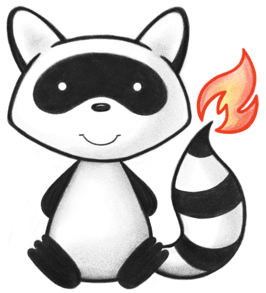
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A list is a curated collection of resources. 049 */ 050@ResourceDef(name = "List", profile = "http://hl7.org/fhir/StructureDefinition/List") 051public class ListResource extends DomainResource { 052 053 public enum ListStatus { 054 /** 055 * The list is considered to be an active part of the patient's record. 056 */ 057 CURRENT, 058 /** 059 * The list is "old" and should no longer be considered accurate or relevant. 060 */ 061 RETIRED, 062 /** 063 * The list was never accurate. It is retained for medico-legal purposes only. 064 */ 065 ENTEREDINERROR, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 071 public static ListStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("current".equals(codeString)) 075 return CURRENT; 076 if ("retired".equals(codeString)) 077 return RETIRED; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 084 } 085 086 public String toCode() { 087 switch (this) { 088 case CURRENT: 089 return "current"; 090 case RETIRED: 091 return "retired"; 092 case ENTEREDINERROR: 093 return "entered-in-error"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getSystem() { 102 switch (this) { 103 case CURRENT: 104 return "http://hl7.org/fhir/list-status"; 105 case RETIRED: 106 return "http://hl7.org/fhir/list-status"; 107 case ENTEREDINERROR: 108 return "http://hl7.org/fhir/list-status"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getDefinition() { 117 switch (this) { 118 case CURRENT: 119 return "The list is considered to be an active part of the patient's record."; 120 case RETIRED: 121 return "The list is \"old\" and should no longer be considered accurate or relevant."; 122 case ENTEREDINERROR: 123 return "The list was never accurate. It is retained for medico-legal purposes only."; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getDisplay() { 132 switch (this) { 133 case CURRENT: 134 return "Current"; 135 case RETIRED: 136 return "Retired"; 137 case ENTEREDINERROR: 138 return "Entered In Error"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 } 146 147 public static class ListStatusEnumFactory implements EnumFactory<ListStatus> { 148 public ListStatus fromCode(String codeString) throws IllegalArgumentException { 149 if (codeString == null || "".equals(codeString)) 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("current".equals(codeString)) 153 return ListStatus.CURRENT; 154 if ("retired".equals(codeString)) 155 return ListStatus.RETIRED; 156 if ("entered-in-error".equals(codeString)) 157 return ListStatus.ENTEREDINERROR; 158 throw new IllegalArgumentException("Unknown ListStatus code '" + codeString + "'"); 159 } 160 161 public Enumeration<ListStatus> fromType(PrimitiveType<?> code) throws FHIRException { 162 if (code == null) 163 return null; 164 if (code.isEmpty()) 165 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 166 String codeString = code.asStringValue(); 167 if (codeString == null || "".equals(codeString)) 168 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 169 if ("current".equals(codeString)) 170 return new Enumeration<ListStatus>(this, ListStatus.CURRENT, code); 171 if ("retired".equals(codeString)) 172 return new Enumeration<ListStatus>(this, ListStatus.RETIRED, code); 173 if ("entered-in-error".equals(codeString)) 174 return new Enumeration<ListStatus>(this, ListStatus.ENTEREDINERROR, code); 175 throw new FHIRException("Unknown ListStatus code '" + codeString + "'"); 176 } 177 178 public String toCode(ListStatus code) { 179 if (code == ListStatus.CURRENT) 180 return "current"; 181 if (code == ListStatus.RETIRED) 182 return "retired"; 183 if (code == ListStatus.ENTEREDINERROR) 184 return "entered-in-error"; 185 return "?"; 186 } 187 188 public String toSystem(ListStatus code) { 189 return code.getSystem(); 190 } 191 } 192 193 public enum ListMode { 194 /** 195 * This list is the master list, maintained in an ongoing fashion with regular 196 * updates as the real world list it is tracking changes. 197 */ 198 WORKING, 199 /** 200 * This list was prepared as a snapshot. It should not be assumed to be current. 201 */ 202 SNAPSHOT, 203 /** 204 * A point-in-time list that shows what changes have been made or recommended. 205 * E.g. a discharge medication list showing what was added and removed during an 206 * encounter. 207 */ 208 CHANGES, 209 /** 210 * added to help the parsers with the generic types 211 */ 212 NULL; 213 214 public static ListMode fromCode(String codeString) throws FHIRException { 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("working".equals(codeString)) 218 return WORKING; 219 if ("snapshot".equals(codeString)) 220 return SNAPSHOT; 221 if ("changes".equals(codeString)) 222 return CHANGES; 223 if (Configuration.isAcceptInvalidEnums()) 224 return null; 225 else 226 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 227 } 228 229 public String toCode() { 230 switch (this) { 231 case WORKING: 232 return "working"; 233 case SNAPSHOT: 234 return "snapshot"; 235 case CHANGES: 236 return "changes"; 237 case NULL: 238 return null; 239 default: 240 return "?"; 241 } 242 } 243 244 public String getSystem() { 245 switch (this) { 246 case WORKING: 247 return "http://hl7.org/fhir/list-mode"; 248 case SNAPSHOT: 249 return "http://hl7.org/fhir/list-mode"; 250 case CHANGES: 251 return "http://hl7.org/fhir/list-mode"; 252 case NULL: 253 return null; 254 default: 255 return "?"; 256 } 257 } 258 259 public String getDefinition() { 260 switch (this) { 261 case WORKING: 262 return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes."; 263 case SNAPSHOT: 264 return "This list was prepared as a snapshot. It should not be assumed to be current."; 265 case CHANGES: 266 return "A point-in-time list that shows what changes have been made or recommended. E.g. a discharge medication list showing what was added and removed during an encounter."; 267 case NULL: 268 return null; 269 default: 270 return "?"; 271 } 272 } 273 274 public String getDisplay() { 275 switch (this) { 276 case WORKING: 277 return "Working List"; 278 case SNAPSHOT: 279 return "Snapshot List"; 280 case CHANGES: 281 return "Change List"; 282 case NULL: 283 return null; 284 default: 285 return "?"; 286 } 287 } 288 } 289 290 public static class ListModeEnumFactory implements EnumFactory<ListMode> { 291 public ListMode fromCode(String codeString) throws IllegalArgumentException { 292 if (codeString == null || "".equals(codeString)) 293 if (codeString == null || "".equals(codeString)) 294 return null; 295 if ("working".equals(codeString)) 296 return ListMode.WORKING; 297 if ("snapshot".equals(codeString)) 298 return ListMode.SNAPSHOT; 299 if ("changes".equals(codeString)) 300 return ListMode.CHANGES; 301 throw new IllegalArgumentException("Unknown ListMode code '" + codeString + "'"); 302 } 303 304 public Enumeration<ListMode> fromType(PrimitiveType<?> code) throws FHIRException { 305 if (code == null) 306 return null; 307 if (code.isEmpty()) 308 return new Enumeration<ListMode>(this, ListMode.NULL, code); 309 String codeString = code.asStringValue(); 310 if (codeString == null || "".equals(codeString)) 311 return new Enumeration<ListMode>(this, ListMode.NULL, code); 312 if ("working".equals(codeString)) 313 return new Enumeration<ListMode>(this, ListMode.WORKING, code); 314 if ("snapshot".equals(codeString)) 315 return new Enumeration<ListMode>(this, ListMode.SNAPSHOT, code); 316 if ("changes".equals(codeString)) 317 return new Enumeration<ListMode>(this, ListMode.CHANGES, code); 318 throw new FHIRException("Unknown ListMode code '" + codeString + "'"); 319 } 320 321 public String toCode(ListMode code) { 322 if (code == ListMode.WORKING) 323 return "working"; 324 if (code == ListMode.SNAPSHOT) 325 return "snapshot"; 326 if (code == ListMode.CHANGES) 327 return "changes"; 328 return "?"; 329 } 330 331 public String toSystem(ListMode code) { 332 return code.getSystem(); 333 } 334 } 335 336 @Block() 337 public static class ListEntryComponent extends BackboneElement implements IBaseBackboneElement { 338 /** 339 * The flag allows the system constructing the list to indicate the role and 340 * significance of the item in the list. 341 */ 342 @Child(name = "flag", type = { 343 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 344 @Description(shortDefinition = "Status/Workflow information about this item", formalDefinition = "The flag allows the system constructing the list to indicate the role and significance of the item in the list.") 345 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-item-flag") 346 protected CodeableConcept flag; 347 348 /** 349 * True if this item is marked as deleted in the list. 350 */ 351 @Child(name = "deleted", type = { 352 BooleanType.class }, order = 2, min = 0, max = 1, modifier = true, summary = false) 353 @Description(shortDefinition = "If this item is actually marked as deleted", formalDefinition = "True if this item is marked as deleted in the list.") 354 protected BooleanType deleted; 355 356 /** 357 * When this item was added to the list. 358 */ 359 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 360 @Description(shortDefinition = "When item added to list", formalDefinition = "When this item was added to the list.") 361 protected DateTimeType date; 362 363 /** 364 * A reference to the actual resource from which data was derived. 