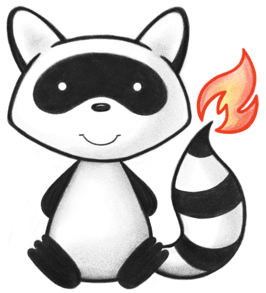
001package org.hl7.fhir.r4.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * Raw data describing a biological sequence. 050 */ 051@ResourceDef(name = "MolecularSequence", profile = "http://hl7.org/fhir/StructureDefinition/MolecularSequence") 052public class MolecularSequence extends DomainResource { 053 054 public enum SequenceType { 055 /** 056 * Amino acid sequence. 057 */ 058 AA, 059 /** 060 * DNA Sequence. 061 */ 062 DNA, 063 /** 064 * RNA Sequence. 065 */ 066 RNA, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 072 public static SequenceType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("aa".equals(codeString)) 076 return AA; 077 if ("dna".equals(codeString)) 078 return DNA; 079 if ("rna".equals(codeString)) 080 return RNA; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown SequenceType code '" + codeString + "'"); 085 } 086 087 public String toCode() { 088 switch (this) { 089 case AA: 090 return "aa"; 091 case DNA: 092 return "dna"; 093 case RNA: 094 return "rna"; 095 case NULL: 096 return null; 097 default: 098 return "?"; 099 } 100 } 101 102 public String getSystem() { 103 switch (this) { 104 case AA: 105 return "http://hl7.org/fhir/sequence-type"; 106 case DNA: 107 return "http://hl7.org/fhir/sequence-type"; 108 case RNA: 109 return "http://hl7.org/fhir/sequence-type"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getDefinition() { 118 switch (this) { 119 case AA: 120 return "Amino acid sequence."; 121 case DNA: 122 return "DNA Sequence."; 123 case RNA: 124 return "RNA Sequence."; 125 case NULL: 126 return null; 127 default: 128 return "?"; 129 } 130 } 131 132 public String getDisplay() { 133 switch (this) { 134 case AA: 135 return "AA Sequence"; 136 case DNA: 137 return "DNA Sequence"; 138 case RNA: 139 return "RNA Sequence"; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 } 147 148 public static class SequenceTypeEnumFactory implements EnumFactory<SequenceType> { 149 public SequenceType fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("aa".equals(codeString)) 154 return SequenceType.AA; 155 if ("dna".equals(codeString)) 156 return SequenceType.DNA; 157 if ("rna".equals(codeString)) 158 return SequenceType.RNA; 159 throw new IllegalArgumentException("Unknown SequenceType code '" + codeString + "'"); 160 } 161 162 public Enumeration<SequenceType> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 167 String codeString = code.asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<SequenceType>(this, SequenceType.NULL, code); 170 if ("aa".equals(codeString)) 171 return new Enumeration<SequenceType>(this, SequenceType.AA, code); 172 if ("dna".equals(codeString)) 173 return new Enumeration<SequenceType>(this, SequenceType.DNA, code); 174 if ("rna".equals(codeString)) 175 return new Enumeration<SequenceType>(this, SequenceType.RNA, code); 176 throw new FHIRException("Unknown SequenceType code '" + codeString + "'"); 177 } 178 179 public String toCode(SequenceType code) { 180 if (code == SequenceType.NULL) 181 return null; 182 if (code == SequenceType.AA) 183 return "aa"; 184 if (code == SequenceType.DNA) 185 return "dna"; 186 if (code == SequenceType.RNA) 187 return "rna"; 188 return "?"; 189 } 190 191 public String toSystem(SequenceType code) { 192 return code.getSystem(); 193 } 194 } 195 196 public enum OrientationType { 197 /** 198 * Sense orientation of reference sequence. 199 */ 200 SENSE, 201 /** 202 * Antisense orientation of reference sequence. 203 */ 204 ANTISENSE, 205 /** 206 * added to help the parsers with the generic types 207 */ 208 NULL; 209 210 public static OrientationType fromCode(String codeString) throws FHIRException { 211 if (codeString == null || "".equals(codeString)) 212 return null; 213 if ("sense".equals(codeString)) 214 return SENSE; 215 if ("antisense".equals(codeString)) 216 return ANTISENSE; 217 if (Configuration.isAcceptInvalidEnums()) 218 return null; 219 else 220 throw new FHIRException("Unknown OrientationType code '" + codeString + "'"); 221 } 222 223 public String toCode() { 224 switch (this) { 225 case SENSE: 226 return "sense"; 227 case ANTISENSE: 228 return "antisense"; 229 case NULL: 230 return null; 231 default: 232 return "?"; 233 } 234 } 235 236 public String getSystem() { 237 switch (this) { 238 case SENSE: 239 return "http://hl7.org/fhir/orientation-type"; 240 case ANTISENSE: 241 return "http://hl7.org/fhir/orientation-type"; 242 case NULL: 243 return null; 244 default: 245 return "?"; 246 } 247 } 248 249 public String getDefinition() { 250 switch (this) { 251 case SENSE: 252 return "Sense orientation of reference sequence."; 253 case ANTISENSE: 254 return "Antisense orientation of reference sequence."; 255 case NULL: 256 return null; 257 default: 258 return "?"; 259 } 260 } 261 262 public String getDisplay() { 263 switch (this) { 264 case SENSE: 265 return "Sense orientation of referenceSeq"; 266 case ANTISENSE: 267 return "Antisense orientation of referenceSeq"; 268 case NULL: 269 return null; 270 default: 271 return "?"; 272 } 273 } 274 } 275 276 public static class OrientationTypeEnumFactory implements EnumFactory<OrientationType> { 277 public OrientationType fromCode(String codeString) throws IllegalArgumentException { 278 if (codeString == null || "".equals(codeString)) 279 if (codeString == null || "".equals(codeString)) 280 return null; 281 if ("sense".equals(codeString)) 282 return OrientationType.SENSE; 283 if ("antisense".equals(codeString)) 284 return OrientationType.ANTISENSE; 285 throw new IllegalArgumentException("Unknown OrientationType code '" + codeString + "'"); 286 } 287 288 public Enumeration<OrientationType> fromType(PrimitiveType<?> code) throws FHIRException { 289 if (code == null) 290 return null; 291 if (code.isEmpty()) 292 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 293 String codeString = code.asStringValue(); 294 if (codeString == null || "".equals(codeString)) 295 return new Enumeration<OrientationType>(this, OrientationType.NULL, code); 296 if ("sense".equals(codeString)) 297 return new Enumeration<OrientationType>(this, OrientationType.SENSE, code); 298 if ("antisense".equals(codeString)) 299 return new Enumeration<OrientationType>(this, OrientationType.ANTISENSE, code); 300 throw new FHIRException("Unknown OrientationType code '" + codeString + "'"); 301 } 302 303 public String toCode(OrientationType code) { 304 if (code == OrientationType.NULL) 305 return null; 306 if (code == OrientationType.SENSE) 307 return "sense"; 308 if (code == OrientationType.ANTISENSE) 309 return "antisense"; 310 return "?"; 311 } 312 313 public String toSystem(OrientationType code) { 314 return code.getSystem(); 315 } 316 } 317 318 public enum StrandType { 319 /** 320 * Watson strand of reference sequence. 321 */ 322 WATSON, 323 /** 324 * Crick strand of reference sequence. 325 */ 326 CRICK, 327 /** 328 * added to help the parsers with the generic types 329 */ 330 NULL; 331 332 public static StrandType fromCode(String codeString) throws FHIRException { 333 if (codeString == null || "".equals(codeString)) 334 return null; 335 if ("watson".equals(codeString)) 336 return WATSON; 337 if ("crick".equals(codeString)) 338 return CRICK; 339 if (Configuration.isAcceptInvalidEnums()) 340 return null; 341 else 342 throw new FHIRException("Unknown StrandType code '" + codeString + "'"); 343 } 344 345 public String toCode() { 346 switch (this) { 347 case WATSON: 348 return "watson"; 349 case CRICK: 350 return "crick"; 351 case NULL: 352 return null; 353 default: 354 return "?"; 355 } 356 } 357 358 public String getSystem() { 359 switch (this) { 360 case WATSON: 361 return "http://hl7.org/fhir/strand-type"; 362 case CRICK: 363 return "http://hl7.org/fhir/strand-type"; 364 case NULL: 365 return null; 366 default: 367 return "?"; 368 } 369 } 370 371 public String getDefinition() { 372 switch (this) { 373 case WATSON: 374 return "Watson strand of reference sequence."; 375 case CRICK: 376 return "Crick strand of reference sequence."; 377 case NULL: 378 return null; 379 default: 380 return "?"; 381 } 382 } 383 384 public String getDisplay() { 385 switch (this) { 386 case WATSON: 387 return "Watson strand of referenceSeq"; 388 case CRICK: 389 return "Crick strand of referenceSeq"; 390 case NULL: 391 return null; 392 default: 393 return "?"; 394 } 395 } 396 } 397 398 public static class StrandTypeEnumFactory implements EnumFactory<StrandType> { 399 public StrandType fromCode(String codeString) throws IllegalArgumentException { 400 if (codeString == null || "".equals(codeString)) 401 if (codeString == null || "".equals(codeString)) 402 return null; 403 if ("watson".equals(codeString)) 404 return StrandType.WATSON; 405 if ("crick".equals(codeString)) 406 return StrandType.CRICK; 407 throw new IllegalArgumentException("Unknown StrandType code '" + codeString + "'"); 408 } 409 410 public Enumeration<StrandType> fromType(PrimitiveType<?> code) throws FHIRException { 411 if (code == null) 412 return null; 413 if (code.isEmpty()) 414 return new Enumeration<StrandType>(this, StrandType.NULL, code); 415 String codeString = code.asStringValue(); 416 if (codeString == null || "".equals(codeString)) 417 return new Enumeration<StrandType>(this, StrandType.NULL, code); 418 if ("watson".equals(codeString)) 419 return new Enumeration<StrandType>(this, StrandType.WATSON, code); 420 if ("crick".equals(codeString)) 421 return new Enumeration<StrandType>(this, StrandType.CRICK, code); 422 throw new FHIRException("Unknown StrandType code '" + codeString + "'"); 423 } 424 425 public String toCode(StrandType code) { 426 if (code == StrandType.NULL) 427 return null; 428 if (code == StrandType.WATSON) 429 return "watson"; 430 if (code == StrandType.CRICK) 431 return "crick"; 432 return "?"; 433 } 434 435 public String toSystem(StrandType code) { 436 return code.getSystem(); 437 } 438 } 439 440 public enum QualityType { 441 /** 442 * INDEL Comparison. 443 */ 444 INDEL, 445 /** 446 * SNP Comparison. 447 */ 448 SNP, 449 /** 450 * UNKNOWN Comparison. 451 */ 452 UNKNOWN, 453 /** 454 * added to help the parsers with the generic types 455 */ 456 NULL; 457 458 public static QualityType fromCode(String codeString) throws FHIRException { 459 if (codeString == null || "".equals(codeString)) 460 return null; 461 if ("indel".equals(codeString)) 462 return INDEL; 463 if ("snp".equals(codeString)) 464 return SNP; 465 if ("unknown".equals(codeString)) 466 return UNKNOWN; 467 if (Configuration.isAcceptInvalidEnums()) 468 return null; 469 else 470 throw new FHIRException("Unknown QualityType code '" + codeString + "'"); 471 } 472 473 public String toCode() { 474 switch (this) { 475 case INDEL: 476 return "indel"; 477 case SNP: 478 return "snp"; 479 case UNKNOWN: 480 return "unknown"; 481 case NULL: 482 return null; 483 default: 484 return "?"; 485 } 486 } 487 488 public String getSystem() { 489 switch (this) { 490 case INDEL: 491 return "http://hl7.org/fhir/quality-type"; 492 case SNP: 493 return "http://hl7.org/fhir/quality-type"; 494 case UNKNOWN: 495 return "http://hl7.org/fhir/quality-type"; 496 case NULL: 497 return null; 498 default: 499 return "?"; 500 } 501 } 502 503 public String getDefinition() { 504 switch (this) { 505 case INDEL: 506 return "INDEL Comparison."; 507 case SNP: 508 return "SNP Comparison."; 509 case UNKNOWN: 510 return "UNKNOWN Comparison."; 511 case NULL: 512 return null; 513 default: 514 return "?"; 515 } 516 } 517 518 public String getDisplay() { 519 switch (this) { 520 case INDEL: 521 return "INDEL Comparison"; 522 case SNP: 523 return "SNP Comparison"; 524 case UNKNOWN: 525 return "UNKNOWN Comparison"; 526 case NULL: 527 return null; 528 default: 529 return "?"; 530 } 531 } 532 } 533 534 public static class QualityTypeEnumFactory implements EnumFactory<QualityType> { 535 public QualityType fromCode(String codeString) throws IllegalArgumentException { 536 if (codeString == null || "".equals(codeString)) 537 if (codeString == null || "".equals(codeString)) 538 return null; 539 if ("indel".equals(codeString)) 540 return QualityType.INDEL; 541 if ("snp".equals(codeString)) 542 return QualityType.SNP; 543 if ("unknown".equals(codeString)) 544 return QualityType.UNKNOWN; 545 throw new IllegalArgumentException("Unknown QualityType code '" + codeString + "'"); 546 } 547 548 public Enumeration<QualityType> fromType(PrimitiveType<?> code) throws FHIRException { 549 if (code == null) 550 return null; 551 if (code.isEmpty()) 552 return new Enumeration<QualityType>(this, QualityType.NULL, code); 553 String codeString = code.asStringValue(); 554 if (codeString == null || "".equals(codeString)) 555 return new Enumeration<QualityType>(this, QualityType.NULL, code); 556 if ("indel".equals(codeString)) 557 return new Enumeration<QualityType>(this, QualityType.INDEL, code); 558 if ("snp".equals(codeString)) 559 return new Enumeration<QualityType>(this, QualityType.SNP, code); 560 if ("unknown".equals(codeString)) 561 return new Enumeration<QualityType>(this, QualityType.UNKNOWN, code); 562 throw new FHIRException("Unknown QualityType code '" + codeString + "'"); 563 } 564 565 public String toCode(QualityType code) { 566 if (code == QualityType.NULL) 567 return null; 568 if (code == QualityType.INDEL) 569 return "indel"; 570 if (code == QualityType.SNP) 571 return "snp"; 572 if (code == QualityType.UNKNOWN) 573 return "unknown"; 574 return "?"; 575 } 576 577 public String toSystem(QualityType code) { 578 return code.getSystem(); 579 } 580 } 581 582 public enum RepositoryType { 583 /** 584 * When URL is clicked, the resource can be seen directly (by webpage or by 585 * download link format). 586 */ 587 DIRECTLINK, 588 /** 589 * When the API method (e.g. [base_url]/[parameter]) related with the URL of the 590 * website is executed, the resource can be seen directly (usually in JSON or 591 * XML format). 592 */ 593 OPENAPI, 594 /** 595 * When logged into the website, the resource can be seen. 596 */ 597 LOGIN, 598 /** 599 * When logged in and follow the API in the website related with URL, the 600 * resource can be seen. 601 */ 602 OAUTH, 603 /** 604 * Some other complicated or particular way to get resource from URL. 605 */ 606 OTHER, 607 /** 608 * added to help the parsers with the generic types 609 */ 610 NULL; 611 612 public static RepositoryType fromCode(String codeString) throws FHIRException { 613 if (codeString == null || "".equals(codeString)) 614 return null; 615 if ("directlink".equals(codeString)) 616 return DIRECTLINK; 617 if ("openapi".equals(codeString)) 618 return OPENAPI; 619 if ("login".equals(codeString)) 620 return LOGIN; 621 if ("oauth".equals(codeString)) 622 return OAUTH; 623 if ("other".equals(codeString)) 624 return OTHER; 625 if (Configuration.isAcceptInvalidEnums()) 626 return null; 627 else 628 throw new FHIRException("Unknown RepositoryType code '" + codeString + "'"); 629 } 630 631 public String toCode() { 632 switch (this) { 633 case DIRECTLINK: 634 return "directlink"; 635 case OPENAPI: 636 return "openapi"; 637 case LOGIN: 638 return "login"; 639 case OAUTH: 640 return "oauth"; 641 case OTHER: 642 return "other"; 643 case NULL: 644 return null; 645 default: 646 return "?"; 647 } 648 } 649 650 public String getSystem() { 651 switch (this) { 652 case DIRECTLINK: 653 return "http://hl7.org/fhir/repository-type"; 654 case OPENAPI: 655 return "http://hl7.org/fhir/repository-type"; 656 case LOGIN: 657 return "http://hl7.org/fhir/repository-type"; 658 case OAUTH: 659 return "http://hl7.org/fhir/repository-type"; 660 case OTHER: 661 return "http://hl7.org/fhir/repository-type"; 662 case NULL: 663 return null; 664 default: 665 return "?"; 666 } 667 } 668 669 public String getDefinition() { 670 switch (this) { 671 case DIRECTLINK: 672 return "When URL is clicked, the resource can be seen directly (by webpage or by download link format)."; 673 case OPENAPI: 674 return "When the API method (e.g. [base_url]/[parameter]) related with the URL of the website is executed, the resource can be seen directly (usually in JSON or XML format)."; 675 case LOGIN: 676 return "When logged into the website, the resource can be seen."; 677 case OAUTH: 678 return "When logged in and follow the API in the website related with URL, the resource can be seen."; 679 case OTHER: 680 return "Some other complicated or particular way to get resource from URL."; 681 case NULL: 682 return null; 683 default: 684 return "?"; 685 } 686 } 687 688 public String getDisplay() { 689 switch (this) { 690 case DIRECTLINK: 691 return "Click and see"; 692 case OPENAPI: 693 return "The URL is the RESTful or other kind of API that can access to the result."; 694 case LOGIN: 695 return "Result cannot be access unless an account is logged in"; 696 case OAUTH: 697 return "Result need to be fetched with API and need LOGIN( or cookies are required when visiting the link of resource)"; 698 case OTHER: 699 return "Some other complicated or particular way to get resource from URL."; 700 case NULL: 701 return null; 702 default: 703 return "?"; 704 } 705 } 706 } 707 708 public static class RepositoryTypeEnumFactory implements EnumFactory<RepositoryType> { 709 public RepositoryType fromCode(String codeString) throws IllegalArgumentException { 710 if (codeString == null || "".equals(codeString)) 711 if (codeString == null || "".equals(codeString)) 712 return null; 713 if ("directlink".equals(codeString)) 714 return RepositoryType.DIRECTLINK; 715 if ("openapi".equals(codeString)) 716 return RepositoryType.OPENAPI; 717 if ("login".equals(codeString)) 718 return RepositoryType.LOGIN; 719 if ("oauth".equals(codeString)) 720 return RepositoryType.OAUTH; 721 if ("other".equals(codeString)) 722 return RepositoryType.OTHER; 723 throw new IllegalArgumentException("Unknown RepositoryType code '" + codeString + "'"); 724 } 725 726 public Enumeration<RepositoryType> fromType(PrimitiveType<?> code) throws FHIRException { 727 if (code == null) 728 return null; 729 if (code.isEmpty()) 730 return new Enumeration<RepositoryType>(this, RepositoryType.NULL, code); 731 String codeString = code.asStringValue(); 732 if (codeString == null || "".equals(codeString)) 733 return new Enumeration<RepositoryType>(this, RepositoryType.NULL, code); 734 if ("directlink".equals(codeString)) 735 return new Enumeration<RepositoryType>(this, RepositoryType.DIRECTLINK, code); 736 if ("openapi".equals(codeString)) 737 return new Enumeration<RepositoryType>(this, RepositoryType.OPENAPI, code); 738 if ("login".equals(codeString)) 739 return new Enumeration<RepositoryType>(this, RepositoryType.LOGIN, code); 740 if ("oauth".equals(codeString)) 741 return new Enumeration<RepositoryType>(this, RepositoryType.OAUTH, code); 742 if ("other".equals(codeString)) 743 return new Enumeration<RepositoryType>(this, RepositoryType.OTHER, code); 744 throw new FHIRException("Unknown RepositoryType code '" + codeString + "'"); 745 } 746 747 public String toCode(RepositoryType code) { 748 if (code == RepositoryType.NULL) 749 return null; 750 if (code == RepositoryType.DIRECTLINK) 751 return "directlink"; 752 if (code == RepositoryType.OPENAPI) 753 return "openapi"; 754 if (code == RepositoryType.LOGIN) 755 return "login"; 756 if (code == RepositoryType.OAUTH) 757 return "oauth"; 758 if (code == RepositoryType.OTHER) 759 return "other"; 760 return "?"; 761 } 762 763 public String toSystem(RepositoryType code) { 764 return code.getSystem(); 765 } 766 } 767 768 @Block() 769 public static class MolecularSequenceReferenceSeqComponent extends BackboneElement implements IBaseBackboneElement { 770 /** 771 * Structural unit composed of a nucleic acid molecule which controls its own 772 * replication through the interaction of specific proteins at one or more 773 * origins of replication 774 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)). 775 */ 776 @Child(name = "chromosome", type = { 777 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 778 @Description(shortDefinition = "Chromosome containing genetic finding", formalDefinition = "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).") 779 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/chromosome-human") 780 protected CodeableConcept chromosome; 781 782 /** 783 * The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 784 * 37'. Version number must be included if a versioned release of a primary 785 * build was used. 786 */ 787 @Child(name = "genomeBuild", type = { 788 StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 789 @Description(shortDefinition = "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'", formalDefinition = "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.") 790 protected StringType genomeBuild; 791 792 /** 793 * A relative reference to a DNA strand based on gene orientation. The strand 794 * that contains the open reading frame of the gene is the "sense" strand, and 795 * the opposite complementary strand is the "antisense" strand. 796 */ 797 @Child(name = "orientation", type = { 798 CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 799 @Description(shortDefinition = "sense | antisense", formalDefinition = "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.") 800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/orientation-type") 801 protected Enumeration<OrientationType> orientation; 802 803 /** 804 * Reference identifier of reference sequence submitted to NCBI. It must match 805 * the type in the MolecularSequence.type field. For example, the prefix, ?NG_? 806 * identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, 807 * and ?NP_? for amino acid sequences. 808 */ 809 @Child(name = "referenceSeqId", type = { 810 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 811 @Description(shortDefinition = "Reference identifier", formalDefinition = "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.") 812 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-referenceSeq") 813 protected CodeableConcept referenceSeqId; 814 815 /** 816 * A pointer to another MolecularSequence entity as reference sequence. 817 */ 818 @Child(name = "referenceSeqPointer", type = { 819 MolecularSequence.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 820 @Description(shortDefinition = "A pointer to another MolecularSequence entity as reference sequence", formalDefinition = "A pointer to another MolecularSequence entity as reference sequence.") 821 protected Reference referenceSeqPointer; 822 823 /** 824 * The actual object that is the target of the reference (A pointer to another 825 * MolecularSequence entity as reference sequence.) 826 */ 827 protected MolecularSequence referenceSeqPointerTarget; 828 829 /** 830 * A string like "ACGT". 831 */ 832 @Child(name = "referenceSeqString", type = { 833 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 834 @Description(shortDefinition = "A string to represent reference sequence", formalDefinition = "A string like \"ACGT\".") 835 protected StringType referenceSeqString; 836 837 /** 838 * An absolute reference to a strand. The Watson strand is the strand whose 839 * 5'-end is on the short arm of the chromosome, and the Crick strand as the one 840 * whose 5'-end is on the long arm. 841 */ 842 @Child(name = "strand", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 843 @Description(shortDefinition = "watson | crick", formalDefinition = "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.") 844 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/strand-type") 845 protected Enumeration<StrandType> strand; 846 847 /** 848 * Start position of the window on the reference sequence. If the coordinate 849 * system is either 0-based or 1-based, then start position is inclusive. 850 */ 851 @Child(name = "windowStart", type = { 852 IntegerType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 853 @Description(shortDefinition = "Start position of the window on the reference sequence", formalDefinition = "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 854 protected IntegerType windowStart; 855 856 /** 857 * End position of the window on the reference sequence. If the coordinate 858 * system is 0-based then end is exclusive and does not include the last 859 * position. If the coordinate system is 1-base, then end is inclusive and 860 * includes the last position. 861 */ 862 @Child(name = "windowEnd", type = { 863 IntegerType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 864 @Description(shortDefinition = "End position of the window on the reference sequence", formalDefinition = "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 865 protected IntegerType windowEnd; 866 867 private static final long serialVersionUID = 307364267L; 868 869 /** 870 * Constructor 871 */ 872 public MolecularSequenceReferenceSeqComponent() { 873 super(); 874 } 875 876 /** 877 * @return {@link #chromosome} (Structural unit composed of a nucleic acid 878 * molecule which controls its own replication through the interaction 879 * of specific proteins at one or more origins of replication 880 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 881 */ 882 public CodeableConcept getChromosome() { 883 if (this.chromosome == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.chromosome"); 886 else if (Configuration.doAutoCreate()) 887 this.chromosome = new CodeableConcept(); // cc 888 return this.chromosome; 889 } 890 891 public boolean hasChromosome() { 892 return this.chromosome != null && !this.chromosome.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #chromosome} (Structural unit composed of a nucleic acid 897 * molecule which controls its own replication through the 898 * interaction of specific proteins at one or more origins of 899 * replication 900 * ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).) 901 */ 902 public MolecularSequenceReferenceSeqComponent setChromosome(CodeableConcept value) { 903 this.chromosome = value; 904 return this; 905 } 906 907 /** 908 * @return {@link #genomeBuild} (The Genome Build used for reference, following 909 * GRCh build versions e.g. 'GRCh 37'. Version number must be included 910 * if a versioned release of a primary build was used.). This is the 911 * underlying object with id, value and extensions. The accessor 912 * "getGenomeBuild" gives direct access to the value 913 */ 914 public StringType getGenomeBuildElement() { 915 if (this.genomeBuild == null) 916 if (Configuration.errorOnAutoCreate()) 917 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.genomeBuild"); 918 else if (Configuration.doAutoCreate()) 919 this.genomeBuild = new StringType(); // bb 920 return this.genomeBuild; 921 } 922 923 public boolean hasGenomeBuildElement() { 924 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 925 } 926 927 public boolean hasGenomeBuild() { 928 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 929 } 930 931 /** 932 * @param value {@link #genomeBuild} (The Genome Build used for reference, 933 * following GRCh build versions e.g. 'GRCh 37'. Version number 934 * must be included if a versioned release of a primary build was 935 * used.). This is the underlying object with id, value and 936 * extensions. The accessor "getGenomeBuild" gives direct access to 937 * the value 938 */ 939 public MolecularSequenceReferenceSeqComponent setGenomeBuildElement(StringType value) { 940 this.genomeBuild = value; 941 return this; 942 } 943 944 /** 945 * @return The Genome Build used for reference, following GRCh build versions 946 * e.g. 'GRCh 37'. Version number must be included if a versioned 947 * release of a primary build was used. 948 */ 949 public String getGenomeBuild() { 950 return this.genomeBuild == null ? null : this.genomeBuild.getValue(); 951 } 952 953 /** 954 * @param value The Genome Build used for reference, following GRCh build 955 * versions e.g. 'GRCh 37'. Version number must be included if a 956 * versioned release of a primary build was used. 957 */ 958 public MolecularSequenceReferenceSeqComponent setGenomeBuild(String value) { 959 if (Utilities.noString(value)) 960 this.genomeBuild = null; 961 else { 962 if (this.genomeBuild == null) 963 this.genomeBuild = new StringType(); 964 this.genomeBuild.setValue(value); 965 } 966 return this; 967 } 968 969 /** 970 * @return {@link #orientation} (A relative reference to a DNA strand based on 971 * gene orientation. The strand that contains the open reading frame of 972 * the gene is the "sense" strand, and the opposite complementary strand 973 * is the "antisense" strand.). This is the underlying object with id, 974 * value and extensions. The accessor "getOrientation" gives direct 975 * access to the value 976 */ 977 public Enumeration<OrientationType> getOrientationElement() { 978 if (this.orientation == null) 979 if (Configuration.errorOnAutoCreate()) 980 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.orientation"); 981 else if (Configuration.doAutoCreate()) 982 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); // bb 983 return this.orientation; 984 } 985 986 public boolean hasOrientationElement() { 987 return this.orientation != null && !this.orientation.isEmpty(); 988 } 989 990 public boolean hasOrientation() { 991 return this.orientation != null && !this.orientation.isEmpty(); 992 } 993 994 /** 995 * @param value {@link #orientation} (A relative reference to a DNA strand based 996 * on gene orientation. The strand that contains the open reading 997 * frame of the gene is the "sense" strand, and the opposite 998 * complementary strand is the "antisense" strand.). This is the 999 * underlying object with id, value and extensions. The accessor 1000 * "getOrientation" gives direct access to the value 1001 */ 1002 public MolecularSequenceReferenceSeqComponent setOrientationElement(Enumeration<OrientationType> value) { 1003 this.orientation = value; 1004 return this; 1005 } 1006 1007 /** 1008 * @return A relative reference to a DNA strand based on gene orientation. The 1009 * strand that contains the open reading frame of the gene is the 1010 * "sense" strand, and the opposite complementary strand is the 1011 * "antisense" strand. 1012 */ 1013 public OrientationType getOrientation() { 1014 return this.orientation == null ? null : this.orientation.getValue(); 1015 } 1016 1017 /** 1018 * @param value A relative reference to a DNA strand based on gene orientation. 1019 * The strand that contains the open reading frame of the gene is 1020 * the "sense" strand, and the opposite complementary strand is the 1021 * "antisense" strand. 1022 */ 1023 public MolecularSequenceReferenceSeqComponent setOrientation(OrientationType value) { 1024 if (value == null) 1025 this.orientation = null; 1026 else { 1027 if (this.orientation == null) 1028 this.orientation = new Enumeration<OrientationType>(new OrientationTypeEnumFactory()); 1029 this.orientation.setValue(value); 1030 } 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #referenceSeqId} (Reference identifier of reference sequence 1036 * submitted to NCBI. It must match the type in the 1037 * MolecularSequence.type field. For example, the prefix, ?NG_? 1038 * identifies reference sequence for genes, ?NM_? for messenger RNA 1039 * transcripts, and ?NP_? for amino acid sequences.) 1040 */ 1041 public CodeableConcept getReferenceSeqId() { 1042 if (this.referenceSeqId == null) 1043 if (Configuration.errorOnAutoCreate()) 1044 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqId"); 1045 else if (Configuration.doAutoCreate()) 1046 this.referenceSeqId = new CodeableConcept(); // cc 1047 return this.referenceSeqId; 1048 } 1049 1050 public boolean hasReferenceSeqId() { 1051 return this.referenceSeqId != null && !this.referenceSeqId.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #referenceSeqId} (Reference identifier of reference 1056 * sequence submitted to NCBI. It must match the type in the 1057 * MolecularSequence.type field. For example, the prefix, ?NG_? 1058 * identifies reference sequence for genes, ?NM_? for messenger RNA 1059 * transcripts, and ?NP_? for amino acid sequences.) 1060 */ 1061 public MolecularSequenceReferenceSeqComponent setReferenceSeqId(CodeableConcept value) { 1062 this.referenceSeqId = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #referenceSeqPointer} (A pointer to another MolecularSequence 1068 * entity as reference sequence.) 1069 */ 1070 public Reference getReferenceSeqPointer() { 1071 if (this.referenceSeqPointer == null) 1072 if (Configuration.errorOnAutoCreate()) 1073 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqPointer"); 1074 else if (Configuration.doAutoCreate()) 1075 this.referenceSeqPointer = new Reference(); // cc 1076 return this.referenceSeqPointer; 1077 } 1078 1079 public boolean hasReferenceSeqPointer() { 1080 return this.referenceSeqPointer != null && !this.referenceSeqPointer.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #referenceSeqPointer} (A pointer to another 1085 * MolecularSequence entity as reference sequence.) 1086 */ 1087 public MolecularSequenceReferenceSeqComponent setReferenceSeqPointer(Reference value) { 1088 this.referenceSeqPointer = value; 1089 return this; 1090 } 1091 1092 /** 1093 * @return {@link #referenceSeqPointer} The actual object that is the target of 1094 * the reference. The reference library doesn't populate this, but you 1095 * can use it to hold the resource if you resolve it. (A pointer to 1096 * another MolecularSequence entity as reference sequence.) 1097 */ 1098 public MolecularSequence getReferenceSeqPointerTarget() { 1099 if (this.referenceSeqPointerTarget == null) 1100 if (Configuration.errorOnAutoCreate()) 1101 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqPointer"); 1102 else if (Configuration.doAutoCreate()) 1103 this.referenceSeqPointerTarget = new MolecularSequence(); // aa 1104 return this.referenceSeqPointerTarget; 1105 } 1106 1107 /** 1108 * @param value {@link #referenceSeqPointer} The actual object that is the 1109 * target of the reference. The reference library doesn't use 1110 * these, but you can use it to hold the resource if you resolve 1111 * it. (A pointer to another MolecularSequence entity as reference 1112 * sequence.) 1113 */ 1114 public MolecularSequenceReferenceSeqComponent setReferenceSeqPointerTarget(MolecularSequence value) { 1115 this.referenceSeqPointerTarget = value; 1116 return this; 1117 } 1118 1119 /** 1120 * @return {@link #referenceSeqString} (A string like "ACGT".). This is the 1121 * underlying object with id, value and extensions. The accessor 1122 * "getReferenceSeqString" gives direct access to the value 1123 */ 1124 public StringType getReferenceSeqStringElement() { 1125 if (this.referenceSeqString == null) 1126 if (Configuration.errorOnAutoCreate()) 1127 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.referenceSeqString"); 1128 else if (Configuration.doAutoCreate()) 1129 this.referenceSeqString = new StringType(); // bb 1130 return this.referenceSeqString; 1131 } 1132 1133 public boolean hasReferenceSeqStringElement() { 1134 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 1135 } 1136 1137 public boolean hasReferenceSeqString() { 1138 return this.referenceSeqString != null && !this.referenceSeqString.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #referenceSeqString} (A string like "ACGT".). This is the 1143 * underlying object with id, value and extensions. The accessor 1144 * "getReferenceSeqString" gives direct access to the value 1145 */ 1146 public MolecularSequenceReferenceSeqComponent setReferenceSeqStringElement(StringType value) { 1147 this.referenceSeqString = value; 1148 return this; 1149 } 1150 1151 /** 1152 * @return A string like "ACGT". 1153 */ 1154 public String getReferenceSeqString() { 1155 return this.referenceSeqString == null ? null : this.referenceSeqString.getValue(); 1156 } 1157 1158 /** 1159 * @param value A string like "ACGT". 1160 */ 1161 public MolecularSequenceReferenceSeqComponent setReferenceSeqString(String value) { 1162 if (Utilities.noString(value)) 1163 this.referenceSeqString = null; 1164 else { 1165 if (this.referenceSeqString == null) 1166 this.referenceSeqString = new StringType(); 1167 this.referenceSeqString.setValue(value); 1168 } 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #strand} (An absolute reference to a strand. The Watson strand 1174 * is the strand whose 5'-end is on the short arm of the chromosome, and 1175 * the Crick strand as the one whose 5'-end is on the long arm.). This 1176 * is the underlying object with id, value and extensions. The accessor 1177 * "getStrand" gives direct access to the value 1178 */ 1179 public Enumeration<StrandType> getStrandElement() { 1180 if (this.strand == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.strand"); 1183 else if (Configuration.doAutoCreate()) 1184 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); // bb 1185 return this.strand; 1186 } 1187 1188 public boolean hasStrandElement() { 1189 return this.strand != null && !this.strand.isEmpty(); 1190 } 1191 1192 public boolean hasStrand() { 1193 return this.strand != null && !this.strand.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #strand} (An absolute reference to a strand. The Watson 1198 * strand is the strand whose 5'-end is on the short arm of the 1199 * chromosome, and the Crick strand as the one whose 5'-end is on 1200 * the long arm.). This is the underlying object with id, value and 1201 * extensions. The accessor "getStrand" gives direct access to the 1202 * value 1203 */ 1204 public MolecularSequenceReferenceSeqComponent setStrandElement(Enumeration<StrandType> value) { 1205 this.strand = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return An absolute reference to a strand. The Watson strand is the strand 1211 * whose 5'-end is on the short arm of the chromosome, and the Crick 1212 * strand as the one whose 5'-end is on the long arm. 1213 */ 1214 public StrandType getStrand() { 1215 return this.strand == null ? null : this.strand.getValue(); 1216 } 1217 1218 /** 1219 * @param value An absolute reference to a strand. The Watson strand is the 1220 * strand whose 5'-end is on the short arm of the chromosome, and 1221 * the Crick strand as the one whose 5'-end is on the long arm. 1222 */ 1223 public MolecularSequenceReferenceSeqComponent setStrand(StrandType value) { 1224 if (value == null) 1225 this.strand = null; 1226 else { 1227 if (this.strand == null) 1228 this.strand = new Enumeration<StrandType>(new StrandTypeEnumFactory()); 1229 this.strand.setValue(value); 1230 } 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #windowStart} (Start position of the window on the reference 1236 * sequence. If the coordinate system is either 0-based or 1-based, then 1237 * start position is inclusive.). This is the underlying object with id, 1238 * value and extensions. The accessor "getWindowStart" gives direct 1239 * access to the value 1240 */ 1241 public IntegerType getWindowStartElement() { 1242 if (this.windowStart == null) 1243 if (Configuration.errorOnAutoCreate()) 1244 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.windowStart"); 1245 else if (Configuration.doAutoCreate()) 1246 this.windowStart = new IntegerType(); // bb 1247 return this.windowStart; 1248 } 1249 1250 public boolean hasWindowStartElement() { 1251 return this.windowStart != null && !this.windowStart.isEmpty(); 1252 } 1253 1254 public boolean hasWindowStart() { 1255 return this.windowStart != null && !this.windowStart.isEmpty(); 1256 } 1257 1258 /** 1259 * @param value {@link #windowStart} (Start position of the window on the 1260 * reference sequence. If the coordinate system is either 0-based 1261 * or 1-based, then start position is inclusive.). This is the 1262 * underlying object with id, value and extensions. The accessor 1263 * "getWindowStart" gives direct access to the value 1264 */ 1265 public MolecularSequenceReferenceSeqComponent setWindowStartElement(IntegerType value) { 1266 this.windowStart = value; 1267 return this; 1268 } 1269 1270 /** 1271 * @return Start position of the window on the reference sequence. If the 1272 * coordinate system is either 0-based or 1-based, then start position 1273 * is inclusive. 1274 */ 1275 public int getWindowStart() { 1276 return this.windowStart == null || this.windowStart.isEmpty() ? 0 : this.windowStart.getValue(); 1277 } 1278 1279 /** 1280 * @param value Start position of the window on the reference sequence. If the 1281 * coordinate system is either 0-based or 1-based, then start 1282 * position is inclusive. 