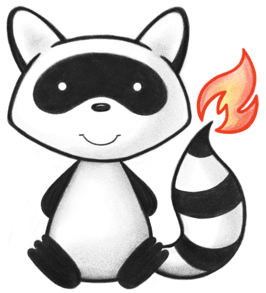
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * A photo, video, or audio recording acquired or used in healthcare. The actual 049 * content may be inline or provided by direct reference. 050 */ 051@ResourceDef(name = "Media", profile = "http://hl7.org/fhir/StructureDefinition/Media") 052public class Media extends DomainResource { 053 054 public enum MediaStatus { 055 /** 056 * The core event has not started yet, but some staging activities have begun 057 * (e.g. surgical suite preparation). Preparation stages may be tracked for 058 * billing purposes. 059 */ 060 PREPARATION, 061 /** 062 * The event is currently occurring. 063 */ 064 INPROGRESS, 065 /** 066 * The event was terminated prior to any activity beyond preparation. I.e. The 067 * 'main' activity has not yet begun. The boundary between preparatory and the 068 * 'main' activity is context-specific. 069 */ 070 NOTDONE, 071 /** 072 * The event has been temporarily stopped but is expected to resume in the 073 * future. 074 */ 075 ONHOLD, 076 /** 077 * The event was terminated prior to the full completion of the intended 078 * activity but after at least some of the 'main' activity (beyond preparation) 079 * has occurred. 080 */ 081 STOPPED, 082 /** 083 * The event has now concluded. 084 */ 085 COMPLETED, 086 /** 087 * This electronic record should never have existed, though it is possible that 088 * real-world decisions were based on it. (If real-world activity has occurred, 089 * the status should be "stopped" rather than "entered-in-error".). 090 */ 091 ENTEREDINERROR, 092 /** 093 * The authoring/source system does not know which of the status values 094 * currently applies for this event. Note: This concept is not to be used for 095 * "other" - one of the listed statuses is presumed to apply, but the 096 * authoring/source system does not know which. 097 */ 098 UNKNOWN, 099 /** 100 * added to help the parsers with the generic types 101 */ 102 NULL; 103 104 public static MediaStatus fromCode(String codeString) throws FHIRException { 105 if (codeString == null || "".equals(codeString)) 106 return null; 107 if ("preparation".equals(codeString)) 108 return PREPARATION; 109 if ("in-progress".equals(codeString)) 110 return INPROGRESS; 111 if ("not-done".equals(codeString)) 112 return NOTDONE; 113 if ("on-hold".equals(codeString)) 114 return ONHOLD; 115 if ("stopped".equals(codeString)) 116 return STOPPED; 117 if ("completed".equals(codeString)) 118 return COMPLETED; 119 if ("entered-in-error".equals(codeString)) 120 return ENTEREDINERROR; 121 if ("unknown".equals(codeString)) 122 return UNKNOWN; 123 if (Configuration.isAcceptInvalidEnums()) 124 return null; 125 else 126 throw new FHIRException("Unknown MediaStatus code '" + codeString + "'"); 127 } 128 129 public String toCode() { 130 switch (this) { 131 case PREPARATION: 132 return "preparation"; 133 case INPROGRESS: 134 return "in-progress"; 135 case NOTDONE: 136 return "not-done"; 137 case ONHOLD: 138 return "on-hold"; 139 case STOPPED: 140 return "stopped"; 141 case COMPLETED: 142 return "completed"; 143 case ENTEREDINERROR: 144 return "entered-in-error"; 145 case UNKNOWN: 146 return "unknown"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case PREPARATION: 157 return "http://hl7.org/fhir/event-status"; 158 case INPROGRESS: 159 return "http://hl7.org/fhir/event-status"; 160 case NOTDONE: 161 return "http://hl7.org/fhir/event-status"; 162 case ONHOLD: 163 return "http://hl7.org/fhir/event-status"; 164 case STOPPED: 165 return "http://hl7.org/fhir/event-status"; 166 case COMPLETED: 167 return "http://hl7.org/fhir/event-status"; 168 case ENTEREDINERROR: 169 return "http://hl7.org/fhir/event-status"; 170 case UNKNOWN: 171 return "http://hl7.org/fhir/event-status"; 172 case NULL: 173 return null; 174 default: 175 return "?"; 176 } 177 } 178 179 public String getDefinition() { 180 switch (this) { 181 case PREPARATION: 182 return "The core event has not started yet, but some staging activities have begun (e.g. surgical suite preparation). Preparation stages may be tracked for billing purposes."; 183 case INPROGRESS: 184 return "The event is currently occurring."; 185 case NOTDONE: 186 return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 187 case ONHOLD: 188 return "The event has been temporarily stopped but is expected to resume in the future."; 189 case STOPPED: 190 return "The event was terminated prior to the full completion of the intended activity but after at least some of the 'main' activity (beyond preparation) has occurred."; 191 case COMPLETED: 192 return "The event has now concluded."; 193 case ENTEREDINERROR: 194 return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 195 case UNKNOWN: 196 return "The authoring/source system does not know which of the status values currently applies for this event. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 204 public String getDisplay() { 205 switch (this) { 206 case PREPARATION: 207 return "Preparation"; 208 case INPROGRESS: 209 return "In Progress"; 210 case NOTDONE: 211 return "Not Done"; 212 case ONHOLD: 213 return "On Hold"; 214 case STOPPED: 215 return "Stopped"; 216 case COMPLETED: 217 return "Completed"; 218 case ENTEREDINERROR: 219 return "Entered in Error"; 220 case UNKNOWN: 221 return "Unknown"; 222 case NULL: 223 return null; 224 default: 225 return "?"; 226 } 227 } 228 } 229 230 public static class MediaStatusEnumFactory implements EnumFactory<MediaStatus> { 231 public MediaStatus fromCode(String codeString) throws IllegalArgumentException { 232 if (codeString == null || "".equals(codeString)) 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("preparation".equals(codeString)) 236 return MediaStatus.PREPARATION; 237 if ("in-progress".equals(codeString)) 238 return MediaStatus.INPROGRESS; 239 if ("not-done".equals(codeString)) 240 return MediaStatus.NOTDONE; 241 if ("on-hold".equals(codeString)) 242 return MediaStatus.ONHOLD; 243 if ("stopped".equals(codeString)) 244 return MediaStatus.STOPPED; 245 if ("completed".equals(codeString)) 246 return MediaStatus.COMPLETED; 247 if ("entered-in-error".equals(codeString)) 248 return MediaStatus.ENTEREDINERROR; 249 if ("unknown".equals(codeString)) 250 return MediaStatus.UNKNOWN; 251 throw new IllegalArgumentException("Unknown MediaStatus code '" + codeString + "'"); 252 } 253 254 public Enumeration<MediaStatus> fromType(PrimitiveType<?> code) throws FHIRException { 255 if (code == null) 256 return null; 257 if (code.isEmpty()) 258 return new Enumeration<MediaStatus>(this, MediaStatus.NULL, code); 259 String codeString = code.asStringValue(); 260 if (codeString == null || "".equals(codeString)) 261 return new Enumeration<MediaStatus>(this, MediaStatus.NULL, code); 262 if ("preparation".equals(codeString)) 263 return new Enumeration<MediaStatus>(this, MediaStatus.PREPARATION, code); 264 if ("in-progress".equals(codeString)) 265 return new Enumeration<MediaStatus>(this, MediaStatus.INPROGRESS, code); 266 if ("not-done".equals(codeString)) 267 return new Enumeration<MediaStatus>(this, MediaStatus.NOTDONE, code); 268 if ("on-hold".equals(codeString)) 269 return new Enumeration<MediaStatus>(this, MediaStatus.ONHOLD, code); 270 if ("stopped".equals(codeString)) 271 return new Enumeration<MediaStatus>(this, MediaStatus.STOPPED, code); 272 if ("completed".equals(codeString)) 273 return new Enumeration<MediaStatus>(this, MediaStatus.COMPLETED, code); 274 if ("entered-in-error".equals(codeString)) 275 return new Enumeration<MediaStatus>(this, MediaStatus.ENTEREDINERROR, code); 276 if ("unknown".equals(codeString)) 277 return new Enumeration<MediaStatus>(this, MediaStatus.UNKNOWN, code); 278 throw new FHIRException("Unknown MediaStatus code '" + codeString + "'"); 279 } 280 281 public String toCode(MediaStatus code) { 282 if (code == MediaStatus.NULL) 283 return null; 284 if (code == MediaStatus.PREPARATION) 285 return "preparation"; 286 if (code == MediaStatus.INPROGRESS) 287 return "in-progress"; 288 if (code == MediaStatus.NOTDONE) 289 return "not-done"; 290 if (code == MediaStatus.ONHOLD) 291 return "on-hold"; 292 if (code == MediaStatus.STOPPED) 293 return "stopped"; 294 if (code == MediaStatus.COMPLETED) 295 return "completed"; 296 if (code == MediaStatus.ENTEREDINERROR) 297 return "entered-in-error"; 298 if (code == MediaStatus.UNKNOWN) 299 return "unknown"; 300 return "?"; 301 } 302 303 public String toSystem(MediaStatus code) { 304 return code.getSystem(); 305 } 306 } 307 308 /** 309 * Identifiers associated with the image - these may include identifiers for the 310 * image itself, identifiers for the context of its collection (e.g. series ids) 311 * and context ids such as accession numbers or other workflow identifiers. 312 */ 313 @Child(name = "identifier", type = { 314 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 315 @Description(shortDefinition = "Identifier(s) for the image", formalDefinition = "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.") 316 protected List<Identifier> identifier; 317 318 /** 319 * A procedure that is fulfilled in whole or in part by the creation of this 320 * media. 321 */ 322 @Child(name = "basedOn", type = { ServiceRequest.class, 323 CarePlan.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 324 @Description(shortDefinition = "Procedure that caused this media to be created", formalDefinition = "A procedure that is fulfilled in whole or in part by the creation of this media.") 325 protected List<Reference> basedOn; 326 /** 327 * The actual objects that are the target of the reference (A procedure that is 328 * fulfilled in whole or in part by the creation of this media.) 329 */ 330 protected List<Resource> basedOnTarget; 331 332 /** 333 * A larger event of which this particular event is a component or step. 334 */ 335 @Child(name = "partOf", type = { 336 Reference.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 337 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 338 protected List<Reference> partOf; 339 /** 340 * The actual objects that are the target of the reference (A larger event of 341 * which this particular event is a component or step.) 342 */ 343 protected List<Resource> partOfTarget; 344 345 /** 346 * The current state of the {{title}}. 347 */ 348 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 349 @Description(shortDefinition = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", formalDefinition = "The current state of the {{title}}.") 350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/event-status") 351 protected Enumeration<MediaStatus> status; 352 353 /** 354 * A code that classifies whether the media is an image, video or audio 355 * recording or some other media category. 356 */ 357 @Child(name = "type", type = { CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 358 @Description(shortDefinition = "Classification of media as image, video, or audio", formalDefinition = "A code that classifies whether the media is an image, video or audio recording or some other media category.") 359 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-type") 360 protected CodeableConcept type; 361 362 /** 363 * Details of the type of the media - usually, how it was acquired (what type of 364 * device). If images sourced from a DICOM system, are wrapped in a Media 365 * resource, then this is the modality. 366 */ 367 @Child(name = "modality", type = { 368 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 369 @Description(shortDefinition = "The type of acquisition equipment/process", formalDefinition = "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.") 