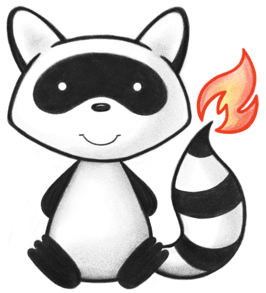
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * Describes the event of a patient consuming or otherwise being administered a 048 * medication. This may be as simple as swallowing a tablet or it may be a long 049 * running infusion. Related resources tie this event to the authorizing 050 * prescription, and the specific encounter between patient and health care 051 * practitioner. 052 */ 053@ResourceDef(name = "MedicationAdministration", profile = "http://hl7.org/fhir/StructureDefinition/MedicationAdministration") 054public class MedicationAdministration extends DomainResource { 055 056 public enum MedicationAdministrationStatus { 057 /** 058 * The administration has started but has not yet completed. 059 */ 060 INPROGRESS, 061 /** 062 * The administration was terminated prior to any impact on the subject (though 063 * preparatory actions may have been taken) 064 */ 065 NOTDONE, 066 /** 067 * Actions implied by the administration have been temporarily halted, but are 068 * expected to continue later. May also be called 'suspended'. 069 */ 070 ONHOLD, 071 /** 072 * All actions that are implied by the administration have occurred. 073 */ 074 COMPLETED, 075 /** 076 * The administration was entered in error and therefore nullified. 077 */ 078 ENTEREDINERROR, 079 /** 080 * Actions implied by the administration have been permanently halted, before 081 * all of them occurred. 082 */ 083 STOPPED, 084 /** 085 * The authoring system does not know which of the status values currently 086 * applies for this request. Note: This concept is not to be used for 'other' - 087 * one of the listed statuses is presumed to apply, it's just not known which 088 * one. 089 */ 090 UNKNOWN, 091 /** 092 * added to help the parsers with the generic types 093 */ 094 NULL; 095 096 public static MedicationAdministrationStatus fromCode(String codeString) throws FHIRException { 097 if (codeString == null || "".equals(codeString)) 098 return null; 099 if ("in-progress".equals(codeString)) 100 return INPROGRESS; 101 if ("not-done".equals(codeString)) 102 return NOTDONE; 103 if ("on-hold".equals(codeString)) 104 return ONHOLD; 105 if ("completed".equals(codeString)) 106 return COMPLETED; 107 if ("entered-in-error".equals(codeString)) 108 return ENTEREDINERROR; 109 if ("stopped".equals(codeString)) 110 return STOPPED; 111 if ("unknown".equals(codeString)) 112 return UNKNOWN; 113 if (Configuration.isAcceptInvalidEnums()) 114 return null; 115 else 116 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 117 } 118 119 public String toCode() { 120 switch (this) { 121 case INPROGRESS: 122 return "in-progress"; 123 case NOTDONE: 124 return "not-done"; 125 case ONHOLD: 126 return "on-hold"; 127 case COMPLETED: 128 return "completed"; 129 case ENTEREDINERROR: 130 return "entered-in-error"; 131 case STOPPED: 132 return "stopped"; 133 case UNKNOWN: 134 return "unknown"; 135 case NULL: 136 return null; 137 default: 138 return "?"; 139 } 140 } 141 142 public String getSystem() { 143 switch (this) { 144 case INPROGRESS: 145 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 146 case NOTDONE: 147 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 148 case ONHOLD: 149 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 150 case COMPLETED: 151 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 152 case ENTEREDINERROR: 153 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 154 case STOPPED: 155 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 156 case UNKNOWN: 157 return "http://terminology.hl7.org/CodeSystem/medication-admin-status"; 158 case NULL: 159 return null; 160 default: 161 return "?"; 162 } 163 } 164 165 public String getDefinition() { 166 switch (this) { 167 case INPROGRESS: 168 return "The administration has started but has not yet completed."; 169 case NOTDONE: 170 return "The administration was terminated prior to any impact on the subject (though preparatory actions may have been taken)"; 171 case ONHOLD: 172 return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called 'suspended'."; 173 case COMPLETED: 174 return "All actions that are implied by the administration have occurred."; 175 case ENTEREDINERROR: 176 return "The administration was entered in error and therefore nullified."; 177 case STOPPED: 178 return "Actions implied by the administration have been permanently halted, before all of them occurred."; 179 case UNKNOWN: 180 return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, it's just not known which one."; 181 case NULL: 182 return null; 183 default: 184 return "?"; 185 } 186 } 187 188 public String getDisplay() { 189 switch (this) { 190 case INPROGRESS: 191 return "In Progress"; 192 case NOTDONE: 193 return "Not Done"; 194 case ONHOLD: 195 return "On Hold"; 196 case COMPLETED: 197 return "Completed"; 198 case ENTEREDINERROR: 199 return "Entered in Error"; 200 case STOPPED: 201 return "Stopped"; 202 case UNKNOWN: 203 return "Unknown"; 204 case NULL: 205 return null; 206 default: 207 return "?"; 208 } 209 } 210 } 211 212 public static class MedicationAdministrationStatusEnumFactory implements EnumFactory<MedicationAdministrationStatus> { 213 public MedicationAdministrationStatus fromCode(String codeString) throws IllegalArgumentException { 214 if (codeString == null || "".equals(codeString)) 215 if (codeString == null || "".equals(codeString)) 216 return null; 217 if ("in-progress".equals(codeString)) 218 return MedicationAdministrationStatus.INPROGRESS; 219 if ("not-done".equals(codeString)) 220 return MedicationAdministrationStatus.NOTDONE; 221 if ("on-hold".equals(codeString)) 222 return MedicationAdministrationStatus.ONHOLD; 223 if ("completed".equals(codeString)) 224 return MedicationAdministrationStatus.COMPLETED; 225 if ("entered-in-error".equals(codeString)) 226 return MedicationAdministrationStatus.ENTEREDINERROR; 227 if ("stopped".equals(codeString)) 228 return MedicationAdministrationStatus.STOPPED; 229 if ("unknown".equals(codeString)) 230 return MedicationAdministrationStatus.UNKNOWN; 231 throw new IllegalArgumentException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 232 } 233 234 public Enumeration<MedicationAdministrationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 235 if (code == null) 236 return null; 237 if (code.isEmpty()) 238 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NULL, code); 239 String codeString = code.asStringValue(); 240 if (codeString == null || "".equals(codeString)) 241 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NULL, code); 242 if ("in-progress".equals(codeString)) 243 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.INPROGRESS, code); 244 if ("not-done".equals(codeString)) 245 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.NOTDONE, code); 246 if ("on-hold".equals(codeString)) 247 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ONHOLD, code); 248 if ("completed".equals(codeString)) 249 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.COMPLETED, code); 250 if ("entered-in-error".equals(codeString)) 251 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ENTEREDINERROR, 252 code); 253 if ("stopped".equals(codeString)) 254 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.STOPPED, code); 255 if ("unknown".equals(codeString)) 256 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.UNKNOWN, code); 257 throw new FHIRException("Unknown MedicationAdministrationStatus code '" + codeString + "'"); 258 } 259 260 public String toCode(MedicationAdministrationStatus code) { 261 if (code == MedicationAdministrationStatus.INPROGRESS) 262 return "in-progress"; 263 if (code == MedicationAdministrationStatus.NOTDONE) 264 return "not-done"; 265 if (code == MedicationAdministrationStatus.ONHOLD) 266 return "on-hold"; 267 if (code == MedicationAdministrationStatus.COMPLETED) 268 return "completed"; 269 if (code == MedicationAdministrationStatus.ENTEREDINERROR) 270 return "entered-in-error"; 271 if (code == MedicationAdministrationStatus.STOPPED) 272 return "stopped"; 273 if (code == MedicationAdministrationStatus.UNKNOWN) 274 return "unknown"; 275 return "?"; 276 } 277 278 public String toSystem(MedicationAdministrationStatus code) { 279 return code.getSystem(); 280 } 281 } 282 283 @Block() 284 public static class MedicationAdministrationPerformerComponent extends BackboneElement 285 implements IBaseBackboneElement { 286 /** 287 * Distinguishes the type of involvement of the performer in the medication 288 * administration. 289 */ 290 @Child(name = "function", type = { 291 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 292 @Description(shortDefinition = "Type of performance", formalDefinition = "Distinguishes the type of involvement of the performer in the medication administration.") 293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/med-admin-perform-function") 294 protected CodeableConcept function; 295 296 /** 297 * Indicates who or what performed the medication administration. 298 */ 299 @Child(name = "actor", type = { Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, 300 Device.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 301 @Description(shortDefinition = "Who performed the medication administration", formalDefinition = "Indicates who or what performed the medication administration.") 302 protected Reference actor; 303 304 /** 305 * The actual object that is the target of the reference (Indicates who or what 306 * performed the medication administration.) 307 */ 308 protected Resource actorTarget; 309 310 private static final long serialVersionUID = 1424001049L; 311 312 /** 313 * Constructor 314 */ 315 public MedicationAdministrationPerformerComponent() { 316 super(); 317 } 318 319 /** 320 * Constructor 321 */ 322 public MedicationAdministrationPerformerComponent(Reference actor) { 323 super(); 324 this.actor = actor; 325 } 326 327 /** 328 * @return {@link #function} (Distinguishes the type of involvement of the 329 * performer in the medication administration.) 330 */ 331 public CodeableConcept getFunction() { 332 if (this.function == null) 333 if (Configuration.errorOnAutoCreate()) 334 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.function"); 335 else if (Configuration.doAutoCreate()) 336 this.function = new CodeableConcept(); // cc 337 return this.function; 338 } 339 340 public boolean hasFunction() { 341 return this.function != null && !this.function.isEmpty(); 342 } 343 344 /** 345 * @param value {@link #function} (Distinguishes the type of involvement of the 346 * performer in the medication administration.) 347 */ 348 public MedicationAdministrationPerformerComponent setFunction(CodeableConcept value) { 349 this.function = value; 350 return this; 351 } 352 353 /** 354 * @return {@link #actor} (Indicates who or what performed the medication 355 * administration.) 356 */ 357 public Reference getActor() { 358 if (this.actor == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.actor"); 361 else if (Configuration.doAutoCreate()) 362 this.actor = new Reference(); // cc 363 return this.actor; 364 } 365 366 public boolean hasActor() { 367 return this.actor != null && !this.actor.isEmpty(); 368 } 369 370 /** 371 * @param value {@link #actor} (Indicates who or what performed the medication 372 * administration.) 373 */ 374 public MedicationAdministrationPerformerComponent setActor(Reference value) { 375 this.actor = value; 376 return this; 377 } 378 379 /** 380 * @return {@link #actor} The actual object that is the target of the reference. 381 * The reference library doesn't populate this, but you can use it to 382 * hold the resource if you resolve it. (Indicates who or what performed 383 * the medication administration.) 384 */ 385 public Resource getActorTarget() { 386 return this.actorTarget; 387 } 388 389 /** 390 * @param value {@link #actor} The actual object that is the target of the 391 * reference. The reference library doesn't use these, but you can 392 * use it to hold the resource if you resolve it. (Indicates who or 393 * what performed the medication administration.) 394 */ 395 public MedicationAdministrationPerformerComponent setActorTarget(Resource value) { 396 this.actorTarget = value; 397 return this; 398 } 399 400 protected void listChildren(List<Property> children) { 401 super.listChildren(children); 402 children.add(new Property("function", "CodeableConcept", 403 "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function)); 404 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", 405 "Indicates who or what performed the medication administration.", 0, 1, actor)); 406 } 407 408 @Override 409 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 410 switch (_hash) { 411 case 1380938712: 412 /* function */ return new Property("function", "CodeableConcept", 413 "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function); 414 case 92645877: 415 /* actor */ return new Property("actor", 416 "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", 417 "Indicates who or what performed the medication administration.", 0, 1, actor); 418 default: 419 return super.getNamedProperty(_hash, _name, _checkValid); 420 } 421 422 } 423 424 @Override 425 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 426 switch (hash) { 427 case 1380938712: 428 /* function */ return this.function == null ? new Base[0] : new Base[] { this.function }; // CodeableConcept 429 case 92645877: 430 /* actor */ return this.actor == null ? new Base[0] : new Base[] { this.actor }; // Reference 431 default: 432 return super.getProperty(hash, name, checkValid); 433 } 434 435 } 436 437 @Override 438 public Base setProperty(int hash, String name, Base value) throws FHIRException { 439 switch (hash) { 440 case 1380938712: // function 441 this.function = castToCodeableConcept(value); // CodeableConcept 442 return value; 443 case 92645877: // actor 444 this.actor = castToReference(value); // Reference 445 return value; 446 default: 447 return super.setProperty(hash, name, value); 448 } 449 450 } 451 452 @Override 453 public Base setProperty(String name, Base value) throws FHIRException { 454 if (name.equals("function")) { 455 this.function = castToCodeableConcept(value); // CodeableConcept 456 } else if (name.equals("actor")) { 457 this.actor = castToReference(value); // Reference 458 } else 459 return super.setProperty(name, value); 460 return value; 461 } 462 463 @Override 464 public Base makeProperty(int hash, String name) throws FHIRException { 465 switch (hash) { 466 case 1380938712: 467 return getFunction(); 468 case 92645877: 469 return getActor(); 470 default: 471 return super.makeProperty(hash, name); 472 } 473 474 } 475 476 @Override 477 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 478 switch (hash) { 479 case 1380938712: 480 /* function */ return new String[] { "CodeableConcept" }; 481 case 92645877: 482 /* actor */ return new String[] { "Reference" }; 483 default: 484 return super.getTypesForProperty(hash, name); 485 } 486 487 } 488 489 @Override 490 public Base addChild(String name) throws FHIRException { 491 if (name.equals("function")) { 492 this.function = new CodeableConcept(); 493 return this.function; 494 } else if (name.equals("actor")) { 495 this.actor = new Reference(); 496 return this.actor; 497 } else 498 return super.addChild(name); 499 } 500 501 public MedicationAdministrationPerformerComponent copy() { 502 MedicationAdministrationPerformerComponent dst = new MedicationAdministrationPerformerComponent(); 503 copyValues(dst); 504 return dst; 505 } 506 507 public void copyValues(MedicationAdministrationPerformerComponent dst) { 508 super.copyValues(dst); 509 dst.function = function == null ? null : function.copy(); 510 dst.actor = actor == null ? null : actor.copy(); 511 } 512 513 @Override 514 public boolean equalsDeep(Base other_) { 515 if (!super.equalsDeep(other_)) 516 return false; 517 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 518 return false; 519 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 520 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 521 } 522 523 @Override 524 public boolean equalsShallow(Base other_) { 525 if (!super.equalsShallow(other_)) 526 return false; 527 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 528 return false; 529 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 530 return true; 531 } 532 533 public boolean isEmpty() { 534 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 535 } 536 537 public String fhirType() { 538 return "MedicationAdministration.performer"; 539 540 } 541 542 } 543 544 @Block() 545 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 546 /** 547 * Free text dosage can be used for cases where the dosage administered is too 548 * complex to code. When coded dosage is present, the free text dosage may still 549 * be present for display to humans. 