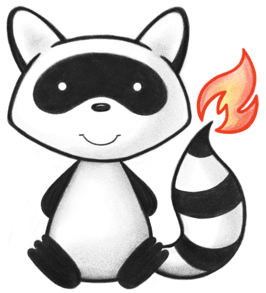
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Block; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045 046/** 047 * A pharmaceutical product described in terms of its composition and dose form. 048 */ 049@ResourceDef(name = "MedicinalProductPharmaceutical", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductPharmaceutical") 050public class MedicinalProductPharmaceutical extends DomainResource { 051 052 @Block() 053 public static class MedicinalProductPharmaceuticalCharacteristicsComponent extends BackboneElement 054 implements IBaseBackboneElement { 055 /** 056 * A coded characteristic. 057 */ 058 @Child(name = "code", type = { 059 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "A coded characteristic", formalDefinition = "A coded characteristic.") 061 protected CodeableConcept code; 062 063 /** 064 * The status of characteristic e.g. assigned or pending. 065 */ 066 @Child(name = "status", type = { 067 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "The status of characteristic e.g. assigned or pending", formalDefinition = "The status of characteristic e.g. assigned or pending.") 069 protected CodeableConcept status; 070 071 private static final long serialVersionUID = 1414556635L; 072 073 /** 074 * Constructor 075 */ 076 public MedicinalProductPharmaceuticalCharacteristicsComponent() { 077 super(); 078 } 079 080 /** 081 * Constructor 082 */ 083 public MedicinalProductPharmaceuticalCharacteristicsComponent(CodeableConcept code) { 084 super(); 085 this.code = code; 086 } 087 088 /** 089 * @return {@link #code} (A coded characteristic.) 090 */ 091 public CodeableConcept getCode() { 092 if (this.code == null) 093 if (Configuration.errorOnAutoCreate()) 094 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalCharacteristicsComponent.code"); 095 else if (Configuration.doAutoCreate()) 096 this.code = new CodeableConcept(); // cc 097 return this.code; 098 } 099 100 public boolean hasCode() { 101 return this.code != null && !this.code.isEmpty(); 102 } 103 104 /** 105 * @param value {@link #code} (A coded characteristic.) 106 */ 107 public MedicinalProductPharmaceuticalCharacteristicsComponent setCode(CodeableConcept value) { 108 this.code = value; 109 return this; 110 } 111 112 /** 113 * @return {@link #status} (The status of characteristic e.g. assigned or 114 * pending.) 115 */ 116 public CodeableConcept getStatus() { 117 if (this.status == null) 118 if (Configuration.errorOnAutoCreate()) 119 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalCharacteristicsComponent.status"); 120 else if (Configuration.doAutoCreate()) 121 this.status = new CodeableConcept(); // cc 122 return this.status; 123 } 124 125 public boolean hasStatus() { 126 return this.status != null && !this.status.isEmpty(); 127 } 128 129 /** 130 * @param value {@link #status} (The status of characteristic e.g. assigned or 131 * pending.) 132 */ 133 public MedicinalProductPharmaceuticalCharacteristicsComponent setStatus(CodeableConcept value) { 134 this.status = value; 135 return this; 136 } 137 138 protected void listChildren(List<Property> children) { 139 super.listChildren(children); 140 children.add(new Property("code", "CodeableConcept", "A coded characteristic.", 0, 1, code)); 141 children.add(new Property("status", "CodeableConcept", "The status of characteristic e.g. assigned or pending.", 142 0, 1, status)); 143 } 144 145 @Override 146 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 147 switch (_hash) { 148 case 3059181: 149 /* code */ return new Property("code", "CodeableConcept", "A coded characteristic.", 0, 1, code); 150 case -892481550: 151 /* status */ return new Property("status", "CodeableConcept", 152 "The status of characteristic e.g. assigned or pending.", 0, 1, status); 153 default: 154 return super.getNamedProperty(_hash, _name, _checkValid); 155 } 156 157 } 158 159 @Override 160 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 161 switch (hash) { 162 case 3059181: 163 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 164 case -892481550: 165 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // CodeableConcept 166 default: 167 return super.getProperty(hash, name, checkValid); 168 } 169 170 } 171 172 @Override 173 public Base setProperty(int hash, String name, Base value) throws FHIRException { 174 switch (hash) { 175 case 3059181: // code 176 this.code = castToCodeableConcept(value); // CodeableConcept 177 return value; 178 case -892481550: // status 179 this.status = castToCodeableConcept(value); // CodeableConcept 180 return value; 181 default: 182 return super.setProperty(hash, name, value); 183 } 184 185 } 186 187 @Override 188 public Base setProperty(String name, Base value) throws FHIRException { 189 if (name.equals("code")) { 190 this.code = castToCodeableConcept(value); // CodeableConcept 191 } else if (name.equals("status")) { 192 this.status = castToCodeableConcept(value); // CodeableConcept 193 } else 194 return super.setProperty(name, value); 195 return value; 196 } 197 198 @Override 199 public Base makeProperty(int hash, String name) throws FHIRException { 200 switch (hash) { 201 case 3059181: 202 return getCode(); 203 case -892481550: 204 return getStatus(); 205 default: 206 return super.makeProperty(hash, name); 207 } 208 209 } 210 211 @Override 212 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 213 switch (hash) { 214 case 3059181: 215 /* code */ return new String[] { "CodeableConcept" }; 216 case -892481550: 217 /* status */ return new String[] { "CodeableConcept" }; 218 default: 219 return super.getTypesForProperty(hash, name); 220 } 221 222 } 223 224 @Override 225 public Base addChild(String name) throws FHIRException { 226 if (name.equals("code")) { 227 this.code = new CodeableConcept(); 228 return this.code; 229 } else if (name.equals("status")) { 230 this.status = new CodeableConcept(); 231 return this.status; 232 } else 233 return super.addChild(name); 234 } 235 236 public MedicinalProductPharmaceuticalCharacteristicsComponent copy() { 237 MedicinalProductPharmaceuticalCharacteristicsComponent dst = new MedicinalProductPharmaceuticalCharacteristicsComponent(); 238 copyValues(dst); 239 return dst; 240 } 241 242 public void copyValues(MedicinalProductPharmaceuticalCharacteristicsComponent dst) { 243 super.copyValues(dst); 244 dst.code = code == null ? null : code.copy(); 245 dst.status = status == null ? null : status.copy(); 246 } 247 248 @Override 249 public boolean equalsDeep(Base other_) { 250 if (!super.equalsDeep(other_)) 251 return false; 252 if (!(other_ instanceof MedicinalProductPharmaceuticalCharacteristicsComponent)) 253 return false; 254 MedicinalProductPharmaceuticalCharacteristicsComponent o = (MedicinalProductPharmaceuticalCharacteristicsComponent) other_; 255 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true); 256 } 257 258 @Override 259 public boolean equalsShallow(Base other_) { 260 if (!super.equalsShallow(other_)) 261 return false; 262 if (!(other_ instanceof MedicinalProductPharmaceuticalCharacteristicsComponent)) 263 return false; 264 MedicinalProductPharmaceuticalCharacteristicsComponent o = (MedicinalProductPharmaceuticalCharacteristicsComponent) other_; 265 return true; 266 } 267 268 public boolean isEmpty() { 269 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status); 270 } 271 272 public String fhirType() { 273 return "MedicinalProductPharmaceutical.characteristics"; 274 275 } 276 277 } 278 279 @Block() 280 public static class MedicinalProductPharmaceuticalRouteOfAdministrationComponent extends BackboneElement 281 implements IBaseBackboneElement { 282 /** 283 * Coded expression for the route. 284 */ 285 @Child(name = "code", type = { 286 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 287 @Description(shortDefinition = "Coded expression for the route", formalDefinition = "Coded expression for the route.") 288 protected CodeableConcept code; 289 290 /** 291 * The first dose (dose quantity) administered in humans can be specified, for a 292 * product under investigation, using a numerical value and its unit of 293 * measurement. 294 */ 295 @Child(name = "firstDose", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 296 @Description(shortDefinition = "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement", formalDefinition = "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.") 297 protected Quantity firstDose; 298 299 /** 300 * The maximum single dose that can be administered as per the protocol of a 301 * clinical trial can be specified using a numerical value and its unit of 302 * measurement. 303 */ 304 @Child(name = "maxSingleDose", type = { 305 Quantity.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 306 @Description(shortDefinition = "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement", formalDefinition = "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.") 307 protected Quantity maxSingleDose; 308 309 /** 310 * The maximum dose per day (maximum dose quantity to be administered in any one 311 * 24-h period) that can be administered as per the protocol referenced in the 312 * clinical trial authorisation. 313 */ 314 @Child(name = "maxDosePerDay", type = { 315 Quantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 316 @Description(shortDefinition = "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.") 317 protected Quantity maxDosePerDay; 318 319 /** 320 * The maximum dose per treatment period that can be administered as per the 321 * protocol referenced in the clinical trial authorisation. 322 */ 323 @Child(name = "maxDosePerTreatmentPeriod", type = { 324 Ratio.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 325 @Description(shortDefinition = "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.") 326 protected Ratio maxDosePerTreatmentPeriod; 327 328 /** 329 * The maximum treatment period during which an Investigational Medicinal 330 * Product can be administered as per the protocol referenced in the clinical 331 * trial authorisation. 