365 */ 366 @Child(name = "item", type = { Reference.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 367 @Description(shortDefinition = "Actual entry", formalDefinition = "A reference to the actual resource from which data was derived.") 368 protected Reference item; 369 370 /** 371 * The actual object that is the target of the reference (A reference to the 372 * actual resource from which data was derived.) 373 */ 374 protected Resource itemTarget; 375 376 private static final long serialVersionUID = -758164425L; 377 378 /** 379 * Constructor 380 */ 381 public ListEntryComponent() { 382 super(); 383 } 384 385 /** 386 * Constructor 387 */ 388 public ListEntryComponent(Reference item) { 389 super(); 390 this.item = item; 391 } 392 393 /** 394 * @return {@link #flag} (The flag allows the system constructing the list to 395 * indicate the role and significance of the item in the list.) 396 */ 397 public CodeableConcept getFlag() { 398 if (this.flag == null) 399 if (Configuration.errorOnAutoCreate()) 400 throw new Error("Attempt to auto-create ListEntryComponent.flag"); 401 else if (Configuration.doAutoCreate()) 402 this.flag = new CodeableConcept(); // cc 403 return this.flag; 404 } 405 406 public boolean hasFlag() { 407 return this.flag != null && !this.flag.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #flag} (The flag allows the system constructing the list 412 * to indicate the role and significance of the item in the list.) 413 */ 414 public ListEntryComponent setFlag(CodeableConcept value) { 415 this.flag = value; 416 return this; 417 } 418 419 /** 420 * @return {@link #deleted} (True if this item is marked as deleted in the 421 * list.). This is the underlying object with id, value and extensions. 422 * The accessor "getDeleted" gives direct access to the value 423 */ 424 public BooleanType getDeletedElement() { 425 if (this.deleted == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create ListEntryComponent.deleted"); 428 else if (Configuration.doAutoCreate()) 429 this.deleted = new BooleanType(); // bb 430 return this.deleted; 431 } 432 433 public boolean hasDeletedElement() { 434 return this.deleted != null && !this.deleted.isEmpty(); 435 } 436 437 public boolean hasDeleted() { 438 return this.deleted != null && !this.deleted.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #deleted} (True if this item is marked as deleted in the 443 * list.). This is the underlying object with id, value and 444 * extensions. The accessor "getDeleted" gives direct access to the 445 * value 446 */ 447 public ListEntryComponent setDeletedElement(BooleanType value) { 448 this.deleted = value; 449 return this; 450 } 451 452 /** 453 * @return True if this item is marked as deleted in the list. 454 */ 455 public boolean getDeleted() { 456 return this.deleted == null || this.deleted.isEmpty() ? false : this.deleted.getValue(); 457 } 458 459 /** 460 * @param value True if this item is marked as deleted in the list. 461 */ 462 public ListEntryComponent setDeleted(boolean value) { 463 if (this.deleted == null) 464 this.deleted = new BooleanType(); 465 this.deleted.setValue(value); 466 return this; 467 } 468 469 /** 470 * @return {@link #date} (When this item was added to the list.). This is the 471 * underlying object with id, value and extensions. The accessor 472 * "getDate" gives direct access to the value 473 */ 474 public DateTimeType getDateElement() { 475 if (this.date == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create ListEntryComponent.date"); 478 else if (Configuration.doAutoCreate()) 479 this.date = new DateTimeType(); // bb 480 return this.date; 481 } 482 483 public boolean hasDateElement() { 484 return this.date != null && !this.date.isEmpty(); 485 } 486 487 public boolean hasDate() { 488 return this.date != null && !this.date.isEmpty(); 489 } 490 491 /** 492 * @param value {@link #date} (When this item was added to the list.). This is 493 * the underlying object with id, value and extensions. The 494 * accessor "getDate" gives direct access to the value 495 */ 496 public ListEntryComponent setDateElement(DateTimeType value) { 497 this.date = value; 498 return this; 499 } 500 501 /** 502 * @return When this item was added to the list. 503 */ 504 public Date getDate() { 505 return this.date == null ? null : this.date.getValue(); 506 } 507 508 /** 509 * @param value When this item was added to the list. 510 */ 511 public ListEntryComponent setDate(Date value) { 512 if (value == null) 513 this.date = null; 514 else { 515 if (this.date == null) 516 this.date = new DateTimeType(); 517 this.date.setValue(value); 518 } 519 return this; 520 } 521 522 /** 523 * @return {@link #item} (A reference to the actual resource from which data was 524 * derived.) 525 */ 526 public Reference getItem() { 527 if (this.item == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create ListEntryComponent.item"); 530 else if (Configuration.doAutoCreate()) 531 this.item = new Reference(); // cc 532 return this.item; 533 } 534 535 public boolean hasItem() { 536 return this.item != null && !this.item.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #item} (A reference to the actual resource from which 541 * data was derived.) 542 */ 543 public ListEntryComponent setItem(Reference value) { 544 this.item = value; 545 return this; 546 } 547 548 /** 549 * @return {@link #item} The actual object that is the target of the reference. 550 * The reference library doesn't populate this, but you can use it to 551 * hold the resource if you resolve it. (A reference to the actual 552 * resource from which data was derived.) 553 */ 554 public Resource getItemTarget() { 555 return this.itemTarget; 556 } 557 558 /** 559 * @param value {@link #item} The actual object that is the target of the 560 * reference. The reference library doesn't use these, but you can 561 * use it to hold the resource if you resolve it. (A reference to 562 * the actual resource from which data was derived.) 563 */ 564 public ListEntryComponent setItemTarget(Resource value) { 565 this.itemTarget = value; 566 return this; 567 } 568 569 protected void listChildren(List<Property> children) { 570 super.listChildren(children); 571 children.add(new Property("flag", "CodeableConcept", 572 "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 573 0, 1, flag)); 574 children.add( 575 new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted)); 576 children.add(new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date)); 577 children.add(new Property("item", "Reference(Any)", 578 "A reference to the actual resource from which data was derived.", 0, 1, item)); 579 } 580 581 @Override 582 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 583 switch (_hash) { 584 case 3145580: 585 /* flag */ return new Property("flag", "CodeableConcept", 586 "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 587 0, 1, flag); 588 case 1550463001: 589 /* deleted */ return new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 590 0, 1, deleted); 591 case 3076014: 592 /* date */ return new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date); 593 case 3242771: 594 /* item */ return new Property("item", "Reference(Any)", 595 "A reference to the actual resource from which data was derived.", 0, 1, item); 596 default: 597 return super.getNamedProperty(_hash, _name, _checkValid); 598 } 599 600 } 601 602 @Override 603 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 604 switch (hash) { 605 case 3145580: 606 /* flag */ return this.flag == null ? new Base[0] : new Base[] { this.flag }; // CodeableConcept 607 case 1550463001: 608 /* deleted */ return this.deleted == null ? new Base[0] : new Base[] { this.deleted }; // BooleanType 609 case 3076014: 610 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 611 case 3242771: 612 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Reference 613 default: 614 return super.getProperty(hash, name, checkValid); 615 } 616 617 } 618 619 @Override 620 public Base setProperty(int hash, String name, Base value) throws FHIRException { 621 switch (hash) { 622 case 3145580: // flag 623 this.flag = castToCodeableConcept(value); // CodeableConcept 624 return value; 625 case 1550463001: // deleted 626 this.deleted = castToBoolean(value); // BooleanType 627 return value; 628 case 3076014: // date 629 this.date = castToDateTime(value); // DateTimeType 630 return value; 631 case 3242771: // item 632 this.item = castToReference(value); // Reference 633 return value; 634 default: 635 return super.setProperty(hash, name, value); 636 } 637 638 } 639 640 @Override 641 public Base setProperty(String name, Base value) throws FHIRException { 642 if (name.equals("flag")) { 643 this.flag = castToCodeableConcept(value); // CodeableConcept 644 } else if (name.equals("deleted")) { 645 this.deleted = castToBoolean(value); // BooleanType 646 } else if (name.equals("date")) { 647 this.date = castToDateTime(value); // DateTimeType 648 } else if (name.equals("item")) { 649 this.item = castToReference(value); // Reference 650 } else 651 return super.setProperty(name, value); 652 return value; 653 } 654 655 @Override 656 public Base makeProperty(int hash, String name) throws FHIRException { 657 switch (hash) { 658 case 3145580: 659 return getFlag(); 660 case 1550463001: 661 return getDeletedElement(); 662 case 3076014: 663 return getDateElement(); 664 case 3242771: 665 return getItem(); 666 default: 667 return super.makeProperty(hash, name); 668 } 669 670 } 671 672 @Override 673 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 674 switch (hash) { 675 case 3145580: 676 /* flag */ return new String[] { "CodeableConcept" }; 677 case 1550463001: 678 /* deleted */ return new String[] { "boolean" }; 679 case 3076014: 680 /* date */ return new String[] { "dateTime" }; 681 case 3242771: 682 /* item */ return new String[] { "Reference" }; 683 default: 684 return super.getTypesForProperty(hash, name); 685 } 686 687 } 688 689 @Override 690 public Base addChild(String name) throws FHIRException { 691 if (name.equals("flag")) { 692 this.flag = new CodeableConcept(); 693 return this.flag; 694 } else if (name.equals("deleted")) { 695 throw new FHIRException("Cannot call addChild on a singleton property List.deleted"); 696 } else if (name.equals("date")) { 697 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 698 } else if (name.equals("item")) { 699 this.item = new Reference(); 700 return this.item; 701 } else 702 return super.addChild(name); 703 } 704 705 public ListEntryComponent copy() { 706 ListEntryComponent dst = new ListEntryComponent(); 707 copyValues(dst); 708 return dst; 709 } 710 711 public void copyValues(ListEntryComponent dst) { 712 super.copyValues(dst); 713 dst.flag = flag == null ? null : flag.copy(); 714 dst.deleted = deleted == null ? null : deleted.copy(); 715 dst.date = date == null ? null : date.copy(); 716 dst.item = item == null ? null : item.copy(); 717 } 718 719 @Override 720 public boolean equalsDeep(Base other_) { 721 if (!super.equalsDeep(other_)) 722 return false; 723 if (!(other_ instanceof ListEntryComponent)) 724 return false; 725 ListEntryComponent o = (ListEntryComponent) other_; 726 return compareDeep(flag, o.flag, true) && compareDeep(deleted, o.deleted, true) && compareDeep(date, o.date, true) 727 && compareDeep(item, o.item, true); 728 } 729 730 @Override 731 public boolean equalsShallow(Base other_) { 732 if (!super.equalsShallow(other_)) 733 return false; 734 if (!(other_ instanceof ListEntryComponent)) 735 return false; 736 ListEntryComponent o = (ListEntryComponent) other_; 737 return compareValues(deleted, o.deleted, true) && compareValues(date, o.date, true); 738 } 739 740 public boolean isEmpty() { 741 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(flag, deleted, date, item); 742 } 743 744 public String fhirType() { 745 return "List.entry"; 746 747 } 748 749 } 750 751 /** 752 * Identifier for the List assigned for business purposes outside the context of 753 * FHIR. 754 */ 755 @Child(name = "identifier", type = { 756 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 757 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier for the List assigned for business purposes outside the context of FHIR.") 758 protected List<Identifier> identifier; 759 760 /** 761 * Indicates the current state of this list. 762 */ 763 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 764 @Description(shortDefinition = "current | retired | entered-in-error", formalDefinition = "Indicates the current state of this list.") 765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-status") 766 protected Enumeration<ListStatus> status; 767 768 /** 769 * How this list was prepared - whether it is a working list that is suitable 770 * for being maintained on an ongoing basis, or if it represents a snapshot of a 771 * list of items from another source, or whether it is a prepared list where 772 * items may be marked as added, modified or deleted. 773 */ 774 @Child(name = "mode", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 775 @Description(shortDefinition = "working | snapshot | changes", formalDefinition = "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.") 776 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-mode") 777 protected Enumeration<ListMode> mode; 778 779 /** 780 * A label for the list assigned by the author. 781 */ 782 @Child(name = "title", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 783 @Description(shortDefinition = "Descriptive name for the list", formalDefinition = "A label for the list assigned by the author.") 784 protected StringType title; 785 786 /** 787 * This code defines the purpose of the list - why it was created. 788 */ 789 @Child(name = "code", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 790 @Description(shortDefinition = "What the purpose of this list is", formalDefinition = "This code defines the purpose of the list - why it was created.") 791 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-example-codes") 792 protected CodeableConcept code; 793 794 /** 795 * The common subject (or patient) of the resources that are in the list if 796 * there is one. 797 */ 798 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 799 Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 800 @Description(shortDefinition = "If all resources have the same subject", formalDefinition = "The common subject (or patient) of the resources that are in the list if there is one.") 801 protected Reference subject; 802 803 /** 804 * The actual object that is the target of the reference (The common subject (or 805 * patient) of the resources that are in the list if there is one.) 806 */ 807 protected Resource subjectTarget; 808 809 /** 810 * The encounter that is the context in which this list was created. 811 */ 812 @Child(name = "encounter", type = { Encounter.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 813 @Description(shortDefinition = "Context in which list created", formalDefinition = "The encounter that is the context in which this list was created.") 814 protected Reference encounter; 815 816 /** 817 * The actual object that is the target of the reference (The encounter that is 818 * the context in which this list was created.) 819 */ 820 protected Encounter encounterTarget; 821 822 /** 823 * The date that the list was prepared. 824 */ 825 @Child(name = "date", type = { DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 826 @Description(shortDefinition = "When the list was prepared", formalDefinition = "The date that the list was prepared.") 827 protected DateTimeType date; 828 829 /** 830 * The entity responsible for deciding what the contents of the list were. Where 831 * the list was created by a human, this is the same as the author of the list. 832 */ 833 @Child(name = "source", type = { Practitioner.class, PractitionerRole.class, Patient.class, 834 Device.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 835 @Description(shortDefinition = "Who and/or what defined the list contents (aka Author)", formalDefinition = "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.") 836 protected Reference source; 837 838 /** 839 * The actual object that is the target of the reference (The entity responsible 840 * for deciding what the contents of the list were. Where the list was created 841 * by a human, this is the same as the author of the list.) 842 */ 843 protected Resource sourceTarget; 844 845 /** 846 * What order applies to the items in the list. 847 */ 848 @Child(name = "orderedBy", type = { 849 CodeableConcept.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 850 @Description(shortDefinition = "What order the list has", formalDefinition = "What order applies to the items in the list.") 851 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-order") 852 protected CodeableConcept orderedBy; 853 854 /** 855 * Comments that apply to the overall list. 856 */ 857 @Child(name = "note", type = { 858 Annotation.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 859 @Description(shortDefinition = "Comments about the list", formalDefinition = "Comments that apply to the overall list.") 860 protected List<Annotation> note; 861 862 /** 863 * Entries in this list. 864 */ 865 @Child(name = "entry", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 866 @Description(shortDefinition = "Entries in the list", formalDefinition = "Entries in this list.") 867 protected List<ListEntryComponent> entry; 868 869 /** 870 * If the list is empty, why the list is empty. 871 */ 872 @Child(name = "emptyReason", type = { 873 CodeableConcept.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 874 @Description(shortDefinition = "Why list is empty", formalDefinition = "If the list is empty, why the list is empty.") 