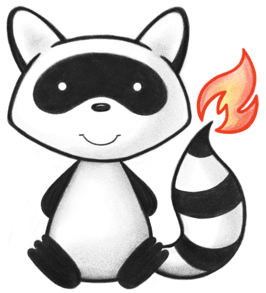
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * Common Ancestor declaration for conformance and knowledge artifact resources. 047 */ 048public abstract class MetadataResource extends DomainResource { 049 050 /** 051 * An absolute URI that is used to identify this metadata resource when it is 052 * referenced in a specification, model, design or an instance; also called its 053 * canonical identifier. This SHOULD be globally unique and SHOULD be a literal 054 * address at which at which an authoritative instance of this metadata resource 055 * is (or will be) published. This URL can be the target of a canonical 056 * reference. It SHALL remain the same when the metadata resource is stored on 057 * different servers. 058 */ 059 @Child(name = "url", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "Canonical identifier for this metadata resource, represented as a URI (globally unique)", formalDefinition = "An absolute URI that is used to identify this metadata resource when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this metadata resource is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the metadata resource is stored on different servers.") 061 protected UriType url; 062 063 /** 064 * The identifier that is used to identify this version of the metadata resource 065 * when it is referenced in a specification, model, design or instance. This is 066 * an arbitrary value managed by the metadata resource author and is not 067 * expected to be globally unique. For example, it might be a timestamp (e.g. 068 * yyyymmdd) if a managed version is not available. There is also no expectation 069 * that versions can be placed in a lexicographical sequence. 070 */ 071 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Business version of the metadata resource", formalDefinition = "The identifier that is used to identify this version of the metadata resource when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the metadata resource author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.") 073 protected StringType version; 074 075 /** 076 * A natural language name identifying the metadata resource. This name should 077 * be usable as an identifier for the module by machine processing applications 078 * such as code generation. 079 */ 080 @Child(name = "name", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 081 @Description(shortDefinition = "Name for this metadata resource (computer friendly)", formalDefinition = "A natural language name identifying the metadata resource. This name should be usable as an identifier for the module by machine processing applications such as code generation.") 082 protected StringType name; 083 084 /** 085 * A short, descriptive, user-friendly title for the metadata resource. 086 */ 087 @Child(name = "title", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 088 @Description(shortDefinition = "Name for this metadata resource (human friendly)", formalDefinition = "A short, descriptive, user-friendly title for the metadata resource.") 089 protected StringType title; 090 091 /** 092 * The status of this metadata resource. Enables tracking the life-cycle of the 093 * content. 094 */ 095 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = true, summary = true) 096 @Description(shortDefinition = "draft | active | retired | unknown", formalDefinition = "The status of this metadata resource. Enables tracking the life-cycle of the content.") 097 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/publication-status") 098 protected Enumeration<PublicationStatus> status; 099 100 /** 101 * A Boolean value to indicate that this metadata resource is authored for 102 * testing purposes (or education/evaluation/marketing) and is not intended to 103 * be used for genuine usage. 104 */ 105 @Child(name = "experimental", type = { 106 BooleanType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 107 @Description(shortDefinition = "For testing purposes, not real usage", formalDefinition = "A Boolean value to indicate that this metadata resource is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.") 108 protected BooleanType experimental; 109 110 /** 111 * The date (and optionally time) when the metadata resource was published. The 112 * date must change when the business version changes and it must change if the 113 * status code changes. In addition, it should change when the substantive 114 * content of the metadata resource changes. 115 */ 116 @Child(name = "date", type = { DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 117 @Description(shortDefinition = "Date last changed", formalDefinition = "The date (and optionally time) when the metadata resource was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the metadata resource changes.") 118 protected DateTimeType date; 119 120 /** 121 * The name of the organization or individual that published the metadata 122 * resource. 123 */ 124 @Child(name = "publisher", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 125 @Description(shortDefinition = "Name of the publisher (organization or individual)", formalDefinition = "The name of the organization or individual that published the metadata resource.") 126 protected StringType publisher; 127 128 /** 129 * Contact details to assist a user in finding and communicating with the 130 * publisher. 131 */ 132 @Child(name = "contact", type = { 133 ContactDetail.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 134 @Description(shortDefinition = "Contact details for the publisher", formalDefinition = "Contact details to assist a user in finding and communicating with the publisher.") 