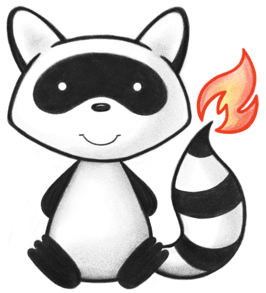
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * An order or request for both supply of the medication and the instructions 048 * for administration of the medication to a patient. The resource is called 049 * "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" 050 * to generalize the use across inpatient and outpatient settings, including 051 * care plans, etc., and to harmonize with workflow patterns. 052 */ 053@ResourceDef(name = "MedicationRequest", profile = "http://hl7.org/fhir/StructureDefinition/MedicationRequest") 054public class MedicationRequest extends DomainResource { 055 056 public enum MedicationRequestStatus { 057 /** 058 * The prescription is 'actionable', but not all actions that are implied by it 059 * have occurred yet. 060 */ 061 ACTIVE, 062 /** 063 * Actions implied by the prescription are to be temporarily halted, but are 064 * expected to continue later. May also be called 'suspended'. 065 */ 066 ONHOLD, 067 /** 068 * The prescription has been withdrawn before any administrations have occurred 069 */ 070 CANCELLED, 071 /** 072 * All actions that are implied by the prescription have occurred. 073 */ 074 COMPLETED, 075 /** 076 * Some of the actions that are implied by the medication request may have 077 * occurred. For example, the medication may have been dispensed and the patient 078 * may have taken some of the medication. Clinical decision support systems 079 * should take this status into account 080 */ 081 ENTEREDINERROR, 082 /** 083 * Actions implied by the prescription are to be permanently halted, before all 084 * of the administrations occurred. This should not be used if the original 085 * order was entered in error 086 */ 087 STOPPED, 088 /** 089 * The prescription is not yet 'actionable', e.g. it is a work in progress, 090 * requires sign-off, verification or needs to be run through decision support 091 * process. 092 */ 093 DRAFT, 094 /** 095 * The authoring/source system does not know which of the status values 096 * currently applies for this observation. Note: This concept is not to be used 097 * for 'other' - one of the listed statuses is presumed to apply, but the 098 * authoring/source system does not know which. 099 */ 100 UNKNOWN, 101 /** 102 * added to help the parsers with the generic types 103 */ 104 NULL; 105 106 public static MedicationRequestStatus fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("active".equals(codeString)) 110 return ACTIVE; 111 if ("on-hold".equals(codeString)) 112 return ONHOLD; 113 if ("cancelled".equals(codeString)) 114 return CANCELLED; 115 if ("completed".equals(codeString)) 116 return COMPLETED; 117 if ("entered-in-error".equals(codeString)) 118 return ENTEREDINERROR; 119 if ("stopped".equals(codeString)) 120 return STOPPED; 121 if ("draft".equals(codeString)) 122 return DRAFT; 123 if ("unknown".equals(codeString)) 124 return UNKNOWN; 125 if (Configuration.isAcceptInvalidEnums()) 126 return null; 127 else 128 throw new FHIRException("Unknown MedicationRequestStatus code '" + codeString + "'"); 129 } 130 131 public String toCode() { 132 switch (this) { 133 case ACTIVE: 134 return "active"; 135 case ONHOLD: 136 return "on-hold"; 137 case CANCELLED: 138 return "cancelled"; 139 case COMPLETED: 140 return "completed"; 141 case ENTEREDINERROR: 142 return "entered-in-error"; 143 case STOPPED: 144 return "stopped"; 145 case DRAFT: 146 return "draft"; 147 case UNKNOWN: 148 return "unknown"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getSystem() { 157 switch (this) { 158 case ACTIVE: 159 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 160 case ONHOLD: 161 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 162 case CANCELLED: 163 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 164 case COMPLETED: 165 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 166 case ENTEREDINERROR: 167 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 168 case STOPPED: 169 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 170 case DRAFT: 171 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 172 case UNKNOWN: 173 return "http://hl7.org/fhir/CodeSystem/medicationrequest-status"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDefinition() { 182 switch (this) { 183 case ACTIVE: 184 return "The prescription is 'actionable', but not all actions that are implied by it have occurred yet."; 185 case ONHOLD: 186 return "Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called 'suspended'."; 187 case CANCELLED: 188 return "The prescription has been withdrawn before any administrations have occurred"; 189 case COMPLETED: 190 return "All actions that are implied by the prescription have occurred."; 191 case ENTEREDINERROR: 192 return "Some of the actions that are implied by the medication request may have occurred. For example, the medication may have been dispensed and the patient may have taken some of the medication. Clinical decision support systems should take this status into account"; 193 case STOPPED: 194 return "Actions implied by the prescription are to be permanently halted, before all of the administrations occurred. This should not be used if the original order was entered in error"; 195 case DRAFT: 196 return "The prescription is not yet 'actionable', e.g. it is a work in progress, requires sign-off, verification or needs to be run through decision support process."; 197 case UNKNOWN: 198 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 199 case NULL: 200 return null; 201 default: 202 return "?"; 203 } 204 } 205 206 public String getDisplay() { 207 switch (this) { 208 case ACTIVE: 209 return "Active"; 210 case ONHOLD: 211 return "On Hold"; 212 case CANCELLED: 213 return "Cancelled"; 214 case COMPLETED: 215 return "Completed"; 216 case ENTEREDINERROR: 217 return "Entered in Error"; 218 case STOPPED: 219 return "Stopped"; 220 case DRAFT: 221 return "Draft"; 222 case UNKNOWN: 223 return "Unknown"; 224 case NULL: 225 return null; 226 default: 227 return "?"; 228 } 229 } 230 } 231 232 public static class MedicationRequestStatusEnumFactory implements EnumFactory<MedicationRequestStatus> { 233 public MedicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 234 if (codeString == null || "".equals(codeString)) 235 if (codeString == null || "".equals(codeString)) 236 return null; 237 if ("active".equals(codeString)) 238 return MedicationRequestStatus.ACTIVE; 239 if ("on-hold".equals(codeString)) 240 return MedicationRequestStatus.ONHOLD; 241 if ("cancelled".equals(codeString)) 242 return MedicationRequestStatus.CANCELLED; 243 if ("completed".equals(codeString)) 244 return MedicationRequestStatus.COMPLETED; 245 if ("entered-in-error".equals(codeString)) 246 return MedicationRequestStatus.ENTEREDINERROR; 247 if ("stopped".equals(codeString)) 248 return MedicationRequestStatus.STOPPED; 249 if ("draft".equals(codeString)) 250 return MedicationRequestStatus.DRAFT; 251 if ("unknown".equals(codeString)) 252 return MedicationRequestStatus.UNKNOWN; 253 throw new IllegalArgumentException("Unknown MedicationRequestStatus code '" + codeString + "'"); 254 } 255 256 public Enumeration<MedicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 257 if (code == null) 258 return null; 259 if (code.isEmpty()) 260 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.NULL, code); 261 String codeString = code.asStringValue(); 262 if (codeString == null || "".equals(codeString)) 263 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.NULL, code); 264 if ("active".equals(codeString)) 265 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ACTIVE, code); 266 if ("on-hold".equals(codeString)) 267 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ONHOLD, code); 268 if ("cancelled".equals(codeString)) 269 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.CANCELLED, code); 270 if ("completed".equals(codeString)) 271 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.COMPLETED, code); 272 if ("entered-in-error".equals(codeString)) 273 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ENTEREDINERROR, code); 274 if ("stopped".equals(codeString)) 275 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.STOPPED, code); 276 if ("draft".equals(codeString)) 277 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.DRAFT, code); 278 if ("unknown".equals(codeString)) 279 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.UNKNOWN, code); 280 throw new FHIRException("Unknown MedicationRequestStatus code '" + codeString + "'"); 281 } 282 283 public String toCode(MedicationRequestStatus code) { 284 if (code == MedicationRequestStatus.NULL) 285 return null; 286 if (code == MedicationRequestStatus.ACTIVE) 287 return "active"; 288 if (code == MedicationRequestStatus.ONHOLD) 289 return "on-hold"; 290 if (code == MedicationRequestStatus.CANCELLED) 291 return "cancelled"; 292 if (code == MedicationRequestStatus.COMPLETED) 293 return "completed"; 294 if (code == MedicationRequestStatus.ENTEREDINERROR) 295 return "entered-in-error"; 296 if (code == MedicationRequestStatus.STOPPED) 297 return "stopped"; 298 if (code == MedicationRequestStatus.DRAFT) 299 return "draft"; 300 if (code == MedicationRequestStatus.UNKNOWN) 301 return "unknown"; 302 return "?"; 303 } 304 305 public String toSystem(MedicationRequestStatus code) { 306 return code.getSystem(); 307 } 308 } 309 310 public enum MedicationRequestIntent { 311 /** 312 * The request is a suggestion made by someone/something that doesn't have an 313 * intention to ensure it occurs and without providing an authorization to act 314 */ 315 PROPOSAL, 316 /** 317 * The request represents an intention to ensure something occurs without 318 * providing an authorization for others to act. 319 */ 320 PLAN, 321 /** 322 * The request represents a request/demand and authorization for action 323 */ 324 ORDER, 325 /** 326 * The request represents the original authorization for the medication request. 327 */ 328 ORIGINALORDER, 329 /** 330 * The request represents an automatically generated supplemental authorization 331 * for action based on a parent authorization together with initial results of 332 * the action taken against that parent authorization.. 333 */ 334 REFLEXORDER, 335 /** 336 * The request represents the view of an authorization instantiated by a 337 * fulfilling system representing the details of the fulfiller's intention to 338 * act upon a submitted order. 339 */ 340 FILLERORDER, 341 /** 342 * The request represents an instance for the particular order, for example a 343 * medication administration record. 344 */ 345 INSTANCEORDER, 346 /** 347 * The request represents a component or option for a RequestGroup that 348 * establishes timing, conditionality and/or other constraints among a set of 349 * requests. 350 */ 351 OPTION, 352 /** 353 * added to help the parsers with the generic types 354 */ 355 NULL; 356 357 public static MedicationRequestIntent fromCode(String codeString) throws FHIRException { 358 if (codeString == null || "".equals(codeString)) 359 return null; 360 if ("proposal".equals(codeString)) 361 return PROPOSAL; 362 if ("plan".equals(codeString)) 363 return PLAN; 364 if ("order".equals(codeString)) 365 return ORDER; 366 if ("original-order".equals(codeString)) 367 return ORIGINALORDER; 368 if ("reflex-order".equals(codeString)) 369 return REFLEXORDER; 370 if ("filler-order".equals(codeString)) 371 return FILLERORDER; 372 if ("instance-order".equals(codeString)) 373 return INSTANCEORDER; 374 if ("option".equals(codeString)) 375 return OPTION; 376 if (Configuration.isAcceptInvalidEnums()) 377 return null; 378 else 379 throw new FHIRException("Unknown MedicationRequestIntent code '" + codeString + "'"); 380 } 381 382 public String toCode() { 383 switch (this) { 384 case PROPOSAL: 385 return "proposal"; 386 case PLAN: 387 return "plan"; 388 case ORDER: 389 return "order"; 390 case ORIGINALORDER: 391 return "original-order"; 392 case REFLEXORDER: 393 return "reflex-order"; 394 case FILLERORDER: 395 return "filler-order"; 396 case INSTANCEORDER: 397 return "instance-order"; 398 case OPTION: 399 return "option"; 400 case NULL: 401 return null; 402 default: 403 return "?"; 404 } 405 } 406 407 public String getSystem() { 408 switch (this) { 409 case PROPOSAL: 410 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 411 case PLAN: 412 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 413 case ORDER: 414 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 415 case ORIGINALORDER: 416 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 417 case REFLEXORDER: 418 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 419 case FILLERORDER: 420 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 421 case INSTANCEORDER: 422 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 423 case OPTION: 424 return "http://hl7.org/fhir/CodeSystem/medicationrequest-intent"; 425 case NULL: 426 return null; 427 default: 428 return "?"; 429 } 430 } 431 432 public String getDefinition() { 433 switch (this) { 434 case PROPOSAL: 435 return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 436 case PLAN: 437 return "The request represents an intention to ensure something occurs without providing an authorization for others to act."; 438 case ORDER: 439 return "The request represents a request/demand and authorization for action"; 440 case ORIGINALORDER: 441 return "The request represents the original authorization for the medication request."; 442 case REFLEXORDER: 443 return "The request represents an automatically generated supplemental authorization for action based on a parent authorization together with initial results of the action taken against that parent authorization.."; 444 case FILLERORDER: 445 return "The request represents the view of an authorization instantiated by a fulfilling system representing the details of the fulfiller's intention to act upon a submitted order."; 446 case INSTANCEORDER: 447 return "The request represents an instance for the particular order, for example a medication administration record."; 448 case OPTION: 449 return "The request represents a component or option for a RequestGroup that establishes timing, conditionality and/or other constraints among a set of requests."; 450 case NULL: 451 return null; 452 default: 453 return "?"; 454 } 455 } 456 457 public String getDisplay() { 458 switch (this) { 459 case PROPOSAL: 460 return "Proposal"; 461 case PLAN: 462 return "Plan"; 463 case ORDER: 464 return "Order"; 465 case ORIGINALORDER: 466 return "Original Order"; 467 case REFLEXORDER: 468 return "Reflex Order"; 469 case FILLERORDER: 470 return "Filler Order"; 471 case INSTANCEORDER: 472 return "Instance Order"; 473 case OPTION: 474 return "Option"; 475 case NULL: 476 return null; 477 default: 478 return "?"; 479 } 480 } 481 } 482 483 public static class MedicationRequestIntentEnumFactory implements EnumFactory<MedicationRequestIntent> { 484 public MedicationRequestIntent fromCode(String codeString) throws IllegalArgumentException { 485 if (codeString == null || "".equals(codeString)) 486 if (codeString == null || "".equals(codeString)) 487 return null; 488 if ("proposal".equals(codeString)) 489 return MedicationRequestIntent.PROPOSAL; 490 if ("plan".equals(codeString)) 491 return MedicationRequestIntent.PLAN; 492 if ("order".equals(codeString)) 493 return MedicationRequestIntent.ORDER; 494 if ("original-order".equals(codeString)) 495 return MedicationRequestIntent.ORIGINALORDER; 496 if ("reflex-order".equals(codeString)) 497 return MedicationRequestIntent.REFLEXORDER; 498 if ("filler-order".equals(codeString)) 499 return MedicationRequestIntent.FILLERORDER; 500 if ("instance-order".equals(codeString)) 501 return MedicationRequestIntent.INSTANCEORDER; 502 if ("option".equals(codeString)) 503 return MedicationRequestIntent.OPTION; 504 throw new IllegalArgumentException("Unknown MedicationRequestIntent code '" + codeString + "'"); 505 } 506 507 public Enumeration<MedicationRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 508 if (code == null) 509 return null; 510 if (code.isEmpty()) 511 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 512 String codeString = code.asStringValue(); 513 if (codeString == null || "".equals(codeString)) 514 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.NULL, code); 515 if ("proposal".equals(codeString)) 516 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PROPOSAL, code); 517 if ("plan".equals(codeString)) 518 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PLAN, code); 519 if ("order".equals(codeString)) 520 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORDER, code); 521 if ("original-order".equals(codeString)) 522 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORIGINALORDER, code); 523 if ("reflex-order".equals(codeString)) 524 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.REFLEXORDER, code); 525 if ("filler-order".equals(codeString)) 526 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.FILLERORDER, code); 527 if ("instance-order".equals(codeString)) 528 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.INSTANCEORDER, code); 529 if ("option".equals(codeString)) 530 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.OPTION, code); 531 throw new FHIRException("Unknown MedicationRequestIntent code '" + codeString + "'"); 532 } 533 534 public String toCode(MedicationRequestIntent code) { 535 if (code == MedicationRequestIntent.NULL) 536 return null; 537 if (code == MedicationRequestIntent.PROPOSAL) 538 return "proposal"; 539 if (code == MedicationRequestIntent.PLAN) 540 return "plan"; 541 if (code == MedicationRequestIntent.ORDER) 542 return "order"; 543 if (code == MedicationRequestIntent.ORIGINALORDER) 544 return "original-order"; 545 if (code == MedicationRequestIntent.REFLEXORDER) 546 return "reflex-order"; 547 if (code == MedicationRequestIntent.FILLERORDER) 548 return "filler-order"; 549 if (code == MedicationRequestIntent.INSTANCEORDER) 550 return "instance-order"; 551 if (code == MedicationRequestIntent.OPTION) 552 return "option"; 553 return "?"; 554 } 555 556 public String toSystem(MedicationRequestIntent code) { 557 return code.getSystem(); 558 } 559 } 560 561 public enum MedicationRequestPriority { 562 /** 563 * The request has normal priority. 564 */ 565 ROUTINE, 566 /** 567 * The request should be actioned promptly - higher priority than routine. 568 */ 569 URGENT, 570 /** 571 * The request should be actioned as soon as possible - higher priority than 572 * urgent. 573 */ 574 ASAP, 575 /** 576 * The request should be actioned immediately - highest possible priority. E.g. 577 * an emergency. 578 */ 579 STAT, 580 /** 581 * added to help the parsers with the generic types 582 */ 583 NULL; 584 585 public static MedicationRequestPriority fromCode(String codeString) throws FHIRException { 586 if (codeString == null || "".equals(codeString)) 587 return null; 588 if ("routine".equals(codeString)) 589 return ROUTINE; 590 if ("urgent".equals(codeString)) 591 return URGENT; 592 if ("asap".equals(codeString)) 593 return ASAP; 594 if ("stat".equals(codeString)) 595 return STAT; 596 if (Configuration.isAcceptInvalidEnums()) 597 return null; 598 else 599 throw new FHIRException("Unknown MedicationRequestPriority code '" + codeString + "'"); 600 } 601 602 public String toCode() { 603 switch (this) { 604 case ROUTINE: 605 return "routine"; 606 case URGENT: 607 return "urgent"; 608 case ASAP: 609 return "asap"; 610 case STAT: 611 return "stat"; 612 case NULL: 613 return null; 614 default: 615 return "?"; 616 } 617 } 618 619 public String getSystem() { 620 switch (this) { 621 case ROUTINE: 622 return "http://hl7.org/fhir/request-priority"; 623 case URGENT: 624 return "http://hl7.org/fhir/request-priority"; 625 case ASAP: 626 return "http://hl7.org/fhir/request-priority"; 627 case STAT: 628 return "http://hl7.org/fhir/request-priority"; 629 case NULL: 630 return null; 631 default: 632 return "?"; 633 } 634 } 635 636 public String getDefinition() { 637 switch (this) { 638 case ROUTINE: 639 return "The request has normal priority."; 640 case URGENT: 641 return "The request should be actioned promptly - higher priority than routine."; 642 case ASAP: 643 return "The request should be actioned as soon as possible - higher priority than urgent."; 644 case STAT: 645 return "The request should be actioned immediately - highest possible priority. E.g. an emergency."; 646 case NULL: 647 return null; 648 default: 649 return "?"; 650 } 651 } 652 653 public String getDisplay() { 654 switch (this) { 655 case ROUTINE: 656 return "Routine"; 657 case URGENT: 658 return "Urgent"; 659 case ASAP: 660 return "ASAP"; 661 case STAT: 662 return "STAT"; 663 case NULL: 664 return null; 665 default: 666 return "?"; 667 } 668 } 669 } 670 671 public static class MedicationRequestPriorityEnumFactory implements EnumFactory<MedicationRequestPriority> { 672 public MedicationRequestPriority fromCode(String codeString) throws IllegalArgumentException { 673 if (codeString == null || "".equals(codeString)) 674 if (codeString == null || "".equals(codeString)) 675 return null; 676 if ("routine".equals(codeString)) 677 return MedicationRequestPriority.ROUTINE; 678 if ("urgent".equals(codeString)) 679 return MedicationRequestPriority.URGENT; 680 if ("asap".equals(codeString)) 681 return MedicationRequestPriority.ASAP; 682 if ("stat".equals(codeString)) 683 return MedicationRequestPriority.STAT; 684 throw new IllegalArgumentException("Unknown MedicationRequestPriority code '" + codeString + "'"); 685 } 686 687 public Enumeration<MedicationRequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 688 if (code == null) 689 return null; 690 if (code.isEmpty()) 691 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.NULL, code); 692 String codeString = code.asStringValue(); 693 if (codeString == null || "".equals(codeString)) 694 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.NULL, code); 695 if ("routine".equals(codeString)) 696 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.ROUTINE, code); 697 if ("urgent".equals(codeString)) 698 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.URGENT, code); 699 if ("asap".equals(codeString)) 700 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.ASAP, code); 701 if ("stat".equals(codeString)) 702 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.STAT, code); 703 throw new FHIRException("Unknown MedicationRequestPriority code '" + codeString + "'"); 704 } 705 706 public String toCode(MedicationRequestPriority code) { 707 if (code == MedicationRequestPriority.NULL) 708 return null; 709 if (code == MedicationRequestPriority.ROUTINE) 710 return "routine"; 711 if (code == MedicationRequestPriority.URGENT) 712 return "urgent"; 713 if (code == MedicationRequestPriority.ASAP) 714 return "asap"; 715 if (code == MedicationRequestPriority.STAT) 716 return "stat"; 717 return "?"; 718 } 719 720 public String toSystem(MedicationRequestPriority code) { 721 return code.getSystem(); 722 } 723 } 724 725 @Block() 726 public static class MedicationRequestDispenseRequestComponent extends BackboneElement 727 implements IBaseBackboneElement { 728 /** 729 * Indicates the quantity or duration for the first dispense of the medication. 730 */ 731 @Child(name = "initialFill", type = {}, order = 1, min = 0, max = 1, modifier = false, summary = false) 732 @Description(shortDefinition = "First fill details", formalDefinition = "Indicates the quantity or duration for the first dispense of the medication.") 733 protected MedicationRequestDispenseRequestInitialFillComponent initialFill; 734 735 /** 736 * The minimum period of time that must occur between dispenses of the 737 * medication. 738 */ 739 @Child(name = "dispenseInterval", type = { 740 Duration.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 741 @Description(shortDefinition = "Minimum period of time between dispenses", formalDefinition = "The minimum period of time that must occur between dispenses of the medication.") 742 protected Duration dispenseInterval; 743 744 /** 745 * This indicates the validity period of a prescription (stale dating the 746 * Prescription). 747 */ 748 @Child(name = "validityPeriod", type = { 749 Period.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 750 @Description(shortDefinition = "Time period supply is authorized for", formalDefinition = "This indicates the validity period of a prescription (stale dating the Prescription).") 751 protected Period validityPeriod; 752 753 /** 754 * An integer indicating the number of times, in addition to the original 755 * dispense, (aka refills or repeats) that the patient can receive the 756 * prescribed medication. Usage Notes: This integer does not include the 757 * original order dispense. This means that if an order indicates dispense 30 758 * tablets plus "3 repeats", then the order can be dispensed a total of 4 times 759 * and the patient can receive a total of 120 tablets. A prescriber may 760 * explicitly say that zero refills are permitted after the initial dispense. 761 */ 762 @Child(name = "numberOfRepeatsAllowed", type = { 763 UnsignedIntType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 764 @Description(shortDefinition = "Number of refills authorized", formalDefinition = "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.") 765 protected UnsignedIntType numberOfRepeatsAllowed; 766 767 /** 768 * The amount that is to be dispensed for one fill. 769 */ 770 @Child(name = "quantity", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 771 @Description(shortDefinition = "Amount of medication to supply per dispense", formalDefinition = "The amount that is to be dispensed for one fill.") 772 protected Quantity quantity; 773 774 /** 775 * Identifies the period time over which the supplied product is expected to be 776 * used, or the length of time the dispense is expected to last. 777 */ 778 @Child(name = "expectedSupplyDuration", type = { 779 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 780 @Description(shortDefinition = "Number of days supply per dispense", formalDefinition = "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.") 