875 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/list-empty-reason") 876 protected CodeableConcept emptyReason; 877 878 private static final long serialVersionUID = 2071342704L; 879 880 /** 881 * Constructor 882 */ 883 public ListResource() { 884 super(); 885 } 886 887 /** 888 * Constructor 889 */ 890 public ListResource(Enumeration<ListStatus> status, Enumeration<ListMode> mode) { 891 super(); 892 this.status = status; 893 this.mode = mode; 894 } 895 896 /** 897 * @return {@link #identifier} (Identifier for the List assigned for business 898 * purposes outside the context of FHIR.) 899 */ 900 public List<Identifier> getIdentifier() { 901 if (this.identifier == null) 902 this.identifier = new ArrayList<Identifier>(); 903 return this.identifier; 904 } 905 906 /** 907 * @return Returns a reference to <code>this</code> for easy method chaining 908 */ 909 public ListResource setIdentifier(List<Identifier> theIdentifier) { 910 this.identifier = theIdentifier; 911 return this; 912 } 913 914 public boolean hasIdentifier() { 915 if (this.identifier == null) 916 return false; 917 for (Identifier item : this.identifier) 918 if (!item.isEmpty()) 919 return true; 920 return false; 921 } 922 923 public Identifier addIdentifier() { // 3 924 Identifier t = new Identifier(); 925 if (this.identifier == null) 926 this.identifier = new ArrayList<Identifier>(); 927 this.identifier.add(t); 928 return t; 929 } 930 931 public ListResource addIdentifier(Identifier t) { // 3 932 if (t == null) 933 return this; 934 if (this.identifier == null) 935 this.identifier = new ArrayList<Identifier>(); 936 this.identifier.add(t); 937 return this; 938 } 939 940 /** 941 * @return The first repetition of repeating field {@link #identifier}, creating 942 * it if it does not already exist 943 */ 944 public Identifier getIdentifierFirstRep() { 945 if (getIdentifier().isEmpty()) { 946 addIdentifier(); 947 } 948 return getIdentifier().get(0); 949 } 950 951 /** 952 * @return {@link #status} (Indicates the current state of this list.). This is 953 * the underlying object with id, value and extensions. The accessor 954 * "getStatus" gives direct access to the value 955 */ 956 public Enumeration<ListStatus> getStatusElement() { 957 if (this.status == null) 958 if (Configuration.errorOnAutoCreate()) 959 throw new Error("Attempt to auto-create List.status"); 960 else if (Configuration.doAutoCreate()) 961 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); // bb 962 return this.status; 963 } 964 965 public boolean hasStatusElement() { 966 return this.status != null && !this.status.isEmpty(); 967 } 968 969 public boolean hasStatus() { 970 return this.status != null && !this.status.isEmpty(); 971 } 972 973 /** 974 * @param value {@link #status} (Indicates the current state of this list.). 975 * This is the underlying object with id, value and extensions. The 976 * accessor "getStatus" gives direct access to the value 977 */ 978 public ListResource setStatusElement(Enumeration<ListStatus> value) { 979 this.status = value; 980 return this; 981 } 982 983 /** 984 * @return Indicates the current state of this list. 985 */ 986 public ListStatus getStatus() { 987 return this.status == null ? null : this.status.getValue(); 988 } 989 990 /** 991 * @param value Indicates the current state of this list. 992 */ 993 public ListResource setStatus(ListStatus value) { 994 if (this.status == null) 995 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); 996 this.status.setValue(value); 997 return this; 998 } 999 1000 /** 1001 * @return {@link #mode} (How this list was prepared - whether it is a working 1002 * list that is suitable for being maintained on an ongoing basis, or if 1003 * it represents a snapshot of a list of items from another source, or 1004 * whether it is a prepared list where items may be marked as added, 1005 * modified or deleted.). This is the underlying object with id, value 1006 * and extensions. The accessor "getMode" gives direct access to the 1007 * value 1008 */ 1009 public Enumeration<ListMode> getModeElement() { 1010 if (this.mode == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create List.mode"); 1013 else if (Configuration.doAutoCreate()) 1014 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 1015 return this.mode; 1016 } 1017 1018 public boolean hasModeElement() { 1019 return this.mode != null && !this.mode.isEmpty(); 1020 } 1021 1022 public boolean hasMode() { 1023 return this.mode != null && !this.mode.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #mode} (How this list was prepared - whether it is a 1028 * working list that is suitable for being maintained on an ongoing 1029 * basis, or if it represents a snapshot of a list of items from 1030 * another source, or whether it is a prepared list where items may 1031 * be marked as added, modified or deleted.). This is the 1032 * underlying object with id, value and extensions. The accessor 1033 * "getMode" gives direct access to the value 1034 */ 1035 public ListResource setModeElement(Enumeration<ListMode> value) { 1036 this.mode = value; 1037 return this; 1038 } 1039 1040 /** 1041 * @return How this list was prepared - whether it is a working list that is 1042 * suitable for being maintained on an ongoing basis, or if it 1043 * represents a snapshot of a list of items from another source, or 1044 * whether it is a prepared list where items may be marked as added, 1045 * modified or deleted. 1046 */ 1047 public ListMode getMode() { 1048 return this.mode == null ? null : this.mode.getValue(); 1049 } 1050 1051 /** 1052 * @param value How this list was prepared - whether it is a working list that 1053 * is suitable for being maintained on an ongoing basis, or if it 1054 * represents a snapshot of a list of items from another source, or 1055 * whether it is a prepared list where items may be marked as 1056 * added, modified or deleted. 1057 */ 1058 public ListResource setMode(ListMode value) { 1059 if (this.mode == null) 1060 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 1061 this.mode.setValue(value); 1062 return this; 1063 } 1064 1065 /** 1066 * @return {@link #title} (A label for the list assigned by the author.). This 1067 * is the underlying object with id, value and extensions. The accessor 1068 * "getTitle" gives direct access to the value 1069 */ 1070 public StringType getTitleElement() { 1071 if (this.title == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create List.title"); 1074 else if (Configuration.doAutoCreate()) 1075 this.title = new StringType(); // bb 1076 return this.title; 1077 } 1078 1079 public boolean hasTitleElement() { 1080 return this.title != null && !this.title.isEmpty(); 1081 } 1082 1083 public boolean hasTitle() { 1084 return this.title != null && !this.title.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #title} (A label for the list assigned by the author.). 1089 * This is the underlying object with id, value and extensions. The 1090 * accessor "getTitle" gives direct access to the value 1091 */ 1092 public ListResource setTitleElement(StringType value) { 1093 this.title = value; 1094 return this; 1095 } 1096 1097 /** 1098 * @return A label for the list assigned by the author. 1099 */ 1100 public String getTitle() { 1101 return this.title == null ? null : this.title.getValue(); 1102 } 1103 1104 /** 1105 * @param value A label for the list assigned by the author. 1106 */ 1107 public ListResource setTitle(String value) { 1108 if (Utilities.noString(value)) 1109 this.title = null; 1110 else { 1111 if (this.title == null) 1112 this.title = new StringType(); 1113 this.title.setValue(value); 1114 } 1115 return this; 1116 } 1117 1118 /** 1119 * @return {@link #code} (This code defines the purpose of the list - why it was 1120 * created.) 1121 */ 1122 public CodeableConcept getCode() { 1123 if (this.code == null) 1124 if (Configuration.errorOnAutoCreate()) 1125 throw new Error("Attempt to auto-create List.code"); 1126 else if (Configuration.doAutoCreate()) 1127 this.code = new CodeableConcept(); // cc 1128 return this.code; 1129 } 1130 1131 public boolean hasCode() { 1132 return this.code != null && !this.code.isEmpty(); 1133 } 1134 1135 /** 1136 * @param value {@link #code} (This code defines the purpose of the list - why 1137 * it was created.) 