370 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-modality") 371 protected CodeableConcept modality; 372 373 /** 374 * The name of the imaging view e.g. Lateral or Antero-posterior (AP). 375 */ 376 @Child(name = "view", type = { CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 377 @Description(shortDefinition = "Imaging view, e.g. Lateral or Antero-posterior", formalDefinition = "The name of the imaging view e.g. Lateral or Antero-posterior (AP).") 378 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/media-view") 379 protected CodeableConcept view; 380 381 /** 382 * Who/What this Media is a record of. 383 */ 384 @Child(name = "subject", type = { Patient.class, Practitioner.class, PractitionerRole.class, Group.class, 385 Device.class, Specimen.class, Location.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 386 @Description(shortDefinition = "Who/What this Media is a record of", formalDefinition = "Who/What this Media is a record of.") 387 protected Reference subject; 388 389 /** 390 * The actual object that is the target of the reference (Who/What this Media is 391 * a record of.) 392 */ 393 protected Resource subjectTarget; 394 395 /** 396 * The encounter that establishes the context for this media. 397 */ 398 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 399 @Description(shortDefinition = "Encounter associated with media", formalDefinition = "The encounter that establishes the context for this media.") 400 protected Reference encounter; 401 402 /** 403 * The actual object that is the target of the reference (The encounter that 404 * establishes the context for this media.) 405 */ 406 protected Encounter encounterTarget; 407 408 /** 409 * The date and time(s) at which the media was collected. 410 */ 411 @Child(name = "created", type = { DateTimeType.class, 412 Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 413 @Description(shortDefinition = "When Media was collected", formalDefinition = "The date and time(s) at which the media was collected.") 414 protected Type created; 415 416 /** 417 * The date and time this version of the media was made available to providers, 418 * typically after having been reviewed. 419 */ 420 @Child(name = "issued", type = { InstantType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 421 @Description(shortDefinition = "Date/Time this version was made available", formalDefinition = "The date and time this version of the media was made available to providers, typically after having been reviewed.") 422 protected InstantType issued; 423 424 /** 425 * The person who administered the collection of the image. 426 */ 427 @Child(name = "operator", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 428 Patient.class, Device.class, 429 RelatedPerson.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 430 @Description(shortDefinition = "The person who generated the image", formalDefinition = "The person who administered the collection of the image.") 431 protected Reference operator; 432 433 /** 434 * The actual object that is the target of the reference (The person who 435 * administered the collection of the image.) 436 */ 437 protected Resource operatorTarget; 438 439 /** 440 * Describes why the event occurred in coded or textual form. 441 */ 442 @Child(name = "reasonCode", type = { 443 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 444 @Description(shortDefinition = "Why was event performed?", formalDefinition = "Describes why the event occurred in coded or textual form.") 445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/procedure-reason") 446 protected List<CodeableConcept> reasonCode; 447 448 /** 449 * Indicates the site on the subject's body where the observation was made (i.e. 450 * the target site). 451 */ 452 @Child(name = "bodySite", type = { 453 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 454 @Description(shortDefinition = "Observed body part", formalDefinition = "Indicates the site on the subject's body where the observation was made (i.e. the target site).") 455 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 456 protected CodeableConcept bodySite; 457 458 /** 459 * The name of the device / manufacturer of the device that was used to make the 460 * recording. 461 */ 462 @Child(name = "deviceName", type = { 463 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 464 @Description(shortDefinition = "Name of the device/manufacturer", formalDefinition = "The name of the device / manufacturer of the device that was used to make the recording.") 465 protected StringType deviceName; 466 467 /** 468 * The device used to collect the media. 469 */ 470 @Child(name = "device", type = { Device.class, DeviceMetric.class, 471 Device.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 472 @Description(shortDefinition = "Observing Device", formalDefinition = "The device used to collect the media.") 473 protected Reference device; 474 475 /** 476 * The actual object that is the target of the reference (The device used to 477 * collect the media.) 478 */ 479 protected Resource deviceTarget; 480 481 /** 482 * Height of the image in pixels (photo/video). 483 */ 484 @Child(name = "height", type = { 485 PositiveIntType.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 486 @Description(shortDefinition = "Height of the image in pixels (photo/video)", formalDefinition = "Height of the image in pixels (photo/video).") 487 protected PositiveIntType height; 488 489 /** 490 * Width of the image in pixels (photo/video). 491 */ 492 @Child(name = "width", type = { 493 PositiveIntType.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 494 @Description(shortDefinition = "Width of the image in pixels (photo/video)", formalDefinition = "Width of the image in pixels (photo/video).") 495 protected PositiveIntType width; 496 497 /** 498 * The number of frames in a photo. This is used with a multi-page fax, or an 499 * imaging acquisition context that takes multiple slices in a single image, or 500 * an animated gif. If there is more than one frame, this SHALL have a value in 501 * order to alert interface software that a multi-frame capable rendering widget 502 * is required. 503 */ 504 @Child(name = "frames", type = { 505 PositiveIntType.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 506 @Description(shortDefinition = "Number of frames if > 1 (photo)", formalDefinition = "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.") 507 protected PositiveIntType frames; 508 509 /** 510 * The duration of the recording in seconds - for audio and video. 511 */ 512 @Child(name = "duration", type = { 513 DecimalType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 514 @Description(shortDefinition = "Length in seconds (audio / video)", formalDefinition = "The duration of the recording in seconds - for audio and video.") 515 protected DecimalType duration; 516 517 /** 518 * The actual content of the media - inline or by direct reference to the media 519 * source file. 520 */ 521 @Child(name = "content", type = { Attachment.class }, order = 20, min = 1, max = 1, modifier = false, summary = true) 522 @Description(shortDefinition = "Actual Media - reference or data", formalDefinition = "The actual content of the media - inline or by direct reference to the media source file.") 523 protected Attachment content; 524 525 /** 526 * Comments made about the media by the performer, subject or other 527 * participants. 528 */ 529 @Child(name = "note", type = { 530 Annotation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 531 @Description(shortDefinition = "Comments made about the media", formalDefinition = "Comments made about the media by the performer, subject or other participants.") 532 protected List<Annotation> note; 533 534 private static final long serialVersionUID = 2069980126L; 535 536 /** 537 * Constructor 538 */ 539 public Media() { 540 super(); 541 } 542 543 /** 544 * Constructor 545 */ 546 public Media(Enumeration<MediaStatus> status, Attachment content) { 547 super(); 548 this.status = status; 549 this.content = content; 550 } 551 552 /** 553 * @return {@link #identifier} (Identifiers associated with the image - these 554 * may include identifiers for the image itself, identifiers for the 555 * context of its collection (e.g. series ids) and context ids such as 556 * accession numbers or other workflow identifiers.) 557 */ 558 public List<Identifier> getIdentifier() { 559 if (this.identifier == null) 560 this.identifier = new ArrayList<Identifier>(); 561 return this.identifier; 562 } 563 564 /** 565 * @return Returns a reference to <code>this</code> for easy method chaining 566 */ 567 public Media setIdentifier(List<Identifier> theIdentifier) { 568 this.identifier = theIdentifier; 569 return this; 570 } 571 572 public boolean hasIdentifier() { 573 if (this.identifier == null) 574 return false; 575 for (Identifier item : this.identifier) 576 if (!item.isEmpty()) 577 return true; 578 return false; 579 } 580 581 public Identifier addIdentifier() { // 3 582 Identifier t = new Identifier(); 583 if (this.identifier == null) 584 this.identifier = new ArrayList<Identifier>(); 585 this.identifier.add(t); 586 return t; 587 } 588 589 public Media addIdentifier(Identifier t) { // 3 590 if (t == null) 591 return this; 592 if (this.identifier == null) 593 this.identifier = new ArrayList<Identifier>(); 594 this.identifier.add(t); 595 return this; 596 } 597 598 /** 599 * @return The first repetition of repeating field {@link #identifier}, creating 600 * it if it does not already exist 601 */ 602 public Identifier getIdentifierFirstRep() { 603 if (getIdentifier().isEmpty()) { 604 addIdentifier(); 605 } 606 return getIdentifier().get(0); 607 } 608 609 /** 610 * @return {@link #basedOn} (A procedure that is fulfilled in whole or in part 611 * by the creation of this media.) 