1283 */ 1284 public MolecularSequenceReferenceSeqComponent setWindowStart(int value) { 1285 if (this.windowStart == null) 1286 this.windowStart = new IntegerType(); 1287 this.windowStart.setValue(value); 1288 return this; 1289 } 1290 1291 /** 1292 * @return {@link #windowEnd} (End position of the window on the reference 1293 * sequence. If the coordinate system is 0-based then end is exclusive 1294 * and does not include the last position. If the coordinate system is 1295 * 1-base, then end is inclusive and includes the last position.). This 1296 * is the underlying object with id, value and extensions. The accessor 1297 * "getWindowEnd" gives direct access to the value 1298 */ 1299 public IntegerType getWindowEndElement() { 1300 if (this.windowEnd == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create MolecularSequenceReferenceSeqComponent.windowEnd"); 1303 else if (Configuration.doAutoCreate()) 1304 this.windowEnd = new IntegerType(); // bb 1305 return this.windowEnd; 1306 } 1307 1308 public boolean hasWindowEndElement() { 1309 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1310 } 1311 1312 public boolean hasWindowEnd() { 1313 return this.windowEnd != null && !this.windowEnd.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #windowEnd} (End position of the window on the reference 1318 * sequence. If the coordinate system is 0-based then end is 1319 * exclusive and does not include the last position. If the 1320 * coordinate system is 1-base, then end is inclusive and includes 1321 * the last position.). This is the underlying object with id, 1322 * value and extensions. The accessor "getWindowEnd" gives direct 1323 * access to the value 1324 */ 1325 public MolecularSequenceReferenceSeqComponent setWindowEndElement(IntegerType value) { 1326 this.windowEnd = value; 1327 return this; 1328 } 1329 1330 /** 1331 * @return End position of the window on the reference sequence. If the 1332 * coordinate system is 0-based then end is exclusive and does not 1333 * include the last position. If the coordinate system is 1-base, then 1334 * end is inclusive and includes the last position. 1335 */ 1336 public int getWindowEnd() { 1337 return this.windowEnd == null || this.windowEnd.isEmpty() ? 0 : this.windowEnd.getValue(); 1338 } 1339 1340 /** 1341 * @param value End position of the window on the reference sequence. If the 1342 * coordinate system is 0-based then end is exclusive and does not 1343 * include the last position. If the coordinate system is 1-base, 1344 * then end is inclusive and includes the last position. 1345 */ 1346 public MolecularSequenceReferenceSeqComponent setWindowEnd(int value) { 1347 if (this.windowEnd == null) 1348 this.windowEnd = new IntegerType(); 1349 this.windowEnd.setValue(value); 1350 return this; 1351 } 1352 1353 protected void listChildren(List<Property> children) { 1354 super.listChildren(children); 1355 children.add(new Property("chromosome", "CodeableConcept", 1356 "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 1357 0, 1, chromosome)); 1358 children.add(new Property("genomeBuild", "string", 1359 "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 1360 0, 1, genomeBuild)); 1361 children.add(new Property("orientation", "code", 1362 "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 1363 0, 1, orientation)); 1364 children.add(new Property("referenceSeqId", "CodeableConcept", 1365 "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 1366 0, 1, referenceSeqId)); 1367 children.add(new Property("referenceSeqPointer", "Reference(MolecularSequence)", 1368 "A pointer to another MolecularSequence entity as reference sequence.", 0, 1, referenceSeqPointer)); 1369 children.add(new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, referenceSeqString)); 1370 children.add(new Property("strand", "code", 1371 "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 1372 0, 1, strand)); 1373 children.add(new Property("windowStart", "integer", 1374 "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 1375 0, 1, windowStart)); 1376 children.add(new Property("windowEnd", "integer", 1377 "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 1378 0, 1, windowEnd)); 1379 } 1380 1381 @Override 1382 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1383 switch (_hash) { 1384 case -1499470472: 1385 /* chromosome */ return new Property("chromosome", "CodeableConcept", 1386 "Structural unit composed of a nucleic acid molecule which controls its own replication through the interaction of specific proteins at one or more origins of replication ([SO:0000340](http://www.sequenceontology.org/browser/current_svn/term/SO:0000340)).", 1387 0, 1, chromosome); 1388 case 1061239735: 1389 /* genomeBuild */ return new Property("genomeBuild", "string", 1390 "The Genome Build used for reference, following GRCh build versions e.g. 'GRCh 37'. Version number must be included if a versioned release of a primary build was used.", 1391 0, 1, genomeBuild); 1392 case -1439500848: 1393 /* orientation */ return new Property("orientation", "code", 1394 "A relative reference to a DNA strand based on gene orientation. The strand that contains the open reading frame of the gene is the \"sense\" strand, and the opposite complementary strand is the \"antisense\" strand.", 1395 0, 1, orientation); 1396 case -1911500465: 1397 /* referenceSeqId */ return new Property("referenceSeqId", "CodeableConcept", 1398 "Reference identifier of reference sequence submitted to NCBI. It must match the type in the MolecularSequence.type field. For example, the prefix, ?NG_? identifies reference sequence for genes, ?NM_? for messenger RNA transcripts, and ?NP_? for amino acid sequences.", 1399 0, 1, referenceSeqId); 1400 case 1923414665: 1401 /* referenceSeqPointer */ return new Property("referenceSeqPointer", "Reference(MolecularSequence)", 1402 "A pointer to another MolecularSequence entity as reference sequence.", 0, 1, referenceSeqPointer); 1403 case -1648301499: 1404 /* referenceSeqString */ return new Property("referenceSeqString", "string", "A string like \"ACGT\".", 0, 1, 1405 referenceSeqString); 1406 case -891993594: 1407 /* strand */ return new Property("strand", "code", 1408 "An absolute reference to a strand. The Watson strand is the strand whose 5'-end is on the short arm of the chromosome, and the Crick strand as the one whose 5'-end is on the long arm.", 1409 0, 1, strand); 1410 case 1903685202: 1411 /* windowStart */ return new Property("windowStart", "integer", 1412 "Start position of the window on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 1413 0, 1, windowStart); 1414 case -217026869: 1415 /* windowEnd */ return new Property("windowEnd", "integer", 1416 "End position of the window on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 1417 0, 1, windowEnd); 1418 default: 1419 return super.getNamedProperty(_hash, _name, _checkValid); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1426 switch (hash) { 1427 case -1499470472: 1428 /* chromosome */ return this.chromosome == null ? new Base[0] : new Base[] { this.chromosome }; // CodeableConcept 1429 case 1061239735: 1430 /* genomeBuild */ return this.genomeBuild == null ? new Base[0] : new Base[] { this.genomeBuild }; // StringType 1431 case -1439500848: 1432 /* orientation */ return this.orientation == null ? new Base[0] : new Base[] { this.orientation }; // Enumeration<OrientationType> 1433 case -1911500465: 1434 /* referenceSeqId */ return this.referenceSeqId == null ? new Base[0] : new Base[] { this.referenceSeqId }; // CodeableConcept 1435 case 1923414665: 1436 /* referenceSeqPointer */ return this.referenceSeqPointer == null ? new Base[0] 1437 : new Base[] { this.referenceSeqPointer }; // Reference 1438 case -1648301499: 1439 /* referenceSeqString */ return this.referenceSeqString == null ? new Base[0] 1440 : new Base[] { this.referenceSeqString }; // StringType 1441 case -891993594: 1442 /* strand */ return this.strand == null ? new Base[0] : new Base[] { this.strand }; // Enumeration<StrandType> 1443 case 1903685202: 1444 /* windowStart */ return this.windowStart == null ? new Base[0] : new Base[] { this.windowStart }; // IntegerType 1445 case -217026869: 1446 /* windowEnd */ return this.windowEnd == null ? new Base[0] : new Base[] { this.windowEnd }; // IntegerType 1447 default: 1448 return super.getProperty(hash, name, checkValid); 1449 } 1450 1451 } 1452 1453 @Override 1454 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1455 switch (hash) { 1456 case -1499470472: // chromosome 1457 this.chromosome = castToCodeableConcept(value); // CodeableConcept 1458 return value; 1459 case 1061239735: // genomeBuild 1460 this.genomeBuild = castToString(value); // StringType 1461 return value; 1462 case -1439500848: // orientation 1463 value = new OrientationTypeEnumFactory().fromType(castToCode(value)); 1464 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1465 return value; 1466 case -1911500465: // referenceSeqId 1467 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 1468 return value; 1469 case 1923414665: // referenceSeqPointer 1470 this.referenceSeqPointer = castToReference(value); // Reference 1471 return value; 1472 case -1648301499: // referenceSeqString 1473 this.referenceSeqString = castToString(value); // StringType 1474 return value; 1475 case -891993594: // strand 1476 value = new StrandTypeEnumFactory().fromType(castToCode(value)); 1477 this.strand = (Enumeration) value; // Enumeration<StrandType> 1478 return value; 1479 case 1903685202: // windowStart 1480 this.windowStart = castToInteger(value); // IntegerType 1481 return value; 1482 case -217026869: // windowEnd 1483 this.windowEnd = castToInteger(value); // IntegerType 1484 return value; 1485 default: 1486 return super.setProperty(hash, name, value); 1487 } 1488 1489 } 1490 1491 @Override 1492 public Base setProperty(String name, Base value) throws FHIRException { 1493 if (name.equals("chromosome")) { 1494 this.chromosome = castToCodeableConcept(value); // CodeableConcept 1495 } else if (name.equals("genomeBuild")) { 1496 this.genomeBuild = castToString(value); // StringType 1497 } else if (name.equals("orientation")) { 1498 value = new OrientationTypeEnumFactory().fromType(castToCode(value)); 1499 this.orientation = (Enumeration) value; // Enumeration<OrientationType> 1500 } else if (name.equals("referenceSeqId")) { 1501 this.referenceSeqId = castToCodeableConcept(value); // CodeableConcept 1502 } else if (name.equals("referenceSeqPointer")) { 1503 this.referenceSeqPointer = castToReference(value); // Reference 1504 } else if (name.equals("referenceSeqString")) { 1505 this.referenceSeqString = castToString(value); // StringType 1506 } else if (name.equals("strand")) { 1507 value = new StrandTypeEnumFactory().fromType(castToCode(value)); 1508 this.strand = (Enumeration) value; // Enumeration<StrandType> 1509 } else if (name.equals("windowStart")) { 1510 this.windowStart = castToInteger(value); // IntegerType 1511 } else if (name.equals("windowEnd")) { 1512 this.windowEnd = castToInteger(value); // IntegerType 1513 } else 1514 return super.setProperty(name, value); 1515 return value; 1516 } 1517 1518 @Override 1519 public void removeChild(String name, Base value) throws FHIRException { 1520 if (name.equals("chromosome")) { 1521 this.chromosome = null; 1522 } else if (name.equals("genomeBuild")) { 1523 this.genomeBuild = null; 1524 } else if (name.equals("orientation")) { 1525 this.orientation = null; 1526 } else if (name.equals("referenceSeqId")) { 1527 this.referenceSeqId = null; 1528 } else if (name.equals("referenceSeqPointer")) { 1529 this.referenceSeqPointer = null; 1530 } else if (name.equals("referenceSeqString")) { 1531 this.referenceSeqString = null; 1532 } else if (name.equals("strand")) { 1533 this.strand = null; 1534 } else if (name.equals("windowStart")) { 1535 this.windowStart = null; 1536 } else if (name.equals("windowEnd")) { 1537 this.windowEnd = null; 1538 } else 1539 super.removeChild(name, value); 1540 1541 } 1542 1543 @Override 1544 public Base makeProperty(int hash, String name) throws FHIRException { 1545 switch (hash) { 1546 case -1499470472: 1547 return getChromosome(); 1548 case 1061239735: 1549 return getGenomeBuildElement(); 1550 case -1439500848: 1551 return getOrientationElement(); 1552 case -1911500465: 1553 return getReferenceSeqId(); 1554 case 1923414665: 1555 return getReferenceSeqPointer(); 1556 case -1648301499: 1557 return getReferenceSeqStringElement(); 1558 case -891993594: 1559 return getStrandElement(); 1560 case 1903685202: 1561 return getWindowStartElement(); 1562 case -217026869: 1563 return getWindowEndElement(); 1564 default: 1565 return super.makeProperty(hash, name); 1566 } 1567 1568 } 1569 1570 @Override 1571 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1572 switch (hash) { 1573 case -1499470472: 1574 /* chromosome */ return new String[] { "CodeableConcept" }; 1575 case 1061239735: 1576 /* genomeBuild */ return new String[] { "string" }; 1577 case -1439500848: 1578 /* orientation */ return new String[] { "code" }; 1579 case -1911500465: 1580 /* referenceSeqId */ return new String[] { "CodeableConcept" }; 1581 case 1923414665: 1582 /* referenceSeqPointer */ return new String[] { "Reference" }; 1583 case -1648301499: 1584 /* referenceSeqString */ return new String[] { "string" }; 1585 case -891993594: 1586 /* strand */ return new String[] { "code" }; 1587 case 1903685202: 1588 /* windowStart */ return new String[] { "integer" }; 1589 case -217026869: 1590 /* windowEnd */ return new String[] { "integer" }; 1591 default: 1592 return super.getTypesForProperty(hash, name); 1593 } 1594 1595 } 1596 1597 @Override 1598 public Base addChild(String name) throws FHIRException { 1599 if (name.equals("chromosome")) { 1600 this.chromosome = new CodeableConcept(); 1601 return this.chromosome; 1602 } else if (name.equals("genomeBuild")) { 1603 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.genomeBuild"); 1604 } else if (name.equals("orientation")) { 1605 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.orientation"); 1606 } else if (name.equals("referenceSeqId")) { 1607 this.referenceSeqId = new CodeableConcept(); 1608 return this.referenceSeqId; 1609 } else if (name.equals("referenceSeqPointer")) { 1610 this.referenceSeqPointer = new Reference(); 1611 return this.referenceSeqPointer; 1612 } else if (name.equals("referenceSeqString")) { 1613 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.referenceSeqString"); 1614 } else if (name.equals("strand")) { 1615 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.strand"); 1616 } else if (name.equals("windowStart")) { 1617 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.windowStart"); 1618 } else if (name.equals("windowEnd")) { 1619 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.windowEnd"); 1620 } else 1621 return super.addChild(name); 1622 } 1623 1624 public MolecularSequenceReferenceSeqComponent copy() { 1625 MolecularSequenceReferenceSeqComponent dst = new MolecularSequenceReferenceSeqComponent(); 1626 copyValues(dst); 1627 return dst; 1628 } 1629 1630 public void copyValues(MolecularSequenceReferenceSeqComponent dst) { 1631 super.copyValues(dst); 1632 dst.chromosome = chromosome == null ? null : chromosome.copy(); 1633 dst.genomeBuild = genomeBuild == null ? null : genomeBuild.copy(); 1634 dst.orientation = orientation == null ? null : orientation.copy(); 1635 dst.referenceSeqId = referenceSeqId == null ? null : referenceSeqId.copy(); 1636 dst.referenceSeqPointer = referenceSeqPointer == null ? null : referenceSeqPointer.copy(); 1637 dst.referenceSeqString = referenceSeqString == null ? null : referenceSeqString.copy(); 1638 dst.strand = strand == null ? null : strand.copy(); 1639 dst.windowStart = windowStart == null ? null : windowStart.copy(); 1640 dst.windowEnd = windowEnd == null ? null : windowEnd.copy(); 1641 } 1642 1643 @Override 1644 public boolean equalsDeep(Base other_) { 1645 if (!super.equalsDeep(other_)) 1646 return false; 1647 if (!(other_ instanceof MolecularSequenceReferenceSeqComponent)) 1648 return false; 1649 MolecularSequenceReferenceSeqComponent o = (MolecularSequenceReferenceSeqComponent) other_; 1650 return compareDeep(chromosome, o.chromosome, true) && compareDeep(genomeBuild, o.genomeBuild, true) 1651 && compareDeep(orientation, o.orientation, true) && compareDeep(referenceSeqId, o.referenceSeqId, true) 1652 && compareDeep(referenceSeqPointer, o.referenceSeqPointer, true) 1653 && compareDeep(referenceSeqString, o.referenceSeqString, true) && compareDeep(strand, o.strand, true) 1654 && compareDeep(windowStart, o.windowStart, true) && compareDeep(windowEnd, o.windowEnd, true); 1655 } 1656 1657 @Override 1658 public boolean equalsShallow(Base other_) { 1659 if (!super.equalsShallow(other_)) 1660 return false; 1661 if (!(other_ instanceof MolecularSequenceReferenceSeqComponent)) 1662 return false; 1663 MolecularSequenceReferenceSeqComponent o = (MolecularSequenceReferenceSeqComponent) other_; 1664 return compareValues(genomeBuild, o.genomeBuild, true) && compareValues(orientation, o.orientation, true) 1665 && compareValues(referenceSeqString, o.referenceSeqString, true) && compareValues(strand, o.strand, true) 1666 && compareValues(windowStart, o.windowStart, true) && compareValues(windowEnd, o.windowEnd, true); 1667 } 1668 1669 public boolean isEmpty() { 1670 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(chromosome, genomeBuild, orientation, 1671 referenceSeqId, referenceSeqPointer, referenceSeqString, strand, windowStart, windowEnd); 1672 } 1673 1674 public String fhirType() { 1675 return "MolecularSequence.referenceSeq"; 1676 1677 } 1678 1679 } 1680 1681 @Block() 1682 public static class MolecularSequenceVariantComponent extends BackboneElement implements IBaseBackboneElement { 1683 /** 1684 * Start position of the variant on the reference sequence. If the coordinate 1685 * system is either 0-based or 1-based, then start position is inclusive. 1686 */ 1687 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1688 @Description(shortDefinition = "Start position of the variant on the reference sequence", formalDefinition = "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 1689 protected IntegerType start; 1690 1691 /** 1692 * End position of the variant on the reference sequence. If the coordinate 1693 * system is 0-based then end is exclusive and does not include the last 1694 * position. If the coordinate system is 1-base, then end is inclusive and 1695 * includes the last position. 1696 */ 1697 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1698 @Description(shortDefinition = "End position of the variant on the reference sequence", formalDefinition = "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 1699 protected IntegerType end; 1700 1701 /** 1702 * An allele is one of a set of coexisting sequence variants of a gene 1703 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1704 * Nucleotide(s)/amino acids from start position of sequence to stop position of 1705 * sequence on the positive (+) strand of the observed sequence. When the 1706 * sequence type is DNA, it should be the sequence on the positive (+) strand. 1707 * This will lay in the range between variant.start and variant.end. 1708 */ 1709 @Child(name = "observedAllele", type = { 1710 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1711 @Description(shortDefinition = "Allele that was observed", formalDefinition = "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.") 1712 protected StringType observedAllele; 1713 1714 /** 1715 * An allele is one of a set of coexisting sequence variants of a gene 1716 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1717 * Nucleotide(s)/amino acids from start position of sequence to stop position of 1718 * sequence on the positive (+) strand of the reference sequence. When the 1719 * sequence type is DNA, it should be the sequence on the positive (+) strand. 1720 * This will lay in the range between variant.start and variant.end. 1721 */ 1722 @Child(name = "referenceAllele", type = { 1723 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1724 @Description(shortDefinition = "Allele in the reference sequence", formalDefinition = "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.") 1725 protected StringType referenceAllele; 1726 1727 /** 1728 * Extended CIGAR string for aligning the sequence with reference bases. See 1729 * detailed documentation 1730 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 1731 */ 1732 @Child(name = "cigar", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1733 @Description(shortDefinition = "Extended CIGAR string for aligning the sequence with reference bases", formalDefinition = "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).") 1734 protected StringType cigar; 1735 1736 /** 1737 * A pointer to an Observation containing variant information. 1738 */ 1739 @Child(name = "variantPointer", type = { 1740 Observation.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1741 @Description(shortDefinition = "Pointer to observed variant information", formalDefinition = "A pointer to an Observation containing variant information.") 1742 protected Reference variantPointer; 1743 1744 /** 1745 * The actual object that is the target of the reference (A pointer to an 1746 * Observation containing variant information.) 1747 */ 1748 protected Observation variantPointerTarget; 1749 1750 private static final long serialVersionUID = 105611837L; 1751 1752 /** 1753 * Constructor 1754 */ 1755 public MolecularSequenceVariantComponent() { 1756 super(); 1757 } 1758 1759 /** 1760 * @return {@link #start} (Start position of the variant on the reference 1761 * sequence. If the coordinate system is either 0-based or 1-based, then 1762 * start position is inclusive.). This is the underlying object with id, 1763 * value and extensions. The accessor "getStart" gives direct access to 1764 * the value 1765 */ 1766 public IntegerType getStartElement() { 1767 if (this.start == null) 1768 if (Configuration.errorOnAutoCreate()) 1769 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.start"); 1770 else if (Configuration.doAutoCreate()) 1771 this.start = new IntegerType(); // bb 1772 return this.start; 1773 } 1774 1775 public boolean hasStartElement() { 1776 return this.start != null && !this.start.isEmpty(); 1777 } 1778 1779 public boolean hasStart() { 1780 return this.start != null && !this.start.isEmpty(); 1781 } 1782 1783 /** 1784 * @param value {@link #start} (Start position of the variant on the reference 1785 * sequence. If the coordinate system is either 0-based or 1-based, 1786 * then start position is inclusive.). This is the underlying 1787 * object with id, value and extensions. The accessor "getStart" 1788 * gives direct access to the value 1789 */ 1790 public MolecularSequenceVariantComponent setStartElement(IntegerType value) { 1791 this.start = value; 1792 return this; 1793 } 1794 1795 /** 1796 * @return Start position of the variant on the reference sequence. If the 1797 * coordinate system is either 0-based or 1-based, then start position 1798 * is inclusive. 1799 */ 1800 public int getStart() { 1801 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 1802 } 1803 1804 /** 1805 * @param value Start position of the variant on the reference sequence. If the 1806 * coordinate system is either 0-based or 1-based, then start 1807 * position is inclusive. 1808 */ 1809 public MolecularSequenceVariantComponent setStart(int value) { 1810 if (this.start == null) 1811 this.start = new IntegerType(); 1812 this.start.setValue(value); 1813 return this; 1814 } 1815 1816 /** 1817 * @return {@link #end} (End position of the variant on the reference sequence. 1818 * If the coordinate system is 0-based then end is exclusive and does 1819 * not include the last position. If the coordinate system is 1-base, 1820 * then end is inclusive and includes the last position.). This is the 1821 * underlying object with id, value and extensions. The accessor 1822 * "getEnd" gives direct access to the value 1823 */ 1824 public IntegerType getEndElement() { 1825 if (this.end == null) 1826 if (Configuration.errorOnAutoCreate()) 1827 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.end"); 1828 else if (Configuration.doAutoCreate()) 1829 this.end = new IntegerType(); // bb 1830 return this.end; 1831 } 1832 1833 public boolean hasEndElement() { 1834 return this.end != null && !this.end.isEmpty(); 1835 } 1836 1837 public boolean hasEnd() { 1838 return this.end != null && !this.end.isEmpty(); 1839 } 1840 1841 /** 1842 * @param value {@link #end} (End position of the variant on the reference 1843 * sequence. If the coordinate system is 0-based then end is 1844 * exclusive and does not include the last position. If the 1845 * coordinate system is 1-base, then end is inclusive and includes 1846 * the last position.). This is the underlying object with id, 1847 * value and extensions. The accessor "getEnd" gives direct access 1848 * to the value 1849 */ 1850 public MolecularSequenceVariantComponent setEndElement(IntegerType value) { 1851 this.end = value; 1852 return this; 1853 } 1854 1855 /** 1856 * @return End position of the variant on the reference sequence. If the 1857 * coordinate system is 0-based then end is exclusive and does not 1858 * include the last position. If the coordinate system is 1-base, then 1859 * end is inclusive and includes the last position. 1860 */ 1861 public int getEnd() { 1862 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 1863 } 1864 1865 /** 1866 * @param value End position of the variant on the reference sequence. If the 1867 * coordinate system is 0-based then end is exclusive and does not 1868 * include the last position. If the coordinate system is 1-base, 1869 * then end is inclusive and includes the last position. 1870 */ 1871 public MolecularSequenceVariantComponent setEnd(int value) { 1872 if (this.end == null) 1873 this.end = new IntegerType(); 1874 this.end.setValue(value); 1875 return this; 1876 } 1877 1878 /** 1879 * @return {@link #observedAllele} (An allele is one of a set of coexisting 1880 * sequence variants of a gene 1881 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1882 * Nucleotide(s)/amino acids from start position of sequence to stop 1883 * position of sequence on the positive (+) strand of the observed 1884 * sequence. When the sequence type is DNA, it should be the sequence on 1885 * the positive (+) strand. This will lay in the range between 1886 * variant.start and variant.end.). This is the underlying object with 1887 * id, value and extensions. The accessor "getObservedAllele" gives 1888 * direct access to the value 1889 */ 1890 public StringType getObservedAlleleElement() { 1891 if (this.observedAllele == null) 1892 if (Configuration.errorOnAutoCreate()) 1893 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.observedAllele"); 1894 else if (Configuration.doAutoCreate()) 1895 this.observedAllele = new StringType(); // bb 1896 return this.observedAllele; 1897 } 1898 1899 public boolean hasObservedAlleleElement() { 1900 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1901 } 1902 1903 public boolean hasObservedAllele() { 1904 return this.observedAllele != null && !this.observedAllele.isEmpty(); 1905 } 1906 1907 /** 1908 * @param value {@link #observedAllele} (An allele is one of a set of coexisting 1909 * sequence variants of a gene 1910 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1911 * Nucleotide(s)/amino acids from start position of sequence to 1912 * stop position of sequence on the positive (+) strand of the 1913 * observed sequence. When the sequence type is DNA, it should be 1914 * the sequence on the positive (+) strand. This will lay in the 1915 * range between variant.start and variant.end.). This is the 1916 * underlying object with id, value and extensions. The accessor 1917 * "getObservedAllele" gives direct access to the value 1918 */ 1919 public MolecularSequenceVariantComponent setObservedAlleleElement(StringType value) { 1920 this.observedAllele = value; 1921 return this; 1922 } 1923 1924 /** 1925 * @return An allele is one of a set of coexisting sequence variants of a gene 1926 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1927 * Nucleotide(s)/amino acids from start position of sequence to stop 1928 * position of sequence on the positive (+) strand of the observed 1929 * sequence. When the sequence type is DNA, it should be the sequence on 1930 * the positive (+) strand. This will lay in the range between 1931 * variant.start and variant.end. 1932 */ 1933 public String getObservedAllele() { 1934 return this.observedAllele == null ? null : this.observedAllele.getValue(); 1935 } 1936 1937 /** 1938 * @param value An allele is one of a set of coexisting sequence variants of a 1939 * gene 1940 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1941 * Nucleotide(s)/amino acids from start position of sequence to 1942 * stop position of sequence on the positive (+) strand of the 1943 * observed sequence. When the sequence type is DNA, it should be 1944 * the sequence on the positive (+) strand. This will lay in the 1945 * range between variant.start and variant.end. 1946 */ 1947 public MolecularSequenceVariantComponent setObservedAllele(String value) { 1948 if (Utilities.noString(value)) 1949 this.observedAllele = null; 1950 else { 1951 if (this.observedAllele == null) 1952 this.observedAllele = new StringType(); 1953 this.observedAllele.setValue(value); 1954 } 1955 return this; 1956 } 1957 1958 /** 1959 * @return {@link #referenceAllele} (An allele is one of a set of coexisting 1960 * sequence variants of a gene 1961 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1962 * Nucleotide(s)/amino acids from start position of sequence to stop 1963 * position of sequence on the positive (+) strand of the reference 1964 * sequence. When the sequence type is DNA, it should be the sequence on 1965 * the positive (+) strand. This will lay in the range between 1966 * variant.start and variant.end.). This is the underlying object with 1967 * id, value and extensions. The accessor "getReferenceAllele" gives 1968 * direct access to the value 1969 */ 1970 public StringType getReferenceAlleleElement() { 1971 if (this.referenceAllele == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.referenceAllele"); 1974 else if (Configuration.doAutoCreate()) 1975 this.referenceAllele = new StringType(); // bb 1976 return this.referenceAllele; 1977 } 1978 1979 public boolean hasReferenceAlleleElement() { 1980 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1981 } 1982 1983 public boolean hasReferenceAllele() { 1984 return this.referenceAllele != null && !this.referenceAllele.isEmpty(); 1985 } 1986 1987 /** 1988 * @param value {@link #referenceAllele} (An allele is one of a set of 1989 * coexisting sequence variants of a gene 1990 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 1991 * Nucleotide(s)/amino acids from start position of sequence to 1992 * stop position of sequence on the positive (+) strand of the 1993 * reference sequence. When the sequence type is DNA, it should be 1994 * the sequence on the positive (+) strand. This will lay in the 1995 * range between variant.start and variant.end.). This is the 1996 * underlying object with id, value and extensions. The accessor 1997 * "getReferenceAllele" gives direct access to the value 1998 */ 1999 public MolecularSequenceVariantComponent setReferenceAlleleElement(StringType value) { 2000 this.referenceAllele = value; 2001 return this; 2002 } 2003 2004 /** 2005 * @return An allele is one of a set of coexisting sequence variants of a gene 2006 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 2007 * Nucleotide(s)/amino acids from start position of sequence to stop 2008 * position of sequence on the positive (+) strand of the reference 2009 * sequence. When the sequence type is DNA, it should be the sequence on 2010 * the positive (+) strand. This will lay in the range between 2011 * variant.start and variant.end. 2012 */ 2013 public String getReferenceAllele() { 2014 return this.referenceAllele == null ? null : this.referenceAllele.getValue(); 2015 } 2016 2017 /** 2018 * @param value An allele is one of a set of coexisting sequence variants of a 2019 * gene 2020 * ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). 2021 * Nucleotide(s)/amino acids from start position of sequence to 2022 * stop position of sequence on the positive (+) strand of the 2023 * reference sequence. When the sequence type is DNA, it should be 2024 * the sequence on the positive (+) strand. This will lay in the 2025 * range between variant.start and variant.end. 2026 */ 2027 public MolecularSequenceVariantComponent setReferenceAllele(String value) { 2028 if (Utilities.noString(value)) 2029 this.referenceAllele = null; 2030 else { 2031 if (this.referenceAllele == null) 2032 this.referenceAllele = new StringType(); 2033 this.referenceAllele.setValue(value); 2034 } 2035 return this; 2036 } 2037 2038 /** 2039 * @return {@link #cigar} (Extended CIGAR string for aligning the sequence with 2040 * reference bases. See detailed documentation 2041 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). 2042 * This is the underlying object with id, value and extensions. The 2043 * accessor "getCigar" gives direct access to the value 2044 */ 2045 public StringType getCigarElement() { 2046 if (this.cigar == null) 2047 if (Configuration.errorOnAutoCreate()) 2048 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.cigar"); 2049 else if (Configuration.doAutoCreate()) 2050 this.cigar = new StringType(); // bb 2051 return this.cigar; 2052 } 2053 2054 public boolean hasCigarElement() { 2055 return this.cigar != null && !this.cigar.isEmpty(); 2056 } 2057 2058 public boolean hasCigar() { 2059 return this.cigar != null && !this.cigar.isEmpty(); 2060 } 2061 2062 /** 2063 * @param value {@link #cigar} (Extended CIGAR string for aligning the sequence 2064 * with reference bases. See detailed documentation 2065 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).). 2066 * This is the underlying object with id, value and extensions. The 2067 * accessor "getCigar" gives direct access to the value 2068 */ 2069 public MolecularSequenceVariantComponent setCigarElement(StringType value) { 2070 this.cigar = value; 2071 return this; 2072 } 2073 2074 /** 2075 * @return Extended CIGAR string for aligning the sequence with reference bases. 2076 * See detailed documentation 2077 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 2078 */ 2079 public String getCigar() { 2080 return this.cigar == null ? null : this.cigar.getValue(); 2081 } 2082 2083 /** 2084 * @param value Extended CIGAR string for aligning the sequence with reference 2085 * bases. See detailed documentation 2086 * [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm). 2087 */ 2088 public MolecularSequenceVariantComponent setCigar(String value) { 2089 if (Utilities.noString(value)) 2090 this.cigar = null; 2091 else { 2092 if (this.cigar == null) 2093 this.cigar = new StringType(); 2094 this.cigar.setValue(value); 2095 } 2096 return this; 2097 } 2098 2099 /** 2100 * @return {@link #variantPointer} (A pointer to an Observation containing 2101 * variant information.) 2102 */ 2103 public Reference getVariantPointer() { 2104 if (this.variantPointer == null) 2105 if (Configuration.errorOnAutoCreate()) 2106 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.variantPointer"); 2107 else if (Configuration.doAutoCreate()) 2108 this.variantPointer = new Reference(); // cc 2109 return this.variantPointer; 2110 } 2111 2112 public boolean hasVariantPointer() { 2113 return this.variantPointer != null && !this.variantPointer.isEmpty(); 2114 } 2115 2116 /** 2117 * @param value {@link #variantPointer} (A pointer to an Observation containing 2118 * variant information.) 2119 */ 2120 public MolecularSequenceVariantComponent setVariantPointer(Reference value) { 2121 this.variantPointer = value; 2122 return this; 2123 } 2124 2125 /** 2126 * @return {@link #variantPointer} The actual object that is the target of the 2127 * reference. The reference library doesn't populate this, but you can 2128 * use it to hold the resource if you resolve it. (A pointer to an 2129 * Observation containing variant information.) 2130 */ 2131 public Observation getVariantPointerTarget() { 2132 if (this.variantPointerTarget == null) 2133 if (Configuration.errorOnAutoCreate()) 2134 throw new Error("Attempt to auto-create MolecularSequenceVariantComponent.variantPointer"); 2135 else if (Configuration.doAutoCreate()) 2136 this.variantPointerTarget = new Observation(); // aa 2137 return this.variantPointerTarget; 2138 } 2139 2140 /** 2141 * @param value {@link #variantPointer} The actual object that is the target of 2142 * the reference. The reference library doesn't use these, but you 2143 * can use it to hold the resource if you resolve it. (A pointer to 2144 * an Observation containing variant information.) 