1138 */ 1139 public ListResource setCode(CodeableConcept value) { 1140 this.code = value; 1141 return this; 1142 } 1143 1144 /** 1145 * @return {@link #subject} (The common subject (or patient) of the resources 1146 * that are in the list if there is one.) 1147 */ 1148 public Reference getSubject() { 1149 if (this.subject == null) 1150 if (Configuration.errorOnAutoCreate()) 1151 throw new Error("Attempt to auto-create List.subject"); 1152 else if (Configuration.doAutoCreate()) 1153 this.subject = new Reference(); // cc 1154 return this.subject; 1155 } 1156 1157 public boolean hasSubject() { 1158 return this.subject != null && !this.subject.isEmpty(); 1159 } 1160 1161 /** 1162 * @param value {@link #subject} (The common subject (or patient) of the 1163 * resources that are in the list if there is one.) 1164 */ 1165 public ListResource setSubject(Reference value) { 1166 this.subject = value; 1167 return this; 1168 } 1169 1170 /** 1171 * @return {@link #subject} The actual object that is the target of the 1172 * reference. The reference library doesn't populate this, but you can 1173 * use it to hold the resource if you resolve it. (The common subject 1174 * (or patient) of the resources that are in the list if there is one.) 1175 */ 1176 public Resource getSubjectTarget() { 1177 return this.subjectTarget; 1178 } 1179 1180 /** 1181 * @param value {@link #subject} The actual object that is the target of the 1182 * reference. The reference library doesn't use these, but you can 1183 * use it to hold the resource if you resolve it. (The common 1184 * subject (or patient) of the resources that are in the list if 1185 * there is one.) 1186 */ 1187 public ListResource setSubjectTarget(Resource value) { 1188 this.subjectTarget = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #encounter} (The encounter that is the context in which this 1194 * list was created.) 1195 */ 1196 public Reference getEncounter() { 1197 if (this.encounter == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create List.encounter"); 1200 else if (Configuration.doAutoCreate()) 1201 this.encounter = new Reference(); // cc 1202 return this.encounter; 1203 } 1204 1205 public boolean hasEncounter() { 1206 return this.encounter != null && !this.encounter.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #encounter} (The encounter that is the context in which 1211 * this list was created.) 1212 */ 1213 public ListResource setEncounter(Reference value) { 1214 this.encounter = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #encounter} The actual object that is the target of the 1220 * reference. The reference library doesn't populate this, but you can 1221 * use it to hold the resource if you resolve it. (The encounter that is 1222 * the context in which this list was created.) 1223 */ 1224 public Encounter getEncounterTarget() { 1225 if (this.encounterTarget == null) 1226 if (Configuration.errorOnAutoCreate()) 1227 throw new Error("Attempt to auto-create List.encounter"); 1228 else if (Configuration.doAutoCreate()) 1229 this.encounterTarget = new Encounter(); // aa 1230 return this.encounterTarget; 1231 } 1232 1233 /** 1234 * @param value {@link #encounter} The actual object that is the target of the 1235 * reference. The reference library doesn't use these, but you can 1236 * use it to hold the resource if you resolve it. (The encounter 1237 * that is the context in which this list was created.) 1238 */ 1239 public ListResource setEncounterTarget(Encounter value) { 1240 this.encounterTarget = value; 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #date} (The date that the list was prepared.). This is the 1246 * underlying object with id, value and extensions. The accessor 1247 * "getDate" gives direct access to the value 1248 */ 1249 public DateTimeType getDateElement() { 1250 if (this.date == null) 1251 if (Configuration.errorOnAutoCreate()) 1252 throw new Error("Attempt to auto-create List.date"); 1253 else if (Configuration.doAutoCreate()) 1254 this.date = new DateTimeType(); // bb 1255 return this.date; 1256 } 1257 1258 public boolean hasDateElement() { 1259 return this.date != null && !this.date.isEmpty(); 1260 } 1261 1262 public boolean hasDate() { 1263 return this.date != null && !this.date.isEmpty(); 1264 } 1265 1266 /** 1267 * @param value {@link #date} (The date that the list was prepared.). This is 1268 * the underlying object with id, value and extensions. The 1269 * accessor "getDate" gives direct access to the value 1270 */ 1271 public ListResource setDateElement(DateTimeType value) { 1272 this.date = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return The date that the list was prepared. 1278 */ 1279 public Date getDate() { 1280 return this.date == null ? null : this.date.getValue(); 1281 } 1282 1283 /** 1284 * @param value The date that the list was prepared. 1285 */ 1286 public ListResource setDate(Date value) { 1287 if (value == null) 1288 this.date = null; 1289 else { 1290 if (this.date == null) 1291 this.date = new DateTimeType(); 1292 this.date.setValue(value); 1293 } 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #source} (The entity responsible for deciding what the 1299 * contents of the list were. Where the list was created by a human, 1300 * this is the same as the author of the list.) 1301 */ 1302 public Reference getSource() { 1303 if (this.source == null) 1304 if (Configuration.errorOnAutoCreate()) 1305 throw new Error("Attempt to auto-create List.source"); 1306 else if (Configuration.doAutoCreate()) 1307 this.source = new Reference(); // cc 1308 return this.source; 1309 } 1310 1311 public boolean hasSource() { 1312 return this.source != null && !this.source.isEmpty(); 1313 } 1314 1315 /** 1316 * @param value {@link #source} (The entity responsible for deciding what the 1317 * contents of the list were. Where the list was created by a 1318 * human, this is the same as the author of the list.) 1319 */ 1320 public ListResource setSource(Reference value) { 1321 this.source = value; 1322 return this; 1323 } 1324 1325 /** 1326 * @return {@link #source} The actual object that is the target of the 1327 * reference. The reference library doesn't populate this, but you can 1328 * use it to hold the resource if you resolve it. (The entity 1329 * responsible for deciding what the contents of the list were. Where 1330 * the list was created by a human, this is the same as the author of 1331 * the list.) 1332 */ 1333 public Resource getSourceTarget() { 1334 return this.sourceTarget; 1335 } 1336 1337 /** 1338 * @param value {@link #source} The actual object that is the target of the 1339 * reference. The reference library doesn't use these, but you can 1340 * use it to hold the resource if you resolve it. (The entity 1341 * responsible for deciding what the contents of the list were. 1342 * Where the list was created by a human, this is the same as the 1343 * author of the list.) 1344 */ 1345 public ListResource setSourceTarget(Resource value) { 1346 this.sourceTarget = value; 1347 return this; 1348 } 1349 1350 /** 1351 * @return {@link #orderedBy} (What order applies to the items in the list.) 1352 */ 1353 public CodeableConcept getOrderedBy() { 1354 if (this.orderedBy == null) 1355 if (Configuration.errorOnAutoCreate()) 1356 throw new Error("Attempt to auto-create List.orderedBy"); 1357 else if (Configuration.doAutoCreate()) 1358 this.orderedBy = new CodeableConcept(); // cc 1359 return this.orderedBy; 1360 } 1361 1362 public boolean hasOrderedBy() { 1363 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1364 } 1365 1366 /** 1367 * @param value {@link #orderedBy} (What order applies to the items in the 1368 * list.) 1369 */ 1370 public ListResource setOrderedBy(CodeableConcept value) { 1371 this.orderedBy = value; 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #note} (Comments that apply to the overall list.) 1377 */ 1378 public List<Annotation> getNote() { 1379 if (this.note == null) 1380 this.note = new ArrayList<Annotation>(); 1381 return this.note; 1382 } 1383 1384 /** 1385 * @return Returns a reference to <code>this</code> for easy method chaining 1386 */ 1387 public ListResource setNote(List<Annotation> theNote) { 1388 this.note = theNote; 1389 return this; 1390 } 1391 1392 public boolean hasNote() { 1393 if (this.note == null) 1394 return false; 1395 for (Annotation item : this.note) 1396 if (!item.isEmpty()) 1397 return true; 1398 return false; 1399 } 1400 1401 public Annotation addNote() { // 3 1402 Annotation t = new Annotation(); 1403 if (this.note == null) 1404 this.note = new ArrayList<Annotation>(); 1405 this.note.add(t); 1406 return t; 1407 } 1408 1409 public ListResource addNote(Annotation t) { // 3 1410 if (t == null) 1411 return this; 1412 if (this.note == null) 1413 this.note = new ArrayList<Annotation>(); 1414 this.note.add(t); 1415 return this; 1416 } 1417 1418 /** 1419 * @return The first repetition of repeating field {@link #note}, creating it if 1420 * it does not already exist 1421 */ 1422 public Annotation getNoteFirstRep() { 1423 if (getNote().isEmpty()) { 1424 addNote(); 1425 } 1426 return getNote().get(0); 1427 } 1428 1429 /** 1430 * @return {@link #entry} (Entries in this list.) 1431 */ 1432 public List<ListEntryComponent> getEntry() { 1433 if (this.entry == null) 1434 this.entry = new ArrayList<ListEntryComponent>(); 1435 return this.entry; 1436 } 1437 1438 /** 1439 * @return Returns a reference to <code>this</code> for easy method chaining 1440 */ 1441 public ListResource setEntry(List<ListEntryComponent> theEntry) { 1442 this.entry = theEntry; 1443 return this; 1444 } 1445 1446 public boolean hasEntry() { 1447 if (this.entry == null) 1448 return false; 1449 for (ListEntryComponent item : this.entry) 1450 if (!item.isEmpty()) 1451 return true; 1452 return false; 1453 } 1454 1455 public ListEntryComponent addEntry() { // 3 1456 ListEntryComponent t = new ListEntryComponent(); 1457 if (this.entry == null) 1458 this.entry = new ArrayList<ListEntryComponent>(); 1459 this.entry.add(t); 1460 return t; 1461 } 1462 1463 public ListResource addEntry(ListEntryComponent t) { // 3 1464 if (t == null) 1465 return this; 1466 if (this.entry == null) 1467 this.entry = new ArrayList<ListEntryComponent>(); 1468 this.entry.add(t); 1469 return this; 1470 } 1471 1472 /** 1473 * @return The first repetition of repeating field {@link #entry}, creating it 1474 * if it does not already exist 1475 */ 1476 public ListEntryComponent getEntryFirstRep() { 1477 if (getEntry().isEmpty()) { 1478 addEntry(); 1479 } 1480 return getEntry().get(0); 1481 } 1482 1483 /** 1484 * @return {@link #emptyReason} (If the list is empty, why the list is empty.) 