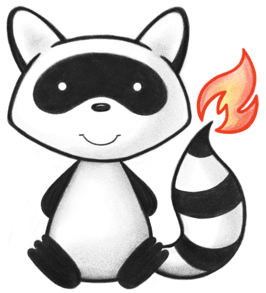
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044 045/** 046 * An ingredient of a manufactured item or pharmaceutical product. 047 */ 048@ResourceDef(name = "MedicinalProductIngredient", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductIngredient") 049public class MedicinalProductIngredient extends DomainResource { 050 051 @Block() 052 public static class MedicinalProductIngredientSpecifiedSubstanceComponent extends BackboneElement 053 implements IBaseBackboneElement { 054 /** 055 * The specified substance. 056 */ 057 @Child(name = "code", type = { 058 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 059 @Description(shortDefinition = "The specified substance", formalDefinition = "The specified substance.") 060 protected CodeableConcept code; 061 062 /** 063 * The group of specified substance, e.g. group 1 to 4. 064 */ 065 @Child(name = "group", type = { 066 CodeableConcept.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "The group of specified substance, e.g. group 1 to 4", formalDefinition = "The group of specified substance, e.g. group 1 to 4.") 068 protected CodeableConcept group; 069 070 /** 071 * Confidentiality level of the specified substance as the ingredient. 072 */ 073 @Child(name = "confidentiality", type = { 074 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Confidentiality level of the specified substance as the ingredient", formalDefinition = "Confidentiality level of the specified substance as the ingredient.") 076 protected CodeableConcept confidentiality; 077 078 /** 079 * Quantity of the substance or specified substance present in the manufactured 080 * item or pharmaceutical product. 081 */ 082 @Child(name = "strength", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 083 @Description(shortDefinition = "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product", formalDefinition = "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.") 084 protected List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> strength; 085 086 private static final long serialVersionUID = -272590200L; 087 088 /** 089 * Constructor 090 */ 091 public MedicinalProductIngredientSpecifiedSubstanceComponent() { 092 super(); 093 } 094 095 /** 096 * Constructor 097 */ 098 public MedicinalProductIngredientSpecifiedSubstanceComponent(CodeableConcept code, CodeableConcept group) { 099 super(); 100 this.code = code; 101 this.group = group; 102 } 103 104 /** 105 * @return {@link #code} (The specified substance.) 106 */ 107 public CodeableConcept getCode() { 108 if (this.code == null) 109 if (Configuration.errorOnAutoCreate()) 110 throw new Error("Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceComponent.code"); 111 else if (Configuration.doAutoCreate()) 112 this.code = new CodeableConcept(); // cc 113 return this.code; 114 } 115 116 public boolean hasCode() { 117 return this.code != null && !this.code.isEmpty(); 118 } 119 120 /** 121 * @param value {@link #code} (The specified substance.) 122 */ 123 public MedicinalProductIngredientSpecifiedSubstanceComponent setCode(CodeableConcept value) { 124 this.code = value; 125 return this; 126 } 127 128 /** 129 * @return {@link #group} (The group of specified substance, e.g. group 1 to 4.) 130 */ 131 public CodeableConcept getGroup() { 132 if (this.group == null) 133 if (Configuration.errorOnAutoCreate()) 134 throw new Error("Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceComponent.group"); 135 else if (Configuration.doAutoCreate()) 136 this.group = new CodeableConcept(); // cc 137 return this.group; 138 } 139 140 public boolean hasGroup() { 141 return this.group != null && !this.group.isEmpty(); 142 } 143 144 /** 145 * @param value {@link #group} (The group of specified substance, e.g. group 1 146 * to 4.) 147 */ 148 public MedicinalProductIngredientSpecifiedSubstanceComponent setGroup(CodeableConcept value) { 149 this.group = value; 150 return this; 151 } 152 153 /** 154 * @return {@link #confidentiality} (Confidentiality level of the specified 155 * substance as the ingredient.) 156 */ 157 public CodeableConcept getConfidentiality() { 158 if (this.confidentiality == null) 159 if (Configuration.errorOnAutoCreate()) 160 throw new Error( 161 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceComponent.confidentiality"); 162 else if (Configuration.doAutoCreate()) 163 this.confidentiality = new CodeableConcept(); // cc 164 return this.confidentiality; 165 } 166 167 public boolean hasConfidentiality() { 168 return this.confidentiality != null && !this.confidentiality.isEmpty(); 169 } 170 171 /** 172 * @param value {@link #confidentiality} (Confidentiality level of the specified 173 * substance as the ingredient.) 174 */ 175 public MedicinalProductIngredientSpecifiedSubstanceComponent setConfidentiality(CodeableConcept value) { 176 this.confidentiality = value; 177 return this; 178 } 179 180 /** 181 * @return {@link #strength} (Quantity of the substance or specified substance 182 * present in the manufactured item or pharmaceutical product.) 183 */ 184 public List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> getStrength() { 185 if (this.strength == null) 186 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 187 return this.strength; 188 } 189 190 /** 191 * @return Returns a reference to <code>this</code> for easy method chaining 192 */ 193 public MedicinalProductIngredientSpecifiedSubstanceComponent setStrength( 194 List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> theStrength) { 195 this.strength = theStrength; 196 return this; 197 } 198 199 public boolean hasStrength() { 200 if (this.strength == null) 201 return false; 202 for (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent item : this.strength) 203 if (!item.isEmpty()) 204 return true; 205 return false; 206 } 207 208 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent addStrength() { // 3 209 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent t = new MedicinalProductIngredientSpecifiedSubstanceStrengthComponent(); 210 if (this.strength == null) 211 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 212 this.strength.add(t); 213 return t; 214 } 215 216 public MedicinalProductIngredientSpecifiedSubstanceComponent addStrength( 217 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent t) { // 3 218 if (t == null) 219 return this; 220 if (this.strength == null) 221 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 222 this.strength.add(t); 223 return this; 224 } 225 226 /** 227 * @return The first repetition of repeating field {@link #strength}, creating 228 * it if it does not already exist 229 */ 230 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent getStrengthFirstRep() { 231 if (getStrength().isEmpty()) { 232 addStrength(); 233 } 234 return getStrength().get(0); 235 } 236 237 protected void listChildren(List<Property> children) { 238 super.listChildren(children); 239 children.add(new Property("code", "CodeableConcept", "The specified substance.", 0, 1, code)); 240 children.add(new Property("group", "CodeableConcept", "The group of specified substance, e.g. group 1 to 4.", 0, 241 1, group)); 242 children.add(new Property("confidentiality", "CodeableConcept", 243 "Confidentiality level of the specified substance as the ingredient.", 0, 1, confidentiality)); 244 children.add(new Property("strength", "", 245 "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.", 246 0, java.lang.Integer.MAX_VALUE, strength)); 247 } 248 249 @Override 250 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 251 switch (_hash) { 252 case 3059181: 253 /* code */ return new Property("code", "CodeableConcept", "The specified substance.", 0, 1, code); 254 case 98629247: 255 /* group */ return new Property("group", "CodeableConcept", 256 "The group of specified substance, e.g. group 1 to 4.", 0, 1, group); 257 case -1923018202: 258 /* confidentiality */ return new Property("confidentiality", "CodeableConcept", 259 "Confidentiality level of the specified substance as the ingredient.", 0, 1, confidentiality); 260 case 1791316033: 261 /* strength */ return new Property("strength", "", 262 "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.", 263 0, java.lang.Integer.MAX_VALUE, strength); 264 default: 265 return super.getNamedProperty(_hash, _name, _checkValid); 266 } 267 268 } 269 270 @Override 271 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 272 switch (hash) { 273 case 3059181: 274 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 275 case 98629247: 276 /* group */ return this.group == null ? new Base[0] : new Base[] { this.group }; // CodeableConcept 277 case -1923018202: 278 /* confidentiality */ return this.confidentiality == null ? new Base[0] : new Base[] { this.confidentiality }; // CodeableConcept 279 case 1791316033: 280 /* strength */ return this.strength == null ? new Base[0] 281 : this.strength.toArray(new Base[this.strength.size()]); // MedicinalProductIngredientSpecifiedSubstanceStrengthComponent 282 default: 283 return super.getProperty(hash, name, checkValid); 284 } 285 286 } 287 288 @Override 289 public Base setProperty(int hash, String name, Base value) throws FHIRException { 290 switch (hash) { 291 case 3059181: // code 292 this.code = castToCodeableConcept(value); // CodeableConcept 293 return value; 294 case 98629247: // group 295 this.group = castToCodeableConcept(value); // CodeableConcept 296 return value; 297 case -1923018202: // confidentiality 298 this.confidentiality = castToCodeableConcept(value); // CodeableConcept 299 return value; 300 case 1791316033: // strength 301 this.getStrength().add((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); // MedicinalProductIngredientSpecifiedSubstanceStrengthComponent 302 return value; 303 default: 304 return super.setProperty(hash, name, value); 305 } 306 307 } 308 309 @Override 310 public Base setProperty(String name, Base value) throws FHIRException { 311 if (name.equals("code")) { 312 this.code = castToCodeableConcept(value); // CodeableConcept 313 } else if (name.equals("group")) { 314 this.group = castToCodeableConcept(value); // CodeableConcept 315 } else if (name.equals("confidentiality")) { 316 this.confidentiality = castToCodeableConcept(value); // CodeableConcept 317 } else if (name.equals("strength")) { 318 this.getStrength().add((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); 319 } else 320 return super.setProperty(name, value); 321 return value; 322 } 323 324 @Override 325 public void removeChild(String name, Base value) throws FHIRException { 326 if (name.equals("code")) { 327 this.code = null; 328 } else if (name.equals("group")) { 329 this.group = null; 330 } else if (name.equals("confidentiality")) { 331 this.confidentiality = null; 332 } else if (name.equals("strength")) { 333 this.getStrength().remove((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); 334 } else 335 super.removeChild(name, value); 336 337 } 338 339 @Override 340 public Base makeProperty(int hash, String name) throws FHIRException { 341 switch (hash) { 342 case 3059181: 343 return getCode(); 344 case 98629247: 345 return getGroup(); 346 case -1923018202: 347 return getConfidentiality(); 348 case 1791316033: 349 return addStrength(); 350 default: 351 return super.makeProperty(hash, name); 352 } 353 354 } 355 356 @Override 357 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 358 switch (hash) { 359 case 3059181: 360 /* code */ return new String[] { "CodeableConcept" }; 361 case 98629247: 362 /* group */ return new String[] { "CodeableConcept" }; 363 case -1923018202: 364 /* confidentiality */ return new String[] { "CodeableConcept" }; 365 case 1791316033: 366 /* strength */ return new String[] {}; 367 default: 368 return super.getTypesForProperty(hash, name); 369 } 370 371 } 372 373 @Override 374 public Base addChild(String name) throws FHIRException { 375 if (name.equals("code")) { 376 this.code = new CodeableConcept(); 377 return this.code; 378 } else if (name.equals("group")) { 379 this.group = new CodeableConcept(); 380 return this.group; 381 } else if (name.equals("confidentiality")) { 382 this.confidentiality = new CodeableConcept(); 383 return this.confidentiality; 384 } else if (name.equals("strength")) { 385 return addStrength(); 386 } else 387 return super.addChild(name); 388 } 389 390 public MedicinalProductIngredientSpecifiedSubstanceComponent copy() { 391 MedicinalProductIngredientSpecifiedSubstanceComponent dst = new MedicinalProductIngredientSpecifiedSubstanceComponent(); 392 copyValues(dst); 393 return dst; 394 } 395 396 public void copyValues(MedicinalProductIngredientSpecifiedSubstanceComponent dst) { 397 super.copyValues(dst); 398 dst.code = code == null ? null : code.copy(); 399 dst.group = group == null ? null : group.copy(); 400 dst.confidentiality = confidentiality == null ? null : confidentiality.copy(); 401 if (strength != null) { 402 dst.