612 */ 613 public List<Reference> getBasedOn() { 614 if (this.basedOn == null) 615 this.basedOn = new ArrayList<Reference>(); 616 return this.basedOn; 617 } 618 619 /** 620 * @return Returns a reference to <code>this</code> for easy method chaining 621 */ 622 public Media setBasedOn(List<Reference> theBasedOn) { 623 this.basedOn = theBasedOn; 624 return this; 625 } 626 627 public boolean hasBasedOn() { 628 if (this.basedOn == null) 629 return false; 630 for (Reference item : this.basedOn) 631 if (!item.isEmpty()) 632 return true; 633 return false; 634 } 635 636 public Reference addBasedOn() { // 3 637 Reference t = new Reference(); 638 if (this.basedOn == null) 639 this.basedOn = new ArrayList<Reference>(); 640 this.basedOn.add(t); 641 return t; 642 } 643 644 public Media addBasedOn(Reference t) { // 3 645 if (t == null) 646 return this; 647 if (this.basedOn == null) 648 this.basedOn = new ArrayList<Reference>(); 649 this.basedOn.add(t); 650 return this; 651 } 652 653 /** 654 * @return The first repetition of repeating field {@link #basedOn}, creating it 655 * if it does not already exist 656 */ 657 public Reference getBasedOnFirstRep() { 658 if (getBasedOn().isEmpty()) { 659 addBasedOn(); 660 } 661 return getBasedOn().get(0); 662 } 663 664 /** 665 * @deprecated Use Reference#setResource(IBaseResource) instead 666 */ 667 @Deprecated 668 public List<Resource> getBasedOnTarget() { 669 if (this.basedOnTarget == null) 670 this.basedOnTarget = new ArrayList<Resource>(); 671 return this.basedOnTarget; 672 } 673 674 /** 675 * @return {@link #partOf} (A larger event of which this particular event is a 676 * component or step.) 677 */ 678 public List<Reference> getPartOf() { 679 if (this.partOf == null) 680 this.partOf = new ArrayList<Reference>(); 681 return this.partOf; 682 } 683 684 /** 685 * @return Returns a reference to <code>this</code> for easy method chaining 686 */ 687 public Media setPartOf(List<Reference> thePartOf) { 688 this.partOf = thePartOf; 689 return this; 690 } 691 692 public boolean hasPartOf() { 693 if (this.partOf == null) 694 return false; 695 for (Reference item : this.partOf) 696 if (!item.isEmpty()) 697 return true; 698 return false; 699 } 700 701 public Reference addPartOf() { // 3 702 Reference t = new Reference(); 703 if (this.partOf == null) 704 this.partOf = new ArrayList<Reference>(); 705 this.partOf.add(t); 706 return t; 707 } 708 709 public Media addPartOf(Reference t) { // 3 710 if (t == null) 711 return this; 712 if (this.partOf == null) 713 this.partOf = new ArrayList<Reference>(); 714 this.partOf.add(t); 715 return this; 716 } 717 718 /** 719 * @return The first repetition of repeating field {@link #partOf}, creating it 720 * if it does not already exist 721 */ 722 public Reference getPartOfFirstRep() { 723 if (getPartOf().isEmpty()) { 724 addPartOf(); 725 } 726 return getPartOf().get(0); 727 } 728 729 /** 730 * @deprecated Use Reference#setResource(IBaseResource) instead 731 */ 732 @Deprecated 733 public List<Resource> getPartOfTarget() { 734 if (this.partOfTarget == null) 735 this.partOfTarget = new ArrayList<Resource>(); 736 return this.partOfTarget; 737 } 738 739 /** 740 * @return {@link #status} (The current state of the {{title}}.). This is the 741 * underlying object with id, value and extensions. The accessor 742 * "getStatus" gives direct access to the value 743 */ 744 public Enumeration<MediaStatus> getStatusElement() { 745 if (this.status == null) 746 if (Configuration.errorOnAutoCreate()) 747 throw new Error("Attempt to auto-create Media.status"); 748 else if (Configuration.doAutoCreate()) 749 this.status = new Enumeration<MediaStatus>(new MediaStatusEnumFactory()); // bb 750 return this.status; 751 } 752 753 public boolean hasStatusElement() { 754 return this.status != null && !this.status.isEmpty(); 755 } 756 757 public boolean hasStatus() { 758 return this.status != null && !this.status.isEmpty(); 759 } 760 761 /** 762 * @param value {@link #status} (The current state of the {{title}}.). This is 763 * the underlying object with id, value and extensions. The 764 * accessor "getStatus" gives direct access to the value 765 */ 766 public Media setStatusElement(Enumeration<MediaStatus> value) { 767 this.status = value; 768 return this; 769 } 770 771 /** 772 * @return The current state of the {{title}}. 773 */ 774 public MediaStatus getStatus() { 775 return this.status == null ? null : this.status.getValue(); 776 } 777 778 /** 779 * @param value The current state of the {{title}}. 780 */ 781 public Media setStatus(MediaStatus value) { 782 if (this.status == null) 783 this.status = new Enumeration<MediaStatus>(new MediaStatusEnumFactory()); 784 this.status.setValue(value); 785 return this; 786 } 787 788 /** 789 * @return {@link #type} (A code that classifies whether the media is an image, 790 * video or audio recording or some other media category.) 791 */ 792 public CodeableConcept getType() { 793 if (this.type == null) 794 if (Configuration.errorOnAutoCreate()) 795 throw new Error("Attempt to auto-create Media.type"); 796 else if (Configuration.doAutoCreate()) 797 this.type = new CodeableConcept(); // cc 798 return this.type; 799 } 800 801 public boolean hasType() { 802 return this.type != null && !this.type.isEmpty(); 803 } 804 805 /** 806 * @param value {@link #type} (A code that classifies whether the media is an 807 * image, video or audio recording or some other media category.) 808 */ 809 public Media setType(CodeableConcept value) { 810 this.type = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #modality} (Details of the type of the media - usually, how it 816 * was acquired (what type of device). If images sourced from a DICOM 817 * system, are wrapped in a Media resource, then this is the modality.) 818 */ 819 public CodeableConcept getModality() { 820 if (this.modality == null) 821 if (Configuration.errorOnAutoCreate()) 822 throw new Error("Attempt to auto-create Media.modality"); 823 else if (Configuration.doAutoCreate()) 824 this.modality = new CodeableConcept(); // cc 825 return this.modality; 826 } 827 828 public boolean hasModality() { 829 return this.modality != null && !this.modality.isEmpty(); 830 } 831 832 /** 833 * @param value {@link #modality} (Details of the type of the media - usually, 834 * how it was acquired (what type of device). If images sourced 835 * from a DICOM system, are wrapped in a Media resource, then this 836 * is the modality.) 837 */ 838 public Media setModality(CodeableConcept value) { 839 this.modality = value; 840 return this; 841 } 842 843 /** 844 * @return {@link #view} (The name of the imaging view e.g. Lateral or 845 * Antero-posterior (AP).) 846 */ 847 public CodeableConcept getView() { 848 if (this.view == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create Media.view"); 851 else if (Configuration.doAutoCreate()) 852 this.view = new CodeableConcept(); // cc 853 return this.view; 854 } 855 856 public boolean hasView() { 857 return this.view != null && !this.view.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #view} (The name of the imaging view e.g. Lateral or 862 * Antero-posterior (AP).) 863 */ 864 public Media setView(CodeableConcept value) { 865 this.view = value; 866 return this; 867 } 868 869 /** 870 * @return {@link #subject} (Who/What this Media is a record of.) 871 */ 872 public Reference getSubject() { 873 if (this.subject == null) 874 if (Configuration.errorOnAutoCreate()) 875 throw new Error("Attempt to auto-create Media.subject"); 876 else if (Configuration.doAutoCreate()) 877 this.subject = new Reference(); // cc 878 return this.subject; 879 } 880 881 public boolean hasSubject() { 882 return this.subject != null && !this.subject.isEmpty(); 883 } 884 885 /** 886 * @param value {@link #subject} (Who/What this Media is a record of.) 887 */ 888 public Media setSubject(Reference value) { 889 this.subject = value; 890 return this; 891 } 892 893 /** 894 * @return {@link #subject} The actual object that is the target of the 895 * reference. The reference library doesn't populate this, but you can 896 * use it to hold the resource if you resolve it. (Who/What this Media 897 * is a record of.) 898 */ 899 public Resource getSubjectTarget() { 900 return this.subjectTarget; 901 } 902 903 /** 904 * @param value {@link #subject} The actual object that is the target of the 905 * reference. The reference library doesn't use these, but you can 906 * use it to hold the resource if you resolve it. (Who/What this 907 * Media is a record of.) 908 */ 909 public Media setSubjectTarget(Resource value) { 910 this.subjectTarget = value; 911 return this; 912 } 913 914 /** 915 * @return {@link #encounter} (The encounter that establishes the context for 916 * this media.) 917 */ 918 public Reference getEncounter() { 919 if (this.encounter == null) 920 if (Configuration.errorOnAutoCreate()) 921 throw new Error("Attempt to auto-create Media.encounter"); 922 else if (Configuration.doAutoCreate()) 923 this.encounter = new Reference(); // cc 924 return this.encounter; 925 } 926 927 public boolean hasEncounter() { 928 return this.encounter != null && !this.encounter.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #encounter} (The encounter that establishes the context 933 * for this media.) 934 */ 935 public Media setEncounter(Reference value) { 936 this.encounter = value; 937 return this; 938 } 939 940 /** 941 * @return {@link #encounter} The actual object that is the target of the 942 * reference. The reference library doesn't populate this, but you can 943 * use it to hold the resource if you resolve it. (The encounter that 944 * establishes the context for this media.) 945 */ 946 public Encounter getEncounterTarget() { 947 if (this.encounterTarget == null) 948 if (Configuration.errorOnAutoCreate()) 949 throw new Error("Attempt to auto-create Media.encounter"); 950 else if (Configuration.doAutoCreate()) 951 this.encounterTarget = new Encounter(); // aa 952 return this.encounterTarget; 953 } 954 955 /** 956 * @param value {@link #encounter} The actual object that is the target of the 957 * reference. The reference library doesn't use these, but you can 958 * use it to hold the resource if you resolve it. (The encounter 959 * that establishes the context for this media.) 960 */ 961 public Media setEncounterTarget(Encounter value) { 962 this.encounterTarget = value; 963 return this; 964 } 965 966 /** 967 * @return {@link #created} (The date and time(s) at which the media was 968 * collected.) 969 */ 970 public Type getCreated() { 971 return this.created; 972 } 973 974 /** 975 * @return {@link #created} (The date and time(s) at which the media was 976 * collected.) 977 */ 978 public DateTimeType getCreatedDateTimeType() throws FHIRException { 979 if (this.created == null) 980 this.created = new DateTimeType(); 981 if (!(this.created instanceof DateTimeType)) 982 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 983 + this.created.getClass().getName() + " was encountered"); 984 return (DateTimeType) this.created; 985 } 986 987 public boolean hasCreatedDateTimeType() { 988 return this != null && this.created instanceof DateTimeType; 989 } 990 991 /** 992 * @return {@link #created} (The date and time(s) at which the media was 993 * collected.) 994 */ 995 public Period getCreatedPeriod() throws FHIRException { 996 if (this.created == null) 997 this.created = new Period(); 998 if (!(this.created instanceof Period)) 999 throw new FHIRException( 1000 "Type mismatch: the type Period was expected, but " + this.created.getClass().getName() + " was encountered"); 1001 return (Period) this.created; 1002 } 1003 1004 public boolean hasCreatedPeriod() { 1005 return this != null && this.created instanceof Period; 1006 } 1007 1008 public boolean hasCreated() { 1009 return this.created != null && !this.created.isEmpty(); 1010 } 1011 1012 /** 1013 * @param value {@link #created} (The date and time(s) at which the media was 1014 * collected.) 1015 */ 1016 public Media setCreated(Type value) { 1017 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1018 throw new Error("Not the right type for Media.created[x]: " + value.fhirType()); 1019 this.created = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #issued} (The date and time this version of the media was made 1025 * available to providers, typically after having been reviewed.). This 1026 * is the underlying object with id, value and extensions. The accessor 1027 * "getIssued" gives direct access to the value 1028 */ 1029 public InstantType getIssuedElement() { 1030 if (this.issued == null) 1031 if (Configuration.errorOnAutoCreate()) 1032 throw new Error("Attempt to auto-create Media.issued"); 1033 else if (Configuration.doAutoCreate()) 1034 this.issued = new InstantType(); // bb 1035 return this.issued; 1036 } 1037 1038 public boolean hasIssuedElement() { 1039 return this.issued != null && !this.issued.isEmpty(); 1040 } 1041 1042 public boolean hasIssued() { 1043 return this.issued != null && !this.issued.