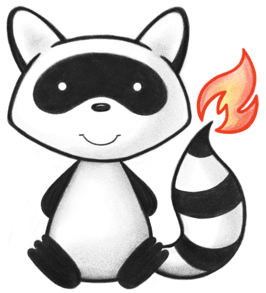
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Details and position information for a physical place where services are 050 * provided and resources and participants may be stored, found, contained, or 051 * accommodated. 052 */ 053@ResourceDef(name = "Location", profile = "http://hl7.org/fhir/StructureDefinition/Location") 054public class Location extends DomainResource { 055 056 public enum LocationStatus { 057 /** 058 * The location is operational. 059 */ 060 ACTIVE, 061 /** 062 * The location is temporarily closed. 063 */ 064 SUSPENDED, 065 /** 066 * The location is no longer used. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 074 public static LocationStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("suspended".equals(codeString)) 080 return SUSPENDED; 081 if ("inactive".equals(codeString)) 082 return INACTIVE; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case ACTIVE: 092 return "active"; 093 case SUSPENDED: 094 return "suspended"; 095 case INACTIVE: 096 return "inactive"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case ACTIVE: 107 return "http://hl7.org/fhir/location-status"; 108 case SUSPENDED: 109 return "http://hl7.org/fhir/location-status"; 110 case INACTIVE: 111 return "http://hl7.org/fhir/location-status"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case ACTIVE: 122 return "The location is operational."; 123 case SUSPENDED: 124 return "The location is temporarily closed."; 125 case INACTIVE: 126 return "The location is no longer used."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case ACTIVE: 137 return "Active"; 138 case SUSPENDED: 139 return "Suspended"; 140 case INACTIVE: 141 return "Inactive"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class LocationStatusEnumFactory implements EnumFactory<LocationStatus> { 151 public LocationStatus fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return LocationStatus.ACTIVE; 157 if ("suspended".equals(codeString)) 158 return LocationStatus.SUSPENDED; 159 if ("inactive".equals(codeString)) 160 return LocationStatus.INACTIVE; 161 throw new IllegalArgumentException("Unknown LocationStatus code '" + codeString + "'"); 162 } 163 164 public Enumeration<LocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 165 if (code == null) 166 return null; 167 if (code.isEmpty()) 168 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 169 String codeString = code.asStringValue(); 170 if (codeString == null || "".equals(codeString)) 171 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 172 if ("active".equals(codeString)) 173 return new Enumeration<LocationStatus>(this, LocationStatus.ACTIVE, code); 174 if ("suspended".equals(codeString)) 175 return new Enumeration<LocationStatus>(this, LocationStatus.SUSPENDED, code); 176 if ("inactive".equals(codeString)) 177 return new Enumeration<LocationStatus>(this, LocationStatus.INACTIVE, code); 178 throw new FHIRException("Unknown LocationStatus code '" + codeString + "'"); 179 } 180 181 public String toCode(LocationStatus code) { 182 if (code == LocationStatus.ACTIVE) 183 return "active"; 184 if (code == LocationStatus.SUSPENDED) 185 return "suspended"; 186 if (code == LocationStatus.INACTIVE) 187 return "inactive"; 188 return "?"; 189 } 190 191 public String toSystem(LocationStatus code) { 192 return code.getSystem(); 193 } 194 } 195 196 public enum LocationMode { 197 /** 198 * The Location resource represents a specific instance of a location (e.g. 199 * Operating Theatre 1A). 200 */ 201 INSTANCE, 202 /** 203 * The Location represents a class of locations (e.g. Any Operating Theatre) 204 * although this class of locations could be constrained within a specific 205 * boundary (such as organization, or parent location, address etc.). 206 */ 207 KIND, 208 /** 209 * added to help the parsers with the generic types 210 */ 211 NULL; 212 213 public static LocationMode fromCode(String codeString) throws FHIRException { 214 if (codeString == null || "".equals(codeString)) 215 return null; 216 if ("instance".equals(codeString)) 217 return INSTANCE; 218 if ("kind".equals(codeString)) 219 return KIND; 220 if (Configuration.isAcceptInvalidEnums()) 221 return null; 222 else 223 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 224 } 225 226 public String toCode() { 227 switch (this) { 228 case INSTANCE: 229 return "instance"; 230 case KIND: 231 return "kind"; 232 case NULL: 233 return null; 234 default: 235 return "?"; 236 } 237 } 238 239 public String getSystem() { 240 switch (this) { 241 case INSTANCE: 242 return "http://hl7.org/fhir/location-mode"; 243 case KIND: 244 return "http://hl7.org/fhir/location-mode"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getDefinition() { 253 switch (this) { 254 case INSTANCE: 255 return "The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A)."; 256 case KIND: 257 return "The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.)."; 258 case NULL: 259 return null; 260 default: 261 return "?"; 262 } 263 } 264 265 public String getDisplay() { 266 switch (this) { 267 case INSTANCE: 268 return "Instance"; 269 case KIND: 270 return "Kind"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 } 278 279 public static class LocationModeEnumFactory implements EnumFactory<LocationMode> { 280 public LocationMode fromCode(String codeString) throws IllegalArgumentException { 281 if (codeString == null || "".equals(codeString)) 282 if (codeString == null || "".equals(codeString)) 283 return null; 284 if ("instance".equals(codeString)) 285 return LocationMode.INSTANCE; 286 if ("kind".equals(codeString)) 287 return LocationMode.KIND; 288 throw new IllegalArgumentException("Unknown LocationMode code '" + codeString + "'"); 289 } 290 291 public Enumeration<LocationMode> fromType(PrimitiveType<?> code) throws FHIRException { 292 if (code == null) 293 return null; 294 if (code.isEmpty()) 295 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 296 String codeString = code.asStringValue(); 297 if (codeString == null || "".equals(codeString)) 298 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 299 if ("instance".equals(codeString)) 300 return new Enumeration<LocationMode>(this, LocationMode.INSTANCE, code); 301 if ("kind".equals(codeString)) 302 return new Enumeration<LocationMode>(this, LocationMode.KIND, code); 303 throw new FHIRException("Unknown LocationMode code '" + codeString + "'"); 304 } 305 306 public String toCode(LocationMode code) { 307 if (code == LocationMode.INSTANCE) 308 return "instance"; 309 if (code == LocationMode.KIND) 310 return "kind"; 311 return "?"; 312 } 313 314 public String toSystem(LocationMode code) { 315 return code.getSystem(); 316 } 317 } 318 319 public enum DaysOfWeek { 320 /** 321 * Monday. 322 */ 323 MON, 324 /** 325 * Tuesday. 326 */ 327 TUE, 328 /** 329 * Wednesday. 330 */ 331 WED, 332 /** 333 * Thursday. 334 */ 335 THU, 336 /** 337 * Friday. 338 */ 339 FRI, 340 /** 341 * Saturday. 342 */ 343 SAT, 344 /** 345 * Sunday. 346 */ 347 SUN, 348 /** 349 * added to help the parsers with the generic types 350 */ 351 NULL; 352 353 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 354 if (codeString == null || "".equals(codeString)) 355 return null; 356 if ("mon".equals(codeString)) 357 return MON; 358 if ("tue".equals(codeString)) 359 return TUE; 360 if ("wed".equals(codeString)) 361 return WED; 362 if ("thu".equals(codeString)) 363 return THU; 364 if ("fri".equals(codeString)) 365 return FRI; 366 if ("sat".equals(codeString)) 367 return SAT; 368 if ("sun".equals(codeString)) 369 return SUN; 370 if (Configuration.isAcceptInvalidEnums()) 371 return null; 372 else 373 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 374 } 375 376 public String toCode() { 377 switch (this) { 378 case MON: 379 return "mon"; 380 case TUE: 381 return "tue"; 382 case WED: 383 return "wed"; 384 case THU: 385 return "thu"; 386 case FRI: 387 return "fri"; 388 case SAT: 389 return "sat"; 390 case SUN: 391 return "sun"; 392 case NULL: 393 return null; 394 default: 395 return "?"; 396 } 397 } 398 399 public String getSystem() { 400 switch (this) { 401 case MON: 402 return "http://hl7.org/fhir/days-of-week"; 403 case TUE: 404 return "http://hl7.org/fhir/days-of-week"; 405 case WED: 406 return "http://hl7.org/fhir/days-of-week"; 407 case THU: 408 return "http://hl7.org/fhir/days-of-week"; 409 case FRI: 410 return "http://hl7.org/fhir/days-of-week"; 411 case SAT: 412 return "http://hl7.org/fhir/days-of-week"; 413 case SUN: 414 return "http://hl7.org/fhir/days-of-week"; 415 case NULL: 416 return null; 417 default: 418 return "?"; 419 } 420 } 421 422 public String getDefinition() { 423 switch (this) { 424 case MON: 425 return "Monday."; 426 case TUE: 427 return "Tuesday."; 428 case WED: 429 return "Wednesday."; 430 case THU: 431 return "Thursday."; 432 case FRI: 433 return "Friday."; 434 case SAT: 435 return "Saturday."; 436 case SUN: 437 return "Sunday."; 438 case NULL: 439 return null; 440 default: 441 return "?"; 442 } 443 } 444 445 public String getDisplay() { 446 switch (this) { 447 case MON: 448 return "Monday"; 449 case TUE: 450 return "Tuesday"; 451 case WED: 452 return "Wednesday"; 453 case THU: 454 return "Thursday"; 455 case FRI: 456 return "Friday"; 457 case SAT: 458 return "Saturday"; 459 case SUN: 460 return "Sunday"; 461 case NULL: 462 return null; 463 default: 464 return "?"; 465 } 466 } 467 } 468 469 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 470 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 471 if (codeString == null || "".equals(codeString)) 472 if (codeString == null || "".equals(codeString)) 473 return null; 474 if ("mon".equals(codeString)) 475 return DaysOfWeek.MON; 476 if ("tue".equals(codeString)) 477 return DaysOfWeek.TUE; 478 if ("wed".equals(codeString)) 479 return DaysOfWeek.WED; 480 if ("thu".equals(codeString)) 481 return DaysOfWeek.THU; 482 if ("fri".equals(codeString)) 483 return DaysOfWeek.FRI; 484 if ("sat".equals(codeString)) 485 return DaysOfWeek.SAT; 486 if ("sun".equals(codeString)) 487 return DaysOfWeek.SUN; 488 throw new IllegalArgumentException("Unknown DaysOfWeek code '" + codeString + "'"); 489 } 490 491 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 492 if (code == null) 493 return null; 494 if (code.isEmpty()) 495 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 496 String codeString = code.asStringValue(); 497 if (codeString == null || "".equals(codeString)) 498 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.NULL, code); 499 if ("mon".equals(codeString)) 500 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON, code); 501 if ("tue".equals(codeString)) 502 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE, code); 503 if ("wed".equals(codeString)) 504 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED, code); 505 if ("thu".equals(codeString)) 506 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU, code); 507 if ("fri".equals(codeString)) 508 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI, code); 509 if ("sat".equals(codeString)) 510 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT, code); 511 if ("sun".equals(codeString)) 512 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN, code); 513 throw new FHIRException("Unknown DaysOfWeek code '" + codeString + "'"); 514 } 515 516 public String toCode(DaysOfWeek code) { 517 if (code == DaysOfWeek.MON) 518 return "mon"; 519 if (code == DaysOfWeek.TUE) 520 return "tue"; 521 if (code == DaysOfWeek.WED) 522 return "wed"; 523 if (code == DaysOfWeek.THU) 524 return "thu"; 525 if (code == DaysOfWeek.FRI) 526 return "fri"; 527 if (code == DaysOfWeek.SAT) 528 return "sat"; 529 if (code == DaysOfWeek.SUN) 530 return "sun"; 531 return "?"; 532 } 533 534 public String toSystem(DaysOfWeek code) { 535 return code.getSystem(); 536 } 537 } 538 539 @Block() 540 public static class LocationPositionComponent extends BackboneElement implements IBaseBackboneElement { 541 /** 542 * Longitude. The value domain and the interpretation are the same as for the 543 * text of the longitude element in KML (see notes below). 544 */ 545 @Child(name = "longitude", type = { 546 DecimalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 547 @Description(shortDefinition = "Longitude with WGS84 datum", formalDefinition = "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).") 548 protected DecimalType longitude; 549 550 /** 551 * Latitude. The value domain and the interpretation are the same as for the 552 * text of the latitude element in KML (see notes below). 553 */ 554 @Child(name = "latitude", type = { 555 DecimalType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 556 @Description(shortDefinition = "Latitude with WGS84 datum", formalDefinition = "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).") 557 protected DecimalType latitude; 558 559 /** 560 * Altitude. The value domain and the interpretation are the same as for the 561 * text of the altitude element in KML (see notes below). 562 */ 563 @Child(name = "altitude", type = { 564 DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 565 @Description(shortDefinition = "Altitude with WGS84 datum", formalDefinition = "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).") 566 protected DecimalType altitude; 567 568 private static final long serialVersionUID = -74276134L; 569 570 /** 571 * Constructor 572 */ 573 public LocationPositionComponent() { 574 super(); 575 } 576 577 /** 578 * Constructor 579 */ 580 public LocationPositionComponent(DecimalType longitude, DecimalType latitude) { 581 super(); 582 this.longitude = longitude; 583 this.latitude = latitude; 584 } 585 586 /** 587 * @return {@link #longitude} (Longitude. The value domain and the 588 * interpretation are the same as for the text of the longitude element 589 * in KML (see notes below).). This is the underlying object with id, 590 * value and extensions. The accessor "getLongitude" gives direct access 591 * to the value 592 */ 593 public DecimalType getLongitudeElement() { 594 if (this.longitude == null) 595 if (Configuration.errorOnAutoCreate()) 596 throw new Error("Attempt to auto-create LocationPositionComponent.longitude"); 597 else if (Configuration.doAutoCreate()) 598 this.longitude = new DecimalType(); // bb 599 return this.longitude; 600 } 601 602 public boolean hasLongitudeElement() { 603 return this.longitude != null && !this.longitude.isEmpty(); 604 } 605 606 public boolean hasLongitude() { 607 return this.longitude != null && !this.longitude.