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 403 for (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent i : strength) 404 dst.strength.add(i.copy()); 405 } 406 ; 407 } 408 409 @Override 410 public boolean equalsDeep(Base other_) { 411 if (!super.equalsDeep(other_)) 412 return false; 413 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceComponent)) 414 return false; 415 MedicinalProductIngredientSpecifiedSubstanceComponent o = (MedicinalProductIngredientSpecifiedSubstanceComponent) other_; 416 return compareDeep(code, o.code, true) && compareDeep(group, o.group, true) 417 && compareDeep(confidentiality, o.confidentiality, true) && compareDeep(strength, o.strength, true); 418 } 419 420 @Override 421 public boolean equalsShallow(Base other_) { 422 if (!super.equalsShallow(other_)) 423 return false; 424 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceComponent)) 425 return false; 426 MedicinalProductIngredientSpecifiedSubstanceComponent o = (MedicinalProductIngredientSpecifiedSubstanceComponent) other_; 427 return true; 428 } 429 430 public boolean isEmpty() { 431 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, group, confidentiality, strength); 432 } 433 434 public String fhirType() { 435 return "MedicinalProductIngredient.specifiedSubstance"; 436 437 } 438 439 } 440 441 @Block() 442 public static class MedicinalProductIngredientSpecifiedSubstanceStrengthComponent extends BackboneElement 443 implements IBaseBackboneElement { 444 /** 445 * The quantity of substance in the unit of presentation, or in the volume (or 446 * mass) of the single pharmaceutical product or manufactured item. 447 */ 448 @Child(name = "presentation", type = { Ratio.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 449 @Description(shortDefinition = "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item", formalDefinition = "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item.") 450 protected Ratio presentation; 451 452 /** 453 * A lower limit for the quantity of substance in the unit of presentation. For 454 * use when there is a range of strengths, this is the lower limit, with the 455 * presentation attribute becoming the upper limit. 456 */ 457 @Child(name = "presentationLowLimit", type = { 458 Ratio.class }, order = 2, min = 0, max = 1, modifier = true, summary = true) 459 @Description(shortDefinition = "A lower limit for the quantity of substance in the unit of presentation. For use when there is a range of strengths, this is the lower limit, with the presentation attribute becoming the upper limit", formalDefinition = "A lower limit for the quantity of substance in the unit of presentation. For use when there is a range of strengths, this is the lower limit, with the presentation attribute becoming the upper limit.") 460 protected Ratio presentationLowLimit; 461 462 /** 463 * The strength per unitary volume (or mass). 464 */ 465 @Child(name = "concentration", type = { 466 Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 467 @Description(shortDefinition = "The strength per unitary volume (or mass)", formalDefinition = "The strength per unitary volume (or mass).") 468 protected Ratio concentration; 469 470 /** 471 * A lower limit for the strength per unitary volume (or mass), for when there 472 * is a range. The concentration attribute then becomes the upper limit. 473 */ 474 @Child(name = "concentrationLowLimit", type = { 475 Ratio.class }, order = 4, min = 0, max = 1, modifier = true, summary = true) 476 @Description(shortDefinition = "A lower limit for the strength per unitary volume (or mass), for when there is a range. The concentration attribute then becomes the upper limit", formalDefinition = "A lower limit for the strength per unitary volume (or mass), for when there is a range. The concentration attribute then becomes the upper limit.") 477 protected Ratio concentrationLowLimit; 478 479 /** 480 * For when strength is measured at a particular point or distance. 481 */ 482 @Child(name = "measurementPoint", type = { 483 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 484 @Description(shortDefinition = "For when strength is measured at a particular point or distance", formalDefinition = "For when strength is measured at a particular point or distance.") 485 protected StringType measurementPoint; 486 487 /** 488 * The country or countries for which the strength range applies. 489 */ 490 @Child(name = "country", type = { 491 CodeableConcept.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 492 @Description(shortDefinition = "The country or countries for which the strength range applies", formalDefinition = "The country or countries for which the strength range applies.") 493 protected List<CodeableConcept> country; 494 495 /** 496 * Strength expressed in terms of a reference substance. 497 */ 498 @Child(name = "referenceStrength", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 499 @Description(shortDefinition = "Strength expressed in terms of a reference substance", formalDefinition = "Strength expressed in terms of a reference substance.") 500 protected List<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent> referenceStrength; 501 502 private static final long serialVersionUID = 1981438822L; 503 504 /** 505 * Constructor 506 */ 507 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent() { 508 super(); 509 } 510 511 /** 512 * Constructor 513 */ 514 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent(Ratio presentation) { 515 super(); 516 this.presentation = presentation; 517 } 518 519 /** 520 * @return {@link #presentation} (The quantity of substance in the unit of 521 * presentation, or in the volume (or mass) of the single pharmaceutical 522 * product or manufactured item.) 523 */ 524 public Ratio getPresentation() { 525 if (this.presentation == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error( 528 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.presentation"); 529 else if (Configuration.doAutoCreate()) 530 this.presentation = new Ratio(); // cc 531 return this.presentation; 532 } 533 534 public boolean hasPresentation() { 535 return this.presentation != null && !this.presentation.isEmpty(); 536 } 537 538 /** 539 * @param value {@link #presentation} (The quantity of substance in the unit of 540 * presentation, or in the volume (or mass) of the single 541 * pharmaceutical product or manufactured item.) 542 */ 543 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setPresentation(Ratio value) { 544 this.presentation = value; 545 return this; 546 } 547 548 /** 549 * @return {@link #presentationLowLimit} (A lower limit for the quantity of 550 * substance in the unit of presentation. For use when there is a range 551 * of strengths, this is the lower limit, with the presentation 552 * attribute becoming the upper limit.) 553 */ 554 public Ratio getPresentationLowLimit() { 555 if (this.presentationLowLimit == null) 556 if (Configuration.errorOnAutoCreate()) 557 throw new Error( 558 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.presentationLowLimit"); 559 else if (Configuration.doAutoCreate()) 560 this.presentationLowLimit = new Ratio(); // cc 561 return this.presentationLowLimit; 562 } 563 564 public boolean hasPresentationLowLimit() { 565 return this.presentationLowLimit != null && !this.presentationLowLimit.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #presentationLowLimit} (A lower limit for the quantity of 570 * substance in the unit of presentation. For use when there is a 571 * range of strengths, this is the lower limit, with the 572 * presentation attribute becoming the upper limit.) 573 */ 574 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setPresentationLowLimit(Ratio value) { 575 this.presentationLowLimit = value; 576 return this; 577 } 578 579 /** 580 * @return {@link #concentration} (The strength per unitary volume (or mass).) 581 */ 582 public Ratio getConcentration() { 583 if (this.concentration == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error( 586 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.concentration"); 587 else if (Configuration.doAutoCreate()) 588 this.concentration = new Ratio(); // cc 589 return this.concentration; 590 } 591 592 public boolean hasConcentration() { 593 return this.concentration != null && !this.concentration.isEmpty(); 594 } 595 596 /** 597 * @param value {@link #concentration} (The strength per unitary volume (or 598 * mass).) 599 */ 600 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setConcentration(Ratio value) { 601 this.concentration = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #concentrationLowLimit} (A lower limit for the strength per 607 * unitary volume (or mass), for when there is a range. The 608 * concentration attribute then becomes the upper limit.) 609 */ 610 public Ratio getConcentrationLowLimit() { 611 if (this.concentrationLowLimit == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error( 614 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.concentrationLowLimit"); 615 else if (Configuration.doAutoCreate()) 616 this.concentrationLowLimit = new Ratio(); // cc 617 return this.concentrationLowLimit; 618 } 619 620 public boolean hasConcentrationLowLimit() { 621 return this.concentrationLowLimit != null && !this.concentrationLowLimit.isEmpty(); 622 } 623 624 /** 625 * @param value {@link #concentrationLowLimit} (A lower limit for the strength 626 * per unitary volume (or mass), for when there is a range. The 627 * concentration attribute then becomes the upper limit.) 