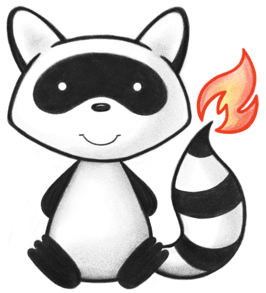
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * The interactions of the medicinal product with other medicinal products, or 048 * other forms of interactions. 049 */ 050@ResourceDef(name = "MedicinalProductInteraction", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductInteraction") 051public class MedicinalProductInteraction extends DomainResource { 052 053 @Block() 054 public static class MedicinalProductInteractionInteractantComponent extends BackboneElement 055 implements IBaseBackboneElement { 056 /** 057 * The specific medication, food or laboratory test that interacts. 058 */ 059 @Child(name = "item", type = { MedicinalProduct.class, Medication.class, Substance.class, 060 ObservationDefinition.class, 061 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 062 @Description(shortDefinition = "The specific medication, food or laboratory test that interacts", formalDefinition = "The specific medication, food or laboratory test that interacts.") 063 protected Type item; 064 065 private static final long serialVersionUID = 1445276561L; 066 067 /** 068 * Constructor 069 */ 070 public MedicinalProductInteractionInteractantComponent() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public MedicinalProductInteractionInteractantComponent(Type item) { 078 super(); 079 this.item = item; 080 } 081 082 /** 083 * @return {@link #item} (The specific medication, food or laboratory test that 084 * interacts.) 085 */ 086 public Type getItem() { 087 return this.item; 088 } 089 090 /** 091 * @return {@link #item} (The specific medication, food or laboratory test that 092 * interacts.) 093 */ 094 public Reference getItemReference() throws FHIRException { 095 if (this.item == null) 096 this.item = new Reference(); 097 if (!(this.item instanceof Reference)) 098 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.item.getClass().getName() 099 + " was encountered"); 100 return (Reference) this.item; 101 } 102 103 public boolean hasItemReference() { 104 return this != null && this.item instanceof Reference; 105 } 106 107 /** 108 * @return {@link #item} (The specific medication, food or laboratory test that 109 * interacts.) 110 */ 111 public CodeableConcept getItemCodeableConcept() throws FHIRException { 112 if (this.item == null) 113 this.item = new CodeableConcept(); 114 if (!(this.item instanceof CodeableConcept)) 115 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 116 + this.item.getClass().getName() + " was encountered"); 117 return (CodeableConcept) this.item; 118 } 119 120 public boolean hasItemCodeableConcept() { 121 return this != null && this.item instanceof CodeableConcept; 122 } 123 124 public boolean hasItem() { 125 return this.item != null && !this.item.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #item} (The specific medication, food or laboratory test 130 * that interacts.) 131 */ 132 public MedicinalProductInteractionInteractantComponent setItem(Type value) { 133 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 134 throw new Error("Not the right type for MedicinalProductInteraction.interactant.item[x]: " + value.fhirType()); 135 this.item = value; 136 return this; 137 } 138 139 protected void listChildren(List<Property> children) { 140 super.listChildren(children); 141 children.add(new Property("item[x]", 142 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 143 "The specific medication, food or laboratory test that interacts.", 0, 1, item)); 144 } 145 146 @Override 147 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 148 switch (_hash) { 149 case 2116201613: 150 /* item[x] */ return new Property("item[x]", 151 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 152 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 153 case 3242771: 154 /* item */ return new Property("item[x]", 155 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 156 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 157 case 1376364920: 158 /* itemReference */ return new Property("item[x]", 159 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 160 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 161 case 106644494: 162 /* itemCodeableConcept */ return new Property("item[x]", 163 "Reference(MedicinalProduct|Medication|Substance|ObservationDefinition)|CodeableConcept", 164 "The specific medication, food or laboratory test that interacts.", 0, 1, item); 165 default: 166 return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3242771: 175 /* item */ return this.item == null ? new Base[0] : new Base[] { this.item }; // Type 176 default: 177 return super.getProperty(hash, name, checkValid); 178 } 179 180 } 181 182 @Override 183 public Base setProperty(int hash, String name, Base value) throws FHIRException { 184 switch (hash) { 185 case 3242771: // item 186 this.item = castToType(value); // Type 187 return value; 188 default: 189 return super.setProperty(hash, name, value); 190 } 191 192 } 193 194 @Override 195 public Base setProperty(String name, Base value) throws FHIRException { 196 if (name.equals("item[x]")) { 197 this.item = castToType(value); // Type 198 } else 199 return super.setProperty(name, value); 200 return value; 201 } 202 203 @Override 204 public Base makeProperty(int hash, String name) throws FHIRException { 205 switch (hash) { 206 case 2116201613: 207 return getItem(); 208 case 3242771: 209 return getItem(); 210 default: 211 return super.makeProperty(hash, name); 212 } 213 214 } 215 216 @Override 217 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 218 switch (hash) { 219 case 3242771: 220 /* item */ return new String[] { "Reference", "CodeableConcept" }; 221 default: 222 return super.getTypesForProperty(hash, name); 223 } 224 225 } 226 227 @Override 228 public Base addChild(String name) throws FHIRException { 229 if (name.equals("itemReference")) { 230 this.item = new Reference(); 231 return this.item; 232 } else if (name.equals("itemCodeableConcept")) { 233 this.item = new CodeableConcept(); 234 return this.item; 235 } else 236 return super.addChild(name); 237 } 238 239 public MedicinalProductInteractionInteractantComponent copy() { 240 MedicinalProductInteractionInteractantComponent dst = new MedicinalProductInteractionInteractantComponent(); 241 copyValues(dst); 242 return dst; 243 } 244 245 public void copyValues(MedicinalProductInteractionInteractantComponent dst) { 246 super.copyValues(dst); 247 dst.item = item == null ? null : item.copy(); 248 } 249 250 @Override 251 public boolean equalsDeep(Base other_) { 252 if (!super.equalsDeep(other_)) 253 return false; 254 if (!(other_ instanceof MedicinalProductInteractionInteractantComponent)) 255 return false; 256 MedicinalProductInteractionInteractantComponent o = (MedicinalProductInteractionInteractantComponent) other_; 257 return compareDeep(item, o.item, true); 258 } 259 260 @Override 261 public boolean equalsShallow(Base other_) { 262 if (!super.equalsShallow(other_)) 263 return false; 264 if (!(other_ instanceof MedicinalProductInteractionInteractantComponent)) 265 return false; 266 MedicinalProductInteractionInteractantComponent o = (MedicinalProductInteractionInteractantComponent) other_; 267 return true; 268 } 269 270 public boolean isEmpty() { 271 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item); 272 } 273 274 public String fhirType() { 275 return "MedicinalProductInteraction.interactant"; 276 277 } 278 279 } 280 281 /** 282 * The medication for which this is a described interaction. 283 */ 284 @Child(name = "subject", type = { MedicinalProduct.class, Medication.class, 285 Substance.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 286 @Description(shortDefinition = "The medication for which this is a described interaction", formalDefinition = "The medication for which this is a described interaction.") 287 protected List<Reference> subject; 288 /** 289 * The actual objects that are the target of the reference (The medication for 290 * which this is a described interaction.) 291 */ 292 protected List<Resource> subjectTarget; 293 294 /** 295 * The interaction described. 296 */ 297 @Child(name = "description", type = { 298 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 299 @Description(shortDefinition = "The interaction described", formalDefinition = "The interaction described.") 300 protected StringType description; 301 302 /** 303 * The specific medication, food or laboratory test that interacts. 304 */ 305 @Child(name = "interactant", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 306 @Description(shortDefinition = "The specific medication, food or laboratory test that interacts", formalDefinition = "The specific medication, food or laboratory test that interacts.") 