332 */ 333 @Child(name = "maxTreatmentPeriod", type = { 334 Duration.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 335 @Description(shortDefinition = "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation", formalDefinition = "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.") 336 protected Duration maxTreatmentPeriod; 337 338 /** 339 * A species for which this route applies. 340 */ 341 @Child(name = "targetSpecies", type = {}, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 342 @Description(shortDefinition = "A species for which this route applies", formalDefinition = "A species for which this route applies.") 343 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> targetSpecies; 344 345 private static final long serialVersionUID = 854394783L; 346 347 /** 348 * Constructor 349 */ 350 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent() { 351 super(); 352 } 353 354 /** 355 * Constructor 356 */ 357 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent(CodeableConcept code) { 358 super(); 359 this.code = code; 360 } 361 362 /** 363 * @return {@link #code} (Coded expression for the route.) 364 */ 365 public CodeableConcept getCode() { 366 if (this.code == null) 367 if (Configuration.errorOnAutoCreate()) 368 throw new Error("Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.code"); 369 else if (Configuration.doAutoCreate()) 370 this.code = new CodeableConcept(); // cc 371 return this.code; 372 } 373 374 public boolean hasCode() { 375 return this.code != null && !this.code.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #code} (Coded expression for the route.) 380 */ 381 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setCode(CodeableConcept value) { 382 this.code = value; 383 return this; 384 } 385 386 /** 387 * @return {@link #firstDose} (The first dose (dose quantity) administered in 388 * humans can be specified, for a product under investigation, using a 389 * numerical value and its unit of measurement.) 390 */ 391 public Quantity getFirstDose() { 392 if (this.firstDose == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error( 395 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.firstDose"); 396 else if (Configuration.doAutoCreate()) 397 this.firstDose = new Quantity(); // cc 398 return this.firstDose; 399 } 400 401 public boolean hasFirstDose() { 402 return this.firstDose != null && !this.firstDose.isEmpty(); 403 } 404 405 /** 406 * @param value {@link #firstDose} (The first dose (dose quantity) administered 407 * in humans can be specified, for a product under investigation, 408 * using a numerical value and its unit of measurement.) 409 */ 410 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setFirstDose(Quantity value) { 411 this.firstDose = value; 412 return this; 413 } 414 415 /** 416 * @return {@link #maxSingleDose} (The maximum single dose that can be 417 * administered as per the protocol of a clinical trial can be specified 418 * using a numerical value and its unit of measurement.) 419 */ 420 public Quantity getMaxSingleDose() { 421 if (this.maxSingleDose == null) 422 if (Configuration.errorOnAutoCreate()) 423 throw new Error( 424 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxSingleDose"); 425 else if (Configuration.doAutoCreate()) 426 this.maxSingleDose = new Quantity(); // cc 427 return this.maxSingleDose; 428 } 429 430 public boolean hasMaxSingleDose() { 431 return this.maxSingleDose != null && !this.maxSingleDose.isEmpty(); 432 } 433 434 /** 435 * @param value {@link #maxSingleDose} (The maximum single dose that can be 436 * administered as per the protocol of a clinical trial can be 437 * specified using a numerical value and its unit of measurement.) 438 */ 439 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxSingleDose(Quantity value) { 440 this.maxSingleDose = value; 441 return this; 442 } 443 444 /** 445 * @return {@link #maxDosePerDay} (The maximum dose per day (maximum dose 446 * quantity to be administered in any one 24-h period) that can be 447 * administered as per the protocol referenced in the clinical trial 448 * authorisation.) 449 */ 450 public Quantity getMaxDosePerDay() { 451 if (this.maxDosePerDay == null) 452 if (Configuration.errorOnAutoCreate()) 453 throw new Error( 454 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxDosePerDay"); 455 else if (Configuration.doAutoCreate()) 456 this.maxDosePerDay = new Quantity(); // cc 457 return this.maxDosePerDay; 458 } 459 460 public boolean hasMaxDosePerDay() { 461 return this.maxDosePerDay != null && !this.maxDosePerDay.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #maxDosePerDay} (The maximum dose per day (maximum dose 466 * quantity to be administered in any one 24-h period) that can be 467 * administered as per the protocol referenced in the clinical 468 * trial authorisation.) 469 */ 470 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxDosePerDay(Quantity value) { 471 this.maxDosePerDay = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #maxDosePerTreatmentPeriod} (The maximum dose per treatment 477 * period that can be administered as per the protocol referenced in the 478 * clinical trial authorisation.) 479 */ 480 public Ratio getMaxDosePerTreatmentPeriod() { 481 if (this.maxDosePerTreatmentPeriod == null) 482 if (Configuration.errorOnAutoCreate()) 483 throw new Error( 484 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxDosePerTreatmentPeriod"); 485 else if (Configuration.doAutoCreate()) 486 this.maxDosePerTreatmentPeriod = new Ratio(); // cc 487 return this.maxDosePerTreatmentPeriod; 488 } 489 490 public boolean hasMaxDosePerTreatmentPeriod() { 491 return this.maxDosePerTreatmentPeriod != null && !this.maxDosePerTreatmentPeriod.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #maxDosePerTreatmentPeriod} (The maximum dose per 496 * treatment period that can be administered as per the protocol 497 * referenced in the clinical trial authorisation.) 498 */ 499 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxDosePerTreatmentPeriod(Ratio value) { 500 this.maxDosePerTreatmentPeriod = value; 501 return this; 502 } 503 504 /** 505 * @return {@link #maxTreatmentPeriod} (The maximum treatment period during 506 * which an Investigational Medicinal Product can be administered as per 507 * the protocol referenced in the clinical trial authorisation.) 508 */ 509 public Duration getMaxTreatmentPeriod() { 510 if (this.maxTreatmentPeriod == null) 511 if (Configuration.errorOnAutoCreate()) 512 throw new Error( 513 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationComponent.maxTreatmentPeriod"); 514 else if (Configuration.doAutoCreate()) 515 this.maxTreatmentPeriod = new Duration(); // cc 516 return this.maxTreatmentPeriod; 517 } 518 519 public boolean hasMaxTreatmentPeriod() { 520 return this.maxTreatmentPeriod != null && !this.maxTreatmentPeriod.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #maxTreatmentPeriod} (The maximum treatment period during 525 * which an Investigational Medicinal Product can be administered 526 * as per the protocol referenced in the clinical trial 527 * authorisation.) 528 */ 529 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setMaxTreatmentPeriod(Duration value) { 530 this.maxTreatmentPeriod = value; 531 return this; 532 } 533 534 /** 535 * @return {@link #targetSpecies} (A species for which this route applies.) 536 */ 537 public List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> getTargetSpecies() { 538 if (this.targetSpecies == null) 539 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 540 return this.targetSpecies; 541 } 542 543 /** 544 * @return Returns a reference to <code>this</code> for easy method chaining 545 */ 546 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent setTargetSpecies( 547 List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent> theTargetSpecies) { 548 this.targetSpecies = theTargetSpecies; 549 return this; 550 } 551 552 public boolean hasTargetSpecies() { 553 if (this.targetSpecies == null) 554 return false; 555 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent item : this.targetSpecies) 556 if (!item.isEmpty()) 557 return true; 558 return false; 559 } 560 561 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent addTargetSpecies() { // 3 562 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(); 563 if (this.targetSpecies == null) 564 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 565 this.targetSpecies.add(t); 566 return t; 567 } 568 569 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent addTargetSpecies( 570 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent t) { // 3 571 if (t == null) 572 return this; 573 if (this.targetSpecies == null) 574 this.