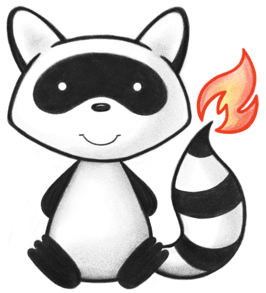
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049 050/** 051 * A curated namespace that issues unique symbols within that namespace for the 052 * identification of concepts, people, devices, etc. Represents a "System" used 053 * within the Identifier and Coding data types. 054 */ 055@ResourceDef(name = "NamingSystem", profile = "http://hl7.org/fhir/StructureDefinition/NamingSystem") 056@ChildOrder(names = { "name", "status", "kind", "date", "publisher", "contact", "responsible", "type", "description", 057 "useContext", "jurisdiction", "usage", "uniqueId" }) 058public class NamingSystem extends MetadataResource { 059 060 public enum NamingSystemType { 061 /** 062 * The naming system is used to define concepts and symbols to represent those 063 * concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc. 064 */ 065 CODESYSTEM, 066 /** 067 * The naming system is used to manage identifiers (e.g. license numbers, order 068 * numbers, etc.). 069 */ 070 IDENTIFIER, 071 /** 072 * The naming system is used as the root for other identifiers and naming 073 * systems. 074 */ 075 ROOT, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 081 public static NamingSystemType fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("codesystem".equals(codeString)) 085 return CODESYSTEM; 086 if ("identifier".equals(codeString)) 087 return IDENTIFIER; 088 if ("root".equals(codeString)) 089 return ROOT; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case CODESYSTEM: 099 return "codesystem"; 100 case IDENTIFIER: 101 return "identifier"; 102 case ROOT: 103 return "root"; 104 case NULL: 105 return null; 106 default: 107 return "?"; 108 } 109 } 110 111 public String getSystem() { 112 switch (this) { 113 case CODESYSTEM: 114 return "http://hl7.org/fhir/namingsystem-type"; 115 case IDENTIFIER: 116 return "http://hl7.org/fhir/namingsystem-type"; 117 case ROOT: 118 return "http://hl7.org/fhir/namingsystem-type"; 119 case NULL: 120 return null; 121 default: 122 return "?"; 123 } 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case CODESYSTEM: 129 return "The naming system is used to define concepts and symbols to represent those concepts; e.g. UCUM, LOINC, NDC code, local lab codes, etc."; 130 case IDENTIFIER: 131 return "The naming system is used to manage identifiers (e.g. license numbers, order numbers, etc.)."; 132 case ROOT: 133 return "The naming system is used as the root for other identifiers and naming systems."; 134 case NULL: 135 return null; 136 default: 137 return "?"; 138 } 139 } 140 141 public String getDisplay() { 142 switch (this) { 143 case CODESYSTEM: 144 return "Code System"; 145 case IDENTIFIER: 146 return "Identifier"; 147 case ROOT: 148 return "Root"; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 } 156 157 public static class NamingSystemTypeEnumFactory implements EnumFactory<NamingSystemType> { 158 public NamingSystemType fromCode(String codeString) throws IllegalArgumentException { 159 if (codeString == null || "".equals(codeString)) 160 if (codeString == null || "".equals(codeString)) 161 return null; 162 if ("codesystem".equals(codeString)) 163 return NamingSystemType.CODESYSTEM; 164 if ("identifier".equals(codeString)) 165 return NamingSystemType.IDENTIFIER; 166 if ("root".equals(codeString)) 167 return NamingSystemType.ROOT; 168 throw new IllegalArgumentException("Unknown NamingSystemType code '" + codeString + "'"); 169 } 170 171 public Enumeration<NamingSystemType> fromType(PrimitiveType<?> code) throws FHIRException { 172 if (code == null) 173 return null; 174 if (code.isEmpty()) 175 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 176 String codeString = code.asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return new Enumeration<NamingSystemType>(this, NamingSystemType.NULL, code); 179 if ("codesystem".equals(codeString)) 180 return new Enumeration<NamingSystemType>(this, NamingSystemType.CODESYSTEM, code); 181 if ("identifier".equals(codeString)) 182 return new Enumeration<NamingSystemType>(this, NamingSystemType.IDENTIFIER, code); 183 if ("root".equals(codeString)) 184 return new Enumeration<NamingSystemType>(this, NamingSystemType.ROOT, code); 185 throw new FHIRException("Unknown NamingSystemType code '" + codeString + "'"); 186 } 187 188 public String toCode(NamingSystemType code) { 189 if (code == NamingSystemType.NULL) 190 return null; 191 if (code == NamingSystemType.CODESYSTEM) 192 return "codesystem"; 193 if (code == NamingSystemType.IDENTIFIER) 194 return "identifier"; 195 if (code == NamingSystemType.ROOT) 196 return "root"; 197 return "?"; 198 } 199 200 public String toSystem(NamingSystemType code) { 201 return code.getSystem(); 202 } 203 } 204 205 public enum NamingSystemIdentifierType { 206 /** 207 * An ISO object identifier; e.g. 1.2.3.4.5. 208 */ 209 OID, 210 /** 211 * A universally unique identifier of the form 212 * a5afddf4-e880-459b-876e-e4591b0acc11. 213 */ 214 UUID, 215 /** 216 * A uniform resource identifier (ideally a URL - uniform resource locator); 217 * e.g. http://unitsofmeasure.org. 218 */ 219 URI, 220 /** 221 * Some other type of unique identifier; e.g. HL7-assigned reserved string such 222 * as LN for LOINC. 223 */ 224 OTHER, 225 /** 226 * added to help the parsers with the generic types 227 */ 228 NULL; 229 230 public static NamingSystemIdentifierType fromCode(String codeString) throws FHIRException { 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("oid".equals(codeString)) 234 return OID; 235 if ("uuid".equals(codeString)) 236 return UUID; 237 if ("uri".equals(codeString)) 238 return URI; 239 if ("other".equals(codeString)) 240 return OTHER; 241 if (Configuration.isAcceptInvalidEnums()) 242 return null; 243 else 244 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 245 } 246 247 public String toCode() { 248 switch (this) { 249 case OID: 250 return "oid"; 251 case UUID: 252 return "uuid"; 253 case URI: 254 return "uri"; 255 case OTHER: 256 return "other"; 257 case NULL: 258 return null; 259 default: 260 return "?"; 261 } 262 } 263 264 public String getSystem() { 265 switch (this) { 266 case OID: 267 return "http://hl7.org/fhir/namingsystem-identifier-type"; 268 case UUID: 269 return "http://hl7.org/fhir/namingsystem-identifier-type"; 270 case URI: 271 return "http://hl7.org/fhir/namingsystem-identifier-type"; 272 case OTHER: 273 return "http://hl7.org/fhir/namingsystem-identifier-type"; 274 case NULL: 275 return null; 276 default: 277 return "?"; 278 } 279 } 280 281 public String getDefinition() { 282 switch (this) { 283 case OID: 284 return "An ISO object identifier; e.g. 1.2.3.4.5."; 285 case UUID: 286 return "A universally unique identifier of the form a5afddf4-e880-459b-876e-e4591b0acc11."; 287 case URI: 288 return "A uniform resource identifier (ideally a URL - uniform resource locator); e.g. http://unitsofmeasure.org."; 289 case OTHER: 290 return "Some other type of unique identifier; e.g. HL7-assigned reserved string such as LN for LOINC."; 291 case NULL: 292 return null; 293 default: 294 return "?"; 295 } 296 } 297 298 public String getDisplay() { 299 switch (this) { 300 case OID: 301 return "OID"; 302 case UUID: 303 return "UUID"; 304 case URI: 305 return "URI"; 306 case OTHER: 307 return "Other"; 308 case NULL: 309 return null; 310 default: 311 return "?"; 312 } 313 } 314 } 315 316 public static class NamingSystemIdentifierTypeEnumFactory implements EnumFactory<NamingSystemIdentifierType> { 317 public NamingSystemIdentifierType fromCode(String codeString) throws IllegalArgumentException { 318 if (codeString == null || "".equals(codeString)) 319 if (codeString == null || "".equals(codeString)) 320 return null; 321 if ("oid".equals(codeString)) 322 return NamingSystemIdentifierType.OID; 323 if ("uuid".equals(codeString)) 324 return NamingSystemIdentifierType.UUID; 325 if ("uri".equals(codeString)) 326 return NamingSystemIdentifierType.URI; 327 if ("other".equals(codeString)) 328 return NamingSystemIdentifierType.OTHER; 329 throw new IllegalArgumentException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 330 } 331 332 public Enumeration<NamingSystemIdentifierType> fromType(PrimitiveType<?> code) throws FHIRException { 333 if (code == null) 334 return null; 335 if (code.isEmpty()) 336 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 337 String codeString = code.asStringValue(); 338 if (codeString == null || "".equals(codeString)) 339 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.NULL, code); 340 if ("oid".equals(codeString)) 341 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OID, code); 342 if ("uuid".equals(codeString)) 343 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.UUID, code); 344 if ("uri".equals(codeString)) 345 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.URI, code); 346 if ("other".equals(codeString)) 347 return new Enumeration<NamingSystemIdentifierType>(this, NamingSystemIdentifierType.OTHER, code); 348 throw new FHIRException("Unknown NamingSystemIdentifierType code '" + codeString + "'"); 349 } 350 351 public String toCode(NamingSystemIdentifierType code) { 352 if (code == NamingSystemIdentifierType.NULL) 353 return null; 354 if (code == NamingSystemIdentifierType.NULL) 355 return null; 356 if (code == NamingSystemIdentifierType.OID) 357 return "oid"; 358 if (code == NamingSystemIdentifierType.UUID) 359 return "uuid"; 360 if (code == NamingSystemIdentifierType.URI) 361 return "uri"; 362 if (code == NamingSystemIdentifierType.OTHER) 363 return "other"; 364 return "?"; 365 } 366 367 public String toSystem(NamingSystemIdentifierType code) { 368 return code.getSystem(); 369 } 370 } 371 372 @Block() 373 public static class NamingSystemUniqueIdComponent extends BackboneElement implements IBaseBackboneElement { 374 /** 375 * Identifies the unique identifier scheme used for this particular identifier. 376 */ 377 @Child(name = "type", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 378 @Description(shortDefinition = "oid | uuid | uri | other", formalDefinition = "Identifies the unique identifier scheme used for this particular identifier.") 379 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/namingsystem-identifier-type") 380 protected Enumeration<NamingSystemIdentifierType> type; 381 382 /** 383 * The string that should be sent over the wire to identify the code system or 384 * identifier system. 385 */ 386 @Child(name = "value", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 387 @Description(shortDefinition = "The unique identifier", formalDefinition = "The string that should be sent over the wire to identify the code system or identifier system.") 388 protected StringType value; 389 390 /** 391 * Indicates whether this identifier is the "preferred" identifier of this type. 392 */ 393 @Child(name = "preferred", type = { 394 BooleanType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 395 @Description(shortDefinition = "Is this the id that should be used for this type", formalDefinition = "Indicates whether this identifier is the \"preferred\" identifier of this type.") 396 protected BooleanType preferred; 397 398 /** 399 * Notes about the past or intended usage of this identifier. 