781 protected Duration expectedSupplyDuration; 782 783 /** 784 * Indicates the intended dispensing Organization specified by the prescriber. 785 */ 786 @Child(name = "performer", type = { 787 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 788 @Description(shortDefinition = "Intended dispenser", formalDefinition = "Indicates the intended dispensing Organization specified by the prescriber.") 789 protected Reference performer; 790 791 /** 792 * The actual object that is the target of the reference (Indicates the intended 793 * dispensing Organization specified by the prescriber.) 794 */ 795 protected Organization performerTarget; 796 797 private static final long serialVersionUID = -1680129929L; 798 799 /** 800 * Constructor 801 */ 802 public MedicationRequestDispenseRequestComponent() { 803 super(); 804 } 805 806 /** 807 * @return {@link #initialFill} (Indicates the quantity or duration for the 808 * first dispense of the medication.) 809 */ 810 public MedicationRequestDispenseRequestInitialFillComponent getInitialFill() { 811 if (this.initialFill == null) 812 if (Configuration.errorOnAutoCreate()) 813 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.initialFill"); 814 else if (Configuration.doAutoCreate()) 815 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); // cc 816 return this.initialFill; 817 } 818 819 public boolean hasInitialFill() { 820 return this.initialFill != null && !this.initialFill.isEmpty(); 821 } 822 823 /** 824 * @param value {@link #initialFill} (Indicates the quantity or duration for the 825 * first dispense of the medication.) 826 */ 827 public MedicationRequestDispenseRequestComponent setInitialFill( 828 MedicationRequestDispenseRequestInitialFillComponent value) { 829 this.initialFill = value; 830 return this; 831 } 832 833 /** 834 * @return {@link #dispenseInterval} (The minimum period of time that must occur 835 * between dispenses of the medication.) 836 */ 837 public Duration getDispenseInterval() { 838 if (this.dispenseInterval == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.dispenseInterval"); 841 else if (Configuration.doAutoCreate()) 842 this.dispenseInterval = new Duration(); // cc 843 return this.dispenseInterval; 844 } 845 846 public boolean hasDispenseInterval() { 847 return this.dispenseInterval != null && !this.dispenseInterval.isEmpty(); 848 } 849 850 /** 851 * @param value {@link #dispenseInterval} (The minimum period of time that must 852 * occur between dispenses of the medication.) 853 */ 854 public MedicationRequestDispenseRequestComponent setDispenseInterval(Duration value) { 855 this.dispenseInterval = value; 856 return this; 857 } 858 859 /** 860 * @return {@link #validityPeriod} (This indicates the validity period of a 861 * prescription (stale dating the Prescription).) 862 */ 863 public Period getValidityPeriod() { 864 if (this.validityPeriod == null) 865 if (Configuration.errorOnAutoCreate()) 866 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.validityPeriod"); 867 else if (Configuration.doAutoCreate()) 868 this.validityPeriod = new Period(); // cc 869 return this.validityPeriod; 870 } 871 872 public boolean hasValidityPeriod() { 873 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 874 } 875 876 /** 877 * @param value {@link #validityPeriod} (This indicates the validity period of a 878 * prescription (stale dating the Prescription).) 879 */ 880 public MedicationRequestDispenseRequestComponent setValidityPeriod(Period value) { 881 this.validityPeriod = value; 882 return this; 883 } 884 885 /** 886 * @return {@link #numberOfRepeatsAllowed} (An integer indicating the number of 887 * times, in addition to the original dispense, (aka refills or repeats) 888 * that the patient can receive the prescribed medication. Usage Notes: 889 * This integer does not include the original order dispense. This means 890 * that if an order indicates dispense 30 tablets plus "3 repeats", then 891 * the order can be dispensed a total of 4 times and the patient can 892 * receive a total of 120 tablets. A prescriber may explicitly say that 893 * zero refills are permitted after the initial dispense.). This is the 894 * underlying object with id, value and extensions. The accessor 895 * "getNumberOfRepeatsAllowed" gives direct access to the value 896 */ 897 public UnsignedIntType getNumberOfRepeatsAllowedElement() { 898 if (this.numberOfRepeatsAllowed == null) 899 if (Configuration.errorOnAutoCreate()) 900 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.numberOfRepeatsAllowed"); 901 else if (Configuration.doAutoCreate()) 902 this.numberOfRepeatsAllowed = new UnsignedIntType(); // bb 903 return this.numberOfRepeatsAllowed; 904 } 905 906 public boolean hasNumberOfRepeatsAllowedElement() { 907 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 908 } 909 910 public boolean hasNumberOfRepeatsAllowed() { 911 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 912 } 913 914 /** 915 * @param value {@link #numberOfRepeatsAllowed} (An integer indicating the 916 * number of times, in addition to the original dispense, (aka 917 * refills or repeats) that the patient can receive the prescribed 918 * medication. Usage Notes: This integer does not include the 919 * original order dispense. This means that if an order indicates 920 * dispense 30 tablets plus "3 repeats", then the order can be 921 * dispensed a total of 4 times and the patient can receive a total 922 * of 120 tablets. A prescriber may explicitly say that zero 923 * refills are permitted after the initial dispense.). This is the 924 * underlying object with id, value and extensions. The accessor 925 * "getNumberOfRepeatsAllowed" gives direct access to the value 926 */ 927 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowedElement(UnsignedIntType value) { 928 this.numberOfRepeatsAllowed = value; 929 return this; 930 } 931 932 /** 933 * @return An integer indicating the number of times, in addition to the 934 * original dispense, (aka refills or repeats) that the patient can 935 * receive the prescribed medication. Usage Notes: This integer does not 936 * include the original order dispense. This means that if an order 937 * indicates dispense 30 tablets plus "3 repeats", then the order can be 938 * dispensed a total of 4 times and the patient can receive a total of 939 * 120 tablets. A prescriber may explicitly say that zero refills are 940 * permitted after the initial dispense. 941 */ 942 public int getNumberOfRepeatsAllowed() { 943 return this.numberOfRepeatsAllowed == null || this.numberOfRepeatsAllowed.isEmpty() ? 0 944 : this.numberOfRepeatsAllowed.getValue(); 945 } 946 947 /** 948 * @param value An integer indicating the number of times, in addition to the 949 * original dispense, (aka refills or repeats) that the patient can 950 * receive the prescribed medication. Usage Notes: This integer 951 * does not include the original order dispense. This means that if 952 * an order indicates dispense 30 tablets plus "3 repeats", then 953 * the order can be dispensed a total of 4 times and the patient 954 * can receive a total of 120 tablets. A prescriber may explicitly 955 * say that zero refills are permitted after the initial dispense. 956 */ 957 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowed(int value) { 958 if (this.numberOfRepeatsAllowed == null) 959 this.numberOfRepeatsAllowed = new UnsignedIntType(); 960 this.numberOfRepeatsAllowed.setValue(value); 961 return this; 962 } 963 964 /** 965 * @return {@link #quantity} (The amount that is to be dispensed for one fill.) 966 */ 967 public Quantity getQuantity() { 968 if (this.quantity == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.quantity"); 971 else if (Configuration.doAutoCreate()) 972 this.quantity = new Quantity(); // cc 973 return this.quantity; 974 } 975 976 public boolean hasQuantity() { 977 return this.quantity != null && !this.quantity.isEmpty(); 978 } 979 980 /** 981 * @param value {@link #quantity} (The amount that is to be dispensed for one 982 * fill.) 983 */ 984 public MedicationRequestDispenseRequestComponent setQuantity(Quantity value) { 985 this.quantity = value; 986 return this; 987 } 988 989 /** 990 * @return {@link #expectedSupplyDuration} (Identifies the period time over 991 * which the supplied product is expected to be used, or the length of 992 * time the dispense is expected to last.) 993 */ 994 public Duration getExpectedSupplyDuration() { 995 if (this.expectedSupplyDuration == null) 996 if (Configuration.errorOnAutoCreate()) 997 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.expectedSupplyDuration"); 998 else if (Configuration.doAutoCreate()) 999 this.expectedSupplyDuration = new Duration(); // cc 1000 return this.expectedSupplyDuration; 1001 } 1002 1003 public boolean hasExpectedSupplyDuration() { 1004 return this.expectedSupplyDuration != null && !this.expectedSupplyDuration.isEmpty(); 1005 } 1006 1007 /** 1008 * @param value {@link #expectedSupplyDuration} (Identifies the period time over 1009 * which the supplied product is expected to be used, or the length 1010 * of time the dispense is expected to last.) 1011 */ 1012 public MedicationRequestDispenseRequestComponent setExpectedSupplyDuration(Duration value) { 1013 this.expectedSupplyDuration = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #performer} (Indicates the intended dispensing Organization 1019 * specified by the prescriber.) 1020 */ 1021 public Reference getPerformer() { 1022 if (this.performer == null) 1023 if (Configuration.errorOnAutoCreate()) 1024 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.performer"); 1025 else if (Configuration.doAutoCreate()) 1026 this.performer = new Reference(); // cc 1027 return this.performer; 1028 } 1029 1030 public boolean hasPerformer() { 1031 return this.performer != null && !this.performer.isEmpty(); 1032 } 1033 1034 /** 1035 * @param value {@link #performer} (Indicates the intended dispensing 1036 * Organization specified by the prescriber.) 1037 */ 1038 public MedicationRequestDispenseRequestComponent setPerformer(Reference value) { 1039 this.performer = value; 1040 return this; 1041 } 1042 1043 /** 1044 * @return {@link #performer} The actual object that is the target of the 1045 * reference. The reference library doesn't populate this, but you can 1046 * use it to hold the resource if you resolve it. (Indicates the 1047 * intended dispensing Organization specified by the prescriber.) 1048 */ 1049 public Organization getPerformerTarget() { 1050 if (this.performerTarget == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.performer"); 1053 else if (Configuration.doAutoCreate()) 1054 this.performerTarget = new Organization(); // aa 1055 return this.performerTarget; 1056 } 1057 1058 /** 1059 * @param value {@link #performer} The actual object that is the target of the 1060 * reference. The reference library doesn't use these, but you can 1061 * use it to hold the resource if you resolve it. (Indicates the 1062 * intended dispensing Organization specified by the prescriber.) 1063 */ 1064 public MedicationRequestDispenseRequestComponent setPerformerTarget(Organization value) { 1065 this.performerTarget = value; 1066 return this; 1067 } 1068 1069 protected void listChildren(List<Property> children) { 1070 super.listChildren(children); 1071 children.add(new Property("initialFill", "", 1072 "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill)); 1073 children.add(new Property("dispenseInterval", "Duration", 1074 "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval)); 1075 children.add(new Property("validityPeriod", "Period", 1076 "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, 1077 validityPeriod)); 1078 children.add(new Property("numberOfRepeatsAllowed", "unsignedInt", 1079 "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 1080 0, 1, numberOfRepeatsAllowed)); 1081 children.add(new Property("quantity", "SimpleQuantity", "The amount that is to be dispensed for one fill.", 0, 1, 1082 quantity)); 1083 children.add(new Property("expectedSupplyDuration", "Duration", 1084 "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 1085 0, 1, expectedSupplyDuration)); 1086 children.add(new Property("performer", "Reference(Organization)", 1087 "Indicates the intended dispensing Organization specified by the prescriber.", 0, 1, performer)); 1088 } 1089 1090 @Override 1091 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1092 switch (_hash) { 1093 case 1232961255: 1094 /* initialFill */ return new Property("initialFill", "", 1095 "Indicates the quantity or duration for the first dispense of the medication.", 0, 1, initialFill); 1096 case 757112130: 1097 /* dispenseInterval */ return new Property("dispenseInterval", "Duration", 1098 "The minimum period of time that must occur between dispenses of the medication.", 0, 1, dispenseInterval); 1099 case -1434195053: 1100 /* validityPeriod */ return new Property("validityPeriod", "Period", 1101 "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, 1102 validityPeriod); 1103 case -239736976: 1104 /* numberOfRepeatsAllowed */ return new Property("numberOfRepeatsAllowed", "unsignedInt", 1105 "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. A prescriber may explicitly say that zero refills are permitted after the initial dispense.", 1106 0, 1, numberOfRepeatsAllowed); 1107 case -1285004149: 1108 /* quantity */ return new Property("quantity", "SimpleQuantity", 1109 "The amount that is to be dispensed for one fill.", 0, 1, quantity); 1110 case -1910182789: 1111 /* expectedSupplyDuration */ return new Property("expectedSupplyDuration", "Duration", 1112 "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 1113 0, 1, expectedSupplyDuration); 1114 case 481140686: 1115 /* performer */ return new Property("performer", "Reference(Organization)", 1116 "Indicates the intended dispensing Organization specified by the prescriber.", 0, 1, performer); 1117 default: 1118 return super.getNamedProperty(_hash, _name, _checkValid); 1119 } 1120 1121 } 1122 1123 @Override 1124 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1125 switch (hash) { 1126 case 1232961255: 1127 /* initialFill */ return this.initialFill == null ? new Base[0] : new Base[] { this.initialFill }; // MedicationRequestDispenseRequestInitialFillComponent 1128 case 757112130: 1129 /* dispenseInterval */ return this.dispenseInterval == null ? new Base[0] 1130 : new Base[] { this.dispenseInterval }; // Duration 1131 case -1434195053: 1132 /* validityPeriod */ return this.validityPeriod == null ? new Base[0] : new Base[] { this.validityPeriod }; // Period 1133 case -239736976: 1134 /* numberOfRepeatsAllowed */ return this.numberOfRepeatsAllowed == null ? new Base[0] 1135 : new Base[] { this.numberOfRepeatsAllowed }; // UnsignedIntType 1136 case -1285004149: 1137 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1138 case -1910182789: 1139 /* expectedSupplyDuration */ return this.expectedSupplyDuration == null ? new Base[0] 1140 : new Base[] { this.expectedSupplyDuration }; // Duration 1141 case 481140686: 1142 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 1143 default: 1144 return super.getProperty(hash, name, checkValid); 1145 } 1146 1147 } 1148 1149 @Override 1150 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1151 switch (hash) { 1152 case 1232961255: // initialFill 1153 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 1154 return value; 1155 case 757112130: // dispenseInterval 1156 this.dispenseInterval = castToDuration(value); // Duration 1157 return value; 1158 case -1434195053: // validityPeriod 1159 this.validityPeriod = castToPeriod(value); // Period 1160 return value; 1161 case -239736976: // numberOfRepeatsAllowed 1162 this.numberOfRepeatsAllowed = castToUnsignedInt(value); // UnsignedIntType 1163 return value; 1164 case -1285004149: // quantity 1165 this.quantity = castToQuantity(value); // Quantity 1166 return value; 1167 case -1910182789: // expectedSupplyDuration 1168 this.expectedSupplyDuration = castToDuration(value); // Duration 1169 return value; 1170 case 481140686: // performer 1171 this.performer = castToReference(value); // Reference 1172 return value; 1173 default: 1174 return super.setProperty(hash, name, value); 1175 } 1176 1177 } 1178 1179 @Override 1180 public Base setProperty(String name, Base value) throws FHIRException { 1181 if (name.equals("initialFill")) { 1182 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 1183 } else if (name.equals("dispenseInterval")) { 1184 this.dispenseInterval = castToDuration(value); // Duration 1185 } else if (name.equals("validityPeriod")) { 1186 this.validityPeriod = castToPeriod(value); // Period 1187 } else if (name.equals("numberOfRepeatsAllowed")) { 1188 this.numberOfRepeatsAllowed = castToUnsignedInt(value); // UnsignedIntType 1189 } else if (name.equals("quantity")) { 1190 this.quantity = castToQuantity(value); // Quantity 1191 } else if (name.equals("expectedSupplyDuration")) { 1192 this.expectedSupplyDuration = castToDuration(value); // Duration 1193 } else if (name.equals("performer")) { 1194 this.performer = castToReference(value); // Reference 1195 } else 1196 return super.setProperty(name, value); 1197 return value; 1198 } 1199 1200 @Override 1201 public void removeChild(String name, Base value) throws FHIRException { 1202 if (name.equals("initialFill")) { 1203 this.initialFill = (MedicationRequestDispenseRequestInitialFillComponent) value; // MedicationRequestDispenseRequestInitialFillComponent 1204 } else if (name.equals("dispenseInterval")) { 1205 this.dispenseInterval = null; 1206 } else if (name.equals("validityPeriod")) { 1207 this.validityPeriod = null; 1208 } else if (name.equals("numberOfRepeatsAllowed")) { 1209 this.numberOfRepeatsAllowed = null; 1210 } else if (name.equals("quantity")) { 1211 this.quantity = null; 1212 } else if (name.equals("expectedSupplyDuration")) { 1213 this.expectedSupplyDuration = null; 1214 } else if (name.equals("performer")) { 1215 this.performer = null; 1216 } else 1217 super.removeChild(name, value); 1218 1219 } 1220 1221 @Override 1222 public Base makeProperty(int hash, String name) throws FHIRException { 1223 switch (hash) { 1224 case 1232961255: 1225 return getInitialFill(); 1226 case 757112130: 1227 return getDispenseInterval(); 1228 case -1434195053: 1229 return getValidityPeriod(); 1230 case -239736976: 1231 return getNumberOfRepeatsAllowedElement(); 1232 case -1285004149: 1233 return getQuantity(); 1234 case -1910182789: 1235 return getExpectedSupplyDuration(); 1236 case 481140686: 1237 return getPerformer(); 1238 default: 1239 return super.makeProperty(hash, name); 1240 } 1241 1242 } 1243 1244 @Override 1245 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1246 switch (hash) { 1247 case 1232961255: 1248 /* initialFill */ return new String[] {}; 1249 case 757112130: 1250 /* dispenseInterval */ return new String[] { "Duration" }; 1251 case -1434195053: 1252 /* validityPeriod */ return new String[] { "Period" }; 1253 case -239736976: 1254 /* numberOfRepeatsAllowed */ return new String[] { "unsignedInt" }; 1255 case -1285004149: 1256 /* quantity */ return new String[] { "SimpleQuantity" }; 1257 case -1910182789: 1258 /* expectedSupplyDuration */ return new String[] { "Duration" }; 1259 case 481140686: 1260 /* performer */ return new String[] { "Reference" }; 1261 default: 1262 return super.getTypesForProperty(hash, name); 1263 } 1264 1265 } 1266 1267 @Override 1268 public Base addChild(String name) throws FHIRException { 1269 if (name.equals("initialFill")) { 1270 this.initialFill = new MedicationRequestDispenseRequestInitialFillComponent(); 1271 return this.initialFill; 1272 } else if (name.equals("dispenseInterval")) { 1273 this.dispenseInterval = new Duration(); 1274 return this.dispenseInterval; 1275 } else if (name.equals("validityPeriod")) { 1276 this.validityPeriod = new Period(); 1277 return this.validityPeriod; 1278 } else if (name.equals("numberOfRepeatsAllowed")) { 1279 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.numberOfRepeatsAllowed"); 1280 } else if (name.equals("quantity")) { 1281 this.quantity = new Quantity(); 1282 return this.quantity; 1283 } else if (name.equals("expectedSupplyDuration")) { 1284 this.expectedSupplyDuration = new Duration(); 1285 return this.expectedSupplyDuration; 1286 } else if (name.equals("performer")) { 1287 this.performer = new Reference(); 1288 return this.performer; 1289 } else 1290 return super.addChild(name); 1291 } 1292 1293 public MedicationRequestDispenseRequestComponent copy() { 1294 MedicationRequestDispenseRequestComponent dst = new MedicationRequestDispenseRequestComponent(); 1295 copyValues(dst); 1296 return dst; 1297 } 1298 1299 public void copyValues(MedicationRequestDispenseRequestComponent dst) { 1300 super.copyValues(dst); 1301 dst.initialFill = initialFill == null ? null : initialFill.copy(); 1302 dst.dispenseInterval = dispenseInterval == null ? null : dispenseInterval.copy(); 1303 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1304 dst.numberOfRepeatsAllowed = numberOfRepeatsAllowed == null ? null : numberOfRepeatsAllowed.copy(); 1305 dst.quantity = quantity == null ? null : quantity.copy(); 1306 dst.expectedSupplyDuration = expectedSupplyDuration == null ? null : expectedSupplyDuration.copy(); 1307 dst.performer = performer == null ? null : performer.copy(); 1308 } 1309 1310 @Override 1311 public boolean equalsDeep(Base other_) { 1312 if (!super.equalsDeep(other_)) 1313 return false; 1314 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1315 return false; 1316 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1317 return compareDeep(initialFill, o.initialFill, true) && compareDeep(dispenseInterval, o.dispenseInterval, true) 1318 && compareDeep(validityPeriod, o.validityPeriod, true) 1319 && compareDeep(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true) 1320 && compareDeep(quantity, o.quantity, true) 1321 && compareDeep(expectedSupplyDuration, o.expectedSupplyDuration, true) 1322 && compareDeep(performer, o.performer, true); 1323 } 1324 1325 @Override 1326 public boolean equalsShallow(Base other_) { 1327 if (!super.equalsShallow(other_)) 1328 return false; 1329 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1330 return false; 1331 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1332 return compareValues(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true); 1333 } 1334 1335 public boolean isEmpty() { 1336 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(initialFill, dispenseInterval, validityPeriod, 1337 numberOfRepeatsAllowed, quantity, expectedSupplyDuration, performer); 1338 } 1339 1340 public String fhirType() { 1341 return "MedicationRequest.dispenseRequest"; 1342 1343 } 1344 1345 } 1346 1347 @Block() 1348 public static class MedicationRequestDispenseRequestInitialFillComponent extends BackboneElement 1349 implements IBaseBackboneElement { 1350 /** 1351 * The amount or quantity to provide as part of the first dispense. 1352 */ 1353 @Child(name = "quantity", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1354 @Description(shortDefinition = "First fill quantity", formalDefinition = "The amount or quantity to provide as part of the first dispense.") 1355 protected Quantity quantity; 1356 1357 /** 1358 * The length of time that the first dispense is expected to last. 1359 */ 1360 @Child(name = "duration", type = { Duration.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1361 @Description(shortDefinition = "First fill duration", formalDefinition = "The length of time that the first dispense is expected to last.") 1362 protected Duration duration; 1363 1364 private static final long serialVersionUID = 1223227956L; 1365 1366 /** 1367 * Constructor 1368 */ 1369 public MedicationRequestDispenseRequestInitialFillComponent() { 1370 super(); 1371 } 1372 1373 /** 1374 * @return {@link #quantity} (The amount or quantity to provide as part of the 1375 * first dispense.) 1376 */ 1377 public Quantity getQuantity() { 1378 if (this.quantity == null) 1379 if (Configuration.errorOnAutoCreate()) 1380 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.quantity"); 1381 else if (Configuration.doAutoCreate()) 1382 this.quantity = new Quantity(); // cc 1383 return this.quantity; 1384 } 1385 1386 public boolean hasQuantity() { 1387 return this.quantity != null && !this.quantity.isEmpty(); 1388 } 1389 1390 /** 1391 * @param value {@link #quantity} (The amount or quantity to provide as part of 1392 * the first dispense.) 1393 */ 1394 public MedicationRequestDispenseRequestInitialFillComponent setQuantity(Quantity value) { 1395 this.quantity = value; 1396 return this; 1397 } 1398 1399 /** 1400 * @return {@link #duration} (The length of time that the first dispense is 1401 * expected to last.) 1402 */ 1403 public Duration getDuration() { 1404 if (this.duration == null) 1405 if (Configuration.errorOnAutoCreate()) 1406 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestInitialFillComponent.duration"); 1407 else if (Configuration.doAutoCreate()) 1408 this.duration = new Duration(); // cc 1409 return this.duration; 1410 } 1411 1412 public boolean hasDuration() { 1413 return this.duration != null && !this.duration.