628 */ 629 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setConcentrationLowLimit(Ratio value) { 630 this.concentrationLowLimit = value; 631 return this; 632 } 633 634 /** 635 * @return {@link #measurementPoint} (For when strength is measured at a 636 * particular point or distance.). This is the underlying object with 637 * id, value and extensions. The accessor "getMeasurementPoint" gives 638 * direct access to the value 639 */ 640 public StringType getMeasurementPointElement() { 641 if (this.measurementPoint == null) 642 if (Configuration.errorOnAutoCreate()) 643 throw new Error( 644 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.measurementPoint"); 645 else if (Configuration.doAutoCreate()) 646 this.measurementPoint = new StringType(); // bb 647 return this.measurementPoint; 648 } 649 650 public boolean hasMeasurementPointElement() { 651 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 652 } 653 654 public boolean hasMeasurementPoint() { 655 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #measurementPoint} (For when strength is measured at a 660 * particular point or distance.). This is the underlying object 661 * with id, value and extensions. The accessor 662 * "getMeasurementPoint" gives direct access to the value 663 */ 664 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setMeasurementPointElement(StringType value) { 665 this.measurementPoint = value; 666 return this; 667 } 668 669 /** 670 * @return For when strength is measured at a particular point or distance. 671 */ 672 public String getMeasurementPoint() { 673 return this.measurementPoint == null ? null : this.measurementPoint.getValue(); 674 } 675 676 /** 677 * @param value For when strength is measured at a particular point or distance. 678 */ 679 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setMeasurementPoint(String value) { 680 if (Utilities.noString(value)) 681 this.measurementPoint = null; 682 else { 683 if (this.measurementPoint == null) 684 this.measurementPoint = new StringType(); 685 this.measurementPoint.setValue(value); 686 } 687 return this; 688 } 689 690 /** 691 * @return {@link #country} (The country or countries for which the strength 692 * range applies.) 693 */ 694 public List<CodeableConcept> getCountry() { 695 if (this.country == null) 696 this.country = new ArrayList<CodeableConcept>(); 697 return this.country; 698 } 699 700 /** 701 * @return Returns a reference to <code>this</code> for easy method chaining 702 */ 703 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setCountry(List<CodeableConcept> theCountry) { 704 this.country = theCountry; 705 return this; 706 } 707 708 public boolean hasCountry() { 709 if (this.country == null) 710 return false; 711 for (CodeableConcept item : this.country) 712 if (!item.isEmpty()) 713 return true; 714 return false; 715 } 716 717 public CodeableConcept addCountry() { // 3 718 CodeableConcept t = new CodeableConcept(); 719 if (this.country == null) 720 this.country = new ArrayList<CodeableConcept>(); 721 this.country.add(t); 722 return t; 723 } 724 725 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent addCountry(CodeableConcept t) { // 3 726 if (t == null) 727 return this; 728 if (this.country == null) 729 this.country = new ArrayList<CodeableConcept>(); 730 this.country.add(t); 731 return this; 732 } 733 734 /** 735 * @return The first repetition of repeating field {@link #country}, creating it 736 * if it does not already exist 737 */ 738 public CodeableConcept getCountryFirstRep() { 739 if (getCountry().isEmpty()) { 740 addCountry(); 741 } 742 return getCountry().get(0); 743 } 744 745 /** 746 * @return {@link #referenceStrength} (Strength expressed in terms of a 747 * reference substance.) 748 */ 749 public List<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent> getReferenceStrength() { 750 if (this.referenceStrength == null) 751 this.referenceStrength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent>(); 752 return this.referenceStrength; 753 } 754 755 /** 756 * @return Returns a reference to <code>this</code> for easy method chaining 757 */ 758 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent setReferenceStrength( 759 List<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent> theReferenceStrength) { 760 this.referenceStrength = theReferenceStrength; 761 return this; 762 } 763 764 public boolean hasReferenceStrength() { 765 if (this.referenceStrength == null) 766 return false; 767 for (MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent item : this.referenceStrength) 768 if (!item.isEmpty()) 769 return true; 770 return false; 771 } 772 773 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent addReferenceStrength() { // 3 774 MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent t = new MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent(); 775 if (this.referenceStrength == null) 776 this.referenceStrength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent>(); 777 this.referenceStrength.add(t); 778 return t; 779 } 780 781 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent addReferenceStrength( 782 MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent t) { // 3 783 if (t == null) 784 return this; 785 if (this.referenceStrength == null) 786 this.referenceStrength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent>(); 787 this.referenceStrength.add(t); 788 return this; 789 } 790 791 /** 792 * @return The first repetition of repeating field {@link #referenceStrength}, 793 * creating it if it does not already exist 794 */ 795 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent getReferenceStrengthFirstRep() { 796 if (getReferenceStrength().isEmpty()) { 797 addReferenceStrength(); 798 } 799 return getReferenceStrength().get(0); 800 } 801 802 protected void listChildren(List<Property> children) { 803 super.listChildren(children); 804 children.add(new Property("presentation", "Ratio", 805 "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item.", 806 0, 1, presentation)); 807 children.add(new Property("presentationLowLimit", "Ratio", 808 "A lower limit for the quantity of substance in the unit of presentation. For use when there is a range of strengths, this is the lower limit, with the presentation attribute becoming the upper limit.", 809 0, 1, presentationLowLimit)); 810 children.add( 811 new Property("concentration", "Ratio", "The strength per unitary volume (or mass).", 0, 1, concentration)); 812 children.add(new Property("concentrationLowLimit", "Ratio", 813 "A lower limit for the strength per unitary volume (or mass), for when there is a range. The concentration attribute then becomes the upper limit.", 814 0, 1, concentrationLowLimit)); 815 children.add(new Property("measurementPoint", "string", 816 "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint)); 817 children.add(new Property("country", "CodeableConcept", 818 "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country)); 819 children.add(new Property("referenceStrength", "", "Strength expressed in terms of a reference substance.", 0, 820 java.lang.Integer.MAX_VALUE, referenceStrength)); 821 } 822 823 @Override 824 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 825 switch (_hash) { 826 case 696975130: 827 /* presentation */ return new Property("presentation", "Ratio", 828 "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item.", 829 0, 1, presentation); 830 case -819112447: 831 /* presentationLowLimit */ return new Property("presentationLowLimit", "Ratio", 832 "A lower limit for the quantity of substance in the unit of presentation. For use when there is a range of strengths, this is the lower limit, with the presentation attribute becoming the upper limit.", 833 0, 1, presentationLowLimit); 834 case -410557331: 835 /* concentration */ return new Property("concentration", "Ratio", "The strength per unitary volume (or mass).", 836 0, 1, concentration); 837 case -484132780: 838 /* concentrationLowLimit */ return new Property("concentrationLowLimit", "Ratio", 839 "A lower limit for the strength per unitary volume (or mass), for when there is a range. The concentration attribute then becomes the upper limit.", 840 0, 1, concentrationLowLimit); 841 case 235437876: 842 /* measurementPoint */ return new Property("measurementPoint", "string", 843 "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint); 844 case 957831062: 845 /* country */ return new Property("country", "CodeableConcept", 846 "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country); 847 case 1943566508: 848 /* referenceStrength */ return new Property("referenceStrength", "", 849 "Strength expressed in terms of a reference substance.", 0, java.lang.Integer.MAX_VALUE, referenceStrength); 850 default: 851 return super.getNamedProperty(_hash, _name, _checkValid); 852 } 853 854 } 855 856 @Override 857 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 858 switch (hash) { 859 case 696975130: 860 /* presentation */ return this.presentation == null ? new Base[0] : new Base[] { this.presentation }; // Ratio 861 case -819112447: 862 /* presentationLowLimit */ return this.presentationLowLimit == null ? new Base[0] 863 : new Base[] { this.presentationLowLimit }; // Ratio 864 case -410557331: 865 /* concentration */ return this.concentration == null ? new Base[0] : new Base[] { this.concentration }; // Ratio 866 case -484132780: 867 /* concentrationLowLimit */ return this.concentrationLowLimit == null ? new Base[0] 868 : new Base[] { this.concentrationLowLimit }; // Ratio 869 case 235437876: 870 /* measurementPoint */ return this.measurementPoint == null ? new Base[0] 871 : new Base[] { this.measurementPoint }; // StringType 872 case 957831062: 873 /* country */ return this.country == null ? new Base[0] : this.country.toArray(new Base[this.country.size()]); // CodeableConcept 874 case 1943566508: 875 /* referenceStrength */ return this.referenceStrength == null ? new Base[0] 876 : this.referenceStrength.toArray(new Base[this.referenceStrength.size()]); // MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent 877 default: 878 return super.getProperty(hash, name, checkValid); 879 } 880 881 } 882 883 @Override 884 public Base setProperty(int hash, String name, Base value) throws FHIRException { 885 switch (hash) { 886 case 696975130: // presentation 887 this.presentation = castToRatio(value); // Ratio 888 return value; 889 case -819112447: // presentationLowLimit 890 this.presentationLowLimit = castToRatio(value); // Ratio 891 return value; 892 case -410557331: // concentration 893 this.concentration = castToRatio(value); // Ratio 894 return value; 895 case -484132780: // concentrationLowLimit 896 this.concentrationLowLimit = castToRatio(value); // Ratio 897 return value; 898 case 235437876: // measurementPoint 899 this.measurementPoint = castToString(value); // StringType 900 return value; 901 case 957831062: // country 902 this.getCountry().add(castToCodeableConcept(value)); // CodeableConcept 903 return value; 904 case 1943566508: // referenceStrength 905 this.getReferenceStrength() 906 .add((MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent) value); // MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent 907 return value; 908 default: 909 return super.setProperty(hash, name, value); 