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #issued} (The date and time this version of the media was 1048 * made available to providers, typically after having been 1049 * reviewed.). This is the underlying object with id, value and 1050 * extensions. The accessor "getIssued" gives direct access to the 1051 * value 1052 */ 1053 public Media setIssuedElement(InstantType value) { 1054 this.issued = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return The date and time this version of the media was made available to 1060 * providers, typically after having been reviewed. 1061 */ 1062 public Date getIssued() { 1063 return this.issued == null ? null : this.issued.getValue(); 1064 } 1065 1066 /** 1067 * @param value The date and time this version of the media was made available 1068 * to providers, typically after having been reviewed. 1069 */ 1070 public Media setIssued(Date value) { 1071 if (value == null) 1072 this.issued = null; 1073 else { 1074 if (this.issued == null) 1075 this.issued = new InstantType(); 1076 this.issued.setValue(value); 1077 } 1078 return this; 1079 } 1080 1081 /** 1082 * @return {@link #operator} (The person who administered the collection of the 1083 * image.) 1084 */ 1085 public Reference getOperator() { 1086 if (this.operator == null) 1087 if (Configuration.errorOnAutoCreate()) 1088 throw new Error("Attempt to auto-create Media.operator"); 1089 else if (Configuration.doAutoCreate()) 1090 this.operator = new Reference(); // cc 1091 return this.operator; 1092 } 1093 1094 public boolean hasOperator() { 1095 return this.operator != null && !this.operator.isEmpty(); 1096 } 1097 1098 /** 1099 * @param value {@link #operator} (The person who administered the collection of 1100 * the image.) 1101 */ 1102 public Media setOperator(Reference value) { 1103 this.operator = value; 1104 return this; 1105 } 1106 1107 /** 1108 * @return {@link #operator} The actual object that is the target of the 1109 * reference. The reference library doesn't populate this, but you can 1110 * use it to hold the resource if you resolve it. (The person who 1111 * administered the collection of the image.) 1112 */ 1113 public Resource getOperatorTarget() { 1114 return this.operatorTarget; 1115 } 1116 1117 /** 1118 * @param value {@link #operator} The actual object that is the target of the 1119 * reference. The reference library doesn't use these, but you can 1120 * use it to hold the resource if you resolve it. (The person who 1121 * administered the collection of the image.) 1122 */ 1123 public Media setOperatorTarget(Resource value) { 1124 this.operatorTarget = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #reasonCode} (Describes why the event occurred in coded or 1130 * textual form.) 1131 */ 1132 public List<CodeableConcept> getReasonCode() { 1133 if (this.reasonCode == null) 1134 this.reasonCode = new ArrayList<CodeableConcept>(); 1135 return this.reasonCode; 1136 } 1137 1138 /** 1139 * @return Returns a reference to <code>this</code> for easy method chaining 1140 */ 1141 public Media setReasonCode(List<CodeableConcept> theReasonCode) { 1142 this.reasonCode = theReasonCode; 1143 return this; 1144 } 1145 1146 public boolean hasReasonCode() { 1147 if (this.reasonCode == null) 1148 return false; 1149 for (CodeableConcept item : this.reasonCode) 1150 if (!item.isEmpty()) 1151 return true; 1152 return false; 1153 } 1154 1155 public CodeableConcept addReasonCode() { // 3 1156 CodeableConcept t = new CodeableConcept(); 1157 if (this.reasonCode == null) 1158 this.reasonCode = new ArrayList<CodeableConcept>(); 1159 this.reasonCode.add(t); 1160 return t; 1161 } 1162 1163 public Media addReasonCode(CodeableConcept t) { // 3 1164 if (t == null) 1165 return this; 1166 if (this.reasonCode == null) 1167 this.reasonCode = new ArrayList<CodeableConcept>(); 1168 this.reasonCode.add(t); 1169 return this; 1170 } 1171 1172 /** 1173 * @return The first repetition of repeating field {@link #reasonCode}, creating 1174 * it if it does not already exist 1175 */ 1176 public CodeableConcept getReasonCodeFirstRep() { 1177 if (getReasonCode().isEmpty()) { 1178 addReasonCode(); 1179 } 1180 return getReasonCode().get(0); 1181 } 1182 1183 /** 1184 * @return {@link #bodySite} (Indicates the site on the subject's body where the 1185 * observation was made (i.e. the target site).) 1186 */ 1187 public CodeableConcept getBodySite() { 1188 if (this.bodySite == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create Media.bodySite"); 1191 else if (Configuration.doAutoCreate()) 1192 this.bodySite = new CodeableConcept(); // cc 1193 return this.bodySite; 1194 } 1195 1196 public boolean hasBodySite() { 1197 return this.bodySite != null && !this.bodySite.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #bodySite} (Indicates the site on the subject's body 1202 * where the observation was made (i.e. the target site).) 1203 */ 1204 public Media setBodySite(CodeableConcept value) { 1205 this.bodySite = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return {@link #deviceName} (The name of the device / manufacturer of the 1211 * device that was used to make the recording.). This is the underlying 1212 * object with id, value and extensions. The accessor "getDeviceName" 1213 * gives direct access to the value 1214 */ 1215 public StringType getDeviceNameElement() { 1216 if (this.deviceName == null) 1217 if (Configuration.errorOnAutoCreate()) 1218 throw new Error("Attempt to auto-create Media.deviceName"); 1219 else if (Configuration.doAutoCreate()) 1220 this.deviceName = new StringType(); // bb 1221 return this.deviceName; 1222 } 1223 1224 public boolean hasDeviceNameElement() { 1225 return this.deviceName != null && !this.deviceName.isEmpty(); 1226 } 1227 1228 public boolean hasDeviceName() { 1229 return this.deviceName != null && !this.deviceName.isEmpty(); 1230 } 1231 1232 /** 1233 * @param value {@link #deviceName} (The name of the device / manufacturer of 1234 * the device that was used to make the recording.). This is the 1235 * underlying object with id, value and extensions. The accessor 1236 * "getDeviceName" gives direct access to the value 1237 */ 1238 public Media setDeviceNameElement(StringType value) { 1239 this.deviceName = value; 1240 return this; 1241 } 1242 1243 /** 1244 * @return The name of the device / manufacturer of the device that was used to 1245 * make the recording. 1246 */ 1247 public String getDeviceName() { 1248 return this.deviceName == null ? null : this.deviceName.getValue(); 1249 } 1250 1251 /** 1252 * @param value The name of the device / manufacturer of the device that was 1253 * used to make the recording. 1254 */ 1255 public Media setDeviceName(String value) { 1256 if (Utilities.noString(value)) 1257 this.deviceName = null; 1258 else { 1259 if (this.deviceName == null) 1260 this.deviceName = new StringType(); 1261 this.deviceName.setValue(value); 1262 } 1263 return this; 1264 } 1265 1266 /** 1267 * @return {@link #device} (The device used to collect the media.) 1268 */ 1269 public Reference getDevice() { 1270 if (this.device == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create Media.device"); 1273 else if (Configuration.doAutoCreate()) 1274 this.device = new Reference(); // cc 1275 return this.device; 1276 } 1277 1278 public boolean hasDevice() { 1279 return this.device != null && !this.device.isEmpty(); 1280 } 1281 1282 /** 1283 * @param value {@link #device} (The device used to collect the media.) 1284 */ 1285 public Media setDevice(Reference value) { 1286 this.device = value; 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #device} The actual object that is the target of the 1292 * reference. The reference library doesn't populate this, but you can 1293 * use it to hold the resource if you resolve it. (The device used to 1294 * collect the media.) 1295 */ 1296 public Resource getDeviceTarget() { 1297 return this.deviceTarget; 1298 } 1299 1300 /** 1301 * @param value {@link #device} The actual object that is the target of the 1302 * reference. The reference library doesn't use these, but you can 1303 * use it to hold the resource if you resolve it. (The device used 1304 * to collect the media.) 1305 */ 1306 public Media setDeviceTarget(Resource value) { 1307 this.deviceTarget = value; 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #height} (Height of the image in pixels (photo/video).). This 1313 * is the underlying object with id, value and extensions. The accessor 1314 * "getHeight" gives direct access to the value 1315 */ 1316 public PositiveIntType getHeightElement() { 1317 if (this.height == null) 1318 if (Configuration.errorOnAutoCreate()) 1319 throw new Error("Attempt to auto-create Media.height"); 1320 else if (Configuration.doAutoCreate()) 1321 this.height = new PositiveIntType(); // bb 1322 return this.height; 1323 } 1324 1325 public boolean hasHeightElement() { 1326 return this.height != null && !this.height.isEmpty(); 1327 } 1328 1329 public boolean hasHeight() { 1330 return this.height != null && !this.height.isEmpty(); 1331 } 1332 1333 /** 1334 * @param value {@link #height} (Height of the image in pixels (photo/video).). 1335 * This is the underlying object with id, value and extensions. The 1336 * accessor "getHeight" gives direct access to the value 1337 */ 1338 public Media setHeightElement(PositiveIntType value) { 1339 this.height = value; 1340 return this; 1341 } 1342 1343 /** 1344 * @return Height of the image in pixels (photo/video). 1345 */ 1346 public int getHeight() { 1347 return this.height == null || this.height.isEmpty() ? 0 : this.height.getValue(); 1348 } 1349 1350 /** 1351 * @param value Height of the image in pixels (photo/video). 1352 */ 1353 public Media setHeight(int value) { 1354 if (this.height == null) 1355 this.height = new PositiveIntType(); 1356 this.height.setValue(value); 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #width} (Width of the image in pixels (photo/video).). This is 1362 * the underlying object with id, value and extensions. The accessor 1363 * "getWidth" gives direct access to the value 1364 */ 1365 public PositiveIntType getWidthElement() { 1366 if (this.width == null) 1367 if (Configuration.errorOnAutoCreate()) 1368 throw new Error("Attempt to auto-create Media.width"); 1369 else if (Configuration.doAutoCreate()) 1370 this.width = new PositiveIntType(); // bb 1371 return this.width; 1372 } 1373 1374 public boolean hasWidthElement() { 1375 return this.width != null && !this.width.isEmpty(); 1376 } 1377 1378 public boolean hasWidth() { 1379 return this.width != null && !this.width.isEmpty(); 1380 } 1381 1382 /** 1383 * @param value {@link #width} (Width of the image in pixels (photo/video).). 1384 * This is the underlying object with id, value and extensions. The 1385 * accessor "getWidth" gives direct access to the value 1386 */ 1387 public Media setWidthElement(PositiveIntType value) { 1388 this.width = value; 1389 return this; 1390 } 1391 1392 /** 1393 * @return Width of the image in pixels (photo/video). 1394 */ 1395 public int getWidth() { 1396 return this.width == null || this.width.isEmpty() ? 0 : this.width.getValue(); 1397 } 1398 1399 /** 1400 * @param value Width of the image in pixels (photo/video). 1401 */ 1402 public Media setWidth(int value) { 1403 if (this.width == null) 1404 this.width = new PositiveIntType(); 1405 this.width.setValue(value); 1406 return this; 1407 } 1408 1409 /** 1410 * @return {@link #frames} (The number of frames in a photo. This is used with a 1411 * multi-page fax, or an imaging acquisition context that takes multiple 1412 * slices in a single image, or an animated gif. If there is more than 1413 * one frame, this SHALL have a value in order to alert interface 1414 * software that a multi-frame capable rendering widget is required.). 