550 * 551 * The dosage instructions should reflect the dosage of the medication that was 552 * administered. 553 */ 554 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 555 @Description(shortDefinition = "Free text dosage instructions e.g. SIG", formalDefinition = "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.") 556 protected StringType text; 557 558 /** 559 * A coded specification of the anatomic site where the medication first entered 560 * the body. For example, "left arm". 561 */ 562 @Child(name = "site", type = { 563 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 564 @Description(shortDefinition = "Body site administered to", formalDefinition = "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".") 565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/approach-site-codes") 566 protected CodeableConcept site; 567 568 /** 569 * A code specifying the route or physiological path of administration of a 570 * therapeutic agent into or onto the patient. For example, topical, 571 * intravenous, etc. 572 */ 573 @Child(name = "route", type = { 574 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 575 @Description(shortDefinition = "Path of substance into body", formalDefinition = "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.") 576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/route-codes") 577 protected CodeableConcept route; 578 579 /** 580 * A coded value indicating the method by which the medication is intended to be 581 * or was introduced into or on the body. This attribute will most often NOT be 582 * populated. It is most commonly used for injections. For example, Slow Push, 583 * Deep IV. 584 */ 585 @Child(name = "method", type = { 586 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 587 @Description(shortDefinition = "How drug was administered", formalDefinition = "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.") 588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/administration-method-codes") 589 protected CodeableConcept method; 590 591 /** 592 * The amount of the medication given at one administration event. Use this 593 * value when the administration is essentially an instantaneous event such as a 594 * swallowing a tablet or giving an injection. 595 */ 596 @Child(name = "dose", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 597 @Description(shortDefinition = "Amount of medication per dose", formalDefinition = "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.") 598 protected Quantity dose; 599 600 /** 601 * Identifies the speed with which the medication was or will be introduced into 602 * the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 603 * 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 604 * 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours. 605 */ 606 @Child(name = "rate", type = { Ratio.class, 607 Quantity.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 608 @Description(shortDefinition = "Dose quantity per unit of time", formalDefinition = "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.") 609 protected Type rate; 610 611 private static final long serialVersionUID = 947835626L; 612 613 /** 614 * Constructor 615 */ 616 public MedicationAdministrationDosageComponent() { 617 super(); 618 } 619 620 /** 621 * @return {@link #text} (Free text dosage can be used for cases where the 622 * dosage administered is too complex to code. When coded dosage is 623 * present, the free text dosage may still be present for display to 624 * humans. 625 * 626 * The dosage instructions should reflect the dosage of the medication 627 * that was administered.). This is the underlying object with id, value 628 * and extensions. The accessor "getText" gives direct access to the 629 * value 630 */ 631 public StringType getTextElement() { 632 if (this.text == null) 633 if (Configuration.errorOnAutoCreate()) 634 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 635 else if (Configuration.doAutoCreate()) 636 this.text = new StringType(); // bb 637 return this.text; 638 } 639 640 public boolean hasTextElement() { 641 return this.text != null && !this.text.isEmpty(); 642 } 643 644 public boolean hasText() { 645 return this.text != null && !this.text.isEmpty(); 646 } 647 648 /** 649 * @param value {@link #text} (Free text dosage can be used for cases where the 650 * dosage administered is too complex to code. When coded dosage is 651 * present, the free text dosage may still be present for display 652 * to humans. 653 * 654 * The dosage instructions should reflect the dosage of the 655 * medication that was administered.). This is the underlying 656 * object with id, value and extensions. The accessor "getText" 657 * gives direct access to the value 658 */ 659 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 660 this.text = value; 661 return this; 662 } 663 664 /** 665 * @return Free text dosage can be used for cases where the dosage administered 666 * is too complex to code. When coded dosage is present, the free text 667 * dosage may still be present for display to humans. 668 * 669 * The dosage instructions should reflect the dosage of the medication 670 * that was administered. 671 */ 672 public String getText() { 673 return this.text == null ? null : this.text.getValue(); 674 } 675 676 /** 677 * @param value Free text dosage can be used for cases where the dosage 678 * administered is too complex to code. When coded dosage is 679 * present, the free text dosage may still be present for display 680 * to humans. 681 * 682 * The dosage instructions should reflect the dosage of the 683 * medication that was administered. 684 */ 685 public MedicationAdministrationDosageComponent setText(String value) { 686 if (Utilities.noString(value)) 687 this.text = null; 688 else { 689 if (this.text == null) 690 this.text = new StringType(); 691 this.text.setValue(value); 692 } 693 return this; 694 } 695 696 /** 697 * @return {@link #site} (A coded specification of the anatomic site where the 698 * medication first entered the body. For example, "left arm".) 699 */ 700 public CodeableConcept getSite() { 701 if (this.site == null) 702 if (Configuration.errorOnAutoCreate()) 703 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.site"); 704 else if (Configuration.doAutoCreate()) 705 this.site = new CodeableConcept(); // cc 706 return this.site; 707 } 708 709 public boolean hasSite() { 710 return this.site != null && !this.site.isEmpty(); 711 } 712 713 /** 714 * @param value {@link #site} (A coded specification of the anatomic site where 715 * the medication first entered the body. For example, "left arm".) 716 */ 717 public MedicationAdministrationDosageComponent setSite(CodeableConcept value) { 718 this.site = value; 719 return this; 720 } 721 722 /** 723 * @return {@link #route} (A code specifying the route or physiological path of 724 * administration of a therapeutic agent into or onto the patient. For 725 * example, topical, intravenous, etc.) 726 */ 727 public CodeableConcept getRoute() { 728 if (this.route == null) 729 if (Configuration.errorOnAutoCreate()) 730 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 731 else if (Configuration.doAutoCreate()) 732 this.route = new CodeableConcept(); // cc 733 return this.route; 734 } 735 736 public boolean hasRoute() { 737 return this.route != null && !this.route.isEmpty(); 738 } 739 740 /** 741 * @param value {@link #route} (A code specifying the route or physiological 742 * path of administration of a therapeutic agent into or onto the 743 * patient. For example, topical, intravenous, etc.) 744 */ 745 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 746 this.route = value; 747 return this; 748 } 749 750 /** 751 * @return {@link #method} (A coded value indicating the method by which the 752 * medication is intended to be or was introduced into or on the body. 753 * This attribute will most often NOT be populated. It is most commonly 754 * used for injections. For example, Slow Push, Deep IV.) 755 */ 756 public CodeableConcept getMethod() { 757 if (this.method == null) 758 if (Configuration.errorOnAutoCreate()) 759 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 760 else if (Configuration.doAutoCreate()) 761 this.method = new CodeableConcept(); // cc 762 return this.method; 763 } 764 765 public boolean hasMethod() { 766 return this.method != null && !this.method.isEmpty(); 767 } 768 769 /** 770 * @param value {@link #method} (A coded value indicating the method by which 771 * the medication is intended to be or was introduced into or on 772 * the body. This attribute will most often NOT be populated. It is 773 * most commonly used for injections. For example, Slow Push, Deep 774 * IV.) 775 */ 776 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 777 this.method = value; 778 return this; 779 } 780 781 /** 782 * @return {@link #dose} (The amount of the medication given at one 783 * administration event. Use this value when the administration is 784 * essentially an instantaneous event such as a swallowing a tablet or 785 * giving an injection.) 786 */ 787 public Quantity getDose() { 788 if (this.dose == null) 789 if (Configuration.errorOnAutoCreate()) 790 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.dose"); 791 else if (Configuration.doAutoCreate()) 792 this.dose = new Quantity(); // cc 793 return this.dose; 794 } 795 796 public boolean hasDose() { 797 return this.dose != null && !this.dose.isEmpty(); 798 } 799 800 /** 801 * @param value {@link #dose} (The amount of the medication given at one 802 * administration event. Use this value when the administration is 803 * essentially an instantaneous event such as a swallowing a tablet 804 * or giving an injection.) 805 */ 806 public MedicationAdministrationDosageComponent setDose(Quantity value) { 807 this.dose = value; 808 return this; 809 } 810 811 /** 812 * @return {@link #rate} (Identifies the speed with which the medication was or 813 * will be introduced into the patient. Typically, the rate for an 814 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 815 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 816 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 817 */ 818 public Type getRate() { 819 return this.rate; 820 } 821 822 /** 823 * @return {@link #rate} (Identifies the speed with which the medication was or 824 * will be introduced into the patient. Typically, the rate for an 825 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 826 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 827 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 828 */ 829 public Ratio getRateRatio() throws FHIRException { 830 if (this.rate == null) 831 this.rate = new Ratio(); 832 if (!(this.rate instanceof Ratio)) 833 throw new FHIRException( 834 "Type mismatch: the type Ratio was expected, but " + this.rate.getClass().getName() + " was encountered"); 835 return (Ratio) this.rate; 836 } 837 838 public boolean hasRateRatio() { 839 return this != null && this.rate instanceof Ratio; 840 } 841 842 /** 843 * @return {@link #rate} (Identifies the speed with which the medication was or 844 * will be introduced into the patient. Typically, the rate for an 845 * infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed 846 * as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 847 * 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 848 */ 849 public Quantity getRateQuantity() throws FHIRException { 850 if (this.rate == null) 851 this.rate = new Quantity(); 852 if (!(this.rate instanceof Quantity)) 853 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.rate.getClass().getName() 854 + " was encountered"); 855 return (Quantity) this.rate; 856 } 857 858 public boolean hasRateQuantity() { 859 return this != null && this.rate instanceof Quantity; 860 } 861 862 public boolean hasRate() { 863 return this.rate != null && !this.rate.isEmpty(); 864 } 865 866 /** 867 * @param value {@link #rate} (Identifies the speed with which the medication 868 * was or will be introduced into the patient. Typically, the rate 869 * for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be 870 * expressed as a rate per unit of time, e.g. 500 ml per 2 hours. 871 * Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 872 * hours.) 873 */ 874 public MedicationAdministrationDosageComponent setRate(Type value) { 875 if (value != null && !