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 575 this.targetSpecies.add(t); 576 return this; 577 } 578 579 /** 580 * @return The first repetition of repeating field {@link #targetSpecies}, 581 * creating it if it does not already exist 582 */ 583 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent getTargetSpeciesFirstRep() { 584 if (getTargetSpecies().isEmpty()) { 585 addTargetSpecies(); 586 } 587 return getTargetSpecies().get(0); 588 } 589 590 protected void listChildren(List<Property> children) { 591 super.listChildren(children); 592 children.add(new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code)); 593 children.add(new Property("firstDose", "Quantity", 594 "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.", 595 0, 1, firstDose)); 596 children.add(new Property("maxSingleDose", "Quantity", 597 "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.", 598 0, 1, maxSingleDose)); 599 children.add(new Property("maxDosePerDay", "Quantity", 600 "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.", 601 0, 1, maxDosePerDay)); 602 children.add(new Property("maxDosePerTreatmentPeriod", "Ratio", 603 "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.", 604 0, 1, maxDosePerTreatmentPeriod)); 605 children.add(new Property("maxTreatmentPeriod", "Duration", 606 "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.", 607 0, 1, maxTreatmentPeriod)); 608 children.add(new Property("targetSpecies", "", "A species for which this route applies.", 0, 609 java.lang.Integer.MAX_VALUE, targetSpecies)); 610 } 611 612 @Override 613 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 614 switch (_hash) { 615 case 3059181: 616 /* code */ return new Property("code", "CodeableConcept", "Coded expression for the route.", 0, 1, code); 617 case 132551405: 618 /* firstDose */ return new Property("firstDose", "Quantity", 619 "The first dose (dose quantity) administered in humans can be specified, for a product under investigation, using a numerical value and its unit of measurement.", 620 0, 1, firstDose); 621 case -259207927: 622 /* maxSingleDose */ return new Property("maxSingleDose", "Quantity", 623 "The maximum single dose that can be administered as per the protocol of a clinical trial can be specified using a numerical value and its unit of measurement.", 624 0, 1, maxSingleDose); 625 case -2017475520: 626 /* maxDosePerDay */ return new Property("maxDosePerDay", "Quantity", 627 "The maximum dose per day (maximum dose quantity to be administered in any one 24-h period) that can be administered as per the protocol referenced in the clinical trial authorisation.", 628 0, 1, maxDosePerDay); 629 case -608040195: 630 /* maxDosePerTreatmentPeriod */ return new Property("maxDosePerTreatmentPeriod", "Ratio", 631 "The maximum dose per treatment period that can be administered as per the protocol referenced in the clinical trial authorisation.", 632 0, 1, maxDosePerTreatmentPeriod); 633 case 920698453: 634 /* maxTreatmentPeriod */ return new Property("maxTreatmentPeriod", "Duration", 635 "The maximum treatment period during which an Investigational Medicinal Product can be administered as per the protocol referenced in the clinical trial authorisation.", 636 0, 1, maxTreatmentPeriod); 637 case 295481963: 638 /* targetSpecies */ return new Property("targetSpecies", "", "A species for which this route applies.", 0, 639 java.lang.Integer.MAX_VALUE, targetSpecies); 640 default: 641 return super.getNamedProperty(_hash, _name, _checkValid); 642 } 643 644 } 645 646 @Override 647 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 648 switch (hash) { 649 case 3059181: 650 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 651 case 132551405: 652 /* firstDose */ return this.firstDose == null ? new Base[0] : new Base[] { this.firstDose }; // Quantity 653 case -259207927: 654 /* maxSingleDose */ return this.maxSingleDose == null ? new Base[0] : new Base[] { this.maxSingleDose }; // Quantity 655 case -2017475520: 656 /* maxDosePerDay */ return this.maxDosePerDay == null ? new Base[0] : new Base[] { this.maxDosePerDay }; // Quantity 657 case -608040195: 658 /* maxDosePerTreatmentPeriod */ return this.maxDosePerTreatmentPeriod == null ? new Base[0] 659 : new Base[] { this.maxDosePerTreatmentPeriod }; // Ratio 660 case 920698453: 661 /* maxTreatmentPeriod */ return this.maxTreatmentPeriod == null ? new Base[0] 662 : new Base[] { this.maxTreatmentPeriod }; // Duration 663 case 295481963: 664 /* targetSpecies */ return this.targetSpecies == null ? new Base[0] 665 : this.targetSpecies.toArray(new Base[this.targetSpecies.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent 666 default: 667 return super.getProperty(hash, name, checkValid); 668 } 669 670 } 671 672 @Override 673 public Base setProperty(int hash, String name, Base value) throws FHIRException { 674 switch (hash) { 675 case 3059181: // code 676 this.code = castToCodeableConcept(value); // CodeableConcept 677 return value; 678 case 132551405: // firstDose 679 this.firstDose = castToQuantity(value); // Quantity 680 return value; 681 case -259207927: // maxSingleDose 682 this.maxSingleDose = castToQuantity(value); // Quantity 683 return value; 684 case -2017475520: // maxDosePerDay 685 this.maxDosePerDay = castToQuantity(value); // Quantity 686 return value; 687 case -608040195: // maxDosePerTreatmentPeriod 688 this.maxDosePerTreatmentPeriod = castToRatio(value); // Ratio 689 return value; 690 case 920698453: // maxTreatmentPeriod 691 this.maxTreatmentPeriod = castToDuration(value); // Duration 692 return value; 693 case 295481963: // targetSpecies 694 this.getTargetSpecies().add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent 695 return value; 696 default: 697 return super.setProperty(hash, name, value); 698 } 699 700 } 701 702 @Override 703 public Base setProperty(String name, Base value) throws FHIRException { 704 if (name.equals("code")) { 705 this.code = castToCodeableConcept(value); // CodeableConcept 706 } else if (name.equals("firstDose")) { 707 this.firstDose = castToQuantity(value); // Quantity 708 } else if (name.equals("maxSingleDose")) { 709 this.maxSingleDose = castToQuantity(value); // Quantity 710 } else if (name.equals("maxDosePerDay")) { 711 this.maxDosePerDay = castToQuantity(value); // Quantity 712 } else if (name.equals("maxDosePerTreatmentPeriod")) { 713 this.maxDosePerTreatmentPeriod = castToRatio(value); // Ratio 714 } else if (name.equals("maxTreatmentPeriod")) { 715 this.maxTreatmentPeriod = castToDuration(value); // Duration 716 } else if (name.equals("targetSpecies")) { 717 this.getTargetSpecies().add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) value); 718 } else 719 return super.setProperty(name, value); 720 return value; 721 } 722 723 @Override 724 public Base makeProperty(int hash, String name) throws FHIRException { 725 switch (hash) { 726 case 3059181: 727 return getCode(); 728 case 132551405: 729 return getFirstDose(); 730 case -259207927: 731 return getMaxSingleDose(); 732 case -2017475520: 733 return getMaxDosePerDay(); 734 case -608040195: 735 return getMaxDosePerTreatmentPeriod(); 736 case 920698453: 737 return getMaxTreatmentPeriod(); 738 case 295481963: 739 return addTargetSpecies(); 740 default: 741 return super.makeProperty(hash, name); 742 } 743 744 } 745 746 @Override 747 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 748 switch (hash) { 749 case 3059181: 750 /* code */ return new String[] { "CodeableConcept" }; 751 case 132551405: 752 /* firstDose */ return new String[] { "Quantity" }; 753 case -259207927: 754 /* maxSingleDose */ return new String[] { "Quantity" }; 755 case -2017475520: 756 /* maxDosePerDay */ return new String[] { "Quantity" }; 757 case -608040195: 758 /* maxDosePerTreatmentPeriod */ return new String[] { "Ratio" }; 759 case 920698453: 760 /* maxTreatmentPeriod */ return new String[] { "Duration" }; 761 case 295481963: 762 /* targetSpecies */ return new String[] {}; 763 default: 764 return super.getTypesForProperty(hash, name); 765 } 766 767 } 768 769 @Override 770 public Base addChild(String name) throws FHIRException { 771 if (name.equals("code")) { 772 this.code = new CodeableConcept(); 773 return this.code; 774 } else if (name.equals("firstDose")) { 775 this.firstDose = new Quantity(); 776 return this.firstDose; 777 } else if (name.equals("maxSingleDose")) { 778 this.maxSingleDose = new Quantity(); 779 return this.maxSingleDose; 780 } else if (name.equals("maxDosePerDay")) { 781 this.maxDosePerDay = new Quantity(); 782 return this.maxDosePerDay; 783 } else if (name.equals("maxDosePerTreatmentPeriod")) { 784 this.maxDosePerTreatmentPeriod = new Ratio(); 785 return this.maxDosePerTreatmentPeriod; 786 } else if (name.equals("maxTreatmentPeriod")) { 787 this.maxTreatmentPeriod = new Duration(); 788 return this.maxTreatmentPeriod; 789 } else if (name.equals("targetSpecies")) { 790 return addTargetSpecies(); 791 } else 792 return super.addChild(name); 793 } 794 795 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent copy() { 796 MedicinalProductPharmaceuticalRouteOfAdministrationComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationComponent(); 797 copyValues(dst); 798 return dst; 799 } 800 801 public void copyValues(MedicinalProductPharmaceuticalRouteOfAdministrationComponent dst) { 802 super.copyValues(dst); 803 dst.code = code == null ? null : code.copy(); 804 dst.firstDose = firstDose == null ? null : firstDose.copy(); 805 dst.maxSingleDose = maxSingleDose == null ? null : maxSingleDose.copy(); 806 dst.maxDosePerDay = maxDosePerDay == null ? null : maxDosePerDay.copy(); 807 dst.maxDosePerTreatmentPeriod = maxDosePerTreatmentPeriod == null ? null : maxDosePerTreatmentPeriod.copy(); 808 dst.maxTreatmentPeriod = maxTreatmentPeriod == null ? null : maxTreatmentPeriod.copy(); 809 if (targetSpecies != null) { 810 dst.targetSpecies = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent>(); 811 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent i : targetSpecies) 812 dst.targetSpecies.add(i.copy()); 813 } 814 ; 815 } 816 817 @Override 818 public boolean equalsDeep(Base other_) { 819 if (!super.equalsDeep(other_)) 820 return false; 821 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationComponent)) 822 return false; 823 MedicinalProductPharmaceuticalRouteOfAdministrationComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationComponent) other_; 824 return compareDeep(code, o.code, true) && compareDeep(firstDose, o.firstDose, true) 825 && compareDeep(maxSingleDose, o.maxSingleDose, true) && compareDeep(maxDosePerDay, o.maxDosePerDay, true) 826 && compareDeep(maxDosePerTreatmentPeriod, o.maxDosePerTreatmentPeriod, true) 827 && compareDeep(maxTreatmentPeriod, o.maxTreatmentPeriod, true) 828 && compareDeep(targetSpecies, o.targetSpecies, true); 829 } 830 831 @Override 832 public boolean equalsShallow(Base other_) { 833 if (!super.equalsShallow(other_)) 834 return false; 835 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationComponent)) 836 return false; 837 MedicinalProductPharmaceuticalRouteOfAdministrationComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationComponent) other_; 838 return true; 839 } 840 841 public boolean isEmpty() { 842 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, firstDose, maxSingleDose, maxDosePerDay, 843 maxDosePerTreatmentPeriod, maxTreatmentPeriod, targetSpecies); 844 } 845 846 public String fhirType() { 847 return "MedicinalProductPharmaceutical.routeOfAdministration"; 848 849 } 850 851 } 852 853 @Block() 854 public static class MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent extends BackboneElement 855 implements IBaseBackboneElement { 856 /** 857 * Coded expression for the species. 