307 protected List<MedicinalProductInteractionInteractantComponent> interactant; 308 309 /** 310 * The type of the interaction e.g. drug-drug interaction, drug-food 311 * interaction, drug-lab test interaction. 312 */ 313 @Child(name = "type", type = { CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 314 @Description(shortDefinition = "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction", formalDefinition = "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.") 315 protected CodeableConcept type; 316 317 /** 318 * The effect of the interaction, for example "reduced gastric absorption of 319 * primary medication". 320 */ 321 @Child(name = "effect", type = { 322 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 323 @Description(shortDefinition = "The effect of the interaction, for example \"reduced gastric absorption of primary medication\"", formalDefinition = "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".") 324 protected CodeableConcept effect; 325 326 /** 327 * The incidence of the interaction, e.g. theoretical, observed. 328 */ 329 @Child(name = "incidence", type = { 330 CodeableConcept.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 331 @Description(shortDefinition = "The incidence of the interaction, e.g. theoretical, observed", formalDefinition = "The incidence of the interaction, e.g. theoretical, observed.") 332 protected CodeableConcept incidence; 333 334 /** 335 * Actions for managing the interaction. 336 */ 337 @Child(name = "management", type = { 338 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 339 @Description(shortDefinition = "Actions for managing the interaction", formalDefinition = "Actions for managing the interaction.") 340 protected CodeableConcept management; 341 342 private static final long serialVersionUID = -1872687169L; 343 344 /** 345 * Constructor 346 */ 347 public MedicinalProductInteraction() { 348 super(); 349 } 350 351 /** 352 * @return {@link #subject} (The medication for which this is a described 353 * interaction.) 354 */ 355 public List<Reference> getSubject() { 356 if (this.subject == null) 357 this.subject = new ArrayList<Reference>(); 358 return this.subject; 359 } 360 361 /** 362 * @return Returns a reference to <code>this</code> for easy method chaining 363 */ 364 public MedicinalProductInteraction setSubject(List<Reference> theSubject) { 365 this.subject = theSubject; 366 return this; 367 } 368 369 public boolean hasSubject() { 370 if (this.subject == null) 371 return false; 372 for (Reference item : this.subject) 373 if (!item.isEmpty()) 374 return true; 375 return false; 376 } 377 378 public Reference addSubject() { // 3 379 Reference t = new Reference(); 380 if (this.subject == null) 381 this.subject = new ArrayList<Reference>(); 382 this.subject.add(t); 383 return t; 384 } 385 386 public MedicinalProductInteraction addSubject(Reference t) { // 3 387 if (t == null) 388 return this; 389 if (this.subject == null) 390 this.subject = new ArrayList<Reference>(); 391 this.subject.add(t); 392 return this; 393 } 394 395 /** 396 * @return The first repetition of repeating field {@link #subject}, creating it 397 * if it does not already exist 398 */ 399 public Reference getSubjectFirstRep() { 400 if (getSubject().isEmpty()) { 401 addSubject(); 402 } 403 return getSubject().get(0); 404 } 405 406 /** 407 * @deprecated Use Reference#setResource(IBaseResource) instead 408 */ 409 @Deprecated 410 public List<Resource> getSubjectTarget() { 411 if (this.subjectTarget == null) 412 this.subjectTarget = new ArrayList<Resource>(); 413 return this.subjectTarget; 414 } 415 416 /** 417 * @return {@link #description} (The interaction described.). This is the 418 * underlying object with id, value and extensions. The accessor 419 * "getDescription" gives direct access to the value 420 */ 421 public StringType getDescriptionElement() { 422 if (this.description == null) 423 if (Configuration.errorOnAutoCreate()) 424 throw new Error("Attempt to auto-create MedicinalProductInteraction.description"); 425 else if (Configuration.doAutoCreate()) 426 this.description = new StringType(); // bb 427 return this.description; 428 } 429 430 public boolean hasDescriptionElement() { 431 return this.description != null && !this.description.isEmpty(); 432 } 433 434 public boolean hasDescription() { 435 return this.description != null && !this.description.isEmpty(); 436 } 437 438 /** 439 * @param value {@link #description} (The interaction described.). This is the 440 * underlying object with id, value and extensions. The accessor 441 * "getDescription" gives direct access to the value 442 */ 443 public MedicinalProductInteraction setDescriptionElement(StringType value) { 444 this.description = value; 445 return this; 446 } 447 448 /** 449 * @return The interaction described. 