep(totalNet, o.totalNet, true) && compareDeep(totalGross, o.totalGross, true) 3002 && compareDeep(paymentTerms, o.paymentTerms, true) && compareDeep(note, o.note, true); 3003 } 3004 3005 @Override 3006 public boolean equalsShallow(Base other_) { 3007 if (!super.equalsShallow(other_)) 3008 return false; 3009 if (!(other_ instanceof Invoice)) 3010 return false; 3011 Invoice o = (Invoice) other_; 3012 return compareValues(status, o.status, true) && compareValues(cancelledReason, o.cancelledReason, true) 3013 && compareValues(date, o.date, true) && compareValues(paymentTerms, o.paymentTerms, true); 3014 } 3015 3016 public boolean isEmpty() { 3017 return super.isEmpty() 3018 && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancelledReason, type, subject, recipient, date, 3019 participant, issuer, account, lineItem, totalPriceComponent, totalNet, totalGross, paymentTerms, note); 3020 } 3021 3022 @Override 3023 public ResourceType getResourceType() { 3024 return ResourceType.Invoice; 3025 } 3026 3027 /** 3028 * Search parameter: <b>date</b> 3029 * <p> 3030 * Description: <b>Invoice date / posting date</b><br> 3031 * Type: <b>date</b><br> 3032 * Path: <b>Invoice.date</b><br> 3033 * </p> 3034 */ 3035 @SearchParamDefinition(name = "date", path = "Invoice.date", description = "Invoice date / posting date", type = "date") 3036 public static final String SP_DATE = "date"; 3037 /** 3038 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3039 * <p> 3040 * Description: <b>Invoice date / posting date</b><br> 3041 * Type: <b>date</b><br> 3042 * Path: <b>Invoice.date</b><br> 3043 * </p> 3044 */ 3045 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3046 SP_DATE); 3047 3048 /** 3049 * Search parameter: <b>identifier</b> 3050 * <p> 3051 * Description: <b>Business Identifier for item</b><br> 3052 * Type: <b>token</b><br> 3053 * Path: <b>Invoice.identifier</b><br> 3054 * </p> 3055 */ 3056 @SearchParamDefinition(name = "identifier", path = "Invoice.identifier", description = "Business Identifier for item", type = "token") 3057 public static final String SP_IDENTIFIER = "identifier"; 3058 /** 3059 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3060 * <p> 3061 * Description: <b>Business Identifier for item</b><br> 3062 * Type: <b>token</b><br> 3063 * Path: <b>Invoice.identifier</b><br> 3064 * </p> 3065 */ 3066 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3067 SP_IDENTIFIER); 3068 3069 /** 3070 * Search parameter: <b>totalgross</b> 3071 * <p> 3072 * Description: <b>Gross total of this Invoice</b><br> 3073 * Type: <b>quantity</b><br> 3074 * Path: <b>Invoice.totalGross</b><br> 3075 * </p> 3076 */ 3077 @SearchParamDefinition(name = "totalgross", path = "Invoice.totalGross", description = "Gross total of this Invoice", type = "quantity") 3078 public static final String SP_TOTALGROSS = "totalgross"; 3079 /** 3080 * <b>Fluent Client</b> search parameter constant for <b>totalgross</b> 3081 * <p> 3082 * Description: <b>Gross total of this Invoice</b><br> 3083 * Type: <b>quantity</b><br> 3084 * Path: <b>Invoice.totalGross</b><br> 3085 * </p> 3086 */ 3087 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALGROSS = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3088 SP_TOTALGROSS); 3089 3090 /** 3091 * Search parameter: <b>subject</b> 3092 * <p> 3093 * Description: <b>Recipient(s) of goods and services</b><br> 3094 * Type: <b>reference</b><br> 3095 * Path: <b>Invoice.subject</b><br> 3096 * </p> 3097 */ 3098 @SearchParamDefinition(name = "subject", path = "Invoice.subject", description = "Recipient(s) of goods and services", type = "reference", providesMembershipIn = { 3099 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3100 public static final String SP_SUBJECT = "subject"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3103 * <p> 3104 * Description: <b>Recipient(s) of goods and services</b><br> 3105 * Type: <b>reference</b><br> 3106 * Path: <b>Invoice.subject</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3110 SP_SUBJECT); 3111 3112 /** 3113 * Constant for fluent queries to be used to add include statements. Specifies 3114 * the path value of "<b>Invoice:subject</b>". 