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #duration} (The length of time that the first dispense is 1418 * expected to last.) 1419 */ 1420 public MedicationRequestDispenseRequestInitialFillComponent setDuration(Duration value) { 1421 this.duration = value; 1422 return this; 1423 } 1424 1425 protected void listChildren(List<Property> children) { 1426 super.listChildren(children); 1427 children.add(new Property("quantity", "SimpleQuantity", 1428 "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity)); 1429 children.add(new Property("duration", "Duration", 1430 "The length of time that the first dispense is expected to last.", 0, 1, duration)); 1431 } 1432 1433 @Override 1434 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1435 switch (_hash) { 1436 case -1285004149: 1437 /* quantity */ return new Property("quantity", "SimpleQuantity", 1438 "The amount or quantity to provide as part of the first dispense.", 0, 1, quantity); 1439 case -1992012396: 1440 /* duration */ return new Property("duration", "Duration", 1441 "The length of time that the first dispense is expected to last.", 0, 1, duration); 1442 default: 1443 return super.getNamedProperty(_hash, _name, _checkValid); 1444 } 1445 1446 } 1447 1448 @Override 1449 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1450 switch (hash) { 1451 case -1285004149: 1452 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 1453 case -1992012396: 1454 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Duration 1455 default: 1456 return super.getProperty(hash, name, checkValid); 1457 } 1458 1459 } 1460 1461 @Override 1462 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1463 switch (hash) { 1464 case -1285004149: // quantity 1465 this.quantity = castToQuantity(value); // Quantity 1466 return value; 1467 case -1992012396: // duration 1468 this.duration = castToDuration(value); // Duration 1469 return value; 1470 default: 1471 return super.setProperty(hash, name, value); 1472 } 1473 1474 } 1475 1476 @Override 1477 public Base setProperty(String name, Base value) throws FHIRException { 1478 if (name.equals("quantity")) { 1479 this.quantity = castToQuantity(value); // Quantity 1480 } else if (name.equals("duration")) { 1481 this.duration = castToDuration(value); // Duration 1482 } else 1483 return super.setProperty(name, value); 1484 return value; 1485 } 1486 1487 @Override 1488 public void removeChild(String name, Base value) throws FHIRException { 1489 if (name.equals("quantity")) { 1490 this.quantity = null; 1491 } else if (name.equals("duration")) { 1492 this.duration = null; 1493 } else 1494 super.removeChild(name, value); 1495 1496 } 1497 1498 @Override 1499 public Base makeProperty(int hash, String name) throws FHIRException { 1500 switch (hash) { 1501 case -1285004149: 1502 return getQuantity(); 1503 case -1992012396: 1504 return getDuration(); 1505 default: 1506 return super.makeProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1513 switch (hash) { 1514 case -1285004149: 1515 /* quantity */ return new String[] { "SimpleQuantity" }; 1516 case -1992012396: 1517 /* duration */ return new String[] { "Duration" }; 1518 default: 1519 return super.getTypesForProperty(hash, name); 1520 } 1521 1522 } 1523 1524 @Override 1525 public Base addChild(String name) throws FHIRException { 1526 if (name.equals("quantity")) { 1527 this.quantity = new Quantity(); 1528 return this.quantity; 1529 } else if (name.equals("duration")) { 1530 this.duration = new Duration(); 1531 return this.duration; 1532 } else 1533 return super.addChild(name); 1534 } 1535 1536 public MedicationRequestDispenseRequestInitialFillComponent copy() { 1537 MedicationRequestDispenseRequestInitialFillComponent dst = new MedicationRequestDispenseRequestInitialFillComponent(); 1538 copyValues(dst); 1539 return dst; 1540 } 1541 1542 public void copyValues(MedicationRequestDispenseRequestInitialFillComponent dst) { 1543 super.copyValues(dst); 1544 dst.quantity = quantity == null ? null : quantity.copy(); 1545 dst.duration = duration == null ? null : duration.copy(); 1546 } 1547 1548 @Override 1549 public boolean equalsDeep(Base other_) { 1550 if (!super.equalsDeep(other_)) 1551 return false; 1552 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1553 return false; 1554 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1555 return compareDeep(quantity, o.quantity, true) && compareDeep(duration, o.duration, true); 1556 } 1557 1558 @Override 1559 public boolean equalsShallow(Base other_) { 1560 if (!super.equalsShallow(other_)) 1561 return false; 1562 if (!(other_ instanceof MedicationRequestDispenseRequestInitialFillComponent)) 1563 return false; 1564 MedicationRequestDispenseRequestInitialFillComponent o = (MedicationRequestDispenseRequestInitialFillComponent) other_; 1565 return true; 1566 } 1567 1568 public boolean isEmpty() { 1569 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, duration); 1570 } 1571 1572 public String fhirType() { 1573 return "MedicationRequest.dispenseRequest.initialFill"; 1574 1575 } 1576 1577 } 1578 1579 @Block() 1580 public static class MedicationRequestSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 1581 /** 1582 * True if the prescriber allows a different drug to be dispensed from what was 1583 * prescribed. 1584 */ 1585 @Child(name = "allowed", type = { BooleanType.class, 1586 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 1587 @Description(shortDefinition = "Whether substitution is allowed or not", formalDefinition = "True if the prescriber allows a different drug to be dispensed from what was prescribed.") 1588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 1589 protected Type allowed; 1590 1591 /** 1592 * Indicates the reason for the substitution, or why substitution must or must 1593 * not be performed. 1594 */ 1595 @Child(name = "reason", type = { 1596 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1597 @Description(shortDefinition = "Why should (not) substitution be made", formalDefinition = "Indicates the reason for the substitution, or why substitution must or must not be performed.") 1598 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://terminology.hl7.org/ValueSet/v3-SubstanceAdminSubstitutionReason") 1599 protected CodeableConcept reason; 1600 1601 private static final long serialVersionUID = 547265407L; 1602 1603 /** 1604 * Constructor 1605 */ 1606 public MedicationRequestSubstitutionComponent() { 1607 super(); 1608 } 1609 1610 /** 1611 * Constructor 1612 */ 1613 public MedicationRequestSubstitutionComponent(Type allowed) { 1614 super(); 1615 this.allowed = allowed; 1616 } 1617 1618 /** 1619 * @return {@link #allowed} (True if the prescriber allows a different drug to 1620 * be dispensed from what was prescribed.) 1621 */ 1622 public Type getAllowed() { 1623 return this.allowed; 1624 } 1625 1626 /** 1627 * @return {@link #allowed} (True if the prescriber allows a different drug to 1628 * be dispensed from what was prescribed.) 1629 */ 1630 public BooleanType getAllowedBooleanType() throws FHIRException { 1631 if (this.allowed == null) 1632 this.allowed = new BooleanType(); 1633 if (!(this.allowed instanceof BooleanType)) 1634 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1635 + this.allowed.getClass().getName() + " was encountered"); 1636 return (BooleanType) this.allowed; 1637 } 1638 1639 public boolean hasAllowedBooleanType() { 1640 return this.allowed instanceof BooleanType; 1641 } 1642 1643 /** 1644 * @return {@link #allowed} (True if the prescriber allows a different drug to 1645 * be dispensed from what was prescribed.) 1646 */ 1647 public CodeableConcept getAllowedCodeableConcept() throws FHIRException { 1648 if (this.allowed == null) 1649 this.allowed = new CodeableConcept(); 1650 if (!(this.allowed instanceof CodeableConcept)) 1651 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1652 + this.allowed.getClass().getName() + " was encountered"); 1653 return (CodeableConcept) this.allowed; 1654 } 1655 1656 public boolean hasAllowedCodeableConcept() { 1657 return this.allowed instanceof CodeableConcept; 1658 } 1659 1660 public boolean hasAllowed() { 1661 return this.allowed != null && !this.allowed.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #allowed} (True if the prescriber allows a different drug 1666 * to be dispensed from what was prescribed.) 1667 */ 1668 public MedicationRequestSubstitutionComponent setAllowed(Type value) { 1669 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 1670 throw new Error("Not the right type for MedicationRequest.substitution.allowed[x]: " + value.fhirType()); 1671 this.allowed = value; 1672 return this; 1673 } 1674 1675 /** 1676 * @return {@link #reason} (Indicates the reason for the substitution, or why 1677 * substitution must or must not be performed.) 1678 */ 1679 public CodeableConcept getReason() { 1680 if (this.reason == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create MedicationRequestSubstitutionComponent.reason"); 1683 else if (Configuration.doAutoCreate()) 1684 this.reason = new CodeableConcept(); // cc 1685 return this.reason; 1686 } 1687 1688 public boolean hasReason() { 1689 return this.reason != null && !this.reason.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #reason} (Indicates the reason for the substitution, or 1694 * why substitution must or must not be performed.) 1695 */ 1696 public MedicationRequestSubstitutionComponent setReason(CodeableConcept value) { 1697 this.reason = value; 1698 return this; 1699 } 1700 1701 protected void listChildren(List<Property> children) { 1702 super.listChildren(children); 1703 children.add(new Property("allowed[x]", "boolean|CodeableConcept", 1704 "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed)); 1705 children.add(new Property("reason", "CodeableConcept", 1706 "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, 1707 reason)); 1708 } 1709 1710 @Override 1711 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1712 switch (_hash) { 1713 case -1336663592: 1714 /* allowed[x] */ return new Property("allowed[x]", "boolean|CodeableConcept", 1715 "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1716 case -911343192: 1717 /* allowed */ return new Property("allowed[x]", "boolean|CodeableConcept", 1718 "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1719 case 177755488: 1720 /* allowedBoolean */ return new Property("allowed[x]", "boolean|CodeableConcept", 1721 "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1722 case 963125465: 1723 /* allowedCodeableConcept */ return new Property("allowed[x]", "boolean|CodeableConcept", 1724 "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1725 case -934964668: 1726 /* reason */ return new Property("reason", "CodeableConcept", 1727 "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, 1728 reason); 1729 default: 1730 return super.getNamedProperty(_hash, _name, _checkValid); 1731 } 1732 1733 } 1734 1735 @Override 1736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1737 switch (hash) { 1738 case -911343192: 1739 /* allowed */ return this.allowed == null ? new Base[0] : new Base[] { this.allowed }; // Type 1740 case -934964668: 1741 /* reason */ return this.reason == null ? new Base[0] : new Base[] { this.reason }; // CodeableConcept 1742 default: 1743 return super.getProperty(hash, name, checkValid); 1744 } 1745 1746 } 1747 1748 @Override 1749 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1750 switch (hash) { 1751 case -911343192: // allowed 1752 this.allowed = castToType(value); // Type 1753 return value; 1754 case -934964668: // reason 1755 this.reason = castToCodeableConcept(value); // CodeableConcept 1756 return value; 1757 default: 1758 return super.setProperty(hash, name, value); 1759 } 1760 1761 } 1762 1763 @Override 1764 public Base setProperty(String name, Base value) throws FHIRException { 1765 if (name.equals("allowed[x]")) { 1766 this.allowed = castToType(value); // Type 1767 } else if (name.equals("reason")) { 1768 this.reason = castToCodeableConcept(value); // CodeableConcept 1769 } else 1770 return super.setProperty(name, value); 1771 return value; 1772 } 1773 1774 @Override 1775 public void removeChild(String name, Base value) throws FHIRException { 1776 if (name.equals("allowed[x]")) { 1777 this.allowed = null; 1778 } else if (name.equals("reason")) { 1779 this.reason = null; 1780 } else 1781 super.removeChild(name, value); 1782 1783 } 1784 1785 @Override 1786 public Base makeProperty(int hash, String name) throws FHIRException { 1787 switch (hash) { 1788 case -1336663592: 1789 return getAllowed(); 1790 case -911343192: 1791 return getAllowed(); 1792 case -934964668: 1793 return getReason(); 1794 default: 1795 return super.makeProperty(hash, name); 1796 } 1797 1798 } 1799 1800 @Override 1801 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1802 switch (hash) { 1803 case -911343192: 1804 /* allowed */ return new String[] { "boolean", "CodeableConcept" }; 1805 case -934964668: 1806 /* reason */ return new String[] { "CodeableConcept" }; 1807 default: 1808 return super.getTypesForProperty(hash, name); 1809 } 1810 1811 } 1812 1813 @Override 1814 public Base addChild(String name) throws FHIRException { 1815 if (name.equals("allowedBoolean")) { 1816 this.allowed = new BooleanType(); 1817 return this.allowed; 1818 } else if (name.equals("allowedCodeableConcept")) { 1819 this.allowed = new CodeableConcept(); 1820 return this.allowed; 1821 } else if (name.equals("reason")) { 1822 this.reason = new CodeableConcept(); 1823 return this.reason; 1824 } else 1825 return super.addChild(name); 1826 } 1827 1828 public MedicationRequestSubstitutionComponent copy() { 1829 MedicationRequestSubstitutionComponent dst = new MedicationRequestSubstitutionComponent(); 1830 copyValues(dst); 1831 return dst; 1832 } 1833 1834 public void copyValues(MedicationRequestSubstitutionComponent dst) { 1835 super.copyValues(dst); 1836 dst.allowed = allowed == null ? null : allowed.copy(); 1837 dst.reason = reason == null ? null : reason.copy(); 1838 } 1839 1840 @Override 1841 public boolean equalsDeep(Base other_) { 1842 if (!super.equalsDeep(other_)) 1843 return false; 1844 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1845 return false; 1846 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1847 return compareDeep(allowed, o.allowed, true) && compareDeep(reason, o.reason, true); 1848 } 1849 1850 @Override 1851 public boolean equalsShallow(Base other_) { 1852 if (!super.equalsShallow(other_)) 1853 return false; 1854 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1855 return false; 1856 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1857 return true; 1858 } 1859 1860 public boolean isEmpty() { 1861 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(allowed, reason); 1862 } 1863 1864 public String fhirType() { 1865 return "MedicationRequest.substitution"; 1866 1867 } 1868 1869 } 1870 1871 /** 1872 * Identifiers associated with this medication request that are defined by 1873 * business processes and/or used to refer to it when a direct URL reference to 1874 * the resource itself is not appropriate. They are business identifiers 1875 * assigned to this resource by the performer or other systems and remain 1876 * constant as the resource is updated and propagates from server to server. 1877 */ 1878 @Child(name = "identifier", type = { 1879 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1880 @Description(shortDefinition = "External ids for this request", formalDefinition = "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.") 1881 protected List<Identifier> identifier; 1882 1883 /** 1884 * A code specifying the current state of the order. Generally, this will be 1885 * active or completed state. 1886 */ 1887 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 1888 @Description(shortDefinition = "active | on-hold | cancelled | completed | entered-in-error | stopped | draft | unknown", formalDefinition = "A code specifying the current state of the order. Generally, this will be active or completed state.") 1889 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationrequest-status") 1890 protected Enumeration<MedicationRequestStatus> status; 1891 1892 /** 1893 * Captures the reason for the current state of the MedicationRequest. 1894 */ 1895 @Child(name = "statusReason", type = { 1896 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1897 @Description(shortDefinition = "Reason for current status", formalDefinition = "Captures the reason for the current state of the MedicationRequest.") 1898 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationrequest-status-reason") 1899 protected CodeableConcept statusReason; 1900 1901 /** 1902 * Whether the request is a proposal, plan, or an original order. 1903 */ 1904 @Child(name = "intent", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 1905 @Description(shortDefinition = "proposal | plan | order | original-order | reflex-order | filler-order | instance-order | option", formalDefinition = "Whether the request is a proposal, plan, or an original order.") 1906 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationrequest-intent") 1907 protected Enumeration<MedicationRequestIntent> intent; 1908 1909 /** 1910 * Indicates the type of medication request (for example, where the medication 1911 * is expected to be consumed or administered (i.e. inpatient or outpatient)). 1912 */ 1913 @Child(name = "category", type = { 1914 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1915 @Description(shortDefinition = "Type of medication usage", formalDefinition = "Indicates the type of medication request (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).") 1916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationrequest-category") 1917 protected List<CodeableConcept> category; 1918 1919 /** 1920 * Indicates how quickly the Medication Request should be addressed with respect 1921 * to other requests. 1922 */ 1923 @Child(name = "priority", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1924 @Description(shortDefinition = "routine | urgent | asap | stat", formalDefinition = "Indicates how quickly the Medication Request should be addressed with respect to other requests.") 1925 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/request-priority") 1926 protected Enumeration<MedicationRequestPriority> priority; 1927 1928 /** 1929 * If true indicates that the provider is asking for the medication request not 1930 * to occur. 1931 */ 1932 @Child(name = "doNotPerform", type = { 1933 BooleanType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 1934 @Description(shortDefinition = "True if request is prohibiting action", formalDefinition = "If true indicates that the provider is asking for the medication request not to occur.") 1935 protected BooleanType doNotPerform; 1936 1937 /** 1938 * Indicates if this record was captured as a secondary 'reported' record rather 1939 * than as an original primary source-of-truth record. It may also indicate the 1940 * source of the report. 1941 */ 1942 @Child(name = "reported", type = { BooleanType.class, Patient.class, Practitioner.class, PractitionerRole.class, 1943 RelatedPerson.class, Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1944 @Description(shortDefinition = "Reported rather than primary record", formalDefinition = "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.") 1945 protected Type reported; 1946 1947 /** 1948 * Identifies the medication being requested. This is a link to a resource that 1949 * represents the medication which may be the details of the medication or 1950 * simply an attribute carrying a code that identifies the medication from a 1951 * known list of medications. 1952 */ 1953 @Child(name = "medication", type = { CodeableConcept.class, 1954 Medication.class }, order = 8, min = 1, max = 1, modifier = false, summary = true) 1955 @Description(shortDefinition = "Medication to be taken", formalDefinition = "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.") 1956 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medication-codes") 1957 protected Type medication; 1958 1959 /** 1960 * A link to a resource representing the person or set of individuals to whom 1961 * the medication will be given. 1962 */ 1963 @Child(name = "subject", type = { Patient.class, 1964 Group.class }, order = 9, min = 1, max = 1, modifier = false, summary = true) 1965 @Description(shortDefinition = "Who or group medication request is for", formalDefinition = "A link to a resource representing the person or set of individuals to whom the medication will be given.") 1966 protected Reference subject; 1967 1968 /** 1969 * The actual object that is the target of the reference (A link to a resource 1970 * representing the person or set of individuals to whom the medication will be 1971 * given.) 1972 */ 1973 protected Resource subjectTarget; 1974 1975 /** 1976 * The Encounter during which this [x] was created or to which the creation of 1977 * this record is tightly associated. 1978 */ 1979 @Child(name = "encounter", type = { 1980 Encounter.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1981 @Description(shortDefinition = "Encounter created as part of encounter/admission/stay", formalDefinition = "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.") 1982 protected Reference encounter; 1983 1984 /** 1985 * The actual object that is the target of the reference (The Encounter during 1986 * which this [x] was created or to which the creation of this record is tightly 1987 * associated.) 1988 */ 1989 protected Encounter encounterTarget; 1990 1991 /** 1992 * Include additional information (for example, patient height and weight) that 1993 * supports the ordering of the medication. 1994 */ 1995 @Child(name = "supportingInformation", type = { 1996 Reference.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1997 @Description(shortDefinition = "Information to support ordering of the medication", formalDefinition = "Include additional information (for example, patient height and weight) that supports the ordering of the medication.") 1998 protected List<Reference> supportingInformation; 1999 /** 2000 * The actual objects that are the target of the reference (Include additional 2001 * information (for example, patient height and weight) that supports the 2002 * ordering of the medication.) 2003 */ 2004 protected List<Resource> supportingInformationTarget; 2005 2006 /** 2007 * The date (and perhaps time) when the prescription was initially written or 2008 * authored on. 2009 */ 2010 @Child(name = "authoredOn", type = { 2011 DateTimeType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2012 @Description(shortDefinition = "When request was initially authored", formalDefinition = "The date (and perhaps time) when the prescription was initially written or authored on.") 2013 protected DateTimeType authoredOn; 2014 2015 /** 2016 * The individual, organization, or device that initiated the request and has 2017 * responsibility for its activation. 2018 */ 2019 @Child(name = "requester", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 2020 RelatedPerson.class, Device.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 2021 @Description(shortDefinition = "Who/What requested the Request", formalDefinition = "The individual, organization, or device that initiated the request and has responsibility for its activation.") 2022 protected Reference requester; 2023 2024 /** 2025 * The actual object that is the target of the reference (The individual, 2026 * organization, or device that initiated the request and has responsibility for 2027 * its activation.) 2028 */ 2029 protected Resource requesterTarget; 2030 2031 /** 2032 * The specified desired performer of the medication treatment (e.g. the 2033 * performer of the medication administration). 2034 */ 2035 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, 2036 Device.class, RelatedPerson.class, 2037 CareTeam.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 2038 @Description(shortDefinition = "Intended performer of administration", formalDefinition = "The specified desired performer of the medication treatment (e.g. the performer of the medication administration).") 2039 protected Reference performer; 2040 2041 /** 2042 * The actual object that is the target of the reference (The specified desired 2043 * performer of the medication treatment (e.g. the performer of the medication 2044 * administration).) 2045 */ 2046 protected Resource performerTarget; 2047 2048 /** 2049 * Indicates the type of performer of the administration of the medication. 2050 */ 2051 @Child(name = "performerType", type = { 2052 CodeableConcept.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 2053 @Description(shortDefinition = "Desired kind of performer of the medication administration", formalDefinition = "Indicates the type of performer of the administration of the medication.") 2054 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/performer-role") 2055 protected CodeableConcept performerType; 2056 2057 /** 2058 * The person who entered the order on behalf of another individual for example 2059 * in the case of a verbal or a telephone order. 2060 */ 2061 @Child(name = "recorder", type = { Practitioner.class, 2062 PractitionerRole.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 2063 @Description(shortDefinition = "Person who entered the request", formalDefinition = "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.") 2064 protected Reference recorder; 2065 2066 /** 2067 * The actual object that is the target of the reference (The person who entered 2068 * the order on behalf of another individual for example in the case of a verbal 2069 * or a telephone order.) 2070 */ 2071 protected Resource recorderTarget; 2072 2073 /** 2074 * The reason or the indication for ordering or not ordering the medication. 2075 */ 2076 @Child(name = "reasonCode", type = { 2077 CodeableConcept.class }, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2078 @Description(shortDefinition = "Reason or indication for ordering or not ordering the medication", formalDefinition = "The reason or the indication for ordering or not ordering the medication.") 