450 */ 451 public String getDescription() { 452 return this.description == null ? null : this.description.getValue(); 453 } 454 455 /** 456 * @param value The interaction described. 457 */ 458 public MedicinalProductInteraction setDescription(String value) { 459 if (Utilities.noString(value)) 460 this.description = null; 461 else { 462 if (this.description == null) 463 this.description = new StringType(); 464 this.description.setValue(value); 465 } 466 return this; 467 } 468 469 /** 470 * @return {@link #interactant} (The specific medication, food or laboratory 471 * test that interacts.) 472 */ 473 public List<MedicinalProductInteractionInteractantComponent> getInteractant() { 474 if (this.interactant == null) 475 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 476 return this.interactant; 477 } 478 479 /** 480 * @return Returns a reference to <code>this</code> for easy method chaining 481 */ 482 public MedicinalProductInteraction setInteractant( 483 List<MedicinalProductInteractionInteractantComponent> theInteractant) { 484 this.interactant = theInteractant; 485 return this; 486 } 487 488 public boolean hasInteractant() { 489 if (this.interactant == null) 490 return false; 491 for (MedicinalProductInteractionInteractantComponent item : this.interactant) 492 if (!item.isEmpty()) 493 return true; 494 return false; 495 } 496 497 public MedicinalProductInteractionInteractantComponent addInteractant() { // 3 498 MedicinalProductInteractionInteractantComponent t = new MedicinalProductInteractionInteractantComponent(); 499 if (this.interactant == null) 500 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 501 this.interactant.add(t); 502 return t; 503 } 504 505 public MedicinalProductInteraction addInteractant(MedicinalProductInteractionInteractantComponent t) { // 3 506 if (t == null) 507 return this; 508 if (this.interactant == null) 509 this.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 510 this.interactant.add(t); 511 return this; 512 } 513 514 /** 515 * @return The first repetition of repeating field {@link #interactant}, 516 * creating it if it does not already exist 517 */ 518 public MedicinalProductInteractionInteractantComponent getInteractantFirstRep() { 519 if (getInteractant().isEmpty()) { 520 addInteractant(); 521 } 522 return getInteractant().get(0); 523 } 524 525 /** 526 * @return {@link #type} (The type of the interaction e.g. drug-drug 527 * interaction, drug-food interaction, drug-lab test interaction.) 528 */ 529 public CodeableConcept getType() { 530 if (this.type == null) 531 if (Configuration.errorOnAutoCreate()) 532 throw new Error("Attempt to auto-create MedicinalProductInteraction.type"); 533 else if (Configuration.doAutoCreate()) 534 this.type = new CodeableConcept(); // cc 535 return this.type; 536 } 537 538 public boolean hasType() { 539 return this.type != null && !this.type.isEmpty(); 540 } 541 542 /** 543 * @param value {@link #type} (The type of the interaction e.g. drug-drug 544 * interaction, drug-food interaction, drug-lab test interaction.) 545 */ 546 public MedicinalProductInteraction setType(CodeableConcept value) { 547 this.type = value; 548 return this; 549 } 550 551 /** 552 * @return {@link #effect} (The effect of the interaction, for example "reduced 553 * gastric absorption of primary medication".) 554 */ 555 public CodeableConcept getEffect() { 556 if (this.effect == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create MedicinalProductInteraction.effect"); 559 else if (Configuration.doAutoCreate()) 560 this.effect = new CodeableConcept(); // cc 561 return this.effect; 562 } 563 564 public boolean hasEffect() { 565 return this.effect != null && !this.effect.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #effect} (The effect of the interaction, for example 570 * "reduced gastric absorption of primary medication".) 