3115 */ 3116 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3117 "Invoice:subject").toLocked(); 3118 3119 /** 3120 * Search parameter: <b>participant-role</b> 3121 * <p> 3122 * Description: <b>Type of involvement in creation of this Invoice</b><br> 3123 * Type: <b>token</b><br> 3124 * Path: <b>Invoice.participant.role</b><br> 3125 * </p> 3126 */ 3127 @SearchParamDefinition(name = "participant-role", path = "Invoice.participant.role", description = "Type of involvement in creation of this Invoice", type = "token") 3128 public static final String SP_PARTICIPANT_ROLE = "participant-role"; 3129 /** 3130 * <b>Fluent Client</b> search parameter constant for <b>participant-role</b> 3131 * <p> 3132 * Description: <b>Type of involvement in creation of this Invoice</b><br> 3133 * Type: <b>token</b><br> 3134 * Path: <b>Invoice.participant.role</b><br> 3135 * </p> 3136 */ 3137 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3138 SP_PARTICIPANT_ROLE); 3139 3140 /** 3141 * Search parameter: <b>type</b> 3142 * <p> 3143 * Description: <b>Type of Invoice</b><br> 3144 * Type: <b>token</b><br> 3145 * Path: <b>Invoice.type</b><br> 3146 * </p> 3147 */ 3148 @SearchParamDefinition(name = "type", path = "Invoice.type", description = "Type of Invoice", type = "token") 3149 public static final String SP_TYPE = "type"; 3150 /** 3151 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3152 * <p> 3153 * Description: <b>Type of Invoice</b><br> 3154 * Type: <b>token</b><br> 3155 * Path: <b>Invoice.type</b><br> 3156 * </p> 3157 */ 3158 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3159 SP_TYPE); 3160 3161 /** 3162 * Search parameter: <b>issuer</b> 3163 * <p> 3164 * Description: <b>Issuing Organization of Invoice</b><br> 3165 * Type: <b>reference</b><br> 3166 * Path: <b>Invoice.issuer</b><br> 3167 * </p> 3168 */ 3169 @SearchParamDefinition(name = "issuer", path = "Invoice.issuer", description = "Issuing Organization of Invoice", type = "reference", target = { 3170 Organization.class }) 3171 public static final String SP_ISSUER = "issuer"; 3172 /** 3173 * <b>Fluent Client</b> search parameter constant for <b>issuer</b> 3174 * <p> 3175 * Description: <b>Issuing Organization of Invoice</b><br> 3176 * Type: <b>reference</b><br> 3177 * Path: <b>Invoice.issuer</b><br> 3178 * </p> 3179 */ 3180 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ISSUER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3181 SP_ISSUER); 3182 3183 /** 3184 * Constant for fluent queries to be used to add include statements. Specifies 3185 * the path value of "<b>Invoice:issuer</b>". 3186 */ 3187 public static final ca.uhn.fhir.model.api.Include INCLUDE_ISSUER = new ca.uhn.fhir.model.api.Include("Invoice:issuer") 3188 .toLocked(); 3189 3190 /** 3191 * Search parameter: <b>participant</b> 3192 * <p> 3193 * Description: <b>Individual who was involved</b><br> 3194 * Type: <b>reference</b><br> 3195 * Path: <b>Invoice.participant.actor</b><br> 3196 * </p> 3197 */ 3198 @SearchParamDefinition(name = "participant", path = "Invoice.participant.actor", description = "Individual who was involved", type = "reference", providesMembershipIn = { 3199 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 3200 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 3201 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3202 public static final String SP_PARTICIPANT = "participant"; 3203 /** 3204 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 3205 * <p> 3206 * Description: <b>Individual who was involved</b><br> 3207 * Type: <b>reference</b><br> 3208 * Path: <b>Invoice.participant.actor</b><br> 3209 * </p> 3210 */ 3211 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3212 SP_PARTICIPANT); 3213 3214 /** 3215 * Constant for fluent queries to be used to add include statements. Specifies 3216 * the path value of "<b>Invoice:participant</b>". 3217 */ 3218 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include( 3219 "Invoice:participant").toLocked(); 3220 3221 /** 3222 * Search parameter: <b>totalnet</b> 3223 * <p> 3224 * Description: <b>Net total of this Invoice</b><br> 3225 * Type: <b>quantity</b><br> 3226 * Path: <b>Invoice.totalNet</b><br> 3227 * </p> 3228 */ 3229 @SearchParamDefinition(name = "totalnet", path = "Invoice.totalNet", description = "Net total of this Invoice", type = "quantity") 3230 public static final String SP_TOTALNET = "totalnet"; 3231 /** 3232 * <b>Fluent Client</b> search parameter constant for <b>totalnet</b> 3233 * <p> 3234 * Description: <b>Net total of this Invoice</b><br> 