400 */ 401 @Child(name = "comment", type = { 402 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 403 @Description(shortDefinition = "Notes about identifier usage", formalDefinition = "Notes about the past or intended usage of this identifier.") 404 protected StringType comment; 405 406 /** 407 * Identifies the period of time over which this identifier is considered 408 * appropriate to refer to the naming system. Outside of this window, the 409 * identifier might be non-deterministic. 410 */ 411 @Child(name = "period", type = { Period.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 412 @Description(shortDefinition = "When is identifier valid?", formalDefinition = "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.") 413 protected Period period; 414 415 private static final long serialVersionUID = -1458889328L; 416 417 /** 418 * Constructor 419 */ 420 public NamingSystemUniqueIdComponent() { 421 super(); 422 } 423 424 /** 425 * Constructor 426 */ 427 public NamingSystemUniqueIdComponent(Enumeration<NamingSystemIdentifierType> type, StringType value) { 428 super(); 429 this.type = type; 430 this.value = value; 431 } 432 433 /** 434 * @return {@link #type} (Identifies the unique identifier scheme used for this 435 * particular identifier.). This is the underlying object with id, value 436 * and extensions. The accessor "getType" gives direct access to the 437 * value 438 */ 439 public Enumeration<NamingSystemIdentifierType> getTypeElement() { 440 if (this.type == null) 441 if (Configuration.errorOnAutoCreate()) 442 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.type"); 443 else if (Configuration.doAutoCreate()) 444 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); // bb 445 return this.type; 446 } 447 448 public boolean hasTypeElement() { 449 return this.type != null && !this.type.isEmpty(); 450 } 451 452 public boolean hasType() { 453 return this.type != null && !this.type.isEmpty(); 454 } 455 456 /** 457 * @param value {@link #type} (Identifies the unique identifier scheme used for 458 * this particular identifier.). This is the underlying object with 459 * id, value and extensions. The accessor "getType" gives direct 460 * access to the value 461 */ 462 public NamingSystemUniqueIdComponent setTypeElement(Enumeration<NamingSystemIdentifierType> value) { 463 this.type = value; 464 return this; 465 } 466 467 /** 468 * @return Identifies the unique identifier scheme used for this particular 469 * identifier. 470 */ 471 public NamingSystemIdentifierType getType() { 472 return this.type == null ? null : this.type.getValue(); 473 } 474 475 /** 476 * @param value Identifies the unique identifier scheme used for this particular 477 * identifier. 478 */ 479 public NamingSystemUniqueIdComponent setType(NamingSystemIdentifierType value) { 480 if (this.type == null) 481 this.type = new Enumeration<NamingSystemIdentifierType>(new NamingSystemIdentifierTypeEnumFactory()); 482 this.type.setValue(value); 483 return this; 484 } 485 486 /** 487 * @return {@link #value} (The string that should be sent over the wire to 488 * identify the code system or identifier system.). This is the 489 * underlying object with id, value and extensions. The accessor 490 * "getValue" gives direct access to the value 491 */ 492 public StringType getValueElement() { 493 if (this.value == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.value"); 496 else if (Configuration.doAutoCreate()) 497 this.value = new StringType(); // bb 498 return this.value; 499 } 500 501 public boolean hasValueElement() { 502 return this.value != null && !this.value.isEmpty(); 503 } 504 505 public boolean hasValue() { 506 return this.value != null && !this.value.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #value} (The string that should be sent over the wire to 511 * identify the code system or identifier system.). This is the 512 * underlying object with id, value and extensions. The accessor 513 * "getValue" gives direct access to the value 514 */ 515 public NamingSystemUniqueIdComponent setValueElement(StringType value) { 516 this.value = value; 517 return this; 518 } 519 520 /** 521 * @return The string that should be sent over the wire to identify the code 522 * system or identifier system. 523 */ 524 public String getValue() { 525 return this.value == null ? null : this.value.getValue(); 526 } 527 528 /** 529 * @param value The string that should be sent over the wire to identify the 530 * code system or identifier system. 531 */ 532 public NamingSystemUniqueIdComponent setValue(String value) { 533 if (this.value == null) 534 this.value = new StringType(); 535 this.value.setValue(value); 536 return this; 537 } 538 539 /** 540 * @return {@link #preferred} (Indicates whether this identifier is the 541 * "preferred" identifier of this type.). This is the underlying object 542 * with id, value and extensions. The accessor "getPreferred" gives 543 * direct access to the value 544 */ 545 public BooleanType getPreferredElement() { 546 if (this.preferred == null) 547 if (Configuration.errorOnAutoCreate()) 548 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.preferred"); 549 else if (Configuration.doAutoCreate()) 550 this.preferred = new BooleanType(); // bb 551 return this.preferred; 552 } 553 554 public boolean hasPreferredElement() { 555 return this.preferred != null && !this.preferred.isEmpty(); 556 } 557 558 public boolean hasPreferred() { 559 return this.preferred != null && !this.preferred.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #preferred} (Indicates whether this identifier is the 564 * "preferred" identifier of this type.). This is the underlying 565 * object with id, value and extensions. The accessor 566 * "getPreferred" gives direct access to the value 567 */ 568 public NamingSystemUniqueIdComponent setPreferredElement(BooleanType value) { 569 this.preferred = value; 570 return this; 571 } 572 573 /** 574 * @return Indicates whether this identifier is the "preferred" identifier of 575 * this type. 576 */ 577 public boolean getPreferred() { 578 return this.preferred == null || this.preferred.isEmpty() ? false : this.preferred.getValue(); 579 } 580 581 /** 582 * @param value Indicates whether this identifier is the "preferred" identifier 583 * of this type. 584 */ 585 public NamingSystemUniqueIdComponent setPreferred(boolean value) { 586 if (this.preferred == null) 587 this.preferred = new BooleanType(); 588 this.preferred.setValue(value); 589 return this; 590 } 591 592 /** 593 * @return {@link #comment} (Notes about the past or intended usage of this 594 * identifier.). This is the underlying object with id, value and 595 * extensions. The accessor "getComment" gives direct access to the 596 * value 597 */ 598 public StringType getCommentElement() { 599 if (this.comment == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.comment"); 602 else if (Configuration.doAutoCreate()) 603 this.comment = new StringType(); // bb 604 return this.comment; 605 } 606 607 public boolean hasCommentElement() { 608 return this.comment != null && !this.comment.isEmpty(); 609 } 610 611 public boolean hasComment() { 612 return this.comment != null && !this.comment.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #comment} (Notes about the past or intended usage of this 617 * identifier.). This is the underlying object with id, value and 618 * extensions. The accessor "getComment" gives direct access to the 619 * value 620 */ 621 public NamingSystemUniqueIdComponent setCommentElement(StringType value) { 622 this.comment = value; 623 return this; 624 } 625 626 /** 627 * @return Notes about the past or intended usage of this identifier. 628 */ 629 public String getComment() { 630 return this.comment == null ? null : this.comment.getValue(); 631 } 632 633 /** 634 * @param value Notes about the past or intended usage of this identifier. 635 */ 636 public NamingSystemUniqueIdComponent setComment(String value) { 637 if (Utilities.noString(value)) 638 this.comment = null; 639 else { 640 if (this.comment == null) 641 this.comment = new StringType(); 642 this.comment.setValue(value); 643 } 644 return this; 645 } 646 647 /** 648 * @return {@link #period} (Identifies the period of time over which this 649 * identifier is considered appropriate to refer to the naming system. 650 * Outside of this window, the identifier might be non-deterministic.) 651 */ 652 public Period getPeriod() { 653 if (this.period == null) 654 if (Configuration.errorOnAutoCreate()) 655 throw new Error("Attempt to auto-create NamingSystemUniqueIdComponent.period"); 656 else if (Configuration.doAutoCreate()) 657 this.period = new Period(); // cc 658 return this.period; 659 } 660 661 public boolean hasPeriod() { 662 return this.period != null && !this.period.isEmpty(); 663 } 664 665 /** 666 * @param value {@link #period} (Identifies the period of time over which this 667 * identifier is considered appropriate to refer to the naming 668 * system. Outside of this window, the identifier might be 669 * non-deterministic.) 670 */ 671 public NamingSystemUniqueIdComponent setPeriod(Period value) { 672 this.period = value; 673 return this; 674 } 675 676 protected void listChildren(List<Property> children) { 677 super.listChildren(children); 678 children.add(new Property("type", "code", 679 "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type)); 680 children.add(new Property("value", "string", 681 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, 682 value)); 683 children.add(new Property("preferred", "boolean", 684 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred)); 685 children.add(new Property("comment", "string", "Notes about the past or intended usage of this identifier.", 0, 1, 686 comment)); 687 children.add(new Property("period", "Period", 688 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 689 0, 1, period)); 690 } 691 692 @Override 693 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 694 switch (_hash) { 695 case 3575610: 696 /* type */ return new Property("type", "code", 697 "Identifies the unique identifier scheme used for this particular identifier.", 0, 1, type); 698 case 111972721: 699 /* value */ return new Property("value", "string", 700 "The string that should be sent over the wire to identify the code system or identifier system.", 0, 1, 701 value); 702 case -1294005119: 703 /* preferred */ return new Property("preferred", "boolean", 704 "Indicates whether this identifier is the \"preferred\" identifier of this type.", 0, 1, preferred); 705 case 950398559: 706 /* comment */ return new Property("comment", "string", 707 "Notes about the past or intended usage of this identifier.", 0, 1, comment); 708 case -991726143: 709 /* period */ return new Property("period", "Period", 710 "Identifies the period of time over which this identifier