858 */ 859 @Child(name = "code", type = { 860 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 861 @Description(shortDefinition = "Coded expression for the species", formalDefinition = "Coded expression for the species.") 862 protected CodeableConcept code; 863 864 /** 865 * A species specific time during which consumption of animal product is not 866 * appropriate. 867 */ 868 @Child(name = "withdrawalPeriod", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 869 @Description(shortDefinition = "A species specific time during which consumption of animal product is not appropriate", formalDefinition = "A species specific time during which consumption of animal product is not appropriate.") 870 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> withdrawalPeriod; 871 872 private static final long serialVersionUID = -664052812L; 873 874 /** 875 * Constructor 876 */ 877 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent() { 878 super(); 879 } 880 881 /** 882 * Constructor 883 */ 884 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(CodeableConcept code) { 885 super(); 886 this.code = code; 887 } 888 889 /** 890 * @return {@link #code} (Coded expression for the species.) 891 */ 892 public CodeableConcept getCode() { 893 if (this.code == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error( 896 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent.code"); 897 else if (Configuration.doAutoCreate()) 898 this.code = new CodeableConcept(); // cc 899 return this.code; 900 } 901 902 public boolean hasCode() { 903 return this.code != null && !this.code.isEmpty(); 904 } 905 906 /** 907 * @param value {@link #code} (Coded expression for the species.) 908 */ 909 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent setCode(CodeableConcept value) { 910 this.code = value; 911 return this; 912 } 913 914 /** 915 * @return {@link #withdrawalPeriod} (A species specific time during which 916 * consumption of animal product is not appropriate.) 917 */ 918 public List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> getWithdrawalPeriod() { 919 if (this.withdrawalPeriod == null) 920 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 921 return this.withdrawalPeriod; 922 } 923 924 /** 925 * @return Returns a reference to <code>this</code> for easy method chaining 926 */ 927 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent setWithdrawalPeriod( 928 List<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent> theWithdrawalPeriod) { 929 this.withdrawalPeriod = theWithdrawalPeriod; 930 return this; 931 } 932 933 public boolean hasWithdrawalPeriod() { 934 if (this.withdrawalPeriod == null) 935 return false; 936 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent item : this.withdrawalPeriod) 937 if (!item.isEmpty()) 938 return true; 939 return false; 940 } 941 942 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent addWithdrawalPeriod() { // 3 943 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 944 if (this.withdrawalPeriod == null) 945 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 946 this.withdrawalPeriod.add(t); 947 return t; 948 } 949 950 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent addWithdrawalPeriod( 951 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent t) { // 3 952 if (t == null) 953 return this; 954 if (this.withdrawalPeriod == null) 955 this.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 956 this.withdrawalPeriod.add(t); 957 return this; 958 } 959 960 /** 961 * @return The first repetition of repeating field {@link #withdrawalPeriod}, 962 * creating it if it does not already exist 963 */ 964 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent getWithdrawalPeriodFirstRep() { 965 if (getWithdrawalPeriod().isEmpty()) { 966 addWithdrawalPeriod(); 967 } 968 return getWithdrawalPeriod().get(0); 969 } 970 971 protected void listChildren(List<Property> children) { 972 super.listChildren(children); 973 children.add(new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code)); 974 children.add(new Property("withdrawalPeriod", "", 975 "A species specific time during which consumption of animal product is not appropriate.", 0, 976 java.lang.Integer.MAX_VALUE, withdrawalPeriod)); 977 } 978 979 @Override 980 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 981 switch (_hash) { 982 case 3059181: 983 /* code */ return new Property("code", "CodeableConcept", "Coded expression for the species.", 0, 1, code); 984 case -98450730: 985 /* withdrawalPeriod */ return new Property("withdrawalPeriod", "", 986 "A species specific time during which consumption of animal product is not appropriate.", 0, 987 java.lang.Integer.MAX_VALUE, withdrawalPeriod); 988 default: 989 return super.getNamedProperty(_hash, _name, _checkValid); 990 } 991 992 } 993 994 @Override 995 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 996 switch (hash) { 997 case 3059181: 998 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 999 case -98450730: 1000 /* withdrawalPeriod */ return this.withdrawalPeriod == null ? new Base[0] 1001 : this.withdrawalPeriod.toArray(new Base[this.withdrawalPeriod.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1002 default: 1003 return super.getProperty(hash, name, checkValid); 1004 } 1005 1006 } 1007 1008 @Override 1009 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1010 switch (hash) { 1011 case 3059181: // code 1012 this.code = castToCodeableConcept(value); // CodeableConcept 1013 return value; 1014 case -98450730: // withdrawalPeriod 1015 this.getWithdrawalPeriod() 1016 .add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1017 return value; 1018 default: 1019 return super.setProperty(hash, name, value); 1020 } 1021 1022 } 1023 1024 @Override 1025 public Base setProperty(String name, Base value) throws FHIRException { 1026 if (name.equals("code")) { 1027 this.code = castToCodeableConcept(value); // CodeableConcept 1028 } else if (name.equals("withdrawalPeriod")) { 1029 this.getWithdrawalPeriod() 1030 .add((MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) value); 1031 } else 1032 return super.setProperty(name, value); 1033 return value; 1034 } 1035 1036 @Override 1037 public Base makeProperty(int hash, String name) throws FHIRException { 1038 switch (hash) { 1039 case 3059181: 1040 return getCode(); 1041 case -98450730: 1042 return addWithdrawalPeriod(); 1043 default: 1044 return super.makeProperty(hash, name); 1045 } 1046 1047 } 1048 1049 @Override 1050 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1051 switch (hash) { 1052 case 3059181: 1053 /* code */ return new String[] { "CodeableConcept" }; 1054 case -98450730: 1055 /* withdrawalPeriod */ return new String[] {}; 1056 default: 1057 return super.getTypesForProperty(hash, name); 1058 } 1059 1060 } 1061 1062 @Override 1063 public Base addChild(String name) throws FHIRException { 1064 if (name.equals("code")) { 1065 this.code = new CodeableConcept(); 1066 return this.code; 1067 } else if (name.equals("withdrawalPeriod")) { 1068 return addWithdrawalPeriod(); 1069 } else 1070 return super.addChild(name); 1071 } 1072 1073 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent copy() { 1074 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent(); 1075 copyValues(dst); 1076 return dst; 1077 } 1078 1079 public void copyValues(MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent dst) { 1080 super.copyValues(dst); 1081 dst.code = code == null ? null : code.copy(); 1082 if (withdrawalPeriod != null) { 1083 dst.withdrawalPeriod = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent>(); 1084 for (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent i : withdrawalPeriod) 1085 dst.withdrawalPeriod.add(i.copy()); 1086 } 1087 ; 1088 } 1089 1090 @Override 1091 public boolean equalsDeep(Base other_) { 1092 if (!super.equalsDeep(other_)) 1093 return false; 1094 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent)) 1095 return false; 1096 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) other_; 1097 return compareDeep(code, o.code, true) && compareDeep(withdrawalPeriod, o.withdrawalPeriod, true); 1098 } 1099 1100 @Override 1101 public boolean equalsShallow(Base other_) { 1102 if (!super.equalsShallow(other_)) 1103 return false; 1104 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent)) 1105 return false; 1106 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesComponent) other_; 1107 return true; 1108 } 1109 1110 public boolean isEmpty() { 1111 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, withdrawalPeriod); 1112 } 1113 1114 public String fhirType() { 1115 return "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies"; 1116 1117 } 1118 1119 } 1120 1121 @Block() 1122 public static class MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent 1123 extends BackboneElement implements IBaseBackboneElement { 1124 /** 1125 * Coded expression for the type of tissue for which the withdrawal period 1126 * applues, e.g. meat, milk. 1127 */ 1128 @Child(name = "tissue", type = { 1129 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1130 @Description(shortDefinition = "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk", formalDefinition = "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.") 1131 protected CodeableConcept tissue; 1132 1133 /** 1134 * A value for the time. 1135 */ 1136 @Child(name = "value", type = { Quantity.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1137 @Description(shortDefinition = "A value for the time", formalDefinition = "A value for the time.") 1138 protected Quantity value; 1139 1140 /** 1141 * Extra information about the withdrawal period. 