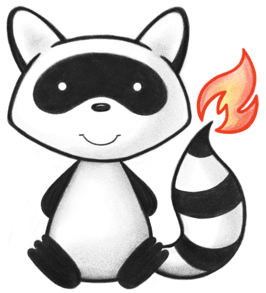
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046 047/** 048 * Measurements and simple assertions made about a patient, device or other 049 * subject. 050 */ 051@ResourceDef(name = "Observation", profile = "http://hl7.org/fhir/StructureDefinition/Observation") 052public class Observation extends DomainResource { 053 054 public enum ObservationStatus { 055 /** 056 * The existence of the observation is registered, but there is no result yet 057 * available. 058 */ 059 REGISTERED, 060 /** 061 * This is an initial or interim observation: data may be incomplete or 062 * unverified. 063 */ 064 PRELIMINARY, 065 /** 066 * The observation is complete and there are no further actions needed. 067 * Additional information such "released", "signed", etc would be represented 068 * using [Provenance](provenance.html) which provides not only the act but also 069 * the actors and dates and other related data. These act states would be 070 * associated with an observation status of `preliminary` until they are all 071 * completed and then a status of `final` would be applied. 072 */ 073 FINAL, 074 /** 075 * Subsequent to being Final, the observation has been modified subsequent. This 076 * includes updates/new information and corrections. 077 */ 078 AMENDED, 079 /** 080 * Subsequent to being Final, the observation has been modified to correct an 081 * error in the test result. 082 */ 083 CORRECTED, 084 /** 085 * The observation is unavailable because the measurement was not started or not 086 * completed (also sometimes called "aborted"). 087 */ 088 CANCELLED, 089 /** 090 * The observation has been withdrawn following previous final release. This 091 * electronic record should never have existed, though it is possible that 092 * real-world decisions were based on it. (If real-world activity has occurred, 093 * the status should be "cancelled" rather than "entered-in-error".). 094 */ 095 ENTEREDINERROR, 096 /** 097 * The authoring/source system does not know which of the status values 098 * currently applies for this observation. Note: This concept is not to be used 099 * for "other" - one of the listed statuses is presumed to apply, but the 100 * authoring/source system does not know which. 101 */ 102 UNKNOWN, 103 /** 104 * added to help the parsers with the generic types 105 */ 106 NULL; 107 108 public static ObservationStatus fromCode(String codeString) throws FHIRException { 109 if (codeString == null || "".equals(codeString)) 110 return null; 111 if ("registered".equals(codeString)) 112 return REGISTERED; 113 if ("preliminary".equals(codeString)) 114 return PRELIMINARY; 115 if ("final".equals(codeString)) 116 return FINAL; 117 if ("amended".equals(codeString)) 118 return AMENDED; 119 if ("corrected".equals(codeString)) 120 return CORRECTED; 121 if ("cancelled".equals(codeString)) 122 return CANCELLED; 123 if ("entered-in-error".equals(codeString)) 124 return ENTEREDINERROR; 125 if ("unknown".equals(codeString)) 126 return UNKNOWN; 127 if (Configuration.isAcceptInvalidEnums()) 128 return null; 129 else 130 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 131 } 132 133 public String toCode() { 134 switch (this) { 135 case REGISTERED: 136 return "registered"; 137 case PRELIMINARY: 138 return "preliminary"; 139 case FINAL: 140 return "final"; 141 case AMENDED: 142 return "amended"; 143 case CORRECTED: 144 return "corrected"; 145 case CANCELLED: 146 return "cancelled"; 147 case ENTEREDINERROR: 148 return "entered-in-error"; 149 case UNKNOWN: 150 return "unknown"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getSystem() { 159 switch (this) { 160 case REGISTERED: 161 return "http://hl7.org/fhir/observation-status"; 162 case PRELIMINARY: 163 return "http://hl7.org/fhir/observation-status"; 164 case FINAL: 165 return "http://hl7.org/fhir/observation-status"; 166 case AMENDED: 167 return "http://hl7.org/fhir/observation-status"; 168 case CORRECTED: 169 return "http://hl7.org/fhir/observation-status"; 170 case CANCELLED: 171 return "http://hl7.org/fhir/observation-status"; 172 case ENTEREDINERROR: 173 return "http://hl7.org/fhir/observation-status"; 174 case UNKNOWN: 175 return "http://hl7.org/fhir/observation-status"; 176 case NULL: 177 return null; 178 default: 179 return "?"; 180 } 181 } 182 183 public String getDefinition() { 184 switch (this) { 185 case REGISTERED: 186 return "The existence of the observation is registered, but there is no result yet available."; 187 case PRELIMINARY: 188 return "This is an initial or interim observation: data may be incomplete or unverified."; 189 case FINAL: 190 return "The observation is complete and there are no further actions needed. Additional information such \"released\", \"signed\", etc would be represented using [Provenance](provenance.html) which provides not only the act but also the actors and dates and other related data. These act states would be associated with an observation status of `preliminary` until they are all completed and then a status of `final` would be applied."; 191 case AMENDED: 192 return "Subsequent to being Final, the observation has been modified subsequent. This includes updates/new information and corrections."; 193 case CORRECTED: 194 return "Subsequent to being Final, the observation has been modified to correct an error in the test result."; 195 case CANCELLED: 196 return "The observation is unavailable because the measurement was not started or not completed (also sometimes called \"aborted\")."; 197 case ENTEREDINERROR: 198 return "The observation has been withdrawn following previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 199 case UNKNOWN: 200 return "The authoring/source system does not know which of the status values currently applies for this observation. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, but the authoring/source system does not know which."; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getDisplay() { 209 switch (this) { 210 case REGISTERED: 211 return "Registered"; 212 case PRELIMINARY: 213 return "Preliminary"; 214 case FINAL: 215 return "Final"; 216 case AMENDED: 217 return "Amended"; 218 case CORRECTED: 219 return "Corrected"; 220 case CANCELLED: 221 return "Cancelled"; 222 case ENTEREDINERROR: 223 return "Entered in Error"; 224 case UNKNOWN: 225 return "Unknown"; 226 case NULL: 227 return null; 228 default: 229 return "?"; 230 } 231 } 232 } 233 234 public static class ObservationStatusEnumFactory implements EnumFactory<ObservationStatus> { 235 public ObservationStatus fromCode(String codeString) throws IllegalArgumentException { 236 if (codeString == null || "".equals(codeString)) 237 if (codeString == null || "".equals(codeString)) 238 return null; 239 if ("registered".equals(codeString)) 240 return ObservationStatus.REGISTERED; 241 if ("preliminary".equals(codeString)) 242 return ObservationStatus.PRELIMINARY; 243 if ("final".equals(codeString)) 244 return ObservationStatus.FINAL; 245 if ("amended".equals(codeString)) 246 return ObservationStatus.AMENDED; 247 if ("corrected".equals(codeString)) 248 return ObservationStatus.CORRECTED; 249 if ("cancelled".equals(codeString)) 250 return ObservationStatus.CANCELLED; 251 if ("entered-in-error".equals(codeString)) 252 return ObservationStatus.ENTEREDINERROR; 253 if ("unknown".equals(codeString)) 254 return ObservationStatus.UNKNOWN; 255 throw new IllegalArgumentException("Unknown ObservationStatus code '" + codeString + "'"); 256 } 257 258 public Enumeration<ObservationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 259 if (code == null) 260 return null; 261 if (code.isEmpty()) 262 return new Enumeration<ObservationStatus>(this, ObservationStatus.NULL, code); 263 String codeString = code.asStringValue(); 264 if (codeString == null || "".equals(codeString)) 265 return new Enumeration<ObservationStatus>(this, ObservationStatus.NULL, code); 266 if ("registered".equals(codeString)) 267 return new Enumeration<ObservationStatus>(this, ObservationStatus.REGISTERED, code); 268 if ("preliminary".equals(codeString)) 269 return new Enumeration<ObservationStatus>(this, ObservationStatus.PRELIMINARY, code); 270 if ("final".equals(codeString)) 271 return new Enumeration<ObservationStatus>(this, ObservationStatus.FINAL, code); 272 if ("amended".equals(codeString)) 273 return new Enumeration<ObservationStatus>(this, ObservationStatus.AMENDED, code); 274 if ("corrected".equals(codeString)) 275 return new Enumeration<ObservationStatus>(this, ObservationStatus.CORRECTED, code); 276 if ("cancelled".equals(codeString)) 277 return new Enumeration<ObservationStatus>(this, ObservationStatus.CANCELLED, code); 278 if ("entered-in-error".equals(codeString)) 279 return new Enumeration<ObservationStatus>(this, ObservationStatus.ENTEREDINERROR, code); 280 if ("unknown".equals(codeString)) 281 return new Enumeration<ObservationStatus>(this, ObservationStatus.UNKNOWN, code); 282 throw new FHIRException("Unknown ObservationStatus code '" + codeString + "'"); 283 } 284 285 public String toCode(ObservationStatus code) { 286 if (code == ObservationStatus.NULL) 287 return null; 288 if (code == ObservationStatus.REGISTERED) 289 return "registered"; 290 if (code == ObservationStatus.PRELIMINARY) 291 return "preliminary"; 292 if (code == ObservationStatus.FINAL) 293 return "final"; 294 if (code == ObservationStatus.AMENDED) 295 return "amended"; 296 if (code == ObservationStatus.CORRECTED) 297 return "corrected"; 298 if (code == ObservationStatus.CANCELLED) 299 return "cancelled"; 300 if (code == ObservationStatus.ENTEREDINERROR) 301 return "entered-in-error"; 302 if (code == ObservationStatus.UNKNOWN) 303 return "unknown"; 304 return "?"; 305 } 306 307 public String toSystem(ObservationStatus code) { 308 return code.getSystem(); 309 } 310 } 311 312 @Block() 313 public static class ObservationReferenceRangeComponent extends BackboneElement implements IBaseBackboneElement { 314 /** 315 * The value of the low bound of the reference range. The low bound of the 316 * reference range endpoint is inclusive of the value (e.g. reference range is 317 * >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless 318 * (e.g. reference range is <=2.3). 319 */ 320 @Child(name = "low", type = { Quantity.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 321 @Description(shortDefinition = "Low Range, if relevant", formalDefinition = "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).") 322 protected Quantity low; 323 324 /** 325 * The value of the high bound of the reference range. The high bound of the 326 * reference range endpoint is inclusive of the value (e.g. reference range is 327 * >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless 328 * (e.g. reference range is >= 2.3). 329 */ 330 @Child(name = "high", type = { Quantity.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 331 @Description(shortDefinition = "High Range, if relevant", formalDefinition = "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).") 332 protected Quantity high; 333 334 /** 335 * Codes to indicate the what part of the targeted reference population it 336 * applies to. For example, the normal or therapeutic range. 337 */ 338 @Child(name = "type", type = { 339 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 340 @Description(shortDefinition = "Reference range qualifier", formalDefinition = "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.") 341 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-meaning") 342 protected CodeableConcept type; 343 344 /** 345 * Codes to indicate the target population this reference range applies to. For 346 * example, a reference range may be based on the normal population or a 347 * particular sex or race. Multiple `appliesTo` are interpreted as an "AND" of 348 * the target populations. For example, to represent a target population of 349 * African American females, both a code of female and a code for African 350 * American would be used. 351 */ 352 @Child(name = "appliesTo", type = { 353 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 354 @Description(shortDefinition = "Reference range population", formalDefinition = "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.") 355 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/referencerange-appliesto") 356 protected List<CodeableConcept> appliesTo; 357 358 /** 359 * The age at which this reference range is applicable. This is a neonatal age 360 * (e.g. number of weeks at term) if the meaning says so. 361 */ 362 @Child(name = "age", type = { Range.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 363 @Description(shortDefinition = "Applicable age range, if relevant", formalDefinition = "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.") 364 protected Range age; 365 366 /** 367 * Text based reference range in an observation which may be used when a 368 * quantitative range is not appropriate for an observation. An example would be 369 * a reference value of "Negative" or a list or table of "normals". 370 */ 371 @Child(name = "text", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 372 @Description(shortDefinition = "Text based reference range in an observation", formalDefinition = "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".") 373 protected StringType text; 374 375 private static final long serialVersionUID = -305128879L; 376 377 /** 378 * Constructor 379 */ 380 public ObservationReferenceRangeComponent() { 381 super(); 382 } 383 384 /** 385 * @return {@link #low} (The value of the low bound of the reference range. The 386 * low bound of the reference range endpoint is inclusive of the value 387 * (e.g. reference range is >=5 - <=9). If the low bound is omitted, it 388 * is assumed to be meaningless (e.g. reference range is <=2.3).) 389 */ 390 public Quantity getLow() { 391 if (this.low == null) 392 if (Configuration.errorOnAutoCreate()) 393 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.low"); 394 else if (Configuration.doAutoCreate()) 395 this.low = new Quantity(); // cc 396 return this.low; 397 } 398 399 public boolean hasLow() { 400 return this.low != null && !this.low.isEmpty(); 401 } 402 403 /** 404 * @param value {@link #low} (The value of the low bound of the reference range. 405 * The low bound of the reference range endpoint is inclusive of 406 * the value (e.g. reference range is >=5 - <=9). If the low bound 407 * is omitted, it is assumed to be meaningless (e.g. reference 408 * range is <=2.3).) 409 */ 410 public ObservationReferenceRangeComponent setLow(Quantity value) { 411 this.low = value; 412 return this; 413 } 414 415 /** 416 * @return {@link #high} (The value of the high bound of the reference range. 417 * The high bound of the reference range endpoint is inclusive of the 418 * value (e.g. reference range is >=5 - <=9). If the high bound is 419 * omitted, it is assumed to be meaningless (e.g. reference range is >= 420 * 2.3).) 421 */ 422 public Quantity getHigh() { 423 if (this.high == null) 424 if (Configuration.errorOnAutoCreate()) 425 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.high"); 426 else if (Configuration.doAutoCreate()) 427 this.high = new Quantity(); // cc 428 return this.high; 429 } 430 431 public boolean hasHigh() { 432 return this.high != null && !this.high.isEmpty(); 433 } 434 435 /** 436 * @param value {@link #high} (The value of the high bound of the reference 437 * range. The high bound of the reference range endpoint is 438 * inclusive of the value (e.g. reference range is >=5 - <=9). If 439 * the high bound is omitted, it is assumed to be meaningless (e.g. 440 * reference range is >= 2.3).) 441 */ 442 public ObservationReferenceRangeComponent setHigh(Quantity value) { 443 this.high = value; 444 return this; 445 } 446 447 /** 448 * @return {@link #type} (Codes to indicate the what part of the targeted 449 * reference population it applies to. For example, the normal or 450 * therapeutic range.) 451 */ 452 public CodeableConcept getType() { 453 if (this.type == null) 454 if (Configuration.errorOnAutoCreate()) 455 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.type"); 456 else if (Configuration.doAutoCreate()) 457 this.type = new CodeableConcept(); // cc 458 return this.type; 459 } 460 461 public boolean hasType() { 462 return this.type != null && !this.type.isEmpty(); 463 } 464 465 /** 466 * @param value {@link #type} (Codes to indicate the what part of the targeted 467 * reference population it applies to. For example, the normal or 468 * therapeutic range.) 469 */ 470 public ObservationReferenceRangeComponent setType(CodeableConcept value) { 471 this.type = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #appliesTo} (Codes to indicate the target population this 477 * reference range applies to. For example, a reference range may be 478 * based on the normal population or a particular sex or race. Multiple 479 * `appliesTo` are interpreted as an "AND" of the target populations. 480 * For example, to represent a target population of African American 481 * females, both a code of female and a code for African American would 482 * be used.) 483 */ 484 public List<CodeableConcept> getAppliesTo() { 485 if (this.appliesTo == null) 486 this.appliesTo = new ArrayList<CodeableConcept>(); 487 return this.appliesTo; 488 } 489 490 /** 491 * @return Returns a reference to <code>this</code> for easy method chaining 492 */ 493 public ObservationReferenceRangeComponent setAppliesTo(List<CodeableConcept> theAppliesTo) { 494 this.appliesTo = theAppliesTo; 495 return this; 496 } 497 498 public boolean hasAppliesTo() { 499 if (this.appliesTo == null) 500 return false; 501 for (CodeableConcept item : this.appliesTo) 502 if (!item.isEmpty()) 503 return true; 504 return false; 505 } 506 507 public CodeableConcept addAppliesTo() { // 3 508 CodeableConcept t = new CodeableConcept(); 509 if (this.appliesTo == null) 510 this.appliesTo = new ArrayList<CodeableConcept>(); 511 this.appliesTo.add(t); 512 return t; 513 } 514 515 public ObservationReferenceRangeComponent addAppliesTo(CodeableConcept t) { // 3 516 if (t == null) 517 return this; 518 if (this.appliesTo == null) 519 this.appliesTo = new ArrayList<CodeableConcept>(); 520 this.appliesTo.add(t); 521 return this; 522 } 523 524 /** 525 * @return The first repetition of repeating field {@link #appliesTo}, creating 526 * it if it does not already exist 527 */ 528 public CodeableConcept getAppliesToFirstRep() { 529 if (getAppliesTo().isEmpty()) { 530 addAppliesTo(); 531 } 532 return getAppliesTo().get(0); 533 } 534 535 /** 536 * @return {@link #age} (The age at which this reference range is applicable. 537 * This is a neonatal age (e.g. number of weeks at term) if the meaning 538 * says so.) 539 */ 540 public Range getAge() { 541 if (this.age == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.age"); 544 else if (Configuration.doAutoCreate()) 545 this.age = new Range(); // cc 546 return this.age; 547 } 548 549 public boolean hasAge() { 550 return this.age != null && !this.age.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #age} (The age at which this reference range is 555 * applicable. This is a neonatal age (e.g. number of weeks at 556 * term) if the meaning says so.) 557 */ 558 public ObservationReferenceRangeComponent setAge(Range value) { 559 this.age = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #text} (Text based reference range in an observation which may 565 * be used when a quantitative range is not appropriate for an 566 * observation. An example would be a reference value of "Negative" or a 567 * list or table of "normals".). This is the underlying object with id, 568 * value and extensions. The accessor "getText" gives direct access to 569 * the value 570 */ 571 public StringType getTextElement() { 572 if (this.text == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create ObservationReferenceRangeComponent.text"); 575 else if (Configuration.doAutoCreate()) 576 this.text = new StringType(); // bb 577 return this.text; 578 } 579 580 public boolean hasTextElement() { 581 return this.text != null && !this.text.isEmpty(); 582 } 583 584 public boolean hasText() { 585 return this.text != null && !this.text.isEmpty(); 586 } 587 588 /** 589 * @param value {@link #text} (Text based reference range in an observation 590 * which may be used when a quantitative range is not appropriate 591 * for an observation. An example would be a reference value of 592 * "Negative" or a list or table of "normals".). This is the 593 * underlying object with id, value and extensions. The accessor 594 * "getText" gives direct access to the value 595 */ 596 public ObservationReferenceRangeComponent setTextElement(StringType value) { 597 this.text = value; 598 return this; 599 } 600 601 /** 602 * @return Text based reference range in an observation which may be used when a 603 * quantitative range is not appropriate for an observation. An example 604 * would be a reference value of "Negative" or a list or table of 605 * "normals". 606 */ 607 public String getText() { 608 return this.text == null ? null : this.text.getValue(); 609 } 610 611 /** 612 * @param value Text based reference range in an observation which may be used 613 * when a quantitative range is not appropriate for an observation. 614 * An example would be a reference value of "Negative" or a list or 615 * table of "normals". 616 */ 617 public ObservationReferenceRangeComponent setText(String value) { 618 if (Utilities.noString(value)) 619 this.text = null; 620 else { 621 if (this.text == null) 622 this.text = new StringType(); 623 this.text.setValue(value); 624 } 625 return this; 626 } 627 628 protected void listChildren(List<Property> children) { 629 super.listChildren(children); 630 children.add(new Property("low", "SimpleQuantity", 631 "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 632 0, 1, low)); 633 children.add(new Property("high", "SimpleQuantity", 634 "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 635 0, 1, high)); 636 children.add(new Property("type", "CodeableConcept", 637 "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 638 0, 1, type)); 639 children.add(new Property("appliesTo", "CodeableConcept", 640 "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 641 0, java.lang.Integer.MAX_VALUE, appliesTo)); 642 children.add(new Property("age", "Range", 643 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 644 0, 1, age)); 645 children.add(new Property("text", "string", 646 "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 647 0, 1, text)); 648 } 649 650 @Override 651 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 652 switch (_hash) { 653 case 107348: 654 /* low */ return new Property("low", "SimpleQuantity", 655 "The value of the low bound of the reference range. The low bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the low bound is omitted, it is assumed to be meaningless (e.g. reference range is <=2.3).", 656 0, 1, low); 657 case 3202466: 658 /* high */ return new Property("high", "SimpleQuantity", 659 "The value of the high bound of the reference range. The high bound of the reference range endpoint is inclusive of the value (e.g. reference range is >=5 - <=9). If the high bound is omitted, it is assumed to be meaningless (e.g. reference range is >= 2.3).", 660 0, 1, high); 661 case 3575610: 662 /* type */ return new Property("type", "CodeableConcept", 663 "Codes to indicate the what part of the targeted reference population it applies to. For example, the normal or therapeutic range.", 664 0, 1, type); 665 case -2089924569: 666 /* appliesTo */ return new Property("appliesTo", "CodeableConcept", 667 "Codes to indicate the target population this reference range applies to. For example, a reference range may be based on the normal population or a particular sex or race. Multiple `appliesTo` are interpreted as an \"AND\" of the target populations. For example, to represent a target population of African American females, both a code of female and a code for African American would be used.", 668 0, java.lang.Integer.MAX_VALUE, appliesTo); 669 case 96511: 670 /* age */ return new Property("age", "Range", 671 "The age at which this reference range is applicable. This is a neonatal age (e.g. number of weeks at term) if the meaning says so.", 672 0, 1, age); 673 case 3556653: 674 /* text */ return new Property("text", "string", 675 "Text based reference range in an observation which may be used when a quantitative range is not appropriate for an observation. An example would be a reference value of \"Negative\" or a list or table of \"normals\".", 676 0, 1, text); 677 default: 678 return super.getNamedProperty(_hash, _name, _checkValid); 679 } 680 681 } 682 683 @Override 684 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 685 switch (hash) { 686 case 107348: 687 /* low */ return this.low == null ? new Base[0] : new Base[] { this.low }; // Quantity 688 case 3202466: 689 /* high */ return this.high == null ? new Base[0] : new Base[] { this.high }; // Quantity 690 case 3575610: 691 /* type */ return this.type == null ? new Base[0] : new Base[] { this.type }; // CodeableConcept 692 case -2089924569: 693 /* appliesTo */ return this.appliesTo == null ? new Base[0] 694 : this.appliesTo.toArray(new Base[this.appliesTo.size()]); // CodeableConcept 695 case 96511: 696 /* age */ return this.age == null ? new Base[0] : new Base[] { this.age }; // Range 697 case 3556653: 698 /* text */ return this.text == null ? new Base[0] : new Base[] { this.text }; // StringType 699 default: 700 return super.getProperty(hash, name, checkValid); 701 } 702 703 } 704 705 @Override 706 public Base setProperty(int hash, String name, Base value) throws FHIRException { 707 switch (hash) { 708 case 