1485 */ 1486 public CodeableConcept getEmptyReason() { 1487 if (this.emptyReason == null) 1488 if (Configuration.errorOnAutoCreate()) 1489 throw new Error("Attempt to auto-create List.emptyReason"); 1490 else if (Configuration.doAutoCreate()) 1491 this.emptyReason = new CodeableConcept(); // cc 1492 return this.emptyReason; 1493 } 1494 1495 public boolean hasEmptyReason() { 1496 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #emptyReason} (If the list is empty, why the list is 1501 * empty.) 1502 */ 1503 public ListResource setEmptyReason(CodeableConcept value) { 1504 this.emptyReason = value; 1505 return this; 1506 } 1507 1508 protected void listChildren(List<Property> children) { 1509 super.listChildren(children); 1510 children.add(new Property("identifier", "Identifier", 1511 "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, 1512 java.lang.Integer.MAX_VALUE, identifier)); 1513 children.add(new Property("status", "code", "Indicates the current state of this list.", 0, 1, status)); 1514 children.add(new Property("mode", "code", 1515 "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1516 0, 1, mode)); 1517 children.add(new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title)); 1518 children.add(new Property("code", "CodeableConcept", 1519 "This code defines the purpose of the list - why it was created.", 0, 1, code)); 1520 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 1521 "The common subject (or patient) of the resources that are in the list if there is one.", 0, 1, subject)); 1522 children.add(new Property("encounter", "Reference(Encounter)", 1523 "The encounter that is the context in which this list was created.", 0, 1, encounter)); 1524 children.add(new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date)); 1525 children.add(new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device)", 1526 "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 1527 0, 1, source)); 1528 children.add( 1529 new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy)); 1530 children.add(new Property("note", "Annotation", "Comments that apply to the overall list.", 0, 1531 java.lang.Integer.MAX_VALUE, note)); 1532 children.add(new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry)); 1533 children.add(new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, 1534 emptyReason)); 1535 } 1536 1537 @Override 1538 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1539 switch (_hash) { 1540 case -1618432855: 1541 /* identifier */ return new Property("identifier", "Identifier", 1542 "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, 1543 java.lang.Integer.MAX_VALUE, identifier); 1544 case -892481550: 1545 /* status */ return new Property("status", "code", "Indicates the current state of this list.", 0, 1, status); 1546 case 3357091: 1547 /* mode */ return new Property("mode", "code", 1548 "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 1549 0, 1, mode); 1550 case 110371416: 1551 /* title */ return new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title); 1552 case 3059181: 1553 /* code */ return new Property("code", "CodeableConcept", 1554 "This code defines the purpose of the list - why it was created.", 0, 1, code); 1555 case -1867885268: 1556 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 1557 "The common subject (or patient) of the resources that are in the list if there is one.", 0, 1, subject); 1558 case 1524132147: 1559 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1560 "The encounter that is the context in which this list was created.", 0, 1, encounter); 1561 case 3076014: 1562 /* date */ return new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date); 1563 case -896505829: 1564 /* source */ return new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device)", 1565 "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 1566 0, 1, source); 1567 case -391079516: 1568 /* orderedBy */ return new Property("orderedBy", "CodeableConcept", 1569 "What order applies to the items in the list.", 0, 1, orderedBy); 1570 case 3387378: 1571 /* note */ return new Property("note", "Annotation", "Comments that apply to the overall list.", 0, 1572 java.lang.Integer.MAX_VALUE, note); 1573 case 96667762: 1574 /* entry */ return new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry); 1575 case 1140135409: 1576 /* emptyReason */ return new Property("emptyReason", "CodeableConcept", 1577 "If the list is empty, why the list is empty.", 0, 1, emptyReason); 1578 default: 1579 return super.getNamedProperty(_hash, _name, _checkValid); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1586 switch (hash) { 1587 case -1618432855: 1588 /* identifier */ return this.identifier == null ? new Base[0] 1589 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1590 case -892481550: 1591 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ListStatus> 1592 case 3357091: 1593 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<ListMode> 1594 case 110371416: 1595 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 1596 case 3059181: 1597 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1598 case -1867885268: 1599 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1600 case 1524132147: 1601 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1602 case 3076014: 1603 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1604 case -896505829: 1605 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // Reference 1606 case -391079516: 1607 /* orderedBy */ return this.orderedBy == null ? new Base[0] : new Base[] { this.orderedBy }; // CodeableConcept 1608 case 3387378: 1609 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1610 case 96667762: 1611 /* entry */ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // ListEntryComponent 1612 case 1140135409: 1613 /* emptyReason */ return this.emptyReason == null ? new Base[0] : new Base[] { this.emptyReason }; // CodeableConcept 1614 default: 1615 return super.getProperty(hash, name, checkValid); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1622 switch (hash) { 1623 case -1618432855: // identifier 1624 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1625 return value; 1626 case -892481550: // status 1627 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1628 this.status = (Enumeration) value; // Enumeration<ListStatus> 1629 return value; 1630 case 3357091: // mode 1631 value = new ListModeEnumFactory().fromType(castToCode(value)); 1632 this.mode = (Enumeration) value; // Enumeration<ListMode> 1633 return value; 1634 case 110371416: // title 1635 this.title = castToString(value); // StringType 1636 return value; 1637 case 3059181: // code 1638 this.code = castToCodeableConcept(value); // CodeableConcept 1639 return value; 1640 case -1867885268: // subject 1641 this.subject = castToReference(value); // Reference 1642 return value; 1643 case 1524132147: // encounter 1644 this.encounter = castToReference(value); // Reference 1645 return value; 1646 case 3076014: // date 1647 this.date = castToDateTime(value); // DateTimeType 1648 return value; 1649 case -896505829: // source 1650 this.source = castToReference(value); // Reference 1651 return value; 1652 case -391079516: // orderedBy 1653 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1654 return value; 1655 case 3387378: // note 1656 this.getNote().add(castToAnnotation(value)); // Annotation 1657 return value; 1658 case 96667762: // entry 1659 this.getEntry().add((ListEntryComponent) value); // ListEntryComponent 1660 return value; 1661 case 1140135409: // emptyReason 1662 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1663 return value; 1664 default: 1665 return super.setProperty(hash, name, value); 1666 } 1667 1668 } 1669 1670 @Override 1671 public Base setProperty(String name, Base value) throws FHIRException { 1672 if (name.equals("identifier")) { 1673 this.getIdentifier().add(castToIdentifier(value)); 1674 } else if (name.equals("status")) { 1675 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1676 this.status = (Enumeration) value; // Enumeration<ListStatus> 1677 } else if (name.equals("mode")) { 1678 value = new ListModeEnumFactory().fromType(castToCode(value)); 