2145 */ 2146 public MolecularSequenceVariantComponent setVariantPointerTarget(Observation value) { 2147 this.variantPointerTarget = value; 2148 return this; 2149 } 2150 2151 protected void listChildren(List<Property> children) { 2152 super.listChildren(children); 2153 children.add(new Property("start", "integer", 2154 "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 2155 0, 1, start)); 2156 children.add(new Property("end", "integer", 2157 "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 2158 0, 1, end)); 2159 children.add(new Property("observedAllele", "string", 2160 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2161 0, 1, observedAllele)); 2162 children.add(new Property("referenceAllele", "string", 2163 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2164 0, 1, referenceAllele)); 2165 children.add(new Property("cigar", "string", 2166 "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 2167 0, 1, cigar)); 2168 children.add(new Property("variantPointer", "Reference(Observation)", 2169 "A pointer to an Observation containing variant information.", 0, 1, variantPointer)); 2170 } 2171 2172 @Override 2173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2174 switch (_hash) { 2175 case 109757538: 2176 /* start */ return new Property("start", "integer", 2177 "Start position of the variant on the reference sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 2178 0, 1, start); 2179 case 100571: 2180 /* end */ return new Property("end", "integer", 2181 "End position of the variant on the reference sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 2182 0, 1, end); 2183 case -1418745787: 2184 /* observedAllele */ return new Property("observedAllele", "string", 2185 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the observed sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2186 0, 1, observedAllele); 2187 case 364045960: 2188 /* referenceAllele */ return new Property("referenceAllele", "string", 2189 "An allele is one of a set of coexisting sequence variants of a gene ([SO:0001023](http://www.sequenceontology.org/browser/current_svn/term/SO:0001023)). Nucleotide(s)/amino acids from start position of sequence to stop position of sequence on the positive (+) strand of the reference sequence. When the sequence type is DNA, it should be the sequence on the positive (+) strand. This will lay in the range between variant.start and variant.end.", 2190 0, 1, referenceAllele); 2191 case 94658738: 2192 /* cigar */ return new Property("cigar", "string", 2193 "Extended CIGAR string for aligning the sequence with reference bases. See detailed documentation [here](http://support.illumina.com/help/SequencingAnalysisWorkflow/Content/Vault/Informatics/Sequencing_Analysis/CASAVA/swSEQ_mCA_ExtendedCIGARFormat.htm).", 2194 0, 1, cigar); 2195 case -1654319624: 2196 /* variantPointer */ return new Property("variantPointer", "Reference(Observation)", 2197 "A pointer to an Observation containing variant information.", 0, 1, variantPointer); 2198 default: 2199 return super.getNamedProperty(_hash, _name, _checkValid); 2200 } 2201 2202 } 2203 2204 @Override 2205 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2206 switch (hash) { 2207 case 109757538: 2208 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 2209 case 100571: 2210 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 2211 case -1418745787: 2212 /* observedAllele */ return this.observedAllele == null ? new Base[0] : new Base[] { this.observedAllele }; // StringType 2213 case 364045960: 2214 /* referenceAllele */ return this.referenceAllele == null ? new Base[0] : new Base[] { this.referenceAllele }; // StringType 2215 case 94658738: 2216 /* cigar */ return this.cigar == null ? new Base[0] : new Base[] { this.cigar }; // StringType 2217 case -1654319624: 2218 /* variantPointer */ return this.variantPointer == null ? new Base[0] : new Base[] { this.variantPointer }; // Reference 2219 default: 2220 return super.getProperty(hash, name, checkValid); 2221 } 2222 2223 } 2224 2225 @Override 2226 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2227 switch (hash) { 2228 case 109757538: // start 2229 this.start = castToInteger(value); // IntegerType 2230 return value; 2231 case 100571: // end 2232 this.end = castToInteger(value); // IntegerType 2233 return value; 2234 case -1418745787: // observedAllele 2235 this.observedAllele = castToString(value); // StringType 2236 return value; 2237 case 364045960: // referenceAllele 2238 this.referenceAllele = castToString(value); // StringType 2239 return value; 2240 case 94658738: // cigar 2241 this.cigar = castToString(value); // StringType 2242 return value; 2243 case -1654319624: // variantPointer 2244 this.variantPointer = castToReference(value); // Reference 2245 return value; 2246 default: 2247 return super.setProperty(hash, name, value); 2248 } 2249 2250 } 2251 2252 @Override 2253 public Base setProperty(String name, Base value) throws FHIRException { 2254 if (name.equals("start")) { 2255 this.start = castToInteger(value); // IntegerType 2256 } else if (name.equals("end")) { 2257 this.end = castToInteger(value); // IntegerType 2258 } else if (name.equals("observedAllele")) { 2259 this.observedAllele = castToString(value); // StringType 2260 } else if (name.equals("referenceAllele")) { 2261 this.referenceAllele = castToString(value); // StringType 2262 } else if (name.equals("cigar")) { 2263 this.cigar = castToString(value); // StringType 2264 } else if (name.equals("variantPointer")) { 2265 this.variantPointer = castToReference(value); // Reference 2266 } else 2267 return super.setProperty(name, value); 2268 return value; 2269 } 2270 2271 @Override 2272 public void removeChild(String name, Base value) throws FHIRException { 2273 if (name.equals("start")) { 2274 this.start = null; 2275 } else if (name.equals("end")) { 2276 this.end = null; 2277 } else if (name.equals("observedAllele")) { 2278 this.observedAllele = null; 2279 } else if (name.equals("referenceAllele")) { 2280 this.referenceAllele = null; 2281 } else if (name.equals("cigar")) { 2282 this.cigar = null; 2283 } else if (name.equals("variantPointer")) { 2284 this.variantPointer = null; 2285 } else 2286 super.removeChild(name, value); 2287 2288 } 2289 2290 @Override 2291 public Base makeProperty(int hash, String name) throws FHIRException { 2292 switch (hash) { 2293 case 109757538: 2294 return getStartElement(); 2295 case 100571: 2296 return getEndElement(); 2297 case -1418745787: 2298 return getObservedAlleleElement(); 2299 case 364045960: 2300 return getReferenceAlleleElement(); 2301 case 94658738: 2302 return getCigarElement(); 2303 case -1654319624: 2304 return getVariantPointer(); 2305 default: 2306 return super.makeProperty(hash, name); 2307 } 2308 2309 } 2310 2311 @Override 2312 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2313 switch (hash) { 2314 case 109757538: 2315 /* start */ return new String[] { "integer" }; 2316 case 100571: 2317 /* end */ return new String[] { "integer" }; 2318 case -1418745787: 2319 /* observedAllele */ return new String[] { "string" }; 2320 case 364045960: 2321 /* referenceAllele */ return new String[] { "string" }; 2322 case 94658738: 2323 /* cigar */ return new String[] { "string" }; 2324 case -1654319624: 2325 /* variantPointer */ return new String[] { "Reference" }; 2326 default: 2327 return super.getTypesForProperty(hash, name); 2328 } 2329 2330 } 2331 2332 @Override 2333 public Base addChild(String name) throws FHIRException { 2334 if (name.equals("start")) { 2335 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 2336 } else if (name.equals("end")) { 2337 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 2338 } else if (name.equals("observedAllele")) { 2339 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.observedAllele"); 2340 } else if (name.equals("referenceAllele")) { 2341 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.referenceAllele"); 2342 } else if (name.equals("cigar")) { 2343 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.cigar"); 2344 } else if (name.equals("variantPointer")) { 2345 this.variantPointer = new Reference(); 2346 return this.variantPointer; 2347 } else 2348 return super.addChild(name); 2349 } 2350 2351 public MolecularSequenceVariantComponent copy() { 2352 MolecularSequenceVariantComponent dst = new MolecularSequenceVariantComponent(); 2353 copyValues(dst); 2354 return dst; 2355 } 2356 2357 public void copyValues(MolecularSequenceVariantComponent dst) { 2358 super.copyValues(dst); 2359 dst.start = start == null ? null : start.copy(); 2360 dst.end = end == null ? null : end.copy(); 2361 dst.observedAllele = observedAllele == null ? null : observedAllele.copy(); 2362 dst.referenceAllele = referenceAllele == null ? null : referenceAllele.copy(); 2363 dst.cigar = cigar == null ? null : cigar.copy(); 2364 dst.variantPointer = variantPointer == null ? null : variantPointer.copy(); 2365 } 2366 2367 @Override 2368 public boolean equalsDeep(Base other_) { 2369 if (!super.equalsDeep(other_)) 2370 return false; 2371 if (!(other_ instanceof MolecularSequenceVariantComponent)) 2372 return false; 2373 MolecularSequenceVariantComponent o = (MolecularSequenceVariantComponent) other_; 2374 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 2375 && compareDeep(observedAllele, o.observedAllele, true) 2376 && compareDeep(referenceAllele, o.referenceAllele, true) && compareDeep(cigar, o.cigar, true) 2377 && compareDeep(variantPointer, o.variantPointer, true); 2378 } 2379 2380 @Override 2381 public boolean equalsShallow(Base other_) { 2382 if (!super.equalsShallow(other_)) 2383 return false; 2384 if (!(other_ instanceof MolecularSequenceVariantComponent)) 2385 return false; 2386 MolecularSequenceVariantComponent o = (MolecularSequenceVariantComponent) other_; 2387 return compareValues(start, o.start, true) && compareValues(end, o.end, true) 2388 && compareValues(observedAllele, o.observedAllele, true) 2389 && compareValues(referenceAllele, o.referenceAllele, true) && compareValues(cigar, o.cigar, true); 2390 } 2391 2392 public boolean isEmpty() { 2393 return super.isEmpty() 2394 && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end, observedAllele, referenceAllele, cigar, variantPointer); 2395 } 2396 2397 public String fhirType() { 2398 return "MolecularSequence.variant"; 2399 2400 } 2401 2402 } 2403 2404 @Block() 2405 public static class MolecularSequenceQualityComponent extends BackboneElement implements IBaseBackboneElement { 2406 /** 2407 * INDEL / SNP / Undefined variant. 2408 */ 2409 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2410 @Description(shortDefinition = "indel | snp | unknown", formalDefinition = "INDEL / SNP / Undefined variant.") 2411 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/quality-type") 2412 protected Enumeration<QualityType> type; 2413 2414 /** 2415 * Gold standard sequence used for comparing against. 2416 */ 2417 @Child(name = "standardSequence", type = { 2418 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2419 @Description(shortDefinition = "Standard sequence for comparison", formalDefinition = "Gold standard sequence used for comparing against.") 2420 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-quality-standardSequence") 2421 protected CodeableConcept standardSequence; 2422 2423 /** 2424 * Start position of the sequence. If the coordinate system is either 0-based or 2425 * 1-based, then start position is inclusive. 2426 */ 2427 @Child(name = "start", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2428 @Description(shortDefinition = "Start position of the sequence", formalDefinition = "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 2429 protected IntegerType start; 2430 2431 /** 2432 * End position of the sequence. If the coordinate system is 0-based then end is 2433 * exclusive and does not include the last position. If the coordinate system is 2434 * 1-base, then end is inclusive and includes the last position. 2435 */ 2436 @Child(name = "end", type = { IntegerType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2437 @Description(shortDefinition = "End position of the sequence", formalDefinition = "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 2438 protected IntegerType end; 2439 2440 /** 2441 * The score of an experimentally derived feature such as a p-value 2442 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)). 2443 */ 2444 @Child(name = "score", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2445 @Description(shortDefinition = "Quality score for the comparison", formalDefinition = "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).") 2446 protected Quantity score; 2447 2448 /** 2449 * Which method is used to get sequence quality. 2450 */ 2451 @Child(name = "method", type = { 2452 CodeableConcept.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2453 @Description(shortDefinition = "Method to get quality", formalDefinition = "Which method is used to get sequence quality.") 2454 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-quality-method") 2455 protected CodeableConcept method; 2456 2457 /** 2458 * True positives, from the perspective of the truth data, i.e. the number of 2459 * sites in the Truth Call Set for which there are paths through the Query Call 2460 * Set that are consistent with all of the alleles at this site, and for which 2461 * there is an accurate genotype call for the event. 2462 */ 2463 @Child(name = "truthTP", type = { 2464 DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2465 @Description(shortDefinition = "True positives from the perspective of the truth data", formalDefinition = "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.") 2466 protected DecimalType truthTP; 2467 2468 /** 2469 * True positives, from the perspective of the query data, i.e. the number of 2470 * sites in the Query Call Set for which there are paths through the Truth Call 2471 * Set that are consistent with all of the alleles at this site, and for which 2472 * there is an accurate genotype call for the event. 2473 */ 2474 @Child(name = "queryTP", type = { 2475 DecimalType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2476 @Description(shortDefinition = "True positives from the perspective of the query data", formalDefinition = "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.") 2477 protected DecimalType queryTP; 2478 2479 /** 2480 * False negatives, i.e. the number of sites in the Truth Call Set for which 2481 * there is no path through the Query Call Set that is consistent with all of 2482 * the alleles at this site, or sites for which there is an inaccurate genotype 2483 * call for the event. Sites with correct variant but incorrect genotype are 2484 * counted here. 2485 */ 2486 @Child(name = "truthFN", type = { 2487 DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2488 @Description(shortDefinition = "False negatives", formalDefinition = "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.") 2489 protected DecimalType truthFN; 2490 2491 /** 2492 * False positives, i.e. the number of sites in the Query Call Set for which 2493 * there is no path through the Truth Call Set that is consistent with this 2494 * site. Sites with correct variant but incorrect genotype are counted here. 2495 */ 2496 @Child(name = "queryFP", type = { 2497 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 2498 @Description(shortDefinition = "False positives", formalDefinition = "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.") 2499 protected DecimalType queryFP; 2500 2501 /** 2502 * The number of false positives where the non-REF alleles in the Truth and 2503 * Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 2504 * or similar). 2505 */ 2506 @Child(name = "gtFP", type = { DecimalType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 2507 @Description(shortDefinition = "False positives where the non-REF alleles in the Truth and Query Call Sets match", formalDefinition = "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).") 2508 protected DecimalType gtFP; 2509 2510 /** 2511 * QUERY.TP / (QUERY.TP + QUERY.FP). 2512 */ 2513 @Child(name = "precision", type = { 2514 DecimalType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 2515 @Description(shortDefinition = "Precision of comparison", formalDefinition = "QUERY.TP / (QUERY.TP + QUERY.FP).") 2516 protected DecimalType precision; 2517 2518 /** 2519 * TRUTH.TP / (TRUTH.TP + TRUTH.FN). 2520 */ 2521 @Child(name = "recall", type = { 2522 DecimalType.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 2523 @Description(shortDefinition = "Recall of comparison", formalDefinition = "TRUTH.TP / (TRUTH.TP + TRUTH.FN).") 2524 protected DecimalType recall; 2525 2526 /** 2527 * Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / 2528 * (precision + recall). 2529 */ 2530 @Child(name = "fScore", type = { 2531 DecimalType.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 2532 @Description(shortDefinition = "F-score", formalDefinition = "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).") 2533 protected DecimalType fScore; 2534 2535 /** 2536 * Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity 2537 * tradeoff. 2538 */ 2539 @Child(name = "roc", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 2540 @Description(shortDefinition = "Receiver Operator Characteristic (ROC) Curve", formalDefinition = "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.") 2541 protected MolecularSequenceQualityRocComponent roc; 2542 2543 private static final long serialVersionUID = -811933526L; 2544 2545 /** 2546 * Constructor 2547 */ 2548 public MolecularSequenceQualityComponent() { 2549 super(); 2550 } 2551 2552 /** 2553 * Constructor 2554 */ 2555 public MolecularSequenceQualityComponent(Enumeration<QualityType> type) { 2556 super(); 2557 this.type = type; 2558 } 2559 2560 /** 2561 * @return {@link #type} (INDEL / SNP / Undefined variant.). This is the 2562 * underlying object with id, value and extensions. The accessor 2563 * "getType" gives direct access to the value 2564 */ 2565 public Enumeration<QualityType> getTypeElement() { 2566 if (this.type == null) 2567 if (Configuration.errorOnAutoCreate()) 2568 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.type"); 2569 else if (Configuration.doAutoCreate()) 2570 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); // bb 2571 return this.type; 2572 } 2573 2574 public boolean hasTypeElement() { 2575 return this.type != null && !this.type.isEmpty(); 2576 } 2577 2578 public boolean hasType() { 2579 return this.type != null && !this.type.isEmpty(); 2580 } 2581 2582 /** 2583 * @param value {@link #type} (INDEL / SNP / Undefined variant.). This is the 2584 * underlying object with id, value and extensions. The accessor 2585 * "getType" gives direct access to the value 2586 */ 2587 public MolecularSequenceQualityComponent setTypeElement(Enumeration<QualityType> value) { 2588 this.type = value; 2589 return this; 2590 } 2591 2592 /** 2593 * @return INDEL / SNP / Undefined variant. 2594 */ 2595 public QualityType getType() { 2596 return this.type == null ? null : this.type.getValue(); 2597 } 2598 2599 /** 2600 * @param value INDEL / SNP / Undefined variant. 2601 */ 2602 public MolecularSequenceQualityComponent setType(QualityType value) { 2603 if (this.type == null) 2604 this.type = new Enumeration<QualityType>(new QualityTypeEnumFactory()); 2605 this.type.setValue(value); 2606 return this; 2607 } 2608 2609 /** 2610 * @return {@link #standardSequence} (Gold standard sequence used for comparing 2611 * against.) 2612 */ 2613 public CodeableConcept getStandardSequence() { 2614 if (this.standardSequence == null) 2615 if (Configuration.errorOnAutoCreate()) 2616 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.standardSequence"); 2617 else if (Configuration.doAutoCreate()) 2618 this.standardSequence = new CodeableConcept(); // cc 2619 return this.standardSequence; 2620 } 2621 2622 public boolean hasStandardSequence() { 2623 return this.standardSequence != null && !this.standardSequence.isEmpty(); 2624 } 2625 2626 /** 2627 * @param value {@link #standardSequence} (Gold standard sequence used for 2628 * comparing against.) 2629 */ 2630 public MolecularSequenceQualityComponent setStandardSequence(CodeableConcept value) { 2631 this.standardSequence = value; 2632 return this; 2633 } 2634 2635 /** 2636 * @return {@link #start} (Start position of the sequence. If the coordinate 2637 * system is either 0-based or 1-based, then start position is 2638 * inclusive.). This is the underlying object with id, value and 2639 * extensions. The accessor "getStart" gives direct access to the value 2640 */ 2641 public IntegerType getStartElement() { 2642 if (this.start == null) 2643 if (Configuration.errorOnAutoCreate()) 2644 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.start"); 2645 else if (Configuration.doAutoCreate()) 2646 this.start = new IntegerType(); // bb 2647 return this.start; 2648 } 2649 2650 public boolean hasStartElement() { 2651 return this.start != null && !this.start.isEmpty(); 2652 } 2653 2654 public boolean hasStart() { 2655 return this.start != null && !this.start.isEmpty(); 2656 } 2657 2658 /** 2659 * @param value {@link #start} (Start position of the sequence. If the 2660 * coordinate system is either 0-based or 1-based, then start 2661 * position is inclusive.). This is the underlying object with id, 2662 * value and extensions. The accessor "getStart" gives direct 2663 * access to the value 2664 */ 2665 public MolecularSequenceQualityComponent setStartElement(IntegerType value) { 2666 this.start = value; 2667 return this; 2668 } 2669 2670 /** 2671 * @return Start position of the sequence. If the coordinate system is either 2672 * 0-based or 1-based, then start position is inclusive. 2673 */ 2674 public int getStart() { 2675 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 2676 } 2677 2678 /** 2679 * @param value Start position of the sequence. If the coordinate system is 2680 * either 0-based or 1-based, then start position is inclusive. 2681 */ 2682 public MolecularSequenceQualityComponent setStart(int value) { 2683 if (this.start == null) 2684 this.start = new IntegerType(); 2685 this.start.setValue(value); 2686 return this; 2687 } 2688 2689 /** 2690 * @return {@link #end} (End position of the sequence. If the coordinate system 2691 * is 0-based then end is exclusive and does not include the last 2692 * position. If the coordinate system is 1-base, then end is inclusive 2693 * and includes the last position.). This is the underlying object with 2694 * id, value and extensions. The accessor "getEnd" gives direct access 2695 * to the value 2696 */ 2697 public IntegerType getEndElement() { 2698 if (this.end == null) 2699 if (Configuration.errorOnAutoCreate()) 2700 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.end"); 2701 else if (Configuration.doAutoCreate()) 2702 this.end = new IntegerType(); // bb 2703 return this.end; 2704 } 2705 2706 public boolean hasEndElement() { 2707 return this.end != null && !this.end.isEmpty(); 2708 } 2709 2710 public boolean hasEnd() { 2711 return this.end != null && !this.end.isEmpty(); 2712 } 2713 2714 /** 2715 * @param value {@link #end} (End position of the sequence. If the coordinate 2716 * system is 0-based then end is exclusive and does not include the 2717 * last position. If the coordinate system is 1-base, then end is 2718 * inclusive and includes the last position.). This is the 2719 * underlying object with id, value and extensions. The accessor 2720 * "getEnd" gives direct access to the value 2721 */ 2722 public MolecularSequenceQualityComponent setEndElement(IntegerType value) { 2723 this.end = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return End position of the sequence. If the coordinate system is 0-based 2729 * then end is exclusive and does not include the last position. If the 2730 * coordinate system is 1-base, then end is inclusive and includes the 2731 * last position. 2732 */ 2733 public int getEnd() { 2734 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 2735 } 2736 2737 /** 2738 * @param value End position of the sequence. If the coordinate system is 2739 * 0-based then end is exclusive and does not include the last 2740 * position. If the coordinate system is 1-base, then end is 2741 * inclusive and includes the last position. 2742 */ 2743 public MolecularSequenceQualityComponent setEnd(int value) { 2744 if (this.end == null) 2745 this.end = new IntegerType(); 2746 this.end.setValue(value); 2747 return this; 2748 } 2749 2750 /** 2751 * @return {@link #score} (The score of an experimentally derived feature such 2752 * as a p-value 2753 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 2754 */ 2755 public Quantity getScore() { 2756 if (this.score == null) 2757 if (Configuration.errorOnAutoCreate()) 2758 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.score"); 2759 else if (Configuration.doAutoCreate()) 2760 this.score = new Quantity(); // cc 2761 return this.score; 2762 } 2763 2764 public boolean hasScore() { 2765 return this.score != null && !this.score.isEmpty(); 2766 } 2767 2768 /** 2769 * @param value {@link #score} (The score of an experimentally derived feature 2770 * such as a p-value 2771 * ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).) 2772 */ 2773 public MolecularSequenceQualityComponent setScore(Quantity value) { 2774 this.score = value; 2775 return this; 2776 } 2777 2778 /** 2779 * @return {@link #method} (Which method is used to get sequence quality.) 2780 */ 2781 public CodeableConcept getMethod() { 2782 if (this.method == null) 2783 if (Configuration.errorOnAutoCreate()) 2784 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.method"); 2785 else if (Configuration.doAutoCreate()) 2786 this.method = new CodeableConcept(); // cc 2787 return this.method; 2788 } 2789 2790 public boolean hasMethod() { 2791 return this.method != null && !this.method.isEmpty(); 2792 } 2793 2794 /** 2795 * @param value {@link #method} (Which method is used to get sequence quality.) 2796 */ 2797 public MolecularSequenceQualityComponent setMethod(CodeableConcept value) { 2798 this.method = value; 2799 return this; 2800 } 2801 2802 /** 2803 * @return {@link #truthTP} (True positives, from the perspective of the truth 2804 * data, i.e. the number of sites in the Truth Call Set for which there 2805 * are paths through the Query Call Set that are consistent with all of 2806 * the alleles at this site, and for which there is an accurate genotype 2807 * call for the event.). This is the underlying object with id, value 2808 * and extensions. The accessor "getTruthTP" gives direct access to the 2809 * value 2810 */ 2811 public DecimalType getTruthTPElement() { 2812 if (this.truthTP == null) 2813 if (Configuration.errorOnAutoCreate()) 2814 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.truthTP"); 2815 else if (Configuration.doAutoCreate()) 2816 this.truthTP = new DecimalType(); // bb 2817 return this.truthTP; 2818 } 2819 2820 public boolean hasTruthTPElement() { 2821 return this.truthTP != null && !this.truthTP.isEmpty(); 2822 } 2823 2824 public boolean hasTruthTP() { 2825 return this.truthTP != null && !this.truthTP.isEmpty(); 2826 } 2827 2828 /** 2829 * @param value {@link #truthTP} (True positives, from the perspective of the 2830 * truth data, i.e. the number of sites in the Truth Call Set for 2831 * which there are paths through the Query Call Set that are 2832 * consistent with all of the alleles at this site, and for which 2833 * there is an accurate genotype call for the event.). This is the 2834 * underlying object with id, value and extensions. The accessor 2835 * "getTruthTP" gives direct access to the value 2836 */ 2837 public MolecularSequenceQualityComponent setTruthTPElement(DecimalType value) { 2838 this.truthTP = value; 2839 return this; 2840 } 2841 2842 /** 2843 * @return True positives, from the perspective of the truth data, i.e. the 2844 * number of sites in the Truth Call Set for which there are paths 2845 * through the Query Call Set that are consistent with all of the 2846 * alleles at this site, and for which there is an accurate genotype 2847 * call for the event. 2848 */ 2849 public BigDecimal getTruthTP() { 2850 return this.truthTP == null ? null : this.truthTP.getValue(); 2851 } 2852 2853 /** 2854 * @param value True positives, from the perspective of the truth data, i.e. the 2855 * number of sites in the Truth Call Set for which there are paths 2856 * through the Query Call Set that are consistent with all of the 2857 * alleles at this site, and for which there is an accurate 2858 * genotype call for the event. 2859 */ 2860 public MolecularSequenceQualityComponent setTruthTP(BigDecimal value) { 2861 if (value == null) 2862 this.truthTP = null; 2863 else { 2864 if (this.truthTP == null) 2865 this.truthTP = new DecimalType(); 2866 this.truthTP.setValue(value); 2867 } 2868 return this; 2869 } 2870 2871 /** 2872 * @param value True positives, from the perspective of the truth data, i.e. the 2873 * number of sites in the Truth Call Set for which there are paths 2874 * through the Query Call Set that are consistent with all of the 2875 * alleles at this site, and for which there is an accurate 2876 * genotype call for the event. 2877 */ 2878 public MolecularSequenceQualityComponent setTruthTP(long value) { 2879 this.truthTP = new DecimalType(); 2880 this.truthTP.setValue(value); 2881 return this; 2882 } 2883 2884 /** 2885 * @param value True positives, from the perspective of the truth data, i.e. the 2886 * number of sites in the Truth Call Set for which there are paths 2887 * through the Query Call Set that are consistent with all of the 2888 * alleles at this site, and for which there is an accurate 2889 * genotype call for the event. 2890 */ 2891 public MolecularSequenceQualityComponent setTruthTP(double value) { 2892 this.truthTP = new DecimalType(); 2893 this.truthTP.setValue(value); 2894 return this; 2895 } 2896 2897 /** 2898 * @return {@link #queryTP} (True positives, from the perspective of the query 2899 * data, i.e. the number of sites in the Query Call Set for which there 2900 * are paths through the Truth Call Set that are consistent with all of 2901 * the alleles at this site, and for which there is an accurate genotype 2902 * call for the event.). This is the underlying object with id, value 2903 * and extensions. The accessor "getQueryTP" gives direct access to the 2904 * value 2905 */ 2906 public DecimalType getQueryTPElement() { 2907 if (this.queryTP == null) 2908 if (Configuration.errorOnAutoCreate()) 2909 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.queryTP"); 2910 else if (Configuration.doAutoCreate()) 2911 this.queryTP = new DecimalType(); // bb 2912 return this.queryTP; 2913 } 2914 2915 public boolean hasQueryTPElement() { 2916 return this.queryTP != null && !this.queryTP.isEmpty(); 2917 } 2918 2919 public boolean hasQueryTP() { 2920 return this.queryTP != null && !this.queryTP.isEmpty(); 2921 } 2922 2923 /** 2924 * @param value {@link #queryTP} (True positives, from the perspective of the 2925 * query data, i.e. the number of sites in the Query Call Set for 2926 * which there are paths through the Truth Call Set that are 2927 * consistent with all of the alleles at this site, and for which 2928 * there is an accurate genotype call for the event.). This is the 2929 * underlying object with id, value and extensions. The accessor 2930 * "getQueryTP" gives direct access to the value 2931 */ 2932 public MolecularSequenceQualityComponent setQueryTPElement(DecimalType value) { 2933 this.queryTP = value; 2934 return this; 2935 } 2936 2937 /** 2938 * @return True positives, from the perspective of the query data, i.e. the 2939 * number of sites in the Query Call Set for which there are paths 2940 * through the Truth Call Set that are consistent with all of the 2941 * alleles at this site, and for which there is an accurate genotype 2942 * call for the event. 2943 */ 2944 public BigDecimal getQueryTP() { 2945 return this.queryTP == null ? null : this.queryTP.getValue(); 2946 } 2947 2948 /** 2949 * @param value True positives, from the perspective of the query data, i.e. the 2950 * number of sites in the Query Call Set for which there are paths 2951 * through the Truth Call Set that are consistent with all of the 2952 * alleles at this site, and for which there is an accurate 2953 * genotype call for the event. 2954 */ 2955 public MolecularSequenceQualityComponent setQueryTP(BigDecimal value) { 2956 if (value == null) 2957 this.queryTP = null; 2958 else { 2959 if (this.queryTP == null) 2960 this.queryTP = new DecimalType(); 2961 this.queryTP.setValue(value); 2962 } 2963 return this; 2964 } 2965 2966 /** 2967 * @param value True positives, from the perspective of the query data, i.e. the 2968 * number of sites in the Query Call Set for which there are paths 2969 * through the Truth Call Set that are consistent with all of the 2970 * alleles at this site, and for which there is an accurate 2971 * genotype call for the event. 2972 */ 2973 public MolecularSequenceQualityComponent setQueryTP(long value) { 2974 this.queryTP = new DecimalType(); 2975 this.queryTP.setValue(value); 2976 return this; 2977 } 2978 2979 /** 2980 * @param value True positives, from the perspective of the query data, i.e. the 2981 * number of sites in the Query Call Set for which there are paths 2982 * through the Truth Call Set that are consistent with all of the 2983 * alleles at this site, and for which there is an accurate 2984 * genotype call for the event. 2985 */ 2986 public MolecularSequenceQualityComponent setQueryTP(double value) { 2987 this.queryTP = new DecimalType(); 2988 this.queryTP.setValue(value); 2989 return this; 2990 } 2991 2992 /** 2993 * @return {@link #truthFN} (False negatives, i.e. the number of sites in the 2994 * Truth Call Set for which there is no path through the Query Call Set 2995 * that is consistent with all of the alleles at this site, or sites for 2996 * which there is an inaccurate genotype call for the event. Sites with 2997 * correct variant but incorrect genotype are counted here.). This is 2998 * the underlying object with id, value and extensions. The accessor 2999 * "getTruthFN" gives direct access to the value 3000 */ 3001 public DecimalType getTruthFNElement() { 3002 if (this.truthFN == null) 3003 if (Configuration.errorOnAutoCreate()) 3004 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.truthFN"); 3005 else if (Configuration.doAutoCreate()) 3006 this.truthFN = new DecimalType(); // bb 3007 return this.truthFN; 3008 } 3009 3010 public boolean hasTruthFNElement() { 3011 return this.truthFN != null && !this.truthFN.isEmpty(); 3012 } 3013 3014 public boolean hasTruthFN() { 3015 return this.truthFN != null && !this.truthFN.isEmpty(); 3016 } 3017 3018 /** 3019 * @param value {@link #truthFN} (False negatives, i.e. the number of sites in 3020 * the Truth Call Set for which there is no path through the Query 3021 * Call Set that is consistent with all of the alleles at this 3022 * site, or sites for which there is an inaccurate genotype call 3023 * for the event. Sites with correct variant but incorrect genotype 3024 * are counted here.). This is the underlying object with id, value 3025 * and extensions. The accessor "getTruthFN" gives direct access to 3026 * the value 3027 */ 3028 public MolecularSequenceQualityComponent setTruthFNElement(DecimalType value) { 3029 this.truthFN = value; 3030 return this; 3031 } 3032 3033 /** 3034 * @return False negatives, i.e. the number of sites in the Truth Call Set for 3035 * which there is no path through the Query Call Set that is consistent 3036 * with all of the alleles at this site, or sites for which there is an 3037 * inaccurate genotype call for the event. Sites with correct variant 3038 * but incorrect genotype are counted here. 3039 */ 3040 public BigDecimal getTruthFN() { 3041 return this.truthFN == null ? null : this.truthFN.getValue(); 3042 } 3043 3044 /** 3045 * @param value False negatives, i.e. the number of sites in the Truth Call Set 3046 * for which there is no path through the Query Call Set that is 3047 * consistent with all of the alleles at this site, or sites for 3048 * which there is an inaccurate genotype call for the event. Sites 3049 * with correct variant but incorrect genotype are counted here. 3050 */ 3051 public MolecularSequenceQualityComponent setTruthFN(BigDecimal value) { 3052 if (value == null) 3053 this.truthFN = null; 3054 else { 3055 if (this.truthFN == null) 3056 this.truthFN = new DecimalType(); 3057 this.truthFN.setValue(value); 3058 } 3059 return this; 3060 } 3061 3062 /** 3063 * @param value False negatives, i.e. the number of sites in the Truth Call Set 3064 * for which there is no path through the Query Call Set that is 3065 * consistent with all of the alleles at this site, or sites for 3066 * which there is an inaccurate genotype call for the event. Sites 3067 * with correct variant but incorrect genotype are counted here. 3068 */ 3069 public MolecularSequenceQualityComponent setTruthFN(long value) { 3070 this.truthFN = new DecimalType(); 3071 this.truthFN.setValue(value); 3072 return this; 3073 } 3074 3075 /** 3076 * @param value False negatives, i.e. the number of sites in the Truth Call Set 3077 * for which there is no path through the Query Call Set that is 3078 * consistent with all of the alleles at this site, or sites for 3079 * which there is an inaccurate genotype call for the event. Sites 3080 * with correct variant but incorrect genotype are counted here. 3081 */ 3082 public MolecularSequenceQualityComponent setTruthFN(double value) { 3083 this.truthFN = new DecimalType(); 3084 this.truthFN.setValue(value); 3085 return this; 3086 } 3087 3088 /** 3089 * @return {@link #queryFP} (False positives, i.e. the number of sites in the 3090 * Query Call Set for which there is no path through the Truth Call Set 3091 * that is consistent with this site. Sites with correct variant but 3092 * incorrect genotype are counted here.). This is the underlying object 3093 * with id, value and extensions. The accessor "getQueryFP" gives direct 3094 * access to the value 3095 */ 3096 public DecimalType getQueryFPElement() { 3097 if (this.queryFP == null) 3098 if (Configuration.errorOnAutoCreate()) 3099 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.queryFP"); 3100 else if (Configuration.doAutoCreate()) 3101 this.queryFP = new DecimalType(); // bb 3102 return this.queryFP; 3103 } 3104 3105 public boolean hasQueryFPElement() { 3106 return this.queryFP != null && !this.queryFP.isEmpty(); 3107 } 3108 3109 public boolean hasQueryFP() { 3110 return this.queryFP != null && !this.queryFP.isEmpty(); 3111 } 3112 3113 /** 3114 * @param value {@link #queryFP} (False positives, i.e. the number of sites in 3115 * the Query Call Set for which there is no path through the Truth 3116 * Call Set that is consistent with this site. Sites with correct 3117 * variant but incorrect genotype are counted here.). This is the 3118 * underlying object with id, value and extensions. The accessor 3119 * "getQueryFP" gives direct access to the value 3120 */ 3121 public MolecularSequenceQualityComponent setQueryFPElement(DecimalType value) { 3122 this.queryFP = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return False positives, i.e. the number of sites in the Query Call Set for 3128 * which there is no path through the Truth Call Set that is consistent 3129 * with this site. Sites with correct variant but incorrect genotype are 3130 * counted here. 3131 */ 3132 public BigDecimal getQueryFP() { 3133 return this.queryFP == null ? null : this.queryFP.getValue(); 3134 } 3135 3136 /** 3137 * @param value False positives, i.e. the number of sites in the Query Call Set 3138 * for which there is no path through the Truth Call Set that is 3139 * consistent with this site. Sites with correct variant but 3140 * incorrect genotype are counted here. 3141 */ 3142 public MolecularSequenceQualityComponent setQueryFP(BigDecimal value) { 3143 if (value == null) 3144 this.queryFP = null; 3145 else { 3146 if (this.queryFP == null) 3147 this.queryFP = new DecimalType(); 3148 this.queryFP.setValue(value); 3149 } 3150 return this; 3151 } 3152 3153 /** 3154 * @param value False positives, i.e. the number of sites in the Query Call Set 3155 * for which there is no path through the Truth Call Set that is 3156 * consistent with this site. Sites with correct variant but 3157 * incorrect genotype are counted here. 3158 */ 3159 public MolecularSequenceQualityComponent setQueryFP(long value) { 3160 this.queryFP = new DecimalType(); 3161 this.queryFP.setValue(value); 3162 return this; 3163 } 3164 3165 /** 3166 * @param value False positives, i.e. the number of sites in the Query Call Set 3167 * for which there is no path through the Truth Call Set that is 3168 * consistent with this site. Sites with correct variant but 3169 * incorrect genotype are counted here. 3170 */ 3171 public MolecularSequenceQualityComponent setQueryFP(double value) { 3172 this.queryFP = new DecimalType(); 3173 this.queryFP.setValue(value); 3174 return this; 3175 } 3176 3177 /** 3178 * @return {@link #gtFP} (The number of false positives where the non-REF 3179 * alleles in the Truth and Query Call Sets match (i.e. cases where the 3180 * truth is 1/1 and the query is 0/1 or similar).). This is the 3181 * underlying object with id, value and extensions. The accessor 3182 * "getGtFP" gives direct access to the value 3183 */ 3184 public DecimalType getGtFPElement() { 3185 if (this.gtFP == null) 3186 if (Configuration.errorOnAutoCreate()) 3187 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.gtFP"); 3188 else if (Configuration.doAutoCreate()) 3189 this.gtFP = new DecimalType(); // bb 3190 return this.gtFP; 3191 } 3192 3193 public boolean hasGtFPElement() { 3194 return this.gtFP != null && !this.gtFP.isEmpty(); 3195 } 3196 3197 public boolean hasGtFP() { 3198 return this.gtFP != null && !this.gtFP.isEmpty(); 3199 } 3200 3201 /** 3202 * @param value {@link #gtFP} (The number of false positives where the non-REF 3203 * alleles in the Truth and Query Call Sets match (i.e. cases where 3204 * the truth is 1/1 and the query is 0/1 or similar).). This is the 3205 * underlying object with id, value and extensions. The accessor 3206 * "getGtFP" gives direct access to the value 3207 */ 3208 public MolecularSequenceQualityComponent setGtFPElement(DecimalType value) { 3209 this.gtFP = value; 3210 return this; 3211 } 3212 3213 /** 3214 * @return The number of false positives where the non-REF alleles in the Truth 3215 * and Query Call Sets match (i.e. cases where the truth is 1/1 and the 3216 * query is 0/1 or similar). 3217 */ 3218 public BigDecimal getGtFP() { 3219 return this.gtFP == null ? null : this.gtFP.getValue(); 3220 } 3221 3222 /** 3223 * @param value The number of false positives where the non-REF alleles in the 3224 * Truth and Query Call Sets match (i.e. cases where the truth is 3225 * 1/1 and the query is 0/1 or similar). 3226 */ 3227 public MolecularSequenceQualityComponent setGtFP(BigDecimal value) { 3228 if (value == null) 3229 this.gtFP = null; 3230 else { 3231 if (this.gtFP == null) 3232 this.gtFP = new DecimalType(); 3233 this.gtFP.setValue(value); 3234 } 3235 return this; 3236 } 3237 3238 /** 3239 * @param value The number of false positives where the non-REF alleles in the 3240 * Truth and Query Call Sets match (i.e. cases where the truth is 3241 * 1/1 and the query is 0/1 or similar). 3242 */ 3243 public MolecularSequenceQualityComponent setGtFP(long value) { 3244 this.gtFP = new DecimalType(); 3245 this.gtFP.setValue(value); 3246 return this; 3247 } 3248 3249 /** 3250 * @param value The number of false positives where the non-REF alleles in the 3251 * Truth and Query Call Sets match (i.e. cases where the truth is 3252 * 1/1 and the query is 0/1 or similar). 