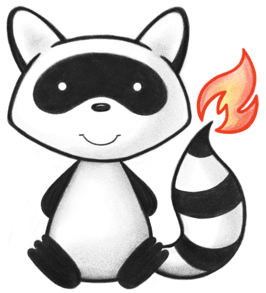
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * This resource is primarily used for the identification and definition of a 049 * medication for the purposes of prescribing, dispensing, and administering a 050 * medication as well as for making statements about medication use. 051 */ 052@ResourceDef(name = "Medication", profile = "http://hl7.org/fhir/StructureDefinition/Medication") 053public class Medication extends DomainResource { 054 055 public enum MedicationStatus { 056 /** 057 * The medication is available for use. 058 */ 059 ACTIVE, 060 /** 061 * The medication is not available for use. 062 */ 063 INACTIVE, 064 /** 065 * The medication was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 073 public static MedicationStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MedicationStatus code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case ACTIVE: 091 return "active"; 092 case INACTIVE: 093 return "inactive"; 094 case ENTEREDINERROR: 095 return "entered-in-error"; 096 case NULL: 097 return null; 098 default: 099 return "?"; 100 } 101 } 102 103 public String getSystem() { 104 switch (this) { 105 case ACTIVE: 106 return "http://hl7.org/fhir/CodeSystem/medication-status"; 107 case INACTIVE: 108 return "http://hl7.org/fhir/CodeSystem/medication-status"; 109 case ENTEREDINERROR: 110 return "http://hl7.org/fhir/CodeSystem/medication-status"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getDefinition() { 119 switch (this) { 120 case ACTIVE: 121 return "The medication is available for use."; 122 case INACTIVE: 123 return "The medication is not available for use."; 124 case ENTEREDINERROR: 125 return "The medication was entered in error."; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getDisplay() { 134 switch (this) { 135 case ACTIVE: 136 return "Active"; 137 case INACTIVE: 138 return "Inactive"; 139 case ENTEREDINERROR: 140 return "Entered in Error"; 141 case NULL: 142 return null; 143 default: 144 return "?"; 145 } 146 } 147 } 148 149 public static class MedicationStatusEnumFactory implements EnumFactory<MedicationStatus> { 150 public MedicationStatus fromCode(String codeString) throws IllegalArgumentException { 151 if (codeString == null || "".equals(codeString)) 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("active".equals(codeString)) 155 return MedicationStatus.ACTIVE; 156 if ("inactive".equals(codeString)) 157 return MedicationStatus.INACTIVE; 158 if ("entered-in-error".equals(codeString)) 159 return MedicationStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown MedicationStatus code '" + codeString + "'"); 161 } 162 163 public Enumeration<MedicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<MedicationStatus>(this, MedicationStatus.NULL, code); 168 String codeString = code.asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<MedicationStatus>(this, MedicationStatus.NULL, code); 171 if ("active".equals(codeString)) 172 return new Enumeration<MedicationStatus>(this, MedicationStatus.ACTIVE, code); 173 if ("inactive".equals(codeString)) 174 return new Enumeration<MedicationStatus>(this, MedicationStatus.INACTIVE, code); 175 if ("entered-in-error".equals(codeString)) 176 return new Enumeration<MedicationStatus>(this, MedicationStatus.ENTEREDINERROR, code); 177 throw new FHIRException("Unknown MedicationStatus code '" + codeString + "'"); 178 } 179 180 public String toCode(MedicationStatus code) { 181 if (code == MedicationStatus.ACTIVE) 182 return "active"; 183 if (code == MedicationStatus.INACTIVE) 184 return "inactive"; 185 if (code == MedicationStatus.ENTEREDINERROR) 186 return "entered-in-error"; 187 return "?"; 188 } 189 190 public String toSystem(MedicationStatus code) { 191 return code.getSystem(); 192 } 193 } 194 195 @Block() 196 public static class MedicationIngredientComponent extends BackboneElement implements IBaseBackboneElement { 197 /** 198 * The actual ingredient - either a substance (simple ingredient) or another 199 * medication of a medication. 200 */ 201 @Child(name = "item", type = { CodeableConcept.class, Substance.class, 202 Medication.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 203 @Description(shortDefinition = "The actual ingredient or content", formalDefinition = "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.") 204 protected Type item; 205 206 /** 207 * Indication of whether this ingredient affects the therapeutic action of the 208 * drug. 209 */ 210 @Child(name = "isActive", type = { 211 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 212 @Description(shortDefinition = "Active ingredient indicator", formalDefinition = "Indication of whether this ingredient affects the therapeutic action of the drug.") 213 protected BooleanType isActive; 214 215 /** 216 * Specifies how many (or how much) of the items there are in this Medication. 217 * For example, 250 mg per tablet. This is expressed as a ratio where the 218 * numerator is 250mg and the denominator is 1 tablet. 219 */ 220 @Child(name = "strength", type = { Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 221 @Description(shortDefinition = "Quantity of ingredient present", formalDefinition = "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.") 222 protected Ratio strength; 223 224 private static final long serialVersionUID = 1365103497L; 225 226 /** 227 * Constructor 228 */ 229 public MedicationIngredientComponent() { 230 super(); 231 } 232 233 /** 234 * Constructor 235 */ 236 public MedicationIngredientComponent(Type item) { 237 super(); 238 this.item = item; 239 } 240 241 /** 242 * @return {@link #item} (The actual ingredient - either a substance (simple 243 * ingredient) or another medication of a medication.) 244 */ 245 public Type getItem() { 246 return this.item; 247 } 248 249 /** 250 * @return {@link #item} (The actual ingredient - either a substance (simple 251 * ingredient) or another medication of a medication.) 252 */ 253 public CodeableConcept getItemCodeableConcept() throws FHIRException { 254 if (this.item == null) 255 this.item = new CodeableConcept(); 256 if (!(this.item instanceof CodeableConcept)) 257 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 258 + this.item.getClass().getName() + " was encountered"); 259 return (CodeableConcept) this.item; 260 } 261 262 public boolean hasItemCodeableConcept() { 263 return this != null && this.item instanceof CodeableConcept; 264 } 265 266 /** 267 * @return {@link #item} (The actual ingredient - either a substance (simple 268 * ingredient) or another medication of a medication.) 269 */ 270 public Reference getItemReference() throws FHIRException { 271 if (this.item == null) 272 this.item = new Reference(); 273 if (!(this.item instanceof Reference)) 274 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 275 + " was encountered"); 276 return (Reference) this.item; 277 } 278 279 public boolean hasItemReference() { 280 return this != null && this.item instanceof Reference; 281 } 282 283 public boolean hasItem() { 284 return this.item != null && !this.item.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #item} (The actual ingredient - either a substance 289 * (simple ingredient) or another medication of a medication.) 