135 protected List<ContactDetail> contact; 136 137 /** 138 * A free text natural language description of the metadata resource from a 139 * consumer's perspective. 140 */ 141 @Child(name = "description", type = { 142 MarkdownType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 143 @Description(shortDefinition = "Natural language description of the metadata resource", formalDefinition = "A free text natural language description of the metadata resource from a consumer's perspective.") 144 protected MarkdownType description; 145 146 /** 147 * The content was developed with a focus and intent of supporting the contexts 148 * that are listed. These contexts may be general categories (gender, age, ...) 149 * or may be references to specific programs (insurance plans, studies, ...) and 150 * may be used to assist with indexing and searching for appropriate metadata 151 * resource instances. 152 */ 153 @Child(name = "useContext", type = { 154 UsageContext.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 155 @Description(shortDefinition = "The context that the content is intended to support", formalDefinition = "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate metadata resource instances.") 156 protected List<UsageContext> useContext; 157 158 /** 159 * A legal or geographic region in which the metadata resource is intended to be 160 * used. 161 */ 162 @Child(name = "jurisdiction", type = { 163 CodeableConcept.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 164 @Description(shortDefinition = "Intended jurisdiction for metadata resource (if applicable)", formalDefinition = "A legal or geographic region in which the metadata resource is intended to be used.") 165 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/jurisdiction") 166 protected List<CodeableConcept> jurisdiction; 167 168 private static final long serialVersionUID = 1952104592L; 169 170 /** 171 * Constructor 172 */ 173 public MetadataResource() { 174 super(); 175 } 176 177 /** 178 * Constructor 179 */ 180 public MetadataResource(Enumeration<PublicationStatus> status) { 181 super(); 182 this.status = status; 183 } 184 185 /** 186 * @return {@link #url} (An absolute URI that is used to identify this metadata 187 * resource when it is referenced in a specification, model, design or 188 * an instance; also called its canonical identifier. This SHOULD be 189 * globally unique and SHOULD be a literal address at which at which an 190 * authoritative instance of this metadata resource is (or will be) 191 * published. This URL can be the target of a canonical reference. It 192 * SHALL remain the same when the metadata resource is stored on 193 * different servers.). This is the underlying object with id, value and 194 * extensions. The accessor "getUrl" gives direct access to the value 195 */ 196 public UriType getUrlElement() { 197 if (this.url == null) 198 if (Configuration.errorOnAutoCreate()) 199 throw new Error("Attempt to auto-create MetadataResource.url"); 200 else if (Configuration.doAutoCreate()) 201 this.url = new UriType(); // bb 202 return this.url; 203 } 204 205 public boolean hasUrlElement() { 206 return this.url != null && !this.url.isEmpty(); 207 } 208 209 public boolean hasUrl() { 210 return this.url != null && !this.url.isEmpty(); 211 } 212 213 /** 214 * @param value {@link #url} (An absolute URI that is used to identify this 215 * metadata resource when it is referenced in a specification, 216 * model, design or an instance; also called its canonical 217 * identifier. This SHOULD be globally unique and SHOULD be a 218 * literal address at which at which an authoritative instance of 219 * this metadata resource is (or will be) published. This URL can 220 * be the target of a canonical reference. It SHALL remain the same 221 * when the metadata resource is stored on different servers.). 222 * This is the underlying object with id, value and extensions. The 223 * accessor "getUrl" gives direct access to the value 224 */ 225 public MetadataResource setUrlElement(UriType value) { 226 this.url = value; 227 return this; 228 } 229 230 /** 231 * @return An absolute URI that is used to identify this metadata resource when 232 * it is referenced in a specification, model, design or an instance; 233 * also called its canonical identifier. This SHOULD be globally unique 234 * and SHOULD be a literal address at which at which an authoritative 235 * instance of this metadata resource is (or will be) published. This 236 * URL can be the target of a canonical reference. It SHALL remain the 237 * same when the metadata resource is stored on different servers. 238 */ 239 public String getUrl() { 240 return this.url == null ? null : this.url.getValue(); 241 } 242 243 /** 244 * @param value An absolute URI that is used to identify this metadata resource 245 * when it is referenced in a specification, model, design or an 246 * instance; also called its canonical identifier. This SHOULD be 247 * globally unique and SHOULD be a literal address at which at 248 * which an authoritative instance of this metadata resource is (or 249 * will be) published. This URL can be the target of a canonical 250 * reference. It SHALL remain the same when the metadata resource 251 * is stored on different servers. 