107348: // low 709 this.low = castToQuantity(value); // Quantity 710 return value; 711 case 3202466: // high 712 this.high = castToQuantity(value); // Quantity 713 return value; 714 case 3575610: // type 715 this.type = castToCodeableConcept(value); // CodeableConcept 716 return value; 717 case -2089924569: // appliesTo 718 this.getAppliesTo().add(castToCodeableConcept(value)); // CodeableConcept 719 return value; 720 case 96511: // age 721 this.age = castToRange(value); // Range 722 return value; 723 case 3556653: // text 724 this.text = castToString(value); // StringType 725 return value; 726 default: 727 return super.setProperty(hash, name, value); 728 } 729 730 } 731 732 @Override 733 public Base setProperty(String name, Base value) throws FHIRException { 734 if (name.equals("low")) { 735 this.low = castToQuantity(value); // Quantity 736 } else if (name.equals("high")) { 737 this.high = castToQuantity(value); // Quantity 738 } else if (name.equals("type")) { 739 this.type = castToCodeableConcept(value); // CodeableConcept 740 } else if (name.equals("appliesTo")) { 741 this.getAppliesTo().add(castToCodeableConcept(value)); 742 } else if (name.equals("age")) { 743 this.age = castToRange(value); // Range 744 } else if (name.equals("text")) { 745 this.text = castToString(value); // StringType 746 } else 747 return super.setProperty(name, value); 748 return value; 749 } 750 751 @Override 752 public void removeChild(String name, Base value) throws FHIRException { 753 if (name.equals("low")) { 754 this.low = null; 755 } else if (name.equals("high")) { 756 this.high = null; 757 } else if (name.equals("type")) { 758 this.type = null; 759 } else if (name.equals("appliesTo")) { 760 this.getAppliesTo().remove(castToCodeableConcept(value)); 761 } else if (name.equals("age")) { 762 this.age = null; 763 } else if (name.equals("text")) { 764 this.text = null; 765 } else 766 super.removeChild(name, value); 767 768 } 769 770 @Override 771 public Base makeProperty(int hash, String name) throws FHIRException { 772 switch (hash) { 773 case 107348: 774 return getLow(); 775 case 3202466: 776 return getHigh(); 777 case 3575610: 778 return getType(); 779 case -2089924569: 780 return addAppliesTo(); 781 case 96511: 782 return getAge(); 783 case 3556653: 784 return getTextElement(); 785 default: 786 return super.makeProperty(hash, name); 787 } 788 789 } 790 791 @Override 792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 793 switch (hash) { 794 case 107348: 795 /* low */ return new String[] { "SimpleQuantity" }; 796 case 3202466: 797 /* high */ return new String[] { "SimpleQuantity" }; 798 case 3575610: 799 /* type */ return new String[] { "CodeableConcept" }; 800 case -2089924569: 801 /* appliesTo */ return new String[] { "CodeableConcept" }; 802 case 96511: 803 /* age */ return new String[] { "Range" }; 804 case 3556653: 805 /* text */ return new String[] { "string" }; 806 default: 807 return super.getTypesForProperty(hash, name); 808 } 809 810 } 811 812 @Override 813 public Base addChild(String name) throws FHIRException { 814 if (name.equals("low")) { 815 this.low = new Quantity(); 816 return this.low; 817 } else if (name.equals("high")) { 818 this.high = new Quantity(); 819 return this.high; 820 } else if (name.equals("type")) { 821 this.type = new CodeableConcept(); 822 return this.type; 823 } else if (name.equals("appliesTo")) { 824 return addAppliesTo(); 825 } else if (name.equals("age")) { 826 this.age = new Range(); 827 return this.age; 828 } else if (name.equals("text")) { 829 throw new FHIRException("Cannot call addChild on a singleton property Observation.text"); 830 } else 831 return super.addChild(name); 832 } 833 834 public ObservationReferenceRangeComponent copy() { 835 ObservationReferenceRangeComponent dst = new ObservationReferenceRangeComponent(); 836 copyValues(dst); 837 return dst; 838 } 839 840 public void copyValues(ObservationReferenceRangeComponent dst) { 841 super.copyValues(dst); 842 dst.low = low == null ? null : low.copy(); 843 dst.high = high == null ? null : high.copy(); 844 dst.type = type == null ? null : type.copy(); 845 if (appliesTo != null) { 846 dst.appliesTo = new ArrayList<CodeableConcept>(); 847 for (CodeableConcept i : appliesTo) 848 dst.appliesTo.add(i.copy()); 849 } 850 ; 851 dst.age = age == null ? null : age.copy(); 852 dst.text = text == null ? null : text.copy(); 853 } 854 855 @Override 856 public boolean equalsDeep(Base other_) { 857 if (!super.equalsDeep(other_)) 858 return false; 859 if (!(other_ instanceof ObservationReferenceRangeComponent)) 860 return false; 861 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 862 return compareDeep(low, o.low, true) && compareDeep(high, o.high, true) && compareDeep(type, o.type, true) 863 && compareDeep(appliesTo, o.appliesTo, true) && compareDeep(age, o.age, true) 864 && compareDeep(text, o.text, true); 865 } 866 867 @Override 868 public boolean equalsShallow(Base other_) { 869 if (!super.equalsShallow(other_)) 870 return false; 871 if (!(other_ instanceof ObservationReferenceRangeComponent)) 872 return false; 873 ObservationReferenceRangeComponent o = (ObservationReferenceRangeComponent) other_; 874 return compareValues(text, o.text, true); 875 } 876 877 public boolean isEmpty() { 878 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(low, high, type, appliesTo, age, text); 879 } 880 881 public String fhirType() { 882 return "Observation.referenceRange"; 883 884 } 885 886 } 887 888 @Block() 889 public static class ObservationComponentComponent extends BackboneElement implements IBaseBackboneElement { 890 /** 891 * Describes what was observed. Sometimes this is called the observation "code". 892 */ 893 @Child(name = "code", type = { 894 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 895 @Description(shortDefinition = "Type of component observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"code\".") 896 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 897 protected CodeableConcept code; 898 899 /** 900 * The information determined as a result of making the observation, if the 901 * information has a simple value. 902 */ 903 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, 904 IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, 905 Period.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 906 @Description(shortDefinition = "Actual component result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 907 protected Type value; 908 909 /** 910 * Provides a reason why the expected value in the element 911 * Observation.component.value[x] is missing. 912 */ 913 @Child(name = "dataAbsentReason", type = { 914 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 915 @Description(shortDefinition = "Why the component result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.component.value[x] is missing.") 916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/data-absent-reason") 917 protected CodeableConcept dataAbsentReason; 918 919 /** 920 * A categorical assessment of an observation value. For example, high, low, 921 * normal. 922 */ 923 @Child(name = "interpretation", type = { 924 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 925 @Description(shortDefinition = "High, low, normal, etc.", formalDefinition = "A categorical assessment of an observation value. For example, high, low, normal.") 926 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-interpretation") 927 protected List<CodeableConcept> interpretation; 928 929 /** 930 * Guidance on how to interpret the value by comparison to a normal or 931 * recommended range. 932 */ 933 @Child(name = "referenceRange", type = { 934 ObservationReferenceRangeComponent.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 935 @Description(shortDefinition = "Provides guide for interpretation of component result", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range.") 936 protected List<ObservationReferenceRangeComponent> referenceRange; 937 938 private static final long serialVersionUID = 576590931L; 939 940 /** 941 * Constructor 942 */ 943 public ObservationComponentComponent() { 944 super(); 945 } 946 947 /** 948 * Constructor 949 */ 950 public ObservationComponentComponent(CodeableConcept code) { 951 super(); 952 this.code = code; 953 } 954 955 /** 956 * @return {@link #code} (Describes what was observed. Sometimes this is called 957 * the observation "code".) 958 */ 959 public CodeableConcept getCode() { 960 if (this.code == null) 961 if (Configuration.errorOnAutoCreate()) 962 throw new Error("Attempt to auto-create ObservationComponentComponent.code"); 963 else if (Configuration.doAutoCreate()) 964 this.code = new CodeableConcept(); // cc 965 return this.code; 966 } 967 968 public boolean hasCode() { 969 return this.code != null && !this.code.isEmpty(); 970 } 971 972 /** 973 * @param value {@link #code} (Describes what was observed. Sometimes this is 974 * called the observation "code".) 975 */ 976 public ObservationComponentComponent setCode(CodeableConcept value) { 977 this.code = value; 978 return this; 979 } 980 981 /** 982 * @return {@link #value} (The information determined as a result of making the 983 * observation, if the information has a simple value.) 984 */ 985 public Type getValue() { 986 return this.value; 987 } 988 989 /** 990 * @return {@link #value} (The information determined as a result of making the 991 * observation, if the information has a simple value.) 992 */ 993 public Quantity getValueQuantity() throws FHIRException { 994 if (this.value == null) 995 this.value = new Quantity(); 996 if (!(this.value instanceof Quantity)) 997 throw new FHIRException("Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() 998 + " was encountered"); 999 return (Quantity) this.value; 1000 } 1001 1002 public boolean hasValueQuantity() { 1003 return this != null && this.value instanceof Quantity; 1004 } 1005 1006 /** 1007 * @return {@link #value} (The information determined as a result of making the 1008 * observation, if the information has a simple value.) 1009 */ 1010 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1011 if (this.value == null) 1012 this.value = new CodeableConcept(); 1013 if (!(this.value instanceof CodeableConcept)) 1014 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1015 + this.value.getClass().getName() + " was encountered"); 1016 return (CodeableConcept) this.value; 1017 } 1018 1019 public boolean hasValueCodeableConcept() { 1020 return this != null && this.value instanceof CodeableConcept; 1021 } 1022 1023 /** 1024 * @return {@link #value} (The information determined as a result of making the 1025 * observation, if the information has a simple value.) 1026 */ 1027 public StringType getValueStringType() throws FHIRException { 1028 if (this.value == null) 1029 this.value = new StringType(); 1030 if (!(this.value instanceof StringType)) 1031 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1032 + this.value.getClass().getName() + " was encountered"); 1033 return (StringType) this.value; 1034 } 1035 1036 public boolean hasValueStringType() { 1037 return this != null && this.value instanceof StringType; 1038 } 1039 1040 /** 1041 * @return {@link #value} (The information determined as a result of making the 1042 * observation, if the information has a simple value.) 1043 */ 1044 public BooleanType getValueBooleanType() throws FHIRException { 1045 if (this.value == null) 1046 this.value = new BooleanType(); 1047 if (!(this.value instanceof BooleanType)) 1048 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " 1049 + this.value.getClass().getName() + " was encountered"); 1050 return (BooleanType) this.value; 1051 } 1052 1053 public boolean hasValueBooleanType() { 1054 return this != null && this.value instanceof BooleanType; 1055 } 1056 1057 /** 1058 * @return {@link #value} (The information determined as a result of making the 1059 * observation, if the information has a simple value.) 1060 */ 1061 public IntegerType getValueIntegerType() throws FHIRException { 1062 if (this.value == null) 1063 this.value = new IntegerType(); 1064 if (!(this.value instanceof IntegerType)) 1065 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " 1066 + this.value.getClass().getName() + " was encountered"); 1067 return (IntegerType) this.value; 1068 } 1069 1070 public boolean hasValueIntegerType() { 1071 return this != null && this.value instanceof IntegerType; 1072 } 1073 1074 /** 1075 * @return {@link #value} (The information determined as a result of making the 1076 * observation, if the information has a simple value.) 1077 */ 1078 public Range getValueRange() throws FHIRException { 1079 if (this.value == null) 1080 this.value = new Range(); 1081 if (!(this.value instanceof Range)) 1082 throw new FHIRException( 1083 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 1084 return (Range) this.value; 1085 } 1086 1087 public boolean hasValueRange() { 1088 return this != null && this.value instanceof Range; 1089 } 1090 1091 /** 1092 * @return {@link #value} (The information determined as a result of making the 1093 * observation, if the information has a simple value.) 1094 */ 1095 public Ratio getValueRatio() throws FHIRException { 1096 if (this.value == null) 1097 this.value = new Ratio(); 1098 if (!(this.value instanceof Ratio)) 1099 throw new FHIRException( 1100 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 1101 return (Ratio) this.value; 1102 } 1103 1104 public boolean hasValueRatio() { 1105 return this != null && this.value instanceof Ratio; 1106 } 1107 1108 /** 1109 * @return {@link #value} (The information determined as a result of making the 1110 * observation, if the information has a simple value.) 1111 */ 1112 public SampledData getValueSampledData() throws FHIRException { 1113 if (this.value == null) 1114 this.value = new SampledData(); 1115 if (!(this.value instanceof SampledData)) 1116 throw new FHIRException("Type mismatch: the type SampledData was expected, but " 1117 + this.value.getClass().getName() + " was encountered"); 1118 return (SampledData) this.value; 1119 } 1120 1121 public boolean hasValueSampledData() { 1122 return this != null && this.value instanceof SampledData; 1123 } 1124 1125 /** 1126 * @return {@link #value} (The information determined as a result of making the 1127 * observation, if the information has a simple value.) 1128 */ 1129 public TimeType getValueTimeType() throws FHIRException { 1130 if (this.value == null) 1131 this.value = new TimeType(); 1132 if (!(this.value instanceof TimeType)) 1133 throw new FHIRException("Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() 1134 + " was encountered"); 1135 return (TimeType) this.value; 1136 } 1137 1138 public boolean hasValueTimeType() { 1139 return this != null && this.value instanceof TimeType; 1140 } 1141 1142 /** 1143 * @return {@link #value} (The information determined as a result of making the 1144 * observation, if the information has a simple value.) 1145 */ 1146 public DateTimeType getValueDateTimeType() throws FHIRException { 1147 if (this.value == null) 1148 this.value = new DateTimeType(); 1149 if (!(this.value instanceof DateTimeType)) 1150 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 1151 + this.value.getClass().getName() + " was encountered"); 1152 return (DateTimeType) this.value; 1153 } 1154 1155 public boolean hasValueDateTimeType() { 1156 return this != null && this.value instanceof DateTimeType; 1157 } 1158 1159 /** 1160 * @return {@link #value} (The information determined as a result of making the 1161 * observation, if the information has a simple value.) 1162 */ 1163 public Period getValuePeriod() throws FHIRException { 1164 if (this.value == null) 1165 this.value = new Period(); 1166 if (!(this.value instanceof Period)) 1167 throw new FHIRException( 1168 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 1169 return (Period) this.value; 1170 } 1171 1172 public boolean hasValuePeriod() { 1173 return this != null && this.value instanceof Period; 1174 } 1175 1176 public boolean hasValue() { 1177 return this.value != null && !this.value.isEmpty(); 1178 } 1179 1180 /** 1181 * @param value {@link #value} (The information determined as a result of making 1182 * the observation, if the information has a simple value.) 1183 */ 1184 public ObservationComponentComponent setValue(Type value) { 1185 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept 1186 || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType 1187 || value instanceof Range || value instanceof Ratio || value instanceof SampledData 1188 || value instanceof TimeType || value instanceof DateTimeType || value instanceof Period)) 1189 throw new Error("Not the right type for Observation.component.value[x]: " + value.fhirType()); 1190 this.value = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 1196 * in the element Observation.component.value[x] is missing.) 1197 */ 1198 public CodeableConcept getDataAbsentReason() { 1199 if (this.dataAbsentReason == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error("Attempt to auto-create ObservationComponentComponent.dataAbsentReason"); 1202 else if (Configuration.doAutoCreate()) 1203 this.dataAbsentReason = new CodeableConcept(); // cc 1204 return this.dataAbsentReason; 1205 } 1206 1207 public boolean hasDataAbsentReason() { 1208 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 1213 * value in the element Observation.component.value[x] is missing.) 1214 */ 1215 public ObservationComponentComponent setDataAbsentReason(CodeableConcept value) { 1216 this.dataAbsentReason = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return {@link #interpretation} (A categorical assessment of an observation 1222 * value. For example, high, low, normal.) 1223 */ 1224 public List<CodeableConcept> getInterpretation() { 1225 if (this.interpretation == null) 1226 this.interpretation = new ArrayList<CodeableConcept>(); 1227 return this.interpretation; 1228 } 1229 1230 /** 1231 * @return Returns a reference to <code>this</code> for easy method chaining 1232 */ 1233 public ObservationComponentComponent setInterpretation(List<CodeableConcept> theInterpretation) { 1234 this.interpretation = theInterpretation; 1235 return this; 1236 } 1237 1238 public boolean hasInterpretation() { 1239 if (this.interpretation == null) 1240 return false; 1241 for (CodeableConcept item : this.interpretation) 1242 if (!item.isEmpty()) 1243 return true; 1244 return false; 1245 } 1246 1247 public CodeableConcept addInterpretation() { // 3 1248 CodeableConcept t = new CodeableConcept(); 1249 if (this.interpretation == null) 1250 this.interpretation = new ArrayList<CodeableConcept>(); 1251 this.interpretation.add(t); 1252 return t; 1253 } 1254 1255 public ObservationComponentComponent addInterpretation(CodeableConcept t) { // 3 1256 if (t == null) 1257 return this; 1258 if (this.interpretation == null) 1259 this.interpretation = new ArrayList<CodeableConcept>(); 1260 this.interpretation.add(t); 1261 return this; 1262 } 1263 1264 /** 1265 * @return The first repetition of repeating field {@link #interpretation}, 1266 * creating it if it does not already exist 1267 */ 1268 public CodeableConcept getInterpretationFirstRep() { 1269 if (getInterpretation().isEmpty()) { 1270 addInterpretation(); 1271 } 1272 return getInterpretation().get(0); 1273 } 1274 1275 /** 1276 * @return {@link #referenceRange} (Guidance on how to interpret the value by 1277 * comparison to a normal or recommended range.) 1278 */ 1279 public List<ObservationReferenceRangeComponent> getReferenceRange() { 1280 if (this.referenceRange == null) 1281 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1282 return this.referenceRange; 1283 } 1284 1285 /** 1286 * @return Returns a reference to <code>this</code> for easy method chaining 1287 */ 1288 public ObservationComponentComponent setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 1289 this.referenceRange = theReferenceRange; 1290 return this; 1291 } 1292 1293 public boolean hasReferenceRange() { 1294 if (this.referenceRange == null) 1295 return false; 1296 for (ObservationReferenceRangeComponent item : this.referenceRange) 1297 if (!item.isEmpty()) 1298 return true; 1299 return false; 1300 } 1301 1302 public ObservationReferenceRangeComponent addReferenceRange() { // 3 1303 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 1304 if (this.referenceRange == null) 1305 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1306 this.referenceRange.add(t); 1307 return t; 1308 } 1309 1310 public ObservationComponentComponent addReferenceRange(ObservationReferenceRangeComponent t) { // 3 1311 if (t == null) 1312 return this; 1313 if (this.referenceRange == null) 1314 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1315 this.referenceRange.add(t); 1316 return this; 1317 } 1318 1319 /** 1320 * @return The first repetition of repeating field {@link #referenceRange}, 1321 * creating it if it does not already exist 1322 */ 1323 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 1324 if (getReferenceRange().isEmpty()) { 1325 addReferenceRange(); 1326 } 1327 return getReferenceRange().get(0); 1328 } 1329 1330 protected void listChildren(List<Property> children) { 1331 super.listChildren(children); 1332 children.add(new Property("code", "CodeableConcept", 1333 "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code)); 1334 children.add(new Property("value[x]", 1335 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1336 "The information determined as a result of making the observation, if the information has a simple value.", 0, 1337 1, value)); 1338 children.add(new Property("dataAbsentReason", "CodeableConcept", 1339 "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, 1340 dataAbsentReason)); 1341 children.add(new Property("interpretation", "CodeableConcept", 1342 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 1343 java.lang.Integer.MAX_VALUE, interpretation)); 1344 children.add(new Property("referenceRange", "@Observation.referenceRange", 1345 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 1346 java.lang.Integer.MAX_VALUE, referenceRange)); 1347 } 1348 1349 @Override 1350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1351 switch (_hash) { 1352 case 3059181: 1353 /* code */ return new Property("code", "CodeableConcept", 1354 "Describes what was observed. Sometimes this is called the observation \"code\".", 0, 1, code); 1355 case -1410166417: 1356 /* value[x] */ return new Property("value[x]", 1357 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1358 "The information determined as a result of making the observation, if the information has a simple value.", 1359 0, 1, value); 1360 case 111972721: 1361 /* value */ return new Property("value[x]", 1362 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1363 "The information determined as a result of making the observation, if the information has a simple value.", 1364 0, 1, value); 1365 case -2029823716: 1366 /* valueQuantity */ return new Property("value[x]", 1367 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1368 "The information determined as a result of making the observation, if the information has a simple value.", 1369 0, 1, value); 1370 case 924902896: 1371 /* valueCodeableConcept */ return new Property("value[x]", 1372 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1373 "The information determined as a result of making the observation, if the information has a simple value.", 1374 0, 1, value); 1375 case -1424603934: 1376 /* valueString */ return new Property("value[x]", 1377 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1378 "The information determined as a result of making the observation, if the information has a simple value.", 1379 0, 1, value); 1380 case 733421943: 1381 /* valueBoolean */ return new Property("value[x]", 1382 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1383 "The information determined as a result of making the observation, if the information has a simple value.", 1384 0, 1, value); 1385 case -1668204915: 1386 /* valueInteger */ return new Property("value[x]", 1387 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1388 "The information determined as a result of making the observation, if the information has a simple value.", 1389 0, 1, value); 1390 case 2030761548: 1391 /* valueRange */ return new Property("value[x]", 1392 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1393 "The information determined as a result of making the observation, if the information has a simple value.", 1394 0, 1, value); 1395 case 2030767386: 1396 /* valueRatio */ return new Property("value[x]", 1397 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1398 "The information determined as a result of making the observation, if the information has a simple value.", 1399 0, 1, value); 1400 case -962229101: 1401 /* valueSampledData */ return new Property("value[x]", 1402 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1403 "The information determined as a result of making the observation, if the information has a simple value.", 1404 0, 1, value); 1405 case -765708322: 1406 /* valueTime */ return new Property("value[x]", 1407 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1408 "The information determined as a result of making the observation, if the information has a simple value.", 1409 0, 1, value); 1410 case 1047929900: 1411 /* valueDateTime */ return new Property("value[x]", 1412 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1413 "The information determined as a result of making the observation, if the information has a simple value.", 1414 0, 1, value); 1415 case -1524344174: 1416 /* valuePeriod */ return new Property("value[x]", 1417 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 1418 "The information