1142 */ 1143 @Child(name = "supportingInformation", type = { 1144 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1145 @Description(shortDefinition = "Extra information about the withdrawal period", formalDefinition = "Extra information about the withdrawal period.") 1146 protected StringType supportingInformation; 1147 1148 private static final long serialVersionUID = -1113691238L; 1149 1150 /** 1151 * Constructor 1152 */ 1153 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent() { 1154 super(); 1155 } 1156 1157 /** 1158 * Constructor 1159 */ 1160 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent( 1161 CodeableConcept tissue, Quantity value) { 1162 super(); 1163 this.tissue = tissue; 1164 this.value = value; 1165 } 1166 1167 /** 1168 * @return {@link #tissue} (Coded expression for the type of tissue for which 1169 * the withdrawal period applues, e.g. meat, milk.) 1170 */ 1171 public CodeableConcept getTissue() { 1172 if (this.tissue == null) 1173 if (Configuration.errorOnAutoCreate()) 1174 throw new Error( 1175 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.tissue"); 1176 else if (Configuration.doAutoCreate()) 1177 this.tissue = new CodeableConcept(); // cc 1178 return this.tissue; 1179 } 1180 1181 public boolean hasTissue() { 1182 return this.tissue != null && !this.tissue.isEmpty(); 1183 } 1184 1185 /** 1186 * @param value {@link #tissue} (Coded expression for the type of tissue for 1187 * which the withdrawal period applues, e.g. meat, milk.) 1188 */ 1189 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setTissue( 1190 CodeableConcept value) { 1191 this.tissue = value; 1192 return this; 1193 } 1194 1195 /** 1196 * @return {@link #value} (A value for the time.) 1197 */ 1198 public Quantity getValue() { 1199 if (this.value == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error( 1202 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.value"); 1203 else if (Configuration.doAutoCreate()) 1204 this.value = new Quantity(); // cc 1205 return this.value; 1206 } 1207 1208 public boolean hasValue() { 1209 return this.value != null && !this.value.isEmpty(); 1210 } 1211 1212 /** 1213 * @param value {@link #value} (A value for the time.) 1214 */ 1215 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setValue( 1216 Quantity value) { 1217 this.value = value; 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #supportingInformation} (Extra information about the 1223 * withdrawal period.). This is the underlying object with id, value and 1224 * extensions. The accessor "getSupportingInformation" gives direct 1225 * access to the value 1226 */ 1227 public StringType getSupportingInformationElement() { 1228 if (this.supportingInformation == null) 1229 if (Configuration.errorOnAutoCreate()) 1230 throw new Error( 1231 "Attempt to auto-create MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent.supportingInformation"); 1232 else if (Configuration.doAutoCreate()) 1233 this.supportingInformation = new StringType(); // bb 1234 return this.supportingInformation; 1235 } 1236 1237 public boolean hasSupportingInformationElement() { 1238 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1239 } 1240 1241 public boolean hasSupportingInformation() { 1242 return this.supportingInformation != null && !this.supportingInformation.isEmpty(); 1243 } 1244 1245 /** 1246 * @param value {@link #supportingInformation} (Extra information about the 1247 * withdrawal period.). This is the underlying object with id, 1248 * value and extensions. The accessor "getSupportingInformation" 1249 * gives direct access to the value 1250 */ 1251 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformationElement( 1252 StringType value) { 1253 this.supportingInformation = value; 1254 return this; 1255 } 1256 1257 /** 1258 * @return Extra information about the withdrawal period. 1259 */ 1260 public String getSupportingInformation() { 1261 return this.supportingInformation == null ? null : this.supportingInformation.getValue(); 1262 } 1263 1264 /** 1265 * @param value Extra information about the withdrawal period. 1266 */ 1267 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent setSupportingInformation( 1268 String value) { 1269 if (Utilities.noString(value)) 1270 this.supportingInformation = null; 1271 else { 1272 if (this.supportingInformation == null) 1273 this.supportingInformation = new StringType(); 1274 this.supportingInformation.setValue(value); 1275 } 1276 return this; 1277 } 1278 1279 protected void listChildren(List<Property> children) { 1280 super.listChildren(children); 1281 children.add(new Property("tissue", "CodeableConcept", 1282 "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.", 0, 1, 1283 tissue)); 1284 children.add(new Property("value", "Quantity", "A value for the time.", 0, 1, value)); 1285 children.add(new Property("supportingInformation", "string", "Extra information about the withdrawal period.", 0, 1286 1, supportingInformation)); 1287 } 1288 1289 @Override 1290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1291 switch (_hash) { 1292 case -873475867: 1293 /* tissue */ return new Property("tissue", "CodeableConcept", 1294 "Coded expression for the type of tissue for which the withdrawal period applues, e.g. meat, milk.", 0, 1, 1295 tissue); 1296 case 111972721: 1297 /* value */ return new Property("value", "Quantity", "A value for the time.", 0, 1, value); 1298 case -1248768647: 1299 /* supportingInformation */ return new Property("supportingInformation", "string", 1300 "Extra information about the withdrawal period.", 0, 1, supportingInformation); 1301 default: 1302 return super.getNamedProperty(_hash, _name, _checkValid); 1303 } 1304 1305 } 1306 1307 @Override 1308 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1309 switch (hash) { 1310 case -873475867: 1311 /* tissue */ return this.tissue == null ? new Base[0] : new Base[] { this.tissue }; // CodeableConcept 1312 case 111972721: 1313 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Quantity 1314 case -1248768647: 1315 /* supportingInformation */ return this.supportingInformation == null ? new Base[0] 1316 : new Base[] { this.supportingInformation }; // StringType 1317 default: 1318 return super.getProperty(hash, name, checkValid); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1325 switch (hash) { 1326 case -873475867: // tissue 1327 this.tissue = castToCodeableConcept(value); // CodeableConcept 1328 return value; 1329 case 111972721: // value 1330 this.value = castToQuantity(value); // Quantity 1331 return value; 1332 case -1248768647: // supportingInformation 1333 this.supportingInformation = castToString(value); // StringType 1334 return value; 1335 default: 1336 return super.setProperty(hash, name, value); 1337 } 1338 1339 } 1340 1341 @Override 1342 public Base setProperty(String name, Base value) throws FHIRException { 1343 if (name.equals("tissue")) { 1344 this.tissue = castToCodeableConcept(value); // CodeableConcept 1345 } else if (name.equals("value")) { 1346 this.value = castToQuantity(value); // Quantity 1347 } else if (name.equals("supportingInformation")) { 1348 this.supportingInformation = castToString(value); // StringType 1349 } else 1350 return super.setProperty(name, value); 1351 return value; 1352 } 1353 1354 @Override 1355 public Base makeProperty(int hash, String name) throws FHIRException { 1356 switch (hash) { 1357 case -873475867: 1358 return getTissue(); 1359 case 111972721: 1360 return getValue(); 1361 case -1248768647: 1362 return getSupportingInformationElement(); 1363 default: 1364 return super.makeProperty(hash, name); 1365 } 1366 1367 } 1368 1369 @Override 1370 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1371 switch (hash) { 1372 case -873475867: 1373 /* tissue */ return new String[] { "CodeableConcept" }; 1374 case 111972721: 1375 /* value */ return new String[] { "Quantity" }; 1376 case -1248768647: 1377 /* supportingInformation */ return new String[] { "string" }; 1378 default: 1379 return super.getTypesForProperty(hash, name); 1380 } 1381 1382 } 1383 1384 @Override 1385 public Base addChild(String name) throws FHIRException { 1386 if (name.equals("tissue")) { 1387 this.tissue = new CodeableConcept(); 1388 return this.tissue; 1389 } else if (name.equals("value")) { 1390 this.value = new Quantity(); 1391 return this.value; 1392 } else if (name.equals("supportingInformation")) { 1393 throw new FHIRException( 1394 "Cannot call addChild on a singleton property MedicinalProductPharmaceutical.supportingInformation"); 1395 } else 1396 return super.addChild(name); 1397 } 1398 1399 public MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent copy() { 1400 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst = new MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent(); 1401 copyValues(dst); 1402 return dst; 1403 } 1404 1405 public void copyValues( 1406 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent dst) { 1407 super.copyValues(dst); 1408 dst.tissue = tissue == null ? null : tissue.copy(); 1409 dst.value = value == null ? null : value.copy(); 1410 dst.supportingInformation = supportingInformation == null ? null : supportingInformation.copy(); 1411 } 1412 1413 @Override 1414 public boolean equalsDeep(Base other_) { 1415 if (!super.equalsDeep(other_)) 1416 return false; 1417 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1418 return false; 1419 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1420 return compareDeep(tissue, o.tissue, true) && compareDeep(value, o.value, true) 1421 && compareDeep(supportingInformation, o.supportingInformation, true); 1422 } 1423 1424 @Override 1425 public boolean equalsShallow(Base other_) { 1426 if (!super.equalsShallow(other_)) 1427 return false; 1428 if (!(other_ instanceof MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent)) 1429 return false; 1430 MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent o = (MedicinalProductPharmaceuticalRouteOfAdministrationTargetSpeciesWithdrawalPeriodComponent) other_; 1431 return compareValues(supportingInformation, o.supportingInformation, true); 1432 } 1433 1434 public boolean isEmpty() { 1435 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(tissue, value, supportingInformation); 1436 } 1437 1438 public String fhirType() { 1439 return "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.withdrawalPeriod"; 1440 1441 } 1442 1443 } 1444 1445 /** 1446 * An identifier for the pharmaceutical medicinal product. 