3253 */ 3254 public MolecularSequenceQualityComponent setGtFP(double value) { 3255 this.gtFP = new DecimalType(); 3256 this.gtFP.setValue(value); 3257 return this; 3258 } 3259 3260 /** 3261 * @return {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is the 3262 * underlying object with id, value and extensions. The accessor 3263 * "getPrecision" gives direct access to the value 3264 */ 3265 public DecimalType getPrecisionElement() { 3266 if (this.precision == null) 3267 if (Configuration.errorOnAutoCreate()) 3268 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.precision"); 3269 else if (Configuration.doAutoCreate()) 3270 this.precision = new DecimalType(); // bb 3271 return this.precision; 3272 } 3273 3274 public boolean hasPrecisionElement() { 3275 return this.precision != null && !this.precision.isEmpty(); 3276 } 3277 3278 public boolean hasPrecision() { 3279 return this.precision != null && !this.precision.isEmpty(); 3280 } 3281 3282 /** 3283 * @param value {@link #precision} (QUERY.TP / (QUERY.TP + QUERY.FP).). This is 3284 * the underlying object with id, value and extensions. The 3285 * accessor "getPrecision" gives direct access to the value 3286 */ 3287 public MolecularSequenceQualityComponent setPrecisionElement(DecimalType value) { 3288 this.precision = value; 3289 return this; 3290 } 3291 3292 /** 3293 * @return QUERY.TP / (QUERY.TP + QUERY.FP). 3294 */ 3295 public BigDecimal getPrecision() { 3296 return this.precision == null ? null : this.precision.getValue(); 3297 } 3298 3299 /** 3300 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3301 */ 3302 public MolecularSequenceQualityComponent setPrecision(BigDecimal value) { 3303 if (value == null) 3304 this.precision = null; 3305 else { 3306 if (this.precision == null) 3307 this.precision = new DecimalType(); 3308 this.precision.setValue(value); 3309 } 3310 return this; 3311 } 3312 3313 /** 3314 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3315 */ 3316 public MolecularSequenceQualityComponent setPrecision(long value) { 3317 this.precision = new DecimalType(); 3318 this.precision.setValue(value); 3319 return this; 3320 } 3321 3322 /** 3323 * @param value QUERY.TP / (QUERY.TP + QUERY.FP). 3324 */ 3325 public MolecularSequenceQualityComponent setPrecision(double value) { 3326 this.precision = new DecimalType(); 3327 this.precision.setValue(value); 3328 return this; 3329 } 3330 3331 /** 3332 * @return {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the 3333 * underlying object with id, value and extensions. The accessor 3334 * "getRecall" gives direct access to the value 3335 */ 3336 public DecimalType getRecallElement() { 3337 if (this.recall == null) 3338 if (Configuration.errorOnAutoCreate()) 3339 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.recall"); 3340 else if (Configuration.doAutoCreate()) 3341 this.recall = new DecimalType(); // bb 3342 return this.recall; 3343 } 3344 3345 public boolean hasRecallElement() { 3346 return this.recall != null && !this.recall.isEmpty(); 3347 } 3348 3349 public boolean hasRecall() { 3350 return this.recall != null && !this.recall.isEmpty(); 3351 } 3352 3353 /** 3354 * @param value {@link #recall} (TRUTH.TP / (TRUTH.TP + TRUTH.FN).). This is the 3355 * underlying object with id, value and extensions. The accessor 3356 * "getRecall" gives direct access to the value 3357 */ 3358 public MolecularSequenceQualityComponent setRecallElement(DecimalType value) { 3359 this.recall = value; 3360 return this; 3361 } 3362 3363 /** 3364 * @return TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3365 */ 3366 public BigDecimal getRecall() { 3367 return this.recall == null ? null : this.recall.getValue(); 3368 } 3369 3370 /** 3371 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3372 */ 3373 public MolecularSequenceQualityComponent setRecall(BigDecimal value) { 3374 if (value == null) 3375 this.recall = null; 3376 else { 3377 if (this.recall == null) 3378 this.recall = new DecimalType(); 3379 this.recall.setValue(value); 3380 } 3381 return this; 3382 } 3383 3384 /** 3385 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3386 */ 3387 public MolecularSequenceQualityComponent setRecall(long value) { 3388 this.recall = new DecimalType(); 3389 this.recall.setValue(value); 3390 return this; 3391 } 3392 3393 /** 3394 * @param value TRUTH.TP / (TRUTH.TP + TRUTH.FN). 3395 */ 3396 public MolecularSequenceQualityComponent setRecall(double value) { 3397 this.recall = new DecimalType(); 3398 this.recall.setValue(value); 3399 return this; 3400 } 3401 3402 /** 3403 * @return {@link #fScore} (Harmonic mean of Recall and Precision, computed as: 3404 * 2 * precision * recall / (precision + recall).). This is the 3405 * underlying object with id, value and extensions. The accessor 3406 * "getFScore" gives direct access to the value 3407 */ 3408 public DecimalType getFScoreElement() { 3409 if (this.fScore == null) 3410 if (Configuration.errorOnAutoCreate()) 3411 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.fScore"); 3412 else if (Configuration.doAutoCreate()) 3413 this.fScore = new DecimalType(); // bb 3414 return this.fScore; 3415 } 3416 3417 public boolean hasFScoreElement() { 3418 return this.fScore != null && !this.fScore.isEmpty(); 3419 } 3420 3421 public boolean hasFScore() { 3422 return this.fScore != null && !this.fScore.isEmpty(); 3423 } 3424 3425 /** 3426 * @param value {@link #fScore} (Harmonic mean of Recall and Precision, computed 3427 * as: 2 * precision * recall / (precision + recall).). This is the 3428 * underlying object with id, value and extensions. The accessor 3429 * "getFScore" gives direct access to the value 3430 */ 3431 public MolecularSequenceQualityComponent setFScoreElement(DecimalType value) { 3432 this.fScore = value; 3433 return this; 3434 } 3435 3436 /** 3437 * @return Harmonic mean of Recall and Precision, computed as: 2 * precision * 3438 * recall / (precision + recall). 3439 */ 3440 public BigDecimal getFScore() { 3441 return this.fScore == null ? null : this.fScore.getValue(); 3442 } 3443 3444 /** 3445 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3446 * precision * recall / (precision + recall). 3447 */ 3448 public MolecularSequenceQualityComponent setFScore(BigDecimal value) { 3449 if (value == null) 3450 this.fScore = null; 3451 else { 3452 if (this.fScore == null) 3453 this.fScore = new DecimalType(); 3454 this.fScore.setValue(value); 3455 } 3456 return this; 3457 } 3458 3459 /** 3460 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3461 * precision * recall / (precision + recall). 3462 */ 3463 public MolecularSequenceQualityComponent setFScore(long value) { 3464 this.fScore = new DecimalType(); 3465 this.fScore.setValue(value); 3466 return this; 3467 } 3468 3469 /** 3470 * @param value Harmonic mean of Recall and Precision, computed as: 2 * 3471 * precision * recall / (precision + recall). 3472 */ 3473 public MolecularSequenceQualityComponent setFScore(double value) { 3474 this.fScore = new DecimalType(); 3475 this.fScore.setValue(value); 3476 return this; 3477 } 3478 3479 /** 3480 * @return {@link #roc} (Receiver Operator Characteristic (ROC) Curve to give 3481 * sensitivity/specificity tradeoff.) 3482 */ 3483 public MolecularSequenceQualityRocComponent getRoc() { 3484 if (this.roc == null) 3485 if (Configuration.errorOnAutoCreate()) 3486 throw new Error("Attempt to auto-create MolecularSequenceQualityComponent.roc"); 3487 else if (Configuration.doAutoCreate()) 3488 this.roc = new MolecularSequenceQualityRocComponent(); // cc 3489 return this.roc; 3490 } 3491 3492 public boolean hasRoc() { 3493 return this.roc != null && !this.roc.isEmpty(); 3494 } 3495 3496 /** 3497 * @param value {@link #roc} (Receiver Operator Characteristic (ROC) Curve to 3498 * give sensitivity/specificity tradeoff.) 3499 */ 3500 public MolecularSequenceQualityComponent setRoc(MolecularSequenceQualityRocComponent value) { 3501 this.roc = value; 3502 return this; 3503 } 3504 3505 protected void listChildren(List<Property> children) { 3506 super.listChildren(children); 3507 children.add(new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type)); 3508 children.add(new Property("standardSequence", "CodeableConcept", 3509 "Gold standard sequence used for comparing against.", 0, 1, standardSequence)); 3510 children.add(new Property("start", "integer", 3511 "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 3512 0, 1, start)); 3513 children.add(new Property("end", "integer", 3514 "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 3515 0, 1, end)); 3516 children.add(new Property("score", "Quantity", 3517 "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 3518 0, 1, score)); 3519 children.add( 3520 new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 0, 1, method)); 3521 children.add(new Property("truthTP", "decimal", 3522 "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3523 0, 1, truthTP)); 3524 children.add(new Property("queryTP", "decimal", 3525 "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3526 0, 1, queryTP)); 3527 children.add(new Property("truthFN", "decimal", 3528 "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 3529 0, 1, truthFN)); 3530 children.add(new Property("queryFP", "decimal", 3531 "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 3532 0, 1, queryFP)); 3533 children.add(new Property("gtFP", "decimal", 3534 "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 3535 0, 1, gtFP)); 3536 children.add(new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, precision)); 3537 children.add(new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall)); 3538 children.add(new Property("fScore", "decimal", 3539 "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, 3540 fScore)); 3541 children.add(new Property("roc", "", 3542 "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.", 0, 1, roc)); 3543 } 3544 3545 @Override 3546 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3547 switch (_hash) { 3548 case 3575610: 3549 /* type */ return new Property("type", "code", "INDEL / SNP / Undefined variant.", 0, 1, type); 3550 case -1861227106: 3551 /* standardSequence */ return new Property("standardSequence", "CodeableConcept", 3552 "Gold standard sequence used for comparing against.", 0, 1, standardSequence); 3553 case 109757538: 3554 /* start */ return new Property("start", "integer", 3555 "Start position of the sequence. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 3556 0, 1, start); 3557 case 100571: 3558 /* end */ return new Property("end", "integer", 3559 "End position of the sequence. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 3560 0, 1, end); 3561 case 109264530: 3562 /* score */ return new Property("score", "Quantity", 3563 "The score of an experimentally derived feature such as a p-value ([SO:0001685](http://www.sequenceontology.org/browser/current_svn/term/SO:0001685)).", 3564 0, 1, score); 3565 case -1077554975: 3566 /* method */ return new Property("method", "CodeableConcept", "Which method is used to get sequence quality.", 3567 0, 1, method); 3568 case -1048421849: 3569 /* truthTP */ return new Property("truthTP", "decimal", 3570 "True positives, from the perspective of the truth data, i.e. the number of sites in the Truth Call Set for which there are paths through the Query Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3571 0, 1, truthTP); 3572 case 655102276: 3573 /* queryTP */ return new Property("queryTP", "decimal", 3574 "True positives, from the perspective of the query data, i.e. the number of sites in the Query Call Set for which there are paths through the Truth Call Set that are consistent with all of the alleles at this site, and for which there is an accurate genotype call for the event.", 3575 0, 1, queryTP); 3576 case -1048422285: 3577 /* truthFN */ return new Property("truthFN", "decimal", 3578 "False negatives, i.e. the number of sites in the Truth Call Set for which there is no path through the Query Call Set that is consistent with all of the alleles at this site, or sites for which there is an inaccurate genotype call for the event. Sites with correct variant but incorrect genotype are counted here.", 3579 0, 1, truthFN); 3580 case 655101842: 3581 /* queryFP */ return new Property("queryFP", "decimal", 3582 "False positives, i.e. the number of sites in the Query Call Set for which there is no path through the Truth Call Set that is consistent with this site. Sites with correct variant but incorrect genotype are counted here.", 3583 0, 1, queryFP); 3584 case 3182199: 3585 /* gtFP */ return new Property("gtFP", "decimal", 3586 "The number of false positives where the non-REF alleles in the Truth and Query Call Sets match (i.e. cases where the truth is 1/1 and the query is 0/1 or similar).", 3587 0, 1, gtFP); 3588 case -1376177026: 3589 /* precision */ return new Property("precision", "decimal", "QUERY.TP / (QUERY.TP + QUERY.FP).", 0, 1, 3590 precision); 3591 case -934922479: 3592 /* recall */ return new Property("recall", "decimal", "TRUTH.TP / (TRUTH.TP + TRUTH.FN).", 0, 1, recall); 3593 case -1295082036: 3594 /* fScore */ return new Property("fScore", "decimal", 3595 "Harmonic mean of Recall and Precision, computed as: 2 * precision * recall / (precision + recall).", 0, 1, 3596 fScore); 3597 case 113094: 3598 /* roc */ return new Property("roc", "", 3599 "Receiver Operator Characteristic (ROC) Curve to give sensitivity/specificity tradeoff.", 0, 1, roc); 3600 default: 3601 return super.getNamedProperty(_hash, _name, _checkValid); 3602 } 3603 3604 } 3605 3606 @Override 3607 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3608 switch (hash) { 3609 case 3575610: 3610 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<QualityType> 3611 case -1861227106: 3612 /* standardSequence */ return this.standardSequence == null ? new Base[0] 3613 : new Base[] { this.standardSequence }; // CodeableConcept 3614 case 109757538: 3615 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 3616 case 100571: 3617 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 3618 case 109264530: 3619 /* score */ return this.score == null ? new Base[0] : new Base[] { this.score }; // Quantity 3620 case -1077554975: 3621 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 3622 case -1048421849: 3623 /* truthTP */ return this.truthTP == null ? new Base[0] : new Base[] { this.truthTP }; // DecimalType 3624 case 655102276: 3625 /* queryTP */ return this.queryTP == null ? new Base[0] : new Base[] { this.queryTP }; // DecimalType 3626 case -1048422285: 3627 /* truthFN */ return this.truthFN == null ? new Base[0] : new Base[] { this.truthFN }; // DecimalType 3628 case 655101842: 3629 /* queryFP */ return this.queryFP == null ? new Base[0] : new Base[] { this.queryFP }; // DecimalType 3630 case 3182199: 3631 /* gtFP */ return this.gtFP == null ? new Base[0] : new Base[] { this.gtFP }; // DecimalType 3632 case -1376177026: 3633 /* precision */ return this.precision == null ? new Base[0] : new Base[] { this.precision }; // DecimalType 3634 case -934922479: 3635 /* recall */ return this.recall == null ? new Base[0] : new Base[] { this.recall }; // DecimalType 3636 case -1295082036: 3637 /* fScore */ return this.fScore == null ? new Base[0] : new Base[] { this.fScore }; // DecimalType 3638 case 113094: 3639 /* roc */ return this.roc == null ? new Base[0] : new Base[] { this.roc }; // MolecularSequenceQualityRocComponent 3640 default: 3641 return super.getProperty(hash, name, checkValid); 3642 } 3643 3644 } 3645 3646 @Override 3647 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3648 switch (hash) { 3649 case 3575610: // type 3650 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 3651 this.type = (Enumeration) value; // Enumeration<QualityType> 3652 return value; 3653 case -1861227106: // standardSequence 3654 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 3655 return value; 3656 case 109757538: // start 3657 this.start = castToInteger(value); // IntegerType 3658 return value; 3659 case 100571: // end 3660 this.end = castToInteger(value); // IntegerType 3661 return value; 3662 case 109264530: // score 3663 this.score = castToQuantity(value); // Quantity 3664 return value; 3665 case -1077554975: // method 3666 this.method = castToCodeableConcept(value); // CodeableConcept 3667 return value; 3668 case -1048421849: // truthTP 3669 this.truthTP = castToDecimal(value); // DecimalType 3670 return value; 3671 case 655102276: // queryTP 3672 this.queryTP = castToDecimal(value); // DecimalType 3673 return value; 3674 case -1048422285: // truthFN 3675 this.truthFN = castToDecimal(value); // DecimalType 3676 return value; 3677 case 655101842: // queryFP 3678 this.queryFP = castToDecimal(value); // DecimalType 3679 return value; 3680 case 3182199: // gtFP 3681 this.gtFP = castToDecimal(value); // DecimalType 3682 return value; 3683 case -1376177026: // precision 3684 this.precision = castToDecimal(value); // DecimalType 3685 return value; 3686 case -934922479: // recall 3687 this.recall = castToDecimal(value); // DecimalType 3688 return value; 3689 case -1295082036: // fScore 3690 this.fScore = castToDecimal(value); // DecimalType 3691 return value; 3692 case 113094: // roc 3693 this.roc = (MolecularSequenceQualityRocComponent) value; // MolecularSequenceQualityRocComponent 3694 return value; 3695 default: 3696 return super.setProperty(hash, name, value); 3697 } 3698 3699 } 3700 3701 @Override 3702 public Base setProperty(String name, Base value) throws FHIRException { 3703 if (name.equals("type")) { 3704 value = new QualityTypeEnumFactory().fromType(castToCode(value)); 3705 this.type = (Enumeration) value; // Enumeration<QualityType> 3706 } else if (name.equals("standardSequence")) { 3707 this.standardSequence = castToCodeableConcept(value); // CodeableConcept 3708 } else if (name.equals("start")) { 3709 this.start = castToInteger(value); // IntegerType 3710 } else if (name.equals("end")) { 3711 this.end = castToInteger(value); // IntegerType 3712 } else if (name.equals("score")) { 3713 this.score = castToQuantity(value); // Quantity 3714 } else if (name.equals("method")) { 3715 this.method = castToCodeableConcept(value); // CodeableConcept 3716 } else if (name.equals("truthTP")) { 3717 this.truthTP = castToDecimal(value); // DecimalType 3718 } else if (name.equals("queryTP")) { 3719 this.queryTP = castToDecimal(value); // DecimalType 3720 } else if (name.equals("truthFN")) { 3721 this.truthFN = castToDecimal(value); // DecimalType 3722 } else if (name.equals("queryFP")) { 3723 this.queryFP = castToDecimal(value); // DecimalType 3724 } else if (name.equals("gtFP")) { 3725 this.gtFP = castToDecimal(value); // DecimalType 3726 } else if (name.equals("precision")) { 3727 this.precision = castToDecimal(value); // DecimalType 3728 } else if (name.equals("recall")) { 3729 this.recall = castToDecimal(value); // DecimalType 3730 } else if (name.equals("fScore")) { 3731 this.fScore = castToDecimal(value); // DecimalType 3732 } else if (name.equals("roc")) { 3733 this.roc = (MolecularSequenceQualityRocComponent) value; // MolecularSequenceQualityRocComponent 3734 } else 3735 return super.setProperty(name, value); 3736 return value; 3737 } 3738 3739 @Override 3740 public void removeChild(String name, Base value) throws FHIRException { 3741 if (name.equals("type")) { 3742 this.type = null; 3743 } else if (name.equals("standardSequence")) { 3744 this.standardSequence = null; 3745 } else if (name.equals("start")) { 3746 this.start = null; 3747 } else if (name.equals("end")) { 3748 this.end = null; 3749 } else if (name.equals("score")) { 3750 this.score = null; 3751 } else if (name.equals("method")) { 3752 this.method = null; 3753 } else if (name.equals("truthTP")) { 3754 this.truthTP = null; 3755 } else if (name.equals("queryTP")) { 3756 this.queryTP = null; 3757 } else if (name.equals("truthFN")) { 3758 this.truthFN = null; 3759 } else if (name.equals("queryFP")) { 3760 this.queryFP = null; 3761 } else if (name.equals("gtFP")) { 3762 this.gtFP = null; 3763 } else if (name.equals("precision")) { 3764 this.precision = null; 3765 } else if (name.equals("recall")) { 3766 this.recall = null; 3767 } else if (name.equals("fScore")) { 3768 this.fScore = null; 3769 } else if (name.equals("roc")) { 3770 this.roc = (MolecularSequenceQualityRocComponent) value; // MolecularSequenceQualityRocComponent 3771 } else 3772 super.removeChild(name, value); 3773 3774 } 3775 3776 @Override 3777 public Base makeProperty(int hash, String name) throws FHIRException { 3778 switch (hash) { 3779 case 3575610: 3780 return getTypeElement(); 3781 case -1861227106: 3782 return getStandardSequence(); 3783 case 109757538: 3784 return getStartElement(); 3785 case 100571: 3786 return getEndElement(); 3787 case 109264530: 3788 return getScore(); 3789 case -1077554975: 3790 return getMethod(); 3791 case -1048421849: 3792 return getTruthTPElement(); 3793 case 655102276: 3794 return getQueryTPElement(); 3795 case -1048422285: 3796 return getTruthFNElement(); 3797 case 655101842: 3798 return getQueryFPElement(); 3799 case 3182199: 3800 return getGtFPElement(); 3801 case -1376177026: 3802 return getPrecisionElement(); 3803 case -934922479: 3804 return getRecallElement(); 3805 case -1295082036: 3806 return getFScoreElement(); 3807 case 113094: 3808 return getRoc(); 3809 default: 3810 return super.makeProperty(hash, name); 3811 } 3812 3813 } 3814 3815 @Override 3816 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3817 switch (hash) { 3818 case 3575610: 3819 /* type */ return new String[] { "code" }; 3820 case -1861227106: 3821 /* standardSequence */ return new String[] { "CodeableConcept" }; 3822 case 109757538: 3823 /* start */ return new String[] { "integer" }; 3824 case 100571: 3825 /* end */ return new String[] { "integer" }; 3826 case 109264530: 3827 /* score */ return new String[] { "Quantity" }; 3828 case -1077554975: 3829 /* method */ return new String[] { "CodeableConcept" }; 3830 case -1048421849: 3831 /* truthTP */ return new String[] { "decimal" }; 3832 case 655102276: 3833 /* queryTP */ return new String[] { "decimal" }; 3834 case -1048422285: 3835 /* truthFN */ return new String[] { "decimal" }; 3836 case 655101842: 3837 /* queryFP */ return new String[] { "decimal" }; 3838 case 3182199: 3839 /* gtFP */ return new String[] { "decimal" }; 3840 case -1376177026: 3841 /* precision */ return new String[] { "decimal" }; 3842 case -934922479: 3843 /* recall */ return new String[] { "decimal" }; 3844 case -1295082036: 3845 /* fScore */ return new String[] { "decimal" }; 3846 case 113094: 3847 /* roc */ return new String[] {}; 3848 default: 3849 return super.getTypesForProperty(hash, name); 3850 } 3851 3852 } 3853 3854 @Override 3855 public Base addChild(String name) throws FHIRException { 3856 if (name.equals("type")) { 3857 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 3858 } else if (name.equals("standardSequence")) { 3859 this.standardSequence = new CodeableConcept(); 3860 return this.standardSequence; 3861 } else if (name.equals("start")) { 3862 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 3863 } else if (name.equals("end")) { 3864 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 3865 } else if (name.equals("score")) { 3866 this.score = new Quantity(); 3867 return this.score; 3868 } else if (name.equals("method")) { 3869 this.method = new CodeableConcept(); 3870 return this.method; 3871 } else if (name.equals("truthTP")) { 3872 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.truthTP"); 3873 } else if (name.equals("queryTP")) { 3874 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.queryTP"); 3875 } else if (name.equals("truthFN")) { 3876 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.truthFN"); 3877 } else if (name.equals("queryFP")) { 3878 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.queryFP"); 3879 } else if (name.equals("gtFP")) { 3880 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.gtFP"); 3881 } else if (name.equals("precision")) { 3882 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.precision"); 3883 } else if (name.equals("recall")) { 3884 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.recall"); 3885 } else if (name.equals("fScore")) { 3886 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.fScore"); 3887 } else if (name.equals("roc")) { 3888 this.roc = new MolecularSequenceQualityRocComponent(); 3889 return this.roc; 3890 } else 3891 return super.addChild(name); 3892 } 3893 3894 public MolecularSequenceQualityComponent copy() { 3895 MolecularSequenceQualityComponent dst = new MolecularSequenceQualityComponent(); 3896 copyValues(dst); 3897 return dst; 3898 } 3899 3900 public void copyValues(MolecularSequenceQualityComponent dst) { 3901 super.copyValues(dst); 3902 dst.type = type == null ? null : type.copy(); 3903 dst.standardSequence = standardSequence == null ? null : standardSequence.copy(); 3904 dst.start = start == null ? null : start.copy(); 3905 dst.end = end == null ? null : end.copy(); 3906 dst.score = score == null ? null : score.copy(); 3907 dst.method = method == null ? null : method.copy(); 3908 dst.truthTP = truthTP == null ? null : truthTP.copy(); 3909 dst.queryTP = queryTP == null ? null : queryTP.copy(); 3910 dst.truthFN = truthFN == null ? null : truthFN.copy(); 3911 dst.queryFP = queryFP == null ? null : queryFP.copy(); 3912 dst.gtFP = gtFP == null ? null : gtFP.copy(); 3913 dst.precision = precision == null ? null : precision.copy(); 3914 dst.recall = recall == null ? null : recall.copy(); 3915 dst.fScore = fScore == null ? null : fScore.copy(); 3916 dst.roc = roc == null ? null : roc.copy(); 3917 } 3918 3919 @Override 3920 public boolean equalsDeep(Base other_) { 3921 if (!super.equalsDeep(other_)) 3922 return false; 3923 if (!(other_ instanceof MolecularSequenceQualityComponent)) 3924 return false; 3925 MolecularSequenceQualityComponent o = (MolecularSequenceQualityComponent) other_; 3926 return compareDeep(type, o.type, true) && compareDeep(standardSequence, o.standardSequence, true) 3927 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) && compareDeep(score, o.score, true) 3928 && compareDeep(method, o.method, true) && compareDeep(truthTP, o.truthTP, true) 3929 && compareDeep(queryTP, o.queryTP, true) && compareDeep(truthFN, o.truthFN, true) 3930 && compareDeep(queryFP, o.queryFP, true) && compareDeep(gtFP, o.gtFP, true) 3931 && compareDeep(precision, o.precision, true) && compareDeep(recall, o.recall, true) 3932 && compareDeep(fScore, o.fScore, true) && compareDeep(roc, o.roc, true); 3933 } 3934 3935 @Override 3936 public boolean equalsShallow(Base other_) { 3937 if (!super.equalsShallow(other_)) 3938 return false; 3939 if (!(other_ instanceof MolecularSequenceQualityComponent)) 3940 return false; 3941 MolecularSequenceQualityComponent o = (MolecularSequenceQualityComponent) other_; 3942 return compareValues(type, o.type, true) && compareValues(start, o.start, true) && compareValues(end, o.end, true) 3943 && compareValues(truthTP, o.truthTP, true) && compareValues(queryTP, o.queryTP, true) 3944 && compareValues(truthFN, o.truthFN, true) && compareValues(queryFP, o.queryFP, true) 3945 && compareValues(gtFP, o.gtFP, true) && compareValues(precision, o.precision, true) 3946 && compareValues(recall, o.recall, true) && compareValues(fScore, o.fScore, true); 3947 } 3948 3949 public boolean isEmpty() { 3950 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, standardSequence, start, end, score, method, 3951 truthTP, queryTP, truthFN, queryFP, gtFP, precision, recall, fScore, roc); 3952 } 3953 3954 public String fhirType() { 3955 return "MolecularSequence.quality"; 3956 3957 } 3958 3959 } 3960 3961 @Block() 3962 public static class MolecularSequenceQualityRocComponent extends BackboneElement implements IBaseBackboneElement { 3963 /** 3964 * Invidual data point representing the GQ (genotype quality) score threshold. 3965 */ 3966 @Child(name = "score", type = { 3967 IntegerType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3968 @Description(shortDefinition = "Genotype quality score", formalDefinition = "Invidual data point representing the GQ (genotype quality) score threshold.") 3969 protected List<IntegerType> score; 3970 3971 /** 3972 * The number of true positives if the GQ score threshold was set to "score" 3973 * field value. 3974 */ 3975 @Child(name = "numTP", type = { 3976 IntegerType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3977 @Description(shortDefinition = "Roc score true positive numbers", formalDefinition = "The number of true positives if the GQ score threshold was set to \"score\" field value.") 3978 protected List<IntegerType> numTP; 3979 3980 /** 3981 * The number of false positives if the GQ score threshold was set to "score" 3982 * field value. 3983 */ 3984 @Child(name = "numFP", type = { 3985 IntegerType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3986 @Description(shortDefinition = "Roc score false positive numbers", formalDefinition = "The number of false positives if the GQ score threshold was set to \"score\" field value.") 3987 protected List<IntegerType> numFP; 3988 3989 /** 3990 * The number of false negatives if the GQ score threshold was set to "score" 3991 * field value. 3992 */ 3993 @Child(name = "numFN", type = { 3994 IntegerType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3995 @Description(shortDefinition = "Roc score false negative numbers", formalDefinition = "The number of false negatives if the GQ score threshold was set to \"score\" field value.") 3996 protected List<IntegerType> numFN; 3997 3998 /** 3999 * Calculated precision if the GQ score threshold was set to "score" field 4000 * value. 4001 */ 4002 @Child(name = "precision", type = { 4003 DecimalType.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4004 @Description(shortDefinition = "Precision of the GQ score", formalDefinition = "Calculated precision if the GQ score threshold was set to \"score\" field value.") 4005 protected List<DecimalType> precision; 4006 4007 /** 4008 * Calculated sensitivity if the GQ score threshold was set to "score" field 4009 * value. 4010 */ 4011 @Child(name = "sensitivity", type = { 4012 DecimalType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4013 @Description(shortDefinition = "Sensitivity of the GQ score", formalDefinition = "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.") 4014 protected List<DecimalType> sensitivity; 4015 4016 /** 4017 * Calculated fScore if the GQ score threshold was set to "score" field value. 4018 */ 4019 @Child(name = "fMeasure", type = { 4020 DecimalType.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4021 @Description(shortDefinition = "FScore of the GQ score", formalDefinition = "Calculated fScore if the GQ score threshold was set to \"score\" field value.") 4022 protected List<DecimalType> fMeasure; 4023 4024 private static final long serialVersionUID = 1923392132L; 4025 4026 /** 4027 * Constructor 4028 */ 4029 public MolecularSequenceQualityRocComponent() { 4030 super(); 4031 } 4032 4033 /** 4034 * @return {@link #score} (Invidual data point representing the GQ (genotype 4035 * quality) score threshold.) 4036 */ 4037 public List<IntegerType> getScore() { 4038 if (this.score == null) 4039 this.score = new ArrayList<IntegerType>(); 4040 return this.score; 4041 } 4042 4043 /** 4044 * @return Returns a reference to <code>this</code> for easy method chaining 4045 */ 4046 public MolecularSequenceQualityRocComponent setScore(List<IntegerType> theScore) { 4047 this.score = theScore; 4048 return this; 4049 } 4050 4051 public boolean hasScore() { 4052 if (this.score == null) 4053 return false; 4054 for (IntegerType item : this.score) 4055 if (!item.isEmpty()) 4056 return true; 4057 return false; 4058 } 4059 4060 /** 4061 * @return {@link #score} (Invidual data point representing the GQ (genotype 4062 * quality) score threshold.) 4063 */ 4064 public IntegerType addScoreElement() {// 2 4065 IntegerType t = new IntegerType(); 4066 if (this.score == null) 4067 this.score = new ArrayList<IntegerType>(); 4068 this.score.add(t); 4069 return t; 4070 } 4071 4072 /** 4073 * @param value {@link #score} (Invidual data point representing the GQ 4074 * (genotype quality) score threshold.) 4075 */ 4076 public MolecularSequenceQualityRocComponent addScore(int value) { // 1 4077 IntegerType t = new IntegerType(); 4078 t.setValue(value); 4079 if (this.score == null) 4080 this.score = new ArrayList<IntegerType>(); 4081 this.score.add(t); 4082 return this; 4083 } 4084 4085 /** 4086 * @param value {@link #score} (Invidual data point representing the GQ 4087 * (genotype quality) score threshold.) 4088 */ 4089 public boolean hasScore(int value) { 4090 if (this.score == null) 4091 return false; 4092 for (IntegerType v : this.score) 4093 if (v.getValue().equals(value)) // integer 4094 return true; 4095 return false; 4096 } 4097 4098 /** 4099 * @return {@link #numTP} (The number of true positives if the GQ score 4100 * threshold was set to "score" field value.) 4101 */ 4102 public List<IntegerType> getNumTP() { 4103 if (this.numTP == null) 4104 this.numTP = new ArrayList<IntegerType>(); 4105 return this.numTP; 4106 } 4107 4108 /** 4109 * @return Returns a reference to <code>this</code> for easy method chaining 4110 */ 4111 public MolecularSequenceQualityRocComponent setNumTP(List<IntegerType> theNumTP) { 4112 this.numTP = theNumTP; 4113 return this; 4114 } 4115 4116 public boolean hasNumTP() { 4117 if (this.numTP == null) 4118 return false; 4119 for (IntegerType item : this.numTP) 4120 if (!item.isEmpty()) 4121 return true; 4122 return false; 4123 } 4124 4125 /** 4126 * @return {@link #numTP} (The number of true positives if the GQ score 4127 * threshold was set to "score" field value.) 4128 */ 4129 public IntegerType addNumTPElement() {// 2 4130 IntegerType t = new IntegerType(); 4131 if (this.numTP == null) 4132 this.numTP = new ArrayList<IntegerType>(); 4133 this.numTP.add(t); 4134 return t; 4135 } 4136 4137 /** 4138 * @param value {@link #numTP} (The number of true positives if the GQ score 4139 * threshold was set to "score" field value.) 4140 */ 4141 public MolecularSequenceQualityRocComponent addNumTP(int value) { // 1 4142 IntegerType t = new IntegerType(); 4143 t.setValue(value); 4144 if (this.numTP == null) 4145 this.numTP = new ArrayList<IntegerType>(); 4146 this.numTP.add(t); 4147 return this; 4148 } 4149 4150 /** 4151 * @param value {@link #numTP} (The number of true positives if the GQ score 4152 * threshold was set to "score" field value.) 4153 */ 4154 public boolean hasNumTP(int value) { 4155 if (this.numTP == null) 4156 return false; 4157 for (IntegerType v : this.numTP) 4158 if (v.getValue().equals(value)) // integer 4159 return true; 4160 return false; 4161 } 4162 4163 /** 4164 * @return {@link #numFP} (The number of false positives if the GQ score 4165 * threshold was set to "score" field value.) 4166 */ 4167 public List<IntegerType> getNumFP() { 4168 if (this.numFP == null) 4169 this.numFP = new ArrayList<IntegerType>(); 4170 return this.numFP; 4171 } 4172 4173 /** 4174 * @return Returns a reference to <code>this</code> for easy method chaining 4175 */ 4176 public MolecularSequenceQualityRocComponent setNumFP(List<IntegerType> theNumFP) { 4177 this.numFP = theNumFP; 4178 return this; 4179 } 4180 4181 public boolean hasNumFP() { 4182 if (this.numFP == null) 4183 return false; 4184 for (IntegerType item : this.numFP) 4185 if (!item.isEmpty()) 4186 return true; 4187 return false; 4188 } 4189 4190 /** 4191 * @return {@link #numFP} (The number of false positives if the GQ score 4192 * threshold was set to "score" field value.) 4193 */ 4194 public IntegerType addNumFPElement() {// 2 4195 IntegerType t = new IntegerType(); 4196 if (this.numFP == null) 4197 this.numFP = new ArrayList<IntegerType>(); 4198 this.numFP.add(t); 4199 return t; 4200 } 4201 4202 /** 4203 * @param value {@link #numFP} (The number of false positives if the GQ score 4204 * threshold was set to "score" field value.) 4205 */ 4206 public MolecularSequenceQualityRocComponent addNumFP(int value) { // 1 4207 IntegerType t = new IntegerType(); 4208 t.setValue(value); 4209 if (this.numFP == null) 4210 this.numFP = new ArrayList<IntegerType>(); 4211 this.numFP.add(t); 4212 return this; 4213 } 4214 4215 /** 4216 * @param value {@link #numFP} (The number of false positives if the GQ score 4217 * threshold was set to "score" field value.) 4218 */ 4219 public boolean hasNumFP(int value) { 4220 if (this.numFP == null) 4221 return false; 4222 for (IntegerType v : this.numFP) 4223 if (v.getValue().equals(value)) // integer 4224 return true; 4225 return false; 4226 } 4227 4228 /** 4229 * @return {@link #numFN} (The number of false negatives if the GQ score 4230 * threshold was set to "score" field value.) 4231 */ 4232 public List<IntegerType> getNumFN() { 4233 if (this.numFN == null) 4234 this.numFN = new ArrayList<IntegerType>(); 4235 return this.numFN; 4236 } 4237 4238 /** 4239 * @return Returns a reference to <code>this</code> for easy method chaining 4240 */ 4241 public MolecularSequenceQualityRocComponent setNumFN(List<IntegerType> theNumFN) { 4242 this.numFN = theNumFN; 4243 return this; 4244 } 4245 4246 public boolean hasNumFN() { 4247 if (this.numFN == null) 4248 return false; 4249 for (IntegerType item : this.numFN) 4250 if (!item.isEmpty()) 4251 return true; 4252 return false; 4253 } 4254 4255 /** 4256 * @return {@link #numFN} (The number of false negatives if the GQ score 4257 * threshold was set to "score" field value.) 4258 */ 4259 public IntegerType addNumFNElement() {// 2 4260 IntegerType t = new IntegerType(); 4261 if (this.numFN == null) 4262 this.numFN = new ArrayList<IntegerType>(); 4263 this.numFN.add(t); 4264 return t; 4265 } 4266 4267 /** 4268 * @param value {@link #numFN} (The number of false negatives if the GQ score 4269 * threshold was set to "score" field value.) 4270 */ 4271 public MolecularSequenceQualityRocComponent addNumFN(int value) { // 1 4272 IntegerType t = new IntegerType(); 4273 t.setValue(value); 4274 if (this.numFN == null) 4275 this.numFN = new ArrayList<IntegerType>(); 4276 this.numFN.add(t); 4277 return this; 4278 } 4279 4280 /** 4281 * @param value {@link #numFN} (The number of false negatives if the GQ score 4282 * threshold was set to "score" field value.) 4283 */ 4284 public boolean hasNumFN(int value) { 4285 if (this.numFN == null) 4286 return false; 4287 for (IntegerType v : this.numFN) 4288 if (v.getValue().equals(value)) // integer 4289 return true; 4290 return false; 4291 } 4292 4293 /** 4294 * @return {@link #precision} (Calculated precision if the GQ score threshold 4295 * was set to "score" field value.) 4296 */ 4297 public List<DecimalType> getPrecision() { 4298 if (this.precision == null) 4299 this.precision = new ArrayList<DecimalType>(); 4300 return this.precision; 4301 } 4302 4303 /** 4304 * @return Returns a reference to <code>this</code> for easy method chaining 4305 */ 4306 public MolecularSequenceQualityRocComponent setPrecision(List<DecimalType> thePrecision) { 4307 this.precision = thePrecision; 4308 return this; 4309 } 4310 4311 public boolean hasPrecision() { 4312 if (this.precision == null) 4313 return false; 4314 for (DecimalType item : this.precision) 4315 if (!item.isEmpty()) 4316 return true; 4317 return false; 4318 } 4319 4320 /** 4321 * @return {@link #precision} (Calculated precision if the GQ score threshold 4322 * was set to "score" field value.) 4323 */ 4324 public DecimalType addPrecisionElement() {// 2 4325 DecimalType t = new DecimalType(); 4326 if (this.precision == null) 4327 this.precision = new ArrayList<DecimalType>(); 4328 this.precision.add(t); 4329 return t; 4330 } 4331 4332 /** 4333 * @param value {@link #precision} (Calculated precision if the GQ score 4334 * threshold was set to "score" field value.) 4335 */ 4336 public MolecularSequenceQualityRocComponent addPrecision(BigDecimal value) { // 1 4337 DecimalType t = new DecimalType(); 4338 t.setValue(value); 4339 if (this.precision == null) 4340 this.precision = new ArrayList<DecimalType>(); 4341 this.precision.add(t); 4342 return this; 4343 } 4344 4345 /** 4346 * @param value {@link #precision} (Calculated precision if the GQ score 4347 * threshold was set to "score" field value.) 4348 */ 4349 public boolean hasPrecision(BigDecimal value) { 4350 if (this.precision == null) 4351 return false; 4352 for (DecimalType v : this.precision) 4353 if (v.getValue().equals(value)) // decimal 4354 return true; 4355 return false; 4356 } 4357 4358 /** 4359 * @return {@link #sensitivity} (Calculated sensitivity if the GQ score 4360 * threshold was set to "score" field value.) 