determined as a result of making the observation, if the information has a simple value.", 1419 0, 1, value); 1420 case 1034315687: 1421 /* dataAbsentReason */ return new Property("dataAbsentReason", "CodeableConcept", 1422 "Provides a reason why the expected value in the element Observation.component.value[x] is missing.", 0, 1, 1423 dataAbsentReason); 1424 case -297950712: 1425 /* interpretation */ return new Property("interpretation", "CodeableConcept", 1426 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 1427 java.lang.Integer.MAX_VALUE, interpretation); 1428 case -1912545102: 1429 /* referenceRange */ return new Property("referenceRange", "@Observation.referenceRange", 1430 "Guidance on how to interpret the value by comparison to a normal or recommended range.", 0, 1431 java.lang.Integer.MAX_VALUE, referenceRange); 1432 default: 1433 return super.getNamedProperty(_hash, _name, _checkValid); 1434 } 1435 1436 } 1437 1438 @Override 1439 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1440 switch (hash) { 1441 case 3059181: 1442 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 1443 case 111972721: 1444 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 1445 case 1034315687: 1446 /* dataAbsentReason */ return this.dataAbsentReason == null ? new Base[0] 1447 : new Base[] { this.dataAbsentReason }; // CodeableConcept 1448 case -297950712: 1449 /* interpretation */ return this.interpretation == null ? new Base[0] 1450 : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 1451 case -1912545102: 1452 /* referenceRange */ return this.referenceRange == null ? new Base[0] 1453 : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 1454 default: 1455 return super.getProperty(hash, name, checkValid); 1456 } 1457 1458 } 1459 1460 @Override 1461 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1462 switch (hash) { 1463 case 3059181: // code 1464 this.code = castToCodeableConcept(value); // CodeableConcept 1465 return value; 1466 case 111972721: // value 1467 this.value = castToType(value); // Type 1468 return value; 1469 case 1034315687: // dataAbsentReason 1470 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1471 return value; 1472 case -297950712: // interpretation 1473 this.getInterpretation().add(castToCodeableConcept(value)); // CodeableConcept 1474 return value; 1475 case -1912545102: // referenceRange 1476 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 1477 return value; 1478 default: 1479 return super.setProperty(hash, name, value); 1480 } 1481 1482 } 1483 1484 @Override 1485 public Base setProperty(String name, Base value) throws FHIRException { 1486 if (name.equals("code")) { 1487 this.code = castToCodeableConcept(value); // CodeableConcept 1488 } else if (name.equals("value[x]")) { 1489 this.value = castToType(value); // Type 1490 } else if (name.equals("dataAbsentReason")) { 1491 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 1492 } else if (name.equals("interpretation")) { 1493 this.getInterpretation().add(castToCodeableConcept(value)); 1494 } else if (name.equals("referenceRange")) { 1495 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 1496 } else 1497 return super.setProperty(name, value); 1498 return value; 1499 } 1500 1501 @Override 1502 public void removeChild(String name, Base value) throws FHIRException { 1503 if (name.equals("code")) { 1504 this.code = null; 1505 } else if (name.equals("value[x]")) { 1506 this.value = null; 1507 } else if (name.equals("dataAbsentReason")) { 1508 this.dataAbsentReason = null; 1509 } else if (name.equals("interpretation")) { 1510 this.getInterpretation().remove(castToCodeableConcept(value)); 1511 } else if (name.equals("referenceRange")) { 1512 this.getReferenceRange().remove((ObservationReferenceRangeComponent) value); 1513 } else 1514 super.removeChild(name, value); 1515 1516 } 1517 1518 @Override 1519 public Base makeProperty(int hash, String name) throws FHIRException { 1520 switch (hash) { 1521 case 3059181: 1522 return getCode(); 1523 case -1410166417: 1524 return getValue(); 1525 case 111972721: 1526 return getValue(); 1527 case 1034315687: 1528 return getDataAbsentReason(); 1529 case -297950712: 1530 return addInterpretation(); 1531 case -1912545102: 1532 return addReferenceRange(); 1533 default: 1534 return super.makeProperty(hash, name); 1535 } 1536 1537 } 1538 1539 @Override 1540 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1541 switch (hash) { 1542 case 3059181: 1543 /* code */ return new String[] { "CodeableConcept" }; 1544 case 111972721: 1545 /* value */ return new String[] { "Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", 1546 "Ratio", "SampledData", "time", "dateTime", "Period" }; 1547 case 1034315687: 1548 /* dataAbsentReason */ return new String[] { "CodeableConcept" }; 1549 case -297950712: 1550 /* interpretation */ return new String[] { "CodeableConcept" }; 1551 case -1912545102: 1552 /* referenceRange */ return new String[] { "@Observation.referenceRange" }; 1553 default: 1554 return super.getTypesForProperty(hash, name); 1555 } 1556 1557 } 1558 1559 @Override 1560 public Base addChild(String name) throws FHIRException { 1561 if (name.equals("code")) { 1562 this.code = new CodeableConcept(); 1563 return this.code; 1564 } else if (name.equals("valueQuantity")) { 1565 this.value = new Quantity(); 1566 return this.value; 1567 } else if (name.equals("valueCodeableConcept")) { 1568 this.value = new CodeableConcept(); 1569 return this.value; 1570 } else if (name.equals("valueString")) { 1571 this.value = new StringType(); 1572 return this.value; 1573 } else if (name.equals("valueBoolean")) { 1574 this.value = new BooleanType(); 1575 return this.value; 1576 } else if (name.equals("valueInteger")) { 1577 this.value = new IntegerType(); 1578 return this.value; 1579 } else if (name.equals("valueRange")) { 1580 this.value = new Range(); 1581 return this.value; 1582 } else if (name.equals("valueRatio")) { 1583 this.value = new Ratio(); 1584 return this.value; 1585 } else if (name.equals("valueSampledData")) { 1586 this.value = new SampledData(); 1587 return this.value; 1588 } else if (name.equals("valueTime")) { 1589 this.value = new TimeType(); 1590 return this.value; 1591 } else if (name.equals("valueDateTime")) { 1592 this.value = new DateTimeType(); 1593 return this.value; 1594 } else if (name.equals("valuePeriod")) { 1595 this.value = new Period(); 1596 return this.value; 1597 } else if (name.equals("dataAbsentReason")) { 1598 this.dataAbsentReason = new CodeableConcept(); 1599 return this.dataAbsentReason; 1600 } else if (name.equals("interpretation")) { 1601 return addInterpretation(); 1602 } else if (name.equals("referenceRange")) { 1603 return addReferenceRange(); 1604 } else 1605 return super.addChild(name); 1606 } 1607 1608 public ObservationComponentComponent copy() { 1609 ObservationComponentComponent dst = new ObservationComponentComponent(); 1610 copyValues(dst); 1611 return dst; 1612 } 1613 1614 public void copyValues(ObservationComponentComponent dst) { 1615 super.copyValues(dst); 1616 dst.code = code == null ? null : code.copy(); 1617 dst.value = value == null ? null : value.copy(); 1618 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 1619 if (interpretation != null) { 1620 dst.interpretation = new ArrayList<CodeableConcept>(); 1621 for (CodeableConcept i : interpretation) 1622 dst.interpretation.add(i.copy()); 1623 } 1624 ; 1625 if (referenceRange != null) { 1626 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 1627 for (ObservationReferenceRangeComponent i : referenceRange) 1628 dst.referenceRange.add(i.copy()); 1629 } 1630 ; 1631 } 1632 1633 @Override 1634 public boolean equalsDeep(Base other_) { 1635 if (!super.equalsDeep(other_)) 1636 return false; 1637 if (!(other_ instanceof ObservationComponentComponent)) 1638 return false; 1639 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1640 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 1641 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 1642 && compareDeep(interpretation, o.interpretation, true) && compareDeep(referenceRange, o.referenceRange, true); 1643 } 1644 1645 @Override 1646 public boolean equalsShallow(Base other_) { 1647 if (!super.equalsShallow(other_)) 1648 return false; 1649 if (!(other_ instanceof ObservationComponentComponent)) 1650 return false; 1651 ObservationComponentComponent o = (ObservationComponentComponent) other_; 1652 return true; 1653 } 1654 1655 public boolean isEmpty() { 1656 return super.isEmpty() 1657 && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, dataAbsentReason, interpretation, referenceRange); 1658 } 1659 1660 public String fhirType() { 1661 return "Observation.component"; 1662 1663 } 1664 1665 } 1666 1667 /** 1668 * A unique identifier assigned to this observation. 1669 */ 1670 @Child(name = "identifier", type = { 1671 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1672 @Description(shortDefinition = "Business Identifier for observation", formalDefinition = "A unique identifier assigned to this observation.") 1673 protected List<Identifier> identifier; 1674 1675 /** 1676 * A plan, proposal or order that is fulfilled in whole or in part by this 1677 * event. For example, a MedicationRequest may require a patient to have 1678 * laboratory test performed before it is dispensed. 1679 */ 1680 @Child(name = "basedOn", type = { CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, 1681 MedicationRequest.class, NutritionOrder.class, 1682 ServiceRequest.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1683 @Description(shortDefinition = "Fulfills plan, proposal or order", formalDefinition = "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.") 1684 protected List<Reference> basedOn; 1685 /** 1686 * The actual objects that are the target of the reference (A plan, proposal or 1687 * order that is fulfilled in whole or in part by this event. For example, a 1688 * MedicationRequest may require a patient to have laboratory test performed 1689 * before it is dispensed.) 1690 */ 1691 protected List<Resource> basedOnTarget; 1692 1693 /** 1694 * A larger event of which this particular Observation is a component or step. 1695 * For example, an observation as part of a procedure. 1696 */ 1697 @Child(name = "partOf", type = { MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, 1698 Procedure.class, Immunization.class, 1699 ImagingStudy.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1700 @Description(shortDefinition = "Part of referenced event", formalDefinition = "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.") 1701 protected List<Reference> partOf; 1702 /** 1703 * The actual objects that are the target of the reference (A larger event of 1704 * which this particular Observation is a component or step. For example, an 1705 * observation as part of a procedure.) 1706 */ 1707 protected List<Resource> partOfTarget; 1708 1709 /** 1710 * The status of the result value. 1711 */ 1712 @Child(name = "status", type = { CodeType.class }, order = 3, min = 1, max = 1, modifier = true, summary = true) 1713 @Description(shortDefinition = "registered | preliminary | final | amended +", formalDefinition = "The status of the result value.") 1714 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-status") 1715 protected Enumeration<ObservationStatus> status; 1716 1717 /** 1718 * A code that classifies the general type of observation being made. 1719 */ 1720 @Child(name = "category", type = { 1721 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1722 @Description(shortDefinition = "Classification of type of observation", formalDefinition = "A code that classifies the general type of observation being made.") 1723 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-category") 1724 protected List<CodeableConcept> category; 1725 1726 /** 1727 * Describes what was observed. Sometimes this is called the observation "name". 1728 */ 1729 @Child(name = "code", type = { CodeableConcept.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1730 @Description(shortDefinition = "Type of observation (code / type)", formalDefinition = "Describes what was observed. Sometimes this is called the observation \"name\".") 1731 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-codes") 1732 protected CodeableConcept code; 1733 1734 /** 1735 * The patient, or group of patients, location, or device this observation is 1736 * about and into whose record the observation is placed. If the actual focus of 1737 * the observation is different from the subject (or a sample of, part, or 1738 * region of the subject), the `focus` element or the `code` itself specifies 1739 * the actual focus of the observation. 1740 */ 1741 @Child(name = "subject", type = { Patient.class, Group.class, Device.class, 1742 Location.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1743 @Description(shortDefinition = "Who and/or what the observation is about", formalDefinition = "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.") 1744 protected Reference subject; 1745 1746 /** 1747 * The actual object that is the target of the reference (The patient, or group 1748 * of patients, location, or device this observation is about and into whose 1749 * record the observation is placed. If the actual focus of the observation is 1750 * different from the subject (or a sample of, part, or region of the subject), 1751 * the `focus` element or the `code` itself specifies the actual focus of the 1752 * observation.) 1753 */ 1754 protected Resource subjectTarget; 1755 1756 /** 1757 * The actual focus of an observation when it is not the patient of record 1758 * representing something or someone associated with the patient such as a 1759 * spouse, parent, fetus, or donor. For example, fetus observations in a 1760 * mother's record. The focus of an observation could also be an existing 1761 * condition, an intervention, the subject's diet, another observation of the 1762 * subject, or a body structure such as tumor or implanted device. An example 1763 * use case would be using the Observation resource to capture whether the 1764 * mother is trained to change her child's tracheostomy tube. In this example, 1765 * the child is the patient of record and the mother is the focus. 1766 */ 1767 @Child(name = "focus", type = { 1768 Reference.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1769 @Description(shortDefinition = "What the observation is about, when it is not about the subject of record", formalDefinition = "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.") 1770 protected List<Reference> focus; 1771 /** 1772 * The actual objects that are the target of the reference (The actual focus of 1773 * an observation when it is not the patient of record representing something or 1774 * someone associated with the patient such as a spouse, parent, fetus, or 1775 * donor. For example, fetus observations in a mother's record. The focus of an 1776 * observation could also be an existing condition, an intervention, the 1777 * subject's diet, another observation of the subject, or a body structure such 1778 * as tumor or implanted device. An example use case would be using the 1779 * Observation resource to capture whether the mother is trained to change her 1780 * child's tracheostomy tube. In this example, the child is the patient of 1781 * record and the mother is the focus.) 1782 */ 1783 protected List<Resource> focusTarget; 1784 1785 /** 1786 * The healthcare event (e.g. a patient and healthcare provider interaction) 1787 * during which this observation is made. 1788 */ 1789 @Child(name = "encounter", type = { Encounter.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1790 @Description(shortDefinition = "Healthcare event during which this observation is made", formalDefinition = "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.") 1791 protected Reference encounter; 1792 1793 /** 1794 * The actual object that is the target of the reference (The healthcare event 1795 * (e.g. a patient and healthcare provider interaction) during which this 1796 * observation is made.) 1797 */ 1798 protected Encounter encounterTarget; 1799 1800 /** 1801 * The time or time-period the observed value is asserted as being true. For 1802 * biological subjects - e.g. human patients - this is usually called the 1803 * "physiologically relevant time". This is usually either the time of the 1804 * procedure or of specimen collection, but very often the source of the 1805 * date/time is not known, only the date/time itself. 1806 */ 1807 @Child(name = "effective", type = { DateTimeType.class, Period.class, Timing.class, 1808 InstantType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1809 @Description(shortDefinition = "Clinically relevant time/time-period for observation", formalDefinition = "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.") 1810 protected Type effective; 1811 1812 /** 1813 * The date and time this version of the observation was made available to 1814 * providers, typically after the results have been reviewed and verified. 1815 */ 1816 @Child(name = "issued", type = { InstantType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1817 @Description(shortDefinition = "Date/Time this version was made available", formalDefinition = "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.") 1818 protected InstantType issued; 1819 1820 /** 1821 * Who was responsible for asserting the observed value as "true". 1822 */ 1823 @Child(name = "performer", type = { Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, 1824 Patient.class, 1825 RelatedPerson.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1826 @Description(shortDefinition = "Who is responsible for the observation", formalDefinition = "Who was responsible for asserting the observed value as \"true\".") 1827 protected List<Reference> performer; 1828 /** 1829 * The actual objects that are the target of the reference (Who was responsible 1830 * for asserting the observed value as "true".) 1831 */ 1832 protected List<Resource> performerTarget; 1833 1834 /** 1835 * The information determined as a result of making the observation, if the 1836 * information has a simple value. 1837 */ 1838 @Child(name = "value", type = { Quantity.class, CodeableConcept.class, StringType.class, BooleanType.class, 1839 IntegerType.class, Range.class, Ratio.class, SampledData.class, TimeType.class, DateTimeType.class, 1840 Period.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1841 @Description(shortDefinition = "Actual result", formalDefinition = "The information determined as a result of making the observation, if the information has a simple value.") 1842 protected Type value; 1843 1844 /** 1845 * Provides a reason why the expected value in the element Observation.value[x] 1846 * is missing. 1847 */ 1848 @Child(name = "dataAbsentReason", type = { 1849 CodeableConcept.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1850 @Description(shortDefinition = "Why the result is missing", formalDefinition = "Provides a reason why the expected value in the element Observation.value[x] is missing.") 1851 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/data-absent-reason") 1852 protected CodeableConcept dataAbsentReason; 1853 1854 /** 1855 * A categorical assessment of an observation value. For example, high, low, 1856 * normal. 1857 */ 1858 @Child(name = "interpretation", type = { 1859 CodeableConcept.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1860 @Description(shortDefinition = "High, low, normal, etc.", formalDefinition = "A categorical assessment of an observation value. For example, high, low, normal.") 1861 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-interpretation") 1862 protected List<CodeableConcept> interpretation; 1863 1864 /** 1865 * Comments about the observation or the results. 1866 */ 1867 @Child(name = "note", type = { 1868 Annotation.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1869 @Description(shortDefinition = "Comments about the observation", formalDefinition = "Comments about the observation or the results.") 1870 protected List<Annotation> note; 1871 1872 /** 1873 * Indicates the site on the subject's body where the observation was made (i.e. 1874 * the target site). 1875 */ 1876 @Child(name = "bodySite", type = { 1877 CodeableConcept.class }, order = 16, min = 0, max = 1, modifier = false, summary = false) 1878 @Description(shortDefinition = "Observed body part", formalDefinition = "Indicates the site on the subject's body where the observation was made (i.e. the target site).") 1879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/body-site") 1880 protected CodeableConcept bodySite; 1881 1882 /** 1883 * Indicates the mechanism used to perform the observation. 1884 */ 1885 @Child(name = "method", type = { 1886 CodeableConcept.class }, order = 17, min = 0, max = 1, modifier = false, summary = false) 1887 @Description(shortDefinition = "How it was done", formalDefinition = "Indicates the mechanism used to perform the observation.") 1888 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/observation-methods") 1889 protected CodeableConcept method; 1890 1891 /** 1892 * The specimen that was used when this observation was made. 1893 */ 1894 @Child(name = "specimen", type = { Specimen.class }, order = 18, min = 0, max = 1, modifier = false, summary = false) 1895 @Description(shortDefinition = "Specimen used for this observation", formalDefinition = "The specimen that was used when this observation was made.") 1896 protected Reference specimen; 1897 1898 /** 1899 * The actual object that is the target of the reference (The specimen that was 1900 * used when this observation was made.) 1901 */ 1902 protected Specimen specimenTarget; 1903 1904 /** 1905 * The device used to generate the observation data. 1906 */ 1907 @Child(name = "device", type = { Device.class, 1908 DeviceMetric.class }, order = 19, min = 0, max = 1, modifier = false, summary = false) 1909 @Description(shortDefinition = "(Measurement) Device", formalDefinition = "The device used to generate the observation data.") 1910 protected Reference device; 1911 1912 /** 1913 * The actual object that is the target of the reference (The device used to 1914 * generate the observation data.) 1915 */ 1916 protected Resource deviceTarget; 1917 1918 /** 1919 * Guidance on how to interpret the value by comparison to a normal or 1920 * recommended range. Multiple reference ranges are interpreted as an "OR". In 1921 * other words, to represent two distinct target populations, two 1922 * `referenceRange` elements would be used. 1923 */ 1924 @Child(name = "referenceRange", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1925 @Description(shortDefinition = "Provides guide for interpretation", formalDefinition = "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.") 1926 protected List<ObservationReferenceRangeComponent> referenceRange; 1927 1928 /** 1929 * This observation is a group observation (e.g. a battery, a panel of tests, a 1930 * set of vital sign measurements) that includes the target as a member of the 1931 * group. 1932 */ 1933 @Child(name = "hasMember", type = { Observation.class, QuestionnaireResponse.class, 1934 MolecularSequence.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1935 @Description(shortDefinition = "Related resource that belongs to the Observation group", formalDefinition = "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.") 1936 protected List<Reference> hasMember; 1937 /** 1938 * The actual objects that are the target of the reference (This observation is 1939 * a group observation (e.g. a battery, a panel of tests, a set of vital sign 1940 * measurements) that includes the target as a member of the group.) 1941 */ 1942 protected List<Resource> hasMemberTarget; 1943 1944 /** 1945 * The target resource that represents a measurement from which this observation 1946 * value is derived. For example, a calculated anion gap or a fetal measurement 1947 * based on an ultrasound image. 1948 */ 1949 @Child(name = "derivedFrom", type = { DocumentReference.class, ImagingStudy.class, Media.class, 1950 QuestionnaireResponse.class, Observation.class, 1951 MolecularSequence.class }, order = 22, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1952 @Description(shortDefinition = "Related measurements the observation is made from", formalDefinition = "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.") 1953 protected List<Reference> derivedFrom; 1954 /** 1955 * The actual objects that are the target of the reference (The target resource 1956 * that represents a measurement from which this observation value is derived. 1957 * For example, a calculated anion gap or a fetal measurement based on an 1958 * ultrasound image.) 1959 */ 1960 protected List<Resource> derivedFromTarget; 1961 1962 /** 1963 * Some observations have multiple component observations. These component 1964 * observations are expressed as separate code value pairs that share the same 1965 * attributes. Examples include systolic and diastolic component observations 1966 * for blood pressure measurement and multiple component observations for 1967 * genetics observations. 1968 */ 1969 @Child(name = "component", type = {}, order = 23, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1970 @Description(shortDefinition = "Component results", formalDefinition = "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.") 1971 protected List<ObservationComponentComponent> component; 1972 1973 private static final long serialVersionUID = -2036786355L; 1974 1975 /** 1976 * Constructor 1977 */ 1978 public Observation() { 1979 super(); 1980 } 1981 1982 /** 1983 * Constructor 1984 */ 1985 public Observation(Enumeration<ObservationStatus> status, CodeableConcept code) { 1986 super(); 1987 this.status = status; 1988 this.code = code; 1989 } 1990 1991 /** 1992 * @return {@link #identifier} (A unique identifier assigned to this 1993 * observation.) 