(value instanceof Ratio || value instanceof Quantity)) 876 throw new Error("Not the right type for MedicationAdministration.dosage.rate[x]: " + value.fhirType()); 877 this.rate = value; 878 return this; 879 } 880 881 protected void listChildren(List<Property> children) { 882 super.listChildren(children); 883 children.add(new Property("text", "string", 884 "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 885 0, 1, text)); 886 children.add(new Property("site", "CodeableConcept", 887 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 888 0, 1, site)); 889 children.add(new Property("route", "CodeableConcept", 890 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 891 0, 1, route)); 892 children.add(new Property("method", "CodeableConcept", 893 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 894 0, 1, method)); 895 children.add(new Property("dose", "SimpleQuantity", 896 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 897 0, 1, dose)); 898 children.add(new Property("rate[x]", "Ratio|SimpleQuantity", 899 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 900 0, 1, rate)); 901 } 902 903 @Override 904 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 905 switch (_hash) { 906 case 3556653: 907 /* text */ return new Property("text", "string", 908 "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 909 0, 1, text); 910 case 3530567: 911 /* site */ return new Property("site", "CodeableConcept", 912 "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 913 0, 1, site); 914 case 108704329: 915 /* route */ return new Property("route", "CodeableConcept", 916 "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 917 0, 1, route); 918 case -1077554975: 919 /* method */ return new Property("method", "CodeableConcept", 920 "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 921 0, 1, method); 922 case 3089437: 923 /* dose */ return new Property("dose", "SimpleQuantity", 924 "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 925 0, 1, dose); 926 case 983460768: 927 /* rate[x] */ return new Property("rate[x]", "Ratio|SimpleQuantity", 928 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 929 0, 1, rate); 930 case 3493088: 931 /* rate */ return new Property("rate[x]", "Ratio|SimpleQuantity", 932 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 933 0, 1, rate); 934 case 204021515: 935 /* rateRatio */ return new Property("rate[x]", "Ratio|SimpleQuantity", 936 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 937 0, 1, rate); 938 case -1085459061: 939 /* rateQuantity */ return new Property("rate[x]", "Ratio|SimpleQuantity", 940 "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 941 0, 1, rate); 942 default: 943 return super.getNamedProperty(_hash, _name, _checkValid); 944 } 945 946 } 947 948 @Override 949 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 950 switch (hash) { 951 case 3556653: 952 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 953 case 3530567: 954 /* site */ return this.site == null ? new Base[0] : new Base[] { this.site }; // CodeableConcept 955 case 108704329: 956 /* route */ return this.route == null ? new Base[0] : new Base[] { this.route }; // CodeableConcept 957 case -1077554975: 958 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 959 case 3089437: 960 /* dose */ return this.dose == null ? new Base[0] : new Base[] { this.dose }; // Quantity 961 case 3493088: 962 /* rate */ return this.rate == null ? new Base[0] : new Base[] { this.rate }; // Type 963 default: 964 return super.getProperty(hash, name, checkValid); 965 } 966 967 } 968 969 @Override 970 public Base setProperty(int hash, String name, Base value) throws FHIRException { 971 switch (hash) { 972 case 3556653: // text 973 this.text = castToString(value); // StringType 974 return value; 975 case 3530567: // site 976 this.site = castToCodeableConcept(value); // CodeableConcept 977 return value; 978 case 108704329: // route 979 this.route = castToCodeableConcept(value); // CodeableConcept 980 return value; 981 case -1077554975: // method 982 this.method = castToCodeableConcept(value); // CodeableConcept 983 return value; 984 case 3089437: // dose 985 this.dose = castToQuantity(value); // Quantity 986 return value; 987 case 3493088: // rate 988 this.rate = castToType(value); // Type 989 return value; 990 default: 991 return super.setProperty(hash, name, value); 992 } 993 994 } 995 996 @Override 997 public Base setProperty(String name, Base value) throws FHIRException { 998 if (name.equals("text")) { 999 this.text = castToString(value); // StringType 1000 } else if (name.equals("site")) { 1001 this.site = castToCodeableConcept(value); // CodeableConcept 1002 } else if (name.equals("route")) { 1003 this.route = castToCodeableConcept(value); // CodeableConcept 1004 } else if (name.equals("method")) { 1005 this.method = castToCodeableConcept(value); // CodeableConcept 1006 } else if (name.equals("dose")) { 1007 this.dose = castToQuantity(value); // Quantity 1008 } else if (name.equals("rate[x]")) { 1009 this.rate = castToType(value); // Type 1010 } else 1011 return super.setProperty(name, value); 1012 return value; 1013 } 1014 1015 @Override 1016 public Base makeProperty(int hash, String name) throws FHIRException { 1017 switch (hash) { 1018 case 3556653: 1019 return getTextElement(); 1020 case 3530567: 1021 return getSite(); 1022 case 108704329: 1023 return getRoute(); 1024 case -1077554975: 1025 return getMethod(); 1026 case 3089437: 1027 return getDose(); 1028 case 983460768: 1029 return getRate(); 1030 case 3493088: 1031 return getRate(); 1032 default: 1033 return super.makeProperty(hash, name); 1034 } 1035 1036 } 1037 1038 @Override 1039 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1040 switch (hash) { 1041 case 3556653: 1042 /* text */ return new String[] { "string" }; 1043 case 3530567: 1044 /* site */ return new String[] { "CodeableConcept" }; 1045 case 108704329: 1046 /* route */ return new String[] { "CodeableConcept" }; 1047 case -1077554975: 1048 /* method */ return new String[] { "CodeableConcept" }; 1049 case 3089437: 1050 /* dose */ return new String[] { "SimpleQuantity" }; 1051 case 3493088: 1052 /* rate */ return new String[] { "Ratio", "SimpleQuantity" }; 1053 default: 1054 return super.getTypesForProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public Base addChild(String name) throws FHIRException { 1061 if (name.equals("text")) { 1062 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.text"); 1063 } else if (name.equals("site")) { 1064 this.site = new CodeableConcept(); 1065 return this.site; 1066 } else if (name.equals("route")) { 1067 this.route = new CodeableConcept(); 1068 return this.route; 1069 } else if (name.equals("method")) { 1070 this.method = new CodeableConcept(); 1071 return this.method; 1072 } else if (name.equals("dose")) { 1073 this.dose = new Quantity(); 1074 return this.dose; 1075 } else if (name.equals("rateRatio")) { 1076 this.rate = new Ratio(); 1077 return this.rate; 1078 } else if (name.equals("rateQuantity")) { 1079 this.rate = new Quantity(); 1080 return this.rate; 1081 } else 1082 return super.addChild(name); 1083 } 1084 1085 public MedicationAdministrationDosageComponent copy() { 1086 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 1087 copyValues(dst); 1088 return dst; 1089 } 1090 1091 public void copyValues(MedicationAdministrationDosageComponent dst) { 1092 super.copyValues(dst); 1093 dst.text = text == null ? null : text.copy(); 1094 dst.site = site == null ? null : site.copy(); 1095 dst.route = route == null ? null : route.copy(); 1096 dst.method = method == null ? null : method.copy(); 1097 dst.dose = dose == null ? null : dose.copy(); 1098 dst.rate = rate == null ? null : rate.copy(); 1099 } 1100 1101 @Override 1102 public boolean equalsDeep(Base other_) { 1103 if (!super.equalsDeep(other_)) 1104 return false; 1105 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 1106 return false; 1107 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 1108 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 1109 && compareDeep(method, o.method, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true); 1110 } 1111 1112 @Override 1113 public boolean equalsShallow(Base other_) { 1114 if (!super.equalsShallow(other_)) 1115 return false; 1116 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 1117 return false; 1118 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 1119 return compareValues(text, o.text, true); 1120 } 1121 1122 public boolean isEmpty() { 1123 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, site, route, method, dose, rate); 1124 } 1125 1126 public String fhirType() { 1127 return "MedicationAdministration.dosage"; 1128 1129 } 1130 1131 } 1132 1133 /** 1134 * Identifiers associated with this Medication Administration that are defined 1135 * by business processes and/or used to refer to it when a direct URL reference 1136 * to the resource itself is not appropriate. They are business identifiers 1137 * assigned to this resource by the performer or other systems and remain 1138 * constant as the resource is updated and propagates from server to server. 1139 */ 1140 @Child(name = "identifier", type = { 1141 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1142 @Description(shortDefinition = "External identifier", formalDefinition = "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.") 1143 protected List<Identifier> identifier; 1144 1145 /** 1146 * A protocol, guideline, orderset, or other definition that was adhered to in 1147 * whole or in part by this event. 1148 */ 1149 @Child(name = "instantiates", type = { 1150 UriType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1151 @Description(shortDefinition = "Instantiates protocol or definition", formalDefinition = "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.") 1152 protected List<UriType> instantiates; 1153 1154 /** 1155 * A larger event of which this particular event is a component or step. 1156 */ 1157 @Child(name = "partOf", type = { MedicationAdministration.class, 1158 Procedure.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1159 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular event is a component or step.") 1160 protected List<Reference> partOf; 1161 /** 1162 * The actual objects that are the target of the reference (A larger event of 1163 * which this particular event is a component or step.) 1164 */ 1165 protected List<Resource> partOfTarget; 1166 1167 /** 1168 * Will generally be set to show that the administration has been completed. For 1169 * some long running administrations such as infusions, it is possible for an 1170 * administration to be started but not completed or it may be paused while some 1171 * other process is under way. 1172 */ 1173 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 1174 @Description(shortDefinition = "in-progress | not-done | on-hold | completed | entered-in-error | stopped | unknown", formalDefinition = "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.") 1175 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-admin-status") 1176 protected Enumeration<MedicationAdministrationStatus> status; 1177 1178 /** 1179 * A code indicating why the administration was not performed. 1180 */ 1181 @Child(name = "statusReason", type = { 1182 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1183 @Description(shortDefinition = "Reason administration not performed", formalDefinition = "A code indicating why the administration was not performed.") 1184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reason-medication-not-given-codes") 1185 protected List<CodeableConcept> statusReason; 1186 1187 /** 1188 * Indicates where the medication is expected to be consumed or administered. 1189 */ 1190 @Child(name = "category", type = { 1191 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1192 @Description(shortDefinition = "Type of medication usage", formalDefinition = "Indicates where the medication is expected to be consumed or administered.") 1193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-admin-category") 1194 protected CodeableConcept category; 1195 1196 /** 1197 * Identifies the medication that was administered. This is either a link to a 1198 * resource representing the details of the medication or a simple attribute 1199 * carrying a code that identifies the medication from a known list of 1200 * medications. 1201 */ 1202 @Child(name = "medication", type = { CodeableConcept.class, 1203 Medication.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1204 @Description(shortDefinition = "What was administered", formalDefinition = "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.") 1205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1206 protected Type medication; 1207 1208 /** 1209 * The person or animal or group receiving the medication. 1210 */ 1211 @Child(name = "subject", type = { Patient.class, 1212 Group.class }, order = 7, min = 1, max = 1, modifier = false, summary = true) 1213 @Description(shortDefinition = "Who received medication", formalDefinition = "The person or animal or group receiving the medication.") 1214 protected Reference subject; 1215 1216 /** 1217 * The actual object that is the target of the reference (The person or animal 1218 * or group receiving the medication.) 1219 */ 1220 protected Resource subjectTarget; 1221 1222 /** 1223 * The visit, admission, or other contact between patient and health care 1224 * provider during which the medication administration was performed. 1225 */ 1226 @Child(name = "context", type = { Encounter.class, 1227 EpisodeOfCare.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1228 @Description(shortDefinition = "Encounter or Episode of Care administered as part of", formalDefinition = "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.") 1229 protected Reference context; 1230 1231 /** 1232 * The actual object that is the target of the reference (The visit, admission, 1233 * or other contact between patient and health care provider during which the 1234 * medication administration was performed.) 1235 */ 1236 protected Resource contextTarget; 1237 1238 /** 1239 * Additional information (for example, patient height and weight) that supports 1240 * the administration of the medication. 1241 */ 1242 @Child(name = "supportingInformation", type = { 1243 Reference.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1244 @Description(shortDefinition = "Additional information to support administration", formalDefinition = "Additional information (for example, patient height and weight) that supports the administration of the medication.") 1245 protected List<Reference> supportingInformation; 1246 /** 1247 * The actual objects that are the target of the reference (Additional 1248 * information (for example, patient height and weight) that supports the 1249 * administration of the medication.) 1250 */ 1251 protected List<Resource> supportingInformationTarget; 1252 1253 /** 1254 * A specific date/time or interval of time during which the administration took 1255 * place (or did not take place, when the 'notGiven' attribute is true). For 1256 * many administrations, such as swallowing a tablet the use of dateTime is more 1257 * appropriate. 1258 */ 1259 @Child(name = "effective", type = { DateTimeType.class, 1260 Period.class }, order = 10, min = 1, max = 1, modifier = false, summary = true) 1261 @Description(shortDefinition = "Start and end time of administration", formalDefinition = "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.") 1262 protected Type effective; 1263 1264 /** 1265 * Indicates who or what performed the medication administration and how they 1266 * were involved. 1267 */ 1268 @Child(name = "performer", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1269 @Description(shortDefinition = "Who performed the medication administration and what they did", formalDefinition = "Indicates who or what performed the medication administration and how they were involved.") 1270 protected List<MedicationAdministrationPerformerComponent> performer; 1271 1272 /** 1273 * A code indicating why the medication was given. 1274 */ 1275 @Child(name = "reasonCode", type = { 1276 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1277 @Description(shortDefinition = "Reason administration performed", formalDefinition = "A code indicating why the medication was given.") 1278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/reason-medication-given-codes") 1279 protected List<CodeableConcept> reasonCode; 1280 1281 /** 1282 * Condition or observation that supports why the medication was administered. 1283 */ 1284 @Child(name = "reasonReference", type = { Condition.class, Observation.class, 1285 DiagnosticReport.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1286 @Description(shortDefinition = "Condition or observation that supports why the medication was administered", formalDefinition = "Condition or observation that supports why the medication was administered.") 1287 protected List<Reference> reasonReference; 1288 /** 1289 * The actual objects that are the target of the reference (Condition or 1290 * observation that supports why the medication was administered.) 1291 */ 1292 protected List<Resource> reasonReferenceTarget; 1293 1294 /** 1295 * The original request, instruction or authority to perform the administration. 1296 */ 1297 @Child(name = "request", type = { 1298 MedicationRequest.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1299 @Description(shortDefinition = "Request administration performed against", formalDefinition = "The original request, instruction or authority to perform the administration.") 1300 protected Reference request; 1301 1302 /** 1303 * The actual object that is the target of the reference (The original request, 1304 * instruction or authority to perform the administration.) 