4361 */ 4362 public List<DecimalType> getSensitivity() { 4363 if (this.sensitivity == null) 4364 this.sensitivity = new ArrayList<DecimalType>(); 4365 return this.sensitivity; 4366 } 4367 4368 /** 4369 * @return Returns a reference to <code>this</code> for easy method chaining 4370 */ 4371 public MolecularSequenceQualityRocComponent setSensitivity(List<DecimalType> theSensitivity) { 4372 this.sensitivity = theSensitivity; 4373 return this; 4374 } 4375 4376 public boolean hasSensitivity() { 4377 if (this.sensitivity == null) 4378 return false; 4379 for (DecimalType item : this.sensitivity) 4380 if (!item.isEmpty()) 4381 return true; 4382 return false; 4383 } 4384 4385 /** 4386 * @return {@link #sensitivity} (Calculated sensitivity if the GQ score 4387 * threshold was set to "score" field value.) 4388 */ 4389 public DecimalType addSensitivityElement() {// 2 4390 DecimalType t = new DecimalType(); 4391 if (this.sensitivity == null) 4392 this.sensitivity = new ArrayList<DecimalType>(); 4393 this.sensitivity.add(t); 4394 return t; 4395 } 4396 4397 /** 4398 * @param value {@link #sensitivity} (Calculated sensitivity if the GQ score 4399 * threshold was set to "score" field value.) 4400 */ 4401 public MolecularSequenceQualityRocComponent addSensitivity(BigDecimal value) { // 1 4402 DecimalType t = new DecimalType(); 4403 t.setValue(value); 4404 if (this.sensitivity == null) 4405 this.sensitivity = new ArrayList<DecimalType>(); 4406 this.sensitivity.add(t); 4407 return this; 4408 } 4409 4410 /** 4411 * @param value {@link #sensitivity} (Calculated sensitivity if the GQ score 4412 * threshold was set to "score" field value.) 4413 */ 4414 public boolean hasSensitivity(BigDecimal value) { 4415 if (this.sensitivity == null) 4416 return false; 4417 for (DecimalType v : this.sensitivity) 4418 if (v.getValue().equals(value)) // decimal 4419 return true; 4420 return false; 4421 } 4422 4423 /** 4424 * @return {@link #fMeasure} (Calculated fScore if the GQ score threshold was 4425 * set to "score" field value.) 4426 */ 4427 public List<DecimalType> getFMeasure() { 4428 if (this.fMeasure == null) 4429 this.fMeasure = new ArrayList<DecimalType>(); 4430 return this.fMeasure; 4431 } 4432 4433 /** 4434 * @return Returns a reference to <code>this</code> for easy method chaining 4435 */ 4436 public MolecularSequenceQualityRocComponent setFMeasure(List<DecimalType> theFMeasure) { 4437 this.fMeasure = theFMeasure; 4438 return this; 4439 } 4440 4441 public boolean hasFMeasure() { 4442 if (this.fMeasure == null) 4443 return false; 4444 for (DecimalType item : this.fMeasure) 4445 if (!item.isEmpty()) 4446 return true; 4447 return false; 4448 } 4449 4450 /** 4451 * @return {@link #fMeasure} (Calculated fScore if the GQ score threshold was 4452 * set to "score" field value.) 4453 */ 4454 public DecimalType addFMeasureElement() {// 2 4455 DecimalType t = new DecimalType(); 4456 if (this.fMeasure == null) 4457 this.fMeasure = new ArrayList<DecimalType>(); 4458 this.fMeasure.add(t); 4459 return t; 4460 } 4461 4462 /** 4463 * @param value {@link #fMeasure} (Calculated fScore if the GQ score threshold 4464 * was set to "score" field value.) 4465 */ 4466 public MolecularSequenceQualityRocComponent addFMeasure(BigDecimal value) { // 1 4467 DecimalType t = new DecimalType(); 4468 t.setValue(value); 4469 if (this.fMeasure == null) 4470 this.fMeasure = new ArrayList<DecimalType>(); 4471 this.fMeasure.add(t); 4472 return this; 4473 } 4474 4475 /** 4476 * @param value {@link #fMeasure} (Calculated fScore if the GQ score threshold 4477 * was set to "score" field value.) 4478 */ 4479 public boolean hasFMeasure(BigDecimal value) { 4480 if (this.fMeasure == null) 4481 return false; 4482 for (DecimalType v : this.fMeasure) 4483 if (v.getValue().equals(value)) // decimal 4484 return true; 4485 return false; 4486 } 4487 4488 protected void listChildren(List<Property> children) { 4489 super.listChildren(children); 4490 children.add(new Property("score", "integer", 4491 "Invidual data point representing the GQ (genotype quality) score threshold.", 0, java.lang.Integer.MAX_VALUE, 4492 score)); 4493 children.add(new Property("numTP", "integer", 4494 "The number of true positives if the GQ score threshold was set to \"score\" field value.", 0, 4495 java.lang.Integer.MAX_VALUE, numTP)); 4496 children.add(new Property("numFP", "integer", 4497 "The number of false positives if the GQ score threshold was set to \"score\" field value.", 0, 4498 java.lang.Integer.MAX_VALUE, numFP)); 4499 children.add(new Property("numFN", "integer", 4500 "The number of false negatives if the GQ score threshold was set to \"score\" field value.", 0, 4501 java.lang.Integer.MAX_VALUE, numFN)); 4502 children.add(new Property("precision", "decimal", 4503 "Calculated precision if the GQ score threshold was set to \"score\" field value.", 0, 4504 java.lang.Integer.MAX_VALUE, precision)); 4505 children.add(new Property("sensitivity", "decimal", 4506 "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.", 0, 4507 java.lang.Integer.MAX_VALUE, sensitivity)); 4508 children.add(new Property("fMeasure", "decimal", 4509 "Calculated fScore if the GQ score threshold was set to \"score\" field value.", 0, 4510 java.lang.Integer.MAX_VALUE, fMeasure)); 4511 } 4512 4513 @Override 4514 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4515 switch (_hash) { 4516 case 109264530: 4517 /* score */ return new Property("score", "integer", 4518 "Invidual data point representing the GQ (genotype quality) score threshold.", 0, 4519 java.lang.Integer.MAX_VALUE, score); 4520 case 105180290: 4521 /* numTP */ return new Property("numTP", "integer", 4522 "The number of true positives if the GQ score threshold was set to \"score\" field value.", 0, 4523 java.lang.Integer.MAX_VALUE, numTP); 4524 case 105179856: 4525 /* numFP */ return new Property("numFP", "integer", 4526 "The number of false positives if the GQ score threshold was set to \"score\" field value.", 0, 4527 java.lang.Integer.MAX_VALUE, numFP); 4528 case 105179854: 4529 /* numFN */ return new Property("numFN", "integer", 4530 "The number of false negatives if the GQ score threshold was set to \"score\" field value.", 0, 4531 java.lang.Integer.MAX_VALUE, numFN); 4532 case -1376177026: 4533 /* precision */ return new Property("precision", "decimal", 4534 "Calculated precision if the GQ score threshold was set to \"score\" field value.", 0, 4535 java.lang.Integer.MAX_VALUE, precision); 4536 case 564403871: 4537 /* sensitivity */ return new Property("sensitivity", "decimal", 4538 "Calculated sensitivity if the GQ score threshold was set to \"score\" field value.", 0, 4539 java.lang.Integer.MAX_VALUE, sensitivity); 4540 case -18997736: 4541 /* fMeasure */ return new Property("fMeasure", "decimal", 4542 "Calculated fScore if the GQ score threshold was set to \"score\" field value.", 0, 4543 java.lang.Integer.MAX_VALUE, fMeasure); 4544 default: 4545 return super.getNamedProperty(_hash, _name, _checkValid); 4546 } 4547 4548 } 4549 4550 @Override 4551 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4552 switch (hash) { 4553 case 109264530: 4554 /* score */ return this.score == null ? new Base[0] : this.score.toArray(new Base[this.score.size()]); // IntegerType 4555 case 105180290: 4556 /* numTP */ return this.numTP == null ? new Base[0] : this.numTP.toArray(new Base[this.numTP.size()]); // IntegerType 4557 case 105179856: 4558 /* numFP */ return this.numFP == null ? new Base[0] : this.numFP.toArray(new Base[this.numFP.size()]); // IntegerType 4559 case 105179854: 4560 /* numFN */ return this.numFN == null ? new Base[0] : this.numFN.toArray(new Base[this.numFN.size()]); // IntegerType 4561 case -1376177026: 4562 /* precision */ return this.precision == null ? new Base[0] 4563 : this.precision.toArray(new Base[this.precision.size()]); // DecimalType 4564 case 564403871: 4565 /* sensitivity */ return this.sensitivity == null ? new Base[0] 4566 : this.sensitivity.toArray(new Base[this.sensitivity.size()]); // DecimalType 4567 case -18997736: 4568 /* fMeasure */ return this.fMeasure == null ? new Base[0] 4569 : this.fMeasure.toArray(new Base[this.fMeasure.size()]); // DecimalType 4570 default: 4571 return super.getProperty(hash, name, checkValid); 4572 } 4573 4574 } 4575 4576 @Override 4577 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4578 switch (hash) { 4579 case 109264530: // score 4580 this.getScore().add(castToInteger(value)); // IntegerType 4581 return value; 4582 case 105180290: // numTP 4583 this.getNumTP().add(castToInteger(value)); // IntegerType 4584 return value; 4585 case 105179856: // numFP 4586 this.getNumFP().add(castToInteger(value)); // IntegerType 4587 return value; 4588 case 105179854: // numFN 4589 this.getNumFN().add(castToInteger(value)); // IntegerType 4590 return value; 4591 case -1376177026: // precision 4592 this.getPrecision().add(castToDecimal(value)); // DecimalType 4593 return value; 4594 case 564403871: // sensitivity 4595 this.getSensitivity().add(castToDecimal(value)); // DecimalType 4596 return value; 4597 case -18997736: // fMeasure 4598 this.getFMeasure().add(castToDecimal(value)); // DecimalType 4599 return value; 4600 default: 4601 return super.setProperty(hash, name, value); 4602 } 4603 4604 } 4605 4606 @Override 4607 public Base setProperty(String name, Base value) throws FHIRException { 4608 if (name.equals("score")) { 4609 this.getScore().add(castToInteger(value)); 4610 } else if (name.equals("numTP")) { 4611 this.getNumTP().add(castToInteger(value)); 4612 } else if (name.equals("numFP")) { 4613 this.getNumFP().add(castToInteger(value)); 4614 } else if (name.equals("numFN")) { 4615 this.getNumFN().add(castToInteger(value)); 4616 } else if (name.equals("precision")) { 4617 this.getPrecision().add(castToDecimal(value)); 4618 } else if (name.equals("sensitivity")) { 4619 this.getSensitivity().add(castToDecimal(value)); 4620 } else if (name.equals("fMeasure")) { 4621 this.getFMeasure().add(castToDecimal(value)); 4622 } else 4623 return super.setProperty(name, value); 4624 return value; 4625 } 4626 4627 @Override 4628 public void removeChild(String name, Base value) throws FHIRException { 4629 if (name.equals("score")) { 4630 this.getScore().remove(castToInteger(value)); 4631 } else if (name.equals("numTP")) { 4632 this.getNumTP().remove(castToInteger(value)); 4633 } else if (name.equals("numFP")) { 4634 this.getNumFP().remove(castToInteger(value)); 4635 } else if (name.equals("numFN")) { 4636 this.getNumFN().remove(castToInteger(value)); 4637 } else if (name.equals("precision")) { 4638 this.getPrecision().remove(castToDecimal(value)); 4639 } else if (name.equals("sensitivity")) { 4640 this.getSensitivity().remove(castToDecimal(value)); 4641 } else if (name.equals("fMeasure")) { 4642 this.getFMeasure().remove(castToDecimal(value)); 4643 } else 4644 super.removeChild(name, value); 4645 4646 } 4647 4648 @Override 4649 public Base makeProperty(int hash, String name) throws FHIRException { 4650 switch (hash) { 4651 case 109264530: 4652 return addScoreElement(); 4653 case 105180290: 4654 return addNumTPElement(); 4655 case 105179856: 4656 return addNumFPElement(); 4657 case 105179854: 4658 return addNumFNElement(); 4659 case -1376177026: 4660 return addPrecisionElement(); 4661 case 564403871: 4662 return addSensitivityElement(); 4663 case -18997736: 4664 return addFMeasureElement(); 4665 default: 4666 return super.makeProperty(hash, name); 4667 } 4668 4669 } 4670 4671 @Override 4672 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4673 switch (hash) { 4674 case 109264530: 4675 /* score */ return new String[] { "integer" }; 4676 case 105180290: 4677 /* numTP */ return new String[] { "integer" }; 4678 case 105179856: 4679 /* numFP */ return new String[] { "integer" }; 4680 case 105179854: 4681 /* numFN */ return new String[] { "integer" }; 4682 case -1376177026: 4683 /* precision */ return new String[] { "decimal" }; 4684 case 564403871: 4685 /* sensitivity */ return new String[] { "decimal" }; 4686 case -18997736: 4687 /* fMeasure */ return new String[] { "decimal" }; 4688 default: 4689 return super.getTypesForProperty(hash, name); 4690 } 4691 4692 } 4693 4694 @Override 4695 public Base addChild(String name) throws FHIRException { 4696 if (name.equals("score")) { 4697 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.score"); 4698 } else if (name.equals("numTP")) { 4699 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numTP"); 4700 } else if (name.equals("numFP")) { 4701 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numFP"); 4702 } else if (name.equals("numFN")) { 4703 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.numFN"); 4704 } else if (name.equals("precision")) { 4705 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.precision"); 4706 } else if (name.equals("sensitivity")) { 4707 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.sensitivity"); 4708 } else if (name.equals("fMeasure")) { 4709 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.fMeasure"); 4710 } else 4711 return super.addChild(name); 4712 } 4713 4714 public MolecularSequenceQualityRocComponent copy() { 4715 MolecularSequenceQualityRocComponent dst = new MolecularSequenceQualityRocComponent(); 4716 copyValues(dst); 4717 return dst; 4718 } 4719 4720 public void copyValues(MolecularSequenceQualityRocComponent dst) { 4721 super.copyValues(dst); 4722 if (score != null) { 4723 dst.score = new ArrayList<IntegerType>(); 4724 for (IntegerType i : score) 4725 dst.score.add(i.copy()); 4726 } 4727 ; 4728 if (numTP != null) { 4729 dst.numTP = new ArrayList<IntegerType>(); 4730 for (IntegerType i : numTP) 4731 dst.numTP.add(i.copy()); 4732 } 4733 ; 4734 if (numFP != null) { 4735 dst.numFP = new ArrayList<IntegerType>(); 4736 for (IntegerType i : numFP) 4737 dst.numFP.add(i.copy()); 4738 } 4739 ; 4740 if (numFN != null) { 4741 dst.numFN = new ArrayList<IntegerType>(); 4742 for (IntegerType i : numFN) 4743 dst.numFN.add(i.copy()); 4744 } 4745 ; 4746 if (precision != null) { 4747 dst.precision = new ArrayList<DecimalType>(); 4748 for (DecimalType i : precision) 4749 dst.precision.add(i.copy()); 4750 } 4751 ; 4752 if (sensitivity != null) { 4753 dst.sensitivity = new ArrayList<DecimalType>(); 4754 for (DecimalType i : sensitivity) 4755 dst.sensitivity.add(i.copy()); 4756 } 4757 ; 4758 if (fMeasure != null) { 4759 dst.fMeasure = new ArrayList<DecimalType>(); 4760 for (DecimalType i : fMeasure) 4761 dst.fMeasure.add(i.copy()); 4762 } 4763 ; 4764 } 4765 4766 @Override 4767 public boolean equalsDeep(Base other_) { 4768 if (!super.equalsDeep(other_)) 4769 return false; 4770 if (!(other_ instanceof MolecularSequenceQualityRocComponent)) 4771 return false; 4772 MolecularSequenceQualityRocComponent o = (MolecularSequenceQualityRocComponent) other_; 4773 return compareDeep(score, o.score, true) && compareDeep(numTP, o.numTP, true) && compareDeep(numFP, o.numFP, true) 4774 && compareDeep(numFN, o.numFN, true) && compareDeep(precision, o.precision, true) 4775 && compareDeep(sensitivity, o.sensitivity, true) && compareDeep(fMeasure, o.fMeasure, true); 4776 } 4777 4778 @Override 4779 public boolean equalsShallow(Base other_) { 4780 if (!super.equalsShallow(other_)) 4781 return false; 4782 if (!(other_ instanceof MolecularSequenceQualityRocComponent)) 4783 return false; 4784 MolecularSequenceQualityRocComponent o = (MolecularSequenceQualityRocComponent) other_; 4785 return compareValues(score, o.score, true) && compareValues(numTP, o.numTP, true) 4786 && compareValues(numFP, o.numFP, true) && compareValues(numFN, o.numFN, true) 4787 && compareValues(precision, o.precision, true) && compareValues(sensitivity, o.sensitivity, true) 4788 && compareValues(fMeasure, o.fMeasure, true); 4789 } 4790 4791 public boolean isEmpty() { 4792 return super.isEmpty() 4793 && ca.uhn.fhir.util.ElementUtil.isEmpty(score, numTP, numFP, numFN, precision, sensitivity, fMeasure); 4794 } 4795 4796 public String fhirType() { 4797 return "MolecularSequence.quality.roc"; 4798 4799 } 4800 4801 } 4802 4803 @Block() 4804 public static class MolecularSequenceRepositoryComponent extends BackboneElement implements IBaseBackboneElement { 4805 /** 4806 * Click and see / RESTful API / Need login to see / RESTful API with 4807 * authentication / Other ways to see resource. 4808 */ 4809 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4810 @Description(shortDefinition = "directlink | openapi | login | oauth | other", formalDefinition = "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.") 4811 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/repository-type") 4812 protected Enumeration<RepositoryType> type; 4813 4814 /** 4815 * URI of an external repository which contains further details about the 4816 * genetics data. 4817 */ 4818 @Child(name = "url", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4819 @Description(shortDefinition = "URI of the repository", formalDefinition = "URI of an external repository which contains further details about the genetics data.") 4820 protected UriType url; 4821 4822 /** 4823 * URI of an external repository which contains further details about the 4824 * genetics data. 4825 */ 4826 @Child(name = "name", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4827 @Description(shortDefinition = "Repository's name", formalDefinition = "URI of an external repository which contains further details about the genetics data.") 4828 protected StringType name; 4829 4830 /** 4831 * Id of the variant in this external repository. The server will understand how 4832 * to use this id to call for more info about datasets in external repository. 4833 */ 4834 @Child(name = "datasetId", type = { 4835 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4836 @Description(shortDefinition = "Id of the dataset that used to call for dataset in repository", formalDefinition = "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.") 4837 protected StringType datasetId; 4838 4839 /** 4840 * Id of the variantset in this external repository. The server will understand 4841 * how to use this id to call for more info about variantsets in external 4842 * repository. 4843 */ 4844 @Child(name = "variantsetId", type = { 4845 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4846 @Description(shortDefinition = "Id of the variantset that used to call for variantset in repository", formalDefinition = "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.") 4847 protected StringType variantsetId; 4848 4849 /** 4850 * Id of the read in this external repository. 4851 */ 4852 @Child(name = "readsetId", type = { 4853 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4854 @Description(shortDefinition = "Id of the read", formalDefinition = "Id of the read in this external repository.") 4855 protected StringType readsetId; 4856 4857 private static final long serialVersionUID = -899243265L; 4858 4859 /** 4860 * Constructor 4861 */ 4862 public MolecularSequenceRepositoryComponent() { 4863 super(); 4864 } 4865 4866 /** 4867 * Constructor 4868 */ 4869 public MolecularSequenceRepositoryComponent(Enumeration<RepositoryType> type) { 4870 super(); 4871 this.type = type; 4872 } 4873 4874 /** 4875 * @return {@link #type} (Click and see / RESTful API / Need login to see / 4876 * RESTful API with authentication / Other ways to see resource.). This 4877 * is the underlying object with id, value and extensions. The accessor 4878 * "getType" gives direct access to the value 4879 */ 4880 public Enumeration<RepositoryType> getTypeElement() { 4881 if (this.type == null) 4882 if (Configuration.errorOnAutoCreate()) 4883 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.type"); 4884 else if (Configuration.doAutoCreate()) 4885 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); // bb 4886 return this.type; 4887 } 4888 4889 public boolean hasTypeElement() { 4890 return this.type != null && !this.type.isEmpty(); 4891 } 4892 4893 public boolean hasType() { 4894 return this.type != null && !this.type.isEmpty(); 4895 } 4896 4897 /** 4898 * @param value {@link #type} (Click and see / RESTful API / Need login to see / 4899 * RESTful API with authentication / Other ways to see resource.). 4900 * This is the underlying object with id, value and extensions. The 4901 * accessor "getType" gives direct access to the value 4902 */ 4903 public MolecularSequenceRepositoryComponent setTypeElement(Enumeration<RepositoryType> value) { 4904 this.type = value; 4905 return this; 4906 } 4907 4908 /** 4909 * @return Click and see / RESTful API / Need login to see / RESTful API with 4910 * authentication / Other ways to see resource. 4911 */ 4912 public RepositoryType getType() { 4913 return this.type == null ? null : this.type.getValue(); 4914 } 4915 4916 /** 4917 * @param value Click and see / RESTful API / Need login to see / RESTful API 4918 * with authentication / Other ways to see resource. 4919 */ 4920 public MolecularSequenceRepositoryComponent setType(RepositoryType value) { 4921 if (this.type == null) 4922 this.type = new Enumeration<RepositoryType>(new RepositoryTypeEnumFactory()); 4923 this.type.setValue(value); 4924 return this; 4925 } 4926 4927 /** 4928 * @return {@link #url} (URI of an external repository which contains further 4929 * details about the genetics data.). This is the underlying object with 4930 * id, value and extensions. The accessor "getUrl" gives direct access 4931 * to the value 4932 */ 4933 public UriType getUrlElement() { 4934 if (this.url == null) 4935 if (Configuration.errorOnAutoCreate()) 4936 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.url"); 4937 else if (Configuration.doAutoCreate()) 4938 this.url = new UriType(); // bb 4939 return this.url; 4940 } 4941 4942 public boolean hasUrlElement() { 4943 return this.url != null && !this.url.isEmpty(); 4944 } 4945 4946 public boolean hasUrl() { 4947 return this.url != null && !this.url.isEmpty(); 4948 } 4949 4950 /** 4951 * @param value {@link #url} (URI of an external repository which contains 4952 * further details about the genetics data.). This is the 4953 * underlying object with id, value and extensions. The accessor 4954 * "getUrl" gives direct access to the value 4955 */ 4956 public MolecularSequenceRepositoryComponent setUrlElement(UriType value) { 4957 this.url = value; 4958 return this; 4959 } 4960 4961 /** 4962 * @return URI of an external repository which contains further details about 4963 * the genetics data. 4964 */ 4965 public String getUrl() { 4966 return this.url == null ? null : this.url.getValue(); 4967 } 4968 4969 /** 4970 * @param value URI of an external repository which contains further details 4971 * about the genetics data. 4972 */ 4973 public MolecularSequenceRepositoryComponent setUrl(String value) { 4974 if (Utilities.noString(value)) 4975 this.url = null; 4976 else { 4977 if (this.url == null) 4978 this.url = new UriType(); 4979 this.url.setValue(value); 4980 } 4981 return this; 4982 } 4983 4984 /** 4985 * @return {@link #name} (URI of an external repository which contains further 4986 * details about the genetics data.). This is the underlying object with 4987 * id, value and extensions. The accessor "getName" gives direct access 4988 * to the value 4989 */ 4990 public StringType getNameElement() { 4991 if (this.name == null) 4992 if (Configuration.errorOnAutoCreate()) 4993 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.name"); 4994 else if (Configuration.doAutoCreate()) 4995 this.name = new StringType(); // bb 4996 return this.name; 4997 } 4998 4999 public boolean hasNameElement() { 5000 return this.name != null && !this.name.isEmpty(); 5001 } 5002 5003 public boolean hasName() { 5004 return this.name != null && !this.name.isEmpty(); 5005 } 5006 5007 /** 5008 * @param value {@link #name} (URI of an external repository which contains 5009 * further details about the genetics data.). This is the 5010 * underlying object with id, value and extensions. The accessor 5011 * "getName" gives direct access to the value 5012 */ 5013 public MolecularSequenceRepositoryComponent setNameElement(StringType value) { 5014 this.name = value; 5015 return this; 5016 } 5017 5018 /** 5019 * @return URI of an external repository which contains further details about 5020 * the genetics data. 5021 */ 5022 public String getName() { 5023 return this.name == null ? null : this.name.getValue(); 5024 } 5025 5026 /** 5027 * @param value URI of an external repository which contains further details 5028 * about the genetics data. 5029 */ 5030 public MolecularSequenceRepositoryComponent setName(String value) { 5031 if (Utilities.noString(value)) 5032 this.name = null; 5033 else { 5034 if (this.name == null) 5035 this.name = new StringType(); 5036 this.name.setValue(value); 5037 } 5038 return this; 5039 } 5040 5041 /** 5042 * @return {@link #datasetId} (Id of the variant in this external repository. 5043 * The server will understand how to use this id to call for more info 5044 * about datasets in external repository.). This is the underlying 5045 * object with id, value and extensions. The accessor "getDatasetId" 5046 * gives direct access to the value 5047 */ 5048 public StringType getDatasetIdElement() { 5049 if (this.datasetId == null) 5050 if (Configuration.errorOnAutoCreate()) 5051 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.datasetId"); 5052 else if (Configuration.doAutoCreate()) 5053 this.datasetId = new StringType(); // bb 5054 return this.datasetId; 5055 } 5056 5057 public boolean hasDatasetIdElement() { 5058 return this.datasetId != null && !this.datasetId.isEmpty(); 5059 } 5060 5061 public boolean hasDatasetId() { 5062 return this.datasetId != null && !this.datasetId.isEmpty(); 5063 } 5064 5065 /** 5066 * @param value {@link #datasetId} (Id of the variant in this external 5067 * repository. The server will understand how to use this id to 5068 * call for more info about datasets in external repository.). This 5069 * is the underlying object with id, value and extensions. The 5070 * accessor "getDatasetId" gives direct access to the value 5071 */ 5072 public MolecularSequenceRepositoryComponent setDatasetIdElement(StringType value) { 5073 this.datasetId = value; 5074 return this; 5075 } 5076 5077 /** 5078 * @return Id of the variant in this external repository. The server will 5079 * understand how to use this id to call for more info about datasets in 5080 * external repository. 5081 */ 5082 public String getDatasetId() { 5083 return this.datasetId == null ? null : this.datasetId.getValue(); 5084 } 5085 5086 /** 5087 * @param value Id of the variant in this external repository. The server will 5088 * understand how to use this id to call for more info about 5089 * datasets in external repository. 5090 */ 5091 public MolecularSequenceRepositoryComponent setDatasetId(String value) { 5092 if (Utilities.noString(value)) 5093 this.datasetId = null; 5094 else { 5095 if (this.datasetId == null) 5096 this.datasetId = new StringType(); 5097 this.datasetId.setValue(value); 5098 } 5099 return this; 5100 } 5101 5102 /** 5103 * @return {@link #variantsetId} (Id of the variantset in this external 5104 * repository. The server will understand how to use this id to call for 5105 * more info about variantsets in external repository.). This is the 5106 * underlying object with id, value and extensions. The accessor 5107 * "getVariantsetId" gives direct access to the value 5108 */ 5109 public StringType getVariantsetIdElement() { 5110 if (this.variantsetId == null) 5111 if (Configuration.errorOnAutoCreate()) 5112 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.variantsetId"); 5113 else if (Configuration.doAutoCreate()) 5114 this.variantsetId = new StringType(); // bb 5115 return this.variantsetId; 5116 } 5117 5118 public boolean hasVariantsetIdElement() { 5119 return this.variantsetId != null && !this.variantsetId.isEmpty(); 5120 } 5121 5122 public boolean hasVariantsetId() { 5123 return this.variantsetId != null && !this.variantsetId.isEmpty(); 5124 } 5125 5126 /** 5127 * @param value {@link #variantsetId} (Id of the variantset in this external 5128 * repository. The server will understand how to use this id to 5129 * call for more info about variantsets in external repository.). 5130 * This is the underlying object with id, value and extensions. The 5131 * accessor "getVariantsetId" gives direct access to the value 5132 */ 5133 public MolecularSequenceRepositoryComponent setVariantsetIdElement(StringType value) { 5134 this.variantsetId = value; 5135 return this; 5136 } 5137 5138 /** 5139 * @return Id of the variantset in this external repository. The server will 5140 * understand how to use this id to call for more info about variantsets 5141 * in external repository. 5142 */ 5143 public String getVariantsetId() { 5144 return this.variantsetId == null ? null : this.variantsetId.getValue(); 5145 } 5146 5147 /** 5148 * @param value Id of the variantset in this external repository. The server 5149 * will understand how to use this id to call for more info about 5150 * variantsets in external repository. 5151 */ 5152 public MolecularSequenceRepositoryComponent setVariantsetId(String value) { 5153 if (Utilities.noString(value)) 5154 this.variantsetId = null; 5155 else { 5156 if (this.variantsetId == null) 5157 this.variantsetId = new StringType(); 5158 this.variantsetId.setValue(value); 5159 } 5160 return this; 5161 } 5162 5163 /** 5164 * @return {@link #readsetId} (Id of the read in this external repository.). 5165 * This is the underlying object with id, value and extensions. The 5166 * accessor "getReadsetId" gives direct access to the value 5167 */ 5168 public StringType getReadsetIdElement() { 5169 if (this.readsetId == null) 5170 if (Configuration.errorOnAutoCreate()) 5171 throw new Error("Attempt to auto-create MolecularSequenceRepositoryComponent.readsetId"); 5172 else if (Configuration.doAutoCreate()) 5173 this.readsetId = new StringType(); // bb 5174 return this.readsetId; 5175 } 5176 5177 public boolean hasReadsetIdElement() { 5178 return this.readsetId != null && !this.readsetId.isEmpty(); 5179 } 5180 5181 public boolean hasReadsetId() { 5182 return this.readsetId != null && !this.readsetId.isEmpty(); 5183 } 5184 5185 /** 5186 * @param value {@link #readsetId} (Id of the read in this external 5187 * repository.). This is the underlying object with id, value and 5188 * extensions. The accessor "getReadsetId" gives direct access to 5189 * the value 5190 */ 5191 public MolecularSequenceRepositoryComponent setReadsetIdElement(StringType value) { 5192 this.readsetId = value; 5193 return this; 5194 } 5195 5196 /** 5197 * @return Id of the read in this external repository. 5198 */ 5199 public String getReadsetId() { 5200 return this.readsetId == null ? null : this.readsetId.getValue(); 5201 } 5202 5203 /** 5204 * @param value Id of the read in this external repository. 5205 */ 5206 public MolecularSequenceRepositoryComponent setReadsetId(String value) { 5207 if (Utilities.noString(value)) 5208 this.readsetId = null; 5209 else { 5210 if (this.readsetId == null) 5211 this.readsetId = new StringType(); 5212 this.readsetId.setValue(value); 5213 } 5214 return this; 5215 } 5216 5217 protected void listChildren(List<Property> children) { 5218 super.listChildren(children); 5219 children.add(new Property("type", "code", 5220 "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 5221 0, 1, type)); 5222 children.add(new Property("url", "uri", 5223 "URI of an external repository which contains further details about the genetics data.", 0, 1, url)); 5224 children.add(new Property("name", "string", 5225 "URI of an external repository which contains further details about the genetics data.", 0, 1, name)); 5226 children.add(new Property("datasetId", "string", 5227 "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 5228 0, 1, datasetId)); 5229 children.add(new Property("variantsetId", "string", 5230 "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 5231 0, 1, variantsetId)); 5232 children.add(new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, readsetId)); 5233 } 5234 5235 @Override 5236 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5237 switch (_hash) { 5238 case 3575610: 5239 /* type */ return new Property("type", "code", 5240 "Click and see / RESTful API / Need login to see / RESTful API with authentication / Other ways to see resource.", 5241 0, 1, type); 5242 case 116079: 5243 /* url */ return new Property("url", "uri", 5244 "URI of an external repository which contains further details about the genetics data.", 0, 1, url); 5245 case 3373707: 5246 /* name */ return new Property("name", "string", 5247 "URI of an external repository which contains further details about the genetics data.", 0, 1, name); 5248 case -345342029: 5249 /* datasetId */ return new Property("datasetId", "string", 5250 "Id of the variant in this external repository. The server will understand how to use this id to call for more info about datasets in external repository.", 5251 0, 1, datasetId); 5252 case 1929752504: 5253 /* variantsetId */ return new Property("variantsetId", "string", 5254 "Id of the variantset in this external repository. The server will understand how to use this id to call for more info about variantsets in external repository.", 5255 0, 1, variantsetId); 5256 case -1095407289: 5257 /* readsetId */ return new Property("readsetId", "string", "Id of the read in this external repository.", 0, 1, 5258 readsetId); 5259 default: 5260 return super.getNamedProperty(_hash, _name, _checkValid); 5261 } 5262 5263 } 5264 5265 @Override 5266 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5267 switch (hash) { 5268 case 3575610: 5269 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<RepositoryType> 5270 case 116079: 5271 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 5272 case 3373707: 5273 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 5274 case -345342029: 5275 /* datasetId */ return this.datasetId == null ? new Base[0] : new Base[] { this.datasetId }; // StringType 5276 case 1929752504: 5277 /* variantsetId */ return this.variantsetId == null ? new Base[0] : new Base[] { this.variantsetId }; // StringType 5278 case -1095407289: 5279 /* readsetId */ return this.readsetId == null ? new Base[0] : new Base[] { this.readsetId }; // StringType 5280 default: 5281 return super.getProperty(hash, name, checkValid); 5282 } 5283 5284 } 5285 5286 @Override 5287 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5288 switch (hash) { 5289 case 3575610: // type 5290 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 5291 this.type = (Enumeration) value; // Enumeration<RepositoryType> 5292 return value; 5293 case 116079: // url 5294 this.url = castToUri(value); // UriType 5295 return value; 5296 case 3373707: // name 5297 this.name = castToString(value); // StringType 5298 return value; 5299 case -345342029: // datasetId 5300 this.datasetId = castToString(value); // StringType 5301 return value; 5302 case 1929752504: // variantsetId 5303 this.variantsetId = castToString(value); // StringType 5304 return value; 5305 case -1095407289: // readsetId 5306 this.readsetId = castToString(value); // StringType 5307 return value; 5308 default: 5309 return super.setProperty(hash, name, value); 5310 } 5311 5312 } 5313 5314 @Override 5315 public Base setProperty(String name, Base value) throws FHIRException { 5316 if (name.equals("type")) { 5317 value = new RepositoryTypeEnumFactory().fromType(castToCode(value)); 5318 this.type = (Enumeration) value; // Enumeration<RepositoryType> 5319 } else if (name.equals("url")) { 5320 this.url = castToUri(value); // UriType 5321 } else if (name.equals("name")) { 5322 this.name = castToString(value); // StringType 5323 } else if (name.equals("datasetId")) { 5324 this.datasetId = castToString(value); // StringType 5325 } else if (name.equals("variantsetId")) { 5326 this.variantsetId = castToString(value); // StringType 5327 } else if (name.equals("readsetId")) { 5328 this.readsetId = castToString(value); // StringType 5329 } else 5330 return super.setProperty(name, value); 5331 return value; 5332 } 5333 5334 @Override 5335 public void removeChild(String name, Base value) throws FHIRException { 5336 if (name.equals("type")) { 5337 this.type = null; 5338 } else if (name.equals("url")) { 5339 this.url = null; 5340 } else if (name.equals("name")) { 5341 this.name = null; 5342 } else if (name.equals("datasetId")) { 5343 this.datasetId = null; 5344 } else if (name.equals("variantsetId")) { 5345 this.variantsetId = null; 5346 } else if (name.equals("readsetId")) { 5347 this.readsetId = null; 5348 } else 5349 super.removeChild(name, value); 5350 5351 } 5352 5353 @Override 5354 public Base makeProperty(int hash, String name) throws FHIRException { 5355 switch (hash) { 5356 case 3575610: 5357 return getTypeElement(); 5358 case 116079: 5359 return getUrlElement(); 5360 case 3373707: 5361 return getNameElement(); 5362 case -345342029: 5363 return getDatasetIdElement(); 5364 case 1929752504: 5365 return getVariantsetIdElement(); 5366 case -1095407289: 5367 return getReadsetIdElement(); 5368 default: 5369 return super.makeProperty(hash, name); 5370 } 5371 5372 } 5373 5374 @Override 5375 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5376 switch (hash) { 5377 case 3575610: 5378 /* type */ return new String[] { "code" }; 5379 case 116079: 5380 /* url */ return new String[] { "uri" }; 5381 case 3373707: 5382 /* name */ return new String[] { "string" }; 5383 case -345342029: 5384 /* datasetId */ return new String[] { "string" }; 5385 case 1929752504: 5386 /* variantsetId */ return new String[] { "string" }; 5387 case -1095407289: 5388 /* readsetId */ return new String[] { "string" }; 5389 default: 5390 return super.getTypesForProperty(hash, name); 5391 } 5392 5393 } 5394 5395 @Override 5396 public Base addChild(String name) throws FHIRException { 5397 if (name.equals("type")) { 5398 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 5399 } else if (name.equals("url")) { 5400 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.url"); 5401 } else if (name.equals("name")) { 5402 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.name"); 5403 } else if (name.equals("datasetId")) { 5404 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.datasetId"); 5405 } else if (name.equals("variantsetId")) { 5406 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.variantsetId"); 5407 } else if (name.equals("readsetId")) { 5408 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.readsetId"); 5409 } else 5410 return super.addChild(name); 5411 } 5412 5413 public MolecularSequenceRepositoryComponent copy() { 5414 MolecularSequenceRepositoryComponent dst = new MolecularSequenceRepositoryComponent(); 5415 copyValues(dst); 5416 return dst; 5417 } 5418 5419 public void copyValues(MolecularSequenceRepositoryComponent dst) { 5420 super.copyValues(dst); 5421 dst.type = type == null ? null : type.copy(); 5422 dst.url = url == null ? null : url.copy(); 5423 dst.name = name == null ? null : name.copy(); 5424 dst.datasetId = datasetId == null ? null : datasetId.copy(); 5425 dst.variantsetId = variantsetId == null ? null : variantsetId.copy(); 5426 dst.readsetId = readsetId == null ? null : readsetId.copy(); 5427 } 5428 5429 @Override 5430 public boolean equalsDeep(Base other_) { 5431 if (!super.equalsDeep(other_)) 5432 return false; 5433 if (!(other_ instanceof MolecularSequenceRepositoryComponent)) 5434 return false; 5435 MolecularSequenceRepositoryComponent o = (MolecularSequenceRepositoryComponent) other_; 5436 return compareDeep(type, o.type, true) && compareDeep(url, o.url, true) && compareDeep(name, o.name, true) 5437 && compareDeep(datasetId, o.datasetId, true) && compareDeep(variantsetId, o.variantsetId, true) 5438 && compareDeep(readsetId, o.readsetId, true); 5439 } 5440 5441 @Override 5442 public boolean equalsShallow(Base other_) { 5443 if (!super.equalsShallow(other_)) 5444 return false; 5445 if (!