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #longitude} (Longitude. The value domain and the 612 * interpretation are the same as for the text of the longitude 613 * element in KML (see notes below).). This is the underlying 614 * object with id, value and extensions. The accessor 615 * "getLongitude" gives direct access to the value 616 */ 617 public LocationPositionComponent setLongitudeElement(DecimalType value) { 618 this.longitude = value; 619 return this; 620 } 621 622 /** 623 * @return Longitude. The value domain and the interpretation are the same as 624 * for the text of the longitude element in KML (see notes below). 625 */ 626 public BigDecimal getLongitude() { 627 return this.longitude == null ? null : this.longitude.getValue(); 628 } 629 630 /** 631 * @param value Longitude. The value domain and the interpretation are the same 632 * as for the text of the longitude element in KML (see notes 633 * below). 634 */ 635 public LocationPositionComponent setLongitude(BigDecimal value) { 636 if (this.longitude == null) 637 this.longitude = new DecimalType(); 638 this.longitude.setValue(value); 639 return this; 640 } 641 642 /** 643 * @param value Longitude. The value domain and the interpretation are the same 644 * as for the text of the longitude element in KML (see notes 645 * below). 646 */ 647 public LocationPositionComponent setLongitude(long value) { 648 this.longitude = new DecimalType(); 649 this.longitude.setValue(value); 650 return this; 651 } 652 653 /** 654 * @param value Longitude. The value domain and the interpretation are the same 655 * as for the text of the longitude element in KML (see notes 656 * below). 657 */ 658 public LocationPositionComponent setLongitude(double value) { 659 this.longitude = new DecimalType(); 660 this.longitude.setValue(value); 661 return this; 662 } 663 664 /** 665 * @return {@link #latitude} (Latitude. The value domain and the interpretation 666 * are the same as for the text of the latitude element in KML (see 667 * notes below).). This is the underlying object with id, value and 668 * extensions. The accessor "getLatitude" gives direct access to the 669 * value 670 */ 671 public DecimalType getLatitudeElement() { 672 if (this.latitude == null) 673 if (Configuration.errorOnAutoCreate()) 674 throw new Error("Attempt to auto-create LocationPositionComponent.latitude"); 675 else if (Configuration.doAutoCreate()) 676 this.latitude = new DecimalType(); // bb 677 return this.latitude; 678 } 679 680 public boolean hasLatitudeElement() { 681 return this.latitude != null && !this.latitude.isEmpty(); 682 } 683 684 public boolean hasLatitude() { 685 return this.latitude != null && !this.latitude.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #latitude} (Latitude. The value domain and the 690 * interpretation are the same as for the text of the latitude 691 * element in KML (see notes below).). This is the underlying 692 * object with id, value and extensions. The accessor "getLatitude" 693 * gives direct access to the value 694 */ 695 public LocationPositionComponent setLatitudeElement(DecimalType value) { 696 this.latitude = value; 697 return this; 698 } 699 700 /** 701 * @return Latitude. The value domain and the interpretation are the same as for 702 * the text of the latitude element in KML (see notes below). 703 */ 704 public BigDecimal getLatitude() { 705 return this.latitude == null ? null : this.latitude.getValue(); 706 } 707 708 /** 709 * @param value Latitude. The value domain and the interpretation are the same 710 * as for the text of the latitude element in KML (see notes 711 * below). 712 */ 713 public LocationPositionComponent setLatitude(BigDecimal value) { 714 if (this.latitude == null) 715 this.latitude = new DecimalType(); 716 this.latitude.setValue(value); 717 return this; 718 } 719 720 /** 721 * @param value Latitude. The value domain and the interpretation are the same 722 * as for the text of the latitude element in KML (see notes 723 * below). 724 */ 725 public LocationPositionComponent setLatitude(long value) { 726 this.latitude = new DecimalType(); 727 this.latitude.setValue(value); 728 return this; 729 } 730 731 /** 732 * @param value Latitude. The value domain and the interpretation are the same 733 * as for the text of the latitude element in KML (see notes 734 * below). 735 */ 736 public LocationPositionComponent setLatitude(double value) { 737 this.latitude = new DecimalType(); 738 this.latitude.setValue(value); 739 return this; 740 } 741 742 /** 743 * @return {@link #altitude} (Altitude. The value domain and the interpretation 744 * are the same as for the text of the altitude element in KML (see 745 * notes below).). This is the underlying object with id, value and 746 * extensions. The accessor "getAltitude" gives direct access to the 747 * value 748 */ 749 public DecimalType getAltitudeElement() { 750 if (this.altitude == null) 751 if (Configuration.errorOnAutoCreate()) 752 throw new Error("Attempt to auto-create LocationPositionComponent.altitude"); 753 else if (Configuration.doAutoCreate()) 754 this.altitude = new DecimalType(); // bb 755 return this.altitude; 756 } 757 758 public boolean hasAltitudeElement() { 759 return this.altitude != null && !this.altitude.isEmpty(); 760 } 761 762 public boolean hasAltitude() { 763 return this.altitude != null && !this.altitude.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #altitude} (Altitude. The value domain and the 768 * interpretation are the same as for the text of the altitude 769 * element in KML (see notes below).). This is the underlying 770 * object with id, value and extensions. The accessor "getAltitude" 771 * gives direct access to the value 772 */ 773 public LocationPositionComponent setAltitudeElement(DecimalType value) { 774 this.altitude = value; 775 return this; 776 } 777 778 /** 779 * @return Altitude. The value domain and the interpretation are the same as for 780 * the text of the altitude element in KML (see notes below). 781 */ 782 public BigDecimal getAltitude() { 783 return this.altitude == null ? null : this.altitude.getValue(); 784 } 785 786 /** 787 * @param value Altitude. The value domain and the interpretation are the same 788 * as for the text of the altitude element in KML (see notes 789 * below). 790 */ 791 public LocationPositionComponent setAltitude(BigDecimal value) { 792 if (value == null) 793 this.altitude = null; 794 else { 795 if (this.altitude == null) 796 this.altitude = new DecimalType(); 797 this.altitude.setValue(value); 798 } 799 return this; 800 } 801 802 /** 803 * @param value Altitude. The value domain and the interpretation are the same 804 * as for the text of the altitude element in KML (see notes 805 * below). 806 */ 807 public LocationPositionComponent setAltitude(long value) { 808 this.altitude = new DecimalType(); 809 this.altitude.setValue(value); 810 return this; 811 } 812 813 /** 814 * @param value Altitude. The value domain and the interpretation are the same 815 * as for the text of the altitude element in KML (see notes 816 * below). 817 */ 818 public LocationPositionComponent setAltitude(double value) { 819 this.altitude = new DecimalType(); 820 this.altitude.setValue(value); 821 return this; 822 } 823 824 protected void listChildren(List<Property> children) { 825 super.listChildren(children); 826 children.add(new Property("longitude", "decimal", 827 "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 828 0, 1, longitude)); 829 children.add(new Property("latitude", "decimal", 830 "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 831 0, 1, latitude)); 832 children.add(new Property("altitude", "decimal", 833 "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 834 0, 1, altitude)); 835 } 836 837 @Override 838 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 839 switch (_hash) { 840 case 137365935: 841 /* longitude */ return new Property("longitude", "decimal", 842 "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 843 0, 1, longitude); 844 case -1439978388: 845 /* latitude */ return new Property("latitude", "decimal", 846 "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 847 0, 1, latitude); 848 case 2036550306: 849 /* altitude */ return new Property("altitude", "decimal", 850 "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 851 0, 1, altitude); 852 default: 853 return super.getNamedProperty(_hash, _name, _checkValid); 854 } 855 856 } 857 858 @Override 859 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 860 switch (hash) { 861 case 137365935: 862 /* longitude */ return this.longitude == null ? new Base[0] : new Base[] { this.longitude }; // DecimalType 863 case -1439978388: 864 /* latitude */ return this.latitude == null ? new Base[0] : new Base[] { this.latitude }; // DecimalType 865 case 2036550306: 866 /* altitude */ return this.altitude == null ? new Base[0] : new Base[] { this.altitude }; // DecimalType 867 default: 868 return super.getProperty(hash, name, checkValid); 869 } 870 871 } 872 873 @Override 874 public Base setProperty(int hash, String name, Base value) throws FHIRException { 875 switch (hash) { 876 case 137365935: // longitude 877 this.longitude = castToDecimal(value); // DecimalType 878 return value; 879 case -1439978388: // latitude 880 this.latitude = castToDecimal(value); // DecimalType 881 return value; 882 case 2036550306: // altitude 883 this.altitude = castToDecimal(value); // DecimalType 884 return value; 885 default: 886 return super.setProperty(hash, name, value); 887 } 888 889 } 890 891 @Override 892 public Base setProperty(String name, Base value) throws FHIRException { 893 if (name.equals("longitude")) { 894 this.longitude = castToDecimal(value); // DecimalType 895 } else if (name.equals("latitude")) { 896 this.latitude = castToDecimal(value); // DecimalType 897 } else if (name.equals("altitude")) { 898 this.altitude = castToDecimal(value); // DecimalType 899 } else 900 return super.setProperty(name, value); 901 return value; 902 } 903 904 @Override 905 public Base makeProperty(int hash, String name) throws FHIRException { 906 switch (hash) { 907 case 137365935: 908 return getLongitudeElement(); 909 case -1439978388: 910 return getLatitudeElement(); 911 case 2036550306: 912 return getAltitudeElement(); 913 default: 914 return super.makeProperty(hash, name); 915 } 916 917 } 918 919 @Override 920 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 921 switch (hash) { 922 case 137365935: 923 /* longitude */ return new String[] { "decimal" }; 924 case -1439978388: 925 /* latitude */ return new String[] { "decimal" }; 926 case 2036550306: 927 /* altitude */ return new String[] { "decimal" }; 928 default: 929 return super.getTypesForProperty(hash, name); 930 } 931 932 } 933 934 @Override 935 public Base addChild(String name) throws FHIRException { 936 if (name.equals("longitude")) { 937 throw new FHIRException("Cannot call addChild on a singleton property Location.longitude"); 938 } else if (name.equals("latitude")) { 939 throw new FHIRException("Cannot call addChild on a singleton property Location.latitude"); 940 } else if (name.equals("altitude")) { 941 throw new FHIRException("Cannot call addChild on a singleton property Location.altitude"); 942 } else 943 return super.addChild(name); 944 } 945 946 public LocationPositionComponent copy() { 947 LocationPositionComponent dst = new LocationPositionComponent(); 948 copyValues(dst); 949 return dst; 950 } 951 952 public void copyValues(LocationPositionComponent dst) { 953 super.copyValues(dst); 954 dst.longitude = longitude == null ? null : longitude.copy(); 955 dst.latitude = latitude == null ? null : latitude.copy(); 956 dst.altitude = altitude == null ? null : altitude.copy(); 957 } 958 959 @Override 960 public boolean equalsDeep(Base other_) { 961 if (!super.equalsDeep(other_)) 962 return false; 963 if (!(other_ instanceof LocationPositionComponent)) 964 return false; 965 LocationPositionComponent o = (LocationPositionComponent) other_; 966 return compareDeep(longitude, o.longitude, true) && compareDeep(latitude, o.latitude, true) 967 && compareDeep(altitude, o.altitude, true); 968 } 969 970 @Override 971 public boolean equalsShallow(Base other_) { 972 if (!super.equalsShallow(other_)) 973 return false; 974 if (!(other_ instanceof LocationPositionComponent)) 975 return false; 976 LocationPositionComponent o = (LocationPositionComponent) other_; 977 return compareValues(longitude, o.longitude, true) && compareValues(latitude, o.latitude, true) 978 && compareValues(altitude, o.altitude, true); 979 } 980 981 public boolean isEmpty() { 982 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(longitude, latitude, altitude); 983 } 984 985 public String fhirType() { 986 return "Location.position"; 987 988 } 989 990 } 991 992 @Block() 993 public static class LocationHoursOfOperationComponent extends BackboneElement implements IBaseBackboneElement { 994 /** 995 * Indicates which days of the week are available between the start and end 996 * Times. 997 */ 998 @Child(name = "daysOfWeek", type = { 999 CodeType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1000 @Description(shortDefinition = "mon | tue | wed | thu | fri | sat | sun", formalDefinition = "Indicates which days of the week are available between the start and end Times.") 1001 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/days-of-week") 1002 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 1003 1004 /** 1005 * The Location is open all day. 1006 */ 1007 @Child(name = "allDay", type = { 1008 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1009 @Description(shortDefinition = "The Location is open all day", formalDefinition = "The Location is open all day.") 1010 protected BooleanType allDay; 1011 1012 /** 1013 * Time that the Location opens. 1014 */ 1015 @Child(name = "openingTime", type = { 1016 TimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1017 @Description(shortDefinition = "Time that the Location opens", formalDefinition = "Time that the Location opens.") 1018 protected TimeType openingTime; 1019 1020 /** 1021 * Time that the Location closes. 1022 */ 1023 @Child(name = "closingTime", type = { 1024 TimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1025 @Description(shortDefinition = "Time that the Location closes", formalDefinition = "Time that the Location closes.") 1026 protected TimeType closingTime; 1027 1028 private static final long serialVersionUID = -932551849L; 1029 1030 /** 1031 * Constructor 1032 */ 1033 public LocationHoursOfOperationComponent() { 1034 super(); 1035 } 1036 1037 /** 1038 * @return {@link #daysOfWeek} (Indicates which days of the week are available 1039 * between the start and end Times.) 