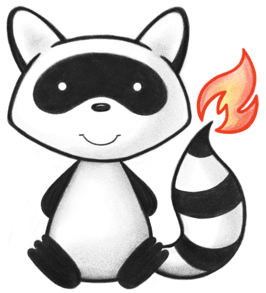
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseMetaType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * The metadata about a resource. This is content in the resource that is 047 * maintained by the infrastructure. Changes to the content might not always be 048 * associated with version changes to the resource. 049 */ 050@DatatypeDef(name = "Meta") 051public class Meta extends Type implements IBaseMetaType { 052 053 /** 054 * The version specific identifier, as it appears in the version portion of the 055 * URL. This value changes when the resource is created, updated, or deleted. 056 */ 057 @Child(name = "versionId", type = { IdType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 058 @Description(shortDefinition = "Version specific identifier", formalDefinition = "The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.") 059 protected IdType versionId; 060 061 /** 062 * When the resource last changed - e.g. when the version changed. 063 */ 064 @Child(name = "lastUpdated", type = { 065 InstantType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 066 @Description(shortDefinition = "When the resource version last changed", formalDefinition = "When the resource last changed - e.g. when the version changed.") 067 protected InstantType lastUpdated; 068 069 /** 070 * A uri that identifies the source system of the resource. This provides a 071 * minimal amount of [[[Provenance]]] information that can be used to track or 072 * differentiate the source of information in the resource. The source may 073 * identify another FHIR server, document, message, database, etc. 074 */ 075 @Child(name = "source", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 076 @Description(shortDefinition = "Identifies where the resource comes from", formalDefinition = "A uri that identifies the source system of the resource. This provides a minimal amount of [[[Provenance]]] information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.") 077 protected UriType source; 078 079 /** 080 * A list of profiles (references to [[[StructureDefinition]]] resources) that 081 * this resource claims to conform to. The URL is a reference to 082 * [[[StructureDefinition.url]]]. 083 */ 084 @Child(name = "profile", type = { 085 CanonicalType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 086 @Description(shortDefinition = "Profiles this resource claims to conform to", formalDefinition = "A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].") 087 protected List<CanonicalType> profile; 088 089 /** 090 * Security labels applied to this resource. These tags connect specific 091 * resources to the overall security policy and infrastructure. 092 */ 093 @Child(name = "security", type = { 094 Coding.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 095 @Description(shortDefinition = "Security Labels applied to this resource", formalDefinition = "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.") 096 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/security-labels") 097 protected List<Coding> security; 098 099 /** 100 * Tags applied to this resource. Tags are intended to be used to identify and 101 * relate resources to process and workflow, and applications are not required 102 * to consider the tags when interpreting the meaning of a resource. 103 */ 104 @Child(name = "tag", type = { 105 Coding.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 106 @Description(shortDefinition = "Tags applied to this resource", formalDefinition = "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.") 107 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/common-tags") 108 protected List<Coding> tag; 109 110 private static final long serialVersionUID = -1386695622L; 111 112 /** 113 * Constructor 114 */ 115 public Meta() { 116 super(); 117 } 118 119 /** 120 * @return {@link #versionId} (The version specific identifier, as it appears in 121 * the version portion of the URL. This value changes when the resource 122 * is created, updated, or deleted.). This is the underlying object with 123 * id, value and extensions. The accessor "getVersionId" gives direct 124 * access to the value 125 */ 126 public IdType getVersionIdElement() { 127 if (this.versionId == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create Meta.versionId"); 130 else if (Configuration.doAutoCreate()) 131 this.versionId = new IdType(); // bb 132 return this.versionId; 133 } 134 135 public boolean hasVersionIdElement() { 136 return this.versionId != null && !this.versionId.isEmpty(); 137 } 138 139 public boolean hasVersionId() { 140 return this.versionId != null && !this.versionId.