1415 * This is the underlying object with id, value and extensions. The 1416 * accessor "getFrames" gives direct access to the value 1417 */ 1418 public PositiveIntType getFramesElement() { 1419 if (this.frames == null) 1420 if (Configuration.errorOnAutoCreate()) 1421 throw new Error("Attempt to auto-create Media.frames"); 1422 else if (Configuration.doAutoCreate()) 1423 this.frames = new PositiveIntType(); // bb 1424 return this.frames; 1425 } 1426 1427 public boolean hasFramesElement() { 1428 return this.frames != null && !this.frames.isEmpty(); 1429 } 1430 1431 public boolean hasFrames() { 1432 return this.frames != null && !this.frames.isEmpty(); 1433 } 1434 1435 /** 1436 * @param value {@link #frames} (The number of frames in a photo. This is used 1437 * with a multi-page fax, or an imaging acquisition context that 1438 * takes multiple slices in a single image, or an animated gif. If 1439 * there is more than one frame, this SHALL have a value in order 1440 * to alert interface software that a multi-frame capable rendering 1441 * widget is required.). This is the underlying object with id, 1442 * value and extensions. The accessor "getFrames" gives direct 1443 * access to the value 1444 */ 1445 public Media setFramesElement(PositiveIntType value) { 1446 this.frames = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return The number of frames in a photo. This is used with a multi-page fax, 1452 * or an imaging acquisition context that takes multiple slices in a 1453 * single image, or an animated gif. If there is more than one frame, 1454 * this SHALL have a value in order to alert interface software that a 1455 * multi-frame capable rendering widget is required. 1456 */ 1457 public int getFrames() { 1458 return this.frames == null || this.frames.isEmpty() ? 0 : this.frames.getValue(); 1459 } 1460 1461 /** 1462 * @param value The number of frames in a photo. This is used with a multi-page 1463 * fax, or an imaging acquisition context that takes multiple 1464 * slices in a single image, or an animated gif. If there is more 1465 * than one frame, this SHALL have a value in order to alert 1466 * interface software that a multi-frame capable rendering widget 1467 * is required. 1468 */ 1469 public Media setFrames(int value) { 1470 if (this.frames == null) 1471 this.frames = new PositiveIntType(); 1472 this.frames.setValue(value); 1473 return this; 1474 } 1475 1476 /** 1477 * @return {@link #duration} (The duration of the recording in seconds - for 1478 * audio and video.). This is the underlying object with id, value and 1479 * extensions. The accessor "getDuration" gives direct access to the 1480 * value 1481 */ 1482 public DecimalType getDurationElement() { 1483 if (this.duration == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create Media.duration"); 1486 else if (Configuration.doAutoCreate()) 1487 this.duration = new DecimalType(); // bb 1488 return this.duration; 1489 } 1490 1491 public boolean hasDurationElement() { 1492 return this.duration != null && !this.duration.isEmpty(); 1493 } 1494 1495 public boolean hasDuration() { 1496 return this.duration != null && !this.duration.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #duration} (The duration of the recording in seconds - 1501 * for audio and video.). This is the underlying object with id, 1502 * value and extensions. The accessor "getDuration" gives direct 1503 * access to the value 1504 */ 1505 public Media setDurationElement(DecimalType value) { 1506 this.duration = value; 1507 return this; 1508 } 1509 1510 /** 1511 * @return The duration of the recording in seconds - for audio and video. 1512 */ 1513 public BigDecimal getDuration() { 1514 return this.duration == null ? null : this.duration.getValue(); 1515 } 1516 1517 /** 1518 * @param value The duration of the recording in seconds - for audio and video. 1519 */ 1520 public Media setDuration(BigDecimal value) { 1521 if (value == null) 1522 this.duration = null; 1523 else { 1524 if (this.duration == null) 1525 this.duration = new DecimalType(); 1526 this.duration.setValue(value); 1527 } 1528 return this; 1529 } 1530 1531 /** 1532 * @param value The duration of the recording in seconds - for audio and video. 1533 */ 1534 public Media setDuration(long value) { 1535 this.duration = new DecimalType(); 1536 this.duration.setValue(value); 1537 return this; 1538 } 1539 1540 /** 1541 * @param value The duration of the recording in seconds - for audio and video. 1542 */ 1543 public Media setDuration(double value) { 1544 this.duration = new DecimalType(); 1545 this.duration.setValue(value); 1546 return this; 1547 } 1548 1549 /** 1550 * @return {@link #content} (The actual content of the media - inline or by 1551 * direct reference to the media source file.) 1552 */ 1553 public Attachment getContent() { 1554 if (this.content == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create Media.content"); 1557 else if (Configuration.doAutoCreate()) 1558 this.content = new Attachment(); // cc 1559 return this.content; 1560 } 1561 1562 public boolean hasContent() { 1563 return this.content != null && !this.content.isEmpty(); 1564 } 1565 1566 /** 1567 * @param value {@link #content} (The actual content of the media - inline or by 1568 * direct reference to the media source file.) 1569 */ 1570 public Media setContent(Attachment value) { 1571 this.content = value; 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #note} (Comments made about the media by the performer, 1577 * subject or other participants.) 1578 */ 1579 public List<Annotation> getNote() { 1580 if (this.note == null) 1581 this.note = new ArrayList<Annotation>(); 1582 return this.note; 1583 } 1584 1585 /** 1586 * @return Returns a reference to <code>this</code> for easy method chaining 1587 */ 1588 public Media setNote(List<Annotation> theNote) { 1589 this.note = theNote; 1590 return this; 1591 } 1592 1593 public boolean hasNote() { 1594 if (this.note == null) 1595 return false; 1596 for (Annotation item : this.note) 1597 if (!item.isEmpty()) 1598 return true; 1599 return false; 1600 } 1601 1602 public Annotation addNote() { // 3 1603 Annotation t = new Annotation(); 1604 if (this.note == null) 1605 this.note = new ArrayList<Annotation>(); 1606 this.note.add(t); 1607 return t; 1608 } 1609 1610 public Media addNote(Annotation t) { // 3 1611 if (t == null) 1612 return this; 1613 if (this.note == null) 1614 this.note = new ArrayList<Annotation>(); 1615 this.note.add(t); 1616 return this; 1617 } 1618 1619 /** 1620 * @return The first repetition of repeating field {@link #note}, creating it if 1621 * it does not already exist 1622 */ 1623 public Annotation getNoteFirstRep() { 1624 if (getNote().isEmpty()) { 1625 addNote(); 1626 } 1627 return getNote().get(0); 1628 } 1629 1630 protected void listChildren(List<Property> children) { 1631 super.listChildren(children); 1632 children.add(new Property("identifier", "Identifier", 1633 "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 1634 0, java.lang.Integer.MAX_VALUE, identifier)); 1635 children.add(new Property("basedOn", "Reference(ServiceRequest|CarePlan)", 1636 "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, 1637 java.lang.Integer.MAX_VALUE, basedOn)); 1638 children.add(new Property("partOf", "Reference(Any)", 1639 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1640 partOf)); 1641 children.add(new Property("status", "code", "The current state of the {{title}}.", 0, 1, status)); 1642 children.add(new Property("type", "CodeableConcept", 1643 "A code that classifies whether the media is an image, video or audio recording or some other media category.", 1644 0, 1, type)); 1645 children.add(new Property("modality", "CodeableConcept", 1646 "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 1647 0, 1, modality)); 1648 children.add(new Property("view", "CodeableConcept", 1649 "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view)); 1650 children 1651 .add(new Property("subject", "Reference(Patient|Practitioner|PractitionerRole|Group|Device|Specimen|Location)", 1652 "Who/What this Media is a record of.", 0, 1, subject)); 1653 children.add(new Property("encounter", "Reference(Encounter)", 1654 "The encounter that establishes the context for this media.", 0, 1, encounter)); 1655 children.add(new Property("created[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 1656 0, 1, created)); 1657 children.add(new Property("issued", "instant", 1658 "The date and time this version of the media was made available to providers, typically after having been reviewed.", 1659 0, 1, issued)); 1660 children.add(new Property("operator", 1661 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1662 "The person who administered the collection of the image.", 0, 1, operator)); 1663 children.add(new Property("reasonCode", "CodeableConcept", 1664 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1665 children.add(new Property("bodySite", "CodeableConcept", 1666 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 1667 bodySite)); 1668 children.add(new Property("deviceName", "string", 1669 "The name of the device / manufacturer of the device that was used to make the recording.", 0, 1, deviceName)); 1670 children.add(new Property("device", "Reference(Device|DeviceMetric|Device)", 1671 "The device used to collect the media.", 0, 1, device)); 1672 children.add(new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height)); 1673 children.add(new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width)); 1674 children.add(new Property("frames", "positiveInt", 1675 "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 1676 0, 1, frames)); 1677 children.add(new Property("duration", "decimal", "The duration of the recording in seconds - for audio and video.", 1678 0, 1, duration)); 1679 children.add(new Property("content", "Attachment", 1680 "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content)); 1681 children.add(new Property("note", "Annotation", 1682 "Comments made about the media by the performer, subject or other participants.", 0, 1683 java.lang.Integer.MAX_VALUE, note)); 1684 } 1685 1686 @Override 1687 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1688 switch (_hash) { 1689 case -1618432855: 1690 /* identifier */ return new Property("identifier", "Identifier", 1691 "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 1692 0, java.lang.Integer.MAX_VALUE, identifier); 1693 case -332612366: 1694 /* basedOn */ return new Property("basedOn", "Reference(ServiceRequest|CarePlan)", 1695 "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, 1696 java.lang.Integer.MAX_VALUE, basedOn); 1697 case -995410646: 1698 /* partOf */ return new Property("partOf", "Reference(Any)", 1699 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 1700 partOf); 1701 case -892481550: 1702 /* status */ return new Property("status", "code", "The current state of the {{title}}.", 0, 1, status); 1703 case 3575610: 1704 /* type */ return new Property("type", "CodeableConcept", 1705 "A code that classifies whether the media is an image, video or audio recording or some other media category.", 1706 0, 1, type); 1707 case -622722335: 1708 /* modality */ return new Property("modality", "CodeableConcept", 1709 "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 1710 0, 1, modality); 1711 case 3619493: 1712 /* view */ return new Property("view", "CodeableConcept", 1713 "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view); 1714 case -1867885268: 1715 /* subject */ return new Property("subject", 