910 } 911 912 } 913 914 @Override 915 public Base setProperty(String name, Base value) throws FHIRException { 916 if (name.equals("presentation")) { 917 this.presentation = castToRatio(value); // Ratio 918 } else if (name.equals("presentationLowLimit")) { 919 this.presentationLowLimit = castToRatio(value); // Ratio 920 } else if (name.equals("concentration")) { 921 this.concentration = castToRatio(value); // Ratio 922 } else if (name.equals("concentrationLowLimit")) { 923 this.concentrationLowLimit = castToRatio(value); // Ratio 924 } else if (name.equals("measurementPoint")) { 925 this.measurementPoint = castToString(value); // StringType 926 } else if (name.equals("country")) { 927 this.getCountry().add(castToCodeableConcept(value)); 928 } else if (name.equals("referenceStrength")) { 929 this.getReferenceStrength() 930 .add((MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent) value); 931 } else 932 return super.setProperty(name, value); 933 return value; 934 } 935 936 @Override 937 public void removeChild(String name, Base value) throws FHIRException { 938 if (name.equals("presentation")) { 939 this.presentation = null; 940 } else if (name.equals("presentationLowLimit")) { 941 this.presentationLowLimit = null; 942 } else if (name.equals("concentration")) { 943 this.concentration = null; 944 } else if (name.equals("concentrationLowLimit")) { 945 this.concentrationLowLimit = null; 946 } else if (name.equals("measurementPoint")) { 947 this.measurementPoint = null; 948 } else if (name.equals("country")) { 949 this.getCountry().remove(castToCodeableConcept(value)); 950 } else if (name.equals("referenceStrength")) { 951 this.getReferenceStrength() 952 .remove((MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent) value); 953 } else 954 super.removeChild(name, value); 955 956 } 957 958 @Override 959 public Base makeProperty(int hash, String name) throws FHIRException { 960 switch (hash) { 961 case 696975130: 962 return getPresentation(); 963 case -819112447: 964 return getPresentationLowLimit(); 965 case -410557331: 966 return getConcentration(); 967 case -484132780: 968 return getConcentrationLowLimit(); 969 case 235437876: 970 return getMeasurementPointElement(); 971 case 957831062: 972 return addCountry(); 973 case 1943566508: 974 return addReferenceStrength(); 975 default: 976 return super.makeProperty(hash, name); 977 } 978 979 } 980 981 @Override 982 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 983 switch (hash) { 984 case 696975130: 985 /* presentation */ return new String[] { "Ratio" }; 986 case -819112447: 987 /* presentationLowLimit */ return new String[] { "Ratio" }; 988 case -410557331: 989 /* concentration */ return new String[] { "Ratio" }; 990 case -484132780: 991 /* concentrationLowLimit */ return new String[] { "Ratio" }; 992 case 235437876: 993 /* measurementPoint */ return new String[] { "string" }; 994 case 957831062: 995 /* country */ return new String[] { "CodeableConcept" }; 996 case 1943566508: 997 /* referenceStrength */ return new String[] {}; 998 default: 999 return super.getTypesForProperty(hash, name); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base addChild(String name) throws FHIRException { 1006 if (name.equals("presentation")) { 1007 this.presentation = new Ratio(); 1008 return this.presentation; 1009 } else if (name.equals("presentationLowLimit")) { 1010 this.presentationLowLimit = new Ratio(); 1011 return this.presentationLowLimit; 1012 } else if (name.equals("concentration")) { 1013 this.concentration = new Ratio(); 1014 return this.concentration; 1015 } else if (name.equals("concentrationLowLimit")) { 1016 this.concentrationLowLimit = new Ratio(); 1017 return this.concentrationLowLimit; 1018 } else if (name.equals("measurementPoint")) { 1019 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductIngredient.measurementPoint"); 1020 } else if (name.equals("country")) { 1021 return addCountry(); 1022 } else if (name.equals("referenceStrength")) { 1023 return addReferenceStrength(); 1024 } else 1025 return super.addChild(name); 1026 } 1027 1028 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent copy() { 1029 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent dst = new MedicinalProductIngredientSpecifiedSubstanceStrengthComponent(); 1030 copyValues(dst); 1031 return dst; 1032 } 1033 1034 public void copyValues(MedicinalProductIngredientSpecifiedSubstanceStrengthComponent dst) { 1035 super.copyValues(dst); 1036 dst.presentation = presentation == null ? null : presentation.copy(); 1037 dst.presentationLowLimit = presentationLowLimit == null ? null : presentationLowLimit.copy(); 1038 dst.concentration = concentration == null ? null : concentration.copy(); 1039 dst.concentrationLowLimit = concentrationLowLimit == null ? null : concentrationLowLimit.copy(); 1040 dst.measurementPoint = measurementPoint == null ? null : measurementPoint.copy(); 1041 if (country != null) { 1042 dst.country = new ArrayList<CodeableConcept>(); 1043 for (CodeableConcept i : country) 1044 dst.country.add(i.copy()); 1045 } 1046 ; 1047 if (referenceStrength != null) { 1048 dst.referenceStrength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent>(); 1049 for (MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent i : referenceStrength) 1050 dst.referenceStrength.add(i.copy()); 1051 } 1052 ; 1053 } 1054 1055 @Override 1056 public boolean equalsDeep(Base other_) { 1057 if (!super.equalsDeep(other_)) 1058 return false; 1059 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceStrengthComponent)) 1060 return false; 1061 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent o = (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) other_; 1062 return compareDeep(presentation, o.presentation, true) 1063 && compareDeep(presentationLowLimit, o.presentationLowLimit, true) 1064 && compareDeep(concentration, o.concentration, true) 1065 && compareDeep(concentrationLowLimit, o.concentrationLowLimit, true) 1066 && compareDeep(measurementPoint, o.measurementPoint, true) && compareDeep(country, o.country, true) 1067 && compareDeep(referenceStrength, o.referenceStrength, true); 1068 } 1069 1070 @Override 1071 public boolean equalsShallow(Base other_) { 1072 if (!super.equalsShallow(other_)) 1073 return false; 1074 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceStrengthComponent)) 1075 return false; 1076 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent o = (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) other_; 1077 return compareValues(measurementPoint, o.measurementPoint, true); 1078 } 1079 1080 public boolean isEmpty() { 1081 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(presentation, presentationLowLimit, concentration, 1082 concentrationLowLimit, measurementPoint, country, referenceStrength); 1083 } 1084 1085 public String fhirType() { 1086 return "MedicinalProductIngredient.specifiedSubstance.strength"; 1087 1088 } 1089 1090 } 1091 1092 @Block() 1093 public static class MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent 1094 extends BackboneElement implements IBaseBackboneElement { 1095 /** 1096 * Relevant reference substance. 1097 */ 1098 @Child(name = "substance", type = { 1099 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1100 @Description(shortDefinition = "Relevant reference substance", formalDefinition = "Relevant reference substance.") 1101 protected CodeableConcept substance; 1102 1103 /** 1104 * Strength expressed in terms of a reference substance. 1105 */ 1106 @Child(name = "strength", type = { Ratio.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1107 @Description(shortDefinition = "Strength expressed in terms of a reference substance", formalDefinition = "Strength expressed in terms of a reference substance.") 1108 protected Ratio strength; 1109 1110 /** 1111 * Strength expressed in terms of a reference substance. 1112 */ 1113 @Child(name = "strengthLowLimit", type = { 1114 Ratio.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1115 @Description(shortDefinition = "Strength expressed in terms of a reference substance", formalDefinition = "Strength expressed in terms of a reference substance.") 1116 protected Ratio strengthLowLimit; 1117 1118 /** 1119 * For when strength is measured at a particular point or distance. 1120 */ 1121 @Child(name = "measurementPoint", type = { 1122 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1123 @Description(shortDefinition = "For when strength is measured at a particular point or distance", formalDefinition = "For when strength is measured at a particular point or distance.") 1124 protected StringType measurementPoint; 1125 1126 /** 1127 * The country or countries for which the strength range applies. 1128 */ 1129 @Child(name = "country", type = { 1130 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1131 @Description(shortDefinition = "The country or countries for which the strength range applies", formalDefinition = "The country or countries for which the strength range applies.") 1132 protected List<CodeableConcept> country; 1133 1134 private static final long serialVersionUID = -839485716L; 1135 1136 /** 1137 * Constructor 1138 */ 1139 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent() { 1140 super(); 1141 } 1142 1143 /** 1144 * Constructor 1145 */ 1146 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent(Ratio strength) { 1147 super(); 1148 this.strength = strength; 1149 } 1150 1151 /** 1152 * @return {@link #substance} (Relevant reference substance.) 1153 */ 1154 public CodeableConcept getSubstance() { 1155 if (this.substance == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error( 1158 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent.substance"); 1159 else if (Configuration.doAutoCreate()) 1160 this.substance = new CodeableConcept(); // cc 1161 return this.substance; 1162 } 1163 1164 public boolean hasSubstance() { 1165 return this.substance != null && !this.substance.isEmpty(); 1166 } 1167 1168 /** 1169 * @param value {@link #substance} (Relevant reference substance.) 1170 */ 1171 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setSubstance( 1172 CodeableConcept value) { 1173 this.substance = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #strength} (Strength expressed in terms of a reference 1179 * substance.) 1180 */ 1181 public Ratio getStrength() { 1182 if (this.strength == null) 1183 if (Configuration.errorOnAutoCreate()) 1184 throw new Error( 1185 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent.strength"); 1186 else if (Configuration.doAutoCreate()) 1187 this.strength = new Ratio(); // cc 1188 return this.strength; 1189 } 1190 1191 public boolean hasStrength() { 1192 return this.strength != null && !this.strength.isEmpty(); 1193 } 1194 1195 /** 1196 * @param value {@link #strength} (Strength expressed in terms of a reference 1197 * substance.) 1198 */ 1199 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setStrength(Ratio value) { 1200 this.strength = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #strengthLowLimit} (Strength expressed in terms of a reference 1206 * substance.) 