1994 */ 1995 public List<Identifier> getIdentifier() { 1996 if (this.identifier == null) 1997 this.identifier = new ArrayList<Identifier>(); 1998 return this.identifier; 1999 } 2000 2001 /** 2002 * @return Returns a reference to <code>this</code> for easy method chaining 2003 */ 2004 public Observation setIdentifier(List<Identifier> theIdentifier) { 2005 this.identifier = theIdentifier; 2006 return this; 2007 } 2008 2009 public boolean hasIdentifier() { 2010 if (this.identifier == null) 2011 return false; 2012 for (Identifier item : this.identifier) 2013 if (!item.isEmpty()) 2014 return true; 2015 return false; 2016 } 2017 2018 public Identifier addIdentifier() { // 3 2019 Identifier t = new Identifier(); 2020 if (this.identifier == null) 2021 this.identifier = new ArrayList<Identifier>(); 2022 this.identifier.add(t); 2023 return t; 2024 } 2025 2026 public Observation addIdentifier(Identifier t) { // 3 2027 if (t == null) 2028 return this; 2029 if (this.identifier == null) 2030 this.identifier = new ArrayList<Identifier>(); 2031 this.identifier.add(t); 2032 return this; 2033 } 2034 2035 /** 2036 * @return The first repetition of repeating field {@link #identifier}, creating 2037 * it if it does not already exist 2038 */ 2039 public Identifier getIdentifierFirstRep() { 2040 if (getIdentifier().isEmpty()) { 2041 addIdentifier(); 2042 } 2043 return getIdentifier().get(0); 2044 } 2045 2046 /** 2047 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in 2048 * whole or in part by this event. For example, a MedicationRequest may 2049 * require a patient to have laboratory test performed before it is 2050 * dispensed.) 2051 */ 2052 public List<Reference> getBasedOn() { 2053 if (this.basedOn == null) 2054 this.basedOn = new ArrayList<Reference>(); 2055 return this.basedOn; 2056 } 2057 2058 /** 2059 * @return Returns a reference to <code>this</code> for easy method chaining 2060 */ 2061 public Observation setBasedOn(List<Reference> theBasedOn) { 2062 this.basedOn = theBasedOn; 2063 return this; 2064 } 2065 2066 public boolean hasBasedOn() { 2067 if (this.basedOn == null) 2068 return false; 2069 for (Reference item : this.basedOn) 2070 if (!item.isEmpty()) 2071 return true; 2072 return false; 2073 } 2074 2075 public Reference addBasedOn() { // 3 2076 Reference t = new Reference(); 2077 if (this.basedOn == null) 2078 this.basedOn = new ArrayList<Reference>(); 2079 this.basedOn.add(t); 2080 return t; 2081 } 2082 2083 public Observation addBasedOn(Reference t) { // 3 2084 if (t == null) 2085 return this; 2086 if (this.basedOn == null) 2087 this.basedOn = new ArrayList<Reference>(); 2088 this.basedOn.add(t); 2089 return this; 2090 } 2091 2092 /** 2093 * @return The first repetition of repeating field {@link #basedOn}, creating it 2094 * if it does not already exist 2095 */ 2096 public Reference getBasedOnFirstRep() { 2097 if (getBasedOn().isEmpty()) { 2098 addBasedOn(); 2099 } 2100 return getBasedOn().get(0); 2101 } 2102 2103 /** 2104 * @deprecated Use Reference#setResource(IBaseResource) instead 2105 */ 2106 @Deprecated 2107 public List<Resource> getBasedOnTarget() { 2108 if (this.basedOnTarget == null) 2109 this.basedOnTarget = new ArrayList<Resource>(); 2110 return this.basedOnTarget; 2111 } 2112 2113 /** 2114 * @return {@link #partOf} (A larger event of which this particular Observation 2115 * is a component or step. For example, an observation as part of a 2116 * procedure.) 2117 */ 2118 public List<Reference> getPartOf() { 2119 if (this.partOf == null) 2120 this.partOf = new ArrayList<Reference>(); 2121 return this.partOf; 2122 } 2123 2124 /** 2125 * @return Returns a reference to <code>this</code> for easy method chaining 2126 */ 2127 public Observation setPartOf(List<Reference> thePartOf) { 2128 this.partOf = thePartOf; 2129 return this; 2130 } 2131 2132 public boolean hasPartOf() { 2133 if (this.partOf == null) 2134 return false; 2135 for (Reference item : this.partOf) 2136 if (!item.isEmpty()) 2137 return true; 2138 return false; 2139 } 2140 2141 public Reference addPartOf() { // 3 2142 Reference t = new Reference(); 2143 if (this.partOf == null) 2144 this.partOf = new ArrayList<Reference>(); 2145 this.partOf.add(t); 2146 return t; 2147 } 2148 2149 public Observation addPartOf(Reference t) { // 3 2150 if (t == null) 2151 return this; 2152 if (this.partOf == null) 2153 this.partOf = new ArrayList<Reference>(); 2154 this.partOf.add(t); 2155 return this; 2156 } 2157 2158 /** 2159 * @return The first repetition of repeating field {@link #partOf}, creating it 2160 * if it does not already exist 2161 */ 2162 public Reference getPartOfFirstRep() { 2163 if (getPartOf().isEmpty()) { 2164 addPartOf(); 2165 } 2166 return getPartOf().get(0); 2167 } 2168 2169 /** 2170 * @deprecated Use Reference#setResource(IBaseResource) instead 2171 */ 2172 @Deprecated 2173 public List<Resource> getPartOfTarget() { 2174 if (this.partOfTarget == null) 2175 this.partOfTarget = new ArrayList<Resource>(); 2176 return this.partOfTarget; 2177 } 2178 2179 /** 2180 * @return {@link #status} (The status of the result value.). This is the 2181 * underlying object with id, value and extensions. The accessor 2182 * "getStatus" gives direct access to the value 2183 */ 2184 public Enumeration<ObservationStatus> getStatusElement() { 2185 if (this.status == null) 2186 if (Configuration.errorOnAutoCreate()) 2187 throw new Error("Attempt to auto-create Observation.status"); 2188 else if (Configuration.doAutoCreate()) 2189 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); // bb 2190 return this.status; 2191 } 2192 2193 public boolean hasStatusElement() { 2194 return this.status != null && !this.status.isEmpty(); 2195 } 2196 2197 public boolean hasStatus() { 2198 return this.status != null && !this.status.isEmpty(); 2199 } 2200 2201 /** 2202 * @param value {@link #status} (The status of the result value.). This is the 2203 * underlying object with id, value and extensions. The accessor 2204 * "getStatus" gives direct access to the value 2205 */ 2206 public Observation setStatusElement(Enumeration<ObservationStatus> value) { 2207 this.status = value; 2208 return this; 2209 } 2210 2211 /** 2212 * @return The status of the result value. 2213 */ 2214 public ObservationStatus getStatus() { 2215 return this.status == null ? null : this.status.getValue(); 2216 } 2217 2218 /** 2219 * @param value The status of the result value. 2220 */ 2221 public Observation setStatus(ObservationStatus value) { 2222 if (this.status == null) 2223 this.status = new Enumeration<ObservationStatus>(new ObservationStatusEnumFactory()); 2224 this.status.setValue(value); 2225 return this; 2226 } 2227 2228 /** 2229 * @return {@link #category} (A code that classifies the general type of 2230 * observation being made.) 2231 */ 2232 public List<CodeableConcept> getCategory() { 2233 if (this.category == null) 2234 this.category = new ArrayList<CodeableConcept>(); 2235 return this.category; 2236 } 2237 2238 /** 2239 * @return Returns a reference to <code>this</code> for easy method chaining 2240 */ 2241 public Observation setCategory(List<CodeableConcept> theCategory) { 2242 this.category = theCategory; 2243 return this; 2244 } 2245 2246 public boolean hasCategory() { 2247 if (this.category == null) 2248 return false; 2249 for (CodeableConcept item : this.category) 2250 if (!item.isEmpty()) 2251 return true; 2252 return false; 2253 } 2254 2255 public CodeableConcept addCategory() { // 3 2256 CodeableConcept t = new CodeableConcept(); 2257 if (this.category == null) 2258 this.category = new ArrayList<CodeableConcept>(); 2259 this.category.add(t); 2260 return t; 2261 } 2262 2263 public Observation addCategory(CodeableConcept t) { // 3 2264 if (t == null) 2265 return this; 2266 if (this.category == null) 2267 this.category = new ArrayList<CodeableConcept>(); 2268 this.category.add(t); 2269 return this; 2270 } 2271 2272 /** 2273 * @return The first repetition of repeating field {@link #category}, creating 2274 * it if it does not already exist 2275 */ 2276 public CodeableConcept getCategoryFirstRep() { 2277 if (getCategory().isEmpty()) { 2278 addCategory(); 2279 } 2280 return getCategory().get(0); 2281 } 2282 2283 /** 2284 * @return {@link #code} (Describes what was observed. Sometimes this is called 2285 * the observation "name".) 2286 */ 2287 public CodeableConcept getCode() { 2288 if (this.code == null) 2289 if (Configuration.errorOnAutoCreate()) 2290 throw new Error("Attempt to auto-create Observation.code"); 2291 else if (Configuration.doAutoCreate()) 2292 this.code = new CodeableConcept(); // cc 2293 return this.code; 2294 } 2295 2296 public boolean hasCode() { 2297 return this.code != null && !this.code.isEmpty(); 2298 } 2299 2300 /** 2301 * @param value {@link #code} (Describes what was observed. Sometimes this is 2302 * called the observation "name".) 2303 */ 2304 public Observation setCode(CodeableConcept value) { 2305 this.code = value; 2306 return this; 2307 } 2308 2309 /** 2310 * @return {@link #subject} (The patient, or group of patients, location, or 2311 * device this observation is about and into whose record the 2312 * observation is placed. If the actual focus of the observation is 2313 * different from the subject (or a sample of, part, or region of the 2314 * subject), the `focus` element or the `code` itself specifies the 2315 * actual focus of the observation.) 2316 */ 2317 public Reference getSubject() { 2318 if (this.subject == null) 2319 if (Configuration.errorOnAutoCreate()) 2320 throw new Error("Attempt to auto-create Observation.subject"); 2321 else if (Configuration.doAutoCreate()) 2322 this.subject = new Reference(); // cc 2323 return this.subject; 2324 } 2325 2326 public boolean hasSubject() { 2327 return this.subject != null && !this.subject.isEmpty(); 2328 } 2329 2330 /** 2331 * @param value {@link #subject} (The patient, or group of patients, location, 2332 * or device this observation is about and into whose record the 2333 * observation is placed. If the actual focus of the observation is 2334 * different from the subject (or a sample of, part, or region of 2335 * the subject), the `focus` element or the `code` itself specifies 2336 * the actual focus of the observation.) 2337 */ 2338 public Observation setSubject(Reference value) { 2339 this.subject = value; 2340 return this; 2341 } 2342 2343 /** 2344 * @return {@link #subject} The actual object that is the target of the 2345 * reference. The reference library doesn't populate this, but you can 2346 * use it to hold the resource if you resolve it. (The patient, or group 2347 * of patients, location, or device this observation is about and into 2348 * whose record the observation is placed. If the actual focus of the 2349 * observation is different from the subject (or a sample of, part, or 2350 * region of the subject), the `focus` element or the `code` itself 2351 * specifies the actual focus of the observation.) 2352 */ 2353 public Resource getSubjectTarget() { 2354 return this.subjectTarget; 2355 } 2356 2357 /** 2358 * @param value {@link #subject} The actual object that is the target of the 2359 * reference. The reference library doesn't use these, but you can 2360 * use it to hold the resource if you resolve it. (The patient, or 2361 * group of patients, location, or device this observation is about 2362 * and into whose record the observation is placed. If the actual 2363 * focus of the observation is different from the subject (or a 2364 * sample of, part, or region of the subject), the `focus` element 2365 * or the `code` itself specifies the actual focus of the 2366 * observation.) 2367 */ 2368 public Observation setSubjectTarget(Resource value) { 2369 this.subjectTarget = value; 2370 return this; 2371 } 2372 2373 /** 2374 * @return {@link #focus} (The actual focus of an observation when it is not the 2375 * patient of record representing something or someone associated with 2376 * the patient such as a spouse, parent, fetus, or donor. For example, 2377 * fetus observations in a mother's record. The focus of an observation 2378 * could also be an existing condition, an intervention, the subject's 2379 * diet, another observation of the subject, or a body structure such as 2380 * tumor or implanted device. An example use case would be using the 2381 * Observation resource to capture whether the mother is trained to 2382 * change her child's tracheostomy tube. In this example, the child is 2383 * the patient of record and the mother is the focus.) 2384 */ 2385 public List<Reference> getFocus() { 2386 if (this.focus == null) 2387 this.focus = new ArrayList<Reference>(); 2388 return this.focus; 2389 } 2390 2391 /** 2392 * @return Returns a reference to <code>this</code> for easy method chaining 2393 */ 2394 public Observation setFocus(List<Reference> theFocus) { 2395 this.focus = theFocus; 2396 return this; 2397 } 2398 2399 public boolean hasFocus() { 2400 if (this.focus == null) 2401 return false; 2402 for (Reference item : this.focus) 2403 if (!item.isEmpty()) 2404 return true; 2405 return false; 2406 } 2407 2408 public Reference addFocus() { // 3 2409 Reference t = new Reference(); 2410 if (this.focus == null) 2411 this.focus = new ArrayList<Reference>(); 2412 this.focus.add(t); 2413 return t; 2414 } 2415 2416 public Observation addFocus(Reference t) { // 3 2417 if (t == null) 2418 return this; 2419 if (this.focus == null) 2420 this.focus = new ArrayList<Reference>(); 2421 this.focus.add(t); 2422 return this; 2423 } 2424 2425 /** 2426 * @return The first repetition of repeating field {@link #focus}, creating it 2427 * if it does not already exist 2428 */ 2429 public Reference getFocusFirstRep() { 2430 if (getFocus().isEmpty()) { 2431 addFocus(); 2432 } 2433 return getFocus().get(0); 2434 } 2435 2436 /** 2437 * @deprecated Use Reference#setResource(IBaseResource) instead 2438 */ 2439 @Deprecated 2440 public List<Resource> getFocusTarget() { 2441 if (this.focusTarget == null) 2442 this.focusTarget = new ArrayList<Resource>(); 2443 return this.focusTarget; 2444 } 2445 2446 /** 2447 * @return {@link #encounter} (The healthcare event (e.g. a patient and 2448 * healthcare provider interaction) during which this observation is 2449 * made.) 2450 */ 2451 public Reference getEncounter() { 2452 if (this.encounter == null) 2453 if (Configuration.errorOnAutoCreate()) 2454 throw new Error("Attempt to auto-create Observation.encounter"); 2455 else if (Configuration.doAutoCreate()) 2456 this.encounter = new Reference(); // cc 2457 return this.encounter; 2458 } 2459 2460 public boolean hasEncounter() { 2461 return this.encounter != null && !this.encounter.isEmpty(); 2462 } 2463 2464 /** 2465 * @param value {@link #encounter} (The healthcare event (e.g. a patient and 2466 * healthcare provider interaction) during which this observation 2467 * is made.) 2468 */ 2469 public Observation setEncounter(Reference value) { 2470 this.encounter = value; 2471 return this; 2472 } 2473 2474 /** 2475 * @return {@link #encounter} The actual object that is the target of the 2476 * reference. The reference library doesn't populate this, but you can 2477 * use it to hold the resource if you resolve it. (The healthcare event 2478 * (e.g. a patient and healthcare provider interaction) during which 2479 * this observation is made.) 2480 */ 2481 public Encounter getEncounterTarget() { 2482 if (this.encounterTarget == null) 2483 if (Configuration.errorOnAutoCreate()) 2484 throw new Error("Attempt to auto-create Observation.encounter"); 2485 else if (Configuration.doAutoCreate()) 2486 this.encounterTarget = new Encounter(); // aa 2487 return this.encounterTarget; 2488 } 2489 2490 /** 2491 * @param value {@link #encounter} The actual object that is the target of the 2492 * reference. The reference library doesn't use these, but you can 2493 * use it to hold the resource if you resolve it. (The healthcare 2494 * event (e.g. a patient and healthcare provider interaction) 2495 * during which this observation is made.) 2496 */ 2497 public Observation setEncounterTarget(Encounter value) { 2498 this.encounterTarget = value; 2499 return this; 2500 } 2501 2502 /** 2503 * @return {@link #effective} (The time or time-period the observed value is 2504 * asserted as being true. For biological subjects - e.g. human patients 2505 * - this is usually called the "physiologically relevant time". This is 2506 * usually either the time of the procedure or of specimen collection, 2507 * but very often the source of the date/time is not known, only the 2508 * date/time itself.) 2509 */ 2510 public Type getEffective() { 2511 return this.effective; 2512 } 2513 2514 /** 2515 * @return {@link #effective} (The time or time-period the observed value is 2516 * asserted as being true. For biological subjects - e.g. human patients 2517 * - this is usually called the "physiologically relevant time". This is 2518 * usually either the time of the procedure or of specimen collection, 2519 * but very often the source of the date/time is not known, only the 2520 * date/time itself.) 2521 */ 2522 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 2523 if (this.effective == null) 2524 this.effective = new DateTimeType(); 2525 if (!(this.effective instanceof DateTimeType)) 2526 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2527 + this.effective.getClass().getName() + " was encountered"); 2528 return (DateTimeType) this.effective; 2529 } 2530 2531 public boolean hasEffectiveDateTimeType() { 2532 return this != null && this.effective instanceof DateTimeType; 2533 } 2534 2535 /** 2536 * @return {@link #effective} (The time or time-period the observed value is 2537 * asserted as being true. For biological subjects - e.g. human patients 2538 * - this is usually called the "physiologically relevant time". This is 2539 * usually either the time of the procedure or of specimen collection, 2540 * but very often the source of the date/time is not known, only the 2541 * date/time itself.) 2542 */ 2543 public Period getEffectivePeriod() throws FHIRException { 2544 if (this.effective == null) 2545 this.effective = new Period(); 2546 if (!(this.effective instanceof Period)) 2547 throw new FHIRException("Type mismatch: the type Period was expected, but " + this.effective.getClass().getName() 2548 + " was encountered"); 2549 return (Period) this.effective; 2550 } 2551 2552 public boolean hasEffectivePeriod() { 2553 return this != null && this.effective instanceof Period; 2554 } 2555 2556 /** 2557 * @return {@link #effective} (The time or time-period the observed value is 2558 * asserted as being true. For biological subjects - e.g. human patients 2559 * - this is usually called the "physiologically relevant time". This is 2560 * usually either the time of the procedure or of specimen collection, 2561 * but very often the source of the date/time is not known, only the 2562 * date/time itself.) 2563 */ 2564 public Timing getEffectiveTiming() throws FHIRException { 2565 if (this.effective == null) 2566 this.effective = new Timing(); 2567 if (!(this.effective instanceof Timing)) 2568 throw new FHIRException("Type mismatch: the type Timing was expected, but " + this.effective.getClass().getName() 2569 + " was encountered"); 2570 return (Timing) this.effective; 2571 } 2572 2573 public boolean hasEffectiveTiming() { 2574 return this != null && this.effective instanceof Timing; 2575 } 2576 2577 /** 2578 * @return {@link #effective} (The time or time-period the observed value is 2579 * asserted as being true. For biological subjects - e.g. human patients 2580 * - this is usually called the "physiologically relevant time". This is 2581 * usually either the time of the procedure or of specimen collection, 2582 * but very often the source of the date/time is not known, only the 2583 * date/time itself.) 2584 */ 2585 public InstantType getEffectiveInstantType() throws FHIRException { 2586 if (this.effective == null) 2587 this.effective = new InstantType(); 2588 if (!(this.effective instanceof InstantType)) 2589 throw new FHIRException("Type mismatch: the type InstantType was expected, but " 2590 + this.effective.getClass().getName() + " was encountered"); 2591 return (InstantType) this.effective; 2592 } 2593 2594 public boolean hasEffectiveInstantType() { 2595 return this != null && this.effective instanceof InstantType; 2596 } 2597 2598 public boolean hasEffective() { 2599 return this.effective != null && !this.effective.isEmpty(); 2600 } 2601 2602 /** 2603 * @param value {@link #effective} (The time or time-period the observed value 2604 * is asserted as being true. For biological subjects - e.g. human 2605 * patients - this is usually called the "physiologically relevant 2606 * time". This is usually either the time of the procedure or of 2607 * specimen collection, but very often the source of the date/time 2608 * is not known, only the date/time itself.) 2609 */ 2610 public Observation setEffective(Type value) { 2611 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing 2612 || value instanceof InstantType)) 2613 throw new Error("Not the right type for Observation.effective[x]: " + value.fhirType()); 2614 this.effective = value; 2615 return this; 2616 } 2617 2618 /** 2619 * @return {@link #issued} (The date and time this version of the observation 2620 * was made available to providers, typically after the results have 2621 * been reviewed and verified.). This is the underlying object with id, 2622 * value and extensions. The accessor "getIssued" gives direct access to 2623 * the value 2624 */ 2625 public InstantType getIssuedElement() { 2626 if (this.issued == null) 2627 if (Configuration.errorOnAutoCreate()) 2628 throw new Error("Attempt to auto-create Observation.issued"); 2629 else if (Configuration.doAutoCreate()) 2630 this.issued = new InstantType(); // bb 2631 return this.issued; 2632 } 2633 2634 public boolean hasIssuedElement() { 2635 return this.issued != null && !this.issued.isEmpty(); 2636 } 2637 2638 public boolean hasIssued() { 2639 return this.issued != null && !this.issued.isEmpty(); 2640 } 2641 2642 /** 2643 * @param value {@link #issued} (The date and time this version of the 2644 * observation was made available to providers, typically after the 2645 * results have been reviewed and verified.). This is the 2646 * underlying object with id, value and extensions. The accessor 2647 * "getIssued" gives direct access to the value 2648 */ 2649 public Observation setIssuedElement(InstantType value) { 2650 this.issued = value; 2651 return this; 2652 } 2653 2654 /** 2655 * @return The date and time this version of the observation was made available 2656 * to providers, typically after the results have been reviewed and 2657 * verified. 2658 */ 2659 public Date getIssued() { 2660 return this.issued == null ? null : this.issued.getValue(); 2661 } 2662 2663 /** 2664 * @param value The date and time this version of the observation was made 2665 * available to providers, typically after the results have been 2666 * reviewed and verified. 2667 */ 2668 public Observation setIssued(Date value) { 2669 if (value == null) 2670 this.issued = null; 2671 else { 2672 if (this.issued == null) 2673 this.issued = new InstantType(); 2674 this.issued.setValue(value); 2675 } 2676 return this; 2677 } 2678 2679 /** 2680 * @return {@link #performer} (Who was responsible for asserting the observed 2681 * value as "true".) 2682 */ 2683 public List<Reference> getPerformer() { 2684 if (this.performer == null) 2685 this.performer = new ArrayList<Reference>(); 2686 return this.performer; 2687 } 2688 2689 /** 2690 * @return Returns a reference to <code>this</code> for easy method chaining 2691 */ 2692 public Observation setPerformer(List<Reference> thePerformer) { 2693 this.performer = thePerformer; 2694 return this; 2695 } 2696 2697 public boolean hasPerformer() { 2698 if (this.performer == null) 2699 return false; 2700 for (Reference item : this.performer) 2701 if (!item.isEmpty()) 2702 return true; 2703 return false; 2704 } 2705 2706 public Reference addPerformer() { // 3 2707 Reference t = new Reference(); 2708 if (this.performer == null) 2709 this.performer = new ArrayList<Reference>(); 2710 this.performer.add(t); 2711 return t; 2712 } 2713 2714 public Observation addPerformer(Reference t) { // 3 2715 if (t == null) 2716 return this; 2717 if (this.performer == null) 2718 this.performer = new ArrayList<Reference>(); 2719 this.performer.add(t); 2720 return this; 2721 } 2722 2723 /** 2724 * @return The first repetition of repeating field {@link #performer}, creating 2725 * it if it does not already exist 2726 */ 2727 public Reference getPerformerFirstRep() { 2728 if (getPerformer().isEmpty()) { 2729 addPerformer(); 2730 } 2731 return getPerformer().get(0); 2732 } 2733 2734 /** 2735 * @deprecated Use Reference#setResource(IBaseResource) instead 2736 */ 2737 @Deprecated 2738 public List<Resource> getPerformerTarget() { 2739 if (this.performerTarget == null) 2740 this.performerTarget = new ArrayList<Resource>(); 2741 return this.performerTarget; 2742 } 2743 2744 /** 2745 * @return {@link #value} (The information determined as a result of making the 2746 * observation, if the information has a simple value.) 2747 */ 2748 public Type getValue() { 2749 return this.value; 2750 } 2751 2752 /** 2753 * @return {@link #value} (The information determined as a result of making the 2754 * observation, if the information has a simple value.) 2755 */ 2756 public Quantity getValueQuantity() throws FHIRException { 2757 if (this.value == null) 2758 this.value = new Quantity(); 2759 if (!