252 */ 253 public MetadataResource setUrl(String value) { 254 if (Utilities.noString(value)) 255 this.url = null; 256 else { 257 if (this.url == null) 258 this.url = new UriType(); 259 this.url.setValue(value); 260 } 261 return this; 262 } 263 264 /** 265 * @return {@link #version} (The identifier that is used to identify this 266 * version of the metadata resource when it is referenced in a 267 * specification, model, design or instance. This is an arbitrary value 268 * managed by the metadata resource author and is not expected to be 269 * globally unique. For example, it might be a timestamp (e.g. yyyymmdd) 270 * if a managed version is not available. There is also no expectation 271 * that versions can be placed in a lexicographical sequence.). This is 272 * the underlying object with id, value and extensions. The accessor 273 * "getVersion" gives direct access to the value 274 */ 275 public StringType getVersionElement() { 276 if (this.version == null) 277 if (Configuration.errorOnAutoCreate()) 278 throw new Error("Attempt to auto-create MetadataResource.version"); 279 else if (Configuration.doAutoCreate()) 280 this.version = new StringType(); // bb 281 return this.version; 282 } 283 284 public boolean hasVersionElement() { 285 return this.version != null && !this.version.isEmpty(); 286 } 287 288 public boolean hasVersion() { 289 return this.version != null && !this.version.isEmpty(); 290 } 291 292 /** 293 * @param value {@link #version} (The identifier that is used to identify this 294 * version of the metadata resource when it is referenced in a 295 * specification, model, design or instance. This is an arbitrary 296 * value managed by the metadata resource author and is not 297 * expected to be globally unique. For example, it might be a 298 * timestamp (e.g. yyyymmdd) if a managed version is not available. 299 * There is also no expectation that versions can be placed in a 300 * lexicographical sequence.). This is the underlying object with 301 * id, value and extensions. The accessor "getVersion" gives direct 302 * access to the value 303 */ 304 public MetadataResource setVersionElement(StringType value) { 305 this.version = value; 306 return this; 307 } 308 309 /** 310 * @return The identifier that is used to identify this version of the metadata 311 * resource when it is referenced in a specification, model, design or 312 * instance. This is an arbitrary value managed by the metadata resource 313 * author and is not expected to be globally unique. For example, it 314 * might be a timestamp (e.g. yyyymmdd) if a managed version is not 315 * available. There is also no expectation that versions can be placed 316 * in a lexicographical sequence. 317 */ 318 public String getVersion() { 319 return this.version == null ? null : this.version.getValue(); 320 } 321 322 /** 323 * @param value The identifier that is used to identify this version of the 324 * metadata resource when it is referenced in a specification, 325 * model, design or instance. This is an arbitrary value managed by 326 * the metadata resource author and is not expected to be globally 327 * unique. For example, it might be a timestamp (e.g. yyyymmdd) if 328 * a managed version is not available. There is also no expectation 329 * that versions can be placed in a lexicographical sequence. 330 */ 331 public MetadataResource setVersion(String value) { 332 if (Utilities.noString(value)) 333 this.version = null; 334 else { 335 if (this.version == null) 336 this.version = new StringType(); 337 this.version.setValue(value); 338 } 339 return this; 340 } 341 342 /** 343 * @return {@link #name} (A natural language name identifying the metadata 344 * resource. This name should be usable as an identifier for the module 345 * by machine processing applications such as code generation.). This is 346 * the underlying object with id, value and extensions. The accessor 347 * "getName" gives direct access to the value 348 */ 349 public StringType getNameElement() { 350 if (this.name == null) 351 if (Configuration.errorOnAutoCreate()) 352 throw new Error("Attempt to auto-create MetadataResource.name"); 353 else if (Configuration.doAutoCreate()) 354 this.name = new StringType(); // bb 355 return this.name; 356 } 357 358 public boolean hasNameElement() { 359 return this.name != null && !this.name.isEmpty(); 360 } 361 362 public boolean hasName() { 363 return this.name != null && !this.name.isEmpty(); 364 } 365 366 /** 367 * @param value {@link #name} (A natural language name identifying the metadata 368 * resource. This name should be usable as an identifier for the 369 * module by machine processing applications such as code 370 * generation.). This is the underlying object with id, value and 371 * extensions. The accessor "getName" gives direct access to the 372 * value 373 */ 374 public MetadataResource setNameElement(StringType value) { 375 this.name = value; 376 return this; 377 } 378 379 /** 380 * @return A natural language name identifying the metadata resource. This name 381 * should be usable as an identifier for the module by machine 382 * processing applications such as code generation. 383 */ 384 public String getName() { 385 return this.name == null ? null : this.name.getValue(); 386 } 387 388 /** 389 * @param value A natural language name identifying the metadata resource. This 390 * name should be usable as an identifier for the module by machine 391 * processing applications such as code generation. 