290 */ 291 public MedicationIngredientComponent setItem(Type value) { 292 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 293 throw new Error("Not the right type for Medication.ingredient.item[x]: " + value.fhirType()); 294 this.item = value; 295 return this; 296 } 297 298 /** 299 * @return {@link #isActive} (Indication of whether this ingredient affects the 300 * therapeutic action of the drug.). This is the underlying object with 301 * id, value and extensions. The accessor "getIsActive" gives direct 302 * access to the value 303 */ 304 public BooleanType getIsActiveElement() { 305 if (this.isActive == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create MedicationIngredientComponent.isActive"); 308 else if (Configuration.doAutoCreate()) 309 this.isActive = new BooleanType(); // bb 310 return this.isActive; 311 } 312 313 public boolean hasIsActiveElement() { 314 return this.isActive != null && !this.isActive.isEmpty(); 315 } 316 317 public boolean hasIsActive() { 318 return this.isActive != null && !this.isActive.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #isActive} (Indication of whether this ingredient affects 323 * the therapeutic action of the drug.). This is the underlying 324 * object with id, value and extensions. The accessor "getIsActive" 325 * gives direct access to the value 326 */ 327 public MedicationIngredientComponent setIsActiveElement(BooleanType value) { 328 this.isActive = value; 329 return this; 330 } 331 332 /** 333 * @return Indication of whether this ingredient affects the therapeutic action 334 * of the drug. 335 */ 336 public boolean getIsActive() { 337 return this.isActive == null || this.isActive.isEmpty() ? false : this.isActive.getValue(); 338 } 339 340 /** 341 * @param value Indication of whether this ingredient affects the therapeutic 342 * action of the drug. 343 */ 344 public MedicationIngredientComponent setIsActive(boolean value) { 345 if (this.isActive == null) 346 this.isActive = new BooleanType(); 347 this.isActive.setValue(value); 348 return this; 349 } 350 351 /** 352 * @return {@link #strength} (Specifies how many (or how much) of the items 353 * there are in this Medication. For example, 250 mg per tablet. This is 354 * expressed as a ratio where the numerator is 250mg and the denominator 355 * is 1 tablet.) 356 */ 357 public Ratio getStrength() { 358 if (this.strength == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create MedicationIngredientComponent.strength"); 361 else if (Configuration.doAutoCreate()) 362 this.strength = new Ratio(); // cc 363 return this.strength; 364 } 365 366 public boolean hasStrength() { 367 return this.strength != null && !this.strength.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #strength} (Specifies how many (or how much) of the items 372 * there are in this Medication. For example, 250 mg per tablet. 373 * This is expressed as a ratio where the numerator is 250mg and 374 * the denominator is 1 tablet.) 375 */ 376 public MedicationIngredientComponent setStrength(Ratio value) { 377 this.strength = value; 378 return this; 379 } 380 381 protected void listChildren(List<Property> children) { 382 super.listChildren(children); 383 children.add(new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 384 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 1, 385 item)); 386 children.add(new Property("isActive", "boolean", 387 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive)); 388 children.add(new Property("strength", "Ratio", 389 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 390 0, 1, strength)); 391 } 392 393 @Override 394 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 395 switch (_hash) { 396 case 2116201613: 397 /* item[x] */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 398 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 399 1, item); 400 case 3242771: 401 /* item */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 402 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 403 1, item); 404 case 106644494: 405 /* itemCodeableConcept */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 406 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 407 1, item); 408 case 1376364920: 409 /* itemReference */ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", 410 "The actual ingredient - either a substance (simple ingredient) or another medication of a medication.", 0, 411 1, item); 412 case -748916528: 413 /* isActive */ return new Property("isActive", "boolean", 414 "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive); 415 case 1791316033: 416 /* strength */ return new Property("strength", "Ratio", 417 "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 418 0, 1, strength); 419 default: 420 return super.getNamedProperty(_hash, _name, _checkValid); 421 } 422 423 } 424 425 @Override 426 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 427 switch (hash) { 428 case 3242771: 429 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 430 case -748916528: 431 /* isActive */ return this.isActive == null ? new Base[0] : new Base[] { this.isActive }; // BooleanType 432 case 1791316033: 433 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Ratio 434 default: 435 return super.getProperty(hash, name, checkValid); 436 } 437 438 } 439 440 @Override 441 public Base setProperty(int hash, String name, Base value) throws FHIRException { 442 switch (hash) { 443 case 3242771: // item 444 this.item = castToType(value); // Type 445 return value; 446 case -748916528: // isActive 447 this.isActive = castToBoolean(value); // BooleanType 448 return value; 449 case 1791316033: // strength 450 this.strength = castToRatio(value); // Ratio 451 return value; 452 default: 453 return super.setProperty(hash, name, value); 454 } 455 456 } 457 458 @Override 459 public Base setProperty(String name, Base value) throws FHIRException { 460 if (name.equals("item[x]")) { 461 this.item = castToType(value); // Type 462 } else if (name.equals("isActive")) { 463 this.isActive = castToBoolean(value); // BooleanType 464 } else if (name.equals("strength")) { 465 this.strength = castToRatio(value); // Ratio 466 } else 467 return super.setProperty(name, value); 468 return value; 469 } 470 471 @Override 472 public Base makeProperty(int hash, String name) throws FHIRException { 473 switch (hash) { 474 case 2116201613: 475 return getItem(); 476 case 3242771: 477 return getItem(); 478 case -748916528: 479 return getIsActiveElement(); 480 case 1791316033: 481 return getStrength(); 482 default: 483 return super.makeProperty(hash, name); 484 } 485 486 } 487 488 @Override 489 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 490 switch (hash) { 491 case 3242771: 492 /* item */ return new String[] { "CodeableConcept", "Reference" }; 493 case -748916528: 494 /* isActive */ return new String[] { "boolean" }; 495 case 1791316033: 496 /* strength */ return new String[] { "Ratio" }; 497 default: 498 return super.getTypesForProperty(hash, name); 499 } 500 501 } 502 503 @Override 504 public Base addChild(String name) throws FHIRException { 505 if (name.equals("itemCodeableConcept")) { 506 this.item = new CodeableConcept(); 507 return this.item; 508 } else if (name.equals("itemReference")) { 509 this.item = new Reference(); 510 return this.item; 511 } else if (name.equals("isActive")) { 512 throw new FHIRException("Cannot call addChild on a singleton property Medication.isActive"); 513 } else if (name.equals("strength")) { 514 this.strength = new Ratio(); 515 return this.strength; 516 } else 517 return super.addChild(name); 518 } 519 520 public MedicationIngredientComponent copy() { 521 MedicationIngredientComponent dst = new MedicationIngredientComponent(); 522 copyValues(dst); 523 return dst; 524 } 525 526 public void copyValues(MedicationIngredientComponent dst) { 527 super.copyValues(dst); 528 dst.item = item == null ? null : item.copy(); 529 dst.isActive = isActive == null ? null : isActive.copy(); 530 dst.strength = strength == null ? null : strength.copy(); 531 } 532 533 @Override 534 public boolean equalsDeep(Base other_) { 535 if (!super.equalsDeep(other_)) 536 return false; 537 if (!