is considered appropriate to refer to the naming system. Outside of this window, the identifier might be non-deterministic.", 711 0, 1, period); 712 default: 713 return super.getNamedProperty(_hash, _name, _checkValid); 714 } 715 716 } 717 718 @Override 719 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 720 switch (hash) { 721 case 3575610: 722 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // Enumeration<NamingSystemIdentifierType> 723 case 111972721: 724 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // StringType 725 case -1294005119: 726 /* preferred */ return this.preferred == null ? new Base[0] : new Base[] { this.preferred }; // BooleanType 727 case 950398559: 728 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 729 case -991726143: 730 /* period */ return this.period == null ? new Base[0] : new Base[] { this.period }; // Period 731 default: 732 return super.getProperty(hash, name, checkValid); 733 } 734 735 } 736 737 @Override 738 public Base setProperty(int hash, String name, Base value) throws FHIRException { 739 switch (hash) { 740 case 3575610: // type 741 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 742 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 743 return value; 744 case 111972721: // value 745 this.value = castToString(value); // StringType 746 return value; 747 case -1294005119: // preferred 748 this.preferred = castToBoolean(value); // BooleanType 749 return value; 750 case 950398559: // comment 751 this.comment = castToString(value); // StringType 752 return value; 753 case -991726143: // period 754 this.period = castToPeriod(value); // Period 755 return value; 756 default: 757 return super.setProperty(hash, name, value); 758 } 759 760 } 761 762 @Override 763 public Base setProperty(String name, Base value) throws FHIRException { 764 if (name.equals("type")) { 765 value = new NamingSystemIdentifierTypeEnumFactory().fromType(castToCode(value)); 766 this.type = (Enumeration) value; // Enumeration<NamingSystemIdentifierType> 767 } else if (name.equals("value")) { 768 this.value = castToString(value); // StringType 769 } else if (name.equals("preferred")) { 770 this.preferred = castToBoolean(value); // BooleanType 771 } else if (name.equals("comment")) { 772 this.comment = castToString(value); // StringType 773 } else if (name.equals("period")) { 774 this.period = castToPeriod(value); // Period 775 } else 776 return super.setProperty(name, value); 777 return value; 778 } 779 780 @Override 781 public void removeChild(String name, Base value) throws FHIRException { 782 if (name.equals("type")) { 783 this.type = null; 784 } else if (name.equals("value")) { 785 this.value = null; 786 } else if (name.equals("preferred")) { 787 this.preferred = null; 788 } else if (name.equals("comment")) { 789 this.comment = null; 790 } else if (name.equals("period")) { 791 this.period = null; 792 } else 793 super.removeChild(name, value); 794 795 } 796 797 @Override 798 public Base makeProperty(int hash, String name) throws FHIRException { 799 switch (hash) { 800 case 3575610: 801 return getTypeElement(); 802 case 111972721: 803 return getValueElement(); 804 case -1294005119: 805 return getPreferredElement(); 806 case 950398559: 807 return getCommentElement(); 808 case -991726143: 809 return getPeriod(); 810 default: 811 return super.makeProperty(hash, name); 812 } 813 814 } 815 816 @Override 817 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case 3575610: 820 /* type */ return new String[] { "code" }; 821 case 111972721: 822 /* value */ return new String[] { "string" }; 823 case -1294005119: 824 /* preferred */ return new String[] { "boolean" }; 825 case 950398559: 826 /* comment */ return new String[] { "string" }; 827 case -991726143: 828 /* period */ return new String[] { "Period" }; 829 default: 830 return super.getTypesForProperty(hash, name); 831 } 832 833 } 834 835 @Override 836 public Base addChild(String name) throws FHIRException { 837 if (name.equals("type")) { 838 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.type"); 839 } else if (name.equals("value")) { 840 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.value"); 841 } else if (name.equals("preferred")) { 842 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.preferred"); 843 } else if (name.equals("comment")) { 844 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.comment"); 845 } else if (name.equals("period")) { 846 this.period = new Period(); 847 return this.period; 848 } else 849 return super.addChild(name); 850 } 851 852 public NamingSystemUniqueIdComponent copy() { 853 NamingSystemUniqueIdComponent dst = new NamingSystemUniqueIdComponent(); 854 copyValues(dst); 855 return dst; 856 } 857 858 public void copyValues(NamingSystemUniqueIdComponent dst) { 859 super.copyValues(dst); 860 dst.type = type == null ? null : type.copy(); 861 dst.value = value == null ? null : value.copy(); 862 dst.preferred = preferred == null ? null : preferred.copy(); 863 dst.comment = comment == null ? null : comment.copy(); 864 dst.period = period == null ? null : period.copy(); 865 } 866 867 @Override 868 public boolean equalsDeep(Base other_) { 869 if (!super.equalsDeep(other_)) 870 return false; 871 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 872 return false; 873 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 874 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true) 875 && compareDeep(preferred, o.preferred, true) && compareDeep(comment, o.comment, true) 876 && compareDeep(period, o.period, true); 877 } 878 879 @Override 880 public boolean equalsShallow(Base other_) { 881 if (!super.equalsShallow(other_)) 882 return false; 883 if (!(other_ instanceof NamingSystemUniqueIdComponent)) 884 return false; 885 NamingSystemUniqueIdComponent o = (NamingSystemUniqueIdComponent) other_; 886 return compareValues(type, o.type, true) && compareValues(value, o.value, true) 887 && compareValues(preferred, o.preferred, true) && compareValues(comment, o.comment, true); 888 } 889 890 public boolean isEmpty() { 891 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value, preferred, comment, period); 892 } 893 894 public String fhirType() { 895 return "NamingSystem.uniqueId"; 896 897 } 898 899 } 900 901 /** 902 * Indicates the purpose for the naming system - what kinds of things does it 903 * make unique? 904 */ 905 @Child(name = "kind", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 906 @Description(shortDefinition = "codesystem | identifier | root", formalDefinition = "Indicates the purpose for the naming system - what kinds of things does it make unique?") 907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/namingsystem-type") 908 protected Enumeration<NamingSystemType> kind; 909 910 /** 911 * The name of the organization that is responsible for issuing identifiers or 912 * codes for this namespace and ensuring their non-collision. 913 */ 914 @Child(name = "responsible", type = { 915 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 916 @Description(shortDefinition = "Who maintains system namespace?", formalDefinition = "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.") 917 protected StringType responsible; 918 919 /** 920 * Categorizes a naming system for easier search by grouping related naming 921 * systems. 922 */ 923 @Child(name = "type", type = { 924 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 925 @Description(shortDefinition = "e.g. driver, provider, patient, bank etc.", formalDefinition = "Categorizes a naming system for easier search by grouping related naming systems.") 926 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/identifier-type") 927 protected CodeableConcept type; 928 929 /** 930 * Provides guidance on the use of the namespace, including the handling of 931 * formatting characters, use of upper vs. lower case, etc. 932 */ 933 @Child(name = "usage", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 934 @Description(shortDefinition = "How/where is it used", formalDefinition = "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.") 935 protected StringType usage; 936 937 /** 938 * Indicates how the system may be identified when referenced in electronic 939 * exchange. 940 */ 941 @Child(name = "uniqueId", type = {}, order = 4, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 942 @Description(shortDefinition = "Unique identifiers used for system", formalDefinition = "Indicates how the system may be identified when referenced in electronic exchange.") 943 protected List<NamingSystemUniqueIdComponent> uniqueId; 944 945 private static final long serialVersionUID = 1686086580L; 946 947 /** 948 * Constructor 949 */ 950 public NamingSystem() { 951 super(); 952 } 953 954 /** 955 * Constructor 956 */ 957 public NamingSystem(StringType name, Enumeration<PublicationStatus> status, Enumeration<NamingSystemType> kind, 958 DateTimeType date) { 959 super(); 960 this.name = name; 961 this.status = status; 962 this.kind = kind; 963 this.date = date; 964 } 965 966 /** 967 * @return {@link #name} (A natural language name identifying the naming system. 968 * This name should be usable as an identifier for the module by machine 969 * processing applications such as code generation.). This is the 970 * underlying object with id, value and extensions. The accessor 971 * "getName" gives direct access to the value 972 */ 973 public StringType getNameElement() { 974 if (this.name == null) 975 if (Configuration.errorOnAutoCreate()) 976 throw new Error("Attempt to auto-create NamingSystem.name"); 977 else if (Configuration.doAutoCreate()) 978 this.name = new StringType(); // bb 979 return this.name; 980 } 981 982 public boolean hasNameElement() { 983 return this.name != null && !this.name.isEmpty(); 984 } 985 986 public boolean hasName() { 987 return this.name != null && !this.name.isEmpty(); 988 } 989 990 /** 991 * @param value {@link #name} (A natural language name identifying the naming 992 * system. This name should be usable as an identifier for the 993 * module by machine processing applications such as code 994 * generation.). This is the underlying object with id, value and 995 * extensions. The accessor "getName" gives direct access to the 996 * value 997 */ 998 public NamingSystem setNameElement(StringType value) { 999 this.name = value; 1000 return this; 1001 } 1002 1003 /** 1004 * @return A natural language name identifying the naming system. This name 1005 * should be usable as an identifier for the module by machine 1006 * processing applications such as code generation. 1007 */ 1008 public String getName() { 1009 return this.name == null ? null : this.name.getValue(); 1010 } 1011 1012 /** 1013 * @param value A natural language name identifying the naming system. This name 1014 * should be usable as an identifier for the module by machine 1015 * processing applications such as code generation. 