392 */ 393 public MetadataResource setName(String value) { 394 if (Utilities.noString(value)) 395 this.name = null; 396 else { 397 if (this.name == null) 398 this.name = new StringType(); 399 this.name.setValue(value); 400 } 401 return this; 402 } 403 404 /** 405 * @return {@link #title} (A short, descriptive, user-friendly title for the 406 * metadata resource.). This is the underlying object with id, value and 407 * extensions. The accessor "getTitle" gives direct access to the value 408 */ 409 public StringType getTitleElement() { 410 if (this.title == null) 411 if (Configuration.errorOnAutoCreate()) 412 throw new Error("Attempt to auto-create MetadataResource.title"); 413 else if (Configuration.doAutoCreate()) 414 this.title = new StringType(); // bb 415 return this.title; 416 } 417 418 public boolean hasTitleElement() { 419 return this.title != null && !this.title.isEmpty(); 420 } 421 422 public boolean hasTitle() { 423 return this.title != null && !this.title.isEmpty(); 424 } 425 426 /** 427 * @param value {@link #title} (A short, descriptive, user-friendly title for 428 * the metadata resource.). This is the underlying object with id, 429 * value and extensions. The accessor "getTitle" gives direct 430 * access to the value 431 */ 432 public MetadataResource setTitleElement(StringType value) { 433 this.title = value; 434 return this; 435 } 436 437 /** 438 * @return A short, descriptive, user-friendly title for the metadata resource. 439 */ 440 public String getTitle() { 441 return this.title == null ? null : this.title.getValue(); 442 } 443 444 /** 445 * @param value A short, descriptive, user-friendly title for the metadata 446 * resource. 447 */ 448 public MetadataResource setTitle(String value) { 449 if (Utilities.noString(value)) 450 this.title = null; 451 else { 452 if (this.title == null) 453 this.title = new StringType(); 454 this.title.setValue(value); 455 } 456 return this; 457 } 458 459 /** 460 * @return {@link #status} (The status of this metadata resource. Enables 461 * tracking the life-cycle of the content.). This is the underlying 462 * object with id, value and extensions. The accessor "getStatus" gives 463 * direct access to the value 464 */ 465 public Enumeration<PublicationStatus> getStatusElement() { 466 if (this.status == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create MetadataResource.status"); 469 else if (Configuration.doAutoCreate()) 470 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 471 return this.status; 472 } 473 474 public boolean hasStatusElement() { 475 return this.status != null && !this.status.isEmpty(); 476 } 477 478 public boolean hasStatus() { 479 return this.status != null && !this.status.isEmpty(); 480 } 481 482 /** 483 * @param value {@link #status} (The status of this metadata resource. Enables 484 * tracking the life-cycle of the content.). This is the underlying 485 * object with id, value and extensions. The accessor "getStatus" 486 * gives direct access to the value 487 */ 488 public MetadataResource setStatusElement(Enumeration<PublicationStatus> value) { 489 this.status = value; 490 return this; 491 } 492 493 /** 494 * @return The status of this metadata resource. Enables tracking the life-cycle 495 * of the content. 496 */ 497 public PublicationStatus getStatus() { 498 return this.status == null ? null : this.status.getValue(); 499 } 500 501 /** 502 * @param value The status of this metadata resource. Enables tracking the 503 * life-cycle of the content. 504 */ 505 public MetadataResource setStatus(PublicationStatus value) { 506 if (this.status == null) 507 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 508 this.status.setValue(value); 509 return this; 510 } 511 512 /** 513 * @return {@link #experimental} (A Boolean value to indicate that this metadata 514 * resource is authored for testing purposes (or 515 * education/evaluation/marketing) and is not intended to be used for 516 * genuine usage.). This is the underlying object with id, value and 517 * extensions. The accessor "getExperimental" gives direct access to the 518 * value 519 */ 520 public BooleanType getExperimentalElement() { 521 if (this.experimental == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create MetadataResource.experimental"); 524 else if (Configuration.doAutoCreate()) 525 this.experimental = new BooleanType(); // bb 526 return this.experimental; 527 } 528 529 public boolean hasExperimentalElement() { 530 return this.experimental != null && !this.experimental.isEmpty(); 531 } 532 533 public boolean hasExperimental() { 534 return this.experimental != null && !this.experimental.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #experimental} (A Boolean value to indicate that this 539 * metadata resource is authored for testing purposes (or 540 * education/evaluation/marketing) and is not intended to be used 541 * for genuine usage.). This is the underlying object with id, 542 * value and extensions. The accessor "getExperimental" gives 543 * direct access to the value 544 */ 545 public MetadataResource setExperimentalElement(BooleanType value) { 546 this.experimental = value; 547 return this; 548 } 549 550 /** 551 * @return A Boolean value to indicate that this metadata resource is authored 552 * for testing purposes (or education/evaluation/marketing) and is not 553 * intended to be used for genuine usage. 554 */ 555 public boolean getExperimental() { 556 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 557 } 558 559 /** 560 * @param value A Boolean value to indicate that this metadata resource is 561 * authored for testing purposes (or 562 * education/evaluation/marketing) and is not intended to be used 563 * for genuine usage. 564 */ 565 public MetadataResource setExperimental(boolean value) { 566 if (this.experimental == null) 567 this.experimental = new BooleanType(); 568 this.experimental.setValue(value); 569 return this; 570 } 571 572 /** 573 * @return {@link #date} (The date (and optionally time) when the metadata 574 * resource was published. The date must change when the business 575 * version changes and it must change if the status code changes. In 576 * addition, it should change when the substantive content of the 577 * metadata resource changes.). This is the underlying object with id, 578 * value and extensions. The accessor "getDate" gives direct access to 579 * the value 580 */ 581 public DateTimeType getDateElement() { 582 if (this.date == null) 583 if (Configuration.errorOnAutoCreate()) 584 throw new Error("Attempt to auto-create MetadataResource.date"); 585 else if (Configuration.doAutoCreate()) 586 this.date = new DateTimeType(); // bb 587 return this.date; 588 } 589 590 public boolean hasDateElement() { 591 return this.date != null && !this.date.isEmpty(); 592 } 593 594 public boolean hasDate() { 595 return this.date != null && !this.date.