(other_ instanceof MedicationIngredientComponent)) 538 return false; 539 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 540 return compareDeep(item, o.item, true) && compareDeep(isActive, o.isActive, true) 541 && compareDeep(strength, o.strength, true); 542 } 543 544 @Override 545 public boolean equalsShallow(Base other_) { 546 if (!super.equalsShallow(other_)) 547 return false; 548 if (!(other_ instanceof MedicationIngredientComponent)) 549 return false; 550 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 551 return compareValues(isActive, o.isActive, true); 552 } 553 554 public boolean isEmpty() { 555 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, isActive, strength); 556 } 557 558 public String fhirType() { 559 return "Medication.ingredient"; 560 561 } 562 563 } 564 565 @Block() 566 public static class MedicationBatchComponent extends BackboneElement implements IBaseBackboneElement { 567 /** 568 * The assigned lot number of a batch of the specified product. 569 */ 570 @Child(name = "lotNumber", type = { 571 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 572 @Description(shortDefinition = "Identifier assigned to batch", formalDefinition = "The assigned lot number of a batch of the specified product.") 573 protected StringType lotNumber; 574 575 /** 576 * When this specific batch of product will expire. 577 */ 578 @Child(name = "expirationDate", type = { 579 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 580 @Description(shortDefinition = "When batch will expire", formalDefinition = "When this specific batch of product will expire.") 581 protected DateTimeType expirationDate; 582 583 private static final long serialVersionUID = 1982738755L; 584 585 /** 586 * Constructor 587 */ 588 public MedicationBatchComponent() { 589 super(); 590 } 591 592 /** 593 * @return {@link #lotNumber} (The assigned lot number of a batch of the 594 * specified product.). This is the underlying object with id, value and 595 * extensions. The accessor "getLotNumber" gives direct access to the 596 * value 597 */ 598 public StringType getLotNumberElement() { 599 if (this.lotNumber == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create MedicationBatchComponent.lotNumber"); 602 else if (Configuration.doAutoCreate()) 603 this.lotNumber = new StringType(); // bb 604 return this.lotNumber; 605 } 606 607 public boolean hasLotNumberElement() { 608 return this.lotNumber != null && !this.lotNumber.isEmpty(); 609 } 610 611 public boolean hasLotNumber() { 612 return this.lotNumber != null && !this.lotNumber.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #lotNumber} (The assigned lot number of a batch of the 617 * specified product.). This is the underlying object with id, 618 * value and extensions. The accessor "getLotNumber" gives direct 619 * access to the value 620 */ 621 public MedicationBatchComponent setLotNumberElement(StringType value) { 622 this.lotNumber = value; 623 return this; 624 } 625 626 /** 627 * @return The assigned lot number of a batch of the specified product. 628 */ 629 public String getLotNumber() { 630 return this.lotNumber == null ? null : this.lotNumber.getValue(); 631 } 632 633 /** 634 * @param value The assigned lot number of a batch of the specified product. 635 */ 636 public MedicationBatchComponent setLotNumber(String value) { 637 if (Utilities.noString(value)) 638 this.lotNumber = null; 639 else { 640 if (this.lotNumber == null) 641 this.lotNumber = new StringType(); 642 this.lotNumber.setValue(value); 643 } 644 return this; 645 } 646 647 /** 648 * @return {@link #expirationDate} (When this specific batch of product will 649 * expire.). This is the underlying object with id, value and 650 * extensions. The accessor "getExpirationDate" gives direct access to 651 * the value 652 */ 653 public DateTimeType getExpirationDateElement() { 654 if (this.expirationDate == null) 655 if (Configuration.errorOnAutoCreate()) 656 throw new Error("Attempt to auto-create MedicationBatchComponent.expirationDate"); 657 else if (Configuration.doAutoCreate()) 658 this.expirationDate = new DateTimeType(); // bb 659 return this.expirationDate; 660 } 661 662 public boolean hasExpirationDateElement() { 663 return this.expirationDate != null && !this.expirationDate.isEmpty(); 664 } 665 666 public boolean hasExpirationDate() { 667 return this.expirationDate != null && !this.expirationDate.isEmpty(); 668 } 669 670 /** 671 * @param value {@link #expirationDate} (When this specific batch of product 672 * will expire.). This is the underlying object with id, value and 673 * extensions. The accessor "getExpirationDate" gives direct access 674 * to the value 675 */ 676 public MedicationBatchComponent setExpirationDateElement(DateTimeType value) { 677 this.expirationDate = value; 678 return this; 679 } 680 681 /** 682 * @return When this specific batch of product will expire. 683 */ 684 public Date getExpirationDate() { 685 return this.expirationDate == null ? null : this.expirationDate.getValue(); 686 } 687 688 /** 689 * @param value When this specific batch of product will expire. 690 */ 691 public MedicationBatchComponent setExpirationDate(Date value) { 692 if (value == null) 693 this.expirationDate = null; 694 else { 695 if (this.expirationDate == null) 696 this.expirationDate = new DateTimeType(); 697 this.expirationDate.setValue(value); 698 } 699 return this; 700 } 701 702 protected void listChildren(List<Property> children) { 703 super.listChildren(children); 704 children.add(new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 705 0, 1, lotNumber)); 706 children.add(new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, 707 expirationDate)); 708 } 709 710 @Override 711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 712 switch (_hash) { 713 case 462547450: 714 /* lotNumber */ return new Property("lotNumber", "string", 715 "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber); 716 case -668811523: 717 /* expirationDate */ return new Property("expirationDate", "dateTime", 718 "When this specific batch of product will expire.", 0, 1, expirationDate); 719 default: 720 return super.getNamedProperty(_hash, _name, _checkValid); 721 } 722 723 } 724 725 @Override 726 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 727 switch (hash) { 728 case 462547450: 729 /* lotNumber */ return this.lotNumber == null ? new Base[0] : new Base[] { this.lotNumber }; // StringType 730 case -668811523: 731 /* expirationDate */ return this.expirationDate == null ? new Base[0] : new Base[] { this.expirationDate }; // DateTimeType 732 default: 733 return super.getProperty(hash, name, checkValid); 734 } 735 736 } 737 738 @Override 739 public Base setProperty(int hash, String name, Base value) throws FHIRException { 740 switch (hash) { 741 case 462547450: // lotNumber 742 this.lotNumber = castToString(value); // StringType 743 return value; 744 case -668811523: // expirationDate 745 this.expirationDate = castToDateTime(value); // DateTimeType 746 return value; 747 default: 748 return super.setProperty(hash, name, value); 749 } 750 751 } 752 753 @Override 754 public Base setProperty(String name, Base value) throws FHIRException { 755 if (name.equals("lotNumber")) { 756 this.lotNumber = castToString(value); // StringType 757 } else if (name.equals("expirationDate")) { 758 this.expirationDate = castToDateTime(value); // DateTimeType 759 } else 760 return super.setProperty(name, value); 761 return value; 762 } 763 764 @Override 765 public Base makeProperty(int hash, String name) throws FHIRException { 766 switch (hash) { 767 case 462547450: 768 return getLotNumberElement(); 769 case -668811523: 770 return getExpirationDateElement(); 771 default: 772 return super.makeProperty(hash, name); 773 } 774 775 } 776 777 @Override 778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 779 switch (hash) { 780 case 462547450: 781 /* lotNumber */ return new String[] { "string" }; 782 case -668811523: 783 /* expirationDate */ return new String[] { "dateTime" }; 784 default: 785 return super.getTypesForProperty(hash, name); 786 } 787 788 } 789 790 @Override 791 public Base addChild(String name) throws FHIRException { 792 if (name.equals("lotNumber")) { 793 throw new FHIRException("Cannot call addChild on a singleton property Medication.lotNumber"); 794 } else if (name.equals("expirationDate")) { 795 throw new FHIRException("Cannot call addChild on a singleton property Medication.expirationDate"); 796 } else 797 return super.addChild(name); 798 } 799 800 public MedicationBatchComponent copy() { 801 MedicationBatchComponent dst = new MedicationBatchComponent(); 802 copyValues(dst); 803 return dst; 804 } 805 806 public void copyValues(MedicationBatchComponent dst) { 807 super.copyValues(dst); 808 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 809 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 810 } 811 812 @Override 813 public boolean equalsDeep(Base other_) { 814 if (!super.equalsDeep(other_)) 815 return false; 816 if (!(other_ instanceof MedicationBatchComponent)) 817 return false; 818 MedicationBatchComponent o = (MedicationBatchComponent) other_; 819 return compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true); 820 } 821 822 @Override 823 public boolean equalsShallow(Base other_) { 824 if (!super.equalsShallow(other_)) 825 return false; 826 if (!(other_ instanceof MedicationBatchComponent)) 827 return false; 828 MedicationBatchComponent o = (MedicationBatchComponent) other_; 829 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true); 830 } 831 832 public boolean isEmpty() { 833 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lotNumber, expirationDate); 834 } 835 836 public String fhirType() { 837 return "Medication.batch"; 838 839 } 840 841 } 842 843 /** 844 * Business identifier for this medication. 845 */ 846 @Child(name = "identifier", type = { 847 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 848 @Description(shortDefinition = "Business identifier for this medication", formalDefinition = "Business identifier for this medication.") 849 protected List<Identifier> identifier; 850 851 /** 852 * A code (or set of codes) that specify this medication, or a textual 853 * description if no code is available. Usage note: This could be a standard 854 * medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could 855 * also be a national or local formulary code, optionally with translations to 856 * other code systems. 857 */ 858 @Child(name = "code", type = { CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 859 @Description(shortDefinition = "Codes that identify this medication", formalDefinition = "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.") 860 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 861 protected CodeableConcept code; 862 863 /** 864 * A code to indicate if the medication is in active use. 865 */ 866 @Child(name = "status", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 867 @Description(shortDefinition = "active | inactive | entered-in-error", formalDefinition = "A code to indicate if the medication is in active use.") 868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-status") 869 protected Enumeration<MedicationStatus> status; 870 871 /** 872 * Describes the details of the manufacturer of the medication product. This is 873 * not intended to represent the distributor of a medication product. 874 */ 875 @Child(name = "manufacturer", type = { 876 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 877 @Description(shortDefinition = "Manufacturer of the item", formalDefinition = "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.") 878 protected Reference manufacturer; 879 880 /** 881 * The actual object that is the target of the reference (Describes the details 882 * of the manufacturer of the medication product. This is not intended to 883 * represent the distributor of a medication product.) 884 */ 885 protected Organization manufacturerTarget; 886 887 /** 888 * Describes the form of the item. Powder; tablets; capsule. 889 */ 890 @Child(name = "form", type = { 891 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 892 @Description(shortDefinition = "powder | tablets | capsule +", formalDefinition = "Describes the form of the item. Powder; tablets; capsule.") 893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-form-codes") 894 protected CodeableConcept form; 895 896 /** 897 * Specific amount of the drug in the packaged product. For example, when 898 * specifying a product that has the same strength (For example, Insulin 899 * glargine 100 unit per mL solution for injection), this attribute provides 900 * additional clarification of the package amount (For example, 3 mL, 10mL, 901 * etc.). 902 */ 903 @Child(name = "amount", type = { Ratio.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 904 @Description(shortDefinition = "Amount of drug in package", formalDefinition = "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).") 905 protected Ratio amount; 906 907 /** 908 * Identifies a particular constituent of interest in the product. 909 */ 910 @Child(name = "ingredient", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 911 @Description(shortDefinition = "Active or inactive ingredient", formalDefinition = "Identifies a particular constituent of interest in the product.") 912 protected List<MedicationIngredientComponent> ingredient; 913 914 /** 915 * Information that only applies to packages (not products). 916 */ 917 @Child(name = "batch", type = {}, order = 7, min = 0, max = 1, modifier = false, summary = false) 918 @Description(shortDefinition = "Details about packaged medications", formalDefinition = "Information that only applies to packages (not products).") 919 protected MedicationBatchComponent batch; 920 921 private static final long serialVersionUID = 781229373L; 922 923 /** 924 * Constructor 925 */ 926 public Medication() { 927 super(); 928 } 929 930 /** 931 * @return {@link #identifier} (Business identifier for this medication.) 