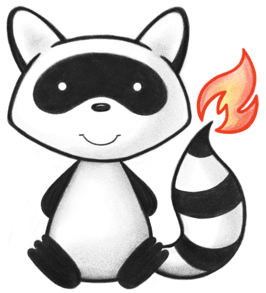
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.List; 034 035import org.hl7.fhir.exceptions.FHIRException; 036import org.hl7.fhir.instance.model.api.INarrative; 037import org.hl7.fhir.utilities.xhtml.NodeType; 038import org.hl7.fhir.utilities.xhtml.XhtmlNode; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * A human-readable summary of the resource conveying the essential clinical and 046 * business information for the resource. 047 */ 048@DatatypeDef(name = "Narrative") 049public class Narrative extends BaseNarrative implements INarrative { 050 051 public enum NarrativeStatus { 052 /** 053 * The contents of the narrative are entirely generated from the core elements 054 * in the content. 055 */ 056 GENERATED, 057 /** 058 * The contents of the narrative are entirely generated from the core elements 059 * in the content and some of the content is generated from extensions. The 060 * narrative SHALL reflect the impact of all modifier extensions. 061 */ 062 EXTENSIONS, 063 /** 064 * The contents of the narrative may contain additional information not found in 065 * the structured data. Note that there is no computable way to determine what 066 * the extra information is, other than by human inspection. 067 */ 068 ADDITIONAL, 069 /** 070 * The contents of the narrative are some equivalent of "No human-readable text 071 * provided in this case". 072 */ 073 EMPTY, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 079 public static NarrativeStatus fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("generated".equals(codeString)) 083 return GENERATED; 084 if ("extensions".equals(codeString)) 085 return EXTENSIONS; 086 if ("additional".equals(codeString)) 087 return ADDITIONAL; 088 if ("empty".equals(codeString)) 089 return EMPTY; 090 if (Configuration.isAcceptInvalidEnums()) 091 return null; 092 else 093 throw new FHIRException("Unknown NarrativeStatus code '" + codeString + "'"); 094 } 095 096 public String toCode() { 097 switch (this) { 098 case GENERATED: 099 return "generated"; 100 case EXTENSIONS: 101 return "extensions"; 102 case ADDITIONAL: 103 return "additional"; 104 case EMPTY: 105 return "empty"; 106 case NULL: 107 return null; 108 default: 109 return "?"; 110 } 111 } 112 113 public String getSystem() { 114 switch (this) { 115 case GENERATED: 116 return "http://hl7.org/fhir/narrative-status"; 117 case EXTENSIONS: 118 return "http://hl7.org/fhir/narrative-status"; 119 case ADDITIONAL: 120 return "http://hl7.org/fhir/narrative-status"; 121 case EMPTY: 122 return "http://hl7.org/fhir/narrative-status"; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDefinition() { 131 switch (this) { 132 case GENERATED: 133 return "The contents of the narrative are entirely generated from the core elements in the content."; 134 case EXTENSIONS: 135 return "The contents of the narrative are entirely generated from the core elements in the content and some of the content is generated from extensions. The narrative SHALL reflect the impact of all modifier extensions."; 136 case ADDITIONAL: 137 return "The contents of the narrative may contain additional information not found in the structured data. Note that there is no computable way to determine what the extra information is, other than by human inspection."; 138 case EMPTY: 139 return "The contents of the narrative are some equivalent of \"No human-readable text provided in this case\"."; 140 case NULL: 141 return null; 142 default: 143 return "?"; 144 } 145 } 146 147 public String getDisplay() { 148 switch (this) { 149 case GENERATED: 150 return "Generated"; 151 case EXTENSIONS: 152 return "Extensions"; 153 case ADDITIONAL: 154 return "Additional"; 155 case EMPTY: 156 return "Empty"; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 } 164 165 public static class NarrativeStatusEnumFactory implements EnumFactory<NarrativeStatus> { 166 public NarrativeStatus fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("generated".equals(codeString)) 171 return NarrativeStatus.GENERATED; 172 if ("extensions".equals(codeString)) 173 return NarrativeStatus.EXTENSIONS; 174 if ("additional".equals(codeString)) 175 return NarrativeStatus.ADDITIONAL; 176 if ("empty".equals(codeString)) 177 return NarrativeStatus.EMPTY; 178 throw new IllegalArgumentException("Unknown NarrativeStatus code '" + codeString + "'"); 179 } 180 181 public Enumeration<NarrativeStatus> fromType(PrimitiveType<?