1040 */ 1041 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 1042 if (this.daysOfWeek == null) 1043 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1044 return this.daysOfWeek; 1045 } 1046 1047 /** 1048 * @return Returns a reference to <code>this</code> for easy method chaining 1049 */ 1050 public LocationHoursOfOperationComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 1051 this.daysOfWeek = theDaysOfWeek; 1052 return this; 1053 } 1054 1055 public boolean hasDaysOfWeek() { 1056 if (this.daysOfWeek == null) 1057 return false; 1058 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 1059 if (!item.isEmpty()) 1060 return true; 1061 return false; 1062 } 1063 1064 /** 1065 * @return {@link #daysOfWeek} (Indicates which days of the week are available 1066 * between the start and end Times.) 1067 */ 1068 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {// 2 1069 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1070 if (this.daysOfWeek == null) 1071 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1072 this.daysOfWeek.add(t); 1073 return t; 1074 } 1075 1076 /** 1077 * @param value {@link #daysOfWeek} (Indicates which days of the week are 1078 * available between the start and end Times.) 1079 */ 1080 public LocationHoursOfOperationComponent addDaysOfWeek(DaysOfWeek value) { // 1 1081 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 1082 t.setValue(value); 1083 if (this.daysOfWeek == null) 1084 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1085 this.daysOfWeek.add(t); 1086 return this; 1087 } 1088 1089 /** 1090 * @param value {@link #daysOfWeek} (Indicates which days of the week are 1091 * available between the start and end Times.) 1092 */ 1093 public boolean hasDaysOfWeek(DaysOfWeek value) { 1094 if (this.daysOfWeek == null) 1095 return false; 1096 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 1097 if (v.getValue().equals(value)) // code 1098 return true; 1099 return false; 1100 } 1101 1102 /** 1103 * @return {@link #allDay} (The Location is open all day.). This is the 1104 * underlying object with id, value and extensions. The accessor 1105 * "getAllDay" gives direct access to the value 1106 */ 1107 public BooleanType getAllDayElement() { 1108 if (this.allDay == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.allDay"); 1111 else if (Configuration.doAutoCreate()) 1112 this.allDay = new BooleanType(); // bb 1113 return this.allDay; 1114 } 1115 1116 public boolean hasAllDayElement() { 1117 return this.allDay != null && !this.allDay.isEmpty(); 1118 } 1119 1120 public boolean hasAllDay() { 1121 return this.allDay != null && !this.allDay.isEmpty(); 1122 } 1123 1124 /** 1125 * @param value {@link #allDay} (The Location is open all day.). This is the 1126 * underlying object with id, value and extensions. The accessor 1127 * "getAllDay" gives direct access to the value 1128 */ 1129 public LocationHoursOfOperationComponent setAllDayElement(BooleanType value) { 1130 this.allDay = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return The Location is open all day. 1136 */ 1137 public boolean getAllDay() { 1138 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 1139 } 1140 1141 /** 1142 * @param value The Location is open all day. 1143 */ 1144 public LocationHoursOfOperationComponent setAllDay(boolean value) { 1145 if (this.allDay == null) 1146 this.allDay = new BooleanType(); 1147 this.allDay.setValue(value); 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #openingTime} (Time that the Location opens.). This is the 1153 * underlying object with id, value and extensions. The accessor 1154 * "getOpeningTime" gives direct access to the value 1155 */ 1156 public TimeType getOpeningTimeElement() { 1157 if (this.openingTime == null) 1158 if (Configuration.errorOnAutoCreate()) 1159 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.openingTime"); 1160 else if (Configuration.doAutoCreate()) 1161 this.openingTime = new TimeType(); // bb 1162 return this.openingTime; 1163 } 1164 1165 public boolean hasOpeningTimeElement() { 1166 return this.openingTime != null && !this.openingTime.isEmpty(); 1167 } 1168 1169 public boolean hasOpeningTime() { 1170 return this.openingTime != null && !this.openingTime.isEmpty(); 1171 } 1172 1173 /** 1174 * @param value {@link #openingTime} (Time that the Location opens.). This is 1175 * the underlying object with id, value and extensions. The 1176 * accessor "getOpeningTime" gives direct access to the value 1177 */ 1178 public LocationHoursOfOperationComponent setOpeningTimeElement(TimeType value) { 1179 this.openingTime = value; 1180 return this; 1181 } 1182 1183 /** 1184 * @return Time that the Location opens. 1185 */ 1186 public String getOpeningTime() { 1187 return this.openingTime == null ? null : this.openingTime.getValue(); 1188 } 1189 1190 /** 1191 * @param value Time that the Location opens. 1192 */ 1193 public LocationHoursOfOperationComponent setOpeningTime(String value) { 1194 if (value == null) 1195 this.openingTime = null; 1196 else { 1197 if (this.openingTime == null) 1198 this.openingTime = new TimeType(); 1199 this.openingTime.setValue(value); 1200 } 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #closingTime} (Time that the Location closes.). This is the 1206 * underlying object with id, value and extensions. The accessor 1207 * "getClosingTime" gives direct access to the value 1208 */ 1209 public TimeType getClosingTimeElement() { 1210 if (this.closingTime == null) 1211 if (Configuration.errorOnAutoCreate()) 1212 throw new Error("Attempt to auto-create LocationHoursOfOperationComponent.closingTime"); 1213 else if (Configuration.doAutoCreate()) 1214 this.closingTime = new TimeType(); // bb 1215 return this.closingTime; 1216 } 1217 1218 public boolean hasClosingTimeElement() { 1219 return this.closingTime != null && !this.closingTime.isEmpty(); 1220 } 1221 1222 public boolean hasClosingTime() { 1223 return this.closingTime != null && !this.closingTime.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #closingTime} (Time that the Location closes.). This is 1228 * the underlying object with id, value and extensions. The 1229 * accessor "getClosingTime" gives direct access to the value 1230 */ 1231 public LocationHoursOfOperationComponent setClosingTimeElement(TimeType value) { 1232 this.closingTime = value; 1233 return this; 1234 } 1235 1236 /** 1237 * @return Time that the Location closes. 1238 */ 1239 public String getClosingTime() { 1240 return this.closingTime == null ? null : this.closingTime.getValue(); 1241 } 1242 1243 /** 1244 * @param value Time that the Location closes. 1245 */ 1246 public LocationHoursOfOperationComponent setClosingTime(String value) { 1247 if (value == null) 1248 this.closingTime = null; 1249 else { 1250 if (this.closingTime == null) 1251 this.closingTime = new TimeType(); 1252 this.closingTime.setValue(value); 1253 } 1254 return this; 1255 } 1256 1257 protected void listChildren(List<Property> children) { 1258 super.listChildren(children); 1259 children.add(new Property("daysOfWeek", "code", 1260 "Indicates which days of the week are available between the start and end Times.", 0, 1261 java.lang.Integer.MAX_VALUE, daysOfWeek)); 1262 children.add(new Property("allDay", "boolean", "The Location is open all day.", 0, 1, allDay)); 1263 children.add(new Property("openingTime", "time", "Time that the Location opens.", 0, 1, openingTime)); 1264 children.add(new Property("closingTime", "time", "Time that the Location closes.", 0, 1, closingTime)); 1265 } 1266 1267 @Override 1268 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1269 switch (_hash) { 1270 case 68050338: 1271 /* daysOfWeek */ return new Property("daysOfWeek", "code", 1272 "Indicates which days of the week are available between the start and end Times.", 0, 1273 java.lang.Integer.MAX_VALUE, daysOfWeek); 1274 case -1414913477: 1275 /* allDay */ return new Property("allDay", "boolean", "The Location is open all day.", 0, 1, allDay); 1276 case 84062277: 1277 /* openingTime */ return new Property("openingTime", "time", "Time that the Location opens.", 0, 1, 1278 openingTime); 1279 case 188137762: 1280 /* closingTime */ return new Property("closingTime", "time", "Time that the Location closes.", 0, 1, 1281 closingTime); 1282 default: 1283 return super.getNamedProperty(_hash, _name, _checkValid); 1284 } 1285 1286 } 1287 1288 @Override 1289 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1290 switch (hash) { 1291 case 68050338: 1292 /* daysOfWeek */ return this.daysOfWeek == null ? new Base[0] 1293 : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 1294 case -1414913477: 1295 /* allDay */ return this.allDay == null ? new Base[0] : new Base[] { this.allDay }; // BooleanType 1296 case 84062277: 1297 /* openingTime */ return this.openingTime == null ? new Base[0] : new Base[] { this.openingTime }; // TimeType 1298 case 188137762: 1299 /* closingTime */ return this.closingTime == null ? new Base[0] : new Base[] { this.closingTime }; // TimeType 1300 default: 1301 return super.getProperty(hash, name, checkValid); 1302 } 1303 1304 } 1305 1306 @Override 1307 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1308 switch (hash) { 1309 case 68050338: // daysOfWeek 1310 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 1311 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 1312 return value; 1313 case -1414913477: // allDay 1314 this.allDay = castToBoolean(value); // BooleanType 1315 return value; 1316 case 84062277: // openingTime 1317 this.openingTime = castToTime(value); // TimeType 1318 return value; 1319 case 188137762: // closingTime 1320 this.closingTime = castToTime(value); // TimeType 1321 return value; 1322 default: 1323 return super.setProperty(hash, name, value); 1324 } 1325 1326 } 1327 1328 @Override 1329 public Base setProperty(String name, Base value) throws FHIRException { 1330 if (name.equals("daysOfWeek")) { 1331 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 1332 this.getDaysOfWeek().add((Enumeration) value); 1333 } else if (name.equals("allDay")) { 1334 this.allDay = castToBoolean(value); // BooleanType 1335 } else if (name.equals("openingTime")) { 1336 this.openingTime = castToTime(value); // TimeType 1337 } else if (name.equals("closingTime")) { 1338 this.closingTime = castToTime(value); // TimeType 1339 } else 1340 return super.setProperty(name, value); 1341 return value; 1342 } 1343 1344 @Override 1345 public Base makeProperty(int hash, String name) throws FHIRException { 1346 switch (hash) { 1347 case 68050338: 1348 return addDaysOfWeekElement(); 1349 case -1414913477: 1350 return getAllDayElement(); 1351 case 84062277: 1352 return getOpeningTimeElement(); 1353 case 188137762: 1354 return getClosingTimeElement(); 1355 default: 1356 return super.makeProperty(hash, name); 1357 } 1358 1359 } 1360 1361 @Override 1362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1363 switch (hash) { 1364 case 68050338: 1365 /* daysOfWeek */ return new String[] { "code" }; 1366 case -1414913477: 1367 /* allDay */ return new String[] { "boolean" }; 1368 case 84062277: 1369 /* openingTime */ return new String[] { "time" }; 1370 case 188137762: 1371 /* closingTime */ return new String[] { "time" }; 1372 default: 1373 return super.getTypesForProperty(hash, name); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base addChild(String name) throws FHIRException { 1380 if (name.equals("daysOfWeek")) { 1381 throw new FHIRException("Cannot call addChild on a singleton property Location.daysOfWeek"); 1382 } else if (name.equals("allDay")) { 1383 throw new FHIRException("Cannot call addChild on a singleton property Location.allDay"); 1384 } else if (name.equals("openingTime")) { 1385 throw new FHIRException("Cannot call addChild on a singleton property Location.openingTime"); 1386 } else if (name.equals("closingTime")) { 1387 throw new FHIRException("Cannot call addChild on a singleton property Location.closingTime"); 1388 } else 1389 return super.addChild(name); 1390 } 1391 1392 public LocationHoursOfOperationComponent copy() { 1393 LocationHoursOfOperationComponent dst = new LocationHoursOfOperationComponent(); 1394 copyValues(dst); 1395 return dst; 1396 } 1397 1398 public void copyValues(LocationHoursOfOperationComponent dst) { 1399 super.copyValues(dst); 1400 if (daysOfWeek != null) { 1401 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 1402 for (Enumeration<DaysOfWeek> i : daysOfWeek) 1403 dst.daysOfWeek.add(i.copy()); 1404 } 1405 ; 1406 dst.allDay = allDay == null ? null : allDay.copy(); 1407 dst.openingTime = openingTime == null ? null : openingTime.copy(); 1408 dst.closingTime = closingTime == null ? null : closingTime.copy(); 1409 } 1410 1411 @Override 1412 public boolean equalsDeep(Base other_) { 1413 if (!super.equalsDeep(other_)) 1414 return false; 1415 if (!(other_ instanceof LocationHoursOfOperationComponent)) 1416 return false; 1417 LocationHoursOfOperationComponent o = (LocationHoursOfOperationComponent) other_; 1418 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) 1419 && compareDeep(openingTime, o.openingTime, true) && compareDeep(closingTime, o.closingTime, true); 1420 } 1421 1422 @Override 1423 public boolean equalsShallow(Base other_) { 1424 if (!super.equalsShallow(other_)) 1425 return false; 1426 if (!(other_ instanceof LocationHoursOfOperationComponent)) 1427 return false; 1428 LocationHoursOfOperationComponent o = (LocationHoursOfOperationComponent) other_; 1429 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) 1430 && compareValues(openingTime, o.openingTime, true) && compareValues(closingTime, o.closingTime, true); 1431 } 1432 1433 public boolean isEmpty() { 1434 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, openingTime, closingTime); 1435 } 1436 1437 public String fhirType() { 1438 return "Location.hoursOfOperation"; 1439 1440 } 1441 1442 } 1443 1444 /** 1445 * Unique code or number identifying the location to its users. 1446 */ 1447 @Child(name = "identifier", type = { 1448 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1449 @Description(shortDefinition = "Unique code or number identifying the location to its users", formalDefinition = "Unique code or number identifying the location to its users.") 