932 */ 933 public List<Identifier> getIdentifier() { 934 if (this.identifier == null) 935 this.identifier = new ArrayList<Identifier>(); 936 return this.identifier; 937 } 938 939 /** 940 * @return Returns a reference to <code>this</code> for easy method chaining 941 */ 942 public Medication setIdentifier(List<Identifier> theIdentifier) { 943 this.identifier = theIdentifier; 944 return this; 945 } 946 947 public boolean hasIdentifier() { 948 if (this.identifier == null) 949 return false; 950 for (Identifier item : this.identifier) 951 if (!item.isEmpty()) 952 return true; 953 return false; 954 } 955 956 public Identifier addIdentifier() { // 3 957 Identifier t = new Identifier(); 958 if (this.identifier == null) 959 this.identifier = new ArrayList<Identifier>(); 960 this.identifier.add(t); 961 return t; 962 } 963 964 public Medication addIdentifier(Identifier t) { // 3 965 if (t == null) 966 return this; 967 if (this.identifier == null) 968 this.identifier = new ArrayList<Identifier>(); 969 this.identifier.add(t); 970 return this; 971 } 972 973 /** 974 * @return The first repetition of repeating field {@link #identifier}, creating 975 * it if it does not already exist 976 */ 977 public Identifier getIdentifierFirstRep() { 978 if (getIdentifier().isEmpty()) { 979 addIdentifier(); 980 } 981 return getIdentifier().get(0); 982 } 983 984 /** 985 * @return {@link #code} (A code (or set of codes) that specify this medication, 986 * or a textual description if no code is available. Usage note: This 987 * could be a standard medication code such as a code from RxNorm, 988 * SNOMED CT, IDMP etc. It could also be a national or local formulary 989 * code, optionally with translations to other code systems.) 990 */ 991 public CodeableConcept getCode() { 992 if (this.code == null) 993 if (Configuration.errorOnAutoCreate()) 994 throw new Error("Attempt to auto-create Medication.code"); 995 else if (Configuration.doAutoCreate()) 996 this.code = new CodeableConcept(); // cc 997 return this.code; 998 } 999 1000 public boolean hasCode() { 1001 return this.code != null && !this.code.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #code} (A code (or set of codes) that specify this 1006 * medication, or a textual description if no code is available. 1007 * Usage note: This could be a standard medication code such as a 1008 * code from RxNorm, SNOMED CT, IDMP etc. It could also be a 1009 * national or local formulary code, optionally with translations 1010 * to other code systems.) 1011 */ 1012 public Medication setCode(CodeableConcept value) { 1013 this.code = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #status} (A code to indicate if the medication is in active 1019 * use.). This is the underlying object with id, value and extensions. 1020 * The accessor "getStatus" gives direct access to the value 1021 */ 1022 public Enumeration<MedicationStatus> getStatusElement() { 1023 if (this.status == null) 1024 if (Configuration.errorOnAutoCreate()) 1025 throw new Error("Attempt to auto-create Medication.status"); 1026 else if (Configuration.doAutoCreate()) 1027 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); // bb 1028 return this.status; 1029 } 1030 1031 public boolean hasStatusElement() { 1032 return this.status != null && !this.status.isEmpty(); 1033 } 1034 1035 public boolean hasStatus() { 1036 return this.status != null && !this.status.isEmpty(); 1037 } 1038 1039 /** 1040 * @param value {@link #status} (A code to indicate if the medication is in 1041 * active use.). This is the underlying object with id, value and 1042 * extensions. The accessor "getStatus" gives direct access to the 1043 * value 1044 */ 1045 public Medication setStatusElement(Enumeration<MedicationStatus> value) { 1046 this.status = value; 1047 return this; 1048 } 1049 1050 /** 1051 * @return A code to indicate if the medication is in active use. 1052 */ 1053 public MedicationStatus getStatus() { 1054 return this.status == null ? null : this.status.getValue(); 1055 } 1056 1057 /** 1058 * @param value A code to indicate if the medication is in active use. 1059 */ 1060 public Medication setStatus(MedicationStatus value) { 1061 if (value == null) 1062 this.status = null; 1063 else { 1064 if (this.status == null) 1065 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); 1066 this.status.setValue(value); 1067 } 1068 return this; 1069 } 1070 1071 /** 1072 * @return {@link #manufacturer} (Describes the details of the manufacturer of 1073 * the medication product. This is not intended to represent the 1074 * distributor of a medication product.) 1075 */ 1076 public Reference getManufacturer() { 1077 if (this.manufacturer == null) 1078 if (Configuration.errorOnAutoCreate()) 1079 throw new Error("Attempt to auto-create Medication.manufacturer"); 1080 else if (Configuration.doAutoCreate()) 1081 this.manufacturer = new Reference(); // cc 1082 return this.manufacturer; 1083 } 1084 1085 public boolean hasManufacturer() { 1086 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1087 } 1088 1089 /** 1090 * @param value {@link #manufacturer} (Describes the details of the manufacturer 1091 * of the medication product. This is not intended to represent the 1092 * distributor of a medication product.) 1093 */ 1094 public Medication setManufacturer(Reference value) { 1095 this.manufacturer = value; 1096 return this; 1097 } 1098 1099 /** 1100 * @return {@link #manufacturer} The actual object that is the target of the 1101 * reference. The reference library doesn't populate this, but you can 1102 * use it to hold the resource if you resolve it. (Describes the details 1103 * of the manufacturer of the medication product. This is not intended 1104 * to represent the distributor of a medication product.) 1105 */ 1106 public Organization getManufacturerTarget() { 1107 if (this.manufacturerTarget == null) 1108 if (Configuration.errorOnAutoCreate()) 1109 throw new Error("Attempt to auto-create Medication.manufacturer"); 1110 else if (Configuration.doAutoCreate()) 1111 this.manufacturerTarget = new Organization(); // aa 1112 return this.manufacturerTarget; 1113 } 1114 1115 /** 1116 * @param value {@link #manufacturer} The actual object that is the target of 1117 * the reference. The reference library doesn't use these, but you 1118 * can use it to hold the resource if you resolve it. (Describes 1119 * the details of the manufacturer of the medication product. This 1120 * is not intended to represent the distributor of a medication 1121 * product.) 1122 */ 1123 public Medication setManufacturerTarget(Organization value) { 1124 this.manufacturerTarget = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #form} (Describes the form of the item. Powder; tablets; 1130 * capsule.) 1131 */ 1132 public CodeableConcept getForm() { 1133 if (this.form == null) 1134 if (Configuration.errorOnAutoCreate()) 1135 throw new Error("Attempt to auto-create Medication.form"); 1136 else if (Configuration.doAutoCreate()) 1137 this.form = new CodeableConcept(); // cc 1138 return this.form; 1139 } 1140 1141 public boolean hasForm() { 1142 return this.form != null && !this.form.isEmpty(); 1143 } 1144 1145 /** 1146 * @param value {@link #form} (Describes the form of the item. Powder; tablets; 1147 * capsule.) 1148 */ 1149 public Medication setForm(CodeableConcept value) { 1150 this.form = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return {@link #amount} (Specific amount of the drug in the packaged product. 