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #date} (The date (and optionally time) when the metadata 600 * resource was published. The date must change when the business 601 * version changes and it must change if the status code changes. 602 * In addition, it should change when the substantive content of 603 * the metadata resource changes.). This is the underlying object 604 * with id, value and extensions. The accessor "getDate" gives 605 * direct access to the value 606 */ 607 public MetadataResource setDateElement(DateTimeType value) { 608 this.date = value; 609 return this; 610 } 611 612 /** 613 * @return The date (and optionally time) when the metadata resource was 614 * published. The date must change when the business version changes and 615 * it must change if the status code changes. In addition, it should 616 * change when the substantive content of the metadata resource changes. 617 */ 618 public Date getDate() { 619 return this.date == null ? null : this.date.getValue(); 620 } 621 622 /** 623 * @param value The date (and optionally time) when the metadata resource was 624 * published. The date must change when the business version 625 * changes and it must change if the status code changes. In 626 * addition, it should change when the substantive content of the 627 * metadata resource changes. 628 */ 629 public MetadataResource setDate(Date value) { 630 if (value == null) 631 this.date = null; 632 else { 633 if (this.date == null) 634 this.date = new DateTimeType(); 635 this.date.setValue(value); 636 } 637 return this; 638 } 639 640 /** 641 * @return {@link #publisher} (The name of the organization or individual that 642 * published the metadata resource.). This is the underlying object with 643 * id, value and extensions. The accessor "getPublisher" gives direct 644 * access to the value 645 */ 646 public StringType getPublisherElement() { 647 if (this.publisher == null) 648 if (Configuration.errorOnAutoCreate()) 649 throw new Error("Attempt to auto-create MetadataResource.publisher"); 650 else if (Configuration.doAutoCreate()) 651 this.publisher = new StringType(); // bb 652 return this.publisher; 653 } 654 655 public boolean hasPublisherElement() { 656 return this.publisher != null && !this.publisher.isEmpty(); 657 } 658 659 public boolean hasPublisher() { 660 return this.publisher != null && !this.publisher.isEmpty(); 661 } 662 663 /** 664 * @param value {@link #publisher} (The name of the organization or individual 665 * that published the metadata resource.). This is the underlying 666 * object with id, value and extensions. The accessor 667 * "getPublisher" gives direct access to the value 668 */ 669 public MetadataResource setPublisherElement(StringType value) { 670 this.publisher = value; 671 return this; 672 } 673 674 /** 675 * @return The name of the organization or individual that published the 676 * metadata resource. 677 */ 678 public String getPublisher() { 679 return this.publisher == null ? null : this.publisher.getValue(); 680 } 681 682 /** 683 * @param value The name of the organization or individual that published the 684 * metadata resource. 685 */ 686 public MetadataResource setPublisher(String value) { 687 if (Utilities.noString(value)) 688 this.publisher = null; 689 else { 690 if (this.publisher == null) 691 this.publisher = new StringType(); 692 this.publisher.setValue(value); 693 } 694 return this; 695 } 696 697 /** 698 * @return {@link #contact} (Contact details to assist a user in finding and 699 * communicating with the publisher.) 700 */ 701 public List<ContactDetail> getContact() { 702 if (this.contact == null) 703 this.contact = new ArrayList<ContactDetail>(); 704 return this.contact; 705 } 706 707 /** 708 * @return Returns a reference to <code>this</code> for easy method chaining 709 */ 710 public MetadataResource setContact(List<ContactDetail> theContact) { 711 this.contact = theContact; 712 return this; 713 } 714 715 public boolean hasContact() { 716 if (this.contact == null) 717 return false; 718 for (ContactDetail item : this.contact) 719 if (!item.isEmpty()) 720 return true; 721 return false; 722 } 723 724 public ContactDetail addContact() { // 3 725 ContactDetail t = new ContactDetail(); 726 if (this.contact == null) 727 this.contact = new ArrayList<ContactDetail>(); 728 this.contact.add(t); 729 return t; 730 } 731 732 public MetadataResource addContact(ContactDetail t) { // 3 733 if (t == null) 734 return this; 735 if (this.contact == null) 736 this.contact = new ArrayList<ContactDetail>(); 737 this.contact.add(t); 738 return this; 739 } 740 741 /** 742 * @return The first repetition of repeating field {@link #contact}, creating it 743 * if it does not already exist 744 */ 745 public ContactDetail getContactFirstRep() { 746 if (getContact().isEmpty()) { 747 addContact(); 748 } 749 return getContact().get(0); 750 } 751 752 /** 753 * @return {@link #description} (A free text natural language description of the 754 * metadata resource from a consumer's perspective.). This is the 755 * underlying object with id, value and extensions. The accessor 756 * "getDescription" gives direct access to