1207 */ 1208 public Ratio getStrengthLowLimit() { 1209 if (this.strengthLowLimit == null) 1210 if (Configuration.errorOnAutoCreate()) 1211 throw new Error( 1212 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent.strengthLowLimit"); 1213 else if (Configuration.doAutoCreate()) 1214 this.strengthLowLimit = new Ratio(); // cc 1215 return this.strengthLowLimit; 1216 } 1217 1218 public boolean hasStrengthLowLimit() { 1219 return this.strengthLowLimit != null && !this.strengthLowLimit.isEmpty(); 1220 } 1221 1222 /** 1223 * @param value {@link #strengthLowLimit} (Strength expressed in terms of a 1224 * reference substance.) 1225 */ 1226 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setStrengthLowLimit( 1227 Ratio value) { 1228 this.strengthLowLimit = value; 1229 return this; 1230 } 1231 1232 /** 1233 * @return {@link #measurementPoint} (For when strength is measured at a 1234 * particular point or distance.). This is the underlying object with 1235 * id, value and extensions. The accessor "getMeasurementPoint" gives 1236 * direct access to the value 1237 */ 1238 public StringType getMeasurementPointElement() { 1239 if (this.measurementPoint == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error( 1242 "Attempt to auto-create MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent.measurementPoint"); 1243 else if (Configuration.doAutoCreate()) 1244 this.measurementPoint = new StringType(); // bb 1245 return this.measurementPoint; 1246 } 1247 1248 public boolean hasMeasurementPointElement() { 1249 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1250 } 1251 1252 public boolean hasMeasurementPoint() { 1253 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #measurementPoint} (For when strength is measured at a 1258 * particular point or distance.). This is the underlying object 1259 * with id, value and extensions. The accessor 1260 * "getMeasurementPoint" gives direct access to the value 1261 */ 1262 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setMeasurementPointElement( 1263 StringType value) { 1264 this.measurementPoint = value; 1265 return this; 1266 } 1267 1268 /** 1269 * @return For when strength is measured at a particular point or distance. 1270 */ 1271 public String getMeasurementPoint() { 1272 return this.measurementPoint == null ? null : this.measurementPoint.getValue(); 1273 } 1274 1275 /** 1276 * @param value For when strength is measured at a particular point or distance. 1277 */ 1278 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setMeasurementPoint( 1279 String value) { 1280 if (Utilities.noString(value)) 1281 this.measurementPoint = null; 1282 else { 1283 if (this.measurementPoint == null) 1284 this.measurementPoint = new StringType(); 1285 this.measurementPoint.setValue(value); 1286 } 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #country} (The country or countries for which the strength 1292 * range applies.) 1293 */ 1294 public List<CodeableConcept> getCountry() { 1295 if (this.country == null) 1296 this.country = new ArrayList<CodeableConcept>(); 1297 return this.country; 1298 } 1299 1300 /** 1301 * @return Returns a reference to <code>this</code> for easy method chaining 1302 */ 1303 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent setCountry( 1304 List<CodeableConcept> theCountry) { 1305 this.country = theCountry; 1306 return this; 1307 } 1308 1309 public boolean hasCountry() { 1310 if (this.country == null) 1311 return false; 1312 for (CodeableConcept item : this.country) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public CodeableConcept addCountry() { // 3 1319 CodeableConcept t = new CodeableConcept(); 1320 if (this.country == null) 1321 this.country = new ArrayList<CodeableConcept>(); 1322 this.country.add(t); 1323 return t; 1324 } 1325 1326 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent addCountry( 1327 CodeableConcept t) { // 3 1328 if (t == null) 1329 return this; 1330 if (this.country == null) 1331 this.country = new ArrayList<CodeableConcept>(); 1332 this.country.add(t); 1333 return this; 1334 } 1335 1336 /** 1337 * @return The first repetition of repeating field {@link #country}, creating it 1338 * if it does not already exist 1339 */ 1340 public CodeableConcept getCountryFirstRep() { 1341 if (getCountry().isEmpty()) { 1342 addCountry(); 1343 } 1344 return getCountry().get(0); 1345 } 1346 1347 protected void listChildren(List<Property> children) { 1348 super.listChildren(children); 1349 children.add(new Property("substance", "CodeableConcept", "Relevant reference substance.", 0, 1, substance)); 1350 children.add( 1351 new Property("strength", "Ratio", "Strength expressed in terms of a reference substance.", 0, 1, strength)); 1352 children.add(new Property("strengthLowLimit", "Ratio", "Strength expressed in terms of a reference substance.", 0, 1353 1, strengthLowLimit)); 1354 children.add(new Property("measurementPoint", "string", 1355 "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint)); 1356 children.add(new Property("country", "CodeableConcept", 1357 "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country)); 1358 } 1359 1360 @Override 1361 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1362 switch (_hash) { 1363 case 530040176: 1364 /* substance */ return new Property("substance", "CodeableConcept", "Relevant reference substance.", 0, 1, 1365 substance); 1366 case 1791316033: 1367 /* strength */ return new Property("strength", "Ratio", "Strength expressed in terms of a reference substance.", 1368 0, 1, strength); 1369 case 1945341992: 1370 /* strengthLowLimit */ return new Property("strengthLowLimit", "Ratio", 1371 "Strength expressed in terms of a reference substance.", 0, 1, strengthLowLimit); 1372 case 235437876: 1373 /* measurementPoint */ return new Property("measurementPoint", "string", 1374 "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint); 1375 case 957831062: 1376 /* country */ return new Property("country", "CodeableConcept", 1377 "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country); 1378 default: 1379 return super.getNamedProperty(_hash, _name, _checkValid); 1380 } 1381 1382 } 1383 1384 @Override 1385 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1386 switch (hash) { 1387 case 530040176: 1388 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // CodeableConcept 1389 case 1791316033: 1390 /* strength */ return this.strength == null ? new Base[0] : new Base[] { this.strength }; // Ratio 1391 case 1945341992: 1392 /* strengthLowLimit */ return this.strengthLowLimit == null ? new Base[0] 1393 : new Base[] { this.strengthLowLimit }; // Ratio 1394 case 235437876: 1395 /* measurementPoint */ return this.measurementPoint == null ? new Base[0] 1396 : new Base[] { this.measurementPoint }; // StringType 1397 case 957831062: 1398 /* country */ return this.country == null ? new Base[0] : this.country.toArray(new Base[this.country.size()]); // CodeableConcept 1399 default: 1400 return super.getProperty(hash, name, checkValid); 1401 } 1402 1403 } 1404 1405 @Override 1406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1407 switch (hash) { 1408 case 530040176: // substance 1409 this.substance = castToCodeableConcept(value); // CodeableConcept 1410 return value; 1411 case 1791316033: // strength 1412 this.strength = castToRatio(value); // Ratio 1413 return value; 1414 case 1945341992: // strengthLowLimit 1415 this.strengthLowLimit = castToRatio(value); // Ratio 1416 return value; 1417 case 235437876: // measurementPoint 1418 this.measurementPoint = castToString(value); // StringType 1419 return value; 1420 case 957831062: // country 1421 this.getCountry().add(castToCodeableConcept(value)); // CodeableConcept 1422 return value; 1423 default: 1424 return super.setProperty(hash, name, value); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base setProperty(String name, Base value) throws FHIRException { 1431 if (name.equals("substance")) { 1432 this.substance = castToCodeableConcept(value); // CodeableConcept 1433 } else if (name.equals("strength")) { 1434 this.strength = castToRatio(value); // Ratio 1435 } else if (name.equals("strengthLowLimit")) { 1436 this.strengthLowLimit = castToRatio(value); // Ratio 1437 } else if (name.equals("measurementPoint")) { 1438 this.measurementPoint = castToString(value); // StringType 1439 } else if (name.equals("country")) { 1440 this.getCountry().add(castToCodeableConcept(value)); 1441 } else 1442 return super.setProperty(name, value); 1443 return value; 1444 } 1445 1446 @Override 1447 public void removeChild(String name, Base value) throws FHIRException { 1448 if (name.equals("substance")) { 1449 this.substance = null; 1450 } else if (name.equals("strength")) { 1451 this.strength = null; 1452 } else if (name.equals("strengthLowLimit")) { 1453 this.strengthLowLimit = null; 1454 } else if (name.equals("measurementPoint")) { 1455 this.measurementPoint = null; 1456 } else if (name.equals("country")) { 1457 this.getCountry().remove(castToCodeableConcept(value)); 1458 } else 1459 super.removeChild(name, value); 1460 1461 } 1462 1463 @Override 1464 public Base makeProperty(int hash, String name) throws FHIRException { 1465 switch (hash) { 1466 case 530040176: 1467 return getSubstance(); 1468 case 1791316033: 1469 return getStrength(); 1470 case 1945341992: 1471 return getStrengthLowLimit(); 1472 case 235437876: 1473 return getMeasurementPointElement(); 1474 case 957831062: 1475 return addCountry(); 1476 default: 1477 return super.makeProperty(hash, name); 1478 } 1479 1480 } 1481 1482 @Override 1483 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1484 switch (hash) { 1485 case 530040176: 1486 /* substance */ return new String[] { "CodeableConcept" }; 1487 case 1791316033: 1488 /* strength */ return new String[] { "Ratio" }; 1489 case 1945341992: 1490 /* strengthLowLimit */ return new String[] { "Ratio" }; 1491 case 235437876: 1492 /* measurementPoint */ return new String[] { "string" }; 1493 case 957831062: 1494 /* country */ return new String[] { "CodeableConcept" }; 1495 default: 1496 return super.getTypesForProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public Base addChild(String name) throws FHIRException { 1503 if (name.equals("substance")) { 1504 this.substance = new CodeableConcept(); 1505 return this.substance; 1506 } else if (name.equals("strength")) { 1507 this.strength = new Ratio(); 1508 return this.strength; 1509 } else if (name.equals("strengthLowLimit")) { 1510 this.strengthLowLimit = new Ratio(); 1511 return this.strengthLowLimit; 1512 } else if (name.equals("measurementPoint")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductIngredient.measurementPoint"); 1514 } else if (name.equals("country")) { 1515 return addCountry(); 1516 } else 1517 return super.addChild(name); 1518 } 1519 1520 public MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent copy() { 1521 MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent dst = new MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent(); 1522 copyValues(dst); 1523 return dst; 1524 } 1525 1526 public void copyValues(MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent dst) { 1527 super.copyValues(dst); 1528 dst.substance = substance == null ? null : substance.copy(); 1529 dst.strength = strength == null ? null : strength.copy(); 1530 dst.strengthLowLimit = strengthLowLimit == null ? null : strengthLowLimit.copy(); 1531 dst.measurementPoint = measurementPoint == null ? null : measurementPoint.copy(); 1532 if (country != null) { 1533 dst.country = new ArrayList<CodeableConcept>(); 1534 for (CodeableConcept i : country) 1535 dst.country.add(i.copy()); 1536 } 1537 ; 1538 } 1539 1540 @Override 1541 public boolean equalsDeep(Base other_) { 1542 if (!super.equalsDeep(other_)) 1543 return false; 1544 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent)) 1545 return false; 1546 MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent o = (MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent) other_; 1547 return compareDeep(substance, o.substance, true) && compareDeep(strength, o.strength, true) 1548 && compareDeep(strengthLowLimit, o.strengthLowLimit, true) 1549 && compareDeep(measurementPoint, o.measurementPoint, true) && compareDeep(country, o.country, true); 1550 } 1551 1552 @Override 1553 public boolean equalsShallow(Base other_) { 1554 if (!super.equalsShallow(other_)) 1555 return false; 1556 if (!