(this.value instanceof Quantity)) 2760 throw new FHIRException( 2761 "Type mismatch: the type Quantity was expected, but " + this.value.getClass().getName() + " was encountered"); 2762 return (Quantity) this.value; 2763 } 2764 2765 public boolean hasValueQuantity() { 2766 return this != null && this.value instanceof Quantity; 2767 } 2768 2769 /** 2770 * @return {@link #value} (The information determined as a result of making the 2771 * observation, if the information has a simple value.) 2772 */ 2773 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2774 if (this.value == null) 2775 this.value = new CodeableConcept(); 2776 if (!(this.value instanceof CodeableConcept)) 2777 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2778 + this.value.getClass().getName() + " was encountered"); 2779 return (CodeableConcept) this.value; 2780 } 2781 2782 public boolean hasValueCodeableConcept() { 2783 return this != null && this.value instanceof CodeableConcept; 2784 } 2785 2786 /** 2787 * @return {@link #value} (The information determined as a result of making the 2788 * observation, if the information has a simple value.) 2789 */ 2790 public StringType getValueStringType() throws FHIRException { 2791 if (this.value == null) 2792 this.value = new StringType(); 2793 if (!(this.value instanceof StringType)) 2794 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.value.getClass().getName() 2795 + " was encountered"); 2796 return (StringType) this.value; 2797 } 2798 2799 public boolean hasValueStringType() { 2800 return this != null && this.value instanceof StringType; 2801 } 2802 2803 /** 2804 * @return {@link #value} (The information determined as a result of making the 2805 * observation, if the information has a simple value.) 2806 */ 2807 public BooleanType getValueBooleanType() throws FHIRException { 2808 if (this.value == null) 2809 this.value = new BooleanType(); 2810 if (!(this.value instanceof BooleanType)) 2811 throw new FHIRException("Type mismatch: the type BooleanType was expected, but " + this.value.getClass().getName() 2812 + " was encountered"); 2813 return (BooleanType) this.value; 2814 } 2815 2816 public boolean hasValueBooleanType() { 2817 return this != null && this.value instanceof BooleanType; 2818 } 2819 2820 /** 2821 * @return {@link #value} (The information determined as a result of making the 2822 * observation, if the information has a simple value.) 2823 */ 2824 public IntegerType getValueIntegerType() throws FHIRException { 2825 if (this.value == null) 2826 this.value = new IntegerType(); 2827 if (!(this.value instanceof IntegerType)) 2828 throw new FHIRException("Type mismatch: the type IntegerType was expected, but " + this.value.getClass().getName() 2829 + " was encountered"); 2830 return (IntegerType) this.value; 2831 } 2832 2833 public boolean hasValueIntegerType() { 2834 return this != null && this.value instanceof IntegerType; 2835 } 2836 2837 /** 2838 * @return {@link #value} (The information determined as a result of making the 2839 * observation, if the information has a simple value.) 2840 */ 2841 public Range getValueRange() throws FHIRException { 2842 if (this.value == null) 2843 this.value = new Range(); 2844 if (!(this.value instanceof Range)) 2845 throw new FHIRException( 2846 "Type mismatch: the type Range was expected, but " + this.value.getClass().getName() + " was encountered"); 2847 return (Range) this.value; 2848 } 2849 2850 public boolean hasValueRange() { 2851 return this != null && this.value instanceof Range; 2852 } 2853 2854 /** 2855 * @return {@link #value} (The information determined as a result of making the 2856 * observation, if the information has a simple value.) 2857 */ 2858 public Ratio getValueRatio() throws FHIRException { 2859 if (this.value == null) 2860 this.value = new Ratio(); 2861 if (!(this.value instanceof Ratio)) 2862 throw new FHIRException( 2863 "Type mismatch: the type Ratio was expected, but " + this.value.getClass().getName() + " was encountered"); 2864 return (Ratio) this.value; 2865 } 2866 2867 public boolean hasValueRatio() { 2868 return this != null && this.value instanceof Ratio; 2869 } 2870 2871 /** 2872 * @return {@link #value} (The information determined as a result of making the 2873 * observation, if the information has a simple value.) 2874 */ 2875 public SampledData getValueSampledData() throws FHIRException { 2876 if (this.value == null) 2877 this.value = new SampledData(); 2878 if (!(this.value instanceof SampledData)) 2879 throw new FHIRException("Type mismatch: the type SampledData was expected, but " + this.value.getClass().getName() 2880 + " was encountered"); 2881 return (SampledData) this.value; 2882 } 2883 2884 public boolean hasValueSampledData() { 2885 return this != null && this.value instanceof SampledData; 2886 } 2887 2888 /** 2889 * @return {@link #value} (The information determined as a result of making the 2890 * observation, if the information has a simple value.) 2891 */ 2892 public TimeType getValueTimeType() throws FHIRException { 2893 if (this.value == null) 2894 this.value = new TimeType(); 2895 if (!(this.value instanceof TimeType)) 2896 throw new FHIRException( 2897 "Type mismatch: the type TimeType was expected, but " + this.value.getClass().getName() + " was encountered"); 2898 return (TimeType) this.value; 2899 } 2900 2901 public boolean hasValueTimeType() { 2902 return this != null && this.value instanceof TimeType; 2903 } 2904 2905 /** 2906 * @return {@link #value} (The information determined as a result of making the 2907 * observation, if the information has a simple value.) 2908 */ 2909 public DateTimeType getValueDateTimeType() throws FHIRException { 2910 if (this.value == null) 2911 this.value = new DateTimeType(); 2912 if (!(this.value instanceof DateTimeType)) 2913 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but " 2914 + this.value.getClass().getName() + " was encountered"); 2915 return (DateTimeType) this.value; 2916 } 2917 2918 public boolean hasValueDateTimeType() { 2919 return this != null && this.value instanceof DateTimeType; 2920 } 2921 2922 /** 2923 * @return {@link #value} (The information determined as a result of making the 2924 * observation, if the information has a simple value.) 2925 */ 2926 public Period getValuePeriod() throws FHIRException { 2927 if (this.value == null) 2928 this.value = new Period(); 2929 if (!(this.value instanceof Period)) 2930 throw new FHIRException( 2931 "Type mismatch: the type Period was expected, but " + this.value.getClass().getName() + " was encountered"); 2932 return (Period) this.value; 2933 } 2934 2935 public boolean hasValuePeriod() { 2936 return this != null && this.value instanceof Period; 2937 } 2938 2939 public boolean hasValue() { 2940 return this.value != null && !this.value.isEmpty(); 2941 } 2942 2943 /** 2944 * @param value {@link #value} (The information determined as a result of making 2945 * the observation, if the information has a simple value.) 2946 */ 2947 public Observation setValue(Type value) { 2948 if (value != null && !(value instanceof Quantity || value instanceof CodeableConcept || value instanceof StringType 2949 || value instanceof BooleanType || value instanceof IntegerType || value instanceof Range 2950 || value instanceof Ratio || value instanceof SampledData || value instanceof TimeType 2951 || value instanceof DateTimeType || value instanceof Period)) 2952 throw new Error("Not the right type for Observation.value[x]: " + value.fhirType()); 2953 this.value = value; 2954 return this; 2955 } 2956 2957 /** 2958 * @return {@link #dataAbsentReason} (Provides a reason why the expected value 2959 * in the element Observation.value[x] is missing.) 2960 */ 2961 public CodeableConcept getDataAbsentReason() { 2962 if (this.dataAbsentReason == null) 2963 if (Configuration.errorOnAutoCreate()) 2964 throw new Error("Attempt to auto-create Observation.dataAbsentReason"); 2965 else if (Configuration.doAutoCreate()) 2966 this.dataAbsentReason = new CodeableConcept(); // cc 2967 return this.dataAbsentReason; 2968 } 2969 2970 public boolean hasDataAbsentReason() { 2971 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 2972 } 2973 2974 /** 2975 * @param value {@link #dataAbsentReason} (Provides a reason why the expected 2976 * value in the element Observation.value[x] is missing.) 2977 */ 2978 public Observation setDataAbsentReason(CodeableConcept value) { 2979 this.dataAbsentReason = value; 2980 return this; 2981 } 2982 2983 /** 2984 * @return {@link #interpretation} (A categorical assessment of an observation 2985 * value. For example, high, low, normal.) 2986 */ 2987 public List<CodeableConcept> getInterpretation() { 2988 if (this.interpretation == null) 2989 this.interpretation = new ArrayList<CodeableConcept>(); 2990 return this.interpretation; 2991 } 2992 2993 /** 2994 * @return Returns a reference to <code>this</code> for easy method chaining 2995 */ 2996 public Observation setInterpretation(List<CodeableConcept> theInterpretation) { 2997 this.interpretation = theInterpretation; 2998 return this; 2999 } 3000 3001 public boolean hasInterpretation() { 3002 if (this.interpretation == null) 3003 return false; 3004 for (CodeableConcept item : this.interpretation) 3005 if (!item.isEmpty()) 3006 return true; 3007 return false; 3008 } 3009 3010 public CodeableConcept addInterpretation() { // 3 3011 CodeableConcept t = new CodeableConcept(); 3012 if (this.interpretation == null) 3013 this.interpretation = new ArrayList<CodeableConcept>(); 3014 this.interpretation.add(t); 3015 return t; 3016 } 3017 3018 public Observation addInterpretation(CodeableConcept t) { // 3 3019 if (t == null) 3020 return this; 3021 if (this.interpretation == null) 3022 this.interpretation = new ArrayList<CodeableConcept>(); 3023 this.interpretation.add(t); 3024 return this; 3025 } 3026 3027 /** 3028 * @return The first repetition of repeating field {@link #interpretation}, 3029 * creating it if it does not already exist 3030 */ 3031 public CodeableConcept getInterpretationFirstRep() { 3032 if (getInterpretation().isEmpty()) { 3033 addInterpretation(); 3034 } 3035 return getInterpretation().get(0); 3036 } 3037 3038 /** 3039 * @return {@link #note} (Comments about the observation or the results.) 3040 */ 3041 public List<Annotation> getNote() { 3042 if (this.note == null) 3043 this.note = new ArrayList<Annotation>(); 3044 return this.note; 3045 } 3046 3047 /** 3048 * @return Returns a reference to <code>this</code> for easy method chaining 3049 */ 3050 public Observation setNote(List<Annotation> theNote) { 3051 this.note = theNote; 3052 return this; 3053 } 3054 3055 public boolean hasNote() { 3056 if (this.note == null) 3057 return false; 3058 for (Annotation item : this.note) 3059 if (!item.isEmpty()) 3060 return true; 3061 return false; 3062 } 3063 3064 public Annotation addNote() { // 3 3065 Annotation t = new Annotation(); 3066 if (this.note == null) 3067 this.note = new ArrayList<Annotation>(); 3068 this.note.add(t); 3069 return t; 3070 } 3071 3072 public Observation addNote(Annotation t) { // 3 3073 if (t == null) 3074 return this; 3075 if (this.note == null) 3076 this.note = new ArrayList<Annotation>(); 3077 this.note.add(t); 3078 return this; 3079 } 3080 3081 /** 3082 * @return The first repetition of repeating field {@link #note}, creating it if 3083 * it does not already exist 3084 */ 3085 public Annotation getNoteFirstRep() { 3086 if (getNote().isEmpty()) { 3087 addNote(); 3088 } 3089 return getNote().get(0); 3090 } 3091 3092 /** 3093 * @return {@link #bodySite} (Indicates the site on the subject's body where the 3094 * observation was made (i.e. the target site).) 3095 */ 3096 public CodeableConcept getBodySite() { 3097 if (this.bodySite == null) 3098 if (Configuration.errorOnAutoCreate()) 3099 throw new Error("Attempt to auto-create Observation.bodySite"); 3100 else if (Configuration.doAutoCreate()) 3101 this.bodySite = new CodeableConcept(); // cc 3102 return this.bodySite; 3103 } 3104 3105 public boolean hasBodySite() { 3106 return this.bodySite != null && !this.bodySite.isEmpty(); 3107 } 3108 3109 /** 3110 * @param value {@link #bodySite} (Indicates the site on the subject's body 3111 * where the observation was made (i.e. the target site).) 3112 */ 3113 public Observation setBodySite(CodeableConcept value) { 3114 this.bodySite = value; 3115 return this; 3116 } 3117 3118 /** 3119 * @return {@link #method} (Indicates the mechanism used to perform the 3120 * observation.) 3121 */ 3122 public CodeableConcept getMethod() { 3123 if (this.method == null) 3124 if (Configuration.errorOnAutoCreate()) 3125 throw new Error("Attempt to auto-create Observation.method"); 3126 else if (Configuration.doAutoCreate()) 3127 this.method = new CodeableConcept(); // cc 3128 return this.method; 3129 } 3130 3131 public boolean hasMethod() { 3132 return this.method != null && !this.method.isEmpty(); 3133 } 3134 3135 /** 3136 * @param value {@link #method} (Indicates the mechanism used to perform the 3137 * observation.) 3138 */ 3139 public Observation setMethod(CodeableConcept value) { 3140 this.method = value; 3141 return this; 3142 } 3143 3144 /** 3145 * @return {@link #specimen} (The specimen that was used when this observation 3146 * was made.) 3147 */ 3148 public Reference getSpecimen() { 3149 if (this.specimen == null) 3150 if (Configuration.errorOnAutoCreate()) 3151 throw new Error("Attempt to auto-create Observation.specimen"); 3152 else if (Configuration.doAutoCreate()) 3153 this.specimen = new Reference(); // cc 3154 return this.specimen; 3155 } 3156 3157 public boolean hasSpecimen() { 3158 return this.specimen != null && !this.specimen.isEmpty(); 3159 } 3160 3161 /** 3162 * @param value {@link #specimen} (The specimen that was used when this 3163 * observation was made.) 3164 */ 3165 public Observation setSpecimen(Reference value) { 3166 this.specimen = value; 3167 return this; 3168 } 3169 3170 /** 3171 * @return {@link #specimen} The actual object that is the target of the 3172 * reference. The reference library doesn't populate this, but you can 3173 * use it to hold the resource if you resolve it. (The specimen that was 3174 * used when this observation was made.) 3175 */ 3176 public Specimen getSpecimenTarget() { 3177 if (this.specimenTarget == null) 3178 if (Configuration.errorOnAutoCreate()) 3179 throw new Error("Attempt to auto-create Observation.specimen"); 3180 else if (Configuration.doAutoCreate()) 3181 this.specimenTarget = new Specimen(); // aa 3182 return this.specimenTarget; 3183 } 3184 3185 /** 3186 * @param value {@link #specimen} The actual object that is the target of the 3187 * reference. The reference library doesn't use these, but you can 3188 * use it to hold the resource if you resolve it. (The specimen 3189 * that was used when this observation was made.) 3190 */ 3191 public Observation setSpecimenTarget(Specimen value) { 3192 this.specimenTarget = value; 3193 return this; 3194 } 3195 3196 /** 3197 * @return {@link #device} (The device used to generate the observation data.) 3198 */ 3199 public Reference getDevice() { 3200 if (this.device == null) 3201 if (Configuration.errorOnAutoCreate()) 3202 throw new Error("Attempt to auto-create Observation.device"); 3203 else if (Configuration.doAutoCreate()) 3204 this.device = new Reference(); // cc 3205 return this.device; 3206 } 3207 3208 public boolean hasDevice() { 3209 return this.device != null && !this.device.isEmpty(); 3210 } 3211 3212 /** 3213 * @param value {@link #device} (The device used to generate the observation 3214 * data.) 3215 */ 3216 public Observation setDevice(Reference value) { 3217 this.device = value; 3218 return this; 3219 } 3220 3221 /** 3222 * @return {@link #device} The actual object that is the target of the 3223 * reference. The reference library doesn't populate this, but you can 3224 * use it to hold the resource if you resolve it. (The device used to 3225 * generate the observation data.) 3226 */ 3227 public Resource getDeviceTarget() { 3228 return this.deviceTarget; 3229 } 3230 3231 /** 3232 * @param value {@link #device} The actual object that is the target of the 3233 * reference. The reference library doesn't use these, but you can 3234 * use it to hold the resource if you resolve it. (The device used 3235 * to generate the observation data.) 3236 */ 3237 public Observation setDeviceTarget(Resource value) { 3238 this.deviceTarget = value; 3239 return this; 3240 } 3241 3242 /** 3243 * @return {@link #referenceRange} (Guidance on how to interpret the value by 3244 * comparison to a normal or recommended range. Multiple reference 3245 * ranges are interpreted as an "OR". In other words, to represent two 3246 * distinct target populations, two `referenceRange` elements would be 3247 * used.) 3248 */ 3249 public List<ObservationReferenceRangeComponent> getReferenceRange() { 3250 if (this.referenceRange == null) 3251 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3252 return this.referenceRange; 3253 } 3254 3255 /** 3256 * @return Returns a reference to <code>this</code> for easy method chaining 3257 */ 3258 public Observation setReferenceRange(List<ObservationReferenceRangeComponent> theReferenceRange) { 3259 this.referenceRange = theReferenceRange; 3260 return this; 3261 } 3262 3263 public boolean hasReferenceRange() { 3264 if (this.referenceRange == null) 3265 return false; 3266 for (ObservationReferenceRangeComponent item : this.referenceRange) 3267 if (!item.isEmpty()) 3268 return true; 3269 return false; 3270 } 3271 3272 public ObservationReferenceRangeComponent addReferenceRange() { // 3 3273 ObservationReferenceRangeComponent t = new ObservationReferenceRangeComponent(); 3274 if (this.referenceRange == null) 3275 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3276 this.referenceRange.add(t); 3277 return t; 3278 } 3279 3280 public Observation addReferenceRange(ObservationReferenceRangeComponent t) { // 3 3281 if (t == null) 3282 return this; 3283 if (this.referenceRange == null) 3284 this.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 3285 this.referenceRange.add(t); 3286 return this; 3287 } 3288 3289 /** 3290 * @return The first repetition of repeating field {@link #referenceRange}, 3291 * creating it if it does not already exist 3292 */ 3293 public ObservationReferenceRangeComponent getReferenceRangeFirstRep() { 3294 if (getReferenceRange().isEmpty()) { 3295 addReferenceRange(); 3296 } 3297 return getReferenceRange().get(0); 3298 } 3299 3300 /** 3301 * @return {@link #hasMember} (This observation is a group observation (e.g. a 3302 * battery, a panel of tests, a set of vital sign measurements) that 3303 * includes the target as a member of the group.) 3304 */ 3305 public List<Reference> getHasMember() { 3306 if (this.hasMember == null) 3307 this.hasMember = new ArrayList<Reference>(); 3308 return this.hasMember; 3309 } 3310 3311 /** 3312 * @return Returns a reference to <code>this</code> for easy method chaining 3313 */ 3314 public Observation setHasMember(List<Reference> theHasMember) { 3315 this.hasMember = theHasMember; 3316 return this; 3317 } 3318 3319 public boolean hasHasMember() { 3320 if (this.hasMember == null) 3321 return false; 3322 for (Reference item : this.hasMember) 3323 if (!item.isEmpty()) 3324 return true; 3325 return false; 3326 } 3327 3328 public Reference addHasMember() { // 3 3329 Reference t = new Reference(); 3330 if (this.hasMember == null) 3331 this.hasMember = new ArrayList<Reference>(); 3332 this.hasMember.add(t); 3333 return t; 3334 } 3335 3336 public Observation addHasMember(Reference t) { // 3 3337 if (t == null) 3338 return this; 3339 if (this.hasMember == null) 3340 this.hasMember = new ArrayList<Reference>(); 3341 this.hasMember.add(t); 3342 return this; 3343 } 3344 3345 /** 3346 * @return The first repetition of repeating field {@link #hasMember}, creating 3347 * it if it does not already exist 3348 */ 3349 public Reference getHasMemberFirstRep() { 3350 if (getHasMember().isEmpty()) { 3351 addHasMember(); 3352 } 3353 return getHasMember().get(0); 3354 } 3355 3356 /** 3357 * @deprecated Use Reference#setResource(IBaseResource) instead 3358 */ 3359 @Deprecated 3360 public List<Resource> getHasMemberTarget() { 3361 if (this.hasMemberTarget == null) 3362 this.hasMemberTarget = new ArrayList<Resource>(); 3363 return this.hasMemberTarget; 3364 } 3365 3366 /** 3367 * @return {@link #derivedFrom} (The target resource that represents a 3368 * measurement from which this observation value is derived. For 3369 * example, a calculated anion gap or a fetal measurement based on an 3370 * ultrasound image.) 3371 */ 3372 public List<Reference> getDerivedFrom() { 3373 if (this.derivedFrom == null) 3374 this.derivedFrom = new ArrayList<Reference>(); 3375 return this.derivedFrom; 3376 } 3377 3378 /** 3379 * @return Returns a reference to <code>this</code> for easy method chaining 3380 */ 3381 public Observation setDerivedFrom(List<Reference> theDerivedFrom) { 3382 this.derivedFrom = theDerivedFrom; 3383 return this; 3384 } 3385 3386 public boolean hasDerivedFrom() { 3387 if (this.derivedFrom == null) 3388 return false; 3389 for (Reference item : this.derivedFrom) 3390 if (!item.isEmpty()) 3391 return true; 3392 return false; 3393 } 3394 3395 public Reference addDerivedFrom() { // 3 3396 Reference t = new Reference(); 3397 if (this.derivedFrom == null) 3398 this.derivedFrom = new ArrayList<Reference>(); 3399 this.derivedFrom.add(t); 3400 return t; 3401 } 3402 3403 public Observation addDerivedFrom(Reference t) { // 3 3404 if (t == null) 3405 return this; 3406 if (this.derivedFrom == null) 3407 this.derivedFrom = new ArrayList<Reference>(); 3408 this.derivedFrom.add(t); 3409 return this; 3410 } 3411 3412 /** 3413 * @return The first repetition of repeating field {@link #derivedFrom}, 3414 * creating it if it does not already exist 3415 */ 3416 public Reference getDerivedFromFirstRep() { 3417 if (getDerivedFrom().isEmpty()) { 3418 addDerivedFrom(); 3419 } 3420 return getDerivedFrom().get(0); 3421 } 3422 3423 /** 3424 * @deprecated Use Reference#setResource(IBaseResource) instead 3425 */ 3426 @Deprecated 3427 public List<Resource> getDerivedFromTarget() { 3428 if (this.derivedFromTarget == null) 3429 this.derivedFromTarget = new ArrayList<Resource>(); 3430 return this.derivedFromTarget; 3431 } 3432 3433 /** 3434 * @return {@link #component} (Some observations have multiple component 3435 * observations. These component observations are expressed as separate 3436 * code value pairs that share the same attributes. Examples include 3437 * systolic and diastolic component observations for blood pressure 3438 * measurement and multiple component observations for genetics 3439 * observations.) 