1450 protected List<Identifier> identifier; 1451 1452 /** 1453 * The status property covers the general availability of the resource, not the 1454 * current value which may be covered by the operationStatus, or by a 1455 * schedule/slots if they are configured for the location. 1456 */ 1457 @Child(name = "status", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 1458 @Description(shortDefinition = "active | suspended | inactive", formalDefinition = "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.") 1459 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-status") 1460 protected Enumeration<LocationStatus> status; 1461 1462 /** 1463 * The operational status covers operation values most relevant to beds (but can 1464 * also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis 1465 * chair). This typically covers concepts such as contamination, housekeeping, 1466 * and other activities like maintenance. 1467 */ 1468 @Child(name = "operationalStatus", type = { 1469 Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1470 @Description(shortDefinition = "The operational status of the location (typically only for a bed/room)", formalDefinition = "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.") 1471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v2-0116") 1472 protected Coding operationalStatus; 1473 1474 /** 1475 * Name of the location as used by humans. Does not need to be unique. 1476 */ 1477 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1478 @Description(shortDefinition = "Name of the location as used by humans", formalDefinition = "Name of the location as used by humans. Does not need to be unique.") 1479 protected StringType name; 1480 1481 /** 1482 * A list of alternate names that the location is known as, or was known as, in 1483 * the past. 1484 */ 1485 @Child(name = "alias", type = { 1486 StringType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1487 @Description(shortDefinition = "A list of alternate names that the location is known as, or was known as, in the past", formalDefinition = "A list of alternate names that the location is known as, or was known as, in the past.") 1488 protected List<StringType> alias; 1489 1490 /** 1491 * Description of the Location, which helps in finding or referencing the place. 1492 */ 1493 @Child(name = "description", type = { 1494 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1495 @Description(shortDefinition = "Additional details about the location that could be displayed as further information to identify the location beyond its name", formalDefinition = "Description of the Location, which helps in finding or referencing the place.") 1496 protected StringType description; 1497 1498 /** 1499 * Indicates whether a resource instance represents a specific location or a 1500 * class of locations. 1501 */ 1502 @Child(name = "mode", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1503 @Description(shortDefinition = "instance | kind", formalDefinition = "Indicates whether a resource instance represents a specific location or a class of locations.") 1504 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-mode") 1505 protected Enumeration<LocationMode> mode; 1506 1507 /** 1508 * Indicates the type of function performed at the location. 1509 */ 1510 @Child(name = "type", type = { 1511 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1512 @Description(shortDefinition = "Type of function performed", formalDefinition = "Indicates the type of function performed at the location.") 1513 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType") 1514 protected List<CodeableConcept> type; 1515 1516 /** 1517 * The contact details of communication devices available at the location. This 1518 * can include phone numbers, fax numbers, mobile numbers, email addresses and 1519 * web sites. 1520 */ 1521 @Child(name = "telecom", type = { 1522 ContactPoint.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1523 @Description(shortDefinition = "Contact details of the location", formalDefinition = "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.") 1524 protected List<ContactPoint> telecom; 1525 1526 /** 1527 * Physical location. 1528 */ 1529 @Child(name = "address", type = { Address.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1530 @Description(shortDefinition = "Physical location", formalDefinition = "Physical location.") 1531 protected Address address; 1532 1533 /** 1534 * Physical form of the location, e.g. building, room, vehicle, road. 1535 */ 1536 @Child(name = "physicalType", type = { 1537 CodeableConcept.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1538 @Description(shortDefinition = "Physical form of the location", formalDefinition = "Physical form of the location, e.g. building, room, vehicle, road.") 1539 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/location-physical-type") 1540 protected CodeableConcept physicalType; 1541 1542 /** 1543 * The absolute geographic location of the Location, expressed using the WGS84 1544 * datum (This is the same co-ordinate system used in KML). 1545 */ 1546 @Child(name = "position", type = {}, order = 11, min = 0, max = 1, modifier = false, summary = false) 1547 @Description(shortDefinition = "The absolute geographic location", formalDefinition = "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).") 1548 protected LocationPositionComponent position; 1549 1550 /** 1551 * The organization responsible for the provisioning and upkeep of the location. 1552 */ 1553 @Child(name = "managingOrganization", type = { 1554 Organization.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1555 @Description(shortDefinition = "Organization responsible for provisioning and upkeep", formalDefinition = "The organization responsible for the provisioning and upkeep of the location.") 1556 protected Reference managingOrganization; 1557 1558 /** 1559 * The actual object that is the target of the reference (The organization 1560 * responsible for the provisioning and upkeep of the location.) 1561 */ 1562 protected Organization managingOrganizationTarget; 1563 1564 /** 1565 * Another Location of which this Location is physically a part of. 1566 */ 1567 @Child(name = "partOf", type = { Location.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1568 @Description(shortDefinition = "Another Location this one is physically a part of", formalDefinition = "Another Location of which this Location is physically a part of.") 1569 protected Reference partOf; 1570 1571 /** 1572 * The actual object that is the target of the reference (Another Location of 1573 * which this Location is physically a part of.) 1574 */ 1575 protected Location partOfTarget; 1576 1577 /** 1578 * What days/times during a week is this location usually open. 1579 */ 1580 @Child(name = "hoursOfOperation", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1581 @Description(shortDefinition = "What days/times during a week is this location usually open", formalDefinition = "What days/times during a week is this location usually open.") 1582 protected List<LocationHoursOfOperationComponent> hoursOfOperation; 1583 1584 /** 1585 * A description of when the locations opening ours are different to normal, 1586 * e.g. public holiday availability. Succinctly describing all possible 1587 * exceptions to normal site availability as detailed in the opening hours 1588 * Times. 1589 */ 1590 @Child(name = "availabilityExceptions", type = { 1591 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1592 @Description(shortDefinition = "Description of availability exceptions", formalDefinition = "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.") 1593 protected StringType availabilityExceptions; 1594 1595 /** 1596 * Technical endpoints providing access to services operated for the location. 1597 */ 1598 @Child(name = "endpoint", type = { 1599 Endpoint.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1600 @Description(shortDefinition = "Technical endpoints providing access to services operated for the location", formalDefinition = "Technical endpoints providing access to services operated for the location.") 1601 protected List<Reference> endpoint; 1602 /** 1603 * The actual objects that are the target of the reference (Technical endpoints 1604 * providing access to services operated for the location.) 1605 */ 1606 protected List<Endpoint> endpointTarget; 1607 1608 private static final long serialVersionUID = -2126621333L; 1609 1610 /** 1611 * Constructor 1612 */ 1613 public Location() { 1614 super(); 1615 } 1616 1617 /** 1618 * @return {@link #identifier} (Unique code or number identifying the location 1619 * to its users.) 1620 */ 1621 public List<Identifier> getIdentifier() { 1622 if (this.identifier == null) 1623 this.identifier = new ArrayList<Identifier>(); 1624 return this.identifier; 1625 } 1626 1627 /** 1628 * @return Returns a reference to <code>this</code> for easy method chaining 1629 */ 1630 public Location setIdentifier(List<Identifier> theIdentifier) { 1631 this.identifier = theIdentifier; 1632 return this; 1633 } 1634 1635 public boolean hasIdentifier() { 1636 if (this.identifier == null) 1637 return false; 1638 for (Identifier item : this.identifier) 1639 if (!item.isEmpty()) 1640 return true; 1641 return false; 1642 } 1643 1644 public Identifier addIdentifier() { // 3 1645 Identifier t = new Identifier(); 1646 if (this.identifier == null) 1647 this.identifier = new ArrayList<Identifier>(); 1648 this.identifier.add(t); 1649 return t; 1650 } 1651 1652 public Location addIdentifier(Identifier t) { // 3 1653 if (t == null) 1654 return this; 1655 if (this.identifier == null) 1656 this.identifier = new ArrayList<Identifier>(); 1657 this.identifier.add(t); 1658 return this; 1659 } 1660 1661 /** 1662 * @return The first repetition of repeating field {@link #identifier}, creating 1663 * it if it does not already exist 1664 */ 1665 public Identifier getIdentifierFirstRep() { 1666 if (getIdentifier().isEmpty()) { 1667 addIdentifier(); 1668 } 1669 return getIdentifier().get(0); 1670 } 1671 1672 /** 1673 * @return {@link #status} (The status property covers the general availability 1674 * of the resource, not the current value which may be covered by the 1675 * operationStatus, or by a schedule/slots if they are configured for 1676 * the location.). This is the underlying object with id, value and 1677 * extensions. The accessor "getStatus" gives direct access to the value 1678 */ 1679 public Enumeration<LocationStatus> getStatusElement() { 1680 if (this.status == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create Location.status"); 1683 else if (Configuration.doAutoCreate()) 1684 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); // bb 1685 return this.status; 1686 } 1687 1688 public boolean hasStatusElement() { 1689 return this.status != null && !this.status.isEmpty(); 1690 } 1691 1692 public boolean hasStatus() { 1693 return this.status != null && !this.status.isEmpty(); 1694 } 1695 1696 /** 1697 * @param value {@link #status} (The status property covers the general 1698 * availability of the resource, not the current value which may be 1699 * covered by the operationStatus, or by a schedule/slots if they 1700 * are configured for the location.). This is the underlying object 1701 * with id, value and extensions. The accessor "getStatus" gives 1702 * direct access to the value 1703 */ 1704 public Location setStatusElement(Enumeration<LocationStatus> value) { 1705 this.status = value; 1706 return this; 1707 } 1708 1709 /** 1710 * @return The status property covers the general availability of the resource, 1711 * not the current value which may be covered by the operationStatus, or 1712 * by a schedule/slots if they are configured for the location. 1713 */ 1714 public LocationStatus getStatus() { 1715 return this.status == null ? null : this.status.getValue(); 1716 } 1717 1718 /** 1719 * @param value The status property covers the general availability of the 1720 * resource, not the current value which may be covered by the 1721 * operationStatus, or by a schedule/slots if they are configured 1722 * for the location. 1723 */ 1724 public Location setStatus(LocationStatus value) { 1725 if (value == null) 1726 this.status = null; 1727 else { 1728 if (this.status == null) 1729 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); 1730 this.status.setValue(value); 1731 } 1732 return this; 1733 } 1734 1735 /** 1736 * @return {@link #operationalStatus} (The operational status covers operation 1737 * values most relevant to beds (but can also apply to 1738 * rooms/units/chairs/etc. such as an isolation unit/dialysis chair). 1739 * This typically covers concepts such as contamination, housekeeping, 1740 * and other activities like maintenance.) 1741 */ 1742 public Coding getOperationalStatus() { 1743 if (this.operationalStatus == null) 1744 if (Configuration.errorOnAutoCreate()) 1745 throw new Error("Attempt to auto-create Location.operationalStatus"); 1746 else if (Configuration.doAutoCreate()) 1747 this.operationalStatus = new Coding(); // cc 1748 return this.operationalStatus; 1749 } 1750 1751 public boolean hasOperationalStatus() { 1752 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 1753 } 1754 1755 /** 1756 * @param value {@link #operationalStatus} (The operational status covers 1757 * operation values most relevant to beds (but can also apply to 1758 * rooms/units/chairs/etc. such as an isolation unit/dialysis 1759 * chair). This typically covers concepts such as contamination, 1760 * housekeeping, and other activities like maintenance.) 1761 */ 1762 public Location setOperationalStatus(Coding value) { 1763 this.operationalStatus = value; 1764 return this; 1765 } 1766 1767 /** 1768 * @return {@link #name} (Name of the location as used by humans. Does not need 1769 * to be unique.). This is the underlying object with id, value and 1770 * extensions. The accessor "getName" gives direct access to the value 1771 */ 1772 public StringType getNameElement() { 1773 if (this.name == null) 1774 if (Configuration.errorOnAutoCreate()) 1775 throw new Error("Attempt to auto-create Location.name"); 1776 else if (Configuration.doAutoCreate()) 1777 this.name = new StringType(); // bb 1778 return this.name; 1779 } 1780 1781 public boolean hasNameElement() { 1782 return this.name != null && !this.name.isEmpty(); 1783 } 1784 1785 public boolean hasName() { 1786 return this.name != null && !this.name.isEmpty(); 1787 } 1788 1789 /** 1790 * @param value {@link #name} (Name of the location as used by humans. Does not 1791 * need to be unique.). This is the underlying object with id, 1792 * value and extensions. The accessor "getName" gives direct access 1793 * to the value 1794 */ 1795 public Location setNameElement(StringType value) { 1796 this.name = value; 1797 return this; 1798 } 1799 1800 /** 1801 * @return Name of the location as used by humans. Does not need to be unique. 