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #versionId} (The version specific identifier, as it 145 * appears in the version portion of the URL. This value changes 146 * when the resource is created, updated, or deleted.). This is the 147 * underlying object with id, value and extensions. The accessor 148 * "getVersionId" gives direct access to the value 149 */ 150 public Meta setVersionIdElement(IdType value) { 151 this.versionId = value; 152 return this; 153 } 154 155 /** 156 * @return The version specific identifier, as it appears in the version portion 157 * of the URL. This value changes when the resource is created, updated, 158 * or deleted. 159 */ 160 public String getVersionId() { 161 return this.versionId == null ? null : this.versionId.getValue(); 162 } 163 164 /** 165 * @param value The version specific identifier, as it appears in the version 166 * portion of the URL. This value changes when the resource is 167 * created, updated, or deleted. 168 */ 169 public Meta setVersionId(String value) { 170 if (Utilities.noString(value)) 171 this.versionId = null; 172 else { 173 if (this.versionId == null) 174 this.versionId = new IdType(); 175 this.versionId.setValue(value); 176 } 177 return this; 178 } 179 180 /** 181 * @return {@link #lastUpdated} (When the resource last changed - e.g. when the 182 * version changed.). This is the underlying object with id, value and 183 * extensions. The accessor "getLastUpdated" gives direct access to the 184 * value 185 */ 186 public InstantType getLastUpdatedElement() { 187 if (this.lastUpdated == null) 188 if (Configuration.errorOnAutoCreate()) 189 throw new Error("Attempt to auto-create Meta.lastUpdated"); 190 else if (Configuration.doAutoCreate()) 191 this.lastUpdated = new InstantType(); // bb 192 return this.lastUpdated; 193 } 194 195 public boolean hasLastUpdatedElement() { 196 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 197 } 198 199 public boolean hasLastUpdated() { 200 return this.lastUpdated != null && !this.lastUpdated.isEmpty(); 201 } 202 203 /** 204 * @param value {@link #lastUpdated} (When the resource last changed - e.g. when 205 * the version changed.). This is the underlying object with id, 206 * value and extensions. The accessor "getLastUpdated" gives direct 207 * access to the value 208 */ 209 public Meta setLastUpdatedElement(InstantType value) { 210 this.lastUpdated = value; 211 return this; 212 } 213 214 /** 215 * @return When the resource last changed - e.g. when the version changed. 216 */ 217 public Date getLastUpdated() { 218 return this.lastUpdated == null ? null : this.lastUpdated.getValue(); 219 } 220 221 /** 222 * @param value When the resource last changed - e.g. when the version changed. 223 */ 224 public Meta setLastUpdated(Date value) { 225 if (value == null) 226 this.lastUpdated = null; 227 else { 228 if (this.lastUpdated == null) 229 this.lastUpdated = new InstantType(); 230 this.lastUpdated.setValue(value); 231 } 232 return this; 233 } 234 235 /** 236 * @return {@link #source} (A uri that identifies the source system of the 237 * resource. This provides a minimal amount of [[[Provenance]]] 238 * information that can be used to track or differentiate the source of 239 * information in the resource. The source may identify another FHIR 240 * server, document, message, database, etc.). This is the underlying 241 * object with id, value and extensions. The accessor "getSource" gives 242 * direct access to the value 243 */ 244 public UriType getSourceElement() { 245 if (this.source == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create Meta.source"); 248 else if (Configuration.doAutoCreate()) 249 this.source = new UriType(); // bb 250 return this.source; 251 } 252 253 public boolean hasSourceElement() { 254 return this.source != null && !this.source.isEmpty(); 255 } 256 257 public boolean hasSource() { 258 return this.source != null && !this.source.isEmpty(); 259 } 260 261 /** 262 * @param value {@link #source} (A uri that identifies the source system of the 263 * resource. This provides a minimal amount of [[[Provenance]]] 264 * information that can be used to track or differentiate the 265 * source of information in the resource. The source may identify 266 * another FHIR server, document, message, database, etc.). This is 267 * the