1016 */ 1017 public NamingSystem setName(String value) { 1018 if (this.name == null) 1019 this.name = new StringType(); 1020 this.name.setValue(value); 1021 return this; 1022 } 1023 1024 /** 1025 * @return {@link #status} (The status of this naming system. Enables tracking 1026 * the life-cycle of the content.). This is the underlying object with 1027 * id, value and extensions. The accessor "getStatus" gives direct 1028 * access to the value 1029 */ 1030 public Enumeration<PublicationStatus> getStatusElement() { 1031 if (this.status == null) 1032 if (Configuration.errorOnAutoCreate()) 1033 throw new Error("Attempt to auto-create NamingSystem.status"); 1034 else if (Configuration.doAutoCreate()) 1035 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1036 return this.status; 1037 } 1038 1039 public boolean hasStatusElement() { 1040 return this.status != null && !this.status.isEmpty(); 1041 } 1042 1043 public boolean hasStatus() { 1044 return this.status != null && !this.status.isEmpty(); 1045 } 1046 1047 /** 1048 * @param value {@link #status} (The status of this naming system. Enables 1049 * tracking the life-cycle of the content.). This is the underlying 1050 * object with id, value and extensions. The accessor "getStatus" 1051 * gives direct access to the value 1052 */ 1053 public NamingSystem setStatusElement(Enumeration<PublicationStatus> value) { 1054 this.status = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return The status of this naming system. Enables tracking the life-cycle of 1060 * the content. 1061 */ 1062 public PublicationStatus getStatus() { 1063 return this.status == null ? null : this.status.getValue(); 1064 } 1065 1066 /** 1067 * @param value The status of this naming system. Enables tracking the 1068 * life-cycle of the content. 1069 */ 1070 public NamingSystem setStatus(PublicationStatus value) { 1071 if (this.status == null) 1072 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1073 this.status.setValue(value); 1074 return this; 1075 } 1076 1077 /** 1078 * @return {@link #kind} (Indicates the purpose for the naming system - what 1079 * kinds of things does it make unique?). This is the underlying object 1080 * with id, value and extensions. The accessor "getKind" gives direct 1081 * access to the value 1082 */ 1083 public Enumeration<NamingSystemType> getKindElement() { 1084 if (this.kind == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create NamingSystem.kind"); 1087 else if (Configuration.doAutoCreate()) 1088 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); // bb 1089 return this.kind; 1090 } 1091 1092 public boolean hasKindElement() { 1093 return this.kind != null && !this.kind.isEmpty(); 1094 } 1095 1096 public boolean hasKind() { 1097 return this.kind != null && !this.kind.isEmpty(); 1098 } 1099 1100 /** 1101 * @param value {@link #kind} (Indicates the purpose for the naming system - 1102 * what kinds of things does it make unique?). This is the 1103 * underlying object with id, value and extensions. The accessor 1104 * "getKind" gives direct access to the value 1105 */ 1106 public NamingSystem setKindElement(Enumeration<NamingSystemType> value) { 1107 this.kind = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return Indicates the purpose for the naming system - what kinds of things 1113 * does it make unique? 1114 */ 1115 public NamingSystemType getKind() { 1116 return this.kind == null ? null : this.kind.getValue(); 1117 } 1118 1119 /** 1120 * @param value Indicates the purpose for the naming system - what kinds of 1121 * things does it make unique? 1122 */ 1123 public NamingSystem setKind(NamingSystemType value) { 1124 if (this.kind == null) 1125 this.kind = new Enumeration<NamingSystemType>(new NamingSystemTypeEnumFactory()); 1126 this.kind.setValue(value); 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #date} (The date (and optionally time) when the naming system 1132 * was published. The date must change when the business version changes 1133 * and it must change if the status code changes. In addition, it should 1134 * change when the substantive content of the naming system changes.). 1135 * This is the underlying object with id, value and extensions. The 1136 * accessor "getDate" gives direct access to the value 1137 */ 1138 public DateTimeType getDateElement() { 1139 if (this.date == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create NamingSystem.date"); 1142 else if (Configuration.doAutoCreate()) 1143 this.date = new DateTimeType(); // bb 1144 return this.date; 1145 } 1146 1147 public boolean hasDateElement() { 1148 return this.date != null && !this.date.isEmpty(); 1149 } 1150 1151 public boolean hasDate() { 1152 return this.date != null && !this.date.isEmpty(); 1153 } 1154 1155 /** 1156 * @param value {@link #date} (The date (and optionally time) when the naming 1157 * system was published. The date must change when the business 1158 * version changes and it must change if the status code changes. 1159 * In addition, it should change when the substantive content of 1160 * the naming system changes.). This is the underlying object with 1161 * id, value and extensions. The accessor "getDate" gives direct 1162 * access to the value 1163 */ 1164 public NamingSystem setDateElement(DateTimeType value) { 1165 this.date = value; 1166 return this; 1167 } 1168 1169 /** 1170 * @return The date (and optionally time) when the naming system was published. 1171 * The date must change when the business version changes and it must 1172 * change if the status code changes. In addition, it should change when 1173 * the substantive content of the naming system changes. 1174 */ 1175 public Date getDate() { 1176 return this.date == null ? null : this.date.getValue(); 1177 } 1178 1179 /** 1180 * @param value The date (and optionally time) when the naming system was 1181 * published. The date must change when the business version 1182 * changes and it must change if the status code changes. In 1183 * addition, it should change when the substantive content of the 1184 * naming system changes. 1185 */ 1186 public NamingSystem setDate(Date value) { 1187 if (this.date == null) 1188 this.date = new DateTimeType(); 1189 this.date.setValue(value); 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #publisher} (The name of the organization or individual that 1195 * published the naming system.). This is the underlying object with id, 1196 * value and extensions. The accessor "getPublisher" gives direct access 1197 * to the value 1198 */ 1199 public StringType getPublisherElement() { 1200 if (this.publisher == null) 1201 if (Configuration.errorOnAutoCreate()) 1202 throw new Error("Attempt to auto-create NamingSystem.publisher"); 1203 else if (Configuration.doAutoCreate()) 1204 this.publisher = new StringType(); // bb 1205 return this.publisher; 1206 } 1207 1208 public boolean hasPublisherElement() { 1209 return this.publisher != null && !this.publisher.isEmpty(); 1210 } 1211 1212 public boolean hasPublisher() { 1213 return this.publisher != null && !this.publisher.isEmpty(); 1214 } 1215 1216 /** 1217 * @param value {@link #publisher} (The name of the organization or individual 1218 * that published the naming system.). This is the underlying 1219 * object with id, value and extensions. The accessor 1220 * "getPublisher" gives direct access to the value 1221 */ 1222 public NamingSystem setPublisherElement(StringType value) { 1223 this.publisher = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return The name of the organization or individual that published the naming 1229 * system. 1230 */ 1231 public String getPublisher() { 1232 return this.publisher == null ? null : this.publisher.getValue(); 1233 } 1234 1235 /** 1236 * @param value The name of the organization or individual that published the 1237 * naming system. 1238 */ 1239 public NamingSystem setPublisher(String value) { 1240 if (Utilities.noString(value)) 1241 this.publisher = null; 1242 else { 1243 if (this.publisher == null) 1244 this.publisher = new StringType(); 1245 this.publisher.setValue(value); 1246 } 1247 return this; 1248 } 1249 1250 /** 1251 * @return {@link #contact} (Contact details to assist a user in finding and 1252 * communicating with the publisher.) 1253 */ 1254 public List<ContactDetail> getContact() { 1255 if (this.contact == null) 1256 this.contact = new ArrayList<ContactDetail>(); 1257 return this.contact; 1258 } 1259 1260 /** 1261 * @return Returns a reference to <code>this</code> for easy method chaining 1262 */ 1263 public NamingSystem setContact(List<ContactDetail> theContact) { 1264 this.contact = theContact; 1265 return this; 1266 } 1267 1268 public boolean hasContact() { 1269 if (this.contact == null) 1270 return false; 1271 for (ContactDetail item : this.contact) 1272 if (!item.isEmpty()) 1273 return true; 1274 return false; 1275 } 1276 1277 public ContactDetail addContact() { // 3 1278 ContactDetail t = new ContactDetail(); 1279 if (this.contact == null) 1280 this.contact = new ArrayList<ContactDetail>(); 1281 this.contact.add(t); 1282 return t; 1283 } 1284 1285 public NamingSystem addContact(ContactDetail t) { // 3 1286 if (t == null) 1287 return this; 1288 if (this.contact == null) 1289 this.contact = new ArrayList<ContactDetail>(); 1290 this.contact.add(t); 1291 return this; 1292 } 1293 1294 /** 1295 * @return The first repetition of repeating field {@link #contact}, creating it 1296 * if it does not already exist 1297 */ 1298 public ContactDetail getContactFirstRep() { 1299 if (getContact().isEmpty()) { 1300 addContact(); 1301 } 1302 return getContact().get(0); 1303 } 1304 1305 /** 1306 * @return {@link #responsible} (The name of the organization that is 1307 * responsible for issuing identifiers or codes for this namespace and 1308 * ensuring their non-collision.). This is the underlying object with 1309 * id, value and extensions. The accessor "getResponsible" gives direct 1310 * access to the value 1311 */ 1312 public StringType getResponsibleElement() { 1313 if (this.responsible == null) 1314 if (Configuration.errorOnAutoCreate()) 1315 throw new Error("Attempt to auto-create NamingSystem.responsible"); 1316 else if (Configuration.doAutoCreate()) 1317 this.responsible = new StringType(); // bb 1318 return this.responsible; 1319 } 1320 1321 public boolean hasResponsibleElement() { 1322 return this.responsible != null && !this.responsible.isEmpty(); 1323 } 1324 1325 public boolean hasResponsible() { 1326 return this.responsible != null && !this.responsible.isEmpty(); 1327 } 1328 1329 /** 1330 * @param value {@link #responsible} (The name of the organization that is 1331 * responsible for issuing identifiers or codes for this namespace 1332 * and ensuring their non-collision.). This is the underlying 1333 * object with id, value and extensions. The accessor 1334 * "getResponsible" gives direct access to the value 1335 */ 1336 public NamingSystem setResponsibleElement(StringType value) { 1337 this.responsible = value; 1338 return this; 1339 } 1340 1341 /** 1342 * @return The name of the organization that is responsible for issuing 1343 * identifiers or codes for this namespace and ensuring their 1344 * non-collision. 