1802 */ 1803 public String getName() { 1804 return this.name == null ? null : this.name.getValue(); 1805 } 1806 1807 /** 1808 * @param value Name of the location as used by humans. Does not need to be 1809 * unique. 1810 */ 1811 public Location setName(String value) { 1812 if (Utilities.noString(value)) 1813 this.name = null; 1814 else { 1815 if (this.name == null) 1816 this.name = new StringType(); 1817 this.name.setValue(value); 1818 } 1819 return this; 1820 } 1821 1822 /** 1823 * @return {@link #alias} (A list of alternate names that the location is known 1824 * as, or was known as, in the past.) 1825 */ 1826 public List<StringType> getAlias() { 1827 if (this.alias == null) 1828 this.alias = new ArrayList<StringType>(); 1829 return this.alias; 1830 } 1831 1832 /** 1833 * @return Returns a reference to <code>this</code> for easy method chaining 1834 */ 1835 public Location setAlias(List<StringType> theAlias) { 1836 this.alias = theAlias; 1837 return this; 1838 } 1839 1840 public boolean hasAlias() { 1841 if (this.alias == null) 1842 return false; 1843 for (StringType item : this.alias) 1844 if (!item.isEmpty()) 1845 return true; 1846 return false; 1847 } 1848 1849 /** 1850 * @return {@link #alias} (A list of alternate names that the location is known 1851 * as, or was known as, in the past.) 1852 */ 1853 public StringType addAliasElement() {// 2 1854 StringType t = new StringType(); 1855 if (this.alias == null) 1856 this.alias = new ArrayList<StringType>(); 1857 this.alias.add(t); 1858 return t; 1859 } 1860 1861 /** 1862 * @param value {@link #alias} (A list of alternate names that the location is 1863 * known as, or was known as, in the past.) 1864 */ 1865 public Location addAlias(String value) { // 1 1866 StringType t = new StringType(); 1867 t.setValue(value); 1868 if (this.alias == null) 1869 this.alias = new ArrayList<StringType>(); 1870 this.alias.add(t); 1871 return this; 1872 } 1873 1874 /** 1875 * @param value {@link #alias} (A list of alternate names that the location is 1876 * known as, or was known as, in the past.) 1877 */ 1878 public boolean hasAlias(String value) { 1879 if (this.alias == null) 1880 return false; 1881 for (StringType v : this.alias) 1882 if (v.getValue().equals(value)) // string 1883 return true; 1884 return false; 1885 } 1886 1887 /** 1888 * @return {@link #description} (Description of the Location, which helps in 1889 * finding or referencing the place.). This is the underlying object 1890 * with id, value and extensions. The accessor "getDescription" gives 1891 * direct access to the value 1892 */ 1893 public StringType getDescriptionElement() { 1894 if (this.description == null) 1895 if (Configuration.errorOnAutoCreate()) 1896 throw new Error("Attempt to auto-create Location.description"); 1897 else if (Configuration.doAutoCreate()) 1898 this.description = new StringType(); // bb 1899 return this.description; 1900 } 1901 1902 public boolean hasDescriptionElement() { 1903 return this.description != null && !this.description.isEmpty(); 1904 } 1905 1906 public boolean hasDescription() { 1907 return this.description != null && !this.description.isEmpty(); 1908 } 1909 1910 /** 1911 * @param value {@link #description} (Description of the Location, which helps 1912 * in finding or referencing the place.). This is the underlying 1913 * object with id, value and extensions. The accessor 1914 * "getDescription" gives direct access to the value 1915 */ 1916 public Location setDescriptionElement(StringType value) { 1917 this.description = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return Description of the Location, which helps in finding or referencing 1923 * the place. 1924 */ 1925 public String getDescription() { 1926 return this.description == null ? null : this.description.getValue(); 1927 } 1928 1929 /** 1930 * @param value Description of the Location, which helps in finding or 1931 * referencing the place. 1932 */ 1933 public Location setDescription(String value) { 1934 if (Utilities.noString(value)) 1935 this.description = null; 1936 else { 1937 if (this.description == null) 1938 this.description = new StringType(); 1939 this.description.setValue(value); 1940 } 1941 return this; 1942 } 1943 1944 /** 1945 * @return {@link #mode} (Indicates whether a resource instance represents a 1946 * specific location or a class of locations.). This is the underlying 1947 * object with id, value and extensions. The accessor "getMode" gives 1948 * direct access to the value 1949 */ 1950 public Enumeration<LocationMode> getModeElement() { 1951 if (this.mode == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create Location.mode"); 1954 else if (Configuration.doAutoCreate()) 1955 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); // bb 1956 return this.mode; 1957 } 1958 1959 public boolean hasModeElement() { 1960 return this.mode != null && !this.mode.isEmpty(); 1961 } 1962 1963 public boolean hasMode() { 1964 return this.mode != null && !this.mode.isEmpty(); 1965 } 1966 1967 /** 1968 * @param value {@link #mode} (Indicates whether a resource instance represents 1969 * a specific location or a class of locations.). This is the 1970 * underlying object with id, value and extensions. The accessor 1971 * "getMode" gives direct access to the value 1972 */ 1973 public Location setModeElement(Enumeration<LocationMode> value) { 1974 this.mode = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return Indicates whether a resource instance represents a specific location 1980 * or a class of locations. 1981 */ 1982 public LocationMode getMode() { 1983 return this.mode == null ? null : this.mode.getValue(); 1984 } 1985 1986 /** 1987 * @param value Indicates whether a resource instance represents a specific 1988 * location or a class of locations. 1989 */ 1990 public Location setMode(LocationMode value) { 1991 if (value == null) 1992 this.mode = null; 1993 else { 1994 if (this.mode == null) 1995 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); 1996 this.mode.setValue(value); 1997 } 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #type} (Indicates the type of function performed at the 2003 * location.) 2004 */ 2005 public List<CodeableConcept> getType() { 2006 if (this.type == null) 2007 this.type = new ArrayList<CodeableConcept>(); 2008 return this.type; 2009 } 2010 2011 /** 2012 * @return Returns a reference to <code>this</code> for easy method chaining 2013 */ 2014 public Location setType(List<CodeableConcept> theType) { 2015 this.type = theType; 2016 return this; 2017 } 2018 2019 public boolean hasType() { 2020 if (this.type == null) 2021 return false; 2022 for (CodeableConcept item : this.type) 2023 if (!item.isEmpty()) 2024 return true; 2025 return false; 2026 } 2027 2028 public CodeableConcept addType() { // 3 2029 CodeableConcept t = new CodeableConcept(); 2030 if (this.type == null) 2031 this.type = new ArrayList<CodeableConcept>(); 2032 this.type.add(t); 2033 return t; 2034 } 2035 2036 public Location addType(CodeableConcept t) { // 3 2037 if (t == null) 2038 return this; 2039 if (this.type == null) 2040 this.type = new ArrayList<CodeableConcept>(); 2041 this.type.add(t); 2042 return this; 2043 } 2044 2045 /** 2046 * @return The first repetition of repeating field {@link #type}, creating it if 2047 * it does not already exist 2048 */ 2049 public CodeableConcept getTypeFirstRep() { 2050 if (getType().isEmpty()) { 2051 addType(); 2052 } 2053 return getType().get(0); 2054 } 2055 2056 /** 2057 * @return {@link #telecom} (The contact details of communication devices 2058 * available at the location. This can include phone numbers, fax 2059 * numbers, mobile numbers, email addresses and web sites.) 2060 */ 2061 public List<ContactPoint> getTelecom() { 2062 if (this.telecom == null) 2063 this.telecom = new ArrayList<ContactPoint>(); 2064 return this.telecom; 2065 } 2066 2067 /** 2068 * @return Returns a reference to <code>this</code> for easy method chaining 2069 */ 2070 public Location setTelecom(List<ContactPoint> theTelecom) { 2071 this.telecom = theTelecom; 2072 return this; 2073 } 2074 2075 public boolean hasTelecom() { 2076 if (this.telecom == null) 2077 return false; 2078 for (ContactPoint item : this.telecom) 2079 if (!item.isEmpty()) 2080 return true; 2081 return false; 2082 } 2083 2084 public ContactPoint addTelecom() { // 3 2085 ContactPoint t = new ContactPoint(); 2086 if (this.telecom == null) 2087 this.telecom = new ArrayList<ContactPoint>(); 2088 this.telecom.add(t); 2089 return t; 2090 } 2091 2092 public Location addTelecom(ContactPoint t) { // 3 2093 if (t == null) 2094 return this; 2095 if (this.telecom == null) 2096 this.telecom = new ArrayList<ContactPoint>(); 2097 this.telecom.add(t); 2098 return this; 2099 } 2100 2101 /** 2102 * @return The first repetition of repeating field {@link #telecom}, creating it 2103 * if it does not already exist 2104 */ 2105 public ContactPoint getTelecomFirstRep() { 2106 if (getTelecom().isEmpty()) { 2107 addTelecom(); 2108 } 2109 return getTelecom().get(0); 2110 } 2111 2112 /** 2113 * @return {@link #address} (Physical location.) 2114 */ 2115 public Address getAddress() { 2116 if (this.address == null) 2117 if (Configuration.errorOnAutoCreate()) 2118 throw new Error("Attempt to auto-create Location.address"); 2119 else if (Configuration.doAutoCreate()) 2120 this.address = new Address(); // cc 2121 return this.address; 2122 } 2123 2124 public boolean hasAddress() { 2125 return this.address != null && !this.address.isEmpty(); 2126 } 2127 2128 /** 2129 * @param value {@link #address} (Physical location.) 2130 */ 2131 public Location setAddress(Address value) { 2132 this.address = value; 2133 return this; 2134 } 2135 2136 /** 2137 * @return {@link #physicalType} (Physical form of the location, e.g. building, 2138 * room, vehicle, road.) 2139 */ 2140 public CodeableConcept getPhysicalType() { 2141 if (this.physicalType == null) 2142 if (Configuration.errorOnAutoCreate()) 2143 throw new Error("Attempt to auto-create Location.physicalType"); 2144 else if (Configuration.doAutoCreate()) 2145 this.physicalType = new CodeableConcept(); // cc 2146 return this.physicalType; 2147 } 2148 2149 public boolean hasPhysicalType() { 2150 return this.physicalType != null && !this.physicalType.isEmpty(); 2151 } 2152 2153 /** 2154 * @param value {@link #physicalType} (Physical form of the location, e.g. 2155 * building, room, vehicle, road.) 2156 */ 2157 public Location setPhysicalType(CodeableConcept value) { 2158 this.physicalType = value; 2159 return this; 2160 } 2161 2162 /** 2163 * @return {@link #position} (The absolute geographic location of the Location, 2164 * expressed using the WGS84 datum (This is the same co-ordinate system 2165 * used in KML).) 2166 */ 2167 public LocationPositionComponent getPosition() { 2168 if (this.position == null) 2169 if (Configuration.errorOnAutoCreate()) 2170 throw new Error("Attempt to auto-create Location.position"); 2171 else if (Configuration.doAutoCreate()) 2172 this.position = new LocationPositionComponent(); // cc 2173 return this.position; 2174 } 2175 2176 public boolean hasPosition() { 2177 return this.position != null && !this.position.isEmpty(); 2178 } 2179 2180 /** 2181 * @param value {@link #position} (The absolute geographic location of the 2182 * Location, expressed using the WGS84 datum (This is the same 2183 * co-ordinate system used in KML).) 2184 */ 2185 public Location setPosition(LocationPositionComponent value) { 2186 this.position = value; 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #managingOrganization} (The organization responsible for the 2192 * provisioning and upkeep of the location.) 2193 */ 2194 public Reference getManagingOrganization() { 2195 if (this.managingOrganization == null) 2196 if (Configuration.errorOnAutoCreate()) 2197 throw new Error("Attempt to auto-create Location.managingOrganization"); 2198 else if (Configuration.doAutoCreate()) 2199 this.managingOrganization = new Reference(); // cc 2200 return this.managingOrganization; 2201 } 2202 2203 public boolean hasManagingOrganization() { 2204 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 2205 } 2206 2207 /** 2208 * @param value {@link #managingOrganization} (The organization responsible for 2209 * the provisioning and upkeep of the location.) 2210 */ 2211 public Location setManagingOrganization(Reference value) { 2212 this.managingOrganization = value; 2213 return this; 2214 } 2215 2216 /** 2217 * @return {@link #managingOrganization} The actual object that is the target of 2218 * the reference. The reference library doesn't populate this, but you 2219 * can use it to hold the resource if you resolve it. (The organization 2220 * responsible for the provisioning and upkeep of the location.) 2221 */ 2222 public Organization getManagingOrganizationTarget() { 2223 if (this.managingOrganizationTarget == null) 2224 if (Configuration.errorOnAutoCreate()) 2225 throw new Error("Attempt to auto-create Location.managingOrganization"); 2226 else if (Configuration.doAutoCreate()) 2227 this.managingOrganizationTarget = new Organization(); // aa 2228 return this.managingOrganizationTarget; 2229 } 2230 2231 /** 2232 * @param value {@link #managingOrganization} The actual object that is the 2233 * target of the reference. The reference library doesn't use 2234 * these, but you can use it to hold the resource if you resolve 2235 * it. (The organization responsible for the provisioning and 2236 * upkeep of the location.) 