underlying object with id, value and extensions. The 268 * accessor "getSource" gives direct access to the value 269 */ 270 public Meta setSourceElement(UriType value) { 271 this.source = value; 272 return this; 273 } 274 275 /** 276 * @return A uri that identifies the source system of the resource. This 277 * provides a minimal amount of [[[Provenance]]] information that can be 278 * used to track or differentiate the source of information in the 279 * resource. The source may identify another FHIR server, document, 280 * message, database, etc. 281 */ 282 public String getSource() { 283 return this.source == null ? null : this.source.getValue(); 284 } 285 286 /** 287 * @param value A uri that identifies the source system of the resource. This 288 * provides a minimal amount of [[[Provenance]]] information that 289 * can be used to track or differentiate the source of information 290 * in the resource. The source may identify another FHIR server, 291 * document, message, database, etc. 292 */ 293 public Meta setSource(String value) { 294 if (Utilities.noString(value)) 295 this.source = null; 296 else { 297 if (this.source == null) 298 this.source = new UriType(); 299 this.source.setValue(value); 300 } 301 return this; 302 } 303 304 /** 305 * @return {@link #profile} (A list of profiles (references to 306 * [[[StructureDefinition]]] resources) that this resource claims to 307 * conform to. The URL is a reference to [[[StructureDefinition.url]]].) 308 */ 309 public List<CanonicalType> getProfile() { 310 if (this.profile == null) 311 this.profile = new ArrayList<CanonicalType>(); 312 return this.profile; 313 } 314 315 /** 316 * @return Returns a reference to <code>this</code> for easy method chaining 317 */ 318 public Meta setProfile(List<CanonicalType> theProfile) { 319 this.profile = theProfile; 320 return this; 321 } 322 323 public boolean hasProfile() { 324 if (this.profile == null) 325 return false; 326 for (CanonicalType item : this.profile) 327 if (!item.isEmpty()) 328 return true; 329 return false; 330 } 331 332 /** 333 * @return {@link #profile} (A list of profiles (references to 334 * [[[StructureDefinition]]] resources) that this resource claims to 335 * conform to. The URL is a reference to [[[StructureDefinition.url]]].) 336 */ 337 public CanonicalType addProfileElement() {// 2 338 CanonicalType t = new CanonicalType(); 339 if (this.profile == null) 340 this.profile = new ArrayList<CanonicalType>(); 341 this.profile.add(t); 342 return t; 343 } 344 345 /** 346 * @param value {@link #profile} (A list of profiles (references to 347 * [[[StructureDefinition]]] resources) that this resource claims 348 * to conform to. The URL is a reference to 349 * [[[StructureDefinition.url]]].) 350 */ 351 public Meta addProfile(String value) { // 1 352 CanonicalType t = new CanonicalType(); 353 t.setValue(value); 354 if (this.profile == null) 355 this.profile = new ArrayList<CanonicalType>(); 356 this.profile.add(t); 357 return this; 358 } 359 360 /** 361 * @param value {@link #profile} (A list of profiles (references to 362 * [[[StructureDefinition]]] resources) that this resource claims 363 * to conform to. The URL is a reference to 364 * [[[StructureDefinition.url]]].) 365 */ 366 public boolean hasProfile(String value) { 367 if (this.profile == null) 368 return false; 369 for (CanonicalType v : this.profile) 370 if (v.getValue().equals(value)) // canonical(StructureDefinition) 371 return true; 372 return false; 373 } 374 375 /** 376 * @return {@link #security} (Security labels applied to this resource. These 377 * tags connect specific resources to the overall security policy and 378 * infrastructure.) 379 */ 380 public List<Coding> getSecurity() { 381 if (this.security == null) 382 this.security = new ArrayList<Coding>(); 383 return this.security; 384 } 385 386 /** 387 * @return Returns a reference to <code>this</code> for easy method chaining 388 */ 389 public Meta setSecurity(List<Coding> theSecurity) { 390 this.security = theSecurity; 391 return this; 392 } 393 394 public boolean hasSecurity() { 395 if (this.security == null) 396 return false; 397 for (Coding item : this.security) 398 if (!item.isEmpty()) 399 return true; 400 return false; 401 } 402 403 public Coding addSecurity() { // 3 404 Coding t = new Coding(); 405 if (this.security == null) 406 this.security = new ArrayList<Coding>(); 407 this.security.add(t); 408 return t; 409 } 410 411 public Meta addSecurity(Coding t) { // 3 412 if (t == null) 413 return this; 