(other_ instanceof MolecularSequenceRepositoryComponent)) 5446 return false; 5447 MolecularSequenceRepositoryComponent o = (MolecularSequenceRepositoryComponent) other_; 5448 return compareValues(type, o.type, true) && compareValues(url, o.url, true) && compareValues(name, o.name, true) 5449 && compareValues(datasetId, o.datasetId, true) && compareValues(variantsetId, o.variantsetId, true) 5450 && compareValues(readsetId, o.readsetId, true); 5451 } 5452 5453 public boolean isEmpty() { 5454 return super.isEmpty() 5455 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, url, name, datasetId, variantsetId, readsetId); 5456 } 5457 5458 public String fhirType() { 5459 return "MolecularSequence.repository"; 5460 5461 } 5462 5463 } 5464 5465 @Block() 5466 public static class MolecularSequenceStructureVariantComponent extends BackboneElement 5467 implements IBaseBackboneElement { 5468 /** 5469 * Information about chromosome structure variation DNA change type. 5470 */ 5471 @Child(name = "variantType", type = { 5472 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 5473 @Description(shortDefinition = "Structural variant change type", formalDefinition = "Information about chromosome structure variation DNA change type.") 5474 protected CodeableConcept variantType; 5475 5476 /** 5477 * Used to indicate if the outer and inner start-end values have the same 5478 * meaning. 5479 */ 5480 @Child(name = "exact", type = { BooleanType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5481 @Description(shortDefinition = "Does the structural variant have base pair resolution breakpoints?", formalDefinition = "Used to indicate if the outer and inner start-end values have the same meaning.") 5482 protected BooleanType exact; 5483 5484 /** 5485 * Length of the variant chromosome. 5486 */ 5487 @Child(name = "length", type = { IntegerType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 5488 @Description(shortDefinition = "Structural variant length", formalDefinition = "Length of the variant chromosome.") 5489 protected IntegerType length; 5490 5491 /** 5492 * Structural variant outer. 5493 */ 5494 @Child(name = "outer", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = true) 5495 @Description(shortDefinition = "Structural variant outer", formalDefinition = "Structural variant outer.") 5496 protected MolecularSequenceStructureVariantOuterComponent outer; 5497 5498 /** 5499 * Structural variant inner. 5500 */ 5501 @Child(name = "inner", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 5502 @Description(shortDefinition = "Structural variant inner", formalDefinition = "Structural variant inner.") 5503 protected MolecularSequenceStructureVariantInnerComponent inner; 5504 5505 private static final long serialVersionUID = -1943515207L; 5506 5507 /** 5508 * Constructor 5509 */ 5510 public MolecularSequenceStructureVariantComponent() { 5511 super(); 5512 } 5513 5514 /** 5515 * @return {@link #variantType} (Information about chromosome structure 5516 * variation DNA change type.) 5517 */ 5518 public CodeableConcept getVariantType() { 5519 if (this.variantType == null) 5520 if (Configuration.errorOnAutoCreate()) 5521 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.variantType"); 5522 else if (Configuration.doAutoCreate()) 5523 this.variantType = new CodeableConcept(); // cc 5524 return this.variantType; 5525 } 5526 5527 public boolean hasVariantType() { 5528 return this.variantType != null && !this.variantType.isEmpty(); 5529 } 5530 5531 /** 5532 * @param value {@link #variantType} (Information about chromosome structure 5533 * variation DNA change type.) 5534 */ 5535 public MolecularSequenceStructureVariantComponent setVariantType(CodeableConcept value) { 5536 this.variantType = value; 5537 return this; 5538 } 5539 5540 /** 5541 * @return {@link #exact} (Used to indicate if the outer and inner start-end 5542 * values have the same meaning.). This is the underlying object with 5543 * id, value and extensions. The accessor "getExact" gives direct access 5544 * to the value 5545 */ 5546 public BooleanType getExactElement() { 5547 if (this.exact == null) 5548 if (Configuration.errorOnAutoCreate()) 5549 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.exact"); 5550 else if (Configuration.doAutoCreate()) 5551 this.exact = new BooleanType(); // bb 5552 return this.exact; 5553 } 5554 5555 public boolean hasExactElement() { 5556 return this.exact != null && !this.exact.isEmpty(); 5557 } 5558 5559 public boolean hasExact() { 5560 return this.exact != null && !this.exact.isEmpty(); 5561 } 5562 5563 /** 5564 * @param value {@link #exact} (Used to indicate if the outer and inner 5565 * start-end values have the same meaning.). This is the underlying 5566 * object with id, value and extensions. The accessor "getExact" 5567 * gives direct access to the value 5568 */ 5569 public MolecularSequenceStructureVariantComponent setExactElement(BooleanType value) { 5570 this.exact = value; 5571 return this; 5572 } 5573 5574 /** 5575 * @return Used to indicate if the outer and inner start-end values have the 5576 * same meaning. 5577 */ 5578 public boolean getExact() { 5579 return this.exact == null || this.exact.isEmpty() ? false : this.exact.getValue(); 5580 } 5581 5582 /** 5583 * @param value Used to indicate if the outer and inner start-end values have 5584 * the same meaning. 5585 */ 5586 public MolecularSequenceStructureVariantComponent setExact(boolean value) { 5587 if (this.exact == null) 5588 this.exact = new BooleanType(); 5589 this.exact.setValue(value); 5590 return this; 5591 } 5592 5593 /** 5594 * @return {@link #length} (Length of the variant chromosome.). This is the 5595 * underlying object with id, value and extensions. The accessor 5596 * "getLength" gives direct access to the value 5597 */ 5598 public IntegerType getLengthElement() { 5599 if (this.length == null) 5600 if (Configuration.errorOnAutoCreate()) 5601 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.length"); 5602 else if (Configuration.doAutoCreate()) 5603 this.length = new IntegerType(); // bb 5604 return this.length; 5605 } 5606 5607 public boolean hasLengthElement() { 5608 return this.length != null && !this.length.isEmpty(); 5609 } 5610 5611 public boolean hasLength() { 5612 return this.length != null && !this.length.isEmpty(); 5613 } 5614 5615 /** 5616 * @param value {@link #length} (Length of the variant chromosome.). This is the 5617 * underlying object with id, value and extensions. The accessor 5618 * "getLength" gives direct access to the value 5619 */ 5620 public MolecularSequenceStructureVariantComponent setLengthElement(IntegerType value) { 5621 this.length = value; 5622 return this; 5623 } 5624 5625 /** 5626 * @return Length of the variant chromosome. 5627 */ 5628 public int getLength() { 5629 return this.length == null || this.length.isEmpty() ? 0 : this.length.getValue(); 5630 } 5631 5632 /** 5633 * @param value Length of the variant chromosome. 5634 */ 5635 public MolecularSequenceStructureVariantComponent setLength(int value) { 5636 if (this.length == null) 5637 this.length = new IntegerType(); 5638 this.length.setValue(value); 5639 return this; 5640 } 5641 5642 /** 5643 * @return {@link #outer} (Structural variant outer.) 5644 */ 5645 public MolecularSequenceStructureVariantOuterComponent getOuter() { 5646 if (this.outer == null) 5647 if (Configuration.errorOnAutoCreate()) 5648 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.outer"); 5649 else if (Configuration.doAutoCreate()) 5650 this.outer = new MolecularSequenceStructureVariantOuterComponent(); // cc 5651 return this.outer; 5652 } 5653 5654 public boolean hasOuter() { 5655 return this.outer != null && !this.outer.isEmpty(); 5656 } 5657 5658 /** 5659 * @param value {@link #outer} (Structural variant outer.) 5660 */ 5661 public MolecularSequenceStructureVariantComponent setOuter(MolecularSequenceStructureVariantOuterComponent value) { 5662 this.outer = value; 5663 return this; 5664 } 5665 5666 /** 5667 * @return {@link #inner} (Structural variant inner.) 5668 */ 5669 public MolecularSequenceStructureVariantInnerComponent getInner() { 5670 if (this.inner == null) 5671 if (Configuration.errorOnAutoCreate()) 5672 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantComponent.inner"); 5673 else if (Configuration.doAutoCreate()) 5674 this.inner = new MolecularSequenceStructureVariantInnerComponent(); // cc 5675 return this.inner; 5676 } 5677 5678 public boolean hasInner() { 5679 return this.inner != null && !this.inner.isEmpty(); 5680 } 5681 5682 /** 5683 * @param value {@link #inner} (Structural variant inner.) 5684 */ 5685 public MolecularSequenceStructureVariantComponent setInner(MolecularSequenceStructureVariantInnerComponent value) { 5686 this.inner = value; 5687 return this; 5688 } 5689 5690 protected void listChildren(List<Property> children) { 5691 super.listChildren(children); 5692 children.add(new Property("variantType", "CodeableConcept", 5693 "Information about chromosome structure variation DNA change type.", 0, 1, variantType)); 5694 children.add(new Property("exact", "boolean", 5695 "Used to indicate if the outer and inner start-end values have the same meaning.", 0, 1, exact)); 5696 children.add(new Property("length", "integer", "Length of the variant chromosome.", 0, 1, length)); 5697 children.add(new Property("outer", "", "Structural variant outer.", 0, 1, outer)); 5698 children.add(new Property("inner", "", "Structural variant inner.", 0, 1, inner)); 5699 } 5700 5701 @Override 5702 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5703 switch (_hash) { 5704 case -1601222305: 5705 /* variantType */ return new Property("variantType", "CodeableConcept", 5706 "Information about chromosome structure variation DNA change type.", 0, 1, variantType); 5707 case 96946943: 5708 /* exact */ return new Property("exact", "boolean", 5709 "Used to indicate if the outer and inner start-end values have the same meaning.", 0, 1, exact); 5710 case -1106363674: 5711 /* length */ return new Property("length", "integer", "Length of the variant chromosome.", 0, 1, length); 5712 case 106111099: 5713 /* outer */ return new Property("outer", "", "Structural variant outer.", 0, 1, outer); 5714 case 100355670: 5715 /* inner */ return new Property("inner", "", "Structural variant inner.", 0, 1, inner); 5716 default: 5717 return super.getNamedProperty(_hash, _name, _checkValid); 5718 } 5719 5720 } 5721 5722 @Override 5723 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5724 switch (hash) { 5725 case -1601222305: 5726 /* variantType */ return this.variantType == null ? new Base[0] : new Base[] { this.variantType }; // CodeableConcept 5727 case 96946943: 5728 /* exact */ return this.exact == null ? new Base[0] : new Base[] { this.exact }; // BooleanType 5729 case -1106363674: 5730 /* length */ return this.length == null ? new Base[0] : new Base[] { this.length }; // IntegerType 5731 case 106111099: 5732 /* outer */ return this.outer == null ? new Base[0] : new Base[] { this.outer }; // MolecularSequenceStructureVariantOuterComponent 5733 case 100355670: 5734 /* inner */ return this.inner == null ? new Base[0] : new Base[] { this.inner }; // MolecularSequenceStructureVariantInnerComponent 5735 default: 5736 return super.getProperty(hash, name, checkValid); 5737 } 5738 5739 } 5740 5741 @Override 5742 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5743 switch (hash) { 5744 case -1601222305: // variantType 5745 this.variantType = castToCodeableConcept(value); // CodeableConcept 5746 return value; 5747 case 96946943: // exact 5748 this.exact = castToBoolean(value); // BooleanType 5749 return value; 5750 case -1106363674: // length 5751 this.length = castToInteger(value); // IntegerType 5752 return value; 5753 case 106111099: // outer 5754 this.outer = (MolecularSequenceStructureVariantOuterComponent) value; // MolecularSequenceStructureVariantOuterComponent 5755 return value; 5756 case 100355670: // inner 5757 this.inner = (MolecularSequenceStructureVariantInnerComponent) value; // MolecularSequenceStructureVariantInnerComponent 5758 return value; 5759 default: 5760 return super.setProperty(hash, name, value); 5761 } 5762 5763 } 5764 5765 @Override 5766 public Base setProperty(String name, Base value) throws FHIRException { 5767 if (name.equals("variantType")) { 5768 this.variantType = castToCodeableConcept(value); // CodeableConcept 5769 } else if (name.equals("exact")) { 5770 this.exact = castToBoolean(value); // BooleanType 5771 } else if (name.equals("length")) { 5772 this.length = castToInteger(value); // IntegerType 5773 } else if (name.equals("outer")) { 5774 this.outer = (MolecularSequenceStructureVariantOuterComponent) value; // MolecularSequenceStructureVariantOuterComponent 5775 } else if (name.equals("inner")) { 5776 this.inner = (MolecularSequenceStructureVariantInnerComponent) value; // MolecularSequenceStructureVariantInnerComponent 5777 } else 5778 return super.setProperty(name, value); 5779 return value; 5780 } 5781 5782 @Override 5783 public void removeChild(String name, Base value) throws FHIRException { 5784 if (name.equals("variantType")) { 5785 this.variantType = null; 5786 } else if (name.equals("exact")) { 5787 this.exact = null; 5788 } else if (name.equals("length")) { 5789 this.length = null; 5790 } else if (name.equals("outer")) { 5791 this.outer = (MolecularSequenceStructureVariantOuterComponent) value; // MolecularSequenceStructureVariantOuterComponent 5792 } else if (name.equals("inner")) { 5793 this.inner = (MolecularSequenceStructureVariantInnerComponent) value; // MolecularSequenceStructureVariantInnerComponent 5794 } else 5795 super.removeChild(name, value); 5796 5797 } 5798 5799 @Override 5800 public Base makeProperty(int hash, String name) throws FHIRException { 5801 switch (hash) { 5802 case -1601222305: 5803 return getVariantType(); 5804 case 96946943: 5805 return getExactElement(); 5806 case -1106363674: 5807 return getLengthElement(); 5808 case 106111099: 5809 return getOuter(); 5810 case 100355670: 5811 return getInner(); 5812 default: 5813 return super.makeProperty(hash, name); 5814 } 5815 5816 } 5817 5818 @Override 5819 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5820 switch (hash) { 5821 case -1601222305: 5822 /* variantType */ return new String[] { "CodeableConcept" }; 5823 case 96946943: 5824 /* exact */ return new String[] { "boolean" }; 5825 case -1106363674: 5826 /* length */ return new String[] { "integer" }; 5827 case 106111099: 5828 /* outer */ return new String[] {}; 5829 case 100355670: 5830 /* inner */ return new String[] {}; 5831 default: 5832 return super.getTypesForProperty(hash, name); 5833 } 5834 5835 } 5836 5837 @Override 5838 public Base addChild(String name) throws FHIRException { 5839 if (name.equals("variantType")) { 5840 this.variantType = new CodeableConcept(); 5841 return this.variantType; 5842 } else if (name.equals("exact")) { 5843 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.exact"); 5844 } else if (name.equals("length")) { 5845 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.length"); 5846 } else if (name.equals("outer")) { 5847 this.outer = new MolecularSequenceStructureVariantOuterComponent(); 5848 return this.outer; 5849 } else if (name.equals("inner")) { 5850 this.inner = new MolecularSequenceStructureVariantInnerComponent(); 5851 return this.inner; 5852 } else 5853 return super.addChild(name); 5854 } 5855 5856 public MolecularSequenceStructureVariantComponent copy() { 5857 MolecularSequenceStructureVariantComponent dst = new MolecularSequenceStructureVariantComponent(); 5858 copyValues(dst); 5859 return dst; 5860 } 5861 5862 public void copyValues(MolecularSequenceStructureVariantComponent dst) { 5863 super.copyValues(dst); 5864 dst.variantType = variantType == null ? null : variantType.copy(); 5865 dst.exact = exact == null ? null : exact.copy(); 5866 dst.length = length == null ? null : length.copy(); 5867 dst.outer = outer == null ? null : outer.copy(); 5868 dst.inner = inner == null ? null : inner.copy(); 5869 } 5870 5871 @Override 5872 public boolean equalsDeep(Base other_) { 5873 if (!super.equalsDeep(other_)) 5874 return false; 5875 if (!(other_ instanceof MolecularSequenceStructureVariantComponent)) 5876 return false; 5877 MolecularSequenceStructureVariantComponent o = (MolecularSequenceStructureVariantComponent) other_; 5878 return compareDeep(variantType, o.variantType, true) && compareDeep(exact, o.exact, true) 5879 && compareDeep(length, o.length, true) && compareDeep(outer, o.outer, true) 5880 && compareDeep(inner, o.inner, true); 5881 } 5882 5883 @Override 5884 public boolean equalsShallow(Base other_) { 5885 if (!super.equalsShallow(other_)) 5886 return false; 5887 if (!(other_ instanceof MolecularSequenceStructureVariantComponent)) 5888 return false; 5889 MolecularSequenceStructureVariantComponent o = (MolecularSequenceStructureVariantComponent) other_; 5890 return compareValues(exact, o.exact, true) && compareValues(length, o.length, true); 5891 } 5892 5893 public boolean isEmpty() { 5894 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(variantType, exact, length, outer, inner); 5895 } 5896 5897 public String fhirType() { 5898 return "MolecularSequence.structureVariant"; 5899 5900 } 5901 5902 } 5903 5904 @Block() 5905 public static class MolecularSequenceStructureVariantOuterComponent extends BackboneElement 5906 implements IBaseBackboneElement { 5907 /** 5908 * Structural variant outer start. If the coordinate system is either 0-based or 5909 * 1-based, then start position is inclusive. 5910 */ 5911 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 5912 @Description(shortDefinition = "Structural variant outer start", formalDefinition = "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 5913 protected IntegerType start; 5914 5915 /** 5916 * Structural variant outer end. If the coordinate system is 0-based then end is 5917 * exclusive and does not include the last position. If the coordinate system is 5918 * 1-base, then end is inclusive and includes the last position. 5919 */ 5920 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 5921 @Description(shortDefinition = "Structural variant outer end", formalDefinition = "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 5922 protected IntegerType end; 5923 5924 private static final long serialVersionUID = -1798864889L; 5925 5926 /** 5927 * Constructor 5928 */ 5929 public MolecularSequenceStructureVariantOuterComponent() { 5930 super(); 5931 } 5932 5933 /** 5934 * @return {@link #start} (Structural variant outer start. If the coordinate 5935 * system is either 0-based or 1-based, then start position is 5936 * inclusive.). This is the underlying object with id, value and 5937 * extensions. The accessor "getStart" gives direct access to the value 5938 */ 5939 public IntegerType getStartElement() { 5940 if (this.start == null) 5941 if (Configuration.errorOnAutoCreate()) 5942 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantOuterComponent.start"); 5943 else if (Configuration.doAutoCreate()) 5944 this.start = new IntegerType(); // bb 5945 return this.start; 5946 } 5947 5948 public boolean hasStartElement() { 5949 return this.start != null && !this.start.isEmpty(); 5950 } 5951 5952 public boolean hasStart() { 5953 return this.start != null && !this.start.isEmpty(); 5954 } 5955 5956 /** 5957 * @param value {@link #start} (Structural variant outer start. If the 5958 * coordinate system is either 0-based or 1-based, then start 5959 * position is inclusive.). This is the underlying object with id, 5960 * value and extensions. The accessor "getStart" gives direct 5961 * access to the value 5962 */ 5963 public MolecularSequenceStructureVariantOuterComponent setStartElement(IntegerType value) { 5964 this.start = value; 5965 return this; 5966 } 5967 5968 /** 5969 * @return Structural variant outer start. If the coordinate system is either 5970 * 0-based or 1-based, then start position is inclusive. 5971 */ 5972 public int getStart() { 5973 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 5974 } 5975 5976 /** 5977 * @param value Structural variant outer start. If the coordinate system is 5978 * either 0-based or 1-based, then start position is inclusive. 5979 */ 5980 public MolecularSequenceStructureVariantOuterComponent setStart(int value) { 5981 if (this.start == null) 5982 this.start = new IntegerType(); 5983 this.start.setValue(value); 5984 return this; 5985 } 5986 5987 /** 5988 * @return {@link #end} (Structural variant outer end. If the coordinate system 5989 * is 0-based then end is exclusive and does not include the last 5990 * position. If the coordinate system is 1-base, then end is inclusive 5991 * and includes the last position.). This is the underlying object with 5992 * id, value and extensions. The accessor "getEnd" gives direct access 5993 * to the value 5994 */ 5995 public IntegerType getEndElement() { 5996 if (this.end == null) 5997 if (Configuration.errorOnAutoCreate()) 5998 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantOuterComponent.end"); 5999 else if (Configuration.doAutoCreate()) 6000 this.end = new IntegerType(); // bb 6001 return this.end; 6002 } 6003 6004 public boolean hasEndElement() { 6005 return this.end != null && !this.end.isEmpty(); 6006 } 6007 6008 public boolean hasEnd() { 6009 return this.end != null && !this.end.isEmpty(); 6010 } 6011 6012 /** 6013 * @param value {@link #end} (Structural variant outer end. If the coordinate 6014 * system is 0-based then end is exclusive and does not include the 6015 * last position. If the coordinate system is 1-base, then end is 6016 * inclusive and includes the last position.). This is the 6017 * underlying object with id, value and extensions. The accessor 6018 * "getEnd" gives direct access to the value 6019 */ 6020 public MolecularSequenceStructureVariantOuterComponent setEndElement(IntegerType value) { 6021 this.end = value; 6022 return this; 6023 } 6024 6025 /** 6026 * @return Structural variant outer end. If the coordinate system is 0-based 6027 * then end is exclusive and does not include the last position. If the 6028 * coordinate system is 1-base, then end is inclusive and includes the 6029 * last position. 6030 */ 6031 public int getEnd() { 6032 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 6033 } 6034 6035 /** 6036 * @param value Structural variant outer end. If the coordinate system is 6037 * 0-based then end is exclusive and does not include the last 6038 * position. If the coordinate system is 1-base, then end is 6039 * inclusive and includes the last position. 6040 */ 6041 public MolecularSequenceStructureVariantOuterComponent setEnd(int value) { 6042 if (this.end == null) 6043 this.end = new IntegerType(); 6044 this.end.setValue(value); 6045 return this; 6046 } 6047 6048 protected void listChildren(List<Property> children) { 6049 super.listChildren(children); 6050 children.add(new Property("start", "integer", 6051 "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6052 0, 1, start)); 6053 children.add(new Property("end", "integer", 6054 "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6055 0, 1, end)); 6056 } 6057 6058 @Override 6059 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6060 switch (_hash) { 6061 case 109757538: 6062 /* start */ return new Property("start", "integer", 6063 "Structural variant outer start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6064 0, 1, start); 6065 case 100571: 6066 /* end */ return new Property("end", "integer", 6067 "Structural variant outer end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6068 0, 1, end); 6069 default: 6070 return super.getNamedProperty(_hash, _name, _checkValid); 6071 } 6072 6073 } 6074 6075 @Override 6076 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6077 switch (hash) { 6078 case 109757538: 6079 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 6080 case 100571: 6081 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 6082 default: 6083 return super.getProperty(hash, name, checkValid); 6084 } 6085 6086 } 6087 6088 @Override 6089 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6090 switch (hash) { 6091 case 109757538: // start 6092 this.start = castToInteger(value); // IntegerType 6093 return value; 6094 case 100571: // end 6095 this.end = castToInteger(value); // IntegerType 6096 return value; 6097 default: 6098 return super.setProperty(hash, name, value); 6099 } 6100 6101 } 6102 6103 @Override 6104 public Base setProperty(String name, Base value) throws FHIRException { 6105 if (name.equals("start")) { 6106 this.start = castToInteger(value); // IntegerType 6107 } else if (name.equals("end")) { 6108 this.end = castToInteger(value); // IntegerType 6109 } else 6110 return super.setProperty(name, value); 6111 return value; 6112 } 6113 6114 @Override 6115 public void removeChild(String name, Base value) throws FHIRException { 6116 if (name.equals("start")) { 6117 this.start = null; 6118 } else if (name.equals("end")) { 6119 this.end = null; 6120 } else 6121 super.removeChild(name, value); 6122 6123 } 6124 6125 @Override 6126 public Base makeProperty(int hash, String name) throws FHIRException { 6127 switch (hash) { 6128 case 109757538: 6129 return getStartElement(); 6130 case 100571: 6131 return getEndElement(); 6132 default: 6133 return super.makeProperty(hash, name); 6134 } 6135 6136 } 6137 6138 @Override 6139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6140 switch (hash) { 6141 case 109757538: 6142 /* start */ return new String[] { "integer" }; 6143 case 100571: 6144 /* end */ return new String[] { "integer" }; 6145 default: 6146 return super.getTypesForProperty(hash, name); 6147 } 6148 6149 } 6150 6151 @Override 6152 public Base addChild(String name) throws FHIRException { 6153 if (name.equals("start")) { 6154 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 6155 } else if (name.equals("end")) { 6156 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 6157 } else 6158 return super.addChild(name); 6159 } 6160 6161 public MolecularSequenceStructureVariantOuterComponent copy() { 6162 MolecularSequenceStructureVariantOuterComponent dst = new MolecularSequenceStructureVariantOuterComponent(); 6163 copyValues(dst); 6164 return dst; 6165 } 6166 6167 public void copyValues(MolecularSequenceStructureVariantOuterComponent dst) { 6168 super.copyValues(dst); 6169 dst.start = start == null ? null : start.copy(); 6170 dst.end = end == null ? null : end.copy(); 6171 } 6172 6173 @Override 6174 public boolean equalsDeep(Base other_) { 6175 if (!super.equalsDeep(other_)) 6176 return false; 6177 if (!(other_ instanceof MolecularSequenceStructureVariantOuterComponent)) 6178 return false; 6179 MolecularSequenceStructureVariantOuterComponent o = (MolecularSequenceStructureVariantOuterComponent) other_; 6180 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 6181 } 6182 6183 @Override 6184 public boolean equalsShallow(Base other_) { 6185 if (!super.equalsShallow(other_)) 6186 return false; 6187 if (!(other_ instanceof MolecularSequenceStructureVariantOuterComponent)) 6188 return false; 6189 MolecularSequenceStructureVariantOuterComponent o = (MolecularSequenceStructureVariantOuterComponent) other_; 6190 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 6191 } 6192 6193 public boolean isEmpty() { 6194 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 6195 } 6196 6197 public String fhirType() { 6198 return "MolecularSequence.structureVariant.outer"; 6199 6200 } 6201 6202 } 6203 6204 @Block() 6205 public static class MolecularSequenceStructureVariantInnerComponent extends BackboneElement 6206 implements IBaseBackboneElement { 6207 /** 6208 * Structural variant inner start. If the coordinate system is either 0-based or 6209 * 1-based, then start position is inclusive. 6210 */ 6211 @Child(name = "start", type = { IntegerType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 6212 @Description(shortDefinition = "Structural variant inner start", formalDefinition = "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.") 6213 protected IntegerType start; 6214 6215 /** 6216 * Structural variant inner end. If the coordinate system is 0-based then end is 6217 * exclusive and does not include the last position. If the coordinate system is 6218 * 1-base, then end is inclusive and includes the last position. 6219 */ 6220 @Child(name = "end", type = { IntegerType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 6221 @Description(shortDefinition = "Structural variant inner end", formalDefinition = "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.") 6222 protected IntegerType end; 6223 6224 private static final long serialVersionUID = -1798864889L; 6225 6226 /** 6227 * Constructor 6228 */ 6229 public MolecularSequenceStructureVariantInnerComponent() { 6230 super(); 6231 } 6232 6233 /** 6234 * @return {@link #start} (Structural variant inner start. If the coordinate 6235 * system is either 0-based or 1-based, then start position is 6236 * inclusive.). This is the underlying object with id, value and 6237 * extensions. The accessor "getStart" gives direct access to the value 6238 */ 6239 public IntegerType getStartElement() { 6240 if (this.start == null) 6241 if (Configuration.errorOnAutoCreate()) 6242 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantInnerComponent.start"); 6243 else if (Configuration.doAutoCreate()) 6244 this.start = new IntegerType(); // bb 6245 return this.start; 6246 } 6247 6248 public boolean hasStartElement() { 6249 return this.start != null && !this.start.isEmpty(); 6250 } 6251 6252 public boolean hasStart() { 6253 return this.start != null && !this.start.isEmpty(); 6254 } 6255 6256 /** 6257 * @param value {@link #start} (Structural variant inner start. If the 6258 * coordinate system is either 0-based or 1-based, then start 6259 * position is inclusive.). This is the underlying object with id, 6260 * value and extensions. The accessor "getStart" gives direct 6261 * access to the value 6262 */ 6263 public MolecularSequenceStructureVariantInnerComponent setStartElement(IntegerType value) { 6264 this.start = value; 6265 return this; 6266 } 6267 6268 /** 6269 * @return Structural variant inner start. If the coordinate system is either 6270 * 0-based or 1-based, then start position is inclusive. 6271 */ 6272 public int getStart() { 6273 return this.start == null || this.start.isEmpty() ? 0 : this.start.getValue(); 6274 } 6275 6276 /** 6277 * @param value Structural variant inner start. If the coordinate system is 6278 * either 0-based or 1-based, then start position is inclusive. 6279 */ 6280 public MolecularSequenceStructureVariantInnerComponent setStart(int value) { 6281 if (this.start == null) 6282 this.start = new IntegerType(); 6283 this.start.setValue(value); 6284 return this; 6285 } 6286 6287 /** 6288 * @return {@link #end} (Structural variant inner end. If the coordinate system 6289 * is 0-based then end is exclusive and does not include the last 6290 * position. If the coordinate system is 1-base, then end is inclusive 6291 * and includes the last position.). This is the underlying object with 6292 * id, value and extensions. The accessor "getEnd" gives direct access 6293 * to the value 6294 */ 6295 public IntegerType getEndElement() { 6296 if (this.end == null) 6297 if (Configuration.errorOnAutoCreate()) 6298 throw new Error("Attempt to auto-create MolecularSequenceStructureVariantInnerComponent.end"); 6299 else if (Configuration.doAutoCreate()) 6300 this.end = new IntegerType(); // bb 6301 return this.end; 6302 } 6303 6304 public boolean hasEndElement() { 6305 return this.end != null && !this.end.isEmpty(); 6306 } 6307 6308 public boolean hasEnd() { 6309 return this.end != null && !this.end.isEmpty(); 6310 } 6311 6312 /** 6313 * @param value {@link #end} (Structural variant inner end. If the coordinate 6314 * system is 0-based then end is exclusive and does not include the 6315 * last position. If the coordinate system is 1-base, then end is 6316 * inclusive and includes the last position.). This is the 6317 * underlying object with id, value and extensions. The accessor 6318 * "getEnd" gives direct access to the value 6319 */ 6320 public MolecularSequenceStructureVariantInnerComponent setEndElement(IntegerType value) { 6321 this.end = value; 6322 return this; 6323 } 6324 6325 /** 6326 * @return Structural variant inner end. If the coordinate system is 0-based 6327 * then end is exclusive and does not include the last position. If the 6328 * coordinate system is 1-base, then end is inclusive and includes the 6329 * last position. 6330 */ 6331 public int getEnd() { 6332 return this.end == null || this.end.isEmpty() ? 0 : this.end.getValue(); 6333 } 6334 6335 /** 6336 * @param value Structural variant inner end. If the coordinate system is 6337 * 0-based then end is exclusive and does not include the last 6338 * position. If the coordinate system is 1-base, then end is 6339 * inclusive and includes the last position. 6340 */ 6341 public MolecularSequenceStructureVariantInnerComponent setEnd(int value) { 6342 if (this.end == null) 6343 this.end = new IntegerType(); 6344 this.end.setValue(value); 6345 return this; 6346 } 6347 6348 protected void listChildren(List<Property> children) { 6349 super.listChildren(children); 6350 children.add(new Property("start", "integer", 6351 "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6352 0, 1, start)); 6353 children.add(new Property("end", "integer", 6354 "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6355 0, 1, end)); 6356 } 6357 6358 @Override 6359 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6360 switch (_hash) { 6361 case 109757538: 6362 /* start */ return new Property("start", "integer", 6363 "Structural variant inner start. If the coordinate system is either 0-based or 1-based, then start position is inclusive.", 6364 0, 1, start); 6365 case 100571: 6366 /* end */ return new Property("end", "integer", 6367 "Structural variant inner end. If the coordinate system is 0-based then end is exclusive and does not include the last position. If the coordinate system is 1-base, then end is inclusive and includes the last position.", 6368 0, 1, end); 6369 default: 6370 return super.getNamedProperty(_hash, _name, _checkValid); 6371 } 6372 6373 } 6374 6375 @Override 6376 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6377 switch (hash) { 6378 case 109757538: 6379 /* start */ return this.start == null ? new Base[0] : new Base[] { this.start }; // IntegerType 6380 case 100571: 6381 /* end */ return this.end == null ? new Base[0] : new Base[] { this.end }; // IntegerType 6382 default: 6383 return super.getProperty(hash, name, checkValid); 6384 } 6385 6386 } 6387 6388 @Override 6389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6390 switch (hash) { 6391 case 109757538: // start 6392 this.start = castToInteger(value); // IntegerType 6393 return value; 6394 case 100571: // end 6395 this.end = castToInteger(value); // IntegerType 6396 return value; 6397 default: 6398 return super.setProperty(hash, name, value); 6399 } 6400 6401 } 6402 6403 @Override 6404 public Base setProperty(String name, Base value) throws FHIRException { 6405 if (name.equals("start")) { 6406 this.start = castToInteger(value); // IntegerType 6407 } else if (name.equals("end")) { 6408 this.end = castToInteger(value); // IntegerType 6409 } else 6410 return super.setProperty(name, value); 6411 return value; 6412 } 6413 6414 @Override 6415 public void removeChild(String name, Base value) throws FHIRException { 6416 if (name.equals("start")) { 6417 this.start = null; 6418 } else if (name.equals("end")) { 6419 this.end = null; 6420 } else 6421 super.removeChild(name, value); 6422 6423 } 6424 6425 @Override 6426 public Base makeProperty(int hash, String name) throws FHIRException { 6427 switch (hash) { 6428 case 109757538: 6429 return getStartElement(); 6430 case 100571: 6431 return getEndElement(); 6432 default: 6433 return super.makeProperty(hash, name); 6434 } 6435 6436 } 6437 6438 @Override 6439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6440 switch (hash) { 6441 case 109757538: 6442 /* start */ return new String[] { "integer" }; 6443 case 100571: 6444 /* end */ return new String[] { "integer" }; 6445 default: 6446 return super.getTypesForProperty(hash, name); 6447 } 6448 6449 } 6450 6451 @Override 6452 public Base addChild(String name) throws FHIRException { 6453 if (name.equals("start")) { 6454 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.start"); 6455 } else if (name.equals("end")) { 6456 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.end"); 6457 } else 6458 return super.addChild(name); 6459 } 6460 6461 public MolecularSequenceStructureVariantInnerComponent copy() { 6462 MolecularSequenceStructureVariantInnerComponent dst = new MolecularSequenceStructureVariantInnerComponent(); 6463 copyValues(dst); 6464 return dst; 6465 } 6466 6467 public void copyValues(MolecularSequenceStructureVariantInnerComponent dst) { 6468 super.copyValues(dst); 6469 dst.start = start == null ? null : start.copy(); 6470 dst.end = end == null ? null : end.copy(); 6471 } 6472 6473 @Override 6474 public boolean equalsDeep(Base other_) { 6475 if (!super.equalsDeep(other_)) 6476 return false; 6477 if (!(other_ instanceof MolecularSequenceStructureVariantInnerComponent)) 6478 return false; 6479 MolecularSequenceStructureVariantInnerComponent o = (MolecularSequenceStructureVariantInnerComponent) other_; 6480 return compareDeep(start, o.start, true) && compareDeep(end, o.end, true); 6481 } 6482 6483 @Override 6484 public boolean equalsShallow(Base other_) { 6485 if (!super.equalsShallow(other_)) 6486 return false; 6487 if (!(other_ instanceof MolecularSequenceStructureVariantInnerComponent)) 6488 return false; 6489 MolecularSequenceStructureVariantInnerComponent o = (MolecularSequenceStructureVariantInnerComponent) other_; 6490 return compareValues(start, o.start, true) && compareValues(end, o.end, true); 6491 } 6492 6493 public boolean isEmpty() { 6494 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(start, end); 6495 } 6496 6497 public String fhirType() { 6498 return "MolecularSequence.structureVariant.inner"; 6499 6500 } 6501 6502 } 6503 6504 /** 6505 * A unique identifier for this particular sequence instance. This is a 6506 * FHIR-defined id. 6507 */ 6508 @Child(name = "identifier", type = { 6509 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6510 @Description(shortDefinition = "Unique ID for this particular sequence. This is a FHIR-defined id", formalDefinition = "A unique identifier for this particular sequence instance. This is a FHIR-defined id.") 6511 protected List<Identifier> identifier; 6512 6513 /** 6514 * Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6515 */ 6516 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 6517 @Description(shortDefinition = "aa | dna | rna", formalDefinition = "Amino Acid Sequence/ DNA Sequence / RNA Sequence.") 6518 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/sequence-type") 6519 protected Enumeration<SequenceType> type; 6520 6521 /** 6522 * Whether the sequence is numbered starting at 0 (0-based numbering or 6523 * coordinates, inclusive start, exclusive end) or starting at 1 (1-based 6524 * numbering, inclusive start and inclusive end). 6525 */ 6526 @Child(name = "coordinateSystem", type = { 6527 IntegerType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 6528 @Description(shortDefinition = "Base number of coordinate system (0 for 0-based numbering or coordinates, inclusive start, exclusive end, 1 for 1-based numbering, inclusive start, inclusive end)", formalDefinition = "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).") 6529 protected IntegerType coordinateSystem; 6530 6531 /** 6532 * The patient whose sequencing results are described by this resource. 6533 */ 6534 @Child(name = "patient", type = { Patient.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 6535 @Description(shortDefinition = "Who and/or what this is about", formalDefinition = "The patient whose sequencing results are described by this resource.") 6536 protected Reference patient; 6537 6538 /** 6539 * The actual object that is the target of the reference (The patient whose 6540 * sequencing results are described by this resource.) 6541 */ 6542 protected Patient patientTarget; 6543 6544 /** 6545 * Specimen used for sequencing. 6546 */ 6547 @Child(name = "specimen", type = { Specimen.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 6548 @Description(shortDefinition = "Specimen used for sequencing", formalDefinition = "Specimen used for sequencing.") 6549 protected Reference specimen; 6550 6551 /** 6552 * The actual object that is the target of the reference (Specimen used for 6553 * sequencing.) 6554 */ 6555 protected Specimen specimenTarget; 6556 6557 /** 6558 * The method for sequencing, for example, chip information. 6559 */ 6560 @Child(name = "device", type = { Device.