1679 this.mode = (Enumeration) value; // Enumeration<ListMode> 1680 } else if (name.equals("title")) { 1681 this.title = castToString(value); // StringType 1682 } else if (name.equals("code")) { 1683 this.code = castToCodeableConcept(value); // CodeableConcept 1684 } else if (name.equals("subject")) { 1685 this.subject = castToReference(value); // Reference 1686 } else if (name.equals("encounter")) { 1687 this.encounter = castToReference(value); // Reference 1688 } else if (name.equals("date")) { 1689 this.date = castToDateTime(value); // DateTimeType 1690 } else if (name.equals("source")) { 1691 this.source = castToReference(value); // Reference 1692 } else if (name.equals("orderedBy")) { 1693 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1694 } else if (name.equals("note")) { 1695 this.getNote().add(castToAnnotation(value)); 1696 } else if (name.equals("entry")) { 1697 this.getEntry().add((ListEntryComponent) value); 1698 } else if (name.equals("emptyReason")) { 1699 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1700 } else 1701 return super.setProperty(name, value); 1702 return value; 1703 } 1704 1705 @Override 1706 public Base makeProperty(int hash, String name) throws FHIRException { 1707 switch (hash) { 1708 case -1618432855: 1709 return addIdentifier(); 1710 case -892481550: 1711 return getStatusElement(); 1712 case 3357091: 1713 return getModeElement(); 1714 case 110371416: 1715 return getTitleElement(); 1716 case 3059181: 1717 return getCode(); 1718 case -1867885268: 1719 return getSubject(); 1720 case 1524132147: 1721 return getEncounter(); 1722 case 3076014: 1723 return getDateElement(); 1724 case -896505829: 1725 return getSource(); 1726 case -391079516: 1727 return getOrderedBy(); 1728 case 3387378: 1729 return addNote(); 1730 case 96667762: 1731 return addEntry(); 1732 case 1140135409: 1733 return getEmptyReason(); 1734 default: 1735 return super.makeProperty(hash, name); 1736 } 1737 1738 } 1739 1740 @Override 1741 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1742 switch (hash) { 1743 case -1618432855: 1744 /* identifier */ return new String[] { "Identifier" }; 1745 case -892481550: 1746 /* status */ return new String[] { "code" }; 1747 case 3357091: 1748 /* mode */ return new String[] { "code" }; 1749 case 110371416: 1750 /* title */ return new String[] { "string" }; 1751 case 3059181: 1752 /* code */ return new String[] { "CodeableConcept" }; 1753 case -1867885268: 1754 /* subject */ return new String[] { "Reference" }; 1755 case 1524132147: 1756 /* encounter */ return new String[] { "Reference" }; 1757 case 3076014: 1758 /* date */ return new String[] { "dateTime" }; 1759 case -896505829: 1760 /* source */ return new String[] { "Reference" }; 1761 case -391079516: 1762 /* orderedBy */ return new String[] { "CodeableConcept" }; 1763 case 3387378: 1764 /* note */ return new String[] { "Annotation" }; 1765 case 96667762: 1766 /* entry */ return new String[] {}; 1767 case 1140135409: 1768 /* emptyReason */ return new String[] { "CodeableConcept" }; 1769 default: 1770 return super.getTypesForProperty(hash, name); 1771 } 1772 1773 } 1774 1775 @Override 1776 public Base addChild(String name) throws FHIRException { 1777 if (name.equals("identifier")) { 1778 return addIdentifier(); 1779 } else if (name.equals("status")) { 1780 throw new FHIRException("Cannot call addChild on a singleton property List.status"); 1781 } else if (name.equals("mode")) { 1782 throw new FHIRException("Cannot call addChild on a singleton property List.mode"); 1783 } else if (name.equals("title")) { 1784 throw new FHIRException("Cannot call addChild on a singleton property List.title"); 1785 } else if (name.equals("code")) { 1786 this.code = new CodeableConcept(); 1787 return this.code; 1788 } else if (name.equals("subject")) { 1789 this.subject = new Reference(); 1790 return this.subject; 1791 } else if (name.equals("encounter")) { 1792 this.encounter = new Reference(); 1793 return this.encounter; 1794 } else if (name.equals("date")) { 1795 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 1796 } else if (name.equals("source")) { 1797 this.source = new Reference(); 1798 return this.source; 1799 } else if (name.equals("orderedBy")) { 1800 this.orderedBy = new CodeableConcept(); 1801 return this.orderedBy; 1802 } else if (name.equals("note")) { 1803 return addNote(); 1804 } else if (name.equals("entry")) { 1805 return addEntry(); 1806 } else if (name.equals("emptyReason")) { 1807 this.emptyReason = new CodeableConcept(); 1808 return this.emptyReason; 1809 } else 1810 return super.addChild(name); 1811 } 1812 1813 public String fhirType() { 1814 return "List"; 1815 1816 } 1817 1818 public ListResource copy() { 1819 ListResource dst = new ListResource(); 1820 copyValues(dst); 1821 return dst; 1822 } 1823 1824 public void copyValues(ListResource dst) { 1825 super.copyValues(dst); 1826 if (identifier != null) { 1827 dst.identifier = new ArrayList<Identifier>(); 1828 for (Identifier i : identifier) 1829 dst.identifier.add(i.copy()); 1830 } 1831 ; 1832 dst.status = status == null ? null : status.copy(); 1833 dst.mode = mode == null ? null : mode.copy(); 1834 dst.title = title == null ? null : title.copy(); 1835 dst.code = code == null ? null : code.copy(); 1836 dst.subject = subject == null ? null : subject.copy(); 1837 dst.encounter = encounter == null ? null : encounter.copy(); 1838 dst.date = date == null ? null : date.copy(); 1839 dst.source = source == null ? null : source.copy(); 1840 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1841 if (note != null) { 1842 dst.note = new ArrayList<Annotation>(); 1843 for (Annotation i : note) 1844 dst.note.add(i.copy()); 1845 } 1846 ; 1847 if (entry != null) { 1848 dst.entry = new ArrayList<ListEntryComponent>(); 1849 for (ListEntryComponent i : entry) 1850 dst.entry.add(i.copy()); 1851 } 1852 ; 1853 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1854 } 1855 1856 protected ListResource typedCopy() { 1857 return copy(); 1858 } 1859 1860 @Override 1861 public boolean equalsDeep(Base other_) { 1862 if (!super.equalsDeep(other_)) 1863 return false; 1864 if (!(other_ instanceof ListResource)) 1865 return false; 1866 ListResource o = (ListResource) other_; 1867 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1868 && compareDeep(mode, o.mode, true) && compareDeep(title, o.title, true) && compareDeep(code, o.code, true) 1869 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 1870 && compareDeep(date, o.date, true) && compareDeep(source, o.source, true) 1871 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(note, o.note, true) 1872 && compareDeep(entry, o.entry, true) && compareDeep(emptyReason, o.emptyReason, true); 1873 } 1874 1875 @Override 1876 public boolean equalsShallow(Base other_) { 1877 if (!super.equalsShallow(other_)) 1878 return false; 1879 if (!(other_ instanceof ListResource)) 1880 return false; 1881 ListResource o = (ListResource) other_; 1882 return compareValues(status, o.status, true) && compareValues(mode, o.mode, true) 1883 && compareValues(title, o.title, true) && compareValues(date, o.date, true); 1884 } 1885 1886 public boolean isEmpty() { 1887 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, mode, title, code, subject, 1888 encounter, date, source, orderedBy, note, entry, emptyReason); 1889 } 1890 1891 @Override 1892 public ResourceType getResourceType() { 1893 return ResourceType.List; 1894 } 1895 1896 /** 1897 * Search parameter: <b>date</b> 1898 * <p> 1899 * Description: <b>When the list was prepared</b><br> 1900 * Type: <b>date</b><br> 1901 * Path: <b>List.date</b><br> 1902 * </p> 1903 */ 1904 @SearchParamDefinition(name = "date", path = "List.date", description = "When the list was prepared", type = "date") 1905 public static final String SP_DATE = "date"; 1906 /** 1907 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1908 * <p> 1909 * Description: <b>When the list was prepared</b><br> 1910 * Type: <b>date</b><br> 1911 * Path: <b>List.date</b><br> 1912 * </p> 1913 */ 1914 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1915 SP_DATE); 1916 1917 /** 1918 * Search parameter: <b>identifier</b> 1919 * <p> 1920 * Description: <b>Business identifier</b><br> 1921 * Type: <b>token</b><br> 1922 * Path: <b>List.identifier</b><br> 1923 * </p> 1924 */ 1925 @SearchParamDefinition(name = "identifier", path = "List.identifier", description = "Business identifier", type = "token") 1926 public static final String SP_IDENTIFIER = "identifier"; 1927 /** 1928 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1929 * <p> 1930 * Description: <b>Business identifier</b><br> 1931 * Type: <b>token</b><br> 1932 * Path: <b>List.identifier</b><br> 1933 * </p> 1934 */ 1935 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1936 SP_IDENTIFIER); 1937 1938 /** 1939 * Search parameter: <b>item</b> 1940 * <p> 1941 * Description: <b>Actual entry</b><br> 1942 * Type: <b>reference</b><br> 1943 * Path: <b>List.entry.item</b><br> 1944 * </p> 1945 */ 1946 @SearchParamDefinition(name = "item", path = "List.entry.item", description = "Actual entry", type = "reference") 1947 public static final String SP_ITEM = "item"; 1948 /** 1949 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1950 * <p> 1951 * Description: <b>Actual entry</b><br> 1952 * Type: <b>reference</b><br> 1953 * Path: <b>List.entry.item</b><br> 1954 * </p> 1955 */ 1956 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1957 SP_ITEM); 1958 1959 /** 1960 * Constant for fluent queries to be used to add include statements. Specifies 1961 * the path value of "<b>List:item</b>". 