414 if (this.security == null) 415 this.security = new ArrayList<Coding>(); 416 this.security.add(t); 417 return this; 418 } 419 420 /** 421 * @return The first repetition of repeating field {@link #security}, creating 422 * it if it does not already exist 423 */ 424 public Coding getSecurityFirstRep() { 425 if (getSecurity().isEmpty()) { 426 addSecurity(); 427 } 428 return getSecurity().get(0); 429 } 430 431 /** 432 * @return {@link #tag} (Tags applied to this resource. Tags are intended to be 433 * used to identify and relate resources to process and workflow, and 434 * applications are not required to consider the tags when interpreting 435 * the meaning of a resource.) 436 */ 437 public List<Coding> getTag() { 438 if (this.tag == null) 439 this.tag = new ArrayList<Coding>(); 440 return this.tag; 441 } 442 443 /** 444 * @return Returns a reference to <code>this</code> for easy method chaining 445 */ 446 public Meta setTag(List<Coding> theTag) { 447 this.tag = theTag; 448 return this; 449 } 450 451 public boolean hasTag() { 452 if (this.tag == null) 453 return false; 454 for (Coding item : this.tag) 455 if (!item.isEmpty()) 456 return true; 457 return false; 458 } 459 460 public Coding addTag() { // 3 461 Coding t = new Coding(); 462 if (this.tag == null) 463 this.tag = new ArrayList<Coding>(); 464 this.tag.add(t); 465 return t; 466 } 467 468 public Meta addTag(Coding t) { // 3 469 if (t == null) 470 return this; 471 if (this.tag == null) 472 this.tag = new ArrayList<Coding>(); 473 this.tag.add(t); 474 return this; 475 } 476 477 /** 478 * @return The first repetition of repeating field {@link #tag}, creating it if 479 * it does not already exist 480 */ 481 public Coding getTagFirstRep() { 482 if (getTag().isEmpty()) { 483 addTag(); 484 } 485 return getTag().get(0); 486 } 487 488 /** 489 * Convenience method which adds a tag 490 * 491 * @param theSystem The code system 492 * @param theCode The code 493 * @param theDisplay The display name 494 * @return Returns a reference to <code>this</code> for easy chaining 495 */ 496 public Meta addTag(String theSystem, String theCode, String theDisplay) { 497 addTag().setSystem(theSystem).setCode(theCode).setDisplay(theDisplay); 498 return this; 499 } 500 501 /** 502 * Convenience method which adds a security tag 503 * 504 * @param theSystem The code system 505 * @param theCode The code 506 * @param theDisplay The display name 507 * @return Returns a reference to <code>this</code> for easy chaining 508 */ 509 public Meta addSecurity(String theSystem, String theCode, String theDisplay) { 510 addSecurity().setSystem(theSystem).setCode(theCode).setDisplay(theDisplay); 511 return this; 512 } 513 514 /** 515 * Returns the first tag (if any) that has the given system and code, or returns 516 * <code>null</code> if none 517 */ 518 public Coding getTag(String theSystem, String theCode) { 519 for (Coding next : getTag()) { 520 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) 521 && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 522 return next; 523 } 524 } 525 return null; 526 } 527 528 /** 529 * Returns the first security label (if any) that has the given system and code, 530 * or returns <code>null</code> if none 531 */ 532 public Coding getSecurity(String theSystem, String theCode) { 533 for (Coding next : getSecurity()) { 534 if (ca.uhn.fhir.util.ObjectUtil.equals(next.getSystem(), theSystem) 535 && ca.uhn.fhir.util.ObjectUtil.equals(next.getCode(), theCode)) { 536 return next; 537 } 538 } 539 return null; 540 } 541 542 protected void listChildren(List<Property> children) { 543 super.listChildren(children); 544 children.add(new Property("versionId", "id", 545 "The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.", 546 0, 1, versionId)); 547 children.add(new Property("lastUpdated", "instant", 548 "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated)); 549 children.add(new Property("source", "uri", 550 "A uri that identifies the source system of the resource. This provides a minimal amount of [[[Provenance]]] information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.", 551 0, 1, source)); 552 children.add(new Property("profile", "canonical(StructureDefinition)", 553 "A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].", 554 0, java.lang.Integer.MAX_VALUE, profile)); 555 children.add(new Property("security", "Coding", 556 "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 557 0, java.lang.Integer.MAX_VALUE, security)); 558 children.add(new