1345 */ 1346 public String getResponsible() { 1347 return this.responsible == null ? null : this.responsible.getValue(); 1348 } 1349 1350 /** 1351 * @param value The name of the organization that is responsible for issuing 1352 * identifiers or codes for this namespace and ensuring their 1353 * non-collision. 1354 */ 1355 public NamingSystem setResponsible(String value) { 1356 if (Utilities.noString(value)) 1357 this.responsible = null; 1358 else { 1359 if (this.responsible == null) 1360 this.responsible = new StringType(); 1361 this.responsible.setValue(value); 1362 } 1363 return this; 1364 } 1365 1366 /** 1367 * @return {@link #type} (Categorizes a naming system for easier search by 1368 * grouping related naming systems.) 1369 */ 1370 public CodeableConcept getType() { 1371 if (this.type == null) 1372 if (Configuration.errorOnAutoCreate()) 1373 throw new Error("Attempt to auto-create NamingSystem.type"); 1374 else if (Configuration.doAutoCreate()) 1375 this.type = new CodeableConcept(); // cc 1376 return this.type; 1377 } 1378 1379 public boolean hasType() { 1380 return this.type != null && !this.type.isEmpty(); 1381 } 1382 1383 /** 1384 * @param value {@link #type} (Categorizes a naming system for easier search by 1385 * grouping related naming systems.) 1386 */ 1387 public NamingSystem setType(CodeableConcept value) { 1388 this.type = value; 1389 return this; 1390 } 1391 1392 /** 1393 * @return {@link #description} (A free text natural language description of the 1394 * naming system from a consumer's perspective. Details about what the 1395 * namespace identifies including scope, granularity, version labeling, 1396 * etc.). This is the underlying object with id, value and extensions. 1397 * The accessor "getDescription" gives direct access to the value 1398 */ 1399 public MarkdownType getDescriptionElement() { 1400 if (this.description == null) 1401 if (Configuration.errorOnAutoCreate()) 1402 throw new Error("Attempt to auto-create NamingSystem.description"); 1403 else if (Configuration.doAutoCreate()) 1404 this.description = new MarkdownType(); // bb 1405 return this.description; 1406 } 1407 1408 public boolean hasDescriptionElement() { 1409 return this.description != null && !this.description.isEmpty(); 1410 } 1411 1412 public boolean hasDescription() { 1413 return this.description != null && !this.description.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #description} (A free text natural language description 1418 * of the naming system from a consumer's perspective. Details 1419 * about what the namespace identifies including scope, 1420 * granularity, version labeling, etc.). This is the underlying 1421 * object with id, value and extensions. The accessor 1422 * "getDescription" gives direct access to the value 1423 */ 1424 public NamingSystem setDescriptionElement(MarkdownType value) { 1425 this.description = value; 1426 return this; 1427 } 1428 1429 /** 1430 * @return A free text natural language description of the naming system from a 1431 * consumer's perspective. Details about what the namespace identifies 1432 * including scope, granularity, version labeling, etc. 1433 */ 1434 public String getDescription() { 1435 return this.description == null ? null : this.description.getValue(); 1436 } 1437 1438 /** 1439 * @param value A free text natural language description of the naming system 1440 * from a consumer's perspective. Details about what the namespace 1441 * identifies including scope, granularity, version labeling, etc. 1442 */ 1443 public NamingSystem setDescription(String value) { 1444 if (value == null) 1445 this.description = null; 1446 else { 1447 if (this.description == null) 1448 this.description = new MarkdownType(); 1449 this.description.setValue(value); 1450 } 1451 return this; 1452 } 1453 1454 /** 1455 * @return {@link #useContext} (The content was developed with a focus and 1456 * intent of supporting the contexts that are listed. These contexts may 1457 * be general categories (gender, age, ...) or may be references to 1458 * specific programs (insurance plans, studies, ...) and may be used to 1459 * assist with indexing and searching for appropriate naming system 1460 * instances.) 1461 */ 1462 public List<UsageContext> getUseContext() { 1463 if (this.useContext == null) 1464 this.useContext = new ArrayList<UsageContext>(); 1465 return this.useContext; 1466 } 1467 1468 /** 1469 * @return Returns a reference to <code>this</code> for easy method chaining 1470 */ 1471 public NamingSystem setUseContext(List<UsageContext> theUseContext) { 1472 this.useContext = theUseContext; 1473 return this; 1474 } 1475 1476 public boolean hasUseContext() { 1477 if (this.useContext == null) 1478 return false; 1479 for (UsageContext item : this.useContext) 1480 if (!item.isEmpty()) 1481 return true; 1482 return false; 1483 } 1484 1485 public UsageContext addUseContext() { // 3 1486 UsageContext t = new UsageContext(); 1487 if (this.useContext == null) 1488 this.useContext = new ArrayList<UsageContext>(); 1489 this.useContext.add(t); 1490 return t; 1491 } 1492 1493 public NamingSystem addUseContext(UsageContext t) { // 3 1494 if (t == null) 1495 return this; 1496 if (this.useContext == null) 1497 this.useContext = new ArrayList<UsageContext>(); 1498 this.useContext.add(t); 1499 return this; 1500 } 1501 1502 /** 1503 * @return The first repetition of repeating field {@link #useContext}, creating 1504 * it if it does not already exist 1505 */ 1506 public UsageContext getUseContextFirstRep() { 1507 if (getUseContext().isEmpty()) { 1508 addUseContext(); 1509 } 1510 return getUseContext().get(0); 1511 } 1512 1513 /** 1514 * @return {@link #jurisdiction} (A legal or geographic region in which the 1515 * naming system is intended to be used.) 1516 */ 1517 public List<CodeableConcept> getJurisdiction() { 1518 if (this.jurisdiction == null) 1519 this.jurisdiction = new ArrayList<CodeableConcept>(); 1520 return this.jurisdiction; 1521 } 1522 1523 /** 1524 * @return Returns a reference to <code>this</code> for easy method chaining 1525 */ 1526 public NamingSystem setJurisdiction(List<CodeableConcept> theJurisdiction) { 1527 this.jurisdiction = theJurisdiction; 1528 return this; 1529 } 1530 1531 public boolean hasJurisdiction() { 1532 if (this.jurisdiction == null) 1533 return false; 1534 for (CodeableConcept item : this.jurisdiction) 1535 if (!item.isEmpty()) 1536 return true; 1537 return false; 1538 } 1539 1540 public CodeableConcept addJurisdiction() { // 3 1541 CodeableConcept t = new CodeableConcept(); 1542 if (this.jurisdiction == null) 1543 this.jurisdiction = new ArrayList<CodeableConcept>(); 1544 this.jurisdiction.add(t); 1545 return t; 1546 } 1547 1548 public NamingSystem addJurisdiction(CodeableConcept t) { // 3 1549 if (t == null) 1550 return this; 1551 if (this.jurisdiction == null) 1552 this.jurisdiction = new ArrayList<CodeableConcept>(); 1553 this.jurisdiction.add(t); 1554 return this; 1555 } 1556 1557 /** 1558 * @return The first repetition of repeating field {@link #jurisdiction}, 1559 * creating it if it does not already exist 1560 */ 1561 public CodeableConcept getJurisdictionFirstRep() { 1562 if (getJurisdiction().isEmpty()) { 1563 addJurisdiction(); 1564 } 1565 return getJurisdiction().get(0); 1566 } 1567 1568 /** 1569 * @return {@link #usage} (Provides guidance on the use of the namespace, 1570 * including the handling of formatting characters, use of upper vs. 1571 * lower case, etc.). This is the underlying object with id, value and 1572 * extensions. The accessor "getUsage" gives direct access to the value 1573 */ 1574 public StringType getUsageElement() { 1575 if (this.usage == null) 1576 if (Configuration.errorOnAutoCreate()) 1577 throw new Error("Attempt to auto-create NamingSystem.usage"); 1578 else if (Configuration.doAutoCreate()) 1579 this.usage = new StringType(); // bb 1580 return this.usage; 1581 } 1582 1583 public boolean hasUsageElement() { 1584 return this.usage != null && !this.usage.isEmpty(); 1585 } 1586 1587 public boolean hasUsage() { 1588 return this.usage != null && !this.usage.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #usage} (Provides guidance on the use of the namespace, 1593 * including the handling of formatting characters, use of upper 1594 * vs. lower case, etc.). This is the underlying object with id, 1595 * value and extensions. The accessor "getUsage" gives direct 1596 * access to the value 1597 */ 1598 public NamingSystem setUsageElement(StringType value) { 1599 this.usage = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return Provides guidance on the use of the namespace, including the handling 1605 * of formatting characters, use of upper vs. lower case, etc. 1606 */ 1607 public String getUsage() { 1608 return this.usage == null ? null : this.usage.getValue(); 1609 } 1610 1611 /** 1612 * @param value Provides guidance on the use of the namespace, including the 1613 * handling of formatting characters, use of upper vs. lower case, 1614 * etc. 1615 */ 1616 public NamingSystem setUsage(String value) { 1617 if (Utilities.noString(value)) 1618 this.usage = null; 1619 else { 1620 if (this.usage == null) 1621 this.usage = new StringType(); 1622 this.usage.setValue(value); 1623 } 1624 return this; 1625 } 1626 1627 /** 1628 * @return {@link #uniqueId} (Indicates how the system may be identified when 1629 * referenced in electronic exchange.) 1630 */ 1631 public List<NamingSystemUniqueIdComponent> getUniqueId() { 1632 if (this.uniqueId == null) 1633 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1634 return this.uniqueId; 1635 } 1636 1637 /** 1638 * @return Returns a reference to <code>this</code> for easy method chaining 1639 */ 1640 public NamingSystem setUniqueId(List<NamingSystemUniqueIdComponent> theUniqueId) { 1641 this.uniqueId = theUniqueId; 1642 return this; 1643 } 1644 1645 public boolean hasUniqueId() { 1646 if (this.uniqueId == null) 1647 return false; 1648 for (NamingSystemUniqueIdComponent item : this.uniqueId) 1649 if (!item.isEmpty()) 1650 return true; 1651 return false; 1652 } 1653 1654 public NamingSystemUniqueIdComponent addUniqueId() { // 3 1655 NamingSystemUniqueIdComponent t = new NamingSystemUniqueIdComponent(); 1656 if (this.uniqueId == null) 1657 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1658 this.uniqueId.add(t); 1659 return t; 1660 } 1661 1662 public NamingSystem addUniqueId(NamingSystemUniqueIdComponent t) { // 3 1663 if (t == null) 1664 return this; 1665 if (this.uniqueId == null) 1666 this.