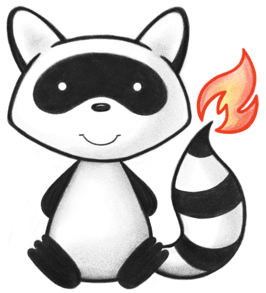
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * Defines the characteristics of a message that can be shared between systems, 052 * including the type of event that initiates the message, the content to be 053 * transmitted and what response(s), if any, are permitted. 054 */ 055@ResourceDef(name = "MessageDefinition", profile = "http://hl7.org/fhir/StructureDefinition/MessageDefinition") 056@ChildOrder(names = { "url", "identifier", "version", "name", "title", "replaces", "status", "experimental", "date", 057 "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "base", "parent", 058 "event[x]", "category", "focus", "responseRequired", "allowedResponse", "graph" }) 059public class MessageDefinition extends MetadataResource { 060 061 public enum MessageSignificanceCategory { 062 /** 063 * The message represents/requests a change that should not be processed more 064 * than once; e.g., making a booking for an appointment. 065 */ 066 CONSEQUENCE, 067 /** 068 * The message represents a response to query for current information. 069 * Retrospective processing is wrong and/or wasteful. 070 */ 071 CURRENCY, 072 /** 073 * The content is not necessarily intended to be current, and it can be 074 * reprocessed, though there may be version issues created by processing old 075 * notifications. 076 */ 077 NOTIFICATION, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 083 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("consequence".equals(codeString)) 087 return CONSEQUENCE; 088 if ("currency".equals(codeString)) 089 return CURRENCY; 090 if ("notification".equals(codeString)) 091 return NOTIFICATION; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case CONSEQUENCE: 101 return "consequence"; 102 case CURRENCY: 103 return "currency"; 104 case NOTIFICATION: 105 return "notification"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case CONSEQUENCE: 116 return "http://hl7.org/fhir/message-significance-category"; 117 case CURRENCY: 118 return "http://hl7.org/fhir/message-significance-category"; 119 case NOTIFICATION: 120 return "http://hl7.org/fhir/message-significance-category"; 121 case NULL: 122 return null; 123 default: 124 return "?"; 125 } 126 } 127 128 public String getDefinition() { 129 switch (this) { 130 case CONSEQUENCE: 131 return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 132 case CURRENCY: 133 return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 134 case NOTIFICATION: 135 return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDisplay() { 144 switch (this) { 145 case CONSEQUENCE: 146 return "Consequence"; 147 case CURRENCY: 148 return "Currency"; 149 case NOTIFICATION: 150 return "Notification"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 } 158 159 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 160 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 161 if (codeString == null || "".equals(codeString)) 162 if (codeString == null || "".equals(codeString)) 163 return null; 164 if ("consequence".equals(codeString)) 165 return MessageSignificanceCategory.CONSEQUENCE; 166 if ("currency".equals(codeString)) 167 return MessageSignificanceCategory.CURRENCY; 168 if ("notification".equals(codeString)) 169 return MessageSignificanceCategory.NOTIFICATION; 170 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 171 } 172 173 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NULL, code); 181 if ("consequence".equals(codeString)) 182 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE, code); 183 if ("currency".equals(codeString)) 184 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY, code); 185 if ("notification".equals(codeString)) 186 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION, code); 187 throw new FHIRException("Unknown MessageSignificanceCategory code '" + codeString + "'"); 188 } 189 190 public String toCode(MessageSignificanceCategory code) { 191 if (code == MessageSignificanceCategory.CONSEQUENCE) 192 return "consequence"; 193 if (code == MessageSignificanceCategory.CURRENCY) 194 return "currency"; 195 if (code == MessageSignificanceCategory.NOTIFICATION) 196 return "notification"; 197 return "?"; 198 } 199 200 public String toSystem(MessageSignificanceCategory code) { 201 return code.getSystem(); 202 } 203 } 204 205 public enum MessageheaderResponseRequest { 206 /** 207 * initiator expects a response for this message. 208 */ 209 ALWAYS, 210 /** 211 * initiator expects a response only if in error. 212 */ 213 ONERROR, 214 /** 215 * initiator does not expect a response. 216 */ 217 NEVER, 218 /** 219 * initiator expects a response only if successful. 220 */ 221 ONSUCCESS, 222 /** 223 * added to help the parsers with the generic types 224 */ 225 NULL; 226 227 public static MessageheaderResponseRequest fromCode(String codeString) throws FHIRException { 228 if (codeString == null || "".equals(codeString)) 229 return null; 230 if ("always".equals(codeString)) 231 return ALWAYS; 232 if ("on-error".equals(codeString)) 233 return ONERROR; 234 if ("never".equals(codeString)) 235 return NEVER; 236 if ("on-success".equals(codeString)) 237 return ONSUCCESS; 238 if (Configuration.isAcceptInvalidEnums()) 239 return null; 240 else 241 throw new FHIRException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 242 } 243 244 public String toCode() { 245 switch (this) { 246 case ALWAYS: 247 return "always"; 248 case ONERROR: 249 return "on-error"; 250 case NEVER: 251 return "never"; 252 case ONSUCCESS: 253 return "on-success"; 254 case NULL: 255 return null; 256 default: 257 return "?"; 258 } 259 } 260 261 public String getSystem() { 262 switch (this) { 263 case ALWAYS: 264 return "http://hl7.org/fhir/messageheader-response-request"; 265 case ONERROR: 266 return "http://hl7.org/fhir/messageheader-response-request"; 267 case NEVER: 268 return "http://hl7.org/fhir/messageheader-response-request"; 269 case ONSUCCESS: 270 return "http://hl7.org/fhir/messageheader-response-request"; 271 case NULL: 272 return null; 273 default: 274 return "?"; 275 } 276 } 277 278 public String getDefinition() { 279 switch (this) { 280 case ALWAYS: 281 return "initiator expects a response for this message."; 282 case ONERROR: 283 return "initiator expects a response only if in error."; 284 case NEVER: 285 return "initiator does not expect a response."; 286 case ONSUCCESS: 287 return "initiator expects a response only if successful."; 288 case NULL: 289 return null; 290 default: 291 return "?"; 292 } 293 } 294 295 public String getDisplay() { 296 switch (this) { 297 case ALWAYS: 298 return "Always"; 299 case ONERROR: 300 return "Error/reject conditions only"; 301 case NEVER: 302 return "Never"; 303 case ONSUCCESS: 304 return "Successful completion only"; 305 case NULL: 306 return null; 307 default: 308 return "?"; 309 } 310 } 311 } 312 313 public static class MessageheaderResponseRequestEnumFactory implements EnumFactory<MessageheaderResponseRequest> { 314 public MessageheaderResponseRequest fromCode(String codeString) throws IllegalArgumentException { 315 if (codeString == null || "".equals(codeString)) 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("always".equals(codeString)) 319 return MessageheaderResponseRequest.ALWAYS; 320 if ("on-error".equals(codeString)) 321 return MessageheaderResponseRequest.ONERROR; 322 if ("never".equals(codeString)) 323 return MessageheaderResponseRequest.NEVER; 324 if ("on-success".equals(codeString)) 325 return MessageheaderResponseRequest.ONSUCCESS; 326 throw new IllegalArgumentException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 327 } 328 329 public Enumeration<MessageheaderResponseRequest> fromType(PrimitiveType<?> code) throws FHIRException { 330 if (code == null) 331 return null; 332 if (code.isEmpty()) 333 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 334 String codeString = code.asStringValue(); 335 if (codeString == null || "".equals(codeString)) 336 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NULL, code); 337 if ("always".equals(codeString)) 338 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ALWAYS, code); 339 if ("on-error".equals(codeString)) 340 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONERROR, code); 341 if ("never".equals(codeString)) 342 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.NEVER, code); 343 if ("on-success".equals(codeString)) 344 return new Enumeration<MessageheaderResponseRequest>(this, MessageheaderResponseRequest.ONSUCCESS, code); 345 throw new FHIRException("Unknown MessageheaderResponseRequest code '" + codeString + "'"); 346 } 347 348 public String toCode(MessageheaderResponseRequest code) { 349 if (code == MessageheaderResponseRequest.ALWAYS) 350 return "always"; 351 if (code == MessageheaderResponseRequest.ONERROR) 352 return "on-error"; 353 if (code == MessageheaderResponseRequest.NEVER) 354 return "never"; 355 if (code == MessageheaderResponseRequest.ONSUCCESS) 356 return "on-success"; 357 return "?"; 358 } 359 360 public String toSystem(MessageheaderResponseRequest code) { 361 return code.getSystem(); 362 } 363 } 364 365 @Block() 366 public static class MessageDefinitionFocusComponent extends BackboneElement implements IBaseBackboneElement { 367 /** 368 * The kind of resource that must be the focus for this message. 369 */ 370 @Child(name = "code", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 371 @Description(shortDefinition = "Type of resource", formalDefinition = "The kind of resource that must be the focus for this message.") 372 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/resource-types") 373 protected CodeType code; 374 375 /** 376 * A profile that reflects constraints for the focal resource (and potentially 377 * for related resources). 378 */ 379 @Child(name = "profile", type = { 380 CanonicalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 381 @Description(shortDefinition = "Profile that must be adhered to by focus", formalDefinition = "A profile that reflects constraints for the focal resource (and potentially for related resources).") 382 protected CanonicalType profile; 383 384 /** 385 * Identifies the minimum number of resources of this type that must be pointed 386 * to by a message in order for it to be valid against this MessageDefinition. 387 */ 388 @Child(name = "min", type = { 389 UnsignedIntType.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 390 @Description(shortDefinition = "Minimum number of focuses of this type", formalDefinition = "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.") 391 protected UnsignedIntType min; 392 393 /** 394 * Identifies the maximum number of resources of this type that must be pointed 395 * to by a message in order for it to be valid against this MessageDefinition. 396 */ 397 @Child(name = "max", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 398 @Description(shortDefinition = "Maximum number of focuses of this type", formalDefinition = "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.") 399 protected StringType max; 400 401 private static final long serialVersionUID = -68504836L; 402 403 /** 404 * Constructor 405 */ 406 public MessageDefinitionFocusComponent() { 407 super(); 408 } 409 410 /** 411 * Constructor 412 */ 413 public MessageDefinitionFocusComponent(CodeType code, UnsignedIntType min) { 414 super(); 415 this.code = code; 416 this.min = min; 417 } 418 419 /** 420 * @return {@link #code} (The kind of resource that must be the focus for this 421 * message.). This is the underlying object with id, value and 422 * extensions. The accessor "getCode" gives direct access to the value 423 */ 424 public CodeType getCodeElement() { 425 if (this.code == null) 426 if (Configuration.errorOnAutoCreate()) 427 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.code"); 428 else if (Configuration.doAutoCreate()) 429 this.code = new CodeType(); // bb 430 return this.code; 431 } 432 433 public boolean hasCodeElement() { 434 return this.code != null && !this.code.isEmpty(); 435 } 436 437 public boolean hasCode() { 438 return this.code != null && !this.code.isEmpty(); 439 } 440 441 /** 442 * @param value {@link #code} (The kind of resource that must be the focus for 443 * this message.). This is the underlying object with id, value and 444 * extensions. The accessor "getCode" gives direct access to the 445 * value 446 */ 447 public MessageDefinitionFocusComponent setCodeElement(CodeType value) { 448 this.code = value; 449 return this; 450 } 451 452 /** 453 * @return The kind of resource that must be the focus for this message. 454 */ 455 public String getCode() { 456 return this.code == null ? null : this.code.getValue(); 457 } 458 459 /** 460 * @param value The kind of resource that must be the focus for this message. 461 */ 462 public MessageDefinitionFocusComponent setCode(String value) { 463 if (this.code == null) 464 this.code = new CodeType(); 465 this.code.setValue(value); 466 return this; 467 } 468 469 /** 470 * @return {@link #profile} (A profile that reflects constraints for the focal 471 * resource (and potentially for related resources).). This is the 472 * underlying object with id, value and extensions. The accessor 473 * "getProfile" gives direct access to the value 474 */ 475 public CanonicalType getProfileElement() { 476 if (this.profile == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 479 else if (Configuration.doAutoCreate()) 480 this.profile = new CanonicalType(); // bb 481 return this.profile; 482 } 483 484 public boolean hasProfileElement() { 485 return this.profile != null && !this.profile.isEmpty(); 486 } 487 488 public boolean hasProfile() { 489 return this.profile != null && !this.profile.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #profile} (A profile that reflects constraints for the 494 * focal resource (and potentially for related resources).). This 495 * is the underlying object with id, value and extensions. The 496 * accessor "getProfile" gives direct access to the value 497 */ 498 public MessageDefinitionFocusComponent setProfileElement(CanonicalType value) { 499 this.profile = value; 500 return this; 501 } 502 503 /** 504 * @return A profile that reflects constraints for the focal resource (and 505 * potentially for related resources). 506 */ 507 public String getProfile() { 508 return this.profile == null ? null : this.profile.getValue(); 509 } 510 511 /** 512 * @param value A profile that reflects constraints for the focal resource (and 513 * potentially for related resources). 514 */ 515 public MessageDefinitionFocusComponent setProfile(String value) { 516 if (Utilities.noString(value)) 517 this.profile = null; 518 else { 519 if (this.profile == null) 520 this.profile = new CanonicalType(); 521 this.profile.setValue(value); 522 } 523 return this; 524 } 525 526 /** 527 * @return {@link #min} (Identifies the minimum number of resources of this type 528 * that must be pointed to by a message in order for it to be valid 529 * against this MessageDefinition.). This is the underlying object with 530 * id, value and extensions. The accessor "getMin" gives direct access 531 * to the value 532 */ 533 public UnsignedIntType getMinElement() { 534 if (this.min == null) 535 if (Configuration.errorOnAutoCreate()) 536 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.min"); 537 else if (Configuration.doAutoCreate()) 538 this.min = new UnsignedIntType(); // bb 539 return this.min; 540 } 541 542 public boolean hasMinElement() { 543 return this.min != null && !this.min.isEmpty(); 544 } 545 546 public boolean hasMin() { 547 return this.min != null && !this.min.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #min} (Identifies the minimum number of resources of this 552 * type that must be pointed to by a message in order for it to be 553 * valid against this MessageDefinition.). This is the underlying 554 * object with id, value and extensions. The accessor "getMin" 555 * gives direct access to the value 556 */ 557 public MessageDefinitionFocusComponent setMinElement(UnsignedIntType value) { 558 this.min = value; 559 return this; 560 } 561 562 /** 563 * @return Identifies the minimum number of resources of this type that must be 564 * pointed to by a message in order for it to be valid against this 565 * MessageDefinition. 566 */ 567 public int getMin() { 568 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 569 } 570 571 /** 572 * @param value Identifies the minimum number of resources of this type that 573 * must be pointed to by a message in order for it to be valid 574 * against this MessageDefinition. 