Property("tag", "Coding", 559 "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 560 0, java.lang.Integer.MAX_VALUE, tag)); 561 } 562 563 @Override 564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 565 switch (_hash) { 566 case -1407102957: 567 /* versionId */ return new Property("versionId", "id", 568 "The version specific identifier, as it appears in the version portion of the URL. This value changes when the resource is created, updated, or deleted.", 569 0, 1, versionId); 570 case 1649733957: 571 /* lastUpdated */ return new Property("lastUpdated", "instant", 572 "When the resource last changed - e.g. when the version changed.", 0, 1, lastUpdated); 573 case -896505829: 574 /* source */ return new Property("source", "uri", 575 "A uri that identifies the source system of the resource. This provides a minimal amount of [[[Provenance]]] information that can be used to track or differentiate the source of information in the resource. The source may identify another FHIR server, document, message, database, etc.", 576 0, 1, source); 577 case -309425751: 578 /* profile */ return new Property("profile", "canonical(StructureDefinition)", 579 "A list of profiles (references to [[[StructureDefinition]]] resources) that this resource claims to conform to. The URL is a reference to [[[StructureDefinition.url]]].", 580 0, java.lang.Integer.MAX_VALUE, profile); 581 case 949122880: 582 /* security */ return new Property("security", "Coding", 583 "Security labels applied to this resource. These tags connect specific resources to the overall security policy and infrastructure.", 584 0, java.lang.Integer.MAX_VALUE, security); 585 case 114586: 586 /* tag */ return new Property("tag", "Coding", 587 "Tags applied to this resource. Tags are intended to be used to identify and relate resources to process and workflow, and applications are not required to consider the tags when interpreting the meaning of a resource.", 588 0, java.lang.Integer.MAX_VALUE, tag); 589 default: 590 return super.getNamedProperty(_hash, _name, _checkValid); 591 } 592 593 } 594 595 @Override 596 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 597 switch (hash) { 598 case -1407102957: 599 /* versionId */ return this.versionId == null ? new Base[0] : new Base[] { this.versionId }; // IdType 600 case 1649733957: 601 /* lastUpdated */ return this.lastUpdated == null ? new Base[0] : new Base[] { this.lastUpdated }; // InstantType 602 case -896505829: 603 /* source */ return this.source == null ? new Base[0] : new Base[] { this.source }; // UriType 604 case -309425751: 605 /* profile */ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 606 case 949122880: 607 /* security */ return this.security == null ? new Base[0] : this.security.toArray(new Base[this.security.size()]); // Coding 608 case 114586: 609 /* tag */ return this.tag == null ? new Base[0] : this.tag.toArray(new Base[this.tag.size()]); // Coding 610 default: 611 return super.getProperty(hash, name, checkValid); 612 } 613 614 } 615 616 @Override 617 public Base setProperty(int hash, String name, Base value) throws FHIRException { 618 switch (hash) { 619 case -1407102957: // versionId 620 this.versionId = castToId(value); // IdType 621 return value; 622 case 1649733957: // lastUpdated 623 this.lastUpdated = castToInstant(value); // InstantType 624 return value; 625 case -896505829: // source 626 this.source = castToUri(value); // UriType 627 return value; 628 case -309425751: // profile 629 this.getProfile().add(castToCanonical(value)); // CanonicalType 630 return value; 631 case 949122880: // security 632 this.getSecurity().add(castToCoding(value)); // Coding 633 return value; 634 case 114586: // tag 635 this.getTag().add(castToCoding(value)); // Coding 636 return value; 637 default: 638 return super.setProperty(hash, name, value); 639 } 640 641 } 642 643 @Override 644 public Base setProperty(String name, Base value) throws FHIRException { 645 if (name.equals("versionId")) { 646 this.versionId = castToId(value); // IdType 647 } else if (name.equals("lastUpdated")) { 648 this.lastUpdated = castToInstant(value); // InstantType 649 } else if (name.equals("source")) { 650 this.source = castToUri(value); // UriType 651 } else if (name.equals("profile")) { 652 this.getProfile().add(castToCanonical(value)); 653 } else if (name.equals("security")) { 654 this.getSecurity().add(castToCoding(value)); 655 } else if (name.equals("tag")) { 656 this.getTag().add(castToCoding(value)); 657 } else 658 