1716 "Reference(Patient|Practitioner|PractitionerRole|Group|Device|Specimen|Location)", 1717 "Who/What this Media is a record of.", 0, 1, subject); 1718 case 1524132147: 1719 /* encounter */ return new Property("encounter", "Reference(Encounter)", 1720 "The encounter that establishes the context for this media.", 0, 1, encounter); 1721 case 1369676952: 1722 /* created[x] */ return new Property("created[x]", "dateTime|Period", 1723 "The date and time(s) at which the media was collected.", 0, 1, created); 1724 case 1028554472: 1725 /* created */ return new Property("created[x]", "dateTime|Period", 1726 "The date and time(s) at which the media was collected.", 0, 1, created); 1727 case -1968526685: 1728 /* createdDateTime */ return new Property("created[x]", "dateTime|Period", 1729 "The date and time(s) at which the media was collected.", 0, 1, created); 1730 case 1525027529: 1731 /* createdPeriod */ return new Property("created[x]", "dateTime|Period", 1732 "The date and time(s) at which the media was collected.", 0, 1, created); 1733 case -1179159893: 1734 /* issued */ return new Property("issued", "instant", 1735 "The date and time this version of the media was made available to providers, typically after having been reviewed.", 1736 0, 1, issued); 1737 case -500553564: 1738 /* operator */ return new Property("operator", 1739 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson)", 1740 "The person who administered the collection of the image.", 0, 1, operator); 1741 case 722137681: 1742 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 1743 "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1744 case 1702620169: 1745 /* bodySite */ return new Property("bodySite", "CodeableConcept", 1746 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 1747 bodySite); 1748 case 780988929: 1749 /* deviceName */ return new Property("deviceName", "string", 1750 "The name of the device / manufacturer of the device that was used to make the recording.", 0, 1, 1751 deviceName); 1752 case -1335157162: 1753 /* device */ return new Property("device", "Reference(Device|DeviceMetric|Device)", 1754 "The device used to collect the media.", 0, 1, device); 1755 case -1221029593: 1756 /* height */ return new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, 1757 height); 1758 case 113126854: 1759 /* width */ return new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, 1760 width); 1761 case -1266514778: 1762 /* frames */ return new Property("frames", "positiveInt", 1763 "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 1764 0, 1, frames); 1765 case -1992012396: 1766 /* duration */ return new Property("duration", "decimal", 1767 "The duration of the recording in seconds - for audio and video.", 0, 1, duration); 1768 case 951530617: 1769 /* content */ return new Property("content", "Attachment", 1770 "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content); 1771 case 3387378: 1772 /* note */ return new Property("note", "Annotation", 1773 "Comments made about the media by the performer, subject or other participants.", 0, 1774 java.lang.Integer.MAX_VALUE, note); 1775 default: 1776 return super.getNamedProperty(_hash, _name, _checkValid); 1777 } 1778 1779 } 1780 1781 @Override 1782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1783 switch (hash) { 1784 case -1618432855: 1785 /* identifier */ return this.identifier == null ? new Base[0] 1786 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1787 case -332612366: 1788 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1789 case -995410646: 1790 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1791 case -892481550: 1792 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MediaStatus> 1793 case 3575610: 1794 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1795 case -622722335: 1796 /* modality */ return this.modality == null ? new Base[0] : new Base[] { this.modality }; // CodeableConcept 1797 case 3619493: 1798 /* view */ return this.view == null ? new Base[0] : new Base[] { this.view }; // CodeableConcept 1799 case -1867885268: 1800 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 1801 case 1524132147: 1802 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 1803 case 1028554472: 1804 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // Type 1805 case -1179159893: 1806 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 1807 case -500553564: 1808 /* operator */ return this.operator == null ? new Base[0] : new Base[] { this.operator }; // Reference 1809 case 722137681: 1810 /* reasonCode */ return this.reasonCode == null ? new Base[0] 1811 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1812 case 1702620169: 1813 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 1814 case 780988929: 1815 /* deviceName */ return this.deviceName == null ? new Base[0] : new Base[] { this.deviceName }; // StringType 1816 case -1335157162: 1817 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 1818 case -1221029593: 1819 /* height */ return this.height == null ? new Base[0] : new Base[] { this.height }; // PositiveIntType 1820 case 113126854: 1821 /* width */ return this.width == null ? new Base[0] : new Base[] { this.width }; // PositiveIntType 1822 case -1266514778: 1823 /* frames */ return this.frames == null ? new Base[0] : new Base[] { this.frames }; // PositiveIntType 1824 case -1992012396: 1825 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // DecimalType 1826 case 951530617: 1827 /* content */ return this.content == null ? new Base[0] : new Base[] { this.content }; // Attachment 1828 case 3387378: 1829 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1830 default: 1831 return super.getProperty(hash, name, checkValid); 1832 } 1833 1834 } 1835 1836 @Override 1837 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1838 switch (hash) { 1839 case -1618432855: // identifier 1840 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1841 return value; 1842 case -332612366: // basedOn 1843 this.getBasedOn().add(castToReference(value)); // Reference 1844 return value; 1845 case -995410646: // partOf 1846 this.getPartOf().add(castToReference(value)); // Reference 1847 return value; 1848 case -892481550: // status 1849 value = new MediaStatusEnumFactory().fromType(castToCode(value)); 1850 this.status = (Enumeration) value; // Enumeration<MediaStatus> 1851 return value; 1852 case 3575610: // type 1853 this.type = castToCodeableConcept(value); // CodeableConcept 1854 return value; 1855 case -622722335: // modality 1856 this.modality = castToCodeableConcept(value); // CodeableConcept 1857 return value; 1858 case 3619493: // view 1859 this.view = castToCodeableConcept(value); // CodeableConcept 1860 return value; 1861 case -1867885268: // subject 1862 this.subject = castToReference(value); // Reference 1863 return value; 1864 case 1524132147: // encounter 1865 this.encounter = castToReference(value); // Reference 1866 return value; 1867 case 1028554472: // created 1868 this.created = castToType(value); // Type 1869 return value; 1870 case -1179159893: // issued 1871 this.issued = castToInstant(value); // InstantType 1872 return value; 1873 case -500553564: // operator 1874 this.operator = castToReference(value); // Reference 1875 return value; 1876 case 722137681: // reasonCode 1877 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1878 return value; 1879 case 1702620169: // bodySite 1880 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1881 return value; 1882 case 780988929: // deviceName 1883 this.deviceName = castToString(value); // StringType 1884 return value; 1885 case -1335157162: // device 1886 this.device = castToReference(value); // Reference 1887 return value; 1888 case -1221029593: // height 1889 this.height = castToPositiveInt(value); // PositiveIntType 1890 return value; 1891 case 113126854: // width 1892 this.width = castToPositiveInt(value); // PositiveIntType 1893 return value; 1894 case -1266514778: // frames 1895 this.frames = castToPositiveInt(value); // PositiveIntType 1896 return value; 1897 case -1992012396: // duration 1898 this.duration = castToDecimal(value); // DecimalType 1899 return value; 1900 case 951530617: // content 1901 this.content = castToAttachment(value); // Attachment 1902 return value; 1903 case 3387378: // note 1904 this.getNote().add(castToAnnotation(value)); // Annotation 1905 return value; 1906 default: 1907 return super.setProperty(hash, name, value); 1908 } 1909 1910 } 1911 1912 @Override 1913 public Base setProperty(String name, Base value) throws FHIRException { 1914 if (name.equals("identifier")) { 1915 this.getIdentifier().add(castToIdentifier(value)); 1916 } else if (name.equals("basedOn")) { 1917 this.getBasedOn().add(castToReference(value)); 1918 } else if (name.equals("partOf")) { 1919 this.getPartOf().add(castToReference(value)); 1920 } else if (name.equals("status")) { 1921 value = new MediaStatusEnumFactory().fromType(castToCode(value)); 1922 this.status = (Enumeration) value; // Enumeration<MediaStatus> 1923 } else if (name.equals("type")) { 1924 this.type = castToCodeableConcept(value); // CodeableConcept 1925 } else if (name.equals("modality")) { 1926 this.modality = castToCodeableConcept(value); // CodeableConcept 1927 } else if (name.equals("view")) { 1928 this.view = castToCodeableConcept(value); // CodeableConcept 1929 } else if (name.equals("subject")) { 1930 this.subject = castToReference(value); // Reference 1931 } else if (name.equals("encounter")) { 1932 this.encounter = castToReference(value); // Reference 1933 } else if (name.equals("created[x]")) { 1934 this.created = castToType(value); // Type 1935 } else if (name.equals("issued")) { 1936 this.issued = castToInstant(value); // InstantType 1937 } else if (name.equals("operator")) { 1938 this.operator = castToReference(value); // Reference 1939 } else if (name.equals("reasonCode")) { 1940 this.getReasonCode().add(castToCodeableConcept(value)); 1941 } else if (name.equals("bodySite")) { 1942 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1943 } else if (name.equals("deviceName")) { 1944 this.deviceName = castToString(value); // StringType 1945 } else if (name.equals("device")) { 1946 this.device = castToReference(value); // Reference 1947 } else if (name.equals("height")) { 1948 this.height = castToPositiveInt(value); // PositiveIntType 1949 } else if (name.equals("width")) { 1950 this.width = castToPositiveInt(value); // PositiveIntType 1951 } else if (name.equals("frames")) { 1952 this.frames = castToPositiveInt(value); // PositiveIntType 1953 } else if (name.equals("duration")) { 1954 this.duration = castToDecimal(value); // DecimalType 1955 } else if (name.equals("content")) { 1956 this.content = castToAttachment(value); // Attachment 1957 } else if (name.equals("note")) { 1958 this.getNote().add(castToAnnotation(value)); 1959 } else 1960 return super.setProperty(name, value); 1961 return value; 1962 } 1963 1964 @Override 1965 public void removeChild(String name, Base value) throws FHIRException { 1966 if (name.equals("identifier")) { 1967 this.getIdentifier().remove(castToIdentifier(value)); 1968 } else if (name.equals("basedOn")) { 1969 this.getBasedOn().remove(castToReference(value)); 1970 } else if (name.equals("partOf")) { 1971 this.getPartOf().remove(castToReference(value)); 1972 } else if (name.equals("status")) { 1973 this.status = null; 1974 } else if (name.equals("type")) { 1975 this.type = null; 1976 } else if (name.equals("modality")) { 1977 this.modality = null; 1978 } else if (name.equals("view")) { 1979 