3235 * Type: <b>quantity</b><br> 3236 * Path: <b>Invoice.totalNet</b><br> 3237 * </p> 3238 */ 3239 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALNET = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3240 SP_TOTALNET); 3241 3242 /** 3243 * Search parameter: <b>patient</b> 3244 * <p> 3245 * Description: <b>Recipient(s) of goods and services</b><br> 3246 * Type: <b>reference</b><br> 3247 * Path: <b>Invoice.subject</b><br> 3248 * </p> 3249 */ 3250 @SearchParamDefinition(name = "patient", path = "Invoice.subject.where(resolve() is Patient)", description = "Recipient(s) of goods and services", type = "reference", providesMembershipIn = { 3251 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3252 public static final String SP_PATIENT = "patient"; 3253 /** 3254 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3255 * <p> 3256 * Description: <b>Recipient(s) of goods and services</b><br> 3257 * Type: <b>reference</b><br> 3258 * Path: <b>Invoice.subject</b><br> 3259 * </p> 3260 */ 3261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3262 SP_PATIENT); 3263 3264 /** 3265 * Constant for fluent queries to be used to add include statements. Specifies 3266 * the path value of "<b>Invoice:patient</b>". 3267 */ 3268 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3269 "Invoice:patient").toLocked(); 3270 3271 /** 3272 * Search parameter: <b>recipient</b> 3273 * <p> 3274 * Description: <b>Recipient of this invoice</b><br> 3275 * Type: <b>reference</b><br> 3276 * Path: <b>Invoice.recipient</b><br> 3277 * </p> 3278 */ 3279 @SearchParamDefinition(name = "recipient", path = "Invoice.recipient", description = "Recipient of this invoice", type = "reference", providesMembershipIn = { 3280 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3281 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 3282 Patient.class, RelatedPerson.class }) 3283 public static final String SP_RECIPIENT = "recipient"; 3284 /** 3285 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 3286 * <p> 3287 * Description: <b>Recipient of this invoice</b><br> 3288 * Type: <b>reference</b><br> 3289 * Path: <b>Invoice.recipient</b><br> 3290 * </p> 3291 */ 3292 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3293 SP_RECIPIENT); 3294 3295 /** 3296 * Constant for fluent queries to be used to add include statements. Specifies 3297 * the path value of "<b>Invoice:recipient</b>". 3298 */ 3299 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include( 3300 "Invoice:recipient").toLocked(); 3301 3302 /** 3303 * Search parameter: <b>account</b> 3304 * <p> 3305 * Description: <b>Account that is being balanced</b><br> 3306 * Type: <b>reference</b><br> 3307 * Path: <b>Invoice.account</b><br> 3308 * </p> 3309 */ 3310 @SearchParamDefinition(name = "account", path = "Invoice.account", description = "Account that is being balanced", type = "reference", target = { 3311 Account.class }) 3312 public static final String SP_ACCOUNT = "account"; 3313 /** 3314 * <b>Fluent Client</b> search parameter constant for <b>account</b> 3315 * <p> 3316 * Description: <b>Account that is being balanced</b><br> 3317 * Type: <b>reference</b><br> 3318 * Path: <b>Invoice.account</b><br> 3319 * </p> 3320 */ 3321 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3322 SP_ACCOUNT); 3323 3324 /** 3325 * Constant for fluent queries to be used to add include statements. Specifies 3326 * the path value of "<b>Invoice:account</b>". 3327 */ 3328 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include( 3329 "Invoice:account").toLocked(); 3330 3331 /** 3332 * Search parameter: <b>status</b> 3333 * <p> 3334 * Description: <b>draft | issued | balanced | cancelled | 3335 * entered-in-error</b><br> 3336 * Type: <b>token</b><br> 3337 * Path: <b>Invoice.status</b><br> 3338 * </p> 3339 */ 3340 @SearchParamDefinition(name = "status", path = "Invoice.status", description = "draft | issued | balanced | cancelled | entered-in-error", type = "token") 3341 public static final String SP_STATUS = "status"; 3342 /** 3343 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3344 * <p> 3345 * Description: <b>draft | issued | balanced | cancelled | 3346 * entered-in-error</b><br> 3347 * Type: <b>token</b><br> 3348 * Path: <b>Invoice.status</b><br> 3349 * </p> 3350 */ 3351 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3352 SP_STATUS); 3353 3354}