3440 */ 3441 public List<ObservationComponentComponent> getComponent() { 3442 if (this.component == null) 3443 this.component = new ArrayList<ObservationComponentComponent>(); 3444 return this.component; 3445 } 3446 3447 /** 3448 * @return Returns a reference to <code>this</code> for easy method chaining 3449 */ 3450 public Observation setComponent(List<ObservationComponentComponent> theComponent) { 3451 this.component = theComponent; 3452 return this; 3453 } 3454 3455 public boolean hasComponent() { 3456 if (this.component == null) 3457 return false; 3458 for (ObservationComponentComponent item : this.component) 3459 if (!item.isEmpty()) 3460 return true; 3461 return false; 3462 } 3463 3464 public ObservationComponentComponent addComponent() { // 3 3465 ObservationComponentComponent t = new ObservationComponentComponent(); 3466 if (this.component == null) 3467 this.component = new ArrayList<ObservationComponentComponent>(); 3468 this.component.add(t); 3469 return t; 3470 } 3471 3472 public Observation addComponent(ObservationComponentComponent t) { // 3 3473 if (t == null) 3474 return this; 3475 if (this.component == null) 3476 this.component = new ArrayList<ObservationComponentComponent>(); 3477 this.component.add(t); 3478 return this; 3479 } 3480 3481 /** 3482 * @return The first repetition of repeating field {@link #component}, creating 3483 * it if it does not already exist 3484 */ 3485 public ObservationComponentComponent getComponentFirstRep() { 3486 if (getComponent().isEmpty()) { 3487 addComponent(); 3488 } 3489 return getComponent().get(0); 3490 } 3491 3492 protected void listChildren(List<Property> children) { 3493 super.listChildren(children); 3494 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this observation.", 0, 3495 java.lang.Integer.MAX_VALUE, identifier)); 3496 children.add(new Property("basedOn", 3497 "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 3498 "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 3499 0, java.lang.Integer.MAX_VALUE, basedOn)); 3500 children.add(new Property("partOf", 3501 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy)", 3502 "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 3503 0, java.lang.Integer.MAX_VALUE, partOf)); 3504 children.add(new Property("status", "code", "The status of the result value.", 0, 1, status)); 3505 children.add(new Property("category", "CodeableConcept", 3506 "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, 3507 category)); 3508 children.add(new Property("code", "CodeableConcept", 3509 "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code)); 3510 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", 3511 "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 3512 0, 1, subject)); 3513 children.add(new Property("focus", "Reference(Any)", 3514 "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 3515 0, java.lang.Integer.MAX_VALUE, focus)); 3516 children.add(new Property("encounter", "Reference(Encounter)", 3517 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 3518 0, 1, encounter)); 3519 children.add(new Property("effective[x]", "dateTime|Period|Timing|instant", 3520 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3521 0, 1, effective)); 3522 children.add(new Property("issued", "instant", 3523 "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 3524 0, 1, issued)); 3525 children.add(new Property("performer", 3526 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", 3527 "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, 3528 performer)); 3529 children.add(new Property("value[x]", 3530 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3531 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3532 1, value)); 3533 children.add(new Property("dataAbsentReason", "CodeableConcept", 3534 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, 3535 dataAbsentReason)); 3536 children.add(new Property("interpretation", "CodeableConcept", 3537 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 3538 java.lang.Integer.MAX_VALUE, interpretation)); 3539 children.add(new Property("note", "Annotation", "Comments about the observation or the results.", 0, 3540 java.lang.Integer.MAX_VALUE, note)); 3541 children.add(new Property("bodySite", "CodeableConcept", 3542 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 3543 bodySite)); 3544 children.add(new Property("method", "CodeableConcept", "Indicates the mechanism used to perform the observation.", 3545 0, 1, method)); 3546 children.add(new Property("specimen", "Reference(Specimen)", 3547 "The specimen that was used when this observation was made.", 0, 1, specimen)); 3548 children.add(new Property("device", "Reference(Device|DeviceMetric)", 3549 "The device used to generate the observation data.", 0, 1, device)); 3550 children.add(new Property("referenceRange", "", 3551 "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 3552 0, java.lang.Integer.MAX_VALUE, referenceRange)); 3553 children.add(new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", 3554 "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 3555 0, java.lang.Integer.MAX_VALUE, hasMember)); 3556 children.add(new Property("derivedFrom", 3557 "Reference(DocumentReference|ImagingStudy|Media|QuestionnaireResponse|Observation|MolecularSequence)", 3558 "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 3559 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 3560 children.add(new Property("component", "", 3561 "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 3562 0, java.lang.Integer.MAX_VALUE, component)); 3563 } 3564 3565 @Override 3566 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3567 switch (_hash) { 3568 case -1618432855: 3569 /* identifier */ return new Property("identifier", "Identifier", 3570 "A unique identifier assigned to this observation.", 0, java.lang.Integer.MAX_VALUE, identifier); 3571 case -332612366: 3572 /* basedOn */ return new Property("basedOn", 3573 "Reference(CarePlan|DeviceRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|ServiceRequest)", 3574 "A plan, proposal or order that is fulfilled in whole or in part by this event. For example, a MedicationRequest may require a patient to have laboratory test performed before it is dispensed.", 3575 0, java.lang.Integer.MAX_VALUE, basedOn); 3576 case -995410646: 3577 /* partOf */ return new Property("partOf", 3578 "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Immunization|ImagingStudy)", 3579 "A larger event of which this particular Observation is a component or step. For example, an observation as part of a procedure.", 3580 0, java.lang.Integer.MAX_VALUE, partOf); 3581 case -892481550: 3582 /* status */ return new Property("status", "code", "The status of the result value.", 0, 1, status); 3583 case 50511102: 3584 /* category */ return new Property("category", "CodeableConcept", 3585 "A code that classifies the general type of observation being made.", 0, java.lang.Integer.MAX_VALUE, 3586 category); 3587 case 3059181: 3588 /* code */ return new Property("code", "CodeableConcept", 3589 "Describes what was observed. Sometimes this is called the observation \"name\".", 0, 1, code); 3590 case -1867885268: 3591 /* subject */ return new Property("subject", "Reference(Patient|Group|Device|Location)", 3592 "The patient, or group of patients, location, or device this observation is about and into whose record the observation is placed. If the actual focus of the observation is different from the subject (or a sample of, part, or region of the subject), the `focus` element or the `code` itself specifies the actual focus of the observation.", 3593 0, 1, subject); 3594 case 97604824: 3595 /* focus */ return new Property("focus", "Reference(Any)", 3596 "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 3597 0, java.lang.Integer.MAX_VALUE, focus); 3598 case 1524132147: 3599 /* encounter */ return new Property("encounter", "Reference(Encounter)", 3600 "The healthcare event (e.g. a patient and healthcare provider interaction) during which this observation is made.", 3601 0, 1, encounter); 3602 case 247104889: 3603 /* effective[x] */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3604 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3605 0, 1, effective); 3606 case -1468651097: 3607 /* effective */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3608 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3609 0, 1, effective); 3610 case -275306910: 3611 /* effectiveDateTime */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3612 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3613 0, 1, effective); 3614 case -403934648: 3615 /* effectivePeriod */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3616 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3617 0, 1, effective); 3618 case -285872943: 3619 /* effectiveTiming */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3620 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3621 0, 1, effective); 3622 case -1295730118: 3623 /* effectiveInstant */ return new Property("effective[x]", "dateTime|Period|Timing|instant", 3624 "The time or time-period the observed value is asserted as being true. For biological subjects - e.g. human patients - this is usually called the \"physiologically relevant time\". This is usually either the time of the procedure or of specimen collection, but very often the source of the date/time is not known, only the date/time itself.", 3625 0, 1, effective); 3626 case -1179159893: 3627 /* issued */ return new Property("issued", "instant", 3628 "The date and time this version of the observation was made available to providers, typically after the results have been reviewed and verified.", 3629 0, 1, issued); 3630 case 481140686: 3631 /* performer */ return new Property("performer", 3632 "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|RelatedPerson)", 3633 "Who was responsible for asserting the observed value as \"true\".", 0, java.lang.Integer.MAX_VALUE, 3634 performer); 3635 case -1410166417: 3636 /* value[x] */ return new Property("value[x]", 3637 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3638 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3639 1, value); 3640 case 111972721: 3641 /* value */ return new Property("value[x]", 3642 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3643 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3644 1, value); 3645 case -2029823716: 3646 /* valueQuantity */ return new Property("value[x]", 3647 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3648 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3649 1, value); 3650 case 924902896: 3651 /* valueCodeableConcept */ return new Property("value[x]", 3652 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3653 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3654 1, value); 3655 case -1424603934: 3656 /* valueString */ return new Property("value[x]", 3657 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3658 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3659 1, value); 3660 case 733421943: 3661 /* valueBoolean */ return new Property("value[x]", 3662 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3663 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3664 1, value); 3665 case -1668204915: 3666 /* valueInteger */ return new Property("value[x]", 3667 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3668 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3669 1, value); 3670 case 2030761548: 3671 /* valueRange */ return new Property("value[x]", 3672 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3673 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3674 1, value); 3675 case 2030767386: 3676 /* valueRatio */ return new Property("value[x]", 3677 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3678 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3679 1, value); 3680 case -962229101: 3681 /* valueSampledData */ return new Property("value[x]", 3682 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3683 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3684 1, value); 3685 case -765708322: 3686 /* valueTime */ return new Property("value[x]", 3687 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3688 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3689 1, value); 3690 case 1047929900: 3691 /* valueDateTime */ return new Property("value[x]", 3692 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3693 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3694 1, value); 3695 case -1524344174: 3696 /* valuePeriod */ return new Property("value[x]", 3697 "Quantity|CodeableConcept|string|boolean|integer|Range|Ratio|SampledData|time|dateTime|Period", 3698 "The information determined as a result of making the observation, if the information has a simple value.", 0, 3699 1, value); 3700 case 1034315687: 3701 /* dataAbsentReason */ return new Property("dataAbsentReason", "CodeableConcept", 3702 "Provides a reason why the expected value in the element Observation.value[x] is missing.", 0, 1, 3703 dataAbsentReason); 3704 case -297950712: 3705 /* interpretation */ return new Property("interpretation", "CodeableConcept", 3706 "A categorical assessment of an observation value. For example, high, low, normal.", 0, 3707 java.lang.Integer.MAX_VALUE, interpretation); 3708 case 3387378: 3709 /* note */ return new Property("note", "Annotation", "Comments about the observation or the results.", 0, 3710 java.lang.Integer.MAX_VALUE, note); 3711 case 1702620169: 3712 /* bodySite */ return new Property("bodySite", "CodeableConcept", 3713 "Indicates the site on the subject's body where the observation was made (i.e. the target site).", 0, 1, 3714 bodySite); 3715 case -1077554975: 3716 /* method */ return new Property("method", "CodeableConcept", 3717 "Indicates the mechanism used to perform the observation.", 0, 1, method); 3718 case -2132868344: 3719 /* specimen */ return new Property("specimen", "Reference(Specimen)", 3720 "The specimen that was used when this observation was made.", 0, 1, specimen); 3721 case -1335157162: 3722 /* device */ return new Property("device", "Reference(Device|DeviceMetric)", 3723 "The device used to generate the observation data.", 0, 1, device); 3724 case -1912545102: 3725 /* referenceRange */ return new Property("referenceRange", "", 3726 "Guidance on how to interpret the value by comparison to a normal or recommended range. Multiple reference ranges are interpreted as an \"OR\". In other words, to represent two distinct target populations, two `referenceRange` elements would be used.", 3727 0, java.lang.Integer.MAX_VALUE, referenceRange); 3728 case -458019372: 3729 /* hasMember */ return new Property("hasMember", "Reference(Observation|QuestionnaireResponse|MolecularSequence)", 3730 "This observation is a group observation (e.g. a battery, a panel of tests, a set of vital sign measurements) that includes the target as a member of the group.", 3731 0, java.lang.Integer.MAX_VALUE, hasMember); 3732 case 1077922663: 3733 /* derivedFrom */ return new Property("derivedFrom", 3734 "Reference(DocumentReference|ImagingStudy|Media|QuestionnaireResponse|Observation|MolecularSequence)", 3735 "The target resource that represents a measurement from which this observation value is derived. For example, a calculated anion gap or a fetal measurement based on an ultrasound image.", 3736 0, java.lang.Integer.MAX_VALUE, derivedFrom); 3737 case -1399907075: 3738 /* component */ return new Property("component", "", 3739 "Some observations have multiple component observations. These component observations are expressed as separate code value pairs that share the same attributes. Examples include systolic and diastolic component observations for blood pressure measurement and multiple component observations for genetics observations.", 3740 0, java.lang.Integer.MAX_VALUE, component); 3741 default: 3742 return super.getNamedProperty(_hash, _name, _checkValid); 3743 } 3744 3745 } 3746 3747 @Override 3748 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3749 switch (hash) { 3750 case -1618432855: 3751 /* identifier */ return this.identifier == null ? new Base[0] 3752 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3753 case -332612366: 3754 /* basedOn */ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3755 case -995410646: 3756 /* partOf */ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 3757 case -892481550: 3758 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<ObservationStatus> 3759 case 50511102: 3760 /* category */ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 3761 case 3059181: 3762 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 3763 case -1867885268: 3764 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 3765 case 97604824: 3766 /* focus */ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 3767 case 1524132147: 3768 /* encounter */ return this.encounter == null ? new Base[0] : new Base[] { this.encounter }; // Reference 3769 case -1468651097: 3770 /* effective */ return this.effective == null ? new Base[0] : new Base[] { this.effective }; // Type 3771 case -1179159893: 3772 /* issued */ return this.issued == null ? new Base[0] : new Base[] { this.issued }; // InstantType 3773 case 481140686: 3774 /* performer */ return this.performer == null ? new Base[0] 3775 : this.performer.toArray(new Base[this.performer.size()]); // Reference 3776 case 111972721: 3777 /* value */ return this.value == null ? new Base[0] : new Base[] { this.value }; // Type 3778 case 1034315687: 3779 /* dataAbsentReason */ return this.dataAbsentReason == null ? new Base[0] : new Base[] { this.dataAbsentReason }; // CodeableConcept 3780 case -297950712: 3781 /* interpretation */ return this.interpretation == null ? new Base[0] 3782 : this.interpretation.toArray(new Base[this.interpretation.size()]); // CodeableConcept 3783 case 3387378: 3784 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3785 case 1702620169: 3786 /* bodySite */ return this.bodySite == null ? new Base[0] : new Base[] { this.bodySite }; // CodeableConcept 3787 case -1077554975: 3788 /* method */ return this.method == null ? new Base[0] : new Base[] { this.method }; // CodeableConcept 3789 case -2132868344: 3790 /* specimen */ return this.specimen == null ? new Base[0] : new Base[] { this.specimen }; // Reference 3791 case -1335157162: 3792 /* device */ return this.device == null ? new Base[0] : new Base[] { this.device }; // Reference 3793 case -1912545102: 3794 /* referenceRange */ return this.referenceRange == null ? new Base[0] 3795 : this.referenceRange.toArray(new Base[this.referenceRange.size()]); // ObservationReferenceRangeComponent 3796 case -458019372: 3797 /* hasMember */ return this.hasMember == null ? new Base[0] 3798 : this.hasMember.toArray(new Base[this.hasMember.size()]); // Reference 3799 case 1077922663: 3800 /* derivedFrom */ return this.derivedFrom == null ? new Base[0] 3801 : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 3802 case -1399907075: 3803 /* component */ return this.component == null ? new Base[0] 3804 : this.component.toArray(new Base[this.component.size()]); // ObservationComponentComponent 3805 default: 3806 return super.getProperty(hash, name, checkValid); 3807 } 3808 3809 } 3810 3811 @Override 3812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3813 switch (hash) { 3814 case -1618432855: // identifier 3815 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3816 return value; 3817 case -332612366: // basedOn 3818 this.getBasedOn().add(castToReference(value)); // Reference 3819 return value; 3820 case -995410646: // partOf 3821 this.getPartOf().add(castToReference(value)); // Reference 3822 return value; 3823 case -892481550: // status 3824 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3825 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3826 return value; 3827 case 50511102: // category 3828 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 3829 return value; 3830 case 3059181: // code 3831 this.code = castToCodeableConcept(value); // CodeableConcept 3832 return value; 3833 case -1867885268: // subject 3834 this.subject = castToReference(value); // Reference 3835 return value; 3836 case 97604824: // focus 3837 this.getFocus().add(castToReference(value)); // Reference 3838 return value; 3839 case 1524132147: // encounter 3840 this.encounter = castToReference(value); // Reference 3841 return value; 3842 case -1468651097: // effective 3843 this.effective = castToType(value); // Type 3844 return value; 3845 case -1179159893: // issued 3846 this.issued = castToInstant(value); // InstantType 3847 return value; 3848 case 481140686: // performer 3849 this.getPerformer().add(castToReference(value)); // Reference 3850 return value; 3851 case 111972721: // value 3852 this.value = castToType(value); // Type 3853 return value; 3854 case 1034315687: // dataAbsentReason 3855 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3856 return value; 3857 case -297950712: // interpretation 3858 this.getInterpretation().add(castToCodeableConcept(value)); // CodeableConcept 3859 return value; 3860 case 3387378: // note 3861 this.getNote().add(castToAnnotation(value)); // Annotation 3862 return value; 3863 case 1702620169: // bodySite 3864 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3865 return value; 3866 case -1077554975: // method 3867 this.method = castToCodeableConcept(value); // CodeableConcept 3868 return value; 3869 case -2132868344: // specimen 3870 this.specimen = castToReference(value); // Reference 3871 return value; 3872 case -1335157162: // device 3873 this.device = castToReference(value); // Reference 3874 return value; 3875 case -1912545102: // referenceRange 3876 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); // ObservationReferenceRangeComponent 3877 return value; 3878 case -458019372: // hasMember 3879 this.getHasMember().add(castToReference(value)); // Reference 3880 return value; 3881 case 1077922663: // derivedFrom 3882 this.getDerivedFrom().add(castToReference(value)); // Reference 3883 return value; 3884 case -1399907075: // component 3885 this.getComponent().add((ObservationComponentComponent) value); // ObservationComponentComponent 3886 return value; 3887 default: 3888 return super.setProperty(hash, name, value); 3889 } 3890 3891 } 3892 3893 @Override 3894 public Base setProperty(String name, Base value) throws FHIRException { 3895 if (name.equals("identifier")) { 3896 this.getIdentifier().add(castToIdentifier(value)); 3897 } else if (name.equals("basedOn")) { 3898 this.getBasedOn().add(castToReference(value)); 3899 } else if (name.equals("partOf")) { 3900 this.getPartOf().add(castToReference(value)); 3901 } else if (name.equals("status")) { 3902 value = new ObservationStatusEnumFactory().fromType(castToCode(value)); 3903 this.status = (Enumeration) value; // Enumeration<ObservationStatus> 3904 } else if (name.equals("category")) { 3905 this.getCategory().add(castToCodeableConcept(value)); 3906 } else if (name.equals("code")) { 3907 this.code = castToCodeableConcept(value); // CodeableConcept 3908 } else if (name.equals("subject")) { 3909 this.subject = castToReference(value); // Reference 3910 } else if (name.equals("focus")) { 3911 this.getFocus().add(castToReference(value)); 3912 } else if (name.equals("encounter")) { 3913 this.encounter = castToReference(value); // Reference 3914 } else if (name.equals("effective[x]")) { 3915 this.effective = castToType(value); // Type 3916 } else if (name.equals("issued")) { 3917 this.issued = castToInstant(value); // InstantType 3918 } else if (name.equals("performer")) { 3919 this.getPerformer().add(castToReference(value)); 3920 } else if (name.equals("value[x]")) { 3921 this.value = castToType(value); // Type 3922 } else if (name.equals("dataAbsentReason")) { 3923 this.dataAbsentReason = castToCodeableConcept(value); // CodeableConcept 3924 } else if (name.equals("interpretation")) { 3925 this.getInterpretation().add(castToCodeableConcept(value)); 3926 } else if (name.equals("note")) { 3927 this.getNote().add(castToAnnotation(value)); 3928 } else if (name.equals("bodySite")) { 3929 this.bodySite = castToCodeableConcept(value); // CodeableConcept 3930 } else if (name.equals("method")) { 3931 this.method = castToCodeableConcept(value); // CodeableConcept 3932 } else if (name.equals("specimen")) { 3933 this.specimen = castToReference(value); // Reference 3934 } else if (name.equals("device")) { 3935 this.device = castToReference(value); // Reference 3936 } else if (name.equals("referenceRange")) { 3937 this.getReferenceRange().add((ObservationReferenceRangeComponent) value); 3938 } else if (name.equals("hasMember")) { 3939 this.getHasMember().add(castToReference(value)); 3940 } else if (name.equals("derivedFrom")) { 3941 this.getDerivedFrom().add(castToReference(value)); 3942 } else if (name.equals("component")) { 3943 this.getComponent().add((ObservationComponentComponent) value); 3944 } else 3945 return super.setProperty(name, value); 3946 return value; 3947 } 3948 3949 @Override 3950 public void removeChild(String name, Base value) throws FHIRException { 3951 if (name.equals("identifier")) { 3952 this.getIdentifier().remove(castToIdentifier(value)); 3953 } else if (name.equals("basedOn")) { 3954 this.getBasedOn().remove(castToReference(value)); 3955 } else if (name.equals("partOf")) { 3956 this.getPartOf().remove(castToReference(value)); 3957 } else if (name.equals("status")) { 3958 this.status = null; 3959 } else if (name.equals("category")) { 3960 this.getCategory().remove(castToCodeableConcept(value)); 3961 } else if (name.equals("code")) { 3962 this.code = null; 3963 } else if (name.equals("subject")) { 3964 this.subject = null; 3965 } else if (name.equals("focus")) { 3966 this.getFocus().remove(castToReference(value)); 3967 } else if (name.equals("encounter")) { 3968 this.encounter = null; 3969 } else if (name.equals("effective[x]")) { 3970 this.effective = null; 3971 } else if (name.equals("issued")) { 3972 this.issued = null; 3973 } else if (name.equals("performer")) { 3974 this.getPerformer().remove(castToReference(value)); 3975 } else if (name.equals("value[x]")) { 3976 this.value = null; 3977 } else if (name.equals("dataAbsentReason")) { 3978 this.dataAbsentReason = null; 3979 } else if (name.equals("interpretation")) { 3980 this.getInterpretation().remove(castToCodeableConcept(value)); 3981 } else if (name.equals("note")) { 3982 this.getNote().remove(castToAnnotation(value)); 3983 } else if (name.equals("bodySite")) { 3984 this.bodySite = null; 3985 } else if (name.equals("method")) { 3986 this.method = null; 3987 } else if (name.equals("specimen")) { 3988 this.specimen = null; 3989 } else if (name.equals("device")) { 3990 this.device = null; 3991 } else if (name.equals("referenceRange")) { 3992 this.getReferenceRange().remove((ObservationReferenceRangeComponent) value); 3993 } else if (name.equals("hasMember")) { 3994 this.getHasMember().remove(castToReference(value)); 3995 } else if (name.equals("derivedFrom")) { 3996 this.getDerivedFrom().remove(castToReference(value)); 3997 } else if (name.equals("component")) { 3998 this.getComponent().remove((ObservationComponentComponent) value); 3999 } else 4000 super.removeChild(name, value); 4001 4002 } 4003 4004 @Override 4005 public Base makeProperty(int hash, String name) throws FHIRException { 4006 switch (hash) { 4007 case -1618432855: 4008 return addIdentifier(); 4009 case -332612366: 4010 return addBasedOn(); 4011 case -995410646: 4012 return addPartOf(); 4013 case -892481550: 4014 return getStatusElement(); 4015 case 50511102: 4016 return addCategory(); 4017 case 3059181: 4018 return getCode(); 4019 case -1867885268: 4020 return getSubject(); 4021 case 97604824: 4022 return addFocus(); 4023 case 1524132147: 4024 return getEncounter(); 4025 case 247104889: 4026 return getEffective(); 4027 case -1468651097: 4028 return getEffective(); 4029 case -1179159893: 4030 return getIssuedElement(); 4031 case 481140686: 4032 return addPerformer(); 4033 case -1410166417: 4034 return getValue(); 4035 case 111972721: 4036 return getValue(); 4037 case 1034315687: 4038 return getDataAbsentReason(); 4039 case -297950712: 4040 return addInterpretation(); 4041 case 3387378: 4042 return addNote(); 4043 case 1702620169: 4044 return getBodySite(); 4045 case -1077554975: 4046 return getMethod(); 4047 case -2132868344: 4048 return getSpecimen(); 4049 case -1335157162: 4050 return getDevice(); 4051 case -1912545102: 4052 return addReferenceRange(); 4053 case -458019372: 4054 return addHasMember(); 4055 case 1077922663: 4056 return addDerivedFrom(); 4057 case -1399907075: 4058 return addComponent(); 4059 default: 4060 return super.makeProperty(hash, name); 4061 } 4062 4063 } 4064 4065 @Override 4066 