1305 */ 1306 protected MedicationRequest requestTarget; 1307 1308 /** 1309 * The device used in administering the medication to the patient. For example, 1310 * a particular infusion pump. 1311 */ 1312 @Child(name = "device", type = { 1313 Device.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1314 @Description(shortDefinition = "Device used to administer", formalDefinition = "The device used in administering the medication to the patient. For example, a particular infusion pump.") 1315 protected List<Reference> device; 1316 /** 1317 * The actual objects that are the target of the reference (The device used in 1318 * administering the medication to the patient. For example, a particular 1319 * infusion pump.) 1320 */ 1321 protected List<Device> deviceTarget; 1322 1323 /** 1324 * Extra information about the medication administration that is not conveyed by 1325 * the other attributes. 1326 */ 1327 @Child(name = "note", type = { 1328 Annotation.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1329 @Description(shortDefinition = "Information about the administration", formalDefinition = "Extra information about the medication administration that is not conveyed by the other attributes.") 1330 protected List<Annotation> note; 1331 1332 /** 1333 * Describes the medication dosage information details e.g. dose, rate, site, 1334 * route, etc. 1335 */ 1336 @Child(name = "dosage", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = false) 1337 @Description(shortDefinition = "Details of how medication was taken", formalDefinition = "Describes the medication dosage information details e.g. dose, rate, site, route, etc.") 1338 protected MedicationAdministrationDosageComponent dosage; 1339 1340 /** 1341 * A summary of the events of interest that have occurred, such as when the 1342 * administration was verified. 1343 */ 1344 @Child(name = "eventHistory", type = { 1345 Provenance.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1346 @Description(shortDefinition = "A list of events of interest in the lifecycle", formalDefinition = "A summary of the events of interest that have occurred, such as when the administration was verified.") 1347 protected List<Reference> eventHistory; 1348 /** 1349 * The actual objects that are the target of the reference (A summary of the 1350 * events of interest that have occurred, such as when the administration was 1351 * verified.) 1352 */ 1353 protected List<Provenance> eventHistoryTarget; 1354 1355 private static final long serialVersionUID = 463158971L; 1356 1357 /** 1358 * Constructor 1359 */ 1360 public MedicationAdministration() { 1361 super(); 1362 } 1363 1364 /** 1365 * Constructor 1366 */ 1367 public MedicationAdministration(Enumeration<MedicationAdministrationStatus> status, Type medication, 1368 Reference subject, Type effective) { 1369 super(); 1370 this.status = status; 1371 this.medication = medication; 1372 this.subject = subject; 1373 this.effective = effective; 1374 } 1375 1376 /** 1377 * @return {@link #identifier} (Identifiers associated with this Medication 1378 * Administration that are defined by business processes and/or used to 1379 * refer to it when a direct URL reference to the resource itself is not 1380 * appropriate. They are business identifiers assigned to this resource 1381 * by the performer or other systems and remain constant as the resource 1382 * is updated and propagates from server to server.) 1383 */ 1384 public List<Identifier> getIdentifier() { 1385 if (this.identifier == null) 1386 this.identifier = new ArrayList<Identifier>(); 1387 return this.identifier; 1388 } 1389 1390 /** 1391 * @return Returns a reference to <code>this</code> for easy method chaining 1392 */ 1393 public MedicationAdministration setIdentifier(List<Identifier> theIdentifier) { 1394 this.identifier = theIdentifier; 1395 return this; 1396 } 1397 1398 public boolean hasIdentifier() { 1399 if (this.identifier == null) 1400 return false; 1401 for (Identifier item : this.identifier) 1402 if (!item.isEmpty()) 1403 return true; 1404 return false; 1405 } 1406 1407 public Identifier addIdentifier() { // 3 1408 Identifier t = new Identifier(); 1409 if (this.identifier == null) 1410 this.identifier = new ArrayList<Identifier>(); 1411 this.identifier.add(t); 1412 return t; 1413 } 1414 1415 public MedicationAdministration addIdentifier(Identifier t) { // 3 1416 if (t == null) 1417 return this; 1418 if (this.identifier == null) 1419 this.identifier = new ArrayList<Identifier>(); 1420 this.identifier.add(t); 1421 return this; 1422 } 1423 1424 /** 1425 * @return The first repetition of repeating field {@link #identifier}, creating 1426 * it if it does not already exist 1427 */ 1428 public Identifier getIdentifierFirstRep() { 1429 if (getIdentifier().isEmpty()) { 1430 addIdentifier(); 1431 } 1432 return getIdentifier().get(0); 1433 } 1434 1435 /** 1436 * @return {@link #instantiates} (A protocol, guideline, orderset, or other 1437 * definition that was adhered to in whole or in part by this event.) 1438 */ 1439 public List<UriType> getInstantiates() { 1440 if (this.instantiates == null) 1441 this.instantiates = new ArrayList<UriType>(); 1442 return this.instantiates; 1443 } 1444 1445 /** 1446 * @return Returns a reference to <code>this</code> for easy method chaining 1447 */ 1448 public MedicationAdministration setInstantiates(List<UriType> theInstantiates) { 1449 this.instantiates = theInstantiates; 1450 return this; 1451 } 1452 1453 public boolean hasInstantiates() { 1454 if (this.instantiates == null) 1455 return false; 1456 for (UriType item : this.instantiates) 1457 if (!item.isEmpty()) 1458 return true; 1459 return false; 1460 } 1461 1462 /** 1463 * @return {@link #instantiates} (A protocol, guideline, orderset, or other 1464 * definition that was adhered to in whole or in part by this event.) 1465 */ 1466 public UriType addInstantiatesElement() {// 2 1467 UriType t = new UriType(); 1468 if (this.instantiates == null) 1469 this.instantiates = new ArrayList<UriType>(); 1470 this.instantiates.add(t); 1471 return t; 1472 } 1473 1474 /** 1475 * @param value {@link #instantiates} (A protocol, guideline, orderset, or other 1476 * definition that was adhered to in whole or in part by this 1477 * event.) 1478 */ 1479 public MedicationAdministration addInstantiates(String value) { // 1 1480 UriType t = new UriType(); 1481 t.setValue(value); 1482 if (this.instantiates == null) 1483 this.instantiates = new ArrayList<UriType>(); 1484 this.instantiates.add(t); 1485 return this; 1486 } 1487 1488 /** 1489 * @param value {@link #instantiates} (A protocol, guideline, orderset, or other 1490 * definition that was adhered to in whole or in part by this 1491 * event.) 1492 */ 1493 public boolean hasInstantiates(String value) { 1494 if (this.instantiates == null) 1495 return false; 1496 for (UriType v : this.instantiates) 1497 if (v.getValue().equals(value)) // uri 1498 return true; 1499 return false; 1500 } 1501 1502 /** 1503 * @return {@link #partOf} (A larger event of which this particular event is a 1504 * component or step.) 1505 */ 1506 public List<Reference> getPartOf() { 1507 if (this.partOf == null) 1508 this.partOf = new ArrayList<Reference>(); 1509 return this.partOf; 1510 } 1511 1512 /** 1513 * @return Returns a reference to <code>this</code> for easy method chaining 1514 */ 1515 public MedicationAdministration setPartOf(List<Reference> thePartOf) { 1516 this.partOf = thePartOf; 1517 return this; 1518 } 1519 1520 public boolean hasPartOf() { 1521 if (this.partOf == null) 1522 return false; 1523 for (Reference item : this.partOf) 1524 if (!item.isEmpty()) 1525 return true; 1526 return false; 1527 } 1528 1529 public Reference addPartOf() { // 3 1530 Reference t = new Reference(); 1531 if (this.partOf == null) 1532 this.partOf = new ArrayList<Reference>(); 1533 this.partOf.add(t); 1534 return t; 1535 } 1536 1537 public MedicationAdministration addPartOf(Reference t) { // 3 1538 if (t == null) 1539 return this; 1540 if (this.partOf == null) 1541 this.partOf = new ArrayList<Reference>(); 1542 this.partOf.add(t); 1543 return this; 1544 } 1545 1546 /** 1547 * @return The first repetition of repeating field {@link #partOf}, creating it 1548 * if it does not already exist 1549 */ 1550 public Reference getPartOfFirstRep() { 1551 if (getPartOf().isEmpty()) { 1552 addPartOf(); 1553 } 1554 return getPartOf().get(0); 1555 } 1556 1557 /** 1558 * @deprecated Use Reference#setResource(IBaseResource) instead 1559 */ 1560 @Deprecated 1561 public List<Resource> getPartOfTarget() { 1562 if (this.partOfTarget == null) 1563 this.partOfTarget = new ArrayList<Resource>(); 1564 return this.partOfTarget; 1565 } 1566 1567 /** 1568 * @return {@link #status} (Will generally be set to show that the 1569 * administration has been completed. For some long running 1570 * administrations such as infusions, it is possible for an 1571 * administration to be started but not completed or it may be paused 1572 * while some other process is under way.). This is the underlying 1573 * object with id, value and extensions. The accessor "getStatus" gives 1574 * direct access to the value 1575 */ 1576 public Enumeration<MedicationAdministrationStatus> getStatusElement() { 1577 if (this.status == null) 1578 if (Configuration.errorOnAutoCreate()) 1579 throw new Error("Attempt to auto-create MedicationAdministration.status"); 1580 else if (Configuration.doAutoCreate()) 1581 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); // bb 1582 return this.status; 1583 } 1584 1585 public boolean hasStatusElement() { 1586 return this.status != null && !this.status.isEmpty(); 1587 } 1588 1589 public boolean hasStatus() { 1590 return this.status != null && !this.status.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #status} (Will generally be set to show that the 1595 * administration has been completed. For some long running 1596 * administrations such as infusions, it is possible for an 1597 * administration to be started but not completed or it may be 1598 * paused while some other process is under way.). This is the 1599 * underlying object with id, value and extensions. The accessor 1600 * "getStatus" gives direct access to the value 1601 */ 1602 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatus> value) { 1603 this.status = value; 1604 return this; 1605 } 1606 1607 /** 1608 * @return Will generally be set to show that the administration has been 1609 * completed. For some long running administrations such as infusions, 1610 * it is possible for an administration to be started but not completed 1611 * or it may be paused while some other process is under way. 1612 */ 1613 public MedicationAdministrationStatus getStatus() { 1614 return this.status == null ? null : this.status.getValue(); 1615 } 1616 1617 /** 1618 * @param value Will generally be set to show that the administration has been 1619 * completed. For some long running administrations such as 1620 * infusions, it is possible for an administration to be started 1621 * but not completed or it may be paused while some other process 1622 * is under way. 1623 */ 1624 public MedicationAdministration setStatus(MedicationAdministrationStatus value) { 1625 if (this.status == null) 1626 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); 1627 this.status.setValue(value); 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #statusReason} (A code indicating why the administration was 1633 * not performed.) 1634 */ 1635 public List<CodeableConcept> getStatusReason() { 1636 if (this.statusReason == null) 1637 this.statusReason = new ArrayList<CodeableConcept>(); 1638 return this.statusReason; 1639 } 1640 1641 /** 1642 * @return Returns a reference to <code>this</code> for easy method chaining 1643 */ 1644 public MedicationAdministration setStatusReason(List<CodeableConcept> theStatusReason) { 1645 this.statusReason = theStatusReason; 1646 return this; 1647 } 1648 1649 public boolean hasStatusReason() { 1650 if (this.statusReason == null) 1651 return false; 1652 for (CodeableConcept item : this.statusReason) 1653 if (!item.isEmpty()) 1654 return true; 1655 return false; 1656 } 1657 1658 public CodeableConcept addStatusReason() { // 3 1659 CodeableConcept t = new CodeableConcept(); 1660 if (this.statusReason == null) 1661 this.statusReason = new ArrayList<CodeableConcept>(); 1662 this.statusReason.add(t); 1663 return t; 1664 } 1665 1666 public MedicationAdministration addStatusReason(CodeableConcept t) { // 3 1667 if (t == null) 1668 return this; 1669 if (this.statusReason == null) 1670 this.statusReason = new ArrayList<CodeableConcept>(); 1671 this.statusReason.add(t); 1672 return this; 1673 } 1674 1675 /** 1676 * @return The first repetition of repeating field {@link #statusReason}, 1677 * creating it if it does not already exist 1678 */ 1679 public CodeableConcept getStatusReasonFirstRep() { 1680 if (getStatusReason().isEmpty()) { 1681 addStatusReason(); 1682 } 1683 return getStatusReason().get(0); 1684 } 1685 1686 /** 1687 * @return {@link #category} (Indicates where the medication is expected to be 1688 * consumed or administered.) 1689 */ 1690 public CodeableConcept getCategory() { 1691 if (this.category == null) 1692 if (Configuration.errorOnAutoCreate()) 1693 throw new Error("Attempt to auto-create MedicationAdministration.category"); 1694 else if (Configuration.doAutoCreate()) 1695 this.category = new CodeableConcept(); // cc 1696 return this.category; 1697 } 1698 1699 public boolean hasCategory() { 1700 return this.category != null && !this.category.isEmpty(); 1701 } 1702 1703 /** 1704 * @param value {@link #category} (Indicates where the medication is expected to 1705 * be consumed or administered.) 1706 */ 1707 public MedicationAdministration setCategory(CodeableConcept value) { 1708 this.category = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #medication} (Identifies the medication that was administered. 1714 * This is either a link to a resource representing the details of the 1715 * medication or a simple attribute carrying a code that identifies the 1716 * medication from a known list of medications.) 1717 */ 1718 public Type getMedication() { 1719 return this.medication; 1720 } 1721 1722 /** 1723 * @return {@link #medication} (Identifies the medication that was administered. 1724 * This is either a link to a resource representing the details of the 1725 * medication or a simple attribute carrying a code that identifies the 1726 * medication from a known list of medications.) 1727 */ 1728 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1729 if (this.medication == null) 1730 this.medication = new CodeableConcept(); 1731 if (!(this.medication instanceof CodeableConcept)) 1732 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1733 + this.medication.getClass().getName() + " was encountered"); 1734 return (CodeableConcept) this.medication; 1735 } 1736 1737 public boolean hasMedicationCodeableConcept() { 1738 return this != null && this.medication instanceof CodeableConcept; 1739 } 1740 1741 /** 1742 * @return {@link #medication} (Identifies the medication that was administered. 1743 * This is either a link to a resource representing the details of the 1744 * medication or a simple attribute carrying a code that identifies the 1745 * medication from a known list of medications.) 1746 */ 1747 public Reference getMedicationReference() throws FHIRException { 1748 if (this.medication == null) 1749 this.medication = new Reference(); 1750 if (!