the value 757 */ 758 public MarkdownType getDescriptionElement() { 759 if (this.description == null) 760 if (Configuration.errorOnAutoCreate()) 761 throw new Error("Attempt to auto-create MetadataResource.description"); 762 else if (Configuration.doAutoCreate()) 763 this.description = new MarkdownType(); // bb 764 return this.description; 765 } 766 767 public boolean hasDescriptionElement() { 768 return this.description != null && !this.description.isEmpty(); 769 } 770 771 public boolean hasDescription() { 772 return this.description != null && !this.description.isEmpty(); 773 } 774 775 /** 776 * @param value {@link #description} (A free text natural language description 777 * of the metadata resource from a consumer's perspective.). This 778 * is the underlying object with id, value and extensions. The 779 * accessor "getDescription" gives direct access to the value 780 */ 781 public MetadataResource setDescriptionElement(MarkdownType value) { 782 this.description = value; 783 return this; 784 } 785 786 /** 787 * @return A free text natural language description of the metadata resource 788 * from a consumer's perspective. 789 */ 790 public String getDescription() { 791 return this.description == null ? null : this.description.getValue(); 792 } 793 794 /** 795 * @param value A free text natural language description of the metadata 796 * resource from a consumer's perspective. 797 */ 798 public MetadataResource setDescription(String value) { 799 if (value == null) 800 this.description = null; 801 else { 802 if (this.description == null) 803 this.description = new MarkdownType(); 804 this.description.setValue(value); 805 } 806 return this; 807 } 808 809 /** 810 * @return {@link #useContext} (The content was developed with a focus and 811 * intent of supporting the contexts that are listed. These contexts may 812 * be general categories (gender, age, ...) or may be references to 813 * specific programs (insurance plans, studies, ...) and may be used to 814 * assist with indexing and searching for appropriate metadata resource 815 * instances.) 816 */ 817 public List<UsageContext> getUseContext() { 818 if (this.useContext == null) 819 this.useContext = new ArrayList<UsageContext>(); 820 return this.useContext; 821 } 822 823 /** 824 * @return Returns a reference to <code>this</code> for easy method chaining 825 */ 826 public MetadataResource setUseContext(List<UsageContext> theUseContext) { 827 this.useContext = theUseContext; 828 return this; 829 } 830 831 public boolean hasUseContext() { 832 if (this.useContext == null) 833 return false; 834 for (UsageContext item : this.useContext) 835 if (!item.isEmpty()) 836 return true; 837 return false; 838 } 839 840 public UsageContext addUseContext() { // 3 841 UsageContext t = new UsageContext(); 842 if (this.useContext == null) 843 this.useContext = new ArrayList<UsageContext>(); 844 this.useContext.add(t); 845 return t; 846 } 847 848 public MetadataResource addUseContext(UsageContext t) { // 3 849 if (t == null) 850 return this; 851 if (this.useContext == null) 852 this.useContext = new ArrayList<UsageContext>(); 853 this.useContext.add(t); 854 return this; 855 } 856 857 /** 858 * @return The first repetition of repeating field {@link #useContext}, creating 859 * it if it does not already exist 860 */ 861 public UsageContext getUseContextFirstRep() { 862 if (getUseContext().isEmpty()) { 863 addUseContext(); 864 } 865 return getUseContext().get(0); 866 } 867 868 /** 869 * @return {@link #jurisdiction} (A legal or geographic region in which the 870 * metadata resource is intended to be used.) 871 */ 872 public List<CodeableConcept> getJurisdiction() { 873 if (this.jurisdiction == null) 874 this.jurisdiction = new ArrayList<CodeableConcept>(); 875 return this.jurisdiction; 876 } 877 878 /** 879 * @return Returns a reference to <code>this</code> for easy method chaining 880 */ 881 public MetadataResource setJurisdiction(List<CodeableConcept> theJurisdiction) { 882 this.jurisdiction = theJurisdiction; 883 return this; 884 } 885 886 public boolean hasJurisdiction() { 887 if (this.jurisdiction == null) 888 return false; 889 for (CodeableConcept item : this.jurisdiction) 890 if (!item.isEmpty()) 891 return true; 892 return false; 893 } 894 895 public CodeableConcept addJurisdiction() { // 3 896 CodeableConcept t = new CodeableConcept(); 897 if (this.jurisdiction == null) 898 this.jurisdiction = new ArrayList<CodeableConcept>(); 899 this.jurisdiction.add(t); 900 return t; 901 } 902 903 public MetadataResource addJurisdiction(CodeableConcept t) { // 3 904 if (t == null) 905 return this; 906 if (this.jurisdiction == null) 907 this.jurisdiction = new ArrayList<CodeableConcept>(); 908 this.jurisdiction.add(t); 909 return this; 910 } 911 912 /** 913 * @return The first repetition of repeating field {@link #jurisdiction}, 914 * creating it if it does not already exist 915 */ 916 public CodeableConcept getJurisdictionFirstRep() { 917 if (getJurisdiction().isEmpty()) { 918 addJurisdiction(); 919 } 920 return getJurisdiction().get(0); 921 } 922 923 protected void listChildren(List<Property> children) { 924 // todo: add a flag to decide whether to do this... 925 // super.listChildren(children); 926 } 927 928 @Override 929 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 930 switch (hash) { 931 case 116079: 932 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 933 case 351608024: 934 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 935 case 3373707: 936 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 937 case 110371416: 938 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 939 case -892481550: 940 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 