2079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/condition-code") 2080 protected List<CodeableConcept> reasonCode; 2081 2082 /** 2083 * Condition or observation that supports why the medication was ordered. 2084 */ 2085 @Child(name = "reasonReference", type = { Condition.class, 2086 Observation.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2087 @Description(shortDefinition = "Condition or observation that supports why the prescription is being written", formalDefinition = "Condition or observation that supports why the medication was ordered.") 2088 protected List<Reference> reasonReference; 2089 /** 2090 * The actual objects that are the target of the reference (Condition or 2091 * observation that supports why the medication was ordered.) 2092 */ 2093 protected List<Resource> reasonReferenceTarget; 2094 2095 /** 2096 * The URL pointing to a protocol, guideline, orderset, or other definition that 2097 * is adhered to in whole or in part by this MedicationRequest. 2098 */ 2099 @Child(name = "instantiatesCanonical", type = { 2100 CanonicalType.class }, order = 19, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2101 @Description(shortDefinition = "Instantiates FHIR protocol or definition", formalDefinition = "The URL pointing to a protocol, guideline, orderset, or other definition that is adhered to in whole or in part by this MedicationRequest.") 2102 protected List<CanonicalType> instantiatesCanonical; 2103 2104 /** 2105 * The URL pointing to an externally maintained protocol, guideline, orderset or 2106 * other definition that is adhered to in whole or in part by this 2107 * MedicationRequest. 2108 */ 2109 @Child(name = "instantiatesUri", type = { 2110 UriType.class }, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2111 @Description(shortDefinition = "Instantiates external protocol or definition", formalDefinition = "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this MedicationRequest.") 2112 protected List<UriType> instantiatesUri; 2113 2114 /** 2115 * A plan or request that is fulfilled in whole or in part by this medication 2116 * request. 2117 */ 2118 @Child(name = "basedOn", type = { CarePlan.class, MedicationRequest.class, ServiceRequest.class, 2119 ImmunizationRecommendation.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2120 @Description(shortDefinition = "What request fulfills", formalDefinition = "A plan or request that is fulfilled in whole or in part by this medication request.") 2121 protected List<Reference> basedOn; 2122 /** 2123 * The actual objects that are the target of the reference (A plan or request 2124 * that is fulfilled in whole or in part by this medication request.) 2125 */ 2126 protected List<Resource> basedOnTarget; 2127 2128 /** 2129 * A shared identifier common to all requests that were authorized more or less 2130 * simultaneously by a single author, representing the identifier of the 2131 * requisition or prescription. 2132 */ 2133 @Child(name = "groupIdentifier", type = { 2134 Identifier.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 2135 @Description(shortDefinition = "Composite request this is part of", formalDefinition = "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.") 2136 protected Identifier groupIdentifier; 2137 2138 /** 2139 * The description of the overall patte3rn of the administration of the 2140 * medication to the patient. 2141 */ 2142 @Child(name = "courseOfTherapyType", type = { 2143 CodeableConcept.class }, order = 23, min = 0, max = 1, modifier = false, summary = false) 2144 @Description(shortDefinition = "Overall pattern of medication administration", formalDefinition = "The description of the overall patte3rn of the administration of the medication to the patient.") 2145 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/medicationrequest-course-of-therapy") 2146 protected CodeableConcept courseOfTherapyType; 2147 2148 /** 2149 * Insurance plans, coverage extensions, pre-authorizations and/or 2150 * pre-determinations that may be required for delivering the requested service. 2151 */ 2152 @Child(name = "insurance", type = { Coverage.class, 2153 ClaimResponse.class }, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2154 @Description(shortDefinition = "Associated insurance coverage", formalDefinition = "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.") 2155 protected List<Reference> insurance; 2156 /** 2157 * The actual objects that are the target of the reference (Insurance plans, 2158 * coverage extensions, pre-authorizations and/or pre-determinations that may be 2159 * required for delivering the requested service.) 2160 */ 2161 protected List<Resource> insuranceTarget; 2162 2163 /** 2164 * Extra information about the prescription that could not be conveyed by the 2165 * other attributes. 2166 */ 2167 @Child(name = "note", type = { 2168 Annotation.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2169 @Description(shortDefinition = "Information about the prescription", formalDefinition = "Extra information about the prescription that could not be conveyed by the other attributes.") 2170 protected List<Annotation> note; 2171 2172 /** 2173 * Indicates how the medication is to be used by the patient. 2174 */ 2175 @Child(name = "dosageInstruction", type = { 2176 Dosage.class }, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2177 @Description(shortDefinition = "How the medication should be taken", formalDefinition = "Indicates how the medication is to be used by the patient.") 2178 protected List<Dosage> dosageInstruction; 2179 2180 /** 2181 * Indicates the specific details for the dispense or medication supply part of 2182 * a medication request (also known as a Medication Prescription or Medication 2183 * Order). Note that this information is not always sent with the order. There 2184 * may be in some settings (e.g. hospitals) institutional or system support for 2185 * completing the dispense details in the pharmacy department. 2186 */ 2187 @Child(name = "dispenseRequest", type = {}, order = 27, min = 0, max = 1, modifier = false, summary = false) 2188 @Description(shortDefinition = "Medication supply authorization", formalDefinition = "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.") 2189 protected MedicationRequestDispenseRequestComponent dispenseRequest; 2190 2191 /** 2192 * Indicates whether or not substitution can or should be part of the dispense. 2193 * In some cases, substitution must happen, in other cases substitution must not 2194 * happen. This block explains the prescriber's intent. If nothing is specified 2195 * substitution may be done. 2196 */ 2197 @Child(name = "substitution", type = {}, order = 28, min = 0, max = 1, modifier = false, summary = false) 2198 @Description(shortDefinition = "Any restrictions on medication substitution", formalDefinition = "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.") 2199 protected MedicationRequestSubstitutionComponent substitution; 2200 2201 /** 2202 * A link to a resource representing an earlier order related order or 2203 * prescription. 2204 */ 2205 @Child(name = "priorPrescription", type = { 2206 MedicationRequest.class }, order = 29, min = 0, max = 1, modifier = false, summary = false) 2207 @Description(shortDefinition = "An order/prescription that is being replaced", formalDefinition = "A link to a resource representing an earlier order related order or prescription.") 2208 protected Reference priorPrescription; 2209 2210 /** 2211 * The actual object that is the target of the reference (A link to a resource 2212 * representing an earlier order related order or prescription.) 2213 */ 2214 protected MedicationRequest priorPrescriptionTarget; 2215 2216 /** 2217 * Indicates an actual or potential clinical issue with or between one or more 2218 * active or proposed clinical actions for a patient; e.g. Drug-drug 2219 * interaction, duplicate therapy, dosage alert etc. 2220 */ 2221 @Child(name = "detectedIssue", type = { 2222 DetectedIssue.class }, order = 30, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2223 @Description(shortDefinition = "Clinical Issue with action", formalDefinition = "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.") 2224 protected List<Reference> detectedIssue; 2225 /** 2226 * The actual objects that are the target of the reference (Indicates an actual 2227 * or potential clinical issue with or between one or more active or proposed 2228 * clinical actions for a patient; e.g. Drug-drug interaction, duplicate 2229 * therapy, dosage alert etc.) 2230 */ 2231 protected List<DetectedIssue> detectedIssueTarget; 2232 2233 /** 2234 * Links to Provenance records for past versions of this resource or fulfilling 2235 * request or event resources that identify key state transitions or updates 2236 * that are likely to be relevant to a user looking at the current version of 2237 * the resource. 2238 */ 2239 @Child(name = "eventHistory", type = { 2240 Provenance.class }, order = 31, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2241 @Description(shortDefinition = "A list of events of interest in the lifecycle", formalDefinition = "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.") 2242 protected List<Reference> eventHistory; 2243 /** 2244 * The actual objects that are the target of the reference (Links to Provenance 2245 * records for past versions of this resource or fulfilling request or event 2246 * resources that identify key state transitions or updates that are likely to 2247 * be relevant to a user looking at the current version of the resource.) 2248 */ 2249 protected List<Provenance> eventHistoryTarget; 2250 2251 private static final long serialVersionUID = 1313900480L; 2252 2253 /** 2254 * Constructor 2255 */ 2256 public MedicationRequest() { 2257 super(); 2258 } 2259 2260 /** 2261 * Constructor 2262 */ 2263 public MedicationRequest(Enumeration<MedicationRequestStatus> status, Enumeration<MedicationRequestIntent> intent, 2264 Type medication, Reference subject) { 2265 super(); 2266 this.status = status; 2267 this.intent = intent; 2268 this.medication = medication; 2269 this.subject = subject; 2270 } 2271 2272 /** 2273 * @return {@link #identifier} (Identifiers associated with this medication 2274 * request that are defined by business processes and/or used to refer 2275 * to it when a direct URL reference to the resource itself is not 2276 * appropriate. They are business identifiers assigned to this resource 2277 * by the performer or other systems and remain constant as the resource 2278 * is updated and propagates from server to server.) 2279 */ 2280 public List<Identifier> getIdentifier() { 2281 if (this.identifier == null) 2282 this.identifier = new ArrayList<Identifier>(); 2283 return this.identifier; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public MedicationRequest setIdentifier(List<Identifier> theIdentifier) { 2290 this.identifier = theIdentifier; 2291 return this; 2292 } 2293 2294 public boolean hasIdentifier() { 2295 if (this.identifier == null) 2296 return false; 2297 for (Identifier item : this.identifier) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public Identifier addIdentifier() { // 3 2304 Identifier t = new Identifier(); 2305 if (this.identifier == null) 2306 this.identifier = new ArrayList<Identifier>(); 2307 this.identifier.add(t); 2308 return t; 2309 } 2310 2311 public MedicationRequest addIdentifier(Identifier t) { // 3 2312 if (t == null) 2313 return this; 2314 if (this.identifier == null) 2315 this.identifier = new ArrayList<Identifier>(); 2316 this.identifier.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #identifier}, creating 2322 * it if it does not already exist 2323 */ 2324 public Identifier getIdentifierFirstRep() { 2325 if (getIdentifier().isEmpty()) { 2326 addIdentifier(); 2327 } 2328 return getIdentifier().get(0); 2329 } 2330 2331 /** 2332 * @return {@link #status} (A code specifying the current state of the order. 2333 * Generally, this will be active or completed state.). This is the 2334 * underlying object with id, value and extensions. The accessor 2335 * "getStatus" gives direct access to the value 2336 */ 2337 public Enumeration<MedicationRequestStatus> getStatusElement() { 2338 if (this.status == null) 2339 if (Configuration.errorOnAutoCreate()) 2340 throw new Error("Attempt to auto-create MedicationRequest.status"); 2341 else if (Configuration.doAutoCreate()) 2342 this.status = new Enumeration<MedicationRequestStatus>(new MedicationRequestStatusEnumFactory()); // bb 2343 return this.status; 2344 } 2345 2346 public boolean hasStatusElement() { 2347 return this.status != null && !this.status.isEmpty(); 2348 } 2349 2350 public boolean hasStatus() { 2351 return this.status != null && !this.status.isEmpty(); 2352 } 2353 2354 /** 2355 * @param value {@link #status} (A code specifying the current state of the 2356 * order. Generally, this will be active or completed state.). This 2357 * is the underlying object with id, value and extensions. The 2358 * accessor "getStatus" gives direct access to the value 2359 */ 2360 public MedicationRequest setStatusElement(Enumeration<MedicationRequestStatus> value) { 2361 this.status = value; 2362 return this; 2363 } 2364 2365 /** 2366 * @return A code specifying the current state of the order. Generally, this 2367 * will be active or completed state. 2368 */ 2369 public MedicationRequestStatus getStatus() { 2370 return this.status == null ? null : this.status.getValue(); 2371 } 2372 2373 /** 2374 * @param value A code specifying the current state of the order. Generally, 2375 * this will be active or completed state. 2376 */ 2377 public MedicationRequest setStatus(MedicationRequestStatus value) { 2378 if (this.status == null) 2379 this.status = new Enumeration<MedicationRequestStatus>(new MedicationRequestStatusEnumFactory()); 2380 this.status.setValue(value); 2381 return this; 2382 } 2383 2384 /** 2385 * @return {@link #statusReason} (Captures the reason for the current state of 2386 * the MedicationRequest.) 2387 */ 2388 public CodeableConcept getStatusReason() { 2389 if (this.statusReason == null) 2390 if (Configuration.errorOnAutoCreate()) 2391 throw new Error("Attempt to auto-create MedicationRequest.statusReason"); 2392 else if (Configuration.doAutoCreate()) 2393 this.statusReason = new CodeableConcept(); // cc 2394 return this.statusReason; 2395 } 2396 2397 public boolean hasStatusReason() { 2398 return this.statusReason != null && !this.statusReason.isEmpty(); 2399 } 2400 2401 /** 2402 * @param value {@link #statusReason} (Captures the reason for the current state 2403 * of the MedicationRequest.) 2404 */ 2405 public MedicationRequest setStatusReason(CodeableConcept value) { 2406 this.statusReason = value; 2407 return this; 2408 } 2409 2410 /** 2411 * @return {@link #intent} (Whether the request is a proposal, plan, or an 2412 * original order.). This is the underlying object with id, value and 2413 * extensions. The accessor "getIntent" gives direct access to the value 2414 */ 2415 public Enumeration<MedicationRequestIntent> getIntentElement() { 2416 if (this.intent == null) 2417 if (Configuration.errorOnAutoCreate()) 2418 throw new Error("Attempt to auto-create MedicationRequest.intent"); 2419 else if (Configuration.doAutoCreate()) 2420 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); // bb 2421 return this.intent; 2422 } 2423 2424 public boolean hasIntentElement() { 2425 return this.intent != null && !this.intent.isEmpty(); 2426 } 2427 2428 public boolean hasIntent() { 2429 return this.intent != null && !this.intent.isEmpty(); 2430 } 2431 2432 /** 2433 * @param value {@link #intent} (Whether the request is a proposal, plan, or an 2434 * original order.). This is the underlying object with id, value 2435 * and extensions. The accessor "getIntent" gives direct access to 2436 * the value 2437 */ 2438 public MedicationRequest setIntentElement(Enumeration<MedicationRequestIntent> value) { 2439 this.intent = value; 2440 return this; 2441 } 2442 2443 /** 2444 * @return Whether the request is a proposal, plan, or an original order. 2445 */ 2446 public MedicationRequestIntent getIntent() { 2447 return this.intent == null ? null : this.intent.getValue(); 2448 } 2449 2450 /** 2451 * @param value Whether the request is a proposal, plan, or an original order. 2452 */ 2453 public MedicationRequest setIntent(MedicationRequestIntent value) { 2454 if (this.intent == null) 2455 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); 2456 this.intent.setValue(value); 2457 return this; 2458 } 2459 2460 /** 2461 * @return {@link #category} (Indicates the type of medication request (for 2462 * example, where the medication is expected to be consumed or 2463 * administered (i.e. inpatient or outpatient)).) 2464 */ 2465 public List<CodeableConcept> getCategory() { 2466 if (this.category == null) 2467 this.category = new ArrayList<CodeableConcept>(); 2468 return this.category; 2469 } 2470 2471 /** 2472 * @return Returns a reference to <code>this</code> for easy method chaining 2473 */ 2474 public MedicationRequest setCategory(List<CodeableConcept> theCategory) { 2475 this.category = theCategory; 2476 return this; 2477 } 2478 2479 public boolean hasCategory() { 2480 if (this.category == null) 2481 return false; 2482 for (CodeableConcept item : this.category) 2483 if (!item.isEmpty()) 2484 return true; 2485 return false; 2486 } 2487 2488 public CodeableConcept addCategory() { // 3 2489 CodeableConcept t = new CodeableConcept(); 2490 if (this.category == null) 2491 this.category = new ArrayList<CodeableConcept>(); 2492 this.category.add(t); 2493 return t; 2494 } 2495 2496 public MedicationRequest addCategory(CodeableConcept t) { // 3 2497 if (t == null) 2498 return this; 2499 if (this.category == null) 2500 this.category = new ArrayList<CodeableConcept>(); 2501 this.category.add(t); 2502 return this; 2503 } 2504 2505 /** 2506 * @return The first repetition of repeating field {@link #category}, creating 2507 * it if it does not already exist 2508 */ 2509 public CodeableConcept getCategoryFirstRep() { 2510 if (getCategory().isEmpty()) { 2511 addCategory(); 2512 } 2513 return getCategory().get(0); 2514 } 2515 2516 /** 2517 * @return {@link #priority} (Indicates how quickly the Medication Request 2518 * should be addressed with respect to other requests.). This is the 2519 * underlying object with id, value and extensions. The accessor 2520 * "getPriority" gives direct access to the value 2521 */ 2522 public Enumeration<MedicationRequestPriority> getPriorityElement() { 2523 if (this.priority == null) 2524 if (Configuration.errorOnAutoCreate()) 2525 throw new Error("Attempt to auto-create MedicationRequest.priority"); 2526 else if (Configuration.doAutoCreate()) 2527 this.priority = new Enumeration<MedicationRequestPriority>(new MedicationRequestPriorityEnumFactory()); // bb 2528 return this.priority; 2529 } 2530 2531 public boolean hasPriorityElement() { 2532 return this.priority != null && !this.priority.isEmpty(); 2533 } 2534 2535 public boolean hasPriority() { 2536 return this.priority != null && !this.priority.isEmpty(); 2537 } 2538 2539 /** 2540 * @param value {@link #priority} (Indicates how quickly the Medication Request 2541 * should be addressed with respect to other requests.). This is 2542 * the underlying object with id, value and extensions. The 2543 * accessor "getPriority" gives direct access to the value 2544 */ 2545 public MedicationRequest setPriorityElement(Enumeration<MedicationRequestPriority> value) { 2546 this.priority = value; 2547 return this; 2548 } 2549 2550 /** 2551 * @return Indicates how quickly the Medication Request should be addressed with 2552 * respect to other requests. 2553 */ 2554 public MedicationRequestPriority getPriority() { 2555 return this.priority == null ? null : this.priority.getValue(); 2556 } 2557 2558 /** 2559 * @param value Indicates how quickly the Medication Request should be addressed 2560 * with respect to other requests. 2561 */ 2562 public MedicationRequest setPriority(MedicationRequestPriority value) { 2563 if (value == null) 2564 this.priority = null; 2565 else { 2566 if (this.priority == null) 2567 this.priority = new Enumeration<MedicationRequestPriority>(new MedicationRequestPriorityEnumFactory()); 2568 this.priority.setValue(value); 2569 } 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #doNotPerform} (If true indicates that the provider is asking 2575 * for the medication request not to occur.). This is the underlying 2576 * object with id, value and extensions. The accessor "getDoNotPerform" 2577 * gives direct access to the value 2578 */ 2579 public BooleanType getDoNotPerformElement() { 2580 if (this.doNotPerform == null) 2581 if (Configuration.errorOnAutoCreate()) 2582 throw new Error("Attempt to auto-create MedicationRequest.doNotPerform"); 2583 else if (Configuration.doAutoCreate()) 2584 this.doNotPerform = new BooleanType(); // bb 2585 return this.doNotPerform; 2586 } 2587 2588 public boolean hasDoNotPerformElement() { 2589 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2590 } 2591 2592 public boolean hasDoNotPerform() { 2593 return this.doNotPerform != null && !this.doNotPerform.isEmpty(); 2594 } 2595 2596 /** 2597 * @param value {@link #doNotPerform} (If true indicates that the provider is 2598 * asking for the medication request not to occur.). This is the 2599 * underlying object with id, value and extensions. The accessor 2600 * "getDoNotPerform" gives direct access to the value 2601 */ 2602 public MedicationRequest setDoNotPerformElement(BooleanType value) { 2603 this.doNotPerform = value; 2604 return this; 2605 } 2606 2607 /** 2608 * @return If true indicates that the provider is asking for the medication 2609 * request not to occur. 2610 */ 2611 public boolean getDoNotPerform() { 2612 return this.doNotPerform == null || this.doNotPerform.isEmpty() ? false : this.doNotPerform.getValue(); 2613 } 2614 2615 /** 2616 * @param value If true indicates that the provider is asking for the medication 2617 * request not to occur. 2618 */ 2619 public MedicationRequest setDoNotPerform(boolean value) { 2620 if (this.doNotPerform == null) 2621 this.doNotPerform = new BooleanType(); 2622 this.doNotPerform.setValue(value); 2623 return this; 2624 } 2625 2626 /** 2627 * @return {@link #reported} (Indicates if this record was captured as a 2628 * secondary 'reported' record rather than as an original primary 2629 * source-of-truth record. It may also indicate the source of the 2630 * report.) 2631 */ 2632 public Type getReported() { 2633 return this.reported; 2634 } 2635 2636 /** 2637 * @return {@link #reported} (Indicates if this record was captured as a 2638 * secondary 'reported' record rather than as an original primary 2639 * source-of-truth record. It may also indicate the source of the 2640 * report.) 2641 */ 2642 public BooleanType getReportedBooleanType() throws FHIRException { 2643 if (this.reported == null) 2644 this.reported = new BooleanType(); 2645 if (!(this.reported instanceof BooleanType)) 2646 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 2647 + this.reported.getClass().getName() + " was encountered"); 2648 return (BooleanType) this.reported; 2649 } 2650 2651 public boolean hasReportedBooleanType() { 2652 return this.reported instanceof BooleanType; 2653 } 2654 2655 /** 2656 * @return {@link #reported} (Indicates if this record was captured as a 2657 * secondary 'reported' record rather than as an original primary 2658 * source-of-truth record. It may also indicate the source of the 2659 * report.) 2660 */ 2661 public Reference getReportedReference() throws FHIRException { 2662 if (this.reported == null) 2663 this.reported = new Reference(); 2664 if (!(this.reported instanceof Reference)) 2665 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2666 + this.reported.getClass().getName() + " was encountered"); 2667 return (Reference) this.reported; 2668 } 2669 2670 public boolean hasReportedReference() { 2671 return this.reported instanceof Reference; 2672 } 2673 2674 public boolean hasReported() { 2675 return this.reported != null && !this.reported.isEmpty(); 2676 } 2677 2678 /** 2679 * @param value {@link #reported} (Indicates if this record was captured as a 2680 * secondary 'reported' record rather than as an original primary 2681 * source-of-truth record. It may also indicate the source of the 2682 * report.) 2683 */ 2684 public MedicationRequest setReported(Type value) { 2685 if (value != null && !(value instanceof BooleanType || value instanceof Reference)) 2686 throw new Error("Not the right type for MedicationRequest.reported[x]: " + value.fhirType()); 2687 this.reported = value; 2688 return this; 2689 } 2690 2691 /** 2692 * @return {@link #medication} (Identifies the medication being requested. This 2693 * is a link to a resource that represents the medication which may be 2694 * the details of the medication or simply an attribute carrying a code 2695 * that identifies the medication from a known list of medications.) 2696 */ 2697 public Type getMedication() { 2698 return this.medication; 2699 } 2700 2701 /** 2702 * @return {@link #medication} (Identifies the medication being requested. This 2703 * is a link to a resource that represents the medication which may be 2704 * the details of the medication or simply an attribute carrying a code 2705 * that identifies the medication from a known list of medications.) 2706 */ 2707 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 2708 if (this.medication == null) 2709 this.medication = new CodeableConcept(); 2710 if (!