575 */ 576 public MessageDefinitionFocusComponent setMin(int value) { 577 if (this.min == null) 578 this.min = new UnsignedIntType(); 579 this.min.setValue(value); 580 return this; 581 } 582 583 /** 584 * @return {@link #max} (Identifies the maximum number of resources of this type 585 * that must be pointed to by a message in order for it to be valid 586 * against this MessageDefinition.). This is the underlying object with 587 * id, value and extensions. The accessor "getMax" gives direct access 588 * to the value 589 */ 590 public StringType getMaxElement() { 591 if (this.max == null) 592 if (Configuration.errorOnAutoCreate()) 593 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.max"); 594 else if (Configuration.doAutoCreate()) 595 this.max = new StringType(); // bb 596 return this.max; 597 } 598 599 public boolean hasMaxElement() { 600 return this.max != null && !this.max.isEmpty(); 601 } 602 603 public boolean hasMax() { 604 return this.max != null && !this.max.isEmpty(); 605 } 606 607 /** 608 * @param value {@link #max} (Identifies the maximum number of resources of this 609 * type that must be pointed to by a message in order for it to be 610 * valid against this MessageDefinition.). This is the underlying 611 * object with id, value and extensions. The accessor "getMax" 612 * gives direct access to the value 613 */ 614 public MessageDefinitionFocusComponent setMaxElement(StringType value) { 615 this.max = value; 616 return this; 617 } 618 619 /** 620 * @return Identifies the maximum number of resources of this type that must be 621 * pointed to by a message in order for it to be valid against this 622 * MessageDefinition. 623 */ 624 public String getMax() { 625 return this.max == null ? null : this.max.getValue(); 626 } 627 628 /** 629 * @param value Identifies the maximum number of resources of this type that 630 * must be pointed to by a message in order for it to be valid 631 * against this MessageDefinition. 632 */ 633 public MessageDefinitionFocusComponent setMax(String value) { 634 if (Utilities.noString(value)) 635 this.max = null; 636 else { 637 if (this.max == null) 638 this.max = new StringType(); 639 this.max.setValue(value); 640 } 641 return this; 642 } 643 644 protected void listChildren(List<Property> children) { 645 super.listChildren(children); 646 children.add( 647 new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code)); 648 children.add(new Property("profile", "canonical(StructureDefinition)", 649 "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, 650 profile)); 651 children.add(new Property("min", "unsignedInt", 652 "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 653 0, 1, min)); 654 children.add(new Property("max", "string", 655 "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 656 0, 1, max)); 657 } 658 659 @Override 660 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 661 switch (_hash) { 662 case 3059181: 663 /* code */ return new Property("code", "code", "The kind of resource that must be the focus for this message.", 664 0, 1, code); 665 case -309425751: 666 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 667 "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, 668 profile); 669 case 108114: 670 /* min */ return new Property("min", "unsignedInt", 671 "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 672 0, 1, min); 673 case 107876: 674 /* max */ return new Property("max", "string", 675 "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 676 0, 1, max); 677 default: 678 return super.getNamedProperty(_hash, _name, _checkValid); 679 } 680 681 } 682 683 @Override 684 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 685 switch (hash) { 686 case 3059181: 687 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeType 688 case -309425751: 689 /* profile */ return this.profile == null ? new Base[0] : new Base[] { this.profile }; // CanonicalType 690 case 108114: 691 /* min */ return this.min == null ? new Base[0] : new Base[] { this.min }; // UnsignedIntType 692 case 107876: 693 /* max */ return this.max == null ? new Base[0] : new Base[] { this.max }; // StringType 694 default: 695 return super.getProperty(hash, name, checkValid); 696 } 697 698 } 699 700 @Override 701 public Base setProperty(int hash, String name, Base value) throws FHIRException { 702 switch (hash) { 703 case 3059181: // code 704 this.code = castToCode(value); // CodeType 705 return value; 706 case -309425751: // profile 707 this.profile = castToCanonical(value); // CanonicalType 708 return value; 709 case 108114: // min 710 this.min = castToUnsignedInt(value); // UnsignedIntType 711 return value; 712 case 107876: // max 713 this.max = castToString(value); // StringType 714 return value; 715 default: 716 return super.setProperty(hash, name, value); 717 } 718 719 } 720 721 @Override 722 public Base setProperty(String name, Base value) throws FHIRException { 723 if (name.equals("code")) { 724 this.code = castToCode(value); // CodeType 725 } else if (name.equals("profile")) { 726 this.profile = castToCanonical(value); // CanonicalType 727 } else if (name.equals("min")) { 728 this.min = castToUnsignedInt(value); // UnsignedIntType 729 } else if (name.equals("max")) { 730 this.max = castToString(value); // StringType 731 } else 732 return super.setProperty(name, value); 733 return value; 734 } 735 736 @Override 737 public Base makeProperty(int hash, String name) throws FHIRException { 738 switch (hash) { 739 case 3059181: 740 return getCodeElement(); 741 case -309425751: 742 return getProfileElement(); 743 case 108114: 744 return getMinElement(); 745 case 107876: 746 return getMaxElement(); 747 default: 748 return super.makeProperty(hash, name); 749 } 750 751 } 752 753 @Override 754 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 755 switch (hash) { 756 case 3059181: 757 /* code */ return new String[] { "code" }; 758 case -309425751: 759 /* profile */ return new String[] { "canonical" }; 760 case 108114: 761 /* min */ return new String[] { "unsignedInt" }; 762 case 107876: 763 /* max */ return new String[] { "string" }; 764 default: 765 return super.getTypesForProperty(hash, name); 766 } 767 768 } 769 770 @Override 771 public Base addChild(String name) throws FHIRException { 772 if (name.equals("code")) { 773 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.code"); 774 } else if (name.equals("profile")) { 775 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.profile"); 776 } else if (name.equals("min")) { 777 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.min"); 778 } else if (name.equals("max")) { 779 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.max"); 780 } else 781 return super.addChild(name); 782 } 783 784 public MessageDefinitionFocusComponent copy() { 785 MessageDefinitionFocusComponent dst = new MessageDefinitionFocusComponent(); 786 copyValues(dst); 787 return dst; 788 } 789 790 public void copyValues(MessageDefinitionFocusComponent dst) { 791 super.copyValues(dst); 792 dst.code = code == null ? null : code.copy(); 793 dst.profile = profile == null ? null : profile.copy(); 794 dst.min = min == null ? null : min.copy(); 795 dst.max = max == null ? null : max.copy(); 796 } 797 798 @Override 799 public boolean equalsDeep(Base other_) { 800 if (!super.equalsDeep(other_)) 801 return false; 802 if (!(other_ instanceof MessageDefinitionFocusComponent)) 803 return false; 804 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 805 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(min, o.min, true) 806 && compareDeep(max, o.max, true); 807 } 808 809 @Override 810 public boolean equalsShallow(Base other_) { 811 if (!super.equalsShallow(other_)) 812 return false; 813 if (!(other_ instanceof MessageDefinitionFocusComponent)) 814 return false; 815 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 816 return compareValues(code, o.code, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true); 817 } 818 819 public boolean isEmpty() { 820 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, min, max); 821 } 822 823 public String fhirType() { 824 return "MessageDefinition.focus"; 825 826 } 827 828 } 829 830 @Block() 831 public static class MessageDefinitionAllowedResponseComponent extends BackboneElement 832 implements IBaseBackboneElement { 833 /** 834 * A reference to the message definition that must be adhered to by this 835 * supported response. 836 */ 837 @Child(name = "message", type = { 838 CanonicalType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 839 @Description(shortDefinition = "Reference to allowed message definition response", formalDefinition = "A reference to the message definition that must be adhered to by this supported response.") 840 protected CanonicalType message; 841 842 /** 843 * Provides a description of the circumstances in which this response should be 844 * used (as opposed to one of the alternative responses). 845 */ 846 @Child(name = "situation", type = { 847 MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 848 @Description(shortDefinition = "When should this response be used", formalDefinition = "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).") 849 protected MarkdownType situation; 850 851 private static final long serialVersionUID = -1943810550L; 852 853 /** 854 * Constructor 855 */ 856 public MessageDefinitionAllowedResponseComponent() { 857 super(); 858 } 859 860 /** 861 * Constructor 862 */ 863 public MessageDefinitionAllowedResponseComponent(CanonicalType message) { 864 super(); 865 this.message = message; 866 } 867 868 /** 869 * @return {@link #message} (A reference to the message definition that must be 870 * adhered to by this supported response.). This is the underlying 871 * object with id, value and extensions. The accessor "getMessage" gives 872 * direct access to the value 873 */ 874 public CanonicalType getMessageElement() { 875 if (this.message == null) 876 if (Configuration.errorOnAutoCreate()) 877 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 878 else if (Configuration.doAutoCreate()) 879 this.message = new CanonicalType(); // bb 880 return this.message; 881 } 882 883 public boolean hasMessageElement() { 884 return this.message != null && !this.message.isEmpty(); 885 } 886 887 public boolean hasMessage() { 888 return this.message != null && !this.message.isEmpty(); 889 } 890 891 /** 892 * @param value {@link #message} (A reference to the message definition that 893 * must be adhered to by this supported response.). This is the 894 * underlying object with id, value and extensions. The accessor 895 * "getMessage" gives direct access to the value 896 */ 897 public MessageDefinitionAllowedResponseComponent setMessageElement(CanonicalType value) { 898 this.message = value; 899 return this; 900 } 901 902 /** 903 * @return A reference to the message definition that must be adhered to by this 904 * supported response. 905 */ 906 public String getMessage() { 907 return this.message == null ? null : this.message.getValue(); 908 } 909 910 /** 911 * @param value A reference to the message definition that must be adhered to by 912 * this supported response. 913 */ 914 public MessageDefinitionAllowedResponseComponent setMessage(String value) { 915 if (this.message == null) 916 this.message = new CanonicalType(); 917 this.message.setValue(value); 918 return this; 919 } 920 921 /** 922 * @return {@link #situation} (Provides a description of the circumstances in 923 * which this response should be used (as opposed to one of the 924 * alternative responses).). This is the underlying object with id, 925 * value and extensions. The accessor "getSituation" gives direct access 926 * to the value 927 */ 928 public MarkdownType getSituationElement() { 929 if (this.situation == null) 930 if (Configuration.errorOnAutoCreate()) 931 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.situation"); 932 else if (Configuration.doAutoCreate()) 933 this.situation = new MarkdownType(); // bb 934 return this.situation; 935 } 936 937 public boolean hasSituationElement() { 938 return this.situation != null && !this.situation.isEmpty(); 939 } 940 941 public boolean hasSituation() { 942 return this.situation != null && !this.situation.isEmpty(); 943 } 944 945 /** 946 * @param value {@link #situation} (Provides a description of the circumstances 947 * in which this response should be used (as opposed to one of the 948 * alternative responses).). This is the underlying object with id, 949 * value and extensions. The accessor "getSituation" gives direct 950 * access to the value 951 */ 952 public MessageDefinitionAllowedResponseComponent setSituationElement(MarkdownType value) { 953 this.situation = value; 954 return this; 955 } 956 957 /** 958 * @return Provides a description of the circumstances in which this response 959 * should be used (as opposed to one of the alternative responses). 960 */ 961 public String getSituation() { 962 return this.situation == null ? null : this.situation.getValue(); 963 } 964 965 /** 966 * @param value Provides a description of the circumstances in which this 967 * response should be used (as opposed to one of the alternative 968 * responses). 969 */ 970 public MessageDefinitionAllowedResponseComponent setSituation(String value) { 971 if (value == null) 972 this.situation = null; 973 else { 974 if (this.situation == null) 975 this.situation = new MarkdownType(); 976 this.situation.setValue(value); 977 } 978 return this; 979 } 980 981 protected void listChildren(List<Property> children) { 982 super.listChildren(children); 983 children.add(new Property("message", "canonical(MessageDefinition)", 984 "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message)); 985 children.add(new Property("situation", "markdown", 986 "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 987 0, 1, situation)); 988 } 989 990 @Override 991 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 992 switch (_hash) { 993 case 954925063: 994 /* message */ return new Property("message", "canonical(MessageDefinition)", 995 "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message); 996 case -73377282: 997 /* situation */ return new Property("situation", "markdown", 998 "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 999 0, 1, situation); 1000 default: 1001 return super.getNamedProperty(_hash, _name, _checkValid); 1002 } 1003 1004 } 1005 1006 @Override 1007 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1008 switch (hash) { 1009 case 954925063: 1010 /* message */ return this.message == null ? new Base[0] : new Base[] { this.message }; // CanonicalType 1011 case -73377282: 1012 /* situation */ return this.situation == null ? new Base[0] : new Base[] { this.situation }; // MarkdownType 1013 default: 1014 return super.getProperty(hash, name, checkValid); 1015 } 1016 1017 } 1018 1019 @Override 1020 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1021 switch (hash) { 1022 case 954925063: // message 1023 this.message = castToCanonical(value); // CanonicalType 1024 return value; 1025 case -73377282: // situation 1026 this.situation = castToMarkdown(value); // MarkdownType 1027 return value; 1028 default: 1029 return super.setProperty(hash, name, value); 1030 } 1031 1032 } 1033 1034 @Override 1035 public Base setProperty(String name, Base value) throws FHIRException { 1036 if (name.equals("message")) { 1037 this.message = castToCanonical(value); // CanonicalType 1038 } else if (name.equals("situation")) { 1039 this.situation = castToMarkdown(value); // MarkdownType 1040 } else 1041 return super.setProperty(name, value); 1042 return value; 1043 } 1044 1045 @Override 1046 public Base makeProperty(int hash, String name) throws FHIRException { 1047 switch (hash) { 1048 case 954925063: 1049 return getMessageElement(); 1050 case -73377282: 1051 return getSituationElement(); 1052 default: 1053 return super.makeProperty(hash, name); 1054 } 1055 1056 } 1057 1058 @Override 1059 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1060 switch (hash) { 1061 case 954925063: 1062 /* message */ return new String[] { "canonical" }; 1063 case -73377282: 1064 /* situation */ return new String[] { "markdown" }; 1065 default: 1066 return super.getTypesForProperty(hash, name); 1067 } 1068 1069 } 1070 1071 @Override 1072 public Base addChild(String name) throws FHIRException { 1073 if (name.equals("message")) { 1074 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.message"); 1075 } else if (name.equals("situation")) { 1076 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.situation"); 1077 } else 1078 return super.addChild(name); 1079 } 1080 1081 public MessageDefinitionAllowedResponseComponent copy() { 1082 MessageDefinitionAllowedResponseComponent dst = new MessageDefinitionAllowedResponseComponent(); 1083 copyValues(dst); 1084 return dst; 1085 } 1086 1087 public void copyValues(MessageDefinitionAllowedResponseComponent dst) { 1088 super.copyValues(dst); 1089 dst.message = message == null ? null : message.copy(); 1090 dst.situation = situation == null ? null : situation.copy(); 1091 } 1092 1093 @Override 1094 public boolean equalsDeep(Base other_) { 1095 if (!super.equalsDeep(other_)) 1096 return false; 1097 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 1098 return false; 1099 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 1100 return compareDeep(message, o.message, true) && compareDeep(situation, o.situation, true); 1101 } 1102 1103 @Override 1104 public boolean equalsShallow(Base other_) { 1105 if (!super.equalsShallow(other_)) 1106 return false; 1107 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 1108 return false; 1109 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 1110 return compareValues(situation, o.situation, true); 1111 } 1112 1113 public boolean isEmpty() { 1114 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(message, situation); 1115 } 1116 1117 public String fhirType() { 1118 return "MessageDefinition.allowedResponse"; 1119 1120 } 1121 1122 } 1123 1124 /** 1125 * A formal identifier that is used to identify this message definition when it 1126 * is represented in other formats, or referenced in a specification, model, 1127 * design or an instance. 