2237 */ 2238 public Location setManagingOrganizationTarget(Organization value) { 2239 this.managingOrganizationTarget = value; 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #partOf} (Another Location of which this Location is 2245 * physically a part of.) 2246 */ 2247 public Reference getPartOf() { 2248 if (this.partOf == null) 2249 if (Configuration.errorOnAutoCreate()) 2250 throw new Error("Attempt to auto-create Location.partOf"); 2251 else if (Configuration.doAutoCreate()) 2252 this.partOf = new Reference(); // cc 2253 return this.partOf; 2254 } 2255 2256 public boolean hasPartOf() { 2257 return this.partOf != null && !this.partOf.isEmpty(); 2258 } 2259 2260 /** 2261 * @param value {@link #partOf} (Another Location of which this Location is 2262 * physically a part of.) 2263 */ 2264 public Location setPartOf(Reference value) { 2265 this.partOf = value; 2266 return this; 2267 } 2268 2269 /** 2270 * @return {@link #partOf} The actual object that is the target of the 2271 * reference. The reference library doesn't populate this, but you can 2272 * use it to hold the resource if you resolve it. (Another Location of 2273 * which this Location is physically a part of.) 2274 */ 2275 public Location getPartOfTarget() { 2276 if (this.partOfTarget == null) 2277 if (Configuration.errorOnAutoCreate()) 2278 throw new Error("Attempt to auto-create Location.partOf"); 2279 else if (Configuration.doAutoCreate()) 2280 this.partOfTarget = new Location(); // aa 2281 return this.partOfTarget; 2282 } 2283 2284 /** 2285 * @param value {@link #partOf} The actual object that is the target of the 2286 * reference. The reference library doesn't use these, but you can 2287 * use it to hold the resource if you resolve it. (Another Location 2288 * of which this Location is physically a part of.) 2289 */ 2290 public Location setPartOfTarget(Location value) { 2291 this.partOfTarget = value; 2292 return this; 2293 } 2294 2295 /** 2296 * @return {@link #hoursOfOperation} (What days/times during a week is this 2297 * location usually open.) 2298 */ 2299 public List<LocationHoursOfOperationComponent> getHoursOfOperation() { 2300 if (this.hoursOfOperation == null) 2301 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2302 return this.hoursOfOperation; 2303 } 2304 2305 /** 2306 * @return Returns a reference to <code>this</code> for easy method chaining 2307 */ 2308 public Location setHoursOfOperation(List<LocationHoursOfOperationComponent> theHoursOfOperation) { 2309 this.hoursOfOperation = theHoursOfOperation; 2310 return this; 2311 } 2312 2313 public boolean hasHoursOfOperation() { 2314 if (this.hoursOfOperation == null) 2315 return false; 2316 for (LocationHoursOfOperationComponent item : this.hoursOfOperation) 2317 if (!item.isEmpty()) 2318 return true; 2319 return false; 2320 } 2321 2322 public LocationHoursOfOperationComponent addHoursOfOperation() { // 3 2323 LocationHoursOfOperationComponent t = new LocationHoursOfOperationComponent(); 2324 if (this.hoursOfOperation == null) 2325 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2326 this.hoursOfOperation.add(t); 2327 return t; 2328 } 2329 2330 public Location addHoursOfOperation(LocationHoursOfOperationComponent t) { // 3 2331 if (t == null) 2332 return this; 2333 if (this.hoursOfOperation == null) 2334 this.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2335 this.hoursOfOperation.add(t); 2336 return this; 2337 } 2338 2339 /** 2340 * @return The first repetition of repeating field {@link #hoursOfOperation}, 2341 * creating it if it does not already exist 2342 */ 2343 public LocationHoursOfOperationComponent getHoursOfOperationFirstRep() { 2344 if (getHoursOfOperation().isEmpty()) { 2345 addHoursOfOperation(); 2346 } 2347 return getHoursOfOperation().get(0); 2348 } 2349 2350 /** 2351 * @return {@link #availabilityExceptions} (A description of when the locations 2352 * opening ours are different to normal, e.g. public holiday 2353 * availability. Succinctly describing all possible exceptions to normal 2354 * site availability as detailed in the opening hours Times.). This is 2355 * the underlying object with id, value and extensions. The accessor 2356 * "getAvailabilityExceptions" gives direct access to the value 2357 */ 2358 public StringType getAvailabilityExceptionsElement() { 2359 if (this.availabilityExceptions == null) 2360 if (Configuration.errorOnAutoCreate()) 2361 throw new Error("Attempt to auto-create Location.availabilityExceptions"); 2362 else if (Configuration.doAutoCreate()) 2363 this.availabilityExceptions = new StringType(); // bb 2364 return this.availabilityExceptions; 2365 } 2366 2367 public boolean hasAvailabilityExceptionsElement() { 2368 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2369 } 2370 2371 public boolean hasAvailabilityExceptions() { 2372 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2373 } 2374 2375 /** 2376 * @param value {@link #availabilityExceptions} (A description of when the 2377 * locations opening ours are different to normal, e.g. public 2378 * holiday availability. Succinctly describing all possible 2379 * exceptions to normal site availability as detailed in the 2380 * opening hours Times.). This is the underlying object with id, 2381 * value and extensions. The accessor "getAvailabilityExceptions" 2382 * gives direct access to the value 2383 */ 2384 public Location setAvailabilityExceptionsElement(StringType value) { 2385 this.availabilityExceptions = value; 2386 return this; 2387 } 2388 2389 /** 2390 * @return A description of when the locations opening ours are different to 2391 * normal, e.g. public holiday availability. Succinctly describing all 2392 * possible exceptions to normal site availability as detailed in the 2393 * opening hours Times. 2394 */ 2395 public String getAvailabilityExceptions() { 2396 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2397 } 2398 2399 /** 2400 * @param value A description of when the locations opening ours are different 2401 * to normal, e.g. public holiday availability. Succinctly 2402 * describing all possible exceptions to normal site availability 2403 * as detailed in the opening hours Times. 2404 */ 2405 public Location setAvailabilityExceptions(String value) { 2406 if (Utilities.noString(value)) 2407 this.availabilityExceptions = null; 2408 else { 2409 if (this.availabilityExceptions == null) 2410 this.availabilityExceptions = new StringType(); 2411 this.availabilityExceptions.setValue(value); 2412 } 2413 return this; 2414 } 2415 2416 /** 2417 * @return {@link #endpoint} (Technical endpoints providing access to services 2418 * operated for the location.) 2419 */ 2420 public List<Reference> getEndpoint() { 2421 if (this.endpoint == null) 2422 this.endpoint = new ArrayList<Reference>(); 2423 return this.endpoint; 2424 } 2425 2426 /** 2427 * @return Returns a reference to <code>this</code> for easy method chaining 2428 */ 2429 public Location setEndpoint(List<Reference> theEndpoint) { 2430 this.endpoint = theEndpoint; 2431 return this; 2432 } 2433 2434 public boolean hasEndpoint() { 2435 if (this.endpoint == null) 2436 return false; 2437 for (Reference item : this.endpoint) 2438 if (!item.isEmpty()) 2439 return true; 2440 return false; 2441 } 2442 2443 public Reference addEndpoint() { // 3 2444 Reference t = new Reference(); 2445 if (this.endpoint == null) 2446 this.endpoint = new ArrayList<Reference>(); 2447 this.endpoint.add(t); 2448 return t; 2449 } 2450 2451 public Location addEndpoint(Reference t) { // 3 2452 if (t == null) 2453 return this; 2454 if (this.endpoint == null) 2455 this.endpoint = new ArrayList<Reference>(); 2456 this.endpoint.add(t); 2457 return this; 2458 } 2459 2460 /** 2461 * @return The first repetition of repeating field {@link #endpoint}, creating 2462 * it if it does not already exist 2463 */ 2464 public Reference getEndpointFirstRep() { 2465 if (getEndpoint().isEmpty()) { 2466 addEndpoint(); 2467 } 2468 return getEndpoint().get(0); 2469 } 2470 2471 /** 2472 * @deprecated Use Reference#setResource(IBaseResource) instead 2473 */ 2474 @Deprecated 2475 public List<Endpoint> getEndpointTarget() { 2476 if (this.endpointTarget == null) 2477 this.endpointTarget = new ArrayList<Endpoint>(); 2478 return this.endpointTarget; 2479 } 2480 2481 /** 2482 * @deprecated Use Reference#setResource(IBaseResource) instead 2483 */ 2484 @Deprecated 2485 public Endpoint addEndpointTarget() { 2486 Endpoint r = new Endpoint(); 2487 if (this.endpointTarget == null) 2488 this.endpointTarget = new ArrayList<Endpoint>(); 2489 this.endpointTarget.add(r); 2490 return r; 2491 } 2492 2493 protected void listChildren(List<Property> children) { 2494 super.listChildren(children); 2495 children.add(new Property("identifier", "Identifier", 2496 "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2497 children.add(new Property("status", "code", 2498 "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 2499 0, 1, status)); 2500 children.add(new Property("operationalStatus", "Coding", 2501 "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 2502 0, 1, operationalStatus)); 2503 children.add(new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 2504 0, 1, name)); 2505 children.add(new Property("alias", "string", 2506 "A list of alternate names that the location is known as, or was known as, in the past.", 0, 2507 java.lang.Integer.MAX_VALUE, alias)); 2508 children.add(new Property("description", "string", 2509 "Description of the Location, which helps in finding or referencing the place.", 0, 1, description)); 2510 children.add(new Property("mode", "code", 2511 "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode)); 2512 children.add(new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, 2513 java.lang.Integer.MAX_VALUE, type)); 2514 children.add(new Property("telecom", "ContactPoint", 2515 "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 2516 0, java.lang.Integer.MAX_VALUE, telecom)); 2517 children.add(new Property("address", "Address", "Physical location.", 0, 1, address)); 2518 children.add(new Property("physicalType", "CodeableConcept", 2519 "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType)); 2520 children.add(new Property("position", "", 2521 "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 2522 0, 1, position)); 2523 children.add(new Property("managingOrganization", "Reference(Organization)", 2524 "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization)); 2525 children.add(new Property("partOf", "Reference(Location)", 2526 "Another Location of which this Location is physically a part of.", 0, 1, partOf)); 2527 children.add(new Property("hoursOfOperation", "", "What days/times during a week is this location usually open.", 0, 2528 java.lang.Integer.MAX_VALUE, hoursOfOperation)); 2529 children.add(new Property("availabilityExceptions", "string", 2530 "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.", 2531 0, 1, availabilityExceptions)); 2532 children.add(new Property("endpoint", "Reference(Endpoint)", 2533 "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, 2534 endpoint)); 2535 } 2536 2537 @Override 2538 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2539 switch (_hash) { 2540 case -1618432855: 2541 /* identifier */ return new Property("identifier", "Identifier", 2542 "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier); 2543 case -892481550: 2544 /* status */ return new Property("status", "code", 2545 "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 2546 0, 1, status); 2547 case -2103166364: 2548 /* operationalStatus */ return new Property("operationalStatus", "Coding", 2549 "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 2550 0, 1, operationalStatus); 2551 case 3373707: 2552 /* name */ return new Property("name", "string", 2553 "Name of the location as used by humans. Does not need to be unique.", 0, 1, name); 2554 case 92902992: 2555 /* alias */ return new Property("alias", "string", 2556 "A list of alternate names that the location is known as, or was known as, in the past.", 0, 2557 java.lang.Integer.MAX_VALUE, alias); 2558 case -1724546052: 2559 /* description */ return new Property("description", "string", 2560 "Description of the Location, which helps in finding or referencing the place.", 0, 1, description); 2561 case 3357091: 2562 /* mode */ return new Property("mode", "code", 2563 "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode); 2564 case 3575610: 2565 /* type */ return new Property("type", "CodeableConcept", 2566 "Indicates the type of function performed at the location.", 0, java.lang.Integer.MAX_VALUE, type); 2567 case -1429363305: 2568 /* telecom */ return new Property("telecom", "ContactPoint", 2569 "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 2570 0, java.lang.Integer.MAX_VALUE, telecom); 2571 case -1147692044: 2572 /* address */ return new Property("address", "Address", "Physical location.", 0, 1, address); 2573 case -1474715471: 2574 /* physicalType */ return new Property("physicalType", "CodeableConcept", 2575 "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType); 2576 case 747804969: 2577 /* position */ return new Property("position", "", 2578 "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 2579 0, 1, position); 2580 case -2058947787: 2581 /* managingOrganization */ return new Property("managingOrganization", "Reference(Organization)", 2582 "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization); 2583 case -995410646: 2584 /* partOf */ return new Property("partOf", "Reference(Location)", 2585 "Another Location of which this Location is physically a part of.", 0, 1, partOf); 2586 case -1588872511: 2587 /* hoursOfOperation */ return new Property("hoursOfOperation", "", 2588 "What days/times during a week is this location usually open.", 0, java.lang.Integer.MAX_VALUE, 2589 hoursOfOperation); 2590 case -1149143617: 2591 /* availabilityExceptions */ return new Property("availabilityExceptions", "string", 2592 "A description of when the locations opening ours are different to normal, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as detailed in the opening hours Times.", 2593 0, 1, availabilityExceptions); 2594 case 1741102485: 2595 /* endpoint */ return new Property("endpoint", "Reference(Endpoint)", 2596 "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, 2597 endpoint); 2598 default: 2599 return super.getNamedProperty(_hash, _name, _checkValid); 2600 } 2601 2602 } 2603 2604 @Override 2605 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2606 switch (hash) { 2607 case -1618432855: 2608 /* identifier */ return this.identifier == null ? new Base[0] 2609 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2610 case -892481550: 2611 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<LocationStatus> 2612 case -2103166364: 2613 /* operationalStatus */ return this.operationalStatus == null ? new Base[0] 2614 : new Base[] { this.operationalStatus }; // Coding 2615 case 3373707: 2616 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2617 case 92902992: 2618 /* alias */ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 2619 case -1724546052: 2620 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 2621 case 3357091: 2622 /* mode */ return this.mode == null ? new Base[0] : new