(this.medication instanceof CodeableConcept)) 2711 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2712 + this.medication.getClass().getName() + " was encountered"); 2713 return (CodeableConcept) this.medication; 2714 } 2715 2716 public boolean hasMedicationCodeableConcept() { 2717 return this.medication instanceof CodeableConcept; 2718 } 2719 2720 /** 2721 * @return {@link #medication} (Identifies the medication being requested. This 2722 * is a link to a resource that represents the medication which may be 2723 * the details of the medication or simply an attribute carrying a code 2724 * that identifies the medication from a known list of medications.) 2725 */ 2726 public Reference getMedicationReference() throws FHIRException { 2727 if (this.medication == null) 2728 this.medication = new Reference(); 2729 if (!(this.medication instanceof Reference)) 2730 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2731 + this.medication.getClass().getName() + " was encountered"); 2732 return (Reference) this.medication; 2733 } 2734 2735 public boolean hasMedicationReference() { 2736 return this.medication instanceof Reference; 2737 } 2738 2739 public boolean hasMedication() { 2740 return this.medication != null && !this.medication.isEmpty(); 2741 } 2742 2743 /** 2744 * @param value {@link #medication} (Identifies the medication being requested. 2745 * This is a link to a resource that represents the medication 2746 * which may be the details of the medication or simply an 2747 * attribute carrying a code that identifies the medication from a 2748 * known list of medications.) 2749 */ 2750 public MedicationRequest setMedication(Type value) { 2751 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2752 throw new Error("Not the right type for MedicationRequest.medication[x]: " + value.fhirType()); 2753 this.medication = value; 2754 return this; 2755 } 2756 2757 /** 2758 * @return {@link #subject} (A link to a resource representing the person or set 2759 * of individuals to whom the medication will be given.) 2760 */ 2761 public Reference getSubject() { 2762 if (this.subject == null) 2763 if (Configuration.errorOnAutoCreate()) 2764 throw new Error("Attempt to auto-create MedicationRequest.subject"); 2765 else if (Configuration.doAutoCreate()) 2766 this.subject = new Reference(); // cc 2767 return this.subject; 2768 } 2769 2770 public boolean hasSubject() { 2771 return this.subject != null && !this.subject.isEmpty(); 2772 } 2773 2774 /** 2775 * @param value {@link #subject} (A link to a resource representing the person 2776 * or set of individuals to whom the medication will be given.) 2777 */ 2778 public MedicationRequest setSubject(Reference value) { 2779 this.subject = value; 2780 return this; 2781 } 2782 2783 /** 2784 * @return {@link #subject} The actual object that is the target of the 2785 * reference. The reference library doesn't populate this, but you can 2786 * use it to hold the resource if you resolve it. (A link to a resource 2787 * representing the person or set of individuals to whom the medication 2788 * will be given.) 2789 */ 2790 public Resource getSubjectTarget() { 2791 return this.subjectTarget; 2792 } 2793 2794 /** 2795 * @param value {@link #subject} The actual object that is the target of the 2796 * reference. The reference library doesn't use these, but you can 2797 * use it to hold the resource if you resolve it. (A link to a 2798 * resource representing the person or set of individuals to whom 2799 * the medication will be given.) 2800 */ 2801 public MedicationRequest setSubjectTarget(Resource value) { 2802 this.subjectTarget = value; 2803 return this; 2804 } 2805 2806 /** 2807 * @return {@link #encounter} (The Encounter during which this [x] was created 2808 * or to which the creation of this record is tightly associated.) 2809 */ 2810 public Reference getEncounter() { 2811 if (this.encounter == null) 2812 if (Configuration.errorOnAutoCreate()) 2813 throw new Error("Attempt to auto-create MedicationRequest.encounter"); 2814 else if (Configuration.doAutoCreate()) 2815 this.encounter = new Reference(); // cc 2816 return this.encounter; 2817 } 2818 2819 public boolean hasEncounter() { 2820 return this.encounter != null && !this.encounter.isEmpty(); 2821 } 2822 2823 /** 2824 * @param value {@link #encounter} (The Encounter during which this [x] was 2825 * created or to which the creation of this record is tightly 2826 * associated.) 2827 */ 2828 public MedicationRequest setEncounter(Reference value) { 2829 this.encounter = value; 2830 return this; 2831 } 2832 2833 /** 2834 * @return {@link #encounter} The actual object that is the target of the 2835 * reference. The reference library doesn't populate this, but you can 2836 * use it to hold the resource if you resolve it. (The Encounter during 2837 * which this [x] was created or to which the creation of this record is 2838 * tightly associated.) 2839 */ 2840 public Encounter getEncounterTarget() { 2841 if (this.encounterTarget == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create MedicationRequest.encounter"); 2844 else if (Configuration.doAutoCreate()) 2845 this.encounterTarget = new Encounter(); // aa 2846 return this.encounterTarget; 2847 } 2848 2849 /** 2850 * @param value {@link #encounter} The actual object that is the target of the 2851 * reference. The reference library doesn't use these, but you can 2852 * use it to hold the resource if you resolve it. (The Encounter 2853 * during which this [x] was created or to which the creation of 2854 * this record is tightly associated.) 2855 */ 2856 public MedicationRequest setEncounterTarget(Encounter value) { 2857 this.encounterTarget = value; 2858 return this; 2859 } 2860 2861 /** 2862 * @return {@link #supportingInformation} (Include additional information (for 2863 * example, patient height and weight) that supports the ordering of the 2864 * medication.) 2865 */ 2866 public List<Reference> getSupportingInformation() { 2867 if (this.supportingInformation == null) 2868 this.supportingInformation = new ArrayList<Reference>(); 2869 return this.supportingInformation; 2870 } 2871 2872 /** 2873 * @return Returns a reference to <code>this</code> for easy method chaining 2874 */ 2875 public MedicationRequest setSupportingInformation(List<Reference> theSupportingInformation) { 2876 this.supportingInformation = theSupportingInformation; 2877 return this; 2878 } 2879 2880 public boolean hasSupportingInformation() { 2881 if (this.supportingInformation == null) 2882 return false; 2883 for (Reference item : this.supportingInformation) 2884 if (!item.isEmpty()) 2885 return true; 2886 return false; 2887 } 2888 2889 public Reference addSupportingInformation() { // 3 2890 Reference t = new Reference(); 2891 if (this.supportingInformation == null) 2892 this.supportingInformation = new ArrayList<Reference>(); 2893 this.supportingInformation.add(t); 2894 return t; 2895 } 2896 2897 public MedicationRequest addSupportingInformation(Reference t) { // 3 2898 if (t == null) 2899 return this; 2900 if (this.supportingInformation == null) 2901 this.supportingInformation = new ArrayList<Reference>(); 2902 this.supportingInformation.add(t); 2903 return this; 2904 } 2905 2906 /** 2907 * @return The first repetition of repeating field 2908 * {@link #supportingInformation}, creating it if it does not already 2909 * exist 2910 */ 2911 public Reference getSupportingInformationFirstRep() { 2912 if (getSupportingInformation().isEmpty()) { 2913 addSupportingInformation(); 2914 } 2915 return getSupportingInformation().get(0); 2916 } 2917 2918 /** 2919 * @return {@link #authoredOn} (The date (and perhaps time) when the 2920 * prescription was initially written or authored on.). This is the 2921 * underlying object with id, value and extensions. The accessor 2922 * "getAuthoredOn" gives direct access to the value 2923 */ 2924 public DateTimeType getAuthoredOnElement() { 2925 if (this.authoredOn == null) 2926 if (Configuration.errorOnAutoCreate()) 2927 throw new Error("Attempt to auto-create MedicationRequest.authoredOn"); 2928 else if (Configuration.doAutoCreate()) 2929 this.authoredOn = new DateTimeType(); // bb 2930 return this.authoredOn; 2931 } 2932 2933 public boolean hasAuthoredOnElement() { 2934 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2935 } 2936 2937 public boolean hasAuthoredOn() { 2938 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2939 } 2940 2941 /** 2942 * @param value {@link #authoredOn} (The date (and perhaps time) when the 2943 * prescription was initially written or authored on.). This is the 2944 * underlying object with id, value and extensions. The accessor 2945 * "getAuthoredOn" gives direct access to the value 2946 */ 2947 public MedicationRequest setAuthoredOnElement(DateTimeType value) { 2948 this.authoredOn = value; 2949 return this; 2950 } 2951 2952 /** 2953 * @return The date (and perhaps time) when the prescription was initially 2954 * written or authored on. 2955 */ 2956 public Date getAuthoredOn() { 2957 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2958 } 2959 2960 /** 2961 * @param value The date (and perhaps time) when the prescription was initially 2962 * written or authored on. 2963 */ 2964 public MedicationRequest setAuthoredOn(Date value) { 2965 if (value == null) 2966 this.authoredOn = null; 2967 else { 2968 if (this.authoredOn == null) 2969 this.authoredOn = new DateTimeType(); 2970 this.authoredOn.setValue(value); 2971 } 2972 return this; 2973 } 2974 2975 /** 2976 * @return {@link #requester} (The individual, organization, or device that 2977 * initiated the request and has responsibility for its activation.) 2978 */ 2979 public Reference getRequester() { 2980 if (this.requester == null) 2981 if (Configuration.errorOnAutoCreate()) 2982 throw new Error("Attempt to auto-create MedicationRequest.requester"); 2983 else if (Configuration.doAutoCreate()) 2984 this.requester = new Reference(); // cc 2985 return this.requester; 2986 } 2987 2988 public boolean hasRequester() { 2989 return this.requester != null && !this.requester.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #requester} (The individual, organization, or device that 2994 * initiated the request and has responsibility for its 2995 * activation.) 2996 */ 2997 public MedicationRequest setRequester(Reference value) { 2998 this.requester = value; 2999 return this; 3000 } 3001 3002 /** 3003 * @return {@link #requester} The actual object that is the target of the 3004 * reference. The reference library doesn't populate this, but you can 3005 * use it to hold the resource if you resolve it. (The individual, 3006 * organization, or device that initiated the request and has 3007 * responsibility for its activation.) 3008 */ 3009 public Resource getRequesterTarget() { 3010 return this.requesterTarget; 3011 } 3012 3013 /** 3014 * @param value {@link #requester} The actual object that is the target of the 3015 * reference. The reference library doesn't use these, but you can 3016 * use it to hold the resource if you resolve it. (The individual, 3017 * organization, or device that initiated the request and has 3018 * responsibility for its activation.) 3019 */ 3020 public MedicationRequest setRequesterTarget(Resource value) { 3021 this.requesterTarget = value; 3022 return this; 3023 } 3024 3025 /** 3026 * @return {@link #performer} (The specified desired performer of the medication 3027 * treatment (e.g. the performer of the medication administration).) 3028 */ 3029 public Reference getPerformer() { 3030 if (this.performer == null) 3031 if (Configuration.errorOnAutoCreate()) 3032 throw new Error("Attempt to auto-create MedicationRequest.performer"); 3033 else if (Configuration.doAutoCreate()) 3034 this.performer = new Reference(); // cc 3035 return this.performer; 3036 } 3037 3038 public boolean hasPerformer() { 3039 return this.performer != null && !this.performer.isEmpty(); 3040 } 3041 3042 /** 3043 * @param value {@link #performer} (The specified desired performer of the 3044 * medication treatment (e.g. the performer of the medication 3045 * administration).) 3046 */ 3047 public MedicationRequest setPerformer(Reference value) { 3048 this.performer = value; 3049 return this; 3050 } 3051 3052 /** 3053 * @return {@link #performer} The actual object that is the target of the 3054 * reference. The reference library doesn't populate this, but you can 3055 * use it to hold the resource if you resolve it. (The specified desired 3056 * performer of the medication treatment (e.g. the performer of the 3057 * medication administration).) 3058 */ 3059 public Resource getPerformerTarget() { 3060 return this.performerTarget; 3061 } 3062 3063 /** 3064 * @param value {@link #performer} The actual object that is the target of the 3065 * reference. The reference library doesn't use these, but you can 3066 * use it to hold the resource if you resolve it. (The specified 3067 * desired performer of the medication treatment (e.g. the 3068 * performer of the medication administration).) 3069 */ 3070 public MedicationRequest setPerformerTarget(Resource value) { 3071 this.performerTarget = value; 3072 return this; 3073 } 3074 3075 /** 3076 * @return {@link #performerType} (Indicates the type of performer of the 3077 * administration of the medication.) 3078 */ 3079 public CodeableConcept getPerformerType() { 3080 if (this.performerType == null) 3081 if (Configuration.errorOnAutoCreate()) 3082 throw new Error("Attempt to auto-create MedicationRequest.performerType"); 3083 else if (Configuration.doAutoCreate()) 3084 this.performerType = new CodeableConcept(); // cc 3085 return this.performerType; 3086 } 3087 3088 public boolean hasPerformerType() { 3089 return this.performerType != null && !this.performerType.isEmpty(); 3090 } 3091 3092 /** 3093 * @param value {@link #performerType} (Indicates the type of performer of the 3094 * administration of the medication.) 3095 */ 3096 public MedicationRequest setPerformerType(CodeableConcept value) { 3097 this.performerType = value; 3098 return this; 3099 } 3100 3101 /** 3102 * @return {@link #recorder} (The person who entered the order on behalf of 3103 * another individual for example in the case of a verbal or a telephone 3104 * order.) 3105 */ 3106 public Reference getRecorder() { 3107 if (this.recorder == null) 3108 if (Configuration.errorOnAutoCreate()) 3109 throw new Error("Attempt to auto-create MedicationRequest.recorder"); 3110 else if (Configuration.doAutoCreate()) 3111 this.recorder = new Reference(); // cc 3112 return this.recorder; 3113 } 3114 3115 public boolean hasRecorder() { 3116 return this.recorder != null && !this.recorder.isEmpty(); 3117 } 3118 3119 /** 3120 * @param value {@link #recorder} (The person who entered the order on behalf of 3121 * another individual for example in the case of a verbal or a 3122 * telephone order.) 3123 */ 3124 public MedicationRequest setRecorder(Reference value) { 3125 this.recorder = value; 3126 return this; 3127 } 3128 3129 /** 3130 * @return {@link #recorder} The actual object that is the target of the 3131 * reference. The reference library doesn't populate this, but you can 3132 * use it to hold the resource if you resolve it. (The person who 3133 * entered the order on behalf of another individual for example in the 3134 * case of a verbal or a telephone order.) 3135 */ 3136 public Resource getRecorderTarget() { 3137 return this.recorderTarget; 3138 } 3139 3140 /** 3141 * @param value {@link #recorder} The actual object that is the target of the 3142 * reference. The reference library doesn't use these, but you can 3143 * use it to hold the resource if you resolve it. (The person who 3144 * entered the order on behalf of another individual for example in 3145 * the case of a verbal or a telephone order.) 3146 */ 3147 public MedicationRequest setRecorderTarget(Resource value) { 3148 this.recorderTarget = value; 3149 return this; 3150 } 3151 3152 /** 3153 * @return {@link #reasonCode} (The reason or the indication for ordering or not 3154 * ordering the medication.) 3155 */ 3156 public List<CodeableConcept> getReasonCode() { 3157 if (this.reasonCode == null) 3158 this.reasonCode = new ArrayList<CodeableConcept>(); 3159 return this.reasonCode; 3160 } 3161 3162 /** 3163 * @return Returns a reference to <code>this</code> for easy method chaining 3164 */ 3165 public MedicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 3166 this.reasonCode = theReasonCode; 3167 return this; 3168 } 3169 3170 public boolean hasReasonCode() { 3171 if (this.reasonCode == null) 3172 return false; 3173 for (CodeableConcept item : this.reasonCode) 3174 if (!item.isEmpty()) 3175 return true; 3176 return false; 3177 } 3178 3179 public CodeableConcept addReasonCode() { // 3 3180 CodeableConcept t = new CodeableConcept(); 3181 if (this.reasonCode == null) 3182 this.reasonCode = new ArrayList<CodeableConcept>(); 3183 this.reasonCode.add(t); 3184 return t; 3185 } 3186 3187 public MedicationRequest addReasonCode(CodeableConcept t) { // 3 3188 if (t == null) 3189 return this; 3190 if (this.reasonCode == null) 3191 this.reasonCode = new ArrayList<CodeableConcept>(); 3192 this.reasonCode.add(t); 3193 return this; 3194 } 3195 3196 /** 3197 * @return The first repetition of repeating field {@link #reasonCode}, creating 3198 * it if it does not already exist 3199 */ 3200 public CodeableConcept getReasonCodeFirstRep() { 3201 if (getReasonCode().isEmpty()) { 3202 addReasonCode(); 3203 } 3204 return getReasonCode().get(0); 3205 } 3206 3207 /** 3208 * @return {@link #reasonReference} (Condition or observation that supports why 3209 * the medication was ordered.) 3210 */ 3211 public List<Reference> getReasonReference() { 3212 if (this.reasonReference == null) 3213 this.reasonReference = new ArrayList<Reference>(); 3214 return this.reasonReference; 3215 } 3216 3217 /** 3218 * @return Returns a reference to <code>this</code> for easy method chaining 3219 */ 3220 public MedicationRequest setReasonReference(List<Reference> theReasonReference) { 3221 this.reasonReference = theReasonReference; 3222 return this; 3223 } 3224 3225 public boolean hasReasonReference() { 3226 if (this.reasonReference == null) 3227 return false; 3228 for (Reference item : this.reasonReference) 3229 if (!item.isEmpty()) 3230 return true; 3231 return false; 3232 } 3233 3234 public Reference addReasonReference() { // 3 3235 Reference t = new Reference(); 3236 if (this.reasonReference == null) 3237 this.reasonReference = new ArrayList<Reference>(); 3238 this.reasonReference.add(t); 3239 return t; 3240 } 3241 3242 public MedicationRequest addReasonReference(Reference t) { // 3 3243 if (t == null) 3244 return this; 3245 if (this.reasonReference == null) 3246 this.reasonReference = new ArrayList<Reference>(); 3247 this.reasonReference.add(t); 3248 return this; 3249 } 3250 3251 /** 3252 * @return The first repetition of repeating field {@link #reasonReference}, 3253 * creating it if it does not already exist 3254 */ 3255 public Reference getReasonReferenceFirstRep() { 3256 if (getReasonReference().isEmpty()) { 3257 addReasonReference(); 3258 } 3259 return getReasonReference().get(0); 3260 } 3261 3262 /** 3263 * @return {@link #instantiatesCanonical} (The URL pointing to a protocol, 3264 * guideline, orderset, or other definition that is adhered to in whole 3265 * or in part by this MedicationRequest.) 3266 */ 3267 public List<CanonicalType> getInstantiatesCanonical() { 3268 if (this.instantiatesCanonical == null) 3269 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3270 return this.instantiatesCanonical; 3271 } 3272 3273 /** 3274 * @return Returns a reference to <code>this</code> for easy method chaining 3275 */ 3276 public MedicationRequest setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 3277 this.instantiatesCanonical = theInstantiatesCanonical; 3278 return this; 3279 } 3280 3281 public boolean hasInstantiatesCanonical() { 3282 if (this.instantiatesCanonical == null) 3283 return false; 3284 for (CanonicalType item : this.instantiatesCanonical) 3285 if (!item.isEmpty()) 3286 return true; 3287 return false; 3288 } 3289 3290 /** 3291 * @return {@link #instantiatesCanonical} (The URL pointing to a protocol, 3292 * guideline, orderset, or other definition that is adhered to in whole 3293 * or in part by this MedicationRequest.) 3294 */ 3295 public CanonicalType addInstantiatesCanonicalElement() {// 2 3296 CanonicalType t = new CanonicalType(); 3297 if (this.instantiatesCanonical == null) 3298 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3299 this.instantiatesCanonical.add(t); 3300 return t; 3301 } 3302 3303 /** 3304 * @param value {@link #instantiatesCanonical} (The URL pointing to a protocol, 3305 * guideline, orderset, or other definition that is adhered to in 3306 * whole or in part by this MedicationRequest.) 3307 */ 3308 public MedicationRequest addInstantiatesCanonical(String value) { // 1 3309 CanonicalType t = new CanonicalType(); 3310 t.setValue(value); 3311 if (this.instantiatesCanonical == null) 3312 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 3313 this.instantiatesCanonical.add(t); 3314 return this; 3315 } 3316 3317 /** 3318 * @param value {@link #instantiatesCanonical} (The URL pointing to a protocol, 3319 * guideline, orderset, or other definition that is adhered to in 3320 * whole or in part by this MedicationRequest.) 3321 */ 3322 public boolean hasInstantiatesCanonical(String value) { 3323 if (this.instantiatesCanonical == null) 3324 return false; 3325 for (CanonicalType v : this.instantiatesCanonical) 3326 if (v.getValue().equals(value)) // canonical 3327 return true; 3328 return false; 3329 } 3330 3331 /** 3332 * @return {@link #instantiatesUri} (The URL pointing to an externally 3333 * maintained protocol, guideline, orderset or other definition that is 3334 * adhered to in whole or in part by this MedicationRequest.) 3335 */ 3336 public List<UriType> getInstantiatesUri() { 3337 if (this.instantiatesUri == null) 3338 this.instantiatesUri = new ArrayList<UriType>(); 3339 return this.instantiatesUri; 3340 } 3341 3342 /** 3343 * @return Returns a reference to <code>this</code> for easy method chaining 3344 */ 3345 public MedicationRequest setInstantiatesUri(List<UriType> theInstantiatesUri) { 3346 this.instantiatesUri = theInstantiatesUri; 3347 return this; 3348 } 3349 3350 public boolean hasInstantiatesUri() { 3351 if (this.instantiatesUri == null) 3352 return false; 3353 for (UriType item : this.instantiatesUri) 3354 if (!item.isEmpty()) 3355 return true; 3356 return false; 3357 } 3358 3359 /** 3360 * @return {@link #instantiatesUri} (The URL pointing to an externally 3361 * maintained protocol, guideline, orderset or other definition that is 3362 * adhered to in whole or in part by this MedicationRequest.) 3363 */ 3364 public UriType addInstantiatesUriElement() {// 2 3365 UriType t = new UriType(); 3366 if (this.instantiatesUri == null) 3367 this.instantiatesUri = new ArrayList<UriType>(); 3368 this.instantiatesUri.add(t); 3369 return t; 3370 } 3371 3372 /** 3373 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3374 * maintained protocol, guideline, orderset or other definition 3375 * that is adhered to in whole or in part by this 3376 * MedicationRequest.) 3377 */ 3378 public MedicationRequest addInstantiatesUri(String value) { // 1 3379 UriType t = new UriType(); 3380 t.setValue(value); 3381 if (this.instantiatesUri == null) 3382 this.instantiatesUri = new ArrayList<UriType>(); 3383 this.instantiatesUri.add(t); 3384 return this; 3385 } 3386 3387 /** 3388 * @param value {@link #instantiatesUri} (The URL pointing to an externally 3389 * maintained protocol, guideline, orderset or other definition 3390 * that is adhered to in whole or in part by this 3391 * MedicationRequest.) 3392 */ 3393 public boolean hasInstantiatesUri(String value) { 3394 if (this.instantiatesUri == null) 3395 return false; 3396 for (UriType v : this.instantiatesUri) 3397 if (v.getValue().equals(value)) // uri 3398 return true; 3399 return false; 3400 } 3401 3402 /** 3403 * @return {@link #basedOn} (A plan or request that is fulfilled in whole or in 3404 * part by this medication request.) 3405 */ 3406 public List<Reference> getBasedOn() { 3407 if (this.basedOn == null) 3408 this.basedOn = new ArrayList<Reference>(); 3409 return this.basedOn; 3410 } 3411 3412 /** 3413 * @return Returns a reference to <code>this</code> for easy method chaining 3414 */ 3415 public MedicationRequest setBasedOn(List<Reference> theBasedOn) { 3416 this.basedOn = theBasedOn; 3417 return this; 3418 } 3419 3420 public boolean hasBasedOn() { 3421 if (this.basedOn == null) 3422 return false; 3423 for (Reference item : this.basedOn) 3424 if (!item.isEmpty()) 3425 return true; 3426 return false; 3427 } 3428 3429 public Reference addBasedOn() { // 3 3430 Reference t = new Reference(); 3431 if (this.basedOn == null) 3432 this.basedOn = new ArrayList<Reference>(); 3433 this.basedOn.add(t); 3434 return t; 3435 } 3436 3437 public MedicationRequest addBasedOn(Reference t) { // 3 3438 if (t == null) 3439 return this; 3440 if (this.basedOn == null) 3441 this.basedOn = new ArrayList<Reference>(); 3442 this.basedOn.add(t); 3443 return this; 3444 } 3445 3446 /** 3447 * @return The first repetition of repeating field {@link #basedOn}, creating it 3448 * if it does not already exist 3449 */ 3450 public Reference getBasedOnFirstRep() { 3451 if (getBasedOn().isEmpty()) { 3452 addBasedOn(); 3453 } 3454 return getBasedOn().get(0); 3455 } 3456 3457 /** 3458 * @return {@link #groupIdentifier} (A shared identifier common to all requests 3459 * that were authorized more or less simultaneously by a single author, 3460 * representing the identifier of the requisition or prescription.) 3461 */ 3462 public Identifier getGroupIdentifier() { 3463 if (this.groupIdentifier == null) 3464 if (Configuration.errorOnAutoCreate()) 3465 throw new Error("Attempt to auto-create MedicationRequest.groupIdentifier"); 3466 else if (Configuration.doAutoCreate()) 3467 this.groupIdentifier = new Identifier(); // cc 3468 return this.groupIdentifier; 3469 } 3470 3471 public boolean hasGroupIdentifier() { 3472 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 3473 } 3474 3475 /** 3476 * @param value {@link #groupIdentifier} (A shared identifier common to all 3477 * requests that were authorized more or less simultaneously by a 3478 * single author, representing the identifier of the requisition or 3479 * prescription.) 