1128 */ 1129 @Child(name = "identifier", type = { 1130 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1131 @Description(shortDefinition = "Primary key for the message definition on a given server", formalDefinition = "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.") 1132 protected List<Identifier> identifier; 1133 1134 /** 1135 * A MessageDefinition that is superseded by this definition. 1136 */ 1137 @Child(name = "replaces", type = { 1138 CanonicalType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1139 @Description(shortDefinition = "Takes the place of", formalDefinition = "A MessageDefinition that is superseded by this definition.") 1140 protected List<CanonicalType> replaces; 1141 1142 /** 1143 * Explanation of why this message definition is needed and why it has been 1144 * designed as it has. 1145 */ 1146 @Child(name = "purpose", type = { MarkdownType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1147 @Description(shortDefinition = "Why this message definition is defined", formalDefinition = "Explanation of why this message definition is needed and why it has been designed as it has.") 1148 protected MarkdownType purpose; 1149 1150 /** 1151 * A copyright statement relating to the message definition and/or its contents. 1152 * Copyright statements are generally legal restrictions on the use and 1153 * publishing of the message definition. 1154 */ 1155 @Child(name = "copyright", type = { 1156 MarkdownType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 1157 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.") 1158 protected MarkdownType copyright; 1159 1160 /** 1161 * The MessageDefinition that is the basis for the contents of this resource. 1162 */ 1163 @Child(name = "base", type = { CanonicalType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1164 @Description(shortDefinition = "Definition this one is based on", formalDefinition = "The MessageDefinition that is the basis for the contents of this resource.") 1165 protected CanonicalType base; 1166 1167 /** 1168 * Identifies a protocol or workflow that this MessageDefinition represents a 1169 * step in. 1170 */ 1171 @Child(name = "parent", type = { 1172 CanonicalType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1173 @Description(shortDefinition = "Protocol/workflow this is part of", formalDefinition = "Identifies a protocol or workflow that this MessageDefinition represents a step in.") 1174 protected List<CanonicalType> parent; 1175 1176 /** 1177 * Event code or link to the EventDefinition. 1178 */ 1179 @Child(name = "event", type = { Coding.class, 1180 UriType.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1181 @Description(shortDefinition = "Event code or link to the EventDefinition", formalDefinition = "Event code or link to the EventDefinition.") 1182 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-events") 1183 protected Type event; 1184 1185 /** 1186 * The impact of the content of the message. 1187 */ 1188 @Child(name = "category", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1189 @Description(shortDefinition = "consequence | currency | notification", formalDefinition = "The impact of the content of the message.") 1190 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/message-significance-category") 1191 protected Enumeration<MessageSignificanceCategory> category; 1192 1193 /** 1194 * Identifies the resource (or resources) that are being addressed by the event. 1195 * For example, the Encounter for an admit message or two Account records for a 1196 * merge. 1197 */ 1198 @Child(name = "focus", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1199 @Description(shortDefinition = "Resource(s) that are the subject of the event", formalDefinition = "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.") 1200 protected List<MessageDefinitionFocusComponent> focus; 1201 1202 /** 1203 * Declare at a message definition level whether a response is required or only 1204 * upon error or success, or never. 1205 */ 1206 @Child(name = "responseRequired", type = { 1207 CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1208 @Description(shortDefinition = "always | on-error | never | on-success", formalDefinition = "Declare at a message definition level whether a response is required or only upon error or success, or never.") 1209 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/messageheader-response-request") 1210 protected Enumeration<MessageheaderResponseRequest> responseRequired; 1211 1212 /** 1213 * Indicates what types of messages may be sent as an application-level response 1214 * to this message. 1215 */ 1216 @Child(name = "allowedResponse", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1217 @Description(shortDefinition = "Responses to this message", formalDefinition = "Indicates what types of messages may be sent as an application-level response to this message.") 1218 protected List<MessageDefinitionAllowedResponseComponent> allowedResponse; 1219 1220 /** 1221 * Canonical reference to a GraphDefinition. If a URL is provided, it is the 1222 * canonical reference to a [[[GraphDefinition]]] that it controls what 1223 * resources are to be added to the bundle when building the document. The 1224 * GraphDefinition can also specify profiles that apply to the various 1225 * resources. 1226 */ 1227 @Child(name = "graph", type = { 1228 CanonicalType.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1229 @Description(shortDefinition = "Canonical reference to a GraphDefinition", formalDefinition = "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.") 1230 protected List<CanonicalType> graph; 1231 1232 private static final long serialVersionUID = 927775347L; 1233 1234 /** 1235 * Constructor 1236 */ 1237 public MessageDefinition() { 1238 super(); 1239 } 1240 1241 /** 1242 * Constructor 1243 */ 1244 public MessageDefinition(Enumeration<PublicationStatus> status, DateTimeType date, Type event) { 1245 super(); 1246 this.status = status; 1247 this.date = date; 1248 this.event = event; 1249 } 1250 1251 /** 1252 * @return {@link #url} (The business identifier that is used to reference the 1253 * MessageDefinition and *is* expected to be consistent from server to 1254 * server.). This is the underlying object with id, value and 1255 * extensions. The accessor "getUrl" gives direct access to the value 1256 */ 1257 public UriType getUrlElement() { 1258 if (this.url == null) 1259 if (Configuration.errorOnAutoCreate()) 1260 throw new Error("Attempt to auto-create MessageDefinition.url"); 1261 else if (Configuration.doAutoCreate()) 1262 this.url = new UriType(); // bb 1263 return this.url; 1264 } 1265 1266 public boolean hasUrlElement() { 1267 return this.url != null && !this.url.isEmpty(); 1268 } 1269 1270 public boolean hasUrl() { 1271 return this.url != null && !this.url.isEmpty(); 1272 } 1273 1274 /** 1275 * @param value {@link #url} (The business identifier that is used to reference 1276 * the MessageDefinition and *is* expected to be consistent from 1277 * server to server.). This is the underlying object with id, value 1278 * and extensions. The accessor "getUrl" gives direct access to the 1279 * value 1280 */ 1281 public MessageDefinition setUrlElement(UriType value) { 1282 this.url = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return The business identifier that is used to reference the 1288 * MessageDefinition and *is* expected to be consistent from server to 1289 * server. 1290 */ 1291 public String getUrl() { 1292 return this.url == null ? null : this.url.getValue(); 1293 } 1294 1295 /** 1296 * @param value The business identifier that is used to reference the 1297 * MessageDefinition and *is* expected to be consistent from server 1298 * to server. 1299 */ 1300 public MessageDefinition setUrl(String value) { 1301 if (Utilities.noString(value)) 1302 this.url = null; 1303 else { 1304 if (this.url == null) 1305 this.url = new UriType(); 1306 this.url.setValue(value); 1307 } 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #identifier} (A formal identifier that is used to identify 1313 * this message definition when it is represented in other formats, or 1314 * referenced in a specification, model, design or an instance.) 1315 */ 1316 public List<Identifier> getIdentifier() { 1317 if (this.identifier == null) 1318 this.identifier = new ArrayList<Identifier>(); 1319 return this.identifier; 1320 } 1321 1322 /** 1323 * @return Returns a reference to <code>this</code> for easy method chaining 1324 */ 1325 public MessageDefinition setIdentifier(List<Identifier> theIdentifier) { 1326 this.identifier = theIdentifier; 1327 return this; 1328 } 1329 1330 public boolean hasIdentifier() { 1331 if (this.identifier == null) 1332 return false; 1333 for (Identifier item : this.identifier) 1334 if (!item.isEmpty()) 1335 return true; 1336 return false; 1337 } 1338 1339 public Identifier addIdentifier() { // 3 1340 Identifier t = new Identifier(); 1341 if (this.identifier == null) 1342 this.identifier = new ArrayList<Identifier>(); 1343 this.identifier.add(t); 1344 return t; 1345 } 1346 1347 public MessageDefinition addIdentifier(Identifier t) { // 3 1348 if (t == null) 1349 return this; 1350 if (this.identifier == null) 1351 this.identifier = new ArrayList<Identifier>(); 1352 this.identifier.add(t); 1353 return this; 1354 } 1355 1356 /** 1357 * @return The first repetition of repeating field {@link #identifier}, creating 1358 * it if it does not already exist 1359 */ 1360 public Identifier getIdentifierFirstRep() { 1361 if (getIdentifier().isEmpty()) { 1362 addIdentifier(); 1363 } 1364 return getIdentifier().get(0); 1365 } 1366 1367 /** 1368 * @return {@link #version} (The identifier that is used to identify this 1369 * version of the message definition when it is referenced in a 1370 * specification, model, design or instance. This is an arbitrary value 1371 * managed by the message definition author and is not expected to be 1372 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 1373 * if a managed version is not available. There is also no expectation 1374 * that versions can be placed in a lexicographical sequence.). This is 1375 * the underlying object with id, value and extensions. The accessor 1376 * "getVersion" gives direct access to the value 1377 */ 1378 public StringType getVersionElement() { 1379 if (this.version == null) 1380 if (Configuration.errorOnAutoCreate()) 1381 throw new Error("Attempt to auto-create MessageDefinition.version"); 1382 else if (Configuration.doAutoCreate()) 1383 this.version = new StringType(); // bb 1384 return this.version; 1385 } 1386 1387 public boolean hasVersionElement() { 1388 return this.version != null && !this.version.isEmpty(); 1389 } 1390 1391 public boolean hasVersion() { 1392 return this.version != null && !this.version.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #version} (The identifier that is used to identify this 1397 * version of the message definition when it is referenced in a 1398 * specification, model, design or instance. This is an arbitrary 1399 * value managed by the message definition author and is not 1400 * expected to be globally unique. For example, it might be a 1401 * timestamp (e.g. yyyymmdd) if a managed version is not available. 1402 * There is also no expectation that versions can be placed in a 1403 * lexicographical sequence.). This is the underlying object with 1404 * id, value and extensions. The accessor "getVersion" gives direct 1405 * access to the value 1406 */ 1407 public MessageDefinition setVersionElement(StringType value) { 1408 this.version = value; 1409 return this; 1410 } 1411 1412 /** 1413 * @return The identifier that is used to identify this version of the message 1414 * definition when it is referenced in a specification, model, design or 1415 * instance. This is an arbitrary value managed by the message 1416 * definition author and is not expected to be globally unique. For 1417 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 1418 * is not available. There is also no expectation that versions can be 1419 * placed in a lexicographical sequence. 1420 */ 1421 public String getVersion() { 1422 return this.version == null ? null : this.version.getValue(); 1423 } 1424 1425 /** 1426 * @param value The identifier that is used to identify this version of the 1427 * message definition when it is referenced in a specification, 1428 * model, design or instance. This is an arbitrary value managed by 1429 * the message definition author and is not expected to be globally 1430 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 1431 * a managed version is not available. There is also no expectation 1432 * that versions can be placed in a lexicographical sequence. 1433 */ 1434 public MessageDefinition setVersion(String value) { 1435 if (Utilities.noString(value)) 1436 this.version = null; 1437 else { 1438 if (this.version == null) 1439 this.version = new StringType(); 1440 this.version.setValue(value); 1441 } 1442 return this; 1443 } 1444 1445 /** 1446 * @return {@link #name} (A natural language name identifying the message 1447 * definition. This name should be usable as an identifier for the 1448 * module by machine processing applications such as code generation.). 1449 * This is the underlying object with id, value and extensions. The 1450 * accessor "getName" gives direct access to the value 1451 */ 1452 public StringType getNameElement() { 1453 if (this.name == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create MessageDefinition.name"); 1456 else if (Configuration.doAutoCreate()) 1457 this.name = new StringType(); // bb 1458 return this.name; 1459 } 1460 1461 public boolean hasNameElement() { 1462 return this.name != null && !this.name.isEmpty(); 1463 } 1464 1465 public boolean hasName() { 1466 return this.name != null && !this.name.isEmpty(); 1467 } 1468 1469 /** 1470 * @param value {@link #name} (A natural language name identifying the message 1471 * definition. This name should be usable as an identifier for the 1472 * module by machine processing applications such as code 1473 * generation.). This is the underlying object with id, value and 1474 * extensions. The accessor "getName" gives direct access to the 1475 * value 1476 */ 1477 public MessageDefinition setNameElement(StringType value) { 1478 this.name = value; 1479 return this; 1480 } 1481 1482 /** 1483 * @return A natural language name identifying the message definition. This name 1484 * should be usable as an identifier for the module by machine 1485 * processing applications such as code generation. 1486 */ 1487 public String getName() { 1488 return this.name == null ? null : this.name.getValue(); 1489 } 1490 1491 /** 1492 * @param value A natural language name identifying the message definition. This 1493 * name should be usable as an identifier for the module by machine 1494 * processing applications such as code generation. 