return super.setProperty(name, value); 659 return value; 660 } 661 662 @Override 663 public void removeChild(String name, Base value) throws FHIRException { 664 if (name.equals("versionId")) { 665 this.versionId = null; 666 } else if (name.equals("lastUpdated")) { 667 this.lastUpdated = null; 668 } else if (name.equals("source")) { 669 this.source = null; 670 } else if (name.equals("profile")) { 671 this.getProfile().remove(castToCanonical(value)); 672 } else if (name.equals("security")) { 673 this.getSecurity().remove(castToCoding(value)); 674 } else if (name.equals("tag")) { 675 this.getTag().remove(castToCoding(value)); 676 } else 677 super.removeChild(name, value); 678 679 } 680 681 @Override 682 public Base makeProperty(int hash, String name) throws FHIRException { 683 switch (hash) { 684 case -1407102957: 685 return getVersionIdElement(); 686 case 1649733957: 687 return getLastUpdatedElement(); 688 case -896505829: 689 return getSourceElement(); 690 case -309425751: 691 return addProfileElement(); 692 case 949122880: 693 return addSecurity(); 694 case 114586: 695 return addTag(); 696 default: 697 return super.makeProperty(hash, name); 698 } 699 700 } 701 702 @Override 703 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 704 switch (hash) { 705 case -1407102957: 706 /* versionId */ return new String[] { "id" }; 707 case 1649733957: 708 /* lastUpdated */ return new String[] { "instant" }; 709 case -896505829: 710 /* source */ return new String[] { "uri" }; 711 case -309425751: 712 /* profile */ return new String[] { "canonical" }; 713 case 949122880: 714 /* security */ return new String[] { "Coding" }; 715 case 114586: 716 /* tag */ return new String[] { "Coding" }; 717 default: 718 return super.getTypesForProperty(hash, name); 719 } 720 721 } 722 723 @Override 724 public Base addChild(String name) throws FHIRException { 725 if (name.equals("versionId")) { 726 throw new FHIRException("Cannot call addChild on a singleton property Meta.versionId"); 727 } else if (name.equals("lastUpdated")) { 728 throw new FHIRException("Cannot call addChild on a singleton property Meta.lastUpdated"); 729 } else if (name.equals("source")) { 730 throw new FHIRException("Cannot call addChild on a singleton property Meta.source"); 731 } else if (name.equals("profile")) { 732 throw new FHIRException("Cannot call addChild on a singleton property Meta.profile"); 733 } else if (name.equals("security")) { 734 return addSecurity(); 735 } else if (name.equals("tag")) { 736 return addTag(); 737 } else 738 return super.addChild(name); 739 } 740 741 public String fhirType() { 742 return "Meta"; 743 744 } 745 746 public Meta copy() { 747 Meta dst = new Meta(); 748 copyValues(dst); 749 return dst; 750 } 751 752 public void copyValues(Meta dst) { 753 super.copyValues(dst); 754 dst.versionId = versionId == null ? null : versionId.copy(); 755 dst.lastUpdated = lastUpdated == null ? null : lastUpdated.copy(); 756 dst.source = source == null ? null : source.copy(); 757 if (profile != null) { 758 dst.profile = new ArrayList<CanonicalType>(); 759 for (CanonicalType i : profile) 760 dst.profile.add(i.copy()); 761 } 762 ; 763 if (security != null) { 764 dst.security = new ArrayList<Coding>(); 765 for (Coding i : security) 766 dst.security.add(i.copy()); 767 } 768 ; 769 if (tag != null) { 770 dst.tag = new ArrayList<Coding>(); 771 for (Coding i : tag) 772 dst.tag.add(i.copy()); 773 } 774 ; 775 } 776 777 protected Meta typedCopy() { 778 return copy(); 779 } 780 781 @Override 782 public boolean equalsDeep(Base other_) { 783 if (!super.equalsDeep(other_)) 784 return false; 785 if (!(other_ instanceof Meta)) 786 return false; 787 Meta o = (Meta) other_; 788 return compareDeep(versionId, o.versionId, true) && compareDeep(lastUpdated, o.lastUpdated, true) 789 && compareDeep(source, o.source, true) && compareDeep(profile, o.profile, true) 790 && compareDeep(security, o.security, true) && compareDeep(tag, o.tag, true); 791 } 792 793 @Override 794 public boolean equalsShallow(Base other_) { 795 if (!super.equalsShallow(other_)) 796 return false; 797 if (!(other_ instanceof Meta)) 798 return false; 799 Meta o = (Meta) other_; 800 return compareValues(versionId, o.versionId, true) && compareValues(lastUpdated, o.lastUpdated, true) 801 && compareValues(source, o.source, true); 802 } 803 804 public boolean isEmpty() { 805 return super.isEmpty() 806 && ca.uhn.fhir.util.ElementUtil.isEmpty(versionId, lastUpdated, source, profile, security, tag); 807 } 808 809}