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 6561 @Description(shortDefinition = "The method for sequencing", formalDefinition = "The method for sequencing, for example, chip information.") 6562 protected Reference device; 6563 6564 /** 6565 * The actual object that is the target of the reference (The method for 6566 * sequencing, for example, chip information.) 6567 */ 6568 protected Device deviceTarget; 6569 6570 /** 6571 * The organization or lab that should be responsible for this result. 6572 */ 6573 @Child(name = "performer", type = { 6574 Organization.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 6575 @Description(shortDefinition = "Who should be responsible for test result", formalDefinition = "The organization or lab that should be responsible for this result.") 6576 protected Reference performer; 6577 6578 /** 6579 * The actual object that is the target of the reference (The organization or 6580 * lab that should be responsible for this result.) 6581 */ 6582 protected Organization performerTarget; 6583 6584 /** 6585 * The number of copies of the sequence of interest. (RNASeq). 6586 */ 6587 @Child(name = "quantity", type = { Quantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 6588 @Description(shortDefinition = "The number of copies of the sequence of interest. (RNASeq)", formalDefinition = "The number of copies of the sequence of interest. (RNASeq).") 6589 protected Quantity quantity; 6590 6591 /** 6592 * A sequence that is used as a reference to describe variants that are present 6593 * in a sequence analyzed. 6594 */ 6595 @Child(name = "referenceSeq", type = {}, order = 8, min = 0, max = 1, modifier = false, summary = true) 6596 @Description(shortDefinition = "A sequence used as reference", formalDefinition = "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.") 6597 protected MolecularSequenceReferenceSeqComponent referenceSeq; 6598 6599 /** 6600 * The definition of variant here originates from Sequence ontology 6601 * ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). 6602 * This element can represent amino acid or nucleic sequence change(including 6603 * insertion,deletion,SNP,etc.) It can represent some complex mutation or 6604 * segment variation with the assist of CIGAR string. 6605 */ 6606 @Child(name = "variant", type = {}, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6607 @Description(shortDefinition = "Variant in sequence", formalDefinition = "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.") 6608 protected List<MolecularSequenceVariantComponent> variant; 6609 6610 /** 6611 * Sequence that was observed. It is the result marked by referenceSeq along 6612 * with variant records on referenceSeq. This shall start from 6613 * referenceSeq.windowStart and end by referenceSeq.windowEnd. 6614 */ 6615 @Child(name = "observedSeq", type = { 6616 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 6617 @Description(shortDefinition = "Sequence that was observed", formalDefinition = "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.") 6618 protected StringType observedSeq; 6619 6620 /** 6621 * An experimental feature attribute that defines the quality of the feature in 6622 * a quantitative way, such as a phred quality score 6623 * ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)). 6624 */ 6625 @Child(name = "quality", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6626 @Description(shortDefinition = "An set of value as quality of sequence", formalDefinition = "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).") 6627 protected List<MolecularSequenceQualityComponent> quality; 6628 6629 /** 6630 * Coverage (read depth or depth) is the average number of reads representing a 6631 * given nucleotide in the reconstructed sequence. 6632 */ 6633 @Child(name = "readCoverage", type = { 6634 IntegerType.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 6635 @Description(shortDefinition = "Average number of reads representing a given nucleotide in the reconstructed sequence", formalDefinition = "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.") 6636 protected IntegerType readCoverage; 6637 6638 /** 6639 * Configurations of the external repository. The repository shall store 6640 * target's observedSeq or records related with target's observedSeq. 6641 */ 6642 @Child(name = "repository", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6643 @Description(shortDefinition = "External repository which contains detailed report related with observedSeq in this resource", formalDefinition = "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.") 6644 protected List<MolecularSequenceRepositoryComponent> repository; 6645 6646 /** 6647 * Pointer to next atomic sequence which at most contains one variant. 6648 */ 6649 @Child(name = "pointer", type = { 6650 MolecularSequence.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6651 @Description(shortDefinition = "Pointer to next atomic sequence", formalDefinition = "Pointer to next atomic sequence which at most contains one variant.") 6652 protected List<Reference> pointer; 6653 /** 6654 * The actual objects that are the target of the reference (Pointer to next 6655 * atomic sequence which at most contains one variant.) 6656 */ 6657 protected List<MolecularSequence> pointerTarget; 6658 6659 /** 6660 * Information about chromosome structure variation. 6661 */ 6662 @Child(name = "structureVariant", type = {}, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 6663 @Description(shortDefinition = "Structural variant", formalDefinition = "Information about chromosome structure variation.") 6664 protected List<MolecularSequenceStructureVariantComponent> structureVariant; 6665 6666 private static final long serialVersionUID = -1541133500L; 6667 6668 /** 6669 * Constructor 6670 */ 6671 public MolecularSequence() { 6672 super(); 6673 } 6674 6675 /** 6676 * Constructor 6677 */ 6678 public MolecularSequence(IntegerType coordinateSystem) { 6679 super(); 6680 this.coordinateSystem = coordinateSystem; 6681 } 6682 6683 /** 6684 * @return {@link #identifier} (A unique identifier for this particular sequence 6685 * instance. This is a FHIR-defined id.) 6686 */ 6687 public List<Identifier> getIdentifier() { 6688 if (this.identifier == null) 6689 this.identifier = new ArrayList<Identifier>(); 6690 return this.identifier; 6691 } 6692 6693 /** 6694 * @return Returns a reference to <code>this</code> for easy method chaining 6695 */ 6696 public MolecularSequence setIdentifier(List<Identifier> theIdentifier) { 6697 this.identifier = theIdentifier; 6698 return this; 6699 } 6700 6701 public boolean hasIdentifier() { 6702 if (this.identifier == null) 6703 return false; 6704 for (Identifier item : this.identifier) 6705 if (!item.isEmpty()) 6706 return true; 6707 return false; 6708 } 6709 6710 public Identifier addIdentifier() { // 3 6711 Identifier t = new Identifier(); 6712 if (this.identifier == null) 6713 this.identifier = new ArrayList<Identifier>(); 6714 this.identifier.add(t); 6715 return t; 6716 } 6717 6718 public MolecularSequence addIdentifier(Identifier t) { // 3 6719 if (t == null) 6720 return this; 6721 if (this.identifier == null) 6722 this.identifier = new ArrayList<Identifier>(); 6723 this.identifier.add(t); 6724 return this; 6725 } 6726 6727 /** 6728 * @return The first repetition of repeating field {@link #identifier}, creating 6729 * it if it does not already exist 6730 */ 6731 public Identifier getIdentifierFirstRep() { 6732 if (getIdentifier().isEmpty()) { 6733 addIdentifier(); 6734 } 6735 return getIdentifier().get(0); 6736 } 6737 6738 /** 6739 * @return {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA Sequence.). 6740 * This is the underlying object with id, value and extensions. The 6741 * accessor "getType" gives direct access to the value 6742 */ 6743 public Enumeration<SequenceType> getTypeElement() { 6744 if (this.type == null) 6745 if (Configuration.errorOnAutoCreate()) 6746 throw new Error("Attempt to auto-create MolecularSequence.type"); 6747 else if (Configuration.doAutoCreate()) 6748 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); // bb 6749 return this.type; 6750 } 6751 6752 public boolean hasTypeElement() { 6753 return this.type != null && !this.type.isEmpty(); 6754 } 6755 6756 public boolean hasType() { 6757 return this.type != null && !this.type.isEmpty(); 6758 } 6759 6760 /** 6761 * @param value {@link #type} (Amino Acid Sequence/ DNA Sequence / RNA 6762 * Sequence.). This is the underlying object with id, value and 6763 * extensions. The accessor "getType" gives direct access to the 6764 * value 6765 */ 6766 public MolecularSequence setTypeElement(Enumeration<SequenceType> value) { 6767 this.type = value; 6768 return this; 6769 } 6770 6771 /** 6772 * @return Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6773 */ 6774 public SequenceType getType() { 6775 return this.type == null ? null : this.type.getValue(); 6776 } 6777 6778 /** 6779 * @param value Amino Acid Sequence/ DNA Sequence / RNA Sequence. 6780 */ 6781 public MolecularSequence setType(SequenceType value) { 6782 if (value == null) 6783 this.type = null; 6784 else { 6785 if (this.type == null) 6786 this.type = new Enumeration<SequenceType>(new SequenceTypeEnumFactory()); 6787 this.type.setValue(value); 6788 } 6789 return this; 6790 } 6791 6792 /** 6793 * @return {@link #coordinateSystem} (Whether the sequence is numbered starting 6794 * at 0 (0-based numbering or coordinates, inclusive start, exclusive 6795 * end) or starting at 1 (1-based numbering, inclusive start and 6796 * inclusive end).). This is the underlying object with id, value and 6797 * extensions. The accessor "getCoordinateSystem" gives direct access to 6798 * the value 6799 */ 6800 public IntegerType getCoordinateSystemElement() { 6801 if (this.coordinateSystem == null) 6802 if (Configuration.errorOnAutoCreate()) 6803 throw new Error("Attempt to auto-create MolecularSequence.coordinateSystem"); 6804 else if (Configuration.doAutoCreate()) 6805 this.coordinateSystem = new IntegerType(); // bb 6806 return this.coordinateSystem; 6807 } 6808 6809 public boolean hasCoordinateSystemElement() { 6810 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 6811 } 6812 6813 public boolean hasCoordinateSystem() { 6814 return this.coordinateSystem != null && !this.coordinateSystem.isEmpty(); 6815 } 6816 6817 /** 6818 * @param value {@link #coordinateSystem} (Whether the sequence is numbered 6819 * starting at 0 (0-based numbering or coordinates, inclusive 6820 * start, exclusive end) or starting at 1 (1-based numbering, 6821 * inclusive start and inclusive end).). This is the underlying 6822 * object with id, value and extensions. The accessor 6823 * "getCoordinateSystem" gives direct access to the value 6824 */ 6825 public MolecularSequence setCoordinateSystemElement(IntegerType value) { 6826 this.coordinateSystem = value; 6827 return this; 6828 } 6829 6830 /** 6831 * @return Whether the sequence is numbered starting at 0 (0-based numbering or 6832 * coordinates, inclusive start, exclusive end) or starting at 1 6833 * (1-based numbering, inclusive start and inclusive end). 6834 */ 6835 public int getCoordinateSystem() { 6836 return this.coordinateSystem == null || this.coordinateSystem.isEmpty() ? 0 : this.coordinateSystem.getValue(); 6837 } 6838 6839 /** 6840 * @param value Whether the sequence is numbered starting at 0 (0-based 6841 * numbering or coordinates, inclusive start, exclusive end) or 6842 * starting at 1 (1-based numbering, inclusive start and inclusive 6843 * end). 6844 */ 6845 public MolecularSequence setCoordinateSystem(int value) { 6846 if (this.coordinateSystem == null) 6847 this.coordinateSystem = new IntegerType(); 6848 this.coordinateSystem.setValue(value); 6849 return this; 6850 } 6851 6852 /** 6853 * @return {@link #patient} (The patient whose sequencing results are described 6854 * by this resource.) 6855 */ 6856 public Reference getPatient() { 6857 if (this.patient == null) 6858 if (Configuration.errorOnAutoCreate()) 6859 throw new Error("Attempt to auto-create MolecularSequence.patient"); 6860 else if (Configuration.doAutoCreate()) 6861 this.patient = new Reference(); // cc 6862 return this.patient; 6863 } 6864 6865 public boolean hasPatient() { 6866 return this.patient != null && !this.patient.isEmpty(); 6867 } 6868 6869 /** 6870 * @param value {@link #patient} (The patient whose sequencing results are 6871 * described by this resource.) 6872 */ 6873 public MolecularSequence setPatient(Reference value) { 6874 this.patient = value; 6875 return this; 6876 } 6877 6878 /** 6879 * @return {@link #patient} The actual object that is the target of the 6880 * reference. The reference library doesn't populate this, but you can 6881 * use it to hold the resource if you resolve it. (The patient whose 6882 * sequencing results are described by this resource.) 6883 */ 6884 public Patient getPatientTarget() { 6885 if (this.patientTarget == null) 6886 if (Configuration.errorOnAutoCreate()) 6887 throw new Error("Attempt to auto-create MolecularSequence.patient"); 6888 else if (Configuration.doAutoCreate()) 6889 this.patientTarget = new Patient(); // aa 6890 return this.patientTarget; 6891 } 6892 6893 /** 6894 * @param value {@link #patient} The actual object that is the target of the 6895 * reference. The reference library doesn't use these, but you can 6896 * use it to hold the resource if you resolve it. (The patient 6897 * whose sequencing results are described by this resource.) 6898 */ 6899 public MolecularSequence setPatientTarget(Patient value) { 6900 this.patientTarget = value; 6901 return this; 6902 } 6903 6904 /** 6905 * @return {@link #specimen} (Specimen used for sequencing.) 6906 */ 6907 public Reference getSpecimen() { 6908 if (this.specimen == null) 6909 if (Configuration.errorOnAutoCreate()) 6910 throw new Error("Attempt to auto-create MolecularSequence.specimen"); 6911 else if (Configuration.doAutoCreate()) 6912 this.specimen = new Reference(); // cc 6913 return this.specimen; 6914 } 6915 6916 public boolean hasSpecimen() { 6917 return this.specimen != null && !this.specimen.isEmpty(); 6918 } 6919 6920 /** 6921 * @param value {@link #specimen} (Specimen used for sequencing.) 6922 */ 6923 public MolecularSequence setSpecimen(Reference value) { 6924 this.specimen = value; 6925 return this; 6926 } 6927 6928 /** 6929 * @return {@link #specimen} The actual object that is the target of the 6930 * reference. The reference library doesn't populate this, but you can 6931 * use it to hold the resource if you resolve it. (Specimen used for 6932 * sequencing.) 6933 */ 6934 public Specimen getSpecimenTarget() { 6935 if (this.specimenTarget == null) 6936 if (Configuration.errorOnAutoCreate()) 6937 throw new Error("Attempt to auto-create MolecularSequence.specimen"); 6938 else if (Configuration.doAutoCreate()) 6939 this.specimenTarget = new Specimen(); // aa 6940 return this.specimenTarget; 6941 } 6942 6943 /** 6944 * @param value {@link #specimen} The actual object that is the target of the 6945 * reference. The reference library doesn't use these, but you can 6946 * use it to hold the resource if you resolve it. (Specimen used 6947 * for sequencing.) 6948 */ 6949 public MolecularSequence setSpecimenTarget(Specimen value) { 6950 this.specimenTarget = value; 6951 return this; 6952 } 6953 6954 /** 6955 * @return {@link #device} (The method for sequencing, for example, chip 6956 * information.) 6957 */ 6958 public Reference getDevice() { 6959 if (this.device == null) 6960 if (Configuration.errorOnAutoCreate()) 6961 throw new Error("Attempt to auto-create MolecularSequence.device"); 6962 else if (Configuration.doAutoCreate()) 6963 this.device = new Reference(); // cc 6964 return this.device; 6965 } 6966 6967 public boolean hasDevice() { 6968 return this.device != null && !this.device.isEmpty(); 6969 } 6970 6971 /** 6972 * @param value {@link #device} (The method for sequencing, for example, chip 6973 * information.) 6974 */ 6975 public MolecularSequence setDevice(Reference value) { 6976 this.device = value; 6977 return this; 6978 } 6979 6980 /** 6981 * @return {@link #device} The actual object that is the target of the 6982 * reference. The reference library doesn't populate this, but you can 6983 * use it to hold the resource if you resolve it. (The method for 6984 * sequencing, for example, chip information.) 6985 */ 6986 public Device getDeviceTarget() { 6987 if (this.deviceTarget == null) 6988 if (Configuration.errorOnAutoCreate()) 6989 throw new Error("Attempt to auto-create MolecularSequence.device"); 6990 else if (Configuration.doAutoCreate()) 6991 this.deviceTarget = new Device(); // aa 6992 return this.deviceTarget; 6993 } 6994 6995 /** 6996 * @param value {@link #device} The actual object that is the target of the 6997 * reference. The reference library doesn't use these, but you can 6998 * use it to hold the resource if you resolve it. (The method for 6999 * sequencing, for example, chip information.) 7000 */ 7001 public MolecularSequence setDeviceTarget(Device value) { 7002 this.deviceTarget = value; 7003 return this; 7004 } 7005 7006 /** 7007 * @return {@link #performer} (The organization or lab that should be 7008 * responsible for this result.) 7009 */ 7010 public Reference getPerformer() { 7011 if (this.performer == null) 7012 if (Configuration.errorOnAutoCreate()) 7013 throw new Error("Attempt to auto-create MolecularSequence.performer"); 7014 else if (Configuration.doAutoCreate()) 7015 this.performer = new Reference(); // cc 7016 return this.performer; 7017 } 7018 7019 public boolean hasPerformer() { 7020 return this.performer != null && !this.performer.isEmpty(); 7021 } 7022 7023 /** 7024 * @param value {@link #performer} (The organization or lab that should be 7025 * responsible for this result.) 7026 */ 7027 public MolecularSequence setPerformer(Reference value) { 7028 this.performer = value; 7029 return this; 7030 } 7031 7032 /** 7033 * @return {@link #performer} The actual object that is the target of the 7034 * reference. The reference library doesn't populate this, but you can 7035 * use it to hold the resource if you resolve it. (The organization or 7036 * lab that should be responsible for this result.) 7037 */ 7038 public Organization getPerformerTarget() { 7039 if (this.performerTarget == null) 7040 if (Configuration.errorOnAutoCreate()) 7041 throw new Error("Attempt to auto-create MolecularSequence.performer"); 7042 else if (Configuration.doAutoCreate()) 7043 this.performerTarget = new Organization(); // aa 7044 return this.performerTarget; 7045 } 7046 7047 /** 7048 * @param value {@link #performer} The actual object that is the target of the 7049 * reference. The reference library doesn't use these, but you can 7050 * use it to hold the resource if you resolve it. (The organization 7051 * or lab that should be responsible for this result.) 7052 */ 7053 public MolecularSequence setPerformerTarget(Organization value) { 7054 this.performerTarget = value; 7055 return this; 7056 } 7057 7058 /** 7059 * @return {@link #quantity} (The number of copies of the sequence of interest. 7060 * (RNASeq).) 7061 */ 7062 public Quantity getQuantity() { 7063 if (this.quantity == null) 7064 if (Configuration.errorOnAutoCreate()) 7065 throw new Error("Attempt to auto-create MolecularSequence.quantity"); 7066 else if (Configuration.doAutoCreate()) 7067 this.quantity = new Quantity(); // cc 7068 return this.quantity; 7069 } 7070 7071 public boolean hasQuantity() { 7072 return this.quantity != null && !this.quantity.isEmpty(); 7073 } 7074 7075 /** 7076 * @param value {@link #quantity} (The number of copies of the sequence of 7077 * interest. (RNASeq).) 7078 */ 7079 public MolecularSequence setQuantity(Quantity value) { 7080 this.quantity = value; 7081 return this; 7082 } 7083 7084 /** 7085 * @return {@link #referenceSeq} (A sequence that is used as a reference to 7086 * describe variants that are present in a sequence analyzed.) 7087 */ 7088 public MolecularSequenceReferenceSeqComponent getReferenceSeq() { 7089 if (this.referenceSeq == null) 7090 if (Configuration.errorOnAutoCreate()) 7091 throw new Error("Attempt to auto-create MolecularSequence.referenceSeq"); 7092 else if (Configuration.doAutoCreate()) 7093 this.referenceSeq = new MolecularSequenceReferenceSeqComponent(); // cc 7094 return this.referenceSeq; 7095 } 7096 7097 public boolean hasReferenceSeq() { 7098 return this.referenceSeq != null && !this.referenceSeq.isEmpty(); 7099 } 7100 7101 /** 7102 * @param value {@link #referenceSeq} (A sequence that is used as a reference to 7103 * describe variants that are present in a sequence analyzed.) 7104 */ 7105 public MolecularSequence setReferenceSeq(MolecularSequenceReferenceSeqComponent value) { 7106 this.referenceSeq = value; 7107 return this; 7108 } 7109 7110 /** 7111 * @return {@link #variant} (The definition of variant here originates from 7112 * Sequence ontology 7113 * ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). 7114 * This element can represent amino acid or nucleic sequence 7115 * change(including insertion,deletion,SNP,etc.) It can represent some 7116 * complex mutation or segment variation with the assist of CIGAR 7117 * string.) 7118 */ 7119 public List<MolecularSequenceVariantComponent> getVariant() { 7120 if (this.variant == null) 7121 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 7122 return this.variant; 7123 } 7124 7125 /** 7126 * @return Returns a reference to <code>this</code> for easy method chaining 7127 */ 7128 public MolecularSequence setVariant(List<MolecularSequenceVariantComponent> theVariant) { 7129 this.variant = theVariant; 7130 return this; 7131 } 7132 7133 public boolean hasVariant() { 7134 if (this.variant == null) 7135 return false; 7136 for (MolecularSequenceVariantComponent item : this.variant) 7137 if (!item.isEmpty()) 7138 return true; 7139 return false; 7140 } 7141 7142 public MolecularSequenceVariantComponent addVariant() { // 3 7143 MolecularSequenceVariantComponent t = new MolecularSequenceVariantComponent(); 7144 if (this.variant == null) 7145 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 7146 this.variant.add(t); 7147 return t; 7148 } 7149 7150 public MolecularSequence addVariant(MolecularSequenceVariantComponent t) { // 3 7151 if (t == null) 7152 return this; 7153 if (this.variant == null) 7154 this.variant = new ArrayList<MolecularSequenceVariantComponent>(); 7155 this.variant.add(t); 7156 return this; 7157 } 7158 7159 /** 7160 * @return The first repetition of repeating field {@link #variant}, creating it 7161 * if it does not already exist 7162 */ 7163 public MolecularSequenceVariantComponent getVariantFirstRep() { 7164 if (getVariant().isEmpty()) { 7165 addVariant(); 7166 } 7167 return getVariant().get(0); 7168 } 7169 7170 /** 7171 * @return {@link #observedSeq} (Sequence that was observed. It is the result 7172 * marked by referenceSeq along with variant records on referenceSeq. 7173 * This shall start from referenceSeq.windowStart and end by 7174 * referenceSeq.windowEnd.). This is the underlying object with id, 7175 * value and extensions. The accessor "getObservedSeq" gives direct 7176 * access to the value 7177 */ 7178 public StringType getObservedSeqElement() { 7179 if (this.observedSeq == null) 7180 if (Configuration.errorOnAutoCreate()) 7181 throw new Error("Attempt to auto-create MolecularSequence.observedSeq"); 7182 else if (Configuration.doAutoCreate()) 7183 this.observedSeq = new StringType(); // bb 7184 return this.observedSeq; 7185 } 7186 7187 public boolean hasObservedSeqElement() { 7188 return this.observedSeq != null && !this.observedSeq.isEmpty(); 7189 } 7190 7191 public boolean hasObservedSeq() { 7192 return this.observedSeq != null && !this.observedSeq.isEmpty(); 7193 } 7194 7195 /** 7196 * @param value {@link #observedSeq} (Sequence that was observed. It is the 7197 * result marked by referenceSeq along with variant records on 7198 * referenceSeq. This shall start from referenceSeq.windowStart and 7199 * end by referenceSeq.windowEnd.). This is the underlying object 7200 * with id, value and extensions. The accessor "getObservedSeq" 7201 * gives direct access to the value 7202 */ 7203 public MolecularSequence setObservedSeqElement(StringType value) { 7204 this.observedSeq = value; 7205 return this; 7206 } 7207 7208 /** 7209 * @return Sequence that was observed. It is the result marked by referenceSeq 7210 * along with variant records on referenceSeq. This shall start from 7211 * referenceSeq.windowStart and end by referenceSeq.windowEnd. 7212 */ 7213 public String getObservedSeq() { 7214 return this.observedSeq == null ? null : this.observedSeq.getValue(); 7215 } 7216 7217 /** 7218 * @param value Sequence that was observed. It is the result marked by 7219 * referenceSeq along with variant records on referenceSeq. This 7220 * shall start from referenceSeq.windowStart and end by 7221 * referenceSeq.windowEnd. 7222 */ 7223 public MolecularSequence setObservedSeq(String value) { 7224 if (Utilities.noString(value)) 7225 this.observedSeq = null; 7226 else { 7227 if (this.observedSeq == null) 7228 this.observedSeq = new StringType(); 7229 this.observedSeq.setValue(value); 7230 } 7231 return this; 7232 } 7233 7234 /** 7235 * @return {@link #quality} (An experimental feature attribute that defines the 7236 * quality of the feature in a quantitative way, such as a phred quality 7237 * score 7238 * ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).) 7239 */ 7240 public List<MolecularSequenceQualityComponent> getQuality() { 7241 if (this.quality == null) 7242 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7243 return this.quality; 7244 } 7245 7246 /** 7247 * @return Returns a reference to <code>this</code> for easy method chaining 7248 */ 7249 public MolecularSequence setQuality(List<MolecularSequenceQualityComponent> theQuality) { 7250 this.quality = theQuality; 7251 return this; 7252 } 7253 7254 public boolean hasQuality() { 7255 if (this.quality == null) 7256 return false; 7257 for (MolecularSequenceQualityComponent item : this.quality) 7258 if (!item.isEmpty()) 7259 return true; 7260 return false; 7261 } 7262 7263 public MolecularSequenceQualityComponent addQuality() { // 3 7264 MolecularSequenceQualityComponent t = new MolecularSequenceQualityComponent(); 7265 if (this.quality == null) 7266 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7267 this.quality.add(t); 7268 return t; 7269 } 7270 7271 public MolecularSequence addQuality(MolecularSequenceQualityComponent t) { // 3 7272 if (t == null) 7273 return this; 7274 if (this.quality == null) 7275 this.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7276 this.quality.add(t); 7277 return this; 7278 } 7279 7280 /** 7281 * @return The first repetition of repeating field {@link #quality}, creating it 7282 * if it does not already exist 7283 */ 7284 public MolecularSequenceQualityComponent getQualityFirstRep() { 7285 if (getQuality().isEmpty()) { 7286 addQuality(); 7287 } 7288 return getQuality().get(0); 7289 } 7290 7291 /** 7292 * @return {@link #readCoverage} (Coverage (read depth or depth) is the average 7293 * number of reads representing a given nucleotide in the reconstructed 7294 * sequence.). This is the underlying object with id, value and 7295 * extensions. The accessor "getReadCoverage" gives direct access to the 7296 * value 7297 */ 7298 public IntegerType getReadCoverageElement() { 7299 if (this.readCoverage == null) 7300 if (Configuration.errorOnAutoCreate()) 7301 throw new Error("Attempt to auto-create MolecularSequence.readCoverage"); 7302 else if (Configuration.doAutoCreate()) 7303 this.readCoverage = new IntegerType(); // bb 7304 return this.readCoverage; 7305 } 7306 7307 public boolean hasReadCoverageElement() { 7308 return this.readCoverage != null && !this.readCoverage.isEmpty(); 7309 } 7310 7311 public boolean hasReadCoverage() { 7312 return this.readCoverage != null && !this.readCoverage.isEmpty(); 7313 } 7314 7315 /** 7316 * @param value {@link #readCoverage} (Coverage (read depth or depth) is the 7317 * average number of reads representing a given nucleotide in the 7318 * reconstructed sequence.). This is the underlying object with id, 7319 * value and extensions. The accessor "getReadCoverage" gives 7320 * direct access to the value 7321 */ 7322 public MolecularSequence setReadCoverageElement(IntegerType value) { 7323 this.readCoverage = value; 7324 return this; 7325 } 7326 7327 /** 7328 * @return Coverage (read depth or depth) is the average number of reads 7329 * representing a given nucleotide in the reconstructed sequence. 7330 */ 7331 public int getReadCoverage() { 7332 return this.readCoverage == null || this.readCoverage.isEmpty() ? 0 : this.readCoverage.getValue(); 7333 } 7334 7335 /** 7336 * @param value Coverage (read depth or depth) is the average number of reads 7337 * representing a given nucleotide in the reconstructed sequence. 7338 */ 7339 public MolecularSequence setReadCoverage(int value) { 7340 if (this.readCoverage == null) 7341 this.readCoverage = new IntegerType(); 7342 this.readCoverage.setValue(value); 7343 return this; 7344 } 7345 7346 /** 7347 * @return {@link #repository} (Configurations of the external repository. The 7348 * repository shall store target's observedSeq or records related with 7349 * target's observedSeq.) 7350 */ 7351 public List<MolecularSequenceRepositoryComponent> getRepository() { 7352 if (this.repository == null) 7353 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7354 return this.repository; 7355 } 7356 7357 /** 7358 * @return Returns a reference to <code>this</code> for easy method chaining 7359 */ 7360 public MolecularSequence setRepository(List<MolecularSequenceRepositoryComponent> theRepository) { 7361 this.repository = theRepository; 7362 return this; 7363 } 7364 7365 public boolean hasRepository() { 7366 if (this.repository == null) 7367 return false; 7368 for (MolecularSequenceRepositoryComponent item : this.repository) 7369 if (!item.isEmpty()) 7370 return true; 7371 return false; 7372 } 7373 7374 public MolecularSequenceRepositoryComponent addRepository() { // 3 7375 MolecularSequenceRepositoryComponent t = new MolecularSequenceRepositoryComponent(); 7376 if (this.repository == null) 7377 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7378 this.repository.add(t); 7379 return t; 7380 } 7381 7382 public MolecularSequence addRepository(MolecularSequenceRepositoryComponent t) { // 3 7383 if (t == null) 7384 return this; 7385 if (this.repository == null) 7386 this.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7387 this.repository.add(t); 7388 return this; 7389 } 7390 7391 /** 7392 * @return The first repetition of repeating field {@link #repository}, creating 7393 * it if it does not already exist 7394 */ 7395 public MolecularSequenceRepositoryComponent getRepositoryFirstRep() { 7396 if (getRepository().isEmpty()) { 7397 addRepository(); 7398 } 7399 return getRepository().get(0); 7400 } 7401 7402 /** 7403 * @return {@link #pointer} (Pointer to next atomic sequence which at most 7404 * contains one variant.) 7405 */ 7406 public List<Reference> getPointer() { 7407 if (this.pointer == null) 7408 this.pointer = new ArrayList<Reference>(); 7409 return this.pointer; 7410 } 7411 7412 /** 7413 * @return Returns a reference to <code>this</code> for easy method chaining 7414 */ 7415 public MolecularSequence setPointer(List<Reference> thePointer) { 7416 this.pointer = thePointer; 7417 return this; 7418 } 7419 7420 public boolean hasPointer() { 7421 if (this.pointer == null) 7422 return false; 7423 for (Reference item : this.pointer) 7424 if (!item.isEmpty()) 7425 return true; 7426 return false; 7427 } 7428 7429 public Reference addPointer() { // 3 7430 Reference t = new Reference(); 7431 if (this.pointer == null) 7432 this.pointer = new ArrayList<Reference>(); 7433 this.pointer.add(t); 7434 return t; 7435 } 7436 7437 public MolecularSequence addPointer(Reference t) { // 3 7438 if (t == null) 7439 return this; 7440 if (this.pointer == null) 7441 this.pointer = new ArrayList<Reference>(); 7442 this.pointer.add(t); 7443 return this; 7444 } 7445 7446 /** 7447 * @return The first repetition of repeating field {@link #pointer}, creating it 7448 * if it does not already exist 7449 */ 7450 public Reference getPointerFirstRep() { 7451 if (getPointer().isEmpty()) { 7452 addPointer(); 7453 } 7454 return getPointer().get(0); 7455 } 7456 7457 /** 7458 * @deprecated Use Reference#setResource(IBaseResource) instead 7459 */ 7460 @Deprecated 7461 public List<MolecularSequence> getPointerTarget() { 7462 if (this.pointerTarget == null) 7463 this.pointerTarget = new ArrayList<MolecularSequence>(); 7464 return this.pointerTarget; 7465 } 7466 7467 /** 7468 * @deprecated Use Reference#setResource(IBaseResource) instead 7469 */ 7470 @Deprecated 7471 public MolecularSequence addPointerTarget() { 7472 MolecularSequence r = new MolecularSequence(); 7473 if (this.pointerTarget == null) 7474 this.pointerTarget = new ArrayList<MolecularSequence>(); 7475 this.pointerTarget.add(r); 7476 return r; 7477 } 7478 7479 /** 7480 * @return {@link #structureVariant} (Information about chromosome structure 7481 * variation.) 7482 */ 7483 public List<MolecularSequenceStructureVariantComponent> getStructureVariant() { 7484 if (this.structureVariant == null) 7485 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7486 return this.structureVariant; 7487 } 7488 7489 /** 7490 * @return Returns a reference to <code>this</code> for easy method chaining 7491 */ 7492 public MolecularSequence setStructureVariant(List<MolecularSequenceStructureVariantComponent> theStructureVariant) { 7493 this.structureVariant = theStructureVariant; 7494 return this; 7495 } 7496 7497 public boolean hasStructureVariant() { 7498 if (this.structureVariant == null) 7499 return false; 7500 for (MolecularSequenceStructureVariantComponent item : this.structureVariant) 7501 if (!item.isEmpty()) 7502 return true; 7503 return false; 7504 } 7505 7506 public MolecularSequenceStructureVariantComponent addStructureVariant() { // 3 7507 MolecularSequenceStructureVariantComponent t = new MolecularSequenceStructureVariantComponent(); 7508 if (this.structureVariant == null) 7509 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7510 this.structureVariant.add(t); 7511 return t; 7512 } 7513 7514 public MolecularSequence addStructureVariant(MolecularSequenceStructureVariantComponent t) { // 3 7515 if (t == null) 7516 return this; 7517 if (this.structureVariant == null) 7518 this.