3480 */ 3481 public MedicationRequest setGroupIdentifier(Identifier value) { 3482 this.groupIdentifier = value; 3483 return this; 3484 } 3485 3486 /** 3487 * @return {@link #courseOfTherapyType} (The description of the overall patte3rn 3488 * of the administration of the medication to the patient.) 3489 */ 3490 public CodeableConcept getCourseOfTherapyType() { 3491 if (this.courseOfTherapyType == null) 3492 if (Configuration.errorOnAutoCreate()) 3493 throw new Error("Attempt to auto-create MedicationRequest.courseOfTherapyType"); 3494 else if (Configuration.doAutoCreate()) 3495 this.courseOfTherapyType = new CodeableConcept(); // cc 3496 return this.courseOfTherapyType; 3497 } 3498 3499 public boolean hasCourseOfTherapyType() { 3500 return this.courseOfTherapyType != null && !this.courseOfTherapyType.isEmpty(); 3501 } 3502 3503 /** 3504 * @param value {@link #courseOfTherapyType} (The description of the overall 3505 * patte3rn of the administration of the medication to the 3506 * patient.) 3507 */ 3508 public MedicationRequest setCourseOfTherapyType(CodeableConcept value) { 3509 this.courseOfTherapyType = value; 3510 return this; 3511 } 3512 3513 /** 3514 * @return {@link #insurance} (Insurance plans, coverage extensions, 3515 * pre-authorizations and/or pre-determinations that may be required for 3516 * delivering the requested service.) 3517 */ 3518 public List<Reference> getInsurance() { 3519 if (this.insurance == null) 3520 this.insurance = new ArrayList<Reference>(); 3521 return this.insurance; 3522 } 3523 3524 /** 3525 * @return Returns a reference to <code>this</code> for easy method chaining 3526 */ 3527 public MedicationRequest setInsurance(List<Reference> theInsurance) { 3528 this.insurance = theInsurance; 3529 return this; 3530 } 3531 3532 public boolean hasInsurance() { 3533 if (this.insurance == null) 3534 return false; 3535 for (Reference item : this.insurance) 3536 if (!item.isEmpty()) 3537 return true; 3538 return false; 3539 } 3540 3541 public Reference addInsurance() { // 3 3542 Reference t = new Reference(); 3543 if (this.insurance == null) 3544 this.insurance = new ArrayList<Reference>(); 3545 this.insurance.add(t); 3546 return t; 3547 } 3548 3549 public MedicationRequest addInsurance(Reference t) { // 3 3550 if (t == null) 3551 return this; 3552 if (this.insurance == null) 3553 this.insurance = new ArrayList<Reference>(); 3554 this.insurance.add(t); 3555 return this; 3556 } 3557 3558 /** 3559 * @return The first repetition of repeating field {@link #insurance}, creating 3560 * it if it does not already exist 3561 */ 3562 public Reference getInsuranceFirstRep() { 3563 if (getInsurance().isEmpty()) { 3564 addInsurance(); 3565 } 3566 return getInsurance().get(0); 3567 } 3568 3569 /** 3570 * @return {@link #note} (Extra information about the prescription that could 3571 * not be conveyed by the other attributes.) 3572 */ 3573 public List<Annotation> getNote() { 3574 if (this.note == null) 3575 this.note = new ArrayList<Annotation>(); 3576 return this.note; 3577 } 3578 3579 /** 3580 * @return Returns a reference to <code>this</code> for easy method chaining 3581 */ 3582 public MedicationRequest setNote(List<Annotation> theNote) { 3583 this.note = theNote; 3584 return this; 3585 } 3586 3587 public boolean hasNote() { 3588 if (this.note == null) 3589 return false; 3590 for (Annotation item : this.note) 3591 if (!item.isEmpty()) 3592 return true; 3593 return false; 3594 } 3595 3596 public Annotation addNote() { // 3 3597 Annotation t = new Annotation(); 3598 if (this.note == null) 3599 this.note = new ArrayList<Annotation>(); 3600 this.note.add(t); 3601 return t; 3602 } 3603 3604 public MedicationRequest addNote(Annotation t) { // 3 3605 if (t == null) 3606 return this; 3607 if (this.note == null) 3608 this.note = new ArrayList<Annotation>(); 3609 this.note.add(t); 3610 return this; 3611 } 3612 3613 /** 3614 * @return The first repetition of repeating field {@link #note}, creating it if 3615 * it does not already exist 3616 */ 3617 public Annotation getNoteFirstRep() { 3618 if (getNote().isEmpty()) { 3619 addNote(); 3620 } 3621 return getNote().get(0); 3622 } 3623 3624 /** 3625 * @return {@link #dosageInstruction} (Indicates how the medication is to be 3626 * used by the patient.) 3627 */ 3628 public List<Dosage> getDosageInstruction() { 3629 if (this.dosageInstruction == null) 3630 this.dosageInstruction = new ArrayList<Dosage>(); 3631 return this.dosageInstruction; 3632 } 3633 3634 /** 3635 * @return Returns a reference to <code>this</code> for easy method chaining 3636 */ 3637 public MedicationRequest setDosageInstruction(List<Dosage> theDosageInstruction) { 3638 this.dosageInstruction = theDosageInstruction; 3639 return this; 3640 } 3641 3642 public boolean hasDosageInstruction() { 3643 if (this.dosageInstruction == null) 3644 return false; 3645 for (Dosage item : this.dosageInstruction) 3646 if (!item.isEmpty()) 3647 return true; 3648 return false; 3649 } 3650 3651 public Dosage addDosageInstruction() { // 3 3652 Dosage t = new Dosage(); 3653 if (this.dosageInstruction == null) 3654 this.dosageInstruction = new ArrayList<Dosage>(); 3655 this.dosageInstruction.add(t); 3656 return t; 3657 } 3658 3659 public MedicationRequest addDosageInstruction(Dosage t) { // 3 3660 if (t == null) 3661 return this; 3662 if (this.dosageInstruction == null) 3663 this.dosageInstruction = new ArrayList<Dosage>(); 3664 this.dosageInstruction.add(t); 3665 return this; 3666 } 3667 3668 /** 3669 * @return The first repetition of repeating field {@link #dosageInstruction}, 3670 * creating it if it does not already exist 3671 */ 3672 public Dosage getDosageInstructionFirstRep() { 3673 if (getDosageInstruction().isEmpty()) { 3674 addDosageInstruction(); 3675 } 3676 return getDosageInstruction().get(0); 3677 } 3678 3679 /** 3680 * @return {@link #dispenseRequest} (Indicates the specific details for the 3681 * dispense or medication supply part of a medication request (also 3682 * known as a Medication Prescription or Medication Order). Note that 3683 * this information is not always sent with the order. There may be in 3684 * some settings (e.g. hospitals) institutional or system support for 3685 * completing the dispense details in the pharmacy department.) 3686 */ 3687 public MedicationRequestDispenseRequestComponent getDispenseRequest() { 3688 if (this.dispenseRequest == null) 3689 if (Configuration.errorOnAutoCreate()) 3690 throw new Error("Attempt to auto-create MedicationRequest.dispenseRequest"); 3691 else if (Configuration.doAutoCreate()) 3692 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); // cc 3693 return this.dispenseRequest; 3694 } 3695 3696 public boolean hasDispenseRequest() { 3697 return this.dispenseRequest != null && !this.dispenseRequest.isEmpty(); 3698 } 3699 3700 /** 3701 * @param value {@link #dispenseRequest} (Indicates the specific details for the 3702 * dispense or medication supply part of a medication request (also 3703 * known as a Medication Prescription or Medication Order). Note 3704 * that this information is not always sent with the order. There 3705 * may be in some settings (e.g. hospitals) institutional or system 3706 * support for completing the dispense details in the pharmacy 3707 * department.) 3708 */ 3709 public MedicationRequest setDispenseRequest(MedicationRequestDispenseRequestComponent value) { 3710 this.dispenseRequest = value; 3711 return this; 3712 } 3713 3714 /** 3715 * @return {@link #substitution} (Indicates whether or not substitution can or 3716 * should be part of the dispense. In some cases, substitution must 3717 * happen, in other cases substitution must not happen. This block 3718 * explains the prescriber's intent. If nothing is specified 3719 * substitution may be done.) 3720 */ 3721 public MedicationRequestSubstitutionComponent getSubstitution() { 3722 if (this.substitution == null) 3723 if (Configuration.errorOnAutoCreate()) 3724 throw new Error("Attempt to auto-create MedicationRequest.substitution"); 3725 else if (Configuration.doAutoCreate()) 3726 this.substitution = new MedicationRequestSubstitutionComponent(); // cc 3727 return this.substitution; 3728 } 3729 3730 public boolean hasSubstitution() { 3731 return this.substitution != null && !this.substitution.isEmpty(); 3732 } 3733 3734 /** 3735 * @param value {@link #substitution} (Indicates whether or not substitution can 3736 * or should be part of the dispense. In some cases, substitution 3737 * must happen, in other cases substitution must not happen. This 3738 * block explains the prescriber's intent. If nothing is specified 3739 * substitution may be done.) 3740 */ 3741 public MedicationRequest setSubstitution(MedicationRequestSubstitutionComponent value) { 3742 this.substitution = value; 3743 return this; 3744 } 3745 3746 /** 3747 * @return {@link #priorPrescription} (A link to a resource representing an 3748 * earlier order related order or prescription.) 3749 */ 3750 public Reference getPriorPrescription() { 3751 if (this.priorPrescription == null) 3752 if (Configuration.errorOnAutoCreate()) 3753 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 3754 else if (Configuration.doAutoCreate()) 3755 this.priorPrescription = new Reference(); // cc 3756 return this.priorPrescription; 3757 } 3758 3759 public boolean hasPriorPrescription() { 3760 return this.priorPrescription != null && !this.priorPrescription.isEmpty(); 3761 } 3762 3763 /** 3764 * @param value {@link #priorPrescription} (A link to a resource representing an 3765 * earlier order related order or prescription.) 3766 */ 3767 public MedicationRequest setPriorPrescription(Reference value) { 3768 this.priorPrescription = value; 3769 return this; 3770 } 3771 3772 /** 3773 * @return {@link #priorPrescription} The actual object that is the target of 3774 * the reference. The reference library doesn't populate this, but you 3775 * can use it to hold the resource if you resolve it. (A link to a 3776 * resource representing an earlier order related order or 3777 * prescription.) 3778 */ 3779 public MedicationRequest getPriorPrescriptionTarget() { 3780 if (this.priorPrescriptionTarget == null) 3781 if (Configuration.errorOnAutoCreate()) 3782 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 3783 else if (Configuration.doAutoCreate()) 3784 this.priorPrescriptionTarget = new MedicationRequest(); // aa 3785 return this.priorPrescriptionTarget; 3786 } 3787 3788 /** 3789 * @param value {@link #priorPrescription} The actual object that is the target 3790 * of the reference. The reference library doesn't use these, but 3791 * you can use it to hold the resource if you resolve it. (A link 3792 * to a resource representing an earlier order related order or 3793 * prescription.) 3794 */ 3795 public MedicationRequest setPriorPrescriptionTarget(MedicationRequest value) { 3796 this.priorPrescriptionTarget = value; 3797 return this; 3798 } 3799 3800 /** 3801 * @return {@link #detectedIssue} (Indicates an actual or potential clinical 3802 * issue with or between one or more active or proposed clinical actions 3803 * for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage 3804 * alert etc.) 3805 */ 3806 public List<Reference> getDetectedIssue() { 3807 if (this.detectedIssue == null) 3808 this.detectedIssue = new ArrayList<Reference>(); 3809 return this.detectedIssue; 3810 } 3811 3812 /** 3813 * @return Returns a reference to <code>this</code> for easy method chaining 3814 */ 3815 public MedicationRequest setDetectedIssue(List<Reference> theDetectedIssue) { 3816 this.detectedIssue = theDetectedIssue; 3817 return this; 3818 } 3819 3820 public boolean hasDetectedIssue() { 3821 if (this.detectedIssue == null) 3822 return false; 3823 for (Reference item : this.detectedIssue) 3824 if (!item.isEmpty()) 3825 return true; 3826 return false; 3827 } 3828 3829 public Reference addDetectedIssue() { // 3 3830 Reference t = new Reference(); 3831 if (this.detectedIssue == null) 3832 this.detectedIssue = new ArrayList<Reference>(); 3833 this.detectedIssue.add(t); 3834 return t; 3835 } 3836 3837 public MedicationRequest addDetectedIssue(Reference t) { // 3 3838 if (t == null) 3839 return this; 3840 if (this.detectedIssue == null) 3841 this.detectedIssue = new ArrayList<Reference>(); 3842 this.detectedIssue.add(t); 3843 return this; 3844 } 3845 3846 /** 3847 * @return The first repetition of repeating field {@link #detectedIssue}, 3848 * creating it if it does not already exist 3849 */ 3850 public Reference getDetectedIssueFirstRep() { 3851 if (getDetectedIssue().isEmpty()) { 3852 addDetectedIssue(); 3853 } 3854 return getDetectedIssue().get(0); 3855 } 3856 3857 /** 3858 * @return {@link #eventHistory} (Links to Provenance records for past versions 3859 * of this resource or fulfilling request or event resources that 3860 * identify key state transitions or updates that are likely to be 3861 * relevant to a user looking at the current version of the resource.) 3862 */ 3863 public List<Reference> getEventHistory() { 3864 if (this.eventHistory == null) 3865 this.eventHistory = new ArrayList<Reference>(); 3866 return this.eventHistory; 3867 } 3868 3869 /** 3870 * @return Returns a reference to <code>this</code> for easy method chaining 3871 */ 3872 public MedicationRequest setEventHistory(List<Reference> theEventHistory) { 3873 this.eventHistory = theEventHistory; 3874 return this; 3875 } 3876 3877 public boolean hasEventHistory() { 3878 if (this.eventHistory == null) 3879 return false; 3880 for (Reference item : this.eventHistory) 3881 if (!item.isEmpty()) 3882 return true; 3883 return false; 3884 } 3885 3886 public Reference addEventHistory() { // 3 3887 Reference t = new Reference(); 3888 if (this.eventHistory == null) 3889 this.eventHistory = new ArrayList<Reference>(); 3890 this.eventHistory.add(t); 3891 return t; 3892 } 3893 3894 public MedicationRequest addEventHistory(Reference t) { // 3 3895 if (t == null) 3896 return this; 3897 if (this.eventHistory == null) 3898 this.eventHistory = new ArrayList<Reference>(); 3899 this.eventHistory.add(t); 3900 return this; 3901 } 3902 3903 /** 3904 * @return The first repetition of repeating field {@link #eventHistory}, 3905 * creating it if it does not already exist 3906 */ 3907 public Reference getEventHistoryFirstRep() { 3908 if (getEventHistory().isEmpty()) { 3909 addEventHistory(); 3910 } 3911 return getEventHistory().get(0); 3912 } 3913 3914 protected void listChildren(List<Property> children) { 3915 super.listChildren(children); 3916 children.add(new Property("identifier", "Identifier", 3917 "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 3918 0, java.lang.Integer.MAX_VALUE, identifier)); 3919 children.add(new Property("status", "code", 3920 "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, 3921 status)); 3922 children.add(new Property("statusReason", "CodeableConcept", 3923 "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason)); 3924 children.add( 3925 new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent)); 3926 children.add(new Property("category", "CodeableConcept", 3927 "Indicates the type of medication request (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).", 3928 0, java.lang.Integer.MAX_VALUE, category)); 3929 children.add(new Property("priority", "code", 3930 "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, 3931 priority)); 3932 children.add(new Property("doNotPerform", "boolean", 3933 "If true indicates that the provider is asking for the medication request not to occur.", 0, 1, doNotPerform)); 3934 children.add(new Property("reported[x]", 3935 "boolean|Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 3936 "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 3937 0, 1, reported)); 3938 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", 3939 "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 3940 0, 1, medication)); 3941 children.add(new Property("subject", "Reference(Patient|Group)", 3942 "A link to a resource representing the person or set of individuals to whom the medication will be given.", 0, 3943 1, subject)); 3944 children.add(new Property("encounter", "Reference(Encounter)", 3945 "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 3946 0, 1, encounter)); 3947 children.add(new Property("supportingInformation", "Reference(Any)", 3948 "Include additional information (for example, patient height and weight) that supports the ordering of the medication.", 3949 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 3950 children.add(new Property("authoredOn", "dateTime", 3951 "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn)); 3952 children.add(new Property("requester", 3953 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 3954 "The individual, organization, or device that initiated the request and has responsibility for its activation.", 3955 0, 1, requester)); 3956 children.add(new Property("performer", 3957 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", 3958 "The specified desired performer of the medication treatment (e.g. the performer of the medication administration).", 3959 0, 1, performer)); 3960 children.add(new Property("performerType", "CodeableConcept", 3961 "Indicates the type of performer of the administration of the medication.", 0, 1, performerType)); 3962 children.add(new Property("recorder", "Reference(Practitioner|PractitionerRole)", 3963 "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 3964 0, 1, recorder)); 3965 children.add(new Property("reasonCode", "CodeableConcept", 3966 "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, 3967 reasonCode)); 3968 children.add(new Property("reasonReference", "Reference(Condition|Observation)", 3969 "Condition or observation that supports why the medication was ordered.", 0, java.lang.Integer.MAX_VALUE, 3970 reasonReference)); 3971 children.add(new Property("instantiatesCanonical", "canonical", 3972 "The URL pointing to a protocol, guideline, orderset, or other definition that is adhered to in whole or in part by this MedicationRequest.", 3973 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 3974 children.add(new Property("instantiatesUri", "uri", 3975 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this MedicationRequest.", 3976 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 3977 children 3978 .add(new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", 3979 "A plan or request that is fulfilled in whole or in part by this medication request.", 0, 3980 java.lang.Integer.MAX_VALUE, basedOn)); 3981 children.add(new Property("groupIdentifier", "Identifier", 3982 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.", 3983 0, 1, groupIdentifier)); 3984 children.add(new Property("courseOfTherapyType", "CodeableConcept", 3985 "The description of the overall patte3rn of the administration of the medication to the patient.", 0, 1, 3986 courseOfTherapyType)); 3987 children.add(new Property("insurance", "Reference(Coverage|ClaimResponse)", 3988 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 3989 0, java.lang.Integer.MAX_VALUE, insurance)); 3990 children.add(new Property("note", "Annotation", 3991 "Extra information about the prescription that could not be conveyed by the other attributes.", 0, 3992 java.lang.Integer.MAX_VALUE, note)); 3993 children 3994 .add(new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 3995 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 3996 children.add(new Property("dispenseRequest", "", 3997 "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 3998 0, 1, dispenseRequest)); 3999 children.add(new Property("substitution", "", 4000 "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 4001 0, 1, substitution)); 4002 children.add(new Property("priorPrescription", "Reference(MedicationRequest)", 4003 "A link to a resource representing an earlier order related order or prescription.", 0, 1, priorPrescription)); 4004 children.add(new Property("detectedIssue", "Reference(DetectedIssue)", 4005 "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 4006 0, java.lang.Integer.MAX_VALUE, detectedIssue)); 4007 children.add(new Property("eventHistory", "Reference(Provenance)", 4008 "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 4009 0, java.lang.Integer.MAX_VALUE, eventHistory)); 4010 } 4011 4012 @Override 4013 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4014 switch (_hash) { 4015 case -1618432855: 4016 /* identifier */ return new Property("identifier", "Identifier", 4017 "Identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 4018 0, java.lang.Integer.MAX_VALUE, identifier); 4019 case -892481550: 4020 /* status */ return new Property("status", "code", 4021 "A code specifying the current state of the order. Generally, this will be active or completed state.", 0, 1, 4022 status); 4023 case 2051346646: 4024 /* statusReason */ return new Property("statusReason", "CodeableConcept", 4025 "Captures the reason for the current state of the MedicationRequest.", 0, 1, statusReason); 4026 case -1183762788: 4027 /* intent */ return new Property("intent", "code", 4028 "Whether the request is a proposal, plan, or an original order.", 0, 1, intent); 4029 case 50511102: 4030 /* category */ return new Property("category", "CodeableConcept", 4031 "Indicates the type of medication request (for example, where the medication is expected to be consumed or administered (i.e. inpatient or outpatient)).", 4032 0, java.lang.Integer.MAX_VALUE, category); 4033 case -1165461084: 4034 /* priority */ return new Property("priority", "code", 4035 "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, 4036 priority); 4037 case -1788508167: 4038 /* doNotPerform */ return new Property("doNotPerform", "boolean", 4039 "If true indicates that the provider is asking for the medication request not to occur.", 0, 1, doNotPerform); 4040 case -241505587: 4041 /* reported[x] */ return new Property("reported[x]", 4042 "boolean|Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 4043 "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 4044 0, 1, reported); 4045 case -427039533: 4046 /* reported */ return new Property("reported[x]", 4047 "boolean|Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 4048 "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 4049 0, 1, reported); 4050 case 1219992533: 4051 /* reportedBoolean */ return new Property("reported[x]", 4052 "boolean|Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 4053 "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 4054 0, 1, reported); 4055 case 1198143416: 4056 /* reportedReference */ return new Property("reported[x]", 4057 "boolean|Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", 4058 "Indicates if this record was captured as a secondary 'reported' record rather than as an original primary source-of-truth record. It may also indicate the source of the report.", 4059 0, 1, reported); 4060 case 1458402129: 4061 /* medication[x] */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 4062 "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 4063 0, 1, medication); 4064 case 1998965455: 4065 /* medication */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 4066 "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 4067 0, 1, medication); 4068 case -209845038: 4069 /* medicationCodeableConcept */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 4070 "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 4071 0, 1, medication); 4072 case 2104315196: 4073 /* medicationReference */ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", 4074 "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 4075 0, 1, medication); 4076 case -1867885268: 4077 /* subject */ return new Property("subject", "Reference(Patient|Group)", 4078 "A link to a resource representing the person or set of individuals to whom the medication will be given.", 0, 4079 1, subject); 4080 case 1524132147: 4081 /* encounter */ return new Property("encounter", "Reference(Encounter)", 4082 "The Encounter during which this [x] was created or to which the creation of this record is tightly associated.", 4083 0, 1, encounter); 4084 case -1248768647: 4085 /* supportingInformation */ return new Property("supportingInformation", "Reference(Any)", 4086 "Include additional information (for example, patient height and weight) that supports the ordering of the medication.", 4087 0, java.lang.Integer.MAX_VALUE, supportingInformation); 4088 case -1500852503: 4089 /* authoredOn */ return new Property("authoredOn", "dateTime", 4090 "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn); 4091 case 693933948: 4092 /* requester */ return new Property("requester", 4093 "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson|Device)", 4094 "The individual, organization, or device that initiated the request and has responsibility for its activation.", 4095 0, 1, requester); 4096 case 481140686: 4097 /* performer */ return new Property("performer", 4098 "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", 4099 "The specified desired performer of the medication treatment (e.g. the performer of the medication administration).", 4100 0, 1, performer); 4101 case -901444568: 4102 /* performerType */ return new Property("performerType", "CodeableConcept", 4103 "Indicates the type of performer of the administration of the medication.", 0, 1, performerType); 4104 case -799233858: 4105 /* recorder */ return new Property("recorder", "Reference(Practitioner|PractitionerRole)", 4106 "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 4107 