571 */ 572 public MedicinalProductInteraction setEffect(CodeableConcept value) { 573 this.effect = value; 574 return this; 575 } 576 577 /** 578 * @return {@link #incidence} (The incidence of the interaction, e.g. 579 * theoretical, observed.) 580 */ 581 public CodeableConcept getIncidence() { 582 if (this.incidence == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create MedicinalProductInteraction.incidence"); 585 else if (Configuration.doAutoCreate()) 586 this.incidence = new CodeableConcept(); // cc 587 return this.incidence; 588 } 589 590 public boolean hasIncidence() { 591 return this.incidence != null && !this.incidence.isEmpty(); 592 } 593 594 /** 595 * @param value {@link #incidence} (The incidence of the interaction, e.g. 596 * theoretical, observed.) 597 */ 598 public MedicinalProductInteraction setIncidence(CodeableConcept value) { 599 this.incidence = value; 600 return this; 601 } 602 603 /** 604 * @return {@link #management} (Actions for managing the interaction.) 605 */ 606 public CodeableConcept getManagement() { 607 if (this.management == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create MedicinalProductInteraction.management"); 610 else if (Configuration.doAutoCreate()) 611 this.management = new CodeableConcept(); // cc 612 return this.management; 613 } 614 615 public boolean hasManagement() { 616 return this.management != null && !this.management.isEmpty(); 617 } 618 619 /** 620 * @param value {@link #management} (Actions for managing the interaction.) 621 */ 622 public MedicinalProductInteraction setManagement(CodeableConcept value) { 623 this.management = value; 624 return this; 625 } 626 627 protected void listChildren(List<Property> children) { 628 super.listChildren(children); 629 children.add(new Property("subject", "Reference(MedicinalProduct|Medication|Substance)", 630 "The medication for which this is a described interaction.", 0, java.lang.Integer.MAX_VALUE, subject)); 631 children.add(new Property("description", "string", "The interaction described.", 0, 1, description)); 632 children.add(new Property("interactant", "", "The specific medication, food or laboratory test that interacts.", 0, 633 java.lang.Integer.MAX_VALUE, interactant)); 634 children.add(new Property("type", "CodeableConcept", 635 "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 0, 636 1, type)); 637 children.add(new Property("effect", "CodeableConcept", 638 "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, 639 effect)); 640 children.add(new Property("incidence", "CodeableConcept", 641 "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence)); 642 children 643 .add(new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, 1, management)); 644 } 645 646 @Override 647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 648 switch (_hash) { 649 case -1867885268: 650 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication|Substance)", 651 "The medication for which this is a described interaction.", 0, java.lang.Integer.MAX_VALUE, subject); 652 case -1724546052: 653 /* description */ return new Property("description", "string", "The interaction described.", 0, 1, description); 654 case 1844097009: 655 /* interactant */ return new Property("interactant", "", 656 "The specific medication, food or laboratory test that interacts.", 0, java.lang.Integer.MAX_VALUE, 657 interactant); 658 case 3575610: 659 /* type */ return new Property("type", "CodeableConcept", 660 "The type of the interaction e.g. drug-drug interaction, drug-food interaction, drug-lab test interaction.", 661 0, 1, type); 662 case -1306084975: 663 /* effect */ return new Property("effect", "CodeableConcept", 664 "The effect of the interaction, for example \"reduced gastric absorption of primary medication\".", 0, 1, 665 effect); 666 case -1598467132: 667 /* incidence */ return new Property("incidence", "CodeableConcept", 668 "The incidence of the interaction, e.g. theoretical, observed.", 0, 1, incidence); 669 case -1799980989: 670 /* management */ return new Property("management", "CodeableConcept", "Actions for managing the interaction.", 0, 671 1, management); 672 default: 673 return super.getNamedProperty(_hash, _name, _checkValid); 674 } 675 676 } 677 678 @Override 679 