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4067 switch (hash) { 4068 case -1618432855: 4069 /* identifier */ return new String[] { "Identifier" }; 4070 case -332612366: 4071 /* basedOn */ return new String[] { "Reference" }; 4072 case -995410646: 4073 /* partOf */ return new String[] { "Reference" }; 4074 case -892481550: 4075 /* status */ return new String[] { "code" }; 4076 case 50511102: 4077 /* category */ return new String[] { "CodeableConcept" }; 4078 case 3059181: 4079 /* code */ return new String[] { "CodeableConcept" }; 4080 case -1867885268: 4081 /* subject */ return new String[] { "Reference" }; 4082 case 97604824: 4083 /* focus */ return new String[] { "Reference" }; 4084 case 1524132147: 4085 /* encounter */ return new String[] { "Reference" }; 4086 case -1468651097: 4087 /* effective */ return new String[] { "dateTime", "Period", "Timing", "instant" }; 4088 case -1179159893: 4089 /* issued */ return new String[] { "instant" }; 4090 case 481140686: 4091 /* performer */ return new String[] { "Reference" }; 4092 case 111972721: 4093 /* value */ return new String[] { "Quantity", "CodeableConcept", "string", "boolean", "integer", "Range", "Ratio", 4094 "SampledData", "time", "dateTime", "Period" }; 4095 case 1034315687: 4096 /* dataAbsentReason */ return new String[] { "CodeableConcept" }; 4097 case -297950712: 4098 /* interpretation */ return new String[] { "CodeableConcept" }; 4099 case 3387378: 4100 /* note */ return new String[] { "Annotation" }; 4101 case 1702620169: 4102 /* bodySite */ return new String[] { "CodeableConcept" }; 4103 case -1077554975: 4104 /* method */ return new String[] { "CodeableConcept" }; 4105 case -2132868344: 4106 /* specimen */ return new String[] { "Reference" }; 4107 case -1335157162: 4108 /* device */ return new String[] { "Reference" }; 4109 case -1912545102: 4110 /* referenceRange */ return new String[] {}; 4111 case -458019372: 4112 /* hasMember */ return new String[] { "Reference" }; 4113 case 1077922663: 4114 /* derivedFrom */ return new String[] { "Reference" }; 4115 case -1399907075: 4116 /* component */ return new String[] {}; 4117 default: 4118 return super.getTypesForProperty(hash, name); 4119 } 4120 4121 } 4122 4123 @Override 4124 public Base addChild(String name) throws FHIRException { 4125 if (name.equals("identifier")) { 4126 return addIdentifier(); 4127 } else if (name.equals("basedOn")) { 4128 return addBasedOn(); 4129 } else if (name.equals("partOf")) { 4130 return addPartOf(); 4131 } else if (name.equals("status")) { 4132 throw new FHIRException("Cannot call addChild on a singleton property Observation.status"); 4133 } else if (name.equals("category")) { 4134 return addCategory(); 4135 } else if (name.equals("code")) { 4136 this.code = new CodeableConcept(); 4137 return this.code; 4138 } else if (name.equals("subject")) { 4139 this.subject = new Reference(); 4140 return this.subject; 4141 } else if (name.equals("focus")) { 4142 return addFocus(); 4143 } else if (name.equals("encounter")) { 4144 this.encounter = new Reference(); 4145 return this.encounter; 4146 } else if (name.equals("effectiveDateTime")) { 4147 this.effective = new DateTimeType(); 4148 return this.effective; 4149 } else if (name.equals("effectivePeriod")) { 4150 this.effective = new Period(); 4151 return this.effective; 4152 } else if (name.equals("effectiveTiming")) { 4153 this.effective = new Timing(); 4154 return this.effective; 4155 } else if (name.equals("effectiveInstant")) { 4156 this.effective = new InstantType(); 4157 return this.effective; 4158 } else if (name.equals("issued")) { 4159 throw new FHIRException("Cannot call addChild on a singleton property Observation.issued"); 4160 } else if (name.equals("performer")) { 4161 return addPerformer(); 4162 } else if (name.equals("valueQuantity")) { 4163 this.value = new Quantity(); 4164 return this.value; 4165 } else if (name.equals("valueCodeableConcept")) { 4166 this.value = new CodeableConcept(); 4167 return this.value; 4168 } else if (name.equals("valueString")) { 4169 this.value = new StringType(); 4170 return this.value; 4171 } else if (name.equals("valueBoolean")) { 4172 this.value = new BooleanType(); 4173 return this.value; 4174 } else if (name.equals("valueInteger")) { 4175 this.value = new IntegerType(); 4176 return this.value; 4177 } else if (name.equals("valueRange")) { 4178 this.value = new Range(); 4179 return this.value; 4180 } else if (name.equals("valueRatio")) { 4181 this.value = new Ratio(); 4182 return this.value; 4183 } else if (name.equals("valueSampledData")) { 4184 this.value = new SampledData(); 4185 return this.value; 4186 } else if (name.equals("valueTime")) { 4187 this.value = new TimeType(); 4188 return this.value; 4189 } else if (name.equals("valueDateTime")) { 4190 this.value = new DateTimeType(); 4191 return this.value; 4192 } else if (name.equals("valuePeriod")) { 4193 this.value = new Period(); 4194 return this.value; 4195 } else if (name.equals("dataAbsentReason")) { 4196 this.dataAbsentReason = new CodeableConcept(); 4197 return this.dataAbsentReason; 4198 } else if (name.equals("interpretation")) { 4199 return addInterpretation(); 4200 } else if (name.equals("note")) { 4201 return addNote(); 4202 } else if (name.equals("bodySite")) { 4203 this.bodySite = new CodeableConcept(); 4204 return this.bodySite; 4205 } else if (name.equals("method")) { 4206 this.method = new CodeableConcept(); 4207 return this.method; 4208 } else if (name.equals("specimen")) { 4209 this.specimen = new Reference(); 4210 return this.specimen; 4211 } else if (name.equals("device")) { 4212 this.device = new Reference(); 4213 return this.device; 4214 } else if (name.equals("referenceRange")) { 4215 return addReferenceRange(); 4216 } else if (name.equals("hasMember")) { 4217 return addHasMember(); 4218 } else if (name.equals("derivedFrom")) { 4219 return addDerivedFrom(); 4220 } else if (name.equals("component")) { 4221 return addComponent(); 4222 } else 4223 return super.addChild(name); 4224 } 4225 4226 public String fhirType() { 4227 return "Observation"; 4228 4229 } 4230 4231 public Observation copy() { 4232 Observation dst = new Observation(); 4233 copyValues(dst); 4234 return dst; 4235 } 4236 4237 public void copyValues(Observation dst) { 4238 super.copyValues(dst); 4239 if (identifier != null) { 4240 dst.identifier = new ArrayList<Identifier>(); 4241 for (Identifier i : identifier) 4242 dst.identifier.add(i.copy()); 4243 } 4244 ; 4245 if (basedOn != null) { 4246 dst.basedOn = new ArrayList<Reference>(); 4247 for (Reference i : basedOn) 4248 dst.basedOn.add(i.copy()); 4249 } 4250 ; 4251 if (partOf != null) { 4252 dst.partOf = new ArrayList<Reference>(); 4253 for (Reference i : partOf) 4254 dst.partOf.add(i.copy()); 4255 } 4256 ; 4257 dst.status = status == null ? null : status.copy(); 4258 if (category != null) { 4259 dst.category = new ArrayList<CodeableConcept>(); 4260 for (CodeableConcept i : category) 4261 dst.category.add(i.copy()); 4262 } 4263 ; 4264 dst.code = code == null ? null : code.copy(); 4265 dst.subject = subject == null ? null : subject.copy(); 4266 if (focus != null) { 4267 dst.focus = new ArrayList<Reference>(); 4268 for (Reference i : focus) 4269 dst.focus.add(i.copy()); 4270 } 4271 ; 4272 dst.encounter = encounter == null ? null : encounter.copy(); 4273 dst.effective = effective == null ? null : effective.copy(); 4274 dst.issued = issued == null ? null : issued.copy(); 4275 if (performer != null) { 4276 dst.performer = new ArrayList<Reference>(); 4277 for (Reference i : performer) 4278 dst.performer.add(i.copy()); 4279 } 4280 ; 4281 dst.value = value == null ? null : value.copy(); 4282 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 4283 if (interpretation != null) { 4284 dst.interpretation = new ArrayList<CodeableConcept>(); 4285 for (CodeableConcept i : interpretation) 4286 dst.interpretation.add(i.copy()); 4287 } 4288 ; 4289 if (note != null) { 4290 dst.note = new ArrayList<Annotation>(); 4291 for (Annotation i : note) 4292 dst.note.add(i.copy()); 4293 } 4294 ; 4295 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4296 dst.method = method == null ? null : method.copy(); 4297 dst.specimen = specimen == null ? null : specimen.copy(); 4298 dst.device = device == null ? null : device.copy(); 4299 if (referenceRange != null) { 4300 dst.referenceRange = new ArrayList<ObservationReferenceRangeComponent>(); 4301 for (ObservationReferenceRangeComponent i : referenceRange) 4302 dst.referenceRange.add(i.copy()); 4303 } 4304 ; 4305 if (hasMember != null) { 4306 dst.hasMember = new ArrayList<Reference>(); 4307 for (Reference i : hasMember) 4308 dst.hasMember.add(i.copy()); 4309 } 4310 ; 4311 if (derivedFrom != null) { 4312 dst.derivedFrom = new ArrayList<Reference>(); 4313 for (Reference i : derivedFrom) 4314 dst.derivedFrom.add(i.copy()); 4315 } 4316 ; 4317 if (component != null) { 4318 dst.component = new ArrayList<ObservationComponentComponent>(); 4319 for (ObservationComponentComponent i : component) 4320 dst.component.add(i.copy()); 4321 } 4322 ; 4323 } 4324 4325 protected Observation typedCopy() { 4326 return copy(); 4327 } 4328 4329 @Override 4330 public boolean equalsDeep(Base other_) { 4331 if (!super.equalsDeep(other_)) 4332 return false; 4333 if (!(other_ instanceof Observation)) 4334 return false; 4335 Observation o = (Observation) other_; 4336 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) 4337 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 4338 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 4339 && compareDeep(subject, o.subject, true) && compareDeep(focus, o.focus, true) 4340 && compareDeep(encounter, o.encounter, true) && compareDeep(effective, o.effective, true) 4341 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) 4342 && compareDeep(value, o.value, true) && compareDeep(dataAbsentReason, o.dataAbsentReason, true) 4343 && compareDeep(interpretation, o.interpretation, true) && compareDeep(note, o.note, true) 4344 && compareDeep(bodySite, o.bodySite, true) && compareDeep(method, o.method, true) 4345 && compareDeep(specimen, o.specimen, true) && compareDeep(device, o.device, true) 4346 && compareDeep(referenceRange, o.referenceRange, true) && compareDeep(hasMember, o.hasMember, true) 4347 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(component, o.component, true); 4348 } 4349 4350 @Override 4351 public boolean equalsShallow(Base other_) { 4352 if (!super.equalsShallow(other_)) 4353 return false; 4354 if (!(other_ instanceof Observation)) 4355 return false; 4356 Observation o = (Observation) other_; 4357 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true); 4358 } 4359 4360 public boolean isEmpty() { 4361 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf, status, category, code, 4362 subject, focus, encounter, effective, issued, performer, value, dataAbsentReason, interpretation, note, 4363 bodySite, method, specimen, device, referenceRange, hasMember, derivedFrom, component); 4364 } 4365 4366 @Override 4367 public ResourceType getResourceType() { 4368 return ResourceType.Observation; 4369 } 4370 4371 /** 4372 * Search parameter: <b>date</b> 4373 * <p> 4374 * Description: <b>Obtained date/time. If the obtained element is a period, a 4375 * date that falls in the period</b><br> 4376 * Type: <b>date</b><br> 4377 * Path: <b>Observation.effective[x]</b><br> 4378 * </p> 4379 */ 4380 @SearchParamDefinition(name = "date", path = "Observation.effective", description = "Obtained date/time. If the obtained element is a period, a date that falls in the period", type = "date") 4381 public static final String SP_DATE = "date"; 4382 /** 4383 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4384 * <p> 4385 * Description: <b>Obtained date/time. If the obtained element is a period, a 4386 * date that falls in the period</b><br> 4387 * Type: <b>date</b><br> 4388 * Path: <b>Observation.effective[x]</b><br> 4389 * </p> 4390 */ 4391 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4392 SP_DATE); 4393 4394 /** 4395 * Search parameter: <b>combo-data-absent-reason</b> 4396 * <p> 4397 * Description: <b>The reason why the expected value in the element 4398 * Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4399 * Type: <b>token</b><br> 4400 * Path: <b>Observation.dataAbsentReason, 4401 * Observation.component.dataAbsentReason</b><br> 4402 * </p> 4403 */ 4404 @SearchParamDefinition(name = "combo-data-absent-reason", path = "Observation.dataAbsentReason | Observation.component.dataAbsentReason", description = "The reason why the expected value in the element Observation.value[x] or Observation.component.value[x] is missing.", type = "token") 4405 public static final String SP_COMBO_DATA_ABSENT_REASON = "combo-data-absent-reason"; 4406 /** 4407 * <b>Fluent Client</b> search parameter constant for 4408 * <b>combo-data-absent-reason</b> 4409 * <p> 4410 * Description: <b>The reason why the expected value in the element 4411 * Observation.value[x] or Observation.component.value[x] is missing.</b><br> 4412 * Type: <b>token</b><br> 4413 * Path: <b>Observation.dataAbsentReason, 4414 * Observation.component.dataAbsentReason</b><br> 4415 * </p> 4416 */ 4417 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4418 SP_COMBO_DATA_ABSENT_REASON); 4419 4420 /** 4421 * Search parameter: <b>code</b> 4422 * <p> 4423 * Description: <b>The code of the observation type</b><br> 4424 * Type: <b>token</b><br> 4425 * Path: <b>Observation.code</b><br> 4426 * </p> 4427 */ 4428 @SearchParamDefinition(name = "code", path = "Observation.code", description = "The code of the observation type", type = "token") 4429 public static final String SP_CODE = "code"; 4430 /** 4431 * <b>Fluent Client</b> search parameter constant for <b>code</b> 4432 * <p> 4433 * Description: <b>The code of the observation type</b><br> 4434 * Type: <b>token</b><br> 4435 * Path: <b>Observation.code</b><br> 4436 * </p> 4437 */ 4438 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4439 SP_CODE); 4440 4441 /** 4442 * Search parameter: <b>combo-code-value-quantity</b> 4443 * <p> 4444 * Description: <b>Code and quantity value parameter pair, including in 4445 * components</b><br> 4446 * Type: <b>composite</b><br> 4447 * Path: <b></b><br> 4448 * </p> 4449 */ 4450 @SearchParamDefinition(name = "combo-code-value-quantity", path = "Observation | Observation.component", description = "Code and quantity value parameter pair, including in components", type = "composite", compositeOf = { 4451 "combo-code", "combo-value-quantity" }) 4452 public static final String SP_COMBO_CODE_VALUE_QUANTITY = "combo-code-value-quantity"; 4453 /** 4454 * <b>Fluent Client</b> search parameter constant for 4455 * <b>combo-code-value-quantity</b> 4456 * <p> 4457 * Description: <b>Code and quantity value parameter pair, including in 4458 * components</b><br> 4459 * Type: <b>composite</b><br> 4460 * Path: <b></b><br> 4461 * </p> 4462 */ 4463 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMBO_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4464 SP_COMBO_CODE_VALUE_QUANTITY); 4465 4466 /** 4467 * Search parameter: <b>subject</b> 4468 * <p> 4469 * Description: <b>The subject that the observation is about</b><br> 4470 * Type: <b>reference</b><br> 4471 * Path: <b>Observation.subject</b><br> 4472 * </p> 4473 */ 4474 @SearchParamDefinition(name = "subject", path = "Observation.subject", description = "The subject that the observation is about", type = "reference", providesMembershipIn = { 4475 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 4476 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Device.class, Group.class, 4477 Location.class, Patient.class }) 4478 public static final String SP_SUBJECT = "subject"; 4479 /** 4480 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4481 * <p> 4482 * Description: <b>The subject that the observation is about</b><br> 4483 * Type: <b>reference</b><br> 4484 * Path: <b>Observation.subject</b><br> 4485 * </p> 4486 */ 4487 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4488 SP_SUBJECT); 4489 4490 /** 4491 * Constant for fluent queries to be used to add include statements. Specifies 4492 * the path value of "<b>Observation:subject</b>". 4493 */ 4494 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 4495 "Observation:subject").toLocked(); 4496 4497 /** 4498 * Search parameter: <b>component-data-absent-reason</b> 4499 * <p> 4500 * Description: <b>The reason why the expected value in the element 4501 * Observation.component.value[x] is missing.</b><br> 4502 * Type: <b>token</b><br> 4503 * Path: <b>Observation.component.dataAbsentReason</b><br> 4504 * </p> 4505 */ 4506 @SearchParamDefinition(name = "component-data-absent-reason", path = "Observation.component.dataAbsentReason", description = "The reason why the expected value in the element Observation.component.value[x] is missing.", type = "token") 4507 public static final String SP_COMPONENT_DATA_ABSENT_REASON = "component-data-absent-reason"; 4508 /** 4509 * <b>Fluent Client</b> search parameter constant for 4510 * <b>component-data-absent-reason</b> 4511 * <p> 4512 * Description: <b>The reason why the expected value in the element 4513 * Observation.component.value[x] is missing.</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>Observation.component.dataAbsentReason</b><br> 4516 * </p> 4517 */ 4518 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4519 SP_COMPONENT_DATA_ABSENT_REASON); 4520 4521 /** 4522 * Search parameter: <b>value-concept</b> 4523 * <p> 4524 * Description: <b>The value of the observation, if the value is a 4525 * CodeableConcept</b><br> 4526 * Type: <b>token</b><br> 4527 * Path: <b>Observation.valueCodeableConcept</b><br> 4528 * </p> 4529 */ 4530 @SearchParamDefinition(name = "value-concept", path = "(Observation.value as CodeableConcept)", description = "The value of the observation, if the value is a CodeableConcept", type = "token") 4531 public static final String SP_VALUE_CONCEPT = "value-concept"; 4532 /** 4533 * <b>Fluent Client</b> search parameter constant for <b>value-concept</b> 4534 * <p> 4535 * Description: <b>The value of the observation, if the value is a 4536 * CodeableConcept</b><br> 4537 * Type: <b>token</b><br> 4538 * Path: <b>Observation.valueCodeableConcept</b><br> 4539 * </p> 4540 */ 4541 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4542 SP_VALUE_CONCEPT); 4543 4544 /** 4545 * Search parameter: <b>value-date</b> 4546 * <p> 4547 * Description: <b>The value of the observation, if the value is a date or 4548 * period of time</b><br> 4549 * Type: <b>date</b><br> 4550 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 4551 * </p> 4552 */ 4553 @SearchParamDefinition(name = "value-date", path = "(Observation.value as dateTime) | (Observation.value as Period)", description = "The value of the observation, if the value is a date or period of time", type = "date") 4554 public static final String SP_VALUE_DATE = "value-date"; 4555 /** 4556 * <b>Fluent Client</b> search parameter constant for <b>value-date</b> 4557 * <p> 4558 * Description: <b>The value of the observation, if the value is a date or 4559 * period of time</b><br> 4560 * Type: <b>date</b><br> 4561 * Path: <b>Observation.valueDateTime, Observation.valuePeriod</b><br> 4562 * </p> 4563 */ 4564 public static final ca.uhn.fhir.rest.gclient.DateClientParam VALUE_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 4565 SP_VALUE_DATE); 4566 4567 /** 4568 * Search parameter: <b>focus</b> 4569 * <p> 4570 * Description: <b>The focus of an observation when the focus is not the patient 4571 * of record.</b><br> 4572 * Type: <b>reference</b><br> 4573 * Path: <b>Observation.focus</b><br> 4574 * </p> 4575 */ 4576 @SearchParamDefinition(name = "focus", path = "Observation.focus", description = "The focus of an observation when the focus is not the patient of record.", type = "reference") 4577 public static final String SP_FOCUS = "focus"; 4578 /** 4579 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 4580 * <p> 4581 * Description: <b>The focus of an observation when the focus is not the patient 4582 * of record.</b><br> 4583 * Type: <b>reference</b><br> 4584 * Path: <b>Observation.focus</b><br> 4585 * </p> 4586 */ 4587 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4588 SP_FOCUS); 4589 4590 /** 4591 * Constant for fluent queries to be used to add include statements. Specifies 4592 * the path value of "<b>Observation:focus</b>". 4593 */ 4594 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include( 4595 "Observation:focus").toLocked(); 4596 4597 /** 4598 * Search parameter: <b>derived-from</b> 4599 * <p> 4600 * Description: <b>Related measurements the observation is made from</b><br> 4601 * Type: <b>reference</b><br> 4602 * Path: <b>Observation.derivedFrom</b><br> 4603 * </p> 4604 */ 4605 @SearchParamDefinition(name = "derived-from", path = "Observation.derivedFrom", description = "Related measurements the observation is made from", type = "reference", target = { 4606 DocumentReference.class, ImagingStudy.class, Media.class, MolecularSequence.class, Observation.class, 4607 QuestionnaireResponse.class }) 4608 public static final String SP_DERIVED_FROM = "derived-from"; 4609 /** 4610 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4611 * <p> 4612 * Description: <b>Related measurements the observation is made from</b><br> 4613 * Type: <b>reference</b><br> 4614 * Path: <b>Observation.derivedFrom</b><br> 4615 * </p> 4616 */ 4617 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4618 SP_DERIVED_FROM); 4619 4620 /** 4621 * Constant for fluent queries to be used to add include statements. Specifies 4622 * the path value of "<b>Observation:derived-from</b>". 4623 */ 4624 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 4625 "Observation:derived-from").toLocked(); 4626 4627 /** 4628 * Search parameter: <b>part-of</b> 4629 * <p> 4630 * Description: <b>Part of referenced event</b><br> 4631 * Type: <b>reference</b><br> 4632 * Path: <b>Observation.partOf</b><br> 4633 * </p> 4634 */ 4635 @SearchParamDefinition(name = "part-of", path = "Observation.partOf", description = "Part of referenced event", type = "reference", target = { 4636 ImagingStudy.class, Immunization.class, MedicationAdministration.class, MedicationDispense.class, 4637 MedicationStatement.class, Procedure.class }) 4638 public static final String SP_PART_OF = "part-of"; 4639 /** 4640 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4641 * <p> 4642 * Description: <b>Part of referenced event</b><br> 4643 * Type: <b>reference</b><br> 4644 * Path: <b>Observation.partOf</b><br> 4645 * </p> 4646 */ 4647 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4648 SP_PART_OF); 4649 4650 /** 4651 * Constant for fluent queries to be used to add include statements. Specifies 4652 * the path value of "<b>Observation:part-of</b>". 4653 */ 4654 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include( 4655 "Observation:part-of").toLocked(); 4656 4657 /** 4658 * Search parameter: <b>has-member</b> 4659 * <p> 4660 * Description: <b>Related resource that belongs to the Observation 4661 * group</b><br> 4662 * Type: <b>reference</b><br> 4663 * Path: <b>Observation.hasMember</b><br> 4664 * </p> 4665 */ 4666 @SearchParamDefinition(name = "has-member", path = "Observation.hasMember", description = "Related resource that belongs to the Observation group", type = "reference", target = { 4667 MolecularSequence.class, Observation.class, QuestionnaireResponse.class }) 4668 public static final String SP_HAS_MEMBER = "has-member"; 4669 /** 4670 * <b>Fluent Client</b> search parameter constant for <b>has-member</b> 4671 * <p> 4672 * Description: <b>Related resource that belongs to the Observation 4673 * group</b><br> 4674 * Type: <b>reference</b><br> 4675 * Path: <b>Observation.hasMember</b><br> 4676 * </p> 4677 */ 4678 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam HAS_MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4679 SP_HAS_MEMBER); 4680 4681 /** 4682 * Constant for fluent queries to be used to add include statements. Specifies 4683 * the path value of "<b>Observation:has-member</b>". 4684 */ 4685 public static final ca.uhn.fhir.model.api.Include INCLUDE_HAS_MEMBER = new ca.uhn.fhir.model.api.Include( 4686 "Observation:has-member").toLocked(); 4687 4688 /** 4689 * Search parameter: <b>code-value-string</b> 4690 * <p> 4691 * Description: <b>Code and string value parameter pair</b><br> 4692 * Type: <b>composite</b><br> 4693 * Path: <b></b><br> 4694 * </p> 4695 */ 4696 @SearchParamDefinition(name = "code-value-string", path = "Observation", description = "Code and string value parameter pair", type = "composite", compositeOf = { 4697 "code", "value-string" }) 4698 public static final String SP_CODE_VALUE_STRING = "code-value-string"; 4699 /** 4700 * <b>Fluent Client</b> search parameter constant for <b>code-value-string</b> 4701 * <p> 4702 * Description: <b>Code and string value parameter pair</b><br> 4703 * Type: <b>composite</b><br> 4704 * Path: <b></b><br> 4705 * </p> 4706 */ 4707 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam> CODE_VALUE_STRING = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.StringClientParam>( 4708 SP_CODE_VALUE_STRING); 4709 4710 /** 4711 * Search parameter: <b>component-code-value-quantity</b> 4712 * <p> 4713 * Description: <b>Component code and component quantity value parameter 4714 * pair</b><br> 4715 * Type: <b>composite</b><br> 4716 * Path: <b></b><br> 4717 * </p> 4718 */ 4719 @SearchParamDefinition(name = "component-code-value-quantity", path = "Observation.component", description = "Component code and component quantity value parameter pair", type = "composite", compositeOf = { 4720 "component-code", "component-value-quantity" }) 4721 public static final String SP_COMPONENT_CODE_VALUE_QUANTITY = "component-code-value-quantity"; 4722 /** 4723 * <b>Fluent Client</b> search parameter constant for 4724 * <b>component-code-value-quantity</b> 4725 * <p> 4726 * Description: <b>Component code and component quantity value parameter 4727 * pair</b><br> 4728 * Type: <b>composite</b><br> 4729 * Path: <b></b><br> 4730 * </p> 4731 */ 4732 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> COMPONENT_CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4733 SP_COMPONENT_CODE_VALUE_QUANTITY); 4734 4735 /** 4736 * Search parameter: <b>based-on</b> 4737 * <p> 4738 * Description: <b>Reference to the service request.