Base[] { this.mode }; // Enumeration<LocationMode> 2623 case 3575610: 2624 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2625 case -1429363305: 2626 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2627 case -1147692044: 2628 /* address */ return this.address == null ? new Base[0] : new Base[] { this.address }; // Address 2629 case -1474715471: 2630 /* physicalType */ return this.physicalType == null ? new Base[0] : new Base[] { this.physicalType }; // CodeableConcept 2631 case 747804969: 2632 /* position */ return this.position == null ? new Base[0] : new Base[] { this.position }; // LocationPositionComponent 2633 case -2058947787: 2634 /* managingOrganization */ return this.managingOrganization == null ? new Base[0] 2635 : new Base[] { this.managingOrganization }; // Reference 2636 case -995410646: 2637 /* partOf */ return this.partOf == null ? new Base[0] : new Base[] { this.partOf }; // Reference 2638 case -1588872511: 2639 /* hoursOfOperation */ return this.hoursOfOperation == null ? new Base[0] 2640 : this.hoursOfOperation.toArray(new Base[this.hoursOfOperation.size()]); // LocationHoursOfOperationComponent 2641 case -1149143617: 2642 /* availabilityExceptions */ return this.availabilityExceptions == null ? new Base[0] 2643 : new Base[] { this.availabilityExceptions }; // StringType 2644 case 1741102485: 2645 /* endpoint */ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2646 default: 2647 return super.getProperty(hash, name, checkValid); 2648 } 2649 2650 } 2651 2652 @Override 2653 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2654 switch (hash) { 2655 case -1618432855: // identifier 2656 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2657 return value; 2658 case -892481550: // status 2659 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 2660 this.status = (Enumeration) value; // Enumeration<LocationStatus> 2661 return value; 2662 case -2103166364: // operationalStatus 2663 this.operationalStatus = castToCoding(value); // Coding 2664 return value; 2665 case 3373707: // name 2666 this.name = castToString(value); // StringType 2667 return value; 2668 case 92902992: // alias 2669 this.getAlias().add(castToString(value)); // StringType 2670 return value; 2671 case -1724546052: // description 2672 this.description = castToString(value); // StringType 2673 return value; 2674 case 3357091: // mode 2675 value = new LocationModeEnumFactory().fromType(castToCode(value)); 2676 this.mode = (Enumeration) value; // Enumeration<LocationMode> 2677 return value; 2678 case 3575610: // type 2679 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2680 return value; 2681 case -1429363305: // telecom 2682 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2683 return value; 2684 case -1147692044: // address 2685 this.address = castToAddress(value); // Address 2686 return value; 2687 case -1474715471: // physicalType 2688 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2689 return value; 2690 case 747804969: // position 2691 this.position = (LocationPositionComponent) value; // LocationPositionComponent 2692 return value; 2693 case -2058947787: // managingOrganization 2694 this.managingOrganization = castToReference(value); // Reference 2695 return value; 2696 case -995410646: // partOf 2697 this.partOf = castToReference(value); // Reference 2698 return value; 2699 case -1588872511: // hoursOfOperation 2700 this.getHoursOfOperation().add((LocationHoursOfOperationComponent) value); // LocationHoursOfOperationComponent 2701 return value; 2702 case -1149143617: // availabilityExceptions 2703 this.availabilityExceptions = castToString(value); // StringType 2704 return value; 2705 case 1741102485: // endpoint 2706 this.getEndpoint().add(castToReference(value)); // Reference 2707 return value; 2708 default: 2709 return super.setProperty(hash, name, value); 2710 } 2711 2712 } 2713 2714 @Override 2715 public Base setProperty(String name, Base value) throws FHIRException { 2716 if (name.equals("identifier")) { 2717 this.getIdentifier().add(castToIdentifier(value)); 2718 } else if (name.equals("status")) { 2719 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 2720 this.status = (Enumeration) value; // Enumeration<LocationStatus> 2721 } else if (name.equals("operationalStatus")) { 2722 this.operationalStatus = castToCoding(value); // Coding 2723 } else if (name.equals("name")) { 2724 this.name = castToString(value); // StringType 2725 } else if (name.equals("alias")) { 2726 this.getAlias().add(castToString(value)); 2727 } else if (name.equals("description")) { 2728 this.description = castToString(value); // StringType 2729 } else if (name.equals("mode")) { 2730 value = new LocationModeEnumFactory().fromType(castToCode(value)); 2731 this.mode = (Enumeration) value; // Enumeration<LocationMode> 2732 } else if (name.equals("type")) { 2733 this.getType().add(castToCodeableConcept(value)); 2734 } else if (name.equals("telecom")) { 2735 this.getTelecom().add(castToContactPoint(value)); 2736 } else if (name.equals("address")) { 2737 this.address = castToAddress(value); // Address 2738 } else if (name.equals("physicalType")) { 2739 this.physicalType = castToCodeableConcept(value); // CodeableConcept 2740 } else if (name.equals("position")) { 2741 this.position = (LocationPositionComponent) value; // LocationPositionComponent 2742 } else if (name.equals("managingOrganization")) { 2743 this.managingOrganization = castToReference(value); // Reference 2744 } else if (name.equals("partOf")) { 2745 this.partOf = castToReference(value); // Reference 2746 } else if (name.equals("hoursOfOperation")) { 2747 this.getHoursOfOperation().add((LocationHoursOfOperationComponent) value); 2748 } else if (name.equals("availabilityExceptions")) { 2749 this.availabilityExceptions = castToString(value); // StringType 2750 } else if (name.equals("endpoint")) { 2751 this.getEndpoint().add(castToReference(value)); 2752 } else 2753 return super.setProperty(name, value); 2754 return value; 2755 } 2756 2757 @Override 2758 public Base makeProperty(int hash, String name) throws FHIRException { 2759 switch (hash) { 2760 case -1618432855: 2761 return addIdentifier(); 2762 case -892481550: 2763 return getStatusElement(); 2764 case -2103166364: 2765 return getOperationalStatus(); 2766 case 3373707: 2767 return getNameElement(); 2768 case 92902992: 2769 return addAliasElement(); 2770 case -1724546052: 2771 return getDescriptionElement(); 2772 case 3357091: 2773 return getModeElement(); 2774 case 3575610: 2775 return addType(); 2776 case -1429363305: 2777 return addTelecom(); 2778 case -1147692044: 2779 return getAddress(); 2780 case -1474715471: 2781 return getPhysicalType(); 2782 case 747804969: 2783 return getPosition(); 2784 case -2058947787: 2785 return getManagingOrganization(); 2786 case -995410646: 2787 return getPartOf(); 2788 case -1588872511: 2789 return addHoursOfOperation(); 2790 case -1149143617: 2791 return getAvailabilityExceptionsElement(); 2792 case 1741102485: 2793 return addEndpoint(); 2794 default: 2795 return super.makeProperty(hash, name); 2796 } 2797 2798 } 2799 2800 @Override 2801 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2802 switch (hash) { 2803 case -1618432855: 2804 /* identifier */ return new String[] { "Identifier" }; 2805 case -892481550: 2806 /* status */ return new String[] { "code" }; 2807 case -2103166364: 2808 /* operationalStatus */ return new String[] { "Coding" }; 2809 case 3373707: 2810 /* name */ return new String[] { "string" }; 2811 case 92902992: 2812 /* alias */ return new String[] { "string" }; 2813 case -1724546052: 2814 /* description */ return new String[] { "string" }; 2815 case 3357091: 2816 /* mode */ return new String[] { "code" }; 2817 case 3575610: 2818 /* type */ return new String[] { "CodeableConcept" }; 2819 case -1429363305: 2820 /* telecom */ return new String[] { "ContactPoint" }; 2821 case -1147692044: 2822 /* address */ return new String[] { "Address" }; 2823 case -1474715471: 2824 /* physicalType */ return new String[] { "CodeableConcept" }; 2825 case 747804969: 2826 /* position */ return new String[] {}; 2827 case -2058947787: 2828 /* managingOrganization */ return new String[] { "Reference" }; 2829 case -995410646: 2830 /* partOf */ return new String[] { "Reference" }; 2831 case -1588872511: 2832 /* hoursOfOperation */ return new String[] {}; 2833 case -1149143617: 2834 /* availabilityExceptions */ return new String[] { "string" }; 2835 case 1741102485: 2836 /* endpoint */ return new String[] { "Reference" }; 2837 default: 2838 return super.getTypesForProperty(hash, name); 2839 } 2840 2841 } 2842 2843 @Override 2844 public Base addChild(String name) throws FHIRException { 2845 if (name.equals("identifier")) { 2846 return addIdentifier(); 2847 } else if (name.equals("status")) { 2848 throw new FHIRException("Cannot call addChild on a singleton property Location.status"); 2849 } else if (name.equals("operationalStatus")) { 2850 this.operationalStatus = new Coding(); 2851 return this.operationalStatus; 2852 } else if (name.equals("name")) { 2853 throw new FHIRException("Cannot call addChild on a singleton property Location.name"); 2854 } else if (name.equals("alias")) { 2855 throw new FHIRException("Cannot call addChild on a singleton property Location.alias"); 2856 } else if (name.equals("description")) { 2857 throw new FHIRException("Cannot call addChild on a singleton property Location.description"); 2858 } else if (name.equals("mode")) { 2859 throw new FHIRException("Cannot call addChild on a singleton property Location.mode"); 2860 } else if (name.equals("type")) { 2861 return addType(); 2862 } else if (name.equals("telecom")) { 2863 return addTelecom(); 2864 } else if (name.equals("address")) { 2865 this.address = new Address(); 2866 return this.address; 2867 } else if (name.equals("physicalType")) { 2868 this.physicalType = new CodeableConcept(); 2869 return this.physicalType; 2870 } else if (name.equals("position")) { 2871 this.position = new LocationPositionComponent(); 2872 return this.position; 2873 } else if (name.equals("managingOrganization")) { 2874 this.managingOrganization = new Reference(); 2875 return this.managingOrganization; 2876 } else if (name.equals("partOf")) { 2877 this.partOf = new Reference(); 2878 return this.partOf; 2879 } else if (name.equals("hoursOfOperation")) { 2880 return addHoursOfOperation(); 2881 } else if (name.equals("availabilityExceptions")) { 2882 throw new FHIRException("Cannot call addChild on a singleton property Location.availabilityExceptions"); 2883 } else if (name.equals("endpoint")) { 2884 return addEndpoint(); 2885 } else 2886 return super.addChild(name); 2887 } 2888 2889 public String fhirType() { 2890 return "Location"; 2891 2892 } 2893 2894 public Location copy() { 2895 Location dst = new Location(); 2896 copyValues(dst); 2897 return dst; 2898 } 2899 2900 public void copyValues(Location dst) { 2901 super.copyValues(dst); 2902 if (identifier != null) { 2903 dst.identifier = new ArrayList<Identifier>(); 2904 for (Identifier i : identifier) 2905 dst.identifier.add(i.copy()); 2906 } 2907 ; 2908 dst.status = status == null ? null : status.copy(); 2909 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 2910 dst.name = name == null ? null : name.copy(); 2911 if (alias != null) { 2912 dst.alias = new ArrayList<StringType>(); 2913 for (StringType i : alias) 2914 dst.alias.add(i.copy()); 2915 } 2916 ; 2917 dst.description = description == null ? null : description.copy(); 2918 dst.mode = mode == null ? null : mode.copy(); 2919 if (type != null) { 2920 dst.type = new ArrayList<CodeableConcept>(); 2921 for (CodeableConcept i : type) 2922 dst.type.add(i.copy()); 2923 } 2924 ; 2925 if (telecom != null) { 2926 dst.telecom = new ArrayList<ContactPoint>(); 2927 for (ContactPoint i : telecom) 2928 dst.telecom.add(i.copy()); 2929 } 2930 ; 2931 dst.address = address == null ? null : address.copy(); 2932 dst.physicalType = physicalType == null ? null : physicalType.copy(); 2933 dst.position = position == null ? null : position.copy(); 2934 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 2935 dst.partOf = partOf == null ? null : partOf.copy(); 2936 if (hoursOfOperation != null) { 2937 dst.hoursOfOperation = new ArrayList<LocationHoursOfOperationComponent>(); 2938 for (LocationHoursOfOperationComponent i : hoursOfOperation) 2939 dst.hoursOfOperation.add(i.copy()); 2940 } 2941 ; 2942 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2943 if (endpoint != null) { 2944 dst.endpoint = new ArrayList<Reference>(); 2945 for (Reference i : endpoint) 2946 dst.endpoint.add(i.copy()); 2947 } 2948 ; 2949 } 2950 2951 protected Location typedCopy() { 2952 return copy(); 2953 } 2954 2955 @Override 2956 public boolean equalsDeep(Base other_) { 2957 if (!super.equalsDeep(other_)) 2958 return false; 2959 if (!(other_ instanceof Location)) 2960 return false; 2961 Location o = (Location) other_; 2962 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 2963 && compareDeep(operationalStatus, o.operationalStatus, true) && compareDeep(name, o.name, true) 2964 && compareDeep(alias, o.alias, true) && compareDeep(description, o.description, true) 2965 && compareDeep(mode, o.mode, true) && compareDeep(type, o.type, true) && compareDeep(telecom, o.telecom, true) 2966 && compareDeep(address, o.address, true) && compareDeep(physicalType, o.physicalType, true) 2967 && compareDeep(position, o.position, true) && compareDeep(managingOrganization, o.managingOrganization, true) 2968 && compareDeep(partOf, o.partOf, true) && compareDeep(hoursOfOperation, o.hoursOfOperation, true) 2969 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) 2970 && compareDeep(endpoint, o.endpoint, true); 2971 } 2972 2973 @Override 2974 public boolean equalsShallow(Base other_) { 2975 if (!super.equalsShallow(other_)) 2976 return false; 2977 if (!