(other_ instanceof MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent)) 1557 return false; 1558 MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent o = (MedicinalProductIngredientSpecifiedSubstanceStrengthReferenceStrengthComponent) other_; 1559 return compareValues(measurementPoint, o.measurementPoint, true); 1560 } 1561 1562 public boolean isEmpty() { 1563 return super.isEmpty() 1564 && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, strength, strengthLowLimit, measurementPoint, country); 1565 } 1566 1567 public String fhirType() { 1568 return "MedicinalProductIngredient.specifiedSubstance.strength.referenceStrength"; 1569 1570 } 1571 1572 } 1573 1574 @Block() 1575 public static class MedicinalProductIngredientSubstanceComponent extends BackboneElement 1576 implements IBaseBackboneElement { 1577 /** 1578 * The ingredient substance. 1579 */ 1580 @Child(name = "code", type = { 1581 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1582 @Description(shortDefinition = "The ingredient substance", formalDefinition = "The ingredient substance.") 1583 protected CodeableConcept code; 1584 1585 /** 1586 * Quantity of the substance or specified substance present in the manufactured 1587 * item or pharmaceutical product. 1588 */ 1589 @Child(name = "strength", type = { 1590 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1591 @Description(shortDefinition = "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product", formalDefinition = "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.") 1592 protected List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> strength; 1593 1594 private static final long serialVersionUID = 1325868149L; 1595 1596 /** 1597 * Constructor 1598 */ 1599 public MedicinalProductIngredientSubstanceComponent() { 1600 super(); 1601 } 1602 1603 /** 1604 * Constructor 1605 */ 1606 public MedicinalProductIngredientSubstanceComponent(CodeableConcept code) { 1607 super(); 1608 this.code = code; 1609 } 1610 1611 /** 1612 * @return {@link #code} (The ingredient substance.) 1613 */ 1614 public CodeableConcept getCode() { 1615 if (this.code == null) 1616 if (Configuration.errorOnAutoCreate()) 1617 throw new Error("Attempt to auto-create MedicinalProductIngredientSubstanceComponent.code"); 1618 else if (Configuration.doAutoCreate()) 1619 this.code = new CodeableConcept(); // cc 1620 return this.code; 1621 } 1622 1623 public boolean hasCode() { 1624 return this.code != null && !this.code.isEmpty(); 1625 } 1626 1627 /** 1628 * @param value {@link #code} (The ingredient substance.) 1629 */ 1630 public MedicinalProductIngredientSubstanceComponent setCode(CodeableConcept value) { 1631 this.code = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return {@link #strength} (Quantity of the substance or specified substance 1637 * present in the manufactured item or pharmaceutical product.) 1638 */ 1639 public List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> getStrength() { 1640 if (this.strength == null) 1641 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 1642 return this.strength; 1643 } 1644 1645 /** 1646 * @return Returns a reference to <code>this</code> for easy method chaining 1647 */ 1648 public MedicinalProductIngredientSubstanceComponent setStrength( 1649 List<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent> theStrength) { 1650 this.strength = theStrength; 1651 return this; 1652 } 1653 1654 public boolean hasStrength() { 1655 if (this.strength == null) 1656 return false; 1657 for (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent item : this.strength) 1658 if (!item.isEmpty()) 1659 return true; 1660 return false; 1661 } 1662 1663 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent addStrength() { // 3 1664 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent t = new MedicinalProductIngredientSpecifiedSubstanceStrengthComponent(); 1665 if (this.strength == null) 1666 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 1667 this.strength.add(t); 1668 return t; 1669 } 1670 1671 public MedicinalProductIngredientSubstanceComponent addStrength( 1672 MedicinalProductIngredientSpecifiedSubstanceStrengthComponent t) { // 3 1673 if (t == null) 1674 return this; 1675 if (this.strength == null) 1676 this.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 1677 this.strength.add(t); 1678 return this; 1679 } 1680 1681 /** 1682 * @return The first repetition of repeating field {@link #strength}, creating 1683 * it if it does not already exist 1684 */ 1685 public MedicinalProductIngredientSpecifiedSubstanceStrengthComponent getStrengthFirstRep() { 1686 if (getStrength().isEmpty()) { 1687 addStrength(); 1688 } 1689 return getStrength().get(0); 1690 } 1691 1692 protected void listChildren(List<Property> children) { 1693 super.listChildren(children); 1694 children.add(new Property("code", "CodeableConcept", "The ingredient substance.", 0, 1, code)); 1695 children.add(new Property("strength", "@MedicinalProductIngredient.specifiedSubstance.strength", 1696 "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.", 1697 0, java.lang.Integer.MAX_VALUE, strength)); 1698 } 1699 1700 @Override 1701 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1702 switch (_hash) { 1703 case 3059181: 1704 /* code */ return new Property("code", "CodeableConcept", "The ingredient substance.", 0, 1, code); 1705 case 1791316033: 1706 /* strength */ return new Property("strength", "@MedicinalProductIngredient.specifiedSubstance.strength", 1707 "Quantity of the substance or specified substance present in the manufactured item or pharmaceutical product.", 1708 0, java.lang.Integer.MAX_VALUE, strength); 1709 default: 1710 return super.getNamedProperty(_hash, _name, _checkValid); 1711 } 1712 1713 } 1714 1715 @Override 1716 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1717 switch (hash) { 1718 case 3059181: 1719 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1720 case 1791316033: 1721 /* strength */ return this.strength == null ? new Base[0] 1722 : this.strength.toArray(new Base[this.strength.size()]); // MedicinalProductIngredientSpecifiedSubstanceStrengthComponent 1723 default: 1724 return super.getProperty(hash, name, checkValid); 1725 } 1726 1727 } 1728 1729 @Override 1730 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1731 switch (hash) { 1732 case 3059181: // code 1733 this.code = castToCodeableConcept(value); // CodeableConcept 1734 return value; 1735 case 1791316033: // strength 1736 this.getStrength().add((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); // MedicinalProductIngredientSpecifiedSubstanceStrengthComponent 1737 return value; 1738 default: 1739 return super.setProperty(hash, name, value); 1740 } 1741 1742 } 1743 1744 @Override 1745 public Base setProperty(String name, Base value) throws FHIRException { 1746 if (name.equals("code")) { 1747 this.code = castToCodeableConcept(value); // CodeableConcept 1748 } else if (name.equals("strength")) { 1749 this.getStrength().add((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); 1750 } else 1751 return super.setProperty(name, value); 1752 return value; 1753 } 1754 1755 @Override 1756 public void removeChild(String name, Base value) throws FHIRException { 1757 if (name.equals("code")) { 1758 this.code = null; 1759 } else if (name.equals("strength")) { 1760 this.getStrength().remove((MedicinalProductIngredientSpecifiedSubstanceStrengthComponent) value); 1761 } else 1762 super.removeChild(name, value); 1763 1764 } 1765 1766 @Override 1767 public Base makeProperty(int hash, String name) throws FHIRException { 1768 switch (hash) { 1769 case 3059181: 1770 return getCode(); 1771 case 1791316033: 1772 return addStrength(); 1773 default: 1774 return super.makeProperty(hash, name); 1775 } 1776 1777 } 1778 1779 @Override 1780 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1781 switch (hash) { 1782 case 3059181: 1783 /* code */ return new String[] { "CodeableConcept" }; 1784 case 1791316033: 1785 /* strength */ return new String[] { "@MedicinalProductIngredient.specifiedSubstance.strength" }; 1786 default: 1787 return super.getTypesForProperty(hash, name); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base addChild(String name) throws FHIRException { 1794 if (name.equals("code")) { 1795 this.code = new CodeableConcept(); 1796 return this.code; 1797 } else if (name.equals("strength")) { 1798 return addStrength(); 1799 } else 1800 return super.addChild(name); 1801 } 1802 1803 public MedicinalProductIngredientSubstanceComponent copy() { 1804 MedicinalProductIngredientSubstanceComponent dst = new MedicinalProductIngredientSubstanceComponent(); 1805 copyValues(dst); 1806 return dst; 1807 } 1808 1809 public void copyValues(MedicinalProductIngredientSubstanceComponent dst) { 1810 super.copyValues(dst); 1811 dst.code = code == null ? null : code.copy(); 1812 if (strength != null) { 1813 dst.strength = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceStrengthComponent>(); 1814 for (MedicinalProductIngredientSpecifiedSubstanceStrengthComponent i : strength) 1815 dst.strength.add(i.copy()); 1816 } 1817 ; 1818 } 1819 1820 @Override 1821 public boolean equalsDeep(Base other_) { 1822 if (!super.equalsDeep(other_)) 1823 return false; 1824 if (!(other_ instanceof MedicinalProductIngredientSubstanceComponent)) 1825 return false; 1826 MedicinalProductIngredientSubstanceComponent o = (MedicinalProductIngredientSubstanceComponent) other_; 1827 return compareDeep(code, o.code, true) && compareDeep(strength, o.strength, true); 1828 } 1829 1830 @Override 1831 public boolean equalsShallow(Base other_) { 1832 if (!super.equalsShallow(other_)) 1833 return false; 1834 if (!(other_ instanceof MedicinalProductIngredientSubstanceComponent)) 1835 return false; 1836 MedicinalProductIngredientSubstanceComponent o = (MedicinalProductIngredientSubstanceComponent) other_; 1837 return true; 1838 } 1839 1840 public boolean isEmpty() { 1841 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, strength); 1842 } 1843 1844 public String fhirType() { 1845 return "MedicinalProductIngredient.substance"; 1846 1847 } 1848 1849 } 1850 1851 /** 1852 * The identifier(s) of this Ingredient that are assigned by business processes 1853 * and/or used to refer to it when a direct URL reference to the resource itself 1854 * is not appropriate. 