1447 */ 1448 @Child(name = "identifier", type = { 1449 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1450 @Description(shortDefinition = "An identifier for the pharmaceutical medicinal product", formalDefinition = "An identifier for the pharmaceutical medicinal product.") 1451 protected List<Identifier> identifier; 1452 1453 /** 1454 * The administrable dose form, after necessary reconstitution. 1455 */ 1456 @Child(name = "administrableDoseForm", type = { 1457 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1458 @Description(shortDefinition = "The administrable dose form, after necessary reconstitution", formalDefinition = "The administrable dose form, after necessary reconstitution.") 1459 protected CodeableConcept administrableDoseForm; 1460 1461 /** 1462 * Todo. 1463 */ 1464 @Child(name = "unitOfPresentation", type = { 1465 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1466 @Description(shortDefinition = "Todo", formalDefinition = "Todo.") 1467 protected CodeableConcept unitOfPresentation; 1468 1469 /** 1470 * Ingredient. 1471 */ 1472 @Child(name = "ingredient", type = { 1473 MedicinalProductIngredient.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1474 @Description(shortDefinition = "Ingredient", formalDefinition = "Ingredient.") 1475 protected List<Reference> ingredient; 1476 /** 1477 * The actual objects that are the target of the reference (Ingredient.) 1478 */ 1479 protected List<MedicinalProductIngredient> ingredientTarget; 1480 1481 /** 1482 * Accompanying device. 1483 */ 1484 @Child(name = "device", type = { 1485 DeviceDefinition.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1486 @Description(shortDefinition = "Accompanying device", formalDefinition = "Accompanying device.") 1487 protected List<Reference> device; 1488 /** 1489 * The actual objects that are the target of the reference (Accompanying 1490 * device.) 1491 */ 1492 protected List<DeviceDefinition> deviceTarget; 1493 1494 /** 1495 * Characteristics e.g. a products onset of action. 1496 */ 1497 @Child(name = "characteristics", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1498 @Description(shortDefinition = "Characteristics e.g. a products onset of action", formalDefinition = "Characteristics e.g. a products onset of action.") 1499 protected List<MedicinalProductPharmaceuticalCharacteristicsComponent> characteristics; 1500 1501 /** 1502 * The path by which the pharmaceutical product is taken into or makes contact 1503 * with the body. 1504 */ 1505 @Child(name = "routeOfAdministration", type = {}, order = 6, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1506 @Description(shortDefinition = "The path by which the pharmaceutical product is taken into or makes contact with the body", formalDefinition = "The path by which the pharmaceutical product is taken into or makes contact with the body.") 1507 protected List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> routeOfAdministration; 1508 1509 private static final long serialVersionUID = -1201548050L; 1510 1511 /** 1512 * Constructor 1513 */ 1514 public MedicinalProductPharmaceutical() { 1515 super(); 1516 } 1517 1518 /** 1519 * Constructor 1520 */ 1521 public MedicinalProductPharmaceutical(CodeableConcept administrableDoseForm) { 1522 super(); 1523 this.administrableDoseForm = administrableDoseForm; 1524 } 1525 1526 /** 1527 * @return {@link #identifier} (An identifier for the pharmaceutical medicinal 1528 * product.) 1529 */ 1530 public List<Identifier> getIdentifier() { 1531 if (this.identifier == null) 1532 this.identifier = new ArrayList<Identifier>(); 1533 return this.identifier; 1534 } 1535 1536 /** 1537 * @return Returns a reference to <code>this</code> for easy method chaining 1538 */ 1539 public MedicinalProductPharmaceutical setIdentifier(List<Identifier> theIdentifier) { 1540 this.identifier = theIdentifier; 1541 return this; 1542 } 1543 1544 public boolean hasIdentifier() { 1545 if (this.identifier == null) 1546 return false; 1547 for (Identifier item : this.identifier) 1548 if (!item.isEmpty()) 1549 return true; 1550 return false; 1551 } 1552 1553 public Identifier addIdentifier() { // 3 1554 Identifier t = new Identifier(); 1555 if (this.identifier == null) 1556 this.identifier = new ArrayList<Identifier>(); 1557 this.identifier.add(t); 1558 return t; 1559 } 1560 1561 public MedicinalProductPharmaceutical addIdentifier(Identifier t) { // 3 1562 if (t == null) 1563 return this; 1564 if (this.identifier == null) 1565 this.identifier = new ArrayList<Identifier>(); 1566 this.identifier.add(t); 1567 return this; 1568 } 1569 1570 /** 1571 * @return The first repetition of repeating field {@link #identifier}, creating 1572 * it if it does not already exist 1573 */ 1574 public Identifier getIdentifierFirstRep() { 1575 if (getIdentifier().isEmpty()) { 1576 addIdentifier(); 1577 } 1578 return getIdentifier().get(0); 1579 } 1580 1581 /** 1582 * @return {@link #administrableDoseForm} (The administrable dose form, after 1583 * necessary reconstitution.) 1584 */ 1585 public CodeableConcept getAdministrableDoseForm() { 1586 if (this.administrableDoseForm == null) 1587 if (Configuration.errorOnAutoCreate()) 1588 throw new Error("Attempt to auto-create MedicinalProductPharmaceutical.administrableDoseForm"); 1589 else if (Configuration.doAutoCreate()) 1590 this.administrableDoseForm = new CodeableConcept(); // cc 1591 return this.administrableDoseForm; 1592 } 1593 1594 public boolean hasAdministrableDoseForm() { 1595 return this.administrableDoseForm != null && !this.administrableDoseForm.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #administrableDoseForm} (The administrable dose form, 1600 * after necessary reconstitution.) 1601 */ 1602 public MedicinalProductPharmaceutical setAdministrableDoseForm(CodeableConcept value) { 1603 this.administrableDoseForm = value; 1604 return this; 1605 } 1606 1607 /** 1608 * @return {@link #unitOfPresentation} (Todo.) 1609 */ 1610 public CodeableConcept getUnitOfPresentation() { 1611 if (this.unitOfPresentation == null) 1612 if (Configuration.errorOnAutoCreate()) 1613 throw new Error("Attempt to auto-create MedicinalProductPharmaceutical.unitOfPresentation"); 1614 else if (Configuration.doAutoCreate()) 1615 this.unitOfPresentation = new CodeableConcept(); // cc 1616 return this.unitOfPresentation; 1617 } 1618 1619 public boolean hasUnitOfPresentation() { 1620 return this.unitOfPresentation != null && !this.unitOfPresentation.isEmpty(); 1621 } 1622 1623 /** 1624 * @param value {@link #unitOfPresentation} (Todo.) 1625 */ 1626 public MedicinalProductPharmaceutical setUnitOfPresentation(CodeableConcept value) { 1627 this.unitOfPresentation = value; 1628 return this; 1629 } 1630 1631 /** 1632 * @return {@link #ingredient} (Ingredient.) 1633 */ 1634 public List<Reference> getIngredient() { 1635 if (this.ingredient == null) 1636 this.ingredient = new ArrayList<Reference>(); 1637 return this.ingredient; 1638 } 1639 1640 /** 1641 * @return Returns a reference to <code>this</code> for easy method chaining 1642 */ 1643 public MedicinalProductPharmaceutical setIngredient(List<Reference> theIngredient) { 1644 this.ingredient = theIngredient; 1645 return this; 1646 } 1647 1648 public boolean hasIngredient() { 1649 if (this.ingredient == null) 1650 return false; 1651 for (Reference item : this.ingredient) 1652 if (!item.isEmpty()) 1653 return true; 1654 return false; 1655 } 1656 1657 public Reference addIngredient() { // 3 1658 Reference t = new Reference(); 1659 if (this.ingredient == null) 1660 this.ingredient = new ArrayList<Reference>(); 1661 this.ingredient.add(t); 1662 return t; 1663 } 1664 1665 public MedicinalProductPharmaceutical addIngredient(Reference t) { // 3 1666 if (t == null) 1667 return this; 1668 if (this.ingredient == null) 1669 this.ingredient = new ArrayList<Reference>(); 1670 this.ingredient.add(t); 1671 return this; 1672 } 1673 1674 /** 1675 * @return The first repetition of repeating field {@link #ingredient}, creating 1676 * it if it does not already exist 1677 */ 1678 public Reference getIngredientFirstRep() { 1679 if (getIngredient().isEmpty()) { 1680 addIngredient(); 1681 } 1682 return getIngredient().get(0); 1683 } 1684 1685 /** 1686 * @deprecated Use Reference#setResource(IBaseResource) instead 1687 */ 1688 @Deprecated 1689 public List<MedicinalProductIngredient> getIngredientTarget() { 1690 if (this.ingredientTarget == null) 1691 this.ingredientTarget = new ArrayList<MedicinalProductIngredient>(); 1692 return this.ingredientTarget; 1693 } 1694 1695 /** 1696 * @deprecated Use Reference#setResource(IBaseResource) instead 1697 */ 1698 @Deprecated 1699 public MedicinalProductIngredient addIngredientTarget() { 1700 MedicinalProductIngredient r = new MedicinalProductIngredient(); 1701 if (this.ingredientTarget == null) 1702 this.ingredientTarget = new ArrayList<MedicinalProductIngredient>(); 1703 this.ingredientTarget.add(r); 1704 return r; 1705 } 1706 1707 /** 1708 * @return {@link #device} (Accompanying device.) 