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 1667 this.uniqueId.add(t); 1668 return this; 1669 } 1670 1671 /** 1672 * @return The first repetition of repeating field {@link #uniqueId}, creating 1673 * it if it does not already exist 1674 */ 1675 public NamingSystemUniqueIdComponent getUniqueIdFirstRep() { 1676 if (getUniqueId().isEmpty()) { 1677 addUniqueId(); 1678 } 1679 return getUniqueId().get(0); 1680 } 1681 1682 protected void listChildren(List<Property> children) { 1683 super.listChildren(children); 1684 children.add(new Property("name", "string", 1685 "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1686 0, 1, name)); 1687 children.add(new Property("status", "code", 1688 "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status)); 1689 children.add(new Property("kind", "code", 1690 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind)); 1691 children.add(new Property("date", "dateTime", 1692 "The date (and optionally time) when the naming system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 1693 0, 1, date)); 1694 children.add(new Property("publisher", "string", 1695 "The name of the organization or individual that published the naming system.", 0, 1, publisher)); 1696 children.add(new Property("contact", "ContactDetail", 1697 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1698 java.lang.Integer.MAX_VALUE, contact)); 1699 children.add(new Property("responsible", "string", 1700 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1701 0, 1, responsible)); 1702 children.add(new Property("type", "CodeableConcept", 1703 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type)); 1704 children.add(new Property("description", "markdown", 1705 "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 1706 0, 1, description)); 1707 children.add(new Property("useContext", "UsageContext", 1708 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 1709 0, java.lang.Integer.MAX_VALUE, useContext)); 1710 children.add(new Property("jurisdiction", "CodeableConcept", 1711 "A legal or geographic region in which the naming system is intended to be used.", 0, 1712 java.lang.Integer.MAX_VALUE, jurisdiction)); 1713 children.add(new Property("usage", "string", 1714 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1715 0, 1, usage)); 1716 children.add(new Property("uniqueId", "", 1717 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1718 java.lang.Integer.MAX_VALUE, uniqueId)); 1719 } 1720 1721 @Override 1722 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1723 switch (_hash) { 1724 case 3373707: 1725 /* name */ return new Property("name", "string", 1726 "A natural language name identifying the naming system. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1727 0, 1, name); 1728 case -892481550: 1729 /* status */ return new Property("status", "code", 1730 "The status of this naming system. Enables tracking the life-cycle of the content.", 0, 1, status); 1731 case 3292052: 1732 /* kind */ return new Property("kind", "code", 1733 "Indicates the purpose for the naming system - what kinds of things does it make unique?", 0, 1, kind); 1734 case 3076014: 1735 /* date */ return new Property("date", "dateTime", 1736 "The date (and optionally time) when the naming system was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the naming system changes.", 1737 0, 1, date); 1738 case 1447404028: 1739 /* publisher */ return new Property("publisher", "string", 1740 "The name of the organization or individual that published the naming system.", 0, 1, publisher); 1741 case 951526432: 1742 /* contact */ return new Property("contact", "ContactDetail", 1743 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1744 java.lang.Integer.MAX_VALUE, contact); 1745 case 1847674614: 1746 /* responsible */ return new Property("responsible", "string", 1747 "The name of the organization that is responsible for issuing identifiers or codes for this namespace and ensuring their non-collision.", 1748 0, 1, responsible); 1749 case 3575610: 1750 /* type */ return new Property("type", "CodeableConcept", 1751 "Categorizes a naming system for easier search by grouping related naming systems.", 0, 1, type); 1752 case -1724546052: 1753 /* description */ return new Property("description", "markdown", 1754 "A free text natural language description of the naming system from a consumer's perspective. Details about what the namespace identifies including scope, granularity, version labeling, etc.", 1755 0, 1, description); 1756 case -669707736: 1757 /* useContext */ return new Property("useContext", "UsageContext", 1758 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate naming system instances.", 1759 0, java.lang.Integer.MAX_VALUE, useContext); 1760 case -507075711: 1761 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 1762 "A legal or geographic region in which the naming system is intended to be used.", 0, 1763 java.lang.Integer.MAX_VALUE, jurisdiction); 1764 case 111574433: 1765 /* usage */ return new Property("usage", "string", 1766 "Provides guidance on the use of the namespace, including the handling of formatting characters, use of upper vs. lower case, etc.", 1767 0, 1, usage); 1768 case -294460212: 1769 /* uniqueId */ return new Property("uniqueId", "", 1770 "Indicates how the system may be identified when referenced in electronic exchange.", 0, 1771 java.lang.Integer.MAX_VALUE, uniqueId); 1772 default: 1773 return super.getNamedProperty(_hash, _name, _checkValid); 1774 } 1775 1776 } 1777 1778 @Override 1779 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1780 switch (hash) { 1781 case 3373707: 1782 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 1783 case -892481550: 1784 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 1785 case 3292052: 1786 /* kind */ return this.kind == null ? new Base[0] : new Base[] { this.kind }; // Enumeration<NamingSystemType> 1787 case 3076014: 1788 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 1789 case 1447404028: 1790 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 1791 case 951526432: 1792 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1793 case 1847674614: 1794 /* responsible */ return this.responsible == null ? new Base[0] : new Base[] { this.responsible }; // StringType 1795 case 3575610: 1796 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 1797 case -1724546052: 1798 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 1799 case -669707736: 1800 /* useContext */ return this.useContext == null ? new Base[0] 1801 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1802 case -507075711: 1803 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 1804 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1805 case 111574433: 1806 /* usage */ return this.usage == null ? new Base[0] : new Base[] { this.usage }; // StringType 1807 case -294460212: 1808 /* uniqueId */ return this.uniqueId == null ? new Base[0] : this.uniqueId.toArray(new Base[this.uniqueId.size()]); // NamingSystemUniqueIdComponent 1809 default: 1810 return super.getProperty(hash, name, checkValid); 1811 } 1812 1813 } 1814 1815 @Override 1816 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1817 switch (hash) { 1818 case 3373707: // name 1819 this.name = castToString(value); // StringType 1820 return value; 1821 case -892481550: // status 1822 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1823 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1824 return value; 1825 case 3292052: // kind 1826 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1827 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1828 return value; 1829 case 3076014: // date 1830 this.date = castToDateTime(value); // DateTimeType 1831 return value; 1832 case 1447404028: // publisher 1833 this.publisher = castToString(value); // StringType 1834 return value; 1835 case 951526432: // contact 1836 this.getContact().add(castToContactDetail(value)); // ContactDetail 1837 return value; 1838 case 1847674614: // responsible 1839 this.responsible = castToString(value); // StringType 1840 return value; 1841 case 3575610: // type 1842 this.type = castToCodeableConcept(value); // CodeableConcept 1843 return value; 1844 case -1724546052: // description 1845 this.description = castToMarkdown(value); // MarkdownType 1846 return value; 1847 case -669707736: // useContext 1848 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1849 return value; 1850 case -507075711: // jurisdiction 1851 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1852 return value; 1853 case 111574433: // usage 1854 this.usage = castToString(value); // StringType 1855 return value; 1856 case -294460212: // uniqueId 1857 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); // NamingSystemUniqueIdComponent 1858 return value; 1859 default: 1860 return super.setProperty(hash, name, value); 1861 } 1862 1863 } 1864 1865 @Override 1866 public Base setProperty(String name, Base value) throws FHIRException { 1867 if (name.equals("name")) { 1868 this.name = castToString(value); // StringType 1869 } else if (name.equals("status")) { 1870 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1871 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1872 } else if (name.equals("kind")) { 1873 value = new NamingSystemTypeEnumFactory().fromType(castToCode(value)); 1874 this.kind = (Enumeration) value; // Enumeration<NamingSystemType> 1875 } else if (name.equals("date")) { 1876 this.date = castToDateTime(value); // DateTimeType 1877 } else if (name.equals("publisher")) { 1878 this.publisher = castToString(value); // StringType 1879 } else if (name.equals("contact")) { 1880 this.getContact().add(castToContactDetail(value)); 1881 } else if (name.equals("responsible")) { 1882 this.responsible = castToString(value); // StringType 1883 } else if (name.equals("type")) { 1884 this.type = castToCodeableConcept(value); // CodeableConcept 1885 } else if (name.equals("description")) { 1886 this.description = castToMarkdown(value); // MarkdownType 1887 } else if (name.equals("useContext")) { 1888 this.getUseContext().add(castToUsageContext(value)); 1889 } else if (name.equals("jurisdiction")) { 1890 this.getJurisdiction().add(castToCodeableConcept(value)); 1891 } else if (name.equals("usage")) { 1892 this.usage = castToString(value); // StringType 1893 } else if (name.equals("uniqueId")) { 1894 this.getUniqueId().add((NamingSystemUniqueIdComponent) value); 1895 } else 1896 return super.setProperty(name, value); 1897 return value; 1898 } 1899 1900 @Override 1901 public void removeChild(String name, Base value) throws FHIRException { 1902 if (name.equals("name")) { 1903 this.name = null; 1904 } else if (name.equals("status")) { 1905 this.status = null; 1906 } else if (name.equals("kind")) { 1907 this.kind = null; 1908 } else if (name.equals("date")) { 1909 this.date = null; 1910 } else if (name.equals("publisher")) { 1911 this.publisher = null; 1912 } else if (name.equals("contact")) { 1913 this.getContact().remove(castToContactDetail(value)); 1914 } else if (name.equals("responsible")) { 1915 this.responsible = null; 1916 } else if (name.equals("type")) { 1917 this.type = null; 1918 } else if (name.equals("description")) { 1919 this.description = null; 1920 } else if (name.equals("useContext")) { 1921 this.getUseContext().remove(castToUsageContext(value)); 1922 } else if (name.equals("jurisdiction")) { 1923 this.getJurisdiction().remove(castToCodeableConcept(value)); 1924 } else if (name.equals("usage")) { 1925 this.usage = null; 1926 } else if (name.equals("uniqueId")) { 1927 this.getUniqueId().remove((NamingSystemUniqueIdComponent) value); 1928 } else 1929 super.removeChild(name, value); 1930 1931 } 1932 1933 @Override 1934 public Base makeProperty(int hash, String name) throws FHIRException { 1935 switch (hash) { 1936 case 3373707: 1937 return getNameElement(); 1938 case -892481550: 1939 return getStatusElement(); 1940 case 3292052: 1941 return getKindElement(); 1942 case 3076014: 1943 return getDateElement(); 1944 case 1447404028: 1945 return getPublisherElement(); 1946 case 951526432: 1947 return addContact(); 1948 case 1847674614: 1949 return getResponsibleElement(); 1950 case 3575610: 1951 return getType(); 1952 case -1724546052: 1953 return getDescriptionElement(); 1954 case -669707736: 1955 return addUseContext(); 1956 case -507075711: 1957 return addJurisdiction(); 1958 case 111574433: 1959 return getUsageElement(); 1960 case -294460212: 1961 return addUniqueId(); 1962 default: 1963 return super.makeProperty(hash, name); 1964 } 1965 1966 } 1967 1968 @Override 1969 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1970 switch (hash) { 1971 case 3373707: 1972 /* name */ return new String[] { "string" }; 1973 case -892481550: 1974 /* status */ return new String[] { "code" }; 1975 case 3292052: 1976 /* kind */ return new String[] { "code" }; 1977 case 3076014: 1978 /* date */ return new String[] { "dateTime" }; 1979 case 1447404028: 1980 /* publisher */ return new String[] { "string" }; 1981 case 951526432: 1982 /* contact */ return new String[] { "ContactDetail" }; 1983 case 1847674614: 1984 /* responsible */ return new String[] { "string" }; 1985 case 3575610: 1986 /* type */ return new String[] { "CodeableConcept" }; 1987 case -1724546052: 1988 /* description */ return new String[] { "markdown" }; 1989 case -669707736: 1990 /* useContext */ return new String[] { "UsageContext" }; 1991 case -507075711: 1992 /* jurisdiction */ return new String[] { "CodeableConcept" }; 1993 case 111574433: 1994 /* usage */ return new String[] { "string" }; 1995 case -294460212: 1996 /* uniqueId */ return new String[] {}; 1997 default: 1998 return super.getTypesForProperty(hash, name); 1999 } 2000 2001 } 2002 2003 @Override 2004 public Base addChild(String name) throws FHIRException { 2005 if (name.equals("name")) { 2006 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.name"); 2007 } else if (name.equals("status")) { 2008 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.status"); 2009 } else if (name.equals("kind")) { 2010 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.kind"); 2011 } else if (name.equals("date")) { 2012 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.date"); 2013 } else if (name.equals("publisher")) { 2014 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.publisher"); 2015 } else if (name.equals("contact")) { 2016 return addContact(); 2017 } else if (name.equals("responsible")) { 2018 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.responsible"); 2019 } else if (name.equals("type")) { 2020 this.type = new CodeableConcept(); 2021 return this.type; 2022 } else if (name.equals("description")) { 2023 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.description"); 2024 } else if (name.equals("useContext")) { 2025 return addUseContext(); 2026 } else if (name.equals("jurisdiction")) { 2027 return addJurisdiction(); 2028 } else if (name.equals("usage")) { 2029 throw new FHIRException("Cannot call addChild on a singleton property NamingSystem.usage"); 2030 } else if (name.equals("uniqueId")) { 2031 return addUniqueId(); 2032 } else 2033 return super.addChild(name); 2034 } 2035 2036 public String fhirType() { 2037 return "NamingSystem"; 2038 2039 } 2040 2041 public NamingSystem copy() { 2042 NamingSystem dst = new NamingSystem(); 2043 copyValues(dst); 2044 return dst; 2045 } 2046 2047 public void copyValues(NamingSystem dst) { 2048 super.copyValues(dst); 2049 dst.name = name == null ? null : name.copy(); 2050 dst.status = status == null ? null : status.copy(); 2051 dst.kind = kind == null ? null : kind.copy(); 2052 dst.date = date == null ? null : date.copy(); 2053 dst.publisher = publisher == null ? null : publisher.copy(); 2054 if (contact != null) { 2055 dst.contact = new ArrayList<ContactDetail>(); 2056 for (ContactDetail i : contact) 2057 dst.contact.add(i.copy()); 2058 } 2059 ; 2060 dst.responsible = responsible == null ? null : responsible.copy(); 2061 dst.type = type == null ? null : type.copy(); 2062 dst.description = description == null ? null : description.copy(); 2063 if (useContext != null) { 2064 dst.useContext = new ArrayList<UsageContext>(); 2065 for (UsageContext i : useContext) 2066 dst.useContext.add(i.copy()); 2067 } 2068 ; 2069 if (jurisdiction != null) { 2070 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2071 for (CodeableConcept i : jurisdiction) 2072 dst.jurisdiction.add(i.copy()); 2073 } 2074 ; 2075 dst.usage = usage == null ? null : usage.copy(); 2076 if (uniqueId != null) { 2077 dst.uniqueId = new ArrayList<NamingSystemUniqueIdComponent>(); 2078 for (NamingSystemUniqueIdComponent i : uniqueId) 2079 dst.uniqueId.add(i.copy()); 2080 } 2081 ; 2082 } 2083 2084 protected NamingSystem typedCopy() { 2085 return copy(); 2086 } 2087 2088 @Override 2089 public boolean equalsDeep(Base other_) { 2090 if (!super.equalsDeep(other_)) 2091 return false; 2092 if (!(other_ instanceof NamingSystem)) 2093 return false; 2094 NamingSystem o = (NamingSystem) other_; 2095 return compareDeep(kind, o.kind, true) && compareDeep(responsible, o.responsible, true) 2096 && compareDeep(type, o.type, true) && compareDeep(usage, o.usage, true) 2097 && compareDeep(uniqueId, o.uniqueId, true); 2098 } 2099 2100 @Override 2101 public boolean equalsShallow(Base other_) { 2102 if (!super.equalsShallow(other_)) 2103 return false; 2104 if (!(other_ instanceof NamingSystem)) 2105 return false; 2106 NamingSystem o = (NamingSystem) other_; 2107 return compareValues(kind, o.kind, true) && compareValues(responsible, o.responsible, true) 2108 && compareValues(usage, o.usage, true); 2109 } 2110 2111 public boolean isEmpty() { 2112 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(kind, responsible, type, usage, uniqueId); 2113 } 2114 2115 @Override 2116 public ResourceType getResourceType() { 2117 return ResourceType.NamingSystem; 2118 } 2119 2120 /** 2121 * Search parameter: <b>date</b> 2122 * <p> 2123 * Description: <b>The naming system publication date</b><br> 2124 * Type: <b>date</b><br> 2125 * Path: <b>NamingSystem.date</b><br> 2126 * </p> 2127 */ 2128 @SearchParamDefinition(name = "date", path = "NamingSystem.date", description = "The naming system publication date", type = "date") 2129 public static final String SP_DATE = "date"; 2130 /** 2131 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2132 * <p> 2133 * Description: <b>The naming system publication date</b><br> 2134 * Type: <b>date</b><br> 2135 * Path: <b>NamingSystem.date</b><br> 2136 * </p> 2137 */ 2138 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2139 SP_DATE); 2140 2141 /** 2142 * Search parameter: <b>period</b> 2143 * <p> 2144 * Description: <b>When is identifier valid?</b><br> 2145 * Type: <b>date</b><br> 2146 * Path: <b>NamingSystem.uniqueId.period</b><br> 2147 * </p> 2148 */ 2149 @SearchParamDefinition(name = "period", path = "NamingSystem.uniqueId.period", description = "When is identifier valid?", type = "date") 2150 public static final String SP_PERIOD = "period"; 2151 /** 2152 * <b>Fluent Client</b> search parameter constant for <b>period</b> 2153 * <p> 2154 * Description: <b>When is identifier valid?</b><br> 2155 * Type: <b>date</b><br> 2156 * Path: <b>NamingSystem.uniqueId.period</b><br> 2157 * </p> 2158 */ 2159 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam( 2160 SP_PERIOD); 2161 2162 /** 2163 * Search parameter: <b>context-type-value</b> 2164 * <p> 2165 * Description: <b>A use context type and value assigned to the naming 2166 * system</b><br> 2167 * Type: <b>composite</b><br> 2168 * Path: <b></b><br> 2169 * </p> 2170 */ 2171 @SearchParamDefinition(name = "context-type-value", path = "NamingSystem.useContext", description = "A use context type and value assigned to the naming system", type = "composite", compositeOf = { 2172 "context-type", "context" }) 2173 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2174 /** 2175 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2176 * <p> 2177 * Description: <b>A use context type and value assigned to the naming 2178 * system</b><br> 2179 * Type: <b>composite</b><br> 2180 * Path: <b></b><br> 2181 * </p> 2182 */ 2183 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2184 SP_CONTEXT_TYPE_VALUE); 2185 2186 /** 2187 * Search parameter: <b>kind</b> 2188 * <p> 2189 * Description: <b>codesystem | identifier | root</b><br> 2190 * Type: <b>token</b><br> 2191 * Path: <b>NamingSystem.kind</b><br> 2192 * </p> 2193 */ 2194 @SearchParamDefinition(name = "kind", path = "NamingSystem.kind", description = "codesystem | identifier | root", type = "token") 2195 public static final String SP_KIND = "kind"; 2196 /** 2197 * <b>Fluent Client</b> search parameter constant for <b>kind</b> 2198 * <p> 2199 * Description: <b>codesystem | identifier | root</b><br> 2200 * Type: <b>token</b><br> 2201 * Path: <b>NamingSystem.kind</b><br> 2202 * </p> 2203 */ 2204 public static final ca.uhn.fhir.rest.gclient.TokenClientParam KIND = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2205 SP_KIND); 2206 2207 /** 2208 * Search parameter: <b>jurisdiction</b> 2209 * <p> 2210 * Description: <b>Intended jurisdiction for the naming system</b><br> 2211 * Type: <b>token</b><br> 2212 * Path: <b>NamingSystem.jurisdiction</b><br> 2213 * </p> 2214 */ 2215 @SearchParamDefinition(name = "jurisdiction", path = "NamingSystem.jurisdiction", description = "Intended jurisdiction for the naming system", type = "token") 2216 public static final String SP_JURISDICTION = "jurisdiction"; 2217 /** 2218 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2219 * <p> 2220 * Description: <b>Intended jurisdiction for the naming system</b><br> 2221 * Type: <b>token</b><br> 2222 * Path: <b>NamingSystem.jurisdiction</b><br> 2223 * </p> 2224 */ 2225 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2226 SP_JURISDICTION); 2227 2228 /** 2229 * Search parameter: <b>description</b> 2230 * <p> 2231 * Description: <b>The description of the naming system</b><br> 2232 * Type: <b>string</b><br> 2233 * Path: <b>NamingSystem.description</b><br> 2234 * </p> 2235 */ 2236 @SearchParamDefinition(name = "description", path = "NamingSystem.description", description = "The description of the naming system", type = "string") 2237 public static final String SP_DESCRIPTION = "description"; 2238 /** 2239 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2240 * <p> 2241 * Description: <b>The description of the naming system</b><br> 2242 * Type: <b>string</b><br> 2243 * Path: <b>NamingSystem.description</b><br> 2244 * </p> 2245 */ 2246 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2247 SP_DESCRIPTION); 2248 2249 /** 2250 * Search parameter: <b>context-type</b> 2251 * <p> 2252 * Description: <b>A type of use context assigned to the naming system</b><br> 2253 * Type: <b>token</b><br> 2254 * Path: <b>NamingSystem.useContext.code</b><br> 2255 * </p> 2256 */ 2257 @SearchParamDefinition(name = "context-type", path = "NamingSystem.useContext.code", description = "A type of use context assigned to the naming system", type = "token") 2258 public static final String SP_CONTEXT_TYPE = "context-type"; 2259 /** 2260 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2261 * <p> 2262 * Description: <b>A type of use context assigned to the naming system</b><br> 2263 * Type: <b>token</b><br> 2264 * Path: <b>NamingSystem.useContext.code</b><br> 2265 * </p> 2266 */ 2267 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2268 SP_CONTEXT_TYPE); 2269 2270 /** 2271 * Search parameter: <b>type</b> 2272 * <p> 2273 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 2274 * Type: <b>token</b><br> 2275 * Path: <b>NamingSystem.type</b><br> 2276 * </p> 2277 */ 2278 @SearchParamDefinition(name = "type", path = "NamingSystem.type", description = "e.g. driver, provider, patient, bank etc.", type = "token") 2279 public static final String SP_TYPE = "type"; 2280 /** 2281 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2282 * <p> 2283 * Description: <b>e.g. driver, provider, patient, bank etc.