1855 */ 1856 @Child(name = "identifier", type = { 1857 Identifier.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 1858 @Description(shortDefinition = "Identifier for the ingredient", formalDefinition = "The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.") 1859 protected Identifier identifier; 1860 1861 /** 1862 * Ingredient role e.g. Active ingredient, excipient. 1863 */ 1864 @Child(name = "role", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1865 @Description(shortDefinition = "Ingredient role e.g. Active ingredient, excipient", formalDefinition = "Ingredient role e.g. Active ingredient, excipient.") 1866 protected CodeableConcept role; 1867 1868 /** 1869 * If the ingredient is a known or suspected allergen. 1870 */ 1871 @Child(name = "allergenicIndicator", type = { 1872 BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1873 @Description(shortDefinition = "If the ingredient is a known or suspected allergen", formalDefinition = "If the ingredient is a known or suspected allergen.") 1874 protected BooleanType allergenicIndicator; 1875 1876 /** 1877 * Manufacturer of this Ingredient. 1878 */ 1879 @Child(name = "manufacturer", type = { 1880 Organization.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1881 @Description(shortDefinition = "Manufacturer of this Ingredient", formalDefinition = "Manufacturer of this Ingredient.") 1882 protected List<Reference> manufacturer; 1883 /** 1884 * The actual objects that are the target of the reference (Manufacturer of this 1885 * Ingredient.) 1886 */ 1887 protected List<Organization> manufacturerTarget; 1888 1889 /** 1890 * A specified substance that comprises this ingredient. 1891 */ 1892 @Child(name = "specifiedSubstance", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1893 @Description(shortDefinition = "A specified substance that comprises this ingredient", formalDefinition = "A specified substance that comprises this ingredient.") 1894 protected List<MedicinalProductIngredientSpecifiedSubstanceComponent> specifiedSubstance; 1895 1896 /** 1897 * The ingredient substance. 1898 */ 1899 @Child(name = "substance", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 1900 @Description(shortDefinition = "The ingredient substance", formalDefinition = "The ingredient substance.") 1901 protected MedicinalProductIngredientSubstanceComponent substance; 1902 1903 private static final long serialVersionUID = -1454686641L; 1904 1905 /** 1906 * Constructor 1907 */ 1908 public MedicinalProductIngredient() { 1909 super(); 1910 } 1911 1912 /** 1913 * Constructor 1914 */ 1915 public MedicinalProductIngredient(CodeableConcept role) { 1916 super(); 1917 this.role = role; 1918 } 1919 1920 /** 1921 * @return {@link #identifier} (The identifier(s) of this Ingredient that are 1922 * assigned by business processes and/or used to refer to it when a 1923 * direct URL reference to the resource itself is not appropriate.) 1924 */ 1925 public Identifier getIdentifier() { 1926 if (this.identifier == null) 1927 if (Configuration.errorOnAutoCreate()) 1928 throw new Error("Attempt to auto-create MedicinalProductIngredient.identifier"); 1929 else if (Configuration.doAutoCreate()) 1930 this.identifier = new Identifier(); // cc 1931 return this.identifier; 1932 } 1933 1934 public boolean hasIdentifier() { 1935 return this.identifier != null && !this.identifier.isEmpty(); 1936 } 1937 1938 /** 1939 * @param value {@link #identifier} (The identifier(s) of this Ingredient that 1940 * are assigned by business processes and/or used to refer to it 1941 * when a direct URL reference to the resource itself is not 1942 * appropriate.) 1943 */ 1944 public MedicinalProductIngredient setIdentifier(Identifier value) { 1945 this.identifier = value; 1946 return this; 1947 } 1948 1949 /** 1950 * @return {@link #role} (Ingredient role e.g. Active ingredient, excipient.) 1951 */ 1952 public CodeableConcept getRole() { 1953 if (this.role == null) 1954 if (Configuration.errorOnAutoCreate()) 1955 throw new Error("Attempt to auto-create MedicinalProductIngredient.role"); 1956 else if (Configuration.doAutoCreate()) 1957 this.role = new CodeableConcept(); // cc 1958 return this.role; 1959 } 1960 1961 public boolean hasRole() { 1962 return this.role != null && !this.role.isEmpty(); 1963 } 1964 1965 /** 1966 * @param value {@link #role} (Ingredient role e.g. Active ingredient, 1967 * excipient.) 1968 */ 1969 public MedicinalProductIngredient setRole(CodeableConcept value) { 1970 this.role = value; 1971 return this; 1972 } 1973 1974 /** 1975 * @return {@link #allergenicIndicator} (If the ingredient is a known or 1976 * suspected allergen.). This is the underlying object with id, value 1977 * and extensions. The accessor "getAllergenicIndicator" gives direct 1978 * access to the value 1979 */ 1980 public BooleanType getAllergenicIndicatorElement() { 1981 if (this.allergenicIndicator == null) 1982 if (Configuration.errorOnAutoCreate()) 1983 throw new Error("Attempt to auto-create MedicinalProductIngredient.allergenicIndicator"); 1984 else if (Configuration.doAutoCreate()) 1985 this.allergenicIndicator = new BooleanType(); // bb 1986 return this.allergenicIndicator; 1987 } 1988 1989 public boolean hasAllergenicIndicatorElement() { 1990 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 1991 } 1992 1993 public boolean hasAllergenicIndicator() { 1994 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 1995 } 1996 1997 /** 1998 * @param value {@link #allergenicIndicator} (If the ingredient is a known or 1999 * suspected allergen.). This is the underlying object with id, 2000 * value and extensions. The accessor "getAllergenicIndicator" 2001 * gives direct access to the value 2002 */ 2003 public MedicinalProductIngredient setAllergenicIndicatorElement(BooleanType value) { 2004 this.allergenicIndicator = value; 2005 return this; 2006 } 2007 2008 /** 2009 * @return If the ingredient is a known or suspected allergen. 2010 */ 2011 public boolean getAllergenicIndicator() { 2012 return this.allergenicIndicator == null || this.allergenicIndicator.isEmpty() ? false 2013 : this.allergenicIndicator.getValue(); 2014 } 2015 2016 /** 2017 * @param value If the ingredient is a known or suspected allergen. 2018 */ 2019 public MedicinalProductIngredient setAllergenicIndicator(boolean value) { 2020 if (this.allergenicIndicator == null) 2021 this.allergenicIndicator = new BooleanType(); 2022 this.allergenicIndicator.setValue(value); 2023 return this; 2024 } 2025 2026 /** 2027 * @return {@link #manufacturer} (Manufacturer of this Ingredient.) 2028 */ 2029 public List<Reference> getManufacturer() { 2030 if (this.manufacturer == null) 2031 this.manufacturer = new ArrayList<Reference>(); 2032 return this.manufacturer; 2033 } 2034 2035 /** 2036 * @return Returns a reference to <code>this</code> for easy method chaining 2037 */ 2038 public MedicinalProductIngredient setManufacturer(List<Reference> theManufacturer) { 2039 this.manufacturer = theManufacturer; 2040 return this; 2041 } 2042 2043 public boolean hasManufacturer() { 2044 if (this.manufacturer == null) 2045 return false; 2046 for (Reference item : this.manufacturer) 2047 if (!item.isEmpty()) 2048 return true; 2049 return false; 2050 } 2051 2052 public Reference addManufacturer() { // 3 2053 Reference t = new Reference(); 2054 if (this.manufacturer == null) 2055 this.manufacturer = new ArrayList<Reference>(); 2056 this.manufacturer.add(t); 2057 return t; 2058 } 2059 2060 public MedicinalProductIngredient addManufacturer(Reference t) { // 3 2061 if (t == null) 2062 return this; 2063 if (this.manufacturer == null) 2064 this.manufacturer = new ArrayList<Reference>(); 2065 this.manufacturer.add(t); 2066 return this; 2067 } 2068 2069 /** 2070 * @return The first repetition of repeating field {@link #manufacturer}, 2071 * creating it if it does not already exist 2072 */ 2073 public Reference getManufacturerFirstRep() { 2074 if (getManufacturer().isEmpty()) { 2075 addManufacturer(); 2076 } 2077 return getManufacturer().get(0); 2078 } 2079 2080 /** 2081 * @deprecated Use Reference#setResource(IBaseResource) instead 2082 */ 2083 @Deprecated 2084 public List<Organization> getManufacturerTarget() { 2085 if (this.manufacturerTarget == null) 2086 this.manufacturerTarget = new ArrayList<Organization>(); 2087 return this.manufacturerTarget; 2088 } 2089 2090 /** 2091 * @deprecated Use Reference#setResource(IBaseResource) instead 2092 */ 2093 @Deprecated 2094 public Organization addManufacturerTarget() { 2095 Organization r = new Organization(); 2096 if (this.manufacturerTarget == null) 2097 this.manufacturerTarget = new ArrayList<Organization>(); 2098 this.manufacturerTarget.add(r); 2099 return r; 2100 } 2101 2102 /** 2103 * @return {@link #specifiedSubstance} (A specified substance that comprises 2104 * this ingredient.) 2105 */ 2106 public List<MedicinalProductIngredientSpecifiedSubstanceComponent> getSpecifiedSubstance() { 2107 if (this.specifiedSubstance == null) 2108 this.specifiedSubstance = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceComponent>(); 2109 return this.specifiedSubstance; 2110 } 2111 2112 /** 2113 * @return Returns a reference to <code>this</code> for easy method chaining 2114 */ 2115 public MedicinalProductIngredient setSpecifiedSubstance( 2116 List<MedicinalProductIngredientSpecifiedSubstanceComponent> theSpecifiedSubstance) { 2117 this.specifiedSubstance = theSpecifiedSubstance; 2118 return this; 2119 } 2120 2121 public boolean hasSpecifiedSubstance() { 2122 if (this.specifiedSubstance == null) 2123 return false; 2124 for (MedicinalProductIngredientSpecifiedSubstanceComponent item : this.specifiedSubstance) 2125 if (!item.isEmpty()) 2126 return true; 2127 return false; 2128 } 2129 2130 public MedicinalProductIngredientSpecifiedSubstanceComponent addSpecifiedSubstance() { // 3 2131 MedicinalProductIngredientSpecifiedSubstanceComponent t = new MedicinalProductIngredientSpecifiedSubstanceComponent(); 2132 if (this.specifiedSubstance == null) 2133 this.specifiedSubstance = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceComponent>(); 2134 this.specifiedSubstance.add(t); 2135 return t; 2136 } 2137 2138 public MedicinalProductIngredient addSpecifiedSubstance(MedicinalProductIngredientSpecifiedSubstanceComponent t) { // 3 2139 if (t == null) 2140 return this; 2141 if (this.specifiedSubstance == null) 2142 this.specifiedSubstance = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceComponent>(); 2143 this.specifiedSubstance.add(t); 2144 return this; 2145 } 2146 2147 /** 2148 * @return The first repetition of repeating field {@link #specifiedSubstance}, 2149 * creating it if it does not already exist 2150 */ 2151 public MedicinalProductIngredientSpecifiedSubstanceComponent getSpecifiedSubstanceFirstRep() { 2152 if (getSpecifiedSubstance().isEmpty()) { 2153 addSpecifiedSubstance(); 2154 } 2155 return getSpecifiedSubstance().get(0); 2156 } 2157 2158 /** 2159 * @return {@link #substance} (The ingredient substance.) 