0, 1, recorder); 4108 case 722137681: 4109 /* reasonCode */ return new Property("reasonCode", "CodeableConcept", 4110 "The reason or the indication for ordering or not ordering the medication.", 0, java.lang.Integer.MAX_VALUE, 4111 reasonCode); 4112 case -1146218137: 4113 /* reasonReference */ return new Property("reasonReference", "Reference(Condition|Observation)", 4114 "Condition or observation that supports why the medication was ordered.", 0, java.lang.Integer.MAX_VALUE, 4115 reasonReference); 4116 case 8911915: 4117 /* instantiatesCanonical */ return new Property("instantiatesCanonical", "canonical", 4118 "The URL pointing to a protocol, guideline, orderset, or other definition that is adhered to in whole or in part by this MedicationRequest.", 4119 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 4120 case -1926393373: 4121 /* instantiatesUri */ return new Property("instantiatesUri", "uri", 4122 "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this MedicationRequest.", 4123 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 4124 case -332612366: 4125 /* basedOn */ return new Property("basedOn", 4126 "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", 4127 "A plan or request that is fulfilled in whole or in part by this medication request.", 0, 4128 java.lang.Integer.MAX_VALUE, basedOn); 4129 case -445338488: 4130 /* groupIdentifier */ return new Property("groupIdentifier", "Identifier", 4131 "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.", 4132 0, 1, groupIdentifier); 4133 case -447282031: 4134 /* courseOfTherapyType */ return new Property("courseOfTherapyType", "CodeableConcept", 4135 "The description of the overall patte3rn of the administration of the medication to the patient.", 0, 1, 4136 courseOfTherapyType); 4137 case 73049818: 4138 /* insurance */ return new Property("insurance", "Reference(Coverage|ClaimResponse)", 4139 "Insurance plans, coverage extensions, pre-authorizations and/or pre-determinations that may be required for delivering the requested service.", 4140 0, java.lang.Integer.MAX_VALUE, insurance); 4141 case 3387378: 4142 /* note */ return new Property("note", "Annotation", 4143 "Extra information about the prescription that could not be conveyed by the other attributes.", 0, 4144 java.lang.Integer.MAX_VALUE, note); 4145 case -1201373865: 4146 /* dosageInstruction */ return new Property("dosageInstruction", "Dosage", 4147 "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, 4148 dosageInstruction); 4149 case 824620658: 4150 /* dispenseRequest */ return new Property("dispenseRequest", "", 4151 "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 4152 0, 1, dispenseRequest); 4153 case 826147581: 4154 /* substitution */ return new Property("substitution", "", 4155 "Indicates whether or not substitution can or should be part of the dispense. In some cases, substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 4156 0, 1, substitution); 4157 case -486355964: 4158 /* priorPrescription */ return new Property("priorPrescription", "Reference(MedicationRequest)", 4159 "A link to a resource representing an earlier order related order or prescription.", 0, 1, priorPrescription); 4160 case 51602295: 4161 /* detectedIssue */ return new Property("detectedIssue", "Reference(DetectedIssue)", 4162 "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 4163 0, java.lang.Integer.MAX_VALUE, detectedIssue); 4164 case 1835190426: 4165 /* eventHistory */ return new Property("eventHistory", "Reference(Provenance)", 4166 "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 4167 0, java.lang.Integer.MAX_VALUE, eventHistory); 4168 default: 4169 return super.getNamedProperty(_hash, _name, _checkValid); 4170 } 4171 4172 } 4173 4174 @Override 4175 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4176 switch (hash) { 4177 case -1618432855: 4178 /* identifier */ return this.identifier == null ? new Base[0] 4179 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4180 case -892481550: 4181 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<MedicationRequestStatus> 4182 case 2051346646: 4183 /* statusReason */ return this.statusReason == null ? new Base[0] : new Base[] { this.statusReason }; // CodeableConcept 4184 case -1183762788: 4185 /* intent */ return this.intent == null ? new Base[0] : new Base[] { this.intent }; // Enumeration<MedicationRequestIntent> 4186 case 50511102: 4187 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 4188 case -1165461084: 4189 /* priority */ return this.priority == null ? new Base[0] : new Base[] { this.priority }; // Enumeration<MedicationRequestPriority> 4190 case -1788508167: 4191 /* doNotPerform */ return this.doNotPerform == null ? new Base[0] : new Base[] { this.doNotPerform }; // BooleanType 4192 case -427039533: 4193 /* reported */ return this.reported == null ? new Base[0] : new Base[] { this.reported }; // Type 4194 case 1998965455: 4195 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 4196 case -1867885268: 4197 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 4198 case 1524132147: 4199 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 4200 case -1248768647: 4201 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 4202 : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 4203 case -1500852503: 4204 /* authoredOn */ return this.authoredOn == null ? new Base[0] : new Base[] { this.authoredOn }; // DateTimeType 4205 case 693933948: 4206 /* requester */ return this.requester == null ? new Base[0] : new Base[] { this.requester }; // Reference 4207 case 481140686: 4208 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 4209 case -901444568: 4210 /* performerType */ return this.performerType == null ? new Base[0] : new Base[] { this.performerType }; // CodeableConcept 4211 case -799233858: 4212 /* recorder */ return this.recorder == null ? new Base[0] : new Base[] { this.recorder }; // Reference 4213 case 722137681: 4214 /* reasonCode */ return this.reasonCode == null ? new Base[0] 4215 : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 4216 case -1146218137: 4217 /* reasonReference */ return this.reasonReference == null ? new Base[0] 4218 : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 4219 case 8911915: 4220 /* instantiatesCanonical */ return this.instantiatesCanonical == null ? new Base[0] 4221 : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 4222 case -1926393373: 4223 /* instantiatesUri */ return this.instantiatesUri == null ? new Base[0] 4224 : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 4225 case -332612366: 4226 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 4227 case -445338488: 4228 /* groupIdentifier */ return this.groupIdentifier == null ? new Base[0] : new Base[] { this.groupIdentifier }; // Identifier 4229 case -447282031: 4230 /* courseOfTherapyType */ return this.courseOfTherapyType == null ? new Base[0] 4231 : new Base[] { this.courseOfTherapyType }; // CodeableConcept 4232 case 73049818: 4233 /* insurance */ return this.insurance == null ? new Base[0] 4234 : this.insurance.toArray(new Base[this.insurance.size()]); // Reference 4235 case 3387378: 4236 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 4237 case -1201373865: 4238 /* dosageInstruction */ return this.dosageInstruction == null ? new Base[0] 4239 : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 4240 case 824620658: 4241 /* dispenseRequest */ return this.dispenseRequest == null ? new Base[0] : new Base[] { this.dispenseRequest }; // MedicationRequestDispenseRequestComponent 4242 case 826147581: 4243 /* substitution */ return this.substitution == null ? new Base[0] : new Base[] { this.substitution }; // MedicationRequestSubstitutionComponent 4244 case -486355964: 4245 /* priorPrescription */ return this.priorPrescription == null ? new Base[0] 4246 : new Base[] { this.priorPrescription }; // Reference 4247 case 51602295: 4248 /* detectedIssue */ return this.detectedIssue == null ? new Base[0] 4249 : this.detectedIssue.toArray(new Base[this.detectedIssue.size()]); // Reference 4250 case 1835190426: 4251 /* eventHistory */ return this.eventHistory == null ? new Base[0] 4252 : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 4253 default: 4254 return super.getProperty(hash, name, checkValid); 4255 } 4256 4257 } 4258 4259 @Override 4260 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4261 switch (hash) { 4262 case -1618432855: // identifier 4263 this.getIdentifier().add(castToIdentifier(value)); // Identifier 4264 return value; 4265 case -892481550: // status 4266 value = new MedicationRequestStatusEnumFactory().fromType(castToCode(value)); 4267 this.status = (Enumeration) value; // Enumeration<MedicationRequestStatus> 4268 return value; 4269 case 2051346646: // statusReason 4270 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4271 return value; 4272 case -1183762788: // intent 4273 value = new MedicationRequestIntentEnumFactory().fromType(castToCode(value)); 4274 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 4275 return value; 4276 case 50511102: // category 4277 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 4278 return value; 4279 case -1165461084: // priority 4280 value = new MedicationRequestPriorityEnumFactory().fromType(castToCode(value)); 4281 this.priority = (Enumeration) value; // Enumeration<MedicationRequestPriority> 4282 return value; 4283 case -1788508167: // doNotPerform 4284 this.doNotPerform = castToBoolean(value); // BooleanType 4285 return value; 4286 case -427039533: // reported 4287 this.reported = castToType(value); // Type 4288 return value; 4289 case 1998965455: // medication 4290 this.medication = castToType(value); // Type 4291 return value; 4292 case -1867885268: // subject 4293 this.subject = castToReference(value); // Reference 4294 return value; 4295 case 1524132147: // encounter 4296 this.encounter = castToReference(value); // Reference 4297 return value; 4298 case -1248768647: // supportingInformation 4299 this.getSupportingInformation().add(castToReference(value)); // Reference 4300 return value; 4301 case -1500852503: // authoredOn 4302 this.authoredOn = castToDateTime(value); // DateTimeType 4303 return value; 4304 case 693933948: // requester 4305 this.requester = castToReference(value); // Reference 4306 return value; 4307 case 481140686: // performer 4308 this.performer = castToReference(value); // Reference 4309 return value; 4310 case -901444568: // performerType 4311 this.performerType = castToCodeableConcept(value); // CodeableConcept 4312 return value; 4313 case -799233858: // recorder 4314 this.recorder = castToReference(value); // Reference 4315 return value; 4316 case 722137681: // reasonCode 4317 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 4318 return value; 4319 case -1146218137: // reasonReference 4320 this.getReasonReference().add(castToReference(value)); // Reference 4321 return value; 4322 case 8911915: // instantiatesCanonical 4323 this.getInstantiatesCanonical().add(castToCanonical(value)); // CanonicalType 4324 return value; 4325 case -1926393373: // instantiatesUri 4326 this.getInstantiatesUri().add(castToUri(value)); // UriType 4327 return value; 4328 case -332612366: // basedOn 4329 this.getBasedOn().add(castToReference(value)); // Reference 4330 return value; 4331 case -445338488: // groupIdentifier 4332 this.groupIdentifier = castToIdentifier(value); // Identifier 4333 return value; 4334 case -447282031: // courseOfTherapyType 4335 this.courseOfTherapyType = castToCodeableConcept(value); // CodeableConcept 4336 return value; 4337 case 73049818: // insurance 4338 this.getInsurance().add(castToReference(value)); // Reference 4339 return value; 4340 case 3387378: // note 4341 this.getNote().add(castToAnnotation(value)); // Annotation 4342 return value; 4343 case -1201373865: // dosageInstruction 4344 this.getDosageInstruction().add(castToDosage(value)); // Dosage 4345 return value; 4346 case 824620658: // dispenseRequest 4347 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 4348 return value; 4349 case 826147581: // substitution 4350 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 4351 return value; 4352 case -486355964: // priorPrescription 4353 this.priorPrescription = castToReference(value); // Reference 4354 return value; 4355 case 51602295: // detectedIssue 4356 this.getDetectedIssue().add(castToReference(value)); // Reference 4357 return value; 4358 case 1835190426: // eventHistory 4359 this.getEventHistory().add(castToReference(value)); // Reference 4360 return value; 4361 default: 4362 return super.setProperty(hash, name, value); 4363 } 4364 4365 } 4366 4367 @Override 4368 public Base setProperty(String name, Base value) throws FHIRException { 4369 if (name.equals("identifier")) { 4370 this.getIdentifier().add(castToIdentifier(value)); 4371 } else if (name.equals("status")) { 4372 value = new MedicationRequestStatusEnumFactory().fromType(castToCode(value)); 4373 this.status = (Enumeration) value; // Enumeration<MedicationRequestStatus> 4374 } else if (name.equals("statusReason")) { 4375 this.statusReason = castToCodeableConcept(value); // CodeableConcept 4376 } else if (name.equals("intent")) { 4377 value = new MedicationRequestIntentEnumFactory().fromType(castToCode(value)); 4378 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 4379 } else if (name.equals("category")) { 4380 this.getCategory().add(castToCodeableConcept(value)); 4381 } else if (name.equals("priority")) { 4382 value = new MedicationRequestPriorityEnumFactory().fromType(castToCode(value)); 4383 this.priority = (Enumeration) value; // Enumeration<MedicationRequestPriority> 4384 } else if (name.equals("doNotPerform")) { 4385 this.doNotPerform = castToBoolean(value); // BooleanType 4386 } else if (name.equals("reported[x]")) { 4387 this.reported = castToType(value); // Type 4388 } else if (name.equals("medication[x]")) { 4389 this.medication = castToType(value); // Type 4390 } else if (name.equals("subject")) { 4391 this.subject = castToReference(value); // Reference 4392 } else if (name.equals("encounter")) { 4393 this.encounter = castToReference(value); // Reference 4394 } else if (name.equals("supportingInformation")) { 4395 this.getSupportingInformation().add(castToReference(value)); 4396 } else if (name.equals("authoredOn")) { 4397 this.authoredOn = castToDateTime(value); // DateTimeType 4398 } else if (name.equals("requester")) { 4399 this.requester = castToReference(value); // Reference 4400 } else if (name.equals("performer")) { 4401 this.performer = castToReference(value); // Reference 4402 } else if (name.equals("performerType")) { 4403 this.performerType = castToCodeableConcept(value); // CodeableConcept 4404 } else if (name.equals("recorder")) { 4405 this.recorder = castToReference(value); // Reference 4406 } else if (name.equals("reasonCode")) { 4407 this.getReasonCode().add(castToCodeableConcept(value)); 4408 } else if (name.equals("reasonReference")) { 4409 this.getReasonReference().add(castToReference(value)); 4410 } else if (name.equals("instantiatesCanonical")) { 4411 this.getInstantiatesCanonical().add(castToCanonical(value)); 4412 } else if (name.equals("instantiatesUri")) { 4413 this.getInstantiatesUri().add(castToUri(value)); 4414 } else if (name.equals("basedOn")) { 4415 this.getBasedOn().add(castToReference(value)); 4416 } else if (name.equals("groupIdentifier")) { 4417 this.groupIdentifier = castToIdentifier(value); // Identifier 4418 } else if (name.equals("courseOfTherapyType")) { 4419 this.courseOfTherapyType = castToCodeableConcept(value); // CodeableConcept 4420 } else if (name.equals("insurance")) { 4421 this.getInsurance().add(castToReference(value)); 4422 } else if (name.equals("note")) { 4423 this.getNote().add(castToAnnotation(value)); 4424 } else if (name.equals("dosageInstruction")) { 4425 this.getDosageInstruction().add(castToDosage(value)); 4426 } else if (name.equals("dispenseRequest")) { 4427 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 4428 } else if (name.equals("substitution")) { 4429 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 4430 } else if (name.equals("priorPrescription")) { 4431 this.priorPrescription = castToReference(value); // Reference 4432 } else if (name.equals("detectedIssue")) { 4433 this.getDetectedIssue().add(castToReference(value)); 4434 } else if (name.equals("eventHistory")) { 4435 this.getEventHistory().add(castToReference(value)); 4436 } else 4437 return super.setProperty(name, value); 4438 return value; 4439 } 4440 4441 @Override 4442 public void removeChild(String name, Base value) throws FHIRException { 4443 if (name.equals("identifier")) { 4444 this.getIdentifier().remove(castToIdentifier(value)); 4445 } else if (name.equals("status")) { 4446 this.status = null; 4447 } else if (name.equals("statusReason")) { 4448 this.statusReason = null; 4449 } else if (name.equals("intent")) { 4450 this.intent = null; 4451 } else if (name.equals("category")) { 4452 this.getCategory().remove(castToCodeableConcept(value)); 4453 } else if (name.equals("priority")) { 4454 this.priority = null; 4455 } else if (name.equals("doNotPerform")) { 4456 this.doNotPerform = null; 4457 } else if (name.equals("reported[x]")) { 4458 this.reported = null; 4459 } else if (name.equals("medication[x]")) { 4460 this.medication = null; 4461 } else if (name.equals("subject")) { 4462 this.subject = null; 4463 } else if (name.equals("encounter")) { 4464 this.encounter = null; 4465 } else if (name.equals("supportingInformation")) { 4466 this.getSupportingInformation().remove(castToReference(value)); 4467 } else if (name.equals("authoredOn")) { 4468 this.authoredOn = null; 4469 } else if (name.equals("requester")) { 4470 this.requester = null; 4471 } else if (name.equals("performer")) { 4472 this.performer = null; 4473 } else if (name.equals("performerType")) { 4474 this.performerType = null; 4475 } else if (name.equals("recorder")) { 4476 this.recorder = null; 4477 } else if (name.equals("reasonCode")) { 4478 this.getReasonCode().remove(castToCodeableConcept(value)); 4479 } else if (name.equals("reasonReference")) { 4480 this.getReasonReference().remove(castToReference(value)); 4481 } else if (name.equals("instantiatesCanonical")) { 4482 this.getInstantiatesCanonical().remove(castToCanonical(value)); 4483 } else if (name.equals("instantiatesUri")) { 4484 this.getInstantiatesUri().remove(castToUri(value)); 4485 } else if (name.equals("basedOn")) { 4486 this.getBasedOn().remove(castToReference(value)); 4487 } else if (name.equals("groupIdentifier")) { 4488 this.groupIdentifier = null; 4489 } else if (name.equals("courseOfTherapyType")) { 4490 this.courseOfTherapyType = null; 4491 } else if (name.equals("insurance")) { 4492 this.getInsurance().remove(castToReference(value)); 4493 } else if (name.equals("note")) { 4494 this.getNote().remove(castToAnnotation(value)); 4495 } else if (name.equals("dosageInstruction")) { 4496 this.getDosageInstruction().remove(castToDosage(value)); 4497 } else if (name.equals("dispenseRequest")) { 4498 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 4499 } else if (name.equals("substitution")) { 4500 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 4501 } else if (name.equals("priorPrescription")) { 4502 this.priorPrescription = null; 4503 } else if (name.equals("detectedIssue")) { 4504 this.getDetectedIssue().remove(castToReference(value)); 4505 } else if (name.equals("eventHistory")) { 4506 this.getEventHistory().remove(castToReference(value)); 4507 } else 4508 super.removeChild(name, value); 4509 4510 } 4511 4512 @Override 4513 public Base makeProperty(int hash, String name) throws FHIRException { 4514 switch (hash) { 4515 case -1618432855: 4516 return addIdentifier(); 4517 case -892481550: 4518 return getStatusElement(); 4519 case 2051346646: 4520 return getStatusReason(); 4521 case -1183762788: 4522 return getIntentElement(); 4523 case 50511102: 4524 return addCategory(); 4525 case -1165461084: 4526 return getPriorityElement(); 4527 case -1788508167: 4528 return getDoNotPerformElement(); 4529 case -241505587: 4530 return getReported(); 4531 case -427039533: 4532 return getReported(); 4533 case 1458402129: 4534 return getMedication(); 4535 case 1998965455: 4536 return getMedication(); 4537 case -1867885268: 4538 return getSubject(); 4539 case 1524132147: 4540 return getEncounter(); 4541 case -1248768647: 4542 return addSupportingInformation(); 4543 case -1500852503: 4544 return getAuthoredOnElement(); 4545 case 693933948: 4546 return getRequester(); 4547 case 481140686: 4548 return getPerformer(); 4549 case -901444568: 4550 return getPerformerType(); 4551 case -799233858: 4552 return getRecorder(); 4553 case 722137681: 4554 return addReasonCode(); 4555 case -1146218137: 4556 return addReasonReference(); 4557 case 8911915: 4558 return addInstantiatesCanonicalElement(); 4559 case -1926393373: 4560 return addInstantiatesUriElement(); 4561 case -332612366: 4562 return addBasedOn(); 4563 case -445338488: 4564 return getGroupIdentifier(); 4565 case -447282031: 4566 return getCourseOfTherapyType(); 4567 case 73049818: 4568 return addInsurance(); 4569 case 3387378: 4570 return addNote(); 4571 case -1201373865: 4572 return addDosageInstruction(); 4573 case 824620658: 4574 return getDispenseRequest(); 4575 case 826147581: 4576 return getSubstitution(); 4577 case -486355964: 4578 return getPriorPrescription(); 4579 case 51602295: 4580 return addDetectedIssue(); 4581 case 1835190426: 4582 return addEventHistory(); 4583 default: 4584 return super.makeProperty(hash, name); 4585 } 4586 4587 } 4588 4589 @Override 4590 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4591 switch (hash) { 4592 case -1618432855: 4593 /* identifier */ return new String[] { "Identifier" }; 4594 case -892481550: 4595 /* status */ return new String[] { "code" }; 4596 case 2051346646: 4597 /* statusReason */ return new String[] { "CodeableConcept" }; 4598 case -1183762788: 4599 /* intent */ return new String[] { "code" }; 4600 case 50511102: 4601 /* category */ return new String[] { "CodeableConcept" }; 4602 case -1165461084: 4603 /* priority */ return new String[] { "code" }; 4604 case -1788508167: 4605 /* doNotPerform */ return new String[] { "boolean" }; 4606 case -427039533: 4607 /* reported */ return new String[] { "boolean", "Reference" }; 4608 case 1998965455: 4609 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 4610 case -1867885268: 4611 /* subject */ return new String[] { "Reference" }; 4612 case 1524132147: 4613 /* encounter */ return new String[] { "Reference" }; 4614 case -1248768647: 4615 /* supportingInformation */ return new String[] { "Reference" }; 4616 case -1500852503: 4617 /* authoredOn */ return new String[] { "dateTime" }; 4618 case 693933948: 4619 /* requester */ return new String[] { "Reference" }; 4620 case 481140686: 4621 /* performer */ return new String[] { "Reference" }; 4622 case -901444568: 4623 /* performerType */ return new String[] { "CodeableConcept" }; 4624 case -799233858: 4625 /* recorder */ return new String[] { "Reference" }; 4626 case 722137681: 4627 /* reasonCode */ return new String[] { "CodeableConcept" }; 4628 case -1146218137: 4629 /* reasonReference */ return new String[] { "Reference" }; 4630 case 8911915: 4631 /* instantiatesCanonical */ return new String[] { "canonical" }; 4632 case -1926393373: 4633 /* instantiatesUri */ return new String[] { "uri" }; 4634 case -332612366: 4635 /* basedOn */ return new String[] { "Reference" }; 4636 case -445338488: 4637 /* groupIdentifier */ return new String[] { "Identifier" }; 4638 case -447282031: 4639 /* courseOfTherapyType */ return new String[] { "CodeableConcept" }; 4640 case 73049818: 4641 /* insurance */ return new String[] { "Reference" }; 4642 case 3387378: 4643 /* note */ return new String[] { "Annotation" }; 4644 case -1201373865: 4645 /* dosageInstruction */ return new String[] { "Dosage" }; 4646 case 824620658: 4647 /* dispenseRequest */ return new String[] {}; 4648 case 826147581: 4649 /* substitution */ return new String[] {}; 4650 case -486355964: 4651 /* priorPrescription */ return new String[] { "Reference" }; 4652 case 51602295: 4653 /* detectedIssue */ return new String[] { "Reference" }; 4654 case 1835190426: 4655 /* eventHistory */ return new String[] { "Reference" }; 4656 default: 4657 return super.getTypesForProperty(hash, name); 4658 } 4659 4660 } 4661 4662 @Override 4663 public Base addChild(String name) throws FHIRException { 4664 if (name.equals("identifier")) { 4665 return addIdentifier(); 4666 } else if (name.equals("status")) { 4667 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.status"); 4668 } else if (name.equals("statusReason")) { 4669 this.statusReason = new CodeableConcept(); 4670 return this.statusReason; 4671 } else if (name.equals("intent")) { 4672 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.intent"); 4673 } else if (name.equals("category")) { 4674 return addCategory(); 4675 } else if (name.equals("priority")) { 4676 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.priority"); 4677 } else if (name.equals("doNotPerform")) { 4678 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.doNotPerform"); 4679 } else if (name.equals("reportedBoolean")) { 4680 this.reported = new BooleanType(); 4681 return this.reported; 4682 } else if (name.equals("reportedReference")) { 4683 this.reported = new Reference(); 4684 return this.reported; 4685 } else if (name.equals("medicationCodeableConcept")) { 4686 this.medication = new CodeableConcept(); 4687 return this.medication; 4688 } else if (name.equals("medicationReference")) { 4689 this.medication = new Reference(); 4690 return this.medication; 4691 } else if (name.equals("subject")) { 4692 this.subject = new Reference(); 4693 return this.subject; 4694 } else if (name.equals("encounter")) { 4695 this.encounter = new Reference(); 