941 case -404562712: 942 /* experimental */ return this.experimental == null ? new Base[0] : new Base[] { this.experimental }; // BooleanType 943 case 3076014: 944 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 945 case 1447404028: 946 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 947 case 951526432: 948 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 949 case -1724546052: 950 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 951 case -669707736: 952 /* useContext */ return this.useContext == null ? new Base[0] 953 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 954 case -507075711: 955 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 956 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 957 default: 958 return super.getProperty(hash, name, checkValid); 959 } 960 961 } 962 963 @Override 964 public Base setProperty(int hash, String name, Base value) throws FHIRException { 965 switch (hash) { 966 case 116079: // url 967 this.url = castToUri(value); // UriType 968 return value; 969 case 351608024: // version 970 this.version = castToString(value); // StringType 971 return value; 972 case 3373707: // name 973 this.name = castToString(value); // StringType 974 return value; 975 case 110371416: // title 976 this.title = castToString(value); // StringType 977 return value; 978 case -892481550: // status 979 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 980 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 981 return value; 982 case -404562712: // experimental 983 this.experimental = castToBoolean(value); // BooleanType 984 return value; 985 case 3076014: // date 986 this.date = castToDateTime(value); // DateTimeType 987 return value; 988 case 1447404028: // publisher 989 this.publisher = castToString(value); // StringType 990 return value; 991 case 951526432: // contact 992 this.getContact().add(castToContactDetail(value)); // ContactDetail 993 return value; 994 case -1724546052: // description 995 this.description = castToMarkdown(value); // MarkdownType 996 return value; 997 case -669707736: // useContext 998 this.getUseContext().add(castToUsageContext(value)); // UsageContext 999 return value; 1000 case -507075711: // jurisdiction 1001 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1002 return value; 1003 default: 1004 return super.setProperty(hash, name, value); 1005 } 1006 1007 } 1008 1009 @Override 1010 public Base setProperty(String name, Base value) throws FHIRException { 1011 if (name.equals("url")) { 1012 this.url = castToUri(value); // UriType 1013 } else if (name.equals("version")) { 1014 this.version = castToString(value); // StringType 1015 } else if (name.equals("name")) { 1016 this.name = castToString(value); // StringType 1017 } else if (name.equals("title")) { 1018 this.title = castToString(value); // StringType 1019 } else if (name.equals("status")) { 1020 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1021 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1022 } else if (name.equals("experimental")) { 1023 this.experimental = castToBoolean(value); // BooleanType 1024 } else if (name.equals("date")) { 1025 this.date = castToDateTime(value); // DateTimeType 1026 } else if (name.equals("publisher")) { 1027 this.publisher = castToString(value); // StringType 1028 } else if (name.equals("contact")) { 1029 this.getContact().add(castToContactDetail(value)); 1030 } else if (name.equals("description")) { 1031 this.description = castToMarkdown(value); // MarkdownType 1032 } else if (name.equals("useContext")) { 1033 this.getUseContext().add(castToUsageContext(value)); 1034 } else if (name.equals("jurisdiction")) { 1035 this.getJurisdiction().add(castToCodeableConcept(value)); 1036 } else 1037 return super.setProperty(name, value); 1038 return value; 1039 } 1040 1041 @Override 1042 public void removeChild(String name, Base value) throws FHIRException { 1043 if (name.equals("url")) { 1044 this.url = null; 1045 } else if (name.equals("version")) { 1046 this.version = null; 1047 } else if (name.equals("name")) { 1048 this.name = null; 1049 } else if (name.equals("title")) { 1050 this.title = null; 1051 } else if (name.equals("status")) { 1052 this.status = null; 1053 } else if (name.equals("experimental")) { 1054 this.experimental = null; 1055 } else if (name.equals("date")) { 1056 this.date = null; 1057 } else if (name.equals("publisher")) { 1058 this.publisher = null; 1059 } else if (name.equals("contact")) { 1060 this.getContact().remove(castToContactDetail(value)); 1061 } else if (name.equals("description")) { 1062 this.description = null; 1063 } else if (name.equals("useContext")) { 1064 this.getUseContext().remove(castToUsageContext(value)); 1065 } else if (name.equals("jurisdiction")) { 1066 this.getJurisdiction().remove(castToCodeableConcept(value)); 1067 } else 1068 super.removeChild(name, value); 1069 1070 } 1071 1072 @Override 1073 public Base makeProperty(int hash, String name) throws FHIRException { 1074 switch (hash) { 1075 case 116079: 1076 return getUrlElement(); 1077 case 351608024: 1078 return getVersionElement(); 1079 case 3373707: 1080 return getNameElement(); 1081 case 110371416: 1082 return getTitleElement(); 1083 case -892481550: 1084 return getStatusElement(); 1085 case -404562712: 1086 return getExperimentalElement(); 1087 case 3076014: 1088 return getDateElement(); 1089 case 1447404028: 1090 return getPublisherElement(); 1091 case 951526432: 1092 return addContact(); 1093 case -1724546052: 1094 return getDescriptionElement(); 1095 case -669707736: 1096 return addUseContext(); 1097 case -507075711: 1098 return addJurisdiction(); 1099 default: 1100 return