1495 */ 1496 public MessageDefinition setName(String value) { 1497 if (Utilities.noString(value)) 1498 this.name = null; 1499 else { 1500 if (this.name == null) 1501 this.name = new StringType(); 1502 this.name.setValue(value); 1503 } 1504 return this; 1505 } 1506 1507 /** 1508 * @return {@link #title} (A short, descriptive, user-friendly title for the 1509 * message definition.). This is the underlying object with id, value 1510 * and extensions. The accessor "getTitle" gives direct access to the 1511 * value 1512 */ 1513 public StringType getTitleElement() { 1514 if (this.title == null) 1515 if (Configuration.errorOnAutoCreate()) 1516 throw new Error("Attempt to auto-create MessageDefinition.title"); 1517 else if (Configuration.doAutoCreate()) 1518 this.title = new StringType(); // bb 1519 return this.title; 1520 } 1521 1522 public boolean hasTitleElement() { 1523 return this.title != null && !this.title.isEmpty(); 1524 } 1525 1526 public boolean hasTitle() { 1527 return this.title != null && !this.title.isEmpty(); 1528 } 1529 1530 /** 1531 * @param value {@link #title} (A short, descriptive, user-friendly title for 1532 * the message definition.). This is the underlying object with id, 1533 * value and extensions. The accessor "getTitle" gives direct 1534 * access to the value 1535 */ 1536 public MessageDefinition setTitleElement(StringType value) { 1537 this.title = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return A short, descriptive, user-friendly title for the message definition. 1543 */ 1544 public String getTitle() { 1545 return this.title == null ? null : this.title.getValue(); 1546 } 1547 1548 /** 1549 * @param value A short, descriptive, user-friendly title for the message 1550 * definition. 1551 */ 1552 public MessageDefinition setTitle(String value) { 1553 if (Utilities.noString(value)) 1554 this.title = null; 1555 else { 1556 if (this.title == null) 1557 this.title = new StringType(); 1558 this.title.setValue(value); 1559 } 1560 return this; 1561 } 1562 1563 /** 1564 * @return {@link #replaces} (A MessageDefinition that is superseded by this 1565 * definition.) 1566 */ 1567 public List<CanonicalType> getReplaces() { 1568 if (this.replaces == null) 1569 this.replaces = new ArrayList<CanonicalType>(); 1570 return this.replaces; 1571 } 1572 1573 /** 1574 * @return Returns a reference to <code>this</code> for easy method chaining 1575 */ 1576 public MessageDefinition setReplaces(List<CanonicalType> theReplaces) { 1577 this.replaces = theReplaces; 1578 return this; 1579 } 1580 1581 public boolean hasReplaces() { 1582 if (this.replaces == null) 1583 return false; 1584 for (CanonicalType item : this.replaces) 1585 if (!item.isEmpty()) 1586 return true; 1587 return false; 1588 } 1589 1590 /** 1591 * @return {@link #replaces} (A MessageDefinition that is superseded by this 1592 * definition.) 1593 */ 1594 public CanonicalType addReplacesElement() {// 2 1595 CanonicalType t = new CanonicalType(); 1596 if (this.replaces == null) 1597 this.replaces = new ArrayList<CanonicalType>(); 1598 this.replaces.add(t); 1599 return t; 1600 } 1601 1602 /** 1603 * @param value {@link #replaces} (A MessageDefinition that is superseded by 1604 * this definition.) 1605 */ 1606 public MessageDefinition addReplaces(String value) { // 1 1607 CanonicalType t = new CanonicalType(); 1608 t.setValue(value); 1609 if (this.replaces == null) 1610 this.replaces = new ArrayList<CanonicalType>(); 1611 this.replaces.add(t); 1612 return this; 1613 } 1614 1615 /** 1616 * @param value {@link #replaces} (A MessageDefinition that is superseded by 1617 * this definition.) 1618 */ 1619 public boolean hasReplaces(String value) { 1620 if (this.replaces == null) 1621 return false; 1622 for (CanonicalType v : this.replaces) 1623 if (v.getValue().equals(value)) // canonical(MessageDefinition) 1624 return true; 1625 return false; 1626 } 1627 1628 /** 1629 * @return {@link #status} (The status of this message definition. Enables 1630 * tracking the life-cycle of the content.). This is the underlying 1631 * object with id, value and extensions. The accessor "getStatus" gives 1632 * direct access to the value 1633 */ 1634 public Enumeration<PublicationStatus> getStatusElement() { 1635 if (this.status == null) 1636 if (Configuration.errorOnAutoCreate()) 1637 throw new Error("Attempt to auto-create MessageDefinition.status"); 1638 else if (Configuration.doAutoCreate()) 1639 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1640 return this.status; 1641 } 1642 1643 public boolean hasStatusElement() { 1644 return this.status != null && !this.status.isEmpty(); 1645 } 1646 1647 public boolean hasStatus() { 1648 return this.status != null && !this.status.isEmpty(); 1649 } 1650 1651 /** 1652 * @param value {@link #status} (The status of this message definition. Enables 1653 * tracking the life-cycle of the content.). This is the underlying 1654 * object with id, value and extensions. The accessor "getStatus" 1655 * gives direct access to the value 1656 */ 1657 public MessageDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1658 this.status = value; 1659 return this; 1660 } 1661 1662 /** 1663 * @return The status of this message definition. Enables tracking the 1664 * life-cycle of the content. 1665 */ 1666 public PublicationStatus getStatus() { 1667 return this.status == null ? null : this.status.getValue(); 1668 } 1669 1670 /** 1671 * @param value The status of this message definition. Enables tracking the 1672 * life-cycle of the content. 1673 */ 1674 public MessageDefinition setStatus(PublicationStatus value) { 1675 if (this.status == null) 1676 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1677 this.status.setValue(value); 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #experimental} (A Boolean value to indicate that this message 1683 * definition is authored for testing purposes (or 1684 * education/evaluation/marketing) and is not intended to be used for 1685 * genuine usage.). This is the underlying object with id, value and 1686 * extensions. The accessor "getExperimental" gives direct access to the 1687 * value 1688 */ 1689 public BooleanType getExperimentalElement() { 1690 if (this.experimental == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create MessageDefinition.experimental"); 1693 else if (Configuration.doAutoCreate()) 1694 this.experimental = new BooleanType(); // bb 1695 return this.experimental; 1696 } 1697 1698 public boolean hasExperimentalElement() { 1699 return this.experimental != null && !this.experimental.isEmpty(); 1700 } 1701 1702 public boolean hasExperimental() { 1703 return this.experimental != null && !this.experimental.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #experimental} (A Boolean value to indicate that this 1708 * message definition is authored for testing purposes (or 1709 * education/evaluation/marketing) and is not intended to be used 1710 * for genuine usage.). This is the underlying object with id, 1711 * value and extensions. The accessor "getExperimental" gives 1712 * direct access to the value 1713 */ 1714 public MessageDefinition setExperimentalElement(BooleanType value) { 1715 this.experimental = value; 1716 return this; 1717 } 1718 1719 /** 1720 * @return A Boolean value to indicate that this message definition is authored 1721 * for testing purposes (or education/evaluation/marketing) and is not 1722 * intended to be used for genuine usage. 1723 */ 1724 public boolean getExperimental() { 1725 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1726 } 1727 1728 /** 1729 * @param value A Boolean value to indicate that this message definition is 1730 * authored for testing purposes (or 1731 * education/evaluation/marketing) and is not intended to be used 1732 * for genuine usage. 1733 */ 1734 public MessageDefinition setExperimental(boolean value) { 1735 if (this.experimental == null) 1736 this.experimental = new BooleanType(); 1737 this.experimental.setValue(value); 1738 return this; 1739 } 1740 1741 /** 1742 * @return {@link #date} (The date (and optionally time) when the message 1743 * definition was published. The date must change when the business 1744 * version changes and it must change if the status code changes. In 1745 * addition, it should change when the substantive content of the 1746 * message definition changes.). This is the underlying object with id, 1747 * value and extensions. The accessor "getDate" gives direct access to 1748 * the value 1749 */ 1750 public DateTimeType getDateElement() { 1751 if (this.date == null) 1752 if (Configuration.errorOnAutoCreate()) 1753 throw new Error("Attempt to auto-create MessageDefinition.date"); 1754 else if (Configuration.doAutoCreate()) 1755 this.date = new DateTimeType(); // bb 1756 return this.date; 1757 } 1758 1759 public boolean hasDateElement() { 1760 return this.date != null && !this.date.isEmpty(); 1761 } 1762 1763 public boolean hasDate() { 1764 return this.date != null && !this.date.isEmpty(); 1765 } 1766 1767 /** 1768 * @param value {@link #date} (The date (and optionally time) when the message 1769 * definition was published. The date must change when the business 1770 * version changes and it must change if the status code changes. 1771 * In addition, it should change when the substantive content of 1772 * the message definition changes.). This is the underlying object 1773 * with id, value and extensions. The accessor "getDate" gives 1774 * direct access to the value 1775 */ 1776 public MessageDefinition setDateElement(DateTimeType value) { 1777 this.date = value; 1778 return this; 1779 } 1780 1781 /** 1782 * @return The date (and optionally time) when the message definition was 1783 * published. The date must change when the business version changes and 1784 * it must change if the status code changes. In addition, it should 1785 * change when the substantive content of the message definition 1786 * changes. 1787 */ 1788 public Date getDate() { 1789 return this.date == null ? null : this.date.getValue(); 1790 } 1791 1792 /** 1793 * @param value The date (and optionally time) when the message definition was 1794 * published. The date must change when the business version 1795 * changes and it must change if the status code changes. In 1796 * addition, it should change when the substantive content of the 1797 * message definition changes. 1798 */ 1799 public MessageDefinition setDate(Date value) { 1800 if (this.date == null) 1801 this.date = new DateTimeType(); 1802 this.date.setValue(value); 1803 return this; 1804 } 1805 1806 /** 1807 * @return {@link #publisher} (The name of the organization or individual that 1808 * published the message definition.). This is the underlying object 1809 * with id, value and extensions. The accessor "getPublisher" gives 1810 * direct access to the value 1811 */ 1812 public StringType getPublisherElement() { 1813 if (this.publisher == null) 1814 if (Configuration.errorOnAutoCreate()) 1815 throw new Error("Attempt to auto-create MessageDefinition.publisher"); 1816 else if (Configuration.doAutoCreate()) 1817 this.publisher = new StringType(); // bb 1818 return this.publisher; 1819 } 1820 1821 public boolean hasPublisherElement() { 1822 return this.publisher != null && !this.publisher.isEmpty(); 1823 } 1824 1825 public boolean hasPublisher() { 1826 return this.publisher != null && !this.publisher.isEmpty(); 1827 } 1828 1829 /** 1830 * @param value {@link #publisher} (The name of the organization or individual 1831 * that published the message definition.). This is the underlying 1832 * object with id, value and extensions. The accessor 1833 * "getPublisher" gives direct access to the value 1834 */ 1835 public MessageDefinition setPublisherElement(StringType value) { 1836 this.publisher = value; 1837 return this; 1838 } 1839 1840 /** 1841 * @return The name of the organization or individual that published the message 1842 * definition. 1843 */ 1844 public String getPublisher() { 1845 return this.publisher == null ? null : this.publisher.getValue(); 1846 } 1847 1848 /** 1849 * @param value The name of the organization or individual that published the 1850 * message definition. 1851 */ 1852 public MessageDefinition setPublisher(String value) { 1853 if (Utilities.noString(value)) 1854 this.publisher = null; 1855 else { 1856 if (this.publisher == null) 1857 this.publisher = new StringType(); 1858 this.publisher.setValue(value); 1859 } 1860 return this; 1861 } 1862 1863 /** 1864 * @return {@link #contact} (Contact details to assist a user in finding and 1865 * communicating with the publisher.) 1866 */ 1867 public List<ContactDetail> getContact() { 1868 if (this.contact == null) 1869 this.contact = new ArrayList<ContactDetail>(); 1870 return this.contact; 1871 } 1872 1873 /** 1874 * @return Returns a reference to <code>this</code> for easy method chaining 1875 */ 1876 public MessageDefinition setContact(List<ContactDetail> theContact) { 1877 this.contact = theContact; 1878 return this; 1879 } 1880 1881 public boolean hasContact() { 1882 if (this.contact == null) 1883 return false; 1884 for (ContactDetail item : this.contact) 1885 if (!item.isEmpty()) 1886 return true; 1887 return false; 1888 } 1889 1890 public ContactDetail addContact() { // 3 1891 ContactDetail t = new ContactDetail(); 1892 if (this.contact == null) 1893 this.contact = new ArrayList<ContactDetail>(); 1894 this.contact.add(t); 1895 return t; 1896 } 1897 1898 public MessageDefinition addContact(ContactDetail t) { // 3 1899 if (t == null) 1900 return this; 1901 if (this.contact == null) 1902 this.contact = new ArrayList<ContactDetail>(); 1903 this.contact.add(t); 1904 return this; 1905 } 1906 1907 /** 1908 * @return The first repetition of repeating field {@link #contact}, creating it 1909 * if it does not already exist 1910 */ 1911 public ContactDetail getContactFirstRep() { 1912 if (getContact().isEmpty()) { 1913 addContact(); 1914 } 1915 return getContact().get(0); 1916 } 1917 1918 /** 1919 * @return {@link #description} (A free text natural language description of the 1920 * message definition from a consumer's perspective.). This is the 1921 * underlying object with id, value and extensions. The accessor 1922 * "getDescription" gives direct access to the value 1923 */ 1924 public MarkdownType getDescriptionElement() { 1925 if (this.description == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create MessageDefinition.description"); 1928 else if (Configuration.doAutoCreate()) 1929 this.description = new MarkdownType(); // bb 1930 return this.description; 1931 } 1932 1933 public boolean hasDescriptionElement() { 1934 return this.description != null && !this.description.isEmpty(); 1935 } 1936 1937 public boolean hasDescription() { 1938 return this.description != null && !this.description.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #description} (A free text natural language description 1943 * of the message definition from a consumer's perspective.). This 1944 * is the underlying object with id, value and extensions. The 1945 * accessor "getDescription" gives direct access to the value 1946 */ 1947 public MessageDefinition setDescriptionElement(MarkdownType value) { 1948 this.description = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return A free text natural language description of the message definition 1954 * from a consumer's perspective. 1955 */ 1956 public String getDescription() { 1957 return this.description == null ? null : this.description.getValue(); 1958 } 1959 1960 /** 1961 * @param value A free text natural language description of the message 1962 * definition from a consumer's perspective. 1963 */ 1964 public MessageDefinition setDescription(String value) { 1965 if (value == null) 1966 this.description = null; 1967 else { 1968 if (this.description == null) 1969 this.description = new MarkdownType(); 1970 this.description.setValue(value); 1971 } 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #useContext} (The content was developed with a focus and 1977 * intent of supporting the contexts that are listed. These contexts may 1978 * be general categories (gender, age, ...) or may be references to 1979 * specific programs (insurance plans, studies, ...) and may be used to 1980 * assist with indexing and searching for appropriate message definition 1981 * instances.) 