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 680 switch (hash) { 681 case -1867885268: 682 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 683 case -1724546052: 684 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // StringType 685 case 1844097009: 686 /* interactant */ return this.interactant == null ? new Base[0] 687 : this.interactant.toArray(new Base[this.interactant.size()]); // MedicinalProductInteractionInteractantComponent 688 case 3575610: 689 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 690 case -1306084975: 691 /* effect */ return this.effect == null ? new Base[0] : new Base[] { this.effect }; // CodeableConcept 692 case -1598467132: 693 /* incidence */ return this.incidence == null ? new Base[0] : new Base[] { this.incidence }; // CodeableConcept 694 case -1799980989: 695 /* management */ return this.management == null ? new Base[0] : new Base[] { this.management }; // CodeableConcept 696 default: 697 return super.getProperty(hash, name, checkValid); 698 } 699 700 } 701 702 @Override 703 public Base setProperty(int hash, String name, Base value) throws FHIRException { 704 switch (hash) { 705 case -1867885268: // subject 706 this.getSubject().add(castToReference(value)); // Reference 707 return value; 708 case -1724546052: // description 709 this.description = castToString(value); // StringType 710 return value; 711 case 1844097009: // interactant 712 this.getInteractant().add((MedicinalProductInteractionInteractantComponent) value); // MedicinalProductInteractionInteractantComponent 713 return value; 714 case 3575610: // type 715 this.type = castToCodeableConcept(value); // CodeableConcept 716 return value; 717 case -1306084975: // effect 718 this.effect = castToCodeableConcept(value); // CodeableConcept 719 return value; 720 case -1598467132: // incidence 721 this.incidence = castToCodeableConcept(value); // CodeableConcept 722 return value; 723 case -1799980989: // management 724 this.management = castToCodeableConcept(value); // CodeableConcept 725 return value; 726 default: 727 return super.setProperty(hash, name, value); 728 } 729 730 } 731 732 @Override 733 public Base setProperty(String name, Base value) throws FHIRException { 734 if (name.equals("subject")) { 735 this.getSubject().add(castToReference(value)); 736 } else if (name.equals("description")) { 737 this.description = castToString(value); // StringType 738 } else if (name.equals("interactant")) { 739 this.getInteractant().add((MedicinalProductInteractionInteractantComponent) value); 740 } else if (name.equals("type")) { 741 this.type = castToCodeableConcept(value); // CodeableConcept 742 } else if (name.equals("effect")) { 743 this.effect = castToCodeableConcept(value); // CodeableConcept 744 } else if (name.equals("incidence")) { 745 this.incidence = castToCodeableConcept(value); // CodeableConcept 746 } else if (name.equals("management")) { 747 this.management = castToCodeableConcept(value); // CodeableConcept 748 } else 749 return super.setProperty(name, value); 750 return value; 751 } 752 753 @Override 754 public Base makeProperty(int hash, String name) throws FHIRException { 755 switch (hash) { 756 case -1867885268: 757 return addSubject(); 758 case -1724546052: 759 return getDescriptionElement(); 760 case 1844097009: 761 return addInteractant(); 762 case 3575610: 763 return getType(); 764 case -1306084975: 765 return getEffect(); 766 case -1598467132: 767 return getIncidence(); 768 case -1799980989: 769 return getManagement(); 770 default: 771 return super.makeProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 778 switch (hash) { 779 case -1867885268: 780 /* subject */ return new String[] { "Reference" }; 781 case -1724546052: 782 /* description */ return new String[] { "string" }; 783 case 1844097009: 784 /* interactant */ return new String[] {}; 785 case 3575610: 786 /* type */ return new String[] { "CodeableConcept" }; 787 case -1306084975: 788 /* effect */ return new String[] { "CodeableConcept" }; 789 case -1598467132: 790 /* incidence */ return new String[] { "CodeableConcept" }; 791 case -1799980989: 792 /* management */ return new String[] { "CodeableConcept" }; 793 default: 794 return super.getTypesForProperty(hash, name); 795 } 796 797 } 798 799 @Override 800 public Base addChild(String name) throws FHIRException { 801 if (name.equals("subject")) { 