(this.medication instanceof Reference)) 1751 throw new FHIRException("Type mismatch: the type Reference was expected, but " 1752 + this.medication.getClass().getName() + " was encountered"); 1753 return (Reference) this.medication; 1754 } 1755 1756 public boolean hasMedicationReference() { 1757 return this != null && this.medication instanceof Reference; 1758 } 1759 1760 public boolean hasMedication() { 1761 return this.medication != null && !this.medication.isEmpty(); 1762 } 1763 1764 /** 1765 * @param value {@link #medication} (Identifies the medication that was 1766 * administered. This is either a link to a resource representing 1767 * the details of the medication or a simple attribute carrying a 1768 * code that identifies the medication from a known list of 1769 * medications.) 1770 */ 1771 public MedicationAdministration setMedication(Type value) { 1772 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1773 throw new Error("Not the right type for MedicationAdministration.medication[x]: " + value.fhirType()); 1774 this.medication = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #subject} (The person or animal or group receiving the 1780 * medication.) 1781 */ 1782 public Reference getSubject() { 1783 if (this.subject == null) 1784 if (Configuration.errorOnAutoCreate()) 1785 throw new Error("Attempt to auto-create MedicationAdministration.subject"); 1786 else if (Configuration.doAutoCreate()) 1787 this.subject = new Reference(); // cc 1788 return this.subject; 1789 } 1790 1791 public boolean hasSubject() { 1792 return this.subject != null && !this.subject.isEmpty(); 1793 } 1794 1795 /** 1796 * @param value {@link #subject} (The person or animal or group receiving the 1797 * medication.) 1798 */ 1799 public MedicationAdministration setSubject(Reference value) { 1800 this.subject = value; 1801 return this; 1802 } 1803 1804 /** 1805 * @return {@link #subject} The actual object that is the target of the 1806 * reference. The reference library doesn't populate this, but you can 1807 * use it to hold the resource if you resolve it. (The person or animal 1808 * or group receiving the medication.) 1809 */ 1810 public Resource getSubjectTarget() { 1811 return this.subjectTarget; 1812 } 1813 1814 /** 1815 * @param value {@link #subject} The actual object that is the target of the 1816 * reference. The reference library doesn't use these, but you can 1817 * use it to hold the resource if you resolve it. (The person or 1818 * animal or group receiving the medication.) 1819 */ 1820 public MedicationAdministration setSubjectTarget(Resource value) { 1821 this.subjectTarget = value; 1822 return this; 1823 } 1824 1825 /** 1826 * @return {@link #context} (The visit, admission, or other contact between 1827 * patient and health care provider during which the medication 1828 * administration was performed.) 1829 */ 1830 public Reference getContext() { 1831 if (this.context == null) 1832 if (Configuration.errorOnAutoCreate()) 1833 throw new Error("Attempt to auto-create MedicationAdministration.context"); 1834 else if (Configuration.doAutoCreate()) 1835 this.context = new Reference(); // cc 1836 return this.context; 1837 } 1838 1839 public boolean hasContext() { 1840 return this.context != null && !this.context.isEmpty(); 1841 } 1842 1843 /** 1844 * @param value {@link #context} (The visit, admission, or other contact between 1845 * patient and health care provider during which the medication 1846 * administration was performed.) 1847 */ 1848 public MedicationAdministration setContext(Reference value) { 1849 this.context = value; 1850 return this; 1851 } 1852 1853 /** 1854 * @return {@link #context} The actual object that is the target of the 1855 * reference. The reference library doesn't populate this, but you can 1856 * use it to hold the resource if you resolve it. (The visit, admission, 1857 * or other contact between patient and health care provider during 1858 * which the medication administration was performed.) 1859 */ 1860 public Resource getContextTarget() { 1861 return this.contextTarget; 1862 } 1863 1864 /** 1865 * @param value {@link #context} The actual object that is the target of the 1866 * reference. The reference library doesn't use these, but you can 1867 * use it to hold the resource if you resolve it. (The visit, 1868 * admission, or other contact between patient and health care 1869 * provider during which the medication administration was 1870 * performed.) 1871 */ 1872 public MedicationAdministration setContextTarget(Resource value) { 1873 this.contextTarget = value; 1874 return this; 1875 } 1876 1877 /** 1878 * @return {@link #supportingInformation} (Additional information (for example, 1879 * patient height and weight) that supports the administration of the 1880 * medication.) 1881 */ 1882 public List<Reference> getSupportingInformation() { 1883 if (this.supportingInformation == null) 1884 this.supportingInformation = new ArrayList<Reference>(); 1885 return this.supportingInformation; 1886 } 1887 1888 /** 1889 * @return Returns a reference to <code>this</code> for easy method chaining 1890 */ 1891 public MedicationAdministration setSupportingInformation(List<Reference> theSupportingInformation) { 1892 this.supportingInformation = theSupportingInformation; 1893 return this; 1894 } 1895 1896 public boolean hasSupportingInformation() { 1897 if (this.supportingInformation == null) 1898 return false; 1899 for (Reference item : this.supportingInformation) 1900 if (!item.isEmpty()) 1901 return true; 1902 return false; 1903 } 1904 1905 public Reference addSupportingInformation() { // 3 1906 Reference t = new Reference(); 1907 if (this.supportingInformation == null) 1908 this.supportingInformation = new ArrayList<Reference>(); 1909 this.supportingInformation.add(t); 1910 return t; 1911 } 1912 1913 public MedicationAdministration addSupportingInformation(Reference t) { // 3 1914 if (t == null) 1915 return this; 1916 if (this.supportingInformation == null) 1917 this.supportingInformation = new ArrayList<Reference>(); 1918 this.supportingInformation.add(t); 1919 return this; 1920 } 1921 1922 /** 1923 * @return The first repetition of repeating field 1924 * {@link #supportingInformation}, creating it if it does not already 1925 * exist 1926 */ 1927 public Reference getSupportingInformationFirstRep() { 1928 if (getSupportingInformation().isEmpty()) { 1929 addSupportingInformation(); 1930 } 1931 return getSupportingInformation().get(0); 1932 } 1933 1934 /** 1935 * @deprecated Use Reference#setResource(IBaseResource) instead 1936 */ 1937 @Deprecated 1938 public List<Resource> getSupportingInformationTarget() { 1939 if (this.supportingInformationTarget == null) 1940 this.supportingInformationTarget = new ArrayList<Resource>(); 1941 return this.supportingInformationTarget; 1942 } 1943 1944 /** 1945 * @return {@link #effective} (A specific date/time or interval of time during 1946 * which the administration took place (or did not take place, when the 1947 * 'notGiven' attribute is true). For many administrations, such as 1948 * swallowing a tablet the use of dateTime is more appropriate.) 1949 */ 1950 public Type getEffective() { 1951 return this.effective; 1952 } 1953 1954 /** 1955 * @return {@link #effective} (A specific date/time or interval of time during 1956 * which the administration took place (or did not take place, when the 1957 * 'notGiven' attribute is true). For many administrations, such as 1958 * swallowing a tablet the use of dateTime is more appropriate.) 1959 */ 1960 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1961 if (this.effective == null) 1962 this.effective = new DateTimeType(); 1963 if (!(this.effective instanceof DateTimeType)) 1964 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1965 + this.effective.getClass().getName() + " was encountered"); 1966 return (DateTimeType) this.effective; 1967 } 1968 1969 public boolean hasEffectiveDateTimeType() { 1970 return this != null && this.effective instanceof DateTimeType; 1971 } 1972 1973 /** 1974 * @return {@link #effective} (A specific date/time or interval of time during 1975 * which the administration took place (or did not take place, when the 1976 * 'notGiven' attribute is true). For many administrations, such as 1977 * swallowing a tablet the use of dateTime is more appropriate.) 1978 */ 1979 public Period getEffectivePeriod() throws FHIRException { 1980 if (this.effective == null) 1981 this.effective = new Period(); 1982 if (!(this.effective instanceof Period)) 1983 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 1984 + " was encountered"); 1985 return (Period) this.effective; 1986 } 1987 1988 public boolean hasEffectivePeriod() { 1989 return this != null && this.effective instanceof Period; 1990 } 1991 1992 public boolean hasEffective() { 1993 return this.effective != null && !this.effective.isEmpty(); 1994 } 1995 1996 /** 1997 * @param value {@link #effective} (A specific date/time or interval of time 1998 * during which the administration took place (or did not take 1999 * place, when the 'notGiven' attribute is true). For many 2000 * administrations, such as swallowing a tablet the use of dateTime 2001 * is more appropriate.) 2002 */ 2003 public MedicationAdministration setEffective(Type value) { 2004 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 2005 throw new Error("Not the right type for MedicationAdministration.effective[x]: " + value.fhirType()); 2006 this.effective = value; 2007 return this; 2008 } 2009 2010 /** 2011 * @return {@link #performer} (Indicates who or what performed the medication 2012 * administration and how they were involved.) 2013 */ 2014 public List<MedicationAdministrationPerformerComponent> getPerformer() { 2015 if (this.performer == null) 2016 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2017 return this.performer; 2018 } 2019 2020 /** 2021 * @return Returns a reference to <code>this</code> for easy method chaining 2022 */ 2023 public MedicationAdministration setPerformer(List<MedicationAdministrationPerformerComponent> thePerformer) { 2024 this.performer = thePerformer; 2025 return this; 2026 } 2027 2028 public boolean hasPerformer() { 2029 if (this.performer == null) 2030 return false; 2031 for (MedicationAdministrationPerformerComponent item : this.performer) 2032 if (!item.isEmpty()) 2033 return true; 2034 return false; 2035 } 2036 2037 public MedicationAdministrationPerformerComponent addPerformer() { // 3 2038 MedicationAdministrationPerformerComponent t = new MedicationAdministrationPerformerComponent(); 2039 if (this.performer == null) 2040 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2041 this.performer.add(t); 2042 return t; 2043 } 2044 2045 public MedicationAdministration addPerformer(MedicationAdministrationPerformerComponent t) { // 3 2046 if (t == null) 2047 return this; 2048 if (this.performer == null) 2049 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2050 this.performer.add(t); 2051 return this; 2052 } 2053 2054 /** 2055 * @return The first repetition of repeating field {@link #performer}, creating 2056 * it if it does not already exist 2057 */ 2058 public MedicationAdministrationPerformerComponent getPerformerFirstRep() { 2059 if (getPerformer().isEmpty()) { 2060 addPerformer(); 2061 } 2062 return getPerformer().get(0); 2063 } 2064 2065 /** 2066 * @return {@link #reasonCode} (A code indicating why the medication was given.) 2067 */ 2068 public List<CodeableConcept> getReasonCode() { 2069 if (this.reasonCode == null) 2070 this.reasonCode = new ArrayList<CodeableConcept>(); 2071 return this.reasonCode; 2072 } 2073 2074 /** 2075 * @return Returns a reference to <code>this</code> for easy method chaining 2076 */ 2077 public MedicationAdministration setReasonCode(List<CodeableConcept> theReasonCode) { 2078 this.reasonCode = theReasonCode; 2079 return this; 2080 } 2081 2082 public boolean hasReasonCode() { 2083 if (this.reasonCode == null) 2084 return false; 2085 for (CodeableConcept item : this.reasonCode) 2086 if (!item.isEmpty()) 2087 return true; 2088 return false; 2089 } 2090 2091 public CodeableConcept addReasonCode() { // 3 2092 CodeableConcept t = new CodeableConcept(); 2093 if (this.reasonCode == null) 2094 this.reasonCode = new ArrayList<CodeableConcept>(); 2095 this.reasonCode.add(t); 2096 return t; 2097 } 2098 2099 public MedicationAdministration addReasonCode(CodeableConcept t) { // 3 2100 if (t == null) 2101 return this; 2102 if (this.reasonCode == null) 2103 this.reasonCode = new ArrayList<CodeableConcept>(); 2104 this.reasonCode.add(t); 2105 return this; 2106 } 2107 2108 /** 2109 * @return The first repetition of repeating field {@link #reasonCode}, creating 2110 * it if it does not already exist 2111 */ 2112 public CodeableConcept getReasonCodeFirstRep() { 2113 if (getReasonCode().isEmpty()) { 2114 addReasonCode(); 2115 } 2116 return getReasonCode().get(0); 2117 } 2118 2119 /** 2120 * @return {@link #reasonReference} (Condition or observation that supports why 2121 * the medication was administered.) 2122 */ 2123 public List<Reference> getReasonReference() { 2124 if (this.reasonReference == null) 2125 this.reasonReference = new ArrayList<Reference>(); 2126 return this.reasonReference; 2127 } 2128 2129 /** 2130 * @return Returns a reference to <code>this</code> for easy method chaining 2131 */ 2132 public MedicationAdministration setReasonReference(List<Reference> theReasonReference) { 2133 this.reasonReference = theReasonReference; 2134 return this; 2135 } 2136 2137 public boolean hasReasonReference() { 2138 if (this.reasonReference == null) 2139 return false; 2140 for (Reference item : this.reasonReference) 2141 if (!item.isEmpty()) 2142 return true; 2143 return false; 2144 } 2145 2146 public Reference addReasonReference() { // 3 2147 Reference t = new Reference(); 2148 if (this.reasonReference == null) 2149 this.reasonReference = new ArrayList<Reference>(); 2150 this.reasonReference.add(t); 2151 return t; 2152 } 2153 2154 public MedicationAdministration addReasonReference(Reference t) { // 3 2155 if (t == null) 2156 return this; 2157 if (this.reasonReference == null) 2158 this.reasonReference = new ArrayList<Reference>(); 2159 this.reasonReference.add(t); 2160 return this; 2161 } 2162 2163 /** 2164 * @return The first repetition of repeating field {@link #reasonReference}, 2165 * creating it if it does not already exist 2166 */ 2167 public Reference getReasonReferenceFirstRep() { 2168 if (getReasonReference().isEmpty()) { 2169 addReasonReference(); 2170 } 2171 return getReasonReference().get(0); 2172 } 2173 2174 /** 2175 * @deprecated Use Reference#setResource(IBaseResource) instead 2176 */ 2177 @Deprecated 2178 public List<Resource> getReasonReferenceTarget() { 2179 if (this.reasonReferenceTarget == null) 2180 this.reasonReferenceTarget = new ArrayList<Resource>(); 2181 return this.reasonReferenceTarget; 2182 } 2183 2184 /** 2185 * @return {@link #request} (The original request, instruction or authority to 2186 * perform the administration.) 2187 */ 2188 public Reference getRequest() { 2189 if (this.request == null) 2190 if (Configuration.errorOnAutoCreate()) 2191 throw new Error("Attempt to auto-create MedicationAdministration.request"); 2192 else if (Configuration.doAutoCreate()) 2193 this.request = new Reference(); // cc 2194 return this.request; 2195 } 2196 2197 public boolean hasRequest() { 2198 return this.request != null && !this.request.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #request} (The original request, instruction or authority 2203 * to perform the administration.) 2204 */ 2205 public MedicationAdministration setRequest(Reference value) { 2206 this.request = value; 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #request} The actual object that is the target of the 2212 * reference. The reference library doesn't populate this, but you can 2213 * use it to hold the resource if you resolve it. (The original request, 2214 * instruction or authority to perform the administration.) 