(other_ instanceof Location)) 2978 return false; 2979 Location o = (Location) other_; 2980 return compareValues(status, o.status, true) && compareValues(name, o.name, true) 2981 && compareValues(alias, o.alias, true) && compareValues(description, o.description, true) 2982 && compareValues(mode, o.mode, true) && compareValues(availabilityExceptions, o.availabilityExceptions, true); 2983 } 2984 2985 public boolean isEmpty() { 2986 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, operationalStatus, name, alias, 2987 description, mode, type, telecom, address, physicalType, position, managingOrganization, partOf, 2988 hoursOfOperation, availabilityExceptions, endpoint); 2989 } 2990 2991 @Override 2992 public ResourceType getResourceType() { 2993 return ResourceType.Location; 2994 } 2995 2996 /** 2997 * Search parameter: <b>identifier</b> 2998 * <p> 2999 * Description: <b>An identifier for the location</b><br> 3000 * Type: <b>token</b><br> 3001 * Path: <b>Location.identifier</b><br> 3002 * </p> 3003 */ 3004 @SearchParamDefinition(name = "identifier", path = "Location.identifier", description = "An identifier for the location", type = "token") 3005 public static final String SP_IDENTIFIER = "identifier"; 3006 /** 3007 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3008 * <p> 3009 * Description: <b>An identifier for the location</b><br> 3010 * Type: <b>token</b><br> 3011 * Path: <b>Location.identifier</b><br> 3012 * </p> 3013 */ 3014 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3015 SP_IDENTIFIER); 3016 3017 /** 3018 * Search parameter: <b>partof</b> 3019 * <p> 3020 * Description: <b>A location of which this location is a part</b><br> 3021 * Type: <b>reference</b><br> 3022 * Path: <b>Location.partOf</b><br> 3023 * </p> 3024 */ 3025 @SearchParamDefinition(name = "partof", path = "Location.partOf", description = "A location of which this location is a part", type = "reference", target = { 3026 Location.class }) 3027 public static final String SP_PARTOF = "partof"; 3028 /** 3029 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 3030 * <p> 3031 * Description: <b>A location of which this location is a part</b><br> 3032 * Type: <b>reference</b><br> 3033 * Path: <b>Location.partOf</b><br> 3034 * </p> 3035 */ 3036 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3037 SP_PARTOF); 3038 3039 /** 3040 * Constant for fluent queries to be used to add include statements. Specifies 3041 * the path value of "<b>Location:partof</b>". 3042 */ 3043 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include( 3044 "Location:partof").toLocked(); 3045 3046 /** 3047 * Search parameter: <b>address</b> 3048 * <p> 3049 * Description: <b>A (part of the) address of the location</b><br> 3050 * Type: <b>string</b><br> 3051 * Path: <b>Location.address</b><br> 3052 * </p> 3053 */ 3054 @SearchParamDefinition(name = "address", path = "Location.address", description = "A (part of the) address of the location", type = "string") 3055 public static final String SP_ADDRESS = "address"; 3056 /** 3057 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3058 * <p> 3059 * Description: <b>A (part of the) address of the location</b><br> 3060 * Type: <b>string</b><br> 3061 * Path: <b>Location.address</b><br> 3062 * </p> 3063 */ 3064 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam( 3065 SP_ADDRESS); 3066 3067 /** 3068 * Search parameter: <b>address-state</b> 3069 * <p> 3070 * Description: <b>A state specified in an address</b><br> 3071 * Type: <b>string</b><br> 3072 * Path: <b>Location.address.state</b><br> 3073 * </p> 3074 */ 3075 @SearchParamDefinition(name = "address-state", path = "Location.address.state", description = "A state specified in an address", type = "string") 3076 public static final String SP_ADDRESS_STATE = "address-state"; 3077 /** 3078 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3079 * <p> 3080 * Description: <b>A state specified in an address</b><br> 3081 * Type: <b>string</b><br> 3082 * Path: <b>Location.address.state</b><br> 3083 * </p> 3084 */ 3085 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3086 SP_ADDRESS_STATE); 3087 3088 /** 3089 * Search parameter: <b>operational-status</b> 3090 * <p> 3091 * Description: <b>Searches for locations (typically bed/room) that have an 3092 * operational status (e.g. contaminated, housekeeping)</b><br> 3093 * Type: <b>token</b><br> 3094 * Path: <b>Location.operationalStatus</b><br> 3095 * </p> 3096 */ 3097 @SearchParamDefinition(name = "operational-status", path = "Location.operationalStatus", description = "Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)", type = "token") 3098 public static final String SP_OPERATIONAL_STATUS = "operational-status"; 3099 /** 3100 * <b>Fluent Client</b> search parameter constant for <b>operational-status</b> 3101 * <p> 3102 * Description: <b>Searches for locations (typically bed/room) that have an 3103 * operational status (e.g. contaminated, housekeeping)</b><br> 3104 * Type: <b>token</b><br> 3105 * Path: <b>Location.operationalStatus</b><br> 3106 * </p> 3107 */ 3108 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OPERATIONAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3109 SP_OPERATIONAL_STATUS); 3110 3111 /** 3112 * Search parameter: <b>type</b> 3113 * <p> 3114 * Description: <b>A code for the type of location</b><br> 3115 * Type: <b>token</b><br> 3116 * Path: <b>Location.type</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name = "type", path = "Location.type", description = "A code for the type of location", type = "token") 3120 public static final String SP_TYPE = "type"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3123 * <p> 3124 * Description: <b>A code for the type of location</b><br> 3125 * Type: <b>token</b><br> 3126 * Path: <b>Location.type</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3130 SP_TYPE); 3131 3132 /** 3133 * Search parameter: <b>address-postalcode</b> 3134 * <p> 3135 * Description: <b>A postal code specified in an address</b><br> 3136 * Type: <b>string</b><br> 3137 * Path: <b>Location.address.postalCode</b><br> 3138 * </p> 3139 */ 3140 @SearchParamDefinition(name = "address-postalcode", path = "Location.address.postalCode", description = "A postal code specified in an address", type = "string") 3141 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3142 /** 3143 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3144 * <p> 3145 * Description: <b>A postal code specified in an address</b><br> 3146 * Type: <b>string</b><br> 3147 * Path: <b>Location.address.postalCode</b><br> 3148 * </p> 3149 */ 3150 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3151 SP_ADDRESS_POSTALCODE); 3152 3153 /** 3154 * Search parameter: <b>address-country</b> 3155 * <p> 3156 * Description: <b>A country specified in an address</b><br> 3157 * Type: <b>string</b><br> 3158 * Path: <b>Location.address.country</b><br> 3159 * </p> 3160 */ 3161 @SearchParamDefinition(name = "address-country", path = "Location.address.country", description = "A country specified in an address", type = "string") 3162 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3163 /** 3164 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3165 * <p> 3166 * Description: <b>A country specified in an address</b><br> 3167 * Type: <b>string</b><br> 3168 * Path: <b>Location.address.country</b><br> 3169 * </p> 3170 */ 3171 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3172 SP_ADDRESS_COUNTRY); 3173 3174 /** 3175 * Search parameter: <b>endpoint</b> 3176 * <p> 3177 * Description: <b>Technical endpoints providing access to services operated for 3178 * the location</b><br> 3179 * Type: <b>reference</b><br> 3180 * Path: <b>Location.endpoint</b><br> 3181 * </p> 3182 */ 3183 @SearchParamDefinition(name = "endpoint", path = "Location.endpoint", description = "Technical endpoints providing access to services operated for the location", type = "reference", target = { 3184 Endpoint.class }) 3185 public static final String SP_ENDPOINT = "endpoint"; 3186 /** 3187 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3188 * <p> 3189 * Description: <b>Technical endpoints providing access to services operated for 3190 * the location</b><br> 3191 * Type: <b>reference</b><br> 3192 * Path: <b>Location.endpoint</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3196 SP_ENDPOINT); 3197 3198 /** 3199 * Constant for fluent queries to be used to add include statements. Specifies 3200 * the path value of "<b>Location:endpoint</b>". 3201 */ 3202 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include( 3203 "Location:endpoint").toLocked(); 3204 3205 /** 3206 * Search parameter: <b>organization</b> 3207 * <p> 3208 * Description: <b>Searches for locations that are managed by the provided 3209 * organization</b><br> 3210 * Type: <b>reference</b><br> 3211 * Path: <b>Location.managingOrganization</b><br> 3212 * </p> 3213 */ 3214 @SearchParamDefinition(name = "organization", path = "Location.managingOrganization", description = "Searches for locations that are managed by the provided organization", type = "reference", target = { 3215 Organization.class }) 3216 public static final String SP_ORGANIZATION = "organization"; 3217 /** 3218 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 3219 * <p> 3220 * Description: <b>Searches for locations that are managed by the provided 3221 * organization</b><br> 3222 * Type: <b>reference</b><br> 3223 * Path: <b>Location.managingOrganization</b><br> 3224 * </p> 3225 */ 3226 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3227 SP_ORGANIZATION); 3228 3229 /** 3230 * Constant for fluent queries to be used to add include statements. Specifies 3231 * the path value of "<b>Location:organization</b>". 3232 */ 3233 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include( 3234 "Location:organization").toLocked(); 3235 3236 /** 3237 * Search parameter: <b>name</b> 3238 * <p> 3239 * Description: <b>A portion of the location's name or alias</b><br> 3240 * Type: <b>string</b><br> 3241 * Path: <b>Location.name, Location.alias</b><br> 3242 * </p> 3243 */ 3244 @SearchParamDefinition(name = "name", path = "Location.name | Location.alias", description = "A portion of the location's name or alias", type = "string") 3245 public static final String SP_NAME = "name"; 3246 /** 3247 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3248 * <p> 3249 * Description: <b>A portion of the location's name or alias</b><br> 3250 * Type: <b>string</b><br> 3251 * Path: <b>Location.name, Location.alias</b><br> 3252 * </p> 3253 */ 3254 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3255 SP_NAME); 3256 3257 /** 3258 * Search parameter: <b>address-use</b> 3259 * <p> 3260 * Description: <b>A use code specified in an address</b><br> 3261 * Type: <b>token</b><br> 3262 * Path: <b>Location.address.use</b><br> 3263 * </p> 3264 */ 3265 @SearchParamDefinition(name = "address-use", path = "Location.address.use", description = "A use code specified in an address", type = "token") 3266 public static final String SP_ADDRESS_USE = "address-use"; 3267 /** 3268 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3269 * <p> 3270 * Description: <b>A use code specified in an address</b><br> 3271 * Type: <b>token</b><br> 3272 * Path: <b>Location.address.use</b><br> 3273 * </p> 3274 */ 3275 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3276 SP_ADDRESS_USE); 3277 3278 /** 3279 * Search parameter: <b>near</b> 3280 * <p> 3281 * Description: <b>Search for locations where the location.position is near to, 3282 * or within a specified distance of, the provided coordinates expressed as 3283 * [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 3284 * If the units are omitted, then kms should be assumed. If the distance is 3285 * omitted, then the server can use its own discretion as to what distances 3286 * should be considered near (and units are irrelevant) 3287 * 3288 * Servers may search using various techniques that might have differing 3289 * accuracies, depending on implementation efficiency. 3290 * 3291 * Requires the near-distance parameter to be provided also</b><br> 3292 * Type: <b>special</b><br> 3293 * Path: <b>Location.position</b><br> 3294 * </p> 3295 */ 3296 @SearchParamDefinition(name = "near", path = "Location.position", description = "Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).\nIf the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant)\n\nServers may search using various techniques that might have differing accuracies, depending on implementation efficiency.\n\nRequires the near-distance parameter to be provided also", type = "special") 3297 public static final String SP_NEAR = "near"; 3298 /** 3299 * <b>Fluent Client</b> search parameter constant for <b>near</b> 3300 * <p> 3301 * Description: <b>Search for locations where the location.position is near to, 3302 * or within a specified distance of, the provided coordinates expressed as 3303 * [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 3304 * If the units are omitted, then kms should be assumed. If the distance is 3305 * omitted, then the server can use its own discretion as to what distances 3306 * should be considered near (and units are irrelevant) 3307 * 3308 * Servers may search using various techniques that might have differing 3309 * accuracies, depending on implementation efficiency. 3310 * 3311 * Requires the near-distance parameter to be provided also</b><br> 3312 * Type: <b>special</b><br> 3313 * Path: <b>Location.position</b><br> 3314 * </p> 3315 */ 3316 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam NEAR = new ca.uhn.fhir.rest.gclient.SpecialClientParam( 3317 SP_NEAR); 3318 3319 /** 3320 * Search parameter: <b>address-city</b> 3321 * <p> 3322 * Description: <b>A city specified in an address</b><br> 3323 * Type: <b>string</b><br> 3324 * Path: <b>Location.address.city</b><br> 3325 * </p> 3326 */ 3327 @SearchParamDefinition(name = "address-city", path = "Location.address.city", description = "A city specified in an address", type = "string") 3328 public static final String SP_ADDRESS_CITY = "address-city"; 3329 /** 3330 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3331 * <p> 3332 * Description: <b>A city specified in an address</b><br> 3333 * Type: <b>string</b><br> 3334 * Path: <b>Location.address.city</b><br> 3335 * </p> 3336 */ 3337 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam( 3338 SP_ADDRESS_CITY); 3339 3340 /** 3341 * Search parameter: <b>status</b> 3342 * <p> 3343 * Description: <b>Searches for locations with a specific kind of status</b><br> 3344 * Type: <b>token</b><br> 3345 * Path: <b>Location.status</b><br> 3346 * </p> 3347 */ 3348 @SearchParamDefinition(name = "status", path = "Location.status", description = "Searches for locations with a specific kind of status", type = "token") 3349 public static final String SP_STATUS = "status"; 3350 /** 3351 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3352 * <p> 3353 * Description: <b>Searches for locations with a specific kind of status</b><br> 3354 * Type: <b>token</b><br> 3355 * Path: <b>Location.status</b><br> 3356 * </p> 3357 */ 3358 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3359 SP_STATUS); 3360 3361}