2160 */ 2161 public MedicinalProductIngredientSubstanceComponent getSubstance() { 2162 if (this.substance == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create MedicinalProductIngredient.substance"); 2165 else if (Configuration.doAutoCreate()) 2166 this.substance = new MedicinalProductIngredientSubstanceComponent(); // cc 2167 return this.substance; 2168 } 2169 2170 public boolean hasSubstance() { 2171 return this.substance != null && !this.substance.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #substance} (The ingredient substance.) 2176 */ 2177 public MedicinalProductIngredient setSubstance(MedicinalProductIngredientSubstanceComponent value) { 2178 this.substance = value; 2179 return this; 2180 } 2181 2182 protected void listChildren(List<Property> children) { 2183 super.listChildren(children); 2184 children.add(new Property("identifier", "Identifier", 2185 "The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 2186 0, 1, identifier)); 2187 children 2188 .add(new Property("role", "CodeableConcept", "Ingredient role e.g. Active ingredient, excipient.", 0, 1, role)); 2189 children.add(new Property("allergenicIndicator", "boolean", "If the ingredient is a known or suspected allergen.", 2190 0, 1, allergenicIndicator)); 2191 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of this Ingredient.", 0, 2192 java.lang.Integer.MAX_VALUE, manufacturer)); 2193 children.add(new Property("specifiedSubstance", "", "A specified substance that comprises this ingredient.", 0, 2194 java.lang.Integer.MAX_VALUE, specifiedSubstance)); 2195 children.add(new Property("substance", "", "The ingredient substance.", 0, 1, substance)); 2196 } 2197 2198 @Override 2199 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2200 switch (_hash) { 2201 case -1618432855: 2202 /* identifier */ return new Property("identifier", "Identifier", 2203 "The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 2204 0, 1, identifier); 2205 case 3506294: 2206 /* role */ return new Property("role", "CodeableConcept", "Ingredient role e.g. Active ingredient, excipient.", 0, 2207 1, role); 2208 case 75406931: 2209 /* allergenicIndicator */ return new Property("allergenicIndicator", "boolean", 2210 "If the ingredient is a known or suspected allergen.", 0, 1, allergenicIndicator); 2211 case -1969347631: 2212 /* manufacturer */ return new Property("manufacturer", "Reference(Organization)", 2213 "Manufacturer of this Ingredient.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2214 case -331477600: 2215 /* specifiedSubstance */ return new Property("specifiedSubstance", "", 2216 "A specified substance that comprises this ingredient.", 0, java.lang.Integer.MAX_VALUE, specifiedSubstance); 2217 case 530040176: 2218 /* substance */ return new Property("substance", "", "The ingredient substance.", 0, 1, substance); 2219 default: 2220 return super.getNamedProperty(_hash, _name, _checkValid); 2221 } 2222 2223 } 2224 2225 @Override 2226 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2227 switch (hash) { 2228 case -1618432855: 2229 /* identifier */ return this.identifier == null ? new Base[0] : new Base[] { this.identifier }; // Identifier 2230 case 3506294: 2231 /* role */ return this.role == null ? new Base[0] : new Base[] { this.role }; // CodeableConcept 2232 case 75406931: 2233 /* allergenicIndicator */ return this.allergenicIndicator == null ? new Base[0] 2234 : new Base[] { this.allergenicIndicator }; // BooleanType 2235 case -1969347631: 2236 /* manufacturer */ return this.manufacturer == null ? new Base[0] 2237 : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 2238 case -331477600: 2239 /* specifiedSubstance */ return this.specifiedSubstance == null ? new Base[0] 2240 : this.specifiedSubstance.toArray(new Base[this.specifiedSubstance.size()]); // MedicinalProductIngredientSpecifiedSubstanceComponent 2241 case 530040176: 2242 /* substance */ return this.substance == null ? new Base[0] : new Base[] { this.substance }; // MedicinalProductIngredientSubstanceComponent 2243 default: 2244 return super.getProperty(hash, name, checkValid); 2245 } 2246 2247 } 2248 2249 @Override 2250 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2251 switch (hash) { 2252 case -1618432855: // identifier 2253 this.identifier = castToIdentifier(value); // Identifier 2254 return value; 2255 case 3506294: // role 2256 this.role = castToCodeableConcept(value); // CodeableConcept 2257 return value; 2258 case 75406931: // allergenicIndicator 2259 this.allergenicIndicator = castToBoolean(value); // BooleanType 2260 return value; 2261 case -1969347631: // manufacturer 2262 this.getManufacturer().add(castToReference(value)); // Reference 2263 return value; 2264 case -331477600: // specifiedSubstance 2265 this.getSpecifiedSubstance().add((MedicinalProductIngredientSpecifiedSubstanceComponent) value); // MedicinalProductIngredientSpecifiedSubstanceComponent 2266 return value; 2267 case 530040176: // substance 2268 this.substance = (MedicinalProductIngredientSubstanceComponent) value; // MedicinalProductIngredientSubstanceComponent 2269 return value; 2270 default: 2271 return super.setProperty(hash, name, value); 2272 } 2273 2274 } 2275 2276 @Override 2277 public Base setProperty(String name, Base value) throws FHIRException { 2278 if (name.equals("identifier")) { 2279 this.identifier = castToIdentifier(value); // Identifier 2280 } else if (name.equals("role")) { 2281 this.role = castToCodeableConcept(value); // CodeableConcept 2282 } else if (name.equals("allergenicIndicator")) { 2283 this.allergenicIndicator = castToBoolean(value); // BooleanType 2284 } else if (name.equals("manufacturer")) { 2285 this.getManufacturer().add(castToReference(value)); 2286 } else if (name.equals("specifiedSubstance")) { 2287 this.getSpecifiedSubstance().add((MedicinalProductIngredientSpecifiedSubstanceComponent) value); 2288 } else if (name.equals("substance")) { 2289 this.substance = (MedicinalProductIngredientSubstanceComponent) value; // MedicinalProductIngredientSubstanceComponent 2290 } else 2291 return super.setProperty(name, value); 2292 return value; 2293 } 2294 2295 @Override 2296 public void removeChild(String name, Base value) throws FHIRException { 2297 if (name.equals("identifier")) { 2298 this.identifier = null; 2299 } else if (name.equals("role")) { 2300 this.role = null; 2301 } else if (name.equals("allergenicIndicator")) { 2302 this.allergenicIndicator = null; 2303 } else if (name.equals("manufacturer")) { 2304 this.getManufacturer().remove(castToReference(value)); 2305 } else if (name.equals("specifiedSubstance")) { 2306 this.getSpecifiedSubstance().remove((MedicinalProductIngredientSpecifiedSubstanceComponent) value); 2307 } else if (name.equals("substance")) { 2308 this.substance = (MedicinalProductIngredientSubstanceComponent) value; // MedicinalProductIngredientSubstanceComponent 2309 } else 2310 super.removeChild(name, value); 2311 2312 } 2313 2314 @Override 2315 public Base makeProperty(int hash, String name) throws FHIRException { 2316 switch (hash) { 2317 case -1618432855: 2318 return getIdentifier(); 2319 case 3506294: 2320 return getRole(); 2321 case 75406931: 2322 return getAllergenicIndicatorElement(); 2323 case -1969347631: 2324 return addManufacturer(); 2325 case -331477600: 2326 return addSpecifiedSubstance(); 2327 case 530040176: 2328 return getSubstance(); 2329 default: 2330 return super.makeProperty(hash, name); 2331 } 2332 2333 } 2334 2335 @Override 2336 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2337 switch (hash) { 2338 case -1618432855: 2339 /* identifier */ return new String[] { "Identifier" }; 2340 case 3506294: 2341 /* role */ return new String[] { "CodeableConcept" }; 2342 case 75406931: 2343 /* allergenicIndicator */ return new String[] { "boolean" }; 2344 case -1969347631: 2345 /* manufacturer */ return new String[] { "Reference" }; 2346 case -331477600: 2347 /* specifiedSubstance */ return new String[] {}; 2348 case 530040176: 2349 /* substance */ return new String[] {}; 2350 default: 2351 return super.getTypesForProperty(hash, name); 2352 } 2353 2354 } 2355 2356 @Override 2357 public Base addChild(String name) throws FHIRException { 2358 if (name.equals("identifier")) { 2359 this.identifier = new Identifier(); 2360 return this.identifier; 2361 } else if (name.equals("role")) { 2362 this.role = new CodeableConcept(); 2363 return this.role; 2364 } else if (name.equals("allergenicIndicator")) { 2365 throw new FHIRException( 2366 "Cannot call addChild on a singleton property MedicinalProductIngredient.allergenicIndicator"); 2367 } else if (name.equals("manufacturer")) { 2368 return addManufacturer(); 2369 } else if (name.equals("specifiedSubstance")) { 2370 return addSpecifiedSubstance(); 2371 } else if (name.equals("substance")) { 2372 this.substance = new MedicinalProductIngredientSubstanceComponent(); 2373 return this.substance; 2374 } else 2375 return super.addChild(name); 2376 } 2377 2378 public String fhirType() { 2379 return "MedicinalProductIngredient"; 2380 2381 } 2382 2383 public MedicinalProductIngredient copy() { 2384 MedicinalProductIngredient dst = new MedicinalProductIngredient(); 2385 copyValues(dst); 2386 return dst; 2387 } 2388 2389 public void copyValues(MedicinalProductIngredient dst) { 2390 super.copyValues(dst); 2391 dst.identifier = identifier == null ? null : identifier.copy(); 2392 dst.role = role == null ? null : role.copy(); 2393 dst.allergenicIndicator = allergenicIndicator == null ? null : allergenicIndicator.copy(); 2394 if (manufacturer != null) { 2395 dst.manufacturer = new ArrayList<Reference>(); 2396 for (Reference i : manufacturer) 2397 dst.manufacturer.add(i.copy()); 2398 } 2399 ; 2400 if (specifiedSubstance != null) { 2401 dst.specifiedSubstance = new ArrayList<MedicinalProductIngredientSpecifiedSubstanceComponent>(); 2402 for (MedicinalProductIngredientSpecifiedSubstanceComponent i : specifiedSubstance) 2403 dst.specifiedSubstance.add(i.copy()); 2404 } 2405 ; 2406 dst.substance = substance == null ? null : substance.copy(); 2407 } 2408 2409 protected MedicinalProductIngredient typedCopy() { 2410 return copy(); 2411 } 2412 2413 @Override 2414 public boolean equalsDeep(Base other_) { 2415 if (!super.equalsDeep(other_)) 2416 return false; 2417 if (!(other_ instanceof MedicinalProductIngredient)) 2418 return false; 2419 MedicinalProductIngredient o = (MedicinalProductIngredient) other_; 2420 return compareDeep(identifier, o.identifier, true) && compareDeep(role, o.role, true) 2421 && compareDeep(allergenicIndicator, o.allergenicIndicator, true) 2422 && compareDeep(manufacturer, o.manufacturer, true) 2423 && compareDeep(specifiedSubstance, o.specifiedSubstance, true) && compareDeep(substance, o.substance, true); 2424 } 2425 2426 @Override 2427 public boolean equalsShallow(Base other_) { 2428 if (!super.equalsShallow(other_)) 2429 return false; 2430 if (!(other_ instanceof MedicinalProductIngredient)) 2431 return false; 2432 MedicinalProductIngredient o = (MedicinalProductIngredient) other_; 2433 return compareValues(allergenicIndicator, o.allergenicIndicator, true); 2434 } 2435 2436 public boolean isEmpty() { 2437 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, role, allergenicIndicator, manufacturer, 2438 specifiedSubstance, substance); 2439 } 2440 2441 @Override 2442 public ResourceType getResourceType() { 2443 return ResourceType.MedicinalProductIngredient; 2444 } 2445 2446}