2215 */ 2216 public MedicationRequest getRequestTarget() { 2217 if (this.requestTarget == null) 2218 if (Configuration.errorOnAutoCreate()) 2219 throw new Error("Attempt to auto-create MedicationAdministration.request"); 2220 else if (Configuration.doAutoCreate()) 2221 this.requestTarget = new MedicationRequest(); // aa 2222 return this.requestTarget; 2223 } 2224 2225 /** 2226 * @param value {@link #request} The actual object that is the target of the 2227 * reference. The reference library doesn't use these, but you can 2228 * use it to hold the resource if you resolve it. (The original 2229 * request, instruction or authority to perform the 2230 * administration.) 2231 */ 2232 public MedicationAdministration setRequestTarget(MedicationRequest value) { 2233 this.requestTarget = value; 2234 return this; 2235 } 2236 2237 /** 2238 * @return {@link #device} (The device used in administering the medication to 2239 * the patient. For example, a particular infusion pump.) 2240 */ 2241 public List<Reference> getDevice() { 2242 if (this.device == null) 2243 this.device = new ArrayList<Reference>(); 2244 return this.device; 2245 } 2246 2247 /** 2248 * @return Returns a reference to <code>this</code> for easy method chaining 2249 */ 2250 public MedicationAdministration setDevice(List<Reference> theDevice) { 2251 this.device = theDevice; 2252 return this; 2253 } 2254 2255 public boolean hasDevice() { 2256 if (this.device == null) 2257 return false; 2258 for (Reference item : this.device) 2259 if (!item.isEmpty()) 2260 return true; 2261 return false; 2262 } 2263 2264 public Reference addDevice() { // 3 2265 Reference t = new Reference(); 2266 if (this.device == null) 2267 this.device = new ArrayList<Reference>(); 2268 this.device.add(t); 2269 return t; 2270 } 2271 2272 public MedicationAdministration addDevice(Reference t) { // 3 2273 if (t == null) 2274 return this; 2275 if (this.device == null) 2276 this.device = new ArrayList<Reference>(); 2277 this.device.add(t); 2278 return this; 2279 } 2280 2281 /** 2282 * @return The first repetition of repeating field {@link #device}, creating it 2283 * if it does not already exist 2284 */ 2285 public Reference getDeviceFirstRep() { 2286 if (getDevice().isEmpty()) { 2287 addDevice(); 2288 } 2289 return getDevice().get(0); 2290 } 2291 2292 /** 2293 * @deprecated Use Reference#setResource(IBaseResource) instead 2294 */ 2295 @Deprecated 2296 public List<Device> getDeviceTarget() { 2297 if (this.deviceTarget == null) 2298 this.deviceTarget = new ArrayList<Device>(); 2299 return this.deviceTarget; 2300 } 2301 2302 /** 2303 * @deprecated Use Reference#setResource(IBaseResource) instead 2304 */ 2305 @Deprecated 2306 public Device addDeviceTarget() { 2307 Device r = new Device(); 2308 if (this.deviceTarget == null) 2309 this.deviceTarget = new ArrayList<Device>(); 2310 this.deviceTarget.add(r); 2311 return r; 2312 } 2313 2314 /** 2315 * @return {@link #note} (Extra information about the medication administration 2316 * that is not conveyed by the other attributes.) 2317 */ 2318 public List<Annotation> getNote() { 2319 if (this.note == null) 2320 this.note = new ArrayList<Annotation>(); 2321 return this.note; 2322 } 2323 2324 /** 2325 * @return Returns a reference to <code>this</code> for easy method chaining 2326 */ 2327 public MedicationAdministration setNote(List<Annotation> theNote) { 2328 this.note = theNote; 2329 return this; 2330 } 2331 2332 public boolean hasNote() { 2333 if (this.note == null) 2334 return false; 2335 for (Annotation item : this.note) 2336 if (!item.isEmpty()) 2337 return true; 2338 return false; 2339 } 2340 2341 public Annotation addNote() { // 3 2342 Annotation t = new Annotation(); 2343 if (this.note == null) 2344 this.note = new ArrayList<Annotation>(); 2345 this.note.add(t); 2346 return t; 2347 } 2348 2349 public MedicationAdministration addNote(Annotation t) { // 3 2350 if (t == null) 2351 return this; 2352 if (this.note == null) 2353 this.note = new ArrayList<Annotation>(); 2354 this.note.add(t); 2355 return this; 2356 } 2357 2358 /** 2359 * @return The first repetition of repeating field {@link #note}, creating it if 2360 * it does not already exist 2361 */ 2362 public Annotation getNoteFirstRep() { 2363 if (getNote().isEmpty()) { 2364 addNote(); 2365 } 2366 return getNote().get(0); 2367 } 2368 2369 /** 2370 * @return {@link #dosage} (Describes the medication dosage information details 2371 * e.g. dose, rate, site, route, etc.) 2372 */ 2373 public MedicationAdministrationDosageComponent getDosage() { 2374 if (this.dosage == null) 2375 if (Configuration.errorOnAutoCreate()) 2376 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 2377 else if (Configuration.doAutoCreate()) 2378 this.dosage = new MedicationAdministrationDosageComponent(); // cc 2379 return this.dosage; 2380 } 2381 2382 public boolean hasDosage() { 2383 return this.dosage != null && !this.dosage.isEmpty(); 2384 } 2385 2386 /** 2387 * @param value {@link #dosage} (Describes the medication dosage information 2388 * details e.g. dose, rate, site, route, etc.) 2389 */ 2390 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 2391 this.dosage = value; 2392 return this; 2393 } 2394 2395 /** 2396 * @return {@link #eventHistory} (A summary of the events of interest that have 2397 * occurred, such as when the administration was verified.) 2398 */ 2399 public List<Reference> getEventHistory() { 2400 if (this.eventHistory == null) 2401 this.eventHistory = new ArrayList<Reference>(); 2402 return this.eventHistory; 2403 } 2404 2405 /** 2406 * @return Returns a reference to <code>this</code> for easy method chaining 2407 */ 2408 public MedicationAdministration setEventHistory(List<Reference> theEventHistory) { 2409 this.eventHistory = theEventHistory; 2410 return this; 2411 } 2412 2413 public boolean hasEventHistory() { 2414 if (this.eventHistory == null) 2415 return false; 2416 for (Reference item : this.eventHistory) 2417 if (!item.isEmpty()) 2418 return true; 2419 return false; 2420 } 2421 2422 public Reference addEventHistory() { // 3 2423 Reference t = new Reference(); 2424 if (this.eventHistory == null) 2425 this.eventHistory = new ArrayList<Reference>(); 2426 this.eventHistory.add(t); 2427 return t; 2428 } 2429 2430 public MedicationAdministration addEventHistory(Reference t) { // 3 2431 if (t == null) 2432 return this; 2433 if (this.eventHistory == null) 2434 this.eventHistory = new ArrayList<Reference>(); 2435 this.eventHistory.add(t); 2436 return this; 2437 } 2438 2439 /** 2440 * @return The first repetition of repeating field {@link #eventHistory}, 2441 * creating it if it does not already exist 2442 */ 2443 public Reference getEventHistoryFirstRep() { 2444 if (getEventHistory().isEmpty()) { 2445 addEventHistory(); 2446 } 2447 return getEventHistory().get(0); 2448 } 2449 2450 /** 2451 * @deprecated Use Reference#setResource(IBaseResource) instead 2452 */ 2453 @Deprecated 2454 public List<Provenance> getEventHistoryTarget() { 2455 if (this.eventHistoryTarget == null) 2456 this.eventHistoryTarget = new ArrayList<Provenance>(); 2457 return this.eventHistoryTarget; 2458 } 2459 2460 /** 2461 * @deprecated Use Reference#setResource(IBaseResource) instead 2462 */ 2463 @Deprecated 2464 public Provenance addEventHistoryTarget() { 2465 Provenance r = new Provenance(); 2466 if (this.eventHistoryTarget == null) 2467 this.eventHistoryTarget = new ArrayList<Provenance>(); 2468 this.eventHistoryTarget.add(r); 2469 return r; 2470 } 2471 2472 protected void listChildren(List<Property> children) { 2473 super.listChildren(children); 2474 children.add(new Property("identifier", "Identifier", 2475 "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2476 0, java.lang.Integer.MAX_VALUE, identifier)); 2477 children.add(new Property("instantiates", "uri", 2478 "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.", 2479 0, java.lang.Integer.MAX_VALUE, instantiates)); 2480 children.add(new Property("partOf", "Reference(MedicationAdministration|Procedure)", 2481 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2482 partOf)); 2483 children.add(new Property("status", "code", 2484 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 2485 0, 1, status)); 2486 children.add(new Property("statusReason", "CodeableConcept", 2487 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 2488 children.add(new Property("category", "CodeableConcept", 2489 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category)); 2490 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2491 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2492 0, 1, medication)); 2493 children.add(new Property("subject", "Reference(Patient|Group)", 2494 "The person or animal or group receiving the medication.", 0, 1, subject)); 2495 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 2496 "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 2497 0, 1, context)); 2498 children.add(new Property("supportingInformation", "Reference(Any)", 2499 "Additional information (for example, patient height and weight) that supports the administration of the medication.", 2500 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2501 children.add(new Property("effective[x]", "dateTime|Period", 2502 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2503 0, 1, effective)); 2504 children.add(new Property("performer", "", 2505 "Indicates who or what performed the medication administration and how they were involved.", 0, 2506 java.lang.Integer.MAX_VALUE, performer)); 2507 children.add(new Property("reasonCode", "CodeableConcept", "A code indicating why the medication was given.", 0, 2508 java.lang.Integer.MAX_VALUE, reasonCode)); 2509 children.add(new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 2510 "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, 2511 reasonReference)); 2512 children.add(new Property("request", "Reference(MedicationRequest)", 2513 "The original request, instruction or authority to perform the administration.", 0, 1, request)); 2514 children.add(new Property("device", "Reference(Device)", 2515 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, 2516 java.lang.Integer.MAX_VALUE, device)); 2517 children.add(new Property("note", "Annotation", 2518 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 2519 java.lang.Integer.MAX_VALUE, note)); 2520 children.add(new Property("dosage", "", 2521 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage)); 2522 children.add(new Property("eventHistory", "Reference(Provenance)", 2523 "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, 2524 java.lang.Integer.MAX_VALUE, eventHistory)); 2525 } 2526 2527 @Override 2528 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2529 switch (_hash) { 2530 case -1618432855: 2531 /* identifier */ return new Property("identifier", "Identifier", 2532 "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 2533 0, java.lang.Integer.MAX_VALUE, identifier); 2534 case -246883639: 2535 /* instantiates */ return new Property("instantiates", "uri", 2536 "A protocol, guideline, orderset, or other definition that was adhered to in whole or in part by this event.", 2537 0, java.lang.Integer.MAX_VALUE, instantiates); 2538 case -995410646: 2539 /* partOf */ return new Property("partOf", "Reference(MedicationAdministration|Procedure)", 2540 "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, 2541 partOf); 2542 case -892481550: 2543 /* status */ return new Property("status", "code", 2544 "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 2545 0, 1, status); 2546 case 2051346646: 2547 /* statusReason */ return new Property("statusReason", "CodeableConcept", 2548 "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason); 2549 case 50511102: 2550 /* category */ return new Property("category", "CodeableConcept", 2551 "Indicates where the medication is expected to be consumed or administered.", 0, 1, category); 2552 case 1458402129: 2553 /* medication[x] */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2554 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2555 0, 1, medication); 2556 case 1998965455: 2557 /* medication */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2558 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2559 0, 1, medication); 2560 case -209845038: 2561 /* medicationCodeableConcept */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2562 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2563 0, 1, medication); 2564 case 2104315196: 2565 /* medicationReference */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 2566 "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 2567 0, 1, medication); 2568 case -1867885268: 2569 /* subject */ return new Property("subject", "Reference(Patient|Group)", 2570 "The person or animal or group receiving the medication.", 0, 1, subject); 2571 case 951530927: 2572 /* context */ return new Property("context", "Reference(Encounter|EpisodeOfCare)", 2573 "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 2574 0, 1, context); 2575 case -1248768647: 2576 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 2577 "Additional information (for example, patient height and weight) that supports the administration of the medication.", 2578 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2579 case 247104889: 2580 /* effective[x] */ return new Property("effective[x]", "dateTime|Period", 2581 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2582 0, 1, effective); 2583 case -1468651097: 2584 /* effective */ return new Property("effective[x]", "dateTime|Period", 2585 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2586 0, 1, effective); 2587 case -275306910: 2588 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period", 2589 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2590 0, 1, effective); 2591 case -403934648: 2592 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period", 2593 "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 2594 0, 1, effective); 2595 case 481140686: 2596 /* performer */ return new Property("performer", "", 2597 "Indicates who or what performed the medication administration and how they were involved.", 0, 2598 java.lang.Integer.MAX_VALUE, performer); 2599 case 722137681: 2600 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 2601 "A code indicating why the medication was given.