this.view = null; 1980 } else if (name.equals("subject")) { 1981 this.subject = null; 1982 } else if (name.equals("encounter")) { 1983 this.encounter = null; 1984 } else if (name.equals("created[x]")) { 1985 this.created = null; 1986 } else if (name.equals("issued")) { 1987 this.issued = null; 1988 } else if (name.equals("operator")) { 1989 this.operator = null; 1990 } else if (name.equals("reasonCode")) { 1991 this.getReasonCode().remove(castToCodeableConcept(value)); 1992 } else if (name.equals("bodySite")) { 1993 this.bodySite = null; 1994 } else if (name.equals("deviceName")) { 1995 this.deviceName = null; 1996 } else if (name.equals("device")) { 1997 this.device = null; 1998 } else if (name.equals("height")) { 1999 this.height = null; 2000 } else if (name.equals("width")) { 2001 this.width = null; 2002 } else if (name.equals("frames")) { 2003 this.frames = null; 2004 } else if (name.equals("duration")) { 2005 this.duration = null; 2006 } else if (name.equals("content")) { 2007 this.content = null; 2008 } else if (name.equals("note")) { 2009 this.getNote().remove(castToAnnotation(value)); 2010 } else 2011 super.removeChild(name, value); 2012 2013 } 2014 2015 @Override 2016 public Base makeProperty(int hash, String name) throws FHIRException { 2017 switch (hash) { 2018 case -1618432855: 2019 return addIdentifier(); 2020 case -332612366: 2021 return addBasedOn(); 2022 case -995410646: 2023 return addPartOf(); 2024 case -892481550: 2025 return getStatusElement(); 2026 case 3575610: 2027 return getType(); 2028 case -622722335: 2029 return getModality(); 2030 case 3619493: 2031 return getView(); 2032 case -1867885268: 2033 return getSubject(); 2034 case 1524132147: 2035 return getEncounter(); 2036 case 1369676952: 2037 return getCreated(); 2038 case 1028554472: 2039 return getCreated(); 2040 case -1179159893: 2041 return getIssuedElement(); 2042 case -500553564: 2043 return getOperator(); 2044 case 722137681: 2045 return addReasonCode(); 2046 case 1702620169: 2047 return getBodySite(); 2048 case 780988929: 2049 return getDeviceNameElement(); 2050 case -1335157162: 2051 return getDevice(); 2052 case -1221029593: 2053 return getHeightElement(); 2054 case 113126854: 2055 return getWidthElement(); 2056 case -1266514778: 2057 return getFramesElement(); 2058 case -1992012396: 2059 return getDurationElement(); 2060 case 951530617: 2061 return getContent(); 2062 case 3387378: 2063 return addNote(); 2064 default: 2065 return super.makeProperty(hash, name); 2066 } 2067 2068 } 2069 2070 @Override 2071 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2072 switch (hash) { 2073 case -1618432855: 2074 /* identifier */ return new String[] { "Identifier" }; 2075 case -332612366: 2076 /* basedOn */ return new String[] { "Reference" }; 2077 case -995410646: 2078 /* partOf */ return new String[] { "Reference" }; 2079 case -892481550: 2080 /* status */ return new String[] { "code" }; 2081 case 3575610: 2082 /* type */ return new String[] { "CodeableConcept" }; 2083 case -622722335: 2084 /* modality */ return new String[] { "CodeableConcept" }; 2085 case 3619493: 2086 /* view */ return new String[] { "CodeableConcept" }; 2087 case -1867885268: 2088 /* subject */ return new String[] { "Reference" }; 2089 case 1524132147: 2090 /* encounter */ return new String[] { "Reference" }; 2091 case 1028554472: 2092 /* created */ return new String[] { "dateTime", "Period" }; 2093 case -1179159893: 2094 /* issued */ return new String[] { "instant" }; 2095 case -500553564: 2096 /* operator */ return new String[] { "Reference" }; 2097 case 722137681: 2098 /* reasonCode */ return new String[] { "CodeableConcept" }; 2099 case 1702620169: 2100 /* bodySite */ return new String[] { "CodeableConcept" }; 2101 case 780988929: 2102 /* deviceName */ return new String[] { "string" }; 2103 case -1335157162: 2104 /* device */ return new String[] { "Reference" }; 2105 case -1221029593: 2106 /* height */ return new String[] { "positiveInt" }; 2107 case 113126854: 2108 /* width */ return new String[] { "positiveInt" }; 2109 case -1266514778: 2110 /* frames */ return new String[] { "positiveInt" }; 2111 case -1992012396: 2112 /* duration */ return new String[] { "decimal" }; 2113 case 951530617: 2114 /* content */ return new String[] { "Attachment" }; 2115 case 3387378: 2116 /* note */ return new String[] { "Annotation" }; 2117 default: 2118 return super.getTypesForProperty(hash, name); 2119 } 2120 2121 } 2122 2123 @Override 2124 public Base addChild(String name) throws FHIRException { 2125 if (name.equals("identifier")) { 2126 return addIdentifier(); 2127 } else if (name.equals("basedOn")) { 2128 return addBasedOn(); 2129 } else if (name.equals("partOf")) { 2130 return addPartOf(); 2131 } else if (name.equals("status")) { 2132 throw new FHIRException("Cannot call addChild on a singleton property Media.status"); 2133 } else if (name.equals("type")) { 2134 this.type = new CodeableConcept(); 2135 return this.type; 2136 } else if (name.equals("modality")) { 2137 this.modality = new CodeableConcept(); 2138 return this.modality; 2139 } else if (name.equals("view")) { 2140 this.view = new CodeableConcept(); 2141 return this.view; 2142 } else if (name.equals("subject")) { 2143 this.subject = new Reference(); 2144 return this.subject; 2145 } else if (name.equals("encounter")) { 2146 this.encounter = new Reference(); 2147 return this.encounter; 2148 } else if (name.equals("createdDateTime")) { 2149 this.created = new DateTimeType(); 2150 return this.created; 2151 } else if (name.equals("createdPeriod")) { 2152 this.created = new Period(); 2153 return this.created; 2154 } else if (name.equals("issued")) { 2155 throw new FHIRException("Cannot call addChild on a singleton property Media.issued"); 2156 } else if (name.equals("operator")) { 2157 this.operator = new Reference(); 2158 return this.operator; 2159 } else if (name.equals("reasonCode")) { 2160 return addReasonCode(); 2161 } else if (name.equals("bodySite")) { 2162 this.bodySite = new CodeableConcept(); 2163 return this.bodySite; 2164 } else if (name.equals("deviceName")) { 2165 throw new FHIRException("Cannot call addChild on a singleton property Media.deviceName"); 2166 } else if (name.equals("device")) { 2167 this.device = new Reference(); 2168 return this.device; 2169 } else if (name.equals("height")) { 2170 throw new FHIRException("Cannot call addChild on a singleton property Media.height"); 2171 } else if (name.equals("width")) { 2172 throw new FHIRException("Cannot call addChild on a singleton property Media.width"); 2173 } else if (name.equals("frames")) { 2174 throw new FHIRException("Cannot call addChild on a singleton property Media.frames"); 2175 } else if (name.equals("duration")) { 2176 throw new FHIRException("Cannot call addChild on a singleton property Media.duration"); 2177 } else if (name.equals("content")) { 2178 this.content = new Attachment(); 2179 return this.content; 2180 } else if (name.equals("note")) { 2181 return addNote(); 2182 } else 2183 return super.addChild(name); 2184 } 2185 2186 public String fhirType() { 2187 return "Media"; 2188 2189 } 2190 2191 public Media copy() { 2192 Media dst = new Media(); 2193 copyValues(dst); 2194 return dst; 2195 } 2196 2197 public void copyValues(Media dst) { 2198 super.copyValues(dst); 2199 if (identifier != null) { 2200 dst.identifier = new ArrayList<Identifier>(); 2201 for (Identifier i : identifier) 2202 dst.identifier.add(i.copy()); 2203 } 2204 ; 2205 if (basedOn != null) { 2206 dst.basedOn = new ArrayList<Reference>(); 2207 for (Reference i : basedOn) 2208 dst.basedOn.add(i.copy()); 2209 } 2210 ; 2211 if (partOf != null) { 2212 dst.partOf = new ArrayList<Reference>(); 2213 for (Reference i : partOf) 2214 dst.partOf.add(i.copy()); 2215 } 2216 ; 2217 dst.status = status == null ? null : status.copy(); 2218 dst.type = type == null ? null : type.copy(); 2219 dst.modality = modality == null ? null : modality.copy(); 2220 dst.view = view == null ? null : view.copy(); 2221 dst.subject = subject == null ? null : subject.copy(); 2222 dst.encounter = encounter == null ? null : encounter.copy(); 2223 dst.created = created == null ? null : created.copy(); 2224 dst.issued = issued == null ? null : issued.copy(); 2225 dst.operator = operator == null ? null : operator.copy(); 2226 if (reasonCode != null) { 2227 dst.reasonCode = new ArrayList<CodeableConcept>(); 2228 for (CodeableConcept i : reasonCode) 2229 dst.reasonCode.add(i.copy()); 2230 } 2231 ; 2232 dst.bodySite = bodySite == null ? null : bodySite.copy(); 2233 dst.deviceName = deviceName == null ? null : deviceName.copy(); 2234 dst.device = device == null ? null : device.copy(); 2235 dst.height = height == null ? null : height.copy(); 2236 dst.width = width == null ? null : width.copy(); 2237 dst.frames = frames == null ? null : frames.copy(); 2238 dst.duration = duration == null ? null : duration.copy(); 2239 dst.content = content == null ? null : content.copy(); 2240 if (note != null) { 2241 dst.note = new ArrayList<Annotation>(); 2242 for (Annotation i : note) 2243 dst.note.add(i.copy()); 2244 } 2245 ; 2246 } 2247 2248 protected Media typedCopy() { 2249 return copy(); 2250 } 2251 2252 @Override 2253 public boolean equalsDeep(Base other_) { 2254 if (!super.equalsDeep(other_)) 2255 return false; 2256 if (!(other_ instanceof Media)) 2257 return false; 2258 Media o = (Media) other_; 2259 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 2260 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 2261 && compareDeep(modality, o.modality, true) && compareDeep(view, o.view, true) 2262 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2263 && compareDeep(created, o.created, true) && compareDeep(issued, o.issued, true) 2264 && compareDeep(operator, o.operator, true) && compareDeep(reasonCode, o.reasonCode, true) 2265 && compareDeep(bodySite, o.bodySite, true) && compareDeep(deviceName, o.deviceName, true) 2266 && compareDeep(device, o.device, true) && compareDeep(height, o.height, true) 2267 && compareDeep(width, o.width, true) && compareDeep(frames, o.frames, true) 2268 && compareDeep(duration, o.duration, true) && compareDeep(content, o.content, true) 2269 && compareDeep(note, o.note, true); 2270 } 2271 2272 @Override 2273 public boolean equalsShallow(Base other_) { 2274 if (!super.equalsShallow(other_)) 2275 return false; 2276 if (!