1709 */ 1710 public List<Reference> getDevice() { 1711 if (this.device == null) 1712 this.device = new ArrayList<Reference>(); 1713 return this.device; 1714 } 1715 1716 /** 1717 * @return Returns a reference to <code>this</code> for easy method chaining 1718 */ 1719 public MedicinalProductPharmaceutical setDevice(List<Reference> theDevice) { 1720 this.device = theDevice; 1721 return this; 1722 } 1723 1724 public boolean hasDevice() { 1725 if (this.device == null) 1726 return false; 1727 for (Reference item : this.device) 1728 if (!item.isEmpty()) 1729 return true; 1730 return false; 1731 } 1732 1733 public Reference addDevice() { // 3 1734 Reference t = new Reference(); 1735 if (this.device == null) 1736 this.device = new ArrayList<Reference>(); 1737 this.device.add(t); 1738 return t; 1739 } 1740 1741 public MedicinalProductPharmaceutical addDevice(Reference t) { // 3 1742 if (t == null) 1743 return this; 1744 if (this.device == null) 1745 this.device = new ArrayList<Reference>(); 1746 this.device.add(t); 1747 return this; 1748 } 1749 1750 /** 1751 * @return The first repetition of repeating field {@link #device}, creating it 1752 * if it does not already exist 1753 */ 1754 public Reference getDeviceFirstRep() { 1755 if (getDevice().isEmpty()) { 1756 addDevice(); 1757 } 1758 return getDevice().get(0); 1759 } 1760 1761 /** 1762 * @deprecated Use Reference#setResource(IBaseResource) instead 1763 */ 1764 @Deprecated 1765 public List<DeviceDefinition> getDeviceTarget() { 1766 if (this.deviceTarget == null) 1767 this.deviceTarget = new ArrayList<DeviceDefinition>(); 1768 return this.deviceTarget; 1769 } 1770 1771 /** 1772 * @deprecated Use Reference#setResource(IBaseResource) instead 1773 */ 1774 @Deprecated 1775 public DeviceDefinition addDeviceTarget() { 1776 DeviceDefinition r = new DeviceDefinition(); 1777 if (this.deviceTarget == null) 1778 this.deviceTarget = new ArrayList<DeviceDefinition>(); 1779 this.deviceTarget.add(r); 1780 return r; 1781 } 1782 1783 /** 1784 * @return {@link #characteristics} (Characteristics e.g. a products onset of 1785 * action.) 1786 */ 1787 public List<MedicinalProductPharmaceuticalCharacteristicsComponent> getCharacteristics() { 1788 if (this.characteristics == null) 1789 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1790 return this.characteristics; 1791 } 1792 1793 /** 1794 * @return Returns a reference to <code>this</code> for easy method chaining 1795 */ 1796 public MedicinalProductPharmaceutical setCharacteristics( 1797 List<MedicinalProductPharmaceuticalCharacteristicsComponent> theCharacteristics) { 1798 this.characteristics = theCharacteristics; 1799 return this; 1800 } 1801 1802 public boolean hasCharacteristics() { 1803 if (this.characteristics == null) 1804 return false; 1805 for (MedicinalProductPharmaceuticalCharacteristicsComponent item : this.characteristics) 1806 if (!item.isEmpty()) 1807 return true; 1808 return false; 1809 } 1810 1811 public MedicinalProductPharmaceuticalCharacteristicsComponent addCharacteristics() { // 3 1812 MedicinalProductPharmaceuticalCharacteristicsComponent t = new MedicinalProductPharmaceuticalCharacteristicsComponent(); 1813 if (this.characteristics == null) 1814 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1815 this.characteristics.add(t); 1816 return t; 1817 } 1818 1819 public MedicinalProductPharmaceutical addCharacteristics(MedicinalProductPharmaceuticalCharacteristicsComponent t) { // 3 1820 if (t == null) 1821 return this; 1822 if (this.characteristics == null) 1823 this.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 1824 this.characteristics.add(t); 1825 return this; 1826 } 1827 1828 /** 1829 * @return The first repetition of repeating field {@link #characteristics}, 1830 * creating it if it does not already exist 1831 */ 1832 public MedicinalProductPharmaceuticalCharacteristicsComponent getCharacteristicsFirstRep() { 1833 if (getCharacteristics().isEmpty()) { 1834 addCharacteristics(); 1835 } 1836 return getCharacteristics().get(0); 1837 } 1838 1839 /** 1840 * @return {@link #routeOfAdministration} (The path by which the pharmaceutical 1841 * product is taken into or makes contact with the body.) 1842 */ 1843 public List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> getRouteOfAdministration() { 1844 if (this.routeOfAdministration == null) 1845 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1846 return this.routeOfAdministration; 1847 } 1848 1849 /** 1850 * @return Returns a reference to <code>this</code> for easy method chaining 1851 */ 1852 public MedicinalProductPharmaceutical setRouteOfAdministration( 1853 List<MedicinalProductPharmaceuticalRouteOfAdministrationComponent> theRouteOfAdministration) { 1854 this.routeOfAdministration = theRouteOfAdministration; 1855 return this; 1856 } 1857 1858 public boolean hasRouteOfAdministration() { 1859 if (this.routeOfAdministration == null) 1860 return false; 1861 for (MedicinalProductPharmaceuticalRouteOfAdministrationComponent item : this.routeOfAdministration) 1862 if (!item.isEmpty()) 1863 return true; 1864 return false; 1865 } 1866 1867 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent addRouteOfAdministration() { // 3 1868 MedicinalProductPharmaceuticalRouteOfAdministrationComponent t = new MedicinalProductPharmaceuticalRouteOfAdministrationComponent(); 1869 if (this.routeOfAdministration == null) 1870 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1871 this.routeOfAdministration.add(t); 1872 return t; 1873 } 1874 1875 public MedicinalProductPharmaceutical addRouteOfAdministration( 1876 MedicinalProductPharmaceuticalRouteOfAdministrationComponent t) { // 3 1877 if (t == null) 1878 return this; 1879 if (this.routeOfAdministration == null) 1880 this.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 1881 this.routeOfAdministration.add(t); 1882 return this; 1883 } 1884 1885 /** 1886 * @return The first repetition of repeating field 1887 * {@link #routeOfAdministration}, creating it if it does not already 1888 * exist 1889 */ 1890 public MedicinalProductPharmaceuticalRouteOfAdministrationComponent getRouteOfAdministrationFirstRep() { 1891 if (getRouteOfAdministration().isEmpty()) { 1892 addRouteOfAdministration(); 1893 } 1894 return getRouteOfAdministration().get(0); 1895 } 1896 1897 protected void listChildren(List<Property> children) { 1898 super.listChildren(children); 1899 children.add(new Property("identifier", "Identifier", "An identifier for the pharmaceutical medicinal product.", 0, 1900 java.lang.Integer.MAX_VALUE, identifier)); 1901 children.add(new Property("administrableDoseForm", "CodeableConcept", 1902 "The administrable dose form, after necessary reconstitution.", 0, 1, administrableDoseForm)); 1903 children.add(new Property("unitOfPresentation", "CodeableConcept", "Todo.", 0, 1, unitOfPresentation)); 1904 children.add(new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 1905 java.lang.Integer.MAX_VALUE, ingredient)); 1906 children.add(new Property("device", "Reference(DeviceDefinition)", "Accompanying device.", 0, 1907 java.lang.Integer.MAX_VALUE, device)); 1908 children.add(new Property("characteristics", "", "Characteristics e.g. a products onset of action.", 0, 1909 java.lang.Integer.MAX_VALUE, characteristics)); 1910 children.add(new Property("routeOfAdministration", "", 1911 "The path by which the pharmaceutical product is taken into or makes contact with the body.", 0, 1912 java.lang.Integer.MAX_VALUE, routeOfAdministration)); 1913 } 1914 1915 @Override 1916 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1917 switch (_hash) { 1918 case -1618432855: 1919 /* identifier */ return new Property("identifier", "Identifier", 1920 "An identifier for the pharmaceutical medicinal product.", 0, java.lang.Integer.MAX_VALUE, identifier); 1921 case 1446105202: 1922 /* administrableDoseForm */ return new Property("administrableDoseForm", "CodeableConcept", 1923 "The administrable dose form, after necessary reconstitution.", 0, 1, administrableDoseForm); 1924 case -1427765963: 1925 /* unitOfPresentation */ return new Property("unitOfPresentation", "CodeableConcept", "Todo.", 0, 1, 1926 unitOfPresentation); 1927 case -206409263: 1928 /* ingredient */ return new Property("ingredient", "Reference(MedicinalProductIngredient)", "Ingredient.", 0, 1929 java.lang.Integer.MAX_VALUE, ingredient); 1930 case -1335157162: 1931 /* device */ return new Property("device", "Reference(DeviceDefinition)", "Accompanying device.", 0, 1932 java.lang.Integer.MAX_VALUE, device); 1933 case -1529171400: 1934 /* characteristics */ return new Property("characteristics", "", 1935 "Characteristics e.g. a products onset of action.", 0, java.lang.Integer.MAX_VALUE, characteristics); 1936 case 1742084734: 1937 /* routeOfAdministration */ return new Property("routeOfAdministration", "", 1938 "The path by which the pharmaceutical product is taken into or makes contact with the body.", 0, 1939 java.lang.Integer.MAX_VALUE, routeOfAdministration); 1940 default: 1941 return super.getNamedProperty(_hash, _name, _checkValid); 1942 } 1943 1944 } 1945 1946 @Override 1947 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1948 switch (hash) { 1949 case -1618432855: 1950 /* identifier */ return this.identifier == null ? new Base[0] 1951 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1952 case 1446105202: 1953 /* administrableDoseForm */ return this.administrableDoseForm == null ? new Base[0] 1954 : new Base[] { this.administrableDoseForm }; // CodeableConcept 1955 case -1427765963: 1956 /* unitOfPresentation */ return this.unitOfPresentation == null ? new Base[0] 1957 : new Base[] { this.unitOfPresentation }; // CodeableConcept 1958 case -206409263: 1959 /* ingredient */ return this.ingredient == null ? new Base[0] 1960 : this.ingredient.toArray(new Base[this.ingredient.size()]); // Reference 1961 case -1335157162: 1962 /* device */ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 1963 case -1529171400: 1964 /* characteristics */ return this.characteristics == null ? new Base[0] 1965 : this.characteristics.toArray(new Base[this.characteristics.size()]); // MedicinalProductPharmaceuticalCharacteristicsComponent 1966 case 1742084734: 1967 /* routeOfAdministration */ return this.routeOfAdministration == null ? new Base[0] 1968 : this.routeOfAdministration.toArray(new Base[this.routeOfAdministration.size()]); // MedicinalProductPharmaceuticalRouteOfAdministrationComponent 1969 default: 1970 return super.getProperty(hash, name, checkValid); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1977 switch (hash) { 1978 case -1618432855: // identifier 1979 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1980 return value; 1981 case 1446105202: // administrableDoseForm 1982 this.administrableDoseForm = castToCodeableConcept(value); // CodeableConcept 1983 return