> code) throws FHIRException { 182 if (code == null) 183 return null; 184 if (code.isEmpty()) 185 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.NULL, code); 186 String codeString = code.asStringValue(); 187 if (codeString == null || "".equals(codeString)) 188 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.NULL, code); 189 if ("generated".equals(codeString)) 190 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.GENERATED, code); 191 if ("extensions".equals(codeString)) 192 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EXTENSIONS, code); 193 if ("additional".equals(codeString)) 194 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.ADDITIONAL, code); 195 if ("empty".equals(codeString)) 196 return new Enumeration<NarrativeStatus>(this, NarrativeStatus.EMPTY, code); 197 throw new FHIRException("Unknown NarrativeStatus code '" + codeString + "'"); 198 } 199 200 public String toCode(NarrativeStatus code) { 201 if (code == NarrativeStatus.NULL) 202 return null; 203 if (code == NarrativeStatus.GENERATED) 204 return "generated"; 205 if (code == NarrativeStatus.EXTENSIONS) 206 return "extensions"; 207 if (code == NarrativeStatus.ADDITIONAL) 208 return "additional"; 209 if (code == NarrativeStatus.EMPTY) 210 return "empty"; 211 return "?"; 212 } 213 214 public String toSystem(NarrativeStatus code) { 215 return code.getSystem(); 216 } 217 } 218 219 /** 220 * The status of the narrative - whether it's entirely generated (from just the 221 * defined data or the extensions too), or whether a human authored it and it 222 * may contain additional data. 223 */ 224 @Child(name = "status", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = false) 225 @Description(shortDefinition = "generated | extensions | additional | empty", formalDefinition = "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.") 226 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/narrative-status") 227 protected Enumeration<NarrativeStatus> status; 228 229 /** 230 * The actual narrative content, a stripped down version of XHTML. 231 */ 232 @Child(name = "div", type = {}, order = 1, min = 1, max = 1, modifier = false, summary = false) 233 @Description(shortDefinition = "Limited xhtml content", formalDefinition = "The actual narrative content, a stripped down version of XHTML.") 234 protected XhtmlNode div; 235 236 private static final long serialVersionUID = 1463852859L; 237 238 /** 239 * Constructor 240 */ 241 public Narrative() { 242 super(); 243 } 244 245 /** 246 * Constructor 247 */ 248 public Narrative(Enumeration<NarrativeStatus> status, XhtmlNode div) { 249 super(); 250 this.status = status; 251 this.div = div; 252 } 253 254 /** 255 * @return {@link #status} (The status of the narrative - whether it's entirely 256 * generated (from just the defined data or the extensions too), or 257 * whether a human authored it and it may contain additional data.). 258 * This is the underlying object with id, value and extensions. The 259 * accessor "getStatus" gives direct access to the value 260 */ 261 public Enumeration<NarrativeStatus> getStatusElement() { 262 if (this.status == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create Narrative.status"); 265 else if (Configuration.doAutoCreate()) 266 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); // bb 267 return this.status; 268 } 269 270 public boolean hasStatusElement() { 271 return this.status != null && !this.status.isEmpty(); 272 } 273 274 public boolean hasStatus() { 275 return this.status != null && !this.status.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #status} (The status of the narrative - whether it's 280 * entirely generated (from just the defined data or the extensions 281 * too), or whether a human authored it and it may contain 282 * additional data.). This is the underlying object with id, value 283 * and extensions. The accessor "getStatus" gives direct access to 284 * the value 285 */ 286 public Narrative setStatusElement(Enumeration<NarrativeStatus> value) { 287 this.status = value; 288 return this; 289 } 290 291 /** 292 * @return The status of the narrative - whether it's entirely generated (from 293 * just the defined data or the extensions too), or whether a human 294 * authored it and it may contain additional data. 295 */ 296 public NarrativeStatus getStatus() { 297 return this.status == null ? null : this.status.getValue(); 298 } 299 300 /** 301 * @param value The status of the narrative - whether it's entirely generated 302 * (from just the defined data or the extensions too), or whether a 303 * human authored it and it may contain additional data. 304 */ 305 public Narrative setStatus(NarrativeStatus value) { 306 if (this.status == null) 307 this.status = new Enumeration<NarrativeStatus>(new NarrativeStatusEnumFactory()); 308 this.status.setValue(value); 309 return this; 310 } 311 312 /** 313 * @return {@link #div} (The actual narrative content, a stripped down version 314 * of XHTML.) 315 */ 316 public XhtmlNode getDiv() { 317 if (this.div == null) 318 if (Configuration.errorOnAutoCreate()) 319 throw new Error("Attempt to auto-create Narrative.div"); 320 else if (Configuration.doAutoCreate()) 321 this.div = new XhtmlNode(NodeType.Element, "div"); // cc 322 return this.div; 323 } 324 325 public boolean hasDiv() { 326 return this.div != null && !this.div.