1982 */ 1983 public List<UsageContext> getUseContext() { 1984 if (this.useContext == null) 1985 this.useContext = new ArrayList<UsageContext>(); 1986 return this.useContext; 1987 } 1988 1989 /** 1990 * @return Returns a reference to <code>this</code> for easy method chaining 1991 */ 1992 public MessageDefinition setUseContext(List<UsageContext> theUseContext) { 1993 this.useContext = theUseContext; 1994 return this; 1995 } 1996 1997 public boolean hasUseContext() { 1998 if (this.useContext == null) 1999 return false; 2000 for (UsageContext item : this.useContext) 2001 if (!item.isEmpty()) 2002 return true; 2003 return false; 2004 } 2005 2006 public UsageContext addUseContext() { // 3 2007 UsageContext t = new UsageContext(); 2008 if (this.useContext == null) 2009 this.useContext = new ArrayList<UsageContext>(); 2010 this.useContext.add(t); 2011 return t; 2012 } 2013 2014 public MessageDefinition addUseContext(UsageContext t) { // 3 2015 if (t == null) 2016 return this; 2017 if (this.useContext == null) 2018 this.useContext = new ArrayList<UsageContext>(); 2019 this.useContext.add(t); 2020 return this; 2021 } 2022 2023 /** 2024 * @return The first repetition of repeating field {@link #useContext}, creating 2025 * it if it does not already exist 2026 */ 2027 public UsageContext getUseContextFirstRep() { 2028 if (getUseContext().isEmpty()) { 2029 addUseContext(); 2030 } 2031 return getUseContext().get(0); 2032 } 2033 2034 /** 2035 * @return {@link #jurisdiction} (A legal or geographic region in which the 2036 * message definition is intended to be used.) 2037 */ 2038 public List<CodeableConcept> getJurisdiction() { 2039 if (this.jurisdiction == null) 2040 this.jurisdiction = new ArrayList<CodeableConcept>(); 2041 return this.jurisdiction; 2042 } 2043 2044 /** 2045 * @return Returns a reference to <code>this</code> for easy method chaining 2046 */ 2047 public MessageDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2048 this.jurisdiction = theJurisdiction; 2049 return this; 2050 } 2051 2052 public boolean hasJurisdiction() { 2053 if (this.jurisdiction == null) 2054 return false; 2055 for (CodeableConcept item : this.jurisdiction) 2056 if (!item.isEmpty()) 2057 return true; 2058 return false; 2059 } 2060 2061 public CodeableConcept addJurisdiction() { // 3 2062 CodeableConcept t = new CodeableConcept(); 2063 if (this.jurisdiction == null) 2064 this.jurisdiction = new ArrayList<CodeableConcept>(); 2065 this.jurisdiction.add(t); 2066 return t; 2067 } 2068 2069 public MessageDefinition addJurisdiction(CodeableConcept t) { // 3 2070 if (t == null) 2071 return this; 2072 if (this.jurisdiction == null) 2073 this.jurisdiction = new ArrayList<CodeableConcept>(); 2074 this.jurisdiction.add(t); 2075 return this; 2076 } 2077 2078 /** 2079 * @return The first repetition of repeating field {@link #jurisdiction}, 2080 * creating it if it does not already exist 2081 */ 2082 public CodeableConcept getJurisdictionFirstRep() { 2083 if (getJurisdiction().isEmpty()) { 2084 addJurisdiction(); 2085 } 2086 return getJurisdiction().get(0); 2087 } 2088 2089 /** 2090 * @return {@link #purpose} (Explanation of why this message definition is 2091 * needed and why it has been designed as it has.). This is the 2092 * underlying object with id, value and extensions. The accessor 2093 * "getPurpose" gives direct access to the value 2094 */ 2095 public MarkdownType getPurposeElement() { 2096 if (this.purpose == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create MessageDefinition.purpose"); 2099 else if (Configuration.doAutoCreate()) 2100 this.purpose = new MarkdownType(); // bb 2101 return this.purpose; 2102 } 2103 2104 public boolean hasPurposeElement() { 2105 return this.purpose != null && !this.purpose.isEmpty(); 2106 } 2107 2108 public boolean hasPurpose() { 2109 return this.purpose != null && !this.purpose.isEmpty(); 2110 } 2111 2112 /** 2113 * @param value {@link #purpose} (Explanation of why this message definition is 2114 * needed and why it has been designed as it has.). This is the 2115 * underlying object with id, value and extensions. The accessor 2116 * "getPurpose" gives direct access to the value 2117 */ 2118 public MessageDefinition setPurposeElement(MarkdownType value) { 2119 this.purpose = value; 2120 return this; 2121 } 2122 2123 /** 2124 * @return Explanation of why this message definition is needed and why it has 2125 * been designed as it has. 2126 */ 2127 public String getPurpose() { 2128 return this.purpose == null ? null : this.purpose.getValue(); 2129 } 2130 2131 /** 2132 * @param value Explanation of why this message definition is needed and why it 2133 * has been designed as it has. 2134 */ 2135 public MessageDefinition setPurpose(String value) { 2136 if (value == null) 2137 this.purpose = null; 2138 else { 2139 if (this.purpose == null) 2140 this.purpose = new MarkdownType(); 2141 this.purpose.setValue(value); 2142 } 2143 return this; 2144 } 2145 2146 /** 2147 * @return {@link #copyright} (A copyright statement relating to the message 2148 * definition and/or its contents. Copyright statements are generally 2149 * legal restrictions on the use and publishing of the message 2150 * definition.). This is the underlying object with id, value and 2151 * extensions. The accessor "getCopyright" gives direct access to the 2152 * value 2153 */ 2154 public MarkdownType getCopyrightElement() { 2155 if (this.copyright == null) 2156 if (Configuration.errorOnAutoCreate()) 2157 throw new Error("Attempt to auto-create MessageDefinition.copyright"); 2158 else if (Configuration.doAutoCreate()) 2159 this.copyright = new MarkdownType(); // bb 2160 return this.copyright; 2161 } 2162 2163 public boolean hasCopyrightElement() { 2164 return this.copyright != null && !this.copyright.isEmpty(); 2165 } 2166 2167 public boolean hasCopyright() { 2168 return this.copyright != null && !this.copyright.isEmpty(); 2169 } 2170 2171 /** 2172 * @param value {@link #copyright} (A copyright statement relating to the 2173 * message definition and/or its contents. Copyright statements are 2174 * generally legal restrictions on the use and publishing of the 2175 * message definition.). This is the underlying object with id, 2176 * value and extensions. The accessor "getCopyright" gives direct 2177 * access to the value 2178 */ 2179 public MessageDefinition setCopyrightElement(MarkdownType value) { 2180 this.copyright = value; 2181 return this; 2182 } 2183 2184 /** 2185 * @return A copyright statement relating to the message definition and/or its 2186 * contents. Copyright statements are generally legal restrictions on 2187 * the use and publishing of the message definition. 2188 */ 2189 public String getCopyright() { 2190 return this.copyright == null ? null : this.copyright.getValue(); 2191 } 2192 2193 /** 2194 * @param value A copyright statement relating to the message definition and/or 2195 * its contents. Copyright statements are generally legal 2196 * restrictions on the use and publishing of the message 2197 * definition. 2198 */ 2199 public MessageDefinition setCopyright(String value) { 2200 if (value == null) 2201 this.copyright = null; 2202 else { 2203 if (this.copyright == null) 2204 this.copyright = new MarkdownType(); 2205 this.copyright.setValue(value); 2206 } 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #base} (The MessageDefinition that is the basis for the 2212 * contents of this resource.). This is the underlying object with id, 2213 * value and extensions. The accessor "getBase" gives direct access to 2214 * the value 2215 */ 2216 public CanonicalType getBaseElement() { 2217 if (this.base == null) 2218 if (Configuration.errorOnAutoCreate()) 2219 throw new Error("Attempt to auto-create MessageDefinition.base"); 2220 else if (Configuration.doAutoCreate()) 2221 this.base = new CanonicalType(); // bb 2222 return this.base; 2223 } 2224 2225 public boolean hasBaseElement() { 2226 return this.base != null && !this.base.isEmpty(); 2227 } 2228 2229 public boolean hasBase() { 2230 return this.base != null && !this.base.isEmpty(); 2231 } 2232 2233 /** 2234 * @param value {@link #base} (The MessageDefinition that is the basis for the 2235 * contents of this resource.). This is the underlying object with 2236 * id, value and extensions. The accessor "getBase" gives direct 2237 * access to the value 2238 */ 2239 public MessageDefinition setBaseElement(CanonicalType value) { 2240 this.base = value; 2241 return this; 2242 } 2243 2244 /** 2245 * @return The MessageDefinition that is the basis for the contents of this 2246 * resource. 2247 */ 2248 public String getBase() { 2249 return this.base == null ? null : this.base.getValue(); 2250 } 2251 2252 /** 2253 * @param value The MessageDefinition that is the basis for the contents of this 2254 * resource. 2255 */ 2256 public MessageDefinition setBase(String value) { 2257 if (Utilities.noString(value)) 2258 this.base = null; 2259 else { 2260 if (this.base == null) 2261 this.base = new CanonicalType(); 2262 this.base.setValue(value); 2263 } 2264 return this; 2265 } 2266 2267 /** 2268 * @return {@link #parent} (Identifies a protocol or workflow that this 2269 * MessageDefinition represents a step in.) 2270 */ 2271 public List<CanonicalType> getParent() { 2272 if (this.parent == null) 2273 this.parent = new ArrayList<CanonicalType>(); 2274 return this.parent; 2275 } 2276 2277 /** 2278 * @return Returns a reference to <code>this</code> for easy method chaining 2279 */ 2280 public MessageDefinition setParent(List<CanonicalType> theParent) { 2281 this.parent = theParent; 2282 return this; 2283 } 2284 2285 public boolean hasParent() { 2286 if (this.parent == null) 2287 return false; 2288 for (CanonicalType item : this.parent) 2289 if (!item.isEmpty()) 2290 return true; 2291 return false; 2292 } 2293 2294 /** 2295 * @return {@link #parent} (Identifies a protocol or workflow that this 2296 * MessageDefinition represents a step in.) 2297 */ 2298 public CanonicalType addParentElement() {// 2 2299 CanonicalType t = new CanonicalType(); 2300 if (this.parent == null) 2301 this.parent = new ArrayList<CanonicalType>(); 2302 this.parent.add(t); 2303 return t; 2304 } 2305 2306 /** 2307 * @param value {@link #parent} (Identifies a protocol or workflow that this 2308 * MessageDefinition represents a step in.) 2309 */ 2310 public MessageDefinition addParent(String value) { // 1 2311 CanonicalType t = new CanonicalType(); 2312 t.setValue(value); 2313 if (this.parent == null) 2314 this.parent = new ArrayList<CanonicalType>(); 2315 this.parent.add(t); 2316 return this; 2317 } 2318 2319 /** 2320 * @param value {@link #parent} (Identifies a protocol or workflow that this 2321 * MessageDefinition represents a step in.) 2322 */ 2323 public boolean hasParent(String value) { 2324 if (this.parent == null) 2325 return false; 2326 for (CanonicalType v : this.parent) 2327 if (v.getValue().equals(value)) // canonical(ActivityDefinition|PlanDefinition) 2328 return true; 2329 return false; 2330 } 2331 2332 /** 2333 * @return {@link #event} (Event code or link to the EventDefinition.) 2334 */ 2335 public Type getEvent() { 2336 return this.event; 2337 } 2338 2339 /** 2340 * @return {@link #event} (Event code or link to the EventDefinition.) 2341 */ 2342 public Coding getEventCoding() throws FHIRException { 2343 if (this.event == null) 2344 this.event = new Coding(); 2345 if (!(this.event instanceof Coding)) 2346 throw new FHIRException( 2347 "Type mismatch: the type Coding was expected, but " + this.event.getClass().getName() + " was encountered"); 2348 return (Coding) this.event; 2349 } 2350 2351 public boolean hasEventCoding() { 2352 return this != null && this.event instanceof Coding; 2353 } 2354 2355 /** 2356 * @return {@link #event} (Event code or link to the EventDefinition.) 2357 */ 2358 public UriType getEventUriType() throws FHIRException { 2359 if (this.event == null) 2360 this.event = new UriType(); 2361 if (!(this.event instanceof UriType)) 2362 throw new FHIRException( 2363 "Type mismatch: the type UriType was expected, but " + this.event.getClass().getName() + " was encountered"); 2364 return (UriType) this.event; 2365 } 2366 2367 public boolean hasEventUriType() { 2368 return this != null && this.event instanceof UriType; 2369 } 2370 2371 public boolean hasEvent() { 2372 return this.event != null && !this.event.isEmpty(); 2373 } 2374 2375 /** 2376 * @param value {@link #event} (Event code or link to the EventDefinition.) 2377 */ 2378 public MessageDefinition setEvent(Type value) { 2379 if (value != null && !(value instanceof Coding || value instanceof UriType)) 2380 throw new Error("Not the right type for MessageDefinition.event[x]: " + value.fhirType()); 2381 this.event = value; 2382 return this; 2383 } 2384 2385 /** 2386 * @return {@link #category} (The impact of the content of the message.). This 2387 * is the underlying object with id, value and extensions. The accessor 2388 * "getCategory" gives direct access to the value 2389 */ 2390 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 2391 if (this.category == null) 2392 if (Configuration.errorOnAutoCreate()) 2393 throw new Error("Attempt to auto-create MessageDefinition.category"); 2394 else if (Configuration.doAutoCreate()) 2395 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 2396 return this.category; 2397 } 2398 2399 public boolean hasCategoryElement() { 2400 return this.category != null && !this.category.isEmpty(); 2401 } 2402 2403 public boolean hasCategory() { 2404 return this.category != null && !this.category.isEmpty(); 2405 } 2406 2407 /** 2408 * @param value {@link #category} (The impact of the content of the message.). 2409 * This is the underlying object with id, value and extensions. The 2410 * accessor "getCategory" gives direct access to the value 2411 */ 2412 public MessageDefinition setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 2413 this.category = value; 2414 return this; 2415 } 2416 2417 /** 2418 * @return The impact of the content of the message. 2419 */ 2420 public MessageSignificanceCategory getCategory() { 2421 return this.category == null ? null : this.category.getValue(); 2422 } 2423 2424 /** 2425 * @param value The impact of the content of the message. 2426 */ 2427 public MessageDefinition setCategory(MessageSignificanceCategory value) { 2428 if (value == null) 2429 this.category = null; 2430 else { 2431 if (this.category == null) 2432 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 2433 this.category.setValue(value); 2434 } 2435 return this; 2436 } 2437 2438 /** 2439 * @return {@link #focus} (Identifies the resource (or resources) that are being 2440 * addressed by the event. For example, the Encounter for an admit 2441 * message or two Account records for a merge.) 2442 */ 2443 public List<MessageDefinitionFocusComponent> getFocus() { 2444 if (this.focus == null) 2445 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2446 return this.focus; 2447 } 2448 2449 /** 2450 * @return Returns a reference to <code>this</code> for easy method chaining 2451 */ 2452 public MessageDefinition setFocus(List<MessageDefinitionFocusComponent> theFocus) { 2453 this.focus = theFocus; 2454 return this; 2455 } 2456 2457 public boolean hasFocus() { 2458 if (this.focus == null) 2459 return false; 2460 for (MessageDefinitionFocusComponent item : this.focus) 2461 if (!item.isEmpty()) 2462 return true; 2463 return false; 2464 } 2465 2466 public MessageDefinitionFocusComponent addFocus() { // 3 2467 MessageDefinitionFocusComponent t = new MessageDefinitionFocusComponent(); 2468 if (this.focus == null) 2469 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2470 this.focus.add(t); 2471 return t; 2472 } 2473 2474 public MessageDefinition addFocus(MessageDefinitionFocusComponent t) { // 3 2475 if (t == null) 2476 return this; 2477 if (this.focus == null) 2478 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2479 this.focus.add(t); 2480 return this; 2481 } 2482 2483 /** 2484 * @return The first repetition of repeating field {@link #focus}, creating it 2485 * if it does not already exist 2486 */ 2487 public MessageDefinitionFocusComponent getFocusFirstRep() { 2488 if (getFocus().isEmpty()) { 2489 addFocus(); 2490 } 2491 return getFocus().get(0); 2492 } 2493 2494 /** 2495 * @return {@link #responseRequired} (Declare at a message definition level 2496 * whether a response is required or only upon error or success, or 2497 * never.). This is the underlying object with id, value and extensions. 2498 * The accessor "getResponseRequired" gives direct access to the value 2499 */ 2500 public Enumeration<MessageheaderResponseRequest> getResponseRequiredElement() { 2501 if (this.responseRequired == null) 2502 if (Configuration.errorOnAutoCreate()) 2503 throw new Error("Attempt to auto-create MessageDefinition.responseRequired"); 2504 else if (Configuration.doAutoCreate()) 2505 this.responseRequired = new Enumeration<MessageheaderResponseRequest>( 2506 new MessageheaderResponseRequestEnumFactory()); // bb 2507 return this.responseRequired; 2508 } 2509 2510 public boolean hasResponseRequiredElement() { 2511 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2512 } 2513 2514 public boolean hasResponseRequired() { 2515 return this.responseRequired != null && !this.responseRequired.isEmpty(); 2516 } 2517 2518 /** 2519 * @param value {@link #responseRequired} (Declare at a message definition level 2520 * whether a response is required or only upon error or success, or 2521 * never.). This is the underlying object with id, value and 2522 * extensions. The accessor "getResponseRequired" gives direct 2523 * access to the value 2524 */ 2525 public MessageDefinition setResponseRequiredElement(Enumeration<MessageheaderResponseRequest> value) { 2526 this.responseRequired = value; 2527 return this; 2528 } 2529 2530 /** 2531 * @return Declare at a message definition level whether a response is required 2532 * or only upon error or success, or never. 