4696 return this.encounter; 4697 } else if (name.equals("supportingInformation")) { 4698 return addSupportingInformation(); 4699 } else if (name.equals("authoredOn")) { 4700 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.authoredOn"); 4701 } else if (name.equals("requester")) { 4702 this.requester = new Reference(); 4703 return this.requester; 4704 } else if (name.equals("performer")) { 4705 this.performer = new Reference(); 4706 return this.performer; 4707 } else if (name.equals("performerType")) { 4708 this.performerType = new CodeableConcept(); 4709 return this.performerType; 4710 } else if (name.equals("recorder")) { 4711 this.recorder = new Reference(); 4712 return this.recorder; 4713 } else if (name.equals("reasonCode")) { 4714 return addReasonCode(); 4715 } else if (name.equals("reasonReference")) { 4716 return addReasonReference(); 4717 } else if (name.equals("instantiatesCanonical")) { 4718 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.instantiatesCanonical"); 4719 } else if (name.equals("instantiatesUri")) { 4720 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.instantiatesUri"); 4721 } else if (name.equals("basedOn")) { 4722 return addBasedOn(); 4723 } else if (name.equals("groupIdentifier")) { 4724 this.groupIdentifier = new Identifier(); 4725 return this.groupIdentifier; 4726 } else if (name.equals("courseOfTherapyType")) { 4727 this.courseOfTherapyType = new CodeableConcept(); 4728 return this.courseOfTherapyType; 4729 } else if (name.equals("insurance")) { 4730 return addInsurance(); 4731 } else if (name.equals("note")) { 4732 return addNote(); 4733 } else if (name.equals("dosageInstruction")) { 4734 return addDosageInstruction(); 4735 } else if (name.equals("dispenseRequest")) { 4736 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); 4737 return this.dispenseRequest; 4738 } else if (name.equals("substitution")) { 4739 this.substitution = new MedicationRequestSubstitutionComponent(); 4740 return this.substitution; 4741 } else if (name.equals("priorPrescription")) { 4742 this.priorPrescription = new Reference(); 4743 return this.priorPrescription; 4744 } else if (name.equals("detectedIssue")) { 4745 return addDetectedIssue(); 4746 } else if (name.equals("eventHistory")) { 4747 return addEventHistory(); 4748 } else 4749 return super.addChild(name); 4750 } 4751 4752 public String fhirType() { 4753 return "MedicationRequest"; 4754 4755 } 4756 4757 public MedicationRequest copy() { 4758 MedicationRequest dst = new MedicationRequest(); 4759 copyValues(dst); 4760 return dst; 4761 } 4762 4763 public void copyValues(MedicationRequest dst) { 4764 super.copyValues(dst); 4765 if (identifier != null) { 4766 dst.identifier = new ArrayList<Identifier>(); 4767 for (Identifier i : identifier) 4768 dst.identifier.add(i.copy()); 4769 } 4770 ; 4771 dst.status = status == null ? null : status.copy(); 4772 dst.statusReason = statusReason == null ? null : statusReason.copy(); 4773 dst.intent = intent == null ? null : intent.copy(); 4774 if (category != null) { 4775 dst.category = new ArrayList<CodeableConcept>(); 4776 for (CodeableConcept i : category) 4777 dst.category.add(i.copy()); 4778 } 4779 ; 4780 dst.priority = priority == null ? null : priority.copy(); 4781 dst.doNotPerform = doNotPerform == null ? null : doNotPerform.copy(); 4782 dst.reported = reported == null ? null : reported.copy(); 4783 dst.medication = medication == null ? null : medication.copy(); 4784 dst.subject = subject == null ? null : subject.copy(); 4785 dst.encounter = encounter == null ? null : encounter.copy(); 4786 if (supportingInformation != null) { 4787 dst.supportingInformation = new ArrayList<Reference>(); 4788 for (Reference i : supportingInformation) 4789 dst.supportingInformation.add(i.copy()); 4790 } 4791 ; 4792 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 4793 dst.requester = requester == null ? null : requester.copy(); 4794 dst.performer = performer == null ? null : performer.copy(); 4795 dst.performerType = performerType == null ? null : performerType.copy(); 4796 dst.recorder = recorder == null ? null : recorder.copy(); 4797 if (reasonCode != null) { 4798 dst.reasonCode = new ArrayList<CodeableConcept>(); 4799 for (CodeableConcept i : reasonCode) 4800 dst.reasonCode.add(i.copy()); 4801 } 4802 ; 4803 if (reasonReference != null) { 4804 dst.reasonReference = new ArrayList<Reference>(); 4805 for (Reference i : reasonReference) 4806 dst.reasonReference.add(i.copy()); 4807 } 4808 ; 4809 if (instantiatesCanonical != null) { 4810 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 4811 for (CanonicalType i : instantiatesCanonical) 4812 dst.instantiatesCanonical.add(i.copy()); 4813 } 4814 ; 4815 if (instantiatesUri != null) { 4816 dst.instantiatesUri = new ArrayList<UriType>(); 4817 for (UriType i : instantiatesUri) 4818 dst.instantiatesUri.add(i.copy()); 4819 } 4820 ; 4821 if (basedOn != null) { 4822 dst.basedOn = new ArrayList<Reference>(); 4823 for (Reference i : basedOn) 4824 dst.basedOn.add(i.copy()); 4825 } 4826 ; 4827 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 4828 dst.courseOfTherapyType = courseOfTherapyType == null ? null : courseOfTherapyType.copy(); 4829 if (insurance != null) { 4830 dst.insurance = new ArrayList<Reference>(); 4831 for (Reference i : insurance) 4832 dst.insurance.add(i.copy()); 4833 } 4834 ; 4835 if (note != null) { 4836 dst.note = new ArrayList<Annotation>(); 4837 for (Annotation i : note) 4838 dst.note.add(i.copy()); 4839 } 4840 ; 4841 if (dosageInstruction != null) { 4842 dst.dosageInstruction = new ArrayList<Dosage>(); 4843 for (Dosage i : dosageInstruction) 4844 dst.dosageInstruction.add(i.copy()); 4845 } 4846 ; 4847 dst.dispenseRequest = dispenseRequest == null ? null : dispenseRequest.copy(); 4848 dst.substitution = substitution == null ? null : substitution.copy(); 4849 dst.priorPrescription = priorPrescription == null ? null : priorPrescription.copy(); 4850 if (detectedIssue != null) { 4851 dst.detectedIssue = new ArrayList<Reference>(); 4852 for (Reference i : detectedIssue) 4853 dst.detectedIssue.add(i.copy()); 4854 } 4855 ; 4856 if (eventHistory != null) { 4857 dst.eventHistory = new ArrayList<Reference>(); 4858 for (Reference i : eventHistory) 4859 dst.eventHistory.add(i.copy()); 4860 } 4861 ; 4862 } 4863 4864 protected MedicationRequest typedCopy() { 4865 return copy(); 4866 } 4867 4868 @Override 4869 public boolean equalsDeep(Base other_) { 4870 if (!super.equalsDeep(other_)) 4871 return false; 4872 if (!(other_ instanceof MedicationRequest)) 4873 return false; 4874 MedicationRequest o = (MedicationRequest) other_; 4875 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 4876 && compareDeep(statusReason, o.statusReason, true) && compareDeep(intent, o.intent, true) 4877 && compareDeep(category, o.category, true) && compareDeep(priority, o.priority, true) 4878 && compareDeep(doNotPerform, o.doNotPerform, true) && compareDeep(reported, o.reported, true) 4879 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 4880 && compareDeep(encounter, o.encounter, true) 4881 && compareDeep(supportingInformation, o.supportingInformation, true) 4882 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) 4883 && compareDeep(performer, o.performer, true) && compareDeep(performerType, o.performerType, true) 4884 && compareDeep(recorder, o.recorder, true) && compareDeep(reasonCode, o.reasonCode, true) 4885 && compareDeep(reasonReference, o.reasonReference, true) 4886 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 4887 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(basedOn, o.basedOn, true) 4888 && compareDeep(groupIdentifier, o.groupIdentifier, true) 4889 && compareDeep(courseOfTherapyType, o.courseOfTherapyType, true) && compareDeep(insurance, o.insurance, true) 4890 && compareDeep(note, o.note, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 4891 && compareDeep(dispenseRequest, o.dispenseRequest, true) && compareDeep(substitution, o.substitution, true) 4892 && compareDeep(priorPrescription, o.priorPrescription, true) 4893 && compareDeep(detectedIssue, o.detectedIssue, true) && compareDeep(eventHistory, o.eventHistory, true); 4894 } 4895 4896 @Override 4897 public boolean equalsShallow(Base other_) { 4898 if (!super.equalsShallow(other_)) 4899 return false; 4900 if (!(other_ instanceof MedicationRequest)) 4901 return false; 4902 MedicationRequest o = (MedicationRequest) other_; 4903 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) 4904 && compareValues(priority, o.priority, true) && compareValues(doNotPerform, o.doNotPerform, true) 4905 && compareValues(authoredOn, o.authoredOn, true) 4906 && compareValues(instantiatesCanonical, o.instantiatesCanonical, true) 4907 && compareValues(instantiatesUri, o.instantiatesUri, true); 4908 } 4909 4910 public boolean isEmpty() { 4911 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusReason, intent, category, 4912 priority, doNotPerform, reported, medication, subject, encounter, supportingInformation, authoredOn, requester, 4913 performer, performerType, recorder, reasonCode, reasonReference, instantiatesCanonical, instantiatesUri, 4914 basedOn, groupIdentifier, courseOfTherapyType, insurance, note, dosageInstruction, dispenseRequest, 4915 substitution, priorPrescription, detectedIssue, eventHistory); 4916 } 4917 4918 @Override 4919 public ResourceType getResourceType() { 4920 return ResourceType.MedicationRequest; 4921 } 4922 4923 /** 4924 * Search parameter: <b>requester</b> 4925 * <p> 4926 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4927 * Type: <b>reference</b><br> 4928 * Path: <b>MedicationRequest.requester</b><br> 4929 * </p> 4930 */ 4931 @SearchParamDefinition(name = "requester", path = "MedicationRequest.requester", description = "Returns prescriptions prescribed by this prescriber", type = "reference", providesMembershipIn = { 4932 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner") }, target = { Device.class, 4933 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4934 public static final String SP_REQUESTER = "requester"; 4935 /** 4936 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 4937 * <p> 4938 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 4939 * Type: <b>reference</b><br> 4940 * Path: <b>MedicationRequest.requester</b><br> 4941 * </p> 4942 */ 4943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4944 SP_REQUESTER); 4945 4946 /** 4947 * Constant for fluent queries to be used to add include statements. Specifies 4948 * the path value of "<b>MedicationRequest:requester</b>". 4949 */ 4950 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include( 4951 "MedicationRequest:requester").toLocked(); 4952 4953 /** 4954 * Search parameter: <b>date</b> 4955 * <p> 4956 * Description: <b>Returns medication request to be administered on a specific 4957 * date</b><br> 4958 * Type: <b>date</b><br> 4959 * Path: <b>MedicationRequest.dosageInstruction.timing.event</b><br> 4960 * </p> 4961 */ 4962 @SearchParamDefinition(name = "date", path = "MedicationRequest.dosageInstruction.timing.event", description = "Returns medication request to be administered on a specific date", type = "date") 4963 public static final String SP_DATE = "date"; 4964 /** 4965 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4966 * <p> 4967 * Description: <b>Returns medication request to be administered on a specific 4968 * date</b><br> 4969 * Type: <b>date</b><br> 4970 * Path: <b>MedicationRequest.dosageInstruction.timing.event</b><br> 4971 * </p> 4972 */ 4973 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4974 SP_DATE); 4975 4976 /** 4977 * Search parameter: <b>identifier</b> 4978 * <p> 4979 * Description: <b>Return prescriptions with this external identifier</b><br> 4980 * Type: <b>token</b><br> 4981 * Path: <b>MedicationRequest.identifier</b><br> 4982 * </p> 4983 */ 4984 @SearchParamDefinition(name = "identifier", path = "MedicationRequest.identifier", description = "Return prescriptions with this external identifier", type = "token") 4985 public static final String SP_IDENTIFIER = "identifier"; 4986 /** 4987 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4988 * <p> 4989 * Description: <b>Return prescriptions with this external identifier</b><br> 4990 * Type: <b>token</b><br> 4991 * Path: <b>MedicationRequest.identifier</b><br> 4992 * </p> 4993 */ 4994 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4995 SP_IDENTIFIER); 4996 4997 /** 4998 * Search parameter: <b>intended-dispenser</b> 4999 * <p> 5000 * Description: <b>Returns prescriptions intended to be dispensed by this 5001 * Organization</b><br> 5002 * Type: <b>reference</b><br> 5003 * Path: <b>MedicationRequest.dispenseRequest.performer</b><br> 5004 * </p> 5005 */ 5006 @SearchParamDefinition(name = "intended-dispenser", path = "MedicationRequest.dispenseRequest.performer", description = "Returns prescriptions intended to be dispensed by this Organization", type = "reference", target = { 5007 Organization.class }) 5008 public static final String SP_INTENDED_DISPENSER = "intended-dispenser"; 5009 /** 5010 * <b>Fluent Client</b> search parameter constant for <b>intended-dispenser</b> 5011 * <p> 5012 * Description: <b>Returns prescriptions intended to be dispensed by this 5013 * Organization</b><br> 5014 * Type: <b>reference</b><br> 5015 * Path: <b>MedicationRequest.dispenseRequest.performer</b><br> 5016 * </p> 5017 */ 5018 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_DISPENSER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5019 SP_INTENDED_DISPENSER); 5020 5021 /** 5022 * Constant for fluent queries to be used to add include statements. Specifies 5023 * the path value of "<b>MedicationRequest:intended-dispenser</b>". 5024 */ 5025 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_DISPENSER = new ca.uhn.fhir.model.api.Include( 5026 "MedicationRequest:intended-dispenser").toLocked(); 5027 5028 /** 5029 * Search parameter: <b>authoredon</b> 5030 * <p> 5031 * Description: <b>Return prescriptions written on this date</b><br> 5032 * Type: <b>date</b><br> 5033 * Path: <b>MedicationRequest.authoredOn</b><br> 5034 * </p> 5035 */ 5036 @SearchParamDefinition(name = "authoredon", path = "MedicationRequest.authoredOn", description = "Return prescriptions written on this date", type = "date") 5037 public static final String SP_AUTHOREDON = "authoredon"; 5038 /** 5039 * <b>Fluent Client</b> search parameter constant for <b>authoredon</b> 5040 * <p> 5041 * Description: <b>Return prescriptions written on this date</b><br> 5042 * Type: <b>date</b><br> 5043 * Path: <b>MedicationRequest.authoredOn</b><br> 5044 * </p> 5045 */ 5046 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHOREDON = new ca.uhn.fhir.rest.gclient.DateClientParam( 5047 SP_AUTHOREDON); 5048 5049 /** 5050 * Search parameter: <b>code</b> 5051 * <p> 5052 * Description: <b>Return prescriptions of this medication code</b><br> 5053 * Type: <b>token</b><br> 5054 * Path: <b>MedicationRequest.medicationCodeableConcept</b><br> 5055 * </p> 5056 */ 5057 @SearchParamDefinition(name = "code", path = "(MedicationRequest.medication as CodeableConcept)", description = "Return prescriptions of this medication code", type = "token") 5058 public static final String SP_CODE = "code"; 5059 /** 5060 * <b>Fluent Client</b> search parameter constant for <b>code</b> 5061 * <p> 5062 * Description: <b>Return prescriptions of this medication code</b><br> 5063 * Type: <b>token</b><br> 5064 * Path: <b>MedicationRequest.medicationCodeableConcept</b><br> 5065 * </p> 5066 */ 5067 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5068 SP_CODE); 5069 5070 /** 5071 * Search parameter: <b>subject</b> 5072 * <p> 5073 * Description: <b>The identity of a patient to list orders for</b><br> 5074 * Type: <b>reference</b><br> 5075 * Path: <b>MedicationRequest.subject</b><br> 5076 * </p> 5077 */ 5078 @SearchParamDefinition(name = "subject", path = "MedicationRequest.subject", description = "The identity of a patient to list orders for", type = "reference", providesMembershipIn = { 5079 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Group.class, Patient.class }) 5080 public static final String SP_SUBJECT = "subject"; 5081 /** 5082 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 5083 * <p> 5084 * Description: <b>The identity of a patient to list orders for</b><br> 5085 * Type: <b>reference</b><br> 5086 * Path: <b>MedicationRequest.subject</b><br> 5087 * </p> 5088 */ 5089 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5090 SP_SUBJECT); 5091 5092 /** 5093 * Constant for fluent queries to be used to add include statements. Specifies 5094 * the path value of "<b>MedicationRequest:subject</b>". 5095 */ 5096 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 5097 "MedicationRequest:subject").toLocked(); 5098 5099 /** 5100 * Search parameter: <b>medication</b> 5101 * <p> 5102 * Description: <b>Return prescriptions for this medication reference</b><br> 5103 * Type: <b>reference</b><br> 5104 * Path: <b>MedicationRequest.medicationReference</b><br> 5105 * </p> 5106 */ 5107 @SearchParamDefinition(name = "medication", path = "(MedicationRequest.medication as Reference)", description = "Return prescriptions for this medication reference", type = "reference", target = { 5108 Medication.class }) 5109 public static final String SP_MEDICATION = "medication"; 5110 /** 5111 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 5112 * <p> 5113 * Description: <b>Return prescriptions for this medication reference</b><br> 5114 * Type: <b>reference</b><br> 5115 * Path: <b>MedicationRequest.medicationReference</b><br> 5116 * </p> 5117 */ 5118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5119 SP_MEDICATION); 5120 5121 /** 5122 * Constant for fluent queries to be used to add include statements. Specifies 5123 * the path value of "<b>MedicationRequest:medication</b>". 5124 */ 5125 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include( 5126 "MedicationRequest:medication").toLocked(); 5127 5128 /** 5129 * Search parameter: <b>encounter</b> 5130 * <p> 5131 * Description: <b>Return prescriptions with this encounter identifier</b><br> 5132 * Type: <b>reference</b><br> 5133 * Path: <b>MedicationRequest.encounter</b><br> 5134 * </p> 5135 */ 5136 @SearchParamDefinition(name = "encounter", path = "MedicationRequest.encounter", description = "Return prescriptions with this encounter identifier", type = "reference", providesMembershipIn = { 5137 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5138 public static final String SP_ENCOUNTER = "encounter"; 5139 /** 5140 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5141 * <p> 5142 * Description: <b>Return prescriptions with this encounter identifier</b><br> 5143 * Type: <b>reference</b><br> 5144 * Path: <b>MedicationRequest.encounter</b><br> 5145 * </p> 5146 */ 5147 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5148 SP_ENCOUNTER); 5149 5150 /** 5151 * Constant for fluent queries to be used to add include statements. Specifies 5152 * the path value of "<b>MedicationRequest:encounter</b>". 5153 */ 5154 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5155 "MedicationRequest:encounter").toLocked(); 5156 5157 /** 5158 * Search parameter: <b>priority</b> 5159 * <p> 5160 * Description: <b>Returns prescriptions with different priorities</b><br> 5161 * Type: <b>token</b><br> 5162 * Path: <b>MedicationRequest.priority</b><br> 5163 * </p> 5164 */ 5165 @SearchParamDefinition(name = "priority", path = "MedicationRequest.priority", description = "Returns prescriptions with different priorities", type = "token") 5166 public static final String SP_PRIORITY = "priority"; 5167 /** 5168 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 5169 * <p> 5170 * Description: <b>Returns prescriptions with different priorities</b><br> 5171 * Type: <b>token</b><br> 5172 * Path: <b>MedicationRequest.priority</b><br> 5173 * </p> 5174 */ 5175 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5176 SP_PRIORITY); 5177 5178 /** 5179 * Search parameter: <b>intent</b> 5180 * <p> 5181 * Description: <b>Returns prescriptions with different intents</b><br> 5182 * Type: <b>token</b><br> 5183 * Path: <b>MedicationRequest.intent</b><br> 5184 * </p> 5185 */ 5186 @SearchParamDefinition(name = "intent", path = "MedicationRequest.intent", description = "Returns prescriptions with different intents", type = "token") 5187 public static final String SP_INTENT = "intent"; 5188 /** 5189 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 5190 * <p> 5191 * Description: <b>Returns prescriptions with different intents</b><br> 5192 * Type: <b>token</b><br> 5193 * Path: <b>MedicationRequest.intent</b><br> 5194 * </p> 5195 */ 5196 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5197 SP_INTENT); 5198 5199 /** 5200 * Search parameter: <b>patient</b> 5201 * <p> 5202 * Description: <b>Returns prescriptions for a specific patient</b><br> 5203 * Type: <b>reference</b><br> 5204 * Path: <b>MedicationRequest.subject</b><br> 5205 * </p> 5206 */ 5207 @SearchParamDefinition(name = "patient", path = "MedicationRequest.subject.where(resolve() is Patient)", description = "Returns prescriptions for a specific patient", type = "reference", target = { 5208 Patient.class }) 5209 public static final String SP_PATIENT = "patient"; 5210 /** 5211 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 5212 * <p> 5213 * Description: <b>Returns prescriptions for a specific patient</b><br> 5214 * Type: <b>reference</b><br> 5215 * Path: <b>MedicationRequest.subject</b><br> 5216 * </p> 5217 */ 5218 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5219 SP_PATIENT); 5220 5221 /** 5222 * Constant for fluent queries to be used to add include statements. Specifies 5223 * the path value of "<b>MedicationRequest:patient</b>". 5224 */ 5225 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 5226 "MedicationRequest:patient").toLocked(); 5227 5228 /** 5229 * Search parameter: <b>intended-performer</b> 5230 * <p> 5231 * Description: <b>Returns the intended performer of the administration of the 5232 * medication request</b><br> 5233 * Type: <b>reference</b><br> 5234 * Path: <b>MedicationRequest.performer</b><br> 5235 * </p> 5236 */ 5237 @SearchParamDefinition(name = "intended-performer", path = "MedicationRequest.performer", description = "Returns the intended performer of the administration of the medication request", type = "reference", target = { 5238 CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, 5239 RelatedPerson.class }) 5240 public static final String SP_INTENDED_PERFORMER = "intended-performer"; 5241 /** 5242 * <b>Fluent Client</b> search parameter constant for <b>intended-performer</b> 5243 * <p> 5244 * Description: <b>Returns the intended performer of the administration of the 5245 * medication request</b><br> 5246 * Type: <b>reference</b><br> 5247 * Path: <b>MedicationRequest.performer</b><br> 5248 * </p> 5249 */ 5250 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5251 SP_INTENDED_PERFORMER); 5252 5253 /** 5254 * Constant for fluent queries to be used to add include statements. Specifies 5255 * the path value of "<b>MedicationRequest:intended-performer</b>". 5256 */ 5257 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_PERFORMER = new ca.uhn.fhir.model.api.Include( 5258 "MedicationRequest:intended-performer").toLocked(); 5259 5260 /** 5261 * Search parameter: <b>intended-performertype</b> 5262 * <p> 5263 * Description: <b>Returns requests for a specific type of performer</b><br> 5264 * Type: <b>token</b><br> 5265 * Path: <b>MedicationRequest.performerType</b><br> 5266 * </p> 5267 */ 5268 @SearchParamDefinition(name = "intended-performertype", path = "MedicationRequest.performerType", description = "Returns requests for a specific type of performer", type = "token") 5269 public static final String SP_INTENDED_PERFORMERTYPE = "intended-performertype"; 5270 /** 5271 * <b>Fluent Client</b> search parameter constant for 5272 * <b>intended-performertype</b> 5273 * <p> 5274 * Description: <b>Returns requests for a specific type of performer</b><br> 5275 * Type: <b>token</b><br> 5276 * Path: <b>MedicationRequest.performerType</b><br> 5277 * </p> 5278 */ 5279 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENDED_PERFORMERTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5280 SP_INTENDED_PERFORMERTYPE); 5281 5282 /** 5283 * Search parameter: <b>category</b> 5284 * <p> 5285 * Description: <b>Returns prescriptions with different categories</b><br> 5286 * Type: <b>token</b><br> 5287 * Path: <b>MedicationRequest.category</b><br> 5288 * </p> 5289 */ 5290 @SearchParamDefinition(name = "category", path = "MedicationRequest.category", description = "Returns prescriptions with different categories", type = "token") 5291 public static final String SP_CATEGORY = "category"; 5292 /** 5293 * <b>Fluent Client</b> search parameter constant for <b>category</b> 5294 * <p> 5295 * Description: <b>Returns prescriptions with different categories</b><br> 5296 * Type: <b>token</b><br> 5297 * Path: <b>MedicationRequest.category</b><br> 5298 * </p> 5299 */ 5300 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5301 SP_CATEGORY); 5302 5303 /** 5304 * Search parameter: <b>status</b> 5305 * <p> 5306 * Description: <b>Status of the prescription</b><br> 5307 * Type: <b>token</b><br> 5308 * Path: <b>MedicationRequest.status</b><br> 5309 * </p> 5310 */ 5311 @SearchParamDefinition(name = "status", path = "MedicationRequest.status", description = "Status of the prescription", type = "token") 5312 public static final String SP_STATUS = "status"; 5313 /** 5314 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5315 * <p> 5316 * Description: <b>Status of the prescription</b><br> 5317 * Type: <b>token</b><br> 5318 * Path: <b>MedicationRequest.status</b><br> 5319 * </p> 5320 */ 5321 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5322 SP_STATUS); 5323 5324}