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 7519 this.structureVariant.add(t); 7520 return this; 7521 } 7522 7523 /** 7524 * @return The first repetition of repeating field {@link #structureVariant}, 7525 * creating it if it does not already exist 7526 */ 7527 public MolecularSequenceStructureVariantComponent getStructureVariantFirstRep() { 7528 if (getStructureVariant().isEmpty()) { 7529 addStructureVariant(); 7530 } 7531 return getStructureVariant().get(0); 7532 } 7533 7534 protected void listChildren(List<Property> children) { 7535 super.listChildren(children); 7536 children.add(new Property("identifier", "Identifier", 7537 "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, 7538 java.lang.Integer.MAX_VALUE, identifier)); 7539 children.add(new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type)); 7540 children.add(new Property("coordinateSystem", "integer", 7541 "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 7542 0, 1, coordinateSystem)); 7543 children.add(new Property("patient", "Reference(Patient)", 7544 "The patient whose sequencing results are described by this resource.", 0, 1, patient)); 7545 children.add(new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, specimen)); 7546 children.add(new Property("device", "Reference(Device)", 7547 "The method for sequencing, for example, chip information.", 0, 1, device)); 7548 children.add(new Property("performer", "Reference(Organization)", 7549 "The organization or lab that should be responsible for this result.", 0, 1, performer)); 7550 children.add(new Property("quantity", "Quantity", "The number of copies of the sequence of interest. (RNASeq).", 0, 7551 1, quantity)); 7552 children.add(new Property("referenceSeq", "", 7553 "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, 7554 referenceSeq)); 7555 children.add(new Property("variant", "", 7556 "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 7557 0, java.lang.Integer.MAX_VALUE, variant)); 7558 children.add(new Property("observedSeq", "string", 7559 "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 7560 0, 1, observedSeq)); 7561 children.add(new Property("quality", "", 7562 "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 7563 0, java.lang.Integer.MAX_VALUE, quality)); 7564 children.add(new Property("readCoverage", "integer", 7565 "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 7566 0, 1, readCoverage)); 7567 children.add(new Property("repository", "", 7568 "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 7569 0, java.lang.Integer.MAX_VALUE, repository)); 7570 children.add(new Property("pointer", "Reference(MolecularSequence)", 7571 "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, 7572 pointer)); 7573 children.add(new Property("structureVariant", "", "Information about chromosome structure variation.", 0, 7574 java.lang.Integer.MAX_VALUE, structureVariant)); 7575 } 7576 7577 @Override 7578 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7579 switch (_hash) { 7580 case -1618432855: 7581 /* identifier */ return new Property("identifier", "Identifier", 7582 "A unique identifier for this particular sequence instance. This is a FHIR-defined id.", 0, 7583 java.lang.Integer.MAX_VALUE, identifier); 7584 case 3575610: 7585 /* type */ return new Property("type", "code", "Amino Acid Sequence/ DNA Sequence / RNA Sequence.", 0, 1, type); 7586 case 354212295: 7587 /* coordinateSystem */ return new Property("coordinateSystem", "integer", 7588 "Whether the sequence is numbered starting at 0 (0-based numbering or coordinates, inclusive start, exclusive end) or starting at 1 (1-based numbering, inclusive start and inclusive end).", 7589 0, 1, coordinateSystem); 7590 case -791418107: 7591 /* patient */ return new Property("patient", "Reference(Patient)", 7592 "The patient whose sequencing results are described by this resource.", 0, 1, patient); 7593 case -2132868344: 7594 /* specimen */ return new Property("specimen", "Reference(Specimen)", "Specimen used for sequencing.", 0, 1, 7595 specimen); 7596 case -1335157162: 7597 /* device */ return new Property("device", "Reference(Device)", 7598 "The method for sequencing, for example, chip information.", 0, 1, device); 7599 case 481140686: 7600 /* performer */ return new Property("performer", "Reference(Organization)", 7601 "The organization or lab that should be responsible for this result.", 0, 1, performer); 7602 case -1285004149: 7603 /* quantity */ return new Property("quantity", "Quantity", 7604 "The number of copies of the sequence of interest. (RNASeq).", 0, 1, quantity); 7605 case -502547180: 7606 /* referenceSeq */ return new Property("referenceSeq", "", 7607 "A sequence that is used as a reference to describe variants that are present in a sequence analyzed.", 0, 1, 7608 referenceSeq); 7609 case 236785797: 7610 /* variant */ return new Property("variant", "", 7611 "The definition of variant here originates from Sequence ontology ([variant_of](http://www.sequenceontology.org/browser/current_svn/term/variant_of)). This element can represent amino acid or nucleic sequence change(including insertion,deletion,SNP,etc.) It can represent some complex mutation or segment variation with the assist of CIGAR string.", 7612 0, java.lang.Integer.MAX_VALUE, variant); 7613 case 125541495: 7614 /* observedSeq */ return new Property("observedSeq", "string", 7615 "Sequence that was observed. It is the result marked by referenceSeq along with variant records on referenceSeq. This shall start from referenceSeq.windowStart and end by referenceSeq.windowEnd.", 7616 0, 1, observedSeq); 7617 case 651215103: 7618 /* quality */ return new Property("quality", "", 7619 "An experimental feature attribute that defines the quality of the feature in a quantitative way, such as a phred quality score ([SO:0001686](http://www.sequenceontology.org/browser/current_svn/term/SO:0001686)).", 7620 0, java.lang.Integer.MAX_VALUE, quality); 7621 case -1798816354: 7622 /* readCoverage */ return new Property("readCoverage", "integer", 7623 "Coverage (read depth or depth) is the average number of reads representing a given nucleotide in the reconstructed sequence.", 7624 0, 1, readCoverage); 7625 case 1950800714: 7626 /* repository */ return new Property("repository", "", 7627 "Configurations of the external repository. The repository shall store target's observedSeq or records related with target's observedSeq.", 7628 0, java.lang.Integer.MAX_VALUE, repository); 7629 case -400605635: 7630 /* pointer */ return new Property("pointer", "Reference(MolecularSequence)", 7631 "Pointer to next atomic sequence which at most contains one variant.", 0, java.lang.Integer.MAX_VALUE, 7632 pointer); 7633 case 757269394: 7634 /* structureVariant */ return new Property("structureVariant", "", 7635 "Information about chromosome structure variation.", 0, java.lang.Integer.MAX_VALUE, structureVariant); 7636 default: 7637 return super.getNamedProperty(_hash, _name, _checkValid); 7638 } 7639 7640 } 7641 7642 @Override 7643 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7644 switch (hash) { 7645 case -1618432855: 7646 /* identifier */ return this.identifier == null ? new Base[0] 7647 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 7648 case 3575610: 7649 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<SequenceType> 7650 case 354212295: 7651 /* coordinateSystem */ return this.coordinateSystem == null ? new Base[0] : new Base[] { this.coordinateSystem }; // IntegerType 7652 case -791418107: 7653 /* patient */ return this.patient == null ? new Base[0] : new Base[] { this.patient }; // Reference 7654 case -2132868344: 7655 /* specimen */ return this.specimen == null ? new Base[0] : new Base[] { this.specimen }; // Reference 7656 case -1335157162: 7657 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 7658 case 481140686: 7659 /* performer */ return this.performer == null ? new Base[0] : new Base[] { this.performer }; // Reference 7660 case -1285004149: 7661 /* quantity */ return this.quantity == null ? new Base[0] : new Base[] { this.quantity }; // Quantity 7662 case -502547180: 7663 /* referenceSeq */ return this.referenceSeq == null ? new Base[0] : new Base[] { this.referenceSeq }; // MolecularSequenceReferenceSeqComponent 7664 case 236785797: 7665 /* variant */ return this.variant == null ? new Base[0] : this.variant.toArray(new Base[this.variant.size()]); // MolecularSequenceVariantComponent 7666 case 125541495: 7667 /* observedSeq */ return this.observedSeq == null ? new Base[0] : new Base[] { this.observedSeq }; // StringType 7668 case 651215103: 7669 /* quality */ return this.quality == null ? new Base[0] : this.quality.toArray(new Base[this.quality.size()]); // MolecularSequenceQualityComponent 7670 case -1798816354: 7671 /* readCoverage */ return this.readCoverage == null ? new Base[0] : new Base[] { this.readCoverage }; // IntegerType 7672 case 1950800714: 7673 /* repository */ return this.repository == null ? new Base[0] 7674 : this.repository.toArray(new Base[this.repository.size()]); // MolecularSequenceRepositoryComponent 7675 case -400605635: 7676 /* pointer */ return this.pointer == null ? new Base[0] : this.pointer.toArray(new Base[this.pointer.size()]); // Reference 7677 case 757269394: 7678 /* structureVariant */ return this.structureVariant == null ? new Base[0] 7679 : this.structureVariant.toArray(new Base[this.structureVariant.size()]); // MolecularSequenceStructureVariantComponent 7680 default: 7681 return super.getProperty(hash, name, checkValid); 7682 } 7683 7684 } 7685 7686 @Override 7687 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7688 switch (hash) { 7689 case -1618432855: // identifier 7690 this.getIdentifier().add(castToIdentifier(value)); // Identifier 7691 return value; 7692 case 3575610: // type 7693 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 7694 this.type = (Enumeration) value; // Enumeration<SequenceType> 7695 return value; 7696 case 354212295: // coordinateSystem 7697 this.coordinateSystem = castToInteger(value); // IntegerType 7698 return value; 7699 case -791418107: // patient 7700 this.patient = castToReference(value); // Reference 7701 return value; 7702 case -2132868344: // specimen 7703 this.specimen = castToReference(value); // Reference 7704 return value; 7705 case -1335157162: // device 7706 this.device = castToReference(value); // Reference 7707 return value; 7708 case 481140686: // performer 7709 this.performer = castToReference(value); // Reference 7710 return value; 7711 case -1285004149: // quantity 7712 this.quantity = castToQuantity(value); // Quantity 7713 return value; 7714 case -502547180: // referenceSeq 7715 this.referenceSeq = (MolecularSequenceReferenceSeqComponent) value; // MolecularSequenceReferenceSeqComponent 7716 return value; 7717 case 236785797: // variant 7718 this.getVariant().add((MolecularSequenceVariantComponent) value); // MolecularSequenceVariantComponent 7719 return value; 7720 case 125541495: // observedSeq 7721 this.observedSeq = castToString(value); // StringType 7722 return value; 7723 case 651215103: // quality 7724 this.getQuality().add((MolecularSequenceQualityComponent) value); // MolecularSequenceQualityComponent 7725 return value; 7726 case -1798816354: // readCoverage 7727 this.readCoverage = castToInteger(value); // IntegerType 7728 return value; 7729 case 1950800714: // repository 7730 this.getRepository().add((MolecularSequenceRepositoryComponent) value); // MolecularSequenceRepositoryComponent 7731 return value; 7732 case -400605635: // pointer 7733 this.getPointer().add(castToReference(value)); // Reference 7734 return value; 7735 case 757269394: // structureVariant 7736 this.getStructureVariant().add((MolecularSequenceStructureVariantComponent) value); // MolecularSequenceStructureVariantComponent 7737 return value; 7738 default: 7739 return super.setProperty(hash, name, value); 7740 } 7741 7742 } 7743 7744 @Override 7745 public Base setProperty(String name, Base value) throws FHIRException { 7746 if (name.equals("identifier")) { 7747 this.getIdentifier().add(castToIdentifier(value)); 7748 } else if (name.equals("type")) { 7749 value = new SequenceTypeEnumFactory().fromType(castToCode(value)); 7750 this.type = (Enumeration) value; // Enumeration<SequenceType> 7751 } else if (name.equals("coordinateSystem")) { 7752 this.coordinateSystem = castToInteger(value); // IntegerType 7753 } else if (name.equals("patient")) { 7754 this.patient = castToReference(value); // Reference 7755 } else if (name.equals("specimen")) { 7756 this.specimen = castToReference(value); // Reference 7757 } else if (name.equals("device")) { 7758 this.device = castToReference(value); // Reference 7759 } else if (name.equals("performer")) { 7760 this.performer = castToReference(value); // Reference 7761 } else if (name.equals("quantity")) { 7762 this.quantity = castToQuantity(value); // Quantity 7763 } else if (name.equals("referenceSeq")) { 7764 this.referenceSeq = (MolecularSequenceReferenceSeqComponent) value; // MolecularSequenceReferenceSeqComponent 7765 } else if (name.equals("variant")) { 7766 this.getVariant().add((MolecularSequenceVariantComponent) value); 7767 } else if (name.equals("observedSeq")) { 7768 this.observedSeq = castToString(value); // StringType 7769 } else if (name.equals("quality")) { 7770 this.getQuality().add((MolecularSequenceQualityComponent) value); 7771 } else if (name.equals("readCoverage")) { 7772 this.readCoverage = castToInteger(value); // IntegerType 7773 } else if (name.equals("repository")) { 7774 this.getRepository().add((MolecularSequenceRepositoryComponent) value); 7775 } else if (name.equals("pointer")) { 7776 this.getPointer().add(castToReference(value)); 7777 } else if (name.equals("structureVariant")) { 7778 this.getStructureVariant().add((MolecularSequenceStructureVariantComponent) value); 7779 } else 7780 return super.setProperty(name, value); 7781 return value; 7782 } 7783 7784 @Override 7785 public void removeChild(String name, Base value) throws FHIRException { 7786 if (name.equals("identifier")) { 7787 this.getIdentifier().remove(castToIdentifier(value)); 7788 } else if (name.equals("type")) { 7789 this.type = null; 7790 } else if (name.equals("coordinateSystem")) { 7791 this.coordinateSystem = null; 7792 } else if (name.equals("patient")) { 7793 this.patient = null; 7794 } else if (name.equals("specimen")) { 7795 this.specimen = null; 7796 } else if (name.equals("device")) { 7797 this.device = null; 7798 } else if (name.equals("performer")) { 7799 this.performer = null; 7800 } else if (name.equals("quantity")) { 7801 this.quantity = null; 7802 } else if (name.equals("referenceSeq")) { 7803 this.referenceSeq = (MolecularSequenceReferenceSeqComponent) value; // MolecularSequenceReferenceSeqComponent 7804 } else if (name.equals("variant")) { 7805 this.getVariant().remove((MolecularSequenceVariantComponent) value); 7806 } else if (name.equals("observedSeq")) { 7807 this.observedSeq = null; 7808 } else if (name.equals("quality")) { 7809 this.getQuality().remove((MolecularSequenceQualityComponent) value); 7810 } else if (name.equals("readCoverage")) { 7811 this.readCoverage = null; 7812 } else if (name.equals("repository")) { 7813 this.getRepository().remove((MolecularSequenceRepositoryComponent) value); 7814 } else if (name.equals("pointer")) { 7815 this.getPointer().remove(castToReference(value)); 7816 } else if (name.equals("structureVariant")) { 7817 this.getStructureVariant().remove((MolecularSequenceStructureVariantComponent) value); 7818 } else 7819 super.removeChild(name, value); 7820 7821 } 7822 7823 @Override 7824 public Base makeProperty(int hash, String name) throws FHIRException { 7825 switch (hash) { 7826 case -1618432855: 7827 return addIdentifier(); 7828 case 3575610: 7829 return getTypeElement(); 7830 case 354212295: 7831 return getCoordinateSystemElement(); 7832 case -791418107: 7833 return getPatient(); 7834 case -2132868344: 7835 return getSpecimen(); 7836 case -1335157162: 7837 return getDevice(); 7838 case 481140686: 7839 return getPerformer(); 7840 case -1285004149: 7841 return getQuantity(); 7842 case -502547180: 7843 return getReferenceSeq(); 7844 case 236785797: 7845 return addVariant(); 7846 case 125541495: 7847 return getObservedSeqElement(); 7848 case 651215103: 7849 return addQuality(); 7850 case -1798816354: 7851 return getReadCoverageElement(); 7852 case 1950800714: 7853 return addRepository(); 7854 case -400605635: 7855 return addPointer(); 7856 case 757269394: 7857 return addStructureVariant(); 7858 default: 7859 return super.makeProperty(hash, name); 7860 } 7861 7862 } 7863 7864 @Override 7865 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7866 switch (hash) { 7867 case -1618432855: 7868 /* identifier */ return new String[] { "Identifier" }; 7869 case 3575610: 7870 /* type */ return new String[] { "code" }; 7871 case 354212295: 7872 /* coordinateSystem */ return new String[] { "integer" }; 7873 case -791418107: 7874 /* patient */ return new String[] { "Reference" }; 7875 case -2132868344: 7876 /* specimen */ return new String[] { "Reference" }; 7877 case -1335157162: 7878 /* device */ return new String[] { "Reference" }; 7879 case 481140686: 7880 /* performer */ return new String[] { "Reference" }; 7881 case -1285004149: 7882 /* quantity */ return new String[] { "Quantity" }; 7883 case -502547180: 7884 /* referenceSeq */ return new String[] {}; 7885 case 236785797: 7886 /* variant */ return new String[] {}; 7887 case 125541495: 7888 /* observedSeq */ return new String[] { "string" }; 7889 case 651215103: 7890 /* quality */ return new String[] {}; 7891 case -1798816354: 7892 /* readCoverage */ return new String[] { "integer" }; 7893 case 1950800714: 7894 /* repository */ return new String[] {}; 7895 case -400605635: 7896 /* pointer */ return new String[] { "Reference" }; 7897 case 757269394: 7898 /* structureVariant */ return new String[] {}; 7899 default: 7900 return super.getTypesForProperty(hash, name); 7901 } 7902 7903 } 7904 7905 @Override 7906 public Base addChild(String name) throws FHIRException { 7907 if (name.equals("identifier")) { 7908 return addIdentifier(); 7909 } else if (name.equals("type")) { 7910 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.type"); 7911 } else if (name.equals("coordinateSystem")) { 7912 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.coordinateSystem"); 7913 } else if (name.equals("patient")) { 7914 this.patient = new Reference(); 7915 return this.patient; 7916 } else if (name.equals("specimen")) { 7917 this.specimen = new Reference(); 7918 return this.specimen; 7919 } else if (name.equals("device")) { 7920 this.device = new Reference(); 7921 return this.device; 7922 } else if (name.equals("performer")) { 7923 this.performer = new Reference(); 7924 return this.performer; 7925 } else if (name.equals("quantity")) { 7926 this.quantity = new Quantity(); 7927 return this.quantity; 7928 } else if (name.equals("referenceSeq")) { 7929 this.referenceSeq = new MolecularSequenceReferenceSeqComponent(); 7930 return this.referenceSeq; 7931 } else if (name.equals("variant")) { 7932 return addVariant(); 7933 } else if (name.equals("observedSeq")) { 7934 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.observedSeq"); 7935 } else if (name.equals("quality")) { 7936 return addQuality(); 7937 } else if (name.equals("readCoverage")) { 7938 throw new FHIRException("Cannot call addChild on a singleton property MolecularSequence.readCoverage"); 7939 } else if (name.equals("repository")) { 7940 return addRepository(); 7941 } else if (name.equals("pointer")) { 7942 return addPointer(); 7943 } else if (name.equals("structureVariant")) { 7944 return addStructureVariant(); 7945 } else 7946 return super.addChild(name); 7947 } 7948 7949 public String fhirType() { 7950 return "MolecularSequence"; 7951 7952 } 7953 7954 public MolecularSequence copy() { 7955 MolecularSequence dst = new MolecularSequence(); 7956 copyValues(dst); 7957 return dst; 7958 } 7959 7960 public void copyValues(MolecularSequence dst) { 7961 super.copyValues(dst); 7962 if (identifier != null) { 7963 dst.identifier = new ArrayList<Identifier>(); 7964 for (Identifier i : identifier) 7965 dst.identifier.add(i.copy()); 7966 } 7967 ; 7968 dst.type = type == null ? null : type.copy(); 7969 dst.coordinateSystem = coordinateSystem == null ? null : coordinateSystem.copy(); 7970 dst.patient = patient == null ? null : patient.copy(); 7971 dst.specimen = specimen == null ? null : specimen.copy(); 7972 dst.device = device == null ? null : device.copy(); 7973 dst.performer = performer == null ? null : performer.copy(); 7974 dst.quantity = quantity == null ? null : quantity.copy(); 7975 dst.referenceSeq = referenceSeq == null ? null : referenceSeq.copy(); 7976 if (variant != null) { 7977 dst.variant = new ArrayList<MolecularSequenceVariantComponent>(); 7978 for (MolecularSequenceVariantComponent i : variant) 7979 dst.variant.add(i.copy()); 7980 } 7981 ; 7982 dst.observedSeq = observedSeq == null ? null : observedSeq.copy(); 7983 if (quality != null) { 7984 dst.quality = new ArrayList<MolecularSequenceQualityComponent>(); 7985 for (MolecularSequenceQualityComponent i : quality) 7986 dst.quality.add(i.copy()); 7987 } 7988 ; 7989 dst.readCoverage = readCoverage == null ? null : readCoverage.copy(); 7990 if (repository != null) { 7991 dst.repository = new ArrayList<MolecularSequenceRepositoryComponent>(); 7992 for (MolecularSequenceRepositoryComponent i : repository) 7993 dst.repository.add(i.copy()); 7994 } 7995 ; 7996 if (pointer != null) { 7997 dst.pointer = new ArrayList<Reference>(); 7998 for (Reference i : pointer) 7999 dst.pointer.add(i.copy()); 8000 } 8001 ; 8002 if (structureVariant != null) { 8003 dst.structureVariant = new ArrayList<MolecularSequenceStructureVariantComponent>(); 8004 for (MolecularSequenceStructureVariantComponent i : structureVariant) 8005 dst.structureVariant.add(i.copy()); 8006 } 8007 ; 8008 } 8009 8010 protected MolecularSequence typedCopy() { 8011 return copy(); 8012 } 8013 8014 @Override 8015 public boolean equalsDeep(Base other_) { 8016 if (!super.equalsDeep(other_)) 8017 return false; 8018 if (!(other_ instanceof MolecularSequence)) 8019 return false; 8020 MolecularSequence o = (MolecularSequence) other_; 8021 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) 8022 && compareDeep(coordinateSystem, o.coordinateSystem, true) && compareDeep(patient, o.patient, true) 8023 && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 8024 && compareDeep(performer, o.performer, true) && compareDeep(quantity, o.quantity, true) 8025 && compareDeep(referenceSeq, o.referenceSeq, true) && compareDeep(variant, o.variant, true) 8026 && compareDeep(observedSeq, o.observedSeq, true) && compareDeep(quality, o.quality, true) 8027 && compareDeep(readCoverage, o.readCoverage, true) && compareDeep(repository, o.repository, true) 8028 && compareDeep(pointer, o.pointer, true) && compareDeep(structureVariant, o.structureVariant, true); 8029 } 8030 8031 @Override 8032 public boolean equalsShallow(Base other_) { 8033 if (!super.equalsShallow(other_)) 8034 return false; 8035 if (!(other_ instanceof MolecularSequence)) 8036 return false; 8037 MolecularSequence o = (MolecularSequence) other_; 8038 return compareValues(type, o.type, true) && compareValues(coordinateSystem, o.coordinateSystem, true) 8039 && compareValues(observedSeq, o.observedSeq, true) && compareValues(readCoverage, o.readCoverage, true); 8040 } 8041 8042 public boolean isEmpty() { 8043 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coordinateSystem, patient, 8044 specimen, device, performer, quantity, referenceSeq, variant, observedSeq, quality, readCoverage, repository, 8045 pointer, structureVariant); 8046 } 8047 8048 @Override 8049 public ResourceType getResourceType() { 8050 return ResourceType.MolecularSequence; 8051 } 8052 8053 /** 8054 * Search parameter: <b>identifier</b> 8055 * <p> 8056 * Description: <b>The unique identity for a particular sequence</b><br> 8057 * Type: <b>token</b><br> 8058 * Path: <b>MolecularSequence.identifier</b><br> 8059 * </p> 8060 */ 8061 @SearchParamDefinition(name = "identifier", path = "MolecularSequence.identifier", description = "The unique identity for a particular sequence", type = "token") 8062 public static final String SP_IDENTIFIER = "identifier"; 8063 /** 8064 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 8065 * <p> 8066 * Description: <b>The unique identity for a particular sequence</b><br> 8067 * Type: <b>token</b><br> 8068 * Path: <b>MolecularSequence.identifier</b><br> 8069 * </p> 8070 */ 8071 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8072 SP_IDENTIFIER); 8073 8074 /** 8075 * Search parameter: <b>referenceseqid-variant-coordinate</b> 8076 * <p> 8077 * Description: <b>Search parameter by reference sequence and variant 8078 * coordinate. This will refer to part of a locus or part of a gene where search 8079 * region will be represented in 1-based system. Since the coordinateSystem can 8080 * either be 0-based or 1-based, this search query will include the result of 8081 * both coordinateSystem that contains the equivalent segment of the gene or 8082 * whole genome sequence. For example, a search for sequence can be represented 8083 * as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means 8084 * it will search for the MolecularSequence resource with variants on 8085 * NC_000001.11 and with position >123 and <345, where in 1-based system 8086 * resource, all strings within region NC_000001.11:124-344 will be revealed, 8087 * while in 0-based system resource, all strings within region 8088 * NC_000001.11:123-344 will be revealed. You may want to check detail about 8089 * 0-based v.s. 1-based above.</b><br> 8090 * Type: <b>composite</b><br> 8091 * Path: <b></b><br> 8092 * </p> 8093 */ 8094 @SearchParamDefinition(name = "referenceseqid-variant-coordinate", path = "MolecularSequence.variant", description = "Search parameter by reference sequence and variant coordinate. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means it will search for the MolecularSequence resource with variants on NC_000001.11 and with position >123 and <345, where in 1-based system resource, all strings within region NC_000001.11:124-344 will be revealed, while in 0-based system resource, all strings within region NC_000001.11:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8095 "referenceseqid", "variant-start" }) 8096 public static final String SP_REFERENCESEQID_VARIANT_COORDINATE = "referenceseqid-variant-coordinate"; 8097 /** 8098 * <b>Fluent Client</b> search parameter constant for 8099 * <b>referenceseqid-variant-coordinate</b> 8100 * <p> 8101 * Description: <b>Search parameter by reference sequence and variant 8102 * coordinate. This will refer to part of a locus or part of a gene where search 8103 * region will be represented in 1-based system. Since the coordinateSystem can 8104 * either be 0-based or 1-based, this search query will include the result of 8105 * both coordinateSystem that contains the equivalent segment of the gene or 8106 * whole genome sequence. For example, a search for sequence can be represented 8107 * as `referenceSeqId-variant-coordinate=NC_000001.11$lt345$gt123`, this means 8108 * it will search for the MolecularSequence resource with variants on 8109 * NC_000001.11 and with position >123 and <345, where in 1-based system 8110 * resource, all strings within region NC_000001.11:124-344 will be revealed, 8111 * while in 0-based system resource, all strings within region 8112 * NC_000001.11:123-344 will be revealed. You may want to check detail about 8113 * 0-based v.s. 1-based above.</b><br> 8114 * Type: <b>composite</b><br> 8115 * Path: <b></b><br> 8116 * </p> 8117 */ 8118 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> REFERENCESEQID_VARIANT_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8119 SP_REFERENCESEQID_VARIANT_COORDINATE); 8120 8121 /** 8122 * Search parameter: <b>chromosome</b> 8123 * <p> 8124 * Description: <b>Chromosome number of the reference sequence</b><br> 8125 * Type: <b>token</b><br> 8126 * Path: <b>MolecularSequence.referenceSeq.chromosome</b><br> 8127 * </p> 8128 */ 8129 @SearchParamDefinition(name = "chromosome", path = "MolecularSequence.referenceSeq.chromosome", description = "Chromosome number of the reference sequence", type = "token") 8130 public static final String SP_CHROMOSOME = "chromosome"; 8131 /** 8132 * <b>Fluent Client</b> search parameter constant for <b>chromosome</b> 8133 * <p> 8134 * Description: <b>Chromosome number of the reference sequence</b><br> 8135 * Type: <b>token</b><br> 8136 * Path: <b>MolecularSequence.referenceSeq.chromosome</b><br> 8137 * </p> 8138 */ 8139 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHROMOSOME = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8140 SP_CHROMOSOME); 8141 8142 /** 8143 * Search parameter: <b>window-end</b> 8144 * <p> 8145 * Description: <b>End position (0-based exclusive, which menas the acid at this 8146 * position will not be included, 1-based inclusive, which means the acid at 8147 * this position will be included) of the reference sequence.</b><br> 8148 * Type: <b>number</b><br> 8149 * Path: <b>MolecularSequence.referenceSeq.windowEnd</b><br> 8150 * </p> 8151 */ 8152 @SearchParamDefinition(name = "window-end", path = "MolecularSequence.referenceSeq.windowEnd", description = "End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the reference sequence.", type = "number") 8153 public static final String SP_WINDOW_END = "window-end"; 8154 /** 8155 * <b>Fluent Client</b> search parameter constant for <b>window-end</b> 8156 * <p> 8157 * Description: <b>End position (0-based exclusive, which menas the acid at this 8158 * position will not be included, 1-based inclusive, which means the acid at 8159 * this position will be included) of the reference sequence.</b><br> 8160 * Type: <b>number</b><br> 8161 * Path: <b>MolecularSequence.referenceSeq.windowEnd</b><br> 8162 * </p> 8163 */ 8164 public static final ca.uhn.fhir.rest.gclient.NumberClientParam WINDOW_END = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8165 SP_WINDOW_END); 8166 8167 /** 8168 * Search parameter: <b>type</b> 8169 * <p> 8170 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 8171 * Type: <b>token</b><br> 8172 * Path: <b>MolecularSequence.type</b><br> 8173 * </p> 8174 */ 8175 @SearchParamDefinition(name = "type", path = "MolecularSequence.type", description = "Amino Acid Sequence/ DNA Sequence / RNA Sequence", type = "token") 8176 public static final String SP_TYPE = "type"; 8177 /** 8178 * <b>Fluent Client</b> search parameter constant for <b>type</b> 8179 * <p> 8180 * Description: <b>Amino Acid Sequence/ DNA Sequence / RNA Sequence</b><br> 8181 * Type: <b>token</b><br> 8182 * Path: <b>MolecularSequence.type</b><br> 8183 * </p> 8184 */ 8185 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8186 SP_TYPE); 8187 8188 /** 8189 * Search parameter: <b>window-start</b> 8190 * <p> 8191 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8192 * means the nucleic acid or amino acid at this position will be included) of 8193 * the reference sequence.</b><br> 8194 * Type: <b>number</b><br> 8195 * Path: <b>MolecularSequence.referenceSeq.windowStart</b><br> 8196 * </p> 8197 */ 8198 @SearchParamDefinition(name = "window-start", path = "MolecularSequence.referenceSeq.windowStart", description = "Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the reference sequence.", type = "number") 8199 public static final String SP_WINDOW_START = "window-start"; 8200 /** 8201 * <b>Fluent Client</b> search parameter constant for <b>window-start</b> 8202 * <p> 8203 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8204 * means the nucleic acid or amino acid at this position will be included) of 8205 * the reference sequence.</b><br> 8206 * Type: <b>number</b><br> 8207 * Path: <b>MolecularSequence.referenceSeq.windowStart</b><br> 8208 * </p> 8209 */ 8210 public static final ca.uhn.fhir.rest.gclient.NumberClientParam WINDOW_START = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8211 SP_WINDOW_START); 8212 8213 /** 8214 * Search parameter: <b>variant-end</b> 8215 * <p> 8216 * Description: <b>End position (0-based exclusive, which menas the acid at this 8217 * position will not be included, 1-based inclusive, which means the acid at 8218 * this position will be included) of the variant.</b><br> 8219 * Type: <b>number</b><br> 8220 * Path: <b>MolecularSequence.variant.end</b><br> 8221 * </p> 8222 */ 8223 @SearchParamDefinition(name = "variant-end", path = "MolecularSequence.variant.end", description = "End position (0-based exclusive, which menas the acid at this position will not be included, 1-based inclusive, which means the acid at this position will be included) of the variant.", type = "number") 8224 public static final String SP_VARIANT_END = "variant-end"; 8225 /** 8226 * <b>Fluent Client</b> search parameter constant for <b>variant-end</b> 8227 * <p> 8228 * Description: <b>End position (0-based exclusive, which menas the acid at this 8229 * position will not be included, 1-based inclusive, which means the acid at 8230 * this position will be included) of the variant.</b><br> 8231 * Type: <b>number</b><br> 8232 * Path: <b>MolecularSequence.variant.end</b><br> 8233 * </p> 8234 */ 8235 public static final ca.uhn.fhir.rest.gclient.NumberClientParam VARIANT_END = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8236 SP_VARIANT_END); 8237 8238 /** 8239 * Search parameter: <b>chromosome-variant-coordinate</b> 8240 * <p> 8241 * Description: <b>Search parameter by chromosome and variant coordinate. This 8242 * will refer to part of a locus or part of a gene where search region will be 8243 * represented in 1-based system. Since the coordinateSystem can either be 8244 * 0-based or 1-based, this search query will include the result of both 8245 * coordinateSystem that contains the equivalent segment of the gene or whole 8246 * genome sequence. For example, a search for sequence can be represented as 8247 * `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for 8248 * the MolecularSequence resource with variants on chromosome 1 and with 8249 * position >123 and <345, where in 1-based system resource, all strings within 8250 * region 1:124-344 will be revealed, while in 0-based system resource, all 8251 * strings within region 1:123-344 will be revealed. You may want to check 8252 * detail about 0-based v.s. 1-based above.</b><br> 8253 * Type: <b>composite</b><br> 8254 * Path: <b></b><br> 8255 * </p> 8256 */ 8257 @SearchParamDefinition(name = "chromosome-variant-coordinate", path = "MolecularSequence.variant", description = "Search parameter by chromosome and variant coordinate. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for the MolecularSequence resource with variants on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8258 "chromosome", "variant-start" }) 8259 public static final String SP_CHROMOSOME_VARIANT_COORDINATE = "chromosome-variant-coordinate"; 8260 /** 8261 * <b>Fluent Client</b> search parameter constant for 8262 * <b>chromosome-variant-coordinate</b> 8263 * <p> 8264 * Description: <b>Search parameter by chromosome and variant coordinate. This 8265 * will refer to part of a locus or part of a gene where search region will be 8266 * represented in 1-based system. Since the coordinateSystem can either be 8267 * 0-based or 1-based, this search query will include the result of both 8268 * coordinateSystem that contains the equivalent segment of the gene or whole 8269 * genome sequence. For example, a search for sequence can be represented as 8270 * `chromosome-variant-coordinate=1$lt345$gt123`, this means it will search for 8271 * the MolecularSequence resource with variants on chromosome 1 and with 8272 * position >123 and <345, where in 1-based system resource, all strings within 8273 * region 1:124-344 will be revealed, while in 0-based system resource, all 8274 * strings within region 1:123-344 will be revealed. You may want to check 8275 * detail about 0-based v.s. 1-based above.</b><br> 8276 * Type: <b>composite</b><br> 8277 * Path: <b></b><br> 8278 * </p> 8279 */ 8280 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> CHROMOSOME_VARIANT_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8281 SP_CHROMOSOME_VARIANT_COORDINATE); 8282 8283 /** 8284 * Search parameter: <b>patient</b> 8285 * <p> 8286 * Description: <b>The subject that the observation is about</b><br> 8287 * Type: <b>reference</b><br> 8288 * Path: <b>MolecularSequence.patient</b><br> 8289 * </p> 8290 */ 8291 @SearchParamDefinition(name = "patient", path = "MolecularSequence.patient", description = "The subject that the observation is about", type = "reference", providesMembershipIn = { 8292 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 8293 public static final String SP_PATIENT = "patient"; 8294 /** 8295 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 8296 * <p> 8297 * Description: <b>The subject that the observation is about</b><br> 8298 * Type: <b>reference</b><br> 8299 * Path: <b>MolecularSequence.patient</b><br> 8300 * </p> 8301 */ 8302 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 8303 SP_PATIENT); 8304 8305 /** 8306 * Constant for fluent queries to be used to add include statements. Specifies 8307 * the path value of "<b>MolecularSequence:patient</b>". 8308 */ 8309 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 8310 "MolecularSequence:patient").toLocked(); 8311 8312 /** 8313 * Search parameter: <b>variant-start</b> 8314 * <p> 8315 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8316 * means the nucleic acid or amino acid at this position will be included) of 8317 * the variant.</b><br> 8318 * Type: <b>number</b><br> 8319 * Path: <b>MolecularSequence.variant.start</b><br> 8320 * </p> 8321 */ 8322 @SearchParamDefinition(name = "variant-start", path = "MolecularSequence.variant.start", description = "Start position (0-based inclusive, 1-based inclusive, that means the nucleic acid or amino acid at this position will be included) of the variant.", type = "number") 8323 public static final String SP_VARIANT_START = "variant-start"; 8324 /** 8325 * <b>Fluent Client</b> search parameter constant for <b>variant-start</b> 8326 * <p> 8327 * Description: <b>Start position (0-based inclusive, 1-based inclusive, that 8328 * means the nucleic acid or amino acid at this position will be included) of 8329 * the variant.</b><br> 8330 * Type: <b>number</b><br> 8331 * Path: <b>MolecularSequence.variant.start</b><br> 8332 * </p> 8333 */ 8334 public static final ca.uhn.fhir.rest.gclient.NumberClientParam VARIANT_START = new ca.uhn.fhir.rest.gclient.NumberClientParam( 8335 SP_VARIANT_START); 8336 8337 /** 8338 * Search parameter: <b>chromosome-window-coordinate</b> 8339 * <p> 8340 * Description: <b>Search parameter by chromosome and window. This will refer to 8341 * part of a locus or part of a gene where search region will be represented in 8342 * 1-based system. Since the coordinateSystem can either be 0-based or 1-based, 8343 * this search query will include the result of both coordinateSystem that 8344 * contains the equivalent segment of the gene or whole genome sequence. For 8345 * example, a search for sequence can be represented as 8346 * `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for 8347 * the MolecularSequence resource with a window on chromosome 1 and with 8348 * position >123 and <345, where in 1-based system resource, all strings within 8349 * region 1:124-344 will be revealed, while in 0-based system resource, all 8350 * strings within region 1:123-344 will be revealed. You may want to check 8351 * detail about 0-based v.s. 1-based above.</b><br> 8352 * Type: <b>composite</b><br> 8353 * Path: <b></b><br> 8354 * </p> 8355 */ 8356 @SearchParamDefinition(name = "chromosome-window-coordinate", path = "MolecularSequence.referenceSeq", description = "Search parameter by chromosome and window. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for the MolecularSequence resource with a window on chromosome 1 and with position >123 and <345, where in 1-based system resource, all strings within region 1:124-344 will be revealed, while in 0-based system resource, all strings within region 1:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8357 "chromosome", "window-start" }) 8358 public static final String SP_CHROMOSOME_WINDOW_COORDINATE = "chromosome-window-coordinate"; 8359 /** 8360 * <b>Fluent Client</b> search parameter constant for 8361 * <b>chromosome-window-coordinate</b> 8362 * <p> 8363 * Description: <b>Search parameter by chromosome and window. This will refer to 8364 * part of a locus or part of a gene where search region will be represented in 8365 * 1-based system. Since the coordinateSystem can either be 0-based or 1-based, 8366 * this search query will include the result of both coordinateSystem that 8367 * contains the equivalent segment of the gene or whole genome sequence. For 8368 * example, a search for sequence can be represented as 8369 * `chromosome-window-coordinate=1$lt345$gt123`, this means it will search for 8370 * the MolecularSequence resource with a window on chromosome 1 and with 8371 * position >123 and <345, where in 1-based system resource, all strings within 8372 * region 1:124-344 will be revealed, while in 0-based system resource, all 8373 * strings within region 1:123-344 will be revealed. You may want to check 8374 * detail about 0-based v.s. 1-based above.</b><br> 8375 * Type: <b>composite</b><br> 8376 * Path: <b></b><br> 8377 * </p> 8378 */ 8379 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> CHROMOSOME_WINDOW_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8380 SP_CHROMOSOME_WINDOW_COORDINATE); 8381 8382 /** 8383 * Search parameter: <b>referenceseqid-window-coordinate</b> 8384 * <p> 8385 * Description: <b>Search parameter by reference sequence and window. This will 8386 * refer to part of a locus or part of a gene where search region will be 8387 * represented in 1-based system. Since the coordinateSystem can either be 8388 * 0-based or 1-based, this search query will include the result of both 8389 * coordinateSystem that contains the equivalent segment of the gene or whole 8390 * genome sequence. For example, a search for sequence can be represented as 8391 * `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it 8392 * will search for the MolecularSequence resource with a window on NC_000001.11 8393 * and with position >123 and <345, where in 1-based system resource, all 8394 * strings within region NC_000001.11:124-344 will be revealed, while in 0-based 8395 * system resource, all strings within region NC_000001.11:123-344 will be 8396 * revealed. You may want to check detail about 0-based v.s. 1-based 8397 * above.</b><br> 8398 * Type: <b>composite</b><br> 8399 * Path: <b></b><br> 8400 * </p> 8401 */ 8402 @SearchParamDefinition(name = "referenceseqid-window-coordinate", path = "MolecularSequence.referenceSeq", description = "Search parameter by reference sequence and window. This will refer to part of a locus or part of a gene where search region will be represented in 1-based system. Since the coordinateSystem can either be 0-based or 1-based, this search query will include the result of both coordinateSystem that contains the equivalent segment of the gene or whole genome sequence. For example, a search for sequence can be represented as `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it will search for the MolecularSequence resource with a window on NC_000001.11 and with position >123 and <345, where in 1-based system resource, all strings within region NC_000001.11:124-344 will be revealed, while in 0-based system resource, all strings within region NC_000001.11:123-344 will be revealed. You may want to check detail about 0-based v.s. 1-based above.", type = "composite", compositeOf = { 8403 "referenceseqid", "window-start" }) 8404 public static final String SP_REFERENCESEQID_WINDOW_COORDINATE = "referenceseqid-window-coordinate"; 8405 /** 8406 * <b>Fluent Client</b> search parameter constant for 8407 * <b>referenceseqid-window-coordinate</b> 8408 * <p> 8409 * Description: <b>Search parameter by reference sequence and window. This will 8410 * refer to part of a locus or part of a gene where search region will be 8411 * represented in 1-based system. Since the coordinateSystem can either be 8412 * 0-based or 1-based, this search query will include the result of both 8413 * coordinateSystem that contains the equivalent segment of the gene or whole 8414 * genome sequence. For example, a search for sequence can be represented as 8415 * `referenceSeqId-window-coordinate=NC_000001.11$lt345$gt123`, this means it 8416 * will search for the MolecularSequence resource with a window on NC_000001.11 8417 * and with position >123 and <345, where in 1-based system resource, all 8418 * strings within region NC_000001.11:124-344 will be revealed, while in 0-based 8419 * system resource, all strings within region NC_000001.11:123-344 will be 8420 * revealed. You may want to check detail about 0-based v.s. 1-based 8421 * above.</b><br> 8422 * Type: <b>composite</b><br> 8423 * Path: <b></b><br> 8424 * </p> 8425 */ 8426 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam> REFERENCESEQID_WINDOW_COORDINATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.NumberClientParam>( 8427 SP_REFERENCESEQID_WINDOW_COORDINATE); 8428 8429 /** 8430 * Search parameter: <b>referenceseqid</b> 8431 * <p> 8432 * Description: <b>Reference Sequence of the sequence</b><br> 8433 * Type: <b>token</b><br> 8434 * Path: <b>MolecularSequence.referenceSeq.referenceSeqId</b><br> 8435 * </p> 8436 */ 8437 @SearchParamDefinition(name = "referenceseqid", path = "MolecularSequence.referenceSeq.referenceSeqId", description = "Reference Sequence of the sequence", type = "token") 8438 public static final String SP_REFERENCESEQID = "referenceseqid"; 8439 /** 8440 * <b>Fluent Client</b> search parameter constant for <b>referenceseqid</b> 8441 * <p> 8442 * Description: <b>Reference Sequence of the sequence</b><br> 8443 * Type: <b>token</b><br> 8444 * Path: <b>MolecularSequence.referenceSeq.referenceSeqId</b><br> 8445 * </p> 8446 */ 8447 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REFERENCESEQID = new ca.uhn.fhir.rest.gclient.TokenClientParam( 8448 SP_REFERENCESEQID); 8449 8450}