2533 */ 2534 public MessageheaderResponseRequest getResponseRequired() { 2535 return this.responseRequired == null ? null : this.responseRequired.getValue(); 2536 } 2537 2538 /** 2539 * @param value Declare at a message definition level whether a response is 2540 * required or only upon error or success, or never. 2541 */ 2542 public MessageDefinition setResponseRequired(MessageheaderResponseRequest value) { 2543 if (value == null) 2544 this.responseRequired = null; 2545 else { 2546 if (this.responseRequired == null) 2547 this.responseRequired = new Enumeration<MessageheaderResponseRequest>( 2548 new MessageheaderResponseRequestEnumFactory()); 2549 this.responseRequired.setValue(value); 2550 } 2551 return this; 2552 } 2553 2554 /** 2555 * @return {@link #allowedResponse} (Indicates what types of messages may be 2556 * sent as an application-level response to this message.) 2557 */ 2558 public List<MessageDefinitionAllowedResponseComponent> getAllowedResponse() { 2559 if (this.allowedResponse == null) 2560 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2561 return this.allowedResponse; 2562 } 2563 2564 /** 2565 * @return Returns a reference to <code>this</code> for easy method chaining 2566 */ 2567 public MessageDefinition setAllowedResponse(List<MessageDefinitionAllowedResponseComponent> theAllowedResponse) { 2568 this.allowedResponse = theAllowedResponse; 2569 return this; 2570 } 2571 2572 public boolean hasAllowedResponse() { 2573 if (this.allowedResponse == null) 2574 return false; 2575 for (MessageDefinitionAllowedResponseComponent item : this.allowedResponse) 2576 if (!item.isEmpty()) 2577 return true; 2578 return false; 2579 } 2580 2581 public MessageDefinitionAllowedResponseComponent addAllowedResponse() { // 3 2582 MessageDefinitionAllowedResponseComponent t = new MessageDefinitionAllowedResponseComponent(); 2583 if (this.allowedResponse == null) 2584 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2585 this.allowedResponse.add(t); 2586 return t; 2587 } 2588 2589 public MessageDefinition addAllowedResponse(MessageDefinitionAllowedResponseComponent t) { // 3 2590 if (t == null) 2591 return this; 2592 if (this.allowedResponse == null) 2593 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2594 this.allowedResponse.add(t); 2595 return this; 2596 } 2597 2598 /** 2599 * @return The first repetition of repeating field {@link #allowedResponse}, 2600 * creating it if it does not already exist 2601 */ 2602 public MessageDefinitionAllowedResponseComponent getAllowedResponseFirstRep() { 2603 if (getAllowedResponse().isEmpty()) { 2604 addAllowedResponse(); 2605 } 2606 return getAllowedResponse().get(0); 2607 } 2608 2609 /** 2610 * @return {@link #graph} (Canonical reference to a GraphDefinition. If a URL is 2611 * provided, it is the canonical reference to a [[[GraphDefinition]]] 2612 * that it controls what resources are to be added to the bundle when 2613 * building the document. The GraphDefinition can also specify profiles 2614 * that apply to the various resources.) 2615 */ 2616 public List<CanonicalType> getGraph() { 2617 if (this.graph == null) 2618 this.graph = new ArrayList<CanonicalType>(); 2619 return this.graph; 2620 } 2621 2622 /** 2623 * @return Returns a reference to <code>this</code> for easy method chaining 2624 */ 2625 public MessageDefinition setGraph(List<CanonicalType> theGraph) { 2626 this.graph = theGraph; 2627 return this; 2628 } 2629 2630 public boolean hasGraph() { 2631 if (this.graph == null) 2632 return false; 2633 for (CanonicalType item : this.graph) 2634 if (!item.isEmpty()) 2635 return true; 2636 return false; 2637 } 2638 2639 /** 2640 * @return {@link #graph} (Canonical reference to a GraphDefinition. If a URL is 2641 * provided, it is the canonical reference to a [[[GraphDefinition]]] 2642 * that it controls what resources are to be added to the bundle when 2643 * building the document. The GraphDefinition can also specify profiles 2644 * that apply to the various resources.) 2645 */ 2646 public CanonicalType addGraphElement() {// 2 2647 CanonicalType t = new CanonicalType(); 2648 if (this.graph == null) 2649 this.graph = new ArrayList<CanonicalType>(); 2650 this.graph.add(t); 2651 return t; 2652 } 2653 2654 /** 2655 * @param value {@link #graph} (Canonical reference to a GraphDefinition. If a 2656 * URL is provided, it is the canonical reference to a 2657 * [[[GraphDefinition]]] that it controls what resources are to be 2658 * added to the bundle when building the document. The 2659 * GraphDefinition can also specify profiles that apply to the 2660 * various resources.) 2661 */ 2662 public MessageDefinition addGraph(String value) { // 1 2663 CanonicalType t = new CanonicalType(); 2664 t.setValue(value); 2665 if (this.graph == null) 2666 this.graph = new ArrayList<CanonicalType>(); 2667 this.graph.add(t); 2668 return this; 2669 } 2670 2671 /** 2672 * @param value {@link #graph} (Canonical reference to a GraphDefinition. If a 2673 * URL is provided, it is the canonical reference to a 2674 * [[[GraphDefinition]]] that it controls what resources are to be 2675 * added to the bundle when building the document. The 2676 * GraphDefinition can also specify profiles that apply to the 2677 * various resources.) 2678 */ 2679 public boolean hasGraph(String value) { 2680 if (this.graph == null) 2681 return false; 2682 for (CanonicalType v : this.graph) 2683 if (v.getValue().equals(value)) // canonical(GraphDefinition) 2684 return true; 2685 return false; 2686 } 2687 2688 protected void listChildren(List<Property> children) { 2689 super.listChildren(children); 2690 children.add(new Property("url", "uri", 2691 "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 2692 0, 1, url)); 2693 children.add(new Property("identifier", "Identifier", 2694 "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2695 0, java.lang.Integer.MAX_VALUE, identifier)); 2696 children.add(new Property("version", "string", 2697 "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2698 0, 1, version)); 2699 children.add(new Property("name", "string", 2700 "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2701 0, 1, name)); 2702 children.add(new Property("title", "string", 2703 "A short, descriptive, user-friendly title for the message definition.", 0, 1, title)); 2704 children.add(new Property("replaces", "canonical(MessageDefinition)", 2705 "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2706 children.add(new Property("status", "code", 2707 "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2708 children.add(new Property("experimental", "boolean", 2709 "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2710 0, 1, experimental)); 2711 children.add(new Property("date", "dateTime", 2712 "The date (and optionally time) when the message definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 2713 0, 1, date)); 2714 children.add(new Property("publisher", "string", 2715 "The name of the organization or individual that published the message definition.", 0, 1, publisher)); 2716 children.add(new Property("contact", "ContactDetail", 2717 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2718 java.lang.Integer.MAX_VALUE, contact)); 2719 children.add(new Property("description", "markdown", 2720 "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, 2721 description)); 2722 children.add(new Property("useContext", "UsageContext", 2723 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 2724 0, java.lang.Integer.MAX_VALUE, useContext)); 2725 children.add(new Property("jurisdiction", "CodeableConcept", 2726 "A legal or geographic region in which the message definition is intended to be used.", 0, 2727 java.lang.Integer.MAX_VALUE, jurisdiction)); 2728 children.add(new Property("purpose", "markdown", 2729 "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2730 children.add(new Property("copyright", "markdown", 2731 "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 2732 0, 1, copyright)); 2733 children.add(new Property("base", "canonical(MessageDefinition)", 2734 "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base)); 2735 children.add(new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", 2736 "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, 2737 java.lang.Integer.MAX_VALUE, parent)); 2738 children.add(new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, event)); 2739 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 2740 children.add(new Property("focus", "", 2741 "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 2742 0, java.lang.Integer.MAX_VALUE, focus)); 2743 children.add(new Property("responseRequired", "code", 2744 "Declare at a message definition level whether a response is required or only upon error or success, or never.", 2745 0, 1, responseRequired)); 2746 children.add(new Property("allowedResponse", "", 2747 "Indicates what types of messages may be sent as an application-level response to this message.", 0, 2748 java.lang.Integer.MAX_VALUE, allowedResponse)); 2749 children.add(new Property("graph", "canonical(GraphDefinition)", 2750 "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.", 2751 0, java.lang.Integer.MAX_VALUE, graph)); 2752 } 2753 2754 @Override 2755 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2756 switch (_hash) { 2757 case 116079: 2758 /* url */ return new Property("url", "uri", 2759 "The business identifier that is used to reference the MessageDefinition and *is* expected to be consistent from server to server.", 2760 0, 1, url); 2761 case -1618432855: 2762 /* identifier */ return new Property("identifier", "Identifier", 2763 "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2764 0, java.lang.Integer.MAX_VALUE, identifier); 2765 case 351608024: 2766 /* version */ return new Property("version", "string", 2767 "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 2768 0, 1, version); 2769 case 3373707: 2770 /* name */ return new Property("name", "string", 2771 "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2772 0, 1, name); 2773 case 110371416: 2774 /* title */ return new Property("title", "string", 2775 "A short, descriptive, user-friendly title for the message definition.", 0, 1, title); 2776 case -430332865: 2777 /* replaces */ return new Property("replaces", "canonical(MessageDefinition)", 2778 "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces); 2779 case -892481550: 2780 /* status */ return new Property("status", "code", 2781 "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2782 case -404562712: 2783 /* experimental */ return new Property("experimental", "boolean", 2784 "A Boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 2785 0, 1, experimental); 2786 case 3076014: 2787 /* date */ return new Property("date", "dateTime", 2788 "The date (and optionally time) when the message definition was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 2789 0, 1, date); 2790 case 1447404028: 2791 /* publisher */ return new Property("publisher", "string", 2792 "The name of the organization or individual that published the message definition.", 0, 1, publisher); 2793 case 951526432: 2794 /* contact */ return new Property("contact", "ContactDetail", 2795 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2796 java.lang.Integer.MAX_VALUE, contact); 2797 case -1724546052: 2798 /* description */ return new Property("description", "markdown", 2799 "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, 2800 description); 2801 case -669707736: 2802 /* useContext */ return new Property("useContext", "UsageContext", 2803 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate message definition instances.", 2804 0, java.lang.Integer.MAX_VALUE, useContext); 2805 case -507075711: 2806 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2807 "A legal or geographic region in which the message definition is intended to be used.", 0, 2808 java.lang.Integer.MAX_VALUE, jurisdiction); 2809 case -220463842: 2810 /* purpose */ return new Property("purpose", "markdown", 2811 "Explanation of why this message definition is needed and why it has been designed as it has.", 0, 1, 2812 purpose); 2813 case 1522889671: 2814 /* copyright */ return new Property("copyright", "markdown", 2815 "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 2816 0, 1, copyright); 2817 case 3016401: 2818 /* base */ return new Property("base", "canonical(MessageDefinition)", 2819 "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base); 2820 case -995424086: 2821 /* parent */ return new Property("parent", "canonical(ActivityDefinition|PlanDefinition)", 2822 "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, 2823 java.lang.Integer.MAX_VALUE, parent); 2824 case 278115238: 2825 /* event[x] */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2826 event); 2827 case 96891546: 2828 /* event */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2829 event); 2830 case -355957084: 2831 /* eventCoding */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 2832 1, event); 2833 case 278109298: 2834 /* eventUri */ return new Property("event[x]", "Coding|uri", "Event code or link to the EventDefinition.", 0, 1, 2835 event); 2836 case 50511102: 2837 /* category */ return new Property("category", "code", "The impact of the content of the message.", 0, 1, 2838 category); 2839 case 97604824: 2840 /* focus */ return new Property("focus", "", 2841 "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 2842 0, java.lang.Integer.MAX_VALUE, focus); 2843 case 791597824: 2844 /* responseRequired */ return new Property("responseRequired", "code", 2845 "Declare at a message definition level whether a response is required or only upon error or success, or never.", 2846 0, 1, responseRequired); 2847 case -1130933751: 2848 /* allowedResponse */ return new Property("allowedResponse", "", 2849 "Indicates what types of messages may be sent as an application-level response to this message.", 0, 2850 java.lang.Integer.MAX_VALUE, allowedResponse); 2851 case 98615630: 2852 /* graph */ return new Property("graph", "canonical(GraphDefinition)", 2853 "Canonical reference to a GraphDefinition. If a URL is provided, it is the canonical reference to a [[[GraphDefinition]]] that it controls what resources are to be added to the bundle when building the document. The GraphDefinition can also specify profiles that apply to the various resources.", 2854 0, java.lang.Integer.MAX_VALUE, graph); 2855 default: 2856 return super.getNamedProperty(_hash, _name, _checkValid); 2857 } 2858 2859 } 2860 2861 @Override 2862 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2863 switch (hash) { 2864 case 116079: 2865 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2866 case -1618432855: 2867 /* identifier */ return this.identifier == null ? new Base[0] 2868 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2869 case 351608024: 2870 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2871 case 3373707: 2872 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2873 case 110371416: 2874 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2875 case -430332865: 2876 /* replaces */ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // CanonicalType 2877 case -892481550: 2878 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2879 case -404562712: 2880 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 2881 case 3076014: 2882 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2883 case 1447404028: 2884 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2885 case 951526432: 2886 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2887 case -1724546052: 2888 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2889 case -669707736: 2890 /* useContext */ return this.useContext == null ? new Base[0] 2891 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2892 case -507075711: 2893 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2894 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2895 case -220463842: 2896 /* purpose */ return this.purpose == null ? new Base[0] : new Base[] { this.purpose }; // MarkdownType 2897 case 1522889671: 2898 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2899 case 3016401: 2900 /* base */ return this.base == null ? new Base[0] : new Base[] { this.base }; // CanonicalType 2901 case -995424086: 2902 /* parent */ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // CanonicalType 2903 case 96891546: 2904 /* event */ return this.event == null ? new Base[0] : new Base[] { this.event }; // Type 2905 case 50511102: 2906 /* category */ return this.category == null ? new Base[0] : new Base[] { this.category }; // Enumeration<MessageSignificanceCategory> 2907 case 97604824: 2908 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // MessageDefinitionFocusComponent 2909 case 791597824: 2910 /* responseRequired */ return this.responseRequired == null ? new Base[0] : new Base[] { this.responseRequired }; // Enumeration<MessageheaderResponseRequest> 2911 case -1130933751: 2912 /* allowedResponse */ return this.allowedResponse == null ? new Base[0] 2913 : this.allowedResponse.toArray(new Base[this.allowedResponse.size()]); // MessageDefinitionAllowedResponseComponent 2914 case 98615630: 2915 /* graph */ return this.graph == null ? new Base[0] : this.graph.toArray(new Base[this.graph.size()]); // CanonicalType 2916 default: 2917 return super.getProperty(hash, name, checkValid); 2918 } 2919 2920 } 2921 2922 @Override 2923 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2924 switch (hash) { 2925 case 116079: // url 2926 this.url = castToUri(value); // UriType 2927 return value; 2928 case -1618432855: // identifier 2929 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2930 return value; 2931 case 351608024: // version 2932 this.version = castToString(value); // StringType 2933 return value; 2934 case 3373707: // name 2935 this.name = castToString(value); // StringType 2936 return value; 2937 case 110371416: // title 2938 this.title = castToString(value); // StringType 2939 return value; 2940 case -430332865: // replaces 2941 this.getReplaces().add(castToCanonical(value)); // CanonicalType 2942 return value; 2943 case -892481550: // status 2944 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2945 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2946 return value; 2947 case -404562712: // experimental 2948 this.experimental = castToBoolean(value); // BooleanType 2949 return value; 2950 case 3076014: // date 2951 this.date = castToDateTime(value); // DateTimeType 2952 return value; 2953 case 1447404028: // publisher 2954 this.publisher = castToString(value); // StringType 2955 return value; 2956 case 951526432: // contact 2957 this.getContact().add(castToContactDetail(value)); // ContactDetail 2958 return value; 2959 case -1724546052: // description 2960 this.description = castToMarkdown(value); // MarkdownType 2961 return value; 2962 case -669707736: // useContext 2963 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2964 return value; 2965 case -507075711: // jurisdiction 2966 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2967 return value; 2968 case -220463842: // purpose 2969 this.purpose = castToMarkdown(value); // MarkdownType 2970 return value; 2971 case 1522889671: // copyright 2972 this.copyright = castToMarkdown(value); // MarkdownType 2973 return value; 2974 case 3016401: // base 2975 this.base = castToCanonical(value); // CanonicalType 2976 return value; 2977 case -995424086: // parent 2978 this.getParent().add(castToCanonical(value)); // CanonicalType 2979 return value; 2980 case 96891546: // event 2981 this.event = castToType(value); // Type 2982 return value; 2983 case 50511102: // category 2984 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 2985 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2986 return value; 2987 case 97604824: // focus 2988 this.getFocus().add((MessageDefinitionFocusComponent) value); // MessageDefinitionFocusComponent 2989 return value; 2990 case 791597824: // responseRequired 2991 value = new MessageheaderResponseRequestEnumFactory().fromType(castToCode(value)); 2992 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 2993 return value; 2994 case -1130933751: // allowedResponse 2995 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); // MessageDefinitionAllowedResponseComponent 2996 return value; 2997 case 98615630: // graph 2998 this.getGraph().add(castToCanonical(value)); // CanonicalType 2999 return value; 3000 default: 3001 return super.setProperty(hash, name, value); 3002 } 3003 3004 } 3005 3006 @Override 3007 public Base setProperty(String name, Base value) throws FHIRException { 3008 if (name.equals("url")) { 3009 this.url = castToUri(value); // UriType 3010 } else if (name.equals("identifier")) { 3011 this.getIdentifier().add(castToIdentifier(value)); 3012 } else if (name.equals("version")) { 3013 this.version = castToString(value); // StringType 3014 } else if (name.equals("name")) { 3015 this.name = castToString(value); // StringType 3016 } else if (name.equals("title")) { 3017 this.title = castToString(value); // StringType 3018 } else if (name.equals("replaces")) { 3019 this.getReplaces().add(castToCanonical(value)); 3020 } else if (name.equals("status")) { 3021 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3022 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3023 } else if (name.equals("experimental")) { 3024 this.experimental = castToBoolean(value); // BooleanType 3025 } else if (name.equals("date")) { 3026 this.date = castToDateTime(value); // DateTimeType 3027 } else if (name.equals("publisher")) { 3028 this.publisher = castToString(value); // StringType 3029 } else if (name.equals("contact")) { 3030 this.getContact().add(castToContactDetail(value)); 3031 } else if (name.equals("description")) { 3032 this.description = castToMarkdown(value); // MarkdownType 3033 } else if (name.equals("useContext")) { 3034 this.getUseContext().add(castToUsageContext(value)); 3035 } else if (name.equals("jurisdiction")) { 3036 this.getJurisdiction().add(castToCodeableConcept(value)); 3037 } else if (name.equals("purpose")) { 3038 this.purpose = castToMarkdown(value); // MarkdownType 3039 } else if (name.equals("copyright")) { 3040 this.copyright = castToMarkdown(value); // MarkdownType 3041 } else if (name.equals("base")) { 3042 this.base = castToCanonical(value); // CanonicalType 3043 } else if (name.equals("parent")) { 3044 this.getParent().add(castToCanonical(value)); 3045 } else if (name.equals("event[x]")) { 3046 this.event = castToType(value); // Type 3047 } else if (name.equals("category")) { 3048 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 3049 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 3050 } else if (name.equals("focus")) { 3051 this.getFocus().add((MessageDefinitionFocusComponent) value); 3052 } else if (name.equals("responseRequired")) { 3053 value = new MessageheaderResponseRequestEnumFactory().fromType(castToCode(value)); 3054 this.responseRequired = (Enumeration) value; // Enumeration<MessageheaderResponseRequest> 3055 } else if (name.equals("allowedResponse")) { 3056 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); 3057 } else if (name.equals("graph")) { 3058 this.getGraph().add(castToCanonical(value)); 3059 } else 3060 return super.setProperty(name, value); 3061 return value; 3062 } 3063 3064 @Override 3065 public Base makeProperty(int hash, String name) throws FHIRException { 3066 switch (hash) { 3067 case 116079: 3068 return getUrlElement(); 3069 case -1618432855: 3070 return addIdentifier(); 3071 case 351608024: 3072 return getVersionElement(); 3073 case 3373707: 3074 return getNameElement(); 3075 case 110371416: 3076 return getTitleElement(); 3077 case -430332865: 3078 return addReplacesElement(); 3079 case -892481550: 3080 return getStatusElement(); 3081 case -404562712: 3082 return getExperimentalElement(); 3083 case 3076014: 3084 return getDateElement(); 3085 case 1447404028: 3086 return getPublisherElement(); 3087 case 951526432: 3088 return addContact(); 3089 case -1724546052: 3090 return getDescriptionElement(); 3091 case -669707736: 3092 return addUseContext(); 3093 case -507075711: 3094 return addJurisdiction(); 3095 case -220463842: 3096 return getPurposeElement(); 3097 case 1522889671: 3098 return getCopyrightElement(); 3099 case 3016401: 3100 return getBaseElement(); 3101 case -995424086: 3102 return addParentElement(); 3103 case 278115238: 3104 return getEvent(); 3105 case 96891546: 3106 return getEvent(); 3107 case 50511102: 3108 return getCategoryElement(); 3109 case 97604824: 3110 return addFocus(); 3111 case 791597824: 3112 return getResponseRequiredElement(); 3113 case -1130933751: 3114 return addAllowedResponse(); 3115 case 98615630: 3116 return addGraphElement(); 3117 default: 3118 return super.makeProperty(hash, name); 3119 } 3120 3121 } 3122 3123 @Override 3124 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3125 switch (hash) { 3126 case 116079: 3127 /* url */ return new String[] { "uri" }; 3128 case -1618432855: 3129 /* identifier */ return new String[] { "Identifier" }; 3130 case 351608024: 3131 /* version */ return new String[] { "string" }; 3132 case 3373707: 3133 /* name */ return new String[] { "string" }; 3134 case 110371416: 3135 /* title */ return new String[] { "string" }; 3136 case -430332865: 3137 /* replaces */ return new String[] { "canonical" }; 3138 case -892481550: 3139 /* status */ return new String[] { "code" }; 3140 case -404562712: 3141 /* experimental */ return new String[] { "boolean" }; 3142 case 3076014: 3143 /* date */ return new String[] { "dateTime" }; 3144 case 1447404028: 3145 /* publisher */ return new String[] { "string" }; 3146 case 951526432: 3147 /* contact */ return new String[] { "ContactDetail" }; 3148 case -1724546052: 3149 /* description */ return new String[] { "markdown" }; 3150 case -669707736: 3151 /* useContext */ return new String[] { "UsageContext" }; 3152 case -507075711: 3153 /* jurisdiction */ return new String[] { "CodeableConcept" }; 3154 case -220463842: 3155 /* purpose */ return new String[] { "markdown" }; 3156 case 1522889671: 3157 /* copyright */ return new String[] { "markdown" }; 3158 case 3016401: 3159 /* base */ return new String[] { "canonical" }; 3160 case -995424086: 3161 /* parent */ return new String[] { "canonical" }; 3162 case 96891546: 3163 /* event */ return new String[] { "Coding", "uri" }; 3164 case 50511102: 3165 /* category */ return new String[] { "code" }; 3166 case 97604824: 3167 /* focus */ return new String[] {}; 3168 case 791597824: 3169 /* responseRequired */ return new String[] { "code" }; 3170 case -1130933751: 3171 /* allowedResponse */ return new String[] {}; 3172 case 98615630: 3173 /* graph */ return new String[] { "canonical" }; 3174 default: 3175 return super.getTypesForProperty(hash, name); 3176 } 3177 3178 } 3179 3180 @Override 3181 public Base addChild(String name) throws FHIRException { 3182 if (name.equals("url")) { 3183 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.url"); 3184 } else if (name.equals("identifier")) { 3185 return addIdentifier(); 3186 } else if (name.equals("version")) { 3187 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.version"); 3188 } else if (name.equals("name")) { 3189 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.name"); 3190 } else if (name.equals("title")) { 3191 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.title"); 3192 } else if (name.equals("replaces")) { 3193 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.replaces"); 3194 } else if (name.equals("status")) { 3195 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.status"); 3196 } else if (name.equals("experimental")) { 3197 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.experimental"); 3198 } else if (name.equals("date")) { 3199 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.date"); 3200 } else if (name.equals("publisher")) { 3201 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.publisher"); 3202 } else if (name.equals("contact")) { 3203 return addContact(); 3204 } else if (name.equals("description")) { 3205 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.description"); 3206 } else if (name.equals("useContext")) { 3207 return addUseContext(); 3208 } else if (name.equals("jurisdiction")) { 3209 return addJurisdiction(); 3210 } else if (name.equals("purpose")) { 3211 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.purpose"); 3212 } else if (name.equals("copyright")) { 3213 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyright"); 3214 } else if (name.equals("base")) { 3215 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.base"); 3216 } else if (name.equals("parent")) { 3217 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.parent"); 3218 } else if (name.equals("eventCoding")) { 3219 this.event = new Coding(); 3220 return this.event; 3221 } else if (name.equals("eventUri")) { 3222 this.event = new UriType(); 3223 return this.event; 3224 } else if (name.equals("category")) { 3225 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.category"); 3226 } else if (name.equals("focus")) { 3227 return addFocus(); 3228 } else if (name.equals("responseRequired")) { 3229 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.responseRequired"); 3230 } else if (name.equals("allowedResponse")) { 3231 return addAllowedResponse(); 3232 } else if (name.equals("graph")) { 3233 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.graph"); 3234 } else 3235 return super.addChild(name); 3236 } 3237 3238 public String fhirType() { 3239 return "MessageDefinition"; 3240 3241 } 3242 3243 public MessageDefinition copy() { 3244 MessageDefinition dst = new MessageDefinition(); 3245 copyValues(dst); 3246 return dst; 3247 } 3248 3249 public void copyValues(MessageDefinition dst) { 3250 super.copyValues(dst); 3251 dst.url = url == null ? null : url.copy(); 3252 if (identifier != null) { 3253 dst.identifier = new ArrayList<Identifier>(); 3254 for (Identifier i : identifier) 3255 dst.identifier.add(i.copy()); 3256 } 3257 ; 3258 dst.version = version == null ? null : version.copy(); 3259 dst.name = name == null ? null : name.copy(); 3260 dst.title = title == null ? null : title.copy(); 3261 if (replaces != null) { 3262 dst.replaces = new ArrayList<CanonicalType>(); 3263 for (CanonicalType i : replaces) 3264 dst.replaces.add(i.copy()); 3265 } 3266 ; 3267 dst.status = status == null ? null : status.copy(); 3268 dst.experimental = experimental == null ? null : experimental.copy(); 3269 dst.date = date == null ? null : date.copy(); 3270 dst.publisher = publisher == null ? null : publisher.copy(); 3271 if (contact != null) { 3272 dst.contact = new ArrayList<ContactDetail>(); 3273 for (ContactDetail i : contact) 3274 dst.contact.add(i.copy()); 3275 } 3276 ; 3277 dst.description = description == null ? null : description.copy(); 3278 if (useContext != null) { 3279 dst.useContext = new ArrayList<UsageContext>(); 3280 for (UsageContext i : useContext) 3281 dst.useContext.add(i.copy()); 3282 } 3283 ; 3284 if (jurisdiction != null) { 3285 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3286 for (CodeableConcept i : jurisdiction) 3287 dst.jurisdiction.add(i.copy()); 3288 } 3289 ; 3290 dst.purpose = purpose == null ? null : purpose.copy(); 3291 dst.copyright = copyright == null ? null : copyright.copy(); 3292 dst.base = base == null ? null : base.copy(); 3293 if (parent != null) { 3294 dst.parent = new ArrayList<CanonicalType>(); 3295 for (CanonicalType i : parent) 3296 dst.parent.add(i.copy()); 3297 } 3298 ; 3299 dst.event = event == null ? null : event.copy(); 3300 dst.category = category == null ? null : category.copy(); 3301 if (focus != null) { 3302 dst.focus = new ArrayList<MessageDefinitionFocusComponent>(); 3303 for (MessageDefinitionFocusComponent i : focus) 3304 dst.focus.add(i.copy()); 3305 } 3306 ; 3307 dst.responseRequired = responseRequired == null ? null : responseRequired.copy(); 3308 if (allowedResponse != null) { 3309 dst.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 3310 for (MessageDefinitionAllowedResponseComponent i : allowedResponse) 3311 dst.allowedResponse.add(i.copy()); 3312 } 3313 ; 3314 if (graph != null) { 3315 dst.graph = new ArrayList<CanonicalType>(); 3316 for (CanonicalType i : graph) 3317 dst.graph.add(i.copy()); 3318 } 3319 ; 3320 } 3321 3322 protected MessageDefinition typedCopy() { 3323 return copy(); 3324 } 3325 3326 @Override 3327 public boolean equalsDeep(Base other_) { 3328 if (!super.equalsDeep(other_)) 3329 return false; 3330 if (!(other_ instanceof MessageDefinition)) 3331 return false; 3332 MessageDefinition o = (MessageDefinition) other_; 3333 return compareDeep(identifier, o.identifier, true) && compareDeep(replaces, o.replaces, true) 3334 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 3335 && compareDeep(base, o.base, true) && compareDeep(parent, o.parent, true) && compareDeep(event, o.event, true) 3336 && compareDeep(category, o.category, true) && compareDeep(focus, o.focus, true) 3337 && compareDeep(responseRequired, o.responseRequired, true) 3338 && compareDeep(allowedResponse, o.allowedResponse, true) && compareDeep(graph, o.graph, true); 3339 } 3340 3341 @Override 3342 public boolean equalsShallow(Base other_) { 3343 if (!super.equalsShallow(other_)) 3344 return false; 3345 if (!