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2602 case -1146218137: 2603 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation|DiagnosticReport)", 2604 "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, 2605 reasonReference); 2606 case 1095692943: 2607 /* request */ return new Property("request", "Reference(MedicationRequest)", 2608 "The original request, instruction or authority to perform the administration.", 0, 1, request); 2609 case -1335157162: 2610 /* device */ return new Property("device", "Reference(Device)", 2611 "The device used in administering the medication to the patient. For example, a particular infusion pump.", 2612 0, java.lang.Integer.MAX_VALUE, device); 2613 case 3387378: 2614 /* note */ return new Property("note", "Annotation", 2615 "Extra information about the medication administration that is not conveyed by the other attributes.", 0, 2616 java.lang.Integer.MAX_VALUE, note); 2617 case -1326018889: 2618 /* dosage */ return new Property("dosage", "", 2619 "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage); 2620 case 1835190426: 2621 /* eventHistory */ return new Property("eventHistory", "Reference(Provenance)", 2622 "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, 2623 java.lang.Integer.MAX_VALUE, eventHistory); 2624 default: 2625 return super.getNamedProperty(_hash, _name, _checkValid); 2626 } 2627 2628 } 2629 2630 @Override 2631 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2632 switch (hash) { 2633 case -1618432855: 2634 /* identifier */ return this.identifier == null ? new Base[0] 2635 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2636 case -246883639: 2637 /* instantiates */ return this.instantiates == null ? new Base[0] 2638 : this.instantiates.toArray(new Base[this.instantiates.size()]); // UriType 2639 case -995410646: 2640 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2641 case -892481550: 2642 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationAdministrationStatus> 2643 case 2051346646: 2644 /* statusReason */ return this.statusReason == null ? new Base[0] 2645 : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 2646 case 50511102: 2647 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // CodeableConcept 2648 case 1998965455: 2649 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 2650 case -1867885268: 2651 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 2652 case 951530927: 2653 /* context */ return this.context == null ? new Base[0] : new Base[] { this.context }; // Reference 2654 case -1248768647: 2655 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 2656 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2657 case -1468651097: 2658 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 2659 case 481140686: 2660 /* performer */ return this.performer == null ? new Base[0] 2661 : this.performer.toArray(new Base[this.performer.size()]); // MedicationAdministrationPerformerComponent 2662 case 722137681: 2663 /* reasonCode */ return this.reasonCode == null ? new Base[0] 2664 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2665 case -1146218137: 2666 /* reasonReference */ return this.reasonReference == null ? new Base[0] 2667 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2668 case 1095692943: 2669 /* request */ return this.request == null ? new Base[0] : new Base[] { this.request }; // Reference 2670 case -1335157162: 2671 /* device */ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 2672 case 3387378: 2673 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2674 case -1326018889: 2675 /* dosage */ return this.dosage == null ? new Base[0] : new Base[] { this.dosage }; // MedicationAdministrationDosageComponent 2676 case 1835190426: 2677 /* eventHistory */ return this.eventHistory == null ? new Base[0] 2678 : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2679 default: 2680 return super.getProperty(hash, name, checkValid); 2681 } 2682 2683 } 2684 2685 @Override 2686 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2687 switch (hash) { 2688 case -1618432855: // identifier 2689 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2690 return value; 2691 case -246883639: // instantiates 2692 this.getInstantiates().add(castToUri(value)); // UriType 2693 return value; 2694 case -995410646: // partOf 2695 this.getPartOf().add(castToReference(value)); // Reference 2696 return value; 2697 case -892481550: // status 2698 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2699 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2700 return value; 2701 case 2051346646: // statusReason 2702 this.getStatusReason().add(castToCodeableConcept(value)); // CodeableConcept 2703 return value; 2704 case 50511102: // category 2705 this.category = castToCodeableConcept(value); // CodeableConcept 2706 return value; 2707 case 1998965455: // medication 2708 this.medication = castToType(value); // Type 2709 return value; 2710 case -1867885268: // subject 2711 this.subject = castToReference(value); // Reference 2712 return value; 2713 case 951530927: // context 2714 this.context = castToReference(value); // Reference 2715 return value; 2716 case -1248768647: // supportingInformation 2717 this.getSupportingInformation().add(castToReference(value)); // Reference 2718 return value; 2719 case -1468651097: // effective 2720 this.effective = castToType(value); // Type 2721 return value; 2722 case 481140686: // performer 2723 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); // MedicationAdministrationPerformerComponent 2724 return value; 2725 case 722137681: // reasonCode 2726 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2727 return value; 2728 case -1146218137: // reasonReference 2729 this.getReasonReference().add(castToReference(value)); // Reference 2730 return value; 2731 case 1095692943: // request 2732 this.request = castToReference(value); // Reference 2733 return value; 2734 case -1335157162: // device 2735 this.getDevice().add(castToReference(value)); // Reference 2736 return value; 2737 case 3387378: // note 2738 this.getNote().add(castToAnnotation(value)); // Annotation 2739 return value; 2740 case -1326018889: // dosage 2741 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2742 return value; 2743 case 1835190426: // eventHistory 2744 this.getEventHistory().add(castToReference(value)); // Reference 2745 return value; 2746 default: 2747 return super.setProperty(hash, name, value); 2748 } 2749 2750 } 2751 2752 @Override 2753 public Base setProperty(String name, Base value) throws FHIRException { 2754 if (name.equals("identifier")) { 2755 this.getIdentifier().add(castToIdentifier(value)); 2756 } else if (name.equals("instantiates")) { 2757 this.getInstantiates().add(castToUri(value)); 2758 } else if (name.equals("partOf")) { 2759 this.getPartOf().add(castToReference(value)); 2760 } else if (name.equals("status")) { 2761 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2762 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2763 } else if (name.equals("statusReason")) { 2764 this.getStatusReason().add(castToCodeableConcept(value)); 2765 } else if (name.equals("category")) { 2766 this.category = castToCodeableConcept(value); // CodeableConcept 2767 } else if (name.equals("medication[x]")) { 2768 this.medication = castToType(value); // Type 2769 } else if (name.equals("subject")) { 2770 this.subject = castToReference(value); // Reference 2771 } else if (name.equals("context")) { 2772 this.context = castToReference(value); // Reference 2773 } else if (name.equals("supportingInformation")) { 2774 this.getSupportingInformation().add(castToReference(value)); 2775 } else if (name.equals("effective[x]")) { 2776 this.effective = castToType(value); // Type 2777 } else if (name.equals("performer")) { 2778 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); 2779 } else if (name.equals("reasonCode")) { 2780 this.getReasonCode().add(castToCodeableConcept(value)); 2781 } else if (name.equals("reasonReference")) { 2782 this.getReasonReference().add(castToReference(value)); 2783 } else if (name.equals("request")) { 2784 this.request = castToReference(value); // Reference 2785 } else if (name.equals("device")) { 2786 this.getDevice().add(castToReference(value)); 2787 } else if (name.equals("note")) { 2788 this.getNote().add(castToAnnotation(value)); 2789 } else if (name.equals("dosage")) { 2790 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2791 } else if (name.equals("eventHistory")) { 2792 this.getEventHistory().add(castToReference(value)); 2793 } else 2794 return super.setProperty(name, value); 2795 return value; 2796 } 2797 2798 @Override 2799 public Base makeProperty(int hash, String name) throws FHIRException { 2800 switch (hash) { 2801 case -1618432855: 2802 return addIdentifier(); 2803 case -246883639: 2804 return addInstantiatesElement(); 2805 case -995410646: 2806 return addPartOf(); 2807 case -892481550: 2808 return getStatusElement(); 2809 case 2051346646: 2810 return addStatusReason(); 2811 case 50511102: 2812 return getCategory(); 2813 case 1458402129: 2814 return getMedication(); 2815 case 1998965455: 2816 return getMedication(); 2817 case -1867885268: 2818 return getSubject(); 2819 case 951530927: 2820 return getContext(); 2821 case -1248768647: 2822 return addSupportingInformation(); 2823 case 247104889: 2824 return getEffective(); 2825 case -1468651097: 2826 return getEffective(); 2827 case 481140686: 2828 return addPerformer(); 2829 case 722137681: 2830 return addReasonCode(); 2831 case -1146218137: 2832 return addReasonReference(); 2833 case 1095692943: 2834 return getRequest(); 2835 case -1335157162: 2836 return addDevice(); 2837 case 3387378: 2838 return addNote(); 2839 case -1326018889: 2840 return getDosage(); 2841 case 1835190426: 2842 return addEventHistory(); 2843 default: 2844 return super.makeProperty(hash, name); 2845 } 2846 2847 } 2848 2849 @Override 2850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2851 switch (hash) { 2852 case -1618432855: 2853 /* identifier */ return new String[] { "Identifier" }; 2854 case -246883639: 2855 /* instantiates */ return new String[] { "uri" }; 2856 case -995410646: 2857 /* partOf */ return new String[] { "Reference" }; 2858 case -892481550: 2859 /* status */ return new String[] { "code" }; 2860 case 2051346646: 2861 /* statusReason */ return new String[] { "CodeableConcept" }; 2862 case 50511102: 2863 /* category */ return new String[] { "CodeableConcept" }; 2864 case 1998965455: 2865 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 2866 case -1867885268: 2867 /* subject */ return new String[] { "Reference" }; 2868 case 951530927: 2869 /* context */ return new String[] { "Reference" }; 2870 case -1248768647: 2871 /* supportingInformation */ return new String[] { "Reference" }; 2872 case -1468651097: 2873 /* effective */ return new String[] { "dateTime", "Period" }; 2874 case 481140686: 2875 /* performer */ return new String[] {}; 2876 case 722137681: 2877 /* reasonCode */ return new String[] { "CodeableConcept" }; 2878 case -1146218137: 2879 /* reasonReference */ return new String[] { "Reference" }; 2880 case 1095692943: 2881 /* request */ return new String[] { "Reference" }; 2882 case -1335157162: 2883 /* device */ return new String[] { "Reference" }; 2884 case 3387378: 2885 /* note */ return new String[] { "Annotation" }; 2886 case -1326018889: 2887 /* dosage */ return new String[] {}; 2888 case 1835190426: 2889 /* eventHistory */ return new String[] { "Reference" }; 2890 default: 2891 return super.getTypesForProperty(hash, name); 2892 } 2893 2894 } 2895 2896 @Override 2897 public Base addChild(String name) throws FHIRException { 2898 if (name.equals("identifier")) { 2899 return addIdentifier(); 2900 } else if (name.equals("instantiates")) { 2901 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.instantiates"); 2902 } else if (name.equals("partOf")) { 2903 return addPartOf(); 2904 } else if (name.equals("status")) { 2905 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 2906 } else if (name.equals("statusReason")) { 2907 return addStatusReason(); 2908 } else if (name.equals("category")) { 2909 this.category = new CodeableConcept(); 2910 return this.category; 2911 } else if (name.equals("medicationCodeableConcept")) { 2912 this.medication = new CodeableConcept(); 2913 return this.medication; 2914 } else if (name.equals("medicationReference")) { 2915 this.medication = new Reference(); 2916 return this.medication; 2917 } else if (name.equals("subject")) { 2918 this.subject = new Reference(); 2919 return this.subject; 2920 } else if (name.equals("context")) { 2921 this.context = new Reference(); 2922 return this.context; 2923 } else if (name.equals("supportingInformation")) { 2924 return addSupportingInformation(); 2925 } else if (name.equals("effectiveDateTime")) { 2926 this.effective = new DateTimeType(); 2927 return this.effective; 2928 } else if (name.equals("effectivePeriod")) { 2929 this.effective = new Period(); 2930 return this.effective; 2931 } else if (name.equals("performer")) { 2932 return addPerformer(); 2933 } else if (name.equals("reasonCode")) { 2934 return addReasonCode(); 2935 } else if (name.equals("reasonReference")) { 2936 return addReasonReference(); 2937 } else if (name.equals("request")) { 2938 this.request = new Reference(); 2939 return this.request; 2940 } else if (name.equals("device")) { 2941 return addDevice(); 2942 } else if (name.equals("note")) { 2943 return addNote(); 2944 } else if (name.equals("dosage")) { 2945 this.dosage = new MedicationAdministrationDosageComponent(); 2946 return this.dosage; 2947 } else if (name.equals("eventHistory")) { 2948 return addEventHistory(); 2949 } else 2950 return super.addChild(name); 2951 } 2952 2953 public String fhirType() { 2954 return "MedicationAdministration"; 2955 2956 } 2957 2958 public MedicationAdministration copy() { 2959 MedicationAdministration dst = new MedicationAdministration(); 2960 copyValues(dst); 2961 return dst; 2962 } 2963 2964 public void copyValues(MedicationAdministration dst) { 2965 super.copyValues(dst); 2966 if (identifier != null) { 2967 dst.identifier = new ArrayList<Identifier>(); 2968 for (Identifier i : identifier) 2969 dst.identifier.add(i.copy()); 2970 } 2971 ; 2972 if (instantiates != null) { 2973 dst.instantiates = new ArrayList<UriType>(); 2974 for (UriType i : instantiates) 2975 dst.instantiates.add(i.copy()); 2976 } 2977 ; 2978 if (partOf != null) { 2979 dst.partOf = new ArrayList<Reference>(); 2980 for (Reference i : partOf) 2981 dst.partOf.add(i.copy()); 2982 } 2983 ; 2984 dst.status = status == null ? null : status.copy(); 2985 if (statusReason != null) { 2986 dst.statusReason = new ArrayList<CodeableConcept>(); 2987 for (CodeableConcept i : statusReason) 2988 dst.statusReason.add(i.copy()); 2989 } 2990 ; 2991 dst.category = category == null ? null : category.copy(); 2992 dst.medication = medication == null ? null : medication.copy(); 2993 dst.subject = subject == null ? null : subject.copy(); 2994 dst.context = context == null ? null : context.copy(); 2995 if (supportingInformation != null) { 2996 dst.supportingInformation = new ArrayList<Reference>(); 2997 for (Reference i : supportingInformation) 2998 dst.supportingInformation.add(i.copy()); 2999 } 3000 ; 3001 dst.effective = effective == null ? null : effective.copy(); 3002 if (performer != null) { 3003 dst.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 3004 for (MedicationAdministrationPerformerComponent i : performer) 3005 dst.performer.add(i.copy()); 3006 } 3007 ; 3008 if (reasonCode != null) { 3009 dst.reasonCode = new ArrayList<CodeableConcept>(); 3010 for (CodeableConcept i : reasonCode) 3011 dst.reasonCode.add(i.copy()); 3012 } 3013 ; 3014 if (reasonReference != null) { 3015 dst.reasonReference = new ArrayList<Reference>(); 3016 for (Reference i : reasonReference) 3017 dst.reasonReference.add(i.copy()); 3018 } 3019 ; 3020 dst.request = request == null ? null : request.copy(); 3021 if (device != null) { 3022 dst.device = new ArrayList<Reference>(); 3023 for (Reference i : device) 3024 dst.device.add(i.copy()); 3025 } 3026 ; 3027 if (note != null) { 3028 dst.note = new ArrayList<Annotation>(); 3029 for (Annotation i : note) 3030 dst.note.add(i.copy()); 3031 } 3032 ; 3033 dst.dosage = dosage == null ? null : dosage.copy(); 3034 if (eventHistory != null) { 3035 dst.eventHistory = new ArrayList<Reference>(); 3036 for (Reference i : eventHistory) 3037 dst.eventHistory.add(i.copy()); 3038 } 3039 ; 3040 } 3041 3042 protected MedicationAdministration typedCopy() { 3043 return copy(); 3044 } 3045 3046 @Override 3047 public boolean equalsDeep(Base other_) { 3048 if (!super.equalsDeep(other_)) 3049 return false; 3050 if (!