</b><br> 4739 * Type: <b>reference</b><br> 4740 * Path: <b>Observation.basedOn</b><br> 4741 * </p> 4742 */ 4743 @SearchParamDefinition(name = "based-on", path = "Observation.basedOn", description = "Reference to the service request.", type = "reference", target = { 4744 CarePlan.class, DeviceRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, 4745 NutritionOrder.class, ServiceRequest.class }) 4746 public static final String SP_BASED_ON = "based-on"; 4747 /** 4748 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 4749 * <p> 4750 * Description: <b>Reference to the service request.</b><br> 4751 * Type: <b>reference</b><br> 4752 * Path: <b>Observation.basedOn</b><br> 4753 * </p> 4754 */ 4755 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4756 SP_BASED_ON); 4757 4758 /** 4759 * Constant for fluent queries to be used to add include statements. Specifies 4760 * the path value of "<b>Observation:based-on</b>". 4761 */ 4762 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include( 4763 "Observation:based-on").toLocked(); 4764 4765 /** 4766 * Search parameter: <b>code-value-date</b> 4767 * <p> 4768 * Description: <b>Code and date/time value parameter pair</b><br> 4769 * Type: <b>composite</b><br> 4770 * Path: <b></b><br> 4771 * </p> 4772 */ 4773 @SearchParamDefinition(name = "code-value-date", path = "Observation", description = "Code and date/time value parameter pair", type = "composite", compositeOf = { 4774 "code", "value-date" }) 4775 public static final String SP_CODE_VALUE_DATE = "code-value-date"; 4776 /** 4777 * <b>Fluent Client</b> search parameter constant for <b>code-value-date</b> 4778 * <p> 4779 * Description: <b>Code and date/time value parameter pair</b><br> 4780 * Type: <b>composite</b><br> 4781 * Path: <b></b><br> 4782 * </p> 4783 */ 4784 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam> CODE_VALUE_DATE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.DateClientParam>( 4785 SP_CODE_VALUE_DATE); 4786 4787 /** 4788 * Search parameter: <b>patient</b> 4789 * <p> 4790 * Description: <b>The subject that the observation is about (if 4791 * patient)</b><br> 4792 * Type: <b>reference</b><br> 4793 * Path: <b>Observation.subject</b><br> 4794 * </p> 4795 */ 4796 @SearchParamDefinition(name = "patient", path = "Observation.subject.where(resolve() is Patient)", description = "The subject that the observation is about (if patient)", type = "reference", target = { 4797 Patient.class }) 4798 public static final String SP_PATIENT = "patient"; 4799 /** 4800 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4801 * <p> 4802 * Description: <b>The subject that the observation is about (if 4803 * patient)</b><br> 4804 * Type: <b>reference</b><br> 4805 * Path: <b>Observation.subject</b><br> 4806 * </p> 4807 */ 4808 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4809 SP_PATIENT); 4810 4811 /** 4812 * Constant for fluent queries to be used to add include statements. Specifies 4813 * the path value of "<b>Observation:patient</b>". 4814 */ 4815 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include( 4816 "Observation:patient").toLocked(); 4817 4818 /** 4819 * Search parameter: <b>specimen</b> 4820 * <p> 4821 * Description: <b>Specimen used for this observation</b><br> 4822 * Type: <b>reference</b><br> 4823 * Path: <b>Observation.specimen</b><br> 4824 * </p> 4825 */ 4826 @SearchParamDefinition(name = "specimen", path = "Observation.specimen", description = "Specimen used for this observation", type = "reference", target = { 4827 Specimen.class }) 4828 public static final String SP_SPECIMEN = "specimen"; 4829 /** 4830 * <b>Fluent Client</b> search parameter constant for <b>specimen</b> 4831 * <p> 4832 * Description: <b>Specimen used for this observation</b><br> 4833 * Type: <b>reference</b><br> 4834 * Path: <b>Observation.specimen</b><br> 4835 * </p> 4836 */ 4837 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SPECIMEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4838 SP_SPECIMEN); 4839 4840 /** 4841 * Constant for fluent queries to be used to add include statements. Specifies 4842 * the path value of "<b>Observation:specimen</b>". 4843 */ 4844 public static final ca.uhn.fhir.model.api.Include INCLUDE_SPECIMEN = new ca.uhn.fhir.model.api.Include( 4845 "Observation:specimen").toLocked(); 4846 4847 /** 4848 * Search parameter: <b>component-code</b> 4849 * <p> 4850 * Description: <b>The component code of the observation type</b><br> 4851 * Type: <b>token</b><br> 4852 * Path: <b>Observation.component.code</b><br> 4853 * </p> 4854 */ 4855 @SearchParamDefinition(name = "component-code", path = "Observation.component.code", description = "The component code of the observation type", type = "token") 4856 public static final String SP_COMPONENT_CODE = "component-code"; 4857 /** 4858 * <b>Fluent Client</b> search parameter constant for <b>component-code</b> 4859 * <p> 4860 * Description: <b>The component code of the observation type</b><br> 4861 * Type: <b>token</b><br> 4862 * Path: <b>Observation.component.code</b><br> 4863 * </p> 4864 */ 4865 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4866 SP_COMPONENT_CODE); 4867 4868 /** 4869 * Search parameter: <b>code-value-quantity</b> 4870 * <p> 4871 * Description: <b>Code and quantity value parameter pair</b><br> 4872 * Type: <b>composite</b><br> 4873 * Path: <b></b><br> 4874 * </p> 4875 */ 4876 @SearchParamDefinition(name = "code-value-quantity", path = "Observation", description = "Code and quantity value parameter pair", type = "composite", compositeOf = { 4877 "code", "value-quantity" }) 4878 public static final String SP_CODE_VALUE_QUANTITY = "code-value-quantity"; 4879 /** 4880 * <b>Fluent Client</b> search parameter constant for <b>code-value-quantity</b> 4881 * <p> 4882 * Description: <b>Code and quantity value parameter pair</b><br> 4883 * Type: <b>composite</b><br> 4884 * Path: <b></b><br> 4885 * </p> 4886 */ 4887 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CODE_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 4888 SP_CODE_VALUE_QUANTITY); 4889 4890 /** 4891 * Search parameter: <b>combo-code-value-concept</b> 4892 * <p> 4893 * Description: <b>Code and coded value parameter pair, including in 4894 * components</b><br> 4895 * Type: <b>composite</b><br> 4896 * Path: <b></b><br> 4897 * </p> 4898 */ 4899 @SearchParamDefinition(name = "combo-code-value-concept", path = "Observation | Observation.component", description = "Code and coded value parameter pair, including in components", type = "composite", compositeOf = { 4900 "combo-code", "combo-value-concept" }) 4901 public static final String SP_COMBO_CODE_VALUE_CONCEPT = "combo-code-value-concept"; 4902 /** 4903 * <b>Fluent Client</b> search parameter constant for 4904 * <b>combo-code-value-concept</b> 4905 * <p> 4906 * Description: <b>Code and coded value parameter pair, including in 4907 * components</b><br> 4908 * Type: <b>composite</b><br> 4909 * Path: <b></b><br> 4910 * </p> 4911 */ 4912 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMBO_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 4913 SP_COMBO_CODE_VALUE_CONCEPT); 4914 4915 /** 4916 * Search parameter: <b>value-string</b> 4917 * <p> 4918 * Description: <b>The value of the observation, if the value is a string, and 4919 * also searches in CodeableConcept.text</b><br> 4920 * Type: <b>string</b><br> 4921 * Path: <b>Observation.value[x]</b><br> 4922 * </p> 4923 */ 4924 @SearchParamDefinition(name = "value-string", path = "(Observation.value as string) | (Observation.value as CodeableConcept).text", description = "The value of the observation, if the value is a string, and also searches in CodeableConcept.text", type = "string") 4925 public static final String SP_VALUE_STRING = "value-string"; 4926 /** 4927 * <b>Fluent Client</b> search parameter constant for <b>value-string</b> 4928 * <p> 4929 * Description: <b>The value of the observation, if the value is a string, and 4930 * also searches in CodeableConcept.text</b><br> 4931 * Type: <b>string</b><br> 4932 * Path: <b>Observation.value[x]</b><br> 4933 * </p> 4934 */ 4935 public static final ca.uhn.fhir.rest.gclient.StringClientParam VALUE_STRING = new ca.uhn.fhir.rest.gclient.StringClientParam( 4936 SP_VALUE_STRING); 4937 4938 /** 4939 * Search parameter: <b>identifier</b> 4940 * <p> 4941 * Description: <b>The unique id for a particular observation</b><br> 4942 * Type: <b>token</b><br> 4943 * Path: <b>Observation.identifier</b><br> 4944 * </p> 4945 */ 4946 @SearchParamDefinition(name = "identifier", path = "Observation.identifier", description = "The unique id for a particular observation", type = "token") 4947 public static final String SP_IDENTIFIER = "identifier"; 4948 /** 4949 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4950 * <p> 4951 * Description: <b>The unique id for a particular observation</b><br> 4952 * Type: <b>token</b><br> 4953 * Path: <b>Observation.identifier</b><br> 4954 * </p> 4955 */ 4956 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 4957 SP_IDENTIFIER); 4958 4959 /** 4960 * Search parameter: <b>performer</b> 4961 * <p> 4962 * Description: <b>Who performed the observation</b><br> 4963 * Type: <b>reference</b><br> 4964 * Path: <b>Observation.performer</b><br> 4965 * </p> 4966 */ 4967 @SearchParamDefinition(name = "performer", path = "Observation.performer", description = "Who performed the observation", type = "reference", providesMembershipIn = { 4968 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 4969 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 4970 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { CareTeam.class, 4971 Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 4972 public static final String SP_PERFORMER = "performer"; 4973 /** 4974 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 4975 * <p> 4976 * Description: <b>Who performed the observation</b><br> 4977 * Type: <b>reference</b><br> 4978 * Path: <b>Observation.performer</b><br> 4979 * </p> 4980 */ 4981 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 4982 SP_PERFORMER); 4983 4984 /** 4985 * Constant for fluent queries to be used to add include statements. Specifies 4986 * the path value of "<b>Observation:performer</b>". 4987 */ 4988 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include( 4989 "Observation:performer").toLocked(); 4990 4991 /** 4992 * Search parameter: <b>combo-code</b> 4993 * <p> 4994 * Description: <b>The code of the observation type or component type</b><br> 4995 * Type: <b>token</b><br> 4996 * Path: <b>Observation.code, Observation.component.code</b><br> 4997 * </p> 4998 */ 4999 @SearchParamDefinition(name = "combo-code", path = "Observation.code | Observation.component.code", description = "The code of the observation type or component type", type = "token") 5000 public static final String SP_COMBO_CODE = "combo-code"; 5001 /** 5002 * <b>Fluent Client</b> search parameter constant for <b>combo-code</b> 5003 * <p> 5004 * Description: <b>The code of the observation type or component type</b><br> 5005 * Type: <b>token</b><br> 5006 * Path: <b>Observation.code, Observation.component.code</b><br> 5007 * </p> 5008 */ 5009 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5010 SP_COMBO_CODE); 5011 5012 /** 5013 * Search parameter: <b>method</b> 5014 * <p> 5015 * Description: <b>The method used for the observation</b><br> 5016 * Type: <b>token</b><br> 5017 * Path: <b>Observation.method</b><br> 5018 * </p> 5019 */ 5020 @SearchParamDefinition(name = "method", path = "Observation.method", description = "The method used for the observation", type = "token") 5021 public static final String SP_METHOD = "method"; 5022 /** 5023 * <b>Fluent Client</b> search parameter constant for <b>method</b> 5024 * <p> 5025 * Description: <b>The method used for the observation</b><br> 5026 * Type: <b>token</b><br> 5027 * Path: <b>Observation.method</b><br> 5028 * </p> 5029 */ 5030 public static final ca.uhn.fhir.rest.gclient.TokenClientParam METHOD = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5031 SP_METHOD); 5032 5033 /** 5034 * Search parameter: <b>value-quantity</b> 5035 * <p> 5036 * Description: <b>The value of the observation, if the value is a Quantity, or 5037 * a SampledData (just search on the bounds of the values in sampled 5038 * data)</b><br> 5039 * Type: <b>quantity</b><br> 5040 * Path: <b>Observation.value[x]</b><br> 5041 * </p> 5042 */ 5043 @SearchParamDefinition(name = "value-quantity", path = "(Observation.value as Quantity) | (Observation.value as SampledData)", description = "The value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 5044 public static final String SP_VALUE_QUANTITY = "value-quantity"; 5045 /** 5046 * <b>Fluent Client</b> search parameter constant for <b>value-quantity</b> 5047 * <p> 5048 * Description: <b>The value of the observation, if the value is a Quantity, or 5049 * a SampledData (just search on the bounds of the values in sampled 5050 * data)</b><br> 5051 * Type: <b>quantity</b><br> 5052 * Path: <b>Observation.value[x]</b><br> 5053 * </p> 5054 */ 5055 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5056 SP_VALUE_QUANTITY); 5057 5058 /** 5059 * Search parameter: <b>component-value-quantity</b> 5060 * <p> 5061 * Description: <b>The value of the component observation, if the value is a 5062 * Quantity, or a SampledData (just search on the bounds of the values in 5063 * sampled data)</b><br> 5064 * Type: <b>quantity</b><br> 5065 * Path: <b>Observation.component.value[x]</b><br> 5066 * </p> 5067 */ 5068 @SearchParamDefinition(name = "component-value-quantity", path = "(Observation.component.value as Quantity) | (Observation.component.value as SampledData)", description = "The value of the component observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 5069 public static final String SP_COMPONENT_VALUE_QUANTITY = "component-value-quantity"; 5070 /** 5071 * <b>Fluent Client</b> search parameter constant for 5072 * <b>component-value-quantity</b> 5073 * <p> 5074 * Description: <b>The value of the component observation, if the value is a 5075 * Quantity, or a SampledData (just search on the bounds of the values in 5076 * sampled data)</b><br> 5077 * Type: <b>quantity</b><br> 5078 * Path: <b>Observation.component.value[x]</b><br> 5079 * </p> 5080 */ 5081 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMPONENT_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5082 SP_COMPONENT_VALUE_QUANTITY); 5083 5084 /** 5085 * Search parameter: <b>data-absent-reason</b> 5086 * <p> 5087 * Description: <b>The reason why the expected value in the element 5088 * Observation.value[x] is missing.</b><br> 5089 * Type: <b>token</b><br> 5090 * Path: <b>Observation.dataAbsentReason</b><br> 5091 * </p> 5092 */ 5093 @SearchParamDefinition(name = "data-absent-reason", path = "Observation.dataAbsentReason", description = "The reason why the expected value in the element Observation.value[x] is missing.", type = "token") 5094 public static final String SP_DATA_ABSENT_REASON = "data-absent-reason"; 5095 /** 5096 * <b>Fluent Client</b> search parameter constant for <b>data-absent-reason</b> 5097 * <p> 5098 * Description: <b>The reason why the expected value in the element 5099 * Observation.value[x] is missing.</b><br> 5100 * Type: <b>token</b><br> 5101 * Path: <b>Observation.dataAbsentReason</b><br> 5102 * </p> 5103 */ 5104 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DATA_ABSENT_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5105 SP_DATA_ABSENT_REASON); 5106 5107 /** 5108 * Search parameter: <b>combo-value-quantity</b> 5109 * <p> 5110 * Description: <b>The value or component value of the observation, if the value 5111 * is a Quantity, or a SampledData (just search on the bounds of the values in 5112 * sampled data)</b><br> 5113 * Type: <b>quantity</b><br> 5114 * Path: <b>Observation.value[x]</b><br> 5115 * </p> 5116 */ 5117 @SearchParamDefinition(name = "combo-value-quantity", path = "(Observation.value as Quantity) | (Observation.value as SampledData) | (Observation.component.value as Quantity) | (Observation.component.value as SampledData)", description = "The value or component value of the observation, if the value is a Quantity, or a SampledData (just search on the bounds of the values in sampled data)", type = "quantity") 5118 public static final String SP_COMBO_VALUE_QUANTITY = "combo-value-quantity"; 5119 /** 5120 * <b>Fluent Client</b> search parameter constant for 5121 * <b>combo-value-quantity</b> 5122 * <p> 5123 * Description: <b>The value or component value of the observation, if the value 5124 * is a Quantity, or a SampledData (just search on the bounds of the values in 5125 * sampled data)</b><br> 5126 * Type: <b>quantity</b><br> 5127 * Path: <b>Observation.value[x]</b><br> 5128 * </p> 5129 */ 5130 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam COMBO_VALUE_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 5131 SP_COMBO_VALUE_QUANTITY); 5132 5133 /** 5134 * Search parameter: <b>encounter</b> 5135 * <p> 5136 * Description: <b>Encounter related to the observation</b><br> 5137 * Type: <b>reference</b><br> 5138 * Path: <b>Observation.encounter</b><br> 5139 * </p> 5140 */ 5141 @SearchParamDefinition(name = "encounter", path = "Observation.encounter", description = "Encounter related to the observation", type = "reference", providesMembershipIn = { 5142 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Encounter") }, target = { Encounter.class }) 5143 public static final String SP_ENCOUNTER = "encounter"; 5144 /** 5145 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 5146 * <p> 5147 * Description: <b>Encounter related to the observation</b><br> 5148 * Type: <b>reference</b><br> 5149 * Path: <b>Observation.encounter</b><br> 5150 * </p> 5151 */ 5152 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5153 SP_ENCOUNTER); 5154 5155 /** 5156 * Constant for fluent queries to be used to add include statements. Specifies 5157 * the path value of "<b>Observation:encounter</b>". 5158 */ 5159 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include( 5160 "Observation:encounter").toLocked(); 5161 5162 /** 5163 * Search parameter: <b>code-value-concept</b> 5164 * <p> 5165 * Description: <b>Code and coded value parameter pair</b><br> 5166 * Type: <b>composite</b><br> 5167 * Path: <b></b><br> 5168 * </p> 5169 */ 5170 @SearchParamDefinition(name = "code-value-concept", path = "Observation", description = "Code and coded value parameter pair", type = "composite", compositeOf = { 5171 "code", "value-concept" }) 5172 public static final String SP_CODE_VALUE_CONCEPT = "code-value-concept"; 5173 /** 5174 * <b>Fluent Client</b> search parameter constant for <b>code-value-concept</b> 5175 * <p> 5176 * Description: <b>Code and coded value parameter pair</b><br> 5177 * Type: <b>composite</b><br> 5178 * Path: <b></b><br> 5179 * </p> 5180 */ 5181 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5182 SP_CODE_VALUE_CONCEPT); 5183 5184 /** 5185 * Search parameter: <b>component-code-value-concept</b> 5186 * <p> 5187 * Description: <b>Component code and component coded value parameter 5188 * pair</b><br> 5189 * Type: <b>composite</b><br> 5190 * Path: <b></b><br> 5191 * </p> 5192 */ 5193 @SearchParamDefinition(name = "component-code-value-concept", path = "Observation.component", description = "Component code and component coded value parameter pair", type = "composite", compositeOf = { 5194 "component-code", "component-value-concept" }) 5195 public static final String SP_COMPONENT_CODE_VALUE_CONCEPT = "component-code-value-concept"; 5196 /** 5197 * <b>Fluent Client</b> search parameter constant for 5198 * <b>component-code-value-concept</b> 5199 * <p> 5200 * Description: <b>Component code and component coded value parameter 5201 * pair</b><br> 5202 * Type: <b>composite</b><br> 5203 * Path: <b></b><br> 5204 * </p> 5205 */ 5206 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> COMPONENT_CODE_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 5207 SP_COMPONENT_CODE_VALUE_CONCEPT); 5208 5209 /** 5210 * Search parameter: <b>component-value-concept</b> 5211 * <p> 5212 * Description: <b>The value of the component observation, if the value is a 5213 * CodeableConcept</b><br> 5214 * Type: <b>token</b><br> 5215 * Path: <b>Observation.component.valueCodeableConcept</b><br> 5216 * </p> 5217 */ 5218 @SearchParamDefinition(name = "component-value-concept", path = "(Observation.component.value as CodeableConcept)", description = "The value of the component observation, if the value is a CodeableConcept", type = "token") 5219 public static final String SP_COMPONENT_VALUE_CONCEPT = "component-value-concept"; 5220 /** 5221 * <b>Fluent Client</b> search parameter constant for 5222 * <b>component-value-concept</b> 5223 * <p> 5224 * Description: <b>The value of the component observation, if the value is a 5225 * CodeableConcept</b><br> 5226 * Type: <b>token</b><br> 5227 * Path: <b>Observation.component.valueCodeableConcept</b><br> 5228 * </p> 5229 */ 5230 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMPONENT_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5231 SP_COMPONENT_VALUE_CONCEPT); 5232 5233 /** 5234 * Search parameter: <b>category</b> 5235 * <p> 5236 * Description: <b>The classification of the type of observation</b><br> 5237 * Type: <b>token</b><br> 5238 * Path: <b>Observation.category</b><br> 5239 * </p> 5240 */ 5241 @SearchParamDefinition(name = "category", path = "Observation.category", description = "The classification of the type of observation", type = "token") 5242 public static final String SP_CATEGORY = "category"; 5243 /** 5244 * <b>Fluent Client</b> search parameter constant for <b>category</b> 5245 * <p> 5246 * Description: <b>The classification of the type of observation</b><br> 5247 * Type: <b>token</b><br> 5248 * Path: <b>Observation.category</b><br> 5249 * </p> 5250 */ 5251 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5252 SP_CATEGORY); 5253 5254 /** 5255 * Search parameter: <b>device</b> 5256 * <p> 5257 * Description: <b>The Device that generated the observation data.</b><br> 5258 * Type: <b>reference</b><br> 5259 * Path: <b>Observation.device</b><br> 5260 * </p> 5261 */ 5262 @SearchParamDefinition(name = "device", path = "Observation.device", description = "The Device that generated the observation data.", type = "reference", providesMembershipIn = { 5263 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device") }, target = { Device.class, DeviceMetric.class }) 5264 public static final String SP_DEVICE = "device"; 5265 /** 5266 * <b>Fluent Client</b> search parameter constant for <b>device</b> 5267 * <p> 5268 * Description: <b>The Device that generated the observation data.</b><br> 5269 * Type: <b>reference</b><br> 5270 * Path: <b>Observation.device</b><br> 5271 * </p> 5272 */ 5273 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 5274 SP_DEVICE); 5275 5276 /** 5277 * Constant for fluent queries to be used to add include statements. Specifies 5278 * the path value of "<b>Observation:device</b>". 5279 */ 5280 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include( 5281 "Observation:device").toLocked(); 5282 5283 /** 5284 * Search parameter: <b>combo-value-concept</b> 5285 * <p> 5286 * Description: <b>The value or component value of the observation, if the value 5287 * is a CodeableConcept</b><br> 5288 * Type: <b>token</b><br> 5289 * Path: <b>Observation.valueCodeableConcept, 5290 * Observation.component.valueCodeableConcept</b><br> 5291 * </p> 5292 */ 5293 @SearchParamDefinition(name = "combo-value-concept", path = "(Observation.value as CodeableConcept) | (Observation.component.value as CodeableConcept)", description = "The value or component value of the observation, if the value is a CodeableConcept", type = "token") 5294 public static final String SP_COMBO_VALUE_CONCEPT = "combo-value-concept"; 5295 /** 5296 * <b>Fluent Client</b> search parameter constant for <b>combo-value-concept</b> 5297 * <p> 5298 * Description: <b>The value or component value of the observation, if the value 5299 * is a CodeableConcept</b><br> 5300 * Type: <b>token</b><br> 5301 * Path: <b>Observation.valueCodeableConcept, 5302 * Observation.component.valueCodeableConcept</b><br> 5303 * </p> 5304 */ 5305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMBO_VALUE_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5306 SP_COMBO_VALUE_CONCEPT); 5307 5308 /** 5309 * Search parameter: <b>status</b> 5310 * <p> 5311 * Description: <b>The status of the observation</b><br> 5312 * Type: <b>token</b><br> 5313 * Path: <b>Observation.status</b><br> 5314 * </p> 5315 */ 5316 @SearchParamDefinition(name = "status", path = "Observation.status", description = "The status of the observation", type = "token") 5317 public static final String SP_STATUS = "status"; 5318 /** 5319 * <b>Fluent Client</b> search parameter constant for <b>status</b> 5320 * <p> 5321 * Description: <b>The status of the observation</b><br> 5322 * Type: <b>token</b><br> 5323 * Path: <b>Observation.status</b><br> 5324 * </p> 5325 */ 5326 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 5327 SP_STATUS); 5328 5329}