(other_ instanceof MessageDefinition)) 3346 return false; 3347 MessageDefinition o = (MessageDefinition) other_; 3348 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) 3349 && compareValues(category, o.category, true) && compareValues(responseRequired, o.responseRequired, true); 3350 } 3351 3352 public boolean isEmpty() { 3353 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, replaces, purpose, copyright, base, 3354 parent, event, category, focus, responseRequired, allowedResponse, graph); 3355 } 3356 3357 @Override 3358 public ResourceType getResourceType() { 3359 return ResourceType.MessageDefinition; 3360 } 3361 3362 /** 3363 * Search parameter: <b>date</b> 3364 * <p> 3365 * Description: <b>The message definition publication date</b><br> 3366 * Type: <b>date</b><br> 3367 * Path: <b>MessageDefinition.date</b><br> 3368 * </p> 3369 */ 3370 @SearchParamDefinition(name = "date", path = "MessageDefinition.date", description = "The message definition publication date", type = "date") 3371 public static final String SP_DATE = "date"; 3372 /** 3373 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3374 * <p> 3375 * Description: <b>The message definition publication date</b><br> 3376 * Type: <b>date</b><br> 3377 * Path: <b>MessageDefinition.date</b><br> 3378 * </p> 3379 */ 3380 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3381 SP_DATE); 3382 3383 /** 3384 * Search parameter: <b>identifier</b> 3385 * <p> 3386 * Description: <b>External identifier for the message definition</b><br> 3387 * Type: <b>token</b><br> 3388 * Path: <b>MessageDefinition.identifier</b><br> 3389 * </p> 3390 */ 3391 @SearchParamDefinition(name = "identifier", path = "MessageDefinition.identifier", description = "External identifier for the message definition", type = "token") 3392 public static final String SP_IDENTIFIER = "identifier"; 3393 /** 3394 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3395 * <p> 3396 * Description: <b>External identifier for the message definition</b><br> 3397 * Type: <b>token</b><br> 3398 * Path: <b>MessageDefinition.identifier</b><br> 3399 * </p> 3400 */ 3401 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3402 SP_IDENTIFIER); 3403 3404 /** 3405 * Search parameter: <b>parent</b> 3406 * <p> 3407 * Description: <b>A resource that is the parent of the definition</b><br> 3408 * Type: <b>reference</b><br> 3409 * Path: <b>MessageDefinition.parent</b><br> 3410 * </p> 3411 */ 3412 @SearchParamDefinition(name = "parent", path = "MessageDefinition.parent", description = "A resource that is the parent of the definition", type = "reference", target = { 3413 ActivityDefinition.class, PlanDefinition.class }) 3414 public static final String SP_PARENT = "parent"; 3415 /** 3416 * <b>Fluent Client</b> search parameter constant for <b>parent</b> 3417 * <p> 3418 * Description: <b>A resource that is the parent of the definition</b><br> 3419 * Type: <b>reference</b><br> 3420 * Path: <b>MessageDefinition.parent</b><br> 3421 * </p> 3422 */ 3423 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3424 SP_PARENT); 3425 3426 /** 3427 * Constant for fluent queries to be used to add include statements. Specifies 3428 * the path value of "<b>MessageDefinition:parent</b>". 3429 */ 3430 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARENT = new ca.uhn.fhir.model.api.Include( 3431 "MessageDefinition:parent").toLocked(); 3432 3433 /** 3434 * Search parameter: <b>context-type-value</b> 3435 * <p> 3436 * Description: <b>A use context type and value assigned to the message 3437 * definition</b><br> 3438 * Type: <b>composite</b><br> 3439 * Path: <b></b><br> 3440 * </p> 3441 */ 3442 @SearchParamDefinition(name = "context-type-value", path = "MessageDefinition.useContext", description = "A use context type and value assigned to the message definition", type = "composite", compositeOf = { 3443 "context-type", "context" }) 3444 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3445 /** 3446 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3447 * <p> 3448 * Description: <b>A use context type and value assigned to the message 3449 * definition</b><br> 3450 * Type: <b>composite</b><br> 3451 * Path: <b></b><br> 3452 * </p> 3453 */ 3454 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 3455 SP_CONTEXT_TYPE_VALUE); 3456 3457 /** 3458 * Search parameter: <b>jurisdiction</b> 3459 * <p> 3460 * Description: <b>Intended jurisdiction for the message definition</b><br> 3461 * Type: <b>token</b><br> 3462 * Path: <b>MessageDefinition.jurisdiction</b><br> 3463 * </p> 3464 */ 3465 @SearchParamDefinition(name = "jurisdiction", path = "MessageDefinition.jurisdiction", description = "Intended jurisdiction for the message definition", type = "token") 3466 public static final String SP_JURISDICTION = "jurisdiction"; 3467 /** 3468 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3469 * <p> 3470 * Description: <b>Intended jurisdiction for the message definition</b><br> 3471 * Type: <b>token</b><br> 3472 * Path: <b>MessageDefinition.jurisdiction</b><br> 3473 * </p> 3474 */ 3475 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3476 SP_JURISDICTION); 3477 3478 /** 3479 * Search parameter: <b>description</b> 3480 * <p> 3481 * Description: <b>The description of the message definition</b><br> 3482 * Type: <b>string</b><br> 3483 * Path: <b>MessageDefinition.description</b><br> 3484 * </p> 3485 */ 3486 @SearchParamDefinition(name = "description", path = "MessageDefinition.description", description = "The description of the message definition", type = "string") 3487 public static final String SP_DESCRIPTION = "description"; 3488 /** 3489 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3490 * <p> 3491 * Description: <b>The description of the message definition</b><br> 3492 * Type: <b>string</b><br> 3493 * Path: <b>MessageDefinition.description</b><br> 3494 * </p> 3495 */ 3496 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 3497 SP_DESCRIPTION); 3498 3499 /** 3500 * Search parameter: <b>focus</b> 3501 * <p> 3502 * Description: <b>A resource that is a permitted focus of the message</b><br> 3503 * Type: <b>token</b><br> 3504 * Path: <b>MessageDefinition.focus.code</b><br> 3505 * </p> 3506 */ 3507 @SearchParamDefinition(name = "focus", path = "MessageDefinition.focus.code", description = "A resource that is a permitted focus of the message", type = "token") 3508 public static final String SP_FOCUS = "focus"; 3509 /** 3510 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 3511 * <p> 3512 * Description: <b>A resource that is a permitted focus of the message</b><br> 3513 * Type: <b>token</b><br> 3514 * Path: <b>MessageDefinition.focus.code</b><br> 3515 * </p> 3516 */ 3517 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3518 SP_FOCUS); 3519 3520 /** 3521 * Search parameter: <b>context-type</b> 3522 * <p> 3523 * Description: <b>A type of use context assigned to the message 3524 * definition</b><br> 3525 * Type: <b>token</b><br> 3526 * Path: <b>MessageDefinition.useContext.code</b><br> 3527 * </p> 3528 */ 3529 @SearchParamDefinition(name = "context-type", path = "MessageDefinition.useContext.code", description = "A type of use context assigned to the message definition", type = "token") 3530 public static final String SP_CONTEXT_TYPE = "context-type"; 3531 /** 3532 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3533 * <p> 3534 * Description: <b>A type of use context assigned to the message 3535 * definition</b><br> 3536 * Type: <b>token</b><br> 3537 * Path: <b>MessageDefinition.useContext.code</b><br> 3538 * </p> 3539 */ 3540 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3541 SP_CONTEXT_TYPE); 3542 3543 /** 3544 * Search parameter: <b>title</b> 3545 * <p> 3546 * Description: <b>The human-friendly name of the message definition</b><br> 3547 * Type: <b>string</b><br> 3548 * Path: <b>MessageDefinition.title</b><br> 3549 * </p> 3550 */ 3551 @SearchParamDefinition(name = "title", path = "MessageDefinition.title", description = "The human-friendly name of the message definition", type = "string") 3552 public static final String SP_TITLE = "title"; 3553 /** 3554 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3555 * <p> 3556 * Description: <b>The human-friendly name of the message definition</b><br> 3557 * Type: <b>string</b><br> 3558 * Path: <b>MessageDefinition.title</b><br> 3559 * </p> 3560 */ 3561 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 3562 SP_TITLE); 3563 3564 /** 3565 * Search parameter: <b>version</b> 3566 * <p> 3567 * Description: <b>The business version of the message definition</b><br> 3568 * Type: <b>token</b><br> 3569 * Path: <b>MessageDefinition.version</b><br> 3570 * </p> 3571 */ 3572 @SearchParamDefinition(name = "version", path = "MessageDefinition.version", description = "The business version of the message definition", type = "token") 3573 public static final String SP_VERSION = "version"; 3574 /** 3575 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3576 * <p> 3577 * Description: <b>The business version of the message definition</b><br> 3578 * Type: <b>token</b><br> 3579 * Path: <b>MessageDefinition.version</b><br> 3580 * </p> 3581 */ 3582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3583 SP_VERSION); 3584 3585 /** 3586 * Search parameter: <b>url</b> 3587 * <p> 3588 * Description: <b>The uri that identifies the message definition</b><br> 3589 * Type: <b>uri</b><br> 3590 * Path: <b>MessageDefinition.url</b><br> 3591 * </p> 3592 */ 3593 @SearchParamDefinition(name = "url", path = "MessageDefinition.url", description = "The uri that identifies the message definition", type = "uri") 3594 public static final String SP_URL = "url"; 3595 /** 3596 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3597 * <p> 3598 * Description: <b>The uri that identifies the message definition</b><br> 3599 * Type: <b>uri</b><br> 3600 * Path: <b>MessageDefinition.url</b><br> 3601 * </p> 3602 */ 3603 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3604 3605 /** 3606 * Search parameter: <b>context-quantity</b> 3607 * <p> 3608 * Description: <b>A quantity- or range-valued use context assigned to the 3609 * message definition</b><br> 3610 * Type: <b>quantity</b><br> 3611 * Path: <b>MessageDefinition.useContext.valueQuantity, 3612 * MessageDefinition.useContext.valueRange</b><br> 3613 * </p> 3614 */ 3615 @SearchParamDefinition(name = "context-quantity", path = "(MessageDefinition.useContext.value as Quantity) | (MessageDefinition.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the message definition", type = "quantity") 3616 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3617 /** 3618 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3619 * <p> 3620 * Description: <b>A quantity- or range-valued use context assigned to the 3621 * message definition</b><br> 3622 * Type: <b>quantity</b><br> 3623 * Path: <b>MessageDefinition.useContext.valueQuantity, 3624 * MessageDefinition.useContext.valueRange</b><br> 3625 * </p> 3626 */ 3627 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3628 SP_CONTEXT_QUANTITY); 3629 3630 /** 3631 * Search parameter: <b>name</b> 3632 * <p> 3633 * Description: <b>Computationally friendly name of the message 3634 * definition</b><br> 3635 * Type: <b>string</b><br> 3636 * Path: <b>MessageDefinition.name</b><br> 3637 * </p> 3638 */ 3639 @SearchParamDefinition(name = "name", path = "MessageDefinition.name", description = "Computationally friendly name of the message definition", type = "string") 3640 public static final String SP_NAME = "name"; 3641 /** 3642 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3643 * <p> 3644 * Description: <b>Computationally friendly name of the message 3645 * definition</b><br> 3646 * Type: <b>string</b><br> 3647 * Path: <b>MessageDefinition.name</b><br> 3648 * </p> 3649 */ 3650 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3651 SP_NAME); 3652 3653 /** 3654 * Search parameter: <b>context</b> 3655 * <p> 3656 * Description: <b>A use context assigned to the message definition</b><br> 3657 * Type: <b>token</b><br> 3658 * Path: <b>MessageDefinition.useContext.valueCodeableConcept</b><br> 3659 * </p> 3660 */ 3661 @SearchParamDefinition(name = "context", path = "(MessageDefinition.useContext.value as CodeableConcept)", description = "A use context assigned to the message definition", type = "token") 3662 public static final String SP_CONTEXT = "context"; 3663 /** 3664 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3665 * <p> 3666 * Description: <b>A use context assigned to the message definition</b><br> 3667 * Type: <b>token</b><br> 3668 * Path: <b>MessageDefinition.useContext.valueCodeableConcept</b><br> 3669 * </p> 3670 */ 3671 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3672 SP_CONTEXT); 3673 3674 /** 3675 * Search parameter: <b>publisher</b> 3676 * <p> 3677 * Description: <b>Name of the publisher of the message definition</b><br> 3678 * Type: <b>string</b><br> 3679 * Path: <b>MessageDefinition.publisher</b><br> 3680 * </p> 3681 */ 3682 @SearchParamDefinition(name = "publisher", path = "MessageDefinition.publisher", description = "Name of the publisher of the message definition", type = "string") 3683 public static final String SP_PUBLISHER = "publisher"; 3684 /** 3685 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3686 * <p> 3687 * Description: <b>Name of the publisher of the message definition</b><br> 3688 * Type: <b>string</b><br> 3689 * Path: <b>MessageDefinition.publisher</b><br> 3690 * </p> 3691 */ 3692 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3693 SP_PUBLISHER); 3694 3695 /** 3696 * Search parameter: <b>event</b> 3697 * <p> 3698 * Description: <b>The event that triggers the message or link to the event 3699 * definition.</b><br> 3700 * Type: <b>token</b><br> 3701 * Path: <b>MessageDefinition.event[x]</b><br> 3702 * </p> 3703 */ 3704 @SearchParamDefinition(name = "event", path = "MessageDefinition.event", description = "The event that triggers the message or link to the event definition.", type = "token") 3705 public static final String SP_EVENT = "event"; 3706 /** 3707 * <b>Fluent Client</b> search parameter constant for <b>event</b> 3708 * <p> 3709 * Description: <b>The event that triggers the message or link to the event 3710 * definition.</b><br> 3711 * Type: <b>token</b><br> 3712 * Path: <b>MessageDefinition.event[x]</b><br> 3713 * </p> 3714 */ 3715 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3716 SP_EVENT); 3717 3718 /** 3719 * Search parameter: <b>category</b> 3720 * <p> 3721 * Description: <b>The behavior associated with the message</b><br> 3722 * Type: <b>token</b><br> 3723 * Path: <b>MessageDefinition.category</b><br> 3724 * </p> 3725 */ 3726 @SearchParamDefinition(name = "category", path = "MessageDefinition.category", description = "The behavior associated with the message", type = "token") 3727 public static final String SP_CATEGORY = "category"; 3728 /** 3729 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3730 * <p> 3731 * Description: <b>The behavior associated with the message</b><br> 3732 * Type: <b>token</b><br> 3733 * Path: <b>MessageDefinition.category</b><br> 3734 * </p> 3735 */ 3736 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3737 SP_CATEGORY); 3738 3739 /** 3740 * Search parameter: <b>context-type-quantity</b> 3741 * <p> 3742 * Description: <b>A use context type and quantity- or range-based value 3743 * assigned to the message definition</b><br> 3744 * Type: <b>composite</b><br> 3745 * Path: <b></b><br> 3746 * </p> 3747 */ 3748 @SearchParamDefinition(name = "context-type-quantity", path = "MessageDefinition.useContext", description = "A use context type and quantity- or range-based value assigned to the message definition", type = "composite", compositeOf = { 3749 "context-type", "context-quantity" }) 3750 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3751 /** 3752 * <b>Fluent Client</b> search parameter constant for 3753 * <b>context-type-quantity</b> 3754 * <p> 3755 * Description: <b>A use context type and quantity- or range-based value 3756 * assigned to the message definition</b><br> 3757 * Type: <b>composite</b><br> 3758 * Path: <b></b><br> 3759 * </p> 3760 */ 3761 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3762 SP_CONTEXT_TYPE_QUANTITY); 3763 3764 /** 3765 * Search parameter: <b>status</b> 3766 * <p> 3767 * Description: <b>The current status of the message definition</b><br> 3768 * Type: <b>token</b><br> 3769 * Path: <b>MessageDefinition.status</b><br> 3770 * </p> 3771 */ 3772 @SearchParamDefinition(name = "status", path = "MessageDefinition.status", description = "The current status of the message definition", type = "token") 3773 public static final String SP_STATUS = "status"; 3774 /** 3775 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3776 * <p> 3777 * Description: <b>The current status of the message definition</b><br> 3778 * Type: <b>token</b><br> 3779 * Path: <b>MessageDefinition.status</b><br> 3780 * </p> 3781 */ 3782 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3783 SP_STATUS); 3784 3785}