1156 * For example, when specifying a product that has the same strength 1157 * (For example, Insulin glargine 100 unit per mL solution for 1158 * injection), this attribute provides additional clarification of the 1159 * package amount (For example, 3 mL, 10mL, etc.).) 1160 */ 1161 public Ratio getAmount() { 1162 if (this.amount == null) 1163 if (Configuration.errorOnAutoCreate()) 1164 throw new Error("Attempt to auto-create Medication.amount"); 1165 else if (Configuration.doAutoCreate()) 1166 this.amount = new Ratio(); // cc 1167 return this.amount; 1168 } 1169 1170 public boolean hasAmount() { 1171 return this.amount != null && !this.amount.isEmpty(); 1172 } 1173 1174 /** 1175 * @param value {@link #amount} (Specific amount of the drug in the packaged 1176 * product. For example, when specifying a product that has the 1177 * same strength (For example, Insulin glargine 100 unit per mL 1178 * solution for injection), this attribute provides additional 1179 * clarification of the package amount (For example, 3 mL, 10mL, 1180 * etc.).) 1181 */ 1182 public Medication setAmount(Ratio value) { 1183 this.amount = value; 1184 return this; 1185 } 1186 1187 /** 1188 * @return {@link #ingredient} (Identifies a particular constituent of interest 1189 * in the product.) 1190 */ 1191 public List<MedicationIngredientComponent> getIngredient() { 1192 if (this.ingredient == null) 1193 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1194 return this.ingredient; 1195 } 1196 1197 /** 1198 * @return Returns a reference to <code>this</code> for easy method chaining 1199 */ 1200 public Medication setIngredient(List<MedicationIngredientComponent> theIngredient) { 1201 this.ingredient = theIngredient; 1202 return this; 1203 } 1204 1205 public boolean hasIngredient() { 1206 if (this.ingredient == null) 1207 return false; 1208 for (MedicationIngredientComponent item : this.ingredient) 1209 if (!item.isEmpty()) 1210 return true; 1211 return false; 1212 } 1213 1214 public MedicationIngredientComponent addIngredient() { // 3 1215 MedicationIngredientComponent t = new MedicationIngredientComponent(); 1216 if (this.ingredient == null) 1217 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1218 this.ingredient.add(t); 1219 return t; 1220 } 1221 1222 public Medication addIngredient(MedicationIngredientComponent t) { // 3 1223 if (t == null) 1224 return this; 1225 if (this.ingredient == null) 1226 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1227 this.ingredient.add(t); 1228 return this; 1229 } 1230 1231 /** 1232 * @return The first repetition of repeating field {@link #ingredient}, creating 1233 * it if it does not already exist 1234 */ 1235 public MedicationIngredientComponent getIngredientFirstRep() { 1236 if (getIngredient().isEmpty()) { 1237 addIngredient(); 1238 } 1239 return getIngredient().get(0); 1240 } 1241 1242 /** 1243 * @return {@link #batch} (Information that only applies to packages (not 1244 * products).) 1245 */ 1246 public MedicationBatchComponent getBatch() { 1247 if (this.batch == null) 1248 if (Configuration.errorOnAutoCreate()) 1249 throw new Error("Attempt to auto-create Medication.batch"); 1250 else if (Configuration.doAutoCreate()) 1251 this.batch = new MedicationBatchComponent(); // cc 1252 return this.batch; 1253 } 1254 1255 public boolean hasBatch() { 1256 return this.batch != null && !this.batch.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #batch} (Information that only applies to packages (not 1261 * products).) 1262 */ 1263 public Medication setBatch(MedicationBatchComponent value) { 1264 this.batch = value; 1265 return this; 1266 } 1267 1268 protected void listChildren(List<Property> children) { 1269 super.listChildren(children); 1270 children.add(new Property("identifier", "Identifier", "Business identifier for this medication.", 0, 1271 java.lang.Integer.MAX_VALUE, identifier)); 1272 children.add(new Property("code", "CodeableConcept", 1273 "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 1274 0, 1, code)); 1275 children 1276 .add(new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status)); 1277 children.add(new Property("manufacturer", "Reference(Organization)", 1278 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 1279 0, 1, manufacturer)); 1280 children.add(new Property("form", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 1281 0, 1, form)); 1282 children.add(new Property("amount", "Ratio", 1283 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 1284 0, 1, amount)); 1285 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, 1286 java.lang.Integer.MAX_VALUE, ingredient)); 1287 children.add(new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, batch)); 1288 } 1289 1290 @Override 1291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1292 switch (_hash) { 1293 case -1618432855: 1294 /* identifier */ return new Property("identifier", "Identifier", "Business identifier for this medication.", 0, 1295 java.lang.Integer.MAX_VALUE, identifier); 1296 case 3059181: 1297 /* code */ return new Property("code", "CodeableConcept", 1298 "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 1299 0, 1, code); 1300 case -892481550: 1301 /* status */ return new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, 1302 status); 1303 case -1969347631: 1304 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 1305 "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 1306 0, 1, manufacturer); 1307 case 3148996: 1308 /* form */ return new Property("form", "CodeableConcept", 1309 "Describes the form of the item. Powder; tablets; capsule.", 0, 1, form); 1310 case -1413853096: 1311 /* amount */ return new Property("amount", "Ratio", 1312 "Specific amount of the drug in the packaged product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 1313 0, 1, amount); 1314 case -206409263: 1315 /* ingredient */ return new Property("ingredient", "", 1316 "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, 1317 ingredient); 1318 case 93509434: 1319 /* batch */ return new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, 1320 batch); 1321 default: 1322 return super.getNamedProperty(_hash, _name, _checkValid); 1323 } 1324 1325 } 1326 1327 @Override 1328 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1329 switch (hash) { 1330 case -1618432855: 1331 /* identifier */ return this.identifier == null ? new Base[0] 1332 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1333 case 3059181: 1334 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1335 case -892481550: 1336 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationStatus> 1337 case -1969347631: 1338 /* manufacturer */ return this.manufacturer == null ? new Base[0] : new Base[] { this.manufacturer }; // Reference 1339 case 3148996: 1340 /* form */ return this.form == null ? new Base[0] : new Base[] { this.form }; // CodeableConcept 1341 case -1413853096: 1342 /* amount */ return this.amount == null ? new Base[0] : new Base[] { this.amount }; // Ratio 1343 case -206409263: 1344 /* ingredient */ return this.ingredient == null ? new Base[0] 1345 : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationIngredientComponent 1346 case 93509434: 1347 /* batch */ return this.batch == null ? new Base[0] : new Base[] { this.batch }; // MedicationBatchComponent 1348 default: 1349 return super.getProperty(hash, name, checkValid); 1350 } 1351 1352 } 1353 1354 @Override 1355 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1356 switch (hash) { 1357 case -1618432855: // identifier 1358 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1359 return value; 1360 case 3059181: // code 1361 this.code = castToCodeableConcept(value); // CodeableConcept 1362 return value; 1363 case -892481550: // status 1364 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1365 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1366 return value; 1367 case -1969347631: // manufacturer 1368 this.manufacturer = castToReference(value); // Reference 1369 return value; 1370 case 3148996: // form 1371 this.form = castToCodeableConcept(value); // CodeableConcept 1372 return value; 1373 case -1413853096: // amount 1374 this.amount = castToRatio(value); // Ratio 1375 return value; 1376 case -206409263: // ingredient 1377 this.getIngredient().add((MedicationIngredientComponent) value); // MedicationIngredientComponent 1378 return value; 1379 case 93509434: // batch 1380 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1381 return value; 1382 default: 1383 return super.setProperty(hash, name, value); 1384 } 1385 1386 } 1387 1388 @Override 1389 public Base setProperty(String name, Base value) throws FHIRException { 1390 if (name.equals("identifier")) { 1391 this.getIdentifier().add(castToIdentifier(value)); 1392 } else if (name.equals("code")) { 1393 this.code = castToCodeableConcept(value); // CodeableConcept 1394 } else if (name.equals("status")) { 1395 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1396 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1397 } else if (name.equals("manufacturer")) { 1398 this.manufacturer = castToReference(value); // Reference 1399 } else if (name.equals("form")) { 1400 this.form = castToCodeableConcept(value); // CodeableConcept 1401 } else if (name.equals("amount")) { 1402 this.amount = castToRatio(value); // Ratio 1403 } else if (name.equals("ingredient")) { 1404 this.getIngredient().add((MedicationIngredientComponent) value); 1405 } else if (name.equals("batch")) { 1406 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1407 } else 1408 return super.setProperty(name, value); 1409 return value; 1410 } 1411 1412 @Override 1413 public Base makeProperty(int hash, String name) throws FHIRException { 1414 switch (hash) { 1415 case -1618432855: 1416 return addIdentifier(); 1417 case 3059181: 1418 return getCode(); 1419 case -892481550: 1420 return getStatusElement(); 1421 case -1969347631: 1422 return getManufacturer(); 1423 case 3148996: 1424 return getForm(); 1425 case -1413853096: 1426 return getAmount(); 1427 case -206409263: 1428 return addIngredient(); 1429 case 93509434: 1430 return getBatch(); 1431 default: 1432 return super.makeProperty(hash, name); 1433 } 1434 1435 } 1436 1437 @Override 1438 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1439 switch (hash) { 1440 case -1618432855: 1441 /* identifier */ return new String[] { "Identifier" }; 1442 case 3059181: 1443 /* code */ return new String[] { "CodeableConcept" }; 1444 case -892481550: 1445 /* status */ return new String[] { "code" }; 1446 case -1969347631: 1447 /* manufacturer */ return new String[] { "Reference" }; 1448 case 3148996: 1449 /* form */ return new String[] { "CodeableConcept" }; 1450 case -1413853096: 1451 /* amount */ return new String[] { "Ratio" }; 1452 case -206409263: 1453 /* ingredient */ return new String[] {}; 1454 case 93509434: 1455 /* batch */ return new String[] {}; 1456 default: 1457 return super.getTypesForProperty(hash, name); 1458 } 1459 1460 } 1461 1462 @Override 1463 public Base addChild(String name) throws FHIRException { 1464 if (name.equals("identifier")) { 1465 return addIdentifier(); 1466 } else if (name.equals("code")) { 1467 this.code = new CodeableConcept(); 1468 return this.code; 1469 } else if (name.equals("status")) { 1470 throw new FHIRException("Cannot call addChild on a singleton property Medication.status"); 1471 } else if (name.equals("manufacturer")) { 1472 this.manufacturer = new Reference(); 1473 return this.manufacturer; 1474 } else if (name.equals("form")) { 1475 this.form = new CodeableConcept(); 1476 return this.form; 1477 } else if (name.equals("amount")) { 1478 this.amount = new Ratio(); 1479 return this.amount; 1480 } else if (name.equals("ingredient")) { 1481 return addIngredient(); 1482 } else if (name.equals("batch")) { 1483 this.batch = new MedicationBatchComponent(); 1484 return this.batch; 1485 } else 1486 return super.addChild(name); 1487 } 1488 1489 public String fhirType() { 1490 return "Medication"; 1491 1492 } 1493 1494 public Medication copy() { 1495 Medication dst = new Medication(); 1496 copyValues(dst); 1497 return dst; 1498 } 1499 1500 public void copyValues(Medication dst) { 1501 super.copyValues(dst); 1502 if (identifier != null) { 1503 dst.identifier = new ArrayList<Identifier>(); 1504 for (Identifier i : identifier) 1505 dst.identifier.add(i.copy()); 1506 } 1507 ; 1508 dst.code = code == null ? null : code.copy(); 1509 dst.status = status == null ? null : status.copy(); 1510 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 1511 dst.form = form == null ? null : form.copy(); 1512 dst.amount = amount == null ? null : amount.copy(); 1513 if (ingredient != null) { 1514 dst.ingredient = new ArrayList<MedicationIngredientComponent>(); 1515 for (MedicationIngredientComponent i : ingredient) 1516 dst.ingredient.add(i.copy()); 1517 } 1518 ; 1519 dst.batch = batch == null ? null : batch.copy(); 1520 } 1521 1522 protected Medication typedCopy() { 1523 return copy(); 1524 } 1525 1526 @Override 1527 public boolean equalsDeep(Base other_) { 1528 if (!super.equalsDeep(other_)) 1529 return false; 1530 if (!(other_ instanceof Medication)) 1531 return false; 1532 Medication o = (Medication) other_; 1533 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 1534 && compareDeep(status, o.status, true) && compareDeep(manufacturer, o.manufacturer, true) 1535 && compareDeep(form, o.form, true) && compareDeep(amount, o.amount, true) 1536 && compareDeep(ingredient, o.ingredient, true) && compareDeep(batch, o.batch, true); 1537 } 1538 1539 @Override 1540 public boolean equalsShallow(Base other_) { 1541 if (!super.equalsShallow(other_)) 1542 return false; 1543 if (!