(other_ instanceof MedicationAdministration)) 3051 return false; 3052 MedicationAdministration o = (MedicationAdministration) other_; 3053 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiates, o.instantiates, true) 3054 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 3055 && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 3056 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 3057 && compareDeep(context, o.context, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3058 && compareDeep(effective, o.effective, true) && compareDeep(performer, o.performer, true) 3059 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3060 && compareDeep(request, o.request, true) && compareDeep(device, o.device, true) 3061 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true) 3062 && compareDeep(eventHistory, o.eventHistory, true); 3063 } 3064 3065 @Override 3066 public boolean equalsShallow(Base other_) { 3067 if (!super.equalsShallow(other_)) 3068 return false; 3069 if (!(other_ instanceof MedicationAdministration)) 3070 return false; 3071 MedicationAdministration o = (MedicationAdministration) other_; 3072 return compareValues(instantiates, o.instantiates, true) && compareValues(status, o.status, true); 3073 } 3074 3075 public boolean isEmpty() { 3076 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiates, partOf, status, 3077 statusReason, category, medication, subject, context, supportingInformation, effective, performer, reasonCode, 3078 reasonReference, request, device, note, dosage, eventHistory); 3079 } 3080 3081 @Override 3082 public ResourceType getResourceType() { 3083 return ResourceType.MedicationAdministration; 3084 } 3085 3086 /** 3087 * Search parameter: <b>identifier</b> 3088 * <p> 3089 * Description: <b>Return administrations with this external identifier</b><br> 3090 * Type: <b>token</b><br> 3091 * Path: <b>MedicationAdministration.identifier</b><br> 3092 * </p> 3093 */ 3094 @SearchParamDefinition(name = "identifier", path = "MedicationAdministration.identifier", description = "Return administrations with this external identifier", type = "token") 3095 public static final String SP_IDENTIFIER = "identifier"; 3096 /** 3097 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3098 * <p> 3099 * Description: <b>Return administrations with this external identifier</b><br> 3100 * Type: <b>token</b><br> 3101 * Path: <b>MedicationAdministration.identifier</b><br> 3102 * </p> 3103 */ 3104 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3105 SP_IDENTIFIER); 3106 3107 /** 3108 * Search parameter: <b>request</b> 3109 * <p> 3110 * Description: <b>The identity of a request to list administrations 3111 * from</b><br> 3112 * Type: <b>reference</b><br> 3113 * Path: <b>MedicationAdministration.request</b><br> 3114 * </p> 3115 */ 3116 @SearchParamDefinition(name = "request", path = "MedicationAdministration.request", description = "The identity of a request to list administrations from", type = "reference", target = { 3117 MedicationRequest.class }) 3118 public static final String SP_REQUEST = "request"; 3119 /** 3120 * <b>Fluent Client</b> search parameter constant for <b>request</b> 3121 * <p> 3122 * Description: <b>The identity of a request to list administrations 3123 * from</b><br> 3124 * Type: <b>reference</b><br> 3125 * Path: <b>MedicationAdministration.request</b><br> 3126 * </p> 3127 */ 3128 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3129 SP_REQUEST); 3130 3131 /** 3132 * Constant for fluent queries to be used to add include statements. Specifies 3133 * the path value of "<b>MedicationAdministration:request</b>". 3134 */ 3135 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include( 3136 "MedicationAdministration:request").toLocked(); 3137 3138 /** 3139 * Search parameter: <b>code</b> 3140 * <p> 3141 * Description: <b>Return administrations of this medication code</b><br> 3142 * Type: <b>token</b><br> 3143 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 3144 * </p> 3145 */ 3146 @SearchParamDefinition(name = "code", path = "(MedicationAdministration.medication as CodeableConcept)", description = "Return administrations of this medication code", type = "token") 3147 public static final String SP_CODE = "code"; 3148 /** 3149 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3150 * <p> 3151 * Description: <b>Return administrations of this medication code</b><br> 3152 * Type: <b>token</b><br> 3153 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 3154 * </p> 3155 */ 3156 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3157 SP_CODE); 3158 3159 /** 3160 * Search parameter: <b>performer</b> 3161 * <p> 3162 * Description: <b>The identity of the individual who administered the 3163 * medication</b><br> 3164 * Type: <b>reference</b><br> 3165 * Path: <b>MedicationAdministration.performer.actor</b><br> 3166 * </p> 3167 */ 3168 @SearchParamDefinition(name = "performer", path = "MedicationAdministration.performer.actor", description = "The identity of the individual who administered the medication", type = "reference", providesMembershipIn = { 3169 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 3170 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 3171 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, Patient.class, 3172 Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 3173 public static final String SP_PERFORMER = "performer"; 3174 /** 3175 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3176 * <p> 3177 * Description: <b>The identity of the individual who administered the 3178 * medication</b><br> 3179 * Type: <b>reference</b><br> 3180 * Path: <b>MedicationAdministration.performer.actor</b><br> 3181 * </p> 3182 */ 3183 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3184 SP_PERFORMER); 3185 3186 /** 3187 * Constant for fluent queries to be used to add include statements. Specifies 3188 * the path value of "<b>MedicationAdministration:performer</b>". 3189 */ 3190 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 3191 "MedicationAdministration:performer").toLocked(); 3192 3193 /** 3194 * Search parameter: <b>subject</b> 3195 * <p> 3196 * Description: <b>The identity of the individual or group to list 3197 * administrations for</b><br> 3198 * Type: <b>reference</b><br> 3199 * Path: <b>MedicationAdministration.subject</b><br> 3200 * </p> 3201 */ 3202 @SearchParamDefinition(name = "subject", path = "MedicationAdministration.subject", description = "The identity of the individual or group to list administrations for", type = "reference", providesMembershipIn = { 3203 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 3204 public static final String SP_SUBJECT = "subject"; 3205 /** 3206 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3207 * <p> 3208 * Description: <b>The identity of the individual or group to list 3209 * administrations for</b><br> 3210 * Type: <b>reference</b><br> 3211 * Path: <b>MedicationAdministration.subject</b><br> 3212 * </p> 3213 */ 3214 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3215 SP_SUBJECT); 3216 3217 /** 3218 * Constant for fluent queries to be used to add include statements. Specifies 3219 * the path value of "<b>MedicationAdministration:subject</b>". 3220 */ 3221 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 3222 "MedicationAdministration:subject").toLocked(); 3223 3224 /** 3225 * Search parameter: <b>medication</b> 3226 * <p> 3227 * Description: <b>Return administrations of this medication resource</b><br> 3228 * Type: <b>reference</b><br> 3229 * Path: <b>MedicationAdministration.medicationReference</b><br> 3230 * </p> 3231 */ 3232 @SearchParamDefinition(name = "medication", path = "(MedicationAdministration.medication as Reference)", description = "Return administrations of this medication resource", type = "reference", target = { 3233 Medication.class }) 3234 public static final String SP_MEDICATION = "medication"; 3235 /** 3236 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3237 * <p> 3238 * Description: <b>Return administrations of this medication resource</b><br> 3239 * Type: <b>reference</b><br> 3240 * Path: <b>MedicationAdministration.medicationReference</b><br> 3241 * </p> 3242 */ 3243 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3244 SP_MEDICATION); 3245 3246 /** 3247 * Constant for fluent queries to be used to add include statements. Specifies 3248 * the path value of "<b>MedicationAdministration:medication</b>". 3249 */ 3250 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include( 3251 "MedicationAdministration:medication").toLocked(); 3252 3253 /** 3254 * Search parameter: <b>reason-given</b> 3255 * <p> 3256 * Description: <b>Reasons for administering the medication</b><br> 3257 * Type: <b>token</b><br> 3258 * Path: <b>MedicationAdministration.reasonCode</b><br> 3259 * </p> 3260 */ 3261 @SearchParamDefinition(name = "reason-given", path = "MedicationAdministration.reasonCode", description = "Reasons for administering the medication", type = "token") 3262 public static final String SP_REASON_GIVEN = "reason-given"; 3263 /** 3264 * <b>Fluent Client</b> search parameter constant for <b>reason-given</b> 3265 * <p> 3266 * Description: <b>Reasons for administering the medication</b><br> 3267 * Type: <b>token</b><br> 3268 * Path: <b>MedicationAdministration.reasonCode</b><br> 3269 * </p> 3270 */ 3271 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3272 SP_REASON_GIVEN); 3273 3274 /** 3275 * Search parameter: <b>patient</b> 3276 * <p> 3277 * Description: <b>The identity of a patient to list administrations for</b><br> 3278 * Type: <b>reference</b><br> 3279 * Path: <b>MedicationAdministration.subject</b><br> 3280 * </p> 3281 */ 3282 @SearchParamDefinition(name = "patient", path = "MedicationAdministration.subject.where(resolve() is Patient)", description = "The identity of a patient to list administrations for", type = "reference", providesMembershipIn = { 3283 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 3284 public static final String SP_PATIENT = "patient"; 3285 /** 3286 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3287 * <p> 3288 * Description: <b>The identity of a patient to list administrations for</b><br> 3289 * Type: <b>reference</b><br> 3290 * Path: <b>MedicationAdministration.subject</b><br> 3291 * </p> 3292 */ 3293 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3294 SP_PATIENT); 3295 3296 /** 3297 * Constant for fluent queries to be used to add include statements. Specifies 3298 * the path value of "<b>MedicationAdministration:patient</b>". 3299 */ 3300 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 3301 "MedicationAdministration:patient").toLocked(); 3302 3303 /** 3304 * Search parameter: <b>effective-time</b> 3305 * <p> 3306 * Description: <b>Date administration happened (or did not happen)</b><br> 3307 * Type: <b>date</b><br> 3308 * Path: <b>MedicationAdministration.effective[x]</b><br> 3309 * </p> 3310 */ 3311 @SearchParamDefinition(name = "effective-time", path = "MedicationAdministration.effective", description = "Date administration happened (or did not happen)", type = "date") 3312 public static final String SP_EFFECTIVE_TIME = "effective-time"; 3313 /** 3314 * <b>Fluent Client</b> search parameter constant for <b>effective-time</b> 3315 * <p> 3316 * Description: <b>Date administration happened (or did not happen)</b><br> 3317 * Type: <b>date</b><br> 3318 * Path: <b>MedicationAdministration.effective[x]</b><br> 3319 * </p> 3320 */ 3321 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE_TIME = new ca.uhn.fhir.rest.gclient.DateClientParam( 3322 SP_EFFECTIVE_TIME); 3323 3324 /** 3325 * Search parameter: <b>context</b> 3326 * <p> 3327 * Description: <b>Return administrations that share this encounter or episode 3328 * of care</b><br> 3329 * Type: <b>reference</b><br> 3330 * Path: <b>MedicationAdministration.context</b><br> 3331 * </p> 3332 */ 3333 @SearchParamDefinition(name = "context", path = "MedicationAdministration.context", description = "Return administrations that share this encounter or episode of care", type = "reference", providesMembershipIn = { 3334 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class, 3335 EpisodeOfCare.class }) 3336 public static final String SP_CONTEXT = "context"; 3337 /** 3338 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3339 * <p> 3340 * Description: <b>Return administrations that share this encounter or episode 3341 * of care</b><br> 3342 * Type: <b>reference</b><br> 3343 * Path: <b>MedicationAdministration.context</b><br> 3344 * </p> 3345 */ 3346 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3347 SP_CONTEXT); 3348 3349 /** 3350 * Constant for fluent queries to be used to add include statements. Specifies 3351 * the path value of "<b>MedicationAdministration:context</b>". 3352 */ 3353 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include( 3354 "MedicationAdministration:context").toLocked(); 3355 3356 /** 3357 * Search parameter: <b>reason-not-given</b> 3358 * <p> 3359 * Description: <b>Reasons for not administering the medication</b><br> 3360 * Type: <b>token</b><br> 3361 * Path: <b>MedicationAdministration.statusReason</b><br> 3362 * </p> 3363 */ 3364 @SearchParamDefinition(name = "reason-not-given", path = "MedicationAdministration.statusReason", description = "Reasons for not administering the medication", type = "token") 3365 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 3366 /** 3367 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 3368 * <p> 3369 * Description: <b>Reasons for not administering the medication</b><br> 3370 * Type: <b>token</b><br> 3371 * Path: <b>MedicationAdministration.statusReason</b><br> 3372 * </p> 3373 */ 3374 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3375 SP_REASON_NOT_GIVEN); 3376 3377 /** 3378 * Search parameter: <b>device</b> 3379 * <p> 3380 * Description: <b>Return administrations with this administration device 3381 * identity</b><br> 3382 * Type: <b>reference</b><br> 3383 * Path: <b>MedicationAdministration.device</b><br> 3384 * </p> 3385 */ 3386 @SearchParamDefinition(name = "device", path = "MedicationAdministration.device", description = "Return administrations with this administration device identity", type = "reference", providesMembershipIn = { 3387 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class }) 3388 public static final String SP_DEVICE = "device"; 3389 /** 3390 * <b>Fluent Client</b> search parameter constant for <b>device</b> 3391 * <p> 3392 * Description: <b>Return administrations with this administration device 3393 * identity</b><br> 3394 * Type: <b>reference</b><br> 3395 * Path: <b>MedicationAdministration.device</b><br> 3396 * </p> 3397 */ 3398 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3399 SP_DEVICE); 3400 3401 /** 3402 * Constant for fluent queries to be used to add include statements. Specifies 3403 * the path value of "<b>MedicationAdministration:device</b>". 3404 */ 3405 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 3406 "MedicationAdministration:device").toLocked(); 3407 3408 /** 3409 * Search parameter: <b>status</b> 3410 * <p> 3411 * Description: <b>MedicationAdministration event status (for example one of 3412 * active/paused/completed/nullified)</b><br> 3413 * Type: <b>token</b><br> 3414 * Path: <b>MedicationAdministration.status</b><br> 3415 * </p> 3416 */ 3417 @SearchParamDefinition(name = "status", path = "MedicationAdministration.status", description = "MedicationAdministration event status (for example one of active/paused/completed/nullified)", type = "token") 3418 public static final String SP_STATUS = "status"; 3419 /** 3420 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3421 * <p> 3422 * Description: <b>MedicationAdministration event status (for example one of 3423 * active/paused/completed/nullified)</b><br> 3424 * Type: <b>token</b><br> 3425 * Path: <b>MedicationAdministration.status</b><br> 3426 * </p> 3427 */ 3428 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3429 SP_STATUS); 3430 3431}