</b><br> 2284 * Type: <b>token</b><br> 2285 * Path: <b>NamingSystem.type</b><br> 2286 * </p> 2287 */ 2288 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2289 SP_TYPE); 2290 2291 /** 2292 * Search parameter: <b>id-type</b> 2293 * <p> 2294 * Description: <b>oid | uuid | uri | other</b><br> 2295 * Type: <b>token</b><br> 2296 * Path: <b>NamingSystem.uniqueId.type</b><br> 2297 * </p> 2298 */ 2299 @SearchParamDefinition(name = "id-type", path = "NamingSystem.uniqueId.type", description = "oid | uuid | uri | other", type = "token") 2300 public static final String SP_ID_TYPE = "id-type"; 2301 /** 2302 * <b>Fluent Client</b> search parameter constant for <b>id-type</b> 2303 * <p> 2304 * Description: <b>oid | uuid | uri | other</b><br> 2305 * Type: <b>token</b><br> 2306 * Path: <b>NamingSystem.uniqueId.type</b><br> 2307 * </p> 2308 */ 2309 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ID_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2310 SP_ID_TYPE); 2311 2312 /** 2313 * Search parameter: <b>context-quantity</b> 2314 * <p> 2315 * Description: <b>A quantity- or range-valued use context assigned to the 2316 * naming system</b><br> 2317 * Type: <b>quantity</b><br> 2318 * Path: <b>NamingSystem.useContext.valueQuantity, 2319 * NamingSystem.useContext.valueRange</b><br> 2320 * </p> 2321 */ 2322 @SearchParamDefinition(name = "context-quantity", path = "(NamingSystem.useContext.value as Quantity) | (NamingSystem.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the naming system", type = "quantity") 2323 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2324 /** 2325 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2326 * <p> 2327 * Description: <b>A quantity- or range-valued use context assigned to the 2328 * naming system</b><br> 2329 * Type: <b>quantity</b><br> 2330 * Path: <b>NamingSystem.useContext.valueQuantity, 2331 * NamingSystem.useContext.valueRange</b><br> 2332 * </p> 2333 */ 2334 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 2335 SP_CONTEXT_QUANTITY); 2336 2337 /** 2338 * Search parameter: <b>responsible</b> 2339 * <p> 2340 * Description: <b>Who maintains system namespace?</b><br> 2341 * Type: <b>string</b><br> 2342 * Path: <b>NamingSystem.responsible</b><br> 2343 * </p> 2344 */ 2345 @SearchParamDefinition(name = "responsible", path = "NamingSystem.responsible", description = "Who maintains system namespace?", type = "string") 2346 public static final String SP_RESPONSIBLE = "responsible"; 2347 /** 2348 * <b>Fluent Client</b> search parameter constant for <b>responsible</b> 2349 * <p> 2350 * Description: <b>Who maintains system namespace?</b><br> 2351 * Type: <b>string</b><br> 2352 * Path: <b>NamingSystem.responsible</b><br> 2353 * </p> 2354 */ 2355 public static final ca.uhn.fhir.rest.gclient.StringClientParam RESPONSIBLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2356 SP_RESPONSIBLE); 2357 2358 /** 2359 * Search parameter: <b>contact</b> 2360 * <p> 2361 * Description: <b>Name of an individual to contact</b><br> 2362 * Type: <b>string</b><br> 2363 * Path: <b>NamingSystem.contact.name</b><br> 2364 * </p> 2365 */ 2366 @SearchParamDefinition(name = "contact", path = "NamingSystem.contact.name", description = "Name of an individual to contact", type = "string") 2367 public static final String SP_CONTACT = "contact"; 2368 /** 2369 * <b>Fluent Client</b> search parameter constant for <b>contact</b> 2370 * <p> 2371 * Description: <b>Name of an individual to contact</b><br> 2372 * Type: <b>string</b><br> 2373 * Path: <b>NamingSystem.contact.name</b><br> 2374 * </p> 2375 */ 2376 public static final ca.uhn.fhir.rest.gclient.StringClientParam CONTACT = new ca.uhn.fhir.rest.gclient.StringClientParam( 2377 SP_CONTACT); 2378 2379 /** 2380 * Search parameter: <b>name</b> 2381 * <p> 2382 * Description: <b>Computationally friendly name of the naming system</b><br> 2383 * Type: <b>string</b><br> 2384 * Path: <b>NamingSystem.name</b><br> 2385 * </p> 2386 */ 2387 @SearchParamDefinition(name = "name", path = "NamingSystem.name", description = "Computationally friendly name of the naming system", type = "string") 2388 public static final String SP_NAME = "name"; 2389 /** 2390 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2391 * <p> 2392 * Description: <b>Computationally friendly name of the naming system</b><br> 2393 * Type: <b>string</b><br> 2394 * Path: <b>NamingSystem.name</b><br> 2395 * </p> 2396 */ 2397 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 2398 SP_NAME); 2399 2400 /** 2401 * Search parameter: <b>context</b> 2402 * <p> 2403 * Description: <b>A use context assigned to the naming system</b><br> 2404 * Type: <b>token</b><br> 2405 * Path: <b>NamingSystem.useContext.valueCodeableConcept</b><br> 2406 * </p> 2407 */ 2408 @SearchParamDefinition(name = "context", path = "(NamingSystem.useContext.value as CodeableConcept)", description = "A use context assigned to the naming system", type = "token") 2409 public static final String SP_CONTEXT = "context"; 2410 /** 2411 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2412 * <p> 2413 * Description: <b>A use context assigned to the naming system</b><br> 2414 * Type: <b>token</b><br> 2415 * Path: <b>NamingSystem.useContext.valueCodeableConcept</b><br> 2416 * </p> 2417 */ 2418 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2419 SP_CONTEXT); 2420 2421 /** 2422 * Search parameter: <b>publisher</b> 2423 * <p> 2424 * Description: <b>Name of the publisher of the naming system</b><br> 2425 * Type: <b>string</b><br> 2426 * Path: <b>NamingSystem.publisher</b><br> 2427 * </p> 2428 */ 2429 @SearchParamDefinition(name = "publisher", path = "NamingSystem.publisher", description = "Name of the publisher of the naming system", type = "string") 2430 public static final String SP_PUBLISHER = "publisher"; 2431 /** 2432 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2433 * <p> 2434 * Description: <b>Name of the publisher of the naming system</b><br> 2435 * Type: <b>string</b><br> 2436 * Path: <b>NamingSystem.publisher</b><br> 2437 * </p> 2438 */ 2439 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 2440 SP_PUBLISHER); 2441 2442 /** 2443 * Search parameter: <b>telecom</b> 2444 * <p> 2445 * Description: <b>Contact details for individual or organization</b><br> 2446 * Type: <b>token</b><br> 2447 * Path: <b>NamingSystem.contact.telecom</b><br> 2448 * </p> 2449 */ 2450 @SearchParamDefinition(name = "telecom", path = "NamingSystem.contact.telecom", description = "Contact details for individual or organization", type = "token") 2451 public static final String SP_TELECOM = "telecom"; 2452 /** 2453 * <b>Fluent Client</b> search parameter constant for <b>telecom</b> 2454 * <p> 2455 * Description: <b>Contact details for individual or organization</b><br> 2456 * Type: <b>token</b><br> 2457 * Path: <b>NamingSystem.contact.telecom</b><br> 2458 * </p> 2459 */ 2460 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TELECOM = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2461 SP_TELECOM); 2462 2463 /** 2464 * Search parameter: <b>value</b> 2465 * <p> 2466 * Description: <b>The unique identifier</b><br> 2467 * Type: <b>string</b><br> 2468 * Path: <b>NamingSystem.uniqueId.value</b><br> 2469 * </p> 2470 */ 2471 @SearchParamDefinition(name = "value", path = "NamingSystem.uniqueId.value", description = "The unique identifier", type = "string") 2472 public static final String SP_VALUE = "value"; 2473 /** 2474 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2475 * <p> 2476 * Description: <b>The unique identifier</b><br> 2477 * Type: <b>string</b><br> 2478 * Path: <b>NamingSystem.uniqueId.value</b><br> 2479 * </p> 2480 */ 2481 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2482 SP_VALUE); 2483 2484 /** 2485 * Search parameter: <b>context-type-quantity</b> 2486 * <p> 2487 * Description: <b>A use context type and quantity- or range-based value 2488 * assigned to the naming system</b><br> 2489 * Type: <b>composite</b><br> 2490 * Path: <b></b><br> 2491 * </p> 2492 */ 2493 @SearchParamDefinition(name = "context-type-quantity", path = "NamingSystem.useContext", description = "A use context type and quantity- or range-based value assigned to the naming system", type = "composite", compositeOf = { 2494 "context-type", "context-quantity" }) 2495 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2496 /** 2497 * <b>Fluent Client</b> search parameter constant for 2498 * <b>context-type-quantity</b> 2499 * <p> 2500 * Description: <b>A use context type and quantity- or range-based value 2501 * assigned to the naming system</b><br> 2502 * Type: <b>composite</b><br> 2503 * Path: <b></b><br> 2504 * </p> 2505 */ 2506 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 2507 SP_CONTEXT_TYPE_QUANTITY); 2508 2509 /** 2510 * Search parameter: <b>status</b> 2511 * <p> 2512 * Description: <b>The current status of the naming system</b><br> 2513 * Type: <b>token</b><br> 2514 * Path: <b>NamingSystem.status</b><br> 2515 * </p> 2516 */ 2517 @SearchParamDefinition(name = "status", path = "NamingSystem.status", description = "The current status of the naming system", type = "token") 2518 public static final String SP_STATUS = "status"; 2519 /** 2520 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2521 * <p> 2522 * Description: <b>The current status of the naming system</b><br> 2523 * Type: <b>token</b><br> 2524 * Path: <b>NamingSystem.status</b><br> 2525 * </p> 2526 */ 2527 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2528 SP_STATUS); 2529 2530}