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #div} (The actual narrative content, a stripped down 331 * version of XHTML.) 332 */ 333 public Narrative setDiv(XhtmlNode value) { 334 this.div = value; 335 return this; 336 } 337 338 protected void listChildren(List<Property> children) { 339 super.listChildren(children); 340 children.add(new Property("status", "code", 341 "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 342 0, 1, status)); 343 children.add(new Property("div", "xhtml", "The actual narrative content, a stripped down version of XHTML", 0, 1, new XhtmlType(this))); 344 } 345 346 @Override 347 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 348 switch (_hash) { 349 case -892481550: 350 /* status */ return new Property("status", "code", 351 "The status of the narrative - whether it's entirely generated (from just the defined data or the extensions too), or whether a human authored it and it may contain additional data.", 352 0, 1, status); 353 default: 354 return super.getNamedProperty(_hash, _name, _checkValid); 355 } 356 357 } 358 359 @Override 360 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 361 switch (hash) { 362 case -892481550: 363 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<NarrativeStatus> 364 case 99473: 365 /* div */ return this.div == null ? new Base[0] 366 : new Base[] { new StringType(new org.hl7.fhir.utilities.xhtml.XhtmlComposer(true).composeEx(this.div)) }; // XhtmlNode 367 default: 368 return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case -892481550: // status 377 value = new NarrativeStatusEnumFactory().fromType(castToCode(value)); 378 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 379 return value; 380 case 99473: // div 381 this.div = castToXhtml(value); // XhtmlNode 382 return value; 383 default: 384 return super.setProperty(hash, name, value); 385 } 386 387 } 388 389 @Override 390 public Base setProperty(String name, Base value) throws FHIRException { 391 if (name.equals("status")) { 392 value = new NarrativeStatusEnumFactory().fromType(castToCode(value)); 393 this.status = (Enumeration) value; // Enumeration<NarrativeStatus> 394 } else if (name.equals("div")) { 395 this.div = castToXhtml(value); // XhtmlNode 396 } else 397 return super.setProperty(name, value); 398 return value; 399 } 400 401 @Override 402 public void removeChild(String name, Base value) throws FHIRException { 403 if (name.equals("status")) { 404 this.status = null; 405 } else if (name.equals("div")) { 406 this.div = null; 407 } else 408 super.removeChild(name, value); 409 410 } 411 412 @Override 413 public Base makeProperty(int hash, String name) throws FHIRException { 414 switch (hash) { 415 case -892481550: 416 return getStatusElement(); 417 case 99473: /* div */ 418 if (div == null) 419 div = new XhtmlNode(NodeType.Element, "div"); 420 return new StringType(new org.hl7.fhir.utilities.xhtml.XhtmlComposer(true).composeEx(this.div)); 421 default: 422 return super.makeProperty(hash, name); 423 } 424 425 } 426 427 @Override 428 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 429 switch (hash) { 430 case -892481550: 431 /* status */ return new String[] { "code" }; 432 case 99473: 433 /* div */ return new String[] { "xhtml" }; 434 default: 435 return super.getTypesForProperty(hash, name); 436 } 437 438 } 439 440 @Override 441 public Base addChild(String name) throws FHIRException { 442 if (name.equals("status")) { 443 throw new FHIRException("Cannot call addChild on a singleton property Narrative.status"); 444 } else 445 return super.addChild(name); 446 } 447 448 public String fhirType() { 449 return "Narrative"; 450 451 } 452 453 public Narrative copy() { 454 Narrative dst = new Narrative(); 455 copyValues(dst); 456 return dst; 457 } 458 459 public void copyValues(Narrative dst) { 460 super.copyValues(dst); 461 dst.status = status == null ? null : status.copy(); 462 dst.div = div == null ? null : div.copy(); 463 } 464 465 protected Narrative typedCopy() { 466 return copy(); 467 } 468 469 @Override 470 public boolean equalsDeep(Base other_) { 471 if (!super.equalsDeep(other_)) 472 return false; 473 if (!(other_ instanceof Narrative)) 474 return false; 475 Narrative o = (Narrative) other_; 476 return compareDeep(status, o.status, true) && compareDeep(div, o.div, true); 477 } 478 479 @Override 480 public boolean equalsShallow(Base other_) { 481 if (!super.equalsShallow(other_)) 482 return false; 483 if (!(other_ instanceof Narrative)) 484 return false; 485 Narrative o = (Narrative) other_; 486 return compareValues(status, o.status, true); 487 } 488 489 public boolean isEmpty() { 490 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, div); 491 } 492 493}