(other_ instanceof Medication)) 1544 return false; 1545 Medication o = (Medication) other_; 1546 return compareValues(status, o.status, true); 1547 } 1548 1549 public boolean isEmpty() { 1550 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status, manufacturer, form, amount, 1551 ingredient, batch); 1552 } 1553 1554 @Override 1555 public ResourceType getResourceType() { 1556 return ResourceType.Medication; 1557 } 1558 1559 /** 1560 * Search parameter: <b>ingredient-code</b> 1561 * <p> 1562 * Description: <b>Returns medications for this ingredient code</b><br> 1563 * Type: <b>token</b><br> 1564 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1565 * </p> 1566 */ 1567 @SearchParamDefinition(name = "ingredient-code", path = "(Medication.ingredient.item as CodeableConcept)", description = "Returns medications for this ingredient code", type = "token") 1568 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 1569 /** 1570 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 1571 * <p> 1572 * Description: <b>Returns medications for this ingredient code</b><br> 1573 * Type: <b>token</b><br> 1574 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1575 * </p> 1576 */ 1577 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1578 SP_INGREDIENT_CODE); 1579 1580 /** 1581 * Search parameter: <b>identifier</b> 1582 * <p> 1583 * Description: <b>Returns medications with this external identifier</b><br> 1584 * Type: <b>token</b><br> 1585 * Path: <b>Medication.identifier</b><br> 1586 * </p> 1587 */ 1588 @SearchParamDefinition(name = "identifier", path = "Medication.identifier", description = "Returns medications with this external identifier", type = "token") 1589 public static final String SP_IDENTIFIER = "identifier"; 1590 /** 1591 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1592 * <p> 1593 * Description: <b>Returns medications with this external identifier</b><br> 1594 * Type: <b>token</b><br> 1595 * Path: <b>Medication.identifier</b><br> 1596 * </p> 1597 */ 1598 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1599 SP_IDENTIFIER); 1600 1601 /** 1602 * Search parameter: <b>code</b> 1603 * <p> 1604 * Description: <b>Returns medications for a specific code</b><br> 1605 * Type: <b>token</b><br> 1606 * Path: <b>Medication.code</b><br> 1607 * </p> 1608 */ 1609 @SearchParamDefinition(name = "code", path = "Medication.code", description = "Returns medications for a specific code", type = "token") 1610 public static final String SP_CODE = "code"; 1611 /** 1612 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1613 * <p> 1614 * Description: <b>Returns medications for a specific code</b><br> 1615 * Type: <b>token</b><br> 1616 * Path: <b>Medication.code</b><br> 1617 * </p> 1618 */ 1619 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1620 SP_CODE); 1621 1622 /** 1623 * Search parameter: <b>ingredient</b> 1624 * <p> 1625 * Description: <b>Returns medications for this ingredient reference</b><br> 1626 * Type: <b>reference</b><br> 1627 * Path: <b>Medication.ingredient.itemReference</b><br> 1628 * </p> 1629 */ 1630 @SearchParamDefinition(name = "ingredient", path = "(Medication.ingredient.item as Reference)", description = "Returns medications for this ingredient reference", type = "reference", target = { 1631 Medication.class, Substance.class }) 1632 public static final String SP_INGREDIENT = "ingredient"; 1633 /** 1634 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 1635 * <p> 1636 * Description: <b>Returns medications for this ingredient reference</b><br> 1637 * Type: <b>reference</b><br> 1638 * Path: <b>Medication.ingredient.itemReference</b><br> 1639 * </p> 1640 */ 1641 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1642 SP_INGREDIENT); 1643 1644 /** 1645 * Constant for fluent queries to be used to add include statements. Specifies 1646 * the path value of "<b>Medication:ingredient</b>". 1647 */ 1648 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include( 1649 "Medication:ingredient").toLocked(); 1650 1651 /** 1652 * Search parameter: <b>form</b> 1653 * <p> 1654 * Description: <b>Returns medications for a specific dose form</b><br> 1655 * Type: <b>token</b><br> 1656 * Path: <b>Medication.form</b><br> 1657 * </p> 1658 */ 1659 @SearchParamDefinition(name = "form", path = "Medication.form", description = "Returns medications for a specific dose form", type = "token") 1660 public static final String SP_FORM = "form"; 1661 /** 1662 * <b>Fluent Client</b> search parameter constant for <b>form</b> 1663 * <p> 1664 * Description: <b>Returns medications for a specific dose form</b><br> 1665 * Type: <b>token</b><br> 1666 * Path: <b>Medication.form</b><br> 1667 * </p> 1668 */ 1669 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1670 SP_FORM); 1671 1672 /** 1673 * Search parameter: <b>lot-number</b> 1674 * <p> 1675 * Description: <b>Returns medications in a batch with this lot number</b><br> 1676 * Type: <b>token</b><br> 1677 * Path: <b>Medication.batch.lotNumber</b><br> 1678 * </p> 1679 */ 1680 @SearchParamDefinition(name = "lot-number", path = "Medication.batch.lotNumber", description = "Returns medications in a batch with this lot number", type = "token") 1681 public static final String SP_LOT_NUMBER = "lot-number"; 1682 /** 1683 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 1684 * <p> 1685 * Description: <b>Returns medications in a batch with this lot number</b><br> 1686 * Type: <b>token</b><br> 1687 * Path: <b>Medication.batch.lotNumber</b><br> 1688 * </p> 1689 */ 1690 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1691 SP_LOT_NUMBER); 1692 1693 /** 1694 * Search parameter: <b>expiration-date</b> 1695 * <p> 1696 * Description: <b>Returns medications in a batch with this expiration 1697 * date</b><br> 1698 * Type: <b>date</b><br> 1699 * Path: <b>Medication.batch.expirationDate</b><br> 1700 * </p> 1701 */ 1702 @SearchParamDefinition(name = "expiration-date", path = "Medication.batch.expirationDate", description = "Returns medications in a batch with this expiration date", type = "date") 1703 public static final String SP_EXPIRATION_DATE = "expiration-date"; 1704 /** 1705 * <b>Fluent Client</b> search parameter constant for <b>expiration-date</b> 1706 * <p> 1707 * Description: <b>Returns medications in a batch with this expiration 1708 * date</b><br> 1709 * Type: <b>date</b><br> 1710 * Path: <b>Medication.batch.expirationDate</b><br> 1711 * </p> 1712 */ 1713 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRATION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 1714 SP_EXPIRATION_DATE); 1715 1716 /** 1717 * Search parameter: <b>manufacturer</b> 1718 * <p> 1719 * Description: <b>Returns medications made or sold for this 1720 * manufacturer</b><br> 1721 * Type: <b>reference</b><br> 1722 * Path: <b>Medication.manufacturer</b><br> 1723 * </p> 1724 */ 1725 @SearchParamDefinition(name = "manufacturer", path = "Medication.manufacturer", description = "Returns medications made or sold for this manufacturer", type = "reference", target = { 1726 Organization.class }) 1727 public static final String SP_MANUFACTURER = "manufacturer"; 1728 /** 1729 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 1730 * <p> 1731 * Description: <b>Returns medications made or sold for this 1732 * manufacturer</b><br> 1733 * Type: <b>reference</b><br> 1734 * Path: <b>Medication.manufacturer</b><br> 1735 * </p> 1736 */ 1737 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1738 SP_MANUFACTURER); 1739 1740 /** 1741 * Constant for fluent queries to be used to add include statements. Specifies 1742 * the path value of "<b>Medication:manufacturer</b>". 1743 */ 1744 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include( 1745 "Medication:manufacturer").toLocked(); 1746 1747 /** 1748 * Search parameter: <b>status</b> 1749 * <p> 1750 * Description: <b>Returns medications for this status</b><br> 1751 * Type: <b>token</b><br> 1752 * Path: <b>Medication.status</b><br> 1753 * </p> 1754 */ 1755 @SearchParamDefinition(name = "status", path = "Medication.status", description = "Returns medications for this status", type = "token") 1756 public static final String SP_STATUS = "status"; 1757 /** 1758 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1759 * <p> 1760 * Description: <b>Returns medications for this status</b><br> 1761 * Type: <b>token</b><br> 1762 * Path: <b>Medication.status</b><br> 1763 * </p> 1764 */ 1765 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1766 SP_STATUS); 1767 1768}