802 return addSubject(); 803 } else if (name.equals("description")) { 804 throw new FHIRException("Cannot call addChild on a singleton property MedicinalProductInteraction.description"); 805 } else if (name.equals("interactant")) { 806 return addInteractant(); 807 } else if (name.equals("type")) { 808 this.type = new CodeableConcept(); 809 return this.type; 810 } else if (name.equals("effect")) { 811 this.effect = new CodeableConcept(); 812 return this.effect; 813 } else if (name.equals("incidence")) { 814 this.incidence = new CodeableConcept(); 815 return this.incidence; 816 } else if (name.equals("management")) { 817 this.management = new CodeableConcept(); 818 return this.management; 819 } else 820 return super.addChild(name); 821 } 822 823 public String fhirType() { 824 return "MedicinalProductInteraction"; 825 826 } 827 828 public MedicinalProductInteraction copy() { 829 MedicinalProductInteraction dst = new MedicinalProductInteraction(); 830 copyValues(dst); 831 return dst; 832 } 833 834 public void copyValues(MedicinalProductInteraction dst) { 835 super.copyValues(dst); 836 if (subject != null) { 837 dst.subject = new ArrayList<Reference>(); 838 for (Reference i : subject) 839 dst.subject.add(i.copy()); 840 } 841 ; 842 dst.description = description == null ? null : description.copy(); 843 if (interactant != null) { 844 dst.interactant = new ArrayList<MedicinalProductInteractionInteractantComponent>(); 845 for (MedicinalProductInteractionInteractantComponent i : interactant) 846 dst.interactant.add(i.copy()); 847 } 848 ; 849 dst.type = type == null ? null : type.copy(); 850 dst.effect = effect == null ? null : effect.copy(); 851 dst.incidence = incidence == null ? null : incidence.copy(); 852 dst.management = management == null ? null : management.copy(); 853 } 854 855 protected MedicinalProductInteraction typedCopy() { 856 return copy(); 857 } 858 859 @Override 860 public boolean equalsDeep(Base other_) { 861 if (!super.equalsDeep(other_)) 862 return false; 863 if (!(other_ instanceof MedicinalProductInteraction)) 864 return false; 865 MedicinalProductInteraction o = (MedicinalProductInteraction) other_; 866 return compareDeep(subject, o.subject, true) && compareDeep(description, o.description, true) 867 && compareDeep(interactant, o.interactant, true) && compareDeep(type, o.type, true) 868 && compareDeep(effect, o.effect, true) && compareDeep(incidence, o.incidence, true) 869 && compareDeep(management, o.management, true); 870 } 871 872 @Override 873 public boolean equalsShallow(Base other_) { 874 if (!super.equalsShallow(other_)) 875 return false; 876 if (!(other_ instanceof MedicinalProductInteraction)) 877 return false; 878 MedicinalProductInteraction o = (MedicinalProductInteraction) other_; 879 return compareValues(description, o.description, true); 880 } 881 882 public boolean isEmpty() { 883 return super.isEmpty() 884 && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, description, interactant, type, effect, incidence, management); 885 } 886 887 @Override 888 public ResourceType getResourceType() { 889 return ResourceType.MedicinalProductInteraction; 890 } 891 892 /** 893 * Search parameter: <b>subject</b> 894 * <p> 895 * Description: <b>The medication for which this is an interaction</b><br> 896 * Type: <b>reference</b><br> 897 * Path: <b>MedicinalProductInteraction.subject</b><br> 898 * </p> 899 */ 900 @SearchParamDefinition(name = "subject", path = "MedicinalProductInteraction.subject", description = "The medication for which this is an interaction", type = "reference", target = { 901 Medication.class, MedicinalProduct.class, Substance.class }) 902 public static final String SP_SUBJECT = "subject"; 903 /** 904 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 905 * <p> 906 * Description: <b>The medication for which this is an interaction</b><br> 907 * Type: <b>reference</b><br> 908 * Path: <b>MedicinalProductInteraction.subject</b><br> 909 * </p> 910 */ 911 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 912 SP_SUBJECT); 913 914 /** 915 * Constant for fluent queries to be used to add include statements. Specifies 916 * the path value of "<b>MedicinalProductInteraction:subject</b>". 917 */ 918 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 919 "MedicinalProductInteraction:subject").toLocked(); 920 921}