super.makeProperty(hash, name); 1101 } 1102 1103 } 1104 1105 @Override 1106 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1107 switch (hash) { 1108 case 116079: 1109 /* url */ return new String[] { "uri" }; 1110 case 351608024: 1111 /* version */ return new String[] { "string" }; 1112 case 3373707: 1113 /* name */ return new String[] { "string" }; 1114 case 110371416: 1115 /* title */ return new String[] { "string" }; 1116 case -892481550: 1117 /* status */ return new String[] { "code" }; 1118 case -404562712: 1119 /* experimental */ return new String[] { "boolean" }; 1120 case 3076014: 1121 /* date */ return new String[] { "dateTime" }; 1122 case 1447404028: 1123 /* publisher */ return new String[] { "string" }; 1124 case 951526432: 1125 /* contact */ return new String[] { "ContactDetail" }; 1126 case -1724546052: 1127 /* description */ return new String[] { "markdown" }; 1128 case -669707736: 1129 /* useContext */ return new String[] { "UsageContext" }; 1130 case -507075711: 1131 /* jurisdiction */ return new String[] { "CodeableConcept" }; 1132 default: 1133 return super.getTypesForProperty(hash, name); 1134 } 1135 1136 } 1137 1138 @Override 1139 public Base addChild(String name) throws FHIRException { 1140 if (name.equals("url")) { 1141 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.url"); 1142 } else if (name.equals("version")) { 1143 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.version"); 1144 } else if (name.equals("name")) { 1145 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.name"); 1146 } else if (name.equals("title")) { 1147 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.title"); 1148 } else if (name.equals("status")) { 1149 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.status"); 1150 } else if (name.equals("experimental")) { 1151 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.experimental"); 1152 } else if (name.equals("date")) { 1153 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.date"); 1154 } else if (name.equals("publisher")) { 1155 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.publisher"); 1156 } else if (name.equals("contact")) { 1157 return addContact(); 1158 } else if (name.equals("description")) { 1159 throw new FHIRException("Cannot call addChild on a singleton property MetadataResource.description"); 1160 } else if (name.equals("useContext")) { 1161 return addUseContext(); 1162 } else if (name.equals("jurisdiction")) { 1163 return addJurisdiction(); 1164 } else 1165 return super.addChild(name); 1166 } 1167 1168 public String fhirType() { 1169 return "MetadataResource"; 1170 1171 } 1172 1173 public abstract MetadataResource copy(); 1174 1175 public void copyValues(MetadataResource dst) { 1176 super.copyValues(dst); 1177 dst.url = url == null ? null : url.copy(); 1178 dst.version = version == null ? null : version.copy(); 1179 dst.name = name == null ? null : name.copy(); 1180 dst.title = title == null ? null : title.copy(); 1181 dst.status = status == null ? null : status.copy(); 1182 dst.experimental = experimental == null ? null : experimental.copy(); 1183 dst.date = date == null ? null : date.copy(); 1184 dst.publisher = publisher == null ? null : publisher.copy(); 1185 if (contact != null) { 1186 dst.contact = new ArrayList<ContactDetail>(); 1187 for (ContactDetail i : contact) 1188 dst.contact.add(i.copy()); 1189 } 1190 ; 1191 dst.description = description == null ? null : description.copy(); 1192 if (useContext != null) { 1193 dst.useContext = new ArrayList<UsageContext>(); 1194 for (UsageContext i : useContext) 1195 dst.useContext.add(i.copy()); 1196 } 1197 ; 1198 if (jurisdiction != null) { 1199 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1200 for (CodeableConcept i : jurisdiction) 1201 dst.jurisdiction.add(i.copy()); 1202 } 1203 ; 1204 } 1205 1206 @Override 1207 public boolean equalsDeep(Base other_) { 1208 if (!super.equalsDeep(other_)) 1209 return false; 1210 if (!(other_ instanceof MetadataResource)) 1211 return false; 1212 MetadataResource o = (MetadataResource) other_; 1213 return compareDeep(url, o.url, true) && compareDeep(version, o.version, true) && compareDeep(name, o.name, true) 1214 && compareDeep(title, o.title, true) && compareDeep(status, o.status, true) 1215 && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 1216 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) 1217 && compareDeep(description, o.description, true) && compareDeep(useContext, o.useContext, true) 1218 && compareDeep(jurisdiction, o.jurisdiction, true); 1219 } 1220 1221 @Override 1222 public boolean equalsShallow(Base other_) { 1223 if (!super.equalsShallow(other_)) 1224 return false; 1225 if (!(other_ instanceof MetadataResource)) 1226 return false; 1227 MetadataResource o = (MetadataResource) other_; 1228 return compareValues(url, o.url, true) && compareValues(version, o.version, true) 1229 && compareValues(name, o.name, true) && compareValues(title, o.title, true) 1230 && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 1231 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 1232 && compareValues(description, o.description, true); 1233 } 1234 1235 public boolean isEmpty() { 1236 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, version, name, title, status, experimental, 1237 date, publisher, contact, description, useContext, jurisdiction); 1238 } 1239 1240// added from java-adornments.txt: 1241 @Override 1242 public String toString() { 1243 return fhirType() + "[" + getUrl() + "]"; 1244 } 1245 1246 public String present() { 1247 if (hasTitle()) 1248 return getTitle(); 1249 if (hasName()) 1250 return getName(); 1251 return toString(); 1252 } 1253 1254// end addition 1255 1256}