value; 1984 case -1427765963: // unitOfPresentation 1985 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 1986 return value; 1987 case -206409263: // ingredient 1988 this.getIngredient().add(castToReference(value)); // Reference 1989 return value; 1990 case -1335157162: // device 1991 this.getDevice().add(castToReference(value)); // Reference 1992 return value; 1993 case -1529171400: // characteristics 1994 this.getCharacteristics().add((MedicinalProductPharmaceuticalCharacteristicsComponent) value); // MedicinalProductPharmaceuticalCharacteristicsComponent 1995 return value; 1996 case 1742084734: // routeOfAdministration 1997 this.getRouteOfAdministration().add((MedicinalProductPharmaceuticalRouteOfAdministrationComponent) value); // MedicinalProductPharmaceuticalRouteOfAdministrationComponent 1998 return value; 1999 default: 2000 return super.setProperty(hash, name, value); 2001 } 2002 2003 } 2004 2005 @Override 2006 public Base setProperty(String name, Base value) throws FHIRException { 2007 if (name.equals("identifier")) { 2008 this.getIdentifier().add(castToIdentifier(value)); 2009 } else if (name.equals("administrableDoseForm")) { 2010 this.administrableDoseForm = castToCodeableConcept(value); // CodeableConcept 2011 } else if (name.equals("unitOfPresentation")) { 2012 this.unitOfPresentation = castToCodeableConcept(value); // CodeableConcept 2013 } else if (name.equals("ingredient")) { 2014 this.getIngredient().add(castToReference(value)); 2015 } else if (name.equals("device")) { 2016 this.getDevice().add(castToReference(value)); 2017 } else if (name.equals("characteristics")) { 2018 this.getCharacteristics().add((MedicinalProductPharmaceuticalCharacteristicsComponent) value); 2019 } else if (name.equals("routeOfAdministration")) { 2020 this.getRouteOfAdministration().add((MedicinalProductPharmaceuticalRouteOfAdministrationComponent) value); 2021 } else 2022 return super.setProperty(name, value); 2023 return value; 2024 } 2025 2026 @Override 2027 public Base makeProperty(int hash, String name) throws FHIRException { 2028 switch (hash) { 2029 case -1618432855: 2030 return addIdentifier(); 2031 case 1446105202: 2032 return getAdministrableDoseForm(); 2033 case -1427765963: 2034 return getUnitOfPresentation(); 2035 case -206409263: 2036 return addIngredient(); 2037 case -1335157162: 2038 return addDevice(); 2039 case -1529171400: 2040 return addCharacteristics(); 2041 case 1742084734: 2042 return addRouteOfAdministration(); 2043 default: 2044 return super.makeProperty(hash, name); 2045 } 2046 2047 } 2048 2049 @Override 2050 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2051 switch (hash) { 2052 case -1618432855: 2053 /* identifier */ return new String[] { "Identifier" }; 2054 case 1446105202: 2055 /* administrableDoseForm */ return new String[] { "CodeableConcept" }; 2056 case -1427765963: 2057 /* unitOfPresentation */ return new String[] { "CodeableConcept" }; 2058 case -206409263: 2059 /* ingredient */ return new String[] { "Reference" }; 2060 case -1335157162: 2061 /* device */ return new String[] { "Reference" }; 2062 case -1529171400: 2063 /* characteristics */ return new String[] {}; 2064 case 1742084734: 2065 /* routeOfAdministration */ return new String[] {}; 2066 default: 2067 return super.getTypesForProperty(hash, name); 2068 } 2069 2070 } 2071 2072 @Override 2073 public Base addChild(String name) throws FHIRException { 2074 if (name.equals("identifier")) { 2075 return addIdentifier(); 2076 } else if (name.equals("administrableDoseForm")) { 2077 this.administrableDoseForm = new CodeableConcept(); 2078 return this.administrableDoseForm; 2079 } else if (name.equals("unitOfPresentation")) { 2080 this.unitOfPresentation = new CodeableConcept(); 2081 return this.unitOfPresentation; 2082 } else if (name.equals("ingredient")) { 2083 return addIngredient(); 2084 } else if (name.equals("device")) { 2085 return addDevice(); 2086 } else if (name.equals("characteristics")) { 2087 return addCharacteristics(); 2088 } else if (name.equals("routeOfAdministration")) { 2089 return addRouteOfAdministration(); 2090 } else 2091 return super.addChild(name); 2092 } 2093 2094 public String fhirType() { 2095 return "MedicinalProductPharmaceutical"; 2096 2097 } 2098 2099 public MedicinalProductPharmaceutical copy() { 2100 MedicinalProductPharmaceutical dst = new MedicinalProductPharmaceutical(); 2101 copyValues(dst); 2102 return dst; 2103 } 2104 2105 public void copyValues(MedicinalProductPharmaceutical dst) { 2106 super.copyValues(dst); 2107 if (identifier != null) { 2108 dst.identifier = new ArrayList<Identifier>(); 2109 for (Identifier i : identifier) 2110 dst.identifier.add(i.copy()); 2111 } 2112 ; 2113 dst.administrableDoseForm = administrableDoseForm == null ? null : administrableDoseForm.copy(); 2114 dst.unitOfPresentation = unitOfPresentation == null ? null : unitOfPresentation.copy(); 2115 if (ingredient != null) { 2116 dst.ingredient = new ArrayList<Reference>(); 2117 for (Reference i : ingredient) 2118 dst.ingredient.add(i.copy()); 2119 } 2120 ; 2121 if (device != null) { 2122 dst.device = new ArrayList<Reference>(); 2123 for (Reference i : device) 2124 dst.device.add(i.copy()); 2125 } 2126 ; 2127 if (characteristics != null) { 2128 dst.characteristics = new ArrayList<MedicinalProductPharmaceuticalCharacteristicsComponent>(); 2129 for (MedicinalProductPharmaceuticalCharacteristicsComponent i : characteristics) 2130 dst.characteristics.add(i.copy()); 2131 } 2132 ; 2133 if (routeOfAdministration != null) { 2134 dst.routeOfAdministration = new ArrayList<MedicinalProductPharmaceuticalRouteOfAdministrationComponent>(); 2135 for (MedicinalProductPharmaceuticalRouteOfAdministrationComponent i : routeOfAdministration) 2136 dst.routeOfAdministration.add(i.copy()); 2137 } 2138 ; 2139 } 2140 2141 protected MedicinalProductPharmaceutical typedCopy() { 2142 return copy(); 2143 } 2144 2145 @Override 2146 public boolean equalsDeep(Base other_) { 2147 if (!super.equalsDeep(other_)) 2148 return false; 2149 if (!(other_ instanceof MedicinalProductPharmaceutical)) 2150 return false; 2151 MedicinalProductPharmaceutical o = (MedicinalProductPharmaceutical) other_; 2152 return compareDeep(identifier, o.identifier, true) 2153 && compareDeep(administrableDoseForm, o.administrableDoseForm, true) 2154 && compareDeep(unitOfPresentation, o.unitOfPresentation, true) && compareDeep(ingredient, o.ingredient, true) 2155 && compareDeep(device, o.device, true) && compareDeep(characteristics, o.characteristics, true) 2156 && compareDeep(routeOfAdministration, o.routeOfAdministration, true); 2157 } 2158 2159 @Override 2160 public boolean equalsShallow(Base other_) { 2161 if (!super.equalsShallow(other_)) 2162 return false; 2163 if (!(other_ instanceof MedicinalProductPharmaceutical)) 2164 return false; 2165 MedicinalProductPharmaceutical o = (MedicinalProductPharmaceutical) other_; 2166 return true; 2167 } 2168 2169 public boolean isEmpty() { 2170 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, administrableDoseForm, 2171 unitOfPresentation, ingredient, device, characteristics, routeOfAdministration); 2172 } 2173 2174 @Override 2175 public ResourceType getResourceType() { 2176 return ResourceType.MedicinalProductPharmaceutical; 2177 } 2178 2179 /** 2180 * Search parameter: <b>identifier</b> 2181 * <p> 2182 * Description: <b>An identifier for the pharmaceutical medicinal 2183 * product</b><br> 2184 * Type: <b>token</b><br> 2185 * Path: <b>MedicinalProductPharmaceutical.identifier</b><br> 2186 * </p> 2187 */ 2188 @SearchParamDefinition(name = "identifier", path = "MedicinalProductPharmaceutical.identifier", description = "An identifier for the pharmaceutical medicinal product", type = "token") 2189 public static final String SP_IDENTIFIER = "identifier"; 2190 /** 2191 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2192 * <p> 2193 * Description: <b>An identifier for the pharmaceutical medicinal 2194 * product</b><br> 2195 * Type: <b>token</b><br> 2196 * Path: <b>MedicinalProductPharmaceutical.identifier</b><br> 2197 * </p> 2198 */ 2199 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2200 SP_IDENTIFIER); 2201 2202 /** 2203 * Search parameter: <b>route</b> 2204 * <p> 2205 * Description: <b>Coded expression for the route</b><br> 2206 * Type: <b>token</b><br> 2207 * Path: <b>MedicinalProductPharmaceutical.routeOfAdministration.code</b><br> 2208 * </p> 2209 */ 2210 @SearchParamDefinition(name = "route", path = "MedicinalProductPharmaceutical.routeOfAdministration.code", description = "Coded expression for the route", type = "token") 2211 public static final String SP_ROUTE = "route"; 2212 /** 2213 * <b>Fluent Client</b> search parameter constant for <b>route</b> 2214 * <p> 2215 * Description: <b>Coded expression for the route</b><br> 2216 * Type: <b>token</b><br> 2217 * Path: <b>MedicinalProductPharmaceutical.routeOfAdministration.code</b><br> 2218 * </p> 2219 */ 2220 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROUTE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2221 SP_ROUTE); 2222 2223 /** 2224 * Search parameter: <b>target-species</b> 2225 * <p> 2226 * Description: <b>Coded expression for the species</b><br> 2227 * Type: <b>token</b><br> 2228 * Path: 2229 * <b>MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code</b><br> 2230 * </p> 2231 */ 2232 @SearchParamDefinition(name = "target-species", path = "MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code", description = "Coded expression for the species", type = "token") 2233 public static final String SP_TARGET_SPECIES = "target-species"; 2234 /** 2235 * <b>Fluent Client</b> search parameter constant for <b>target-species</b> 2236 * <p> 2237 * Description: <b>Coded expression for the species</b><br> 2238 * Type: <b>token</b><br> 2239 * Path: 2240 * <b>MedicinalProductPharmaceutical.routeOfAdministration.targetSpecies.code</b><br> 2241 * </p> 2242 */ 2243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_SPECIES = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2244 SP_TARGET_SPECIES); 2245 2246}