1962 */ 1963 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("List:item") 1964 .toLocked(); 1965 1966 /** 1967 * Search parameter: <b>empty-reason</b> 1968 * <p> 1969 * Description: <b>Why list is empty</b><br> 1970 * Type: <b>token</b><br> 1971 * Path: <b>List.emptyReason</b><br> 1972 * </p> 1973 */ 1974 @SearchParamDefinition(name = "empty-reason", path = "List.emptyReason", description = "Why list is empty", type = "token") 1975 public static final String SP_EMPTY_REASON = "empty-reason"; 1976 /** 1977 * <b>Fluent Client</b> search parameter constant for <b>empty-reason</b> 1978 * <p> 1979 * Description: <b>Why list is empty</b><br> 1980 * Type: <b>token</b><br> 1981 * Path: <b>List.emptyReason</b><br> 1982 * </p> 1983 */ 1984 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMPTY_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1985 SP_EMPTY_REASON); 1986 1987 /** 1988 * Search parameter: <b>code</b> 1989 * <p> 1990 * Description: <b>What the purpose of this list is</b><br> 1991 * Type: <b>token</b><br> 1992 * Path: <b>List.code</b><br> 1993 * </p> 1994 */ 1995 @SearchParamDefinition(name = "code", path = "List.code", description = "What the purpose of this list is", type = "token") 1996 public static final String SP_CODE = "code"; 1997 /** 1998 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1999 * <p> 2000 * Description: <b>What the purpose of this list is</b><br> 2001 * Type: <b>token</b><br> 2002 * Path: <b>List.code</b><br> 2003 * </p> 2004 */ 2005 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2006 SP_CODE); 2007 2008 /** 2009 * Search parameter: <b>notes</b> 2010 * <p> 2011 * Description: <b>The annotation - text content (as markdown)</b><br> 2012 * Type: <b>string</b><br> 2013 * Path: <b>List.note.text</b><br> 2014 * </p> 2015 */ 2016 @SearchParamDefinition(name = "notes", path = "List.note.text", description = "The annotation - text content (as markdown)", type = "string") 2017 public static final String SP_NOTES = "notes"; 2018 /** 2019 * <b>Fluent Client</b> search parameter constant for <b>notes</b> 2020 * <p> 2021 * Description: <b>The annotation - text content (as markdown)</b><br> 2022 * Type: <b>string</b><br> 2023 * Path: <b>List.note.text</b><br> 2024 * </p> 2025 */ 2026 public static final ca.uhn.fhir.rest.gclient.StringClientParam NOTES = new ca.uhn.fhir.rest.gclient.StringClientParam( 2027 SP_NOTES); 2028 2029 /** 2030 * Search parameter: <b>subject</b> 2031 * <p> 2032 * Description: <b>If all resources have the same subject</b><br> 2033 * Type: <b>reference</b><br> 2034 * Path: <b>List.subject</b><br> 2035 * </p> 2036 */ 2037 @SearchParamDefinition(name = "subject", path = "List.subject", description = "If all resources have the same subject", type = "reference", providesMembershipIn = { 2038 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Device"), 2039 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Patient") }, target = { 2040 Device.class, Group.class, Location.class, Patient.class }) 2041 public static final String SP_SUBJECT = "subject"; 2042 /** 2043 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2044 * <p> 2045 * Description: <b>If all resources have the same subject</b><br> 2046 * Type: <b>reference</b><br> 2047 * Path: <b>List.subject</b><br> 2048 * </p> 2049 */ 2050 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2051 SP_SUBJECT); 2052 2053 /** 2054 * Constant for fluent queries to be used to add include statements. Specifies 2055 * the path value of "<b>List:subject</b>". 2056 */ 2057 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("List:subject") 2058 .toLocked(); 2059 2060 /** 2061 * Search parameter: <b>patient</b> 2062 * <p> 2063 * Description: <b>If all resources have the same subject</b><br> 2064 * Type: <b>reference</b><br> 2065 * Path: <b>List.subject</b><br> 2066 * </p> 2067 */ 2068 @SearchParamDefinition(name = "patient", path = "List.subject.where(resolve() is Patient)", description = "If all resources have the same subject", type = "reference", target = { 2069 Patient.class }) 2070 public static final String SP_PATIENT = "patient"; 2071 /** 2072 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2073 * <p> 2074 * Description: <b>If all resources have the same subject</b><br> 2075 * Type: <b>reference</b><br> 2076 * Path: <b>List.subject</b><br> 2077 * </p> 2078 */ 2079 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2080 SP_PATIENT); 2081 2082 /** 2083 * Constant for fluent queries to be used to add include statements. Specifies 2084 * the path value of "<b>List:patient</b>". 2085 */ 2086 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("List:patient") 2087 .toLocked(); 2088 2089 /** 2090 * Search parameter: <b>source</b> 2091 * <p> 2092 * Description: <b>Who and/or what defined the list contents (aka 2093 * Author)</b><br> 2094 * Type: <b>reference</b><br> 2095 * Path: <b>List.source</b><br> 2096 * </p> 2097 */ 2098 @SearchParamDefinition(name = "source", path = "List.source", description = "Who and/or what defined the list contents (aka Author)", type = "reference", providesMembershipIn = { 2099 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Device"), 2100 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Patient"), 2101 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Base FHIR compartment definition for Practitioner") }, target = { 2102 Device.class, Patient.class, Practitioner.class, PractitionerRole.class }) 2103 public static final String SP_SOURCE = "source"; 2104 /** 2105 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2106 * <p> 2107 * Description: <b>Who and/or what defined the list contents (aka 2108 * Author)</b><br> 2109 * Type: <b>reference</b><br> 2110 * Path: <b>List.source</b><br> 2111 * </p> 2112 */ 2113 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2114 SP_SOURCE); 2115 2116 /** 2117 * Constant for fluent queries to be used to add include statements. Specifies 2118 * the path value of "<b>List:source</b>". 2119 */ 2120 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("List:source") 2121 .toLocked(); 2122 2123 /** 2124 * Search parameter: <b>encounter</b> 2125 * <p> 2126 * Description: <b>Context in which list created</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>List.encounter</b><br> 2129 * </p> 2130 */ 2131 @SearchParamDefinition(name = "encounter", path = "List.encounter", description = "Context in which list created", type = "reference", target = { 2132 Encounter.class }) 2133 public static final String SP_ENCOUNTER = "encounter"; 2134 /** 2135 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2136 * <p> 2137 * Description: <b>Context in which list created</b><br> 2138 * Type: <b>reference</b><br> 2139 * Path: <b>List.encounter</b><br> 2140 * </p> 2141 */ 2142 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2143 SP_ENCOUNTER); 2144 2145 /** 2146 * Constant for fluent queries to be used to add include statements. Specifies 2147 * the path value of "<b>List:encounter</b>". 2148 */ 2149 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2150 "List:encounter").toLocked(); 2151 2152 /** 2153 * Search parameter: <b>title</b> 2154 * <p> 2155 * Description: <b>Descriptive name for the list</b><br> 2156 * Type: <b>string</b><br> 2157 * Path: <b>List.title</b><br> 2158 * </p> 2159 */ 2160 @SearchParamDefinition(name = "title", path = "List.title", description = "Descriptive name for the list", type = "string") 2161 public static final String SP_TITLE = "title"; 2162 /** 2163 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2164 * <p> 2165 * Description: <b>Descriptive name for the list</b><br> 2166 * Type: <b>string</b><br> 2167 * Path: <b>List.title</b><br> 2168 * </p> 2169 */ 2170 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2171 SP_TITLE); 2172 2173 /** 2174 * Search parameter: <b>status</b> 2175 * <p> 2176 * Description: <b>current | retired | entered-in-error</b><br> 2177 * Type: <b>token</b><br> 2178 * Path: <b>List.status</b><br> 2179 * </p> 2180 */ 2181 @SearchParamDefinition(name = "status", path = "List.status", description = "current | retired | entered-in-error", type = "token") 2182 public static final String SP_STATUS = "status"; 2183 /** 2184 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2185 * <p> 2186 * Description: <b>current | retired | entered-in-error</b><br> 2187 * Type: <b>token</b><br> 2188 * Path: <b>List.status</b><br> 2189 * </p> 2190 */ 2191 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2192 SP_STATUS); 2193 2194}