(other_ instanceof Media)) 2277 return false; 2278 Media o = (Media) other_; 2279 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) 2280 && compareValues(deviceName, o.deviceName, true) && compareValues(height, o.height, true) 2281 && compareValues(width, o.width, true) && compareValues(frames, o.frames, true) 2282 && compareValues(duration, o.duration, true); 2283 } 2284 2285 public boolean isEmpty() { 2286 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, type, modality, 2287 view, subject, encounter, created, issued, operator, reasonCode, bodySite, deviceName, device, height, width, 2288 frames, duration, content, note); 2289 } 2290 2291 @Override 2292 public ResourceType getResourceType() { 2293 return ResourceType.Media; 2294 } 2295 2296 /** 2297 * Search parameter: <b>identifier</b> 2298 * <p> 2299 * Description: <b>Identifier(s) for the image</b><br> 2300 * Type: <b>token</b><br> 2301 * Path: <b>Media.identifier</b><br> 2302 * </p> 2303 */ 2304 @SearchParamDefinition(name = "identifier", path = "Media.identifier", description = "Identifier(s) for the image", type = "token") 2305 public static final String SP_IDENTIFIER = "identifier"; 2306 /** 2307 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2308 * <p> 2309 * Description: <b>Identifier(s) for the image</b><br> 2310 * Type: <b>token</b><br> 2311 * Path: <b>Media.identifier</b><br> 2312 * </p> 2313 */ 2314 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2315 SP_IDENTIFIER); 2316 2317 /** 2318 * Search parameter: <b>modality</b> 2319 * <p> 2320 * Description: <b>The type of acquisition equipment/process</b><br> 2321 * Type: <b>token</b><br> 2322 * Path: <b>Media.modality</b><br> 2323 * </p> 2324 */ 2325 @SearchParamDefinition(name = "modality", path = "Media.modality", description = "The type of acquisition equipment/process", type = "token") 2326 public static final String SP_MODALITY = "modality"; 2327 /** 2328 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 2329 * <p> 2330 * Description: <b>The type of acquisition equipment/process</b><br> 2331 * Type: <b>token</b><br> 2332 * Path: <b>Media.modality</b><br> 2333 * </p> 2334 */ 2335 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2336 SP_MODALITY); 2337 2338 /** 2339 * Search parameter: <b>subject</b> 2340 * <p> 2341 * Description: <b>Who/What this Media is a record of</b><br> 2342 * Type: <b>reference</b><br> 2343 * Path: <b>Media.subject</b><br> 2344 * </p> 2345 */ 2346 @SearchParamDefinition(name = "subject", path = "Media.subject", description = "Who/What this Media is a record of", type = "reference", providesMembershipIn = { 2347 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 2348 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 2349 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, Group.class, 2350 Location.class, Patient.class, Practitioner.class, PractitionerRole.class, Specimen.class }) 2351 public static final String SP_SUBJECT = "subject"; 2352 /** 2353 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2354 * <p> 2355 * Description: <b>Who/What this Media is a record of</b><br> 2356 * Type: <b>reference</b><br> 2357 * Path: <b>Media.subject</b><br> 2358 * </p> 2359 */ 2360 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2361 SP_SUBJECT); 2362 2363 /** 2364 * Constant for fluent queries to be used to add include statements. Specifies 2365 * the path value of "<b>Media:subject</b>". 2366 */ 2367 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Media:subject") 2368 .toLocked(); 2369 2370 /** 2371 * Search parameter: <b>created</b> 2372 * <p> 2373 * Description: <b>When Media was collected</b><br> 2374 * Type: <b>date</b><br> 2375 * Path: <b>Media.created[x]</b><br> 2376 * </p> 2377 */ 2378 @SearchParamDefinition(name = "created", path = "Media.created", description = "When Media was collected", type = "date") 2379 public static final String SP_CREATED = "created"; 2380 /** 2381 * <b>Fluent Client</b> search parameter constant for <b>created</b> 2382 * <p> 2383 * Description: <b>When Media was collected</b><br> 2384 * Type: <b>date</b><br> 2385 * Path: <b>Media.created[x]</b><br> 2386 * </p> 2387 */ 2388 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 2389 SP_CREATED); 2390 2391 /** 2392 * Search parameter: <b>encounter</b> 2393 * <p> 2394 * Description: <b>Encounter associated with media</b><br> 2395 * Type: <b>reference</b><br> 2396 * Path: <b>Media.encounter</b><br> 2397 * </p> 2398 */ 2399 @SearchParamDefinition(name = "encounter", path = "Media.encounter", description = "Encounter associated with media", type = "reference", providesMembershipIn = { 2400 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 2401 public static final String SP_ENCOUNTER = "encounter"; 2402 /** 2403 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 2404 * <p> 2405 * Description: <b>Encounter associated with media</b><br> 2406 * Type: <b>reference</b><br> 2407 * Path: <b>Media.encounter</b><br> 2408 * </p> 2409 */ 2410 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2411 SP_ENCOUNTER); 2412 2413 /** 2414 * Constant for fluent queries to be used to add include statements. Specifies 2415 * the path value of "<b>Media:encounter</b>". 2416 */ 2417 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 2418 "Media:encounter").toLocked(); 2419 2420 /** 2421 * Search parameter: <b>type</b> 2422 * <p> 2423 * Description: <b>Classification of media as image, video, or audio</b><br> 2424 * Type: <b>token</b><br> 2425 * Path: <b>Media.type</b><br> 2426 * </p> 2427 */ 2428 @SearchParamDefinition(name = "type", path = "Media.type", description = "Classification of media as image, video, or audio", type = "token") 2429 public static final String SP_TYPE = "type"; 2430 /** 2431 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2432 * <p> 2433 * Description: <b>Classification of media as image, video, or audio</b><br> 2434 * Type: <b>token</b><br> 2435 * Path: <b>Media.type</b><br> 2436 * </p> 2437 */ 2438 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2439 SP_TYPE); 2440 2441 /** 2442 * Search parameter: <b>operator</b> 2443 * <p> 2444 * Description: <b>The person who generated the image</b><br> 2445 * Type: <b>reference</b><br> 2446 * Path: <b>Media.operator</b><br> 2447 * </p> 2448 */ 2449 @SearchParamDefinition(name = "operator", path = "Media.operator", description = "The person who generated the image", type = "reference", providesMembershipIn = { 2450 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { CareTeam.class, Device.class, 2451 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 2452 public static final String SP_OPERATOR = "operator"; 2453 /** 2454 * <b>Fluent Client</b> search parameter constant for <b>operator</b> 2455 * <p> 2456 * Description: <b>The person who generated the image</b><br> 2457 * Type: <b>reference</b><br> 2458 * Path: <b>Media.operator</b><br> 2459 * </p> 2460 */ 2461 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OPERATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2462 SP_OPERATOR); 2463 2464 /** 2465 * Constant for fluent queries to be used to add include statements. Specifies 2466 * the path value of "<b>Media:operator</b>". 2467 */ 2468 public static final ca.uhn.fhir.model.api.Include INCLUDE_OPERATOR = new ca.uhn.fhir.model.api.Include( 2469 "Media:operator").toLocked(); 2470 2471 /** 2472 * Search parameter: <b>view</b> 2473 * <p> 2474 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 2475 * Type: <b>token</b><br> 2476 * Path: <b>Media.view</b><br> 2477 * </p> 2478 */ 2479 @SearchParamDefinition(name = "view", path = "Media.view", description = "Imaging view, e.g. Lateral or Antero-posterior", type = "token") 2480 public static final String SP_VIEW = "view"; 2481 /** 2482 * <b>Fluent Client</b> search parameter constant for <b>view</b> 2483 * <p> 2484 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 2485 * Type: <b>token</b><br> 2486 * Path: <b>Media.view</b><br> 2487 * </p> 2488 */ 2489 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VIEW = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2490 SP_VIEW); 2491 2492 /** 2493 * Search parameter: <b>site</b> 2494 * <p> 2495 * Description: <b>Observed body part</b><br> 2496 * Type: <b>token</b><br> 2497 * Path: <b>Media.bodySite</b><br> 2498 * </p> 2499 */ 2500 @SearchParamDefinition(name = "site", path = "Media.bodySite", description = "Observed body part", type = "token") 2501 public static final String SP_SITE = "site"; 2502 /** 2503 * <b>Fluent Client</b> search parameter constant for <b>site</b> 2504 * <p> 2505 * Description: <b>Observed body part</b><br> 2506 * Type: <b>token</b><br> 2507 * Path: <b>Media.bodySite</b><br> 2508 * </p> 2509 */ 2510 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2511 SP_SITE); 2512 2513 /** 2514 * Search parameter: <b>based-on</b> 2515 * <p> 2516 * Description: <b>Procedure that caused this media to be created</b><br> 2517 * Type: <b>reference</b><br> 2518 * Path: <b>Media.basedOn</b><br> 2519 * </p> 2520 */ 2521 @SearchParamDefinition(name = "based-on", path = "Media.basedOn", description = "Procedure that caused this media to be created", type = "reference", target = { 2522 CarePlan.class, ServiceRequest.class }) 2523 public static final String SP_BASED_ON = "based-on"; 2524 /** 2525 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 2526 * <p> 2527 * Description: <b>Procedure that caused this media to be created</b><br> 2528 * Type: <b>reference</b><br> 2529 * Path: <b>Media.basedOn</b><br> 2530 * </p> 2531 */ 2532 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2533 SP_BASED_ON); 2534 2535 /** 2536 * Constant for fluent queries to be used to add include statements. Specifies 2537 * the path value of "<b>Media:based-on</b>". 2538 */ 2539 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 2540 "Media:based-on").toLocked(); 2541 2542 /** 2543 * Search parameter: <b>patient</b> 2544 * <p> 2545 * Description: <b>Who/What this Media is a record of</b><br> 2546 * Type: <b>reference</b><br> 2547 * Path: <b>Media.subject</b><br> 2548 * </p> 2549 */ 2550 @SearchParamDefinition(name = "patient", path = "Media.subject.where(resolve() is Patient)", description = "Who/What this Media is a record of", type = "reference", target = { 2551 Patient.class }) 2552 public static final String SP_PATIENT = "patient"; 2553 /** 2554 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2555 * <p> 2556 * Description: <b>Who/What this Media is a record of</b><br> 2557 * Type: <b>reference</b><br> 2558 * Path: <b>Media.subject</b><br> 2559 * </p> 2560 */ 2561 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2562 SP_PATIENT); 2563 2564 /** 2565 * Constant for fluent queries to be used to add include statements. Specifies 2566 * the path value of "<b>Media:patient</b>". 2567 */ 2568 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Media:patient") 2569 .toLocked(); 2570 2571 /** 2572 * Search parameter: <b>device</b> 2573 * <p> 2574 * Description: <b>Observing Device</b><br> 2575 * Type: <b>reference</b><br> 2576 * Path: <b>Media.device</b><br> 2577 * </p> 2578 */ 2579 @SearchParamDefinition(name = "device", path = "Media.device", description = "Observing Device", type = "reference", target = { 2580 Device.class, DeviceMetric.class }) 2581 public static final String SP_DEVICE = "device"; 2582 /** 2583 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2584 * <p> 2585 * Description: <b>Observing Device</b><br> 2586 * Type: <b>reference</b><br> 2587 * Path: <b>Media.device</b><br> 2588 * </p> 2589 */ 2590 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2591 SP_DEVICE); 2592 2593 /** 2594 * Constant for fluent queries to be used to add include statements. Specifies 2595 * the path value of "<b>Media:device</b>". 2596 */ 2597 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Media:device") 2598 .toLocked(); 2599 2600 /** 2601 * Search parameter: <b>status</b> 2602 * <p> 2603 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 2604 * completed | entered-in-error | unknown</b><br> 2605 * Type: <b>token</b><br> 2606 * Path: <b>Media.status</b><br> 2607 * </p> 2608 */ 2609 @SearchParamDefinition(name = "status", path = "Media.status", description = "preparation | in-progress | not-done | on-hold | stopped | completed | entered-in-error | unknown", type = "token") 2610 public static final String SP_STATUS = "status"; 2611 /** 2612 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2613 * <p> 2614 * Description: <b>preparation | in-progress | not-done | on-hold | stopped | 2615 * completed | entered-in-error | unknown</b><br> 2616 * Type: <b>token</b><br> 2617 * Path: <b>Media.status</b><br> 2618 * </p> 2619 */ 2620 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2621 SP_STATUS); 2622 2623}